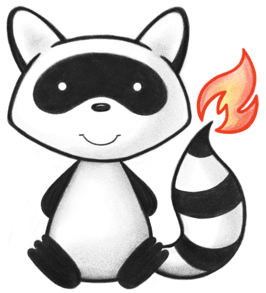
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A curated namespace that issues unique symbols within that namespace for the 050 * identification of concepts, people, devices, etc. Represents a "System" used 051 * within the Identifier and Coding data types. 052 */ 053@ResourceDef(name = "NamingSystem", profile = "http://hl7.org/fhir/Profile/NamingSystem") 054public class NamingSystem extends DomainResource { 055 056 public enum NamingSystemType { 057 /** 058 * The naming system is used to define concepts and symbols to represent those 059 * concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc. 060 */ 061 CODESYSTEM, 062 /** 063 * The naming system is used to manage identifiers (e.g. license numbers, order 064 * numbers, etc.). 065 */ 066 IDENTIFIER, 067 /** 068 * The naming system is used as the root for other identifiers and naming 069 * systems. 070 */ 071 ROOT, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static NamingSystemType fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("codesystem".equals(codeString)) 081 return CODESYSTEM; 082 if ("identifier".equals(codeString)) 083 return IDENTIFIER; 084 if ("root".equals(codeString)) 085 return ROOT; 086 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case CODESYSTEM: 092 return "codesystem"; 093 case IDENTIFIER: 094 return "identifier"; 095 case ROOT: 096 return "root"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case CODESYSTEM: 107 return "http://hl7.org/fhir/namingsystem-type"; 108 case IDENTIFIER: 109 return "http://hl7.org/fhir/namingsystem-type"; 110 case ROOT: 111 return "http://hl7.org/fhir/namingsystem-type"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case CODESYSTEM: 122 return "The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc."; 123 case IDENTIFIER: 124 return "The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.)."; 125 case ROOT: 126 return "The naming system is used as the root for other identifiers and naming systems."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case CODESYSTEM: 137 return "Code System"; 138 case IDENTIFIER: 139 return "Identifier"; 140 case ROOT: 141 return "Root"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class NamingSystemTypeEnumFactory implements EnumFactory<NamingSystemType> { 151 public NamingSystemType fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("codesystem".equals(codeString)) 156 return NamingSystemType.CODESYSTEM; 157 if ("identifier".equals(codeString)) 158 return NamingSystemType.IDENTIFIER; 159 if ("root".equals(codeString)) 160 return NamingSystemType.ROOT; 161 throw new IllegalArgumentException("Unknown NamingSystemType code '" + codeString + "'"); 162 } 163 164 public Enumeration<NamingSystemType> fromType(Base code) throws FHIRException { 165 if (code == null || code.isEmpty()) 166 return null; 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("codesystem".equals(codeString)) 171 return new Enumeration<NamingSystemType>(this, NamingSystemType.CODESYSTEM); 172 if ("identifier".equals(codeString)) 173 return new Enumeration<NamingSystemType>(this, NamingSystemType.IDENTIFIER); 174 if ("root".equals(codeString)) 175 return new Enumeration<NamingSystemType>(this, NamingSystemType.ROOT); 176 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 177 } 178 179 public String toCode(NamingSystemType code) 180 { 181 if (code == NamingSystemType.NULL) 182 return null; 183 if (code == NamingSystemType.CODESYSTEM) 184 return "codesystem"; 185 if (code == NamingSystemType.IDENTIFIER) 186 return "identifier"; 187 if (code == NamingSystemType.ROOT) 188 return "root"; 189 return "?"; 190 } 191 } 192 193 public enum NamingSystemIdentifierType { 194 /** 195 * An ISO object identifier; e.g. 1.2.3.4.5. 196 */ 197 OID, 198 /** 199 * A universally unique identifier of the form 200 * a5afddf4-e880-459b-876e-e4591b0acc11. 201 */ 202 UUID, 203 /** 204 * A uniform resource identifier (ideally a URL - uniform resource locator); 205 * e.g. http://unitsofmeasure.org. 206 */ 207 URI, 208 /** 209 * Some other type of unique identifier; e.g. HL7-assigned reserved string such 210 * as LN for LOINC. 211 */ 212 OTHER, 213 /** 214 * added to help the parsers 215 */ 216 NULL; 217 218 public static NamingSystemIdentifierType fromCode(String codeString) throws FHIRException { 219 if (codeString == null || "".equals(codeString)) 220 return null; 221 if ("oid".equals(codeString)) 222 return OID; 223 if ("uuid".equals(codeString)) 224 return UUID; 225 if ("uri".equals(codeString)) 226 return URI; 227 if ("other".equals(codeString)) 228 return OTHER; 229 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 230 } 231 232 public String toCode() { 233 switch (this) { 234 case OID: 235 return "oid"; 236 case UUID: 237 return "uuid"; 238 case URI: 239 return "uri"; 240 case OTHER: 241 return "other"; 242 case NULL: 243 return null; 244 default: 245 return "?"; 246 } 247 } 248 249 public String getSystem() { 250 switch (this) { 251 case OID: 252 return "http://hl7.org/fhir/namingsystem-identifier-type"; 253 case UUID: 254 return "http://hl7.org/fhir/namingsystem-identifier-type"; 255 case URI: 256 return "http://hl7.org/fhir/namingsystem-identifier-type"; 257 case OTHER: 258 return "http://hl7.org/fhir/namingsystem-identifier-type"; 259 case NULL: 260 return null; 261 default: 262 return "?"; 263 } 264 } 265 266 public String getDefinition() { 267 switch (this) { 268 case OID: 269 return "An ISO object identifier; e.g. 1.2.3.4.5."; 270 case UUID: 271 return "A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11."; 272 case URI: 273 return "A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org."; 274 case OTHER: 275 return "Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC."; 276 case NULL: 277 return null; 278 default: 279 return "?"; 280 } 281 } 282 283 public String getDisplay() { 284 switch (this) { 285 case OID: 286 return "OID"; 287 case UUID: 288 return "UUID"; 289 case URI: 290 return "URI"; 291 case OTHER: 292 return "Other"; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 } 300 301 public static class NamingSystemIdentifierTypeEnumFactory implements EnumFactory<NamingSystemIdentifierType> { 302 public NamingSystemIdentifierType fromCode(String codeString) throws IllegalArgumentException { 303 if (codeString == null || "".equals(codeString)) 304 if (codeString == null || "".equals(codeString)) 305 return null; 306 if ("oid".equals(codeString)) 307 return NamingSystemIdentifierType.OID; 308 if ("uuid".equals(codeString)) 309 return NamingSystemIdentifierType.UUID; 310 if ("uri".equals(codeString)) 311 return NamingSystemIdentifierType.URI; 312 if ("other".equals(codeString)) 313 return NamingSystemIdentifierType.OTHER; 314 throw new IllegalArgumentException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 315 } 316 317 public Enumeration<NamingSystemIdentifierType> fromType(Base code) throws FHIRException { 318 if (code == null || code.isEmpty()) 319 return null; 320 String codeString = ((PrimitiveType) code).asStringValue(); 321 if (codeString == null || "".equals(codeString)) 322 return null; 323 if ("oid".equals(codeString)) 324 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OID); 325 if ("uuid".equals(codeString)) 326 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.UUID); 327 if ("uri".equals(codeString)) 328 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.URI); 329 if ("other".equals(codeString)) 330 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OTHER); 331 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 332 } 333 334 public String toCode(NamingSystemIdentifierType code) 335 { 336 if (code == NamingSystemIdentifierType.NULL) 337 return null; 338 if (code == NamingSystemIdentifierType.OID) 339 return "oid"; 340 if (code == NamingSystemIdentifierType.UUID) 341 return "uuid"; 342 if (code == NamingSystemIdentifierType.URI) 343 return "uri"; 344 if (code == NamingSystemIdentifierType.OTHER) 345 return "other"; 346 return "?"; 347 } 348 } 349 350 @Block() 351 public static class NamingSystemContactComponent extends BackboneElement implements IBaseBackboneElement { 352 /** 353 * The name of an individual to contact regarding the naming system. 354 */ 355 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 356 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the naming system.") 