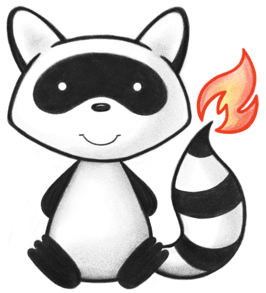
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * An order for both supply of the medication and the instructions for 048 * administration of the medication to a patient. The resource is called 049 * "MedicationOrder" rather than "MedicationPrescription" to generalize the use 050 * across inpatient and outpatient settings as well as for care plans, etc. 051 */ 052@ResourceDef(name = "MedicationOrder", profile = "http://hl7.org/fhir/Profile/MedicationOrder") 053public class MedicationOrder extends DomainResource { 054 055 public enum MedicationOrderStatus { 056 /** 057 * The prescription is 'actionable', but not all actions that are implied by it 058 * have occurred yet. 059 */ 060 ACTIVE, 061 /** 062 * Actions implied by the prescription are to be temporarily halted, but are 063 * expected to continue later. May also be called "suspended". 064 */ 065 ONHOLD, 066 /** 067 * All actions that are implied by the prescription have occurred. 068 */ 069 COMPLETED, 070 /** 071 * The prescription was entered in error. 072 */ 073 ENTEREDINERROR, 074 /** 075 * Actions implied by the prescription are to be permanently halted, before all 076 * of them occurred. 077 */ 078 STOPPED, 079 /** 080 * The prescription is not yet 'actionable', i.e. it is a work in progress, 081 * requires sign-off or verification, and needs to be run through decision 082 * support process. 083 */ 084 DRAFT, 085 /** 086 * added to help the parsers 087 */ 088 NULL; 089 090 public static MedicationOrderStatus fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("active".equals(codeString)) 094 return ACTIVE; 095 if ("on-hold".equals(codeString)) 096 return ONHOLD; 097 if ("completed".equals(codeString)) 098 return COMPLETED; 099 if ("entered-in-error".equals(codeString)) 100 return ENTEREDINERROR; 101 if ("stopped".equals(codeString)) 102 return STOPPED; 103 if ("draft".equals(codeString)) 104 return DRAFT; 105 throw new FHIRException("Unknown MedicationOrderStatus code '" + codeString + "'"); 106 } 107 108 public String toCode() { 109 switch (this) { 110 case ACTIVE: 111 return "active"; 112 case ONHOLD: 113 return "on-hold"; 114 case COMPLETED: 115 return "completed"; 116 case ENTEREDINERROR: 117 return "entered-in-error"; 118 case STOPPED: 119 return "stopped"; 120 case DRAFT: 121 return "draft"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getSystem() { 130 switch (this) { 131 case ACTIVE: 132 return "http://hl7.org/fhir/medication-order-status"; 133 case ONHOLD: 134 return "http://hl7.org/fhir/medication-order-status"; 135 case COMPLETED: 136 return "http://hl7.org/fhir/medication-order-status"; 137 case ENTEREDINERROR: 138 return "http://hl7.org/fhir/medication-order-status"; 139 case STOPPED: 140 return "http://hl7.org/fhir/medication-order-status"; 141 case DRAFT: 142 return "http://hl7.org/fhir/medication-order-status"; 143 case NULL: 144 return null; 145 default: 146 return "?"; 147 } 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case ACTIVE: 153 return "The prescription is 'actionable', but not all actions that are implied by it have occurred yet."; 154 case ONHOLD: 155 return "Actions implied by the prescription are to be temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 156 case COMPLETED: 157 return "All actions that are implied by the prescription have occurred."; 158 case ENTEREDINERROR: 159 return "The prescription was entered in error."; 160 case STOPPED: 161 return "Actions implied by the prescription are to be permanently halted, before all of them occurred."; 162 case DRAFT: 163 return "The prescription is not yet 'actionable', i.e. it is a work in progress, requires sign-off or verification, and needs to be run through decision support process."; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171 public String getDisplay() { 172 switch (this) { 173 case ACTIVE: 174 return "Active"; 175 case ONHOLD: 176 return "On Hold"; 177 case COMPLETED: 178 return "Completed"; 179 case ENTEREDINERROR: 180 return "Entered In Error"; 181 case STOPPED: 182 return "Stopped"; 183 case DRAFT: 184 return "Draft"; 185 case NULL: 186 return null; 187 default: 188 return "?"; 189 } 190 } 191 } 192 193 public static class MedicationOrderStatusEnumFactory implements EnumFactory<MedicationOrderStatus> { 194 public MedicationOrderStatus fromCode(String codeString) throws IllegalArgumentException { 195 if (codeString == null || "".equals(codeString)) 196 if (codeString == null || "".equals(codeString)) 197 return null; 198 if ("active".equals(codeString)) 199 return MedicationOrderStatus.ACTIVE; 200 if ("on-hold".equals(codeString)) 201 return MedicationOrderStatus.ONHOLD; 202 if ("completed".equals(codeString)) 203 return MedicationOrderStatus.COMPLETED; 204 if ("entered-in-error".equals(codeString)) 205 return MedicationOrderStatus.ENTEREDINERROR; 206 if ("stopped".equals(codeString)) 207 return MedicationOrderStatus.STOPPED; 208 if ("draft".equals(codeString)) 209 return MedicationOrderStatus.DRAFT; 210 throw new IllegalArgumentException("Unknown MedicationOrderStatus code '" + codeString + "'"); 211 } 212 213 public Enumeration<MedicationOrderStatus> fromType(Base code) throws FHIRException { 214 if (code == null || code.isEmpty()) 215 return null; 216 String codeString = ((PrimitiveType) code).asStringValue(); 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("active".equals(codeString)) 220 return new Enumeration<MedicationOrderStatus>(this, MedicationOrderStatus.ACTIVE); 221 if ("on-hold".equals(codeString)) 222 return new Enumeration<MedicationOrderStatus>(this, MedicationOrderStatus.ONHOLD); 223 if ("completed".equals(codeString)) 224 return new Enumeration<MedicationOrderStatus>(this, MedicationOrderStatus.COMPLETED); 225 if ("entered-in-error".equals(codeString)) 226 return new Enumeration<MedicationOrderStatus>(this, MedicationOrderStatus.ENTEREDINERROR); 227 if ("stopped".equals(codeString)) 228 return new Enumeration<MedicationOrderStatus>(this, MedicationOrderStatus.STOPPED); 229 if ("draft".equals(codeString)) 230 return new Enumeration<MedicationOrderStatus>(this, MedicationOrderStatus.DRAFT); 231 throw new FHIRException("Unknown MedicationOrderStatus code '" + codeString + "'"); 232 } 233 234 public String toCode(MedicationOrderStatus code) 235 { 236 if (code == MedicationOrderStatus.NULL) 237 return null; 238 if (code == MedicationOrderStatus.ACTIVE) 239 return "active"; 240 if (code == MedicationOrderStatus.ONHOLD) 241 return "on-hold"; 242 if (code == MedicationOrderStatus.COMPLETED) 243 return "completed"; 244 if (code == MedicationOrderStatus.ENTEREDINERROR) 245 return "entered-in-error"; 246 if (code == MedicationOrderStatus.STOPPED) 247 return "stopped"; 248 if (code == MedicationOrderStatus.DRAFT) 249 return "draft"; 250 return "?"; 251 } 252 } 253 254 @Block() 255 public static class MedicationOrderDosageInstructionComponent extends BackboneElement 256 implements IBaseBackboneElement { 257 /** 258 * Free text dosage instructions can be used for cases where the instructions 259 * are too complex to code. The content of this attribute does not include the 260 * name or description of the medication. When coded instructions are present, 261 * the free text instructions may still be present for display to humans taking 262 * or administering the medication. It is expected that the text instructions 263 * will always be populated. If the dosage.timing attribute is also populated, 264 * then the dosage.text should reflect the same information as the timing. 265 */ 266 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 267 @Description(shortDefinition = "Dosage instructions expressed as text", formalDefinition = "Free text dosage instructions can be used for cases where the instructions are too complex to code. The content of this attribute does not include the name or description of the medication. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication. It is expected that the text instructions will always be populated. If the dosage.timing attribute is also populated, then the dosage.text should reflect the same information as the timing.") 268 protected StringType text; 269 270 /** 271 * Additional instructions such as "Swallow with plenty of water" which may or 272 * may not be coded. 273 */ 274 @Child(name = "additionalInstructions", type = { 275 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 276 @Description(shortDefinition = "Supplemental instructions - e.g. \"with meals\"", formalDefinition = "Additional instructions such as \"Swallow with plenty of water\" which may or may not be coded.") 277 protected CodeableConcept additionalInstructions; 278 279 /** 280 * The timing schedule for giving the medication to the patient. The Schedule 281 * data type allows many different expressions. For example: "Every 8 hours"; 282 * "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 283 * 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013". 284 */ 285 @Child(name = "timing", type = { Timing.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 286 @Description(shortDefinition = "When medication should be administered", formalDefinition = "The timing schedule for giving the medication to the patient. The Schedule data type allows many different expressions. For example: \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".") 287 protected Timing timing; 288 289 /** 290 * Indicates whether the Medication is only taken when needed within a specific 291 * dosing schedule (Boolean option), or it indicates the precondition for taking 292 * the Medication (CodeableConcept). 293 */ 294 @Child(name = "asNeeded", type = { BooleanType.class, 295 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 296 @Description(shortDefinition = "Take \"as needed\" (for x)", formalDefinition = "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).") 297 protected Type asNeeded; 298 299 /** 300 * A coded specification of the anatomic site where the medication first enters 301 * the body. 302 */ 303 @Child(name = "site", type = { CodeableConcept.class, 304 BodySite.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 305 @Description(shortDefinition = "Body site to administer to", formalDefinition = "A coded specification of the anatomic site where the medication first enters the body.") 306 protected Type site; 307 308 /** 309 * A code specifying the route or physiological path of administration of a 310 * therapeutic agent into or onto a patient's body. 311 */ 312 @Child(name = "route", type = { 313 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 314 @Description(shortDefinition = "How drug should enter body", formalDefinition = "A code specifying the route or physiological path of administration of a therapeutic agent into or onto a patient's body.") 315 protected CodeableConcept route; 316 317 /** 318 * A coded value indicating the method by which the medication is introduced 319 * into or onto the body. Most commonly used for injections. For examples, Slow 320 * Push; Deep IV. 321 */ 322 @Child(name = "method", type = { 323 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 324 @Description(shortDefinition = "Technique for administering medication", formalDefinition = "A coded value indicating the method by which the medication is introduced into or onto the body. Most commonly used for injections. For examples, Slow Push; Deep IV.") 325 protected CodeableConcept method; 326 327 /** 328 * The amount of therapeutic or other substance given at one administration 329 * event. 330 */ 331 @Child(name = "dose", type = { Range.class, 332 SimpleQuantity.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 333 @Description(shortDefinition = "Amount of medication per dose", formalDefinition = "The amount of therapeutic or other substance given at one administration event.") 334 protected Type dose; 335 336 /** 337 * Identifies the speed with which the medication was or will be introduced into 338 * the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 339 * ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 340 * hours. Currently we do not specify a default of '1' in the denominator, but 341 * this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 342 * liter/8 hours. 343 */ 344 @Child(name = "rate", type = { Ratio.class, 345 Range.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 346 @Description(shortDefinition = "Amount of medication per unit of time", formalDefinition = "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.") 347 protected Type rate; 348 349 /** 350 * The maximum total quantity of a therapeutic substance that may be 351 * administered to a subject over the period of time. For example, 1000mg in 24 352 * hours. 353 */ 354 @Child(name = "maxDosePerPeriod", type = { 355 Ratio.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 356 @Description(shortDefinition = "Upper limit on medication per unit of time", formalDefinition = "The maximum total quantity of a therapeutic substance that may be administered to a subject over the period of time. For example, 1000mg in 24 hours.") 357 protected Ratio maxDosePerPeriod; 358 359 private static final long serialVersionUID = -1470136646L; 360 361 /* 362 * Constructor 363 */ 364 public MedicationOrderDosageInstructionComponent() { 365 super(); 366 } 367 368 /** 369 * @return {@link #text} (Free text dosage instructions can be used for cases 370 * where the instructions are too complex to code. The content of this 371 * attribute does not include the name or description of the medication. 