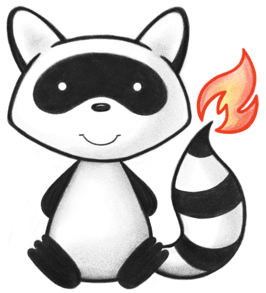
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.utilities.Utilities; 044 045/** 046 * Describes the event of a patient consuming or otherwise being administered a 047 * medication. This may be as simple as swallowing a tablet or it may be a long 048 * running infusion. Related resources tie this event to the authorizing 049 * prescription, and the specific encounter between patient and health care 050 * practitioner. 051 */ 052@ResourceDef(name = "MedicationAdministration", profile = "http://hl7.org/fhir/Profile/MedicationAdministration") 053public class MedicationAdministration extends DomainResource { 054 055 public enum MedicationAdministrationStatus { 056 /** 057 * The administration has started but has not yet completed. 058 */ 059 INPROGRESS, 060 /** 061 * Actions implied by the administration have been temporarily halted, but are 062 * expected to continue later. May also be called "suspended". 063 */ 064 ONHOLD, 065 /** 066 * All actions that are implied by the administration have occurred. 067 */ 068 COMPLETED, 069 /** 070 * The administration was entered in error and therefore nullified. 071 */ 072 ENTEREDINERROR, 073 /** 074 * Actions implied by the administration have been permanently halted, before 075 * all of them occurred. 076 */ 077 STOPPED, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 083 public static MedicationAdministrationStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("in-progress".equals(codeString)) 087 return INPROGRESS; 088 if ("on-hold".equals(codeString)) 089 return ONHOLD; 090 if ("completed".equals(codeString)) 091 return COMPLETED; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if ("stopped".equals(codeString)) 095 return STOPPED; 096 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case INPROGRESS: 102 return "in-progress"; 103 case ONHOLD: 104 return "on-hold"; 105 case COMPLETED: 106 return "completed"; 107 case ENTEREDINERROR: 108 return "entered-in-error"; 109 case STOPPED: 110 return "stopped"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 switch (this) { 120 case INPROGRESS: 121 return "http://hl7.org/fhir/medication-admin-status"; 122 case ONHOLD: 123 return "http://hl7.org/fhir/medication-admin-status"; 124 case COMPLETED: 125 return "http://hl7.org/fhir/medication-admin-status"; 126 case ENTEREDINERROR: 127 return "http://hl7.org/fhir/medication-admin-status"; 128 case STOPPED: 129 return "http://hl7.org/fhir/medication-admin-status"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case INPROGRESS: 140 return "The administration has started but has not yet completed."; 141 case ONHOLD: 142 return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 143 case COMPLETED: 144 return "All actions that are implied by the administration have occurred."; 145 case ENTEREDINERROR: 146 return "The administration was entered in error and therefore nullified."; 147 case STOPPED: 148 return "Actions implied by the administration have been permanently halted, before all of them occurred."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case INPROGRESS: 159 return "In Progress"; 160 case ONHOLD: 161 return "On Hold"; 162 case COMPLETED: 163 return "Completed"; 164 case ENTEREDINERROR: 165 return "Entered in Error"; 166 case STOPPED: 167 return "Stopped"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class MedicationAdministrationStatusEnumFactory implements EnumFactory<MedicationAdministrationStatus> { 177 public MedicationAdministrationStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("in-progress".equals(codeString)) 182 return MedicationAdministrationStatus.INPROGRESS; 183 if ("on-hold".equals(codeString)) 184 return MedicationAdministrationStatus.ONHOLD; 185 if ("completed".equals(codeString)) 186 return MedicationAdministrationStatus.COMPLETED; 187 if ("entered-in-error".equals(codeString)) 188 return MedicationAdministrationStatus.ENTEREDINERROR; 189 if ("stopped".equals(codeString)) 190 return MedicationAdministrationStatus.STOPPED; 191 throw new IllegalArgumentException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 192 } 193 194 public Enumeration<MedicationAdministrationStatus> fromType(Base code) throws FHIRException { 195 if (code == null || code.isEmpty()) 196 return null; 197 String codeString = ((PrimitiveType) code).asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("in-progress".equals(codeString)) 201 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.INPROGRESS); 202 if ("on-hold".equals(codeString)) 203 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ONHOLD); 204 if ("completed".equals(codeString)) 205 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.COMPLETED); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ENTEREDINERROR); 208 if ("stopped".equals(codeString)) 209 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.STOPPED); 210 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 211 } 212 213 public String toCode(MedicationAdministrationStatus code) { 214 if (code == MedicationAdministrationStatus.INPROGRESS) 215 return "in-progress"; 216 if (code == MedicationAdministrationStatus.ONHOLD) 217 return "on-hold"; 218 if (code == MedicationAdministrationStatus.COMPLETED) 219 return "completed"; 220 if (code == MedicationAdministrationStatus.ENTEREDINERROR) 221 return "entered-in-error"; 222 if (code == MedicationAdministrationStatus.STOPPED) 223 return "stopped"; 224 return "?"; 225 } 226 } 227 228 @Block() 229 public static class MedicationAdministrationDosageComponent extends BackboneElement implements IBaseBackboneElement { 230 /** 231 * Free text dosage instructions can be used for cases where the instructions 232 * are too complex to code. When coded instructions are present, the free text 233 * instructions may still be present for display to humans taking or 234 * administering the medication. 235 */ 236 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 237 @Description(shortDefinition = "Dosage Instructions", formalDefinition = "Free text dosage instructions can be used for cases where the instructions are too complex to code. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication.") 238 protected StringType text; 239 240 /** 241 * A coded specification of the anatomic site where the medication first entered 242 * the body. For example, "left arm". 243 */ 244 @Child(name = "site", type = { CodeableConcept.class, 245 BodySite.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 246 @Description(shortDefinition = "Body site administered to", formalDefinition = "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".") 247 protected Type site; 248 249 /** 250 * A code specifying the route or physiological path of administration of a 251 * therapeutic agent into or onto the patient. For example, topical, 252 * intravenous, etc. 253 */ 254 @Child(name = "route", type = { 255 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 256 @Description(shortDefinition = "Path of substance into body", formalDefinition = "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.") 257 protected CodeableConcept route; 258 259 /** 260 * A coded value indicating the method by which the medication is intended to be 261 * or was introduced into or on the body. This attribute will most often NOT be 262 * populated. It is most commonly used for injections. For example, Slow Push, 263 * Deep IV. 264 */ 265 @Child(name = "method", type = { 266 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 267 @Description(shortDefinition = "How drug was administered", formalDefinition = "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.") 268 protected CodeableConcept method; 269 270 /** 271 * The amount of the medication given at one administration event. Use this 272 * value when the administration is essentially an instantaneous event such as a 273 * swallowing a tablet or giving an injection. 274 */ 275 @Child(name = "quantity", type = { 276 SimpleQuantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 277 @Description(shortDefinition = "Amount administered in one dose", formalDefinition = "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.") 278 protected SimpleQuantity quantity; 279 280 /** 281 * Identifies the speed with which the medication was or will be introduced into 282 * the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 283 * ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 284 * hours. Currently we do not specify a default of '1' in the denominator, but 285 * this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 286 * liter/8 hours. 287 */ 288 @Child(name = "rate", type = { Ratio.class, 289 Range.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 290 @Description(shortDefinition = "Dose quantity per unit of time", formalDefinition = "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.") 