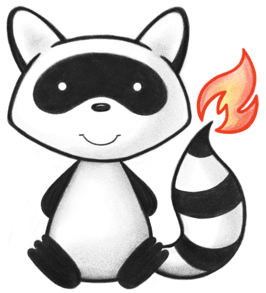
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.utilities.Utilities; 044 045/** 046 * Describes the event of a patient consuming or otherwise being administered a 047 * medication. This may be as simple as swallowing a tablet or it may be a long 048 * running infusion. Related resources tie this event to the authorizing 049 * prescription, and the specific encounter between patient and health care 050 * practitioner. 051 */ 052@ResourceDef(name = "MedicationAdministration", profile = "http://hl7.org/fhir/Profile/MedicationAdministration") 053public class MedicationAdministration extends DomainResource { 054 055 public enum MedicationAdministrationStatus { 056 /** 057 * The administration has started but has not yet completed. 058 */ 059 INPROGRESS, 060 /** 061 * Actions implied by the administration have been temporarily halted, but are 062 * expected to continue later. May also be called "suspended". 063 */ 064 ONHOLD, 065 /** 066 * All actions that are implied by the administration have occurred. 067 */ 068 COMPLETED, 069 /** 070 * The administration was entered in error and therefore nullified. 071 */ 072 ENTEREDINERROR, 073 /** 074 * Actions implied by the administration have been permanently halted, before 075 * all of them occurred. 076 */ 077 STOPPED, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 083 public static MedicationAdministrationStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("in-progress".equals(codeString)) 087 return INPROGRESS; 088 if ("on-hold".equals(codeString)) 089 return ONHOLD; 090 if ("completed".equals(codeString)) 091 return COMPLETED; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if ("stopped".equals(codeString)) 095 return STOPPED; 096 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case INPROGRESS: 102 return "in-progress"; 103 case ONHOLD: 104 return "on-hold"; 105 case COMPLETED: 106 return "completed"; 107 case ENTEREDINERROR: 108 return "entered-in-error"; 109 case STOPPED: 110 return "stopped"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 switch (this) { 120 case INPROGRESS: 121 return "http://hl7.org/fhir/medication-admin-status"; 122 case ONHOLD: 123 return "http://hl7.org/fhir/medication-admin-status"; 124 case COMPLETED: 125 return "http://hl7.org/fhir/medication-admin-status"; 126 case ENTEREDINERROR: 127 return "http://hl7.org/fhir/medication-admin-status"; 128 case STOPPED: 129 return "http://hl7.org/fhir/medication-admin-status"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case INPROGRESS: 140 return "The administration has started but has not yet completed."; 141 case ONHOLD: 142 return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 143 case COMPLETED: 144 return "All actions that are implied by the administration have occurred."; 145 case ENTEREDINERROR: 146 return "The administration was entered in error and therefore nullified."; 147 case STOPPED: 148 return "Actions implied by the administration have been permanently halted, before all of them occurred."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case INPROGRESS: 159 return "In Progress"; 160 case ONHOLD: 161 return "On Hold"; 162 case COMPLETED: 163 return "Completed"; 164 case ENTEREDINERROR: 165 return "Entered in Error"; 166 case STOPPED: 167 return "Stopped"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class MedicationAdministrationStatusEnumFactory implements EnumFactory<MedicationAdministrationStatus> { 177 public MedicationAdministrationStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("in-progress".equals(codeString)) 182 return MedicationAdministrationStatus.INPROGRESS; 183 if ("on-hold".equals(codeString)) 184 return MedicationAdministrationStatus.ONHOLD; 185 if ("completed".equals(codeString)) 186 return MedicationAdministrationStatus.COMPLETED; 187 if ("entered-in-error".equals(codeString)) 188 return MedicationAdministrationStatus.ENTEREDINERROR; 189 if ("stopped".equals(codeString)) 190 return MedicationAdministrationStatus.STOPPED; 191 throw new IllegalArgumentException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 192 } 193 194 public Enumeration<MedicationAdministrationStatus> fromType(Base code) throws FHIRException { 195 if (code == null || code.isEmpty()) 196 return null; 197 String codeString = ((PrimitiveType) code).asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("in-progress".equals(codeString)) 201 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.INPROGRESS); 202 if ("on-hold".equals(codeString)) 203 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ONHOLD); 204 if ("completed".equals(codeString)) 205 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.COMPLETED); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ENTEREDINERROR); 208 if ("stopped".equals(codeString)) 209 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.STOPPED); 210 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 211 } 212 213 public String toCode(MedicationAdministrationStatus code) 214 { 215 if (code == MedicationAdministrationStatus.NULL) 216 return null; 217 if (code == MedicationAdministrationStatus.INPROGRESS) 218 return "in-progress"; 219 if (code == MedicationAdministrationStatus.ONHOLD) 220 return "on-hold"; 221 if (code == MedicationAdministrationStatus.COMPLETED) 222 return "completed"; 223 if (code == MedicationAdministrationStatus.ENTEREDINERROR) 224 return "entered-in-error"; 225 if (code == MedicationAdministrationStatus.STOPPED) 226 return "stopped"; 227 return "?"; 228 } 229 } 230 231 @Block() 232 public static class MedicationAdministrationDosageComponent extends BackboneElement implements IBaseBackboneElement { 233 /** 234 * Free text dosage instructions can be used for cases where the instructions 235 * are too complex to code. When coded instructions are present, the free text 236 * instructions may still be present for display to humans taking or 237 * administering the medication. 238 */ 239 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 240 @Description(shortDefinition = "Dosage Instructions", formalDefinition = "Free text dosage instructions can be used for cases where the instructions are too complex to code. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication.") 241 protected StringType text; 242 243 /** 244 * A coded specification of the anatomic site where the medication first entered 245 * the body. For example, "left arm". 246 */ 247 @Child(name = "site", type = { CodeableConcept.class, 248 BodySite.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 249 @Description(shortDefinition = "Body site administered to", formalDefinition = "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".") 250 protected Type site; 251 252 /** 253 * A code specifying the route or physiological path of administration of a 254 * therapeutic agent into or onto the patient. For example, topical, 255 * intravenous, etc. 256 */ 257 @Child(name = "route", type = { 258 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 259 @Description(shortDefinition = "Path of substance into body", formalDefinition = "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.") 260 protected CodeableConcept route; 261 262 /** 263 * A coded value indicating the method by which the medication is intended to be 264 * or was introduced into or on the body. This attribute will most often NOT be 265 * populated. It is most commonly used for injections. For example, Slow Push, 266 * Deep IV. 267 */ 268 @Child(name = "method", type = { 269 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 270 @Description(shortDefinition = "How drug was administered", formalDefinition = "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.") 271 protected CodeableConcept method; 272 273 /** 274 * The amount of the medication given at one administration event. Use this 275 * value when the administration is essentially an instantaneous event such as a 276 * swallowing a tablet or giving an injection. 277 */ 278 @Child(name = "quantity", type = { 279 SimpleQuantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 280 @Description(shortDefinition = "Amount administered in one dose", formalDefinition = "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.") 281 protected SimpleQuantity quantity; 282 283 /** 284 * Identifies the speed with which the medication was or will be introduced into 285 * the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 286 * ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 287 * hours. Currently we do not specify a default of '1' in the denominator, but 288 * this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 289 * liter/8 hours. 290 */ 291 @Child(name = "rate", type = { Ratio.class, 292 Range.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 293 @Description(shortDefinition = "Dose quantity per unit of time", formalDefinition = "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.") 