372 * When coded instructions are present, the free text instructions may 373 * still be present for display to humans taking or administering the 374 * medication. It is expected that the text instructions will always be 375 * populated. If the dosage.timing attribute is also populated, then the 376 * dosage.text should reflect the same information as the timing.). This 377 * is the underlying object with id, value and extensions. The accessor 378 * "getText" gives direct access to the value 379 */ 380 public StringType getTextElement() { 381 if (this.text == null) 382 if (Configuration.errorOnAutoCreate()) 383 throw new Error("Attempt to auto-create MedicationOrderDosageInstructionComponent.text"); 384 else if (Configuration.doAutoCreate()) 385 this.text = new StringType(); // bb 386 return this.text; 387 } 388 389 public boolean hasTextElement() { 390 return this.text != null && !this.text.isEmpty(); 391 } 392 393 public boolean hasText() { 394 return this.text != null && !this.text.isEmpty(); 395 } 396 397 /** 398 * @param value {@link #text} (Free text dosage instructions can be used for 399 * cases where the instructions are too complex to code. The 400 * content of this attribute does not include the name or 401 * description of the medication. When coded instructions are 402 * present, the free text instructions may still be present for 403 * display to humans taking or administering the medication. It is 404 * expected that the text instructions will always be populated. If 405 * the dosage.timing attribute is also populated, then the 406 * dosage.text should reflect the same information as the timing.). 407 * This is the underlying object with id, value and extensions. The 408 * accessor "getText" gives direct access to the value 409 */ 410 public MedicationOrderDosageInstructionComponent setTextElement(StringType value) { 411 this.text = value; 412 return this; 413 } 414 415 /** 416 * @return Free text dosage instructions can be used for cases where the 417 * instructions are too complex to code. The content of this attribute 418 * does not include the name or description of the medication. When 419 * coded instructions are present, the free text instructions may still 420 * be present for display to humans taking or administering the 421 * medication. It is expected that the text instructions will always be 422 * populated. If the dosage.timing attribute is also populated, then the 423 * dosage.text should reflect the same information as the timing. 424 */ 425 public String getText() { 426 return this.text == null ? null : this.text.getValue(); 427 } 428 429 /** 430 * @param value Free text dosage instructions can be used for cases where the 431 * instructions are too complex to code. The content of this 432 * attribute does not include the name or description of the 433 * medication. When coded instructions are present, the free text 434 * instructions may still be present for display to humans taking 435 * or administering the medication. It is expected that the text 436 * instructions will always be populated. If the dosage.timing 437 * attribute is also populated, then the dosage.text should reflect 438 * the same information as the timing. 439 */ 440 public MedicationOrderDosageInstructionComponent setText(String value) { 441 if (Utilities.noString(value)) 442 this.text = null; 443 else { 444 if (this.text == null) 445 this.text = new StringType(); 446 this.text.setValue(value); 447 } 448 return this; 449 } 450 451 /** 452 * @return {@link #additionalInstructions} (Additional instructions such as 453 * "Swallow with plenty of water" which may or may not be coded.) 454 */ 455 public CodeableConcept getAdditionalInstructions() { 456 if (this.additionalInstructions == null) 457 if (Configuration.errorOnAutoCreate()) 458 throw new Error("Attempt to auto-create MedicationOrderDosageInstructionComponent.additionalInstructions"); 459 else if (Configuration.doAutoCreate()) 460 this.additionalInstructions = new CodeableConcept(); // cc 461 return this.additionalInstructions; 462 } 463 464 public boolean hasAdditionalInstructions() { 465 return this.additionalInstructions != null && !this.additionalInstructions.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #additionalInstructions} (Additional instructions such as 470 * "Swallow with plenty of water" which may or may not be coded.) 471 */ 472 public MedicationOrderDosageInstructionComponent setAdditionalInstructions(CodeableConcept value) { 473 this.additionalInstructions = value; 474 return this; 475 } 476 477 /** 478 * @return {@link #timing} (The timing schedule for giving the medication to the 479 * patient. The Schedule data type allows many different expressions. 480 * For example: "Every 8 hours"; "Three times a day"; "1/2 an hour 481 * before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 482 * 2013 and 1 Nov 2013".) 483 */ 484 public Timing getTiming() { 485 if (this.timing == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create MedicationOrderDosageInstructionComponent.timing"); 488 else if (Configuration.doAutoCreate()) 489 this.timing = new Timing(); // cc 490 return this.timing; 491 } 492 493 public boolean hasTiming() { 494 return this.timing != null && !this.timing.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #timing} (The timing schedule for giving the medication 499 * to the patient. The Schedule data type allows many different 500 * expressions. For example: "Every 8 hours"; "Three times a day"; 501 * "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; 502 * "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 503 */ 504 public MedicationOrderDosageInstructionComponent setTiming(Timing value) { 505 this.timing = value; 506 return this; 507 } 508 509 /** 510 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 511 * when needed within a specific dosing schedule (Boolean option), or it 512 * indicates the precondition for taking the Medication 513 * (CodeableConcept).) 514 */ 515 public Type getAsNeeded() { 516 return this.asNeeded; 517 } 518 519 /** 520 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 521 * when needed within a specific dosing schedule (Boolean option), or it 522 * indicates the precondition for taking the Medication 523 * (CodeableConcept).) 524 */ 525 public BooleanType getAsNeededBooleanType() throws FHIRException { 526 if (!(this.asNeeded instanceof BooleanType)) 527 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 528 + this.asNeeded.getClass().getName() + " was encountered"); 529 return (BooleanType) this.asNeeded; 530 } 531 532 public boolean hasAsNeededBooleanType() { 533 return this.asNeeded instanceof BooleanType; 534 } 535 536 /** 537 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 538 * when needed within a specific dosing schedule (Boolean option), or it 539 * indicates the precondition for taking the Medication 540 * (CodeableConcept).) 541 */ 542 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 543 if (!(this.asNeeded instanceof CodeableConcept)) 544 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 545 + this.asNeeded.getClass().getName() + " was encountered"); 546 return (CodeableConcept) this.asNeeded; 547 } 548 549 public boolean hasAsNeededCodeableConcept() { 550 return this.asNeeded instanceof CodeableConcept; 551 } 552 553 public boolean hasAsNeeded() { 554 return this.asNeeded != null && !this.asNeeded.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #asNeeded} (Indicates whether the Medication is only 559 * taken when needed within a specific dosing schedule (Boolean 560 * option), or it indicates the precondition for taking the 561 * Medication (CodeableConcept).) 562 */ 563 public MedicationOrderDosageInstructionComponent setAsNeeded(Type value) { 564 this.asNeeded = value; 565 return this; 566 } 567 568 /** 569 * @return {@link #site} (A coded specification of the anatomic site where the 570 * medication first enters the body.) 571 */ 572 public Type getSite() { 573 return this.site; 574 } 575 576 /** 577 * @return {@link #site} (A coded specification of the anatomic site where the 578 * medication first enters the body.) 579 */ 580 public CodeableConcept getSiteCodeableConcept() throws FHIRException { 581 if (!(this.site instanceof CodeableConcept)) 582 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 583 + this.site.getClass().getName() + " was encountered"); 584 return (CodeableConcept) this.site; 585 } 586 587 public boolean hasSiteCodeableConcept() { 588 return this.site instanceof CodeableConcept; 589 } 590 591 /** 592 * @return {@link #site} (A coded specification of the anatomic site where the 593 * medication first enters the body.) 594 */ 595 public Reference getSiteReference() throws FHIRException { 596 if (!(this.site instanceof Reference)) 597 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.site.getClass().getName() 598 + " was encountered"); 599 return (Reference) this.site; 600 } 601 602 public boolean hasSiteReference() { 603 return this.site instanceof Reference; 604 } 605 606 public boolean hasSite() { 607 return this.site != null && !this.site.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #site} (A coded specification of the anatomic site where 612 * the medication first enters the body.) 613 */ 614 public MedicationOrderDosageInstructionComponent setSite(Type value) { 615 this.site = value; 616 return this; 617 } 618 619 /** 620 * @return {@link #route} (A code specifying the route or physiological path of 621 * administration of a therapeutic agent into or onto a patient's body.) 622 */ 623 public CodeableConcept getRoute() { 624 if (this.route == null) 625 if (Configuration.errorOnAutoCreate()) 626 throw new Error("Attempt to auto-create MedicationOrderDosageInstructionComponent.route"); 627 else if (Configuration.doAutoCreate()) 628 this.route = new CodeableConcept(); // cc 629 return this.route; 630 } 631 632 public boolean hasRoute() { 633 return this.route != null && !this.route.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #route} (A code specifying the route or physiological 638 * path of administration of a therapeutic agent into or onto a 639 * patient's body.) 640 */ 641 public MedicationOrderDosageInstructionComponent setRoute(CodeableConcept value) { 642 this.route = value; 643 return this; 644 } 645 646 /** 647 * @return {@link #method} (A coded value indicating the method by which the 648 * medication is introduced into or onto the body. Most commonly used 649 * for injections. For examples, Slow Push; Deep IV.) 650 */ 651 public CodeableConcept getMethod() { 652 if (this.method == null) 653 if (Configuration.errorOnAutoCreate()) 654 throw new Error("Attempt to auto-create MedicationOrderDosageInstructionComponent.method"); 655 else if (Configuration.doAutoCreate()) 656 this.method = new CodeableConcept(); // cc 657 return this.method; 658 } 659 660 public boolean hasMethod() { 661 return this.method != null && !this.method.isEmpty(); 662 } 663 664 /** 665 * @param value {@link #method} (A coded value indicating the method by which 666 * the medication is introduced into or onto the body. Most 667 * commonly used for injections. For examples, Slow Push; Deep IV.) 668 */ 669 public MedicationOrderDosageInstructionComponent setMethod(CodeableConcept value) { 670 this.method = value; 671 return this; 672 } 673 674 /** 675 * @return {@link #dose} (The amount of therapeutic or other substance given at 676 * one administration event.) 677 */ 678 public Type getDose() { 679 return this.dose; 680 } 681 682 /** 683 * @return {@link #dose} (The amount of therapeutic or other substance given at 684 * one administration event.) 685 */ 686 public Range getDoseRange() throws FHIRException { 687 if (!(this.dose instanceof Range)) 688 throw new FHIRException( 689 "Type mismatch: the type Range was expected, but " + this.dose.getClass().getName() + " was encountered"); 690 return (Range) this.dose; 691 } 692 693 public boolean hasDoseRange() { 694 return this.dose instanceof Range; 695 } 696 697 /** 698 * @return {@link #dose} (The amount of therapeutic or other substance given at 699 * one administration event.) 700 */ 701 public SimpleQuantity getDoseSimpleQuantity() throws FHIRException { 702 if (!(this.dose instanceof SimpleQuantity)) 703 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but " 704 + this.dose.getClass().getName() + " was encountered"); 705 return (SimpleQuantity) this.dose; 706 } 707 708 public boolean hasDoseSimpleQuantity() { 709 return this.dose instanceof SimpleQuantity; 710 } 711 712 public boolean hasDose() { 713 return this.dose != null && !this.dose.isEmpty(); 714 } 715 716 /** 717 * @param value {@link #dose} (The amount of therapeutic or other substance 718 * given at one administration event.) 719 */ 720 public MedicationOrderDosageInstructionComponent setDose(Type value) { 721 this.dose = value; 722 return this; 723 } 724 725 /** 726 * @return {@link #rate} (Identifies the speed with which the medication was or 727 * will be introduced into the patient. Typically the rate for an 728 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 729 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 730 * not specify a default of '1' in the denominator, but this is being 731 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 732 * hours.) 733 */ 734 public Type getRate() { 735 return this.rate; 736 } 737 738 /** 739 * @return {@link #rate} (Identifies the speed with which the medication was or 740 * will be introduced into the patient. Typically the rate for an 741 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 742 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 743 * not specify a default of '1' in the denominator, but this is being 744 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 745 * hours.) 746 */ 747 public Ratio getRateRatio() throws FHIRException { 748 if (!