291 protected Type rate; 292 293 private static final long serialVersionUID = -1772198879L; 294 295 /* 296 * Constructor 297 */ 298 public MedicationAdministrationDosageComponent() { 299 super(); 300 } 301 302 /** 303 * @return {@link #text} (Free text dosage instructions can be used for cases 304 * where the instructions are too complex to code. When coded 305 * instructions are present, the free text instructions may still be 306 * present for display to humans taking or administering the 307 * medication.). This is the underlying object with id, value and 308 * extensions. The accessor "getText" gives direct access to the value 309 */ 310 public StringType getTextElement() { 311 if (this.text == null) 312 if (Configuration.errorOnAutoCreate()) 313 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.text"); 314 else if (Configuration.doAutoCreate()) 315 this.text = new StringType(); // bb 316 return this.text; 317 } 318 319 public boolean hasTextElement() { 320 return this.text != null && !this.text.isEmpty(); 321 } 322 323 public boolean hasText() { 324 return this.text != null && !this.text.isEmpty(); 325 } 326 327 /** 328 * @param value {@link #text} (Free text dosage instructions can be used for 329 * cases where the instructions are too complex to code. When coded 330 * instructions are present, the free text instructions may still 331 * be present for display to humans taking or administering the 332 * medication.). This is the underlying object with id, value and 333 * extensions. The accessor "getText" gives direct access to the 334 * value 335 */ 336 public MedicationAdministrationDosageComponent setTextElement(StringType value) { 337 this.text = value; 338 return this; 339 } 340 341 /** 342 * @return Free text dosage instructions can be used for cases where the 343 * instructions are too complex to code. When coded instructions are 344 * present, the free text instructions may still be present for display 345 * to humans taking or administering the medication. 346 */ 347 public String getText() { 348 return this.text == null ? null : this.text.getValue(); 349 } 350 351 /** 352 * @param value Free text dosage instructions can be used for cases where the 353 * instructions are too complex to code. When coded instructions 354 * are present, the free text instructions may still be present for 355 * display to humans taking or administering the medication. 356 */ 357 public MedicationAdministrationDosageComponent setText(String value) { 358 if (Utilities.noString(value)) 359 this.text = null; 360 else { 361 if (this.text == null) 362 this.text = new StringType(); 363 this.text.setValue(value); 364 } 365 return this; 366 } 367 368 /** 369 * @return {@link #site} (A coded specification of the anatomic site where the 370 * medication first entered the body. For example, "left arm".) 371 */ 372 public Type getSite() { 373 return this.site; 374 } 375 376 /** 377 * @return {@link #site} (A coded specification of the anatomic site where the 378 * medication first entered the body. For example, "left arm".) 379 */ 380 public CodeableConcept getSiteCodeableConcept() throws FHIRException { 381 if (!(this.site instanceof CodeableConcept)) 382 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 383 + this.site.getClass().getName() + " was encountered"); 384 return (CodeableConcept) this.site; 385 } 386 387 public boolean hasSiteCodeableConcept() { 388 return this.site instanceof CodeableConcept; 389 } 390 391 /** 392 * @return {@link #site} (A coded specification of the anatomic site where the 393 * medication first entered the body. For example, "left arm".) 394 */ 395 public Reference getSiteReference() throws FHIRException { 396 if (!(this.site instanceof Reference)) 397 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.site.getClass().getName() 398 + " was encountered"); 399 return (Reference) this.site; 400 } 401 402 public boolean hasSiteReference() { 403 return this.site instanceof Reference; 404 } 405 406 public boolean hasSite() { 407 return this.site != null && !this.site.isEmpty(); 408 } 409 410 /** 411 * @param value {@link #site} (A coded specification of the anatomic site where 412 * the medication first entered the body. For example, "left arm".) 413 */ 414 public MedicationAdministrationDosageComponent setSite(Type value) { 415 this.site = value; 416 return this; 417 } 418 419 /** 420 * @return {@link #route} (A code specifying the route or physiological path of 421 * administration of a therapeutic agent into or onto the patient. For 422 * example, topical, intravenous, etc.) 423 */ 424 public CodeableConcept getRoute() { 425 if (this.route == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.route"); 428 else if (Configuration.doAutoCreate()) 429 this.route = new CodeableConcept(); // cc 430 return this.route; 431 } 432 433 public boolean hasRoute() { 434 return this.route != null && !this.route.isEmpty(); 435 } 436 437 /** 438 * @param value {@link #route} (A code specifying the route or physiological 439 * path of administration of a therapeutic agent into or onto the 440 * patient. For example, topical, intravenous, etc.) 441 */ 442 public MedicationAdministrationDosageComponent setRoute(CodeableConcept value) { 443 this.route = value; 444 return this; 445 } 446 447 /** 448 * @return {@link #method} (A coded value indicating the method by which the 449 * medication is intended to be or was introduced into or on the body. 450 * This attribute will most often NOT be populated. It is most commonly 451 * used for injections. For example, Slow Push, Deep IV.) 452 */ 453 public CodeableConcept getMethod() { 454 if (this.method == null) 455 if (Configuration.errorOnAutoCreate()) 456 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.method"); 457 else if (Configuration.doAutoCreate()) 458 this.method = new CodeableConcept(); // cc 459 return this.method; 460 } 461 462 public boolean hasMethod() { 463 return this.method != null && !this.method.isEmpty(); 464 } 465 466 /** 467 * @param value {@link #method} (A coded value indicating the method by which 468 * the medication is intended to be or was introduced into or on 469 * the body. This attribute will most often NOT be populated. It is 470 * most commonly used for injections. For example, Slow Push, Deep 471 * IV.) 472 */ 473 public MedicationAdministrationDosageComponent setMethod(CodeableConcept value) { 474 this.method = value; 475 return this; 476 } 477 478 /** 479 * @return {@link #quantity} (The amount of the medication given at one 480 * administration event. Use this value when the administration is 481 * essentially an instantaneous event such as a swallowing a tablet or 482 * giving an injection.) 483 */ 484 public SimpleQuantity getQuantity() { 485 if (this.quantity == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.quantity"); 488 else if (Configuration.doAutoCreate()) 489 this.quantity = new SimpleQuantity(); // cc 490 return this.quantity; 491 } 492 493 public boolean hasQuantity() { 494 return this.quantity != null && !this.quantity.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #quantity} (The amount of the medication given at one 499 * administration event. Use this value when the administration is 500 * essentially an instantaneous event such as a swallowing a tablet 501 * or giving an injection.) 502 */ 503 public MedicationAdministrationDosageComponent setQuantity(SimpleQuantity value) { 504 this.quantity = value; 505 return this; 506 } 507 508 /** 509 * @return {@link #rate} (Identifies the speed with which the medication was or 510 * will be introduced into the patient. Typically the rate for an 511 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 512 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 513 * not specify a default of '1' in the denominator, but this is being 514 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 515 * hours.) 516 */ 517 public Type getRate() { 518 return this.rate; 519 } 520 521 /** 522 * @return {@link #rate} (Identifies the speed with which the medication was or 523 * will be introduced into the patient. Typically the rate for an 524 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 525 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 526 * not specify a default of '1' in the denominator, but this is being 527 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 528 * hours.) 529 */ 530 public Ratio getRateRatio() throws FHIRException { 531 if (!(this.rate instanceof Ratio)) 532 throw new FHIRException( 533 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 534 return (Ratio) this.rate; 535 } 536 537 public boolean hasRateRatio() { 538 return this.rate instanceof Ratio; 539 } 540 541 /** 542 * @return {@link #rate} (Identifies the speed with which the medication was or 543 * will be introduced into the patient. Typically the rate for an 544 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 545 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 546 * not specify a default of '1' in the denominator, but this is being 547 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 548 * hours.) 