357 protected StringType name; 358 359 /** 360 * Contact details for individual (if a name was provided) or the publisher. 361 */ 362 @Child(name = "telecom", type = { 363 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 364 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 365 protected List<ContactPoint> telecom; 366 367 private static final long serialVersionUID = -1179697803L; 368 369 /* 370 * Constructor 371 */ 372 public NamingSystemContactComponent() { 373 super(); 374 } 375 376 /** 377 * @return {@link #name} (The name of an individual to contact regarding the 378 * naming system.). This is the underlying object with id, value and 379 * extensions. The accessor "getName" gives direct access to the value 380 */ 381 public StringType getNameElement() { 382 if (this.name == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create NamingSystemContactComponent.name"); 385 else if (Configuration.doAutoCreate()) 386 this.name = new StringType(); // bb 387 return this.name; 388 } 389 390 public boolean hasNameElement() { 391 return this.name != null && !this.name.isEmpty(); 392 } 393 394 public boolean hasName() { 395 return this.name != null && !this.name.isEmpty(); 396 } 397 398 /** 399 * @param value {@link #name} (The name of an individual to contact regarding 400 * the naming system.). This is the underlying object with id, 401 * value and extensions. The accessor "getName" gives direct access 402 * to the value 403 */ 404 public NamingSystemContactComponent setNameElement(StringType value) { 405 this.name = value; 406 return this; 407 } 408 409 /** 410 * @return The name of an individual to contact regarding the naming system. 411 */ 412 public String getName() { 413 return this.name == null ? null : this.name.getValue(); 414 } 415 416 /** 417 * @param value The name of an individual to contact regarding the naming 418 * system. 419 */ 420 public NamingSystemContactComponent setName(String value) { 421 if (Utilities.noString(value)) 422 this.name = null; 423 else { 424 if (this.name == null) 425 this.name = new StringType(); 426 this.name.setValue(value); 427 } 428 return this; 429 } 430 431 /** 432 * @return {@link #telecom} (Contact details for individual (if a name was 433 * provided) or the publisher.) 434 */ 435 public List<ContactPoint> getTelecom() { 436 if (this.telecom == null) 437 this.telecom = new ArrayList<ContactPoint>(); 438 return this.telecom; 439 } 440 441 public boolean hasTelecom() { 442 if (this.telecom == null) 443 return false; 444 for (ContactPoint item : this.telecom) 445 if (!item.isEmpty()) 446 return true; 447 return false; 448 } 449 450 /** 451 * @return {@link #telecom} (Contact details for individual (if a name was 452 * provided) or the publisher.) 453 */ 454 // syntactic sugar 455 public ContactPoint addTelecom() { // 3 456 ContactPoint t = new ContactPoint(); 457 if (this.telecom == null) 458 this.telecom = new ArrayList<ContactPoint>(); 459 this.telecom.add(t); 460 return t; 461 } 462 463 // syntactic sugar 464 public NamingSystemContactComponent addTelecom(ContactPoint t) { // 3 465 if (t == null) 466 return this; 467 if (this.telecom == null) 468 this.telecom = new ArrayList<ContactPoint>(); 469 this.telecom.add(t); 470 return this; 471 } 472 473 protected void listChildren(List<Property> childrenList) { 474 super.listChildren(childrenList); 475 childrenList.add(new Property("name", "string", 476 "The name of an individual to contact regarding the naming system.", 0, java.lang.Integer.MAX_VALUE, name)); 477 childrenList.add(new Property("telecom", "ContactPoint", 478 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 479 telecom)); 480 } 481 482 @Override 483 public void setProperty(String name, Base value) throws FHIRException { 484 if (name.equals("name")) 485 this.name = castToString(value); // StringType 486 else if (name.equals("telecom")) 487 this.getTelecom().add(castToContactPoint(value)); 488 else 489 super.setProperty(name, value); 490 } 491 492 @Override 493 public Base addChild(String name) throws FHIRException { 494 if (name.equals("name")) { 495 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 496 } else if (name.equals("telecom")) { 497 return addTelecom(); 498 } else 499 return super.addChild(name); 500 } 501 502 public NamingSystemContactComponent copy() { 503 NamingSystemContactComponent dst = new NamingSystemContactComponent(); 504 copyValues(dst); 505 dst.name = name == null ? null : name.copy(); 506 if (telecom != null) { 507 dst.telecom = new ArrayList<ContactPoint>(); 508 for (ContactPoint i : telecom) 509 dst.telecom.add(i.copy()); 510 } 511 ; 512 return dst; 513 } 514 515 @Override 516 public boolean equalsDeep(Base other) { 517 if (!super.equalsDeep(other)) 518 return false; 519 if (!(other instanceof NamingSystemContactComponent)) 520 return false; 521 NamingSystemContactComponent o = (NamingSystemContactComponent) other; 522 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 523 } 524 525 @Override 526 public boolean equalsShallow(Base other) { 527 if (!super.equalsShallow(other)) 528 return false; 529 if (!(other instanceof NamingSystemContactComponent)) 530 return false; 531 NamingSystemContactComponent o = (NamingSystemContactComponent) other; 532 return compareValues(name, o.name, true); 533 } 534 535 public boolean isEmpty() { 536 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 537 } 538 539 public String fhirType() { 540 return "NamingSystem.contact"; 541 542 } 543 544 } 545 546 @Block() 547 public static class NamingSystemUniqueIdComponent extends BackboneElement implements IBaseBackboneElement { 548 /** 549 * Identifies the unique identifier scheme used for this particular identifier. 550 */ 551 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 552 @Description(shortDefinition = "oid | uuid | uri | other", formalDefinition = "Identifies the unique identifier scheme used for this particular identifier.") 553 protected Enumeration<NamingSystemIdentifierType> type; 554 555 /** 556 * The string that should be sent over the wire to identify the code system or 557 * identifier system. 558 */ 559 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 560 @Description(shortDefinition = "The unique identifier", formalDefinition = "The string that should be sent over the wire to identify the code system or identifier system.") 561 protected StringType value; 562 563 /** 564 * Indicates whether this identifier is the "preferred" identifier of this type. 565 */ 566 @Child(name = "preferred", type = { 567 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 568 @Description(shortDefinition = "Is this the id that should be used for this type", formalDefinition = "Indicates whether this identifier is the \"preferred\" identifier of this type.") 569 protected BooleanType preferred; 570 571 /** 572 * Identifies the period of time over which this identifier is considered 573 * appropriate to refer to the naming system. Outside of this window, the 574 * identifier might be non-deterministic. 575 */ 576 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 577 @Description(shortDefinition = "When is identifier valid?", formalDefinition = "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.") 578 protected Period period; 579 580 private static final long serialVersionUID = -193711840L; 581 582 /* 583 * Constructor 584 */ 585 public NamingSystemUniqueIdComponent() { 586 super(); 587 } 588 589 /* 590 * Constructor 591 */ 592 public NamingSystemUniqueIdComponent(Enumeration<NamingSystemIdentifierType> type, StringType value) { 593 super(); 594 this.type = type; 595 this.value = value; 596 } 597 598 /** 599 * @return {@link #type} (Identifies the unique identifier scheme used for this 600 * particular identifier.). This is the underlying object with id, value 601 * and extensions. The accessor "getType" gives direct access to the 602 * value 603 */ 604 public Enumeration<NamingSystemIdentifierType> getTypeElement() { 605 if (this.type == null) 606 if (Configuration.errorOnAutoCreate()) 607 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.type"); 608 else if (Configuration.doAutoCreate()) 609 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); // bb 610 return this.type; 611 } 612 613 public boolean hasTypeElement() { 614 return this.type != null && !this.type.isEmpty(); 615 } 616 617 public boolean hasType() { 618 return this.type != null && !this.type.isEmpty(); 619 } 620 621 /** 622 * @param value {@link #type} (Identifies the unique identifier scheme used for 623 * this particular identifier.). This is the underlying object with 624 * id, value and extensions. The accessor "getType" gives direct 625 * access to the value 626 */ 627 public NamingSystemUniqueIdComponent setTypeElement(Enumeration<NamingSystemIdentifierType> value) { 628 this.type = value; 629 return this; 630 } 631 632 /** 633 * @return Identifies the unique identifier scheme used for this particular 634 * identifier. 