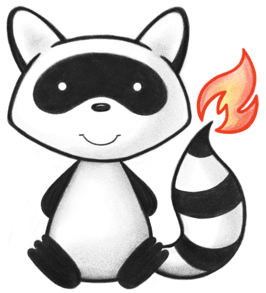
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A curated namespace that issues unique symbols within that namespace for the 050 * identification of concepts, people, devices, etc. Represents a "System" used 051 * within the Identifier and Coding data types. 052 */ 053@ResourceDef(name = "NamingSystem", profile = "http://hl7.org/fhir/Profile/NamingSystem") 054public class NamingSystem extends DomainResource { 055 056 public enum NamingSystemType { 057 /** 058 * The naming system is used to define concepts and symbols to represent those 059 * concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc. 060 */ 061 CODESYSTEM, 062 /** 063 * The naming system is used to manage identifiers (e.g. license numbers, order 064 * numbers, etc.). 065 */ 066 IDENTIFIER, 067 /** 068 * The naming system is used as the root for other identifiers and naming 069 * systems. 070 */ 071 ROOT, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static NamingSystemType fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("codesystem".equals(codeString)) 081 return CODESYSTEM; 082 if ("identifier".equals(codeString)) 083 return IDENTIFIER; 084 if ("root".equals(codeString)) 085 return ROOT; 086 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case CODESYSTEM: 092 return "codesystem"; 093 case IDENTIFIER: 094 return "identifier"; 095 case ROOT: 096 return "root"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case CODESYSTEM: 107 return "http://hl7.org/fhir/namingsystem-type"; 108 case IDENTIFIER: 109 return "http://hl7.org/fhir/namingsystem-type"; 110 case ROOT: 111 return "http://hl7.org/fhir/namingsystem-type"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case CODESYSTEM: 122 return "The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc."; 123 case IDENTIFIER: 124 return "The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.)."; 125 case ROOT: 126 return "The naming system is used as the root for other identifiers and naming systems."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case CODESYSTEM: 137 return "Code System"; 138 case IDENTIFIER: 139 return "Identifier"; 140 case ROOT: 141 return "Root"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class NamingSystemTypeEnumFactory implements EnumFactory<NamingSystemType> { 151 public NamingSystemType fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("codesystem".equals(codeString)) 156 return NamingSystemType.CODESYSTEM; 157 if ("identifier".equals(codeString)) 158 return NamingSystemType.IDENTIFIER; 159 if ("root".equals(codeString)) 160 return NamingSystemType.ROOT; 161 throw new IllegalArgumentException("Unknown NamingSystemType code '" + codeString + "'"); 162 } 163 164 public Enumeration<NamingSystemType> fromType(Base code) throws FHIRException { 165 if (code == null || code.isEmpty()) 166 return null; 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("codesystem".equals(codeString)) 171 return new Enumeration<NamingSystemType>(this, NamingSystemType.CODESYSTEM); 172 if ("identifier".equals(codeString)) 173 return new Enumeration<NamingSystemType>(this, NamingSystemType.IDENTIFIER); 174 if ("root".equals(codeString)) 175 return new Enumeration<NamingSystemType>(this, NamingSystemType.ROOT); 176 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 177 } 178 179 public String toCode(NamingSystemType code) { 180 if (code == NamingSystemType.CODESYSTEM) 181 return "codesystem"; 182 if (code == NamingSystemType.IDENTIFIER) 183 return "identifier"; 184 if (code == NamingSystemType.ROOT) 185 return "root"; 186 return "?"; 187 } 188 } 189 190 public enum NamingSystemIdentifierType { 191 /** 192 * An ISO object identifier; e.g. 1.2.3.4.5. 193 */ 194 OID, 195 /** 196 * A universally unique identifier of the form 197 * a5afddf4-e880-459b-876e-e4591b0acc11. 198 */ 199 UUID, 200 /** 201 * A uniform resource identifier (ideally a URL - uniform resource locator); 202 * e.g. http://unitsofmeasure.org. 203 */ 204 URI, 205 /** 206 * Some other type of unique identifier; e.g. HL7-assigned reserved string such 207 * as LN for LOINC. 208 */ 209 OTHER, 210 /** 211 * added to help the parsers 212 */ 213 NULL; 214 215 public static NamingSystemIdentifierType fromCode(String codeString) throws FHIRException { 216 if (codeString == null || "".equals(codeString)) 217 return null; 218 if ("oid".equals(codeString)) 219 return OID; 220 if ("uuid".equals(codeString)) 221 return UUID; 222 if ("uri".equals(codeString)) 223 return URI; 224 if ("other".equals(codeString)) 225 return OTHER; 226 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 227 } 228 229 public String toCode() { 230 switch (this) { 231 case OID: 232 return "oid"; 233 case UUID: 234 return "uuid"; 235 case URI: 236 return "uri"; 237 case OTHER: 238 return "other"; 239 case NULL: 240 return null; 241 default: 242 return "?"; 243 } 244 } 245 246 public String getSystem() { 247 switch (this) { 248 case OID: 249 return "http://hl7.org/fhir/namingsystem-identifier-type"; 250 case UUID: 251 return "http://hl7.org/fhir/namingsystem-identifier-type"; 252 case URI: 253 return "http://hl7.org/fhir/namingsystem-identifier-type"; 254 case OTHER: 255 return "http://hl7.org/fhir/namingsystem-identifier-type"; 256 case NULL: 257 return null; 258 default: 259 return "?"; 260 } 261 } 262 263 public String getDefinition() { 264 switch (this) { 265 case OID: 266 return "An ISO object identifier; e.g. 1.2.3.4.5."; 267 case UUID: 268 return "A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11."; 269 case URI: 270 return "A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org."; 271 case OTHER: 272 return "Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC."; 273 case NULL: 274 return null; 275 default: 276 return "?"; 277 } 278 } 279 280 public String getDisplay() { 281 switch (this) { 282 case OID: 283 return "OID"; 284 case UUID: 285 return "UUID"; 286 case URI: 287 return "URI"; 288 case OTHER: 289 return "Other"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 } 297 298 public static class NamingSystemIdentifierTypeEnumFactory implements EnumFactory<NamingSystemIdentifierType> { 299 public NamingSystemIdentifierType fromCode(String codeString) throws IllegalArgumentException { 300 if (codeString == null || "".equals(codeString)) 301 if (codeString == null || "".equals(codeString)) 302 return null; 303 if ("oid".equals(codeString)) 304 return NamingSystemIdentifierType.OID; 305 if ("uuid".equals(codeString)) 306 return NamingSystemIdentifierType.UUID; 307 if ("uri".equals(codeString)) 308 return NamingSystemIdentifierType.URI; 309 if ("other".equals(codeString)) 310 return NamingSystemIdentifierType.OTHER; 311 throw new IllegalArgumentException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 312 } 313 314 public Enumeration<NamingSystemIdentifierType> fromType(Base code) throws FHIRException { 315 if (code == null || code.isEmpty()) 316 return null; 317 String codeString = ((PrimitiveType) code).asStringValue(); 318 if (codeString == null || "".equals(codeString)) 319 return null; 320 if ("oid".equals(codeString)) 321 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OID); 322 if ("uuid".equals(codeString)) 323 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.UUID); 324 if ("uri".equals(codeString)) 325 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.URI); 326 if ("other".equals(codeString)) 327 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OTHER); 328 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 329 } 330 331 public String toCode(NamingSystemIdentifierType code) { 332 if (code == NamingSystemIdentifierType.OID) 333 return "oid"; 334 if (code == NamingSystemIdentifierType.UUID) 335 return "uuid"; 336 if (code == NamingSystemIdentifierType.URI) 337 return "uri"; 338 if (code == NamingSystemIdentifierType.OTHER) 339 return "other"; 340 return "?"; 341 } 342 } 343 344 @Block() 345 public static class NamingSystemContactComponent extends BackboneElement implements IBaseBackboneElement { 346 /** 347 * The name of an individual to contact regarding the naming system. 348 */ 349 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 350 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the naming system.") 