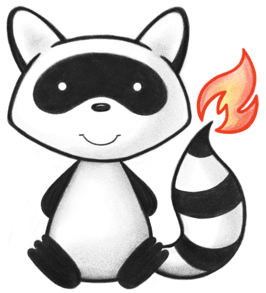
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * The header for a message exchange that is either requesting or responding to 048 * an action. The reference(s) that are the subject of the action as well as 049 * other information related to the action are typically transmitted in a bundle 050 * in which the MessageHeader resource instance is the first resource in the 051 * bundle. 052 */ 053@ResourceDef(name = "MessageHeader", profile = "http://hl7.org/fhir/Profile/MessageHeader") 054public class MessageHeader extends DomainResource { 055 056 public enum ResponseType { 057 /** 058 * The message was accepted and processed without error. 059 */ 060 OK, 061 /** 062 * Some internal unexpected error occurred - wait and try again. Note - this is 063 * usually used for things like database unavailable, which may be expected to 064 * resolve, though human intervention may be required. 065 */ 066 TRANSIENTERROR, 067 /** 068 * The message was rejected because of some content in it. There is no point in 069 * re-sending without change. The response narrative SHALL describe the issue. 070 */ 071 FATALERROR, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static ResponseType fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("ok".equals(codeString)) 081 return OK; 082 if ("transient-error".equals(codeString)) 083 return TRANSIENTERROR; 084 if ("fatal-error".equals(codeString)) 085 return FATALERROR; 086 throw new FHIRException("Unknown ResponseType code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case OK: 092 return "ok"; 093 case TRANSIENTERROR: 094 return "transient-error"; 095 case FATALERROR: 096 return "fatal-error"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case OK: 107 return "http://hl7.org/fhir/response-code"; 108 case TRANSIENTERROR: 109 return "http://hl7.org/fhir/response-code"; 110 case FATALERROR: 111 return "http://hl7.org/fhir/response-code"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case OK: 122 return "The message was accepted and processed without error."; 123 case TRANSIENTERROR: 124 return "Some internal unexpected error occurred - wait and try again. Note - this is usually used for things like database unavailable, which may be expected to resolve, though human intervention may be required."; 125 case FATALERROR: 126 return "The message was rejected because of some content in it. There is no point in re-sending without change. The response narrative SHALL describe the issue."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case OK: 137 return "OK"; 138 case TRANSIENTERROR: 139 return "Transient Error"; 140 case FATALERROR: 141 return "Fatal Error"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class ResponseTypeEnumFactory implements EnumFactory<ResponseType> { 151 public ResponseType fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("ok".equals(codeString)) 156 return ResponseType.OK; 157 if ("transient-error".equals(codeString)) 158 return ResponseType.TRANSIENTERROR; 159 if ("fatal-error".equals(codeString)) 160 return ResponseType.FATALERROR; 161 throw new IllegalArgumentException("Unknown ResponseType code '" + codeString + "'"); 162 } 163 164 public Enumeration<ResponseType> fromType(Base code) throws FHIRException { 165 if (code == null || code.isEmpty()) 166 return null; 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("ok".equals(codeString)) 171 return new Enumeration<ResponseType>(this, ResponseType.OK); 172 if ("transient-error".equals(codeString)) 173 return new Enumeration<ResponseType>(this, ResponseType.TRANSIENTERROR); 174 if ("fatal-error".equals(codeString)) 175 return new Enumeration<ResponseType>(this, ResponseType.FATALERROR); 176 throw new FHIRException("Unknown ResponseType code '" + codeString + "'"); 177 } 178 179 public String toCode(ResponseType code) 180 { 181 if (code == ResponseType.NULL) 182 return null; 183 if (code == ResponseType.OK) 184 return "ok"; 185 if (code == ResponseType.TRANSIENTERROR) 186 return "transient-error"; 187 if (code == ResponseType.FATALERROR) 188 return "fatal-error"; 189 return "?"; 190 } 191 } 192 193 @Block() 194 public static class MessageHeaderResponseComponent extends BackboneElement implements IBaseBackboneElement { 195 /** 196 * The id of the message that this message is a response to. 197 */ 198 @Child(name = "identifier", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 199 @Description(shortDefinition = "Id of original message", formalDefinition = "The id of the message that this message is a response to.") 200 protected IdType identifier; 201 202 /** 203 * Code that identifies the type of response to the message - whether it was 204 * successful or not, and whether it should be resent or not. 205 */ 206 @Child(name = "code", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 207 @Description(shortDefinition = "ok | transient-error | fatal-error", formalDefinition = "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.") 208 protected Enumeration<ResponseType> code; 209 210 /** 211 * Full details of any issues found in the message. 212 */ 213 @Child(name = "details", type = { 214 OperationOutcome.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 215 @Description(shortDefinition = "Specific list of hints/warnings/errors", formalDefinition = "Full details of any issues found in the message.") 216 protected Reference details; 217 218 /** 219 * The actual object that is the target of the reference (Full details of any 220 * issues found in the message.) 221 */ 222 protected OperationOutcome detailsTarget; 223 224 private static final long serialVersionUID = -1008716838L; 225 226 /* 227 * Constructor 228 */ 229 public MessageHeaderResponseComponent() { 230 super(); 231 } 232 233 /* 234 * Constructor 235 */ 236 public MessageHeaderResponseComponent(IdType identifier, Enumeration<ResponseType> code) { 237 super(); 238 this.identifier = identifier; 239 this.code = code; 240 } 241 242 /** 243 * @return {@link #identifier} (The id of the message that this message is a 244 * response to.). This is the underlying object with id, value and 245 * extensions. The accessor "getIdentifier" gives direct access to the 246 * value 247 */ 248 public IdType getIdentifierElement() { 249 if (this.identifier == null) 250 if (Configuration.errorOnAutoCreate()) 251 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.identifier"); 252 else if (Configuration.doAutoCreate()) 253 this.identifier = new IdType(); // bb 254 return this.identifier; 255 } 256 257 public boolean hasIdentifierElement() { 258 return this.identifier != null && !this.identifier.isEmpty(); 259 } 260 261 public boolean hasIdentifier() { 262 return this.identifier != null && !this.identifier.isEmpty(); 263 } 264 265 /** 266 * @param value {@link #identifier} (The id of the message that this message is 267 * a response to.). This is the underlying object with id, value 268 * and extensions. The accessor "getIdentifier" gives direct access 269 * to the value 270 */ 271 public MessageHeaderResponseComponent setIdentifierElement(IdType value) { 272 this.identifier = value; 273 return this; 274 } 275 276 /** 277 * @return The id of the message that this message is a response to. 278 */ 279 public String getIdentifier() { 280 return this.identifier == null ? null : this.identifier.getValue(); 281 } 282 283 /** 284 * @param value The id of the message that this message is a response to. 285 */ 286 public MessageHeaderResponseComponent setIdentifier(String value) { 287 if (this.identifier == null) 288 this.identifier = new IdType(); 289 this.identifier.setValue(value); 290 return this; 291 } 292 293 /** 294 * @return {@link #code} (Code that identifies the type of response to the 295 * message - whether it was successful or not, and whether it should be 296 * resent or not.). This is the underlying object with id, value and 297 * extensions. The accessor "getCode" gives direct access to the value 298 */ 299 public Enumeration<ResponseType> getCodeElement() { 300 if (this.code == null) 301 if (Configuration.errorOnAutoCreate()) 302 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.code"); 303 else if (Configuration.doAutoCreate()) 304 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); // bb 305 return this.code; 306 } 307 308 public boolean hasCodeElement() { 309 return this.code != null && !this.code.isEmpty(); 310 } 311 312 public boolean hasCode() { 313 return this.code != null && !this.code.isEmpty(); 314 } 315 316 /** 317 * @param value {@link #code} (Code that identifies the type of response to the 318 * message - whether it was successful or not, and whether it 319 * should be resent or not.). This is the underlying object with 320 * id, value and extensions. The accessor "getCode" gives direct 321 * access to the value 322 */ 323 public MessageHeaderResponseComponent setCodeElement(Enumeration<ResponseType> value) { 324 this.code = value; 325 return this; 326 } 327 328 /** 329 * @return Code that identifies the type of response to the message - whether it 330 * was successful or not, and whether it should be resent or not. 331 */ 332 public ResponseType getCode() { 333 return this.code == null ? null : this.code.getValue(); 334 } 335 336 /** 337 * @param value Code that identifies the type of response to the message - 338 * whether it was successful or not, and whether it should be 339 * resent or not. 340 */ 341 public MessageHeaderResponseComponent setCode(ResponseType value) { 342 if (this.code == null) 343 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); 344 this.code.setValue(value); 345 return this; 346 } 347 348 /** 349 * @return {@link #details} (Full details of any issues found in the message.) 