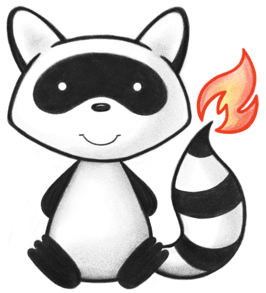
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A record of a medication that is being consumed by a patient. A 048 * MedicationStatement may indicate that the patient may be taking the 049 * medication now, or has taken the medication in the past or will be taking the 050 * medication in the future. The source of this information can be the patient, 051 * significant other (such as a family member or spouse), or a clinician. A 052 * common scenario where this information is captured is during the history 053 * taking process during a patient visit or stay. The medication information may 054 * come from e.g. the patient's memory, from a prescription bottle, or from a 055 * list of medications the patient, clinician or other party maintains 056 * 057 * The primary difference between a medication statement and a medication 058 * administration is that the medication administration has complete 059 * administration information and is based on actual administration information 060 * from the person who administered the medication. A medication statement is 061 * often, if not always, less specific. There is no required date/time when the 062 * medication was administered, in fact we only know that a source has reported 063 * the patient is taking this medication, where details such as time, quantity, 064 * or rate or even medication product may be incomplete or missing or less 065 * precise. As stated earlier, the medication statement information may come 066 * from the patient's memory, from a prescription bottle or from a list of 067 * medications the patient, clinician or other party maintains. Medication 068 * administration is more formal and is not missing detailed information. 069 */ 070@ResourceDef(name = "MedicationStatement", profile = "http://hl7.org/fhir/Profile/MedicationStatement") 071public class MedicationStatement extends DomainResource { 072 073 public enum MedicationStatementStatus { 074 /** 075 * The medication is still being taken. 076 */ 077 ACTIVE, 078 /** 079 * The medication is no longer being taken. 080 */ 081 COMPLETED, 082 /** 083 * The statement was entered in error. 084 */ 085 ENTEREDINERROR, 086 /** 087 * The medication may be taken at some time in the future. 088 */ 089 INTENDED, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 095 public static MedicationStatementStatus fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("active".equals(codeString)) 099 return ACTIVE; 100 if ("completed".equals(codeString)) 101 return COMPLETED; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 if ("intended".equals(codeString)) 105 return INTENDED; 106 throw new FHIRException("Unknown MedicationStatementStatus code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case ACTIVE: 112 return "active"; 113 case COMPLETED: 114 return "completed"; 115 case ENTEREDINERROR: 116 return "entered-in-error"; 117 case INTENDED: 118 return "intended"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getSystem() { 127 switch (this) { 128 case ACTIVE: 129 return "http://hl7.org/fhir/medication-statement-status"; 130 case COMPLETED: 131 return "http://hl7.org/fhir/medication-statement-status"; 132 case ENTEREDINERROR: 133 return "http://hl7.org/fhir/medication-statement-status"; 134 case INTENDED: 135 return "http://hl7.org/fhir/medication-statement-status"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDefinition() { 144 switch (this) { 145 case ACTIVE: 146 return "The medication is still being taken."; 147 case COMPLETED: 148 return "The medication is no longer being taken."; 149 case ENTEREDINERROR: 150 return "The statement was entered in error."; 151 case INTENDED: 152 return "The medication may be taken at some time in the future."; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDisplay() { 161 switch (this) { 162 case ACTIVE: 163 return "Active"; 164 case COMPLETED: 165 return "Completed"; 166 case ENTEREDINERROR: 167 return "Entered in Error"; 168 case INTENDED: 169 return "Intended"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class MedicationStatementStatusEnumFactory implements EnumFactory<MedicationStatementStatus> { 179 public MedicationStatementStatus fromCode(String codeString) throws IllegalArgumentException { 180 if (codeString == null || "".equals(codeString)) 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("active".equals(codeString)) 184 return MedicationStatementStatus.ACTIVE; 185 if ("completed".equals(codeString)) 186 return MedicationStatementStatus.COMPLETED; 187 if ("entered-in-error".equals(codeString)) 188 return MedicationStatementStatus.ENTEREDINERROR; 189 if ("intended".equals(codeString)) 190 return MedicationStatementStatus.INTENDED; 191 throw new IllegalArgumentException("Unknown MedicationStatementStatus code '" + codeString + "'"); 192 } 193 194 public Enumeration<MedicationStatementStatus> fromType(Base code) throws FHIRException { 195 if (code == null || code.isEmpty()) 196 return null; 197 String codeString = ((PrimitiveType) code).asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("active".equals(codeString)) 201 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ACTIVE); 202 if ("completed".equals(codeString)) 203 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.COMPLETED); 204 if ("entered-in-error".equals(codeString)) 205 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ENTEREDINERROR); 206 if ("intended".equals(codeString)) 207 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.INTENDED); 208 throw new FHIRException("Unknown MedicationStatementStatus code '" + codeString + "'"); 209 } 210 211 public String toCode(MedicationStatementStatus code) 212 { 213 if (code == MedicationStatementStatus.NULL) 214 return null; 215 if (code == MedicationStatementStatus.ACTIVE) 216 return "active"; 217 if (code == MedicationStatementStatus.COMPLETED) 218 return "completed"; 219 if (code == MedicationStatementStatus.ENTEREDINERROR) 220 return "entered-in-error"; 221 if (code == MedicationStatementStatus.INTENDED) 222 return "intended"; 223 return "?"; 224 } 225 } 226 227 @Block() 228 public static class MedicationStatementDosageComponent extends BackboneElement implements IBaseBackboneElement { 229 /** 230 * Free text dosage information as reported about a patient's medication use. 231 * When coded dosage information is present, the free text may still be present 232 * for display to humans. 233 */ 234 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 235 @Description(shortDefinition = "Reported dosage information", formalDefinition = "Free text dosage information as reported about a patient's medication use. When coded dosage information is present, the free text may still be present for display to humans.") 236 protected StringType text; 237 238 /** 239 * The timing schedule for giving the medication to the patient. The Schedule 240 * data type allows many different expressions, for example. "Every 8 hours"; 241 * "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 242 * 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013". 243 */ 244 @Child(name = "timing", type = { Timing.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 245 @Description(shortDefinition = "When/how often was medication taken", formalDefinition = "The timing schedule for giving the medication to the patient. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".") 246 protected Timing timing; 247 248 /** 249 * Indicates whether the Medication is only taken when needed within a specific 250 * dosing schedule (Boolean option), or it indicates the precondition for taking 251 * the Medication (CodeableConcept). 252 * 253 * Specifically if 'boolean' datatype is selected, then the following logic 254 * applies: If set to True, this indicates that the medication is only taken 255 * when needed, within the specified schedule. 256 */ 257 @Child(name = "asNeeded", type = { BooleanType.class, 258 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 259 @Description(shortDefinition = "Take \"as needed\" (for x)", formalDefinition = "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept). \n\nSpecifically if 'boolean' datatype is selected, then the following logic applies: If set to True, this indicates that the medication is only taken when needed, within the specified schedule.") 260 protected Type asNeeded; 261 262 /** 263 * A coded specification of or a reference to the anatomic site where the 264 * medication first enters the body. 265 */ 266 @Child(name = "site", type = { CodeableConcept.class, 267 BodySite.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 268 @Description(shortDefinition = "Where (on body) medication is/was administered", formalDefinition = "A coded specification of or a reference to the anatomic site where the medication first enters the body.") 269 protected Type site; 270 271 /** 272 * A code specifying the route or physiological path of administration of a 273 * therapeutic agent into or onto a subject. 274 */ 275 @Child(name = "route", type = { 276 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 277 @Description(shortDefinition = "How the medication entered the body", formalDefinition = "A code specifying the route or physiological path of administration of a therapeutic agent into or onto a subject.") 278 protected CodeableConcept route; 279 280 /** 281 * A coded value indicating the method by which the medication is intended to be 282 * or was introduced into or on the body. This attribute will most often NOT be 283 * populated. It is most commonly used for injections. For example, Slow Push, 284 * Deep IV. 285 */ 286 @Child(name = "method", type = { 287 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 288 @Description(shortDefinition = "Technique used to administer medication", formalDefinition = "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.") 