351 protected StringType name; 352 353 /** 354 * Contact details for individual (if a name was provided) or the publisher. 355 */ 356 @Child(name = "telecom", type = { 357 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 358 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 359 protected List<ContactPoint> telecom; 360 361 private static final long serialVersionUID = -1179697803L; 362 363 /* 364 * Constructor 365 */ 366 public NamingSystemContactComponent() { 367 super(); 368 } 369 370 /** 371 * @return {@link #name} (The name of an individual to contact regarding the 372 * naming system.). This is the underlying object with id, value and 373 * extensions. The accessor "getName" gives direct access to the value 374 */ 375 public StringType getNameElement() { 376 if (this.name == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create NamingSystemContactComponent.name"); 379 else if (Configuration.doAutoCreate()) 380 this.name = new StringType(); // bb 381 return this.name; 382 } 383 384 public boolean hasNameElement() { 385 return this.name != null && !this.name.isEmpty(); 386 } 387 388 public boolean hasName() { 389 return this.name != null && !this.name.isEmpty(); 390 } 391 392 /** 393 * @param value {@link #name} (The name of an individual to contact regarding 394 * the naming system.). This is the underlying object with id, 395 * value and extensions. The accessor "getName" gives direct access 396 * to the value 397 */ 398 public NamingSystemContactComponent setNameElement(StringType value) { 399 this.name = value; 400 return this; 401 } 402 403 /** 404 * @return The name of an individual to contact regarding the naming system. 405 */ 406 public String getName() { 407 return this.name == null ? null : this.name.getValue(); 408 } 409 410 /** 411 * @param value The name of an individual to contact regarding the naming 412 * system. 413 */ 414 public NamingSystemContactComponent setName(String value) { 415 if (Utilities.noString(value)) 416 this.name = null; 417 else { 418 if (this.name == null) 419 this.name = new StringType(); 420 this.name.setValue(value); 421 } 422 return this; 423 } 424 425 /** 426 * @return {@link #telecom} (Contact details for individual (if a name was 427 * provided) or the publisher.) 428 */ 429 public List<ContactPoint> getTelecom() { 430 if (this.telecom == null) 431 this.telecom = new ArrayList<ContactPoint>(); 432 return this.telecom; 433 } 434 435 public boolean hasTelecom() { 436 if (this.telecom == null) 437 return false; 438 for (ContactPoint item : this.telecom) 439 if (!item.isEmpty()) 440 return true; 441 return false; 442 } 443 444 /** 445 * @return {@link #telecom} (Contact details for individual (if a name was 446 * provided) or the publisher.) 447 */ 448 // syntactic sugar 449 public ContactPoint addTelecom() { // 3 450 ContactPoint t = new ContactPoint(); 451 if (this.telecom == null) 452 this.telecom = new ArrayList<ContactPoint>(); 453 this.telecom.add(t); 454 return t; 455 } 456 457 // syntactic sugar 458 public NamingSystemContactComponent addTelecom(ContactPoint t) { // 3 459 if (t == null) 460 return this; 461 if (this.telecom == null) 462 this.telecom = new ArrayList<ContactPoint>(); 463 this.telecom.add(t); 464 return this; 465 } 466 467 protected void listChildren(List<Property> childrenList) { 468 super.listChildren(childrenList); 469 childrenList.add(new Property("name", "string", 470 "The name of an individual to contact regarding the naming system.", 0, java.lang.Integer.MAX_VALUE, name)); 471 childrenList.add(new Property("telecom", "ContactPoint", 472 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 473 telecom)); 474 } 475 476 @Override 477 public void setProperty(String name, Base value) throws FHIRException { 478 if (name.equals("name")) 479 this.name = castToString(value); // StringType 480 else if (name.equals("telecom")) 481 this.getTelecom().add(castToContactPoint(value)); 482 else 483 super.setProperty(name, value); 484 } 485 486 @Override 487 public Base addChild(String name) throws FHIRException { 488 if (name.equals("name")) { 489 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 490 } else if (name.equals("telecom")) { 491 return addTelecom(); 492 } else 493 return super.addChild(name); 494 } 495 496 public NamingSystemContactComponent copy() { 497 NamingSystemContactComponent dst = new NamingSystemContactComponent(); 498 copyValues(dst); 499 dst.name = name == null ? null : name.copy(); 500 if (telecom != null) { 501 dst.telecom = new ArrayList<ContactPoint>(); 502 for (ContactPoint i : telecom) 503 dst.telecom.add(i.copy()); 504 } 505 ; 506 return dst; 507 } 508 509 @Override 510 public boolean equalsDeep(Base other) { 511 if (!super.equalsDeep(other)) 512 return false; 513 if (!(other instanceof NamingSystemContactComponent)) 514 return false; 515 NamingSystemContactComponent o = (NamingSystemContactComponent) other; 516 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 517 } 518 519 @Override 520 public boolean equalsShallow(Base other) { 521 if (!super.equalsShallow(other)) 522 return false; 523 if (!(other instanceof NamingSystemContactComponent)) 524 return false; 525 NamingSystemContactComponent o = (NamingSystemContactComponent) other; 526 return compareValues(name, o.name, true); 527 } 528 529 public boolean isEmpty() { 530 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 531 } 532 533 public String fhirType() { 534 return "NamingSystem.contact"; 535 536 } 537 538 } 539 540 @Block() 541 public static class NamingSystemUniqueIdComponent extends BackboneElement implements IBaseBackboneElement { 542 /** 543 * Identifies the unique identifier scheme used for this particular identifier. 544 */ 545 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 546 @Description(shortDefinition = "oid | uuid | uri | other", formalDefinition = "Identifies the unique identifier scheme used for this particular identifier.") 547 protected Enumeration<NamingSystemIdentifierType> type; 548 549 /** 550 * The string that should be sent over the wire to identify the code system or 551 * identifier system. 552 */ 553 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 554 @Description(shortDefinition = "The unique identifier", formalDefinition = "The string that should be sent over the wire to identify the code system or identifier system.") 555 protected StringType value; 556 557 /** 558 * Indicates whether this identifier is the "preferred" identifier of this type. 559 */ 560 @Child(name = "preferred", type = { 561 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 562 @Description(shortDefinition = "Is this the id that should be used for this type", formalDefinition = "Indicates whether this identifier is the \"preferred\" identifier of this type.") 563 protected BooleanType preferred; 564 565 /** 566 * Identifies the period of time over which this identifier is considered 567 * appropriate to refer to the naming system. Outside of this window, the 568 * identifier might be non-deterministic. 569 */ 570 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 571 @Description(shortDefinition = "When is identifier valid?", formalDefinition = "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.") 572 protected Period period; 573 574 private static final long serialVersionUID = -193711840L; 575 576 /* 577 * Constructor 578 */ 579 public NamingSystemUniqueIdComponent() { 580 super(); 581 } 582 583 /* 584 * Constructor 585 */ 586 public NamingSystemUniqueIdComponent(Enumeration<NamingSystemIdentifierType> type, StringType value) { 587 super(); 588 this.type = type; 589 this.value = value; 590 } 591 592 /** 593 * @return {@link #type} (Identifies the unique identifier scheme used for this 594 * particular identifier.). This is the underlying object with id, value 595 * and extensions. The accessor "getType" gives direct access to the 596 * value 597 */ 598 public Enumeration<NamingSystemIdentifierType> getTypeElement() { 599 if (this.type == null) 600 if (Configuration.errorOnAutoCreate()) 601 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.type"); 602 else if (Configuration.doAutoCreate()) 603 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); // bb 604 return this.type; 605 } 606 607 public boolean hasTypeElement() { 608 return this.type != null && !this.type.isEmpty(); 609 } 610 611 public boolean hasType() { 612 return this.type != null && !this.type.isEmpty(); 613 } 614 615 /** 616 * @param value {@link #type} (Identifies the unique identifier scheme used for 617 * this particular identifier.). This is the underlying object with 618 * id, value and extensions. The accessor "getType" gives direct 619 * access to the value 620 */ 621 public NamingSystemUniqueIdComponent setTypeElement(Enumeration<NamingSystemIdentifierType> value) { 622 this.type = value; 623 return this; 624 } 625 626 /** 627 * @return Identifies the unique identifier scheme used for this particular 628 * identifier. 