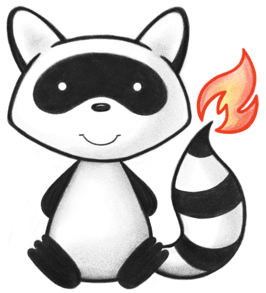
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A request to supply a diet, formula feeding (enteral) or oral nutritional 048 * supplement to a patient/resident. 049 */ 050@ResourceDef(name = "NutritionOrder", profile = "http://hl7.org/fhir/Profile/NutritionOrder") 051public class NutritionOrder extends DomainResource { 052 053 public enum NutritionOrderStatus { 054 /** 055 * The request has been proposed. 056 */ 057 PROPOSED, 058 /** 059 * The request is in preliminary form prior to being sent. 060 */ 061 DRAFT, 062 /** 063 * The request has been planned. 064 */ 065 PLANNED, 066 /** 067 * The request has been placed. 068 */ 069 REQUESTED, 070 /** 071 * The request is 'actionable', but not all actions that are implied by it have 072 * occurred yet. 073 */ 074 ACTIVE, 075 /** 076 * Actions implied by the request have been temporarily halted, but are expected 077 * to continue later. May also be called "suspended". 078 */ 079 ONHOLD, 080 /** 081 * All actions that are implied by the order have occurred and no continuation 082 * is planned (this will rarely be made explicit). 083 */ 084 COMPLETED, 085 /** 086 * The request has been withdrawn and is no longer actionable. 087 */ 088 CANCELLED, 089 /** 090 * added to help the parsers 091 */ 092 NULL; 093 094 public static NutritionOrderStatus fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("proposed".equals(codeString)) 098 return PROPOSED; 099 if ("draft".equals(codeString)) 100 return DRAFT; 101 if ("planned".equals(codeString)) 102 return PLANNED; 103 if ("requested".equals(codeString)) 104 return REQUESTED; 105 if ("active".equals(codeString)) 106 return ACTIVE; 107 if ("on-hold".equals(codeString)) 108 return ONHOLD; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("cancelled".equals(codeString)) 112 return CANCELLED; 113 throw new FHIRException("Unknown NutritionOrderStatus code '" + codeString + "'"); 114 } 115 116 public String toCode() { 117 switch (this) { 118 case PROPOSED: 119 return "proposed"; 120 case DRAFT: 121 return "draft"; 122 case PLANNED: 123 return "planned"; 124 case REQUESTED: 125 return "requested"; 126 case ACTIVE: 127 return "active"; 128 case ONHOLD: 129 return "on-hold"; 130 case COMPLETED: 131 return "completed"; 132 case CANCELLED: 133 return "cancelled"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getSystem() { 142 switch (this) { 143 case PROPOSED: 144 return "http://hl7.org/fhir/nutrition-order-status"; 145 case DRAFT: 146 return "http://hl7.org/fhir/nutrition-order-status"; 147 case PLANNED: 148 return "http://hl7.org/fhir/nutrition-order-status"; 149 case REQUESTED: 150 return "http://hl7.org/fhir/nutrition-order-status"; 151 case ACTIVE: 152 return "http://hl7.org/fhir/nutrition-order-status"; 153 case ONHOLD: 154 return "http://hl7.org/fhir/nutrition-order-status"; 155 case COMPLETED: 156 return "http://hl7.org/fhir/nutrition-order-status"; 157 case CANCELLED: 158 return "http://hl7.org/fhir/nutrition-order-status"; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 166 public String getDefinition() { 167 switch (this) { 168 case PROPOSED: 169 return "The request has been proposed."; 170 case DRAFT: 171 return "The request is in preliminary form prior to being sent."; 172 case PLANNED: 173 return "The request has been planned."; 174 case REQUESTED: 175 return "The request has been placed."; 176 case ACTIVE: 177 return "The request is 'actionable', but not all actions that are implied by it have occurred yet."; 178 case ONHOLD: 179 return "Actions implied by the request have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 180 case COMPLETED: 181 return "All actions that are implied by the order have occurred and no continuation is planned (this will rarely be made explicit)."; 182 case CANCELLED: 183 return "The request has been withdrawn and is no longer actionable."; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getDisplay() { 192 switch (this) { 193 case PROPOSED: 194 return "Proposed"; 195 case DRAFT: 196 return "Draft"; 197 case PLANNED: 198 return "Planned"; 199 case REQUESTED: 200 return "Requested"; 201 case ACTIVE: 202 return "Active"; 203 case ONHOLD: 204 return "On-Hold"; 205 case COMPLETED: 206 return "Completed"; 207 case CANCELLED: 208 return "Cancelled"; 209 case NULL: 210 return null; 211 default: 212 return "?"; 213 } 214 } 215 } 216 217 public static class NutritionOrderStatusEnumFactory implements EnumFactory<NutritionOrderStatus> { 218 public NutritionOrderStatus fromCode(String codeString) throws IllegalArgumentException { 219 if (codeString == null || "".equals(codeString)) 220 if (codeString == null || "".equals(codeString)) 221 return null; 222 if ("proposed".equals(codeString)) 223 return NutritionOrderStatus.PROPOSED; 224 if ("draft".equals(codeString)) 225 return NutritionOrderStatus.DRAFT; 226 if ("planned".equals(codeString)) 227 return NutritionOrderStatus.PLANNED; 228 if ("requested".equals(codeString)) 229 return NutritionOrderStatus.REQUESTED; 230 if ("active".equals(codeString)) 231 return NutritionOrderStatus.ACTIVE; 232 if ("on-hold".equals(codeString)) 233 return NutritionOrderStatus.ONHOLD; 234 if ("completed".equals(codeString)) 235 return NutritionOrderStatus.COMPLETED; 236 if ("cancelled".equals(codeString)) 237 return NutritionOrderStatus.CANCELLED; 238 throw new IllegalArgumentException("Unknown NutritionOrderStatus code '" + codeString + "'"); 239 } 240 241 public Enumeration<NutritionOrderStatus> fromType(Base code) throws FHIRException { 242 if (code == null || code.isEmpty()) 243 return null; 244 String codeString = ((PrimitiveType) code).asStringValue(); 245 if (codeString == null || "".equals(codeString)) 246 return null; 247 if ("proposed".equals(codeString)) 248 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.PROPOSED); 249 if ("draft".equals(codeString)) 250 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.DRAFT); 251 if ("planned".equals(codeString)) 252 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.PLANNED); 253 if ("requested".equals(codeString)) 254 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.REQUESTED); 255 if ("active".equals(codeString)) 256 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ACTIVE); 257 if ("on-hold".equals(codeString)) 258 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ONHOLD); 259 if ("completed".equals(codeString)) 260 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.COMPLETED); 261 if ("cancelled".equals(codeString)) 262 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.CANCELLED); 263 throw new FHIRException("Unknown NutritionOrderStatus code '" + codeString + "'"); 264 } 265 266 public String toCode(NutritionOrderStatus code) 267 { 268 if (code == NutritionOrderStatus.NULL) 269 return null; 270 if (code == NutritionOrderStatus.PROPOSED) 271 return "proposed"; 272 if (code == NutritionOrderStatus.DRAFT) 273 return "draft"; 274 if (code == NutritionOrderStatus.PLANNED) 275 return "planned"; 276 if (code == NutritionOrderStatus.REQUESTED) 277 return "requested"; 278 if (code == NutritionOrderStatus.ACTIVE) 279 return "active"; 280 if (code == NutritionOrderStatus.ONHOLD) 281 return "on-hold"; 282 if (code == NutritionOrderStatus.COMPLETED) 283 return "completed"; 284 if (code == NutritionOrderStatus.CANCELLED) 285 return "cancelled"; 286 return "?"; 287 } 288 } 289 290 @Block() 291 public static class NutritionOrderOralDietComponent extends BackboneElement implements IBaseBackboneElement { 292 /** 293 * The kind of diet or dietary restriction such as fiber restricted diet or 294 * diabetic diet. 295 */ 296 @Child(name = "type", type = { 297 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 298 @Description(shortDefinition = "Type of oral diet or diet restrictions that describe what can be consumed orally", formalDefinition = "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.") 299 protected List<CodeableConcept> type; 300 301 /** 302 * The time period and frequency at which the diet should be given. 303 */ 304 @Child(name = "schedule", type = { 305 Timing.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 306 @Description(shortDefinition = "Scheduled frequency of diet", formalDefinition = "The time period and frequency at which the diet should be given.") 307 protected List<Timing> schedule; 308 309 /** 310 * Class that defines the quantity and type of nutrient modifications required 311 * for the oral diet. 312 */ 313 @Child(name = "nutrient", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 314 @Description(shortDefinition = "Required nutrient modifications", formalDefinition = "Class that defines the quantity and type of nutrient modifications required for the oral diet.") 315 protected List<NutritionOrderOralDietNutrientComponent> nutrient; 316 317 /** 318 * Class that describes any texture modifications required for the patient to 319 * safely consume various types of solid foods. 320 */ 321 @Child(name = "texture", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 322 @Description(shortDefinition = "Required texture modifications", formalDefinition = "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.") 323 protected List<NutritionOrderOralDietTextureComponent> texture; 324 325 /** 326 * The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) 327 * of liquids or fluids served to the patient. 328 */ 329 @Child(name = "fluidConsistencyType", type = { 330 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 331 @Description(shortDefinition = "The required consistency of fluids and liquids provided to the patient", formalDefinition = "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.") 332 protected List<CodeableConcept> fluidConsistencyType; 333 334 /** 335 * Free text or additional instructions or information pertaining to the oral 336 * diet. 337 */ 338 @Child(name = "instruction", type = { 339 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 340 @Description(shortDefinition = "Instructions or additional information about the oral diet", formalDefinition = "Free text or additional instructions or information pertaining to the oral diet.") 341 protected StringType instruction; 342 343 private static final long serialVersionUID = 973058412L; 344 345 /* 346 * Constructor 347 */ 348 public NutritionOrderOralDietComponent() { 349 super(); 350 } 351 352 /** 353 * @return {@link #type} (The kind of diet or dietary restriction such as fiber 354 * restricted diet or diabetic diet.) 355 */ 356 public List<CodeableConcept> getType() { 357 if (this.type == null) 358 this.type = new ArrayList<CodeableConcept>(); 359 return this.type; 360 } 361 362 public boolean hasType() { 363 if (this.type == null) 364 return false; 365 for (CodeableConcept item : this.type) 366 if (!item.isEmpty()) 367 return true; 368 return false; 369 } 370 371 /** 372 * @return {@link #type} (The kind of diet or dietary restriction such as fiber 373 * restricted diet or diabetic diet.) 374 */ 375 // syntactic sugar 376 public CodeableConcept addType() { // 3 377 CodeableConcept t = new CodeableConcept(); 378 if (this.type == null) 379 this.type = new ArrayList<CodeableConcept>(); 380 this.type.add(t); 381 return t; 382 } 383 384 // syntactic sugar 385 public NutritionOrderOralDietComponent addType(CodeableConcept t) { // 3 386 if (t == null) 387 return this; 388 if (this.type == null) 389 this.type = new ArrayList<CodeableConcept>(); 390 this.type.add(t); 391 return this; 392 } 393 394 /** 395 * @return {@link #schedule} (The time period and frequency at which the diet 396 * should be given.) 397 */ 398 public List<Timing> getSchedule() { 399 if (this.schedule == null) 400 this.schedule = new ArrayList<Timing>(); 401 return this.schedule; 402 } 403 404 public boolean hasSchedule() { 405 if (this.schedule == null) 406 return false; 407 for (Timing item : this.schedule) 408 if (!item.isEmpty()) 409 return true; 410 return false; 411 } 412 413 /** 414 * @return {@link #schedule} (The time period and frequency at which the diet 415 * should be given.) 416 */ 417 // syntactic sugar 418 public Timing addSchedule() { // 3 419 Timing t = new Timing(); 420 if (this.schedule == null) 421 this.schedule = new ArrayList<Timing>(); 422 this.schedule.add(t); 423 return t; 424 } 425 426 // syntactic sugar 427 public NutritionOrderOralDietComponent addSchedule(Timing t) { // 3 428 if (t == null) 429 return this; 430 if (this.schedule == null) 431 this.schedule = new ArrayList<Timing>(); 432 this.schedule.add(t); 433 return this; 434 } 435 436 /** 437 * @return {@link #nutrient} (Class that defines the quantity and type of 438 * nutrient modifications required for the oral diet.) 439 */ 440 public List<NutritionOrderOralDietNutrientComponent> getNutrient() { 441 if (this.nutrient == null) 442 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 443 return this.nutrient; 444 } 445 446 public boolean hasNutrient() { 447 if (this.nutrient == null) 448 return false; 449 for (NutritionOrderOralDietNutrientComponent item : this.nutrient) 450 if (!item.isEmpty()) 451 return true; 452 return false; 453 } 454 455 /** 456 * @return {@link #nutrient} (Class that defines the quantity and type of 457 * nutrient modifications required for the oral diet.) 458 */ 459 // syntactic sugar 460 public NutritionOrderOralDietNutrientComponent addNutrient() { // 3 461 NutritionOrderOralDietNutrientComponent t = new NutritionOrderOralDietNutrientComponent(); 462 if (this.nutrient == null) 463 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 464 this.nutrient.add(t); 465 return t; 466 } 467 468 // syntactic sugar 469 public NutritionOrderOralDietComponent addNutrient(NutritionOrderOralDietNutrientComponent t) { // 3 470 if (t == null) 471 return this; 472 if (this.nutrient == null) 473 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 474 this.nutrient.add(t); 475 return this; 476 } 477 478 /** 479 * @return {@link #texture} (Class that describes any texture modifications 480 * required for the patient to safely consume various types of solid 481 * foods.) 482 */ 483 public List<NutritionOrderOralDietTextureComponent> getTexture() { 484 if (this.texture == null) 485 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 486 return this.texture; 487 } 488 489 public boolean hasTexture() { 490 if (this.texture == null) 491 return false; 492 for (NutritionOrderOralDietTextureComponent item : this.texture) 493 if (!item.isEmpty()) 494 return true; 495 return false; 496 } 497 498 /** 499 * @return {@link #texture} (Class that describes any texture modifications 500 * required for the patient to safely consume various types of solid 501 * foods.) 502 */ 503 // syntactic sugar 504 public NutritionOrderOralDietTextureComponent addTexture() { // 3 505 NutritionOrderOralDietTextureComponent t = new NutritionOrderOralDietTextureComponent(); 506 if (this.texture == null) 507 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 508 this.texture.add(t); 509 return t; 510 } 511 512 // syntactic sugar 513 public NutritionOrderOralDietComponent addTexture(NutritionOrderOralDietTextureComponent t) { // 3 514 if (t == null) 515 return this; 516 if (this.texture == null) 517 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 518 this.texture.add(t); 519 return this; 520 } 521 522 /** 523 * @return {@link #fluidConsistencyType} (The required consistency (e.g. 524 * honey-thick, nectar-thick, thin, thickened.) of liquids or fluids 525 * served to the patient.) 