549 */ 550 public Range getRateRange() throws FHIRException { 551 if (!(this.rate instanceof Range)) 552 throw new FHIRException( 553 "Type mismatch: the type Range was expected, but " + this.rate.getClass().getName() + " was encountered"); 554 return (Range) this.rate; 555 } 556 557 public boolean hasRateRange() { 558 return this.rate instanceof Range; 559 } 560 561 public boolean hasRate() { 562 return this.rate != null && !this.rate.isEmpty(); 563 } 564 565 /** 566 * @param value {@link #rate} (Identifies the speed with which the medication 567 * was or will be introduced into the patient. Typically the rate 568 * for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be 569 * expressed as a rate per unit of time e.g. 500 ml per 2 hours. 570 * Currently we do not specify a default of '1' in the denominator, 571 * but this is being discussed. Other examples: 200 mcg/min or 200 572 * mcg/1 minute; 1 liter/8 hours.) 573 */ 574 public MedicationAdministrationDosageComponent setRate(Type value) { 575 this.rate = value; 576 return this; 577 } 578 579 protected void listChildren(List<Property> childrenList) { 580 super.listChildren(childrenList); 581 childrenList.add(new Property("text", "string", 582 "Free text dosage instructions can be used for cases where the instructions are too complex to code. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication.", 583 0, java.lang.Integer.MAX_VALUE, text)); 584 childrenList.add(new Property("site[x]", "CodeableConcept|Reference(BodySite)", 585 "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 586 0, java.lang.Integer.MAX_VALUE, site)); 587 childrenList.add(new Property("route", "CodeableConcept", 588 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 589 0, java.lang.Integer.MAX_VALUE, route)); 590 childrenList.add(new Property("method", "CodeableConcept", 591 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 592 0, java.lang.Integer.MAX_VALUE, method)); 593 childrenList.add(new Property("quantity", "SimpleQuantity", 594 "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 595 0, java.lang.Integer.MAX_VALUE, quantity)); 596 childrenList.add(new Property("rate[x]", "Ratio|Range", 597 "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 598 0, java.lang.Integer.MAX_VALUE, rate)); 599 } 600 601 @Override 602 public void setProperty(String name, Base value) throws FHIRException { 603 if (name.equals("text")) 604 this.text = castToString(value); // StringType 605 else if (name.equals("site[x]")) 606 this.site = (Type) value; // Type 607 else if (name.equals("route")) 608 this.route = castToCodeableConcept(value); // CodeableConcept 609 else if (name.equals("method")) 610 this.method = castToCodeableConcept(value); // CodeableConcept 611 else if (name.equals("quantity")) 612 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 613 else if (name.equals("rate[x]")) 614 this.rate = (Type) value; // Type 615 else 616 super.setProperty(name, value); 617 } 618 619 @Override 620 public Base addChild(String name) throws FHIRException { 621 if (name.equals("text")) { 622 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.text"); 623 } else if (name.equals("siteCodeableConcept")) { 624 this.site = new CodeableConcept(); 625 return this.site; 626 } else if (name.equals("siteReference")) { 627 this.site = new Reference(); 628 return this.site; 629 } else if (name.equals("route")) { 630 this.route = new CodeableConcept(); 631 return this.route; 632 } else if (name.equals("method")) { 633 this.method = new CodeableConcept(); 634 return this.method; 635 } else if (name.equals("quantity")) { 636 this.quantity = new SimpleQuantity(); 637 return this.quantity; 638 } else if (name.equals("rateRatio")) { 639 this.rate = new Ratio(); 640 return this.rate; 641 } else if (name.equals("rateRange")) { 642 this.rate = new Range(); 643 return this.rate; 644 } else 645 return super.addChild(name); 646 } 647 648 public MedicationAdministrationDosageComponent copy() { 649 MedicationAdministrationDosageComponent dst = new MedicationAdministrationDosageComponent(); 650 copyValues(dst); 651 dst.text = text == null ? null : text.copy(); 652 dst.site = site == null ? null : site.copy(); 653 dst.route = route == null ? null : route.copy(); 654 dst.method = method == null ? null : method.copy(); 655 dst.quantity = quantity == null ? null : quantity.copy(); 656 dst.rate = rate == null ? null : rate.copy(); 657 return dst; 658 } 659 660 @Override 661 public boolean equalsDeep(Base other) { 662 if (!super.equalsDeep(other)) 663 return false; 664 if (!(other instanceof MedicationAdministrationDosageComponent)) 665 return false; 666 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other; 667 return compareDeep(text, o.text, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 668 && compareDeep(method, o.method, true) && compareDeep(quantity, o.quantity, true) 669 && compareDeep(rate, o.rate, true); 670 } 671 672 @Override 673 public boolean equalsShallow(Base other) { 674 if (!super.equalsShallow(other)) 675 return false; 676 if (!(other instanceof MedicationAdministrationDosageComponent)) 677 return false; 678 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other; 679 return compareValues(text, o.text, true); 680 } 681 682 public boolean isEmpty() { 683 return super.isEmpty() && (text == null || text.isEmpty()) && (site == null || site.isEmpty()) 684 && (route == null || route.isEmpty()) && (method == null || method.isEmpty()) 685 && (quantity == null || quantity.isEmpty()) && (rate == null || rate.isEmpty()); 686 } 687 688 public String fhirType() { 689 return "MedicationAdministration.dosage"; 690 691 } 692 693 } 694 695 /** 696 * External identifier - FHIR will generate its own internal identifiers 697 * (probably URLs) which do not need to be explicitly managed by the resource. 698 * The identifier here is one that would be used by another non-FHIR system - 699 * for example an automated medication pump would provide a record each time it 700 * operated; an administration while the patient was off the ward might be made 701 * with a different system and entered after the event. Particularly important 702 * if these records have to be updated. 703 */ 704 @Child(name = "identifier", type = { 705 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 706 @Description(shortDefinition = "External identifier", formalDefinition = "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.") 707 protected List<Identifier> identifier; 708 709 /** 710 * Will generally be set to show that the administration has been completed. For 711 * some long running administrations such as infusions it is possible for an 712 * administration to be started but not completed or it may be paused while some 713 * other process is under way. 714 */ 715 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 716 @Description(shortDefinition = "in-progress | on-hold | completed | entered-in-error | stopped", formalDefinition = "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.") 717 protected Enumeration<MedicationAdministrationStatus> status; 718 719 /** 720 * The person or animal receiving the medication. 721 */ 722 @Child(name = "patient", type = { Patient.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 723 @Description(shortDefinition = "Who received medication", formalDefinition = "The person or animal receiving the medication.") 724 protected Reference patient; 725 726 /** 727 * The actual object that is the target of the reference (The person or animal 728 * receiving the medication.) 729 */ 730 protected Patient patientTarget; 731 732 /** 733 * The individual who was responsible for giving the medication to the patient. 734 */ 735 @Child(name = "practitioner", type = { Practitioner.class, Patient.class, 736 RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 737 @Description(shortDefinition = "Who administered substance", formalDefinition = "The individual who was responsible for giving the medication to the patient.") 738 protected Reference practitioner; 739 740 /** 741 * The actual object that is the target of the reference (The individual who was 742 * responsible for giving the medication to the patient.) 743 */ 744 protected Resource practitionerTarget; 745 746 /** 747 * The visit, admission or other contact between patient and health care 748 * provider the medication administration was performed as part of. 749 */ 750 @Child(name = "encounter", type = { Encounter.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 751 @Description(shortDefinition = "Encounter administered as part of", formalDefinition = "The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.") 