(this.rate instanceof Ratio)) 749 throw new FHIRException( 750 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 751 return (Ratio) this.rate; 752 } 753 754 public boolean hasRateRatio() { 755 return this.rate instanceof Ratio; 756 } 757 758 /** 759 * @return {@link #rate} (Identifies the speed with which the medication was or 760 * will be introduced into the patient. Typically the rate for an 761 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 762 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 763 * not specify a default of '1' in the denominator, but this is being 764 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 765 * hours.) 766 */ 767 public Range getRateRange() throws FHIRException { 768 if (!(this.rate instanceof Range)) 769 throw new FHIRException( 770 "Type mismatch: the type Range was expected, but " + this.rate.getClass().getName() + " was encountered"); 771 return (Range) this.rate; 772 } 773 774 public boolean hasRateRange() { 775 return this.rate instanceof Range; 776 } 777 778 public boolean hasRate() { 779 return this.rate != null && !this.rate.isEmpty(); 780 } 781 782 /** 783 * @param value {@link #rate} (Identifies the speed with which the medication 784 * was or will be introduced into the patient. Typically the rate 785 * for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be 786 * expressed as a rate per unit of time e.g. 500 ml per 2 hours. 787 * Currently we do not specify a default of '1' in the denominator, 788 * but this is being discussed. Other examples: 200 mcg/min or 200 789 * mcg/1 minute; 1 liter/8 hours.) 790 */ 791 public MedicationOrderDosageInstructionComponent setRate(Type value) { 792 this.rate = value; 793 return this; 794 } 795 796 /** 797 * @return {@link #maxDosePerPeriod} (The maximum total quantity of a 798 * therapeutic substance that may be administered to a subject over the 799 * period of time. For example, 1000mg in 24 hours.) 800 */ 801 public Ratio getMaxDosePerPeriod() { 802 if (this.maxDosePerPeriod == null) 803 if (Configuration.errorOnAutoCreate()) 804 throw new Error("Attempt to auto-create MedicationOrderDosageInstructionComponent.maxDosePerPeriod"); 805 else if (Configuration.doAutoCreate()) 806 this.maxDosePerPeriod = new Ratio(); // cc 807 return this.maxDosePerPeriod; 808 } 809 810 public boolean hasMaxDosePerPeriod() { 811 return this.maxDosePerPeriod != null && !this.maxDosePerPeriod.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #maxDosePerPeriod} (The maximum total quantity of a 816 * therapeutic substance that may be administered to a subject over 817 * the period of time. For example, 1000mg in 24 hours.) 818 */ 819 public MedicationOrderDosageInstructionComponent setMaxDosePerPeriod(Ratio value) { 820 this.maxDosePerPeriod = value; 821 return this; 822 } 823 824 protected void listChildren(List<Property> childrenList) { 825 super.listChildren(childrenList); 826 childrenList.add(new Property("text", "string", 827 "Free text dosage instructions can be used for cases where the instructions are too complex to code. The content of this attribute does not include the name or description of the medication. When coded instructions are present, the free text instructions may still be present for display to humans taking or administering the medication. It is expected that the text instructions will always be populated. If the dosage.timing attribute is also populated, then the dosage.text should reflect the same information as the timing.", 828 0, java.lang.Integer.MAX_VALUE, text)); 829 childrenList.add(new Property("additionalInstructions", "CodeableConcept", 830 "Additional instructions such as \"Swallow with plenty of water\" which may or may not be coded.", 0, 831 java.lang.Integer.MAX_VALUE, additionalInstructions)); 832 childrenList.add(new Property("timing", "Timing", 833 "The timing schedule for giving the medication to the patient. The Schedule data type allows many different expressions. For example: \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 834 0, java.lang.Integer.MAX_VALUE, timing)); 835 childrenList.add(new Property("asNeeded[x]", "boolean|CodeableConcept", 836 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 837 0, java.lang.Integer.MAX_VALUE, asNeeded)); 838 childrenList.add(new Property("site[x]", "CodeableConcept|Reference(BodySite)", 839 "A coded specification of the anatomic site where the medication first enters the body.", 0, 840 java.lang.Integer.MAX_VALUE, site)); 841 childrenList.add(new Property("route", "CodeableConcept", 842 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto a patient's body.", 843 0, java.lang.Integer.MAX_VALUE, route)); 844 childrenList.add(new Property("method", "CodeableConcept", 845 "A coded value indicating the method by which the medication is introduced into or onto the body. Most commonly used for injections. For examples, Slow Push; Deep IV.", 846 0, java.lang.Integer.MAX_VALUE, method)); 847 childrenList.add(new Property("dose[x]", "Range|SimpleQuantity", 848 "The amount of therapeutic or other substance given at one administration event.", 0, 849 java.lang.Integer.MAX_VALUE, dose)); 850 childrenList.add(new Property("rate[x]", "Ratio|Range", 851 "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 852 0, java.lang.Integer.MAX_VALUE, rate)); 853 childrenList.add(new Property("maxDosePerPeriod", "Ratio", 854 "The maximum total quantity of a therapeutic substance that may be administered to a subject over the period of time. For example, 1000mg in 24 hours.", 855 0, java.lang.Integer.MAX_VALUE, maxDosePerPeriod)); 856 } 857 858 @Override 859 public void setProperty(String name, Base value) throws FHIRException { 860 if (name.equals("text")) 861 this.text = castToString(value); // StringType 862 else if (name.equals("additionalInstructions")) 863 this.additionalInstructions = castToCodeableConcept(value); // CodeableConcept 864 else if (name.equals("timing")) 865 this.timing = castToTiming(value); // Timing 866 else if (name.equals("asNeeded[x]")) 867 this.asNeeded = (Type) value; // Type 868 else if (name.equals("site[x]")) 869 this.site = (Type) value; // Type 870 else if (name.equals("route")) 871 this.route = castToCodeableConcept(value); // CodeableConcept 872 else if (name.equals("method")) 873 this.method = castToCodeableConcept(value); // CodeableConcept 874 else if (name.equals("dose[x]")) 875 this.dose = (Type) value; // Type 876 else if (name.equals("rate[x]")) 877 this.rate = (Type) value; // Type 878 else if (name.equals("maxDosePerPeriod")) 879 this.maxDosePerPeriod = castToRatio(value); // Ratio 880 else 881 super.setProperty(name, value); 882 } 883 884 @Override 885 public Base addChild(String name) throws FHIRException { 886 if (name.equals("text")) { 887 throw new FHIRException("Cannot call addChild on a singleton property MedicationOrder.text"); 888 } else if (name.equals("additionalInstructions")) { 889 this.additionalInstructions = new CodeableConcept(); 890 return this.additionalInstructions; 891 } else if (name.equals("timing")) { 892 this.timing = new Timing(); 893 return this.timing; 894 } else if (name.equals("asNeededBoolean")) { 895 this.asNeeded = new BooleanType(); 896 return this.asNeeded; 897 } else if (name.equals("asNeededCodeableConcept")) { 898 this.asNeeded = new CodeableConcept(); 899 return this.asNeeded; 900 } else if (name.equals("siteCodeableConcept")) { 901 this.site = new CodeableConcept(); 902 return this.site; 903 } else if (name.equals("siteReference")) { 904 this.site = new Reference(); 905 return this.site; 906 } else if (name.equals("route")) { 907 this.route = new CodeableConcept(); 908 return this.route; 909 } else if (name.equals("method")) { 910 this.method = new CodeableConcept(); 911 return this.method; 912 } else if (name.equals("doseRange")) { 913 this.dose = new Range(); 914 return this.dose; 915 } else if (name.equals("doseSimpleQuantity")) { 916 this.dose = new SimpleQuantity(); 917 return this.dose; 918 } else if (name.equals("rateRatio")) { 919 this.rate = new Ratio(); 920 return this.rate; 921 } else if (name.equals("rateRange")) { 922 this.rate = new Range(); 923 return this.rate; 924 } else if (name.equals("maxDosePerPeriod")) { 925 this.maxDosePerPeriod = new Ratio(); 926 return this.maxDosePerPeriod; 927 } else 928 return super.addChild(name); 929 } 930 931 public MedicationOrderDosageInstructionComponent copy() { 932 MedicationOrderDosageInstructionComponent dst = new MedicationOrderDosageInstructionComponent(); 933 copyValues(dst); 934 dst.text = text == null ? null : text.copy(); 935 dst.additionalInstructions = additionalInstructions == null ? null : additionalInstructions.copy(); 936 dst.timing = timing == null ? null : timing.copy(); 937 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 938 dst.site = site == null ? null : site.copy(); 939 dst.route = route == null ? null : route.copy(); 940 dst.method = method == null ? null : method.copy(); 941 dst.dose = dose == null ? null : dose.copy(); 942 dst.rate = rate == null ? null : rate.copy(); 943 dst.maxDosePerPeriod = maxDosePerPeriod == null ? null : maxDosePerPeriod.copy(); 944 return dst; 945 } 946 947 @Override 948 public boolean equalsDeep(Base other) { 949 if (!super.equalsDeep(other)) 950 return false; 951 if (!(other instanceof MedicationOrderDosageInstructionComponent)) 952 return false; 953 MedicationOrderDosageInstructionComponent o = (MedicationOrderDosageInstructionComponent) other; 954 return compareDeep(text, o.text, true) && compareDeep(additionalInstructions, o.additionalInstructions, true) 955 && compareDeep(timing, o.timing, true) && compareDeep(asNeeded, o.asNeeded, true) 956 && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) && compareDeep(method, o.method, true) 957 && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true) 958 && compareDeep(maxDosePerPeriod, o.maxDosePerPeriod, true); 959 } 960 961 @Override 962 public boolean equalsShallow(Base other) { 963 if (!super.equalsShallow(other)) 964 return false; 965 if (!(other instanceof MedicationOrderDosageInstructionComponent)) 966 return false; 967 MedicationOrderDosageInstructionComponent o = (MedicationOrderDosageInstructionComponent) other; 968 return compareValues(text, o.text, true); 969 } 970 971 public boolean isEmpty() { 972 return super.isEmpty() && (text == null || text.isEmpty()) 973 && (additionalInstructions == null || additionalInstructions.isEmpty()) 974 && (timing == null || timing.isEmpty()) && (asNeeded == null || asNeeded.isEmpty()) 975 && (site == null || site.isEmpty()) && (route == null || route.isEmpty()) 976 && (method == null || method.isEmpty()) && (dose == null || dose.isEmpty()) 977 && (rate == null || rate.isEmpty()) && (maxDosePerPeriod == null || maxDosePerPeriod.isEmpty()); 978 } 979 980 public String fhirType() { 981 return "MedicationOrder.dosageInstruction"; 982 983 } 984 985 } 986 987 @Block() 988 public static class MedicationOrderDispenseRequestComponent extends BackboneElement implements IBaseBackboneElement { 989 /** 990 * Identifies the medication being administered. This is a link to a resource 991 * that represents the medication which may be the details of the medication or 992 * simply an attribute carrying a code that identifies the medication from a 993 * known list of medications. 994 */ 995 @Child(name = "medication", type = { CodeableConcept.class, 996 Medication.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 997 @Description(shortDefinition = "Product to be supplied", formalDefinition = "Identifies the medication being administered. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.") 998 protected Type medication; 999 1000 /** 1001 * This indicates the validity period of a prescription (stale dating the 1002 * Prescription). 1003 */ 1004 @Child(name = "validityPeriod", type = { 1005 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1006 @Description(shortDefinition = "Time period supply is authorized for", formalDefinition = "This indicates the validity period of a prescription (stale dating the Prescription).") 1007 protected Period validityPeriod; 1008 1009 /** 1010 * An integer indicating the number of additional times (aka refills or repeats) 1011 * the patient can receive the prescribed medication. Usage Notes: This integer 1012 * does NOT include the original order dispense. This means that if an order 1013 * indicates dispense 30 tablets plus "3 repeats", then the order can be 1014 * dispensed a total of 4 times and the patient can receive a total of 120 1015 * tablets. 1016 */ 1017 @Child(name = "numberOfRepeatsAllowed", type = { 1018 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1019 @Description(shortDefinition = "Number of refills authorized", formalDefinition = "An integer indicating the number of additional times (aka refills or repeats) the patient can receive the prescribed medication. Usage Notes: This integer does NOT include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets.") 1020 protected PositiveIntType numberOfRepeatsAllowed; 1021 1022 /** 1023 * The amount that is to be dispensed for one fill. 1024 */ 1025 @Child(name = "quantity", type = { 1026 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1027 @Description(shortDefinition = "Amount of medication to supply per dispense", formalDefinition = "The amount that is to be dispensed for one fill.") 1028 protected SimpleQuantity quantity; 1029 1030 /** 1031 * Identifies the period time over which the supplied product is expected to be 1032 * used, or the length of time the dispense is expected to last. 1033 */ 1034 @Child(name = "expectedSupplyDuration", type = { 1035 Duration.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1036 @Description(shortDefinition = "Number of days supply per dispense", formalDefinition = "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.") 