629 */ 630 public NamingSystemIdentifierType getType() { 631 return this.type == null ? null : this.type.getValue(); 632 } 633 634 /** 635 * @param value Identifies the unique identifier scheme used for this particular 636 * identifier. 637 */ 638 public NamingSystemUniqueIdComponent setType(NamingSystemIdentifierType value) { 639 if (this.type == null) 640 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); 641 this.type.setValue(value); 642 return this; 643 } 644 645 /** 646 * @return {@link #value} (The string that should be sent over the wire to 647 * identify the code system or identifier system.). This is the 648 * underlying object with id, value and extensions. The accessor 649 * "getValue" gives direct access to the value 650 */ 651 public StringType getValueElement() { 652 if (this.value == null) 653 if (Configuration.errorOnAutoCreate()) 654 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.value"); 655 else if (Configuration.doAutoCreate()) 656 this.value = new StringType(); // bb 657 return this.value; 658 } 659 660 public boolean hasValueElement() { 661 return this.value != null && !this.value.isEmpty(); 662 } 663 664 public boolean hasValue() { 665 return this.value != null && !this.value.isEmpty(); 666 } 667 668 /** 669 * @param value {@link #value} (The string that should be sent over the wire to 670 * identify the code system or identifier system.). This is the 671 * underlying object with id, value and extensions. The accessor 672 * "getValue" gives direct access to the value 673 */ 674 public NamingSystemUniqueIdComponent setValueElement(StringType value) { 675 this.value = value; 676 return this; 677 } 678 679 /** 680 * @return The string that should be sent over the wire to identify the code 681 * system or identifier system. 682 */ 683 public String getValue() { 684 return this.value == null ? null : this.value.getValue(); 685 } 686 687 /** 688 * @param value The string that should be sent over the wire to identify the 689 * code system or identifier system. 690 */ 691 public NamingSystemUniqueIdComponent setValue(String value) { 692 if (this.value == null) 693 this.value = new StringType(); 694 this.value.setValue(value); 695 return this; 696 } 697 698 /** 699 * @return {@link #preferred} (Indicates whether this identifier is the 700 * "preferred" identifier of this type.). This is the underlying object 701 * with id, value and extensions. The accessor "getPreferred" gives 702 * direct access to the value 703 */ 704 public BooleanType getPreferredElement() { 705 if (this.preferred == null) 706 if (Configuration.errorOnAutoCreate()) 707 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.preferred"); 708 else if (Configuration.doAutoCreate()) 709 this.preferred = new BooleanType(); // bb 710 return this.preferred; 711 } 712 713 public boolean hasPreferredElement() { 714 return this.preferred != null && !this.preferred.isEmpty(); 715 } 716 717 public boolean hasPreferred() { 718 return this.preferred != null && !this.preferred.isEmpty(); 719 } 720 721 /** 722 * @param value {@link #preferred} (Indicates whether this identifier is the 723 * "preferred" identifier of this type.). This is the underlying 724 * object with id, value and extensions. The accessor 725 * "getPreferred" gives direct access to the value 726 */ 727 public NamingSystemUniqueIdComponent setPreferredElement(BooleanType value) { 728 this.preferred = value; 729 return this; 730 } 731 732 /** 733 * @return Indicates whether this identifier is the "preferred" identifier of 734 * this type. 735 */ 736 public boolean getPreferred() { 737 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 738 } 739 740 /** 741 * @param value Indicates whether this identifier is the "preferred" identifier 742 * of this type. 743 */ 744 public NamingSystemUniqueIdComponent setPreferred(boolean value) { 745 if (this.preferred == null) 746 this.preferred = new BooleanType(); 747 this.preferred.setValue(value); 748 return this; 749 } 750 751 /** 752 * @return {@link #period} (Identifies the period of time over which this 753 * identifier is considered appropriate to refer to the naming system. 754 * Outside of this window, the identifier might be non-deterministic.) 755 */ 756 public Period getPeriod() { 757 if (this.period == null) 758 if (Configuration.errorOnAutoCreate()) 759 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.period"); 760 else if (Configuration.doAutoCreate()) 761 this.period = new Period(); // cc 762 return this.period; 763 } 764 765 public boolean hasPeriod() { 766 return this.period != null && !this.period.isEmpty(); 767 } 768 769 /** 770 * @param value {@link #period} (Identifies the period of time over which this 771 * identifier is considered appropriate to refer to the naming 772 * system. Outside of this window, the identifier might be 773 * non-deterministic.) 774 */ 775 public NamingSystemUniqueIdComponent setPeriod(Period value) { 776 this.period = value; 777 return this; 778 } 779 780 protected void listChildren(List<Property> childrenList) { 781 super.listChildren(childrenList); 782 childrenList.add( 783 new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 784 0, java.lang.Integer.MAX_VALUE, type)); 785 childrenList.add(new Property("value", "string", 786 "The string that should be sent over the wire to identify the code system or identifier system.", 0, 787 java.lang.Integer.MAX_VALUE, value)); 788 childrenList.add(new Property("preferred", "boolean", 789 "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 790 java.lang.Integer.MAX_VALUE, preferred)); 791 childrenList.add(new Property("period", "Period", 792 "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 793 0, java.lang.Integer.MAX_VALUE, period)); 794 } 795 796 @Override 797 public void setProperty(String name, Base value) throws FHIRException { 798 if (name.equals("type")) 799 this.type = new NamingSystemIdentifierTypeEnumFactory().fromType(value); // Enumeration<NamingSystemIdentifierType> 800 else if (name.equals("value")) 801 this.value = castToString(value); // StringType 802 else if (name.equals("preferred")) 803 this.preferred = castToBoolean(value); // BooleanType 804 else if (name.equals("period")) 805 this.period = castToPeriod(value); // Period 806 else 807 super.setProperty(name, value); 808 } 809 810 @Override 811 public Base addChild(String name) throws FHIRException { 812 if (name.equals("type")) { 813 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.type"); 814 } else if (name.equals("value")) { 815 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.value"); 816 } else if (name.equals("preferred")) { 817 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.preferred"); 818 } else if (name.equals("period")) { 819 this.period = new Period(); 820 return this.period; 821 } else 822 return super.addChild(name); 823 } 824 825 public NamingSystemUniqueIdComponent copy() { 826 NamingSystemUniqueIdComponent dst = new NamingSystemUniqueIdComponent(); 827 copyValues(dst); 828 dst.type = type == null ? null : type.copy(); 829 dst.value = value == null ? null : value.copy(); 830 dst.preferred = preferred == null ? null : preferred.copy(); 831 dst.period = period == null ? null : period.copy(); 832 return dst; 833 } 834 835 @Override 836 public boolean equalsDeep(Base other) { 837 if (!super.equalsDeep(other)) 838 return false; 839 if (!(other instanceof NamingSystemUniqueIdComponent)) 840 return false; 841 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other; 842 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) 843 && compareDeep(preferred, o.preferred, true) && compareDeep(period, o.period, true); 844 } 845 846 @Override 847 public boolean equalsShallow(Base other) { 848 if (!super.equalsShallow(other)) 849 return false; 850 if (!