526 */ 527 public List<CodeableConcept> getFluidConsistencyType() { 528 if (this.fluidConsistencyType == null) 529 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 530 return this.fluidConsistencyType; 531 } 532 533 public boolean hasFluidConsistencyType() { 534 if (this.fluidConsistencyType == null) 535 return false; 536 for (CodeableConcept item : this.fluidConsistencyType) 537 if (!item.isEmpty()) 538 return true; 539 return false; 540 } 541 542 /** 543 * @return {@link #fluidConsistencyType} (The required consistency (e.g. 544 * honey-thick, nectar-thick, thin, thickened.) of liquids or fluids 545 * served to the patient.) 546 */ 547 // syntactic sugar 548 public CodeableConcept addFluidConsistencyType() { // 3 549 CodeableConcept t = new CodeableConcept(); 550 if (this.fluidConsistencyType == null) 551 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 552 this.fluidConsistencyType.add(t); 553 return t; 554 } 555 556 // syntactic sugar 557 public NutritionOrderOralDietComponent addFluidConsistencyType(CodeableConcept t) { // 3 558 if (t == null) 559 return this; 560 if (this.fluidConsistencyType == null) 561 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 562 this.fluidConsistencyType.add(t); 563 return this; 564 } 565 566 /** 567 * @return {@link #instruction} (Free text or additional instructions or 568 * information pertaining to the oral diet.). This is the underlying 569 * object with id, value and extensions. The accessor "getInstruction" 570 * gives direct access to the value 571 */ 572 public StringType getInstructionElement() { 573 if (this.instruction == null) 574 if (Configuration.errorOnAutoCreate()) 575 throw new Error("Attempt to auto-create NutritionOrderOralDietComponent.instruction"); 576 else if (Configuration.doAutoCreate()) 577 this.instruction = new StringType(); // bb 578 return this.instruction; 579 } 580 581 public boolean hasInstructionElement() { 582 return this.instruction != null && !this.instruction.isEmpty(); 583 } 584 585 public boolean hasInstruction() { 586 return this.instruction != null && !this.instruction.isEmpty(); 587 } 588 589 /** 590 * @param value {@link #instruction} (Free text or additional instructions or 591 * information pertaining to the oral diet.). This is the 592 * underlying object with id, value and extensions. The accessor 593 * "getInstruction" gives direct access to the value 594 */ 595 public NutritionOrderOralDietComponent setInstructionElement(StringType value) { 596 this.instruction = value; 597 return this; 598 } 599 600 /** 601 * @return Free text or additional instructions or information pertaining to the 602 * oral diet. 603 */ 604 public String getInstruction() { 605 return this.instruction == null ? null : this.instruction.getValue(); 606 } 607 608 /** 609 * @param value Free text or additional instructions or information pertaining 610 * to the oral diet. 611 */ 612 public NutritionOrderOralDietComponent setInstruction(String value) { 613 if (Utilities.noString(value)) 614 this.instruction = null; 615 else { 616 if (this.instruction == null) 617 this.instruction = new StringType(); 618 this.instruction.setValue(value); 619 } 620 return this; 621 } 622 623 protected void listChildren(List<Property> childrenList) { 624 super.listChildren(childrenList); 625 childrenList.add(new Property("type", "CodeableConcept", 626 "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, 627 java.lang.Integer.MAX_VALUE, type)); 628 childrenList 629 .add(new Property("schedule", "Timing", "The time period and frequency at which the diet should be given.", 0, 630 java.lang.Integer.MAX_VALUE, schedule)); 631 childrenList.add(new Property("nutrient", "", 632 "Class that defines the quantity and type of nutrient modifications required for the oral diet.", 0, 633 java.lang.Integer.MAX_VALUE, nutrient)); 634 childrenList.add(new Property("texture", "", 635 "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 636 0, java.lang.Integer.MAX_VALUE, texture)); 637 childrenList.add(new Property("fluidConsistencyType", "CodeableConcept", 638 "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 639 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType)); 640 childrenList.add(new Property("instruction", "string", 641 "Free text or additional instructions or information pertaining to the oral diet.", 0, 642 java.lang.Integer.MAX_VALUE, instruction)); 643 } 644 645 @Override 646 public void setProperty(String name, Base value) throws FHIRException { 647 if (name.equals("type")) 648 this.getType().add(castToCodeableConcept(value)); 649 else if (name.equals("schedule")) 650 this.getSchedule().add(castToTiming(value)); 651 else if (name.equals("nutrient")) 652 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); 653 else if (name.equals("texture")) 654 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); 655 else if (name.equals("fluidConsistencyType")) 656 this.getFluidConsistencyType().add(castToCodeableConcept(value)); 657 else if (name.equals("instruction")) 658 this.instruction = castToString(value); // StringType 659 else 660 super.setProperty(name, value); 661 } 662 663 @Override 664 public Base addChild(String name) throws FHIRException { 665 if (name.equals("type")) { 666 return addType(); 667 } else if (name.equals("schedule")) { 668 return addSchedule(); 669 } else if (name.equals("nutrient")) { 670 return addNutrient(); 671 } else if (name.equals("texture")) { 672 return addTexture(); 673 } else if (name.equals("fluidConsistencyType")) { 674 return addFluidConsistencyType(); 675 } else if (name.equals("instruction")) { 676 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 677 } else 678 return super.addChild(name); 679 } 680 681 public NutritionOrderOralDietComponent copy() { 682 NutritionOrderOralDietComponent dst = new NutritionOrderOralDietComponent(); 683 copyValues(dst); 684 if (type != null) { 685 dst.type = new ArrayList<CodeableConcept>(); 686 for (CodeableConcept i : type) 687 dst.type.add(i.copy()); 688 } 689 ; 690 if (schedule != null) { 691 dst.schedule = new ArrayList<Timing>(); 692 for (Timing i : schedule) 693 dst.schedule.add(i.copy()); 694 } 695 ; 696 if (nutrient != null) { 697 dst.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 698 for (NutritionOrderOralDietNutrientComponent i : nutrient) 699 dst.nutrient.add(i.copy()); 700 } 701 ; 702 if (texture != null) { 703 dst.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 704 for (NutritionOrderOralDietTextureComponent i : texture) 705 dst.texture.add(i.copy()); 706 } 707 ; 708 if (fluidConsistencyType != null) { 709 dst.fluidConsistencyType = new ArrayList<CodeableConcept>(); 710 for (CodeableConcept i : fluidConsistencyType) 711 dst.fluidConsistencyType.add(i.copy()); 712 } 713 ; 714 dst.instruction = instruction == null ? null : instruction.copy(); 715 return dst; 716 } 717 718 @Override 719 public boolean equalsDeep(Base other) { 720 if (!super.equalsDeep(other)) 721 return false; 722 if (!(other instanceof NutritionOrderOralDietComponent)) 723 return false; 724 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other; 725 return compareDeep(type, o.type, true) && compareDeep(schedule, o.schedule, true) 726 && compareDeep(nutrient, o.nutrient, true) && compareDeep(texture, o.texture, true) 727 && compareDeep(fluidConsistencyType, o.fluidConsistencyType, true) 728 && compareDeep(instruction, o.instruction, true); 729 } 730 731 @Override 732 public boolean equalsShallow(Base other) { 733 if (!super.equalsShallow(other)) 734 return false; 735 if (!(other instanceof NutritionOrderOralDietComponent)) 736 return false; 737 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other; 738 return compareValues(instruction, o.instruction, true); 739 } 740 741 public boolean isEmpty() { 742 return super.isEmpty() && (type == null || type.isEmpty()) && (schedule == null || schedule.isEmpty()) 743 && (nutrient == null || nutrient.isEmpty()) && (texture == null || texture.isEmpty()) 744 && (fluidConsistencyType == null || fluidConsistencyType.isEmpty()) 745 && (instruction == null || instruction.isEmpty()); 746 } 747 748 public String fhirType() { 749 return "NutritionOrder.oralDiet"; 750 751 } 752 753 } 754 755 @Block() 756 public static class NutritionOrderOralDietNutrientComponent extends BackboneElement implements IBaseBackboneElement { 757 /** 758 * The nutrient that is being modified such as carbohydrate or sodium. 759 */ 760 @Child(name = "modifier", type = { 761 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 762 @Description(shortDefinition = "Type of nutrient that is being modified", formalDefinition = "The nutrient that is being modified such as carbohydrate or sodium.") 763 protected CodeableConcept modifier; 764 765 /** 766 * The quantity of the specified nutrient to include in diet. 767 */ 768 @Child(name = "amount", type = { 769 SimpleQuantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 770 @Description(shortDefinition = "Quantity of the specified nutrient", formalDefinition = "The quantity of the specified nutrient to include in diet.") 771 protected SimpleQuantity amount; 772 773 private static final long serialVersionUID = 465107295L; 774 775 /* 776 * Constructor 777 */ 778 public NutritionOrderOralDietNutrientComponent() { 779 super(); 780 } 781 782 /** 783 * @return {@link #modifier} (The nutrient that is being modified such as 784 * carbohydrate or sodium.) 785 */ 786 public CodeableConcept getModifier() { 787 if (this.modifier == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.modifier"); 790 else if (Configuration.doAutoCreate()) 791 this.modifier = new CodeableConcept(); // cc 792 return this.modifier; 793 } 794 795 public boolean hasModifier() { 796 return this.modifier != null && !this.modifier.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #modifier} (The nutrient that is being modified such as 801 * carbohydrate or sodium.) 802 */ 803 public NutritionOrderOralDietNutrientComponent setModifier(CodeableConcept value) { 804 this.modifier = value; 805 return this; 806 } 807 808 /** 809 * @return {@link #amount} (The quantity of the specified nutrient to include in 810 * diet.) 811 */ 812 public SimpleQuantity getAmount() { 813 if (this.amount == null) 814 if (Configuration.errorOnAutoCreate()) 815 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.amount"); 816 else if (Configuration.doAutoCreate()) 817 this.amount = new SimpleQuantity(); // cc 818 return this.amount; 819 } 820 821 public boolean hasAmount() { 822 return this.amount != null && !this.amount.isEmpty(); 823 } 824 825 /** 826 * @param value {@link #amount} (The quantity of the specified nutrient to 827 * include in diet.) 828 */ 829 public NutritionOrderOralDietNutrientComponent setAmount(SimpleQuantity value) { 830 this.amount = value; 831 return this; 832 } 833 834 protected void listChildren(List<Property> childrenList) { 835 super.listChildren(childrenList); 836 childrenList.add(new Property("modifier", "CodeableConcept", 837 "The nutrient that is being modified such as carbohydrate or sodium.", 0, java.lang.Integer.MAX_VALUE, 838 modifier)); 839 childrenList.add(new Property("amount", "SimpleQuantity", 840 "The quantity of the specified nutrient to include in diet.", 0, java.lang.Integer.MAX_VALUE, amount)); 841 } 842 843 @Override 844 public void setProperty(String name, Base value) throws FHIRException { 845 if (name.equals("modifier")) 846 this.modifier = castToCodeableConcept(value); // CodeableConcept 847 else if (name.equals("amount")) 848 this.amount = castToSimpleQuantity(value); // SimpleQuantity 849 else 850 super.setProperty(name, value); 851 } 852 853 @Override 854 public Base addChild(String name) throws FHIRException { 855 if (name.equals("modifier")) { 856 this.modifier = new CodeableConcept(); 857 return this.modifier; 858 } else if (name.equals("amount")) { 859 this.amount = new SimpleQuantity(); 860 return this.amount; 861 } else 862 return super.addChild(name); 863 } 864 865 public NutritionOrderOralDietNutrientComponent copy() { 866 NutritionOrderOralDietNutrientComponent dst = new NutritionOrderOralDietNutrientComponent(); 867 copyValues(dst); 868 dst.modifier = modifier == null ? null : modifier.copy(); 869 dst.amount = amount == null ? null : amount.copy(); 870 return dst; 871 } 872 873 @Override 874 public boolean equalsDeep(Base other) { 875 if (!super.equalsDeep(other)) 876 return false; 877 if (!(other instanceof NutritionOrderOralDietNutrientComponent)) 878 return false; 879 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other; 880 return compareDeep(modifier, o.modifier, true) && compareDeep(amount, o.amount, true); 881 } 882 883 @Override 884 public boolean equalsShallow(Base other) { 885 if (!super.equalsShallow(other)) 886 return false; 887 if (!(other instanceof NutritionOrderOralDietNutrientComponent)) 888 return false; 889 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other; 890 return true; 891 } 892 893 public boolean isEmpty() { 894 return super.isEmpty() && (modifier == null || modifier.isEmpty()) && (amount == null || amount.isEmpty()); 895 } 896 897 public String fhirType() { 898 return "NutritionOrder.oralDiet.nutrient"; 899 900 } 901 902 } 903 904 @Block() 905 public static class NutritionOrderOralDietTextureComponent extends BackboneElement implements IBaseBackboneElement { 906 /** 907 * Any texture modifications (for solid foods) that should be made, e.g. easy to 908 * chew, chopped, ground, and pureed. 909 */ 910 @Child(name = "modifier", type = { 911 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 912 @Description(shortDefinition = "Code to indicate how to alter the texture of the foods, e.g. pureed", formalDefinition = "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.") 913 protected CodeableConcept modifier; 914 915 /** 916 * The food type(s) (e.g. meats, all foods) that the texture modification 917 * applies to. This could be all foods types. 918 */ 919 @Child(name = "foodType", type = { 920 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 921 @Description(shortDefinition = "Concepts that are used to identify an entity that is ingested for nutritional purposes", formalDefinition = "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.") 922 protected CodeableConcept foodType; 923 924 private static final long serialVersionUID = -56402817L; 925 926 /* 927 * Constructor 928 */ 929 public NutritionOrderOralDietTextureComponent() { 930 super(); 931 } 932 933 /** 934 * @return {@link #modifier} (Any texture modifications (for solid foods) that 935 * should be made, e.g. easy to chew, chopped, ground, and pureed.) 936 */ 937 public CodeableConcept getModifier() { 938 if (this.modifier == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.modifier"); 941 else if (Configuration.doAutoCreate()) 942 this.modifier = new CodeableConcept(); // cc 943 return this.modifier; 944 } 945 946 public boolean hasModifier() { 947 return this.modifier != null && !this.modifier.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #modifier} (Any texture modifications (for solid foods) 952 * that should be made, e.g. easy to chew, chopped, ground, and 953 * pureed.) 954 */ 955 public NutritionOrderOralDietTextureComponent setModifier(CodeableConcept value) { 956 this.modifier = value; 957 return this; 958 } 959 960 /** 961 * @return {@link #foodType} (The food type(s) (e.g. meats, all foods) that the 962 * texture modification applies to. This could be all foods types.) 