350 */ 351 public Reference getDetails() { 352 if (this.details == null) 353 if (Configuration.errorOnAutoCreate()) 354 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.details"); 355 else if (Configuration.doAutoCreate()) 356 this.details = new Reference(); // cc 357 return this.details; 358 } 359 360 public boolean hasDetails() { 361 return this.details != null && !this.details.isEmpty(); 362 } 363 364 /** 365 * @param value {@link #details} (Full details of any issues found in the 366 * message.) 367 */ 368 public MessageHeaderResponseComponent setDetails(Reference value) { 369 this.details = value; 370 return this; 371 } 372 373 /** 374 * @return {@link #details} The actual object that is the target of the 375 * reference. The reference library doesn't populate this, but you can 376 * use it to hold the resource if you resolve it. (Full details of any 377 * issues found in the message.) 378 */ 379 public OperationOutcome getDetailsTarget() { 380 if (this.detailsTarget == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.details"); 383 else if (Configuration.doAutoCreate()) 384 this.detailsTarget = new OperationOutcome(); // aa 385 return this.detailsTarget; 386 } 387 388 /** 389 * @param value {@link #details} The actual object that is the target of the 390 * reference. The reference library doesn't use these, but you can 391 * use it to hold the resource if you resolve it. (Full details of 392 * any issues found in the message.) 393 */ 394 public MessageHeaderResponseComponent setDetailsTarget(OperationOutcome value) { 395 this.detailsTarget = value; 396 return this; 397 } 398 399 protected void listChildren(List<Property> childrenList) { 400 super.listChildren(childrenList); 401 childrenList.add(new Property("identifier", "id", "The id of the message that this message is a response to.", 0, 402 java.lang.Integer.MAX_VALUE, identifier)); 403 childrenList.add(new Property("code", "code", 404 "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.", 405 0, java.lang.Integer.MAX_VALUE, code)); 406 childrenList.add(new Property("details", "Reference(OperationOutcome)", 407 "Full details of any issues found in the message.", 0, java.lang.Integer.MAX_VALUE, details)); 408 } 409 410 @Override 411 public void setProperty(String name, Base value) throws FHIRException { 412 if (name.equals("identifier")) 413 this.identifier = castToId(value); // IdType 414 else if (name.equals("code")) 415 this.code = new ResponseTypeEnumFactory().fromType(value); // Enumeration<ResponseType> 416 else if (name.equals("details")) 417 this.details = castToReference(value); // Reference 418 else 419 super.setProperty(name, value); 420 } 421 422 @Override 423 public Base addChild(String name) throws FHIRException { 424 if (name.equals("identifier")) { 425 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.identifier"); 426 } else if (name.equals("code")) { 427 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.code"); 428 } else if (name.equals("details")) { 429 this.details = new Reference(); 430 return this.details; 431 } else 432 return super.addChild(name); 433 } 434 435 public MessageHeaderResponseComponent copy() { 436 MessageHeaderResponseComponent dst = new MessageHeaderResponseComponent(); 437 copyValues(dst); 438 dst.identifier = identifier == null ? null : identifier.copy(); 439 dst.code = code == null ? null : code.copy(); 440 dst.details = details == null ? null : details.copy(); 441 return dst; 442 } 443 444 @Override 445 public boolean equalsDeep(Base other) { 446 if (!super.equalsDeep(other)) 447 return false; 448 if (!(other instanceof MessageHeaderResponseComponent)) 449 return false; 450 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other; 451 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 452 && compareDeep(details, o.details, true); 453 } 454 455 @Override 456 public boolean equalsShallow(Base other) { 457 if (!super.equalsShallow(other)) 458 return false; 459 if (!(other instanceof MessageHeaderResponseComponent)) 460 return false; 461 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other; 462 return compareValues(identifier, o.identifier, true) && compareValues(code, o.code, true); 463 } 464 465 public boolean isEmpty() { 466 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (code == null || code.isEmpty()) 467 && (details == null || details.isEmpty()); 468 } 469 470 public String fhirType() { 471 return "MessageHeader.response"; 472 473 } 474 475 } 476 477 @Block() 478 public static class MessageSourceComponent extends BackboneElement implements IBaseBackboneElement { 479 /** 480 * Human-readable name for the source system. 481 */ 482 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 483 @Description(shortDefinition = "Name of system", formalDefinition = "Human-readable name for the source system.") 484 protected StringType name; 485 486 /** 487 * May include configuration or other information useful in debugging. 488 */ 489 @Child(name = "software", type = { 490 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 491 @Description(shortDefinition = "Name of software running the system", formalDefinition = "May include configuration or other information useful in debugging.") 492 protected StringType software; 493 494 /** 495 * Can convey versions of multiple systems in situations where a message passes 496 * through multiple hands. 497 */ 498 @Child(name = "version", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 499 @Description(shortDefinition = "Version of software running", formalDefinition = "Can convey versions of multiple systems in situations where a message passes through multiple hands.") 500 protected StringType version; 501 502 /** 503 * An e-mail, phone, website or other contact point to use to resolve issues 504 * with message communications. 505 */ 506 @Child(name = "contact", type = { 507 ContactPoint.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 508 @Description(shortDefinition = "Human contact for problems", formalDefinition = "An e-mail, phone, website or other contact point to use to resolve issues with message communications.") 509 protected ContactPoint contact; 510 511 /** 512 * Identifies the routing target to send acknowledgements to. 513 */ 514 @Child(name = "endpoint", type = { UriType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 515 @Description(shortDefinition = "Actual message source address or id", formalDefinition = "Identifies the routing target to send acknowledgements to.") 516 protected UriType endpoint; 517 518 private static final long serialVersionUID = -115878196L; 519 520 /* 521 * Constructor 522 */ 523 public MessageSourceComponent() { 524 super(); 525 } 526 527 /* 528 * Constructor 529 */ 530 public MessageSourceComponent(UriType endpoint) { 531 super(); 532 this.endpoint = endpoint; 533 } 534 535 /** 536 * @return {@link #name} (Human-readable name for the source system.). This is 537 * the underlying object with id, value and extensions. The accessor 538 * "getName" gives direct access to the value 539 */ 540 public StringType getNameElement() { 541 if (this.name == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create MessageSourceComponent.name"); 544 else if (Configuration.doAutoCreate()) 545 this.name = new StringType(); // bb 546 return this.name; 547 } 548 549 public boolean hasNameElement() { 550 return this.name != null && !this.name.isEmpty(); 551 } 552 553 public boolean hasName() { 554 return this.name != null && !this.name.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #name} (Human-readable name for the source system.). This 559 * is the underlying object with id, value and extensions. The 560 * accessor "getName" gives direct access to the value 561 */ 562 public MessageSourceComponent setNameElement(StringType value) { 563 this.name = value; 564 return this; 565 } 566 567 /** 568 * @return Human-readable name for the source system. 569 */ 570 public String getName() { 571 return this.name == null ? null : this.name.getValue(); 572 } 573 574 /** 575 * @param value Human-readable name for the source system. 576 */ 577 public MessageSourceComponent setName(String value) { 578 if (Utilities.noString(value)) 579 this.name = null; 580 else { 581 if (this.name == null) 582 this.name = new StringType(); 583 this.name.setValue(value); 584 } 585 return this; 586 } 587 588 /** 589 * @return {@link #software} (May include configuration or other information 590 * useful in debugging.). This is the underlying object with id, value 591 * and extensions. The accessor "getSoftware" gives direct access to the 592 * value 593 */ 594 public StringType getSoftwareElement() { 595 if (this.software == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create MessageSourceComponent.software"); 598 else if (Configuration.doAutoCreate()) 599 this.software = new StringType(); // bb 600 return this.software; 601 } 602 603 public boolean hasSoftwareElement() { 604 return this.software != null && !this.software.isEmpty(); 605 } 606 607 public boolean hasSoftware() { 608 return this.software != null && !this.software.