(other instanceof NamingSystemUniqueIdComponent)) 851 return false; 852 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other; 853 return compareValues(type, o.type, true) && compareValues(value, o.value, true) 854 && compareValues(preferred, o.preferred, true); 855 } 856 857 public boolean isEmpty() { 858 return super.isEmpty() && (type == null || type.isEmpty()) && (value == null || value.isEmpty()) 859 && (preferred == null || preferred.isEmpty()) && (period == null || period.isEmpty()); 860 } 861 862 public String fhirType() { 863 return "NamingSystem.uniqueId"; 864 865 } 866 867 } 868 869 /** 870 * The descriptive name of this particular identifier type or code system. 871 */ 872 @Child(name = "name", type = { StringType.class }, order = 0, min = 1, max = 1, modifier = false, summary = false) 873 @Description(shortDefinition = "Human-readable label", formalDefinition = "The descriptive name of this particular identifier type or code system.") 874 protected StringType name; 875 876 /** 877 * Indicates whether the naming system is "ready for use" or not. 878 */ 879 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = false) 880 @Description(shortDefinition = "draft | active | retired", formalDefinition = "Indicates whether the naming system is \"ready for use\" or not.") 881 protected Enumeration<ConformanceResourceStatus> status; 882 883 /** 884 * Indicates the purpose for the naming system - what kinds of things does it 885 * make unique? 886 */ 887 @Child(name = "kind", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 888 @Description(shortDefinition = "codesystem | identifier | root", formalDefinition = "Indicates the purpose for the naming system - what kinds of things does it make unique?") 889 protected Enumeration<NamingSystemType> kind; 890 891 /** 892 * The name of the individual or organization that published the naming system. 893 */ 894 @Child(name = "publisher", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 895 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the naming system.") 896 protected StringType publisher; 897 898 /** 899 * Contacts to assist a user in finding and communicating with the publisher. 900 */ 901 @Child(name = "contact", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 902 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 903 protected List<NamingSystemContactComponent> contact; 904 905 /** 906 * The name of the organization that is responsible for issuing identifiers or 907 * codes for this namespace and ensuring their non-collision. 908 */ 909 @Child(name = "responsible", type = { 910 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 911 @Description(shortDefinition = "Who maintains system namespace?", formalDefinition = "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.") 912 protected StringType responsible; 913 914 /** 915 * The date (and optionally time) when the system was registered or published. 916 * The date must change when the business version changes, if it does, and it 917 * must change if the status code changes. In addition, it should change when 918 * the substantive content of the registration changes. 919 */ 920 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 921 @Description(shortDefinition = "Publication Date(/time)", formalDefinition = "The date (and optionally time) when the system was registered or published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the registration changes.") 922 protected DateTimeType date; 923 924 /** 925 * Categorizes a naming system for easier search by grouping related naming 926 * systems. 927 */ 928 @Child(name = "type", type = { 929 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 930 @Description(shortDefinition = "e.g. driver, provider, patient, bank etc.", formalDefinition = "Categorizes a naming system for easier search by grouping related naming systems.") 931 protected CodeableConcept type; 932 933 /** 934 * Details about what the namespace identifies including scope, granularity, 935 * version labeling, etc. 936 */ 937 @Child(name = "description", type = { 938 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 939 @Description(shortDefinition = "What does naming system identify?", formalDefinition = "Details about what the namespace identifies including scope, granularity, version labeling, etc.") 940 protected StringType description; 941 942 /** 943 * The content was developed with a focus and intent of supporting the contexts 944 * that are listed. These terms may be used to assist with indexing and 945 * searching of naming systems. 946 */ 947 @Child(name = "useContext", type = { 948 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 949 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of naming systems.") 950 protected List<CodeableConcept> useContext; 951 952 /** 953 * Provides guidance on the use of the namespace, including the handling of 954 * formatting characters, use of upper vs. lower case, etc. 955 */ 956 @Child(name = "usage", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 957 @Description(shortDefinition = "How/where is it used", formalDefinition = "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.") 958 protected StringType usage; 959 960 /** 961 * Indicates how the system may be identified when referenced in electronic 962 * exchange. 963 */ 964 @Child(name = "uniqueId", type = {}, order = 11, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 965 @Description(shortDefinition = "Unique identifiers used for system", formalDefinition = "Indicates how the system may be identified when referenced in electronic exchange.") 966 protected List<NamingSystemUniqueIdComponent> uniqueId; 967 968 /** 969 * For naming systems that are retired, indicates the naming system that should 970 * be used in their place (if any). 971 */ 972 @Child(name = "replacedBy", type = { 973 NamingSystem.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 974 @Description(shortDefinition = "Use this instead", formalDefinition = "For naming systems that are retired, indicates the naming system that should be used in their place (if any).") 975 protected Reference replacedBy; 976 977 /** 978 * The actual object that is the target of the reference (For naming systems 979 * that are retired, indicates the naming system that should be used in their 980 * place (if any).) 981 */ 982 protected NamingSystem replacedByTarget; 983 984 private static final long serialVersionUID = -1337110053L; 985 986 /* 987 * Constructor 988 */ 989 public NamingSystem() { 990 super(); 991 } 992 993 /* 994 * Constructor 995 */ 996 public NamingSystem(StringType name, Enumeration<ConformanceResourceStatus> status, 997 Enumeration<NamingSystemType> kind, DateTimeType date) { 998 super(); 999 this.name = name; 1000 this.status = status; 1001 this.kind = kind; 1002 this.date = date; 1003 } 1004 1005 /** 1006 * @return {@link #name} (The descriptive name of this particular identifier 1007 * type or code system.). This is the underlying object with id, value 1008 * and extensions. The accessor "getName" gives direct access to the 1009 * value 1010 */ 1011 public StringType getNameElement() { 1012 if (this.name == null) 1013 if (Configuration.errorOnAutoCreate()) 1014 throw new Error("Attempt to auto-create NamingSystem.name"); 1015 else if (Configuration.doAutoCreate()) 1016 this.name = new StringType(); // bb 1017 return this.name; 1018 } 1019 1020 public boolean hasNameElement() { 1021 return this.name != null && !this.name.isEmpty(); 1022 } 1023 1024 public boolean hasName() { 1025 return this.name != null && !this.name.isEmpty(); 1026 } 1027 1028 /** 1029 * @param value {@link #name} (The descriptive name of this particular 1030 * identifier type or code system.). This is the underlying object 1031 * with id, value and extensions. The accessor "getName" gives 1032 * direct access to the value 1033 */ 1034 public NamingSystem setNameElement(StringType value) { 1035 this.name = value; 1036 return this; 1037 } 1038 1039 /** 1040 * @return The descriptive name of this particular identifier type or code 1041 * system. 