635 */ 636 public NamingSystemIdentifierType getType() { 637 return this.type == null ? null : this.type.getValue(); 638 } 639 640 /** 641 * @param value Identifies the unique identifier scheme used for this particular 642 * identifier. 643 */ 644 public NamingSystemUniqueIdComponent setType(NamingSystemIdentifierType value) { 645 if (this.type == null) 646 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); 647 this.type.setValue(value); 648 return this; 649 } 650 651 /** 652 * @return {@link #value} (The string that should be sent over the wire to 653 * identify the code system or identifier system.). This is the 654 * underlying object with id, value and extensions. The accessor 655 * "getValue" gives direct access to the value 656 */ 657 public StringType getValueElement() { 658 if (this.value == null) 659 if (Configuration.errorOnAutoCreate()) 660 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.value"); 661 else if (Configuration.doAutoCreate()) 662 this.value = new StringType(); // bb 663 return this.value; 664 } 665 666 public boolean hasValueElement() { 667 return this.value != null && !this.value.isEmpty(); 668 } 669 670 public boolean hasValue() { 671 return this.value != null && !this.value.isEmpty(); 672 } 673 674 /** 675 * @param value {@link #value} (The string that should be sent over the wire to 676 * identify the code system or identifier system.). This is the 677 * underlying object with id, value and extensions. The accessor 678 * "getValue" gives direct access to the value 679 */ 680 public NamingSystemUniqueIdComponent setValueElement(StringType value) { 681 this.value = value; 682 return this; 683 } 684 685 /** 686 * @return The string that should be sent over the wire to identify the code 687 * system or identifier system. 688 */ 689 public String getValue() { 690 return this.value == null ? null : this.value.getValue(); 691 } 692 693 /** 694 * @param value The string that should be sent over the wire to identify the 695 * code system or identifier system. 696 */ 697 public NamingSystemUniqueIdComponent setValue(String value) { 698 if (this.value == null) 699 this.value = new StringType(); 700 this.value.setValue(value); 701 return this; 702 } 703 704 /** 705 * @return {@link #preferred} (Indicates whether this identifier is the 706 * "preferred" identifier of this type.). This is the underlying object 707 * with id, value and extensions. The accessor "getPreferred" gives 708 * direct access to the value 709 */ 710 public BooleanType getPreferredElement() { 711 if (this.preferred == null) 712 if (Configuration.errorOnAutoCreate()) 713 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.preferred"); 714 else if (Configuration.doAutoCreate()) 715 this.preferred = new BooleanType(); // bb 716 return this.preferred; 717 } 718 719 public boolean hasPreferredElement() { 720 return this.preferred != null && !this.preferred.isEmpty(); 721 } 722 723 public boolean hasPreferred() { 724 return this.preferred != null && !this.preferred.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #preferred} (Indicates whether this identifier is the 729 * "preferred" identifier of this type.). This is the underlying 730 * object with id, value and extensions. The accessor 731 * "getPreferred" gives direct access to the value 732 */ 733 public NamingSystemUniqueIdComponent setPreferredElement(BooleanType value) { 734 this.preferred = value; 735 return this; 736 } 737 738 /** 739 * @return Indicates whether this identifier is the "preferred" identifier of 740 * this type. 741 */ 742 public boolean getPreferred() { 743 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 744 } 745 746 /** 747 * @param value Indicates whether this identifier is the "preferred" identifier 748 * of this type. 749 */ 750 public NamingSystemUniqueIdComponent setPreferred(boolean value) { 751 if (this.preferred == null) 752 this.preferred = new BooleanType(); 753 this.preferred.setValue(value); 754 return this; 755 } 756 757 /** 758 * @return {@link #period} (Identifies the period of time over which this 759 * identifier is considered appropriate to refer to the naming system. 760 * Outside of this window, the identifier might be non-deterministic.) 761 */ 762 public Period getPeriod() { 763 if (this.period == null) 764 if (Configuration.errorOnAutoCreate()) 765 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.period"); 766 else if (Configuration.doAutoCreate()) 767 this.period = new Period(); // cc 768 return this.period; 769 } 770 771 public boolean hasPeriod() { 772 return this.period != null && !this.period.isEmpty(); 773 } 774 775 /** 776 * @param value {@link #period} (Identifies the period of time over which this 777 * identifier is considered appropriate to refer to the naming 778 * system. Outside of this window, the identifier might be 779 * non-deterministic.) 780 */ 781 public NamingSystemUniqueIdComponent setPeriod(Period value) { 782 this.period = value; 783 return this; 784 } 785 786 protected void listChildren(List<Property> childrenList) { 787 super.listChildren(childrenList); 788 childrenList.add( 789 new Property("type", "code", "Identifies the unique identifier scheme used for this particular identifier.", 790 0, java.lang.Integer.MAX_VALUE, type)); 791 childrenList.add(new Property("value", "string", 792 "The string that should be sent over the wire to identify the code system or identifier system.", 0, 793 java.lang.Integer.MAX_VALUE, value)); 794 childrenList.add(new Property("preferred", "boolean", 795 "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 796 java.lang.Integer.MAX_VALUE, preferred)); 797 childrenList.add(new Property("period", "Period", 798 "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 799 0, java.lang.Integer.MAX_VALUE, period)); 800 } 801 802 @Override 803 public void setProperty(String name, Base value) throws FHIRException { 804 if (name.equals("type")) 805 this.type = new NamingSystemIdentifierTypeEnumFactory().fromType(value); // Enumeration<NamingSystemIdentifierType> 806 else if (name.equals("value")) 807 this.value = castToString(value); // StringType 808 else if (name.equals("preferred")) 809 this.preferred = castToBoolean(value); // BooleanType 810 else if (name.equals("period")) 811 this.period = castToPeriod(value); // Period 812 else 813 super.setProperty(name, value); 814 } 815 816 @Override 817 public Base addChild(String name) throws FHIRException { 818 if (name.equals("type")) { 819 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.type"); 820 } else if (name.equals("value")) { 821 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.value"); 822 } else if (name.equals("preferred")) { 823 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.preferred"); 824 } else if (name.equals("period")) { 825 this.period = new Period(); 826 return this.period; 827 } else 828 return super.addChild(name); 829 } 830 831 public NamingSystemUniqueIdComponent copy() { 832 NamingSystemUniqueIdComponent dst = new NamingSystemUniqueIdComponent(); 833 copyValues(dst); 834 dst.type = type == null ? null : type.copy(); 835 dst.value = value == null ? null : value.copy(); 836 dst.preferred = preferred == null ? null : preferred.copy(); 837 dst.period = period == null ? null : period.copy(); 838 return dst; 839 } 840 841 @Override 842 public boolean equalsDeep(Base other) { 843 if (!super.equalsDeep(other)) 844 return false; 845 if (!