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #software} (May include configuration or other 613 * information useful in debugging.). This is the underlying object 614 * with id, value and extensions. The accessor "getSoftware" gives 615 * direct access to the value 616 */ 617 public MessageSourceComponent setSoftwareElement(StringType value) { 618 this.software = value; 619 return this; 620 } 621 622 /** 623 * @return May include configuration or other information useful in debugging. 624 */ 625 public String getSoftware() { 626 return this.software == null ? null : this.software.getValue(); 627 } 628 629 /** 630 * @param value May include configuration or other information useful in 631 * debugging. 632 */ 633 public MessageSourceComponent setSoftware(String value) { 634 if (Utilities.noString(value)) 635 this.software = null; 636 else { 637 if (this.software == null) 638 this.software = new StringType(); 639 this.software.setValue(value); 640 } 641 return this; 642 } 643 644 /** 645 * @return {@link #version} (Can convey versions of multiple systems in 646 * situations where a message passes through multiple hands.). This is 647 * the underlying object with id, value and extensions. The accessor 648 * "getVersion" gives direct access to the value 649 */ 650 public StringType getVersionElement() { 651 if (this.version == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create MessageSourceComponent.version"); 654 else if (Configuration.doAutoCreate()) 655 this.version = new StringType(); // bb 656 return this.version; 657 } 658 659 public boolean hasVersionElement() { 660 return this.version != null && !this.version.isEmpty(); 661 } 662 663 public boolean hasVersion() { 664 return this.version != null && !this.version.isEmpty(); 665 } 666 667 /** 668 * @param value {@link #version} (Can convey versions of multiple systems in 669 * situations where a message passes through multiple hands.). This 670 * is the underlying object with id, value and extensions. The 671 * accessor "getVersion" gives direct access to the value 672 */ 673 public MessageSourceComponent setVersionElement(StringType value) { 674 this.version = value; 675 return this; 676 } 677 678 /** 679 * @return Can convey versions of multiple systems in situations where a message 680 * passes through multiple hands. 681 */ 682 public String getVersion() { 683 return this.version == null ? null : this.version.getValue(); 684 } 685 686 /** 687 * @param value Can convey versions of multiple systems in situations where a 688 * message passes through multiple hands. 689 */ 690 public MessageSourceComponent setVersion(String value) { 691 if (Utilities.noString(value)) 692 this.version = null; 693 else { 694 if (this.version == null) 695 this.version = new StringType(); 696 this.version.setValue(value); 697 } 698 return this; 699 } 700 701 /** 702 * @return {@link #contact} (An e-mail, phone, website or other contact point to 703 * use to resolve issues with message communications.) 704 */ 705 public ContactPoint getContact() { 706 if (this.contact == null) 707 if (Configuration.errorOnAutoCreate()) 708 throw new Error("Attempt to auto-create MessageSourceComponent.contact"); 709 else if (Configuration.doAutoCreate()) 710 this.contact = new ContactPoint(); // cc 711 return this.contact; 712 } 713 714 public boolean hasContact() { 715 return this.contact != null && !this.contact.isEmpty(); 716 } 717 718 /** 719 * @param value {@link #contact} (An e-mail, phone, website or other contact 720 * point to use to resolve issues with message communications.) 721 */ 722 public MessageSourceComponent setContact(ContactPoint value) { 723 this.contact = value; 724 return this; 725 } 726 727 /** 728 * @return {@link #endpoint} (Identifies the routing target to send 729 * acknowledgements to.). This is the underlying object with id, value 730 * and extensions. The accessor "getEndpoint" gives direct access to the 731 * value 732 */ 733 public UriType getEndpointElement() { 734 if (this.endpoint == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create MessageSourceComponent.endpoint"); 737 else if (Configuration.doAutoCreate()) 738 this.endpoint = new UriType(); // bb 739 return this.endpoint; 740 } 741 742 public boolean hasEndpointElement() { 743 return this.endpoint != null && !this.endpoint.isEmpty(); 744 } 745 746 public boolean hasEndpoint() { 747 return this.endpoint != null && !this.endpoint.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #endpoint} (Identifies the routing target to send 752 * acknowledgements to.). This is the underlying object with id, 753 * value and extensions. The accessor "getEndpoint" gives direct 754 * access to the value 755 */ 756 public MessageSourceComponent setEndpointElement(UriType value) { 757 this.endpoint = value; 758 return this; 759 } 760 761 /** 762 * @return Identifies the routing target to send acknowledgements to. 763 */ 764 public String getEndpoint() { 765 return this.endpoint == null ? null : this.endpoint.getValue(); 766 } 767 768 /** 769 * @param value Identifies the routing target to send acknowledgements to. 770 */ 771 public MessageSourceComponent setEndpoint(String value) { 772 if (this.endpoint == null) 773 this.endpoint = new UriType(); 774 this.endpoint.setValue(value); 775 return this; 776 } 777 778 protected void listChildren(List<Property> childrenList) { 779 super.listChildren(childrenList); 780 childrenList.add(new Property("name", "string", "Human-readable name for the source system.", 0, 781 java.lang.Integer.MAX_VALUE, name)); 782 childrenList 783 .add(new Property("software", "string", "May include configuration or other information useful in debugging.", 784 0, java.lang.Integer.MAX_VALUE, software)); 785 childrenList.add(new Property("version", "string", 786 "Can convey versions of multiple systems in situations where a message passes through multiple hands.", 0, 787 java.lang.Integer.MAX_VALUE, version)); 788 childrenList.add(new Property("contact", "ContactPoint", 789 "An e-mail, phone, website or other contact point to use to resolve issues with message communications.", 0, 790 java.lang.Integer.MAX_VALUE, contact)); 791 childrenList.add(new Property("endpoint", "uri", "Identifies the routing target to send acknowledgements to.", 0, 792 java.lang.Integer.MAX_VALUE, endpoint)); 793 } 794 795 @Override 796 public void setProperty(String name, Base value) throws FHIRException { 797 if (name.equals("name")) 798 this.name = castToString(value); // StringType 799 else if (name.equals("software")) 800 this.software = castToString(value); // StringType 801 else if (name.equals("version")) 802 this.version = castToString(value); // StringType 803 else if (name.equals("contact")) 804 this.contact = castToContactPoint(value); // ContactPoint 805 else if (name.equals("endpoint")) 806 this.endpoint = castToUri(value); // UriType 807 else 808 super.setProperty(name, value); 809 } 810 811 @Override 812 public Base addChild(String name) throws FHIRException { 813 if (name.equals("name")) { 814 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.name"); 815 } else if (name.equals("software")) { 816 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.software"); 817 } else if (name.equals("version")) { 818 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.version"); 819 } else if (name.equals("contact")) { 820 this.contact = new ContactPoint(); 821 return this.contact; 822 } else if (name.equals("endpoint")) { 823 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.endpoint"); 824 } else 825 return super.addChild(name); 826 } 827 828 public MessageSourceComponent copy() { 829 MessageSourceComponent dst = new MessageSourceComponent(); 830 copyValues(dst); 831 dst.name = name == null ? null : name.copy(); 832 dst.software = software == null ? null : software.copy(); 833 dst.version = version == null ? null : version.copy(); 834 dst.contact = contact == null ? null : contact.copy(); 835 dst.endpoint = endpoint == null ? null : endpoint.copy(); 836 return dst; 837 } 838 839 @Override 840 public boolean equalsDeep(Base other) { 841 if (!super.equalsDeep(other)) 842 return false; 843 if (!(other instanceof MessageSourceComponent)) 844 return false; 845 MessageSourceComponent o = (MessageSourceComponent) other; 846 return compareDeep(name, o.name, true) && compareDeep(software, o.software, true) 847 && compareDeep(version, o.version, true) && compareDeep(contact, o.contact, true) 848 && compareDeep(endpoint, o.endpoint, true); 849 } 850 851 @Override 852 public boolean equalsShallow(Base other) { 853 if (!super.equalsShallow(other)) 854 return false; 855 if (!