1042 */ 1043 public String getName() { 1044 return this.name == null ? null : this.name.getValue(); 1045 } 1046 1047 /** 1048 * @param value The descriptive name of this particular identifier type or code 1049 * system. 1050 */ 1051 public NamingSystem setName(String value) { 1052 if (this.name == null) 1053 this.name = new StringType(); 1054 this.name.setValue(value); 1055 return this; 1056 } 1057 1058 /** 1059 * @return {@link #status} (Indicates whether the naming system is "ready for 1060 * use" or not.). This is the underlying object with id, value and 1061 * extensions. The accessor "getStatus" gives direct access to the value 1062 */ 1063 public Enumeration<ConformanceResourceStatus> getStatusElement() { 1064 if (this.status == null) 1065 if (Configuration.errorOnAutoCreate()) 1066 throw new Error("Attempt to auto-create NamingSystem.status"); 1067 else if (Configuration.doAutoCreate()) 1068 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 1069 return this.status; 1070 } 1071 1072 public boolean hasStatusElement() { 1073 return this.status != null && !this.status.isEmpty(); 1074 } 1075 1076 public boolean hasStatus() { 1077 return this.status != null && !this.status.isEmpty(); 1078 } 1079 1080 /** 1081 * @param value {@link #status} (Indicates whether the naming system is "ready 1082 * for use" or not.). This is the underlying object with id, value 1083 * and extensions. The accessor "getStatus" gives direct access to 1084 * the value 1085 */ 1086 public NamingSystem setStatusElement(Enumeration<ConformanceResourceStatus> value) { 1087 this.status = value; 1088 return this; 1089 } 1090 1091 /** 1092 * @return Indicates whether the naming system is "ready for use" or not. 1093 */ 1094 public ConformanceResourceStatus getStatus() { 1095 return this.status == null ? null : this.status.getValue(); 1096 } 1097 1098 /** 1099 * @param value Indicates whether the naming system is "ready for use" or not. 1100 */ 1101 public NamingSystem setStatus(ConformanceResourceStatus value) { 1102 if (this.status == null) 1103 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 1104 this.status.setValue(value); 1105 return this; 1106 } 1107 1108 /** 1109 * @return {@link #kind} (Indicates the purpose for the naming system - what 1110 * kinds of things does it make unique?). This is the underlying object 1111 * with id, value and extensions. The accessor "getKind" gives direct 1112 * access to the value 1113 */ 1114 public Enumeration<NamingSystemType> getKindElement() { 1115 if (this.kind == null) 1116 if (Configuration.errorOnAutoCreate()) 1117 throw new Error("Attempt to auto-create NamingSystem.kind"); 1118 else if (Configuration.doAutoCreate()) 1119 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); // bb 1120 return this.kind; 1121 } 1122 1123 public boolean hasKindElement() { 1124 return this.kind != null && !this.kind.isEmpty(); 1125 } 1126 1127 public boolean hasKind() { 1128 return this.kind != null && !this.kind.isEmpty(); 1129 } 1130 1131 /** 1132 * @param value {@link #kind} (Indicates the purpose for the naming system - 1133 * what kinds of things does it make unique?). This is the 1134 * underlying object with id, value and extensions. The accessor 1135 * "getKind" gives direct access to the value 1136 */ 1137 public NamingSystem setKindElement(Enumeration<NamingSystemType> value) { 1138 this.kind = value; 1139 return this; 1140 } 1141 1142 /** 1143 * @return Indicates the purpose for the naming system - what kinds of things 1144 * does it make unique? 1145 */ 1146 public NamingSystemType getKind() { 1147 return this.kind == null ? null : this.kind.getValue(); 1148 } 1149 1150 /** 1151 * @param value Indicates the purpose for the naming system - what kinds of 1152 * things does it make unique? 1153 */ 1154 public NamingSystem setKind(NamingSystemType value) { 1155 if (this.kind == null) 1156 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); 1157 this.kind.setValue(value); 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #publisher} (The name of the individual or organization that 1163 * published the naming system.). This is the underlying object with id, 1164 * value and extensions. The accessor "getPublisher" gives direct access 1165 * to the value 1166 */ 1167 public StringType getPublisherElement() { 1168 if (this.publisher == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create NamingSystem.publisher"); 1171 else if (Configuration.doAutoCreate()) 1172 this.publisher = new StringType(); // bb 1173 return this.publisher; 1174 } 1175 1176 public boolean hasPublisherElement() { 1177 return this.publisher != null && !this.publisher.isEmpty(); 1178 } 1179 1180 public boolean hasPublisher() { 1181 return this.publisher != null && !this.publisher.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #publisher} (The name of the individual or organization 1186 * that published the naming system.). This is the underlying 1187 * object with id, value and extensions. The accessor 1188 * "getPublisher" gives direct access to the value 1189 */ 1190 public NamingSystem setPublisherElement(StringType value) { 1191 this.publisher = value; 1192 return this; 1193 } 1194 1195 /** 1196 * @return The name of the individual or organization that published the naming 1197 * system. 1198 */ 1199 public String getPublisher() { 1200 return this.publisher == null ? null : this.publisher.getValue(); 1201 } 1202 1203 /** 1204 * @param value The name of the individual or organization that published the 1205 * naming system. 1206 */ 1207 public NamingSystem setPublisher(String value) { 1208 if (Utilities.noString(value)) 1209 this.publisher = null; 1210 else { 1211 if (this.publisher == null) 1212 this.publisher = new StringType(); 1213 this.publisher.setValue(value); 1214 } 1215 return this; 1216 } 1217 1218 /** 1219 * @return {@link #contact} (Contacts to assist a user in finding and 1220 * communicating with the publisher.) 1221 */ 1222 public List<NamingSystemContactComponent> getContact() { 1223 if (this.contact == null) 1224 this.contact = new ArrayList<NamingSystemContactComponent>(); 1225 return this.contact; 1226 } 1227 1228 public boolean hasContact() { 1229 if (this.contact == null) 1230 return false; 1231 for (NamingSystemContactComponent item : this.contact) 1232 if (!item.isEmpty()) 1233 return true; 1234 return false; 1235 } 1236 1237 /** 1238 * @return {@link #contact} (Contacts to assist a user in finding and 1239 * communicating with the publisher.) 1240 */ 1241 // syntactic sugar 1242 public NamingSystemContactComponent addContact() { // 3 1243 NamingSystemContactComponent t = new NamingSystemContactComponent(); 1244 if (this.contact == null) 1245 this.contact = new ArrayList<NamingSystemContactComponent>(); 1246 this.contact.add(t); 1247 return t; 1248 } 1249 1250 // syntactic sugar 1251 public NamingSystem addContact(NamingSystemContactComponent t) { // 3 1252 if (t == null) 1253 return this; 1254 if (this.contact == null) 1255 this.contact = new ArrayList<NamingSystemContactComponent>(); 1256 this.contact.add(t); 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #responsible} (The name of the organization that is 1262 * responsible for issuing identifiers or codes for this namespace and 1263 * ensuring their non-collision.). This is the underlying object with 1264 * id, value and extensions. The accessor "getResponsible" gives direct 1265 * access to the value 1266 */ 1267 public StringType getResponsibleElement() { 1268 if (this.responsible == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create NamingSystem.responsible"); 1271 else if (Configuration.doAutoCreate()) 1272 this.responsible = new StringType(); // bb 1273 return this.responsible; 1274 } 1275 1276 public boolean hasResponsibleElement() { 1277 return this.responsible != null && !this.responsible.isEmpty(); 1278 } 1279 1280 public boolean hasResponsible() { 1281 return this.responsible != null && !this.responsible.