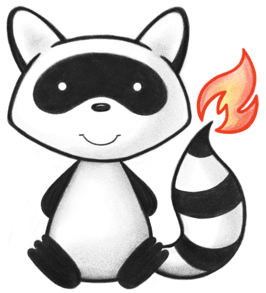
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.INarrative; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.xhtml.XhtmlNode; 041 042/** 043 * A human-readable formatted text, including images. 044 */ 045@DatatypeDef(name = "Narrative") 046public class Narrative extends BaseNarrative implements INarrative { 047 048 public enum NarrativeStatus { 049 /** 050 * The contents of the narrative are entirely generated from the structured data 051 * in the content. 052 */ 053 GENERATED, 054 /** 055 * The contents of the narrative are entirely generated from the structured data 056 * in the content and some of the content is generated from extensions 057 */ 058 EXTENSIONS, 059 /** 060 * The contents of the narrative contain additional information not found in the 061 * structured data 062 */ 063 ADDITIONAL, 064 /** 065 * The contents of the narrative are some equivalent of "No human-readable text 066 * provided in this case" 067 */ 068 EMPTY, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static NarrativeStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("generated".equals(codeString)) 078 return GENERATED; 079 if ("extensions".equals(codeString)) 080 return EXTENSIONS; 081 if ("additional".equals(codeString)) 082 return ADDITIONAL; 083 if ("empty".equals(codeString)) 084 return EMPTY; 085 throw new FHIRException("Unknown NarrativeStatus code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case GENERATED: 091 return "generated"; 092 case EXTENSIONS: 093 return "extensions"; 094 case ADDITIONAL: 095 return "additional"; 096 case EMPTY: 097 return "empty"; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105 public String getSystem() { 106 switch (this) { 107 case GENERATED: 108 return "http://hl7.org/fhir/narrative-status"; 109 case EXTENSIONS: 110 return "http://hl7.org/fhir/narrative-status"; 111 case ADDITIONAL: 112 return "http://hl7.org/fhir/narrative-status"; 113 case EMPTY: 114 return "http://hl7.org/fhir/narrative-status"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getDefinition() { 123 switch (this) { 124 case GENERATED: 125 return "The contents of the narrative are entirely generated from the structured data in the content."; 126 case EXTENSIONS: 127 return "The contents of the narrative are entirely generated from the structured data in the content and some of the content is generated from extensions"; 128 case ADDITIONAL: 129 return "The contents of the narrative contain additional information not found in the structured data"; 130 case EMPTY: 131 return "The contents of the narrative are some equivalent of \"No human-readable text provided in this case\""; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDisplay() { 140 switch (this) { 141 case GENERATED: 142 return "Generated"; 143 case EXTENSIONS: 144 return "Extensions"; 145 case ADDITIONAL: 146 return "Additional"; 147 case EMPTY: 148 return "Empty"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 } 156 157 public static class NarrativeStatusEnumFactory implements EnumFactory<NarrativeStatus> { 158 public NarrativeStatus fromCode(String codeString) throws IllegalArgumentException { 159 if (codeString == null || "".equals(codeString)) 160 if (codeString == null || "".equals(codeString)) 161 return null; 162 if ("generated".equals(codeString)) 163 return NarrativeStatus.GENERATED; 164 if ("extensions".equals(codeString)) 165 return NarrativeStatus.EXTENSIONS; 166 if ("additional".equals(codeString)) 167 return NarrativeStatus.ADDITIONAL; 168 if ("empty".equals(codeString)) 169 return NarrativeStatus.EMPTY; 170 throw new IllegalArgumentException("Unknown NarrativeStatus code '" + codeString + "'"); 171 } 172 173 public Enumeration<NarrativeStatus> fromType(Base code) throws FHIRException { 174 if (code == null || code.isEmpty()) 175 return null; 176 String codeString = ((PrimitiveType) code).asStringValue(); 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("generated".equals(codeString)) 180 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.GENERATED); 181 if ("extensions".equals(codeString)) 182 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EXTENSIONS); 183 if ("additional".equals(codeString)) 184 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.ADDITIONAL); 185 if ("empty".equals(codeString)) 186 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EMPTY); 187 throw new FHIRException("Unknown NarrativeStatus code '" + codeString + "'"); 188 } 189 190 public String toCode(NarrativeStatus code) { 191 if (code == NarrativeStatus.GENERATED) 192 return "generated"; 193 if (code == NarrativeStatus.EXTENSIONS) 194 return "extensions"; 195 if (code == NarrativeStatus.ADDITIONAL) 196 return "additional"; 197 if (code == NarrativeStatus.EMPTY) 198 return "empty"; 199 return "?"; 200 } 201 } 202 203 /** 204 * The status of the narrative - whether it's entirely generated (from just the 205 * defined data or the extensions too), or whether a human authored it and it 206 * may contain additional data. 207 */ 208 @Child(name = "status", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = false) 209 @Description(shortDefinition = "generated | extensions | additional | empty", formalDefinition = "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.") 210 protected Enumeration<NarrativeStatus> status; 211 212 /** 213 * The actual narrative content, a stripped down version of XHTML. 