752 protected Reference encounter; 753 754 /** 755 * The actual object that is the target of the reference (The visit, admission 756 * or other contact between patient and health care provider the medication 757 * administration was performed as part of.) 758 */ 759 protected Encounter encounterTarget; 760 761 /** 762 * The original request, instruction or authority to perform the administration. 763 */ 764 @Child(name = "prescription", type = { 765 MedicationOrder.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 766 @Description(shortDefinition = "Order administration performed against", formalDefinition = "The original request, instruction or authority to perform the administration.") 767 protected Reference prescription; 768 769 /** 770 * The actual object that is the target of the reference (The original request, 771 * instruction or authority to perform the administration.) 772 */ 773 protected MedicationOrder prescriptionTarget; 774 775 /** 776 * Set this to true if the record is saying that the medication was NOT 777 * administered. 778 */ 779 @Child(name = "wasNotGiven", type = { 780 BooleanType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 781 @Description(shortDefinition = "True if medication not administered", formalDefinition = "Set this to true if the record is saying that the medication was NOT administered.") 782 protected BooleanType wasNotGiven; 783 784 /** 785 * A code indicating why the administration was not performed. 786 */ 787 @Child(name = "reasonNotGiven", type = { 788 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 789 @Description(shortDefinition = "Reason administration not performed", formalDefinition = "A code indicating why the administration was not performed.") 790 protected List<CodeableConcept> reasonNotGiven; 791 792 /** 793 * A code indicating why the medication was given. 794 */ 795 @Child(name = "reasonGiven", type = { 796 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 797 @Description(shortDefinition = "Reason administration performed", formalDefinition = "A code indicating why the medication was given.") 798 protected List<CodeableConcept> reasonGiven; 799 800 /** 801 * A specific date/time or interval of time during which the administration took 802 * place (or did not take place, when the 'notGiven' attribute is true). For 803 * many administrations, such as swallowing a tablet the use of dateTime is more 804 * appropriate. 805 */ 806 @Child(name = "effectiveTime", type = { DateTimeType.class, 807 Period.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 808 @Description(shortDefinition = "Start and end time of administration", formalDefinition = "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.") 809 protected Type effectiveTime; 810 811 /** 812 * Identifies the medication that was administered. This is either a link to a 813 * resource representing the details of the medication or a simple attribute 814 * carrying a code that identifies the medication from a known list of 815 * medications. 816 */ 817 @Child(name = "medication", type = { CodeableConcept.class, 818 Medication.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 819 @Description(shortDefinition = "What was administered", formalDefinition = "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 820 protected Type medication; 821 822 /** 823 * The device used in administering the medication to the patient. For example, 824 * a particular infusion pump. 825 */ 826 @Child(name = "device", type = { 827 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 828 @Description(shortDefinition = "Device used to administer", formalDefinition = "The device used in administering the medication to the patient. For example, a particular infusion pump.") 829 protected List<Reference> device; 830 /** 831 * The actual objects that are the target of the reference (The device used in 832 * administering the medication to the patient. For example, a particular 833 * infusion pump.) 834 */ 835 protected List<Device> deviceTarget; 836 837 /** 838 * Extra information about the medication administration that is not conveyed by 839 * the other attributes. 840 */ 841 @Child(name = "note", type = { StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 842 @Description(shortDefinition = "Information about the administration", formalDefinition = "Extra information about the medication administration that is not conveyed by the other attributes.") 843 protected StringType note; 844 845 /** 846 * Describes the medication dosage information details e.g. dose, rate, site, 847 * route, etc. 848 */ 849 @Child(name = "dosage", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = true) 850 @Description(shortDefinition = "Details of how medication was taken", formalDefinition = "Describes the medication dosage information details e.g. dose, rate, site, route, etc.") 851 protected MedicationAdministrationDosageComponent dosage; 852 853 private static final long serialVersionUID = -669616345L; 854 855 /* 856 * Constructor 857 */ 858 public MedicationAdministration() { 859 super(); 860 } 861 862 /* 863 * Constructor 864 */ 865 public MedicationAdministration(Enumeration<MedicationAdministrationStatus> status, Reference patient, 866 Type effectiveTime, Type medication) { 867 super(); 868 this.status = status; 869 this.patient = patient; 870 this.effectiveTime = effectiveTime; 871 this.medication = medication; 872 } 873 874 /** 875 * @return {@link #identifier} (External identifier - FHIR will generate its own 876 * internal identifiers (probably URLs) which do not need to be 877 * explicitly managed by the resource. The identifier here is one that 878 * would be used by another non-FHIR system - for example an automated 879 * medication pump would provide a record each time it operated; an 880 * administration while the patient was off the ward might be made with 881 * a different system and entered after the event. Particularly 882 * important if these records have to be updated.) 883 */ 884 public List<Identifier> getIdentifier() { 885 if (this.identifier == null) 886 this.identifier = new ArrayList<Identifier>(); 887 return this.identifier; 888 } 889 890 public boolean hasIdentifier() { 891 if (this.identifier == null) 892 return false; 893 for (Identifier item : this.identifier) 894 if (!item.isEmpty()) 895 return true; 896 return false; 897 } 898 899 /** 900 * @return {@link #identifier} (External identifier - FHIR will generate its own 901 * internal identifiers (probably URLs) which do not need to be 902 * explicitly managed by the resource. The identifier here is one that 903 * would be used by another non-FHIR system - for example an automated 904 * medication pump would provide a record each time it operated; an 905 * administration while the patient was off the ward might be made with 906 * a different system and entered after the event. Particularly 907 * important if these records have to be updated.) 908 */ 909 // syntactic sugar 910 public Identifier addIdentifier() { // 3 911 Identifier t = new Identifier(); 912 if (this.identifier == null) 913 this.identifier = new ArrayList<Identifier>(); 914 this.identifier.add(t); 915 return t; 916 } 917 918 // syntactic sugar 919 public MedicationAdministration addIdentifier(Identifier t) { // 3 920 if (t == null) 921 return this; 922 if (this.identifier == null) 923 this.identifier = new ArrayList<Identifier>(); 924 this.identifier.add(t); 925 return this; 926 } 927 928 /** 929 * @return {@link #status} (Will generally be set to show that the 930 * administration has been completed. For some long running 931 * administrations such as infusions it is possible for an 932 * administration to be started but not completed or it may be paused 933 * while some other process is under way.). This is the underlying 934 * object with id, value and extensions. The accessor "getStatus" gives 935 * direct access to the value 936 */ 937 public Enumeration<MedicationAdministrationStatus> getStatusElement() { 938 if (this.status == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create MedicationAdministration.status"); 941 else if (Configuration.doAutoCreate()) 942 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); // bb 943 return this.status; 944 } 945 946 public boolean hasStatusElement() { 947 return this.status != null && !this.status.isEmpty(); 948 } 949 950 public boolean hasStatus() { 951 return this.status != null && !this.status.isEmpty(); 952 } 953 954 /** 955 * @param value {@link #status} (Will generally be set to show that the 956 * administration has been completed. For some long running 957 * administrations such as infusions it is possible for an 958 * administration to be started but not completed or it may be 959 * paused while some other process is under way.). This is the 960 * underlying object with id, value and extensions. The accessor 961 * "getStatus" gives direct access to the value 962 */ 963 public MedicationAdministration setStatusElement(Enumeration<MedicationAdministrationStatus> value) { 964 this.status = value; 965 return this; 966 } 967 968 /** 969 * @return Will generally be set to show that the administration has been 970 * completed. For some long running administrations such as infusions it 971 * is possible for an administration to be started but not completed or 972 * it may be paused while some other process is under way. 973 */ 974 public MedicationAdministrationStatus getStatus() { 975 return this.status == null ? null : this.status.getValue(); 976 } 977 978 /** 979 * @param value Will generally be set to show that the administration has been 980 * completed. For some long running administrations such as 981 * infusions it is possible for an administration to be started but 982 * not completed or it may be paused while some other process is 983 * under way. 984 */ 985 public MedicationAdministration setStatus(MedicationAdministrationStatus value) { 986 if (this.status == null) 987 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); 988 this.status.setValue(value); 989 return this; 990 } 991 992 /** 993 * @return {@link #patient} (The person or animal receiving the medication.) 