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #responsible} (The name of the organization that is 1286 * responsible for issuing identifiers or codes for this namespace 1287 * and ensuring their non-collision.). This is the underlying 1288 * object with id, value and extensions. The accessor 1289 * "getResponsible" gives direct access to the value 1290 */ 1291 public NamingSystem setResponsibleElement(StringType value) { 1292 this.responsible = value; 1293 return this; 1294 } 1295 1296 /** 1297 * @return The name of the organization that is responsible for issuing 1298 * identifiers or codes for this namespace and ensuring their 1299 * non-collision. 1300 */ 1301 public String getResponsible() { 1302 return this.responsible == null ? null : this.responsible.getValue(); 1303 } 1304 1305 /** 1306 * @param value The name of the organization that is responsible for issuing 1307 * identifiers or codes for this namespace and ensuring their 1308 * non-collision. 1309 */ 1310 public NamingSystem setResponsible(String value) { 1311 if (Utilities.noString(value)) 1312 this.responsible = null; 1313 else { 1314 if (this.responsible == null) 1315 this.responsible = new StringType(); 1316 this.responsible.setValue(value); 1317 } 1318 return this; 1319 } 1320 1321 /** 1322 * @return {@link #date} (The date (and optionally time) when the system was 1323 * registered or published. The date must change when the business 1324 * version changes, if it does, and it must change if the status code 1325 * changes. In addition, it should change when the substantive content 1326 * of the registration changes.). This is the underlying object with id, 1327 * value and extensions. The accessor "getDate" gives direct access to 1328 * the value 1329 */ 1330 public DateTimeType getDateElement() { 1331 if (this.date == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create NamingSystem.date"); 1334 else if (Configuration.doAutoCreate()) 1335 this.date = new DateTimeType(); // bb 1336 return this.date; 1337 } 1338 1339 public boolean hasDateElement() { 1340 return this.date != null && !this.date.isEmpty(); 1341 } 1342 1343 public boolean hasDate() { 1344 return this.date != null && !this.date.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #date} (The date (and optionally time) when the system 1349 * was registered or published. The date must change when the 1350 * business version changes, if it does, and it must change if the 1351 * status code changes. In addition, it should change when the 1352 * substantive content of the registration changes.). This is the 1353 * underlying object with id, value and extensions. The accessor 1354 * "getDate" gives direct access to the value 1355 */ 1356 public NamingSystem setDateElement(DateTimeType value) { 1357 this.date = value; 1358 return this; 1359 } 1360 1361 /** 1362 * @return The date (and optionally time) when the system was registered or 1363 * published. The date must change when the business version changes, if 1364 * it does, and it must change if the status code changes. In addition, 1365 * it should change when the substantive content of the registration 1366 * changes. 1367 */ 1368 public Date getDate() { 1369 return this.date == null ? null : this.date.getValue(); 1370 } 1371 1372 /** 1373 * @param value The date (and optionally time) when the system was registered or 1374 * published. The date must change when the business version 1375 * changes, if it does, and it must change if the status code 1376 * changes. In addition, it should change when the substantive 1377 * content of the registration changes. 1378 */ 1379 public NamingSystem setDate(Date value) { 1380 if (this.date == null) 1381 this.date = new DateTimeType(); 1382 this.date.setValue(value); 1383 return this; 1384 } 1385 1386 /** 1387 * @return {@link #type} (Categorizes a naming system for easier search by 1388 * grouping related naming systems.) 1389 */ 1390 public CodeableConcept getType() { 1391 if (this.type == null) 1392 if (Configuration.errorOnAutoCreate()) 1393 throw new Error("Attempt to auto-create NamingSystem.type"); 1394 else if (Configuration.doAutoCreate()) 1395 this.type = new CodeableConcept(); // cc 1396 return this.type; 1397 } 1398 1399 public boolean hasType() { 1400 return this.type != null && !this.type.isEmpty(); 1401 } 1402 1403 /** 1404 * @param value {@link #type} (Categorizes a naming system for easier search by 1405 * grouping related naming systems.) 1406 */ 1407 public NamingSystem setType(CodeableConcept value) { 1408 this.type = value; 1409 return this; 1410 } 1411 1412 /** 1413 * @return {@link #description} (Details about what the namespace identifies 1414 * including scope, granularity, version labeling, etc.). This is the 1415 * underlying object with id, value and extensions. The accessor 1416 * "getDescription" gives direct access to the value 1417 */ 1418 public StringType getDescriptionElement() { 1419 if (this.description == null) 1420 if (Configuration.errorOnAutoCreate()) 1421 throw new Error("Attempt to auto-create NamingSystem.description"); 1422 else if (Configuration.doAutoCreate()) 1423 this.description = new StringType(); // bb 1424 return this.description; 1425 } 1426 1427 public boolean hasDescriptionElement() { 1428 return this.description != null && !this.description.isEmpty(); 1429 } 1430 1431 public boolean hasDescription() { 1432 return this.description != null && !this.description.isEmpty(); 1433 } 1434 1435 /** 1436 * @param value {@link #description} (Details about what the namespace 1437 * identifies including scope, granularity, version labeling, 1438 * etc.). This is the underlying object with id, value and 1439 * extensions. The accessor "getDescription" gives direct access to 1440 * the value 1441 */ 1442 public NamingSystem setDescriptionElement(StringType value) { 1443 this.description = value; 1444 return this; 1445 } 1446 1447 /** 1448 * @return Details about what the namespace identifies including scope, 1449 * granularity, version labeling, etc. 1450 */ 1451 public String getDescription() { 1452 return this.description == null ? null : this.description.getValue(); 1453 } 1454 1455 /** 1456 * @param value Details about what the namespace identifies including scope, 1457 * granularity, version labeling, etc. 1458 */ 1459 public NamingSystem setDescription(String value) { 1460 if (Utilities.noString(value)) 1461 this.description = null; 1462 else { 1463 if (this.description == null) 1464 this.description = new StringType(); 1465 this.description.setValue(value); 1466 } 1467 return this; 1468 } 1469 1470 /** 1471 * @return {@link #useContext} (The content was developed with a focus and 1472 * intent of supporting the contexts that are listed. These terms may be 1473 * used to assist with indexing and searching of naming systems.) 1474 */ 1475 public List<CodeableConcept> getUseContext() { 1476 if (this.useContext == null) 1477 this.useContext = new ArrayList<CodeableConcept>(); 1478 return this.useContext; 1479 } 1480 1481 public boolean hasUseContext() { 1482 if (this.useContext == null) 1483 return false; 1484 for (CodeableConcept item : this.useContext) 1485 if (!item.isEmpty()) 1486 return true; 1487 return false; 1488 } 1489 1490 /** 1491 * @return {@link #useContext} (The content was developed with a focus and 1492 * intent of supporting the contexts that are listed. These terms may be 1493 * used to assist with indexing and searching of naming systems.) 