(other instanceof NamingSystemUniqueIdComponent)) 846 return false; 847 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other; 848 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) 849 && compareDeep(preferred, o.preferred, true) && compareDeep(period, o.period, true); 850 } 851 852 @Override 853 public boolean equalsShallow(Base other) { 854 if (!super.equalsShallow(other)) 855 return false; 856 if (!(other instanceof NamingSystemUniqueIdComponent)) 857 return false; 858 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other; 859 return compareValues(type, o.type, true) && compareValues(value, o.value, true) 860 && compareValues(preferred, o.preferred, true); 861 } 862 863 public boolean isEmpty() { 864 return super.isEmpty() && (type == null || type.isEmpty()) && (value == null || value.isEmpty()) 865 && (preferred == null || preferred.isEmpty()) && (period == null || period.isEmpty()); 866 } 867 868 public String fhirType() { 869 return "NamingSystem.uniqueId"; 870 871 } 872 873 } 874 875 /** 876 * The descriptive name of this particular identifier type or code system. 877 */ 878 @Child(name = "name", type = { StringType.class }, order = 0, min = 1, max = 1, modifier = false, summary = false) 879 @Description(shortDefinition = "Human-readable label", formalDefinition = "The descriptive name of this particular identifier type or code system.") 880 protected StringType name; 881 882 /** 883 * Indicates whether the naming system is "ready for use" or not. 884 */ 885 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = false) 886 @Description(shortDefinition = "draft | active | retired", formalDefinition = "Indicates whether the naming system is \"ready for use\" or not.") 887 protected Enumeration<ConformanceResourceStatus> status; 888 889 /** 890 * Indicates the purpose for the naming system - what kinds of things does it 891 * make unique? 892 */ 893 @Child(name = "kind", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 894 @Description(shortDefinition = "codesystem | identifier | root", formalDefinition = "Indicates the purpose for the naming system - what kinds of things does it make unique?") 895 protected Enumeration<NamingSystemType> kind; 896 897 /** 898 * The name of the individual or organization that published the naming system. 899 */ 900 @Child(name = "publisher", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 901 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the naming system.") 902 protected StringType publisher; 903 904 /** 905 * Contacts to assist a user in finding and communicating with the publisher. 906 */ 907 @Child(name = "contact", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 908 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 909 protected List<NamingSystemContactComponent> contact; 910 911 /** 912 * The name of the organization that is responsible for issuing identifiers or 913 * codes for this namespace and ensuring their non-collision. 914 */ 915 @Child(name = "responsible", type = { 916 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 917 @Description(shortDefinition = "Who maintains system namespace?", formalDefinition = "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.") 918 protected StringType responsible; 919 920 /** 921 * The date (and optionally time) when the system was registered or published. 922 * The date must change when the business version changes, if it does, and it 923 * must change if the status code changes. In addition, it should change when 924 * the substantive content of the registration changes. 925 */ 926 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 927 @Description(shortDefinition = "Publication Date(/time)", formalDefinition = "The date (and optionally time) when the system was registered or published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the registration changes.") 928 protected DateTimeType date; 929 930 /** 931 * Categorizes a naming system for easier search by grouping related naming 932 * systems. 933 */ 934 @Child(name = "type", type = { 935 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 936 @Description(shortDefinition = "e.g. driver, provider, patient, bank etc.", formalDefinition = "Categorizes a naming system for easier search by grouping related naming systems.") 937 protected CodeableConcept type; 938 939 /** 940 * Details about what the namespace identifies including scope, granularity, 941 * version labeling, etc. 942 */ 943 @Child(name = "description", type = { 944 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 945 @Description(shortDefinition = "What does naming system identify?", formalDefinition = "Details about what the namespace identifies including scope, granularity, version labeling, etc.") 946 protected StringType description; 947 948 /** 949 * The content was developed with a focus and intent of supporting the contexts 950 * that are listed. These terms may be used to assist with indexing and 951 * searching of naming systems. 952 */ 953 @Child(name = "useContext", type = { 954 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 955 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of naming systems.") 956 protected List<CodeableConcept> useContext; 957 958 /** 959 * Provides guidance on the use of the namespace, including the handling of 960 * formatting characters, use of upper vs. lower case, etc. 961 */ 962 @Child(name = "usage", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 963 @Description(shortDefinition = "How/where is it used", formalDefinition = "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.") 964 protected StringType usage; 965 966 /** 967 * Indicates how the system may be identified when referenced in electronic 968 * exchange. 969 */ 970 @Child(name = "uniqueId", type = {}, order = 11, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 971 @Description(shortDefinition = "Unique identifiers used for system", formalDefinition = "Indicates how the system may be identified when referenced in electronic exchange.") 972 protected List<NamingSystemUniqueIdComponent> uniqueId; 973 974 /** 975 * For naming systems that are retired, indicates the naming system that should 976 * be used in their place (if any). 977 */ 978 @Child(name = "replacedBy", type = { 979 NamingSystem.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 980 @Description(shortDefinition = "Use this instead", formalDefinition = "For naming systems that are retired, indicates the naming system that should be used in their place (if any).") 981 protected Reference replacedBy; 982 983 /** 984 * The actual object that is the target of the reference (For naming systems 985 * that are retired, indicates the naming system that should be used in their 986 * place (if any).) 987 */ 988 protected NamingSystem replacedByTarget; 989 990 private static final long serialVersionUID = -1337110053L; 991 992 /* 993 * Constructor 994 */ 995 public NamingSystem() { 996 super(); 997 } 998 999 /* 1000 * Constructor 1001 */ 1002 public NamingSystem(StringType name, Enumeration<ConformanceResourceStatus> status, 1003 Enumeration<NamingSystemType> kind, DateTimeType date) { 1004 super(); 1005 this.name = name; 1006 this.status = status; 1007 this.kind = kind; 1008 this.date = date; 1009 } 1010 1011 /** 1012 * @return {@link #name} (The descriptive name of this particular identifier 1013 * type or code system.). This is the underlying object with id, value 1014 * and extensions. The accessor "getName" gives direct access to the 1015 * value 1016 */ 1017 public StringType getNameElement() { 1018 if (this.name == null) 1019 if (Configuration.errorOnAutoCreate()) 1020 throw new Error("Attempt to auto-create NamingSystem.name"); 1021 else if (Configuration.doAutoCreate()) 1022 this.name = new StringType(); // bb 1023 return this.name; 1024 } 1025 1026 public boolean hasNameElement() { 1027 return this.name != null && !this.name.isEmpty(); 1028 } 1029 1030 public boolean hasName() { 1031 return this.name != null && !this.name.isEmpty(); 1032 } 1033 1034 /** 1035 * @param value {@link #name} (The descriptive name of this particular 1036 * identifier type or code system.). This is the underlying object 1037 * with id, value and extensions. The accessor "getName" gives 1038 * direct access to the value 1039 */ 1040 public NamingSystem setNameElement(StringType value) { 1041 this.name = value; 1042 return this; 1043 } 1044 1045 /** 1046 * @return The descriptive name of this particular identifier type or code 1047 * system. 