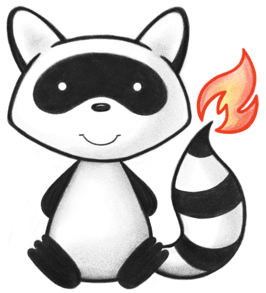
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Indicates that a medication product is to be or has been dispensed for a 048 * named person/patient. This includes a description of the medication product 049 * (supply) provided and the instructions for administering the medication. The 050 * medication dispense is the result of a pharmacy system responding to a 051 * medication order. 052 */ 053@ResourceDef(name = "MedicationDispense", profile = "http://hl7.org/fhir/Profile/MedicationDispense") 054public class MedicationDispense extends DomainResource { 055 056 public enum MedicationDispenseStatus { 057 /** 058 * The dispense has started but has not yet completed. 059 */ 060 INPROGRESS, 061 /** 062 * Actions implied by the administration have been temporarily halted, but are 063 * expected to continue later. May also be called "suspended" 064 */ 065 ONHOLD, 066 /** 067 * All actions that are implied by the dispense have occurred. 068 */ 069 COMPLETED, 070 /** 071 * The dispense was entered in error and therefore nullified. 072 */ 073 ENTEREDINERROR, 074 /** 075 * Actions implied by the dispense have been permanently halted, before all of 076 * them occurred. 077 */ 078 STOPPED, 079 /** 080 * added to help the parsers 081 */ 082 NULL; 083 084 public static MedicationDispenseStatus fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("in-progress".equals(codeString)) 088 return INPROGRESS; 089 if ("on-hold".equals(codeString)) 090 return ONHOLD; 091 if ("completed".equals(codeString)) 092 return COMPLETED; 093 if ("entered-in-error".equals(codeString)) 094 return ENTEREDINERROR; 095 if ("stopped".equals(codeString)) 096 return STOPPED; 097 throw new FHIRException("Unknown MedicationDispenseStatus code '" + codeString + "'"); 098 } 099 100 public String toCode() { 101 switch (this) { 102 case INPROGRESS: 103 return "in-progress"; 104 case ONHOLD: 105 return "on-hold"; 106 case COMPLETED: 107 return "completed"; 108 case ENTEREDINERROR: 109 return "entered-in-error"; 110 case STOPPED: 111 return "stopped"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getSystem() { 120 switch (this) { 121 case INPROGRESS: 122 return "http://hl7.org/fhir/medication-dispense-status"; 123 case ONHOLD: 124 return "http://hl7.org/fhir/medication-dispense-status"; 125 case COMPLETED: 126 return "http://hl7.org/fhir/medication-dispense-status"; 127 case ENTEREDINERROR: 128 return "http://hl7.org/fhir/medication-dispense-status"; 129 case STOPPED: 130 return "http://hl7.org/fhir/medication-dispense-status"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case INPROGRESS: 141 return "The dispense has started but has not yet completed."; 142 case ONHOLD: 143 return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called \"suspended\""; 144 case COMPLETED: 145 return "All actions that are implied by the dispense have occurred."; 146 case ENTEREDINERROR: 147 return "The dispense was entered in error and therefore nullified."; 148 case STOPPED: 149 return "Actions implied by the dispense have been permanently halted, before all of them occurred."; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDisplay() { 158 switch (this) { 159 case INPROGRESS: 160 return "In Progress"; 161 case ONHOLD: 162 return "On Hold"; 163 case COMPLETED: 164 return "Completed"; 165 case ENTEREDINERROR: 166 return "Entered in-Error"; 167 case STOPPED: 168 return "Stopped"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 } 176 177 public static class MedicationDispenseStatusEnumFactory implements EnumFactory<MedicationDispenseStatus> { 178 public MedicationDispenseStatus fromCode(String codeString) throws IllegalArgumentException { 179 if (codeString == null || "".equals(codeString)) 180 if (codeString == null || "".equals(codeString)) 181 return null; 182 if ("in-progress".equals(codeString)) 183 return MedicationDispenseStatus.INPROGRESS; 184 if ("on-hold".equals(codeString)) 185 return MedicationDispenseStatus.ONHOLD; 186 if ("completed".equals(codeString)) 187 return MedicationDispenseStatus.COMPLETED; 188 if ("entered-in-error".equals(codeString)) 189 return MedicationDispenseStatus.ENTEREDINERROR; 190 if ("stopped".equals(codeString)) 191 return MedicationDispenseStatus.STOPPED; 192 throw new IllegalArgumentException("Unknown MedicationDispenseStatus code '" + codeString + "'"); 193 } 194 195 public Enumeration<MedicationDispenseStatus> fromType(Base code) throws FHIRException { 196 if (code == null || code.isEmpty()) 197 return null; 198 String codeString = ((PrimitiveType) code).asStringValue(); 199 if (codeString == null || "".equals(codeString)) 200 return null; 201 if ("in-progress".equals(codeString)) 202 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.INPROGRESS); 203 if ("on-hold".equals(codeString)) 204 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.ONHOLD); 205 if ("completed".equals(codeString)) 206 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.COMPLETED); 207 if ("entered-in-error".equals(codeString)) 208 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.ENTEREDINERROR); 209 if ("stopped".equals(codeString)) 210 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.STOPPED); 211 throw new FHIRException("Unknown MedicationDispenseStatus code '" + codeString + "'"); 212 } 213 214 public String toCode(MedicationDispenseStatus code) 215 { 216 if (code == MedicationDispenseStatus.NULL) 217 return null; 218 if (code == MedicationDispenseStatus.INPROGRESS) 219 return "in-progress"; 220 if (code == MedicationDispenseStatus.ONHOLD) 221 return "on-hold"; 222 if (code == MedicationDispenseStatus.COMPLETED) 223 return "completed"; 224 if (code == MedicationDispenseStatus.ENTEREDINERROR) 225 return "entered-in-error"; 226 if (code == MedicationDispenseStatus.STOPPED) 227 return "stopped"; 228 return "?"; 229 } 230 } 231 232 @Block() 233 public static class MedicationDispenseDosageInstructionComponent extends BackboneElement 234 implements IBaseBackboneElement { 235 /** 236 * Free text dosage instructions can be used for cases where the instructions 237 * are too complex to code. When coded instructions are present, the free text 238 * instructions may still be present for display to humans taking or 239 * administering the medication. 240 */ 241 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 242 @Description(shortDefinition = "Dosage Instructions", formalDefinition = "Free text dosage instructions can be used for cases where the instructions are too complex to code. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication.") 243 protected StringType text; 244 245 /** 246 * Additional instructions such as "Swallow with plenty of water" which may or 247 * may not be coded. 248 */ 249 @Child(name = "additionalInstructions", type = { 250 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 251 @Description(shortDefinition = "E.g. \"Take with food\"", formalDefinition = "Additional instructions such as \"Swallow with plenty of water\" which may or may not be coded.") 252 protected CodeableConcept additionalInstructions; 253 254 /** 255 * The timing schedule for giving the medication to the patient. The Schedule 256 * data type allows many different expressions. For example, "Every 8 hours"; 257 * "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 258 * 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013". 259 */ 260 @Child(name = "timing", type = { Timing.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 261 @Description(shortDefinition = "When medication should be administered", formalDefinition = "The timing schedule for giving the medication to the patient. The Schedule data type allows many different expressions. For example, \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".") 262 protected Timing timing; 263 264 /** 265 * Indicates whether the Medication is only taken when needed within a specific 266 * dosing schedule (Boolean option), or it indicates the precondition for taking 267 * the Medication (CodeableConcept). 268 * 269 * Specifically if 'boolean' datatype is selected, then the following logic 270 * applies: If set to True, this indicates that the medication is only taken 271 * when needed, within the specified schedule. 272 */ 273 @Child(name = "asNeeded", type = { BooleanType.class, 274 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 275 @Description(shortDefinition = "Take \"as needed\" f(or x)", formalDefinition = "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept). \n\nSpecifically if 'boolean' datatype is selected, then the following logic applies: If set to True, this indicates that the medication is only taken when needed, within the specified schedule.") 276 protected Type asNeeded; 277 278 /** 279 * A coded specification of the anatomic site where the medication first enters 280 * the body. 281 */ 282 @Child(name = "site", type = { CodeableConcept.class, 283 BodySite.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 284 @Description(shortDefinition = "Body site to administer to", formalDefinition = "A coded specification of the anatomic site where the medication first enters the body.") 285 protected Type site; 286 287 /** 288 * A code specifying the route or physiological path of administration of a 289 * therapeutic agent into or onto a subject. 290 */ 291 @Child(name = "route", type = { 292 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 293 @Description(shortDefinition = "How drug should enter body", formalDefinition = "A code specifying the route or physiological path of administration of a therapeutic agent into or onto a subject.") 294 protected CodeableConcept route; 295 296 /** 297 * A coded value indicating the method by which the medication is intended to be 298 * or was introduced into or on the body. 299 */ 300 @Child(name = "method", type = { 301 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 302 @Description(shortDefinition = "Technique for administering medication", formalDefinition = "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body.") 303 protected CodeableConcept method; 304 305 /** 306 * The amount of therapeutic or other substance given at one administration 307 * event. 308 */ 309 @Child(name = "dose", type = { Range.class, 310 SimpleQuantity.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 311 @Description(shortDefinition = "Amount of medication per dose", formalDefinition = "The amount of therapeutic or other substance given at one administration event.") 312 protected Type dose; 313 314 /** 315 * Identifies the speed with which the medication was or will be introduced into 316 * the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 317 * ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 318 * hours. Currently we do not specify a default of '1' in the denominator, but 319 * this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 320 * liter/8 hours. 321 */ 322 @Child(name = "rate", type = { Ratio.class, 323 Range.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 324 @Description(shortDefinition = "Amount of medication per unit of time", formalDefinition = "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.") 325 protected Type rate; 326 327 /** 328 * The maximum total quantity of a therapeutic substance that may be 329 * administered to a subject over the period of time, e.g. 1000mg in 24 hours. 330 */ 331 @Child(name = "maxDosePerPeriod", type = { 332 Ratio.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 333 @Description(shortDefinition = "Upper limit on medication per unit of time", formalDefinition = "The maximum total quantity of a therapeutic substance that may be administered to a subject over the period of time, e.g. 1000mg in 24 hours.") 