994 */ 995 public Reference getPatient() { 996 if (this.patient == null) 997 if (Configuration.errorOnAutoCreate()) 998 throw new Error("Attempt to auto-create MedicationAdministration.patient"); 999 else if (Configuration.doAutoCreate()) 1000 this.patient = new Reference(); // cc 1001 return this.patient; 1002 } 1003 1004 public boolean hasPatient() { 1005 return this.patient != null && !this.patient.isEmpty(); 1006 } 1007 1008 /** 1009 * @param value {@link #patient} (The person or animal receiving the 1010 * medication.) 1011 */ 1012 public MedicationAdministration setPatient(Reference value) { 1013 this.patient = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #patient} The actual object that is the target of the 1019 * reference. The reference library doesn't populate this, but you can 1020 * use it to hold the resource if you resolve it. (The person or animal 1021 * receiving the medication.) 1022 */ 1023 public Patient getPatientTarget() { 1024 if (this.patientTarget == null) 1025 if (Configuration.errorOnAutoCreate()) 1026 throw new Error("Attempt to auto-create MedicationAdministration.patient"); 1027 else if (Configuration.doAutoCreate()) 1028 this.patientTarget = new Patient(); // aa 1029 return this.patientTarget; 1030 } 1031 1032 /** 1033 * @param value {@link #patient} The actual object that is the target of the 1034 * reference. The reference library doesn't use these, but you can 1035 * use it to hold the resource if you resolve it. (The person or 1036 * animal receiving the medication.) 1037 */ 1038 public MedicationAdministration setPatientTarget(Patient value) { 1039 this.patientTarget = value; 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #practitioner} (The individual who was responsible for giving 1045 * the medication to the patient.) 1046 */ 1047 public Reference getPractitioner() { 1048 if (this.practitioner == null) 1049 if (Configuration.errorOnAutoCreate()) 1050 throw new Error("Attempt to auto-create MedicationAdministration.practitioner"); 1051 else if (Configuration.doAutoCreate()) 1052 this.practitioner = new Reference(); // cc 1053 return this.practitioner; 1054 } 1055 1056 public boolean hasPractitioner() { 1057 return this.practitioner != null && !this.practitioner.isEmpty(); 1058 } 1059 1060 /** 1061 * @param value {@link #practitioner} (The individual who was responsible for 1062 * giving the medication to the patient.) 1063 */ 1064 public MedicationAdministration setPractitioner(Reference value) { 1065 this.practitioner = value; 1066 return this; 1067 } 1068 1069 /** 1070 * @return {@link #practitioner} The actual object that is the target of the 1071 * reference. The reference library doesn't populate this, but you can 1072 * use it to hold the resource if you resolve it. (The individual who 1073 * was responsible for giving the medication to the patient.) 1074 */ 1075 public Resource getPractitionerTarget() { 1076 return this.practitionerTarget; 1077 } 1078 1079 /** 1080 * @param value {@link #practitioner} The actual object that is the target of 1081 * the reference. The reference library doesn't use these, but you 1082 * can use it to hold the resource if you resolve it. (The 1083 * individual who was responsible for giving the medication to the 1084 * patient.) 1085 */ 1086 public MedicationAdministration setPractitionerTarget(Resource value) { 1087 this.practitionerTarget = value; 1088 return this; 1089 } 1090 1091 /** 1092 * @return {@link #encounter} (The visit, admission or other contact between 1093 * patient and health care provider the medication administration was 1094 * performed as part of.) 1095 */ 1096 public Reference getEncounter() { 1097 if (this.encounter == null) 1098 if (Configuration.errorOnAutoCreate()) 1099 throw new Error("Attempt to auto-create MedicationAdministration.encounter"); 1100 else if (Configuration.doAutoCreate()) 1101 this.encounter = new Reference(); // cc 1102 return this.encounter; 1103 } 1104 1105 public boolean hasEncounter() { 1106 return this.encounter != null && !this.encounter.isEmpty(); 1107 } 1108 1109 /** 1110 * @param value {@link #encounter} (The visit, admission or other contact 1111 * between patient and health care provider the medication 1112 * administration was performed as part of.) 1113 */ 1114 public MedicationAdministration setEncounter(Reference value) { 1115 this.encounter = value; 1116 return this; 1117 } 1118 1119 /** 1120 * @return {@link #encounter} The actual object that is the target of the 1121 * reference. The reference library doesn't populate this, but you can 1122 * use it to hold the resource if you resolve it. (The visit, admission 1123 * or other contact between patient and health care provider the 1124 * medication administration was performed as part of.) 1125 */ 1126 public Encounter getEncounterTarget() { 1127 if (this.encounterTarget == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create MedicationAdministration.encounter"); 1130 else if (Configuration.doAutoCreate()) 1131 this.encounterTarget = new Encounter(); // aa 1132 return this.encounterTarget; 1133 } 1134 1135 /** 1136 * @param value {@link #encounter} The actual object that is the target of the 1137 * reference. The reference library doesn't use these, but you can 1138 * use it to hold the resource if you resolve it. (The visit, 1139 * admission or other contact between patient and health care 1140 * provider the medication administration was performed as part 1141 * of.) 1142 */ 1143 public MedicationAdministration setEncounterTarget(Encounter value) { 1144 this.encounterTarget = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #prescription} (The original request, instruction or authority 1150 * to perform the administration.) 1151 */ 1152 public Reference getPrescription() { 1153 if (this.prescription == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create MedicationAdministration.prescription"); 1156 else if (Configuration.doAutoCreate()) 1157 this.prescription = new Reference(); // cc 1158 return this.prescription; 1159 } 1160 1161 public boolean hasPrescription() { 1162 return this.prescription != null && !this.prescription.isEmpty(); 1163 } 1164 1165 /** 1166 * @param value {@link #prescription} (The original request, instruction or 1167 * authority to perform the administration.) 1168 */ 1169 public MedicationAdministration setPrescription(Reference value) { 1170 this.prescription = value; 1171 return this; 1172 } 1173 1174 /** 1175 * @return {@link #prescription} The actual object that is the target of the 1176 * reference. The reference library doesn't populate this, but you can 1177 * use it to hold the resource if you resolve it. (The original request, 1178 * instruction or authority to perform the administration.) 1179 */ 1180 public MedicationOrder getPrescriptionTarget() { 1181 if (this.prescriptionTarget == null) 1182 if (Configuration.errorOnAutoCreate()) 1183 throw new Error("Attempt to auto-create MedicationAdministration.prescription"); 1184 else if (Configuration.doAutoCreate()) 1185 this.prescriptionTarget = new MedicationOrder(); // aa 1186 return this.prescriptionTarget; 1187 } 1188 1189 /** 1190 * @param value {@link #prescription} The actual object that is the target of 1191 * the reference. The reference library doesn't use these, but you 1192 * can use it to hold the resource if you resolve it. (The original 1193 * request, instruction or authority to perform the 1194 * administration.) 