1048 */ 1049 public String getName() { 1050 return this.name == null ? null : this.name.getValue(); 1051 } 1052 1053 /** 1054 * @param value The descriptive name of this particular identifier type or code 1055 * system. 1056 */ 1057 public NamingSystem setName(String value) { 1058 if (this.name == null) 1059 this.name = new StringType(); 1060 this.name.setValue(value); 1061 return this; 1062 } 1063 1064 /** 1065 * @return {@link #status} (Indicates whether the naming system is "ready for 1066 * use" or not.). This is the underlying object with id, value and 1067 * extensions. The accessor "getStatus" gives direct access to the value 1068 */ 1069 public Enumeration<ConformanceResourceStatus> getStatusElement() { 1070 if (this.status == null) 1071 if (Configuration.errorOnAutoCreate()) 1072 throw new Error("Attempt to auto-create NamingSystem.status"); 1073 else if (Configuration.doAutoCreate()) 1074 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 1075 return this.status; 1076 } 1077 1078 public boolean hasStatusElement() { 1079 return this.status != null && !this.status.isEmpty(); 1080 } 1081 1082 public boolean hasStatus() { 1083 return this.status != null && !this.status.isEmpty(); 1084 } 1085 1086 /** 1087 * @param value {@link #status} (Indicates whether the naming system is "ready 1088 * for use" or not.). This is the underlying object with id, value 1089 * and extensions. The accessor "getStatus" gives direct access to 1090 * the value 1091 */ 1092 public NamingSystem setStatusElement(Enumeration<ConformanceResourceStatus> value) { 1093 this.status = value; 1094 return this; 1095 } 1096 1097 /** 1098 * @return Indicates whether the naming system is "ready for use" or not. 1099 */ 1100 public ConformanceResourceStatus getStatus() { 1101 return this.status == null ? null : this.status.getValue(); 1102 } 1103 1104 /** 1105 * @param value Indicates whether the naming system is "ready for use" or not. 1106 */ 1107 public NamingSystem setStatus(ConformanceResourceStatus value) { 1108 if (this.status == null) 1109 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 1110 this.status.setValue(value); 1111 return this; 1112 } 1113 1114 /** 1115 * @return {@link #kind} (Indicates the purpose for the naming system - what 1116 * kinds of things does it make unique?). This is the underlying object 1117 * with id, value and extensions. The accessor "getKind" gives direct 1118 * access to the value 1119 */ 1120 public Enumeration<NamingSystemType> getKindElement() { 1121 if (this.kind == null) 1122 if (Configuration.errorOnAutoCreate()) 1123 throw new Error("Attempt to auto-create NamingSystem.kind"); 1124 else if (Configuration.doAutoCreate()) 1125 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); // bb 1126 return this.kind; 1127 } 1128 1129 public boolean hasKindElement() { 1130 return this.kind != null && !this.kind.isEmpty(); 1131 } 1132 1133 public boolean hasKind() { 1134 return this.kind != null && !this.kind.isEmpty(); 1135 } 1136 1137 /** 1138 * @param value {@link #kind} (Indicates the purpose for the naming system - 1139 * what kinds of things does it make unique?). This is the 1140 * underlying object with id, value and extensions. The accessor 1141 * "getKind" gives direct access to the value 1142 */ 1143 public NamingSystem setKindElement(Enumeration<NamingSystemType> value) { 1144 this.kind = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return Indicates the purpose for the naming system - what kinds of things 1150 * does it make unique? 1151 */ 1152 public NamingSystemType getKind() { 1153 return this.kind == null ? null : this.kind.getValue(); 1154 } 1155 1156 /** 1157 * @param value Indicates the purpose for the naming system - what kinds of 1158 * things does it make unique? 1159 */ 1160 public NamingSystem setKind(NamingSystemType value) { 1161 if (this.kind == null) 1162 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); 1163 this.kind.setValue(value); 1164 return this; 1165 } 1166 1167 /** 1168 * @return {@link #publisher} (The name of the individual or organization that 1169 * published the naming system.). This is the underlying object with id, 1170 * value and extensions. The accessor "getPublisher" gives direct access 1171 * to the value 1172 */ 1173 public StringType getPublisherElement() { 1174 if (this.publisher == null) 1175 if (Configuration.errorOnAutoCreate()) 1176 throw new Error("Attempt to auto-create NamingSystem.publisher"); 1177 else if (Configuration.doAutoCreate()) 1178 this.publisher = new StringType(); // bb 1179 return this.publisher; 1180 } 1181 1182 public boolean hasPublisherElement() { 1183 return this.publisher != null && !this.publisher.isEmpty(); 1184 } 1185 1186 public boolean hasPublisher() { 1187 return this.publisher != null && !this.publisher.isEmpty(); 1188 } 1189 1190 /** 1191 * @param value {@link #publisher} (The name of the individual or organization 1192 * that published the naming system.). This is the underlying 1193 * object with id, value and extensions. The accessor 1194 * "getPublisher" gives direct access to the value 1195 */ 1196 public NamingSystem setPublisherElement(StringType value) { 1197 this.publisher = value; 1198 return this; 1199 } 1200 1201 /** 1202 * @return The name of the individual or organization that published the naming 1203 * system. 1204 */ 1205 public String getPublisher() { 1206 return this.publisher == null ? null : this.publisher.getValue(); 1207 } 1208 1209 /** 1210 * @param value The name of the individual or organization that published the 1211 * naming system. 1212 */ 1213 public NamingSystem setPublisher(String value) { 1214 if (Utilities.noString(value)) 1215 this.publisher = null; 1216 else { 1217 if (this.publisher == null) 1218 this.publisher = new StringType(); 1219 this.publisher.setValue(value); 1220 } 1221 return this; 1222 } 1223 1224 /** 1225 * @return {@link #contact} (Contacts to assist a user in finding and 1226 * communicating with the publisher.) 1227 */ 1228 public List<NamingSystemContactComponent> getContact() { 1229 if (this.contact == null) 1230 this.contact = new ArrayList<NamingSystemContactComponent>(); 1231 return this.contact; 1232 } 1233 1234 public boolean hasContact() { 1235 if (this.contact == null) 1236 return false; 1237 for (NamingSystemContactComponent item : this.contact) 1238 if (!item.isEmpty()) 1239 return true; 1240 return false; 1241 } 1242 1243 /** 1244 * @return {@link #contact} (Contacts to assist a user in finding and 1245 * communicating with the publisher.) 1246 */ 1247 // syntactic sugar 1248 public NamingSystemContactComponent addContact() { // 3 1249 NamingSystemContactComponent t = new NamingSystemContactComponent(); 1250 if (this.contact == null) 1251 this.contact = new ArrayList<NamingSystemContactComponent>(); 1252 this.contact.add(t); 1253 return t; 1254 } 1255 1256 // syntactic sugar 1257 public NamingSystem addContact(NamingSystemContactComponent t) { // 3 1258 if (t == null) 1259 return this; 1260 if (this.contact == null) 1261 this.contact = new ArrayList<NamingSystemContactComponent>(); 1262 this.contact.add(t); 1263 return this; 1264 } 1265 1266 /** 1267 * @return {@link #responsible} (The name of the organization that is 1268 * responsible for issuing identifiers or codes for this namespace and 1269 * ensuring their non-collision.). This is the underlying object with 1270 * id, value and extensions. The accessor "getResponsible" gives direct 1271 * access to the value 1272 */ 1273 public StringType getResponsibleElement() { 1274 if (this.responsible == null) 1275 if (Configuration.errorOnAutoCreate()) 1276 throw new Error("Attempt to auto-create NamingSystem.responsible"); 1277 else if (Configuration.doAutoCreate()) 1278 this.responsible = new StringType(); // bb 1279 return this.responsible; 1280 } 1281 1282 public boolean hasResponsibleElement() { 1283 return this.responsible != null && !this.responsible.isEmpty(); 1284 } 1285 1286 public boolean hasResponsible() { 1287 return this.responsible != null && !this.responsible.