334 protected Ratio maxDosePerPeriod; 335 336 private static final long serialVersionUID = -1470136646L; 337 338 /* 339 * Constructor 340 */ 341 public MedicationDispenseDosageInstructionComponent() { 342 super(); 343 } 344 345 /** 346 * @return {@link #text} (Free text dosage instructions can be used for cases 347 * where the instructions are too complex to code. When coded 348 * instructions are present, the free text instructions may still be 349 * present for display to humans taking or administering the 350 * medication.). This is the underlying object with id, value and 351 * extensions. The accessor "getText" gives direct access to the value 352 */ 353 public StringType getTextElement() { 354 if (this.text == null) 355 if (Configuration.errorOnAutoCreate()) 356 throw new Error("Attempt to auto-create MedicationDispenseDosageInstructionComponent.text"); 357 else if (Configuration.doAutoCreate()) 358 this.text = new StringType(); // bb 359 return this.text; 360 } 361 362 public boolean hasTextElement() { 363 return this.text != null && !this.text.isEmpty(); 364 } 365 366 public boolean hasText() { 367 return this.text != null && !this.text.isEmpty(); 368 } 369 370 /** 371 * @param value {@link #text} (Free text dosage instructions can be used for 372 * cases where the instructions are too complex to code. When coded 373 * instructions are present, the free text instructions may still 374 * be present for display to humans taking or administering the 375 * medication.). This is the underlying object with id, value and 376 * extensions. The accessor "getText" gives direct access to the 377 * value 378 */ 379 public MedicationDispenseDosageInstructionComponent setTextElement(StringType value) { 380 this.text = value; 381 return this; 382 } 383 384 /** 385 * @return Free text dosage instructions can be used for cases where the 386 * instructions are too complex to code. When coded instructions are 387 * present, the free text instructions may still be present for display 388 * to humans taking or administering the medication. 389 */ 390 public String getText() { 391 return this.text == null ? null : this.text.getValue(); 392 } 393 394 /** 395 * @param value Free text dosage instructions can be used for cases where the 396 * instructions are too complex to code. When coded instructions 397 * are present, the free text instructions may still be present for 398 * display to humans taking or administering the medication. 399 */ 400 public MedicationDispenseDosageInstructionComponent setText(String value) { 401 if (Utilities.noString(value)) 402 this.text = null; 403 else { 404 if (this.text == null) 405 this.text = new StringType(); 406 this.text.setValue(value); 407 } 408 return this; 409 } 410 411 /** 412 * @return {@link #additionalInstructions} (Additional instructions such as 413 * "Swallow with plenty of water" which may or may not be coded.) 414 */ 415 public CodeableConcept getAdditionalInstructions() { 416 if (this.additionalInstructions == null) 417 if (Configuration.errorOnAutoCreate()) 418 throw new Error("Attempt to auto-create MedicationDispenseDosageInstructionComponent.additionalInstructions"); 419 else if (Configuration.doAutoCreate()) 420 this.additionalInstructions = new CodeableConcept(); // cc 421 return this.additionalInstructions; 422 } 423 424 public boolean hasAdditionalInstructions() { 425 return this.additionalInstructions != null && !this.additionalInstructions.isEmpty(); 426 } 427 428 /** 429 * @param value {@link #additionalInstructions} (Additional instructions such as 430 * "Swallow with plenty of water" which may or may not be coded.) 431 */ 432 public MedicationDispenseDosageInstructionComponent setAdditionalInstructions(CodeableConcept value) { 433 this.additionalInstructions = value; 434 return this; 435 } 436 437 /** 438 * @return {@link #timing} (The timing schedule for giving the medication to the 439 * patient. The Schedule data type allows many different expressions. 440 * For example, "Every 8 hours"; "Three times a day"; "1/2 an hour 441 * before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 442 * 2013 and 1 Nov 2013".) 443 */ 444 public Timing getTiming() { 445 if (this.timing == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create MedicationDispenseDosageInstructionComponent.timing"); 448 else if (Configuration.doAutoCreate()) 449 this.timing = new Timing(); // cc 450 return this.timing; 451 } 452 453 public boolean hasTiming() { 454 return this.timing != null && !this.timing.isEmpty(); 455 } 456 457 /** 458 * @param value {@link #timing} (The timing schedule for giving the medication 459 * to the patient. The Schedule data type allows many different 460 * expressions. For example, "Every 8 hours"; "Three times a day"; 461 * "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; 462 * "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 463 */ 464 public MedicationDispenseDosageInstructionComponent setTiming(Timing value) { 465 this.timing = value; 466 return this; 467 } 468 469 /** 470 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 471 * when needed within a specific dosing schedule (Boolean option), or it 472 * indicates the precondition for taking the Medication 473 * (CodeableConcept). 474 * 475 * Specifically if 'boolean' datatype is selected, then the following 476 * logic applies: If set to True, this indicates that the medication is 477 * only taken when needed, within the specified schedule.) 478 */ 479 public Type getAsNeeded() { 480 return this.asNeeded; 481 } 482 483 /** 484 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 485 * when needed within a specific dosing schedule (Boolean option), or it 486 * indicates the precondition for taking the Medication 487 * (CodeableConcept). 488 * 489 * Specifically if 'boolean' datatype is selected, then the following 490 * logic applies: If set to True, this indicates that the medication is 491 * only taken when needed, within the specified schedule.) 492 */ 493 public BooleanType getAsNeededBooleanType() throws FHIRException { 494 if (!(this.asNeeded instanceof BooleanType)) 495 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 496 + this.asNeeded.getClass().getName() + " was encountered"); 497 return (BooleanType) this.asNeeded; 498 } 499 500 public boolean hasAsNeededBooleanType() { 501 return this.asNeeded instanceof BooleanType; 502 } 503 504 /** 505 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 506 * when needed within a specific dosing schedule (Boolean option), or it 507 * indicates the precondition for taking the Medication 508 * (CodeableConcept). 509 * 510 * Specifically if 'boolean' datatype is selected, then the following 511 * logic applies: If set to True, this indicates that the medication is 512 * only taken when needed, within the specified schedule.) 513 */ 514 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 515 if (!(this.asNeeded instanceof CodeableConcept)) 516 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 517 + this.asNeeded.getClass().getName() + " was encountered"); 518 return (CodeableConcept) this.asNeeded; 519 } 520 521 public boolean hasAsNeededCodeableConcept() { 522 return this.asNeeded instanceof CodeableConcept; 523 } 524 525 public boolean hasAsNeeded() { 526 return this.asNeeded != null && !this.asNeeded.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #asNeeded} (Indicates whether the Medication is only 531 * taken when needed within a specific dosing schedule (Boolean 532 * option), or it indicates the precondition for taking the 533 * Medication (CodeableConcept). 534 * 535 * Specifically if 'boolean' datatype is selected, then the 536 * following logic applies: If set to True, this indicates that the 537 * medication is only taken when needed, within the specified 538 * schedule.) 539 */ 540 public MedicationDispenseDosageInstructionComponent setAsNeeded(Type value) { 541 this.asNeeded = value; 542 return this; 543 } 544 545 /** 546 * @return {@link #site} (A coded specification of the anatomic site where the 547 * medication first enters the body.) 548 */ 549 public Type getSite() { 550 return this.site; 551 } 552 553 /** 554 * @return {@link #site} (A coded specification of the anatomic site where the 555 * medication first enters the body.) 556 */ 557 public CodeableConcept getSiteCodeableConcept() throws FHIRException { 558 if (!(this.site instanceof CodeableConcept)) 559 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 560 + this.site.getClass().getName() + " was encountered"); 561 return (CodeableConcept) this.site; 562 } 563 564 public boolean hasSiteCodeableConcept() { 565 return this.site instanceof CodeableConcept; 566 } 567 568 /** 569 * @return {@link #site} (A coded specification of the anatomic site where the 570 * medication first enters the body.) 571 */ 572 public Reference getSiteReference() throws FHIRException { 573 if (!(this.site instanceof Reference)) 574 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.site.getClass().getName() 575 + " was encountered"); 576 return (Reference) this.site; 577 } 578 579 public boolean hasSiteReference() { 580 return this.site instanceof Reference; 581 } 582 583 public boolean hasSite() { 584 return this.site != null && !this.site.isEmpty(); 585 } 586 587 /** 588 * @param value {@link #site} (A coded specification of the anatomic site where 589 * the medication first enters the body.) 590 */ 591 public MedicationDispenseDosageInstructionComponent setSite(Type value) { 592 this.site = value; 593 return this; 594 } 595 596 /** 597 * @return {@link #route} (A code specifying the route or physiological path of 598 * administration of a therapeutic agent into or onto a subject.) 599 */ 600 public CodeableConcept getRoute() { 601 if (this.route == null) 602 if (Configuration.errorOnAutoCreate()) 603 throw new Error("Attempt to auto-create MedicationDispenseDosageInstructionComponent.route"); 604 else if (Configuration.doAutoCreate()) 605 this.route = new CodeableConcept(); // cc 606 return this.route; 607 } 608 609 public boolean hasRoute() { 610 return this.route != null && !this.route.isEmpty(); 611 } 612 613 /** 614 * @param value {@link #route} (A code specifying the route or physiological 615 * path of administration of a therapeutic agent into or onto a 616 * subject.) 617 */ 618 public MedicationDispenseDosageInstructionComponent setRoute(CodeableConcept value) { 619 this.route = value; 620 return this; 621 } 622 623 /** 624 * @return {@link #method} (A coded value indicating the method by which the 625 * medication is intended to be or was introduced into or on the body.) 626 */ 627 public CodeableConcept getMethod() { 628 if (this.method == null) 629 if (Configuration.errorOnAutoCreate()) 630 throw new Error("Attempt to auto-create MedicationDispenseDosageInstructionComponent.method"); 631 else if (Configuration.doAutoCreate()) 632 this.method = new CodeableConcept(); // cc 633 return this.method; 634 } 635 636 public boolean hasMethod() { 637 return this.method != null && !this.method.isEmpty(); 638 } 639 640 /** 641 * @param value {@link #method} (A coded value indicating the method by which 642 * the medication is intended to be or was introduced into or on 643 * the body.) 644 */ 645 public MedicationDispenseDosageInstructionComponent setMethod(CodeableConcept value) { 646 this.method = value; 647 return this; 648 } 649 650 /** 651 * @return {@link #dose} (The amount of therapeutic or other substance given at 652 * one administration event.) 653 */ 654 public Type getDose() { 655 return this.dose; 656 } 657 658 /** 659 * @return {@link #dose} (The amount of therapeutic or other substance given at 660 * one administration event.) 661 */ 662 public Range getDoseRange() throws FHIRException { 663 if (!(this.dose instanceof Range)) 664 throw new FHIRException( 665 "Type mismatch: the type Range was expected, but " + this.dose.getClass().getName() + " was encountered"); 666 return (Range) this.dose; 667 } 668 669 public boolean hasDoseRange() { 670 return this.dose instanceof Range; 671 } 672 673 /** 674 * @return {@link #dose} (The amount of therapeutic or other substance given at 675 * one administration event.) 676 */ 677 public SimpleQuantity getDoseSimpleQuantity() throws FHIRException { 678 if (!