963 */ 964 public CodeableConcept getFoodType() { 965 if (this.foodType == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.foodType"); 968 else if (Configuration.doAutoCreate()) 969 this.foodType = new CodeableConcept(); // cc 970 return this.foodType; 971 } 972 973 public boolean hasFoodType() { 974 return this.foodType != null && !this.foodType.isEmpty(); 975 } 976 977 /** 978 * @param value {@link #foodType} (The food type(s) (e.g. meats, all foods) that 979 * the texture modification applies to. This could be all foods 980 * types.) 981 */ 982 public NutritionOrderOralDietTextureComponent setFoodType(CodeableConcept value) { 983 this.foodType = value; 984 return this; 985 } 986 987 protected void listChildren(List<Property> childrenList) { 988 super.listChildren(childrenList); 989 childrenList.add(new Property("modifier", "CodeableConcept", 990 "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 991 0, java.lang.Integer.MAX_VALUE, modifier)); 992 childrenList.add(new Property("foodType", "CodeableConcept", 993 "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 994 0, java.lang.Integer.MAX_VALUE, foodType)); 995 } 996 997 @Override 998 public void setProperty(String name, Base value) throws FHIRException { 999 if (name.equals("modifier")) 1000 this.modifier = castToCodeableConcept(value); // CodeableConcept 1001 else if (name.equals("foodType")) 1002 this.foodType = castToCodeableConcept(value); // CodeableConcept 1003 else 1004 super.setProperty(name, value); 1005 } 1006 1007 @Override 1008 public Base addChild(String name) throws FHIRException { 1009 if (name.equals("modifier")) { 1010 this.modifier = new CodeableConcept(); 1011 return this.modifier; 1012 } else if (name.equals("foodType")) { 1013 this.foodType = new CodeableConcept(); 1014 return this.foodType; 1015 } else 1016 return super.addChild(name); 1017 } 1018 1019 public NutritionOrderOralDietTextureComponent copy() { 1020 NutritionOrderOralDietTextureComponent dst = new NutritionOrderOralDietTextureComponent(); 1021 copyValues(dst); 1022 dst.modifier = modifier == null ? null : modifier.copy(); 1023 dst.foodType = foodType == null ? null : foodType.copy(); 1024 return dst; 1025 } 1026 1027 @Override 1028 public boolean equalsDeep(Base other) { 1029 if (!super.equalsDeep(other)) 1030 return false; 1031 if (!(other instanceof NutritionOrderOralDietTextureComponent)) 1032 return false; 1033 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other; 1034 return compareDeep(modifier, o.modifier, true) && compareDeep(foodType, o.foodType, true); 1035 } 1036 1037 @Override 1038 public boolean equalsShallow(Base other) { 1039 if (!super.equalsShallow(other)) 1040 return false; 1041 if (!(other instanceof NutritionOrderOralDietTextureComponent)) 1042 return false; 1043 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other; 1044 return true; 1045 } 1046 1047 public boolean isEmpty() { 1048 return super.isEmpty() && (modifier == null || modifier.isEmpty()) && (foodType == null || foodType.isEmpty()); 1049 } 1050 1051 public String fhirType() { 1052 return "NutritionOrder.oralDiet.texture"; 1053 1054 } 1055 1056 } 1057 1058 @Block() 1059 public static class NutritionOrderSupplementComponent extends BackboneElement implements IBaseBackboneElement { 1060 /** 1061 * The kind of nutritional supplement product required such as a high protein or 1062 * pediatric clear liquid supplement. 1063 */ 1064 @Child(name = "type", type = { 1065 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1066 @Description(shortDefinition = "Type of supplement product requested", formalDefinition = "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.") 1067 protected CodeableConcept type; 1068 1069 /** 1070 * The product or brand name of the nutritional supplement such as "Acme Protein 1071 * Shake". 1072 */ 1073 @Child(name = "productName", type = { 1074 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1075 @Description(shortDefinition = "Product or brand name of the nutritional supplement", formalDefinition = "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".") 1076 protected StringType productName; 1077 1078 /** 1079 * The time period and frequency at which the supplement(s) should be given. 1080 */ 1081 @Child(name = "schedule", type = { 1082 Timing.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1083 @Description(shortDefinition = "Scheduled frequency of supplement", formalDefinition = "The time period and frequency at which the supplement(s) should be given.") 1084 protected List<Timing> schedule; 1085 1086 /** 1087 * The amount of the nutritional supplement to be given. 1088 */ 1089 @Child(name = "quantity", type = { 1090 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1091 @Description(shortDefinition = "Amount of the nutritional supplement", formalDefinition = "The amount of the nutritional supplement to be given.") 1092 protected SimpleQuantity quantity; 1093 1094 /** 1095 * Free text or additional instructions or information pertaining to the oral 1096 * supplement. 1097 */ 1098 @Child(name = "instruction", type = { 1099 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1100 @Description(shortDefinition = "Instructions or additional information about the oral supplement", formalDefinition = "Free text or additional instructions or information pertaining to the oral supplement.") 1101 protected StringType instruction; 1102 1103 private static final long serialVersionUID = 297545236L; 1104 1105 /* 1106 * Constructor 1107 */ 1108 public NutritionOrderSupplementComponent() { 1109 super(); 1110 } 1111 1112 /** 1113 * @return {@link #type} (The kind of nutritional supplement product required 1114 * such as a high protein or pediatric clear liquid supplement.) 1115 */ 1116 public CodeableConcept getType() { 1117 if (this.type == null) 1118 if (Configuration.errorOnAutoCreate()) 1119 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.type"); 1120 else if (Configuration.doAutoCreate()) 1121 this.type = new CodeableConcept(); // cc 1122 return this.type; 1123 } 1124 1125 public boolean hasType() { 1126 return this.type != null && !this.type.isEmpty(); 1127 } 1128 1129 /** 1130 * @param value {@link #type} (The kind of nutritional supplement product 1131 * required such as a high protein or pediatric clear liquid 1132 * supplement.) 1133 */ 1134 public NutritionOrderSupplementComponent setType(CodeableConcept value) { 1135 this.type = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #productName} (The product or brand name of the nutritional 1141 * supplement such as "Acme Protein Shake".). This is the underlying 1142 * object with id, value and extensions. The accessor "getProductName" 1143 * gives direct access to the value 1144 */ 1145 public StringType getProductNameElement() { 1146 if (this.productName == null) 1147 if (Configuration.errorOnAutoCreate()) 1148 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.productName"); 1149 else if (Configuration.doAutoCreate()) 1150 this.productName = new StringType(); // bb 1151 return this.productName; 1152 } 1153 1154 public boolean hasProductNameElement() { 1155 return this.productName != null && !this.productName.isEmpty(); 1156 } 1157 1158 public boolean hasProductName() { 1159 return this.productName != null && !this.productName.isEmpty(); 1160 } 1161 1162 /** 1163 * @param value {@link #productName} (The product or brand name of the 1164 * nutritional supplement such as "Acme Protein Shake".). This is 1165 * the underlying object with id, value and extensions. The 1166 * accessor "getProductName" gives direct access to the value 1167 */ 1168 public NutritionOrderSupplementComponent setProductNameElement(StringType value) { 1169 this.productName = value; 1170 return this; 1171 } 1172 1173 /** 1174 * @return The product or brand name of the nutritional supplement such as "Acme 1175 * Protein Shake". 1176 */ 1177 public String getProductName() { 1178 return this.productName == null ? null : this.productName.getValue(); 1179 } 1180 1181 /** 1182 * @param value The product or brand name of the nutritional supplement such as 1183 * "Acme Protein Shake". 1184 */ 1185 public NutritionOrderSupplementComponent setProductName(String value) { 1186 if (Utilities.noString(value)) 1187 this.productName = null; 1188 else { 1189 if (this.productName == null) 1190 this.productName = new StringType(); 1191 this.productName.setValue(value); 1192 } 1193 return this; 1194 } 1195 1196 /** 1197 * @return {@link #schedule} (The time period and frequency at which the 1198 * supplement(s) should be given.) 1199 */ 1200 public List<Timing> getSchedule() { 1201 if (this.schedule == null) 1202 this.schedule = new ArrayList<Timing>(); 1203 return this.schedule; 1204 } 1205 1206 public boolean hasSchedule() { 1207 if (this.schedule == null) 1208 return false; 1209 for (Timing item : this.schedule) 1210 if (!item.isEmpty()) 1211 return true; 1212 return false; 1213 } 1214 1215 /** 1216 * @return {@link #schedule} (The time period and frequency at which the 1217 * supplement(s) should be given.) 1218 */ 1219 // syntactic sugar 1220 public Timing addSchedule() { // 3 1221 Timing t = new Timing(); 1222 if (this.schedule == null) 1223 this.schedule = new ArrayList<Timing>(); 1224 this.schedule.add(t); 1225 return t; 1226 } 1227 1228 // syntactic sugar 1229 public NutritionOrderSupplementComponent addSchedule(Timing t) { // 3 1230 if (t == null) 1231 return this; 1232 if (this.schedule == null) 1233 this.schedule = new ArrayList<Timing>(); 1234 this.schedule.add(t); 1235 return this; 1236 } 1237 1238 /** 1239 * @return {@link #quantity} (The amount of the nutritional supplement to be 1240 * given.) 1241 */ 1242 public SimpleQuantity getQuantity() { 1243 if (this.quantity == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.quantity"); 1246 else if (Configuration.doAutoCreate()) 1247 this.quantity = new SimpleQuantity(); // cc 1248 return this.quantity; 1249 } 1250 1251 public boolean hasQuantity() { 1252 return this.quantity != null && !this.quantity.isEmpty(); 1253 } 1254 1255 /** 1256 * @param value {@link #quantity} (The amount of the nutritional supplement to 1257 * be given.) 1258 */ 1259 public NutritionOrderSupplementComponent setQuantity(SimpleQuantity value) { 1260 this.quantity = value; 1261 return this; 1262 } 1263 1264 /** 1265 * @return {@link #instruction} (Free text or additional instructions or 1266 * information pertaining to the oral supplement.). This is the 1267 * underlying object with id, value and extensions. The accessor 1268 * "getInstruction" gives direct access to the value 1269 */ 1270 public StringType getInstructionElement() { 1271 if (this.instruction == null) 1272 if (Configuration.errorOnAutoCreate()) 1273 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.instruction"); 1274 else if (Configuration.doAutoCreate()) 1275 this.instruction = new StringType(); // bb 1276 return this.instruction; 1277 } 1278 1279 public boolean hasInstructionElement() { 1280 return this.instruction != null && !this.instruction.isEmpty(); 1281 } 1282 1283 public boolean hasInstruction() { 1284 return this.instruction != null && !this.instruction.isEmpty(); 1285 } 1286 1287 /** 1288 * @param value {@link #instruction} (Free text or additional instructions or 1289 * information pertaining to the oral supplement.). This is the 1290 * underlying object with id, value and extensions. The accessor 1291 * "getInstruction" gives direct access to the value 1292 */ 1293 public NutritionOrderSupplementComponent setInstructionElement(StringType value) { 1294 this.instruction = value; 1295 return this; 1296 } 1297 1298 /** 1299 * @return Free text or additional instructions or information pertaining to the 1300 * oral supplement. 1301 */ 1302 public String getInstruction() { 1303 return this.instruction == null ? null : this.instruction.getValue(); 1304 } 1305 1306 /** 1307 * @param value Free text or additional instructions or information pertaining 1308 * to the oral supplement. 1309 */ 1310 public NutritionOrderSupplementComponent setInstruction(String value) { 1311 if (Utilities.noString(value)) 1312 this.instruction = null; 1313 else { 1314 if (this.instruction == null) 1315 this.instruction = new StringType(); 1316 this.instruction.setValue(value); 1317 } 1318 return this; 1319 } 1320 1321 protected void listChildren(List<Property> childrenList) { 1322 super.listChildren(childrenList); 1323 childrenList.add(new Property("type", "CodeableConcept", 1324 "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 1325 0, java.lang.Integer.MAX_VALUE, type)); 1326 childrenList.add(new Property("productName", "string", 1327 "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1328 java.lang.Integer.MAX_VALUE, productName)); 1329 childrenList.add(new Property("schedule", "Timing", 1330 "The time period and frequency at which the supplement(s) should be given.", 0, java.lang.Integer.MAX_VALUE, 1331 schedule)); 1332 childrenList.add(new Property("quantity", "SimpleQuantity", 1333 "The amount of the nutritional supplement to be given.", 0, java.lang.Integer.MAX_VALUE, quantity)); 1334 childrenList.add(new Property("instruction", "string", 1335 "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1336 java.lang.Integer.MAX_VALUE, instruction)); 1337 } 1338 1339 @Override 1340 public void setProperty(String name, Base value) throws FHIRException { 1341 if (name.equals("type")) 1342 this.type = castToCodeableConcept(value); // CodeableConcept 1343 else if (name.equals("productName")) 1344 this.productName = castToString(value); // StringType 1345 else if (name.equals("schedule")) 1346 this.getSchedule().add(castToTiming(value)); 1347 else if (name.equals("quantity")) 1348 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1349 else if (name.equals("instruction")) 1350 this.instruction = castToString(value); // StringType 1351 else 1352 super.setProperty(name, value); 1353 } 1354 1355 @Override 1356 public Base addChild(String name) throws FHIRException { 1357 if (name.equals("type")) { 1358 this.type = new CodeableConcept(); 1359 return this.type; 1360 } else if (name.equals("productName")) { 1361 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.productName"); 1362 } else if (name.equals("schedule")) { 1363 return addSchedule(); 1364 } else if (name.equals("quantity")) { 1365 this.quantity = new SimpleQuantity(); 1366 return this.quantity; 1367 } else if (name.equals("instruction")) { 1368 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 1369 } else 1370 return super.addChild(name); 1371 } 1372 1373 public NutritionOrderSupplementComponent copy() { 1374 NutritionOrderSupplementComponent dst = new NutritionOrderSupplementComponent(); 1375 copyValues(dst); 1376 dst.type = type == null ? null : type.copy(); 1377 dst.productName = productName == null ? null : productName.copy(); 1378 if (schedule != null) { 1379 dst.schedule = new ArrayList<Timing>(); 1380 for (Timing i : schedule) 1381 dst.schedule.add(i.copy()); 1382 } 1383 ; 1384 dst.quantity = quantity == null ? null : quantity.copy(); 1385 dst.instruction = instruction == null ? null : instruction.copy(); 1386 return dst; 1387 } 1388 1389 @Override 1390 public boolean equalsDeep(Base other) { 1391 if (!super.equalsDeep(other)) 1392 return false; 1393 if (!