1195 */ 1196 public MedicationAdministration setPrescriptionTarget(MedicationOrder value) { 1197 this.prescriptionTarget = value; 1198 return this; 1199 } 1200 1201 /** 1202 * @return {@link #wasNotGiven} (Set this to true if the record is saying that 1203 * the medication was NOT administered.). This is the underlying object 1204 * with id, value and extensions. The accessor "getWasNotGiven" gives 1205 * direct access to the value 1206 */ 1207 public BooleanType getWasNotGivenElement() { 1208 if (this.wasNotGiven == null) 1209 if (Configuration.errorOnAutoCreate()) 1210 throw new Error("Attempt to auto-create MedicationAdministration.wasNotGiven"); 1211 else if (Configuration.doAutoCreate()) 1212 this.wasNotGiven = new BooleanType(); // bb 1213 return this.wasNotGiven; 1214 } 1215 1216 public boolean hasWasNotGivenElement() { 1217 return this.wasNotGiven != null && !this.wasNotGiven.isEmpty(); 1218 } 1219 1220 public boolean hasWasNotGiven() { 1221 return this.wasNotGiven != null && !this.wasNotGiven.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #wasNotGiven} (Set this to true if the record is saying 1226 * that the medication was NOT administered.). This is the 1227 * underlying object with id, value and extensions. The accessor 1228 * "getWasNotGiven" gives direct access to the value 1229 */ 1230 public MedicationAdministration setWasNotGivenElement(BooleanType value) { 1231 this.wasNotGiven = value; 1232 return this; 1233 } 1234 1235 /** 1236 * @return Set this to true if the record is saying that the medication was NOT 1237 * administered. 1238 */ 1239 public boolean getWasNotGiven() { 1240 return this.wasNotGiven == null || this.wasNotGiven.isEmpty() ? false : this.wasNotGiven.getValue(); 1241 } 1242 1243 /** 1244 * @param value Set this to true if the record is saying that the medication was 1245 * NOT administered. 1246 */ 1247 public MedicationAdministration setWasNotGiven(boolean value) { 1248 if (this.wasNotGiven == null) 1249 this.wasNotGiven = new BooleanType(); 1250 this.wasNotGiven.setValue(value); 1251 return this; 1252 } 1253 1254 /** 1255 * @return {@link #reasonNotGiven} (A code indicating why the administration was 1256 * not performed.) 1257 */ 1258 public List<CodeableConcept> getReasonNotGiven() { 1259 if (this.reasonNotGiven == null) 1260 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1261 return this.reasonNotGiven; 1262 } 1263 1264 public boolean hasReasonNotGiven() { 1265 if (this.reasonNotGiven == null) 1266 return false; 1267 for (CodeableConcept item : this.reasonNotGiven) 1268 if (!item.isEmpty()) 1269 return true; 1270 return false; 1271 } 1272 1273 /** 1274 * @return {@link #reasonNotGiven} (A code indicating why the administration was 1275 * not performed.) 1276 */ 1277 // syntactic sugar 1278 public CodeableConcept addReasonNotGiven() { // 3 1279 CodeableConcept t = new CodeableConcept(); 1280 if (this.reasonNotGiven == null) 1281 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1282 this.reasonNotGiven.add(t); 1283 return t; 1284 } 1285 1286 // syntactic sugar 1287 public MedicationAdministration addReasonNotGiven(CodeableConcept t) { // 3 1288 if (t == null) 1289 return this; 1290 if (this.reasonNotGiven == null) 1291 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1292 this.reasonNotGiven.add(t); 1293 return this; 1294 } 1295 1296 /** 1297 * @return {@link #reasonGiven} (A code indicating why the medication was 1298 * given.) 1299 */ 1300 public List<CodeableConcept> getReasonGiven() { 1301 if (this.reasonGiven == null) 1302 this.reasonGiven = new ArrayList<CodeableConcept>(); 1303 return this.reasonGiven; 1304 } 1305 1306 public boolean hasReasonGiven() { 1307 if (this.reasonGiven == null) 1308 return false; 1309 for (CodeableConcept item : this.reasonGiven) 1310 if (!item.isEmpty()) 1311 return true; 1312 return false; 1313 } 1314 1315 /** 1316 * @return {@link #reasonGiven} (A code indicating why the medication was 1317 * given.) 1318 */ 1319 // syntactic sugar 1320 public CodeableConcept addReasonGiven() { // 3 1321 CodeableConcept t = new CodeableConcept(); 1322 if (this.reasonGiven == null) 1323 this.reasonGiven = new ArrayList<CodeableConcept>(); 1324 this.reasonGiven.add(t); 1325 return t; 1326 } 1327 1328 // syntactic sugar 1329 public MedicationAdministration addReasonGiven(CodeableConcept t) { // 3 1330 if (t == null) 1331 return this; 1332 if (this.reasonGiven == null) 1333 this.reasonGiven = new ArrayList<CodeableConcept>(); 1334 this.reasonGiven.add(t); 1335 return this; 1336 } 1337 1338 /** 1339 * @return {@link #effectiveTime} (A specific date/time or interval of time 1340 * during which the administration took place (or did not take place, 1341 * when the 'notGiven' attribute is true). For many administrations, 1342 * such as swallowing a tablet the use of dateTime is more appropriate.) 1343 */ 1344 public Type getEffectiveTime() { 1345 return this.effectiveTime; 1346 } 1347 1348 /** 1349 * @return {@link #effectiveTime} (A specific date/time or interval of time 1350 * during which the administration took place (or did not take place, 1351 * when the 'notGiven' attribute is true). For many administrations, 1352 * such as swallowing a tablet the use of dateTime is more appropriate.) 1353 */ 1354 public DateTimeType getEffectiveTimeDateTimeType() throws FHIRException { 1355 if (!(this.effectiveTime instanceof DateTimeType)) 1356 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1357 + this.effectiveTime.getClass().getName() + " was encountered"); 1358 return (DateTimeType) this.effectiveTime; 1359 } 1360 1361 public boolean hasEffectiveTimeDateTimeType() { 1362 return this.effectiveTime instanceof DateTimeType; 1363 } 1364 1365 /** 1366 * @return {@link #effectiveTime} (A specific date/time or interval of time 1367 * during which the administration took place (or did not take place, 1368 * when the 'notGiven' attribute is true). For many administrations, 1369 * such as swallowing a tablet the use of dateTime is more appropriate.) 1370 */ 1371 public Period getEffectiveTimePeriod() throws FHIRException { 1372 if (!(this.effectiveTime instanceof Period)) 1373 throw new FHIRException("Type mismatch: the type Period was expected, but " 1374 + this.effectiveTime.getClass().getName() + " was encountered"); 1375 return (Period) this.effectiveTime; 1376 } 1377 1378 public boolean hasEffectiveTimePeriod() { 1379 return this.effectiveTime instanceof Period; 1380 } 1381 1382 public boolean hasEffectiveTime() { 1383 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 1384 } 1385 1386 /** 1387 * @param value {@link #effectiveTime} (A specific date/time or interval of time 1388 * during which the administration took place (or did not take 1389 * place, when the 'notGiven' attribute is true). For many 1390 * administrations, such as swallowing a tablet the use of dateTime 1391 * is more appropriate.) 1392 */ 1393 public MedicationAdministration setEffectiveTime(Type value) { 1394 this.effectiveTime = value; 1395 return this; 1396 } 1397 1398 /** 1399 * @return {@link #medication} (Identifies the medication that was administered. 1400 * This is either a link to a resource representing the details of the 1401 * medication or a simple attribute carrying a code that identifies the 1402 * medication from a known list of medications.) 1403 */ 1404 public Type getMedication() { 1405 return this.medication; 1406 } 1407 1408 /** 1409 * @return {@link #medication} (Identifies the medication that was administered. 1410 * This is either a link to a resource representing the details of the 1411 * medication or a simple attribute carrying a code that identifies the 1412 * medication from a known list of medications.) 1413 */ 1414 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1415 if (!(this.medication instanceof CodeableConcept)) 1416 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1417 + this.medication.getClass().getName() + " was encountered"); 1418 return (CodeableConcept) this.medication; 1419 } 1420 1421 public boolean hasMedicationCodeableConcept() { 1422 return this.medication instanceof CodeableConcept; 1423 } 1424 1425 /** 1426 * @return {@link #medication} (Identifies the medication that was administered. 1427 * This is either a link to a resource representing the details of the 1428 * medication or a simple attribute carrying a code that identifies the 1429 * medication from a known list of medications.) 1430 */ 1431 public Reference getMedicationReference() throws FHIRException { 1432 if (!(this.medication instanceof Reference)) 1433 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1434 + this.medication.getClass().getName() + " was encountered"); 1435 return (Reference) this.medication; 1436 } 1437 1438 public boolean hasMedicationReference() { 1439 return this.medication instanceof Reference; 1440 } 1441 1442 public boolean hasMedication() { 1443 return this.medication != null && !this.medication.isEmpty(); 1444 } 1445 1446 /** 1447 * @param value {@link #medication} (Identifies the medication that was 1448 * administered. This is either a link to a resource representing 1449 * the details of the medication or a simple attribute carrying a 1450 * code that identifies the medication from a known list of 1451 * medications.) 