(this.dose instanceof SimpleQuantity)) 679 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but " 680 + this.dose.getClass().getName() + " was encountered"); 681 return (SimpleQuantity) this.dose; 682 } 683 684 public boolean hasDoseSimpleQuantity() { 685 return this.dose instanceof SimpleQuantity; 686 } 687 688 public boolean hasDose() { 689 return this.dose != null && !this.dose.isEmpty(); 690 } 691 692 /** 693 * @param value {@link #dose} (The amount of therapeutic or other substance 694 * given at one administration event.) 695 */ 696 public MedicationDispenseDosageInstructionComponent setDose(Type value) { 697 this.dose = value; 698 return this; 699 } 700 701 /** 702 * @return {@link #rate} (Identifies the speed with which the medication was or 703 * will be introduced into the patient. Typically the rate for an 704 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 705 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 706 * not specify a default of '1' in the denominator, but this is being 707 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 708 * hours.) 709 */ 710 public Type getRate() { 711 return this.rate; 712 } 713 714 /** 715 * @return {@link #rate} (Identifies the speed with which the medication was or 716 * will be introduced into the patient. Typically the rate for an 717 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 718 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 719 * not specify a default of '1' in the denominator, but this is being 720 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 721 * hours.) 722 */ 723 public Ratio getRateRatio() throws FHIRException { 724 if (!(this.rate instanceof Ratio)) 725 throw new FHIRException( 726 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 727 return (Ratio) this.rate; 728 } 729 730 public boolean hasRateRatio() { 731 return this.rate instanceof Ratio; 732 } 733 734 /** 735 * @return {@link #rate} (Identifies the speed with which the medication was or 736 * will be introduced into the patient. Typically the rate for an 737 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 738 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 739 * not specify a default of '1' in the denominator, but this is being 740 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 741 * hours.) 742 */ 743 public Range getRateRange() throws FHIRException { 744 if (!(this.rate instanceof Range)) 745 throw new FHIRException( 746 "Type mismatch: the type Range was expected, but " + this.rate.getClass().getName() + " was encountered"); 747 return (Range) this.rate; 748 } 749 750 public boolean hasRateRange() { 751 return this.rate instanceof Range; 752 } 753 754 public boolean hasRate() { 755 return this.rate != null && !this.rate.isEmpty(); 756 } 757 758 /** 759 * @param value {@link #rate} (Identifies the speed with which the medication 760 * was or will be introduced into the patient. Typically the rate 761 * for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be 762 * expressed as a rate per unit of time e.g. 500 ml per 2 hours. 763 * Currently we do not specify a default of '1' in the denominator, 764 * but this is being discussed. Other examples: 200 mcg/min or 200 765 * mcg/1 minute; 1 liter/8 hours.) 766 */ 767 public MedicationDispenseDosageInstructionComponent setRate(Type value) { 768 this.rate = value; 769 return this; 770 } 771 772 /** 773 * @return {@link #maxDosePerPeriod} (The maximum total quantity of a 774 * therapeutic substance that may be administered to a subject over the 775 * period of time, e.g. 1000mg in 24 hours.) 776 */ 777 public Ratio getMaxDosePerPeriod() { 778 if (this.maxDosePerPeriod == null) 779 if (Configuration.errorOnAutoCreate()) 780 throw new Error("Attempt to auto-create MedicationDispenseDosageInstructionComponent.maxDosePerPeriod"); 781 else if (Configuration.doAutoCreate()) 782 this.maxDosePerPeriod = new Ratio(); // cc 783 return this.maxDosePerPeriod; 784 } 785 786 public boolean hasMaxDosePerPeriod() { 787 return this.maxDosePerPeriod != null && !this.maxDosePerPeriod.isEmpty(); 788 } 789 790 /** 791 * @param value {@link #maxDosePerPeriod} (The maximum total quantity of a 792 * therapeutic substance that may be administered to a subject over 793 * the period of time, e.g. 1000mg in 24 hours.) 794 */ 795 public MedicationDispenseDosageInstructionComponent setMaxDosePerPeriod(Ratio value) { 796 this.maxDosePerPeriod = value; 797 return this; 798 } 799 800 protected void listChildren(List<Property> childrenList) { 801 super.listChildren(childrenList); 802 childrenList.add(new Property("text", "string", 803 "Free text dosage instructions can be used for cases where the instructions are too complex to code. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication.", 804 0, java.lang.Integer.MAX_VALUE, text)); 805 childrenList.add(new Property("additionalInstructions", "CodeableConcept", 806 "Additional instructions such as \"Swallow with plenty of water\" which may or may not be coded.", 0, 807 java.lang.Integer.MAX_VALUE, additionalInstructions)); 808 childrenList.add(new Property("timing", "Timing", 809 "The timing schedule for giving the medication to the patient. The Schedule data type allows many different expressions. For example, \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 810 0, java.lang.Integer.MAX_VALUE, timing)); 811 childrenList.add(new Property("asNeeded[x]", "boolean|CodeableConcept", 812 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept). \n\nSpecifically if 'boolean' datatype is selected, then the following logic applies: If set to True, this indicates that the medication is only taken when needed, within the specified schedule.", 813 0, java.lang.Integer.MAX_VALUE, asNeeded)); 814 childrenList.add(new Property("site[x]", "CodeableConcept|Reference(BodySite)", 815 "A coded specification of the anatomic site where the medication first enters the body.", 0, 816 java.lang.Integer.MAX_VALUE, site)); 817 childrenList.add(new Property("route", "CodeableConcept", 818 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto a subject.", 819 0, java.lang.Integer.MAX_VALUE, route)); 820 childrenList.add(new Property("method", "CodeableConcept", 821 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body.", 822 0, java.lang.Integer.MAX_VALUE, method)); 823 childrenList.add(new Property("dose[x]", "Range|SimpleQuantity", 824 "The amount of therapeutic or other substance given at one administration event.", 0, 825 java.lang.Integer.MAX_VALUE, dose)); 826 childrenList.add(new Property("rate[x]", "Ratio|Range", 827 "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 828 0, java.lang.Integer.MAX_VALUE, rate)); 829 childrenList.add(new Property("maxDosePerPeriod", "Ratio", 830 "The maximum total quantity of a therapeutic substance that may be administered to a subject over the period of time, e.g. 1000mg in 24 hours.", 831 0, java.lang.Integer.MAX_VALUE, maxDosePerPeriod)); 832 } 833 834 @Override 835 public void setProperty(String name, Base value) throws FHIRException { 836 if (name.equals("text")) 837 this.text = castToString(value); // StringType 838 else if (name.equals("additionalInstructions")) 839 this.additionalInstructions = castToCodeableConcept(value); // CodeableConcept 840 else if (name.equals("timing")) 841 this.timing = castToTiming(value); // Timing 842 else if (name.equals("asNeeded[x]")) 843 this.asNeeded = (Type) value; // Type 844 else if (name.equals("site[x]")) 845 this.site = (Type) value; // Type 846 else if (name.equals("route")) 847 this.route = castToCodeableConcept(value); // CodeableConcept 848 else if (name.equals("method")) 849 this.method = castToCodeableConcept(value); // CodeableConcept 850 else if (name.equals("dose[x]")) 851 this.dose = (Type) value; // Type 852 else if (name.equals("rate[x]")) 853 this.rate = (Type) value; // Type 854 else if (name.equals("maxDosePerPeriod")) 855 this.maxDosePerPeriod = castToRatio(value); // Ratio 856 else 857 super.setProperty(name, value); 858 } 859 860 @Override 861 public Base addChild(String name) throws FHIRException { 862 if (name.equals("text")) { 863 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.text"); 864 } else if (name.equals("additionalInstructions")) { 865 this.additionalInstructions = new CodeableConcept(); 866 return this.additionalInstructions; 867 } else if (name.equals("timing")) { 868 this.timing = new Timing(); 869 return this.timing; 870 } else if (name.equals("asNeededBoolean")) { 871 this.asNeeded = new BooleanType(); 872 return this.asNeeded; 873 } else if (name.equals("asNeededCodeableConcept")) { 874 this.asNeeded = new CodeableConcept(); 875 return this.asNeeded; 876 } else if (name.equals("siteCodeableConcept")) { 877 this.site = new CodeableConcept(); 878 return this.site; 879 } else if (name.equals("siteReference")) { 880 this.site = new Reference(); 881 return this.site; 882 } else if (name.equals("route")) { 883 this.route = new CodeableConcept(); 884 return this.route; 885 } else if (name.equals("method")) { 886 this.method = new CodeableConcept(); 887 return this.method; 888 } else if (name.equals("doseRange")) { 889 this.dose = new Range(); 890 return this.dose; 891 } else if (name.equals("doseSimpleQuantity")) { 892 this.dose = new SimpleQuantity(); 893 return this.dose; 894 } else if (name.equals("rateRatio")) { 895 this.rate = new Ratio(); 896 return this.rate; 897 } else if (name.equals("rateRange")) { 898 this.rate = new Range(); 899 return this.rate; 900 } else if (name.equals("maxDosePerPeriod")) { 901 this.maxDosePerPeriod = new Ratio(); 902 return this.maxDosePerPeriod; 903 } else 904 return super.addChild(name); 905 } 906 907 public MedicationDispenseDosageInstructionComponent copy() { 908 MedicationDispenseDosageInstructionComponent dst = new MedicationDispenseDosageInstructionComponent(); 909 copyValues(dst); 910 dst.text = text == null ? null : text.copy(); 911 dst.additionalInstructions = additionalInstructions == null ? null : additionalInstructions.copy(); 912 dst.timing = timing == null ? null : timing.copy(); 913 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 914 dst.site = site == null ? null : site.copy(); 915 dst.route = route == null ? null : route.copy(); 916 dst.method = method == null ? null : method.copy(); 917 dst.dose = dose == null ? null : dose.copy(); 918 dst.rate = rate == null ? null : rate.copy(); 919 dst.maxDosePerPeriod = maxDosePerPeriod == null ? null : maxDosePerPeriod.copy(); 920 return dst; 921 } 922 923 @Override 924 public boolean equalsDeep(Base other) { 925 if (!super.equalsDeep(other)) 926 return false; 927 if (!(other instanceof MedicationDispenseDosageInstructionComponent)) 928 return false; 929 MedicationDispenseDosageInstructionComponent o = (MedicationDispenseDosageInstructionComponent) other; 930 return compareDeep(text, o.text, true) && compareDeep(additionalInstructions, o.additionalInstructions, true) 931 && compareDeep(timing, o.timing, true) && compareDeep(asNeeded, o.asNeeded, true) 932 && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) && compareDeep(method, o.method, true) 933 && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true) 934 && compareDeep(maxDosePerPeriod, o.maxDosePerPeriod, true); 935 } 936 937 @Override 938 public boolean equalsShallow(Base other) { 939 if (!super.equalsShallow(other)) 940 return false; 941 if (!(other instanceof MedicationDispenseDosageInstructionComponent)) 942 return false; 943 MedicationDispenseDosageInstructionComponent o = (MedicationDispenseDosageInstructionComponent) other; 944 return compareValues(text, o.text, true); 945 } 946 947 public boolean isEmpty() { 948 return super.isEmpty() && (text == null || text.isEmpty()) 949 && (additionalInstructions == null || additionalInstructions.isEmpty()) 950 && (timing == null || timing.isEmpty()) && (asNeeded == null || asNeeded.isEmpty()) 951 && (site == null || site.isEmpty()) && (route == null || route.isEmpty()) 952 && (method == null || method.isEmpty()) && (dose == null || dose.isEmpty()) 953 && (rate == null || rate.isEmpty()) && (maxDosePerPeriod == null || maxDosePerPeriod.isEmpty()); 954 } 955 956 public String fhirType() { 957 return "MedicationDispense.dosageInstruction"; 958 959 } 960 961 } 962 963 @Block() 964 public static class MedicationDispenseSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 965 /** 966 * A code signifying whether a different drug was dispensed from what was 967 * prescribed. 