(other instanceof NutritionOrderSupplementComponent)) 1394 return false; 1395 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other; 1396 return compareDeep(type, o.type, true) && compareDeep(productName, o.productName, true) 1397 && compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) 1398 && compareDeep(instruction, o.instruction, true); 1399 } 1400 1401 @Override 1402 public boolean equalsShallow(Base other) { 1403 if (!super.equalsShallow(other)) 1404 return false; 1405 if (!(other instanceof NutritionOrderSupplementComponent)) 1406 return false; 1407 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other; 1408 return compareValues(productName, o.productName, true) && compareValues(instruction, o.instruction, true); 1409 } 1410 1411 public boolean isEmpty() { 1412 return super.isEmpty() && (type == null || type.isEmpty()) && (productName == null || productName.isEmpty()) 1413 && (schedule == null || schedule.isEmpty()) && (quantity == null || quantity.isEmpty()) 1414 && (instruction == null || instruction.isEmpty()); 1415 } 1416 1417 public String fhirType() { 1418 return "NutritionOrder.supplement"; 1419 1420 } 1421 1422 } 1423 1424 @Block() 1425 public static class NutritionOrderEnteralFormulaComponent extends BackboneElement implements IBaseBackboneElement { 1426 /** 1427 * The type of enteral or infant formula such as an adult standard formula with 1428 * fiber or a soy-based infant formula. 1429 */ 1430 @Child(name = "baseFormulaType", type = { 1431 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1432 @Description(shortDefinition = "Type of enteral or infant formula", formalDefinition = "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.") 1433 protected CodeableConcept baseFormulaType; 1434 1435 /** 1436 * The product or brand name of the enteral or infant formula product such as 1437 * "ACME Adult Standard Formula". 1438 */ 1439 @Child(name = "baseFormulaProductName", type = { 1440 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1441 @Description(shortDefinition = "Product or brand name of the enteral or infant formula", formalDefinition = "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".") 1442 protected StringType baseFormulaProductName; 1443 1444 /** 1445 * Indicates the type of modular component such as protein, carbohydrate, fat or 1446 * fiber to be provided in addition to or mixed with the base formula. 1447 */ 1448 @Child(name = "additiveType", type = { 1449 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1450 @Description(shortDefinition = "Type of modular component to add to the feeding", formalDefinition = "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.") 1451 protected CodeableConcept additiveType; 1452 1453 /** 1454 * The product or brand name of the type of modular component to be added to the 1455 * formula. 1456 */ 1457 @Child(name = "additiveProductName", type = { 1458 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1459 @Description(shortDefinition = "Product or brand name of the modular additive", formalDefinition = "The product or brand name of the type of modular component to be added to the formula.") 1460 protected StringType additiveProductName; 1461 1462 /** 1463 * The amount of energy (Calories) that the formula should provide per specified 1464 * volume, typically per mL or fluid oz. For example, an infant may require a 1465 * formula that provides 24 Calories per fluid ounce or an adult may require an 1466 * enteral formula that provides 1.5 Calorie/mL. 1467 */ 1468 @Child(name = "caloricDensity", type = { 1469 SimpleQuantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1470 @Description(shortDefinition = "Amount of energy per specified volume that is required", formalDefinition = "The amount of energy (Calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 Calories per fluid ounce or an adult may require an enteral formula that provides 1.5 Calorie/mL.") 1471 protected SimpleQuantity caloricDensity; 1472 1473 /** 1474 * The route or physiological path of administration into the patient's 1475 * gastrointestinal tract for purposes of providing the formula feeding, e.g. 1476 * nasogastric tube. 1477 */ 1478 @Child(name = "routeofAdministration", type = { 1479 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1480 @Description(shortDefinition = "How the formula should enter the patient's gastrointestinal tract", formalDefinition = "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.") 1481 protected CodeableConcept routeofAdministration; 1482 1483 /** 1484 * Formula administration instructions as structured data. This repeating 1485 * structure allows for changing the administration rate or volume over time for 1486 * both bolus and continuous feeding. An example of this would be an instruction 1487 * to increase the rate of continuous feeding every 2 hours. 1488 */ 1489 @Child(name = "administration", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1490 @Description(shortDefinition = "Formula feeding instruction as structured data", formalDefinition = "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.") 1491 protected List<NutritionOrderEnteralFormulaAdministrationComponent> administration; 1492 1493 /** 1494 * The maximum total quantity of formula that may be administered to a subject 1495 * over the period of time, e.g. 1440 mL over 24 hours. 1496 */ 1497 @Child(name = "maxVolumeToDeliver", type = { 1498 SimpleQuantity.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1499 @Description(shortDefinition = "Upper limit on formula volume per unit of time", formalDefinition = "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.") 1500 protected SimpleQuantity maxVolumeToDeliver; 1501 1502 /** 1503 * Free text formula administration, feeding instructions or additional 1504 * instructions or information. 1505 */ 1506 @Child(name = "administrationInstruction", type = { 1507 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1508 @Description(shortDefinition = "Formula feeding instructions expressed as text", formalDefinition = "Free text formula administration, feeding instructions or additional instructions or information.") 1509 protected StringType administrationInstruction; 1510 1511 private static final long serialVersionUID = 292116061L; 1512 1513 /* 1514 * Constructor 1515 */ 1516 public NutritionOrderEnteralFormulaComponent() { 1517 super(); 1518 } 1519 1520 /** 1521 * @return {@link #baseFormulaType} (The type of enteral or infant formula such 1522 * as an adult standard formula with fiber or a soy-based infant 1523 * formula.) 1524 */ 1525 public CodeableConcept getBaseFormulaType() { 1526 if (this.baseFormulaType == null) 1527 if (Configuration.errorOnAutoCreate()) 1528 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaType"); 1529 else if (Configuration.doAutoCreate()) 1530 this.baseFormulaType = new CodeableConcept(); // cc 1531 return this.baseFormulaType; 1532 } 1533 1534 public boolean hasBaseFormulaType() { 1535 return this.baseFormulaType != null && !this.baseFormulaType.isEmpty(); 1536 } 1537 1538 /** 1539 * @param value {@link #baseFormulaType} (The type of enteral or infant formula 1540 * such as an adult standard formula with fiber or a soy-based 1541 * infant formula.) 1542 */ 1543 public NutritionOrderEnteralFormulaComponent setBaseFormulaType(CodeableConcept value) { 1544 this.baseFormulaType = value; 1545 return this; 1546 } 1547 1548 /** 1549 * @return {@link #baseFormulaProductName} (The product or brand name of the 1550 * enteral or infant formula product such as "ACME Adult Standard 1551 * Formula".). This is the underlying object with id, value and 1552 * extensions. The accessor "getBaseFormulaProductName" gives direct 1553 * access to the value 1554 */ 1555 public StringType getBaseFormulaProductNameElement() { 1556 if (this.baseFormulaProductName == null) 1557 if (Configuration.errorOnAutoCreate()) 1558 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaProductName"); 1559 else if (Configuration.doAutoCreate()) 1560 this.baseFormulaProductName = new StringType(); // bb 1561 return this.baseFormulaProductName; 1562 } 1563 1564 public boolean hasBaseFormulaProductNameElement() { 1565 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 1566 } 1567 1568 public boolean hasBaseFormulaProductName() { 1569 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #baseFormulaProductName} (The product or brand name of 1574 * the enteral or infant formula product such as "ACME Adult 1575 * Standard Formula".). This is the underlying object with id, 1576 * value and extensions. The accessor "getBaseFormulaProductName" 1577 * gives direct access to the value 1578 */ 1579 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductNameElement(StringType value) { 1580 this.baseFormulaProductName = value; 1581 return this; 1582 } 1583 1584 /** 1585 * @return The product or brand name of the enteral or infant formula product 1586 * such as "ACME Adult Standard Formula". 1587 */ 1588 public String getBaseFormulaProductName() { 1589 return this.baseFormulaProductName == null ? null : this.baseFormulaProductName.getValue(); 1590 } 1591 1592 /** 1593 * @param value The product or brand name of the enteral or infant formula 1594 * product such as "ACME Adult Standard Formula". 1595 */ 1596 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductName(String value) { 1597 if (Utilities.noString(value)) 1598 this.baseFormulaProductName = null; 1599 else { 1600 if (this.baseFormulaProductName == null) 1601 this.baseFormulaProductName = new StringType(); 1602 this.baseFormulaProductName.setValue(value); 1603 } 1604 return this; 1605 } 1606 1607 /** 1608 * @return {@link #additiveType} (Indicates the type of modular component such 1609 * as protein, carbohydrate, fat or fiber to be provided in addition to 1610 * or mixed with the base formula.) 1611 */ 1612 public CodeableConcept getAdditiveType() { 1613 if (this.additiveType == null) 1614 if (Configuration.errorOnAutoCreate()) 1615 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveType"); 1616 else if (Configuration.doAutoCreate()) 1617 this.additiveType = new CodeableConcept(); // cc 1618 return this.additiveType; 1619 } 1620 1621 public boolean hasAdditiveType() { 1622 return this.additiveType != null && !this.additiveType.isEmpty(); 1623 } 1624 1625 /** 1626 * @param value {@link #additiveType} (Indicates the type of modular component 1627 * such as protein, carbohydrate, fat or fiber to be provided in 1628 * addition to or mixed with the base formula.) 1629 */ 1630 public NutritionOrderEnteralFormulaComponent setAdditiveType(CodeableConcept value) { 1631 this.additiveType = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return {@link #additiveProductName} (The product or brand name of the type 1637 * of modular component to be added to the formula.). This is the 1638 * underlying object with id, value and extensions. The accessor 1639 * "getAdditiveProductName" gives direct access to the value 1640 */ 1641 public StringType getAdditiveProductNameElement() { 1642 if (this.additiveProductName == null) 1643 if (Configuration.errorOnAutoCreate()) 1644 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveProductName"); 1645 else if (Configuration.doAutoCreate()) 1646 this.additiveProductName = new StringType(); // bb 1647 return this.additiveProductName; 1648 } 1649 1650 public boolean hasAdditiveProductNameElement() { 1651 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 1652 } 1653 1654 public boolean hasAdditiveProductName() { 1655 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 1656 } 1657 1658 /** 1659 * @param value {@link #additiveProductName} (The product or brand name of the 1660 * type of modular component to be added to the formula.). This is 1661 * the underlying object with id, value and extensions. The 1662 * accessor "getAdditiveProductName" gives direct access to the 1663 * value 1664 */ 1665 public NutritionOrderEnteralFormulaComponent setAdditiveProductNameElement(StringType value) { 1666 this.additiveProductName = value; 1667 return this; 1668 } 1669 1670 /** 1671 * @return The product or brand name of the type of modular component to be 1672 * added to the formula. 1673 */ 1674 public String getAdditiveProductName() { 1675 return this.additiveProductName == null ? null : this.additiveProductName.getValue(); 1676 } 1677 1678 /** 1679 * @param value The product or brand name of the type of modular component to be 1680 * added to the formula. 1681 */ 1682 public NutritionOrderEnteralFormulaComponent setAdditiveProductName(String value) { 1683 if (Utilities.noString(value)) 1684 this.additiveProductName = null; 1685 else { 1686 if (this.additiveProductName == null) 1687 this.additiveProductName = new StringType(); 1688 this.additiveProductName.setValue(value); 1689 } 1690 return this; 1691 } 1692 1693 /** 1694 * @return {@link #caloricDensity} (The amount of energy (Calories) that the 1695 * formula should provide per specified volume, typically per mL or 1696 * fluid oz. For example, an infant may require a formula that provides 1697 * 24 Calories per fluid ounce or an adult may require an enteral 1698 * formula that provides 1.5 Calorie/mL.) 1699 */ 1700 public SimpleQuantity getCaloricDensity() { 1701 if (this.caloricDensity == null) 1702 if (Configuration.errorOnAutoCreate()) 1703 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.caloricDensity"); 1704 else if (Configuration.doAutoCreate()) 1705 this.caloricDensity = new SimpleQuantity(); // cc 1706 return this.caloricDensity; 1707 } 1708 1709 public boolean hasCaloricDensity() { 1710 return this.caloricDensity != null && !this.caloricDensity.isEmpty(); 1711 } 1712 1713 /** 1714 * @param value {@link #caloricDensity} (The amount of energy (Calories) that 1715 * the formula should provide per specified volume, typically per 1716 * mL or fluid oz. For example, an infant may require a formula 1717 * that provides 24 Calories per fluid ounce or an adult may 1718 * require an enteral formula that provides 1.5 Calorie/mL.) 1719 */ 1720 public NutritionOrderEnteralFormulaComponent setCaloricDensity(SimpleQuantity value) { 1721 this.caloricDensity = value; 1722 return this; 1723 } 1724 1725 /** 1726 * @return {@link #routeofAdministration} (The route or physiological path of 1727 * administration into the patient's gastrointestinal tract for purposes 1728 * of providing the formula feeding, e.g. nasogastric tube.) 1729 */ 1730 public CodeableConcept getRouteofAdministration() { 1731 if (this.routeofAdministration == null) 1732 if (Configuration.errorOnAutoCreate()) 1733 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.routeofAdministration"); 1734 else if (Configuration.doAutoCreate()) 1735 this.routeofAdministration = new CodeableConcept(); // cc 1736 return this.routeofAdministration; 1737 } 1738 1739 public boolean hasRouteofAdministration() { 1740 return this.routeofAdministration != null && !this.routeofAdministration.isEmpty(); 1741 } 1742 1743 /** 1744 * @param value {@link #routeofAdministration} (The route or physiological path 1745 * of administration into the patient's gastrointestinal tract for 1746 * purposes of providing the formula feeding, e.g. nasogastric 1747 * tube.) 1748 */ 1749 public NutritionOrderEnteralFormulaComponent setRouteofAdministration(CodeableConcept value) { 1750 this.routeofAdministration = value; 1751 return this; 1752 } 1753 1754 /** 1755 * @return {@link #administration} (Formula administration instructions as 1756 * structured data. This repeating structure allows for changing the 1757 * administration rate or volume over time for both bolus and continuous 1758 * feeding. An example of this would be an instruction to increase the 1759 * rate of continuous feeding every 2 hours.) 