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #responsible} (The name of the organization that is 1292 * responsible for issuing identifiers or codes for this namespace 1293 * and ensuring their non-collision.). This is the underlying 1294 * object with id, value and extensions. The accessor 1295 * "getResponsible" gives direct access to the value 1296 */ 1297 public NamingSystem setResponsibleElement(StringType value) { 1298 this.responsible = value; 1299 return this; 1300 } 1301 1302 /** 1303 * @return The name of the organization that is responsible for issuing 1304 * identifiers or codes for this namespace and ensuring their 1305 * non-collision. 1306 */ 1307 public String getResponsible() { 1308 return this.responsible == null ? null : this.responsible.getValue(); 1309 } 1310 1311 /** 1312 * @param value The name of the organization that is responsible for issuing 1313 * identifiers or codes for this namespace and ensuring their 1314 * non-collision. 1315 */ 1316 public NamingSystem setResponsible(String value) { 1317 if (Utilities.noString(value)) 1318 this.responsible = null; 1319 else { 1320 if (this.responsible == null) 1321 this.responsible = new StringType(); 1322 this.responsible.setValue(value); 1323 } 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #date} (The date (and optionally time) when the system was 1329 * registered or published. The date must change when the business 1330 * version changes, if it does, and it must change if the status code 1331 * changes. In addition, it should change when the substantive content 1332 * of the registration changes.). This is the underlying object with id, 1333 * value and extensions. The accessor "getDate" gives direct access to 1334 * the value 1335 */ 1336 public DateTimeType getDateElement() { 1337 if (this.date == null) 1338 if (Configuration.errorOnAutoCreate()) 1339 throw new Error("Attempt to auto-create NamingSystem.date"); 1340 else if (Configuration.doAutoCreate()) 1341 this.date = new DateTimeType(); // bb 1342 return this.date; 1343 } 1344 1345 public boolean hasDateElement() { 1346 return this.date != null && !this.date.isEmpty(); 1347 } 1348 1349 public boolean hasDate() { 1350 return this.date != null && !this.date.isEmpty(); 1351 } 1352 1353 /** 1354 * @param value {@link #date} (The date (and optionally time) when the system 1355 * was registered or published. The date must change when the 1356 * business version changes, if it does, and it must change if the 1357 * status code changes. In addition, it should change when the 1358 * substantive content of the registration changes.). This is the 1359 * underlying object with id, value and extensions. The accessor 1360 * "getDate" gives direct access to the value 1361 */ 1362 public NamingSystem setDateElement(DateTimeType value) { 1363 this.date = value; 1364 return this; 1365 } 1366 1367 /** 1368 * @return The date (and optionally time) when the system was registered or 1369 * published. The date must change when the business version changes, if 1370 * it does, and it must change if the status code changes. In addition, 1371 * it should change when the substantive content of the registration 1372 * changes. 1373 */ 1374 public Date getDate() { 1375 return this.date == null ? null : this.date.getValue(); 1376 } 1377 1378 /** 1379 * @param value The date (and optionally time) when the system was registered or 1380 * published. The date must change when the business version 1381 * changes, if it does, and it must change if the status code 1382 * changes. In addition, it should change when the substantive 1383 * content of the registration changes. 1384 */ 1385 public NamingSystem setDate(Date value) { 1386 if (this.date == null) 1387 this.date = new DateTimeType(); 1388 this.date.setValue(value); 1389 return this; 1390 } 1391 1392 /** 1393 * @return {@link #type} (Categorizes a naming system for easier search by 1394 * grouping related naming systems.) 1395 */ 1396 public CodeableConcept getType() { 1397 if (this.type == null) 1398 if (Configuration.errorOnAutoCreate()) 1399 throw new Error("Attempt to auto-create NamingSystem.type"); 1400 else if (Configuration.doAutoCreate()) 1401 this.type = new CodeableConcept(); // cc 1402 return this.type; 1403 } 1404 1405 public boolean hasType() { 1406 return this.type != null && !this.type.isEmpty(); 1407 } 1408 1409 /** 1410 * @param value {@link #type} (Categorizes a naming system for easier search by 1411 * grouping related naming systems.) 1412 */ 1413 public NamingSystem setType(CodeableConcept value) { 1414 this.type = value; 1415 return this; 1416 } 1417 1418 /** 1419 * @return {@link #description} (Details about what the namespace identifies 1420 * including scope, granularity, version labeling, etc.). This is the 1421 * underlying object with id, value and extensions. The accessor 1422 * "getDescription" gives direct access to the value 1423 */ 1424 public StringType getDescriptionElement() { 1425 if (this.description == null) 1426 if (Configuration.errorOnAutoCreate()) 1427 throw new Error("Attempt to auto-create NamingSystem.description"); 1428 else if (Configuration.doAutoCreate()) 1429 this.description = new StringType(); // bb 1430 return this.description; 1431 } 1432 1433 public boolean hasDescriptionElement() { 1434 return this.description != null && !this.description.isEmpty(); 1435 } 1436 1437 public boolean hasDescription() { 1438 return this.description != null && !this.description.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #description} (Details about what the namespace 1443 * identifies including scope, granularity, version labeling, 1444 * etc.). This is the underlying object with id, value and 1445 * extensions. The accessor "getDescription" gives direct access to 1446 * the value 1447 */ 1448 public NamingSystem setDescriptionElement(StringType value) { 1449 this.description = value; 1450 return this; 1451 } 1452 1453 /** 1454 * @return Details about what the namespace identifies including scope, 1455 * granularity, version labeling, etc. 1456 */ 1457 public String getDescription() { 1458 return this.description == null ? null : this.description.getValue(); 1459 } 1460 1461 /** 1462 * @param value Details about what the namespace identifies including scope, 1463 * granularity, version labeling, etc. 1464 */ 1465 public NamingSystem setDescription(String value) { 1466 if (Utilities.noString(value)) 1467 this.description = null; 1468 else { 1469 if (this.description == null) 1470 this.description = new StringType(); 1471 this.description.setValue(value); 1472 } 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #useContext} (The content was developed with a focus and 1478 * intent of supporting the contexts that are listed. These terms may be 1479 * used to assist with indexing and searching of naming systems.) 1480 */ 1481 public List<CodeableConcept> getUseContext() { 1482 if (this.useContext == null) 1483 this.useContext = new ArrayList<CodeableConcept>(); 1484 return this.useContext; 1485 } 1486 1487 public boolean hasUseContext() { 1488 if (this.useContext == null) 1489 return false; 1490 for (CodeableConcept item : this.useContext) 1491 if (!item.isEmpty()) 1492 return true; 1493 return false; 1494 } 1495 1496 /** 1497 * @return {@link #useContext} (The content was developed with a focus and 1498 * intent of supporting the contexts that are listed. These terms may be 1499 * used to assist with indexing and searching of naming systems.) 