968 */ 969 @Child(name = "type", type = { 970 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 971 @Description(shortDefinition = "Type of substitution", formalDefinition = "A code signifying whether a different drug was dispensed from what was prescribed.") 972 protected CodeableConcept type; 973 974 /** 975 * Indicates the reason for the substitution of (or lack of substitution) from 976 * what was prescribed. 977 */ 978 @Child(name = "reason", type = { 979 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 980 @Description(shortDefinition = "Why was substitution made", formalDefinition = "Indicates the reason for the substitution of (or lack of substitution) from what was prescribed.") 981 protected List<CodeableConcept> reason; 982 983 /** 984 * The person or organization that has primary responsibility for the 985 * substitution. 986 */ 987 @Child(name = "responsibleParty", type = { 988 Practitioner.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 989 @Description(shortDefinition = "Who is responsible for the substitution", formalDefinition = "The person or organization that has primary responsibility for the substitution.") 990 protected List<Reference> responsibleParty; 991 /** 992 * The actual objects that are the target of the reference (The person or 993 * organization that has primary responsibility for the substitution.) 994 */ 995 protected List<Practitioner> responsiblePartyTarget; 996 997 private static final long serialVersionUID = 1218245830L; 998 999 /* 1000 * Constructor 1001 */ 1002 public MedicationDispenseSubstitutionComponent() { 1003 super(); 1004 } 1005 1006 /* 1007 * Constructor 1008 */ 1009 public MedicationDispenseSubstitutionComponent(CodeableConcept type) { 1010 super(); 1011 this.type = type; 1012 } 1013 1014 /** 1015 * @return {@link #type} (A code signifying whether a different drug was 1016 * dispensed from what was prescribed.) 1017 */ 1018 public CodeableConcept getType() { 1019 if (this.type == null) 1020 if (Configuration.errorOnAutoCreate()) 1021 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.type"); 1022 else if (Configuration.doAutoCreate()) 1023 this.type = new CodeableConcept(); // cc 1024 return this.type; 1025 } 1026 1027 public boolean hasType() { 1028 return this.type != null && !this.type.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #type} (A code signifying whether a different drug was 1033 * dispensed from what was prescribed.) 1034 */ 1035 public MedicationDispenseSubstitutionComponent setType(CodeableConcept value) { 1036 this.type = value; 1037 return this; 1038 } 1039 1040 /** 1041 * @return {@link #reason} (Indicates the reason for the substitution of (or 1042 * lack of substitution) from what was prescribed.) 1043 */ 1044 public List<CodeableConcept> getReason() { 1045 if (this.reason == null) 1046 this.reason = new ArrayList<CodeableConcept>(); 1047 return this.reason; 1048 } 1049 1050 public boolean hasReason() { 1051 if (this.reason == null) 1052 return false; 1053 for (CodeableConcept item : this.reason) 1054 if (!item.isEmpty()) 1055 return true; 1056 return false; 1057 } 1058 1059 /** 1060 * @return {@link #reason} (Indicates the reason for the substitution of (or 1061 * lack of substitution) from what was prescribed.) 1062 */ 1063 // syntactic sugar 1064 public CodeableConcept addReason() { // 3 1065 CodeableConcept t = new CodeableConcept(); 1066 if (this.reason == null) 1067 this.reason = new ArrayList<CodeableConcept>(); 1068 this.reason.add(t); 1069 return t; 1070 } 1071 1072 // syntactic sugar 1073 public MedicationDispenseSubstitutionComponent addReason(CodeableConcept t) { // 3 1074 if (t == null) 1075 return this; 1076 if (this.reason == null) 1077 this.reason = new ArrayList<CodeableConcept>(); 1078 this.reason.add(t); 1079 return this; 1080 } 1081 1082 /** 1083 * @return {@link #responsibleParty} (The person or organization that has 1084 * primary responsibility for the substitution.) 1085 */ 1086 public List<Reference> getResponsibleParty() { 1087 if (this.responsibleParty == null) 1088 this.responsibleParty = new ArrayList<Reference>(); 1089 return this.responsibleParty; 1090 } 1091 1092 public boolean hasResponsibleParty() { 1093 if (this.responsibleParty == null) 1094 return false; 1095 for (Reference item : this.responsibleParty) 1096 if (!item.isEmpty()) 1097 return true; 1098 return false; 1099 } 1100 1101 /** 1102 * @return {@link #responsibleParty} (The person or organization that has 1103 * primary responsibility for the substitution.) 1104 */ 1105 // syntactic sugar 1106 public Reference addResponsibleParty() { // 3 1107 Reference t = new Reference(); 1108 if (this.responsibleParty == null) 1109 this.responsibleParty = new ArrayList<Reference>(); 1110 this.responsibleParty.add(t); 1111 return t; 1112 } 1113 1114 // syntactic sugar 1115 public MedicationDispenseSubstitutionComponent addResponsibleParty(Reference t) { // 3 1116 if (t == null) 1117 return this; 1118 if (this.responsibleParty == null) 1119 this.responsibleParty = new ArrayList<Reference>(); 1120 this.responsibleParty.add(t); 1121 return this; 1122 } 1123 1124 /** 1125 * @return {@link #responsibleParty} (The actual objects that are the target of 1126 * the reference. The reference library doesn't populate this, but you 1127 * can use this to hold the resources if you resolvethemt. The person or 1128 * organization that has primary responsibility for the substitution.) 1129 */ 1130 public List<Practitioner> getResponsiblePartyTarget() { 1131 if (this.responsiblePartyTarget == null) 1132 this.responsiblePartyTarget = new ArrayList<Practitioner>(); 1133 return this.responsiblePartyTarget; 1134 } 1135 1136 // syntactic sugar 1137 /** 1138 * @return {@link #responsibleParty} (Add an actual object that is the target of 1139 * the reference. The reference library doesn't use these, but you can 1140 * use this to hold the resources if you resolvethemt. The person or 1141 * organization that has primary responsibility for the substitution.) 1142 */ 1143 public Practitioner addResponsiblePartyTarget() { 1144 Practitioner r = new Practitioner(); 1145 if (this.responsiblePartyTarget == null) 1146 this.responsiblePartyTarget = new ArrayList<Practitioner>(); 1147 this.responsiblePartyTarget.add(r); 1148 return r; 1149 } 1150 1151 protected void listChildren(List<Property> childrenList) { 1152 super.listChildren(childrenList); 1153 childrenList.add(new Property("type", "CodeableConcept", 1154 "A code signifying whether a different drug was dispensed from what was prescribed.", 0, 1155 java.lang.Integer.MAX_VALUE, type)); 1156 childrenList.add(new Property("reason", "CodeableConcept", 1157 "Indicates the reason for the substitution of (or lack of substitution) from what was prescribed.", 0, 1158 java.lang.Integer.MAX_VALUE, reason)); 1159 childrenList.add(new Property("responsibleParty", "Reference(Practitioner)", 1160 "The person or organization that has primary responsibility for the substitution.", 0, 1161 java.lang.Integer.MAX_VALUE, responsibleParty)); 1162 } 1163 1164 @Override 1165 public void setProperty(String name, Base value) throws FHIRException { 1166 if (name.equals("type")) 1167 this.type = castToCodeableConcept(value); // CodeableConcept 1168 else if (name.equals("reason")) 1169 this.getReason().add(castToCodeableConcept(value)); 1170 else if (name.equals("responsibleParty")) 1171 this.getResponsibleParty().add(castToReference(value)); 1172 else 1173 super.setProperty(name, value); 1174 } 1175 1176 @Override 1177 public Base addChild(String name) throws FHIRException { 1178 if (name.equals("type")) { 1179 this.type = new CodeableConcept(); 1180 return this.type; 1181 } else if (name.equals("reason")) { 1182 return addReason(); 1183 } else if (name.equals("responsibleParty")) { 1184 return addResponsibleParty(); 1185 } else 1186 return super.addChild(name); 1187 } 1188 1189 public MedicationDispenseSubstitutionComponent copy() { 1190 MedicationDispenseSubstitutionComponent dst = new MedicationDispenseSubstitutionComponent(); 1191 copyValues(dst); 1192 dst.type = type == null ? null : type.copy(); 1193 if (reason != null) { 1194 dst.reason = new ArrayList<CodeableConcept>(); 1195 for (CodeableConcept i : reason) 1196 dst.reason.add(i.copy()); 1197 } 1198 ; 1199 if (responsibleParty != null) { 1200 dst.responsibleParty = new ArrayList<Reference>(); 1201 for (Reference i : responsibleParty) 1202 dst.responsibleParty.add(i.copy()); 1203 } 1204 ; 1205 return dst; 1206 } 1207 1208 @Override 1209 public boolean equalsDeep(Base other) { 1210 if (!super.equalsDeep(other)) 1211 return false; 1212 if (!(other instanceof MedicationDispenseSubstitutionComponent)) 1213 return false; 1214 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other; 1215 return compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true) 1216 && compareDeep(responsibleParty, o.responsibleParty, true); 1217 } 1218 1219 @Override 1220 public boolean equalsShallow(Base other) { 1221 if (!super.equalsShallow(other)) 1222 return false; 1223 if (!(other instanceof MedicationDispenseSubstitutionComponent)) 1224 return false; 1225 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other; 1226 return true; 1227 } 1228 1229 public boolean isEmpty() { 1230 return super.isEmpty() && (type == null || type.isEmpty()) && (reason == null || reason.isEmpty()) 1231 && (responsibleParty == null || responsibleParty.isEmpty()); 1232 } 1233 1234 public String fhirType() { 1235 return "MedicationDispense.substitution"; 1236 1237 } 1238 1239 } 1240 1241 /** 1242 * Identifier assigned by the dispensing facility - this is an identifier 1243 * assigned outside FHIR. 1244 */ 1245 @Child(name = "identifier", type = { 1246 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1247 @Description(shortDefinition = "External identifier", formalDefinition = "Identifier assigned by the dispensing facility - this is an identifier assigned outside FHIR.") 1248 protected Identifier identifier; 1249 1250 /** 1251 * A code specifying the state of the set of dispense events. 1252 */ 1253 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1254 @Description(shortDefinition = "in-progress | on-hold | completed | entered-in-error | stopped", formalDefinition = "A code specifying the state of the set of dispense events.") 1255 protected Enumeration<MedicationDispenseStatus> status; 1256 1257 /** 1258 * A link to a resource representing the person to whom the medication will be 1259 * given. 1260 */ 1261 @Child(name = "patient", type = { Patient.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1262 @Description(shortDefinition = "Who the dispense is for", formalDefinition = "A link to a resource representing the person to whom the medication will be given.") 1263 protected Reference patient; 1264 1265 /** 1266 * The actual object that is the target of the reference (A link to a resource 1267 * representing the person to whom the medication will be given.) 1268 */ 1269 protected Patient patientTarget; 1270 1271 /** 1272 * The individual responsible for dispensing the medication. 1273 */ 1274 @Child(name = "dispenser", type = { 1275 Practitioner.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1276 @Description(shortDefinition = "Practitioner responsible for dispensing medication", formalDefinition = "The individual responsible for dispensing the medication.") 1277 protected Reference dispenser; 1278 1279 /** 1280 * The actual object that is the target of the reference (The individual 1281 * responsible for dispensing the medication.) 