1760 */ 1761 public List<NutritionOrderEnteralFormulaAdministrationComponent> getAdministration() { 1762 if (this.administration == null) 1763 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1764 return this.administration; 1765 } 1766 1767 public boolean hasAdministration() { 1768 if (this.administration == null) 1769 return false; 1770 for (NutritionOrderEnteralFormulaAdministrationComponent item : this.administration) 1771 if (!item.isEmpty()) 1772 return true; 1773 return false; 1774 } 1775 1776 /** 1777 * @return {@link #administration} (Formula administration instructions as 1778 * structured data. This repeating structure allows for changing the 1779 * administration rate or volume over time for both bolus and continuous 1780 * feeding. An example of this would be an instruction to increase the 1781 * rate of continuous feeding every 2 hours.) 1782 */ 1783 // syntactic sugar 1784 public NutritionOrderEnteralFormulaAdministrationComponent addAdministration() { // 3 1785 NutritionOrderEnteralFormulaAdministrationComponent t = new NutritionOrderEnteralFormulaAdministrationComponent(); 1786 if (this.administration == null) 1787 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1788 this.administration.add(t); 1789 return t; 1790 } 1791 1792 // syntactic sugar 1793 public NutritionOrderEnteralFormulaComponent addAdministration( 1794 NutritionOrderEnteralFormulaAdministrationComponent t) { // 3 1795 if (t == null) 1796 return this; 1797 if (this.administration == null) 1798 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1799 this.administration.add(t); 1800 return this; 1801 } 1802 1803 /** 1804 * @return {@link #maxVolumeToDeliver} (The maximum total quantity of formula 1805 * that may be administered to a subject over the period of time, e.g. 1806 * 1440 mL over 24 hours.) 1807 */ 1808 public SimpleQuantity getMaxVolumeToDeliver() { 1809 if (this.maxVolumeToDeliver == null) 1810 if (Configuration.errorOnAutoCreate()) 1811 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.maxVolumeToDeliver"); 1812 else if (Configuration.doAutoCreate()) 1813 this.maxVolumeToDeliver = new SimpleQuantity(); // cc 1814 return this.maxVolumeToDeliver; 1815 } 1816 1817 public boolean hasMaxVolumeToDeliver() { 1818 return this.maxVolumeToDeliver != null && !this.maxVolumeToDeliver.isEmpty(); 1819 } 1820 1821 /** 1822 * @param value {@link #maxVolumeToDeliver} (The maximum total quantity of 1823 * formula that may be administered to a subject over the period of 1824 * time, e.g. 1440 mL over 24 hours.) 1825 */ 1826 public NutritionOrderEnteralFormulaComponent setMaxVolumeToDeliver(SimpleQuantity value) { 1827 this.maxVolumeToDeliver = value; 1828 return this; 1829 } 1830 1831 /** 1832 * @return {@link #administrationInstruction} (Free text formula administration, 1833 * feeding instructions or additional instructions or information.). 1834 * This is the underlying object with id, value and extensions. The 1835 * accessor "getAdministrationInstruction" gives direct access to the 1836 * value 1837 */ 1838 public StringType getAdministrationInstructionElement() { 1839 if (this.administrationInstruction == null) 1840 if (Configuration.errorOnAutoCreate()) 1841 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.administrationInstruction"); 1842 else if (Configuration.doAutoCreate()) 1843 this.administrationInstruction = new StringType(); // bb 1844 return this.administrationInstruction; 1845 } 1846 1847 public boolean hasAdministrationInstructionElement() { 1848 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 1849 } 1850 1851 public boolean hasAdministrationInstruction() { 1852 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 1853 } 1854 1855 /** 1856 * @param value {@link #administrationInstruction} (Free text formula 1857 * administration, feeding instructions or additional instructions 1858 * or information.). This is the underlying object with id, value 1859 * and extensions. The accessor "getAdministrationInstruction" 1860 * gives direct access to the value 1861 */ 1862 public NutritionOrderEnteralFormulaComponent setAdministrationInstructionElement(StringType value) { 1863 this.administrationInstruction = value; 1864 return this; 1865 } 1866 1867 /** 1868 * @return Free text formula administration, feeding instructions or additional 1869 * instructions or information. 1870 */ 1871 public String getAdministrationInstruction() { 1872 return this.administrationInstruction == null ? null : this.administrationInstruction.getValue(); 1873 } 1874 1875 /** 1876 * @param value Free text formula administration, feeding instructions or 1877 * additional instructions or information. 1878 */ 1879 public NutritionOrderEnteralFormulaComponent setAdministrationInstruction(String value) { 1880 if (Utilities.noString(value)) 1881 this.administrationInstruction = null; 1882 else { 1883 if (this.administrationInstruction == null) 1884 this.administrationInstruction = new StringType(); 1885 this.administrationInstruction.setValue(value); 1886 } 1887 return this; 1888 } 1889 1890 protected void listChildren(List<Property> childrenList) { 1891 super.listChildren(childrenList); 1892 childrenList.add(new Property("baseFormulaType", "CodeableConcept", 1893 "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 1894 0, java.lang.Integer.MAX_VALUE, baseFormulaType)); 1895 childrenList.add(new Property("baseFormulaProductName", "string", 1896 "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 1897 0, java.lang.Integer.MAX_VALUE, baseFormulaProductName)); 1898 childrenList.add(new Property("additiveType", "CodeableConcept", 1899 "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 1900 0, java.lang.Integer.MAX_VALUE, additiveType)); 1901 childrenList.add(new Property("additiveProductName", "string", 1902 "The product or brand name of the type of modular component to be added to the formula.", 0, 1903 java.lang.Integer.MAX_VALUE, additiveProductName)); 1904 childrenList.add(new Property("caloricDensity", "SimpleQuantity", 1905 "The amount of energy (Calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 Calories per fluid ounce or an adult may require an enteral formula that provides 1.5 Calorie/mL.", 1906 0, java.lang.Integer.MAX_VALUE, caloricDensity)); 1907 childrenList.add(new Property("routeofAdministration", "CodeableConcept", 1908 "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 1909 0, java.lang.Integer.MAX_VALUE, routeofAdministration)); 1910 childrenList.add(new Property("administration", "", 1911 "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 1912 0, java.lang.Integer.MAX_VALUE, administration)); 1913 childrenList.add(new Property("maxVolumeToDeliver", "SimpleQuantity", 1914 "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 1915 0, java.lang.Integer.MAX_VALUE, maxVolumeToDeliver)); 1916 childrenList.add(new Property("administrationInstruction", "string", 1917 "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1918 java.lang.Integer.MAX_VALUE, administrationInstruction)); 1919 } 1920 1921 @Override 1922 public void setProperty(String name, Base value) throws FHIRException { 1923 if (name.equals("baseFormulaType")) 1924 this.baseFormulaType = castToCodeableConcept(value); // CodeableConcept 1925 else if (name.equals("baseFormulaProductName")) 1926 this.baseFormulaProductName = castToString(value); // StringType 1927 else if (name.equals("additiveType")) 1928 this.additiveType = castToCodeableConcept(value); // CodeableConcept 1929 else if (name.equals("additiveProductName")) 1930 this.additiveProductName = castToString(value); // StringType 1931 else if (name.equals("caloricDensity")) 1932 this.caloricDensity = castToSimpleQuantity(value); // SimpleQuantity 1933 else if (name.equals("routeofAdministration")) 1934 this.routeofAdministration = castToCodeableConcept(value); // CodeableConcept 1935 else if (name.equals("administration")) 1936 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); 1937 else if (name.equals("maxVolumeToDeliver")) 1938 this.maxVolumeToDeliver = castToSimpleQuantity(value); // SimpleQuantity 1939 else if (name.equals("administrationInstruction")) 1940 this.administrationInstruction = castToString(value); // StringType 1941 else 1942 super.setProperty(name, value); 1943 } 1944 1945 @Override 1946 public Base addChild(String name) throws FHIRException { 1947 if (name.equals("baseFormulaType")) { 1948 this.baseFormulaType = new CodeableConcept(); 1949 return this.baseFormulaType; 1950 } else if (name.equals("baseFormulaProductName")) { 1951 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.baseFormulaProductName"); 1952 } else if (name.equals("additiveType")) { 1953 this.additiveType = new CodeableConcept(); 1954 return this.additiveType; 1955 } else if (name.equals("additiveProductName")) { 1956 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.additiveProductName"); 1957 } else if (name.equals("caloricDensity")) { 1958 this.caloricDensity = new SimpleQuantity(); 1959 return this.caloricDensity; 1960 } else if (name.equals("routeofAdministration")) { 1961 this.routeofAdministration = new CodeableConcept(); 1962 return this.routeofAdministration; 1963 } else if (name.equals("administration")) { 1964 return addAdministration(); 1965 } else if (name.equals("maxVolumeToDeliver")) { 1966 this.maxVolumeToDeliver = new SimpleQuantity(); 1967 return this.maxVolumeToDeliver; 1968 } else if (name.equals("administrationInstruction")) { 1969 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.administrationInstruction"); 1970 } else 1971 return super.addChild(name); 1972 } 1973 1974 public NutritionOrderEnteralFormulaComponent copy() { 1975 NutritionOrderEnteralFormulaComponent dst = new NutritionOrderEnteralFormulaComponent(); 1976 copyValues(dst); 1977 dst.baseFormulaType = baseFormulaType == null ? null : baseFormulaType.copy(); 1978 dst.baseFormulaProductName = baseFormulaProductName == null ? null : baseFormulaProductName.copy(); 1979 dst.additiveType = additiveType == null ? null : additiveType.copy(); 1980 dst.additiveProductName = additiveProductName == null ? null : additiveProductName.copy(); 1981 dst.caloricDensity = caloricDensity == null ? null : caloricDensity.copy(); 1982 dst.routeofAdministration = routeofAdministration == null ? null : routeofAdministration.copy(); 1983 if (administration != null) { 1984 dst.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1985 for (NutritionOrderEnteralFormulaAdministrationComponent i : administration) 1986 dst.administration.add(i.copy()); 1987 } 1988 ; 1989 dst.maxVolumeToDeliver = maxVolumeToDeliver == null ? null : maxVolumeToDeliver.copy(); 1990 dst.administrationInstruction = administrationInstruction == null ? null : administrationInstruction.copy(); 1991 return dst; 1992 } 1993 1994 @Override 1995 public boolean equalsDeep(Base other) { 1996 if (!super.equalsDeep(other)) 1997 return false; 1998 if (!(other instanceof NutritionOrderEnteralFormulaComponent)) 1999 return false; 2000 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other; 2001 return compareDeep(baseFormulaType, o.baseFormulaType, true) 2002 && compareDeep(baseFormulaProductName, o.baseFormulaProductName, true) 2003 && compareDeep(additiveType, o.additiveType, true) 2004 && compareDeep(additiveProductName, o.additiveProductName, true) 2005 && compareDeep(caloricDensity, o.caloricDensity, true) 2006 && compareDeep(routeofAdministration, o.routeofAdministration, true) 2007 && compareDeep(administration, o.administration, true) 2008 && compareDeep(maxVolumeToDeliver, o.maxVolumeToDeliver, true) 2009 && compareDeep(administrationInstruction, o.administrationInstruction, true); 2010 } 2011 2012 @Override 2013 public boolean equalsShallow(Base other) { 2014 if (!super.equalsShallow(other)) 2015 return false; 2016 if (!(other instanceof NutritionOrderEnteralFormulaComponent)) 2017 return false; 2018 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other; 2019 return compareValues(baseFormulaProductName, o.baseFormulaProductName, true) 2020 && compareValues(additiveProductName, o.additiveProductName, true) 2021 && compareValues(administrationInstruction, o.administrationInstruction, true); 2022 } 2023 2024 public boolean isEmpty() { 2025 return super.isEmpty() && (baseFormulaType == null || baseFormulaType.isEmpty()) 2026 && (baseFormulaProductName == null || baseFormulaProductName.isEmpty()) 2027 && (additiveType == null || additiveType.isEmpty()) 2028 && (additiveProductName == null || additiveProductName.isEmpty()) 2029 && (caloricDensity == null || caloricDensity.isEmpty()) 2030 && (routeofAdministration == null || routeofAdministration.isEmpty()) 2031 && (administration == null || administration.isEmpty()) 2032 && (maxVolumeToDeliver == null || maxVolumeToDeliver.isEmpty()) 2033 && (administrationInstruction == null || administrationInstruction.isEmpty()); 2034 } 2035 2036 public String fhirType() { 2037 return "NutritionOrder.enteralFormula"; 2038 2039 } 2040 2041 } 2042 2043 @Block() 2044 public static class NutritionOrderEnteralFormulaAdministrationComponent extends BackboneElement 2045 implements IBaseBackboneElement { 2046 /** 2047 * The time period and frequency at which the enteral formula should be 2048 * delivered to the patient. 2049 */ 2050 @Child(name = "schedule", type = { Timing.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2051 @Description(shortDefinition = "Scheduled frequency of enteral feeding", formalDefinition = "The time period and frequency at which the enteral formula should be delivered to the patient.") 2052 protected Timing schedule; 2053 2054 /** 2055 * The volume of formula to provide to the patient per the specified 2056 * administration schedule. 2057 */ 2058 @Child(name = "quantity", type = { 2059 SimpleQuantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2060 @Description(shortDefinition = "The volume of formula to provide", formalDefinition = "The volume of formula to provide to the patient per the specified administration schedule.") 2061 protected SimpleQuantity quantity; 2062 2063 /** 2064 * The rate of administration of formula via a feeding pump, e.g. 60 mL per 2065 * hour, according to the specified schedule. 2066 */ 2067 @Child(name = "rate", type = { SimpleQuantity.class, 2068 Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2069 @Description(shortDefinition = "Speed with which the formula is provided per period of time", formalDefinition = "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.") 2070 protected Type rate; 2071 2072 private static final long serialVersionUID = 1895031997L; 2073 2074 /* 2075 * Constructor 2076 */ 2077 public NutritionOrderEnteralFormulaAdministrationComponent() { 2078 super(); 2079 } 2080 2081 /** 2082 * @return {@link #schedule} (The time period and frequency at which the enteral 2083 * formula should be delivered to the patient.) 2084 */ 2085 public Timing getSchedule() { 2086 if (this.schedule == null) 2087 if (Configuration.errorOnAutoCreate()) 2088 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.schedule"); 2089 else if (Configuration.doAutoCreate()) 2090 this.schedule = new Timing(); // cc 2091 return this.schedule; 2092 } 2093 2094 public boolean hasSchedule() { 2095 return this.schedule != null && !this.schedule.isEmpty(); 2096 } 2097 2098 /** 2099 * @param value {@link #schedule} (The time period and frequency at which the 2100 * enteral formula should be delivered to the patient.) 