294 protected Type rate; 295 296 private static final long serialVersionUID = -1772198879L; 297 298 /* 299 * Constructor 300 */ 301 public MedicationAdministrationDosageComponent() { 302 super(); 303 } 304 305 /** 306 * @return {@link #text} (Free text dosage instructions can be used for cases 307 * where the instructions are too complex to code. When coded 308 * instructions are present, the free text instructions may still be 309 * present for display to humans taking or administering the 310 * medication.). This is the underlying object with id, value and 311 * extensions. The accessor "getText" gives direct access to the value 312 */ 313 public StringType getTextElement() { 314 if (this.text == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.text"); 317 else if (Configuration.doAutoCreate()) 318 this.text = new StringType(); // bb 319 return this.text; 320 } 321 322 public boolean hasTextElement() { 323 return this.text != null && !this.text.isEmpty(); 324 } 325 326 public boolean hasText() { 327 return this.text != null && !this.text.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #text} (Free text dosage instructions can be used for 332 * cases where the instructions are too complex to code. When coded 333 * instructions are present, the free text instructions may still 334 * be present for display to humans taking or administering the 335 * medication.). This is the underlying object with id, value and 336 * extensions. The accessor "getText" gives direct access to the 337 * value 338 */ 339 public MedicationAdministrationDosageComponent setTextElement(StringType value) { 340 this.text = value; 341 return this; 342 } 343 344 /** 345 * @return Free text dosage instructions can be used for cases where the 346 * instructions are too complex to code. When coded instructions are 347 * present, the free text instructions may still be present for display 348 * to humans taking or administering the medication. 349 */ 350 public String getText() { 351 return this.text == null ? null : this.text.getValue(); 352 } 353 354 /** 355 * @param value Free text dosage instructions can be used for cases where the 356 * instructions are too complex to code. When coded instructions 357 * are present, the free text instructions may still be present for 358 * display to humans taking or administering the medication. 359 */ 360 public MedicationAdministrationDosageComponent setText(String value) { 361 if (Utilities.noString(value)) 362 this.text = null; 363 else { 364 if (this.text == null) 365 this.text = new StringType(); 366 this.text.setValue(value); 367 } 368 return this; 369 } 370 371 /** 372 * @return {@link #site} (A coded specification of the anatomic site where the 373 * medication first entered the body. For example, "left arm".) 374 */ 375 public Type getSite() { 376 return this.site; 377 } 378 379 /** 380 * @return {@link #site} (A coded specification of the anatomic site where the 381 * medication first entered the body. For example, "left arm".) 382 */ 383 public CodeableConcept getSiteCodeableConcept() throws FHIRException { 384 if (!(this.site instanceof CodeableConcept)) 385 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 386 + this.site.getClass().getName() + " was encountered"); 387 return (CodeableConcept) this.site; 388 } 389 390 public boolean hasSiteCodeableConcept() { 391 return this.site instanceof CodeableConcept; 392 } 393 394 /** 395 * @return {@link #site} (A coded specification of the anatomic site where the 396 * medication first entered the body. For example, "left arm".) 397 */ 398 public Reference getSiteReference() throws FHIRException { 399 if (!(this.site instanceof Reference)) 400 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.site.getClass().getName() 401 + " was encountered"); 402 return (Reference) this.site; 403 } 404 405 public boolean hasSiteReference() { 406 return this.site instanceof Reference; 407 } 408 409 public boolean hasSite() { 410 return this.site != null && !this.site.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #site} (A coded specification of the anatomic site where 415 * the medication first entered the body. For example, "left arm".) 416 */ 417 public MedicationAdministrationDosageComponent setSite(Type value) { 418 this.site = value; 419 return this; 420 } 421 422 /** 423 * @return {@link #route} (A code specifying the route or physiological path of 424 * administration of a therapeutic agent into or onto the patient. For 425 * example, topical, intravenous, etc.) 426 */ 427 public CodeableConcept getRoute() { 428 if (this.route == null) 429 if (Configuration.errorOnAutoCreate()) 430 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.route"); 431 else if (Configuration.doAutoCreate()) 432 this.route = new CodeableConcept(); // cc 433 return this.route; 434 } 435 436 public boolean hasRoute() { 437 return this.route != null && !this.route.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #route} (A code specifying the route or physiological 442 * path of administration of a therapeutic agent into or onto the 443 * patient. For example, topical, intravenous, etc.) 444 */ 445 public MedicationAdministrationDosageComponent setRoute(CodeableConcept value) { 446 this.route = value; 447 return this; 448 } 449 450 /** 451 * @return {@link #method} (A coded value indicating the method by which the 452 * medication is intended to be or was introduced into or on the body. 453 * This attribute will most often NOT be populated. It is most commonly 454 * used for injections. For example, Slow Push, Deep IV.) 455 */ 456 public CodeableConcept getMethod() { 457 if (this.method == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.method"); 460 else if (Configuration.doAutoCreate()) 461 this.method = new CodeableConcept(); // cc 462 return this.method; 463 } 464 465 public boolean hasMethod() { 466 return this.method != null && !this.method.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #method} (A coded value indicating the method by which 471 * the medication is intended to be or was introduced into or on 472 * the body. This attribute will most often NOT be populated. It is 473 * most commonly used for injections. For example, Slow Push, Deep 474 * IV.) 475 */ 476 public MedicationAdministrationDosageComponent setMethod(CodeableConcept value) { 477 this.method = value; 478 return this; 479 } 480 481 /** 482 * @return {@link #quantity} (The amount of the medication given at one 483 * administration event. Use this value when the administration is 484 * essentially an instantaneous event such as a swallowing a tablet or 485 * giving an injection.) 486 */ 487 public SimpleQuantity getQuantity() { 488 if (this.quantity == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.quantity"); 491 else if (Configuration.doAutoCreate()) 492 this.quantity = new SimpleQuantity(); // cc 493 return this.quantity; 494 } 495 496 public boolean hasQuantity() { 497 return this.quantity != null && !this.quantity.isEmpty(); 498 } 499 500 /** 501 * @param value {@link #quantity} (The amount of the medication given at one 502 * administration event. Use this value when the administration is 503 * essentially an instantaneous event such as a swallowing a tablet 504 * or giving an injection.) 505 */ 506 public MedicationAdministrationDosageComponent setQuantity(SimpleQuantity value) { 507 this.quantity = value; 508 return this; 509 } 510 511 /** 512 * @return {@link #rate} (Identifies the speed with which the medication was or 513 * will be introduced into the patient. Typically the rate for an 514 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 515 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 516 * not specify a default of '1' in the denominator, but this is being 517 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 518 * hours.) 519 */ 520 public Type getRate() { 521 return this.rate; 522 } 523 524 /** 525 * @return {@link #rate} (Identifies the speed with which the medication was or 526 * will be introduced into the patient. Typically the rate for an 527 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 528 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 529 * not specify a default of '1' in the denominator, but this is being 530 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 531 * hours.) 532 */ 533 public Ratio getRateRatio() throws FHIRException { 534 if (!(this.rate instanceof Ratio)) 535 throw new FHIRException( 536 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 537 return (Ratio) this.rate; 538 } 539 540 public boolean hasRateRatio() { 541 return this.rate instanceof Ratio; 542 } 543 544 /** 545 * @return {@link #rate} (Identifies the speed with which the medication was or 546 * will be introduced into the patient. Typically the rate for an 547 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 548 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 549 * not specify a default of '1' in the denominator, but this is being 550 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 551 * hours.) 