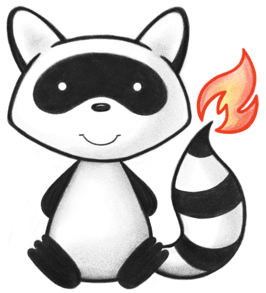
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.DatatypeDef; 039import ca.uhn.fhir.model.api.annotation.Description; 040import org.hl7.fhir.instance.model.api.IBaseMetaType; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * The metadata about a resource. This is content in the resource that is 046 * maintained by the infrastructure. Changes to the content may not always be 047 * associated with version changes to the resource. 048 */ 049@DatatypeDef(name = "Meta") 050public class Meta extends Type implements IBaseMetaType { 051 052 /** 053 * The version specific identifier, as it appears in the version portion of the 054 * URL. This values changes when the resource is created, updated, or deleted. 055 */ 056 @Child(name = "versionId", type = { IdType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 057 @Description(shortDefinition = "Version specific identifier", formalDefinition = "The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted.") 058 protected IdType versionId; 059 060 /** 061 * When the resource last changed - e.g. when the version changed. 062 */ 063 @Child(name = "lastUpdated", type = { 064 InstantType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 065 @Description(shortDefinition = "When the resource version last changed", formalDefinition = "When the resource last changed - e.g. when the version changed.") 066 protected InstantType lastUpdated; 067 068 /** 069 * A list of profiles [[[StructureDefinition]]]s that this resource claims to 070 * conform to. The URL is a reference to [[[StructureDefinition.url]]]. 071 */ 072 @Child(name = "profile", type = { 073 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 074 @Description(shortDefinition = "Profiles this resource claims to conform to", formalDefinition = "A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].") 075 protected List<UriType> profile; 076 077 /** 078 * Security labels applied to this resource. These tags connect specific 079 * resources to the overall security policy and infrastructure. 080 */ 081 @Child(name = "security", type = { 082 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 083 @Description(shortDefinition = "Security Labels applied to this resource", formalDefinition = "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.") 084 protected List<Coding> security; 085 086 /** 087 * Tags applied to this resource. Tags are intended to be used to identify and 088 * relate resources to process and workflow, and applications are not required 089 * to consider the tags when interpreting the meaning of a resource. 090 */ 091 @Child(name = "tag", type = { 092 Coding.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 093 @Description(shortDefinition = "Tags applied to this resource", formalDefinition = "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.") 094 protected List<Coding> tag; 095 096 private static final long serialVersionUID = 867134915L; 097 098 /* 099 * Constructor 100 */ 101 public Meta() { 102 super(); 103 } 104 105 /** 106 * @return {@link #versionId} (The version specific identifier, as it appears in 107 * the version portion of the URL. This values changes when the resource 108 * is created, updated, or deleted.). This is the underlying object with 109 * id, value and extensions. The accessor "getVersionId" gives direct 110 * access to the value 111 */ 112 public IdType getVersionIdElement() { 113 if (this.versionId == null) 114 if (Configuration.errorOnAutoCreate()) 115 throw new Error("Attempt to auto-create Meta.versionId"); 116 else if (Configuration.doAutoCreate()) 117 this.versionId = new IdType(); // bb 118 return this.versionId; 119 } 120 121 public boolean hasVersionIdElement() { 122 return this.versionId != null && !this.versionId.isEmpty(); 123 } 124 125 public boolean hasVersionId() { 126 return this.versionId != null && !this.versionId.isEmpty(); 127 } 128 129 /** 130 * @param value {@link #versionId} (The version specific identifier, as it 131 * appears in the version portion of the URL. This values changes 132 * when the resource is created, updated, or deleted.). This is the 133 * underlying object with id, value and extensions. The accessor 134 * "getVersionId" gives direct access to the value 135 */ 136 public Meta setVersionIdElement(IdType value) { 137 this.versionId = value; 138 return this; 139 } 140 141 /** 142 * @return The version specific identifier, as it appears in the version portion 143 * of the URL. This values changes when the resource is created, 144 * updated, or deleted. 