1282 */ 1283 protected Practitioner dispenserTarget; 1284 1285 /** 1286 * Indicates the medication order that is being dispensed against. 1287 */ 1288 @Child(name = "authorizingPrescription", type = { 1289 MedicationOrder.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1290 @Description(shortDefinition = "Medication order that authorizes the dispense", formalDefinition = "Indicates the medication order that is being dispensed against.") 1291 protected List<Reference> authorizingPrescription; 1292 /** 1293 * The actual objects that are the target of the reference (Indicates the 1294 * medication order that is being dispensed against.) 1295 */ 1296 protected List<MedicationOrder> authorizingPrescriptionTarget; 1297 1298 /** 1299 * Indicates the type of dispensing event that is performed. For example, Trial 1300 * Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 1301 */ 1302 @Child(name = "type", type = { CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1303 @Description(shortDefinition = "Trial fill, partial fill, emergency fill, etc.", formalDefinition = "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.") 1304 protected CodeableConcept type; 1305 1306 /** 1307 * The amount of medication that has been dispensed. Includes unit of measure. 1308 */ 1309 @Child(name = "quantity", type = { 1310 SimpleQuantity.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1311 @Description(shortDefinition = "Amount dispensed", formalDefinition = "The amount of medication that has been dispensed. Includes unit of measure.") 1312 protected SimpleQuantity quantity; 1313 1314 /** 1315 * The amount of medication expressed as a timing amount. 1316 */ 1317 @Child(name = "daysSupply", type = { 1318 SimpleQuantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1319 @Description(shortDefinition = "Days Supply", formalDefinition = "The amount of medication expressed as a timing amount.") 1320 protected SimpleQuantity daysSupply; 1321 1322 /** 1323 * Identifies the medication being administered. This is either a link to a 1324 * resource representing the details of the medication or a simple attribute 1325 * carrying a code that identifies the medication from a known list of 1326 * medications. 1327 */ 1328 @Child(name = "medication", type = { CodeableConcept.class, 1329 Medication.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 1330 @Description(shortDefinition = "What medication was supplied", formalDefinition = "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 1331 protected Type medication; 1332 1333 /** 1334 * The time when the dispensed product was packaged and reviewed. 1335 */ 1336 @Child(name = "whenPrepared", type = { 1337 DateTimeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1338 @Description(shortDefinition = "Dispense processing time", formalDefinition = "The time when the dispensed product was packaged and reviewed.") 1339 protected DateTimeType whenPrepared; 1340 1341 /** 1342 * The time the dispensed product was provided to the patient or their 1343 * representative. 1344 */ 1345 @Child(name = "whenHandedOver", type = { 1346 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1347 @Description(shortDefinition = "When product was given out", formalDefinition = "The time the dispensed product was provided to the patient or their representative.") 1348 protected DateTimeType whenHandedOver; 1349 1350 /** 1351 * Identification of the facility/location where the medication was shipped to, 1352 * as part of the dispense event. 1353 */ 1354 @Child(name = "destination", type = { 1355 Location.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1356 @Description(shortDefinition = "Where the medication was sent", formalDefinition = "Identification of the facility/location where the medication was shipped to, as part of the dispense event.") 1357 protected Reference destination; 1358 1359 /** 1360 * The actual object that is the target of the reference (Identification of the 1361 * facility/location where the medication was shipped to, as part of the 1362 * dispense event.) 1363 */ 1364 protected Location destinationTarget; 1365 1366 /** 1367 * Identifies the person who picked up the medication. This will usually be a 1368 * patient or their caregiver, but some cases exist where it can be a healthcare 1369 * professional. 1370 */ 1371 @Child(name = "receiver", type = { Patient.class, 1372 Practitioner.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1373 @Description(shortDefinition = "Who collected the medication", formalDefinition = "Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.") 1374 protected List<Reference> receiver; 1375 /** 1376 * The actual objects that are the target of the reference (Identifies the 1377 * person who picked up the medication. This will usually be a patient or their 1378 * caregiver, but some cases exist where it can be a healthcare professional.) 1379 */ 1380 protected List<Resource> receiverTarget; 1381 1382 /** 1383 * Extra information about the dispense that could not be conveyed in the other 1384 * attributes. 1385 */ 1386 @Child(name = "note", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1387 @Description(shortDefinition = "Information about the dispense", formalDefinition = "Extra information about the dispense that could not be conveyed in the other attributes.") 1388 protected StringType note; 1389 1390 /** 1391 * Indicates how the medication is to be used by the patient. 1392 */ 1393 @Child(name = "dosageInstruction", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1394 @Description(shortDefinition = "Medicine administration instructions to the patient/caregiver", formalDefinition = "Indicates how the medication is to be used by the patient.") 1395 protected List<MedicationDispenseDosageInstructionComponent> dosageInstruction; 1396 1397 /** 1398 * Indicates whether or not substitution was made as part of the dispense. In 1399 * some cases substitution will be expected but does not happen, in other cases 1400 * substitution is not expected but does happen. This block explains what 1401 * substitution did or did not happen and why. 1402 */ 1403 @Child(name = "substitution", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 1404 @Description(shortDefinition = "Deals with substitution of one medicine for another", formalDefinition = "Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why.") 1405 protected MedicationDispenseSubstitutionComponent substitution; 1406 1407 private static final long serialVersionUID = -2071218407L; 1408 1409 /* 1410 * Constructor 1411 */ 1412 public MedicationDispense() { 1413 super(); 1414 } 1415 1416 /* 1417 * Constructor 1418 */ 1419 public MedicationDispense(Type medication) { 1420 super(); 1421 this.medication = medication; 1422 } 1423 1424 /** 1425 * @return {@link #identifier} (Identifier assigned by the dispensing facility - 1426 * this is an identifier assigned outside FHIR.) 1427 */ 1428 public Identifier getIdentifier() { 1429 if (this.identifier == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create MedicationDispense.identifier"); 1432 else if (Configuration.doAutoCreate()) 1433 this.identifier = new Identifier(); // cc 1434 return this.identifier; 1435 } 1436 1437 public boolean hasIdentifier() { 1438 return this.identifier != null && !this.identifier.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #identifier} (Identifier assigned by the dispensing 1443 * facility - this is an identifier assigned outside FHIR.) 1444 */ 1445 public MedicationDispense setIdentifier(Identifier value) { 1446 this.identifier = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return {@link #status} (A code specifying the state of the set of dispense 1452 * events.). This is the underlying object with id, value and 1453 * extensions. The accessor "getStatus" gives direct access to the value 1454 */ 1455 public Enumeration<MedicationDispenseStatus> getStatusElement() { 1456 if (this.status == null) 1457 if (Configuration.errorOnAutoCreate()) 1458 throw new Error("Attempt to auto-create MedicationDispense.status"); 1459 else if (Configuration.doAutoCreate()) 1460 this.status = new Enumeration<MedicationDispenseStatus>(new MedicationDispenseStatusEnumFactory()); // bb 1461 return this.status; 1462 } 1463 1464 public boolean hasStatusElement() { 1465 return this.status != null && !this.status.isEmpty(); 1466 } 1467 1468 public boolean hasStatus() { 1469 return this.status != null && !this.status.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #status} (A code specifying the state of the set of 1474 * dispense events.). This is the underlying object with id, value 1475 * and extensions. The accessor "getStatus" gives direct access to 1476 * the value 1477 */ 1478 public MedicationDispense setStatusElement(Enumeration<MedicationDispenseStatus> value) { 1479 this.status = value; 1480 return this; 1481 } 1482 1483 /** 1484 * @return A code specifying the state of the set of dispense events. 1485 */ 1486 public MedicationDispenseStatus getStatus() { 1487 return this.status == null ? null : this.status.getValue(); 1488 } 1489 1490 /** 1491 * @param value A code specifying the state of the set of dispense events. 1492 */ 1493 public MedicationDispense setStatus(MedicationDispenseStatus value) { 1494 if (value == null) 1495 this.status = null; 1496 else { 1497 if (this.status == null) 1498 this.status = new Enumeration<MedicationDispenseStatus>(new MedicationDispenseStatusEnumFactory()); 1499 this.status.setValue(value); 1500 } 1501 return this; 1502 } 1503 1504 /** 1505 * @return {@link #patient} (A link to a resource representing the person to 1506 * whom the medication will be given.) 1507 */ 1508 public Reference getPatient() { 1509 if (this.patient == null) 1510 if (Configuration.errorOnAutoCreate()) 1511 throw new Error("Attempt to auto-create MedicationDispense.patient"); 1512 else if (Configuration.doAutoCreate()) 1513 this.patient = new Reference(); // cc 1514 return this.patient; 1515 } 1516 1517 public boolean hasPatient() { 1518 return this.patient != null && !this.patient.isEmpty(); 1519 } 1520 1521 /** 1522 * @param value {@link #patient} (A link to a resource representing the person 1523 * to whom the medication will be given.) 1524 */ 1525 public MedicationDispense setPatient(Reference value) { 1526 this.patient = value; 1527 return this; 1528 } 1529 1530 /** 1531 * @return {@link #patient} The actual object that is the target of the 1532 * reference. The reference library doesn't populate this, but you can 1533 * use it to hold the resource if you resolve it. (A link to a resource 1534 * representing the person to whom the medication will be given.) 1535 */ 1536 public Patient getPatientTarget() { 1537 if (this.patientTarget == null) 1538 if (Configuration.errorOnAutoCreate()) 1539 throw new Error("Attempt to auto-create MedicationDispense.patient"); 1540 else if (Configuration.doAutoCreate()) 1541 this.patientTarget = new Patient(); // aa 1542 return this.patientTarget; 1543 } 1544 1545 /** 1546 * @param value {@link #patient} The actual object that is the target of the 1547 * reference. The reference library doesn't use these, but you can 1548 * use it to hold the resource if you resolve it. (A link to a 1549 * resource representing the person to whom the medication will be 1550 * given.) 1551 */ 1552 public MedicationDispense setPatientTarget(Patient value) { 1553 this.patientTarget = value; 1554 return this; 1555 } 1556 1557 /** 1558 * @return {@link #dispenser} (The individual responsible for dispensing the 1559 * medication.) 1560 */ 1561 public Reference getDispenser() { 1562 if (this.dispenser == null) 1563 if (Configuration.errorOnAutoCreate()) 1564 throw new Error("Attempt to auto-create MedicationDispense.dispenser"); 1565 else if (Configuration.doAutoCreate()) 1566 this.dispenser = new Reference(); // cc 1567 return this.dispenser; 1568 } 1569 1570 public boolean hasDispenser() { 1571 return this.dispenser != null && !this.dispenser.isEmpty(); 1572 } 1573 1574 /** 1575 * @param value {@link #dispenser} (The individual responsible for dispensing 1576 * the medication.) 