552 */ 553 public Range getRateRange() throws FHIRException { 554 if (!(this.rate instanceof Range)) 555 throw new FHIRException( 556 "Type mismatch: the type Range was expected, but " + this.rate.getClass().getName() + " was encountered"); 557 return (Range) this.rate; 558 } 559 560 public boolean hasRateRange() { 561 return this.rate instanceof Range; 562 } 563 564 public boolean hasRate() { 565 return this.rate != null && !this.rate.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #rate} (Identifies the speed with which the medication 570 * was or will be introduced into the patient. Typically the rate 571 * for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be 572 * expressed as a rate per unit of time e.g. 500 ml per 2 hours. 573 * Currently we do not specify a default of '1' in the denominator, 574 * but this is being discussed. Other examples: 200 mcg/min or 200 575 * mcg/1 minute; 1 liter/8 hours.) 576 */ 577 public MedicationAdministrationDosageComponent setRate(Type value) { 578 this.rate = value; 579 return this; 580 } 581 582 protected void listChildren(List<Property> childrenList) { 583 super.listChildren(childrenList); 584 childrenList.add(new Property("text", "string", 585 "Free text dosage instructions can be used for cases where the instructions are too complex to code. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication.", 586 0, java.lang.Integer.MAX_VALUE, text)); 587 childrenList.add(new Property("site[x]", "CodeableConcept|Reference(BodySite)", 588 "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 589 0, java.lang.Integer.MAX_VALUE, site)); 590 childrenList.add(new Property("route", "CodeableConcept", 591 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 592 0, java.lang.Integer.MAX_VALUE, route)); 593 childrenList.add(new Property("method", "CodeableConcept", 594 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 595 0, java.lang.Integer.MAX_VALUE, method)); 596 childrenList.add(new Property("quantity", "SimpleQuantity", 597 "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 598 0, java.lang.Integer.MAX_VALUE, quantity)); 599 childrenList.add(new Property("rate[x]", "Ratio|Range", 600 "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 601 0, java.lang.Integer.MAX_VALUE, rate)); 602 } 603 604 @Override 605 public void setProperty(String name, Base value) throws FHIRException { 606 if (name.equals("text")) 607 this.text = castToString(value); // StringType 608 else if (name.equals("site[x]")) 609 this.site = (Type) value; // Type 610 else if (name.equals("route")) 611 this.route = castToCodeableConcept(value); // CodeableConcept 612 else if (name.equals("method")) 613 this.method = castToCodeableConcept(value); // CodeableConcept 614 else if (name.equals("quantity")) 615 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 616 else if (name.equals("rate[x]")) 617 this.rate = (Type) value; // Type 618 else 619 super.setProperty(name, value); 620 } 621 622 @Override 623 public Base addChild(String name) throws FHIRException { 624 if (name.equals("text")) { 625 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.text"); 626 } else if (name.equals("siteCodeableConcept")) { 627 this.site = new CodeableConcept(); 628 return this.site; 629 } else if (name.equals("siteReference")) { 630 this.site = new Reference(); 631 return this.site; 632 } else if (name.equals("route")) { 633 this.route = new CodeableConcept(); 634 return this.route; 635 } else if (name.equals("method")) { 636 this.method = new CodeableConcept(); 637 return this.method; 638 } else if (name.equals("quantity")) { 639 this.quantity = new SimpleQuantity(); 640 return this.quantity; 641 } else if (name.equals("rateRatio")) { 642 this.rate = new Ratio(); 643 return this.rate; 644 } else if (name.equals("rateRange")) { 645 this.rate = new Range(); 646 return this.rate; 647 } else 648 return super.addChild(name); 649 } 650 651 public MedicationAdministrationDosageComponent copy() { 652 MedicationAdministrationDosageComponent dst = new MedicationAdministrationDosageComponent(); 653 copyValues(dst); 654 dst.text = text == null ? null : text.copy(); 655 dst.site = site == null ? null : site.copy(); 656 dst.route = route == null ? null : route.copy(); 657 dst.method = method == null ? null : method.copy(); 658 dst.quantity = quantity == null ? null : quantity.copy(); 659 dst.rate = rate == null ? null : rate.copy(); 660 return dst; 661 } 662 663 @Override 664 public boolean equalsDeep(Base other) { 665 if (!super.equalsDeep(other)) 666 return false; 667 if (!(other instanceof MedicationAdministrationDosageComponent)) 668 return false; 669 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other; 670 return compareDeep(text, o.text, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 671 && compareDeep(method, o.method, true) && compareDeep(quantity, o.quantity, true) 672 && compareDeep(rate, o.rate, true); 673 } 674 675 @Override 676 public boolean equalsShallow(Base other) { 677 if (!super.equalsShallow(other)) 678 return false; 679 if (!(other instanceof MedicationAdministrationDosageComponent)) 680 return false; 681 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other; 682 return compareValues(text, o.text, true); 683 } 684 685 public boolean isEmpty() { 686 return super.isEmpty() && (text == null || text.isEmpty()) && (site == null || site.isEmpty()) 687 && (route == null || route.isEmpty()) && (method == null || method.isEmpty()) 688 && (quantity == null || quantity.isEmpty()) && (rate == null || rate.isEmpty()); 689 } 690 691 public String fhirType() { 692 return "MedicationAdministration.dosage"; 693 694 } 695 696 } 697 698 /** 699 * External identifier - FHIR will generate its own internal identifiers 700 * (probably URLs) which do not need to be explicitly managed by the resource. 701 * The identifier here is one that would be used by another non-FHIR system - 702 * for example an automated medication pump would provide a record each time it 703 * operated; an administration while the patient was off the ward might be made 704 * with a different system and entered after the event. Particularly important 705 * if these records have to be updated. 706 */ 707 @Child(name = "identifier", type = { 708 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 709 @Description(shortDefinition = "External identifier", formalDefinition = "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.") 710 protected List<Identifier> identifier; 711 712 /** 713 * Will generally be set to show that the administration has been completed. For 714 * some long running administrations such as infusions it is possible for an 715 * administration to be started but not completed or it may be paused while some 716 * other process is under way. 717 */ 718 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 719 @Description(shortDefinition = "in-progress | on-hold | completed | entered-in-error | stopped", formalDefinition = "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.") 720 protected Enumeration<MedicationAdministrationStatus> status; 721 722 /** 723 * The person or animal receiving the medication. 724 */ 725 @Child(name = "patient", type = { Patient.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 726 @Description(shortDefinition = "Who received medication", formalDefinition = "The person or animal receiving the medication.") 727 protected Reference patient; 728 729 /** 730 * The actual object that is the target of the reference (The person or animal 731 * receiving the medication.) 732 */ 733 protected Patient patientTarget; 734 735 /** 736 * The individual who was responsible for giving the medication to the patient. 737 */ 738 @Child(name = "practitioner", type = { Practitioner.class, Patient.class, 739 RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 740 @Description(shortDefinition = "Who administered substance", formalDefinition = "The individual who was responsible for giving the medication to the patient.") 741 protected Reference practitioner; 742 743 /** 744 * The actual object that is the target of the reference (The individual who was 745 * responsible for giving the medication to the patient.) 746 */ 747 protected Resource practitionerTarget; 748 749 /** 750 * The visit, admission or other contact between patient and health care 751 * provider the medication administration was performed as part of. 752 */ 753 @Child(name = "encounter", type = { Encounter.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 754 @Description(shortDefinition = "Encounter administered as part of", formalDefinition = "The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.") 