145 */ 146 public String getVersionId() { 147 return this.versionId == null ? null : this.versionId.getValue(); 148 } 149 150 /** 151 * @param value The version specific identifier, as it appears in the version 152 * portion of the URL. This values changes when the resource is 153 * created, updated, or deleted. 154 */ 155 public Meta setVersionId(String value) { 156 if (Utilities.noString(value)) 157 this.versionId = null; 158 else { 159 if (this.versionId == null) 160 this.versionId = new IdType(); 161 this.versionId.setValue(value); 162 } 163 return this; 164 } 165 166 /** 167 * @return {@link #lastUpdated} (When the resource last changed - e.g. when the 168 * version changed.). This is the underlying object with id, value and 169 * extensions. The accessor "getLastUpdated" gives direct access to the 170 * value 171 */ 172 public InstantType getLastUpdatedElement() { 173 if (this.lastUpdated == null) 174 if (Configuration.errorOnAutoCreate()) 175 throw new Error("Attempt to auto-create Meta.lastUpdated"); 176 else if (Configuration.doAutoCreate()) 177 this.lastUpdated = new InstantType(); // bb 178 return this.lastUpdated; 179 } 180 181 public boolean hasLastUpdatedElement() { 182 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 183 } 184 185 public boolean hasLastUpdated() { 186 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 187 } 188 189 /** 190 * @param value {@link #lastUpdated} (When the resource last changed - e.g. when 191 * the version changed.). This is the underlying object with id, 192 * value and extensions. The accessor "getLastUpdated" gives direct 193 * access to the value 194 */ 195 public Meta setLastUpdatedElement(InstantType value) { 196 this.lastUpdated = value; 197 return this; 198 } 199 200 /** 201 * @return When the resource last changed - e.g. when the version changed. 202 */ 203 public Date getLastUpdated() { 204 return this.lastUpdated == null ? null : this.lastUpdated.getValue(); 205 } 206 207 /** 208 * @param value When the resource last changed - e.g. when the version changed. 209 */ 210 public Meta setLastUpdated(Date value) { 211 if (value == null) 212 this.lastUpdated = null; 213 else { 214 if (this.lastUpdated == null) 215 this.lastUpdated = new InstantType(); 216 this.lastUpdated.setValue(value); 217 } 218 return this; 219 } 220 221 /** 222 * @return {@link #profile} (A list of profiles [[[StructureDefinition]]]s that 223 * this resource claims to conform to. The URL is a reference to 224 * [[[StructureDefinition.url]]].) 225 */ 226 public List<UriType> getProfile() { 227 if (this.profile == null) 228 this.profile = new ArrayList<UriType>(); 229 return this.profile; 230 } 231 232 public boolean hasProfile() { 233 if (this.profile == null) 234 return false; 235 for (UriType item : this.profile) 236 if (!item.isEmpty()) 237 return true; 238 return false; 239 } 240 241 /** 242 * @return {@link #profile} (A list of profiles [[[StructureDefinition]]]s that 243 * this resource claims to conform to. The URL is a reference to 244 * [[[StructureDefinition.url]]].) 245 */ 246 // syntactic sugar 247 public UriType addProfileElement() {// 2 248 UriType t = new UriType(); 249 if (this.profile == null) 250 this.profile = new ArrayList<UriType>(); 251 this.profile.add(t); 252 return t; 253 } 254 255 /** 256 * @param value {@link #profile} (A list of profiles [[[StructureDefinition]]]s 257 * that this resource claims to conform to. The URL is a reference 258 * to [[[StructureDefinition.url]]].) 