1500 */ 1501 // syntactic sugar 1502 public CodeableConcept addUseContext() { // 3 1503 CodeableConcept t = new CodeableConcept(); 1504 if (this.useContext == null) 1505 this.useContext = new ArrayList<CodeableConcept>(); 1506 this.useContext.add(t); 1507 return t; 1508 } 1509 1510 // syntactic sugar 1511 public NamingSystem addUseContext(CodeableConcept t) { // 3 1512 if (t == null) 1513 return this; 1514 if (this.useContext == null) 1515 this.useContext = new ArrayList<CodeableConcept>(); 1516 this.useContext.add(t); 1517 return this; 1518 } 1519 1520 /** 1521 * @return {@link #usage} (Provides guidance on the use of the namespace, 1522 * including the handling of formatting characters, use of upper vs. 1523 * lower case, etc.). This is the underlying object with id, value and 1524 * extensions. The accessor "getUsage" gives direct access to the value 1525 */ 1526 public StringType getUsageElement() { 1527 if (this.usage == null) 1528 if (Configuration.errorOnAutoCreate()) 1529 throw new Error("Attempt to auto-create NamingSystem.usage"); 1530 else if (Configuration.doAutoCreate()) 1531 this.usage = new StringType(); // bb 1532 return this.usage; 1533 } 1534 1535 public boolean hasUsageElement() { 1536 return this.usage != null && !this.usage.isEmpty(); 1537 } 1538 1539 public boolean hasUsage() { 1540 return this.usage != null && !this.usage.isEmpty(); 1541 } 1542 1543 /** 1544 * @param value {@link #usage} (Provides guidance on the use of the namespace, 1545 * including the handling of formatting characters, use of upper 1546 * vs. lower case, etc.). This is the underlying object with id, 1547 * value and extensions. The accessor "getUsage" gives direct 1548 * access to the value 1549 */ 1550 public NamingSystem setUsageElement(StringType value) { 1551 this.usage = value; 1552 return this; 1553 } 1554 1555 /** 1556 * @return Provides guidance on the use of the namespace, including the handling 1557 * of formatting characters, use of upper vs. lower case, etc. 1558 */ 1559 public String getUsage() { 1560 return this.usage == null ? null : this.usage.getValue(); 1561 } 1562 1563 /** 1564 * @param value Provides guidance on the use of the namespace, including the 1565 * handling of formatting characters, use of upper vs. lower case, 1566 * etc. 1567 */ 1568 public NamingSystem setUsage(String value) { 1569 if (Utilities.noString(value)) 1570 this.usage = null; 1571 else { 1572 if (this.usage == null) 1573 this.usage = new StringType(); 1574 this.usage.setValue(value); 1575 } 1576 return this; 1577 } 1578 1579 /** 1580 * @return {@link #uniqueId} (Indicates how the system may be identified when 1581 * referenced in electronic exchange.) 1582 */ 1583 public List<NamingSystemUniqueIdComponent> getUniqueId() { 1584 if (this.uniqueId == null) 1585 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1586 return this.uniqueId; 1587 } 1588 1589 public boolean hasUniqueId() { 1590 if (this.uniqueId == null) 1591 return false; 1592 for (NamingSystemUniqueIdComponent item : this.uniqueId) 1593 if (!item.isEmpty()) 1594 return true; 1595 return false; 1596 } 1597 1598 /** 1599 * @return {@link #uniqueId} (Indicates how the system may be identified when 1600 * referenced in electronic exchange.) 1601 */ 1602 // syntactic sugar 1603 public NamingSystemUniqueIdComponent addUniqueId() { // 3 1604 NamingSystemUniqueIdComponent t = new NamingSystemUniqueIdComponent(); 1605 if (this.uniqueId == null) 1606 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1607 this.uniqueId.add(t); 1608 return t; 1609 } 1610 1611 // syntactic sugar 1612 public NamingSystem addUniqueId(NamingSystemUniqueIdComponent t) { // 3 1613 if (t == null) 1614 return this; 1615 if (this.uniqueId == null) 1616 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1617 this.uniqueId.add(t); 1618 return this; 1619 } 1620 1621 /** 1622 * @return {@link #replacedBy} (For naming systems that are retired, indicates 1623 * the naming system that should be used in their place (if any).) 1624 */ 1625 public Reference getReplacedBy() { 1626 if (this.replacedBy == null) 1627 if (Configuration.errorOnAutoCreate()) 1628 throw new Error("Attempt to auto-create NamingSystem.replacedBy"); 1629 else if (Configuration.doAutoCreate()) 1630 this.replacedBy = new Reference(); // cc 1631 return this.replacedBy; 1632 } 1633 1634 public boolean hasReplacedBy() { 1635 return this.replacedBy != null && !this.replacedBy.isEmpty(); 1636 } 1637 1638 /** 1639 * @param value {@link #replacedBy} (For naming systems that are retired, 1640 * indicates the naming system that should be used in their place 1641 * (if any).) 1642 */ 1643 public NamingSystem setReplacedBy(Reference value) { 1644 this.replacedBy = value; 1645 return this; 1646 } 1647 1648 /** 1649 * @return {@link #replacedBy} The actual object that is the target of the 1650 * reference. The reference library doesn't populate this, but you can 1651 * use it to hold the resource if you resolve it. (For naming systems 1652 * that are retired, indicates the naming system that should be used in 1653 * their place (if any).) 1654 */ 1655 public NamingSystem getReplacedByTarget() { 1656 if (this.replacedByTarget == null) 1657 if (Configuration.errorOnAutoCreate()) 1658 throw new Error("Attempt to auto-create NamingSystem.replacedBy"); 1659 else if (Configuration.doAutoCreate()) 1660 this.replacedByTarget = new NamingSystem(); // aa 1661 return this.replacedByTarget; 1662 } 1663 1664 /** 1665 * @param value {@link #replacedBy} The actual object that is the target of the 1666 * reference. The reference library doesn't use these, but you can 1667 * use it to hold the resource if you resolve it. (For naming 1668 * systems that are retired, indicates the naming system that 1669 * should be used in their place (if any).) 1670 */ 1671 public NamingSystem setReplacedByTarget(NamingSystem value) { 1672 this.replacedByTarget = value; 1673 return this; 1674 } 1675 1676 protected void listChildren(List<Property> childrenList) { 1677 super.listChildren(childrenList); 1678 childrenList 1679 .add(new Property("name", "string", "The descriptive name of this particular identifier type or code system.", 1680 0, java.lang.Integer.MAX_VALUE, name)); 1681 childrenList.add(new Property("status", "code", "Indicates whether the naming system is \"ready for use\" or not.", 1682 0, java.lang.Integer.MAX_VALUE, status)); 1683 childrenList.add(new Property("kind", "code", 1684 "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1685 java.lang.Integer.MAX_VALUE, kind)); 1686 childrenList.add(new Property("publisher", "string", 1687 "The name of the individual or organization that published the naming system.", 0, java.lang.Integer.MAX_VALUE, 1688 publisher)); 1689 childrenList 1690 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 1691 0, java.lang.Integer.MAX_VALUE, contact)); 1692 childrenList.add(new Property("responsible", "string", 1693 "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 1694 0, java.lang.Integer.MAX_VALUE, responsible)); 1695 childrenList.add(new Property("date", "dateTime", 1696 "The date (and optionally time) when the system was registered or published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the registration changes.", 1697 0, java.lang.Integer.MAX_VALUE, date)); 1698 childrenList.add(new Property("type", "CodeableConcept", 1699 "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1700 java.lang.Integer.MAX_VALUE, type)); 1701 childrenList.add(new Property("description", "string", 1702 "Details about what the namespace identifies including scope, granularity, version labeling, etc.", 0, 1703 java.lang.Integer.MAX_VALUE, description)); 1704 childrenList.add(new Property("useContext", "CodeableConcept", 1705 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of naming systems.", 1706 0, java.lang.Integer.MAX_VALUE, useContext)); 1707 childrenList.add(new Property("usage", "string", 1708 "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 1709 0, java.lang.Integer.MAX_VALUE, usage)); 1710 childrenList.add(new Property("uniqueId", "", 1711 "Indicates how the system may be identified when referenced in electronic exchange.", 0, 1712 java.lang.Integer.MAX_VALUE, uniqueId)); 1713 childrenList.add(new Property("replacedBy", "Reference(NamingSystem)", 1714 "For naming systems that are retired, indicates the naming system that should be used in their place (if any).", 1715 0, java.lang.Integer.MAX_VALUE, replacedBy)); 1716 } 1717 1718 @Override 1719 public void setProperty(String name, Base value) throws FHIRException { 1720 if (name.equals("name")) 1721 this.name = castToString(value); // StringType 1722 else if (name.equals("status")) 1723 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 1724 else if (name.equals("kind")) 1725 this.kind = new NamingSystemTypeEnumFactory().fromType(value); // Enumeration<NamingSystemType> 1726 else if (name.equals("publisher")) 1727 this.publisher = castToString(value); // StringType 1728 else if (name.equals("contact")) 1729 this.getContact().add((NamingSystemContactComponent) value); 1730 else if (name.equals("responsible")) 1731 this.responsible = castToString(value); // StringType 1732 else if (name.equals("date")) 1733 this.date = castToDateTime(value); // DateTimeType 1734 else if (name.equals("type")) 1735 this.type = castToCodeableConcept(value); // CodeableConcept 