2101 */ 2102 public NutritionOrderEnteralFormulaAdministrationComponent setSchedule(Timing value) { 2103 this.schedule = value; 2104 return this; 2105 } 2106 2107 /** 2108 * @return {@link #quantity} (The volume of formula to provide to the patient 2109 * per the specified administration schedule.) 2110 */ 2111 public SimpleQuantity getQuantity() { 2112 if (this.quantity == null) 2113 if (Configuration.errorOnAutoCreate()) 2114 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.quantity"); 2115 else if (Configuration.doAutoCreate()) 2116 this.quantity = new SimpleQuantity(); // cc 2117 return this.quantity; 2118 } 2119 2120 public boolean hasQuantity() { 2121 return this.quantity != null && !this.quantity.isEmpty(); 2122 } 2123 2124 /** 2125 * @param value {@link #quantity} (The volume of formula to provide to the 2126 * patient per the specified administration schedule.) 2127 */ 2128 public NutritionOrderEnteralFormulaAdministrationComponent setQuantity(SimpleQuantity value) { 2129 this.quantity = value; 2130 return this; 2131 } 2132 2133 /** 2134 * @return {@link #rate} (The rate of administration of formula via a feeding 2135 * pump, e.g. 60 mL per hour, according to the specified schedule.) 2136 */ 2137 public Type getRate() { 2138 return this.rate; 2139 } 2140 2141 /** 2142 * @return {@link #rate} (The rate of administration of formula via a feeding 2143 * pump, e.g. 60 mL per hour, according to the specified schedule.) 2144 */ 2145 public SimpleQuantity getRateSimpleQuantity() throws FHIRException { 2146 if (!(this.rate instanceof SimpleQuantity)) 2147 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but " 2148 + this.rate.getClass().getName() + " was encountered"); 2149 return (SimpleQuantity) this.rate; 2150 } 2151 2152 public boolean hasRateSimpleQuantity() { 2153 return this.rate instanceof SimpleQuantity; 2154 } 2155 2156 /** 2157 * @return {@link #rate} (The rate of administration of formula via a feeding 2158 * pump, e.g. 60 mL per hour, according to the specified schedule.) 2159 */ 2160 public Ratio getRateRatio() throws FHIRException { 2161 if (!(this.rate instanceof Ratio)) 2162 throw new FHIRException( 2163 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 2164 return (Ratio) this.rate; 2165 } 2166 2167 public boolean hasRateRatio() { 2168 return this.rate instanceof Ratio; 2169 } 2170 2171 public boolean hasRate() { 2172 return this.rate != null && !this.rate.isEmpty(); 2173 } 2174 2175 /** 2176 * @param value {@link #rate} (The rate of administration of formula via a 2177 * feeding pump, e.g. 60 mL per hour, according to the specified 2178 * schedule.) 2179 */ 2180 public NutritionOrderEnteralFormulaAdministrationComponent setRate(Type value) { 2181 this.rate = value; 2182 return this; 2183 } 2184 2185 protected void listChildren(List<Property> childrenList) { 2186 super.listChildren(childrenList); 2187 childrenList.add(new Property("schedule", "Timing", 2188 "The time period and frequency at which the enteral formula should be delivered to the patient.", 0, 2189 java.lang.Integer.MAX_VALUE, schedule)); 2190 childrenList.add(new Property("quantity", "SimpleQuantity", 2191 "The volume of formula to provide to the patient per the specified administration schedule.", 0, 2192 java.lang.Integer.MAX_VALUE, quantity)); 2193 childrenList.add(new Property("rate[x]", "SimpleQuantity|Ratio", 2194 "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 2195 0, java.lang.Integer.MAX_VALUE, rate)); 2196 } 2197 2198 @Override 2199 public void setProperty(String name, Base value) throws FHIRException { 2200 if (name.equals("schedule")) 2201 this.schedule = castToTiming(value); // Timing 2202 else if (name.equals("quantity")) 2203 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2204 else if (name.equals("rate[x]")) 2205 this.rate = (Type) value; // Type 2206 else 2207 super.setProperty(name, value); 2208 } 2209 2210 @Override 2211 public Base addChild(String name) throws FHIRException { 2212 if (name.equals("schedule")) { 2213 this.schedule = new Timing(); 2214 return this.schedule; 2215 } else if (name.equals("quantity")) { 2216 this.quantity = new SimpleQuantity(); 2217 return this.quantity; 2218 } else if (name.equals("rateSimpleQuantity")) { 2219 this.rate = new SimpleQuantity(); 2220 return this.rate; 2221 } else if (name.equals("rateRatio")) { 2222 this.rate = new Ratio(); 2223 return this.rate; 2224 } else 2225 return super.addChild(name); 2226 } 2227 2228 public NutritionOrderEnteralFormulaAdministrationComponent copy() { 2229 NutritionOrderEnteralFormulaAdministrationComponent dst = new NutritionOrderEnteralFormulaAdministrationComponent(); 2230 copyValues(dst); 2231 dst.schedule = schedule == null ? null : schedule.copy(); 2232 dst.quantity = quantity == null ? null : quantity.copy(); 2233 dst.rate = rate == null ? null : rate.copy(); 2234 return dst; 2235 } 2236 2237 @Override 2238 public boolean equalsDeep(Base other) { 2239 if (!super.equalsDeep(other)) 2240 return false; 2241 if (!(other instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 2242 return false; 2243 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other; 2244 return compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) 2245 && compareDeep(rate, o.rate, true); 2246 } 2247 2248 @Override 2249 public boolean equalsShallow(Base other) { 2250 if (!super.equalsShallow(other)) 2251 return false; 2252 if (!(other instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 2253 return false; 2254 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other; 2255 return true; 2256 } 2257 2258 public boolean isEmpty() { 2259 return super.isEmpty() && (schedule == null || schedule.isEmpty()) && (quantity == null || quantity.isEmpty()) 2260 && (rate == null || rate.isEmpty()); 2261 } 2262 2263 public String fhirType() { 2264 return "NutritionOrder.enteralFormula.administration"; 2265 2266 } 2267 2268 } 2269 2270 /** 2271 * The person (patient) who needs the nutrition order for an oral diet, 2272 * nutritional supplement and/or enteral or formula feeding. 2273 */ 2274 @Child(name = "patient", type = { Patient.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 2275 @Description(shortDefinition = "The person who requires the diet, formula or nutritional supplement", formalDefinition = "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.") 2276 protected Reference patient; 2277 2278 /** 2279 * The actual object that is the target of the reference (The person (patient) 2280 * who needs the nutrition order for an oral diet, nutritional supplement and/or 2281 * enteral or formula feeding.) 2282 */ 2283 protected Patient patientTarget; 2284 2285 /** 2286 * The practitioner that holds legal responsibility for ordering the diet, 2287 * nutritional supplement, or formula feedings. 2288 */ 2289 @Child(name = "orderer", type = { Practitioner.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2290 @Description(shortDefinition = "Who ordered the diet, formula or nutritional supplement", formalDefinition = "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.") 2291 protected Reference orderer; 2292 2293 /** 2294 * The actual object that is the target of the reference (The practitioner that 2295 * holds legal responsibility for ordering the diet, nutritional supplement, or 2296 * formula feedings.) 2297 */ 2298 protected Practitioner ordererTarget; 2299 2300 /** 2301 * Identifiers assigned to this order by the order sender or by the order 2302 * receiver. 2303 */ 2304 @Child(name = "identifier", type = { 2305 Identifier.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2306 @Description(shortDefinition = "Identifiers assigned to this order", formalDefinition = "Identifiers assigned to this order by the order sender or by the order receiver.") 2307 protected List<Identifier> identifier; 2308 2309 /** 2310 * An encounter that provides additional information about the healthcare 2311 * context in which this request is made. 2312 */ 2313 @Child(name = "encounter", type = { Encounter.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2314 @Description(shortDefinition = "The encounter associated with this nutrition order", formalDefinition = "An encounter that provides additional information about the healthcare context in which this request is made.") 2315 protected Reference encounter; 2316 2317 /** 2318 * The actual object that is the target of the reference (An encounter that 2319 * provides additional information about the healthcare context in which this 2320 * request is made.) 2321 */ 2322 protected Encounter encounterTarget; 2323 2324 /** 2325 * The date and time that this nutrition order was requested. 2326 */ 2327 @Child(name = "dateTime", type = { 2328 DateTimeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 2329 @Description(shortDefinition = "Date and time the nutrition order was requested", formalDefinition = "The date and time that this nutrition order was requested.") 2330 protected DateTimeType dateTime; 2331 2332 /** 2333 * The workflow status of the nutrition order/request. 2334 */ 2335 @Child(name = "status", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = true, summary = true) 2336 @Description(shortDefinition = "proposed | draft | planned | requested | active | on-hold | completed | cancelled", formalDefinition = "The workflow status of the nutrition order/request.") 2337 protected Enumeration<NutritionOrderStatus> status; 2338 2339 /** 2340 * A link to a record of allergies or intolerances which should be included in 2341 * the nutrition order. 2342 */ 2343 @Child(name = "allergyIntolerance", type = { 2344 AllergyIntolerance.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2345 @Description(shortDefinition = "List of the patient's food and nutrition-related allergies and intolerances", formalDefinition = "A link to a record of allergies or intolerances which should be included in the nutrition order.") 2346 protected List<Reference> allergyIntolerance; 2347 /** 2348 * The actual objects that are the target of the reference (A link to a record 2349 * of allergies or intolerances which should be included in the nutrition 2350 * order.) 2351 */ 2352 protected List<AllergyIntolerance> allergyIntoleranceTarget; 2353 2354 /** 2355 * This modifier is used to convey order-specific modifiers about the type of 2356 * food that should be given. These can be derived from patient allergies, 2357 * intolerances, or preferences such as Halal, Vegan or Kosher. This modifier 2358 * applies to the entire nutrition order inclusive of the oral diet, nutritional 2359 * supplements and enteral formula feedings. 2360 */ 2361 @Child(name = "foodPreferenceModifier", type = { 2362 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2363 @Description(shortDefinition = "Order-specific modifier about the type of food that should be given", formalDefinition = "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.") 2364 protected List<CodeableConcept> foodPreferenceModifier; 2365 2366 /** 2367 * This modifier is used to convey order-specific modifiers about the type of 2368 * food that should NOT be given. These can be derived from patient allergies, 2369 * intolerances, or preferences such as No Red Meat, No Soy or No Wheat or 2370 * Gluten-Free. While it should not be necessary to repeat allergy or 2371 * intolerance information captured in the referenced allergyIntolerance 2372 * resource in the excludeFoodModifier, this element may be used to convey 2373 * additional specificity related to foods that should be eliminated from the 2374 * patient?s diet for any reason. This modifier applies to the entire nutrition 2375 * order inclusive of the oral diet, nutritional supplements and enteral formula 2376 * feedings. 2377 */ 2378 @Child(name = "excludeFoodModifier", type = { 2379 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2380 @Description(shortDefinition = "Order-specific modifier about the type of food that should not be given", formalDefinition = "This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced allergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.") 2381 protected List<CodeableConcept> excludeFoodModifier; 2382 2383 /** 2384 * Diet given orally in contrast to enteral (tube) feeding. 2385 */ 2386 @Child(name = "oralDiet", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 2387 @Description(shortDefinition = "Oral diet components", formalDefinition = "Diet given orally in contrast to enteral (tube) feeding.") 2388 protected NutritionOrderOralDietComponent oralDiet; 2389 2390 /** 2391 * Oral nutritional products given in order to add further nutritional value to 2392 * the patient's diet. 2393 */ 2394 @Child(name = "supplement", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2395 @Description(shortDefinition = "Supplement components", formalDefinition = "Oral nutritional products given in order to add further nutritional value to the patient's diet.") 2396 protected List<NutritionOrderSupplementComponent> supplement; 2397 2398 /** 2399 * Feeding provided through the gastrointestinal tract via a tube, catheter, or 2400 * stoma that delivers nutrition distal to the oral cavity. 2401 */ 2402 @Child(name = "enteralFormula", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = false) 2403 @Description(shortDefinition = "Enteral formula components", formalDefinition = "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.") 2404 protected NutritionOrderEnteralFormulaComponent enteralFormula; 2405 2406 private static final long serialVersionUID = 1139624085L; 2407 2408 /* 2409 * Constructor 2410 */ 2411 public NutritionOrder() { 2412 super(); 2413 } 2414 2415 /* 2416 * Constructor 2417 */ 2418 public NutritionOrder(Reference patient, DateTimeType dateTime) { 2419 super(); 2420 this.patient = patient; 2421 this.dateTime = dateTime; 2422 } 2423 2424 /** 2425 * @return {@link #patient} (The person (patient) who needs the nutrition order 2426 * for an oral diet, nutritional supplement and/or enteral or formula 2427 * feeding.) 2428 */ 2429 public Reference getPatient() { 2430 if (this.patient == null) 2431 if (Configuration.errorOnAutoCreate()) 2432 throw new Error("Attempt to auto-create NutritionOrder.patient"); 2433 else if (Configuration.doAutoCreate()) 2434 this.patient = new Reference(); // cc 2435 return this.patient; 2436 } 2437 2438 public boolean hasPatient() { 2439 return this.patient != null && !this.patient.isEmpty(); 2440 } 2441 2442 /** 2443 * @param value {@link #patient} (The person (patient) who needs the nutrition 2444 * order for an oral diet, nutritional supplement and/or enteral or 2445 * formula feeding.) 2446 */ 2447 public NutritionOrder setPatient(Reference value) { 2448 this.patient = value; 2449 return this; 2450 } 2451 2452 /** 2453 * @return {@link #patient} The actual object that is the target of the 2454 * reference. The reference library doesn't populate this, but you can 2455 * use it to hold the resource if you resolve it. (The person (patient) 2456 * who needs the nutrition order for an oral diet, nutritional 2457 * supplement and/or enteral or formula feeding.) 2458 */ 2459 public Patient getPatientTarget() { 2460 if (this.patientTarget == null) 2461 if (Configuration.errorOnAutoCreate()) 2462 throw new Error("Attempt to auto-create NutritionOrder.patient"); 2463 else if (Configuration.doAutoCreate()) 2464 this.patientTarget = new Patient(); // aa 2465 return this.patientTarget; 2466 } 2467 2468 /** 2469 * @param value {@link #patient} The actual object that is the target of the 2470 * reference. The reference library doesn't use these, but you can 2471 * use it to hold the resource if you resolve it. (The person 2472 * (patient) who needs the nutrition order for an oral diet, 2473 * nutritional supplement and/or enteral or formula feeding.) 