(other instanceof MessageSourceComponent)) 856 return false; 857 MessageSourceComponent o = (MessageSourceComponent) other; 858 return compareValues(name, o.name, true) && compareValues(software, o.software, true) 859 && compareValues(version, o.version, true) && compareValues(endpoint, o.endpoint, true); 860 } 861 862 public boolean isEmpty() { 863 return super.isEmpty() && (name == null || name.isEmpty()) && (software == null || software.isEmpty()) 864 && (version == null || version.isEmpty()) && (contact == null || contact.isEmpty()) 865 && (endpoint == null || endpoint.isEmpty()); 866 } 867 868 public String fhirType() { 869 return "MessageHeader.source"; 870 871 } 872 873 } 874 875 @Block() 876 public static class MessageDestinationComponent extends BackboneElement implements IBaseBackboneElement { 877 /** 878 * Human-readable name for the target system. 879 */ 880 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 881 @Description(shortDefinition = "Name of system", formalDefinition = "Human-readable name for the target system.") 882 protected StringType name; 883 884 /** 885 * Identifies the target end system in situations where the initial message 886 * transmission is to an intermediary system. 887 */ 888 @Child(name = "target", type = { Device.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 889 @Description(shortDefinition = "Particular delivery destination within the destination", formalDefinition = "Identifies the target end system in situations where the initial message transmission is to an intermediary system.") 890 protected Reference target; 891 892 /** 893 * The actual object that is the target of the reference (Identifies the target 894 * end system in situations where the initial message transmission is to an 895 * intermediary system.) 896 */ 897 protected Device targetTarget; 898 899 /** 900 * Indicates where the message should be routed to. 901 */ 902 @Child(name = "endpoint", type = { UriType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 903 @Description(shortDefinition = "Actual destination address or id", formalDefinition = "Indicates where the message should be routed to.") 904 protected UriType endpoint; 905 906 private static final long serialVersionUID = -2097633309L; 907 908 /* 909 * Constructor 910 */ 911 public MessageDestinationComponent() { 912 super(); 913 } 914 915 /* 916 * Constructor 917 */ 918 public MessageDestinationComponent(UriType endpoint) { 919 super(); 920 this.endpoint = endpoint; 921 } 922 923 /** 924 * @return {@link #name} (Human-readable name for the target system.). This is 925 * the underlying object with id, value and extensions. The accessor 926 * "getName" gives direct access to the value 927 */ 928 public StringType getNameElement() { 929 if (this.name == null) 930 if (Configuration.errorOnAutoCreate()) 931 throw new Error("Attempt to auto-create MessageDestinationComponent.name"); 932 else if (Configuration.doAutoCreate()) 933 this.name = new StringType(); // bb 934 return this.name; 935 } 936 937 public boolean hasNameElement() { 938 return this.name != null && !this.name.isEmpty(); 939 } 940 941 public boolean hasName() { 942 return this.name != null && !this.name.isEmpty(); 943 } 944 945 /** 946 * @param value {@link #name} (Human-readable name for the target system.). This 947 * is the underlying object with id, value and extensions. The 948 * accessor "getName" gives direct access to the value 949 */ 950 public MessageDestinationComponent setNameElement(StringType value) { 951 this.name = value; 952 return this; 953 } 954 955 /** 956 * @return Human-readable name for the target system. 957 */ 958 public String getName() { 959 return this.name == null ? null : this.name.getValue(); 960 } 961 962 /** 963 * @param value Human-readable name for the target system. 964 */ 965 public MessageDestinationComponent setName(String value) { 966 if (Utilities.noString(value)) 967 this.name = null; 968 else { 969 if (this.name == null) 970 this.name = new StringType(); 971 this.name.setValue(value); 972 } 973 return this; 974 } 975 976 /** 977 * @return {@link #target} (Identifies the target end system in situations where 978 * the initial message transmission is to an intermediary system.) 979 */ 980 public Reference getTarget() { 981 if (this.target == null) 982 if (Configuration.errorOnAutoCreate()) 983 throw new Error("Attempt to auto-create MessageDestinationComponent.target"); 984 else if (Configuration.doAutoCreate()) 985 this.target = new Reference(); // cc 986 return this.target; 987 } 988 989 public boolean hasTarget() { 990 return this.target != null && !this.target.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #target} (Identifies the target end system in situations 995 * where the initial message transmission is to an intermediary 996 * system.) 997 */ 998 public MessageDestinationComponent setTarget(Reference value) { 999 this.target = value; 1000 return this; 1001 } 1002 1003 /** 1004 * @return {@link #target} The actual object that is the target of the 1005 * reference. The reference library doesn't populate this, but you can 1006 * use it to hold the resource if you resolve it. (Identifies the target 1007 * end system in situations where the initial message transmission is to 1008 * an intermediary system.) 1009 */ 1010 public Device getTargetTarget() { 1011 if (this.targetTarget == null) 1012 if (Configuration.errorOnAutoCreate()) 1013 throw new Error("Attempt to auto-create MessageDestinationComponent.target"); 1014 else if (Configuration.doAutoCreate()) 1015 this.targetTarget = new Device(); // aa 1016 return this.targetTarget; 1017 } 1018 1019 /** 1020 * @param value {@link #target} The actual object that is the target of the 1021 * reference. The reference library doesn't use these, but you can 1022 * use it to hold the resource if you resolve it. (Identifies the 1023 * target end system in situations where the initial message 1024 * transmission is to an intermediary system.) 1025 */ 1026 public MessageDestinationComponent setTargetTarget(Device value) { 1027 this.targetTarget = value; 1028 return this; 1029 } 1030 1031 /** 1032 * @return {@link #endpoint} (Indicates where the message should be routed to.). 1033 * This is the underlying object with id, value and extensions. The 1034 * accessor "getEndpoint" gives direct access to the value 1035 */ 1036 public UriType getEndpointElement() { 1037 if (this.endpoint == null) 1038 if (Configuration.errorOnAutoCreate()) 1039 throw new Error("Attempt to auto-create MessageDestinationComponent.endpoint"); 1040 else if (Configuration.doAutoCreate()) 1041 this.endpoint = new UriType(); // bb 1042 return this.endpoint; 1043 } 1044 1045 public boolean hasEndpointElement() { 1046 return this.endpoint != null && !this.endpoint.isEmpty(); 1047 } 1048 1049 public boolean hasEndpoint() { 1050 return this.endpoint != null && !this.endpoint.isEmpty(); 1051 } 1052 1053 /** 1054 * @param value {@link #endpoint} (Indicates where the message should be routed 1055 * to.). This is the underlying object with id, value and 1056 * extensions. The accessor "getEndpoint" gives direct access to 1057 * the value 1058 */ 1059 public MessageDestinationComponent setEndpointElement(UriType value) { 1060 this.endpoint = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return Indicates where the message should be routed to. 1066 */ 1067 public String getEndpoint() { 1068 return this.endpoint == null ? null : this.endpoint.getValue(); 1069 } 1070 1071 /** 1072 * @param value Indicates where the message should be routed to. 1073 */ 1074 public MessageDestinationComponent setEndpoint(String value) { 1075 if (this.endpoint == null) 1076 this.endpoint = new UriType(); 1077 this.endpoint.setValue(value); 1078 return this; 1079 } 1080 1081 protected void listChildren(List<Property> childrenList) { 1082 super.listChildren(childrenList); 1083 childrenList.add(new Property("name", "string", "Human-readable name for the target system.", 0, 1084 java.lang.Integer.MAX_VALUE, name)); 1085 childrenList.add(new Property("target", "Reference(Device)", 1086 "Identifies the target end system in situations where the initial message transmission is to an intermediary system.", 1087 0, java.lang.Integer.MAX_VALUE, target)); 1088 childrenList.add(new Property("endpoint", "uri", "Indicates where the message should be routed to.", 0, 1089 java.lang.Integer.MAX_VALUE, endpoint)); 1090 } 1091 1092 @Override 1093 public void setProperty(String name, Base value) throws FHIRException { 1094 if (name.equals("name")) 1095 this.name = castToString(value); // StringType 1096 else if (name.equals("target")) 1097 this.target = castToReference(value); // Reference 1098 else if (name.equals("endpoint")) 1099 this.endpoint = castToUri(value); // UriType 1100 else 1101 super.setProperty(name, value); 1102 } 1103 1104 @Override 1105 public Base addChild(String name) throws FHIRException { 1106 if (name.equals("name")) { 1107 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.name"); 1108 } else if (name.equals("target")) { 1109 this.target = new Reference(); 1110 return this.target; 1111 } else if (name.equals("endpoint")) { 1112 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.endpoint"); 1113 } else 1114 return super.addChild(name); 1115 } 1116 1117 public MessageDestinationComponent copy() { 1118 MessageDestinationComponent dst = new MessageDestinationComponent(); 1119 copyValues(dst); 1120 dst.name = name == null ? null : name.copy(); 1121 dst.target = target == null ? null : target.copy(); 1122 dst.endpoint = endpoint == null ? null : endpoint.copy(); 1123 return dst; 1124 } 1125 1126 @Override 1127 public boolean equalsDeep(Base other) { 1128 if (!super.equalsDeep(other)) 1129 return false; 1130 if (!