1452 */ 1453 public MedicationAdministration setMedication(Type value) { 1454 this.medication = value; 1455 return this; 1456 } 1457 1458 /** 1459 * @return {@link #device} (The device used in administering the medication to 1460 * the patient. For example, a particular infusion pump.) 1461 */ 1462 public List<Reference> getDevice() { 1463 if (this.device == null) 1464 this.device = new ArrayList<Reference>(); 1465 return this.device; 1466 } 1467 1468 public boolean hasDevice() { 1469 if (this.device == null) 1470 return false; 1471 for (Reference item : this.device) 1472 if (!item.isEmpty()) 1473 return true; 1474 return false; 1475 } 1476 1477 /** 1478 * @return {@link #device} (The device used in administering the medication to 1479 * the patient. For example, a particular infusion pump.) 1480 */ 1481 // syntactic sugar 1482 public Reference addDevice() { // 3 1483 Reference t = new Reference(); 1484 if (this.device == null) 1485 this.device = new ArrayList<Reference>(); 1486 this.device.add(t); 1487 return t; 1488 } 1489 1490 // syntactic sugar 1491 public MedicationAdministration addDevice(Reference t) { // 3 1492 if (t == null) 1493 return this; 1494 if (this.device == null) 1495 this.device = new ArrayList<Reference>(); 1496 this.device.add(t); 1497 return this; 1498 } 1499 1500 /** 1501 * @return {@link #device} (The actual objects that are the target of the 1502 * reference. The reference library doesn't populate this, but you can 1503 * use this to hold the resources if you resolvethemt. The device used 1504 * in administering the medication to the patient. For example, a 1505 * particular infusion pump.) 1506 */ 1507 public List<Device> getDeviceTarget() { 1508 if (this.deviceTarget == null) 1509 this.deviceTarget = new ArrayList<Device>(); 1510 return this.deviceTarget; 1511 } 1512 1513 // syntactic sugar 1514 /** 1515 * @return {@link #device} (Add an actual object that is the target of the 1516 * reference. The reference library doesn't use these, but you can use 1517 * this to hold the resources if you resolvethemt. The device used in 1518 * administering the medication to the patient. For example, a 1519 * particular infusion pump.) 1520 */ 1521 public Device addDeviceTarget() { 1522 Device r = new Device(); 1523 if (this.deviceTarget == null) 1524 this.deviceTarget = new ArrayList<Device>(); 1525 this.deviceTarget.add(r); 1526 return r; 1527 } 1528 1529 /** 1530 * @return {@link #note} (Extra information about the medication administration 1531 * that is not conveyed by the other attributes.). This is the 1532 * underlying object with id, value and extensions. The accessor 1533 * "getNote" gives direct access to the value 1534 */ 1535 public StringType getNoteElement() { 1536 if (this.note == null) 1537 if (Configuration.errorOnAutoCreate()) 1538 throw new Error("Attempt to auto-create MedicationAdministration.note"); 1539 else if (Configuration.doAutoCreate()) 1540 this.note = new StringType(); // bb 1541 return this.note; 1542 } 1543 1544 public boolean hasNoteElement() { 1545 return this.note != null && !this.note.isEmpty(); 1546 } 1547 1548 public boolean hasNote() { 1549 return this.note != null && !this.note.isEmpty(); 1550 } 1551 1552 /** 1553 * @param value {@link #note} (Extra information about the medication 1554 * administration that is not conveyed by the other attributes.). 1555 * This is the underlying object with id, value and extensions. The 1556 * accessor "getNote" gives direct access to the value 1557 */ 1558 public MedicationAdministration setNoteElement(StringType value) { 1559 this.note = value; 1560 return this; 1561 } 1562 1563 /** 1564 * @return Extra information about the medication administration that is not 1565 * conveyed by the other attributes. 1566 */ 1567 public String getNote() { 1568 return this.note == null ? null : this.note.getValue(); 1569 } 1570 1571 /** 1572 * @param value Extra information about the medication administration that is 1573 * not conveyed by the other attributes. 1574 */ 1575 public MedicationAdministration setNote(String value) { 1576 if (Utilities.noString(value)) 1577 this.note = null; 1578 else { 1579 if (this.note == null) 1580 this.note = new StringType(); 1581 this.note.setValue(value); 1582 } 1583 return this; 1584 } 1585 1586 /** 1587 * @return {@link #dosage} (Describes the medication dosage information details 1588 * e.g. dose, rate, site, route, etc.) 1589 */ 1590 public MedicationAdministrationDosageComponent getDosage() { 1591 if (this.dosage == null) 1592 if (Configuration.errorOnAutoCreate()) 1593 throw new Error("Attempt to auto-create MedicationAdministration.dosage"); 1594 else if (Configuration.doAutoCreate()) 1595 this.dosage = new MedicationAdministrationDosageComponent(); // cc 1596 return this.dosage; 1597 } 1598 1599 public boolean hasDosage() { 1600 return this.dosage != null && !this.dosage.isEmpty(); 1601 } 1602 1603 /** 1604 * @param value {@link #dosage} (Describes the medication dosage information 1605 * details e.g. dose, rate, site, route, etc.) 1606 */ 1607 public MedicationAdministration setDosage(MedicationAdministrationDosageComponent value) { 1608 this.dosage = value; 1609 return this; 1610 } 1611 1612 protected void listChildren(List<Property> childrenList) { 1613 super.listChildren(childrenList); 1614 childrenList.add(new Property("identifier", "Identifier", 1615 "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 1616 0, java.lang.Integer.MAX_VALUE, identifier)); 1617 childrenList.add(new Property("status", "code", 1618 "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 1619 0, java.lang.Integer.MAX_VALUE, status)); 1620 childrenList.add(new Property("patient", "Reference(Patient)", "The person or animal receiving the medication.", 0, 1621 java.lang.Integer.MAX_VALUE, patient)); 1622 childrenList.add(new Property("practitioner", "Reference(Practitioner|Patient|RelatedPerson)", 1623 "The individual who was responsible for giving the medication to the patient.", 0, java.lang.Integer.MAX_VALUE, 1624 practitioner)); 1625 childrenList.add(new Property("encounter", "Reference(Encounter)", 1626 "The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.", 1627 0, java.lang.Integer.MAX_VALUE, encounter)); 1628 childrenList.add(new Property("prescription", "Reference(MedicationOrder)", 1629 "The original request, instruction or authority to perform the administration.", 0, java.lang.Integer.MAX_VALUE, 1630 prescription)); 1631 childrenList.add(new Property("wasNotGiven", "boolean", 1632 "Set this to true if the record is saying that the medication was NOT administered.", 0, 1633 java.lang.Integer.MAX_VALUE, wasNotGiven)); 1634 childrenList.add(new Property("reasonNotGiven", "CodeableConcept", 1635 "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven)); 1636 childrenList.add(new Property("reasonGiven", "CodeableConcept", "A code indicating why the medication was given.", 1637 0, java.lang.Integer.MAX_VALUE, reasonGiven)); 1638 childrenList.add(new Property("effectiveTime[x]", "dateTime|Period", 1639 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 1640 0, java.lang.Integer.MAX_VALUE, effectiveTime)); 1641 childrenList.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1642 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1643 0, java.lang.Integer.MAX_VALUE, medication)); 1644 childrenList.add(new Property("device", "Reference(Device)", 1645 "The device used in administering the medication to the patient. For example, a particular infusion pump.", 0, 1646 java.lang.Integer.MAX_VALUE, device)); 1647 childrenList.add(new Property("note", "string", 1648 "Extra information about the medication administration that is not conveyed by the other attributes.", 0, 1649 java.lang.Integer.MAX_VALUE, note)); 1650 childrenList.add(new Property("dosage", "", 1651 "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1652 java.lang.Integer.MAX_VALUE, dosage)); 1653 } 1654 1655 @Override 1656 public void setProperty(String name, Base value) throws FHIRException { 1657 if (name.equals("identifier")) 1658 this.getIdentifier().add(castToIdentifier(value)); 1659 else if (name.equals("status")) 1660 this.status = new MedicationAdministrationStatusEnumFactory().fromType(value); // Enumeration<MedicationAdministrationStatus> 1661 else if (name.equals("patient")) 1662 this.patient = castToReference(value); // Reference 1663 else if (name.equals("practitioner")) 1664 this.practitioner = castToReference(value); // Reference 1665 else if (name.equals("encounter")) 1666 this.encounter = castToReference(value); // Reference 1667 else if (name.equals("prescription")) 1668 this.prescription = castToReference(value); // Reference 1669 else if (name.equals("wasNotGiven")) 1670 this.wasNotGiven = castToBoolean(value); // BooleanType 1671 else if (name.equals("reasonNotGiven")) 1672 this.getReasonNotGiven().add(castToCodeableConcept(value)); 1673 else if (name.equals("reasonGiven")) 1674 