1736 else if (name.equals("description")) 1737 this.description = castToString(value); // StringType 1738 else if (name.equals("useContext")) 1739 this.getUseContext().add(castToCodeableConcept(value)); 1740 else if (name.equals("usage")) 1741 this.usage = castToString(value); // StringType 1742 else if (name.equals("uniqueId")) 1743 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); 1744 else if (name.equals("replacedBy")) 1745 this.replacedBy = castToReference(value); // Reference 1746 else 1747 super.setProperty(name, value); 1748 } 1749 1750 @Override 1751 public Base addChild(String name) throws FHIRException { 1752 if (name.equals("name")) { 1753 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 1754 } else if (name.equals("status")) { 1755 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.status"); 1756 } else if (name.equals("kind")) { 1757 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.kind"); 1758 } else if (name.equals("publisher")) { 1759 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.publisher"); 1760 } else if (name.equals("contact")) { 1761 return addContact(); 1762 } else if (name.equals("responsible")) { 1763 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.responsible"); 1764 } else if (name.equals("date")) { 1765 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.date"); 1766 } else if (name.equals("type")) { 1767 this.type = new CodeableConcept(); 1768 return this.type; 1769 } else if (name.equals("description")) { 1770 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.description"); 1771 } else if (name.equals("useContext")) { 1772 return addUseContext(); 1773 } else if (name.equals("usage")) { 1774 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.usage"); 1775 } else if (name.equals("uniqueId")) { 1776 return addUniqueId(); 1777 } else if (name.equals("replacedBy")) { 1778 this.replacedBy = new Reference(); 1779 return this.replacedBy; 1780 } else 1781 return super.addChild(name); 1782 } 1783 1784 public String fhirType() { 1785 return "NamingSystem"; 1786 1787 } 1788 1789 public NamingSystem copy() { 1790 NamingSystem dst = new NamingSystem(); 1791 copyValues(dst); 1792 dst.name = name == null ? null : name.copy(); 1793 dst.status = status == null ? null : status.copy(); 1794 dst.kind = kind == null ? null : kind.copy(); 1795 dst.publisher = publisher == null ? null : publisher.copy(); 1796 if (contact != null) { 1797 dst.contact = new ArrayList<NamingSystemContactComponent>(); 1798 for (NamingSystemContactComponent i : contact) 1799 dst.contact.add(i.copy()); 1800 } 1801 ; 1802 dst.responsible = responsible == null ? null : responsible.copy(); 1803 dst.date = date == null ? null : date.copy(); 1804 dst.type = type == null ? null : type.copy(); 1805 dst.description = description == null ? null : description.copy(); 1806 if (useContext != null) { 1807 dst.useContext = new ArrayList<CodeableConcept>(); 1808 for (CodeableConcept i : useContext) 1809 dst.useContext.add(i.copy()); 1810 } 1811 ; 1812 dst.usage = usage == null ? null : usage.copy(); 1813 if (uniqueId != null) { 1814 dst.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1815 for (NamingSystemUniqueIdComponent i : uniqueId) 1816 dst.uniqueId.add(i.copy()); 1817 } 1818 ; 1819 dst.replacedBy = replacedBy == null ? null : replacedBy.copy(); 1820 return dst; 1821 } 1822 1823 protected NamingSystem typedCopy() { 1824 return copy(); 1825 } 1826 1827 @Override 1828 public boolean equalsDeep(Base other) { 1829 if (!super.equalsDeep(other)) 1830 return false; 1831 if (!(other instanceof NamingSystem)) 1832 return false; 1833 NamingSystem o = (NamingSystem) other; 1834 return compareDeep(name, o.name, true) && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) 1835 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 1836 && compareDeep(responsible, o.responsible, true) && compareDeep(date, o.date, true) 1837 && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 1838 && compareDeep(useContext, o.useContext, true) && compareDeep(usage, o.usage, true) 1839 && compareDeep(uniqueId, o.uniqueId, true) && compareDeep(replacedBy, o.replacedBy, true); 1840 } 1841 1842 @Override 1843 public boolean equalsShallow(Base other) { 1844 if (!super.equalsShallow(other)) 1845 return false; 1846 if (!(other instanceof NamingSystem)) 1847 return false; 1848 NamingSystem o = (NamingSystem) other; 1849 return compareValues(name, o.name, true) && compareValues(status, o.status, true) 1850 && compareValues(kind, o.kind, true) && compareValues(publisher, o.publisher, true) 1851 && compareValues(responsible, o.responsible, true) && compareValues(date, o.date, true) 1852 && compareValues(description, o.description, true) && compareValues(usage, o.usage, true); 1853 } 1854 1855 public boolean isEmpty() { 1856 return super.isEmpty() && (name == null || name.isEmpty()) && (status == null || status.isEmpty()) 1857 && (kind == null || kind.isEmpty()) && (publisher == null || publisher.isEmpty()) 1858 && (contact == null || contact.isEmpty()) && (responsible == null || responsible.isEmpty()) 1859 && (date == null || date.isEmpty()) && (type == null || type.isEmpty()) 1860 && (description == null || description.isEmpty()) && (useContext == null || useContext.isEmpty()) 1861 && (usage == null || usage.isEmpty()) && (uniqueId == null || uniqueId.isEmpty()) 1862 && (replacedBy == null || replacedBy.isEmpty()); 1863 } 1864 1865 @Override 1866 public ResourceType getResourceType() { 1867 return ResourceType.NamingSystem; 1868 } 1869 1870 @SearchParamDefinition(name = "date", path = "NamingSystem.date", description = "Publication Date(/time)", type = "date") 1871 public static final String SP_DATE = "date"; 1872 @SearchParamDefinition(name = "period", path = "NamingSystem.uniqueId.period", description = "When is identifier valid?", type = "date") 1873 public static final String SP_PERIOD = "period"; 1874 @SearchParamDefinition(name = "kind", path = "NamingSystem.kind", description = "codesystem | identifier | root", type = "token") 1875 public static final String SP_KIND = "kind"; 1876 @SearchParamDefinition(name = "type", path = "NamingSystem.type", description = "e.g. driver, provider, patient, bank etc.", type = "token") 1877 public static final String SP_TYPE = "type"; 1878 @SearchParamDefinition(name = "id-type", path = "NamingSystem.uniqueId.type", description = "oid | uuid | uri | other", type = "token") 1879 public static final String SP_IDTYPE = "id-type"; 1880 @SearchParamDefinition(name = "responsible", path = "NamingSystem.responsible", description = "Who maintains system namespace?", type = "string") 1881 public static final String SP_RESPONSIBLE = "responsible"; 1882 @SearchParamDefinition(name = "contact", path = "NamingSystem.contact.name", description = "Name of a individual to contact", type = "string") 1883 public static final String SP_CONTACT = "contact"; 1884 @SearchParamDefinition(name = "name", path = "NamingSystem.name", description = "Human-readable label", type = "string") 1885 public static final String SP_NAME = "name"; 1886 @SearchParamDefinition(name = "context", path = "NamingSystem.useContext", description = "Content intends to support these contexts", type = "token") 1887 public static final String SP_CONTEXT = "context"; 1888 @SearchParamDefinition(name = "publisher", path = "NamingSystem.publisher", description = "Name of the publisher (Organization or individual)", type = "string") 1889 public static final String SP_PUBLISHER = "publisher"; 1890 @SearchParamDefinition(name = "telecom", path = "NamingSystem.contact.telecom", description = "Contact details for individual or publisher", type = "token") 1891 public static final String SP_TELECOM = "telecom"; 1892 @SearchParamDefinition(name = "value", path = "NamingSystem.uniqueId.value", description = "The unique identifier", type = "string") 1893 public static final String SP_VALUE = "value"; 1894 @SearchParamDefinition(name = "replaced-by", path = "NamingSystem.replacedBy", description = "Use this instead", type = "reference") 1895 public static final String SP_REPLACEDBY = "replaced-by"; 1896 @SearchParamDefinition(name = "status", path = "NamingSystem.status", description = "draft | active | retired", type = "token") 1897 public static final String SP_STATUS = "status"; 1898 1899}