1037 protected Duration expectedSupplyDuration; 1038 1039 private static final long serialVersionUID = -1690502728L; 1040 1041 /* 1042 * Constructor 1043 */ 1044 public MedicationOrderDispenseRequestComponent() { 1045 super(); 1046 } 1047 1048 /** 1049 * @return {@link #medication} (Identifies the medication being administered. 1050 * This is a link to a resource that represents the medication which may 1051 * be the details of the medication or simply an attribute carrying a 1052 * code that identifies the medication from a known list of 1053 * medications.) 1054 */ 1055 public Type getMedication() { 1056 return this.medication; 1057 } 1058 1059 /** 1060 * @return {@link #medication} (Identifies the medication being administered. 1061 * This is a link to a resource that represents the medication which may 1062 * be the details of the medication or simply an attribute carrying a 1063 * code that identifies the medication from a known list of 1064 * medications.) 1065 */ 1066 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1067 if (!(this.medication instanceof CodeableConcept)) 1068 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1069 + this.medication.getClass().getName() + " was encountered"); 1070 return (CodeableConcept) this.medication; 1071 } 1072 1073 public boolean hasMedicationCodeableConcept() { 1074 return this.medication instanceof CodeableConcept; 1075 } 1076 1077 /** 1078 * @return {@link #medication} (Identifies the medication being administered. 1079 * This is a link to a resource that represents the medication which may 1080 * be the details of the medication or simply an attribute carrying a 1081 * code that identifies the medication from a known list of 1082 * medications.) 1083 */ 1084 public Reference getMedicationReference() throws FHIRException { 1085 if (!(this.medication instanceof Reference)) 1086 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1087 + this.medication.getClass().getName() + " was encountered"); 1088 return (Reference) this.medication; 1089 } 1090 1091 public boolean hasMedicationReference() { 1092 return this.medication instanceof Reference; 1093 } 1094 1095 public boolean hasMedication() { 1096 return this.medication != null && !this.medication.isEmpty(); 1097 } 1098 1099 /** 1100 * @param value {@link #medication} (Identifies the medication being 1101 * administered. This is a link to a resource that represents the 1102 * medication which may be the details of the medication or simply 1103 * an attribute carrying a code that identifies the medication from 1104 * a known list of medications.) 1105 */ 1106 public MedicationOrderDispenseRequestComponent setMedication(Type value) { 1107 this.medication = value; 1108 return this; 1109 } 1110 1111 /** 1112 * @return {@link #validityPeriod} (This indicates the validity period of a 1113 * prescription (stale dating the Prescription).) 1114 */ 1115 public Period getValidityPeriod() { 1116 if (this.validityPeriod == null) 1117 if (Configuration.errorOnAutoCreate()) 1118 throw new Error("Attempt to auto-create MedicationOrderDispenseRequestComponent.validityPeriod"); 1119 else if (Configuration.doAutoCreate()) 1120 this.validityPeriod = new Period(); // cc 1121 return this.validityPeriod; 1122 } 1123 1124 public boolean hasValidityPeriod() { 1125 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #validityPeriod} (This indicates the validity period of a 1130 * prescription (stale dating the Prescription).) 1131 */ 1132 public MedicationOrderDispenseRequestComponent setValidityPeriod(Period value) { 1133 this.validityPeriod = value; 1134 return this; 1135 } 1136 1137 /** 1138 * @return {@link #numberOfRepeatsAllowed} (An integer indicating the number of 1139 * additional times (aka refills or repeats) the patient can receive the 1140 * prescribed medication. Usage Notes: This integer does NOT include the 1141 * original order dispense. This means that if an order indicates 1142 * dispense 30 tablets plus "3 repeats", then the order can be dispensed 1143 * a total of 4 times and the patient can receive a total of 120 1144 * tablets.). This is the underlying object with id, value and 1145 * extensions. The accessor "getNumberOfRepeatsAllowed" gives direct 1146 * access to the value 1147 */ 1148 public PositiveIntType getNumberOfRepeatsAllowedElement() { 1149 if (this.numberOfRepeatsAllowed == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create MedicationOrderDispenseRequestComponent.numberOfRepeatsAllowed"); 1152 else if (Configuration.doAutoCreate()) 1153 this.numberOfRepeatsAllowed = new PositiveIntType(); // bb 1154 return this.numberOfRepeatsAllowed; 1155 } 1156 1157 public boolean hasNumberOfRepeatsAllowedElement() { 1158 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 1159 } 1160 1161 public boolean hasNumberOfRepeatsAllowed() { 1162 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 1163 } 1164 1165 /** 1166 * @param value {@link #numberOfRepeatsAllowed} (An integer indicating the 1167 * number of additional times (aka refills or repeats) the patient 1168 * can receive the prescribed medication. Usage Notes: This integer 1169 * does NOT include the original order dispense. This means that if 1170 * an order indicates dispense 30 tablets plus "3 repeats", then 1171 * the order can be dispensed a total of 4 times and the patient 1172 * can receive a total of 120 tablets.). This is the underlying 1173 * object with id, value and extensions. The accessor 1174 * "getNumberOfRepeatsAllowed" gives direct access to the value 1175 */ 1176 public MedicationOrderDispenseRequestComponent setNumberOfRepeatsAllowedElement(PositiveIntType value) { 1177 this.numberOfRepeatsAllowed = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return An integer indicating the number of additional times (aka refills or 1183 * repeats) the patient can receive the prescribed medication. Usage 1184 * Notes: This integer does NOT include the original order dispense. 1185 * This means that if an order indicates dispense 30 tablets plus "3 1186 * repeats", then the order can be dispensed a total of 4 times and the 1187 * patient can receive a total of 120 tablets. 1188 */ 1189 public int getNumberOfRepeatsAllowed() { 1190 return this.numberOfRepeatsAllowed == null || this.numberOfRepeatsAllowed.isEmpty() ? 0 1191 : this.numberOfRepeatsAllowed.getValue(); 1192 } 1193 1194 /** 1195 * @param value An integer indicating the number of additional times (aka 1196 * refills or repeats) the patient can receive the prescribed 1197 * medication. Usage Notes: This integer does NOT include the 1198 * original order dispense. This means that if an order indicates 1199 * dispense 30 tablets plus "3 repeats", then the order can be 1200 * dispensed a total of 4 times and the patient can receive a total 1201 * of 120 tablets. 1202 */ 1203 public MedicationOrderDispenseRequestComponent setNumberOfRepeatsAllowed(int value) { 1204 if (this.numberOfRepeatsAllowed == null) 1205 this.numberOfRepeatsAllowed = new PositiveIntType(); 1206 this.numberOfRepeatsAllowed.setValue(value); 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #quantity} (The amount that is to be dispensed for one fill.) 1212 */ 1213 public SimpleQuantity getQuantity() { 1214 if (this.quantity == null) 1215 if (Configuration.errorOnAutoCreate()) 1216 throw new Error("Attempt to auto-create MedicationOrderDispenseRequestComponent.quantity"); 1217 else if (Configuration.doAutoCreate()) 1218 this.quantity = new SimpleQuantity(); // cc 1219 return this.quantity; 1220 } 1221 1222 public boolean hasQuantity() { 1223 return this.quantity != null && !this.quantity.isEmpty(); 1224 } 1225 1226 /** 1227 * @param value {@link #quantity} (The amount that is to be dispensed for one 1228 * fill.) 1229 */ 1230 public MedicationOrderDispenseRequestComponent setQuantity(SimpleQuantity value) { 1231 this.quantity = value; 1232 return this; 1233 } 1234 1235 /** 1236 * @return {@link #expectedSupplyDuration} (Identifies the period time over 1237 * which the supplied product is expected to be used, or the length of 1238 * time the dispense is expected to last.) 1239 */ 1240 public Duration getExpectedSupplyDuration() { 1241 if (this.expectedSupplyDuration == null) 1242 if (Configuration.errorOnAutoCreate()) 1243 throw new Error("Attempt to auto-create MedicationOrderDispenseRequestComponent.expectedSupplyDuration"); 1244 else if (Configuration.doAutoCreate()) 1245 this.expectedSupplyDuration = new Duration(); // cc 1246 return this.expectedSupplyDuration; 1247 } 1248 1249 public boolean hasExpectedSupplyDuration() { 1250 return this.expectedSupplyDuration != null && !this.expectedSupplyDuration.isEmpty(); 1251 } 1252 1253 /** 1254 * @param value {@link #expectedSupplyDuration} (Identifies the period time over 1255 * which the supplied product is expected to be used, or the length 1256 * of time the dispense is expected to last.) 1257 */ 1258 public MedicationOrderDispenseRequestComponent setExpectedSupplyDuration(Duration value) { 1259 this.expectedSupplyDuration = value; 1260 return this; 1261 } 1262 1263 protected void listChildren(List<Property> childrenList) { 1264 super.listChildren(childrenList); 1265 childrenList.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1266 "Identifies the medication being administered. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 1267 0, java.lang.Integer.MAX_VALUE, medication)); 1268 childrenList.add(new Property("validityPeriod", "Period", 1269 "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1270 java.lang.Integer.MAX_VALUE, validityPeriod)); 1271 childrenList.add(new Property("numberOfRepeatsAllowed", "positiveInt", 1272 "An integer indicating the number of additional times (aka refills or repeats) the patient can receive the prescribed medication. Usage Notes: This integer does NOT include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets.", 1273 0, java.lang.Integer.MAX_VALUE, numberOfRepeatsAllowed)); 1274 childrenList.add(new Property("quantity", "SimpleQuantity", "The amount that is to be dispensed for one fill.", 0, 1275 java.lang.Integer.MAX_VALUE, quantity)); 1276 childrenList.add(new Property("expectedSupplyDuration", "Duration", 1277 "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 1278 0, java.lang.Integer.MAX_VALUE, expectedSupplyDuration)); 1279 } 1280 1281 @Override 1282 public void setProperty(String name, Base value) throws FHIRException { 1283 if (name.equals("medication[x]")) 1284 this.medication = (Type) value; // Type 1285 else if (name.equals("validityPeriod")) 1286 this.validityPeriod = castToPeriod(value); // Period 1287 else if (name.equals("numberOfRepeatsAllowed")) 1288 this.numberOfRepeatsAllowed = castToPositiveInt(value); // PositiveIntType 1289 else if (name.equals("quantity")) 1290 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1291 else if (name.equals("expectedSupplyDuration")) 1292 this.expectedSupplyDuration = castToDuration(value); // Duration 1293 else 1294 super.setProperty(name, value); 1295 } 1296 1297 @Override 1298 public Base addChild(String name) throws FHIRException { 1299 if (name.equals("medicationCodeableConcept")) { 1300 this.medication = new CodeableConcept(); 1301 return this.medication; 1302 } else if (name.equals("medicationReference")) { 1303 this.medication = new Reference(); 1304 return this.medication; 1305 } else if (name.equals("validityPeriod")) { 1306 this.validityPeriod = new Period(); 1307 return this.validityPeriod; 1308 } else if (name.equals("numberOfRepeatsAllowed")) { 1309 throw new FHIRException("Cannot call addChild on a singleton property MedicationOrder.numberOfRepeatsAllowed"); 1310 } else if (name.equals("quantity")) { 1311 this.quantity = new SimpleQuantity(); 1312 return this.quantity; 1313 } else if (name.equals("expectedSupplyDuration")) { 1314 this.expectedSupplyDuration = new Duration(); 1315 return this.expectedSupplyDuration; 1316 } else 1317 return super.addChild(name); 1318 } 1319 1320 public MedicationOrderDispenseRequestComponent copy() { 1321 MedicationOrderDispenseRequestComponent dst = new MedicationOrderDispenseRequestComponent(); 1322 copyValues(dst); 1323 dst.medication = medication == null ? null : medication.copy(); 1324 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1325 dst.numberOfRepeatsAllowed = numberOfRepeatsAllowed == null ? null : numberOfRepeatsAllowed.copy(); 1326 dst.quantity = quantity == null ? null : quantity.copy(); 1327 dst.expectedSupplyDuration = expectedSupplyDuration == null ? null : expectedSupplyDuration.copy(); 1328 return dst; 1329 } 1330 1331 @Override 1332 public boolean equalsDeep(Base other) { 1333 if (!super.equalsDeep(other)) 1334 return false; 1335 if (!(other instanceof MedicationOrderDispenseRequestComponent)) 1336 return false; 1337 MedicationOrderDispenseRequestComponent o = (MedicationOrderDispenseRequestComponent) other; 1338 return compareDeep(medication, o.medication, true) && compareDeep(validityPeriod, o.validityPeriod, true) 1339 && compareDeep(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true) 1340 && compareDeep(quantity, o.quantity, true) 1341 && compareDeep(expectedSupplyDuration, o.expectedSupplyDuration, true); 1342 } 1343 1344 @Override 1345 public boolean equalsShallow(Base other) { 1346 if (!super.equalsShallow(other)) 1347 return false; 1348 if (!(other instanceof MedicationOrderDispenseRequestComponent)) 1349 return false; 1350 MedicationOrderDispenseRequestComponent o = (MedicationOrderDispenseRequestComponent) other; 1351 return compareValues(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true); 1352 } 1353 1354 public boolean isEmpty() { 1355 return super.isEmpty() && (medication == null || medication.isEmpty()) 1356 && (validityPeriod == null || validityPeriod.isEmpty()) 1357 && (numberOfRepeatsAllowed == null || numberOfRepeatsAllowed.isEmpty()) 1358 && (quantity == null || quantity.isEmpty()) 1359 && (expectedSupplyDuration == null || expectedSupplyDuration.isEmpty()); 1360 } 1361 1362 public String fhirType() { 1363 return "MedicationOrder.dispenseRequest"; 1364 1365 } 1366 1367 } 1368 1369 @Block() 1370 public static class MedicationOrderSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 1371 /** 1372 * A code signifying whether a different drug should be dispensed from what was 1373 * prescribed. 