214 */ 215 @Child(name = "div", type = {}, order = 1, min = 1, max = 1, modifier = false, summary = false) 216 @Description(shortDefinition = "Limited xhtml content", formalDefinition = "The actual narrative content, a stripped down version of XHTML.") 217 protected XhtmlNode div; 218 219 private static final long serialVersionUID = 1463852859L; 220 221 /* 222 * Constructor 223 */ 224 public Narrative() { 225 super(); 226 } 227 228 /* 229 * Constructor 230 */ 231 public Narrative(Enumeration<NarrativeStatus> status, XhtmlNode div) { 232 super(); 233 this.status = status; 234 this.div = div; 235 } 236 237 /** 238 * @return {@link #status} (The status of the narrative - whether it's entirely 239 * generated (from just the defined data or the extensions too), or 240 * whether a human authored it and it may contain additional data.). 241 * This is the underlying object with id, value and extensions. The 242 * accessor "getStatus" gives direct access to the value 243 */ 244 public Enumeration<NarrativeStatus> getStatusElement() { 245 if (this.status == null) 246 if (Configuration.errorOnAutoCreate()) 247 throw new Error("Attempt to auto-create Narrative.status"); 248 else if (Configuration.doAutoCreate()) 249 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); // bb 250 return this.status; 251 } 252 253 public boolean hasStatusElement() { 254 return this.status != null && !this.status.isEmpty(); 255 } 256 257 public boolean hasStatus() { 258 return this.status != null && !this.status.isEmpty(); 259 } 260 261 /** 262 * @param value {@link #status} (The status of the narrative - whether it's 263 * entirely generated (from just the defined data or the extensions 264 * too), or whether a human authored it and it may contain 265 * additional data.). This is the underlying object with id, value 266 * and extensions. The accessor "getStatus" gives direct access to 267 * the value 268 */ 269 public Narrative setStatusElement(Enumeration<NarrativeStatus> value) { 270 this.status = value; 271 return this; 272 } 273 274 /** 275 * @return The status of the narrative - whether it's entirely generated (from 276 * just the defined data or the extensions too), or whether a human 277 * authored it and it may contain additional data. 278 */ 279 public NarrativeStatus getStatus() { 280 return this.status == null ? null : this.status.getValue(); 281 } 282 283 /** 284 * @param value The status of the narrative - whether it's entirely generated 285 * (from just the defined data or the extensions too), or whether a 286 * human authored it and it may contain additional data. 287 */ 288 public Narrative setStatus(NarrativeStatus value) { 289 if (this.status == null) 290 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); 291 this.status.setValue(value); 292 return this; 293 } 294 295 /** 296 * @return {@link #div} (The actual narrative content, a stripped down version 297 * of XHTML.) 298 */ 299 public XhtmlNode getDiv() { 300 if (this.div == null) 301 if (Configuration.errorOnAutoCreate()) 302 throw new Error("Attempt to auto-create Narrative.div"); 303 else if (Configuration.doAutoCreate()) 304 this.div = new XhtmlNode(); // cc 305 return this.div; 306 } 307 308 public boolean hasDiv() { 309 return this.div != null && !this.div.isEmpty(); 310 } 311 312 /** 313 * @param value {@link #div} (The actual narrative content, a stripped down 314 * version of XHTML.) 315 */ 316 public Narrative setDiv(XhtmlNode value) { 317 this.div = value; 318 return this; 319 } 320 321 protected void listChildren(List<Property> childrenList) { 322 super.listChildren(childrenList); 323 childrenList.add(new Property("status", "code", 324 "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.", 325 0, java.lang.Integer.MAX_VALUE, status)); 326 } 327 328 @Override 329 public void setProperty(String name, Base value) throws FHIRException { 330 if (name.equals("status")) 331 this.status = new NarrativeStatusEnumFactory().fromType(value); // Enumeration<NarrativeStatus> 332 else 333 super.setProperty(name, value); 334 } 335 336 @Override 337 public Base addChild(String name) throws FHIRException { 338 if (name.equals("status")) { 339 throw new FHIRException("Cannot call addChild on a singleton property Narrative.status"); 340 } else 341 return super.addChild(name); 342 } 343 344 public String fhirType() { 345 return "Narrative"; 346 347 } 348 349 public Narrative copy() { 350 Narrative dst = new Narrative(); 351 copyValues(dst); 352 dst.status = status == null ? null : status.copy(); 353 dst.div = div == null ? null : div.copy(); 354 return dst; 355 } 356 357 protected Narrative typedCopy() { 358 return copy(); 359 } 360 361 @Override 362 public boolean equalsDeep(Base other) { 363 if (!super.equalsDeep(other)) 364 return false; 365 if (!(other instanceof Narrative)) 366 return false; 367 Narrative o = (Narrative) other; 368 return compareDeep(status, o.status, true) && compareDeep(div, o.div, true); 369 } 370 371 @Override 372 public boolean equalsShallow(Base other) { 373 if (!super.equalsShallow(other)) 374 return false; 375 if (!(other instanceof Narrative)) 376 return false; 377 Narrative o = (Narrative) other; 378 return compareValues(status, o.status, true); 379 } 380 381 public boolean isEmpty() { 382 return super.isEmpty() && (status == null || status.isEmpty()) && (div == null || div.isEmpty()); 383 } 384 385}