289 protected CodeableConcept method; 290 291 /** 292 * The amount of therapeutic or other substance given at one administration 293 * event. 294 */ 295 @Child(name = "quantity", type = { SimpleQuantity.class, 296 Range.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 297 @Description(shortDefinition = "Amount administered in one dose", formalDefinition = "The amount of therapeutic or other substance given at one administration event.") 298 protected Type quantity; 299 300 /** 301 * Identifies the speed with which the medication was or will be introduced into 302 * the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 303 * ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 304 * hours. Currently we do not specify a default of '1' in the denominator, but 305 * this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 306 * liter/8 hours. 307 */ 308 @Child(name = "rate", type = { Ratio.class, 309 Range.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 310 @Description(shortDefinition = "Dose quantity per unit of time", formalDefinition = "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.") 311 protected Type rate; 312 313 /** 314 * The maximum total quantity of a therapeutic substance that may be 315 * administered to a subject over the period of time. For example, 1000mg in 24 316 * hours. 317 */ 318 @Child(name = "maxDosePerPeriod", type = { 319 Ratio.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 320 @Description(shortDefinition = "Maximum dose that was consumed per unit of time", formalDefinition = "The maximum total quantity of a therapeutic substance that may be administered to a subject over the period of time. For example, 1000mg in 24 hours.") 321 protected Ratio maxDosePerPeriod; 322 323 private static final long serialVersionUID = 246880733L; 324 325 /* 326 * Constructor 327 */ 328 public MedicationStatementDosageComponent() { 329 super(); 330 } 331 332 /** 333 * @return {@link #text} (Free text dosage information as reported about a 334 * patient's medication use. When coded dosage information is present, 335 * the free text may still be present for display to humans.). This is 336 * the underlying object with id, value and extensions. The accessor 337 * "getText" gives direct access to the value 338 */ 339 public StringType getTextElement() { 340 if (this.text == null) 341 if (Configuration.errorOnAutoCreate()) 342 throw new Error("Attempt to auto-create MedicationStatementDosageComponent.text"); 343 else if (Configuration.doAutoCreate()) 344 this.text = new StringType(); // bb 345 return this.text; 346 } 347 348 public boolean hasTextElement() { 349 return this.text != null && !this.text.isEmpty(); 350 } 351 352 public boolean hasText() { 353 return this.text != null && !this.text.isEmpty(); 354 } 355 356 /** 357 * @param value {@link #text} (Free text dosage information as reported about a 358 * patient's medication use. When coded dosage information is 359 * present, the free text may still be present for display to 360 * humans.). This is the underlying object with id, value and 361 * extensions. The accessor "getText" gives direct access to the 362 * value 363 */ 364 public MedicationStatementDosageComponent setTextElement(StringType value) { 365 this.text = value; 366 return this; 367 } 368 369 /** 370 * @return Free text dosage information as reported about a patient's medication 371 * use. When coded dosage information is present, the free text may 372 * still be present for display to humans. 373 */ 374 public String getText() { 375 return this.text == null ? null : this.text.getValue(); 376 } 377 378 /** 379 * @param value Free text dosage information as reported about a patient's 380 * medication use. When coded dosage information is present, the 381 * free text may still be present for display to humans. 382 */ 383 public MedicationStatementDosageComponent setText(String value) { 384 if (Utilities.noString(value)) 385 this.text = null; 386 else { 387 if (this.text == null) 388 this.text = new StringType(); 389 this.text.setValue(value); 390 } 391 return this; 392 } 393 394 /** 395 * @return {@link #timing} (The timing schedule for giving the medication to the 396 * patient. The Schedule data type allows many different expressions, 397 * for example. "Every 8 hours"; "Three times a day"; "1/2 an hour 398 * before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 399 * 2013 and 1 Nov 2013".) 400 */ 401 public Timing getTiming() { 402 if (this.timing == null) 403 if (Configuration.errorOnAutoCreate()) 404 throw new Error("Attempt to auto-create MedicationStatementDosageComponent.timing"); 405 else if (Configuration.doAutoCreate()) 406 this.timing = new Timing(); // cc 407 return this.timing; 408 } 409 410 public boolean hasTiming() { 411 return this.timing != null && !this.timing.isEmpty(); 412 } 413 414 /** 415 * @param value {@link #timing} (The timing schedule for giving the medication 416 * to the patient. The Schedule data type allows many different 417 * expressions, for example. "Every 8 hours"; "Three times a day"; 418 * "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; 419 * "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 420 */ 421 public MedicationStatementDosageComponent setTiming(Timing value) { 422 this.timing = value; 423 return this; 424 } 425 426 /** 427 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 428 * when needed within a specific dosing schedule (Boolean option), or it 429 * indicates the precondition for taking the Medication 430 * (CodeableConcept). 431 * 432 * Specifically if 'boolean' datatype is selected, then the following 433 * logic applies: If set to True, this indicates that the medication is 434 * only taken when needed, within the specified schedule.) 435 */ 436 public Type getAsNeeded() { 437 return this.asNeeded; 438 } 439 440 /** 441 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 442 * when needed within a specific dosing schedule (Boolean option), or it 443 * indicates the precondition for taking the Medication 444 * (CodeableConcept). 445 * 446 * Specifically if 'boolean' datatype is selected, then the following 447 * logic applies: If set to True, this indicates that the medication is 448 * only taken when needed, within the specified schedule.) 449 */ 450 public BooleanType getAsNeededBooleanType() throws FHIRException { 451 if (!(this.asNeeded instanceof BooleanType)) 452 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 453 + this.asNeeded.getClass().getName() + " was encountered"); 454 return (BooleanType) this.asNeeded; 455 } 456 457 public boolean hasAsNeededBooleanType() { 458 return this.asNeeded instanceof BooleanType; 459 } 460 461 /** 462 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 463 * when needed within a specific dosing schedule (Boolean option), or it 464 * indicates the precondition for taking the Medication 465 * (CodeableConcept). 466 * 467 * Specifically if 'boolean' datatype is selected, then the following 468 * logic applies: If set to True, this indicates that the medication is 469 * only taken when needed, within the specified schedule.) 470 */ 471 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 472 if (!(this.asNeeded instanceof CodeableConcept)) 473 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 474 + this.asNeeded.getClass().getName() + " was encountered"); 475 return (CodeableConcept) this.asNeeded; 476 } 477 478 public boolean hasAsNeededCodeableConcept() { 479 return this.asNeeded instanceof CodeableConcept; 480 } 481 482 public boolean hasAsNeeded() { 483 return this.asNeeded != null && !this.asNeeded.isEmpty(); 484 } 485 486 /** 487 * @param value {@link #asNeeded} (Indicates whether the Medication is only 488 * taken when needed within a specific dosing schedule (Boolean 489 * option), or it indicates the precondition for taking the 490 * Medication (CodeableConcept). 491 * 492 * Specifically if 'boolean' datatype is selected, then the 493 * following logic applies: If set to True, this indicates that the 494 * medication is only taken when needed, within the specified 495 * schedule.) 496 */ 497 public MedicationStatementDosageComponent setAsNeeded(Type value) { 498 this.asNeeded = value; 499 return this; 500 } 501 502 /** 503 * @return {@link #site} (A coded specification of or a reference to the 504 * anatomic site where the medication first enters the body.) 505 */ 506 public Type getSite() { 507 return this.site; 508 } 509 510 /** 511 * @return {@link #site} (A coded specification of or a reference to the 512 * anatomic site where the medication first enters the body.) 513 */ 514 public CodeableConcept getSiteCodeableConcept() throws FHIRException { 515 if (!(this.site instanceof CodeableConcept)) 516 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 517 + this.site.getClass().getName() + " was encountered"); 518 return (CodeableConcept) this.site; 519 } 520 521 public boolean hasSiteCodeableConcept() { 522 return this.site instanceof CodeableConcept; 523 } 524 525 /** 526 * @return {@link #site} (A coded specification of or a reference to the 527 * anatomic site where the medication first enters the body.) 528 */ 529 public Reference getSiteReference() throws FHIRException { 530 if (!(this.site instanceof Reference)) 531 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.site.getClass().getName() 532 + " was encountered"); 533 return (Reference) this.site; 534 } 535 536 public boolean hasSiteReference() { 537 return this.site instanceof Reference; 538 } 539 540 public boolean hasSite() { 541 return this.site != null && !this.site.