1374 */ 1375 @Child(name = "type", type = { 1376 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1377 @Description(shortDefinition = "generic | formulary +", formalDefinition = "A code signifying whether a different drug should be dispensed from what was prescribed.") 1378 protected CodeableConcept type; 1379 1380 /** 1381 * Indicates the reason for the substitution, or why substitution must or must 1382 * not be performed. 1383 */ 1384 @Child(name = "reason", type = { 1385 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1386 @Description(shortDefinition = "Why should (not) substitution be made", formalDefinition = "Indicates the reason for the substitution, or why substitution must or must not be performed.") 1387 protected CodeableConcept reason; 1388 1389 private static final long serialVersionUID = 1693602518L; 1390 1391 /* 1392 * Constructor 1393 */ 1394 public MedicationOrderSubstitutionComponent() { 1395 super(); 1396 } 1397 1398 /* 1399 * Constructor 1400 */ 1401 public MedicationOrderSubstitutionComponent(CodeableConcept type) { 1402 super(); 1403 this.type = type; 1404 } 1405 1406 /** 1407 * @return {@link #type} (A code signifying whether a different drug should be 1408 * dispensed from what was prescribed.) 1409 */ 1410 public CodeableConcept getType() { 1411 if (this.type == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create MedicationOrderSubstitutionComponent.type"); 1414 else if (Configuration.doAutoCreate()) 1415 this.type = new CodeableConcept(); // cc 1416 return this.type; 1417 } 1418 1419 public boolean hasType() { 1420 return this.type != null && !this.type.isEmpty(); 1421 } 1422 1423 /** 1424 * @param value {@link #type} (A code signifying whether a different drug should 1425 * be dispensed from what was prescribed.) 1426 */ 1427 public MedicationOrderSubstitutionComponent setType(CodeableConcept value) { 1428 this.type = value; 1429 return this; 1430 } 1431 1432 /** 1433 * @return {@link #reason} (Indicates the reason for the substitution, or why 1434 * substitution must or must not be performed.) 1435 */ 1436 public CodeableConcept getReason() { 1437 if (this.reason == null) 1438 if (Configuration.errorOnAutoCreate()) 1439 throw new Error("Attempt to auto-create MedicationOrderSubstitutionComponent.reason"); 1440 else if (Configuration.doAutoCreate()) 1441 this.reason = new CodeableConcept(); // cc 1442 return this.reason; 1443 } 1444 1445 public boolean hasReason() { 1446 return this.reason != null && !this.reason.isEmpty(); 1447 } 1448 1449 /** 1450 * @param value {@link #reason} (Indicates the reason for the substitution, or 1451 * why substitution must or must not be performed.) 1452 */ 1453 public MedicationOrderSubstitutionComponent setReason(CodeableConcept value) { 1454 this.reason = value; 1455 return this; 1456 } 1457 1458 protected void listChildren(List<Property> childrenList) { 1459 super.listChildren(childrenList); 1460 childrenList.add(new Property("type", "CodeableConcept", 1461 "A code signifying whether a different drug should be dispensed from what was prescribed.", 0, 1462 java.lang.Integer.MAX_VALUE, type)); 1463 childrenList.add(new Property("reason", "CodeableConcept", 1464 "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1465 java.lang.Integer.MAX_VALUE, reason)); 1466 } 1467 1468 @Override 1469 public void setProperty(String name, Base value) throws FHIRException { 1470 if (name.equals("type")) 1471 this.type = castToCodeableConcept(value); // CodeableConcept 1472 else if (name.equals("reason")) 1473 this.reason = castToCodeableConcept(value); // CodeableConcept 1474 else 1475 super.setProperty(name, value); 1476 } 1477 1478 @Override 1479 public Base addChild(String name) throws FHIRException { 1480 if (name.equals("type")) { 1481 this.type = new CodeableConcept(); 1482 return this.type; 1483 } else if (name.equals("reason")) { 1484 this.reason = new CodeableConcept(); 1485 return this.reason; 1486 } else 1487 return super.addChild(name); 1488 } 1489 1490 public MedicationOrderSubstitutionComponent copy() { 1491 MedicationOrderSubstitutionComponent dst = new MedicationOrderSubstitutionComponent(); 1492 copyValues(dst); 1493 dst.type = type == null ? null : type.copy(); 1494 dst.reason = reason == null ? null : reason.copy(); 1495 return dst; 1496 } 1497 1498 @Override 1499 public boolean equalsDeep(Base other) { 1500 if (!super.equalsDeep(other)) 1501 return false; 1502 if (!(other instanceof MedicationOrderSubstitutionComponent)) 1503 return false; 1504 MedicationOrderSubstitutionComponent o = (MedicationOrderSubstitutionComponent) other; 1505 return compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true); 1506 } 1507 1508 @Override 1509 public boolean equalsShallow(Base other) { 1510 if (!super.equalsShallow(other)) 1511 return false; 1512 if (!(other instanceof MedicationOrderSubstitutionComponent)) 1513 return false; 1514 MedicationOrderSubstitutionComponent o = (MedicationOrderSubstitutionComponent) other; 1515 return true; 1516 } 1517 1518 public boolean isEmpty() { 1519 return super.isEmpty() && (type == null || type.isEmpty()) && (reason == null || reason.isEmpty()); 1520 } 1521 1522 public String fhirType() { 1523 return "MedicationOrder.substitution"; 1524 1525 } 1526 1527 } 1528 1529 /** 1530 * External identifier - one that would be used by another non-FHIR system - for 1531 * example a re-imbursement system might issue its own id for each prescription 1532 * that is created. This is particularly important where FHIR only provides part 1533 * of an entire workflow process where records have to be tracked through an 1534 * entire system. 1535 */ 1536 @Child(name = "identifier", type = { 1537 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1538 @Description(shortDefinition = "External identifier", formalDefinition = "External identifier - one that would be used by another non-FHIR system - for example a re-imbursement system might issue its own id for each prescription that is created. This is particularly important where FHIR only provides part of an entire workflow process where records have to be tracked through an entire system.") 1539 protected List<Identifier> identifier; 1540 1541 /** 1542 * The date (and perhaps time) when the prescription was written. 1543 */ 1544 @Child(name = "dateWritten", type = { 1545 DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1546 @Description(shortDefinition = "When prescription was authorized", formalDefinition = "The date (and perhaps time) when the prescription was written.") 1547 protected DateTimeType dateWritten; 1548 1549 /** 1550 * A code specifying the state of the order. Generally this will be active or 1551 * completed state. 1552 */ 1553 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 1554 @Description(shortDefinition = "active | on-hold | completed | entered-in-error | stopped | draft", formalDefinition = "A code specifying the state of the order. Generally this will be active or completed state.") 1555 protected Enumeration<MedicationOrderStatus> status; 1556 1557 /** 1558 * The date (and perhaps time) when the prescription was stopped. 1559 */ 1560 @Child(name = "dateEnded", type = { 1561 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1562 @Description(shortDefinition = "When prescription was stopped", formalDefinition = "The date (and perhaps time) when the prescription was stopped.") 1563 protected DateTimeType dateEnded; 1564 1565 /** 1566 * The reason why the prescription was stopped, if it was. 1567 */ 1568 @Child(name = "reasonEnded", type = { 1569 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1570 @Description(shortDefinition = "Why prescription was stopped", formalDefinition = "The reason why the prescription was stopped, if it was.") 1571 protected CodeableConcept reasonEnded; 1572 1573 /** 1574 * A link to a resource representing the person to whom the medication will be 1575 * given. 1576 */ 1577 @Child(name = "patient", type = { Patient.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1578 @Description(shortDefinition = "Who prescription is for", formalDefinition = "A link to a resource representing the person to whom the medication will be given.") 1579 protected Reference patient; 1580 1581 /** 1582 * The actual object that is the target of the reference (A link to a resource 1583 * representing the person to whom the medication will be given.) 1584 */ 1585 protected Patient patientTarget; 1586 1587 /** 1588 * The healthcare professional responsible for authorizing the prescription. 1589 */ 1590 @Child(name = "prescriber", type = { 1591 Practitioner.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1592 @Description(shortDefinition = "Who ordered the medication(s)", formalDefinition = "The healthcare professional responsible for authorizing the prescription.") 1593 protected Reference prescriber; 1594 1595 /** 1596 * The actual object that is the target of the reference (The healthcare 1597 * professional responsible for authorizing the prescription.) 1598 */ 1599 protected Practitioner prescriberTarget; 1600 1601 /** 1602 * A link to a resource that identifies the particular occurrence of contact 1603 * between patient and health care provider. 1604 */ 1605 @Child(name = "encounter", type = { Encounter.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1606 @Description(shortDefinition = "Created during encounter/admission/stay", formalDefinition = "A link to a resource that identifies the particular occurrence of contact between patient and health care provider.") 1607 protected Reference encounter; 1608 1609 /** 1610 * The actual object that is the target of the reference (A link to a resource 1611 * that identifies the particular occurrence of contact between patient and 1612 * health care provider.) 1613 */ 1614 protected Encounter encounterTarget; 1615 1616 /** 1617 * Can be the reason or the indication for writing the prescription. 1618 */ 1619 @Child(name = "reason", type = { CodeableConcept.class, 1620 Condition.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1621 @Description(shortDefinition = "Reason or indication for writing the prescription", formalDefinition = "Can be the reason or the indication for writing the prescription.") 1622 protected Type reason; 1623 1624 /** 1625 * Extra information about the prescription that could not be conveyed by the 1626 * other attributes. 1627 */ 1628 @Child(name = "note", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1629 @Description(shortDefinition = "Information about the prescription", formalDefinition = "Extra information about the prescription that could not be conveyed by the other attributes.") 1630 protected StringType note; 1631 1632 /** 1633 * Identifies the medication being administered. This is a link to a resource 1634 * that represents the medication which may be the details of the medication or 1635 * simply an attribute carrying a code that identifies the medication from a 1636 * known list of medications. 1637 */ 1638 @Child(name = "medication", type = { CodeableConcept.class, 1639 Medication.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 1640 @Description(shortDefinition = "Medication to be taken", formalDefinition = "Identifies the medication being administered. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.") 1641 protected Type medication; 1642 1643 /** 1644 * Indicates how the medication is to be used by the patient. 1645 */ 1646 @Child(name = "dosageInstruction", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1647 @Description(shortDefinition = "How medication should be taken", formalDefinition = "Indicates how the medication is to be used by the patient.") 1648 protected List<MedicationOrderDosageInstructionComponent> dosageInstruction; 1649 1650 /** 1651 * Indicates the specific details for the dispense or medication supply part of 1652 * a medication order (also known as a Medication Prescription). Note that this 1653 * information is NOT always sent with the order. There may be in some settings 1654 * (e.g. hospitals) institutional or system support for completing the dispense 1655 * details in the pharmacy department. 1656 */ 1657 @Child(name = "dispenseRequest", type = {}, order = 12, min = 0, max = 1, modifier = false, summary = true) 1658 @Description(shortDefinition = "Medication supply authorization", formalDefinition = "Indicates the specific details for the dispense or medication supply part of a medication order (also known as a Medication Prescription). Note that this information is NOT always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.") 1659 protected MedicationOrderDispenseRequestComponent dispenseRequest; 1660 1661 /** 1662 * Indicates whether or not substitution can or should be part of the dispense. 1663 * In some cases substitution must happen, in other cases substitution must not 1664 * happen, and in others it does not matter. This block explains the 1665 * prescriber's intent. If nothing is specified substitution may be done. 1666 */ 1667 @Child(name = "substitution", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = true) 1668 @Description(shortDefinition = "Any restrictions on medication substitution", formalDefinition = "Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen, and in others it does not matter. This block explains the prescriber's intent. If nothing is specified substitution may be done.") 1669 protected MedicationOrderSubstitutionComponent substitution; 1670 1671 /** 1672 * A link to a resource representing an earlier order or prescription that this 1673 * order supersedes. 