1577 */ 1578 public MedicationDispense setDispenser(Reference value) { 1579 this.dispenser = value; 1580 return this; 1581 } 1582 1583 /** 1584 * @return {@link #dispenser} The actual object that is the target of the 1585 * reference. The reference library doesn't populate this, but you can 1586 * use it to hold the resource if you resolve it. (The individual 1587 * responsible for dispensing the medication.) 1588 */ 1589 public Practitioner getDispenserTarget() { 1590 if (this.dispenserTarget == null) 1591 if (Configuration.errorOnAutoCreate()) 1592 throw new Error("Attempt to auto-create MedicationDispense.dispenser"); 1593 else if (Configuration.doAutoCreate()) 1594 this.dispenserTarget = new Practitioner(); // aa 1595 return this.dispenserTarget; 1596 } 1597 1598 /** 1599 * @param value {@link #dispenser} The actual object that is the target of the 1600 * reference. The reference library doesn't use these, but you can 1601 * use it to hold the resource if you resolve it. (The individual 1602 * responsible for dispensing the medication.) 1603 */ 1604 public MedicationDispense setDispenserTarget(Practitioner value) { 1605 this.dispenserTarget = value; 1606 return this; 1607 } 1608 1609 /** 1610 * @return {@link #authorizingPrescription} (Indicates the medication order that 1611 * is being dispensed against.) 1612 */ 1613 public List<Reference> getAuthorizingPrescription() { 1614 if (this.authorizingPrescription == null) 1615 this.authorizingPrescription = new ArrayList<Reference>(); 1616 return this.authorizingPrescription; 1617 } 1618 1619 public boolean hasAuthorizingPrescription() { 1620 if (this.authorizingPrescription == null) 1621 return false; 1622 for (Reference item : this.authorizingPrescription) 1623 if (!item.isEmpty()) 1624 return true; 1625 return false; 1626 } 1627 1628 /** 1629 * @return {@link #authorizingPrescription} (Indicates the medication order that 1630 * is being dispensed against.) 1631 */ 1632 // syntactic sugar 1633 public Reference addAuthorizingPrescription() { // 3 1634 Reference t = new Reference(); 1635 if (this.authorizingPrescription == null) 1636 this.authorizingPrescription = new ArrayList<Reference>(); 1637 this.authorizingPrescription.add(t); 1638 return t; 1639 } 1640 1641 // syntactic sugar 1642 public MedicationDispense addAuthorizingPrescription(Reference t) { // 3 1643 if (t == null) 1644 return this; 1645 if (this.authorizingPrescription == null) 1646 this.authorizingPrescription = new ArrayList<Reference>(); 1647 this.authorizingPrescription.add(t); 1648 return this; 1649 } 1650 1651 /** 1652 * @return {@link #authorizingPrescription} (The actual objects that are the 1653 * target of the reference. The reference library doesn't populate this, 1654 * but you can use this to hold the resources if you resolvethemt. 1655 * Indicates the medication order that is being dispensed against.) 1656 */ 1657 public List<MedicationOrder> getAuthorizingPrescriptionTarget() { 1658 if (this.authorizingPrescriptionTarget == null) 1659 this.authorizingPrescriptionTarget = new ArrayList<MedicationOrder>(); 1660 return this.authorizingPrescriptionTarget; 1661 } 1662 1663 // syntactic sugar 1664 /** 1665 * @return {@link #authorizingPrescription} (Add an actual object that is the 1666 * target of the reference. The reference library doesn't use these, but 1667 * you can use this to hold the resources if you resolvethemt. Indicates 1668 * the medication order that is being dispensed against.) 1669 */ 1670 public MedicationOrder addAuthorizingPrescriptionTarget() { 1671 MedicationOrder r = new MedicationOrder(); 1672 if (this.authorizingPrescriptionTarget == null) 1673 this.authorizingPrescriptionTarget = new ArrayList<MedicationOrder>(); 1674 this.authorizingPrescriptionTarget.add(r); 1675 return r; 1676 } 1677 1678 /** 1679 * @return {@link #type} (Indicates the type of dispensing event that is 1680 * performed. For example, Trial Fill, Completion of Trial, Partial 1681 * Fill, Emergency Fill, Samples, etc.) 1682 */ 1683 public CodeableConcept getType() { 1684 if (this.type == null) 1685 if (Configuration.errorOnAutoCreate()) 1686 throw new Error("Attempt to auto-create MedicationDispense.type"); 1687 else if (Configuration.doAutoCreate()) 1688 this.type = new CodeableConcept(); // cc 1689 return this.type; 1690 } 1691 1692 public boolean hasType() { 1693 return this.type != null && !this.type.isEmpty(); 1694 } 1695 1696 /** 1697 * @param value {@link #type} (Indicates the type of dispensing event that is 1698 * performed. For example, Trial Fill, Completion of Trial, Partial 1699 * Fill, Emergency Fill, Samples, etc.) 1700 */ 1701 public MedicationDispense setType(CodeableConcept value) { 1702 this.type = value; 1703 return this; 1704 } 1705 1706 /** 1707 * @return {@link #quantity} (The amount of medication that has been dispensed. 1708 * Includes unit of measure.) 1709 */ 1710 public SimpleQuantity getQuantity() { 1711 if (this.quantity == null) 1712 if (Configuration.errorOnAutoCreate()) 1713 throw new Error("Attempt to auto-create MedicationDispense.quantity"); 1714 else if (Configuration.doAutoCreate()) 1715 this.quantity = new SimpleQuantity(); // cc 1716 return this.quantity; 1717 } 1718 1719 public boolean hasQuantity() { 1720 return this.quantity != null && !this.quantity.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #quantity} (The amount of medication that has been 1725 * dispensed. Includes unit of measure.) 1726 */ 1727 public MedicationDispense setQuantity(SimpleQuantity value) { 1728 this.quantity = value; 1729 return this; 1730 } 1731 1732 /** 1733 * @return {@link #daysSupply} (The amount of medication expressed as a timing 1734 * amount.) 1735 */ 1736 public SimpleQuantity getDaysSupply() { 1737 if (this.daysSupply == null) 1738 if (Configuration.errorOnAutoCreate()) 1739 throw new Error("Attempt to auto-create MedicationDispense.daysSupply"); 1740 else if (Configuration.doAutoCreate()) 1741 this.daysSupply = new SimpleQuantity(); // cc 1742 return this.daysSupply; 1743 } 1744 1745 public boolean hasDaysSupply() { 1746 return this.daysSupply != null && !this.daysSupply.isEmpty(); 1747 } 1748 1749 /** 1750 * @param value {@link #daysSupply} (The amount of medication expressed as a 1751 * timing amount.) 1752 */ 1753 public MedicationDispense setDaysSupply(SimpleQuantity value) { 1754 this.daysSupply = value; 1755 return this; 1756 } 1757 1758 /** 1759 * @return {@link #medication} (Identifies the medication being administered. 1760 * This is either a link to a resource representing the details of the 1761 * medication or a simple attribute carrying a code that identifies the 1762 * medication from a known list of medications.) 1763 */ 1764 public Type getMedication() { 1765 return this.medication; 1766 } 1767 1768 /** 1769 * @return {@link #medication} (Identifies the medication being administered. 1770 * This is either a link to a resource representing the details of the 1771 * medication or a simple attribute carrying a code that identifies the 1772 * medication from a known list of medications.) 1773 */ 1774 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1775 if (!(this.medication instanceof CodeableConcept)) 1776 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1777 + this.medication.getClass().getName() + " was encountered"); 1778 return (CodeableConcept) this.medication; 1779 } 1780 1781 public boolean hasMedicationCodeableConcept() { 1782 return this.medication instanceof CodeableConcept; 1783 } 1784 1785 /** 1786 * @return {@link #medication} (Identifies the medication being administered. 1787 * This is either a link to a resource representing the details of the 1788 * medication or a simple attribute carrying a code that identifies the 1789 * medication from a known list of medications.) 1790 */ 1791 public Reference getMedicationReference() throws FHIRException { 1792 if (!(this.medication instanceof Reference)) 1793 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1794 + this.medication.getClass().getName() + " was encountered"); 1795 return (Reference) this.medication; 1796 } 1797 1798 public boolean hasMedicationReference() { 1799 return this.medication instanceof Reference; 1800 } 1801 1802 public boolean hasMedication() { 1803 return this.medication != null && !this.medication.isEmpty(); 1804 } 1805 1806 /** 1807 * @param value {@link #medication} (Identifies the medication being 1808 * administered. This is either a link to a resource representing 1809 * the details of the medication or a simple attribute carrying a 1810 * code that identifies the medication from a known list of 1811 * medications.) 1812 */ 1813 public MedicationDispense setMedication(Type value) { 1814 this.medication = value; 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #whenPrepared} (The time when the dispensed product was 1820 * packaged and reviewed.). This is the underlying object with id, value 1821 * and extensions. The accessor "getWhenPrepared" gives direct access to 1822 * the value 1823 */ 1824 public DateTimeType getWhenPreparedElement() { 1825 if (this.whenPrepared == null) 1826 if (Configuration.errorOnAutoCreate()) 1827 throw new Error("Attempt to auto-create MedicationDispense.whenPrepared"); 1828 else if (Configuration.doAutoCreate()) 1829 this.whenPrepared = new DateTimeType(); // bb 1830 return this.whenPrepared; 1831 } 1832 1833 public boolean hasWhenPreparedElement() { 1834 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 1835 } 1836 1837 public boolean hasWhenPrepared() { 1838 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 1839 } 1840 1841 /** 1842 * @param value {@link #whenPrepared} (The time when the dispensed product was 1843 * packaged and reviewed.). This is the underlying object with id, 1844 * value and extensions. The accessor "getWhenPrepared" gives 1845 * direct access to the value 1846 */ 1847 public MedicationDispense setWhenPreparedElement(DateTimeType value) { 1848 this.whenPrepared = value; 1849 return this; 1850 } 1851 1852 /** 1853 * @return The time when the dispensed product was packaged and reviewed. 1854 */ 1855 public Date getWhenPrepared() { 1856 return this.whenPrepared == null ? null : this.whenPrepared.getValue(); 1857 } 1858 1859 /** 1860 * @param value The time when the dispensed product was packaged and reviewed. 1861 */ 1862 public MedicationDispense setWhenPrepared(Date value) { 1863 if (value == null) 1864 this.whenPrepared = null; 1865 else { 1866 if (this.whenPrepared == null) 1867 this.whenPrepared = new DateTimeType(); 1868 this.whenPrepared.setValue(value); 1869 } 1870 return this; 1871 } 1872 1873 /** 1874 * @return {@link #whenHandedOver} (The time the dispensed product was provided 1875 * to the patient or their representative.). This is the underlying 1876 * object with id, value and extensions. The accessor 1877 * "getWhenHandedOver" gives direct access to the value 1878 */ 1879 public DateTimeType getWhenHandedOverElement() { 1880 if (this.whenHandedOver == null) 1881 if (Configuration.errorOnAutoCreate()) 1882 throw new Error("Attempt to auto-create MedicationDispense.whenHandedOver"); 1883 else if (Configuration.doAutoCreate()) 1884 this.whenHandedOver = new DateTimeType(); // bb 1885 return this.whenHandedOver; 1886 } 1887 1888 public boolean hasWhenHandedOverElement() { 1889 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1890 } 1891 1892 public boolean hasWhenHandedOver() { 1893 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1894 } 1895 1896 /** 1897 * @param value {@link #whenHandedOver} (The time the dispensed product was 1898 * provided to the patient or their representative.). This is the 1899 * underlying object with id, value and extensions. The accessor 1900 * "getWhenHandedOver" gives direct access to the value 1901 */ 1902 public MedicationDispense setWhenHandedOverElement(DateTimeType value) { 1903 this.whenHandedOver = value; 1904 return this; 1905 } 1906 1907 /** 1908 * @return The time the dispensed product was provided to the patient or their 1909 * representative. 