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #site} (A coded specification of or a reference to the 546 * anatomic site where the medication first enters the body.) 547 */ 548 public MedicationStatementDosageComponent setSite(Type value) { 549 this.site = value; 550 return this; 551 } 552 553 /** 554 * @return {@link #route} (A code specifying the route or physiological path of 555 * administration of a therapeutic agent into or onto a subject.) 556 */ 557 public CodeableConcept getRoute() { 558 if (this.route == null) 559 if (Configuration.errorOnAutoCreate()) 560 throw new Error("Attempt to auto-create MedicationStatementDosageComponent.route"); 561 else if (Configuration.doAutoCreate()) 562 this.route = new CodeableConcept(); // cc 563 return this.route; 564 } 565 566 public boolean hasRoute() { 567 return this.route != null && !this.route.isEmpty(); 568 } 569 570 /** 571 * @param value {@link #route} (A code specifying the route or physiological 572 * path of administration of a therapeutic agent into or onto a 573 * subject.) 574 */ 575 public MedicationStatementDosageComponent setRoute(CodeableConcept value) { 576 this.route = value; 577 return this; 578 } 579 580 /** 581 * @return {@link #method} (A coded value indicating the method by which the 582 * medication is intended to be or was introduced into or on the body. 583 * This attribute will most often NOT be populated. It is most commonly 584 * used for injections. For example, Slow Push, Deep IV.) 585 */ 586 public CodeableConcept getMethod() { 587 if (this.method == null) 588 if (Configuration.errorOnAutoCreate()) 589 throw new Error("Attempt to auto-create MedicationStatementDosageComponent.method"); 590 else if (Configuration.doAutoCreate()) 591 this.method = new CodeableConcept(); // cc 592 return this.method; 593 } 594 595 public boolean hasMethod() { 596 return this.method != null && !this.method.isEmpty(); 597 } 598 599 /** 600 * @param value {@link #method} (A coded value indicating the method by which 601 * the medication is intended to be or was introduced into or on 602 * the body. This attribute will most often NOT be populated. It is 603 * most commonly used for injections. For example, Slow Push, Deep 604 * IV.) 605 */ 606 public MedicationStatementDosageComponent setMethod(CodeableConcept value) { 607 this.method = value; 608 return this; 609 } 610 611 /** 612 * @return {@link #quantity} (The amount of therapeutic or other substance given 613 * at one administration event.) 614 */ 615 public Type getQuantity() { 616 return this.quantity; 617 } 618 619 /** 620 * @return {@link #quantity} (The amount of therapeutic or other substance given 621 * at one administration event.) 622 */ 623 public SimpleQuantity getQuantitySimpleQuantity() throws FHIRException { 624 if (!(this.quantity instanceof SimpleQuantity)) 625 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but " 626 + this.quantity.getClass().getName() + " was encountered"); 627 return (SimpleQuantity) this.quantity; 628 } 629 630 public boolean hasQuantitySimpleQuantity() { 631 return this.quantity instanceof SimpleQuantity; 632 } 633 634 /** 635 * @return {@link #quantity} (The amount of therapeutic or other substance given 636 * at one administration event.) 637 */ 638 public Range getQuantityRange() throws FHIRException { 639 if (!(this.quantity instanceof Range)) 640 throw new FHIRException("Type mismatch: the type Range was expected, but " + this.quantity.getClass().getName() 641 + " was encountered"); 642 return (Range) this.quantity; 643 } 644 645 public boolean hasQuantityRange() { 646 return this.quantity instanceof Range; 647 } 648 649 public boolean hasQuantity() { 650 return this.quantity != null && !this.quantity.isEmpty(); 651 } 652 653 /** 654 * @param value {@link #quantity} (The amount of therapeutic or other substance 655 * given at one administration event.) 656 */ 657 public MedicationStatementDosageComponent setQuantity(Type value) { 658 this.quantity = value; 659 return this; 660 } 661 662 /** 663 * @return {@link #rate} (Identifies the speed with which the medication was or 664 * will be introduced into the patient. Typically the rate for an 665 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 666 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 667 * not specify a default of '1' in the denominator, but this is being 668 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 669 * hours.) 670 */ 671 public Type getRate() { 672 return this.rate; 673 } 674 675 /** 676 * @return {@link #rate} (Identifies the speed with which the medication was or 677 * will be introduced into the patient. Typically the rate for an 678 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 679 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 680 * not specify a default of '1' in the denominator, but this is being 681 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 682 * hours.) 683 */ 684 public Ratio getRateRatio() throws FHIRException { 685 if (!(this.rate instanceof Ratio)) 686 throw new FHIRException( 687 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 688 return (Ratio) this.rate; 689 } 690 691 public boolean hasRateRatio() { 692 return this.rate instanceof Ratio; 693 } 694 695 /** 696 * @return {@link #rate} (Identifies the speed with which the medication was or 697 * will be introduced into the patient. Typically the rate for an 698 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 699 * as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do 700 * not specify a default of '1' in the denominator, but this is being 701 * discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 702 * hours.) 703 */ 704 public Range getRateRange() throws FHIRException { 705 if (!(this.rate instanceof Range)) 706 throw new FHIRException( 707 "Type mismatch: the type Range was expected, but " + this.rate.getClass().getName() + " was encountered"); 708 return (Range) this.rate; 709 } 710 711 public boolean hasRateRange() { 712 return this.rate instanceof Range; 713 } 714 715 public boolean hasRate() { 716 return this.rate != null && !this.rate.isEmpty(); 717 } 718 719 /** 720 * @param value {@link #rate} (Identifies the speed with which the medication 721 * was or will be introduced into the patient. Typically the rate 722 * for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be 723 * expressed as a rate per unit of time e.g. 500 ml per 2 hours. 724 * Currently we do not specify a default of '1' in the denominator, 725 * but this is being discussed. Other examples: 200 mcg/min or 200 726 * mcg/1 minute; 1 liter/8 hours.) 727 */ 728 public MedicationStatementDosageComponent setRate(Type value) { 729 this.rate = value; 730 return this; 731 } 732 733 /** 734 * @return {@link #maxDosePerPeriod} (The maximum total quantity of a 735 * therapeutic substance that may be administered to a subject over the 736 * period of time. For example, 1000mg in 24 hours.) 737 */ 738 public Ratio getMaxDosePerPeriod() { 739 if (this.maxDosePerPeriod == null) 740 if (Configuration.errorOnAutoCreate()) 741 throw new Error("Attempt to auto-create MedicationStatementDosageComponent.maxDosePerPeriod"); 742 else if (Configuration.doAutoCreate()) 743 this.maxDosePerPeriod = new Ratio(); // cc 744 return this.maxDosePerPeriod; 745 } 746 747 public boolean hasMaxDosePerPeriod() { 748 return this.maxDosePerPeriod != null && !this.maxDosePerPeriod.isEmpty(); 749 } 750 751 /** 752 * @param value {@link #maxDosePerPeriod} (The maximum total quantity of a 753 * therapeutic substance that may be administered to a subject over 754 * the period of time. For example, 1000mg in 24 hours.) 755 */ 756 public MedicationStatementDosageComponent setMaxDosePerPeriod(Ratio value) { 757 this.maxDosePerPeriod = value; 758 return this; 759 } 760 761 protected void listChildren(List<Property> childrenList) { 762 super.listChildren(childrenList); 763 childrenList.add(new Property("text", "string", 764 "Free text dosage information as reported about a patient's medication use. When coded dosage information is present, the free text may still be present for display to humans.", 765 0, java.lang.Integer.MAX_VALUE, text)); 766 childrenList.add(new Property("timing", "Timing", 767 "The timing schedule for giving the medication to the patient. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 768 0, java.lang.Integer.MAX_VALUE, timing)); 769 childrenList.add(new Property("asNeeded[x]", "boolean|CodeableConcept", 770 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept). \n\nSpecifically if 'boolean' datatype is selected, then the following logic applies: If set to True, this indicates that the medication is only taken when needed, within the specified schedule.", 771 0, java.lang.Integer.MAX_VALUE, asNeeded)); 772 childrenList.add(new Property("site[x]", "CodeableConcept|Reference(BodySite)", 773 "A coded specification of or a reference to the anatomic site where the medication first enters the body.", 0, 774 java.lang.Integer.MAX_VALUE, site)); 775 childrenList.add(new Property("route", "CodeableConcept", 776 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto a subject.", 777 0, java.lang.Integer.MAX_VALUE, route)); 778 childrenList.add(new Property("method", "CodeableConcept", 779 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 780 0, java.lang.Integer.MAX_VALUE, method)); 781 childrenList.add(new Property("quantity[x]", "SimpleQuantity|Range", 782 "The amount of therapeutic or other substance given at one administration event.", 0, 783 java.lang.Integer.MAX_VALUE, quantity)); 784 childrenList.add(new Property("rate[x]", "Ratio|Range", 785 "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Currently we do not specify a default of '1' in the denominator, but this is being discussed. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 786 0, java.lang.Integer.MAX_VALUE, rate)); 787 childrenList.add(new Property("maxDosePerPeriod", "Ratio", 788 "The maximum total quantity of a therapeutic substance that may be administered to a subject over the period of time. For example, 1000mg in 24 hours.", 789 0, java.lang.Integer.MAX_VALUE, maxDosePerPeriod)); 790 } 791 792 @Override 793 public void setProperty(String name, Base value) throws FHIRException { 794 if (name.equals("text")) 795 this.text = castToString(value); // StringType 796 else if (name.equals("timing")) 797 this.timing = castToTiming(value); // Timing 798 else if (name.equals("asNeeded[x]")) 799 this.asNeeded = (Type) value; // Type 800 else if (name.equals("site[x]")) 801 this.site = (Type) value; // Type 802 else if (name.equals("route")) 803 this.route = castToCodeableConcept(value); // CodeableConcept 804 else if (name.equals("method")) 805 this.method = castToCodeableConcept(value); // CodeableConcept 806 else if (name.equals("quantity[x]")) 807 this.quantity = (Type) value; // Type 808 else if (name.equals("rate[x]")) 809 this.rate = (Type) value; // Type 810 else if (name.equals("maxDosePerPeriod")) 811 this.maxDosePerPeriod = castToRatio(value); // Ratio 812 else 813 super.setProperty(name, value); 814 } 815 816 @Override 817 public Base addChild(String name) throws FHIRException { 818 if (name.equals("text")) { 819 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.text"); 820 } else if (name.equals("timing")) { 821 this.timing = new Timing(); 822 return this.timing; 823 } else if (name.equals("asNeededBoolean")) { 824 this.asNeeded = new BooleanType(); 825 return this.asNeeded; 826 } else if (name.equals("asNeededCodeableConcept")) { 827 this.asNeeded = new CodeableConcept(); 828 return this.asNeeded; 829 } else if (name.equals("siteCodeableConcept")) { 830 this.site = new CodeableConcept(); 831 return this.site; 832 } else if (name.equals("siteReference")) { 833 this.site = new Reference(); 834 return this.site; 835 } else if (name.equals("route")) { 836 this.route = new CodeableConcept(); 837 return this.route; 838 } else if (name.equals("method")) { 839 this.method = new CodeableConcept(); 840 return this.method; 841 } else if (name.equals("quantitySimpleQuantity")) { 842 this.quantity = new SimpleQuantity(); 843 return this.quantity; 844 } else if (name.equals("quantityRange")) { 845 this.quantity = new Range(); 846 return this.quantity; 847 } else if (name.equals("rateRatio")) { 848 this.rate = new Ratio(); 849 return this.rate; 850 } else if (name.equals("rateRange")) { 851 this.rate = new Range(); 852 return this.rate; 853 } else if (name.equals("maxDosePerPeriod")) { 854 this.maxDosePerPeriod = new Ratio(); 855 return this.maxDosePerPeriod; 856 } else 857 return super.addChild(name); 858 } 859 860 public MedicationStatementDosageComponent copy() { 861 MedicationStatementDosageComponent dst = new MedicationStatementDosageComponent(); 862 copyValues(dst); 863 dst.text = text == null ? null : text.copy(); 864 dst.timing = timing == null ? null : timing.copy(); 865 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 866 dst.site = site == null ? null : site.copy(); 867 dst.route = route == null ? null : route.copy(); 868 dst.method = method == null ? null : method.copy(); 869 dst.quantity = quantity == null ? null : quantity.copy(); 870 dst.rate = rate == null ? null : rate.copy(); 871 dst.maxDosePerPeriod = maxDosePerPeriod == null ? null : maxDosePerPeriod.copy(); 872 return dst; 873 } 874 875 @Override 876 public boolean equalsDeep(Base other) { 877 if (!super.equalsDeep(other)) 878 return false; 879 if (!(other instanceof MedicationStatementDosageComponent)) 880 return false; 881 MedicationStatementDosageComponent o = (MedicationStatementDosageComponent) other; 882 return compareDeep(text, o.text, true) && compareDeep(timing, o.timing, true) 883 && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(site, o.site, true) 884 && compareDeep(route, o.route, true) && compareDeep(method, o.method, true) 885 && compareDeep(quantity, o.quantity, true) && compareDeep(rate, o.rate, true) 886 && compareDeep(maxDosePerPeriod, o.maxDosePerPeriod, true); 887 } 888 889 @Override 890 public boolean equalsShallow(Base other) { 891 if (!super.equalsShallow(other)) 892 return false; 893 if (!(other instanceof MedicationStatementDosageComponent)) 894 return false; 895 MedicationStatementDosageComponent o = (MedicationStatementDosageComponent) other; 896 return compareValues(text, o.text, true); 897 } 898 899 public boolean isEmpty() { 900 return super.isEmpty() && (text == null || text.isEmpty()) && (timing == null || timing.isEmpty()) 901 && (asNeeded == null || asNeeded.isEmpty()) && (site == null || site.isEmpty()) 902 && (route == null || route.isEmpty()) && (method == null || method.isEmpty()) 903 && (quantity == null || quantity.isEmpty()) && (rate == null || rate.isEmpty()) 904 && (maxDosePerPeriod == null || maxDosePerPeriod.isEmpty()); 905 } 906 907 public String fhirType() { 908 return "MedicationStatement.dosage"; 909 910 } 911 912 } 913 914 /** 915 * External identifier - FHIR will generate its own internal identifiers 916 * (probably URLs) which do not need to be explicitly managed by the resource. 917 * The identifier here is one that would be used by another non-FHIR system - 918 * for example an automated medication pump would provide a record each time it 919 * operated; an administration while the patient was off the ward might be made 920 * with a different system and entered after the event. Particularly important 921 * if these records have to be updated. 922 */ 923 @Child(name = "identifier", type = { 924 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 925 @Description(shortDefinition = "External identifier", formalDefinition = "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.") 926 protected List<Identifier> identifier; 927 928 /** 929 * The person or animal who is/was taking the medication. 930 */ 931 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 932 @Description(shortDefinition = "Who is/was taking the medication", formalDefinition = "The person or animal who is/was taking the medication.") 933 protected Reference patient; 934 935 /** 936 * The actual object that is the target of the reference (The person or animal 937 * who is/was taking the medication.) 938 */ 939 protected Patient patientTarget; 940 941 /** 942 * The person who provided the information about the taking of this medication. 943 */ 944 @Child(name = "informationSource", type = { Patient.class, Practitioner.class, 945 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 946 @Description(shortDefinition = "", formalDefinition = "The person who provided the information about the taking of this medication.") 947 protected Reference informationSource; 948 949 /** 950 * The actual object that is the target of the reference (The person who 951 * provided the information about the taking of this medication.) 952 */ 953 protected Resource informationSourceTarget; 954 955 /** 956 * The date when the medication statement was asserted by the information 957 * source. 958 */ 959 @Child(name = "dateAsserted", type = { 960 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 961 @Description(shortDefinition = "When the statement was asserted?", formalDefinition = "The date when the medication statement was asserted by the information source.") 962 protected DateTimeType dateAsserted; 963 964 /** 965 * A code representing the patient or other source's judgment about the state of 966 * the medication used that this statement is about. Generally this will be 967 * active or completed. 968 */ 969 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 970 @Description(shortDefinition = "active | completed | entered-in-error | intended", formalDefinition = "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.") 971 protected Enumeration<MedicationStatementStatus> status; 972 973 /** 974 * Set this to true if the record is saying that the medication was NOT taken. 975 */ 976 @Child(name = "wasNotTaken", type = { 977 BooleanType.class }, order = 5, min = 0, max = 1, modifier = true, summary = true) 978 @Description(shortDefinition = "True if medication is/was not being taken", formalDefinition = "Set this to true if the record is saying that the medication was NOT taken.") 979 protected BooleanType wasNotTaken; 980 981 /** 982 * A code indicating why the medication was not taken. 983 */ 984 @Child(name = "reasonNotTaken", type = { 985 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 986 @Description(shortDefinition = "True if asserting medication was not given", formalDefinition = "A code indicating why the medication was not taken.") 987 protected List<CodeableConcept> reasonNotTaken; 988 989 /** 990 * A reason for why the medication is being/was taken. 991 */ 992 @Child(name = "reasonForUse", type = { CodeableConcept.class, 993 Condition.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 994 @Description(shortDefinition = "", formalDefinition = "A reason for why the medication is being/was taken.") 995 protected Type reasonForUse; 996 997 /** 998 * The interval of time during which it is being asserted that the patient was 999 * taking the medication (or was not taking, when the wasNotGiven element is 1000 * true). 