755 protected Reference encounter; 756 757 /** 758 * The actual object that is the target of the reference (The visit, admission 759 * or other contact between patient and health care provider the medication 760 * administration was performed as part of.) 761 */ 762 protected Encounter encounterTarget; 763 764 /** 765 * The original request, instruction or authority to perform the administration. 766 */ 767 @Child(name = "prescription", type = { 768 MedicationOrder.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 769 @Description(shortDefinition = "Order administration performed against", formalDefinition = "The original request, instruction or authority to perform the administration.") 770 protected Reference prescription; 771 772 /** 773 * The actual object that is the target of the reference (The original request, 774 * instruction or authority to perform the administration.) 775 */ 776 protected MedicationOrder prescriptionTarget; 777 778 /** 779 * Set this to true if the record is saying that the medication was NOT 780 * administered. 781 */ 782 @Child(name = "wasNotGiven", type = { 783 BooleanType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 784 @Description(shortDefinition = "True if medication not administered", formalDefinition = "Set this to true if the record is saying that the medication was NOT administered.") 785 protected BooleanType wasNotGiven; 786 787 /** 788 * A code indicating why the administration was not performed. 789 */ 790 @Child(name = "reasonNotGiven", type = { 791 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 792 @Description(shortDefinition = "Reason administration not performed", formalDefinition = "A code indicating why the administration was not performed.") 793 protected List<CodeableConcept> reasonNotGiven; 794 795 /** 796 * A code indicating why the medication was given. 797 */ 798 @Child(name = "reasonGiven", type = { 799 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 800 @Description(shortDefinition = "Reason administration performed", formalDefinition = "A code indicating why the medication was given.") 801 protected List<CodeableConcept> reasonGiven; 802 803 /** 804 * A specific date/time or interval of time during which the administration took 805 * place (or did not take place, when the 'notGiven' attribute is true). For 806 * many administrations, such as swallowing a tablet the use of dateTime is more 807 * appropriate. 808 */ 809 @Child(name = "effectiveTime", type = { DateTimeType.class, 810 Period.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 811 @Description(shortDefinition = "Start and end time of administration", formalDefinition = "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.") 812 protected Type effectiveTime; 813 814 /** 815 * Identifies the medication that was administered. This is either a link to a 816 * resource representing the details of the medication or a simple attribute 817 * carrying a code that identifies the medication from a known list of 818 * medications. 819 */ 820 @Child(name = "medication", type = { CodeableConcept.class, 821 Medication.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 822 @Description(shortDefinition = "What was administered", formalDefinition = "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 823 protected Type medication; 824 825 /** 826 * The device used in administering the medication to the patient. For example, 827 * a particular infusion pump. 828 */ 829 @Child(name = "device", type = { 830 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 831 @Description(shortDefinition = "Device used to administer", formalDefinition = "The device used in administering the medication to the patient. For example, a particular infusion pump.") 832 protected List<Reference> device; 833 /** 834 * The actual objects that are the target of the reference (The device used in 835 * administering the medication to the patient. For example, a particular 836 * infusion pump.) 837 */ 838 protected List<Device> deviceTarget; 839 840 /** 841 * Extra information about the medication administration that is not conveyed by 842 * the other attributes. 843 */ 844 @Child(name = "note", type = { StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 845 @Description(shortDefinition = "Information about the administration", formalDefinition = "Extra information about the medication administration that is not conveyed by the other attributes.") 846 protected StringType note; 847 848 /** 849 * Describes the medication dosage information details e.g. dose, rate, site, 850 * route, etc. 851 */ 852 @Child(name = "dosage", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = true) 853 @Description(shortDefinition = "Details of how medication was taken", formalDefinition = "Describes the medication dosage information details e.g. dose, rate, site, route, etc.") 854 protected MedicationAdministrationDosageComponent dosage; 855 856 private static final long serialVersionUID = -669616345L; 857 858 /* 859 * Constructor 860 */ 861 public MedicationAdministration() { 862 super(); 863 } 864 865 /* 866 * Constructor 867 */ 868 public MedicationAdministration(Enumeration<MedicationAdministrationStatus> status, Reference patient, 869 Type effectiveTime, Type medication) { 870 super(); 871 this.status = status; 872 this.patient = patient; 873 this.effectiveTime = effectiveTime; 874 this.medication = medication; 875 } 876 877 /** 878 * @return {@link #identifier} (External identifier - FHIR will generate its own 879 * internal identifiers (probably URLs) which do not need to be 880 * explicitly managed by the resource. The identifier here is one that 881 * would be used by another non-FHIR system - for example an automated 882 * medication pump would provide a record each time it operated; an 883 * administration while the patient was off the ward might be made with 884 * a different system and entered after the event. Particularly 885 * important if these records have to be updated.) 886 */ 887 public List<Identifier> getIdentifier() { 888 if (this.identifier == null) 889 this.identifier = new ArrayList<Identifier>(); 890 return this.identifier; 891 } 892 893 public boolean hasIdentifier() { 894 if (this.identifier == null) 895 return false; 896 for (Identifier item : this.identifier) 897 if (!item.isEmpty()) 898 return true; 899 return false; 900 } 901 902 /** 903 * @return {@link #identifier} (External identifier - FHIR will generate its own 904 * internal identifiers (probably URLs) which do not need to be 905 * explicitly managed by the resource. The identifier here is one that 906 * would be used by another non-FHIR system - for example an automated 907 * medication pump would provide a record each time it operated; an 908 * administration while the patient was off the ward might be made with 909 * a different system and entered after the event. Particularly 910 * important if these records have to be updated.) 911 */ 912 // syntactic sugar 913 public Identifier addIdentifier() { // 3 914 Identifier t = new Identifier(); 915 if (this.identifier == null) 916 this.identifier = new ArrayList<Identifier>(); 917 this.identifier.add(t); 918 return t; 919 } 920 921 // syntactic sugar 922 public MedicationAdministration addIdentifier(Identifier t) { // 3 923 if (t == null) 924 return this; 925 if (this.identifier == null) 926 this.identifier = new ArrayList<Identifier>(); 927 this.identifier.add(t); 928 return this; 929 } 930 931 /** 932 * @return {@link #status} (Will generally be set to show that the 933 * administration has been completed. For some long running 934 * administrations such as infusions it is possible for an 935 * administration to be started but not completed or it may be paused 936 * while some other process is under way.). This is the underlying 937 * object with id, value and extensions. The accessor "getStatus" gives 938 * direct access to the value 939 */ 940 public Enumeration<MedicationAdministrationStatus> getStatusElement() { 941 if (this.status == null) 942 if (Configuration.errorOnAutoCreate()) 943 throw new Error("Attempt to auto-create MedicationAdministration.status"); 944 else if (Configuration.doAutoCreate()) 945 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); // bb 946 return this.status; 947 } 948 949 public boolean hasStatusElement() { 950 return this.status != null && !this.status.isEmpty(); 951 } 952 953 public boolean hasStatus() { 954 return this.status != null && !this.status.isEmpty(); 955 } 956 957 /** 958 * @param value {@link #status} (Will generally be set to show that the 959 * administration has been completed. For some long running 960 * administrations such as infusions it is possible for an 961 * administration to be started but not completed or it may be 962 * paused while some other process is under way.). This is the 963 * underlying object with id, value and extensions. The accessor 964 * "getStatus" gives direct access to the value 965 */ 966 public MedicationAdministration setStatusElement(Enumeration<MedicationAdministrationStatus> value) { 967 this.status = value; 968 return this; 969 } 970 971 /** 972 * @return Will generally be set to show that the administration has been 973 * completed. For some long running administrations such as infusions it 974 * is possible for an administration to be started but not completed or 975 * it may be paused while some other process is under way. 976 */ 977 public MedicationAdministrationStatus getStatus() { 978 return this.status == null ? null : this.status.getValue(); 979 } 980 981 /** 982 * @param value Will generally be set to show that the administration has been 983 * completed. For some long running administrations such as 984 * infusions it is possible for an administration to be started but 985 * not completed or it may be paused while some other process is 986 * under way. 987 */ 988 public MedicationAdministration setStatus(MedicationAdministrationStatus value) { 989 if (this.status == null) 990 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); 991 this.status.setValue(value); 992 return this; 993 } 994 995 /** 996 * @return {@link #patient} (The person or animal receiving the medication.) 