2474 */ 2475 public NutritionOrder setPatientTarget(Patient value) { 2476 this.patientTarget = value; 2477 return this; 2478 } 2479 2480 /** 2481 * @return {@link #orderer} (The practitioner that holds legal responsibility 2482 * for ordering the diet, nutritional supplement, or formula feedings.) 2483 */ 2484 public Reference getOrderer() { 2485 if (this.orderer == null) 2486 if (Configuration.errorOnAutoCreate()) 2487 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 2488 else if (Configuration.doAutoCreate()) 2489 this.orderer = new Reference(); // cc 2490 return this.orderer; 2491 } 2492 2493 public boolean hasOrderer() { 2494 return this.orderer != null && !this.orderer.isEmpty(); 2495 } 2496 2497 /** 2498 * @param value {@link #orderer} (The practitioner that holds legal 2499 * responsibility for ordering the diet, nutritional supplement, or 2500 * formula feedings.) 2501 */ 2502 public NutritionOrder setOrderer(Reference value) { 2503 this.orderer = value; 2504 return this; 2505 } 2506 2507 /** 2508 * @return {@link #orderer} The actual object that is the target of the 2509 * reference. The reference library doesn't populate this, but you can 2510 * use it to hold the resource if you resolve it. (The practitioner that 2511 * holds legal responsibility for ordering the diet, nutritional 2512 * supplement, or formula feedings.) 2513 */ 2514 public Practitioner getOrdererTarget() { 2515 if (this.ordererTarget == null) 2516 if (Configuration.errorOnAutoCreate()) 2517 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 2518 else if (Configuration.doAutoCreate()) 2519 this.ordererTarget = new Practitioner(); // aa 2520 return this.ordererTarget; 2521 } 2522 2523 /** 2524 * @param value {@link #orderer} The actual object that is the target of the 2525 * reference. The reference library doesn't use these, but you can 2526 * use it to hold the resource if you resolve it. (The practitioner 2527 * that holds legal responsibility for ordering the diet, 2528 * nutritional supplement, or formula feedings.) 2529 */ 2530 public NutritionOrder setOrdererTarget(Practitioner value) { 2531 this.ordererTarget = value; 2532 return this; 2533 } 2534 2535 /** 2536 * @return {@link #identifier} (Identifiers assigned to this order by the order 2537 * sender or by the order receiver.) 2538 */ 2539 public List<Identifier> getIdentifier() { 2540 if (this.identifier == null) 2541 this.identifier = new ArrayList<Identifier>(); 2542 return this.identifier; 2543 } 2544 2545 public boolean hasIdentifier() { 2546 if (this.identifier == null) 2547 return false; 2548 for (Identifier item : this.identifier) 2549 if (!item.isEmpty()) 2550 return true; 2551 return false; 2552 } 2553 2554 /** 2555 * @return {@link #identifier} (Identifiers assigned to this order by the order 2556 * sender or by the order receiver.) 2557 */ 2558 // syntactic sugar 2559 public Identifier addIdentifier() { // 3 2560 Identifier t = new Identifier(); 2561 if (this.identifier == null) 2562 this.identifier = new ArrayList<Identifier>(); 2563 this.identifier.add(t); 2564 return t; 2565 } 2566 2567 // syntactic sugar 2568 public NutritionOrder addIdentifier(Identifier t) { // 3 2569 if (t == null) 2570 return this; 2571 if (this.identifier == null) 2572 this.identifier = new ArrayList<Identifier>(); 2573 this.identifier.add(t); 2574 return this; 2575 } 2576 2577 /** 2578 * @return {@link #encounter} (An encounter that provides additional information 2579 * about the healthcare context in which this request is made.) 2580 */ 2581 public Reference getEncounter() { 2582 if (this.encounter == null) 2583 if (Configuration.errorOnAutoCreate()) 2584 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 2585 else if (Configuration.doAutoCreate()) 2586 this.encounter = new Reference(); // cc 2587 return this.encounter; 2588 } 2589 2590 public boolean hasEncounter() { 2591 return this.encounter != null && !this.encounter.isEmpty(); 2592 } 2593 2594 /** 2595 * @param value {@link #encounter} (An encounter that provides additional 2596 * information about the healthcare context in which this request 2597 * is made.) 2598 */ 2599 public NutritionOrder setEncounter(Reference value) { 2600 this.encounter = value; 2601 return this; 2602 } 2603 2604 /** 2605 * @return {@link #encounter} The actual object that is the target of the 2606 * reference. The reference library doesn't populate this, but you can 2607 * use it to hold the resource if you resolve it. (An encounter that 2608 * provides additional information about the healthcare context in which 2609 * this request is made.) 2610 */ 2611 public Encounter getEncounterTarget() { 2612 if (this.encounterTarget == null) 2613 if (Configuration.errorOnAutoCreate()) 2614 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 2615 else if (Configuration.doAutoCreate()) 2616 this.encounterTarget = new Encounter(); // aa 2617 return this.encounterTarget; 2618 } 2619 2620 /** 2621 * @param value {@link #encounter} The actual object that is the target of the 2622 * reference. The reference library doesn't use these, but you can 2623 * use it to hold the resource if you resolve it. (An encounter 2624 * that provides additional information about the healthcare 2625 * context in which this request is made.) 2626 */ 2627 public NutritionOrder setEncounterTarget(Encounter value) { 2628 this.encounterTarget = value; 2629 return this; 2630 } 2631 2632 /** 2633 * @return {@link #dateTime} (The date and time that this nutrition order was 2634 * requested.). This is the underlying object with id, value and 2635 * extensions. The accessor "getDateTime" gives direct access to the 2636 * value 2637 */ 2638 public DateTimeType getDateTimeElement() { 2639 if (this.dateTime == null) 2640 if (Configuration.errorOnAutoCreate()) 2641 throw new Error("Attempt to auto-create NutritionOrder.dateTime"); 2642 else if (Configuration.doAutoCreate()) 2643 this.dateTime = new DateTimeType(); // bb 2644 return this.dateTime; 2645 } 2646 2647 public boolean hasDateTimeElement() { 2648 return this.dateTime != null && !this.dateTime.isEmpty(); 2649 } 2650 2651 public boolean hasDateTime() { 2652 return this.dateTime != null && !this.dateTime.isEmpty(); 2653 } 2654 2655 /** 2656 * @param value {@link #dateTime} (The date and time that this nutrition order 2657 * was requested.). This is the underlying object with id, value 2658 * and extensions. The accessor "getDateTime" gives direct access 2659 * to the value 2660 */ 2661 public NutritionOrder setDateTimeElement(DateTimeType value) { 2662 this.dateTime = value; 2663 return this; 2664 } 2665 2666 /** 2667 * @return The date and time that this nutrition order was requested. 2668 */ 2669 public Date getDateTime() { 2670 return this.dateTime == null ? null : this.dateTime.getValue(); 2671 } 2672 2673 /** 2674 * @param value The date and time that this nutrition order was requested. 2675 */ 2676 public NutritionOrder setDateTime(Date value) { 2677 if (this.dateTime == null) 2678 this.dateTime = new DateTimeType(); 2679 this.dateTime.setValue(value); 2680 return this; 2681 } 2682 2683 /** 2684 * @return {@link #status} (The workflow status of the nutrition 2685 * order/request.). This is the underlying object with id, value and 2686 * extensions. The accessor "getStatus" gives direct access to the value 2687 */ 2688 public Enumeration<NutritionOrderStatus> getStatusElement() { 2689 if (this.status == null) 2690 if (Configuration.errorOnAutoCreate()) 2691 throw new Error("Attempt to auto-create NutritionOrder.status"); 2692 else if (Configuration.doAutoCreate()) 2693 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); // bb 2694 return this.status; 2695 } 2696 2697 public boolean hasStatusElement() { 2698 return this.status != null && !this.status.isEmpty(); 2699 } 2700 2701 public boolean hasStatus() { 2702 return this.status != null && !this.status.isEmpty(); 2703 } 2704 2705 /** 2706 * @param value {@link #status} (The workflow status of the nutrition 2707 * order/request.). This is the underlying object with id, value 2708 * and extensions. The accessor "getStatus" gives direct access to 2709 * the value 2710 */ 2711 public NutritionOrder setStatusElement(Enumeration<NutritionOrderStatus> value) { 2712 this.status = value; 2713 return this; 2714 } 2715 2716 /** 2717 * @return The workflow status of the nutrition order/request. 2718 */ 2719 public NutritionOrderStatus getStatus() { 2720 return this.status == null ? null : this.status.getValue(); 2721 } 2722 2723 /** 2724 * @param value The workflow status of the nutrition order/request. 2725 */ 2726 public NutritionOrder setStatus(NutritionOrderStatus value) { 2727 if (value == null) 2728 this.status = null; 2729 else { 2730 if (this.status == null) 2731 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); 2732 this.status.setValue(value); 2733 } 2734 return this; 2735 } 2736 2737 /** 2738 * @return {@link #allergyIntolerance} (A link to a record of allergies or 2739 * intolerances which should be included in the nutrition order.) 2740 */ 2741 public List<Reference> getAllergyIntolerance() { 2742 if (this.allergyIntolerance == null) 2743 this.allergyIntolerance = new ArrayList<Reference>(); 2744 return this.allergyIntolerance; 2745 } 2746 2747 public boolean hasAllergyIntolerance() { 2748 if (this.allergyIntolerance == null) 2749 return false; 2750 for (Reference item : this.allergyIntolerance) 2751 if (!item.isEmpty()) 2752 return true; 2753 return false; 2754 } 2755 2756 /** 2757 * @return {@link #allergyIntolerance} (A link to a record of allergies or 2758 * intolerances which should be included in the nutrition order.) 2759 */ 2760 // syntactic sugar 2761 public Reference addAllergyIntolerance() { // 3 2762 Reference t = new Reference(); 2763 if (this.allergyIntolerance == null) 2764 this.allergyIntolerance = new ArrayList<Reference>(); 2765 this.allergyIntolerance.add(t); 2766 return t; 2767 } 2768 2769 // syntactic sugar 2770 public NutritionOrder addAllergyIntolerance(Reference t) { // 3 2771 if (t == null) 2772 return this; 2773 if (this.allergyIntolerance == null) 2774 this.allergyIntolerance = new ArrayList<Reference>(); 2775 this.allergyIntolerance.add(t); 2776 return this; 2777 } 2778 2779 /** 2780 * @return {@link #allergyIntolerance} (The actual objects that are the target 2781 * of the reference. The reference library doesn't populate this, but 2782 * you can use this to hold the resources if you resolvethemt. A link to 2783 * a record of allergies or intolerances which should be included in the 2784 * nutrition order.) 2785 */ 2786 public List<AllergyIntolerance> getAllergyIntoleranceTarget() { 2787 if (this.allergyIntoleranceTarget == null) 2788 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 2789 return this.allergyIntoleranceTarget; 2790 } 2791 2792 // syntactic sugar 2793 /** 2794 * @return {@link #allergyIntolerance} (Add an actual object that is the target 2795 * of the reference. The reference library doesn't use these, but you 2796 * can use this to hold the resources if you resolvethemt. A link to a 2797 * record of allergies or intolerances which should be included in the 2798 * nutrition order.) 2799 */ 2800 public AllergyIntolerance addAllergyIntoleranceTarget() { 2801 AllergyIntolerance r = new AllergyIntolerance(); 2802 if (this.allergyIntoleranceTarget == null) 2803 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 2804 this.allergyIntoleranceTarget.add(r); 2805 return r; 2806 } 2807 2808 /** 2809 * @return {@link #foodPreferenceModifier} (This modifier is used to convey 2810 * order-specific modifiers about the type of food that should be given. 2811 * These can be derived from patient allergies, intolerances, or 2812 * preferences such as Halal, Vegan or Kosher. This modifier applies to 2813 * the entire nutrition order inclusive of the oral diet, nutritional 2814 * supplements and enteral formula feedings.) 2815 */ 2816 public List<CodeableConcept> getFoodPreferenceModifier() { 2817 if (this.foodPreferenceModifier == null) 2818 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 2819 return this.foodPreferenceModifier; 2820 } 2821 2822 public boolean hasFoodPreferenceModifier() { 2823 if (this.foodPreferenceModifier == null) 2824 return false; 2825 for (CodeableConcept item : this.foodPreferenceModifier) 2826 if (!item.isEmpty()) 2827 return true; 2828 return false; 2829 } 2830 2831 /** 2832 * @return {@link #foodPreferenceModifier} (This modifier is used to convey 2833 * order-specific modifiers about the type of food that should be given. 2834 * These can be derived from patient allergies, intolerances, or 2835 * preferences such as Halal, Vegan or Kosher. This modifier applies to 2836 * the entire nutrition order inclusive of the oral diet, nutritional 2837 * supplements and enteral formula feedings.) 2838 */ 2839 // syntactic sugar 2840 public CodeableConcept addFoodPreferenceModifier() { // 3 2841 CodeableConcept t = new CodeableConcept(); 2842 if (this.foodPreferenceModifier == null) 2843 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 2844 this.foodPreferenceModifier.add(t); 2845 return t; 2846 } 2847 2848 // syntactic sugar 2849 public NutritionOrder addFoodPreferenceModifier(CodeableConcept t) { // 3 2850 if (t == null) 2851 return this; 2852 if (this.foodPreferenceModifier == null) 2853 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 2854 this.foodPreferenceModifier.add(t); 2855 return this; 2856 } 2857 2858 /** 2859 * @return {@link #excludeFoodModifier} (This modifier is used to convey 2860 * order-specific modifiers about the type of food that should NOT be 2861 * given. These can be derived from patient allergies, intolerances, or 2862 * preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. 2863 * While it should not be necessary to repeat allergy or intolerance 2864 * information captured in the referenced allergyIntolerance resource in 2865 * the excludeFoodModifier, this element may be used to convey 2866 * additional specificity related to foods that should be eliminated 2867 * from the patient?s diet for any reason. This modifier applies to the 2868 * entire nutrition order inclusive of the oral diet, nutritional 2869 * supplements and enteral formula feedings.) 2870 */ 2871 public List<CodeableConcept> getExcludeFoodModifier() { 2872 if (this.excludeFoodModifier == null) 2873 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 2874 return this.excludeFoodModifier; 2875 } 2876 2877 public boolean hasExcludeFoodModifier() { 2878 if (this.excludeFoodModifier == null) 2879 return false; 2880 for (CodeableConcept item : this.excludeFoodModifier) 2881 if (!item.isEmpty()) 2882 return true; 2883 return false; 2884 } 2885 2886 /** 2887 * @return {@link #excludeFoodModifier} (This modifier is used to convey 2888 * order-specific modifiers about the type of food that should NOT be 2889 * given. These can be derived from patient allergies, intolerances, or 2890 * preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. 