1910 */ 1911 public Date getWhenHandedOver() { 1912 return this.whenHandedOver == null ? null : this.whenHandedOver.getValue(); 1913 } 1914 1915 /** 1916 * @param value The time the dispensed product was provided to the patient or 1917 * their representative. 1918 */ 1919 public MedicationDispense setWhenHandedOver(Date value) { 1920 if (value == null) 1921 this.whenHandedOver = null; 1922 else { 1923 if (this.whenHandedOver == null) 1924 this.whenHandedOver = new DateTimeType(); 1925 this.whenHandedOver.setValue(value); 1926 } 1927 return this; 1928 } 1929 1930 /** 1931 * @return {@link #destination} (Identification of the facility/location where 1932 * the medication was shipped to, as part of the dispense event.) 1933 */ 1934 public Reference getDestination() { 1935 if (this.destination == null) 1936 if (Configuration.errorOnAutoCreate()) 1937 throw new Error("Attempt to auto-create MedicationDispense.destination"); 1938 else if (Configuration.doAutoCreate()) 1939 this.destination = new Reference(); // cc 1940 return this.destination; 1941 } 1942 1943 public boolean hasDestination() { 1944 return this.destination != null && !this.destination.isEmpty(); 1945 } 1946 1947 /** 1948 * @param value {@link #destination} (Identification of the facility/location 1949 * where the medication was shipped to, as part of the dispense 1950 * event.) 1951 */ 1952 public MedicationDispense setDestination(Reference value) { 1953 this.destination = value; 1954 return this; 1955 } 1956 1957 /** 1958 * @return {@link #destination} The actual object that is the target of the 1959 * reference. The reference library doesn't populate this, but you can 1960 * use it to hold the resource if you resolve it. (Identification of the 1961 * facility/location where the medication was shipped to, as part of the 1962 * dispense event.) 1963 */ 1964 public Location getDestinationTarget() { 1965 if (this.destinationTarget == null) 1966 if (Configuration.errorOnAutoCreate()) 1967 throw new Error("Attempt to auto-create MedicationDispense.destination"); 1968 else if (Configuration.doAutoCreate()) 1969 this.destinationTarget = new Location(); // aa 1970 return this.destinationTarget; 1971 } 1972 1973 /** 1974 * @param value {@link #destination} The actual object that is the target of the 1975 * reference. The reference library doesn't use these, but you can 1976 * use it to hold the resource if you resolve it. (Identification 1977 * of the facility/location where the medication was shipped to, as 1978 * part of the dispense event.) 1979 */ 1980 public MedicationDispense setDestinationTarget(Location value) { 1981 this.destinationTarget = value; 1982 return this; 1983 } 1984 1985 /** 1986 * @return {@link #receiver} (Identifies the person who picked up the 1987 * medication. This will usually be a patient or their caregiver, but 1988 * some cases exist where it can be a healthcare professional.) 1989 */ 1990 public List<Reference> getReceiver() { 1991 if (this.receiver == null) 1992 this.receiver = new ArrayList<Reference>(); 1993 return this.receiver; 1994 } 1995 1996 public boolean hasReceiver() { 1997 if (this.receiver == null) 1998 return false; 1999 for (Reference item : this.receiver) 2000 if (!item.isEmpty()) 2001 return true; 2002 return false; 2003 } 2004 2005 /** 2006 * @return {@link #receiver} (Identifies the person who picked up the 2007 * medication. This will usually be a patient or their caregiver, but 2008 * some cases exist where it can be a healthcare professional.) 2009 */ 2010 // syntactic sugar 2011 public Reference addReceiver() { // 3 2012 Reference t = new Reference(); 2013 if (this.receiver == null) 2014 this.receiver = new ArrayList<Reference>(); 2015 this.receiver.add(t); 2016 return t; 2017 } 2018 2019 // syntactic sugar 2020 public MedicationDispense addReceiver(Reference t) { // 3 2021 if (t == null) 2022 return this; 2023 if (this.receiver == null) 2024 this.receiver = new ArrayList<Reference>(); 2025 this.receiver.add(t); 2026 return this; 2027 } 2028 2029 /** 2030 * @return {@link #receiver} (The actual objects that are the target of the 2031 * reference. The reference library doesn't populate this, but you can 2032 * use this to hold the resources if you resolvethemt. Identifies the 2033 * person who picked up the medication. This will usually be a patient 2034 * or their caregiver, but some cases exist where it can be a healthcare 2035 * professional.) 2036 */ 2037 public List<Resource> getReceiverTarget() { 2038 if (this.receiverTarget == null) 2039 this.receiverTarget = new ArrayList<Resource>(); 2040 return this.receiverTarget; 2041 } 2042 2043 /** 2044 * @return {@link #note} (Extra information about the dispense that could not be 2045 * conveyed in the other attributes.). This is the underlying object 2046 * with id, value and extensions. The accessor "getNote" gives direct 2047 * access to the value 2048 */ 2049 public StringType getNoteElement() { 2050 if (this.note == null) 2051 if (Configuration.errorOnAutoCreate()) 2052 throw new Error("Attempt to auto-create MedicationDispense.note"); 2053 else if (Configuration.doAutoCreate()) 2054 this.note = new StringType(); // bb 2055 return this.note; 2056 } 2057 2058 public boolean hasNoteElement() { 2059 return this.note != null && !this.note.isEmpty(); 2060 } 2061 2062 public boolean hasNote() { 2063 return this.note != null && !this.note.isEmpty(); 2064 } 2065 2066 /** 2067 * @param value {@link #note} (Extra information about the dispense that could 2068 * not be conveyed in the other attributes.). This is the 2069 * underlying object with id, value and extensions. The accessor 2070 * "getNote" gives direct access to the value 2071 */ 2072 public MedicationDispense setNoteElement(StringType value) { 2073 this.note = value; 2074 return this; 2075 } 2076 2077 /** 2078 * @return Extra information about the dispense that could not be conveyed in 2079 * the other attributes. 2080 */ 2081 public String getNote() { 2082 return this.note == null ? null : this.note.getValue(); 2083 } 2084 2085 /** 2086 * @param value Extra information about the dispense that could not be conveyed 2087 * in the other attributes. 2088 */ 2089 public MedicationDispense setNote(String value) { 2090 if (Utilities.noString(value)) 2091 this.note = null; 2092 else { 2093 if (this.note == null) 2094 this.note = new StringType(); 2095 this.note.setValue(value); 2096 } 2097 return this; 2098 } 2099 2100 /** 2101 * @return {@link #dosageInstruction} (Indicates how the medication is to be 2102 * used by the patient.) 2103 */ 2104 public List<MedicationDispenseDosageInstructionComponent> getDosageInstruction() { 2105 if (this.dosageInstruction == null) 2106 this.dosageInstruction = new ArrayList<MedicationDispenseDosageInstructionComponent>(); 2107 return this.dosageInstruction; 2108 } 2109 2110 public boolean hasDosageInstruction() { 2111 if (this.dosageInstruction == null) 2112 return false; 2113 for (MedicationDispenseDosageInstructionComponent item : this.dosageInstruction) 2114 if (!item.isEmpty()) 2115 return true; 2116 return false; 2117 } 2118 2119 /** 2120 * @return {@link #dosageInstruction} (Indicates how the medication is to be 2121 * used by the patient.) 2122 */ 2123 // syntactic sugar 2124 public MedicationDispenseDosageInstructionComponent addDosageInstruction() { // 3 2125 MedicationDispenseDosageInstructionComponent t = new MedicationDispenseDosageInstructionComponent(); 2126 if (this.dosageInstruction == null) 2127 this.dosageInstruction = new ArrayList<MedicationDispenseDosageInstructionComponent>(); 2128 this.dosageInstruction.add(t); 2129 return t; 2130 } 2131 2132 // syntactic sugar 2133 public MedicationDispense addDosageInstruction(MedicationDispenseDosageInstructionComponent t) { // 3 2134 if (t == null) 2135 return this; 2136 if (this.dosageInstruction == null) 2137 this.dosageInstruction = new ArrayList<MedicationDispenseDosageInstructionComponent>(); 2138 this.dosageInstruction.add(t); 2139 return this; 2140 } 2141 2142 /** 2143 * @return {@link #substitution} (Indicates whether or not substitution was made 2144 * as part of the dispense. In some cases substitution will be expected 2145 * but does not happen, in other cases substitution is not expected but 2146 * does happen. This block explains what substitution did or did not 2147 * happen and why.) 2148 */ 2149 public MedicationDispenseSubstitutionComponent getSubstitution() { 2150 if (this.substitution == null) 2151 if (Configuration.errorOnAutoCreate()) 2152 throw new Error("Attempt to auto-create MedicationDispense.substitution"); 2153 else if (Configuration.doAutoCreate()) 2154 this.substitution = new MedicationDispenseSubstitutionComponent(); // cc 2155 return this.substitution; 2156 } 2157 2158 public boolean hasSubstitution() { 2159 return this.substitution != null && !this.substitution.isEmpty(); 2160 } 2161 2162 /** 2163 * @param value {@link #substitution} (Indicates whether or not substitution was 2164 * made as part of the dispense. In some cases substitution will be 2165 * expected but does not happen, in other cases substitution is not 2166 * expected but does happen. This block explains what substitution 2167 * did or did not happen and why.) 2168 */ 2169 public MedicationDispense setSubstitution(MedicationDispenseSubstitutionComponent value) { 2170 this.substitution = value; 2171 return this; 2172 } 2173 2174 protected void listChildren(List<Property> childrenList) { 2175 super.listChildren(childrenList); 2176 childrenList.add(new Property("identifier", "Identifier", 2177 "Identifier assigned by the dispensing facility - this is an identifier assigned outside FHIR.", 0, 2178 java.lang.Integer.MAX_VALUE, identifier)); 2179 childrenList.add(new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 2180 java.lang.Integer.MAX_VALUE, status)); 2181 childrenList.add(new Property("patient", "Reference(Patient)", 2182 "A link to a resource representing the person to whom the medication will be given.", 0, 2183 java.lang.Integer.MAX_VALUE, patient)); 2184 childrenList.add(new Property("dispenser", "Reference(Practitioner)", 2185 "The individual responsible for dispensing the medication.", 0, java.lang.Integer.MAX_VALUE, dispenser)); 2186 childrenList.add(new Property("authorizingPrescription", "Reference(MedicationOrder)", 2187 "Indicates the medication order that is being dispensed against.", 0, java.lang.Integer.MAX_VALUE, 2188 authorizingPrescription)); 2189 childrenList.add(new Property("type", "CodeableConcept", 2190 "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 2191 0, java.lang.Integer.MAX_VALUE, type)); 2192 childrenList.add(new Property("quantity", "SimpleQuantity", 2193 "The amount of medication that has been dispensed. Includes unit of measure.", 0, java.lang.Integer.MAX_VALUE, 2194 quantity)); 2195 childrenList.add(new Property("daysSupply", "SimpleQuantity", 2196 "The amount of medication expressed as a timing amount.", 0, java.lang.Integer.MAX_VALUE, daysSupply)); 2197 childrenList.