259 */ 260 public Meta addProfile(String value) { // 1 261 UriType t = new UriType(); 262 t.setValue(value); 263 if (this.profile == null) 264 this.profile = new ArrayList<UriType>(); 265 this.profile.add(t); 266 return this; 267 } 268 269 /** 270 * @param value {@link #profile} (A list of profiles [[[StructureDefinition]]]s 271 * that this resource claims to conform to. The URL is a reference 272 * to [[[StructureDefinition.url]]].) 273 */ 274 public boolean hasProfile(String value) { 275 if (this.profile == null) 276 return false; 277 for (UriType v : this.profile) 278 if (v.equals(value)) // uri 279 return true; 280 return false; 281 } 282 283 /** 284 * @return {@link #security} (Security labels applied to this resource. These 285 * tags connect specific resources to the overall security policy and 286 * infrastructure.) 287 */ 288 public List<Coding> getSecurity() { 289 if (this.security == null) 290 this.security = new ArrayList<Coding>(); 291 return this.security; 292 } 293 294 /** 295 * Returns the first security label (if any) that has the given system and code, 296 * or returns <code>null</code> if none 297 */ 298 public Coding getSecurity(String theSystem, String theCode) { 299 for (Coding next : getSecurity()) { 300 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) 301 && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 302 return next; 303 } 304 } 305 return null; 306 } 307 308 public boolean hasSecurity() { 309 if (this.security == null) 310 return false; 311 for (Coding item : this.security) 312 if (!item.isEmpty()) 313 return true; 314 return false; 315 } 316 317 /** 318 * @return {@link #security} (Security labels applied to this resource. These 319 * tags connect specific resources to the overall security policy and 320 * infrastructure.) 321 */ 322 // syntactic sugar 323 public Coding addSecurity() { // 3 324 Coding t = new Coding(); 325 if (this.security == null) 326 this.security = new ArrayList<Coding>(); 327 this.security.add(t); 328 return t; 329 } 330 331 // syntactic sugar 332 public Meta addSecurity(Coding t) { // 3 333 if (t == null) 334 return this; 335 if (this.security == null) 336 this.security = new ArrayList<Coding>(); 337 this.security.add(t); 338 return this; 339 } 340 341 /** 342 * @return {@link #tag} (Tags applied to this resource. Tags are intended to be 343 * used to identify and relate resources to process and workflow, and 344 * applications are not required to consider the tags when interpreting 345 * the meaning of a resource.) 346 */ 347 public List<Coding> getTag() { 348 if (this.tag == null) 349 this.tag = new ArrayList<Coding>(); 350 return this.tag; 351 } 352 353 /** 354 * Returns the first tag (if any) that has the given system and code, or returns 355 * <code>null</code> if none 356 */ 357 public Coding getTag(String theSystem, String theCode) { 358 for (Coding next : getTag()) { 359 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) 360 && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 361 return next; 362 } 363 } 364 return null; 365 } 366 367 public boolean hasTag() { 368 if (this.tag == null) 369 return false; 370 for (Coding item : this.tag) 371 if (!item.isEmpty()) 372 return true; 373 return false; 374 } 375 376 /** 377 * @return {@link #tag} (Tags applied to this resource. Tags are intended to be 378 * used to identify and relate resources to process and workflow, and 379 * applications are not required to consider the tags when interpreting 380 * the meaning of a resource.) 