this.getReasonGiven().add(castToCodeableConcept(value)); 1675 else if (name.equals("effectiveTime[x]")) 1676 this.effectiveTime = (Type) value; // Type 1677 else if (name.equals("medication[x]")) 1678 this.medication = (Type) value; // Type 1679 else if (name.equals("device")) 1680 this.getDevice().add(castToReference(value)); 1681 else if (name.equals("note")) 1682 this.note = castToString(value); // StringType 1683 else if (name.equals("dosage")) 1684 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 1685 else 1686 super.setProperty(name, value); 1687 } 1688 1689 @Override 1690 public Base addChild(String name) throws FHIRException { 1691 if (name.equals("identifier")) { 1692 return addIdentifier(); 1693 } else if (name.equals("status")) { 1694 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.status"); 1695 } else if (name.equals("patient")) { 1696 this.patient = new Reference(); 1697 return this.patient; 1698 } else if (name.equals("practitioner")) { 1699 this.practitioner = new Reference(); 1700 return this.practitioner; 1701 } else if (name.equals("encounter")) { 1702 this.encounter = new Reference(); 1703 return this.encounter; 1704 } else if (name.equals("prescription")) { 1705 this.prescription = new Reference(); 1706 return this.prescription; 1707 } else if (name.equals("wasNotGiven")) { 1708 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.wasNotGiven"); 1709 } else if (name.equals("reasonNotGiven")) { 1710 return addReasonNotGiven(); 1711 } else if (name.equals("reasonGiven")) { 1712 return addReasonGiven(); 1713 } else if (name.equals("effectiveTimeDateTime")) { 1714 this.effectiveTime = new DateTimeType(); 1715 return this.effectiveTime; 1716 } else if (name.equals("effectiveTimePeriod")) { 1717 this.effectiveTime = new Period(); 1718 return this.effectiveTime; 1719 } else if (name.equals("medicationCodeableConcept")) { 1720 this.medication = new CodeableConcept(); 1721 return this.medication; 1722 } else if (name.equals("medicationReference")) { 1723 this.medication = new Reference(); 1724 return this.medication; 1725 } else if (name.equals("device")) { 1726 return addDevice(); 1727 } else if (name.equals("note")) { 1728 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.note"); 1729 } else if (name.equals("dosage")) { 1730 this.dosage = new MedicationAdministrationDosageComponent(); 1731 return this.dosage; 1732 } else 1733 return super.addChild(name); 1734 } 1735 1736 public String fhirType() { 1737 return "MedicationAdministration"; 1738 1739 } 1740 1741 public MedicationAdministration copy() { 1742 MedicationAdministration dst = new MedicationAdministration(); 1743 copyValues(dst); 1744 if (identifier != null) { 1745 dst.identifier = new ArrayList<Identifier>(); 1746 for (Identifier i : identifier) 1747 dst.identifier.add(i.copy()); 1748 } 1749 ; 1750 dst.status = status == null ? null : status.copy(); 1751 dst.patient = patient == null ? null : patient.copy(); 1752 dst.practitioner = practitioner == null ? null : practitioner.copy(); 1753 dst.encounter = encounter == null ? null : encounter.copy(); 1754 dst.prescription = prescription == null ? null : prescription.copy(); 1755 dst.wasNotGiven = wasNotGiven == null ? null : wasNotGiven.copy(); 1756 if (reasonNotGiven != null) { 1757 dst.reasonNotGiven = new ArrayList<CodeableConcept>(); 1758 for (CodeableConcept i : reasonNotGiven) 1759 dst.reasonNotGiven.add(i.copy()); 1760 } 1761 ; 1762 if (reasonGiven != null) { 1763 dst.reasonGiven = new ArrayList<CodeableConcept>(); 1764 for (CodeableConcept i : reasonGiven) 1765 dst.reasonGiven.add(i.copy()); 1766 } 1767 ; 1768 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 1769 dst.medication = medication == null ? null : medication.copy(); 1770 if (device != null) { 1771 dst.device = new ArrayList<Reference>(); 1772 for (Reference i : device) 1773 dst.device.add(i.copy()); 1774 } 1775 ; 1776 dst.note = note == null ? null : note.copy(); 1777 dst.dosage = dosage == null ? null : dosage.copy(); 1778 return dst; 1779 } 1780 1781 protected MedicationAdministration typedCopy() { 1782 return copy(); 1783 } 1784 1785 @Override 1786 public boolean equalsDeep(Base other) { 1787 if (!super.equalsDeep(other)) 1788 return false; 1789 if (!(other instanceof MedicationAdministration)) 1790 return false; 1791 MedicationAdministration o = (MedicationAdministration) other; 1792 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1793 && compareDeep(patient, o.patient, true) && compareDeep(practitioner, o.practitioner, true) 1794 && compareDeep(encounter, o.encounter, true) && compareDeep(prescription, o.prescription, true) 1795 && compareDeep(wasNotGiven, o.wasNotGiven, true) && compareDeep(reasonNotGiven, o.reasonNotGiven, true) 1796 && compareDeep(reasonGiven, o.reasonGiven, true) && compareDeep(effectiveTime, o.effectiveTime, true) 1797 && compareDeep(medication, o.medication, true) && compareDeep(device, o.device, true) 1798 && compareDeep(note, o.note, true) && compareDeep(dosage, o.dosage, true); 1799 } 1800 1801 @Override 1802 public boolean equalsShallow(Base other) { 1803 if (!super.equalsShallow(other)) 1804 return false; 1805 if (!(other instanceof MedicationAdministration)) 1806 return false; 1807 MedicationAdministration o = (MedicationAdministration) other; 1808 return compareValues(status, o.status, true) && compareValues(wasNotGiven, o.wasNotGiven, true) 1809 && compareValues(note, o.note, true); 1810 } 1811 1812 public boolean isEmpty() { 1813 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 1814 && (patient == null || patient.isEmpty()) && (practitioner == null || practitioner.isEmpty()) 1815 && (encounter == null || encounter.isEmpty()) && (prescription == null || prescription.isEmpty()) 1816 && (wasNotGiven == null || wasNotGiven.isEmpty()) && (reasonNotGiven == null || reasonNotGiven.isEmpty()) 1817 && (reasonGiven == null || reasonGiven.isEmpty()) && (effectiveTime == null || effectiveTime.isEmpty()) 1818 && (medication == null || medication.isEmpty()) && (device == null || device.isEmpty()) 1819 && (note == null || note.isEmpty()) && (dosage == null || dosage.isEmpty()); 1820 } 1821 1822 @Override 1823 public ResourceType getResourceType() { 1824 return ResourceType.MedicationAdministration; 1825 } 1826 1827 @SearchParamDefinition(name = "identifier", path = "MedicationAdministration.identifier", description = "Return administrations with this external identifier", type = "token") 1828 public static final String SP_IDENTIFIER = "identifier"; 1829 @SearchParamDefinition(name = "code", path = "MedicationAdministration.medicationCodeableConcept", description = "Return administrations of this medication code", type = "token") 1830 public static final String SP_CODE = "code"; 1831 @SearchParamDefinition(name = "prescription", path = "MedicationAdministration.prescription", description = "The identity of a prescription to list administrations from", type = "reference") 1832 public static final String SP_PRESCRIPTION = "prescription"; 1833 @SearchParamDefinition(name = "effectivetime", path = "MedicationAdministration.effectiveTime[x]", description = "Date administration happened (or did not happen)", type = "date") 1834 public static final String SP_EFFECTIVETIME = "effectivetime"; 1835 @SearchParamDefinition(name = "practitioner", path = "MedicationAdministration.practitioner", description = "Who administered substance", type = "reference") 1836 public static final String SP_PRACTITIONER = "practitioner"; 1837 @SearchParamDefinition(name = "patient", path = "MedicationAdministration.patient", description = "The identity of a patient to list administrations for", type = "reference") 1838 public static final String SP_PATIENT = "patient"; 1839 @SearchParamDefinition(name = "medication", path = "MedicationAdministration.medicationReference", description = "Return administrations of this medication resource", type = "reference") 1840 public static final String SP_MEDICATION = "medication"; 1841 @SearchParamDefinition(name = "encounter", path = "MedicationAdministration.encounter", description = "Return administrations that share this encounter", type = "reference") 1842 public static final String SP_ENCOUNTER = "encounter"; 1843 @SearchParamDefinition(name = "device", path = "MedicationAdministration.device", description = "Return administrations with this administration device identity", type = "reference") 1844 public static final String SP_DEVICE = "device"; 1845 @SearchParamDefinition(name = "notgiven", path = "MedicationAdministration.wasNotGiven", description = "Administrations that were not made", type = "token") 1846 public static final String SP_NOTGIVEN = "notgiven"; 1847 @SearchParamDefinition(name = "status", path = "MedicationAdministration.status", description = "MedicationAdministration event status (for example one of active/paused/completed/nullified)", type = "token") 1848 public static final String SP_STATUS = "status"; 1849 1850}