(other instanceof MessageDestinationComponent)) 1131 return false; 1132 MessageDestinationComponent o = (MessageDestinationComponent) other; 1133 return compareDeep(name, o.name, true) && compareDeep(target, o.target, true) 1134 && compareDeep(endpoint, o.endpoint, true); 1135 } 1136 1137 @Override 1138 public boolean equalsShallow(Base other) { 1139 if (!super.equalsShallow(other)) 1140 return false; 1141 if (!(other instanceof MessageDestinationComponent)) 1142 return false; 1143 MessageDestinationComponent o = (MessageDestinationComponent) other; 1144 return compareValues(name, o.name, true) && compareValues(endpoint, o.endpoint, true); 1145 } 1146 1147 public boolean isEmpty() { 1148 return super.isEmpty() && (name == null || name.isEmpty()) && (target == null || target.isEmpty()) 1149 && (endpoint == null || endpoint.isEmpty()); 1150 } 1151 1152 public String fhirType() { 1153 return "MessageHeader.destination"; 1154 1155 } 1156 1157 } 1158 1159 /** 1160 * The time that the message was sent. 1161 */ 1162 @Child(name = "timestamp", type = { 1163 InstantType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 1164 @Description(shortDefinition = "Time that the message was sent", formalDefinition = "The time that the message was sent.") 1165 protected InstantType timestamp; 1166 1167 /** 1168 * Code that identifies the event this message represents and connects it with 1169 * its definition. Events defined as part of the FHIR specification have the 1170 * system value "http://hl7.org/fhir/message-events". 1171 */ 1172 @Child(name = "event", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1173 @Description(shortDefinition = "Code for the event this message represents", formalDefinition = "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://hl7.org/fhir/message-events\".") 1174 protected Coding event; 1175 1176 /** 1177 * Information about the message that this message is a response to. Only 1178 * present if this message is a response. 1179 */ 1180 @Child(name = "response", type = {}, order = 2, min = 0, max = 1, modifier = true, summary = true) 1181 @Description(shortDefinition = "If this is a reply to prior message", formalDefinition = "Information about the message that this message is a response to. Only present if this message is a response.") 1182 protected MessageHeaderResponseComponent response; 1183 1184 /** 1185 * The source application from which this message originated. 1186 */ 1187 @Child(name = "source", type = {}, order = 3, min = 1, max = 1, modifier = false, summary = true) 1188 @Description(shortDefinition = "Message Source Application", formalDefinition = "The source application from which this message originated.") 1189 protected MessageSourceComponent source; 1190 1191 /** 1192 * The destination application which the message is intended for. 1193 */ 1194 @Child(name = "destination", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1195 @Description(shortDefinition = "Message Destination Application(s)", formalDefinition = "The destination application which the message is intended for.") 1196 protected List<MessageDestinationComponent> destination; 1197 1198 /** 1199 * The person or device that performed the data entry leading to this message. 1200 * Where there is more than one candidate, pick the most proximal to the 1201 * message. Can provide other enterers in extensions. 1202 */ 1203 @Child(name = "enterer", type = { Practitioner.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1204 @Description(shortDefinition = "The source of the data entry", formalDefinition = "The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.") 1205 protected Reference enterer; 1206 1207 /** 1208 * The actual object that is the target of the reference (The person or device 1209 * that performed the data entry leading to this message. Where there is more 1210 * than one candidate, pick the most proximal to the message. Can provide other 1211 * enterers in extensions.) 1212 */ 1213 protected Practitioner entererTarget; 1214 1215 /** 1216 * The logical author of the message - the person or device that decided the 1217 * described event should happen. Where there is more than one candidate, pick 1218 * the most proximal to the MessageHeader. Can provide other authors in 1219 * extensions. 1220 */ 1221 @Child(name = "author", type = { Practitioner.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1222 @Description(shortDefinition = "The source of the decision", formalDefinition = "The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.") 1223 protected Reference author; 1224 1225 /** 1226 * The actual object that is the target of the reference (The logical author of 1227 * the message - the person or device that decided the described event should 1228 * happen. Where there is more than one candidate, pick the most proximal to the 1229 * MessageHeader. Can provide other authors in extensions.) 1230 */ 1231 protected Practitioner authorTarget; 1232 1233 /** 1234 * Allows data conveyed by a message to be addressed to a particular person or 1235 * department when routing to a specific application isn't sufficient. 1236 */ 1237 @Child(name = "receiver", type = { Practitioner.class, 1238 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1239 @Description(shortDefinition = "Intended \"real-world\" recipient for the data", formalDefinition = "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.") 1240 protected Reference receiver; 1241 1242 /** 1243 * The actual object that is the target of the reference (Allows data conveyed 1244 * by a message to be addressed to a particular person or department when 1245 * routing to a specific application isn't sufficient.) 1246 */ 1247 protected Resource receiverTarget; 1248 1249 /** 1250 * The person or organization that accepts overall responsibility for the 1251 * contents of the message. The implication is that the message event happened 1252 * under the policies of the responsible party. 1253 */ 1254 @Child(name = "responsible", type = { Practitioner.class, 1255 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1256 @Description(shortDefinition = "Final responsibility for event", formalDefinition = "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.") 1257 protected Reference responsible; 1258 1259 /** 1260 * The actual object that is the target of the reference (The person or 1261 * organization that accepts overall responsibility for the contents of the 1262 * message. The implication is that the message event happened under the 1263 * policies of the responsible party.) 1264 */ 1265 protected Resource responsibleTarget; 1266 1267 /** 1268 * Coded indication of the cause for the event - indicates a reason for the 1269 * occurrence of the event that is a focus of this message. 1270 */ 1271 @Child(name = "reason", type = { 1272 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1273 @Description(shortDefinition = "Cause of event", formalDefinition = "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.") 1274 protected CodeableConcept reason; 1275 1276 /** 1277 * The actual data of the message - a reference to the root/focus class of the 1278 * event. 1279 */ 1280 @Child(name = "data", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1281 @Description(shortDefinition = "The actual content of the message", formalDefinition = "The actual data of the message - a reference to the root/focus class of the event.") 1282 protected List<Reference> data; 1283 /** 1284 * The actual objects that are the target of the reference (The actual data of 1285 * the message - a reference to the root/focus class of the event.) 1286 */ 1287 protected List<Resource> dataTarget; 1288 1289 private static final long serialVersionUID = 1429728517L; 1290 1291 /* 1292 * Constructor 1293 */ 1294 public MessageHeader() { 1295 super(); 1296 } 1297 1298 /* 1299 * Constructor 1300 */ 1301 public MessageHeader(InstantType timestamp, Coding event, MessageSourceComponent source) { 1302 super(); 1303 this.timestamp = timestamp; 1304 this.event = event; 1305 this.source = source; 1306 } 1307 1308 /** 1309 * @return {@link #timestamp} (The time that the message was sent.). This is the 1310 * underlying object with id, value and extensions. The accessor 1311 * "getTimestamp" gives direct access to the value 1312 */ 1313 public InstantType getTimestampElement() { 1314 if (this.timestamp == null) 1315 if (Configuration.errorOnAutoCreate()) 1316 throw new Error("Attempt to auto-create MessageHeader.timestamp"); 1317 else if (Configuration.doAutoCreate()) 1318 this.timestamp = new InstantType(); // bb 1319 return this.timestamp; 1320 } 1321 1322 public boolean hasTimestampElement() { 1323 return this.timestamp != null && !this.timestamp.isEmpty(); 1324 } 1325 1326 public boolean hasTimestamp() { 1327 return this.timestamp != null && !this.timestamp.