381 */ 382 // syntactic sugar 383 public Coding addTag() { // 3 384 Coding t = new Coding(); 385 if (this.tag == null) 386 this.tag = new ArrayList<Coding>(); 387 this.tag.add(t); 388 return t; 389 } 390 391 // syntactic sugar 392 public Meta addTag(Coding t) { // 3 393 if (t == null) 394 return this; 395 if (this.tag == null) 396 this.tag = new ArrayList<Coding>(); 397 this.tag.add(t); 398 return this; 399 } 400 401 protected void listChildren(List<Property> childrenList) { 402 super.listChildren(childrenList); 403 childrenList.add(new Property("versionId", "id", 404 "The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted.", 405 0, java.lang.Integer.MAX_VALUE, versionId)); 406 childrenList 407 .add(new Property("lastUpdated", "instant", "When the resource last changed - e.g. when the version changed.", 408 0, java.lang.Integer.MAX_VALUE, lastUpdated)); 409 childrenList.add(new Property("profile", "uri", 410 "A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].", 411 0, java.lang.Integer.MAX_VALUE, profile)); 412 childrenList.add(new Property("security", "Coding", 413 "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.", 414 0, java.lang.Integer.MAX_VALUE, security)); 415 childrenList.add(new Property("tag", "Coding", 416 "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.", 417 0, java.lang.Integer.MAX_VALUE, tag)); 418 } 419 420 @Override 421 public void setProperty(String name, Base value) throws FHIRException { 422 if (name.equals("versionId")) 423 this.versionId = castToId(value); // IdType 424 else if (name.equals("lastUpdated")) 425 this.lastUpdated = castToInstant(value); // InstantType 426 else if (name.equals("profile")) 427 this.getProfile().add(castToUri(value)); 428 else if (name.equals("security")) 429 this.getSecurity().add(castToCoding(value)); 430 else if (name.equals("tag")) 431 this.getTag().add(castToCoding(value)); 432 else 433 super.setProperty(name, value); 434 } 435 436 @Override 437 public Base addChild(String name) throws FHIRException { 438 if (name.equals("versionId")) { 439 throw new FHIRException("Cannot call addChild on a singleton property Meta.versionId"); 440 } else if (name.equals("lastUpdated")) { 441 throw new FHIRException("Cannot call addChild on a singleton property Meta.lastUpdated"); 442 } else if (name.equals("profile")) { 443 throw new FHIRException("Cannot call addChild on a singleton property Meta.profile"); 444 } else if (name.equals("security")) { 445 return addSecurity(); 446 } else if (name.equals("tag")) { 447 return addTag(); 448 } else 449 return super.addChild(name); 450 } 451 452 public String fhirType() { 453 return "Meta"; 454 455 } 456 457 public Meta copy() { 458 Meta dst = new Meta(); 459 copyValues(dst); 460 dst.versionId = versionId == null ? null : versionId.copy(); 461 dst.lastUpdated = lastUpdated == null ? null : lastUpdated.copy(); 462 if (profile != null) { 463 dst.profile = new ArrayList<UriType>(); 464 for (UriType i : profile) 465 dst.profile.add(i.copy()); 466 } 467 ; 468 if (security != null) { 469 dst.security = new ArrayList<Coding>(); 470 for (Coding i : security) 471 dst.security.add(i.copy()); 472 } 473 ; 474 if (tag != null) { 475 dst.tag = new ArrayList<Coding>(); 476 for (Coding i : tag) 477 dst.tag.add(i.copy()); 478 } 479 ; 480 return dst; 481 } 482 483 protected Meta typedCopy() { 484 return copy(); 485 } 486 487 @Override 488 public boolean equalsDeep(Base other) { 489 if (!super.equalsDeep(other)) 490 return false; 491 if (!(other instanceof Meta)) 492 return false; 493 Meta o = (Meta) other; 494 return compareDeep(versionId, o.versionId, true) && compareDeep(lastUpdated, o.lastUpdated, true) 495 && compareDeep(profile, o.profile, true) && compareDeep(security, o.security, true) 496 && compareDeep(tag, o.tag, true); 497 } 498 499 @Override 500 public boolean equalsShallow(Base other) { 501 if (!super.equalsShallow(other)) 502 return false; 503 if (!(other instanceof Meta)) 504 return false; 505 Meta o = (Meta) other; 506 return compareValues(versionId, o.versionId, true) && compareValues(lastUpdated, o.lastUpdated, true) 507 && compareValues(profile, o.profile, true); 508 } 509 510 public boolean isEmpty() { 511 return super.isEmpty() && (versionId == null || versionId.isEmpty()) 512 && (lastUpdated == null || lastUpdated.isEmpty()) && (profile == null || profile.isEmpty()) 513 && (security == null || security.isEmpty()) && (tag == null || tag.isEmpty()); 514 } 515 516}