997 */ 998 public Reference getPatient() { 999 if (this.patient == null) 1000 if (Configuration.errorOnAutoCreate()) 1001 throw new Error("Attempt to auto-create MedicationAdministration.patient"); 1002 else if (Configuration.doAutoCreate()) 1003 this.patient = new Reference(); // cc 1004 return this.patient; 1005 } 1006 1007 public boolean hasPatient() { 1008 return this.patient != null && !this.patient.isEmpty(); 1009 } 1010 1011 /** 1012 * @param value {@link #patient} (The person or animal receiving the 1013 * medication.) 1014 */ 1015 public MedicationAdministration setPatient(Reference value) { 1016 this.patient = value; 1017 return this; 1018 } 1019 1020 /** 1021 * @return {@link #patient} The actual object that is the target of the 1022 * reference. The reference library doesn't populate this, but you can 1023 * use it to hold the resource if you resolve it. (The person or animal 1024 * receiving the medication.) 1025 */ 1026 public Patient getPatientTarget() { 1027 if (this.patientTarget == null) 1028 if (Configuration.errorOnAutoCreate()) 1029 throw new Error("Attempt to auto-create MedicationAdministration.patient"); 1030 else if (Configuration.doAutoCreate()) 1031 this.patientTarget = new Patient(); // aa 1032 return this.patientTarget; 1033 } 1034 1035 /** 1036 * @param value {@link #patient} The actual object that is the target of the 1037 * reference. The reference library doesn't use these, but you can 1038 * use it to hold the resource if you resolve it. (The person or 1039 * animal receiving the medication.) 1040 */ 1041 public MedicationAdministration setPatientTarget(Patient value) { 1042 this.patientTarget = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #practitioner} (The individual who was responsible for giving 1048 * the medication to the patient.) 1049 */ 1050 public Reference getPractitioner() { 1051 if (this.practitioner == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create MedicationAdministration.practitioner"); 1054 else if (Configuration.doAutoCreate()) 1055 this.practitioner = new Reference(); // cc 1056 return this.practitioner; 1057 } 1058 1059 public boolean hasPractitioner() { 1060 return this.practitioner != null && !this.practitioner.isEmpty(); 1061 } 1062 1063 /** 1064 * @param value {@link #practitioner} (The individual who was responsible for 1065 * giving the medication to the patient.) 1066 */ 1067 public MedicationAdministration setPractitioner(Reference value) { 1068 this.practitioner = value; 1069 return this; 1070 } 1071 1072 /** 1073 * @return {@link #practitioner} The actual object that is the target of the 1074 * reference. The reference library doesn't populate this, but you can 1075 * use it to hold the resource if you resolve it. (The individual who 1076 * was responsible for giving the medication to the patient.) 1077 */ 1078 public Resource getPractitionerTarget() { 1079 return this.practitionerTarget; 1080 } 1081 1082 /** 1083 * @param value {@link #practitioner} The actual object that is the target of 1084 * the reference. The reference library doesn't use these, but you 1085 * can use it to hold the resource if you resolve it. (The 1086 * individual who was responsible for giving the medication to the 1087 * patient.) 1088 */ 1089 public MedicationAdministration setPractitionerTarget(Resource value) { 1090 this.practitionerTarget = value; 1091 return this; 1092 } 1093 1094 /** 1095 * @return {@link #encounter} (The visit, admission or other contact between 1096 * patient and health care provider the medication administration was 1097 * performed as part of.) 1098 */ 1099 public Reference getEncounter() { 1100 if (this.encounter == null) 1101 if (Configuration.errorOnAutoCreate()) 1102 throw new Error("Attempt to auto-create MedicationAdministration.encounter"); 1103 else if (Configuration.doAutoCreate()) 1104 this.encounter = new Reference(); // cc 1105 return this.encounter; 1106 } 1107 1108 public boolean hasEncounter() { 1109 return this.encounter != null && !this.encounter.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #encounter} (The visit, admission or other contact 1114 * between patient and health care provider the medication 1115 * administration was performed as part of.) 1116 */ 1117 public MedicationAdministration setEncounter(Reference value) { 1118 this.encounter = value; 1119 return this; 1120 } 1121 1122 /** 1123 * @return {@link #encounter} The actual object that is the target of the 1124 * reference. The reference library doesn't populate this, but you can 1125 * use it to hold the resource if you resolve it. (The visit, admission 1126 * or other contact between patient and health care provider the 1127 * medication administration was performed as part of.) 1128 */ 1129 public Encounter getEncounterTarget() { 1130 if (this.encounterTarget == null) 1131 if (Configuration.errorOnAutoCreate()) 1132 throw new Error("Attempt to auto-create MedicationAdministration.encounter"); 1133 else if (Configuration.doAutoCreate()) 1134 this.encounterTarget = new Encounter(); // aa 1135 return this.encounterTarget; 1136 } 1137 1138 /** 1139 * @param value {@link #encounter} The actual object that is the target of the 1140 * reference. The reference library doesn't use these, but you can 1141 * use it to hold the resource if you resolve it. (The visit, 1142 * admission or other contact between patient and health care 1143 * provider the medication administration was performed as part 1144 * of.) 1145 */ 1146 public MedicationAdministration setEncounterTarget(Encounter value) { 1147 this.encounterTarget = value; 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #prescription} (The original request, instruction or authority 1153 * to perform the administration.) 1154 */ 1155 public Reference getPrescription() { 1156 if (this.prescription == null) 1157 if (Configuration.errorOnAutoCreate()) 1158 throw new Error("Attempt to auto-create MedicationAdministration.prescription"); 1159 else if (Configuration.doAutoCreate()) 1160 this.prescription = new Reference(); // cc 1161 return this.prescription; 1162 } 1163 1164 public boolean hasPrescription() { 1165 return this.prescription != null && !this.prescription.isEmpty(); 1166 } 1167 1168 /** 1169 * @param value {@link #prescription} (The original request, instruction or 1170 * authority to perform the administration.) 1171 */ 1172 public MedicationAdministration setPrescription(Reference value) { 1173 this.prescription = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return {@link #prescription} The actual object that is the target of the 1179 * reference. The reference library doesn't populate this, but you can 1180 * use it to hold the resource if you resolve it. (The original request, 1181 * instruction or authority to perform the administration.) 1182 */ 1183 public MedicationOrder getPrescriptionTarget() { 1184 if (this.prescriptionTarget == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create MedicationAdministration.prescription"); 1187 else if (Configuration.doAutoCreate()) 1188 this.prescriptionTarget = new MedicationOrder(); // aa 1189 return this.prescriptionTarget; 1190 } 1191 1192 /** 1193 * @param value {@link #prescription} The actual object that is the target of 1194 * the reference. The reference library doesn't use these, but you 1195 * can use it to hold the resource if you resolve it. (The original 1196 * request, instruction or authority to perform the 1197 * administration.) 