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #timestamp} (The time that the message was sent.). This 1332 * is the underlying object with id, value and extensions. The 1333 * accessor "getTimestamp" gives direct access to the value 1334 */ 1335 public MessageHeader setTimestampElement(InstantType value) { 1336 this.timestamp = value; 1337 return this; 1338 } 1339 1340 /** 1341 * @return The time that the message was sent. 1342 */ 1343 public Date getTimestamp() { 1344 return this.timestamp == null ? null : this.timestamp.getValue(); 1345 } 1346 1347 /** 1348 * @param value The time that the message was sent. 1349 */ 1350 public MessageHeader setTimestamp(Date value) { 1351 if (this.timestamp == null) 1352 this.timestamp = new InstantType(); 1353 this.timestamp.setValue(value); 1354 return this; 1355 } 1356 1357 /** 1358 * @return {@link #event} (Code that identifies the event this message 1359 * represents and connects it with its definition. Events defined as 1360 * part of the FHIR specification have the system value 1361 * "http://hl7.org/fhir/message-events".) 1362 */ 1363 public Coding getEvent() { 1364 if (this.event == null) 1365 if (Configuration.errorOnAutoCreate()) 1366 throw new Error("Attempt to auto-create MessageHeader.event"); 1367 else if (Configuration.doAutoCreate()) 1368 this.event = new Coding(); // cc 1369 return this.event; 1370 } 1371 1372 public boolean hasEvent() { 1373 return this.event != null && !this.event.isEmpty(); 1374 } 1375 1376 /** 1377 * @param value {@link #event} (Code that identifies the event this message 1378 * represents and connects it with its definition. Events defined 1379 * as part of the FHIR specification have the system value 1380 * "http://hl7.org/fhir/message-events".) 1381 */ 1382 public MessageHeader setEvent(Coding value) { 1383 this.event = value; 1384 return this; 1385 } 1386 1387 /** 1388 * @return {@link #response} (Information about the message that this message is 1389 * a response to. Only present if this message is a response.) 1390 */ 1391 public MessageHeaderResponseComponent getResponse() { 1392 if (this.response == null) 1393 if (Configuration.errorOnAutoCreate()) 1394 throw new Error("Attempt to auto-create MessageHeader.response"); 1395 else if (Configuration.doAutoCreate()) 1396 this.response = new MessageHeaderResponseComponent(); // cc 1397 return this.response; 1398 } 1399 1400 public boolean hasResponse() { 1401 return this.response != null && !this.response.isEmpty(); 1402 } 1403 1404 /** 1405 * @param value {@link #response} (Information about the message that this 1406 * message is a response to. Only present if this message is a 1407 * response.) 1408 */ 1409 public MessageHeader setResponse(MessageHeaderResponseComponent value) { 1410 this.response = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return {@link #source} (The source application from which this message 1416 * originated.) 1417 */ 1418 public MessageSourceComponent getSource() { 1419 if (this.source == null) 1420 if (Configuration.errorOnAutoCreate()) 1421 throw new Error("Attempt to auto-create MessageHeader.source"); 1422 else if (Configuration.doAutoCreate()) 1423 this.source = new MessageSourceComponent(); // cc 1424 return this.source; 1425 } 1426 1427 public boolean hasSource() { 1428 return this.source != null && !this.source.isEmpty(); 1429 } 1430 1431 /** 1432 * @param value {@link #source} (The source application from which this message 1433 * originated.) 1434 */ 1435 public MessageHeader setSource(MessageSourceComponent value) { 1436 this.source = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #destination} (The destination application which the message 1442 * is intended for.) 1443 */ 1444 public List<MessageDestinationComponent> getDestination() { 1445 if (this.destination == null) 1446 this.destination = new ArrayList<MessageDestinationComponent>(); 1447 return this.destination; 1448 } 1449 1450 public boolean hasDestination() { 1451 if (this.destination == null) 1452 return false; 1453 for (MessageDestinationComponent item : this.destination) 1454 if (!item.isEmpty()) 1455 return true; 1456 return false; 1457 } 1458 1459 /** 1460 * @return {@link #destination} (The destination application which the message 1461 * is intended for.) 1462 */ 1463 // syntactic sugar 1464 public MessageDestinationComponent addDestination() { // 3 1465 MessageDestinationComponent t = new MessageDestinationComponent(); 1466 if (this.destination == null) 1467 this.destination = new ArrayList<MessageDestinationComponent>(); 1468 this.destination.add(t); 1469 return t; 1470 } 1471 1472 // syntactic sugar 1473 public MessageHeader addDestination(MessageDestinationComponent t) { // 3 1474 if (t == null) 1475 return this; 1476 if (this.destination == null) 1477 this.destination = new ArrayList<MessageDestinationComponent>(); 1478 this.destination.add(t); 1479 return this; 1480 } 1481 1482 /** 1483 * @return {@link #enterer} (The person or device that performed the data entry 1484 * leading to this message. Where there is more than one candidate, pick 1485 * the most proximal to the message. Can provide other enterers in 1486 * extensions.) 1487 */ 1488 public Reference getEnterer() { 1489 if (this.enterer == null) 1490 if (Configuration.errorOnAutoCreate()) 1491 throw new Error("Attempt to auto-create MessageHeader.enterer"); 1492 else if (Configuration.doAutoCreate()) 1493 this.enterer = new Reference(); // cc 1494 return this.enterer; 1495 } 1496 1497 public boolean hasEnterer() { 1498 return this.enterer != null && !this.enterer.isEmpty(); 1499 } 1500 1501 /** 1502 * @param value {@link #enterer} (The person or device that performed the data 1503 * entry leading to this message. Where there is more than one 1504 * candidate, pick the most proximal to the message. Can provide 1505 * other enterers in extensions.) 1506 */ 1507 public MessageHeader setEnterer(Reference value) { 1508 this.enterer = value; 1509 return this; 1510 } 1511 1512 /** 1513 * @return {@link #enterer} The actual object that is the target of the 1514 * reference. The reference library doesn't populate this, but you can 1515 * use it to hold the resource if you resolve it. (The person or device 1516 * that performed the data entry leading to this message. Where there is 1517 * more than one candidate, pick the most proximal to the message. Can 1518 * provide other enterers in extensions.) 1519 */ 1520 public Practitioner getEntererTarget() { 1521 if (this.entererTarget == null) 1522 if (Configuration.errorOnAutoCreate()) 1523 throw new Error("Attempt to auto-create MessageHeader.enterer"); 1524 else if (Configuration.doAutoCreate()) 1525 this.entererTarget = new Practitioner(); // aa 1526 return this.entererTarget; 1527 } 1528 1529 /** 1530 * @param value {@link #enterer} The actual object that is the target of the 1531 * reference. The reference library doesn't use these, but you can 1532 * use it to hold the resource if you resolve it. (The person or 1533 * device that performed the data entry leading to this message. 1534 * Where there is more than one candidate, pick the most proximal 1535 * to the message. Can provide other enterers in extensions.) 1536 */ 1537 public MessageHeader setEntererTarget(Practitioner value) { 1538 this.entererTarget = value; 1539 return this; 1540 } 1541 1542 /** 1543 * @return {@link #author} (The logical author of the message - the person or 1544 * device that decided the described event should happen. Where there is 1545 * more than one candidate, pick the most proximal to the MessageHeader. 1546 * Can provide other authors in extensions.) 1547 */ 1548 public Reference getAuthor() { 1549 if (this.author == null) 1550 if (Configuration.errorOnAutoCreate()) 1551 throw new Error("Attempt to auto-create MessageHeader.author"); 1552 else if (Configuration.doAutoCreate()) 1553 this.author = new Reference(); // cc 1554 return this.author; 1555 } 1556 1557 public boolean hasAuthor() { 1558 return this.author != null && !this.author.isEmpty(); 1559 } 1560 1561 /** 1562 * @param value {@link #author} (The logical author of the message - the person 1563 * or device that decided the described event should happen. Where 1564 * there is more than one candidate, pick the most proximal to the 1565 * MessageHeader. Can provide other authors in extensions.) 1566 */ 1567 public MessageHeader setAuthor(Reference value) { 1568 this.author = value; 1569 return this; 1570 } 1571 1572 /** 1573 * @return {@link #author} The actual object that is the target of the 1574 * reference. The reference library doesn't populate this, but you can 1575 * use it to hold the resource if you resolve it. (The logical author of 1576 * the message - the person or device that decided the described event 1577 * should happen. Where there is more than one candidate, pick the most 1578 * proximal to the MessageHeader. Can provide other authors in 1579 * extensions.) 