2891 * While it should not be necessary to repeat allergy or intolerance 2892 * information captured in the referenced allergyIntolerance resource in 2893 * the excludeFoodModifier, this element may be used to convey 2894 * additional specificity related to foods that should be eliminated 2895 * from the patient?s diet for any reason. This modifier applies to the 2896 * entire nutrition order inclusive of the oral diet, nutritional 2897 * supplements and enteral formula feedings.) 2898 */ 2899 // syntactic sugar 2900 public CodeableConcept addExcludeFoodModifier() { // 3 2901 CodeableConcept t = new CodeableConcept(); 2902 if (this.excludeFoodModifier == null) 2903 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 2904 this.excludeFoodModifier.add(t); 2905 return t; 2906 } 2907 2908 // syntactic sugar 2909 public NutritionOrder addExcludeFoodModifier(CodeableConcept t) { // 3 2910 if (t == null) 2911 return this; 2912 if (this.excludeFoodModifier == null) 2913 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 2914 this.excludeFoodModifier.add(t); 2915 return this; 2916 } 2917 2918 /** 2919 * @return {@link #oralDiet} (Diet given orally in contrast to enteral (tube) 2920 * feeding.) 2921 */ 2922 public NutritionOrderOralDietComponent getOralDiet() { 2923 if (this.oralDiet == null) 2924 if (Configuration.errorOnAutoCreate()) 2925 throw new Error("Attempt to auto-create NutritionOrder.oralDiet"); 2926 else if (Configuration.doAutoCreate()) 2927 this.oralDiet = new NutritionOrderOralDietComponent(); // cc 2928 return this.oralDiet; 2929 } 2930 2931 public boolean hasOralDiet() { 2932 return this.oralDiet != null && !this.oralDiet.isEmpty(); 2933 } 2934 2935 /** 2936 * @param value {@link #oralDiet} (Diet given orally in contrast to enteral 2937 * (tube) feeding.) 2938 */ 2939 public NutritionOrder setOralDiet(NutritionOrderOralDietComponent value) { 2940 this.oralDiet = value; 2941 return this; 2942 } 2943 2944 /** 2945 * @return {@link #supplement} (Oral nutritional products given in order to add 2946 * further nutritional value to the patient's diet.) 2947 */ 2948 public List<NutritionOrderSupplementComponent> getSupplement() { 2949 if (this.supplement == null) 2950 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 2951 return this.supplement; 2952 } 2953 2954 public boolean hasSupplement() { 2955 if (this.supplement == null) 2956 return false; 2957 for (NutritionOrderSupplementComponent item : this.supplement) 2958 if (!item.isEmpty()) 2959 return true; 2960 return false; 2961 } 2962 2963 /** 2964 * @return {@link #supplement} (Oral nutritional products given in order to add 2965 * further nutritional value to the patient's diet.) 2966 */ 2967 // syntactic sugar 2968 public NutritionOrderSupplementComponent addSupplement() { // 3 2969 NutritionOrderSupplementComponent t = new NutritionOrderSupplementComponent(); 2970 if (this.supplement == null) 2971 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 2972 this.supplement.add(t); 2973 return t; 2974 } 2975 2976 // syntactic sugar 2977 public NutritionOrder addSupplement(NutritionOrderSupplementComponent t) { // 3 2978 if (t == null) 2979 return this; 2980 if (this.supplement == null) 2981 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 2982 this.supplement.add(t); 2983 return this; 2984 } 2985 2986 /** 2987 * @return {@link #enteralFormula} (Feeding provided through the 2988 * gastrointestinal tract via a tube, catheter, or stoma that delivers 2989 * nutrition distal to the oral cavity.) 2990 */ 2991 public NutritionOrderEnteralFormulaComponent getEnteralFormula() { 2992 if (this.enteralFormula == null) 2993 if (Configuration.errorOnAutoCreate()) 2994 throw new Error("Attempt to auto-create NutritionOrder.enteralFormula"); 2995 else if (Configuration.doAutoCreate()) 2996 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); // cc 2997 return this.enteralFormula; 2998 } 2999 3000 public boolean hasEnteralFormula() { 3001 return this.enteralFormula != null && !this.enteralFormula.isEmpty(); 3002 } 3003 3004 /** 3005 * @param value {@link #enteralFormula} (Feeding provided through the 3006 * gastrointestinal tract via a tube, catheter, or stoma that 3007 * delivers nutrition distal to the oral cavity.) 3008 */ 3009 public NutritionOrder setEnteralFormula(NutritionOrderEnteralFormulaComponent value) { 3010 this.enteralFormula = value; 3011 return this; 3012 } 3013 3014 protected void listChildren(List<Property> childrenList) { 3015 super.listChildren(childrenList); 3016 childrenList.add(new Property("patient", "Reference(Patient)", 3017 "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 3018 0, java.lang.Integer.MAX_VALUE, patient)); 3019 childrenList.add(new Property("orderer", "Reference(Practitioner)", 3020 "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 3021 0, java.lang.Integer.MAX_VALUE, orderer)); 3022 childrenList.add(new Property("identifier", "Identifier", 3023 "Identifiers assigned to this order by the order sender or by the order receiver.", 0, 3024 java.lang.Integer.MAX_VALUE, identifier)); 3025 childrenList.add(new Property("encounter", "Reference(Encounter)", 3026 "An encounter that provides additional information about the healthcare context in which this request is made.", 3027 0, java.lang.Integer.MAX_VALUE, encounter)); 3028 childrenList.add(new Property("dateTime", "dateTime", "The date and time that this nutrition order was requested.", 3029 0, java.lang.Integer.MAX_VALUE, dateTime)); 3030 childrenList.add(new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 3031 java.lang.Integer.MAX_VALUE, status)); 3032 childrenList.add(new Property("allergyIntolerance", "Reference(AllergyIntolerance)", 3033 "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, 3034 java.lang.Integer.MAX_VALUE, allergyIntolerance)); 3035 childrenList.add(new Property("foodPreferenceModifier", "CodeableConcept", 3036 "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 3037 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier)); 3038 childrenList.add(new Property("excludeFoodModifier", "CodeableConcept", 3039 "This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced allergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 3040 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier)); 3041 childrenList.add(new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 3042 java.lang.Integer.MAX_VALUE, oralDiet)); 3043 childrenList.add(new Property("supplement", "", 3044 "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, 3045 java.lang.Integer.MAX_VALUE, supplement)); 3046 childrenList.add(new Property("enteralFormula", "", 3047 "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 3048 0, java.lang.Integer.MAX_VALUE, enteralFormula)); 3049 } 3050 3051 @Override 3052 public void setProperty(String name, Base value) throws FHIRException { 3053 if (name.equals("patient")) 3054 this.patient = castToReference(value); // Reference 3055 else if (name.equals("orderer")) 3056 this.orderer = castToReference(value); // Reference 3057 else if (name.equals("identifier")) 3058 this.getIdentifier().add(castToIdentifier(value)); 3059 else if (name.equals("encounter")) 3060 this.encounter = castToReference(value); // Reference 3061 else if (name.equals("dateTime")) 3062 this.dateTime = castToDateTime(value); // DateTimeType 3063 else if (name.equals("status")) 3064 this.status = new NutritionOrderStatusEnumFactory().fromType(value); // Enumeration<NutritionOrderStatus> 3065 else if (name.equals("allergyIntolerance")) 3066 this.getAllergyIntolerance().add(castToReference(value)); 3067 else if (name.equals("foodPreferenceModifier")) 3068 this.getFoodPreferenceModifier().add(castToCodeableConcept(value)); 3069 else if (name.equals("excludeFoodModifier")) 3070 this.getExcludeFoodModifier().add(castToCodeableConcept(value)); 3071 else if (name.equals("oralDiet")) 3072 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 3073 else if (name.equals("supplement")) 3074 this.getSupplement().add((NutritionOrderSupplementComponent) value); 3075 else if (name.equals("enteralFormula")) 3076 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 3077 else 3078 super.setProperty(name, value); 3079 } 3080 3081 @Override 3082 public Base addChild(String name) throws FHIRException { 3083 if (name.equals("patient")) { 3084 this.patient = new Reference(); 3085 return this.patient; 3086 } else if (name.equals("orderer")) { 3087 this.orderer = new Reference(); 3088 return this.orderer; 3089 } else if (name.equals("identifier")) { 3090 return addIdentifier(); 3091 } else if (name.equals("encounter")) { 3092 this.encounter = new Reference(); 3093 return this.encounter; 3094 } else if (name.equals("dateTime")) { 3095 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.dateTime"); 3096 } else if (name.equals("status")) { 3097 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.status"); 3098 } else if (name.equals("allergyIntolerance")) { 3099 return addAllergyIntolerance(); 3100 } else if (name.equals("foodPreferenceModifier")) { 3101 return addFoodPreferenceModifier(); 3102 } else if (name.equals("excludeFoodModifier")) { 3103 return addExcludeFoodModifier(); 3104 } else if (name.equals("oralDiet")) { 3105 this.oralDiet = new NutritionOrderOralDietComponent(); 3106 return this.oralDiet; 3107 } else if (name.equals("supplement")) { 3108 return addSupplement(); 3109 } else if (name.equals("enteralFormula")) { 3110 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); 3111 return this.enteralFormula; 3112 } else 3113 return super.addChild(name); 3114 } 3115 3116 public String fhirType() { 3117 return "NutritionOrder"; 3118 3119 } 3120 3121 public NutritionOrder copy() { 3122 NutritionOrder dst = new NutritionOrder(); 3123 copyValues(dst); 3124 dst.patient = patient == null ? null : patient.copy(); 3125 dst.orderer = orderer == null ? null : orderer.copy(); 3126 if (identifier != null) { 3127 dst.identifier = new ArrayList<Identifier>(); 3128 for (Identifier i : identifier) 3129 dst.identifier.add(i.copy()); 3130 } 3131 ; 3132 dst.encounter = encounter == null ? null : encounter.copy(); 3133 dst.dateTime = dateTime == null ? null : dateTime.copy(); 3134 dst.status = status == null ? null : status.copy(); 3135 if (allergyIntolerance != null) { 3136 dst.allergyIntolerance = new ArrayList<Reference>(); 3137 for (Reference i : allergyIntolerance) 3138 dst.allergyIntolerance.add(i.copy()); 3139 } 3140 ; 3141 if (foodPreferenceModifier != null) { 3142 dst.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 3143 for (CodeableConcept i : foodPreferenceModifier) 3144 dst.foodPreferenceModifier.add(i.copy()); 3145 } 3146 ; 3147 if (excludeFoodModifier != null) { 3148 dst.excludeFoodModifier = new ArrayList<CodeableConcept>(); 3149 for (CodeableConcept i : excludeFoodModifier) 3150 dst.excludeFoodModifier.add(i.copy()); 3151 } 3152 ; 3153 dst.oralDiet = oralDiet == null ? null : oralDiet.copy(); 3154 if (supplement != null) { 3155 dst.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 3156 for (NutritionOrderSupplementComponent i : supplement) 3157 dst.supplement.add(i.copy()); 3158 } 3159 ; 3160 dst.enteralFormula = enteralFormula == null ? null : enteralFormula.copy(); 3161 return dst; 3162 } 3163 3164 protected NutritionOrder typedCopy() { 3165 return copy(); 3166 } 3167 3168 @Override 3169 public boolean equalsDeep(Base other) { 3170 if (!super.equalsDeep(other)) 3171 return false; 3172 if (!(other instanceof NutritionOrder)) 3173 return false; 3174 NutritionOrder o = (NutritionOrder) other; 3175 return compareDeep(patient, o.patient, true) && compareDeep(orderer, o.orderer, true) 3176 && compareDeep(identifier, o.identifier, true) && compareDeep(encounter, o.encounter, true) 3177 && compareDeep(dateTime, o.dateTime, true) && compareDeep(status, o.status, true) 3178 && compareDeep(allergyIntolerance, o.allergyIntolerance, true) 3179 && compareDeep(foodPreferenceModifier, o.foodPreferenceModifier, true) 3180 && compareDeep(excludeFoodModifier, o.excludeFoodModifier, true) && compareDeep(oralDiet, o.oralDiet, true) 3181 && compareDeep(supplement, o.supplement, true) && compareDeep(enteralFormula, o.enteralFormula, true); 3182 } 3183 3184 @Override 3185 public boolean equalsShallow(Base other) { 3186 if (!super.equalsShallow(other)) 3187 return false; 3188 if (!(other instanceof NutritionOrder)) 3189 return false; 3190 NutritionOrder o = (NutritionOrder) other; 3191 return compareValues(dateTime, o.dateTime, true) && compareValues(status, o.status, true); 3192 } 3193 3194 public boolean isEmpty() { 3195 return super.isEmpty() && (patient == null || patient.isEmpty()) && (orderer == null || orderer.isEmpty()) 3196 && (identifier == null || identifier.isEmpty()) && (encounter == null || encounter.isEmpty()) 3197 && (dateTime == null || dateTime.isEmpty()) && (status == null || status.isEmpty()) 3198 && (allergyIntolerance == null || allergyIntolerance.isEmpty()) 3199 && (foodPreferenceModifier == null || foodPreferenceModifier.isEmpty()) 3200 && (excludeFoodModifier == null || excludeFoodModifier.isEmpty()) && (oralDiet == null || oralDiet.isEmpty()) 3201 && (supplement == null || supplement.isEmpty()) && (enteralFormula == null || enteralFormula.isEmpty()); 3202 } 3203 3204 @Override 3205 public ResourceType getResourceType() { 3206 return ResourceType.NutritionOrder; 3207 } 3208 3209 @SearchParamDefinition(name = "identifier", path = "NutritionOrder.identifier", description = "Return nutrition orders with this external identifier", type = "token") 3210 public static final String SP_IDENTIFIER = "identifier"; 3211 @SearchParamDefinition(name = "datetime", path = "NutritionOrder.dateTime", description = "Return nutrition orders requested on this date", type = "date") 3212 public static final String SP_DATETIME = "datetime"; 3213 @SearchParamDefinition(name = "provider", path = "NutritionOrder.orderer", description = "The identify of the provider who placed the nutrition order", type = "reference") 3214 public static final String SP_PROVIDER = "provider"; 3215 @SearchParamDefinition(name = "patient", path = "NutritionOrder.patient", description = "The identity of the person who requires the diet, formula or nutritional supplement", type = "reference") 3216 public static final String SP_PATIENT = "patient"; 3217 @SearchParamDefinition(name = "supplement", path = "NutritionOrder.supplement.type", description = "Type of supplement product requested", type = "token") 3218 public static final String SP_SUPPLEMENT = "supplement"; 3219 @SearchParamDefinition(name = "formula", path = "NutritionOrder.enteralFormula.baseFormulaType", description = "Type of enteral or infant formula", type = "token") 3220 public static final String SP_FORMULA = "formula"; 3221 @SearchParamDefinition(name = "encounter", path = "NutritionOrder.encounter", description = "Return nutrition orders with this encounter identifier", type = "reference") 3222 public static final String SP_ENCOUNTER = "encounter"; 3223 @SearchParamDefinition(name = "oraldiet", path = "NutritionOrder.oralDiet.type", description = "Type of diet that can be consumed orally (i.e., take via the mouth).", type = "token") 3224 public static final String SP_ORALDIET = "oraldiet"; 3225 @SearchParamDefinition(name = "status", path = "NutritionOrder.status", description = "Status of the nutrition order.", type = "token") 3226 public static final String SP_STATUS = "status"; 3227 @SearchParamDefinition(name = "additive", path = "NutritionOrder.enteralFormula.additiveType", description = "Type of module component to add to the feeding", type = "token") 3228 public static final String SP_ADDITIVE = "additive"; 3229 3230}