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2198 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2199 0, java.lang.Integer.MAX_VALUE, medication)); 2200 childrenList 2201 .add(new Property("whenPrepared", "dateTime", "The time when the dispensed product was packaged and reviewed.", 2202 0, java.lang.Integer.MAX_VALUE, whenPrepared)); 2203 childrenList.add(new Property("whenHandedOver", "dateTime", 2204 "The time the dispensed product was provided to the patient or their representative.", 0, 2205 java.lang.Integer.MAX_VALUE, whenHandedOver)); 2206 childrenList.add(new Property("destination", "Reference(Location)", 2207 "Identification of the facility/location where the medication was shipped to, as part of the dispense event.", 2208 0, java.lang.Integer.MAX_VALUE, destination)); 2209 childrenList.add(new Property("receiver", "Reference(Patient|Practitioner)", 2210 "Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.", 2211 0, java.lang.Integer.MAX_VALUE, receiver)); 2212 childrenList.add(new Property("note", "string", 2213 "Extra information about the dispense that could not be conveyed in the other attributes.", 0, 2214 java.lang.Integer.MAX_VALUE, note)); 2215 childrenList.add(new Property("dosageInstruction", "", "Indicates how the medication is to be used by the patient.", 2216 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 2217 childrenList.add(new Property("substitution", "", 2218 "Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why.", 2219 0, java.lang.Integer.MAX_VALUE, substitution)); 2220 } 2221 2222 @Override 2223 public void setProperty(String name, Base value) throws FHIRException { 2224 if (name.equals("identifier")) 2225 this.identifier = castToIdentifier(value); // Identifier 2226 else if (name.equals("status")) 2227 this.status = new MedicationDispenseStatusEnumFactory().fromType(value); // Enumeration<MedicationDispenseStatus> 2228 else if (name.equals("patient")) 2229 this.patient = castToReference(value); // Reference 2230 else if (name.equals("dispenser")) 2231 this.dispenser = castToReference(value); // Reference 2232 else if (name.equals("authorizingPrescription")) 2233 this.getAuthorizingPrescription().add(castToReference(value)); 2234 else if (name.equals("type")) 2235 this.type = castToCodeableConcept(value); // CodeableConcept 2236 else if (name.equals("quantity")) 2237 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2238 else if (name.equals("daysSupply")) 2239 this.daysSupply = castToSimpleQuantity(value); // SimpleQuantity 2240 else if (name.equals("medication[x]")) 2241 this.medication = (Type) value; // Type 2242 else if (name.equals("whenPrepared")) 2243 this.whenPrepared = castToDateTime(value); // DateTimeType 2244 else if (name.equals("whenHandedOver")) 2245 this.whenHandedOver = castToDateTime(value); // DateTimeType 2246 else if (name.equals("destination")) 2247 this.destination = castToReference(value); // Reference 2248 else if (name.equals("receiver")) 2249 this.getReceiver().add(castToReference(value)); 2250 else if (name.equals("note")) 2251 this.note = castToString(value); // StringType 2252 else if (name.equals("dosageInstruction")) 2253 this.getDosageInstruction().add((MedicationDispenseDosageInstructionComponent) value); 2254 else if (name.equals("substitution")) 2255 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 2256 else 2257 super.setProperty(name, value); 2258 } 2259 2260 @Override 2261 public Base addChild(String name) throws FHIRException { 2262 if (name.equals("identifier")) { 2263 this.identifier = new Identifier(); 2264 return this.identifier; 2265 } else if (name.equals("status")) { 2266 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.status"); 2267 } else if (name.equals("patient")) { 2268 this.patient = new Reference(); 2269 return this.patient; 2270 } else if (name.equals("dispenser")) { 2271 this.dispenser = new Reference(); 2272 return this.dispenser; 2273 } else if (name.equals("authorizingPrescription")) { 2274 return addAuthorizingPrescription(); 2275 } else if (name.equals("type")) { 2276 this.type = new CodeableConcept(); 2277 return this.type; 2278 } else if (name.equals("quantity")) { 2279 this.quantity = new SimpleQuantity(); 2280 return this.quantity; 2281 } else if (name.equals("daysSupply")) { 2282 this.daysSupply = new SimpleQuantity(); 2283 return this.daysSupply; 2284 } else if (name.equals("medicationCodeableConcept")) { 2285 this.medication = new CodeableConcept(); 2286 return this.medication; 2287 } else if (name.equals("medicationReference")) { 2288 this.medication = new Reference(); 2289 return this.medication; 2290 } else if (name.equals("whenPrepared")) { 2291 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenPrepared"); 2292 } else if (name.equals("whenHandedOver")) { 2293 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenHandedOver"); 2294 } else if (name.equals("destination")) { 2295 this.destination = new Reference(); 2296 return this.destination; 2297 } else if (name.equals("receiver")) { 2298 return addReceiver(); 2299 } else if (name.equals("note")) { 2300 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.note"); 2301 } else if (name.equals("dosageInstruction")) { 2302 return addDosageInstruction(); 2303 } else if (name.equals("substitution")) { 2304 this.substitution = new MedicationDispenseSubstitutionComponent(); 2305 return this.substitution; 2306 } else 2307 return super.addChild(name); 2308 } 2309 2310 public String fhirType() { 2311 return "MedicationDispense"; 2312 2313 } 2314 2315 public MedicationDispense copy() { 2316 MedicationDispense dst = new MedicationDispense(); 2317 copyValues(dst); 2318 dst.identifier = identifier == null ? null : identifier.copy(); 2319 dst.status = status == null ? null : status.copy(); 2320 dst.patient = patient == null ? null : patient.copy(); 2321 dst.dispenser = dispenser == null ? null : dispenser.copy(); 2322 if (authorizingPrescription != null) { 2323 dst.authorizingPrescription = new ArrayList<Reference>(); 2324 for (Reference i : authorizingPrescription) 2325 dst.authorizingPrescription.add(i.copy()); 2326 } 2327 ; 2328 dst.type = type == null ? null : type.copy(); 2329 dst.quantity = quantity == null ? null : quantity.copy(); 2330 dst.daysSupply = daysSupply == null ? null : daysSupply.copy(); 2331 dst.medication = medication == null ? null : medication.copy(); 2332 dst.whenPrepared = whenPrepared == null ? null : whenPrepared.copy(); 2333 dst.whenHandedOver = whenHandedOver == null ? null : whenHandedOver.copy(); 2334 dst.destination = destination == null ? null : destination.copy(); 2335 if (receiver != null) { 2336 dst.receiver = new ArrayList<Reference>(); 2337 for (Reference i : receiver) 2338 dst.receiver.add(i.copy()); 2339 } 2340 ; 2341 dst.note = note == null ? null : note.copy(); 2342 if (dosageInstruction != null) { 2343 dst.dosageInstruction = new ArrayList<MedicationDispenseDosageInstructionComponent>(); 2344 for (MedicationDispenseDosageInstructionComponent i : dosageInstruction) 2345 dst.dosageInstruction.add(i.copy()); 2346 } 2347 ; 2348 dst.substitution = substitution == null ? null : substitution.copy(); 2349 return dst; 2350 } 2351 2352 protected MedicationDispense typedCopy() { 2353 return copy(); 2354 } 2355 2356 @Override 2357 public boolean equalsDeep(Base other) { 2358 if (!super.equalsDeep(other)) 2359 return false; 2360 if (!(other instanceof MedicationDispense)) 2361 return false; 2362 MedicationDispense o = (MedicationDispense) other; 2363 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2364 && compareDeep(patient, o.patient, true) && compareDeep(dispenser, o.dispenser, true) 2365 && compareDeep(authorizingPrescription, o.authorizingPrescription, true) && compareDeep(type, o.type, true) 2366 && compareDeep(quantity, o.quantity, true) && compareDeep(daysSupply, o.daysSupply, true) 2367 && compareDeep(medication, o.medication, true) && compareDeep(whenPrepared, o.whenPrepared, true) 2368 && compareDeep(whenHandedOver, o.whenHandedOver, true) && compareDeep(destination, o.destination, true) 2369 && compareDeep(receiver, o.receiver, true) && compareDeep(note, o.note, true) 2370 && compareDeep(dosageInstruction, o.dosageInstruction, true) && compareDeep(substitution, o.substitution, true); 2371 } 2372 2373 @Override 2374 public boolean equalsShallow(Base other) { 2375 if (!super.equalsShallow(other)) 2376 return false; 2377 if (!(other instanceof MedicationDispense)) 2378 return false; 2379 MedicationDispense o = (MedicationDispense) other; 2380 return compareValues(status, o.status, true) && compareValues(whenPrepared, o.whenPrepared, true) 2381 && compareValues(whenHandedOver, o.whenHandedOver, true) && compareValues(note, o.note, true); 2382 } 2383 2384 public boolean isEmpty() { 2385 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 2386 && (patient == null || patient.isEmpty()) && (dispenser == null || dispenser.isEmpty()) 2387 && (authorizingPrescription == null || authorizingPrescription.isEmpty()) && (type == null || type.isEmpty()) 2388 && (quantity == null || quantity.isEmpty()) && (daysSupply == null || daysSupply.isEmpty()) 2389 && (medication == null || medication.isEmpty()) && (whenPrepared == null || whenPrepared.isEmpty()) 2390 && (whenHandedOver == null || whenHandedOver.isEmpty()) && (destination == null || destination.isEmpty()) 2391 && (receiver == null || receiver.isEmpty()) && (note == null || note.isEmpty()) 2392 && (dosageInstruction == null || dosageInstruction.isEmpty()) 2393 && (substitution == null || substitution.isEmpty()); 2394 } 2395 2396 @Override 2397 public ResourceType getResourceType() { 2398 return ResourceType.MedicationDispense; 2399 } 2400 2401 @SearchParamDefinition(name = "identifier", path = "MedicationDispense.identifier", description = "Return dispenses with this external identifier", type = "token") 2402 public static final String SP_IDENTIFIER = "identifier"; 2403 @SearchParamDefinition(name = "code", path = "MedicationDispense.medicationCodeableConcept", description = "Return dispenses of this medicine code", type = "token") 2404 public static final String SP_CODE = "code"; 2405 @SearchParamDefinition(name = "receiver", path = "MedicationDispense.receiver", description = "Who collected the medication", type = "reference") 2406 public static final String SP_RECEIVER = "receiver"; 2407 @SearchParamDefinition(name = "destination", path = "MedicationDispense.destination", description = "Return dispenses that should be sent to a specific destination", type = "reference") 2408 public static final String SP_DESTINATION = "destination"; 2409 @SearchParamDefinition(name = "medication", path = "MedicationDispense.medicationReference", description = "Return dispenses of this medicine resource", type = "reference") 2410 public static final String SP_MEDICATION = "medication"; 2411 @SearchParamDefinition(name = "responsibleparty", path = "MedicationDispense.substitution.responsibleParty", description = "Return all dispenses with the specified responsible party", type = "reference") 2412 public static final String SP_RESPONSIBLEPARTY = "responsibleparty"; 2413 @SearchParamDefinition(name = "type", path = "MedicationDispense.type", description = "Return all dispenses of a specific type", type = "token") 2414 public static final String SP_TYPE = "type"; 2415 @SearchParamDefinition(name = "whenhandedover", path = "MedicationDispense.whenHandedOver", description = "Date when medication handed over to patient (outpatient setting), or supplied to ward or clinic (inpatient setting)", type = "date") 2416 public static final String SP_WHENHANDEDOVER = "whenhandedover"; 2417 @SearchParamDefinition(name = "whenprepared", path = "MedicationDispense.whenPrepared", description = "Date when medication prepared", type = "date") 2418 public static final String SP_WHENPREPARED = "whenprepared"; 2419 @SearchParamDefinition(name = "dispenser", path = "MedicationDispense.dispenser", description = "Return all dispenses performed by a specific individual", type = "reference") 2420 public static final String SP_DISPENSER = "dispenser"; 2421 @SearchParamDefinition(name = "prescription", path = "MedicationDispense.authorizingPrescription", description = "The identity of a prescription to list dispenses from", type = "reference") 2422 public static final String SP_PRESCRIPTION = "prescription"; 2423 @SearchParamDefinition(name = "patient", path = "MedicationDispense.patient", description = "The identity of a patient to list dispenses for", type = "reference") 2424 public static final String SP_PATIENT = "patient"; 2425 @SearchParamDefinition(name = "status", path = "MedicationDispense.status", description = "Status of the dispense", type = "token") 2426 public static final String SP_STATUS = "status"; 2427 2428}