1001 */ 1002 @Child(name = "effective", type = { DateTimeType.class, 1003 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1004 @Description(shortDefinition = "Over what period was medication consumed?", formalDefinition = "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).") 1005 protected Type effective; 1006 1007 /** 1008 * Provides extra information about the medication statement that is not 1009 * conveyed by the other attributes. 1010 */ 1011 @Child(name = "note", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1012 @Description(shortDefinition = "Further information about the statement", formalDefinition = "Provides extra information about the medication statement that is not conveyed by the other attributes.") 1013 protected StringType note; 1014 1015 /** 1016 * Allows linking the MedicationStatement to the underlying MedicationOrder, or 1017 * to other information that supports the MedicationStatement. 1018 */ 1019 @Child(name = "supportingInformation", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1020 @Description(shortDefinition = "Additional supporting information", formalDefinition = "Allows linking the MedicationStatement to the underlying MedicationOrder, or to other information that supports the MedicationStatement.") 1021 protected List<Reference> supportingInformation; 1022 /** 1023 * The actual objects that are the target of the reference (Allows linking the 1024 * MedicationStatement to the underlying MedicationOrder, or to other 1025 * information that supports the MedicationStatement.) 1026 */ 1027 protected List<Resource> supportingInformationTarget; 1028 1029 /** 1030 * Identifies the medication being administered. This is either a link to a 1031 * resource representing the details of the medication or a simple attribute 1032 * carrying a code that identifies the medication from a known list of 1033 * medications. 1034 */ 1035 @Child(name = "medication", type = { CodeableConcept.class, 1036 Medication.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 1037 @Description(shortDefinition = "What medication was taken", formalDefinition = "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 1038 protected Type medication; 1039 1040 /** 1041 * Indicates how the medication is/was used by the patient. 1042 */ 1043 @Child(name = "dosage", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1044 @Description(shortDefinition = "Details of how medication was taken", formalDefinition = "Indicates how the medication is/was used by the patient.") 1045 protected List<MedicationStatementDosageComponent> dosage; 1046 1047 private static final long serialVersionUID = 55795672L; 1048 1049 /* 1050 * Constructor 1051 */ 1052 public MedicationStatement() { 1053 super(); 1054 } 1055 1056 /* 1057 * Constructor 1058 */ 1059 public MedicationStatement(Reference patient, Enumeration<MedicationStatementStatus> status, Type medication) { 1060 super(); 1061 this.patient = patient; 1062 this.status = status; 1063 this.medication = medication; 1064 } 1065 1066 /** 1067 * @return {@link #identifier} (External identifier - FHIR will generate its own 1068 * internal identifiers (probably URLs) which do not need to be 1069 * explicitly managed by the resource. The identifier here is one that 1070 * would be used by another non-FHIR system - for example an automated 1071 * medication pump would provide a record each time it operated; an 1072 * administration while the patient was off the ward might be made with 1073 * a different system and entered after the event. Particularly 1074 * important if these records have to be updated.) 1075 */ 1076 public List<Identifier> getIdentifier() { 1077 if (this.identifier == null) 1078 this.identifier = new ArrayList<Identifier>(); 1079 return this.identifier; 1080 } 1081 1082 public boolean hasIdentifier() { 1083 if (this.identifier == null) 1084 return false; 1085 for (Identifier item : this.identifier) 1086 if (!item.isEmpty()) 1087 return true; 1088 return false; 1089 } 1090 1091 /** 1092 * @return {@link #identifier} (External identifier - FHIR will generate its own 1093 * internal identifiers (probably URLs) which do not need to be 1094 * explicitly managed by the resource. The identifier here is one that 1095 * would be used by another non-FHIR system - for example an automated 1096 * medication pump would provide a record each time it operated; an 1097 * administration while the patient was off the ward might be made with 1098 * a different system and entered after the event. Particularly 1099 * important if these records have to be updated.) 1100 */ 1101 // syntactic sugar 1102 public Identifier addIdentifier() { // 3 1103 Identifier t = new Identifier(); 1104 if (this.identifier == null) 1105 this.identifier = new ArrayList<Identifier>(); 1106 this.identifier.add(t); 1107 return t; 1108 } 1109 1110 // syntactic sugar 1111 public MedicationStatement addIdentifier(Identifier t) { // 3 1112 if (t == null) 1113 return this; 1114 if (this.identifier == null) 1115 this.identifier = new ArrayList<Identifier>(); 1116 this.identifier.add(t); 1117 return this; 1118 } 1119 1120 /** 1121 * @return {@link #patient} (The person or animal who is/was taking the 1122 * medication.) 1123 */ 1124 public Reference getPatient() { 1125 if (this.patient == null) 1126 if (Configuration.errorOnAutoCreate()) 1127 throw new Error("Attempt to auto-create MedicationStatement.patient"); 1128 else if (Configuration.doAutoCreate()) 1129 this.patient = new Reference(); // cc 1130 return this.patient; 1131 } 1132 1133 public boolean hasPatient() { 1134 return this.patient != null && !this.patient.isEmpty(); 1135 } 1136 1137 /** 1138 * @param value {@link #patient} (The person or animal who is/was taking the 1139 * medication.) 1140 */ 1141 public MedicationStatement setPatient(Reference value) { 1142 this.patient = value; 1143 return this; 1144 } 1145 1146 /** 1147 * @return {@link #patient} The actual object that is the target of the 1148 * reference. The reference library doesn't populate this, but you can 1149 * use it to hold the resource if you resolve it. (The person or animal 1150 * who is/was taking the medication.) 1151 */ 1152 public Patient getPatientTarget() { 1153 if (this.patientTarget == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create MedicationStatement.patient"); 1156 else if (Configuration.doAutoCreate()) 1157 this.patientTarget = new Patient(); // aa 1158 return this.patientTarget; 1159 } 1160 1161 /** 1162 * @param value {@link #patient} The actual object that is the target of the 1163 * reference. The reference library doesn't use these, but you can 1164 * use it to hold the resource if you resolve it. (The person or 1165 * animal who is/was taking the medication.) 1166 */ 1167 public MedicationStatement setPatientTarget(Patient value) { 1168 this.patientTarget = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #informationSource} (The person who provided the information 1174 * about the taking of this medication.) 1175 */ 1176 public Reference getInformationSource() { 1177 if (this.informationSource == null) 1178 if (Configuration.errorOnAutoCreate()) 1179 throw new Error("Attempt to auto-create MedicationStatement.informationSource"); 1180 else if (Configuration.doAutoCreate()) 1181 this.informationSource = new Reference(); // cc 1182 return this.informationSource; 1183 } 1184 1185 public boolean hasInformationSource() { 1186 return this.informationSource != null && !this.informationSource.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #informationSource} (The person who provided the 1191 * information about the taking of this medication.) 1192 */ 1193 public MedicationStatement setInformationSource(Reference value) { 1194 this.informationSource = value; 1195 return this; 1196 } 1197 1198 /** 1199 * @return {@link #informationSource} The actual object that is the target of 1200 * the reference. The reference library doesn't populate this, but you 1201 * can use it to hold the resource if you resolve it. (The person who 1202 * provided the information about the taking of this medication.) 1203 */ 1204 public Resource getInformationSourceTarget() { 1205 return this.informationSourceTarget; 1206 } 1207 1208 /** 1209 * @param value {@link #informationSource} The actual object that is the target 1210 * of the reference. The reference library doesn't use these, but 1211 * you can use it to hold the resource if you resolve it. (The 1212 * person who provided the information about the taking of this 1213 * medication.) 1214 */ 1215 public MedicationStatement setInformationSourceTarget(Resource value) { 1216 this.informationSourceTarget = value; 1217 return this; 1218 } 1219 1220 /** 1221 * @return {@link #dateAsserted} (The date when the medication statement was 1222 * asserted by the information source.). This is the underlying object 1223 * with id, value and extensions. The accessor "getDateAsserted" gives 1224 * direct access to the value 1225 */ 1226 public DateTimeType getDateAssertedElement() { 1227 if (this.dateAsserted == null) 1228 if (Configuration.errorOnAutoCreate()) 1229 throw new Error("Attempt to auto-create MedicationStatement.dateAsserted"); 1230 else if (Configuration.doAutoCreate()) 1231 this.dateAsserted = new DateTimeType(); // bb 1232 return this.dateAsserted; 1233 } 1234 1235 public boolean hasDateAssertedElement() { 1236 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 1237 } 1238 1239 public boolean hasDateAsserted() { 1240 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 1241 } 1242 1243 /** 1244 * @param value {@link #dateAsserted} (The date when the medication statement 1245 * was asserted by the information source.). This is the underlying 1246 * object with id, value and extensions. The accessor 1247 * "getDateAsserted" gives direct access to the value 1248 */ 1249 public MedicationStatement setDateAssertedElement(DateTimeType value) { 1250 this.dateAsserted = value; 1251 return this; 1252 } 1253 1254 /** 1255 * @return The date when the medication statement was asserted by the 1256 * information source. 