1674 */ 1675 @Child(name = "priorPrescription", type = { 1676 MedicationOrder.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1677 @Description(shortDefinition = "An order/prescription that this supersedes", formalDefinition = "A link to a resource representing an earlier order or prescription that this order supersedes.") 1678 protected Reference priorPrescription; 1679 1680 /** 1681 * The actual object that is the target of the reference (A link to a resource 1682 * representing an earlier order or prescription that this order supersedes.) 1683 */ 1684 protected MedicationOrder priorPrescriptionTarget; 1685 1686 private static final long serialVersionUID = 619326051L; 1687 1688 /* 1689 * Constructor 1690 */ 1691 public MedicationOrder() { 1692 super(); 1693 } 1694 1695 /* 1696 * Constructor 1697 */ 1698 public MedicationOrder(Type medication) { 1699 super(); 1700 this.medication = medication; 1701 } 1702 1703 /** 1704 * @return {@link #identifier} (External identifier - one that would be used by 1705 * another non-FHIR system - for example a re-imbursement system might 1706 * issue its own id for each prescription that is created. This is 1707 * particularly important where FHIR only provides part of an entire 1708 * workflow process where records have to be tracked through an entire 1709 * system.) 1710 */ 1711 public List<Identifier> getIdentifier() { 1712 if (this.identifier == null) 1713 this.identifier = new ArrayList<Identifier>(); 1714 return this.identifier; 1715 } 1716 1717 public boolean hasIdentifier() { 1718 if (this.identifier == null) 1719 return false; 1720 for (Identifier item : this.identifier) 1721 if (!item.isEmpty()) 1722 return true; 1723 return false; 1724 } 1725 1726 /** 1727 * @return {@link #identifier} (External identifier - one that would be used by 1728 * another non-FHIR system - for example a re-imbursement system might 1729 * issue its own id for each prescription that is created. This is 1730 * particularly important where FHIR only provides part of an entire 1731 * workflow process where records have to be tracked through an entire 1732 * system.) 1733 */ 1734 // syntactic sugar 1735 public Identifier addIdentifier() { // 3 1736 Identifier t = new Identifier(); 1737 if (this.identifier == null) 1738 this.identifier = new ArrayList<Identifier>(); 1739 this.identifier.add(t); 1740 return t; 1741 } 1742 1743 // syntactic sugar 1744 public MedicationOrder addIdentifier(Identifier t) { // 3 1745 if (t == null) 1746 return this; 1747 if (this.identifier == null) 1748 this.identifier = new ArrayList<Identifier>(); 1749 this.identifier.add(t); 1750 return this; 1751 } 1752 1753 /** 1754 * @return {@link #dateWritten} (The date (and perhaps time) when the 1755 * prescription was written.). This is the underlying object with id, 1756 * value and extensions. The accessor "getDateWritten" gives direct 1757 * access to the value 1758 */ 1759 public DateTimeType getDateWrittenElement() { 1760 if (this.dateWritten == null) 1761 if (Configuration.errorOnAutoCreate()) 1762 throw new Error("Attempt to auto-create MedicationOrder.dateWritten"); 1763 else if (Configuration.doAutoCreate()) 1764 this.dateWritten = new DateTimeType(); // bb 1765 return this.dateWritten; 1766 } 1767 1768 public boolean hasDateWrittenElement() { 1769 return this.dateWritten != null && !this.dateWritten.isEmpty(); 1770 } 1771 1772 public boolean hasDateWritten() { 1773 return this.dateWritten != null && !this.dateWritten.isEmpty(); 1774 } 1775 1776 /** 1777 * @param value {@link #dateWritten} (The date (and perhaps time) when the 1778 * prescription was written.). This is the underlying object with 1779 * id, value and extensions. The accessor "getDateWritten" gives 1780 * direct access to the value 1781 */ 1782 public MedicationOrder setDateWrittenElement(DateTimeType value) { 1783 this.dateWritten = value; 1784 return this; 1785 } 1786 1787 /** 1788 * @return The date (and perhaps time) when the prescription was written. 1789 */ 1790 public Date getDateWritten() { 1791 return this.dateWritten == null ? null : this.dateWritten.getValue(); 1792 } 1793 1794 /** 1795 * @param value The date (and perhaps time) when the prescription was written. 1796 */ 1797 public MedicationOrder setDateWritten(Date value) { 1798 if (value == null) 1799 this.dateWritten = null; 1800 else { 1801 if (this.dateWritten == null) 1802 this.dateWritten = new DateTimeType(); 1803 this.dateWritten.setValue(value); 1804 } 1805 return this; 1806 } 1807 1808 /** 1809 * @return {@link #status} (A code specifying the state of the order. Generally 1810 * this will be active or completed state.). This is the underlying 1811 * object with id, value and extensions. The accessor "getStatus" gives 1812 * direct access to the value 1813 */ 1814 public Enumeration<MedicationOrderStatus> getStatusElement() { 1815 if (this.status == null) 1816 if (Configuration.errorOnAutoCreate()) 1817 throw new Error("Attempt to auto-create MedicationOrder.status"); 1818 else if (Configuration.doAutoCreate()) 1819 this.status = new Enumeration<MedicationOrderStatus>(new MedicationOrderStatusEnumFactory()); // bb 1820 return this.status; 1821 } 1822 1823 public boolean hasStatusElement() { 1824 return this.status != null && !this.status.isEmpty(); 1825 } 1826 1827 public boolean hasStatus() { 1828 return this.status != null && !this.status.isEmpty(); 1829 } 1830 1831 /** 1832 * @param value {@link #status} (A code specifying the state of the order. 1833 * Generally this will be active or completed state.). This is the 1834 * underlying object with id, value and extensions. The accessor 1835 * "getStatus" gives direct access to the value 1836 */ 1837 public MedicationOrder setStatusElement(Enumeration<MedicationOrderStatus> value) { 1838 this.status = value; 1839 return this; 1840 } 1841 1842 /** 1843 * @return A code specifying the state of the order. Generally this will be 1844 * active or completed state. 1845 */ 1846 public MedicationOrderStatus getStatus() { 1847 return this.status == null ? null : this.status.getValue(); 1848 } 1849 1850 /** 1851 * @param value A code specifying the state of the order. Generally this will be 1852 * active or completed state. 1853 */ 1854 public MedicationOrder setStatus(MedicationOrderStatus value) { 1855 if (value == null) 1856 this.status = null; 1857 else { 1858 if (this.status == null) 1859 this.status = new Enumeration<MedicationOrderStatus>(new MedicationOrderStatusEnumFactory()); 1860 this.status.setValue(value); 1861 } 1862 return this; 1863 } 1864 1865 /** 1866 * @return {@link #dateEnded} (The date (and perhaps time) when the prescription 1867 * was stopped.). This is the underlying object with id, value and 1868 * extensions. The accessor "getDateEnded" gives direct access to the 1869 * value 1870 */ 1871 public DateTimeType getDateEndedElement() { 1872 if (this.dateEnded == null) 1873 if (Configuration.errorOnAutoCreate()) 1874 throw new Error("Attempt to auto-create MedicationOrder.dateEnded"); 1875 else if (Configuration.doAutoCreate()) 1876 this.dateEnded = new DateTimeType(); // bb 1877 return this.dateEnded; 1878 } 1879 1880 public boolean hasDateEndedElement() { 1881 return this.dateEnded != null && !this.dateEnded.isEmpty(); 1882 } 1883 1884 public boolean hasDateEnded() { 1885 return this.dateEnded != null && !this.dateEnded.isEmpty(); 1886 } 1887 1888 /** 1889 * @param value {@link #dateEnded} (The date (and perhaps time) when the 1890 * prescription was stopped.). This is the underlying object with 1891 * id, value and extensions. The accessor "getDateEnded" gives 1892 * direct access to the value 1893 */ 1894 public MedicationOrder setDateEndedElement(DateTimeType value) { 1895 this.dateEnded = value; 1896 return this; 1897 } 1898 1899 /** 1900 * @return The date (and perhaps time) when the prescription was stopped. 1901 */ 1902 public Date getDateEnded() { 1903 return this.dateEnded == null ? null : this.dateEnded.getValue(); 1904 } 1905 1906 /** 1907 * @param value The date (and perhaps time) when the prescription was stopped. 1908 */ 1909 public MedicationOrder setDateEnded(Date value) { 1910 if (value == null) 1911 this.dateEnded = null; 1912 else { 1913 if (this.dateEnded == null) 1914 this.dateEnded = new DateTimeType(); 1915 this.dateEnded.setValue(value); 1916 } 1917 return this; 1918 } 1919 1920 /** 1921 * @return {@link #reasonEnded} (The reason why the prescription was stopped, if 1922 * it was.) 1923 */ 1924 public CodeableConcept getReasonEnded() { 1925 if (this.reasonEnded == null) 1926 if (Configuration.errorOnAutoCreate()) 1927 throw new Error("Attempt to auto-create MedicationOrder.reasonEnded"); 1928 else if (Configuration.doAutoCreate()) 1929 this.reasonEnded = new CodeableConcept(); // cc 1930 return this.reasonEnded; 1931 } 1932 1933 public boolean hasReasonEnded() { 1934 return this.reasonEnded != null && !this.reasonEnded.isEmpty(); 1935 } 1936 1937 /** 1938 * @param value {@link #reasonEnded} (The reason why the prescription was 1939 * stopped, if it was.) 1940 */ 1941 public MedicationOrder setReasonEnded(CodeableConcept value) { 1942 this.reasonEnded = value; 1943 return this; 1944 } 1945 1946 /** 1947 * @return {@link #patient} (A link to a resource representing the person to 1948 * whom the medication will be given.) 1949 */ 1950 public Reference getPatient() { 1951 if (this.patient == null) 1952 if (Configuration.errorOnAutoCreate()) 1953 throw new Error("Attempt to auto-create MedicationOrder.patient"); 1954 else if (Configuration.doAutoCreate()) 1955 this.patient = new Reference(); // cc 1956 return this.patient; 1957 } 1958 1959 public boolean hasPatient() { 1960 return this.patient != null && !this.patient.isEmpty(); 1961 } 1962 1963 /** 1964 * @param value {@link #patient} (A link to a resource representing the person 1965 * to whom the medication will be given.) 1966 */ 1967 public MedicationOrder setPatient(Reference value) { 1968 this.patient = value; 1969 return this; 1970 } 1971 1972 /** 1973 * @return {@link #patient} The actual object that is the target of the 1974 * reference. The reference library doesn't populate this, but you can 1975 * use it to hold the resource if you resolve it. (A link to a resource 1976 * representing the person to whom the medication will be given.) 1977 */ 1978 public Patient getPatientTarget() { 1979 if (this.patientTarget == null) 1980 if (Configuration.errorOnAutoCreate()) 1981 throw new Error("Attempt to auto-create MedicationOrder.patient"); 1982 else if (Configuration.doAutoCreate()) 1983 this.patientTarget = new Patient(); // aa 1984 return this.patientTarget; 1985 } 1986 1987 /** 1988 * @param value {@link #patient} The actual object that is the target of the 1989 * reference. The reference library doesn't use these, but you can 1990 * use it to hold the resource if you resolve it. (A link to a 1991 * resource representing the person to whom the medication will be 1992 * given.) 1993 */ 1994 public MedicationOrder setPatientTarget(Patient value) { 1995 this.patientTarget = value; 1996 return this; 1997 } 1998 1999 /** 2000 * @return {@link #prescriber} (The healthcare professional responsible for 2001 * authorizing the prescription.) 2002 */ 2003 public Reference getPrescriber() { 2004 if (this.prescriber == null) 2005 if (Configuration.errorOnAutoCreate()) 2006 throw new Error("Attempt to auto-create MedicationOrder.prescriber"); 2007 else if (Configuration.doAutoCreate()) 2008 this.prescriber = new Reference(); // cc 2009 return this.prescriber; 2010 } 2011 2012 public boolean hasPrescriber() { 2013 return this.prescriber != null && !this.prescriber.isEmpty(); 2014 } 2015 2016 /** 2017 * @param value {@link #prescriber} (The healthcare professional responsible for 2018 * authorizing the prescription.) 2019 */ 2020 public MedicationOrder setPrescriber(Reference value) { 2021 this.prescriber = value; 2022 return this; 2023 } 2024 2025 /** 2026 * @return {@link #prescriber} The actual object that is the target of the 2027 * reference. The reference library doesn't populate this, but you can 2028 * use it to hold the resource if you resolve it. (The healthcare 2029 * professional responsible for authorizing the prescription.) 2030 */ 2031 public Practitioner getPrescriberTarget() { 2032 if (this.prescriberTarget == null) 2033 if (Configuration.errorOnAutoCreate()) 2034 throw new Error("Attempt to auto-create MedicationOrder.prescriber"); 2035 else if (Configuration.doAutoCreate()) 2036 this.prescriberTarget = new Practitioner(); // aa 2037 return this.prescriberTarget; 2038 } 2039 2040 /** 2041 * @param value {@link #prescriber} The actual object that is the target of the 2042 * reference. The reference library doesn't use these, but you can 2043 * use it to hold the resource if you resolve it. (The healthcare 2044 * professional responsible for authorizing the prescription.) 2045 */ 2046 public MedicationOrder setPrescriberTarget(Practitioner value) { 2047 this.prescriberTarget = value; 2048 return this; 2049 } 2050 2051 /** 2052 * @return {@link #encounter} (A link to a resource that identifies the 2053 * particular occurrence of contact between patient and health care 2054 * provider.) 2055 */ 2056 public Reference getEncounter() { 2057 if (this.encounter == null) 2058 if (Configuration.errorOnAutoCreate()) 2059 throw new Error("Attempt to auto-create MedicationOrder.encounter"); 2060 else if (Configuration.doAutoCreate()) 2061 this.encounter = new Reference(); // cc 2062 return this.encounter; 2063 } 2064 2065 public boolean hasEncounter() { 2066 return this.encounter != null && !this.encounter.isEmpty(); 2067 } 2068 2069 /** 2070 * @param value {@link #encounter} (A link to a resource that identifies the 2071 * particular occurrence of contact between patient and health care 2072 * provider.) 