1198 */ 1199 public MedicationAdministration setPrescriptionTarget(MedicationOrder value) { 1200 this.prescriptionTarget = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #wasNotGiven} (Set this to true if the record is saying that 1206 * the medication was NOT administered.). This is the underlying object 1207 * with id, value and extensions. The accessor "getWasNotGiven" gives 1208 * direct access to the value 1209 */ 1210 public BooleanType getWasNotGivenElement() { 1211 if (this.wasNotGiven == null) 1212 if (Configuration.errorOnAutoCreate()) 1213 throw new Error("Attempt to auto-create MedicationAdministration.wasNotGiven"); 1214 else if (Configuration.doAutoCreate()) 1215 this.wasNotGiven = new BooleanType(); // bb 1216 return this.wasNotGiven; 1217 } 1218 1219 public boolean hasWasNotGivenElement() { 1220 return this.wasNotGiven != null && !this.wasNotGiven.isEmpty(); 1221 } 1222 1223 public boolean hasWasNotGiven() { 1224 return this.wasNotGiven != null && !this.wasNotGiven.isEmpty(); 1225 } 1226 1227 /** 1228 * @param value {@link #wasNotGiven} (Set this to true if the record is saying 1229 * that the medication was NOT administered.). This is the 1230 * underlying object with id, value and extensions. The accessor 1231 * "getWasNotGiven" gives direct access to the value 1232 */ 1233 public MedicationAdministration setWasNotGivenElement(BooleanType value) { 1234 this.wasNotGiven = value; 1235 return this; 1236 } 1237 1238 /** 1239 * @return Set this to true if the record is saying that the medication was NOT 1240 * administered. 1241 */ 1242 public boolean getWasNotGiven() { 1243 return this.wasNotGiven == null || this.wasNotGiven.isEmpty() ? false : this.wasNotGiven.getValue(); 1244 } 1245 1246 /** 1247 * @param value Set this to true if the record is saying that the medication was 1248 * NOT administered. 1249 */ 1250 public MedicationAdministration setWasNotGiven(boolean value) { 1251 if (this.wasNotGiven == null) 1252 this.wasNotGiven = new BooleanType(); 1253 this.wasNotGiven.setValue(value); 1254 return this; 1255 } 1256 1257 /** 1258 * @return {@link #reasonNotGiven} (A code indicating why the administration was 1259 * not performed.) 1260 */ 1261 public List<CodeableConcept> getReasonNotGiven() { 1262 if (this.reasonNotGiven == null) 1263 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1264 return this.reasonNotGiven; 1265 } 1266 1267 public boolean hasReasonNotGiven() { 1268 if (this.reasonNotGiven == null) 1269 return false; 1270 for (CodeableConcept item : this.reasonNotGiven) 1271 if (!item.isEmpty()) 1272 return true; 1273 return false; 1274 } 1275 1276 /** 1277 * @return {@link #reasonNotGiven} (A code indicating why the administration was 1278 * not performed.) 1279 */ 1280 // syntactic sugar 1281 public CodeableConcept addReasonNotGiven() { // 3 1282 CodeableConcept t = new CodeableConcept(); 1283 if (this.reasonNotGiven == null) 1284 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1285 this.reasonNotGiven.add(t); 1286 return t; 1287 } 1288 1289 // syntactic sugar 1290 public MedicationAdministration addReasonNotGiven(CodeableConcept t) { // 3 1291 if (t == null) 1292 return this; 1293 if (this.reasonNotGiven == null) 1294 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1295 this.reasonNotGiven.add(t); 1296 return this; 1297 } 1298 1299 /** 1300 * @return {@link #reasonGiven} (A code indicating why the medication was 1301 * given.) 1302 */ 1303 public List<CodeableConcept> getReasonGiven() { 1304 if (this.reasonGiven == null) 1305 this.reasonGiven = new ArrayList<CodeableConcept>(); 1306 return this.reasonGiven; 1307 } 1308 1309 public boolean hasReasonGiven() { 1310 if (this.reasonGiven == null) 1311 return false; 1312 for (CodeableConcept item : this.reasonGiven) 1313 if (!item.isEmpty()) 1314 return true; 1315 return false; 1316 } 1317 1318 /** 1319 * @return {@link #reasonGiven} (A code indicating why the medication was 1320 * given.) 1321 */ 1322 // syntactic sugar 1323 public CodeableConcept addReasonGiven() { // 3 1324 CodeableConcept t = new CodeableConcept(); 1325 if (this.reasonGiven == null) 1326 this.reasonGiven = new ArrayList<CodeableConcept>(); 1327 this.reasonGiven.add(t); 1328 return t; 1329 } 1330 1331 // syntactic sugar 1332 public MedicationAdministration addReasonGiven(CodeableConcept t) { // 3 1333 if (t == null) 1334 return this; 1335 if (this.reasonGiven == null) 1336 this.reasonGiven = new ArrayList<CodeableConcept>(); 1337 this.reasonGiven.add(t); 1338 return this; 1339 } 1340 1341 /** 1342 * @return {@link #effectiveTime} (A specific date/time or interval of time 1343 * during which the administration took place (or did not take place, 1344 * when the 'notGiven' attribute is true). For many administrations, 1345 * such as swallowing a tablet the use of dateTime is more appropriate.) 1346 */ 1347 public Type getEffectiveTime() { 1348 return this.effectiveTime; 1349 } 1350 1351 /** 1352 * @return {@link #effectiveTime} (A specific date/time or interval of time 1353 * during which the administration took place (or did not take place, 1354 * when the 'notGiven' attribute is true). For many administrations, 1355 * such as swallowing a tablet the use of dateTime is more appropriate.) 1356 */ 1357 public DateTimeType getEffectiveTimeDateTimeType() throws FHIRException { 1358 if (!(this.effectiveTime instanceof DateTimeType)) 1359 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1360 + this.effectiveTime.getClass().getName() + " was encountered"); 1361 return (DateTimeType) this.effectiveTime; 1362 } 1363 1364 public boolean hasEffectiveTimeDateTimeType() { 1365 return this.effectiveTime instanceof DateTimeType; 1366 } 1367 1368 /** 1369 * @return {@link #effectiveTime} (A specific date/time or interval of time 1370 * during which the administration took place (or did not take place, 1371 * when the 'notGiven' attribute is true). For many administrations, 1372 * such as swallowing a tablet the use of dateTime is more appropriate.) 1373 */ 1374 public Period getEffectiveTimePeriod() throws FHIRException { 1375 if (!(this.effectiveTime instanceof Period)) 1376 throw new FHIRException("Type mismatch: the type Period was expected, but " 1377 + this.effectiveTime.getClass().getName() + " was encountered"); 1378 return (Period) this.effectiveTime; 1379 } 1380 1381 public boolean hasEffectiveTimePeriod() { 1382 return this.effectiveTime instanceof Period; 1383 } 1384 1385 public boolean hasEffectiveTime() { 1386 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 1387 } 1388 1389 /** 1390 * @param value {@link #effectiveTime} (A specific date/time or interval of time 1391 * during which the administration took place (or did not take 1392 * place, when the 'notGiven' attribute is true). For many 1393 * administrations, such as swallowing a tablet the use of dateTime 1394 * is more appropriate.) 1395 */ 1396 public MedicationAdministration setEffectiveTime(Type value) { 1397 this.effectiveTime = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #medication} (Identifies the medication that was administered. 1403 * This is either a link to a resource representing the details of the 1404 * medication or a simple attribute carrying a code that identifies the 1405 * medication from a known list of medications.) 1406 */ 1407 public Type getMedication() { 1408 return this.medication; 1409 } 1410 1411 /** 1412 * @return {@link #medication} (Identifies the medication that was administered. 1413 * This is either a link to a resource representing the details of the 1414 * medication or a simple attribute carrying a code that identifies the 1415 * medication from a known list of medications.) 1416 */ 1417 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1418 if (!(this.medication instanceof CodeableConcept)) 1419 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1420 + this.medication.getClass().getName() + " was encountered"); 1421 return (CodeableConcept) this.medication; 1422 } 1423 1424 public boolean hasMedicationCodeableConcept() { 1425 return this.medication instanceof CodeableConcept; 1426 } 1427 1428 /** 1429 * @return {@link #medication} (Identifies the medication that was administered. 1430 * This is either a link to a resource representing the details of the 1431 * medication or a simple attribute carrying a code that identifies the 1432 * medication from a known list of medications.) 1433 */ 1434 public Reference getMedicationReference() throws FHIRException { 1435 if (!(this.medication instanceof Reference)) 1436 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1437 + this.medication.getClass().getName() + " was encountered"); 1438 return (Reference) this.medication; 1439 } 1440 1441 public boolean hasMedicationReference() { 1442 return this.medication instanceof Reference; 1443 } 1444 1445 public boolean hasMedication() { 1446 return this.medication != null && !this.medication.isEmpty(); 1447 } 1448 1449 /** 1450 * @param value {@link #medication} (Identifies the medication that was 1451 * administered. This is either a link to a resource representing 1452 * the details of the medication or a simple attribute carrying a 1453 * code that identifies the medication from a known list of 1454 * medications.) 