1257 */ 1258 public Date getDateAsserted() { 1259 return this.dateAsserted == null ? null : this.dateAsserted.getValue(); 1260 } 1261 1262 /** 1263 * @param value The date when the medication statement was asserted by the 1264 * information source. 1265 */ 1266 public MedicationStatement setDateAsserted(Date value) { 1267 if (value == null) 1268 this.dateAsserted = null; 1269 else { 1270 if (this.dateAsserted == null) 1271 this.dateAsserted = new DateTimeType(); 1272 this.dateAsserted.setValue(value); 1273 } 1274 return this; 1275 } 1276 1277 /** 1278 * @return {@link #status} (A code representing the patient or other source's 1279 * judgment about the state of the medication used that this statement 1280 * is about. Generally this will be active or completed.). This is the 1281 * underlying object with id, value and extensions. The accessor 1282 * "getStatus" gives direct access to the value 1283 */ 1284 public Enumeration<MedicationStatementStatus> getStatusElement() { 1285 if (this.status == null) 1286 if (Configuration.errorOnAutoCreate()) 1287 throw new Error("Attempt to auto-create MedicationStatement.status"); 1288 else if (Configuration.doAutoCreate()) 1289 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); // bb 1290 return this.status; 1291 } 1292 1293 public boolean hasStatusElement() { 1294 return this.status != null && !this.status.isEmpty(); 1295 } 1296 1297 public boolean hasStatus() { 1298 return this.status != null && !this.status.isEmpty(); 1299 } 1300 1301 /** 1302 * @param value {@link #status} (A code representing the patient or other 1303 * source's judgment about the state of the medication used that 1304 * this statement is about. Generally this will be active or 1305 * completed.). This is the underlying object with id, value and 1306 * extensions. The accessor "getStatus" gives direct access to the 1307 * value 1308 */ 1309 public MedicationStatement setStatusElement(Enumeration<MedicationStatementStatus> value) { 1310 this.status = value; 1311 return this; 1312 } 1313 1314 /** 1315 * @return A code representing the patient or other source's judgment about the 1316 * state of the medication used that this statement is about. Generally 1317 * this will be active or completed. 1318 */ 1319 public MedicationStatementStatus getStatus() { 1320 return this.status == null ? null : this.status.getValue(); 1321 } 1322 1323 /** 1324 * @param value A code representing the patient or other source's judgment about 1325 * the state of the medication used that this statement is about. 1326 * Generally this will be active or completed. 1327 */ 1328 public MedicationStatement setStatus(MedicationStatementStatus value) { 1329 if (this.status == null) 1330 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); 1331 this.status.setValue(value); 1332 return this; 1333 } 1334 1335 /** 1336 * @return {@link #wasNotTaken} (Set this to true if the record is saying that 1337 * the medication was NOT taken.). This is the underlying object with 1338 * id, value and extensions. The accessor "getWasNotTaken" gives direct 1339 * access to the value 1340 */ 1341 public BooleanType getWasNotTakenElement() { 1342 if (this.wasNotTaken == null) 1343 if (Configuration.errorOnAutoCreate()) 1344 throw new Error("Attempt to auto-create MedicationStatement.wasNotTaken"); 1345 else if (Configuration.doAutoCreate()) 1346 this.wasNotTaken = new BooleanType(); // bb 1347 return this.wasNotTaken; 1348 } 1349 1350 public boolean hasWasNotTakenElement() { 1351 return this.wasNotTaken != null && !this.wasNotTaken.isEmpty(); 1352 } 1353 1354 public boolean hasWasNotTaken() { 1355 return this.wasNotTaken != null && !this.wasNotTaken.isEmpty(); 1356 } 1357 1358 /** 1359 * @param value {@link #wasNotTaken} (Set this to true if the record is saying 1360 * that the medication was NOT taken.). This is the underlying 1361 * object with id, value and extensions. The accessor 1362 * "getWasNotTaken" gives direct access to the value 1363 */ 1364 public MedicationStatement setWasNotTakenElement(BooleanType value) { 1365 this.wasNotTaken = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return Set this to true if the record is saying that the medication was NOT 1371 * taken. 1372 */ 1373 public boolean getWasNotTaken() { 1374 return this.wasNotTaken == null || this.wasNotTaken.isEmpty() ? false : this.wasNotTaken.getValue(); 1375 } 1376 1377 /** 1378 * @param value Set this to true if the record is saying that the medication was 1379 * NOT taken. 1380 */ 1381 public MedicationStatement setWasNotTaken(boolean value) { 1382 if (this.wasNotTaken == null) 1383 this.wasNotTaken = new BooleanType(); 1384 this.wasNotTaken.setValue(value); 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #reasonNotTaken} (A code indicating why the medication was not 1390 * taken.) 1391 */ 1392 public List<CodeableConcept> getReasonNotTaken() { 1393 if (this.reasonNotTaken == null) 1394 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1395 return this.reasonNotTaken; 1396 } 1397 1398 public boolean hasReasonNotTaken() { 1399 if (this.reasonNotTaken == null) 1400 return false; 1401 for (CodeableConcept item : this.reasonNotTaken) 1402 if (!item.isEmpty()) 1403 return true; 1404 return false; 1405 } 1406 1407 /** 1408 * @return {@link #reasonNotTaken} (A code indicating why the medication was not 1409 * taken.) 1410 */ 1411 // syntactic sugar 1412 public CodeableConcept addReasonNotTaken() { // 3 1413 CodeableConcept t = new CodeableConcept(); 1414 if (this.reasonNotTaken == null) 1415 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1416 this.reasonNotTaken.add(t); 1417 return t; 1418 } 1419 1420 // syntactic sugar 1421 public MedicationStatement addReasonNotTaken(CodeableConcept t) { // 3 1422 if (t == null) 1423 return this; 1424 if (this.reasonNotTaken == null) 1425 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1426 this.reasonNotTaken.add(t); 1427 return this; 1428 } 1429 1430 /** 1431 * @return {@link #reasonForUse} (A reason for why the medication is being/was 1432 * taken.) 1433 */ 1434 public Type getReasonForUse() { 1435 return this.reasonForUse; 1436 } 1437 1438 /** 1439 * @return {@link #reasonForUse} (A reason for why the medication is being/was 1440 * taken.) 1441 */ 1442 public CodeableConcept getReasonForUseCodeableConcept() throws FHIRException { 1443 if (!(this.reasonForUse instanceof CodeableConcept)) 1444 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1445 + this.reasonForUse.getClass().getName() + " was encountered"); 1446 return (CodeableConcept) this.reasonForUse; 1447 } 1448 1449 public boolean hasReasonForUseCodeableConcept() { 1450 return this.reasonForUse instanceof CodeableConcept; 1451 } 1452 1453 /** 1454 * @return {@link #reasonForUse} (A reason for why the medication is being/was 1455 * taken.) 1456 */ 1457 public Reference getReasonForUseReference() throws FHIRException { 1458 if (!(this.reasonForUse instanceof Reference)) 1459 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1460 + this.reasonForUse.getClass().getName() + " was encountered"); 1461 return (Reference) this.reasonForUse; 1462 } 1463 1464 public boolean hasReasonForUseReference() { 1465 return this.reasonForUse instanceof Reference; 1466 } 1467 1468 public boolean hasReasonForUse() { 1469 return this.reasonForUse != null && !this.reasonForUse.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #reasonForUse} (A reason for why the medication is 1474 * being/was taken.) 1475 */ 1476 public MedicationStatement setReasonForUse(Type value) { 1477 this.reasonForUse = value; 1478 return this; 1479 } 1480 1481 /** 1482 * @return {@link #effective} (The interval of time during which it is being 1483 * asserted that the patient was taking the medication (or was not 1484 * taking, when the wasNotGiven element is true).) 1485 */ 1486 public Type getEffective() { 1487 return this.effective; 1488 } 1489 1490 /** 1491 * @return {@link #effective} (The interval of time during which it is being 1492 * asserted that the patient was taking the medication (or was not 1493 * taking, when the wasNotGiven element is true).) 1494 */ 1495 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1496 if (!(this.effective instanceof DateTimeType)) 1497 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1498 + this.effective.getClass().getName() + " was encountered"); 1499 return (DateTimeType) this.effective; 1500 } 1501 1502 public boolean hasEffectiveDateTimeType() { 1503 return this.effective instanceof DateTimeType; 1504 } 1505 1506 /** 1507 * @return {@link #effective} (The interval of time during which it is being 1508 * asserted that the patient was taking the medication (or was not 1509 * taking, when the wasNotGiven element is true).) 1510 */ 1511 public Period getEffectivePeriod() throws FHIRException { 1512 if (!(this.effective instanceof Period)) 1513 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1514 + " was encountered"); 1515 return (Period) this.effective; 1516 } 1517 1518 public boolean hasEffectivePeriod() { 1519 return this.effective instanceof Period; 1520 } 1521 1522 public boolean hasEffective() { 1523 return this.effective != null && !this.effective.isEmpty(); 1524 } 1525 1526 /** 1527 * @param value {@link #effective} (The interval of time during which it is 1528 * being asserted that the patient was taking the medication (or 1529 * was not taking, when the wasNotGiven element is true).) 