1580 */ 1581 public Practitioner getAuthorTarget() { 1582 if (this.authorTarget == null) 1583 if (Configuration.errorOnAutoCreate()) 1584 throw new Error("Attempt to auto-create MessageHeader.author"); 1585 else if (Configuration.doAutoCreate()) 1586 this.authorTarget = new Practitioner(); // aa 1587 return this.authorTarget; 1588 } 1589 1590 /** 1591 * @param value {@link #author} The actual object that is the target of the 1592 * reference. The reference library doesn't use these, but you can 1593 * use it to hold the resource if you resolve it. (The logical 1594 * author of the message - the person or device that decided the 1595 * described event should happen. Where there is more than one 1596 * candidate, pick the most proximal to the MessageHeader. Can 1597 * provide other authors in extensions.) 1598 */ 1599 public MessageHeader setAuthorTarget(Practitioner value) { 1600 this.authorTarget = value; 1601 return this; 1602 } 1603 1604 /** 1605 * @return {@link #receiver} (Allows data conveyed by a message to be addressed 1606 * to a particular person or department when routing to a specific 1607 * application isn't sufficient.) 1608 */ 1609 public Reference getReceiver() { 1610 if (this.receiver == null) 1611 if (Configuration.errorOnAutoCreate()) 1612 throw new Error("Attempt to auto-create MessageHeader.receiver"); 1613 else if (Configuration.doAutoCreate()) 1614 this.receiver = new Reference(); // cc 1615 return this.receiver; 1616 } 1617 1618 public boolean hasReceiver() { 1619 return this.receiver != null && !this.receiver.isEmpty(); 1620 } 1621 1622 /** 1623 * @param value {@link #receiver} (Allows data conveyed by a message to be 1624 * addressed to a particular person or department when routing to a 1625 * specific application isn't sufficient.) 1626 */ 1627 public MessageHeader setReceiver(Reference value) { 1628 this.receiver = value; 1629 return this; 1630 } 1631 1632 /** 1633 * @return {@link #receiver} The actual object that is the target of the 1634 * reference. The reference library doesn't populate this, but you can 1635 * use it to hold the resource if you resolve it. (Allows data conveyed 1636 * by a message to be addressed to a particular person or department 1637 * when routing to a specific application isn't sufficient.) 1638 */ 1639 public Resource getReceiverTarget() { 1640 return this.receiverTarget; 1641 } 1642 1643 /** 1644 * @param value {@link #receiver} The actual object that is the target of the 1645 * reference. The reference library doesn't use these, but you can 1646 * use it to hold the resource if you resolve it. (Allows data 1647 * conveyed by a message to be addressed to a particular person or 1648 * department when routing to a specific application isn't 1649 * sufficient.) 1650 */ 1651 public MessageHeader setReceiverTarget(Resource value) { 1652 this.receiverTarget = value; 1653 return this; 1654 } 1655 1656 /** 1657 * @return {@link #responsible} (The person or organization that accepts overall 1658 * responsibility for the contents of the message. The implication is 1659 * that the message event happened under the policies of the responsible 1660 * party.) 1661 */ 1662 public Reference getResponsible() { 1663 if (this.responsible == null) 1664 if (Configuration.errorOnAutoCreate()) 1665 throw new Error("Attempt to auto-create MessageHeader.responsible"); 1666 else if (Configuration.doAutoCreate()) 1667 this.responsible = new Reference(); // cc 1668 return this.responsible; 1669 } 1670 1671 public boolean hasResponsible() { 1672 return this.responsible != null && !this.responsible.isEmpty(); 1673 } 1674 1675 /** 1676 * @param value {@link #responsible} (The person or organization that accepts 1677 * overall responsibility for the contents of the message. The 1678 * implication is that the message event happened under the 1679 * policies of the responsible party.) 1680 */ 1681 public MessageHeader setResponsible(Reference value) { 1682 this.responsible = value; 1683 return this; 1684 } 1685 1686 /** 1687 * @return {@link #responsible} The actual object that is the target of the 1688 * reference. The reference library doesn't populate this, but you can 1689 * use it to hold the resource if you resolve it. (The person or 1690 * organization that accepts overall responsibility for the contents of 1691 * the message. The implication is that the message event happened under 1692 * the policies of the responsible party.) 1693 */ 1694 public Resource getResponsibleTarget() { 1695 return this.responsibleTarget; 1696 } 1697 1698 /** 1699 * @param value {@link #responsible} The actual object that is the target of the 1700 * reference. The reference library doesn't use these, but you can 1701 * use it to hold the resource if you resolve it. (The person or 1702 * organization that accepts overall responsibility for the 1703 * contents of the message. The implication is that the message 1704 * event happened under the policies of the responsible party.) 1705 */ 1706 public MessageHeader setResponsibleTarget(Resource value) { 1707 this.responsibleTarget = value; 1708 return this; 1709 } 1710 1711 /** 1712 * @return {@link #reason} (Coded indication of the cause for the event - 1713 * indicates a reason for the occurrence of the event that is a focus of 1714 * this message.) 1715 */ 1716 public CodeableConcept getReason() { 1717 if (this.reason == null) 1718 if (Configuration.errorOnAutoCreate()) 1719 throw new Error("Attempt to auto-create MessageHeader.reason"); 1720 else if (Configuration.doAutoCreate()) 1721 this.reason = new CodeableConcept(); // cc 1722 return this.reason; 1723 } 1724 1725 public boolean hasReason() { 1726 return this.reason != null && !this.reason.isEmpty(); 1727 } 1728 1729 /** 1730 * @param value {@link #reason} (Coded indication of the cause for the event - 1731 * indicates a reason for the occurrence of the event that is a 1732 * focus of this message.) 1733 */ 1734 public MessageHeader setReason(CodeableConcept value) { 1735 this.reason = value; 1736 return this; 1737 } 1738 1739 /** 1740 * @return {@link #data} (The actual data of the message - a reference to the 1741 * root/focus class of the event.) 1742 */ 1743 public List<Reference> getData() { 1744 if (this.data == null) 1745 this.data = new ArrayList<Reference>(); 1746 return this.data; 1747 } 1748 1749 public boolean hasData() { 1750 if (this.data == null) 1751 return false; 1752 for (Reference item : this.data) 1753 if (!item.isEmpty()) 1754 return true; 1755 return false; 1756 } 1757 1758 /** 1759 * @return {@link #data} (The actual data of the message - a reference to the 1760 * root/focus class of the event.) 1761 */ 1762 // syntactic sugar 1763 public Reference addData() { // 3 1764 Reference t = new Reference(); 1765 if (this.data == null) 1766 this.data = new ArrayList<Reference>(); 1767 this.data.add(t); 1768 return t; 1769 } 1770 1771 // syntactic sugar 1772 public MessageHeader addData(Reference t) { // 3 1773 if (t == null) 1774 return this; 1775 if (this.data == null) 1776 this.data = new ArrayList<Reference>(); 1777 this.data.add(t); 1778 return this; 1779 } 1780 1781 /** 1782 * @return {@link #data} (The actual objects that are the target of the 1783 * reference. The reference library doesn't populate this, but you can 1784 * use this to hold the resources if you resolvethemt. The actual data 1785 * of the message - a reference to the root/focus class of the event.) 1786 */ 1787 public List<Resource> getDataTarget() { 1788 if (this.dataTarget == null) 1789 this.dataTarget = new ArrayList<Resource>(); 1790 return this.dataTarget; 1791 } 1792 1793 protected void listChildren(List<Property> childrenList) { 1794 super.listChildren(childrenList); 1795 childrenList.add(new Property("timestamp", "instant", "The time that the message was sent.", 0, 1796 java.lang.Integer.MAX_VALUE, timestamp)); 1797 childrenList.add(new Property("event", "Coding", 1798 "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://hl7.org/fhir/message-events\".", 1799 0, java.lang.Integer.MAX_VALUE, event)); 1800 childrenList.add(new Property("response", "", 1801 "Information about the message that this message is a response to. Only present if this message is a response.", 1802 0, java.lang.Integer.MAX_VALUE, response)); 1803 childrenList.add(new Property("source", "", "The source application from which this message originated.", 0, 1804 java.lang.Integer.MAX_VALUE, source)); 1805 childrenList.add(new Property("destination", "", "The destination application which the message is intended for.", 1806 0, java.lang.Integer.MAX_VALUE, destination)); 1807 childrenList.add(new Property("enterer", "Reference(Practitioner)", 1808 "The person or device that performed the data entry leading to this message. Where there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.", 1809 0, java.lang.Integer.MAX_VALUE, enterer)); 1810 childrenList.add(new Property("author", "Reference(Practitioner)", 1811 "The logical author of the message - the person or device that decided the described event should happen. Where there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.", 