2073 */ 2074 public MedicationOrder setEncounter(Reference value) { 2075 this.encounter = value; 2076 return this; 2077 } 2078 2079 /** 2080 * @return {@link #encounter} The actual object that is the target of the 2081 * reference. The reference library doesn't populate this, but you can 2082 * use it to hold the resource if you resolve it. (A link to a resource 2083 * that identifies the particular occurrence of contact between patient 2084 * and health care provider.) 2085 */ 2086 public Encounter getEncounterTarget() { 2087 if (this.encounterTarget == null) 2088 if (Configuration.errorOnAutoCreate()) 2089 throw new Error("Attempt to auto-create MedicationOrder.encounter"); 2090 else if (Configuration.doAutoCreate()) 2091 this.encounterTarget = new Encounter(); // aa 2092 return this.encounterTarget; 2093 } 2094 2095 /** 2096 * @param value {@link #encounter} The actual object that is the target of the 2097 * reference. The reference library doesn't use these, but you can 2098 * use it to hold the resource if you resolve it. (A link to a 2099 * resource that identifies the particular occurrence of contact 2100 * between patient and health care provider.) 2101 */ 2102 public MedicationOrder setEncounterTarget(Encounter value) { 2103 this.encounterTarget = value; 2104 return this; 2105 } 2106 2107 /** 2108 * @return {@link #reason} (Can be the reason or the indication for writing the 2109 * prescription.) 2110 */ 2111 public Type getReason() { 2112 return this.reason; 2113 } 2114 2115 /** 2116 * @return {@link #reason} (Can be the reason or the indication for writing the 2117 * prescription.) 2118 */ 2119 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 2120 if (!(this.reason instanceof CodeableConcept)) 2121 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2122 + this.reason.getClass().getName() + " was encountered"); 2123 return (CodeableConcept) this.reason; 2124 } 2125 2126 public boolean hasReasonCodeableConcept() { 2127 return this.reason instanceof CodeableConcept; 2128 } 2129 2130 /** 2131 * @return {@link #reason} (Can be the reason or the indication for writing the 2132 * prescription.) 2133 */ 2134 public Reference getReasonReference() throws FHIRException { 2135 if (!(this.reason instanceof Reference)) 2136 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.reason.getClass().getName() 2137 + " was encountered"); 2138 return (Reference) this.reason; 2139 } 2140 2141 public boolean hasReasonReference() { 2142 return this.reason instanceof Reference; 2143 } 2144 2145 public boolean hasReason() { 2146 return this.reason != null && !this.reason.isEmpty(); 2147 } 2148 2149 /** 2150 * @param value {@link #reason} (Can be the reason or the indication for writing 2151 * the prescription.) 2152 */ 2153 public MedicationOrder setReason(Type value) { 2154 this.reason = value; 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #note} (Extra information about the prescription that could 2160 * not be conveyed by the other attributes.). This is the underlying 2161 * object with id, value and extensions. The accessor "getNote" gives 2162 * direct access to the value 2163 */ 2164 public StringType getNoteElement() { 2165 if (this.note == null) 2166 if (Configuration.errorOnAutoCreate()) 2167 throw new Error("Attempt to auto-create MedicationOrder.note"); 2168 else if (Configuration.doAutoCreate()) 2169 this.note = new StringType(); // bb 2170 return this.note; 2171 } 2172 2173 public boolean hasNoteElement() { 2174 return this.note != null && !this.note.isEmpty(); 2175 } 2176 2177 public boolean hasNote() { 2178 return this.note != null && !this.note.isEmpty(); 2179 } 2180 2181 /** 2182 * @param value {@link #note} (Extra information about the prescription that 2183 * could not be conveyed by the other attributes.). This is the 2184 * underlying object with id, value and extensions. The accessor 2185 * "getNote" gives direct access to the value 2186 */ 2187 public MedicationOrder setNoteElement(StringType value) { 2188 this.note = value; 2189 return this; 2190 } 2191 2192 /** 2193 * @return Extra information about the prescription that could not be conveyed 2194 * by the other attributes. 2195 */ 2196 public String getNote() { 2197 return this.note == null ? null : this.note.getValue(); 2198 } 2199 2200 /** 2201 * @param value Extra information about the prescription that could not be 2202 * conveyed by the other attributes. 2203 */ 2204 public MedicationOrder setNote(String value) { 2205 if (Utilities.noString(value)) 2206 this.note = null; 2207 else { 2208 if (this.note == null) 2209 this.note = new StringType(); 2210 this.note.setValue(value); 2211 } 2212 return this; 2213 } 2214 2215 /** 2216 * @return {@link #medication} (Identifies the medication being administered. 2217 * This is a link to a resource that represents the medication which may 2218 * be the details of the medication or simply an attribute carrying a 2219 * code that identifies the medication from a known list of 2220 * medications.) 2221 */ 2222 public Type getMedication() { 2223 return this.medication; 2224 } 2225 2226 /** 2227 * @return {@link #medication} (Identifies the medication being administered. 2228 * This is a link to a resource that represents the medication which may 2229 * be the details of the medication or simply an attribute carrying a 2230 * code that identifies the medication from a known list of 2231 * medications.) 2232 */ 2233 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 2234 if (!(this.medication instanceof CodeableConcept)) 2235 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2236 + this.medication.getClass().getName() + " was encountered"); 2237 return (CodeableConcept) this.medication; 2238 } 2239 2240 public boolean hasMedicationCodeableConcept() { 2241 return this.medication instanceof CodeableConcept; 2242 } 2243 2244 /** 2245 * @return {@link #medication} (Identifies the medication being administered. 2246 * This is a link to a resource that represents the medication which may 2247 * be the details of the medication or simply an attribute carrying a 2248 * code that identifies the medication from a known list of 2249 * medications.) 2250 */ 2251 public Reference getMedicationReference() throws FHIRException { 2252 if (!(this.medication instanceof Reference)) 2253 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2254 + this.medication.getClass().getName() + " was encountered"); 2255 return (Reference) this.medication; 2256 } 2257 2258 public boolean hasMedicationReference() { 2259 return this.medication instanceof Reference; 2260 } 2261 2262 public boolean hasMedication() { 2263 return this.medication != null && !this.medication.isEmpty(); 2264 } 2265 2266 /** 2267 * @param value {@link #medication} (Identifies the medication being 2268 * administered. This is a link to a resource that represents the 2269 * medication which may be the details of the medication or simply 2270 * an attribute carrying a code that identifies the medication from 2271 * a known list of medications.) 2272 */ 2273 public MedicationOrder setMedication(Type value) { 2274 this.medication = value; 2275 return this; 2276 } 2277 2278 /** 2279 * @return {@link #dosageInstruction} (Indicates how the medication is to be 2280 * used by the patient.) 2281 */ 2282 public List<MedicationOrderDosageInstructionComponent> getDosageInstruction() { 2283 if (this.dosageInstruction == null) 2284 this.dosageInstruction = new ArrayList<MedicationOrderDosageInstructionComponent>(); 2285 return this.dosageInstruction; 2286 } 2287 2288 public boolean hasDosageInstruction() { 2289 if (this.dosageInstruction == null) 2290 return false; 2291 for (MedicationOrderDosageInstructionComponent item : this.dosageInstruction) 2292 if (!item.isEmpty()) 2293 return true; 2294 return false; 2295 } 2296 2297 /** 2298 * @return {@link #dosageInstruction} (Indicates how the medication is to be 2299 * used by the patient.) 2300 */ 2301 // syntactic sugar 2302 public MedicationOrderDosageInstructionComponent addDosageInstruction() { // 3 2303 MedicationOrderDosageInstructionComponent t = new MedicationOrderDosageInstructionComponent(); 2304 if (this.dosageInstruction == null) 2305 this.dosageInstruction = new ArrayList<MedicationOrderDosageInstructionComponent>(); 2306 this.dosageInstruction.add(t); 2307 return t; 2308 } 2309 2310 // syntactic sugar 2311 public MedicationOrder addDosageInstruction(MedicationOrderDosageInstructionComponent t) { // 3 2312 if (t == null) 2313 return this; 2314 if (this.dosageInstruction == null) 2315 this.dosageInstruction = new ArrayList<MedicationOrderDosageInstructionComponent>(); 2316 this.dosageInstruction.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return {@link #dispenseRequest} (Indicates the specific details for the 2322 * dispense or medication supply part of a medication order (also known 2323 * as a Medication Prescription). Note that this information is NOT 2324 * always sent with the order. There may be in some settings (e.g. 2325 * hospitals) institutional or system support for completing the 2326 * dispense details in the pharmacy department.) 2327 */ 2328 public MedicationOrderDispenseRequestComponent getDispenseRequest() { 2329 if (this.dispenseRequest == null) 2330 if (Configuration.errorOnAutoCreate()) 2331 throw new Error("Attempt to auto-create MedicationOrder.dispenseRequest"); 2332 else if (Configuration.doAutoCreate()) 2333 this.dispenseRequest = new MedicationOrderDispenseRequestComponent(); // cc 2334 return this.dispenseRequest; 2335 } 2336 2337 public boolean hasDispenseRequest() { 2338 return this.dispenseRequest != null && !this.dispenseRequest.isEmpty(); 2339 } 2340 2341 /** 2342 * @param value {@link #dispenseRequest} (Indicates the specific details for the 2343 * dispense or medication supply part of a medication order (also 2344 * known as a Medication Prescription). Note that this information 2345 * is NOT always sent with the order. There may be in some settings 2346 * (e.g. hospitals) institutional or system support for completing 2347 * the dispense details in the pharmacy department.) 2348 */ 2349 public MedicationOrder setDispenseRequest(MedicationOrderDispenseRequestComponent value) { 2350 this.dispenseRequest = value; 2351 return this; 2352 } 2353 2354 /** 2355 * @return {@link #substitution} (Indicates whether or not substitution can or 2356 * should be part of the dispense. In some cases substitution must 2357 * happen, in other cases substitution must not happen, and in others it 2358 * does not matter. This block explains the prescriber's intent. If 2359 * nothing is specified substitution may be done.) 2360 */ 2361 public MedicationOrderSubstitutionComponent getSubstitution() { 2362 if (this.substitution == null) 2363 if (Configuration.errorOnAutoCreate()) 2364 throw new Error("Attempt to auto-create MedicationOrder.substitution"); 2365 else if (Configuration.doAutoCreate()) 2366 this.substitution = new MedicationOrderSubstitutionComponent(); // cc 2367 return this.substitution; 2368 } 2369 2370 public boolean hasSubstitution() { 2371 return this.substitution != null && !this.substitution.isEmpty(); 2372 } 2373 2374 /** 2375 * @param value {@link #substitution} (Indicates whether or not substitution can 2376 * or should be part of the dispense. In some cases substitution 2377 * must happen, in other cases substitution must not happen, and in 2378 * others it does not matter. This block explains the prescriber's 2379 * intent. If nothing is specified substitution may be done.) 2380 */ 2381 public MedicationOrder setSubstitution(MedicationOrderSubstitutionComponent value) { 2382 this.substitution = value; 2383 return this; 2384 } 2385 2386 /** 2387 * @return {@link #priorPrescription} (A link to a resource representing an 2388 * earlier order or prescription that this order supersedes.) 2389 */ 2390 public Reference getPriorPrescription() { 2391 if (this.priorPrescription == null) 2392 if (Configuration.errorOnAutoCreate()) 2393 throw new Error("Attempt to auto-create MedicationOrder.priorPrescription"); 2394 else if (Configuration.doAutoCreate()) 2395 this.priorPrescription = new Reference(); // cc 2396 return this.priorPrescription; 2397 } 2398 2399 public boolean hasPriorPrescription() { 2400 return this.priorPrescription != null && !this.priorPrescription.isEmpty(); 2401 } 2402 2403 /** 2404 * @param value {@link #priorPrescription} (A link to a resource representing an 2405 * earlier order or prescription that this order supersedes.) 2406 */ 2407 public MedicationOrder setPriorPrescription(Reference value) { 2408 this.priorPrescription = value; 2409 return this; 2410 } 2411 2412 /** 2413 * @return {@link #priorPrescription} The actual object that is the target of 2414 * the reference. The reference library doesn't populate this, but you 2415 * can use it to hold the resource if you resolve it. (A link to a 2416 * resource representing an earlier order or prescription that this 2417 * order supersedes.) 2418 */ 2419 public MedicationOrder getPriorPrescriptionTarget() { 2420 if (this.priorPrescriptionTarget == null) 2421 if (Configuration.errorOnAutoCreate()) 2422 throw new Error("Attempt to auto-create MedicationOrder.priorPrescription"); 2423 else if (Configuration.doAutoCreate()) 2424 this.priorPrescriptionTarget = new MedicationOrder(); // aa 2425 return this.priorPrescriptionTarget; 2426 } 2427 2428 /** 2429 * @param value {@link #priorPrescription} The actual object that is the target 2430 * of the reference. The reference library doesn't use these, but 2431 * you can use it to hold the resource if you resolve it. (A link 2432 * to a resource representing an earlier order or prescription that 2433 * this order supersedes.) 