1455 */ 1456 public MedicationAdministration setMedication(Type value) { 1457 this.medication = value; 1458 return this; 1459 } 1460 1461 /** 1462 * @return {@link #device} (The device used in administering the medication to 1463 * the patient. For example, a particular infusion pump.) 1464 */ 1465 public List<Reference> getDevice() { 1466 if (this.device == null) 1467 this.device = new ArrayList<Reference>(); 1468 return this.device; 1469 } 1470 1471 public boolean hasDevice() { 1472 if (this.device == null) 1473 return false; 1474 for (Reference item : this.device) 1475 if (!item.isEmpty()) 1476 return true; 1477 return false; 1478 } 1479 1480 /** 1481 * @return {@link #device} (The device used in administering the medication to 1482 * the patient. For example, a particular infusion pump.) 1483 */ 1484 // syntactic sugar 1485 public Reference addDevice() { // 3 1486 Reference t = new Reference(); 1487 if (this.device == null) 1488 this.device = new ArrayList<Reference>(); 1489 this.device.add(t); 1490 return t; 1491 } 1492 1493 // syntactic sugar 1494 public MedicationAdministration addDevice(Reference t) { // 3 1495 if (t == null) 1496 return this; 1497 if (this.device == null) 1498 this.device = new ArrayList<Reference>(); 1499 this.device.add(t); 1500 return this; 1501 } 1502 1503 /** 1504 * @return {@link #device} (The actual objects that are the target of the 1505 * reference. The reference library doesn't populate this, but you can 1506 * use this to hold the resources if you resolvethemt. The device used 1507 * in administering the medication to the patient. For example, a 1508 * particular infusion pump.) 1509 */ 1510 public List<Device> getDeviceTarget() { 1511 if (this.deviceTarget == null) 1512 this.deviceTarget = new ArrayList<Device>(); 1513 return this.deviceTarget; 1514 } 1515 1516 // syntactic sugar 1517 /** 1518 * @return {@link #device} (Add an actual object that is the target of the 1519 * reference. The reference library doesn't use these, but you can use 1520 * this to hold the resources if you resolvethemt. The device used in 1521 * administering the medication to the patient. For example, a 1522 * particular infusion pump.) 1523 */ 1524 public Device addDeviceTarget() { 1525 Device r = new Device(); 1526 if (this.deviceTarget == null) 1527 this.deviceTarget = new ArrayList<Device>(); 1528 this.deviceTarget.add(r); 1529 return r; 1530 } 1531 1532 /** 1533 * @return {@link #note} (Extra information about the medication administration 1534 * that is not conveyed by the other attributes.). This is the 1535 * underlying object with id, value and extensions. The accessor 1536 * "getNote" gives direct access to the value 1537 */ 1538 public StringType getNoteElement() { 1539 if (this.note == null) 1540 if (Configuration.errorOnAutoCreate()) 1541 throw new Error("Attempt to auto-create MedicationAdministration.note"); 1542 else if (Configuration.doAutoCreate()) 1543 this.note = new StringType(); // bb 1544 return this.note; 1545 } 1546 1547 public boolean hasNoteElement() { 1548 return this.note != null && !this.note.isEmpty(); 1549 } 1550 1551 public boolean hasNote() { 1552 return this.note != null && !this.note.isEmpty(); 1553 } 1554 1555 /** 1556 * @param value {@link #note} (Extra information about the medication 1557 * administration that is not conveyed by the other attributes.). 1558 * This is the underlying object with id, value and extensions. The 1559 * accessor "getNote" gives direct access to the value 1560 */ 1561 public MedicationAdministration setNoteElement(StringType value) { 1562 this.note = value; 1563 return this; 1564 } 1565 1566 /** 1567 * @return Extra information about the medication administration that is not 1568 * conveyed by the other attributes. 1569 */ 1570 public String getNote() { 1571 return this.note == null ? null : this.note.getValue(); 1572 } 1573 1574 /** 1575 * @param value Extra information about the medication administration that is 1576 * not conveyed by the other attributes. 1577 */ 1578 public MedicationAdministration setNote(String value) { 1579 if (Utilities.noString(value)) 1580 this.note = null; 1581 else { 1582 if (this.note == null) 1583 this.note = new StringType(); 1584 this.note.setValue(value); 1585 } 1586 return this; 1587 } 1588 1589 /** 1590 * @return {@link #dosage} (Describes the medication dosage information details 1591 * e.g. dose, rate, site, route, etc.) 1592 */ 1593 public MedicationAdministrationDosageComponent getDosage() { 1594 if (this.dosage == null) 1595 if (Configuration.errorOnAutoCreate()) 1596 throw new Error("Attempt to auto-create MedicationAdministration.dosage"); 1597 else if (Configuration.doAutoCreate()) 1598 this.dosage = new MedicationAdministrationDosageComponent(); // cc 1599 return this.dosage; 1600 } 1601 1602 public boolean hasDosage() { 1603 return this.dosage != null && !this.dosage.isEmpty(); 1604 } 1605 1606 /** 1607 * @param value {@link #dosage} (Describes the medication dosage information 1608 * details e.g. dose, rate, site, route, etc.) 1609 */ 1610 public MedicationAdministration setDosage(MedicationAdministrationDosageComponent value) { 1611 this.dosage = value; 1612 return this; 1613 } 1614 1615 protected void listChildren(List<Property> childrenList) { 1616 super.listChildren(childrenList); 1617 childrenList.add(new Property("identifier", "Identifier", 1618 "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 1619 0, java.lang.Integer.MAX_VALUE, identifier)); 1620 childrenList.add(new Property("status", "code", 1621 "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 1622 0, java.lang.Integer.MAX_VALUE, status)); 1623 childrenList.add(new Property("patient", "Reference(Patient)", "The person or animal receiving the medication.", 0, 1624 java.lang.Integer.MAX_VALUE, patient)); 1625 childrenList.add(new Property("practitioner", "Reference(Practitioner|Patient|RelatedPerson)", 1626 "The individual who was responsible for giving the medication to the patient.", 0, java.lang.Integer.MAX_VALUE, 1627 practitioner)); 1628 childrenList.add(new Property("encounter", "Reference(Encounter)", 1629 "The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.", 1630 0, java.lang.Integer.MAX_VALUE, encounter)); 1631 childrenList.add(new Property("prescription", "Reference(MedicationOrder)", 1632 "The original request, instruction or authority to perform the administration.", 0, java.lang.Integer.MAX_VALUE, 1633 prescription)); 1634 childrenList.add(new Property("wasNotGiven", "boolean", 1635 "Set this to true if the record is saying that the medication was NOT administered.", 0, 1636 java.lang.Integer.MAX_VALUE, wasNotGiven)); 1637 childrenList.add(new Property("reasonNotGiven", "CodeableConcept", 1638 "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven)); 1639 childrenList.add(new Property("reasonGiven", "CodeableConcept", "A code indicating why the medication was given.", 1640 0, java.lang.Integer.MAX_VALUE, reasonGiven)); 1641 childrenList.add(new Property("effectiveTime[x]", "dateTime|Period", 1642 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 1643 0, java.lang.Integer.MAX_VALUE, effectiveTime)); 1644 childrenList.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1645 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1646 0, java.lang.Integer.MAX_VALUE, medication)); 1647 childrenList.add(new Property("device", "Reference(Device)", 1648 "The device used in administering the medication to the patient. For example, a particular infusion pump.", 0, 1649 java.lang.Integer.MAX_VALUE, device)); 1650 childrenList.add(new Property("note", "string", 1651 "Extra information about the medication administration that is not conveyed by the other attributes.", 0, 1652 java.lang.Integer.MAX_VALUE, note)); 1653 childrenList.add(new Property("dosage", "", 1654 "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1655 java.lang.Integer.MAX_VALUE, dosage)); 1656 } 1657 1658 @Override 1659 public void setProperty(String name, Base value) throws FHIRException { 1660 if (name.equals("identifier")) 1661 this.getIdentifier().add(castToIdentifier(value)); 1662 else if (name.equals("status")) 1663 this.status = new MedicationAdministrationStatusEnumFactory().fromType(value); // Enumeration<MedicationAdministrationStatus> 1664 else if (name.equals("patient")) 1665 this.patient = castToReference(value); // Reference 1666 else if (name.equals("practitioner")) 1667 this.practitioner = castToReference(value); // Reference 1668 else if (name.equals("encounter")) 1669 this.encounter = castToReference(value); // Reference 1670 else if (name.equals("prescription")) 1671 this.prescription = castToReference(value); // Reference 1672 else if (name.equals("wasNotGiven")) 1673 this.wasNotGiven = castToBoolean(value); // BooleanType 1674 else if (name.equals("reasonNotGiven")) 1675 this.getReasonNotGiven().add(castToCodeableConcept(value)); 1676 else if (name.equals("reasonGiven")) 1677 