1812 0, java.lang.Integer.MAX_VALUE, author)); 1813 childrenList.add(new Property("receiver", "Reference(Practitioner|Organization)", 1814 "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.", 1815 0, java.lang.Integer.MAX_VALUE, receiver)); 1816 childrenList.add(new Property("responsible", "Reference(Practitioner|Organization)", 1817 "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.", 1818 0, java.lang.Integer.MAX_VALUE, responsible)); 1819 childrenList.add(new Property("reason", "CodeableConcept", 1820 "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.", 1821 0, java.lang.Integer.MAX_VALUE, reason)); 1822 childrenList.add(new Property("data", "Reference(Any)", 1823 "The actual data of the message - a reference to the root/focus class of the event.", 0, 1824 java.lang.Integer.MAX_VALUE, data)); 1825 } 1826 1827 @Override 1828 public void setProperty(String name, Base value) throws FHIRException { 1829 if (name.equals("timestamp")) 1830 this.timestamp = castToInstant(value); // InstantType 1831 else if (name.equals("event")) 1832 this.event = castToCoding(value); // Coding 1833 else if (name.equals("response")) 1834 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 1835 else if (name.equals("source")) 1836 this.source = (MessageSourceComponent) value; // MessageSourceComponent 1837 else if (name.equals("destination")) 1838 this.getDestination().add((MessageDestinationComponent) value); 1839 else if (name.equals("enterer")) 1840 this.enterer = castToReference(value); // Reference 1841 else if (name.equals("author")) 1842 this.author = castToReference(value); // Reference 1843 else if (name.equals("receiver")) 1844 this.receiver = castToReference(value); // Reference 1845 else if (name.equals("responsible")) 1846 this.responsible = castToReference(value); // Reference 1847 else if (name.equals("reason")) 1848 this.reason = castToCodeableConcept(value); // CodeableConcept 1849 else if (name.equals("data")) 1850 this.getData().add(castToReference(value)); 1851 else 1852 super.setProperty(name, value); 1853 } 1854 1855 @Override 1856 public Base addChild(String name) throws FHIRException { 1857 if (name.equals("timestamp")) { 1858 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.timestamp"); 1859 } else if (name.equals("event")) { 1860 this.event = new Coding(); 1861 return this.event; 1862 } else if (name.equals("response")) { 1863 this.response = new MessageHeaderResponseComponent(); 1864 return this.response; 1865 } else if (name.equals("source")) { 1866 this.source = new MessageSourceComponent(); 1867 return this.source; 1868 } else if (name.equals("destination")) { 1869 return addDestination(); 1870 } else if (name.equals("enterer")) { 1871 this.enterer = new Reference(); 1872 return this.enterer; 1873 } else if (name.equals("author")) { 1874 this.author = new Reference(); 1875 return this.author; 1876 } else if (name.equals("receiver")) { 1877 this.receiver = new Reference(); 1878 return this.receiver; 1879 } else if (name.equals("responsible")) { 1880 this.responsible = new Reference(); 1881 return this.responsible; 1882 } else if (name.equals("reason")) { 1883 this.reason = new CodeableConcept(); 1884 return this.reason; 1885 } else if (name.equals("data")) { 1886 return addData(); 1887 } else 1888 return super.addChild(name); 1889 } 1890 1891 public String fhirType() { 1892 return "MessageHeader"; 1893 1894 } 1895 1896 public MessageHeader copy() { 1897 MessageHeader dst = new MessageHeader(); 1898 copyValues(dst); 1899 dst.timestamp = timestamp == null ? null : timestamp.copy(); 1900 dst.event = event == null ? null : event.copy(); 1901 dst.response = response == null ? null : response.copy(); 1902 dst.source = source == null ? null : source.copy(); 1903 if (destination != null) { 1904 dst.destination = new ArrayList<MessageDestinationComponent>(); 1905 for (MessageDestinationComponent i : destination) 1906 dst.destination.add(i.copy()); 1907 } 1908 ; 1909 dst.enterer = enterer == null ? null : enterer.copy(); 1910 dst.author = author == null ? null : author.copy(); 1911 dst.receiver = receiver == null ? null : receiver.copy(); 1912 dst.responsible = responsible == null ? null : responsible.copy(); 1913 dst.reason = reason == null ? null : reason.copy(); 1914 if (data != null) { 1915 dst.data = new ArrayList<Reference>(); 1916 for (Reference i : data) 1917 dst.data.add(i.copy()); 1918 } 1919 ; 1920 return dst; 1921 } 1922 1923 protected MessageHeader typedCopy() { 1924 return copy(); 1925 } 1926 1927 @Override 1928 public boolean equalsDeep(Base other) { 1929 if (!super.equalsDeep(other)) 1930 return false; 1931 if (!(other instanceof MessageHeader)) 1932 return false; 1933 MessageHeader o = (MessageHeader) other; 1934 return compareDeep(timestamp, o.timestamp, true) && compareDeep(event, o.event, true) 1935 && compareDeep(response, o.response, true) && compareDeep(source, o.source, true) 1936 && compareDeep(destination, o.destination, true) && compareDeep(enterer, o.enterer, true) 1937 && compareDeep(author, o.author, true) && compareDeep(receiver, o.receiver, true) 1938 && compareDeep(responsible, o.responsible, true) && compareDeep(reason, o.reason, true) 1939 && compareDeep(data, o.data, true); 1940 } 1941 1942 @Override 1943 public boolean equalsShallow(Base other) { 1944 if (!super.equalsShallow(other)) 1945 return false; 1946 if (!(other instanceof MessageHeader)) 1947 return false; 1948 MessageHeader o = (MessageHeader) other; 1949 return compareValues(timestamp, o.timestamp, true); 1950 } 1951 1952 public boolean isEmpty() { 1953 return super.isEmpty() && (timestamp == null || timestamp.isEmpty()) && (event == null || event.isEmpty()) 1954 && (response == null || response.isEmpty()) && (source == null || source.isEmpty()) 1955 && (destination == null || destination.isEmpty()) && (enterer == null || enterer.isEmpty()) 1956 && (author == null || author.isEmpty()) && (receiver == null || receiver.isEmpty()) 1957 && (responsible == null || responsible.isEmpty()) && (reason == null || reason.isEmpty()) 1958 && (data == null || data.isEmpty()); 1959 } 1960 1961 @Override 1962 public ResourceType getResourceType() { 1963 return ResourceType.MessageHeader; 1964 } 1965 1966 @SearchParamDefinition(name = "code", path = "MessageHeader.response.code", description = "ok | transient-error | fatal-error", type = "token") 1967 public static final String SP_CODE = "code"; 1968 @SearchParamDefinition(name = "data", path = "MessageHeader.data", description = "The actual content of the message", type = "reference") 1969 public static final String SP_DATA = "data"; 1970 @SearchParamDefinition(name = "receiver", path = "MessageHeader.receiver", description = "Intended \"real-world\" recipient for the data", type = "reference") 1971 public static final String SP_RECEIVER = "receiver"; 1972 @SearchParamDefinition(name = "author", path = "MessageHeader.author", description = "The source of the decision", type = "reference") 1973 public static final String SP_AUTHOR = "author"; 1974 @SearchParamDefinition(name = "destination", path = "MessageHeader.destination.name", description = "Name of system", type = "string") 1975 public static final String SP_DESTINATION = "destination"; 1976 @SearchParamDefinition(name = "source", path = "MessageHeader.source.name", description = "Name of system", type = "string") 1977 public static final String SP_SOURCE = "source"; 1978 @SearchParamDefinition(name = "target", path = "MessageHeader.destination.target", description = "Particular delivery destination within the destination", type = "reference") 1979 public static final String SP_TARGET = "target"; 1980 @SearchParamDefinition(name = "destination-uri", path = "MessageHeader.destination.endpoint", description = "Actual destination address or id", type = "uri") 1981 public static final String SP_DESTINATIONURI = "destination-uri"; 1982 @SearchParamDefinition(name = "source-uri", path = "MessageHeader.source.endpoint", description = "Actual message source address or id", type = "uri") 1983 public static final String SP_SOURCEURI = "source-uri"; 1984 @SearchParamDefinition(name = "responsible", path = "MessageHeader.responsible", description = "Final responsibility for event", type = "reference") 1985 public static final String SP_RESPONSIBLE = "responsible"; 1986 @SearchParamDefinition(name = "response-id", path = "MessageHeader.response.identifier", description = "Id of original message", type = "token") 1987 public static final String SP_RESPONSEID = "response-id"; 1988 @SearchParamDefinition(name = "enterer", path = "MessageHeader.enterer", description = "The source of the data entry", type = "reference") 1989 public static final String SP_ENTERER = "enterer"; 1990 @SearchParamDefinition(name = "event", path = "MessageHeader.event", description = "Code for the event this message represents", type = "token") 1991 public static final String SP_EVENT = "event"; 1992 @SearchParamDefinition(name = "timestamp", path = "MessageHeader.timestamp", description = "Time that the message was sent", type = "date") 1993 public static final String SP_TIMESTAMP = "timestamp"; 1994 1995}