1494 */ 1495 // syntactic sugar 1496 public CodeableConcept addUseContext() { // 3 1497 CodeableConcept t = new CodeableConcept(); 1498 if (this.useContext == null) 1499 this.useContext = new ArrayList<CodeableConcept>(); 1500 this.useContext.add(t); 1501 return t; 1502 } 1503 1504 // syntactic sugar 1505 public NamingSystem addUseContext(CodeableConcept t) { // 3 1506 if (t == null) 1507 return this; 1508 if (this.useContext == null) 1509 this.useContext = new ArrayList<CodeableConcept>(); 1510 this.useContext.add(t); 1511 return this; 1512 } 1513 1514 /** 1515 * @return {@link #usage} (Provides guidance on the use of the namespace, 1516 * including the handling of formatting characters, use of upper vs. 1517 * lower case, etc.). This is the underlying object with id, value and 1518 * extensions. The accessor "getUsage" gives direct access to the value 1519 */ 1520 public StringType getUsageElement() { 1521 if (this.usage == null) 1522 if (Configuration.errorOnAutoCreate()) 1523 throw new Error("Attempt to auto-create NamingSystem.usage"); 1524 else if (Configuration.doAutoCreate()) 1525 this.usage = new StringType(); // bb 1526 return this.usage; 1527 } 1528 1529 public boolean hasUsageElement() { 1530 return this.usage != null && !this.usage.isEmpty(); 1531 } 1532 1533 public boolean hasUsage() { 1534 return this.usage != null && !this.usage.isEmpty(); 1535 } 1536 1537 /** 1538 * @param value {@link #usage} (Provides guidance on the use of the namespace, 1539 * including the handling of formatting characters, use of upper 1540 * vs. lower case, etc.). This is the underlying object with id, 1541 * value and extensions. The accessor "getUsage" gives direct 1542 * access to the value 1543 */ 1544 public NamingSystem setUsageElement(StringType value) { 1545 this.usage = value; 1546 return this; 1547 } 1548 1549 /** 1550 * @return Provides guidance on the use of the namespace, including the handling 1551 * of formatting characters, use of upper vs. lower case, etc. 1552 */ 1553 public String getUsage() { 1554 return this.usage == null ? null : this.usage.getValue(); 1555 } 1556 1557 /** 1558 * @param value Provides guidance on the use of the namespace, including the 1559 * handling of formatting characters, use of upper vs. lower case, 1560 * etc. 1561 */ 1562 public NamingSystem setUsage(String value) { 1563 if (Utilities.noString(value)) 1564 this.usage = null; 1565 else { 1566 if (this.usage == null) 1567 this.usage = new StringType(); 1568 this.usage.setValue(value); 1569 } 1570 return this; 1571 } 1572 1573 /** 1574 * @return {@link #uniqueId} (Indicates how the system may be identified when 1575 * referenced in electronic exchange.) 1576 */ 1577 public List<NamingSystemUniqueIdComponent> getUniqueId() { 1578 if (this.uniqueId == null) 1579 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1580 return this.uniqueId; 1581 } 1582 1583 public boolean hasUniqueId() { 1584 if (this.uniqueId == null) 1585 return false; 1586 for (NamingSystemUniqueIdComponent item : this.uniqueId) 1587 if (!item.isEmpty()) 1588 return true; 1589 return false; 1590 } 1591 1592 /** 1593 * @return {@link #uniqueId} (Indicates how the system may be identified when 1594 * referenced in electronic exchange.) 1595 */ 1596 // syntactic sugar 1597 public NamingSystemUniqueIdComponent addUniqueId() { // 3 1598 NamingSystemUniqueIdComponent t = new NamingSystemUniqueIdComponent(); 1599 if (this.uniqueId == null) 1600 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1601 this.uniqueId.add(t); 1602 return t; 1603 } 1604 1605 // syntactic sugar 1606 public NamingSystem addUniqueId(NamingSystemUniqueIdComponent t) { // 3 1607 if (t == null) 1608 return this; 1609 if (this.uniqueId == null) 1610 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1611 this.uniqueId.add(t); 1612 return this; 1613 } 1614 1615 /** 1616 * @return {@link #replacedBy} (For naming systems that are retired, indicates 1617 * the naming system that should be used in their place (if any).) 1618 */ 1619 public Reference getReplacedBy() { 1620 if (this.replacedBy == null) 1621 if (Configuration.errorOnAutoCreate()) 1622 throw new Error("Attempt to auto-create NamingSystem.replacedBy"); 1623 else if (Configuration.doAutoCreate()) 1624 this.replacedBy = new Reference(); // cc 1625 return this.replacedBy; 1626 } 1627 1628 public boolean hasReplacedBy() { 1629 return this.replacedBy != null && !this.replacedBy.isEmpty(); 1630 } 1631 1632 /** 1633 * @param value {@link #replacedBy} (For naming systems that are retired, 1634 * indicates the naming system that should be used in their place 1635 * (if any).) 1636 */ 1637 public NamingSystem setReplacedBy(Reference value) { 1638 this.replacedBy = value; 1639 return this; 1640 } 1641 1642 /** 1643 * @return {@link #replacedBy} The actual object that is the target of the 1644 * reference. The reference library doesn't populate this, but you can 1645 * use it to hold the resource if you resolve it. (For naming systems 1646 * that are retired, indicates the naming system that should be used in 1647 * their place (if any).) 1648 */ 1649 public NamingSystem getReplacedByTarget() { 1650 if (this.replacedByTarget == null) 1651 if (Configuration.errorOnAutoCreate()) 1652 throw new Error("Attempt to auto-create NamingSystem.replacedBy"); 1653 else if (Configuration.doAutoCreate()) 1654 this.replacedByTarget = new NamingSystem(); // aa 1655 return this.replacedByTarget; 1656 } 1657 1658 /** 1659 * @param value {@link #replacedBy} The actual object that is the target of the 1660 * reference. The reference library doesn't use these, but you can 1661 * use it to hold the resource if you resolve it. (For naming 1662 * systems that are retired, indicates the naming system that 1663 * should be used in their place (if any).) 1664 */ 1665 public NamingSystem setReplacedByTarget(NamingSystem value) { 1666 this.replacedByTarget = value; 1667 return this; 1668 } 1669 1670 protected void listChildren(List<Property> childrenList) { 1671 super.listChildren(childrenList); 1672 childrenList 1673 .add(new Property("name", "string", "The descriptive name of this particular identifier type or code system.", 1674 0, java.lang.Integer.MAX_VALUE, name)); 1675 childrenList.add(new Property("status", "code", "Indicates whether the naming system is \"ready for use\" or not.", 1676 0, java.lang.Integer.MAX_VALUE, status)); 1677 childrenList.add(new Property("kind", "code", 1678 "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1679 java.lang.Integer.MAX_VALUE, kind)); 1680 childrenList.add(new Property("publisher", "string", 1681 "The name of the individual or organization that published the naming system.", 0, java.lang.Integer.MAX_VALUE, 1682 publisher)); 1683 childrenList 1684 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 1685 0, java.lang.Integer.MAX_VALUE, contact)); 1686 childrenList.add(new Property("responsible", "string", 1687 "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 1688 0, java.lang.Integer.MAX_VALUE, responsible)); 1689 childrenList.add(new Property("date", "dateTime", 1690 "The date (and optionally time) when the system was registered or published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the registration changes.", 1691 0, java.lang.Integer.MAX_VALUE, date)); 1692 childrenList.add(new Property("type", "CodeableConcept", 1693 "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1694 java.lang.Integer.MAX_VALUE, type)); 1695 childrenList.add(new Property("description", "string", 1696 "Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1697 java.lang.Integer.MAX_VALUE, description)); 1698 childrenList.add(new Property("useContext", "CodeableConcept", 1699 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of naming systems.", 1700 0, java.lang.Integer.MAX_VALUE, useContext)); 1701 childrenList.add(new Property("usage", "string", 1702 "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 1703 0, java.lang.Integer.MAX_VALUE, usage)); 1704 childrenList.add(new Property("uniqueId", "", 1705 "Indicates how the system may be identified when referenced in electronic exchange.", 0, 1706 java.lang.Integer.MAX_VALUE, uniqueId)); 1707 childrenList.add(new Property("replacedBy", "Reference(NamingSystem)", 1708 "For naming systems that are retired, indicates the naming system that should be used in their place (if any).", 1709 0, java.lang.Integer.MAX_VALUE, replacedBy)); 1710 } 1711 1712 @Override 1713 public void setProperty(String name, Base value) throws FHIRException { 1714 if (name.equals("name")) 1715 this.name = castToString(value); // StringType 1716 else if (name.equals("status")) 1717 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 1718 else if (name.equals("kind")) 1719 this.kind = new NamingSystemTypeEnumFactory().fromType(value); // Enumeration<NamingSystemType> 1720 else if (name.equals("publisher")) 1721 this.publisher = castToString(value); // StringType 1722 else if (name.equals("contact")) 1723 this.getContact().add((NamingSystemContactComponent) value); 1724 else if (name.equals("responsible")) 1725 this.responsible = castToString(value); // StringType 1726 else if (name.equals("date")) 1727 this.date = castToDateTime(value); // DateTimeType 1728 else if (name.equals("type")) 1729 this.type = castToCodeableConcept(value); // CodeableConcept 1730 else if (name.equals("description")) 