2434 */ 2435 public MedicationOrder setPriorPrescriptionTarget(MedicationOrder value) { 2436 this.priorPrescriptionTarget = value; 2437 return this; 2438 } 2439 2440 protected void listChildren(List<Property> childrenList) { 2441 super.listChildren(childrenList); 2442 childrenList.add(new Property("identifier", "Identifier", 2443 "External identifier - one that would be used by another non-FHIR system - for example a re-imbursement system might issue its own id for each prescription that is created. This is particularly important where FHIR only provides part of an entire workflow process where records have to be tracked through an entire system.", 2444 0, java.lang.Integer.MAX_VALUE, identifier)); 2445 childrenList.add(new Property("dateWritten", "dateTime", 2446 "The date (and perhaps time) when the prescription was written.", 0, java.lang.Integer.MAX_VALUE, dateWritten)); 2447 childrenList.add(new Property("status", "code", 2448 "A code specifying the state of the order. Generally this will be active or completed state.", 0, 2449 java.lang.Integer.MAX_VALUE, status)); 2450 childrenList.add(new Property("dateEnded", "dateTime", 2451 "The date (and perhaps time) when the prescription was stopped.", 0, java.lang.Integer.MAX_VALUE, dateEnded)); 2452 childrenList.add(new Property("reasonEnded", "CodeableConcept", 2453 "The reason why the prescription was stopped, if it was.", 0, java.lang.Integer.MAX_VALUE, reasonEnded)); 2454 childrenList.add(new Property("patient", "Reference(Patient)", 2455 "A link to a resource representing the person to whom the medication will be given.", 0, 2456 java.lang.Integer.MAX_VALUE, patient)); 2457 childrenList.add(new Property("prescriber", "Reference(Practitioner)", 2458 "The healthcare professional responsible for authorizing the prescription.", 0, java.lang.Integer.MAX_VALUE, 2459 prescriber)); 2460 childrenList.add(new Property("encounter", "Reference(Encounter)", 2461 "A link to a resource that identifies the particular occurrence of contact between patient and health care provider.", 2462 0, java.lang.Integer.MAX_VALUE, encounter)); 2463 childrenList.add(new Property("reason[x]", "CodeableConcept|Reference(Condition)", 2464 "Can be the reason or the indication for writing the prescription.", 0, java.lang.Integer.MAX_VALUE, reason)); 2465 childrenList.add(new Property("note", "string", 2466 "Extra information about the prescription that could not be conveyed by the other attributes.", 0, 2467 java.lang.Integer.MAX_VALUE, note)); 2468 childrenList.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2469 "Identifies the medication being administered. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 2470 0, java.lang.Integer.MAX_VALUE, medication)); 2471 childrenList.add(new Property("dosageInstruction", "", "Indicates how the medication is to be used by the patient.", 2472 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 2473 childrenList.add(new Property("dispenseRequest", "", 2474 "Indicates the specific details for the dispense or medication supply part of a medication order (also known as a Medication Prescription). Note that this information is NOT always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 2475 0, java.lang.Integer.MAX_VALUE, dispenseRequest)); 2476 childrenList.add(new Property("substitution", "", 2477 "Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen, and in others it does not matter. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 2478 0, java.lang.Integer.MAX_VALUE, substitution)); 2479 childrenList.add(new Property("priorPrescription", "Reference(MedicationOrder)", 2480 "A link to a resource representing an earlier order or prescription that this order supersedes.", 0, 2481 java.lang.Integer.MAX_VALUE, priorPrescription)); 2482 } 2483 2484 @Override 2485 public void setProperty(String name, Base value) throws FHIRException { 2486 if (name.equals("identifier")) 2487 this.getIdentifier().add(castToIdentifier(value)); 2488 else if (name.equals("dateWritten")) 2489 this.dateWritten = castToDateTime(value); // DateTimeType 2490 else if (name.equals("status")) 2491 this.status = new MedicationOrderStatusEnumFactory().fromType(value); // Enumeration<MedicationOrderStatus> 2492 else if (name.equals("dateEnded")) 2493 this.dateEnded = castToDateTime(value); // DateTimeType 2494 else if (name.equals("reasonEnded")) 2495 this.reasonEnded = castToCodeableConcept(value); // CodeableConcept 2496 else if (name.equals("patient")) 2497 this.patient = castToReference(value); // Reference 2498 else if (name.equals("prescriber")) 2499 this.prescriber = castToReference(value); // Reference 2500 else if (name.equals("encounter")) 2501 this.encounter = castToReference(value); // Reference 2502 else if (name.equals("reason[x]")) 2503 this.reason = (Type) value; // Type 2504 else if (name.equals("note")) 2505 this.note = castToString(value); // StringType 2506 else if (name.equals("medication[x]")) 2507 this.medication = (Type) value; // Type 2508 else if (name.equals("dosageInstruction")) 2509 this.getDosageInstruction().add((MedicationOrderDosageInstructionComponent) value); 2510 else if (name.equals("dispenseRequest")) 2511 this.dispenseRequest = (MedicationOrderDispenseRequestComponent) value; // MedicationOrderDispenseRequestComponent 2512 else if (name.equals("substitution")) 2513 this.substitution = (MedicationOrderSubstitutionComponent) value; // MedicationOrderSubstitutionComponent 2514 else if (name.equals("priorPrescription")) 2515 this.priorPrescription = castToReference(value); // Reference 2516 else 2517 super.setProperty(name, value); 2518 } 2519 2520 @Override 2521 public Base addChild(String name) throws FHIRException { 2522 if (name.equals("identifier")) { 2523 return addIdentifier(); 2524 } else if (name.equals("dateWritten")) { 2525 throw new FHIRException("Cannot call addChild on a singleton property MedicationOrder.dateWritten"); 2526 } else if (name.equals("status")) { 2527 throw new FHIRException("Cannot call addChild on a singleton property MedicationOrder.status"); 2528 } else if (name.equals("dateEnded")) { 2529 throw new FHIRException("Cannot call addChild on a singleton property MedicationOrder.dateEnded"); 2530 } else if (name.equals("reasonEnded")) { 2531 this.reasonEnded = new CodeableConcept(); 2532 return this.reasonEnded; 2533 } else if (name.equals("patient")) { 2534 this.patient = new Reference(); 2535 return this.patient; 2536 } else if (name.equals("prescriber")) { 2537 this.prescriber = new Reference(); 2538 return this.prescriber; 2539 } else if (name.equals("encounter")) { 2540 this.encounter = new Reference(); 2541 return this.encounter; 2542 } else if (name.equals("reasonCodeableConcept")) { 2543 this.reason = new CodeableConcept(); 2544 return this.reason; 2545 } else if (name.equals("reasonReference")) { 2546 this.reason = new Reference(); 2547 return this.reason; 2548 } else if (name.equals("note")) { 2549 throw new FHIRException("Cannot call addChild on a singleton property MedicationOrder.note"); 2550 } else if (name.equals("medicationCodeableConcept")) { 2551 this.medication = new CodeableConcept(); 2552 return this.medication; 2553 } else if (name.equals("medicationReference")) { 2554 this.medication = new Reference(); 2555 return this.medication; 2556 } else if (name.equals("dosageInstruction")) { 2557 return addDosageInstruction(); 2558 } else if (name.equals("dispenseRequest")) { 2559 this.dispenseRequest = new MedicationOrderDispenseRequestComponent(); 2560 return this.dispenseRequest; 2561 } else if (name.equals("substitution")) { 2562 this.substitution = new MedicationOrderSubstitutionComponent(); 2563 return this.substitution; 2564 } else if (name.equals("priorPrescription")) { 2565 this.priorPrescription = new Reference(); 2566 return this.priorPrescription; 2567 } else 2568 return super.addChild(name); 2569 } 2570 2571 public String fhirType() { 2572 return "MedicationOrder"; 2573 2574 } 2575 2576 public MedicationOrder copy() { 2577 MedicationOrder dst = new MedicationOrder(); 2578 copyValues(dst); 2579 if (identifier != null) { 2580 dst.identifier = new ArrayList<Identifier>(); 2581 for (Identifier i : identifier) 2582 dst.identifier.add(i.copy()); 2583 } 2584 ; 2585 dst.dateWritten = dateWritten == null ? null : dateWritten.copy(); 2586 dst.status = status == null ? null : status.copy(); 2587 dst.dateEnded = dateEnded == null ? null : dateEnded.copy(); 2588 dst.reasonEnded = reasonEnded == null ? null : reasonEnded.copy(); 2589 dst.patient = patient == null ? null : patient.copy(); 2590 dst.prescriber = prescriber == null ? null : prescriber.copy(); 2591 dst.encounter = encounter == null ? null : encounter.copy(); 2592 dst.reason = reason == null ? null : reason.copy(); 2593 dst.note = note == null ? null : note.copy(); 2594 dst.medication = medication == null ? null : medication.copy(); 2595 if (dosageInstruction != null) { 2596 dst.dosageInstruction = new ArrayList<MedicationOrderDosageInstructionComponent>(); 2597 for (MedicationOrderDosageInstructionComponent i : dosageInstruction) 2598 dst.dosageInstruction.add(i.copy()); 2599 } 2600 ; 2601 dst.dispenseRequest = dispenseRequest == null ? null : dispenseRequest.copy(); 2602 dst.substitution = substitution == null ? null : substitution.copy(); 2603 dst.priorPrescription = priorPrescription == null ? null : priorPrescription.copy(); 2604 return dst; 2605 } 2606 2607 protected MedicationOrder typedCopy() { 2608 return copy(); 2609 } 2610 2611 @Override 2612 public boolean equalsDeep(Base other) { 2613 if (!super.equalsDeep(other)) 2614 return false; 2615 if (!(other instanceof MedicationOrder)) 2616 return false; 2617 MedicationOrder o = (MedicationOrder) other; 2618 return compareDeep(identifier, o.identifier, true) && compareDeep(dateWritten, o.dateWritten, true) 2619 && compareDeep(status, o.status, true) && compareDeep(dateEnded, o.dateEnded, true) 2620 && compareDeep(reasonEnded, o.reasonEnded, true) && compareDeep(patient, o.patient, true) 2621 && compareDeep(prescriber, o.prescriber, true) && compareDeep(encounter, o.encounter, true) 2622 && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) 2623 && compareDeep(medication, o.medication, true) && compareDeep(dosageInstruction, o.dosageInstruction, true) 2624 && compareDeep(dispenseRequest, o.dispenseRequest, true) && compareDeep(substitution, o.substitution, true) 2625 && compareDeep(priorPrescription, o.priorPrescription, true); 2626 } 2627 2628 @Override 2629 public boolean equalsShallow(Base other) { 2630 if (!super.equalsShallow(other)) 2631 return false; 2632 if (!(other instanceof MedicationOrder)) 2633 return false; 2634 MedicationOrder o = (MedicationOrder) other; 2635 return compareValues(dateWritten, o.dateWritten, true) && compareValues(status, o.status, true) 2636 && compareValues(dateEnded, o.dateEnded, true) && compareValues(note, o.note, true); 2637 } 2638 2639 public boolean isEmpty() { 2640 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 2641 && (dateWritten == null || dateWritten.isEmpty()) && (status == null || status.isEmpty()) 2642 && (dateEnded == null || dateEnded.isEmpty()) && (reasonEnded == null || reasonEnded.isEmpty()) 2643 && (patient == null || patient.isEmpty()) && (prescriber == null || prescriber.isEmpty()) 2644 && (encounter == null || encounter.isEmpty()) && (reason == null || reason.isEmpty()) 2645 && (note == null || note.isEmpty()) && (medication == null || medication.isEmpty()) 2646 && (dosageInstruction == null || dosageInstruction.isEmpty()) 2647 && (dispenseRequest == null || dispenseRequest.isEmpty()) && (substitution == null || substitution.isEmpty()) 2648 && (priorPrescription == null || priorPrescription.isEmpty()); 2649 } 2650 2651 @Override 2652 public ResourceType getResourceType() { 2653 return ResourceType.MedicationOrder; 2654 } 2655 2656 @SearchParamDefinition(name = "prescriber", path = "MedicationOrder.prescriber", description = "Who ordered the medication(s)", type = "reference") 2657 public static final String SP_PRESCRIBER = "prescriber"; 2658 @SearchParamDefinition(name = "identifier", path = "MedicationOrder.identifier", description = "Return prescriptions with this external identifier", type = "token") 2659 public static final String SP_IDENTIFIER = "identifier"; 2660 @SearchParamDefinition(name = "code", path = "MedicationOrder.medicationCodeableConcept", description = "Return administrations of this medication code", type = "token") 2661 public static final String SP_CODE = "code"; 2662 @SearchParamDefinition(name = "patient", path = "MedicationOrder.patient", description = "The identity of a patient to list orders for", type = "reference") 2663 public static final String SP_PATIENT = "patient"; 2664 @SearchParamDefinition(name = "datewritten", path = "MedicationOrder.dateWritten", description = "Return prescriptions written on this date", type = "date") 2665 public static final String SP_DATEWRITTEN = "datewritten"; 2666 @SearchParamDefinition(name = "medication", path = "MedicationOrder.medicationReference", description = "Return administrations of this medication reference", type = "reference") 2667 public static final String SP_MEDICATION = "medication"; 2668 @SearchParamDefinition(name = "encounter", path = "MedicationOrder.encounter", description = "Return prescriptions with this encounter identifier", type = "reference") 2669 public static final String SP_ENCOUNTER = "encounter"; 2670 @SearchParamDefinition(name = "status", path = "MedicationOrder.status", description = "Status of the prescription", type = "token") 2671 public static final String SP_STATUS = "status"; 2672 2673}