this.getReasonGiven().add(castToCodeableConcept(value)); 1678 else if (name.equals("effectiveTime[x]")) 1679 this.effectiveTime = (Type) value; // Type 1680 else if (name.equals("medication[x]")) 1681 this.medication = (Type) value; // Type 1682 else if (name.equals("device")) 1683 this.getDevice().add(castToReference(value)); 1684 else if (name.equals("note")) 1685 this.note = castToString(value); // StringType 1686 else if (name.equals("dosage")) 1687 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 1688 else 1689 super.setProperty(name, value); 1690 } 1691 1692 @Override 1693 public Base addChild(String name) throws FHIRException { 1694 if (name.equals("identifier")) { 1695 return addIdentifier(); 1696 } else if (name.equals("status")) { 1697 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.status"); 1698 } else if (name.equals("patient")) { 1699 this.patient = new Reference(); 1700 return this.patient; 1701 } else if (name.equals("practitioner")) { 1702 this.practitioner = new Reference(); 1703 return this.practitioner; 1704 } else if (name.equals("encounter")) { 1705 this.encounter = new Reference(); 1706 return this.encounter; 1707 } else if (name.equals("prescription")) { 1708 this.prescription = new Reference(); 1709 return this.prescription; 1710 } else if (name.equals("wasNotGiven")) { 1711 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.wasNotGiven"); 1712 } else if (name.equals("reasonNotGiven")) { 1713 return addReasonNotGiven(); 1714 } else if (name.equals("reasonGiven")) { 1715 return addReasonGiven(); 1716 } else if (name.equals("effectiveTimeDateTime")) { 1717 this.effectiveTime = new DateTimeType(); 1718 return this.effectiveTime; 1719 } else if (name.equals("effectiveTimePeriod")) { 1720 this.effectiveTime = new Period(); 1721 return this.effectiveTime; 1722 } else if (name.equals("medicationCodeableConcept")) { 1723 this.medication = new CodeableConcept(); 1724 return this.medication; 1725 } else if (name.equals("medicationReference")) { 1726 this.medication = new Reference(); 1727 return this.medication; 1728 } else if (name.equals("device")) { 1729 return addDevice(); 1730 } else if (name.equals("note")) { 1731 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.note"); 1732 } else if (name.equals("dosage")) { 1733 this.dosage = new MedicationAdministrationDosageComponent(); 1734 return this.dosage; 1735 } else 1736 return super.addChild(name); 1737 } 1738 1739 public String fhirType() { 1740 return "MedicationAdministration"; 1741 1742 } 1743 1744 public MedicationAdministration copy() { 1745 MedicationAdministration dst = new MedicationAdministration(); 1746 copyValues(dst); 1747 if (identifier != null) { 1748 dst.identifier = new ArrayList<Identifier>(); 1749 for (Identifier i : identifier) 1750 dst.identifier.add(i.copy()); 1751 } 1752 ; 1753 dst.status = status == null ? null : status.copy(); 1754 dst.patient = patient == null ? null : patient.copy(); 1755 dst.practitioner = practitioner == null ? null : practitioner.copy(); 1756 dst.encounter = encounter == null ? null : encounter.copy(); 1757 dst.prescription = prescription == null ? null : prescription.copy(); 1758 dst.wasNotGiven = wasNotGiven == null ? null : wasNotGiven.copy(); 1759 if (reasonNotGiven != null) { 1760 dst.reasonNotGiven = new ArrayList<CodeableConcept>(); 1761 for (CodeableConcept i : reasonNotGiven) 1762 dst.reasonNotGiven.add(i.copy()); 1763 } 1764 ; 1765 if (reasonGiven != null) { 1766 dst.reasonGiven = new ArrayList<CodeableConcept>(); 1767 for (CodeableConcept i : reasonGiven) 1768 dst.reasonGiven.add(i.copy()); 1769 } 1770 ; 1771 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 1772 dst.medication = medication == null ? null : medication.copy(); 1773 if (device != null) { 1774 dst.device = new ArrayList<Reference>(); 1775 for (Reference i : device) 1776 dst.device.add(i.copy()); 1777 } 1778 ; 1779 dst.note = note == null ? null : note.copy(); 1780 dst.dosage = dosage == null ? null : dosage.copy(); 1781 return dst; 1782 } 1783 1784 protected MedicationAdministration typedCopy() { 1785 return copy(); 1786 } 1787 1788 @Override 1789 public boolean equalsDeep(Base other) { 1790 if (!super.equalsDeep(other)) 1791 return false; 1792 if (!(other instanceof MedicationAdministration)) 1793 return false; 1794 MedicationAdministration o = (MedicationAdministration) other; 1795 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1796 && compareDeep(patient, o.patient, true) && compareDeep(practitioner, o.practitioner, true) 1797 && compareDeep(encounter, o.encounter, true) && compareDeep(prescription, o.prescription, true) 1798 && compareDeep(wasNotGiven, o.wasNotGiven, true) && compareDeep(reasonNotGiven, o.reasonNotGiven, true) 1799 && compareDeep(reasonGiven, o.reasonGiven, true) && compareDeep(effectiveTime, o.effectiveTime, true) 1800 && compareDeep(medication, o.medication, true) && compareDeep(device, o.device, true) 1801 && compareDeep(note, o.note, true) && compareDeep(dosage, o.dosage, true); 1802 } 1803 1804 @Override 1805 public boolean equalsShallow(Base other) { 1806 if (!super.equalsShallow(other)) 1807 return false; 1808 if (!(other instanceof MedicationAdministration)) 1809 return false; 1810 MedicationAdministration o = (MedicationAdministration) other; 1811 return compareValues(status, o.status, true) && compareValues(wasNotGiven, o.wasNotGiven, true) 1812 && compareValues(note, o.note, true); 1813 } 1814 1815 public boolean isEmpty() { 1816 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 1817 && (patient == null || patient.isEmpty()) && (practitioner == null || practitioner.isEmpty()) 1818 && (encounter == null || encounter.isEmpty()) && (prescription == null || prescription.isEmpty()) 1819 && (wasNotGiven == null || wasNotGiven.isEmpty()) && (reasonNotGiven == null || reasonNotGiven.isEmpty()) 1820 && (reasonGiven == null || reasonGiven.isEmpty()) && (effectiveTime == null || effectiveTime.isEmpty()) 1821 && (medication == null || medication.isEmpty()) && (device == null || device.isEmpty()) 1822 && (note == null || note.isEmpty()) && (dosage == null || dosage.isEmpty()); 1823 } 1824 1825 @Override 1826 public ResourceType getResourceType() { 1827 return ResourceType.MedicationAdministration; 1828 } 1829 1830 @SearchParamDefinition(name = "identifier", path = "MedicationAdministration.identifier", description = "Return administrations with this external identifier", type = "token") 1831 public static final String SP_IDENTIFIER = "identifier"; 1832 @SearchParamDefinition(name = "code", path = "MedicationAdministration.medicationCodeableConcept", description = "Return administrations of this medication code", type = "token") 1833 public static final String SP_CODE = "code"; 1834 @SearchParamDefinition(name = "prescription", path = "MedicationAdministration.prescription", description = "The identity of a prescription to list administrations from", type = "reference") 1835 public static final String SP_PRESCRIPTION = "prescription"; 1836 @SearchParamDefinition(name = "effectivetime", path = "MedicationAdministration.effectiveTime[x]", description = "Date administration happened (or did not happen)", type = "date") 1837 public static final String SP_EFFECTIVETIME = "effectivetime"; 1838 @SearchParamDefinition(name = "practitioner", path = "MedicationAdministration.practitioner", description = "Who administered substance", type = "reference") 1839 public static final String SP_PRACTITIONER = "practitioner"; 1840 @SearchParamDefinition(name = "patient", path = "MedicationAdministration.patient", description = "The identity of a patient to list administrations for", type = "reference") 1841 public static final String SP_PATIENT = "patient"; 1842 @SearchParamDefinition(name = "medication", path = "MedicationAdministration.medicationReference", description = "Return administrations of this medication resource", type = "reference") 1843 public static final String SP_MEDICATION = "medication"; 1844 @SearchParamDefinition(name = "encounter", path = "MedicationAdministration.encounter", description = "Return administrations that share this encounter", type = "reference") 1845 public static final String SP_ENCOUNTER = "encounter"; 1846 @SearchParamDefinition(name = "device", path = "MedicationAdministration.device", description = "Return administrations with this administration device identity", type = "reference") 1847 public static final String SP_DEVICE = "device"; 1848 @SearchParamDefinition(name = "notgiven", path = "MedicationAdministration.wasNotGiven", description = "Administrations that were not made", type = "token") 1849 public static final String SP_NOTGIVEN = "notgiven"; 1850 @SearchParamDefinition(name = "status", path = "MedicationAdministration.status", description = "MedicationAdministration event status (for example one of active/paused/completed/nullified)", type = "token") 1851 public static final String SP_STATUS = "status"; 1852 1853}