1530 */ 1531 public MedicationStatement setEffective(Type value) { 1532 this.effective = value; 1533 return this; 1534 } 1535 1536 /** 1537 * @return {@link #note} (Provides extra information about the medication 1538 * statement that is not conveyed by the other attributes.). This is the 1539 * underlying object with id, value and extensions. The accessor 1540 * "getNote" gives direct access to the value 1541 */ 1542 public StringType getNoteElement() { 1543 if (this.note == null) 1544 if (Configuration.errorOnAutoCreate()) 1545 throw new Error("Attempt to auto-create MedicationStatement.note"); 1546 else if (Configuration.doAutoCreate()) 1547 this.note = new StringType(); // bb 1548 return this.note; 1549 } 1550 1551 public boolean hasNoteElement() { 1552 return this.note != null && !this.note.isEmpty(); 1553 } 1554 1555 public boolean hasNote() { 1556 return this.note != null && !this.note.isEmpty(); 1557 } 1558 1559 /** 1560 * @param value {@link #note} (Provides extra information about the medication 1561 * statement that is not conveyed by the other attributes.). This 1562 * is the underlying object with id, value and extensions. The 1563 * accessor "getNote" gives direct access to the value 1564 */ 1565 public MedicationStatement setNoteElement(StringType value) { 1566 this.note = value; 1567 return this; 1568 } 1569 1570 /** 1571 * @return Provides extra information about the medication statement that is not 1572 * conveyed by the other attributes. 1573 */ 1574 public String getNote() { 1575 return this.note == null ? null : this.note.getValue(); 1576 } 1577 1578 /** 1579 * @param value Provides extra information about the medication statement that 1580 * is not conveyed by the other attributes. 1581 */ 1582 public MedicationStatement setNote(String value) { 1583 if (Utilities.noString(value)) 1584 this.note = null; 1585 else { 1586 if (this.note == null) 1587 this.note = new StringType(); 1588 this.note.setValue(value); 1589 } 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #supportingInformation} (Allows linking the 1595 * MedicationStatement to the underlying MedicationOrder, or to other 1596 * information that supports the MedicationStatement.) 1597 */ 1598 public List<Reference> getSupportingInformation() { 1599 if (this.supportingInformation == null) 1600 this.supportingInformation = new ArrayList<Reference>(); 1601 return this.supportingInformation; 1602 } 1603 1604 public boolean hasSupportingInformation() { 1605 if (this.supportingInformation == null) 1606 return false; 1607 for (Reference item : this.supportingInformation) 1608 if (!item.isEmpty()) 1609 return true; 1610 return false; 1611 } 1612 1613 /** 1614 * @return {@link #supportingInformation} (Allows linking the 1615 * MedicationStatement to the underlying MedicationOrder, or to other 1616 * information that supports the MedicationStatement.) 1617 */ 1618 // syntactic sugar 1619 public Reference addSupportingInformation() { // 3 1620 Reference t = new Reference(); 1621 if (this.supportingInformation == null) 1622 this.supportingInformation = new ArrayList<Reference>(); 1623 this.supportingInformation.add(t); 1624 return t; 1625 } 1626 1627 // syntactic sugar 1628 public MedicationStatement addSupportingInformation(Reference t) { // 3 1629 if (t == null) 1630 return this; 1631 if (this.supportingInformation == null) 1632 this.supportingInformation = new ArrayList<Reference>(); 1633 this.supportingInformation.add(t); 1634 return this; 1635 } 1636 1637 /** 1638 * @return {@link #supportingInformation} (The actual objects that are the 1639 * target of the reference. The reference library doesn't populate this, 1640 * but you can use this to hold the resources if you resolvethemt. 1641 * Allows linking the MedicationStatement to the underlying 1642 * MedicationOrder, or to other information that supports the 1643 * MedicationStatement.) 1644 */ 1645 public List<Resource> getSupportingInformationTarget() { 1646 if (this.supportingInformationTarget == null) 1647 this.supportingInformationTarget = new ArrayList<Resource>(); 1648 return this.supportingInformationTarget; 1649 } 1650 1651 /** 1652 * @return {@link #medication} (Identifies the medication being administered. 1653 * This is either a link to a resource representing the details of the 1654 * medication or a simple attribute carrying a code that identifies the 1655 * medication from a known list of medications.) 1656 */ 1657 public Type getMedication() { 1658 return this.medication; 1659 } 1660 1661 /** 1662 * @return {@link #medication} (Identifies the medication being administered. 1663 * This is either a link to a resource representing the details of the 1664 * medication or a simple attribute carrying a code that identifies the 1665 * medication from a known list of medications.) 1666 */ 1667 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1668 if (!(this.medication instanceof CodeableConcept)) 1669 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1670 + this.medication.getClass().getName() + " was encountered"); 1671 return (CodeableConcept) this.medication; 1672 } 1673 1674 public boolean hasMedicationCodeableConcept() { 1675 return this.medication instanceof CodeableConcept; 1676 } 1677 1678 /** 1679 * @return {@link #medication} (Identifies the medication being administered. 1680 * This is either a link to a resource representing the details of the 1681 * medication or a simple attribute carrying a code that identifies the 1682 * medication from a known list of medications.) 1683 */ 1684 public Reference getMedicationReference() throws FHIRException { 1685 if (!(this.medication instanceof Reference)) 1686 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1687 + this.medication.getClass().getName() + " was encountered"); 1688 return (Reference) this.medication; 1689 } 1690 1691 public boolean hasMedicationReference() { 1692 return this.medication instanceof Reference; 1693 } 1694 1695 public boolean hasMedication() { 1696 return this.medication != null && !this.medication.isEmpty(); 1697 } 1698 1699 /** 1700 * @param value {@link #medication} (Identifies the medication being 1701 * administered. This is either a link to a resource representing 1702 * the details of the medication or a simple attribute carrying a 1703 * code that identifies the medication from a known list of 1704 * medications.) 1705 */ 1706 public MedicationStatement setMedication(Type value) { 1707 this.medication = value; 1708 return this; 1709 } 1710 1711 /** 1712 * @return {@link #dosage} (Indicates how the medication is/was used by the 1713 * patient.) 1714 */ 1715 public List<MedicationStatementDosageComponent> getDosage() { 1716 if (this.dosage == null) 1717 this.dosage = new ArrayList<MedicationStatementDosageComponent>(); 1718 return this.dosage; 1719 } 1720 1721 public boolean hasDosage() { 1722 if (this.dosage == null) 1723 return false; 1724 for (MedicationStatementDosageComponent item : this.dosage) 1725 if (!item.isEmpty()) 1726 return true; 1727 return false; 1728 } 1729 1730 /** 1731 * @return {@link #dosage} (Indicates how the medication is/was used by the 1732 * patient.) 1733 */ 1734 // syntactic sugar 1735 public MedicationStatementDosageComponent addDosage() { // 3 1736 MedicationStatementDosageComponent t = new MedicationStatementDosageComponent(); 1737 if (this.dosage == null) 1738 this.dosage = new ArrayList<MedicationStatementDosageComponent>(); 1739 this.dosage.add(t); 1740 return t; 1741 } 1742 1743 // syntactic sugar 1744 public MedicationStatement addDosage(MedicationStatementDosageComponent t) { // 3 1745 if (t == null) 1746 return this; 1747 if (this.dosage == null) 1748 this.dosage = new ArrayList<MedicationStatementDosageComponent>(); 1749 this.dosage.add(t); 1750 return this; 1751 } 1752 1753 protected void listChildren(List<Property> childrenList) { 1754 super.listChildren(childrenList); 1755 childrenList.add(new Property("identifier", "Identifier", 1756 "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 1757 0, java.lang.Integer.MAX_VALUE, identifier)); 1758 childrenList.add(new Property("patient", "Reference(Patient)", 1759 "The person or animal who is/was taking the medication.", 0, java.lang.Integer.MAX_VALUE, patient)); 1760 childrenList.add(new Property("informationSource", "Reference(Patient|Practitioner|RelatedPerson)", 1761 "The person who provided the information about the taking of this medication.", 0, java.lang.Integer.MAX_VALUE, 1762 informationSource)); 1763 childrenList.add(new Property("dateAsserted", "dateTime", 1764 "The date when the medication statement was asserted by the information source.", 0, 1765 java.lang.Integer.MAX_VALUE, dateAsserted)); 1766 childrenList.add(new Property("status", "code", 1767 "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.", 1768 0, java.lang.Integer.MAX_VALUE, status)); 1769 childrenList.add(new Property("wasNotTaken", "boolean", 1770 "Set this to true if the record is saying that the medication was NOT taken.", 0, java.lang.Integer.MAX_VALUE, 1771 wasNotTaken)); 1772 childrenList.add(new Property("reasonNotTaken", "CodeableConcept", 1773 "A code indicating why the medication was not taken.", 0, java.lang.Integer.MAX_VALUE, reasonNotTaken)); 1774 childrenList.add(new Property("reasonForUse[x]", "CodeableConcept|Reference(Condition)", 1775 "A reason for why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonForUse)); 1776 childrenList.add(new Property("effective[x]", "dateTime|Period", 1777 "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 1778 0, java.lang.Integer.MAX_VALUE, effective)); 1779 childrenList.add(new Property("note", "string", 1780 "Provides extra information about the medication statement that is not conveyed by the other attributes.", 0, 1781 java.lang.Integer.MAX_VALUE, note)); 1782 childrenList.add(new Property("supportingInformation", "Reference(Any)", 1783 "Allows linking the MedicationStatement to the underlying MedicationOrder, or to other information that supports the MedicationStatement.", 1784 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 1785 childrenList.