1731 this.description = castToString(value); // StringType 1732 else if (name.equals("useContext")) 1733 this.getUseContext().add(castToCodeableConcept(value)); 1734 else if (name.equals("usage")) 1735 this.usage = castToString(value); // StringType 1736 else if (name.equals("uniqueId")) 1737 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); 1738 else if (name.equals("replacedBy")) 1739 this.replacedBy = castToReference(value); // Reference 1740 else 1741 super.setProperty(name, value); 1742 } 1743 1744 @Override 1745 public Base addChild(String name) throws FHIRException { 1746 if (name.equals("name")) { 1747 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 1748 } else if (name.equals("status")) { 1749 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.status"); 1750 } else if (name.equals("kind")) { 1751 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.kind"); 1752 } else if (name.equals("publisher")) { 1753 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.publisher"); 1754 } else if (name.equals("contact")) { 1755 return addContact(); 1756 } else if (name.equals("responsible")) { 1757 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.responsible"); 1758 } else if (name.equals("date")) { 1759 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.date"); 1760 } else if (name.equals("type")) { 1761 this.type = new CodeableConcept(); 1762 return this.type; 1763 } else if (name.equals("description")) { 1764 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.description"); 1765 } else if (name.equals("useContext")) { 1766 return addUseContext(); 1767 } else if (name.equals("usage")) { 1768 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.usage"); 1769 } else if (name.equals("uniqueId")) { 1770 return addUniqueId(); 1771 } else if (name.equals("replacedBy")) { 1772 this.replacedBy = new Reference(); 1773 return this.replacedBy; 1774 } else 1775 return super.addChild(name); 1776 } 1777 1778 public String fhirType() { 1779 return "NamingSystem"; 1780 1781 } 1782 1783 public NamingSystem copy() { 1784 NamingSystem dst = new NamingSystem(); 1785 copyValues(dst); 1786 dst.name = name == null ? null : name.copy(); 1787 dst.status = status == null ? null : status.copy(); 1788 dst.kind = kind == null ? null : kind.copy(); 1789 dst.publisher = publisher == null ? null : publisher.copy(); 1790 if (contact != null) { 1791 dst.contact = new ArrayList<NamingSystemContactComponent>(); 1792 for (NamingSystemContactComponent i : contact) 1793 dst.contact.add(i.copy()); 1794 } 1795 ; 1796 dst.responsible = responsible == null ? null : responsible.copy(); 1797 dst.date = date == null ? null : date.copy(); 1798 dst.type = type == null ? null : type.copy(); 1799 dst.description = description == null ? null : description.copy(); 1800 if (useContext != null) { 1801 dst.useContext = new ArrayList<CodeableConcept>(); 1802 for (CodeableConcept i : useContext) 1803 dst.useContext.add(i.copy()); 1804 } 1805 ; 1806 dst.usage = usage == null ? null : usage.copy(); 1807 if (uniqueId != null) { 1808 dst.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1809 for (NamingSystemUniqueIdComponent i : uniqueId) 1810 dst.uniqueId.add(i.copy()); 1811 } 1812 ; 1813 dst.replacedBy = replacedBy == null ? null : replacedBy.copy(); 1814 return dst; 1815 } 1816 1817 protected NamingSystem typedCopy() { 1818 return copy(); 1819 } 1820 1821 @Override 1822 public boolean equalsDeep(Base other) { 1823 if (!super.equalsDeep(other)) 1824 return false; 1825 if (!(other instanceof NamingSystem)) 1826 return false; 1827 NamingSystem o = (NamingSystem) other; 1828 return compareDeep(name, o.name, true) && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) 1829 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 1830 && compareDeep(responsible, o.responsible, true) && compareDeep(date, o.date, true) 1831 && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 1832 && compareDeep(useContext, o.useContext, true) && compareDeep(usage, o.usage, true) 1833 && compareDeep(uniqueId, o.uniqueId, true) && compareDeep(replacedBy, o.replacedBy, true); 1834 } 1835 1836 @Override 1837 public boolean equalsShallow(Base other) { 1838 if (!super.equalsShallow(other)) 1839 return false; 1840 if (!(other instanceof NamingSystem)) 1841 return false; 1842 NamingSystem o = (NamingSystem) other; 1843 return compareValues(name, o.name, true) && compareValues(status, o.status, true) 1844 && compareValues(kind, o.kind, true) && compareValues(publisher, o.publisher, true) 1845 && compareValues(responsible, o.responsible, true) && compareValues(date, o.date, true) 1846 && compareValues(description, o.description, true) && compareValues(usage, o.usage, true); 1847 } 1848 1849 public boolean isEmpty() { 1850 return super.isEmpty() && (name == null || name.isEmpty()) && (status == null || status.isEmpty()) 1851 && (kind == null || kind.isEmpty()) && (publisher == null || publisher.isEmpty()) 1852 && (contact == null || contact.isEmpty()) && (responsible == null || responsible.isEmpty()) 1853 && (date == null || date.isEmpty()) && (type == null || type.isEmpty()) 1854 && (description == null || description.isEmpty()) && (useContext == null || useContext.isEmpty()) 1855 && (usage == null || usage.isEmpty()) && (uniqueId == null || uniqueId.isEmpty()) 1856 && (replacedBy == null || replacedBy.isEmpty()); 1857 } 1858 1859 @Override 1860 public ResourceType getResourceType() { 1861 return ResourceType.NamingSystem; 1862 } 1863 1864 @SearchParamDefinition(name = "date", path = "NamingSystem.date", description = "Publication Date(/time)", type = "date") 1865 public static final String SP_DATE = "date"; 1866 @SearchParamDefinition(name = "period", path = "NamingSystem.uniqueId.period", description = "When is identifier valid?", type = "date") 1867 public static final String SP_PERIOD = "period"; 1868 @SearchParamDefinition(name = "kind", path = "NamingSystem.kind", description = "codesystem | identifier | root", type = "token") 1869 public static final String SP_KIND = "kind"; 1870 @SearchParamDefinition(name = "type", path = "NamingSystem.type", description = "e.g. driver, provider, patient, bank etc.", type = "token") 1871 public static final String SP_TYPE = "type"; 1872 @SearchParamDefinition(name = "id-type", path = "NamingSystem.uniqueId.type", description = "oid | uuid | uri | other", type = "token") 1873 public static final String SP_IDTYPE = "id-type"; 1874 @SearchParamDefinition(name = "responsible", path = "NamingSystem.responsible", description = "Who maintains system namespace?", type = "string") 1875 public static final String SP_RESPONSIBLE = "responsible"; 1876 @SearchParamDefinition(name = "contact", path = "NamingSystem.contact.name", description = "Name of a individual to contact", type = "string") 1877 public static final String SP_CONTACT = "contact"; 1878 @SearchParamDefinition(name = "name", path = "NamingSystem.name", description = "Human-readable label", type = "string") 1879 public static final String SP_NAME = "name"; 1880 @SearchParamDefinition(name = "context", path = "NamingSystem.useContext", description = "Content intends to support these contexts", type = "token") 1881 public static final String SP_CONTEXT = "context"; 1882 @SearchParamDefinition(name = "publisher", path = "NamingSystem.publisher", description = "Name of the publisher (Organization or individual)", type = "string") 1883 public static final String SP_PUBLISHER = "publisher"; 1884 @SearchParamDefinition(name = "telecom", path = "NamingSystem.contact.telecom", description = "Contact details for individual or publisher", type = "token") 1885 public static final String SP_TELECOM = "telecom"; 1886 @SearchParamDefinition(name = "value", path = "NamingSystem.uniqueId.value", description = "The unique identifier", type = "string") 1887 public static final String SP_VALUE = "value"; 1888 @SearchParamDefinition(name = "replaced-by", path = "NamingSystem.replacedBy", description = "Use this instead", type = "reference") 1889 public static final String SP_REPLACEDBY = "replaced-by"; 1890 @SearchParamDefinition(name = "status", path = "NamingSystem.status", description = "draft | active | retired", type = "token") 1891 public static final String SP_STATUS = "status"; 1892 1893}