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1786 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1787 0, java.lang.Integer.MAX_VALUE, medication)); 1788 childrenList.add(new Property("dosage", "", "Indicates how the medication is/was used by the patient.", 0, 1789 java.lang.Integer.MAX_VALUE, dosage)); 1790 } 1791 1792 @Override 1793 public void setProperty(String name, Base value) throws FHIRException { 1794 if (name.equals("identifier")) 1795 this.getIdentifier().add(castToIdentifier(value)); 1796 else if (name.equals("patient")) 1797 this.patient = castToReference(value); // Reference 1798 else if (name.equals("informationSource")) 1799 this.informationSource = castToReference(value); // Reference 1800 else if (name.equals("dateAsserted")) 1801 this.dateAsserted = castToDateTime(value); // DateTimeType 1802 else if (name.equals("status")) 1803 this.status = new MedicationStatementStatusEnumFactory().fromType(value); // Enumeration<MedicationStatementStatus> 1804 else if (name.equals("wasNotTaken")) 1805 this.wasNotTaken = castToBoolean(value); // BooleanType 1806 else if (name.equals("reasonNotTaken")) 1807 this.getReasonNotTaken().add(castToCodeableConcept(value)); 1808 else if (name.equals("reasonForUse[x]")) 1809 this.reasonForUse = (Type) value; // Type 1810 else if (name.equals("effective[x]")) 1811 this.effective = (Type) value; // Type 1812 else if (name.equals("note")) 1813 this.note = castToString(value); // StringType 1814 else if (name.equals("supportingInformation")) 1815 this.getSupportingInformation().add(castToReference(value)); 1816 else if (name.equals("medication[x]")) 1817 this.medication = (Type) value; // Type 1818 else if (name.equals("dosage")) 1819 this.getDosage().add((MedicationStatementDosageComponent) value); 1820 else 1821 super.setProperty(name, value); 1822 } 1823 1824 @Override 1825 public Base addChild(String name) throws FHIRException { 1826 if (name.equals("identifier")) { 1827 return addIdentifier(); 1828 } else if (name.equals("patient")) { 1829 this.patient = new Reference(); 1830 return this.patient; 1831 } else if (name.equals("informationSource")) { 1832 this.informationSource = new Reference(); 1833 return this.informationSource; 1834 } else if (name.equals("dateAsserted")) { 1835 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.dateAsserted"); 1836 } else if (name.equals("status")) { 1837 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.status"); 1838 } else if (name.equals("wasNotTaken")) { 1839 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.wasNotTaken"); 1840 } else if (name.equals("reasonNotTaken")) { 1841 return addReasonNotTaken(); 1842 } else if (name.equals("reasonForUseCodeableConcept")) { 1843 this.reasonForUse = new CodeableConcept(); 1844 return this.reasonForUse; 1845 } else if (name.equals("reasonForUseReference")) { 1846 this.reasonForUse = new Reference(); 1847 return this.reasonForUse; 1848 } else if (name.equals("effectiveDateTime")) { 1849 this.effective = new DateTimeType(); 1850 return this.effective; 1851 } else if (name.equals("effectivePeriod")) { 1852 this.effective = new Period(); 1853 return this.effective; 1854 } else if (name.equals("note")) { 1855 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.note"); 1856 } else if (name.equals("supportingInformation")) { 1857 return addSupportingInformation(); 1858 } else if (name.equals("medicationCodeableConcept")) { 1859 this.medication = new CodeableConcept(); 1860 return this.medication; 1861 } else if (name.equals("medicationReference")) { 1862 this.medication = new Reference(); 1863 return this.medication; 1864 } else if (name.equals("dosage")) { 1865 return addDosage(); 1866 } else 1867 return super.addChild(name); 1868 } 1869 1870 public String fhirType() { 1871 return "MedicationStatement"; 1872 1873 } 1874 1875 public MedicationStatement copy() { 1876 MedicationStatement dst = new MedicationStatement(); 1877 copyValues(dst); 1878 if (identifier != null) { 1879 dst.identifier = new ArrayList<Identifier>(); 1880 for (Identifier i : identifier) 1881 dst.identifier.add(i.copy()); 1882 } 1883 ; 1884 dst.patient = patient == null ? null : patient.copy(); 1885 dst.informationSource = informationSource == null ? null : informationSource.copy(); 1886 dst.dateAsserted = dateAsserted == null ? null : dateAsserted.copy(); 1887 dst.status = status == null ? null : status.copy(); 1888 dst.wasNotTaken = wasNotTaken == null ? null : wasNotTaken.copy(); 1889 if (reasonNotTaken != null) { 1890 dst.reasonNotTaken = new ArrayList<CodeableConcept>(); 1891 for (CodeableConcept i : reasonNotTaken) 1892 dst.reasonNotTaken.add(i.copy()); 1893 } 1894 ; 1895 dst.reasonForUse = reasonForUse == null ? null : reasonForUse.copy(); 1896 dst.effective = effective == null ? null : effective.copy(); 1897 dst.note = note == null ? null : note.copy(); 1898 if (supportingInformation != null) { 1899 dst.supportingInformation = new ArrayList<Reference>(); 1900 for (Reference i : supportingInformation) 1901 dst.supportingInformation.add(i.copy()); 1902 } 1903 ; 1904 dst.medication = medication == null ? null : medication.copy(); 1905 if (dosage != null) { 1906 dst.dosage = new ArrayList<MedicationStatementDosageComponent>(); 1907 for (MedicationStatementDosageComponent i : dosage) 1908 dst.dosage.add(i.copy()); 1909 } 1910 ; 1911 return dst; 1912 } 1913 1914 protected MedicationStatement typedCopy() { 1915 return copy(); 1916 } 1917 1918 @Override 1919 public boolean equalsDeep(Base other) { 1920 if (!super.equalsDeep(other)) 1921 return false; 1922 if (!(other instanceof MedicationStatement)) 1923 return false; 1924 MedicationStatement o = (MedicationStatement) other; 1925 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) 1926 && compareDeep(informationSource, o.informationSource, true) && compareDeep(dateAsserted, o.dateAsserted, true) 1927 && compareDeep(status, o.status, true) && compareDeep(wasNotTaken, o.wasNotTaken, true) 1928 && compareDeep(reasonNotTaken, o.reasonNotTaken, true) && compareDeep(reasonForUse, o.reasonForUse, true) 1929 && compareDeep(effective, o.effective, true) && compareDeep(note, o.note, true) 1930 && compareDeep(supportingInformation, o.supportingInformation, true) 1931 && compareDeep(medication, o.medication, true) && compareDeep(dosage, o.dosage, true); 1932 } 1933 1934 @Override 1935 public boolean equalsShallow(Base other) { 1936 if (!super.equalsShallow(other)) 1937 return false; 1938 if (!(other instanceof MedicationStatement)) 1939 return false; 1940 MedicationStatement o = (MedicationStatement) other; 1941 return compareValues(dateAsserted, o.dateAsserted, true) && compareValues(status, o.status, true) 1942 && compareValues(wasNotTaken, o.wasNotTaken, true) && compareValues(note, o.note, true); 1943 } 1944 1945 public boolean isEmpty() { 1946 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (patient == null || patient.isEmpty()) 1947 && (informationSource == null || informationSource.isEmpty()) 1948 && (dateAsserted == null || dateAsserted.isEmpty()) && (status == null || status.isEmpty()) 1949 && (wasNotTaken == null || wasNotTaken.isEmpty()) && (reasonNotTaken == null || reasonNotTaken.isEmpty()) 1950 && (reasonForUse == null || reasonForUse.isEmpty()) && (effective == null || effective.isEmpty()) 1951 && (note == null || note.isEmpty()) && (supportingInformation == null || supportingInformation.isEmpty()) 1952 && (medication == null || medication.isEmpty()) && (dosage == null || dosage.isEmpty()); 1953 } 1954 1955 @Override 1956 public ResourceType getResourceType() { 1957 return ResourceType.MedicationStatement; 1958 } 1959 1960 @SearchParamDefinition(name = "identifier", path = "MedicationStatement.identifier", description = "Return statements with this external identifier", type = "token") 1961 public static final String SP_IDENTIFIER = "identifier"; 1962 @SearchParamDefinition(name = "code", path = "MedicationStatement.medicationCodeableConcept", description = "Return administrations of this medication code", type = "token") 1963 public static final String SP_CODE = "code"; 1964 @SearchParamDefinition(name = "patient", path = "MedicationStatement.patient", description = "The identity of a patient to list statements for", type = "reference") 1965 public static final String SP_PATIENT = "patient"; 1966 @SearchParamDefinition(name = "medication", path = "MedicationStatement.medicationReference", description = "Return administrations of this medication reference", type = "reference") 1967 public static final String SP_MEDICATION = "medication"; 1968 @SearchParamDefinition(name = "source", path = "MedicationStatement.informationSource", description = "Who the information in the statement came from", type = "reference") 1969 public static final String SP_SOURCE = "source"; 1970 @SearchParamDefinition(name = "effectivedate", path = "MedicationStatement.effective[x]", description = "Date when patient was taking (or not taking) the medication", type = "date") 1971 public static final String SP_EFFECTIVEDATE = "effectivedate"; 1972 @SearchParamDefinition(name = "status", path = "MedicationStatement.status", description = "Return statements that match the given status", type = "token") 1973 public static final String SP_STATUS = "status"; 1974 1975}