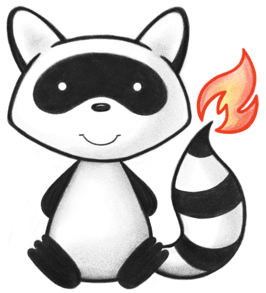
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A request to supply a diet, formula feeding (enteral) or oral nutritional 048 * supplement to a patient/resident. 049 */ 050@ResourceDef(name = "NutritionOrder", profile = "http://hl7.org/fhir/Profile/NutritionOrder") 051public class NutritionOrder extends DomainResource { 052 053 public enum NutritionOrderStatus { 054 /** 055 * The request has been proposed. 056 */ 057 PROPOSED, 058 /** 059 * The request is in preliminary form prior to being sent. 060 */ 061 DRAFT, 062 /** 063 * The request has been planned. 064 */ 065 PLANNED, 066 /** 067 * The request has been placed. 068 */ 069 REQUESTED, 070 /** 071 * The request is 'actionable', but not all actions that are implied by it have 072 * occurred yet. 073 */ 074 ACTIVE, 075 /** 076 * Actions implied by the request have been temporarily halted, but are expected 077 * to continue later. May also be called "suspended". 078 */ 079 ONHOLD, 080 /** 081 * All actions that are implied by the order have occurred and no continuation 082 * is planned (this will rarely be made explicit). 083 */ 084 COMPLETED, 085 /** 086 * The request has been withdrawn and is no longer actionable. 087 */ 088 CANCELLED, 089 /** 090 * added to help the parsers 091 */ 092 NULL; 093 094 public static NutritionOrderStatus fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("proposed".equals(codeString)) 098 return PROPOSED; 099 if ("draft".equals(codeString)) 100 return DRAFT; 101 if ("planned".equals(codeString)) 102 return PLANNED; 103 if ("requested".equals(codeString)) 104 return REQUESTED; 105 if ("active".equals(codeString)) 106 return ACTIVE; 107 if ("on-hold".equals(codeString)) 108 return ONHOLD; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("cancelled".equals(codeString)) 112 return CANCELLED; 113 throw new FHIRException("Unknown NutritionOrderStatus code '" + codeString + "'"); 114 } 115 116 public String toCode() { 117 switch (this) { 118 case PROPOSED: 119 return "proposed"; 120 case DRAFT: 121 return "draft"; 122 case PLANNED: 123 return "planned"; 124 case REQUESTED: 125 return "requested"; 126 case ACTIVE: 127 return "active"; 128 case ONHOLD: 129 return "on-hold"; 130 case COMPLETED: 131 return "completed"; 132 case CANCELLED: 133 return "cancelled"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getSystem() { 142 switch (this) { 143 case PROPOSED: 144 return "http://hl7.org/fhir/nutrition-order-status"; 145 case DRAFT: 146 return "http://hl7.org/fhir/nutrition-order-status"; 147 case PLANNED: 148 return "http://hl7.org/fhir/nutrition-order-status"; 149 case REQUESTED: 150 return "http://hl7.org/fhir/nutrition-order-status"; 151 case ACTIVE: 152 return "http://hl7.org/fhir/nutrition-order-status"; 153 case ONHOLD: 154 return "http://hl7.org/fhir/nutrition-order-status"; 155 case COMPLETED: 156 return "http://hl7.org/fhir/nutrition-order-status"; 157 case CANCELLED: 158 return "http://hl7.org/fhir/nutrition-order-status"; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 166 public String getDefinition() { 167 switch (this) { 168 case PROPOSED: 169 return "The request has been proposed."; 170 case DRAFT: 171 return "The request is in preliminary form prior to being sent."; 172 case PLANNED: 173 return "The request has been planned."; 174 case REQUESTED: 175 return "The request has been placed."; 176 case ACTIVE: 177 return "The request is 'actionable', but not all actions that are implied by it have occurred yet."; 178 case ONHOLD: 179 return "Actions implied by the request have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 180 case COMPLETED: 181 return "All actions that are implied by the order have occurred and no continuation is planned (this will rarely be made explicit)."; 182 case CANCELLED: 183 return "The request has been withdrawn and is no longer actionable."; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getDisplay() { 192 switch (this) { 193 case PROPOSED: 194 return "Proposed"; 195 case DRAFT: 196 return "Draft"; 197 case PLANNED: 198 return "Planned"; 199 case REQUESTED: 200 return "Requested"; 201 case ACTIVE: 202 return "Active"; 203 case ONHOLD: 204 return "On-Hold"; 205 case COMPLETED: 206 return "Completed"; 207 case CANCELLED: 208 return "Cancelled"; 209 case NULL: 210 return null; 211 default: 212 return "?"; 213 } 214 } 215 } 216 217 public static class NutritionOrderStatusEnumFactory implements EnumFactory<NutritionOrderStatus> { 218 public NutritionOrderStatus fromCode(String codeString) throws IllegalArgumentException { 219 if (codeString == null || "".equals(codeString)) 220 if (codeString == null || "".equals(codeString)) 221 return null; 222 if ("proposed".equals(codeString)) 223 return NutritionOrderStatus.PROPOSED; 224 if ("draft".equals(codeString)) 225 return NutritionOrderStatus.DRAFT; 226 if ("planned".equals(codeString)) 227 return NutritionOrderStatus.PLANNED; 228 if ("requested".equals(codeString)) 229 return NutritionOrderStatus.REQUESTED; 230 if ("active".equals(codeString)) 231 return NutritionOrderStatus.ACTIVE; 232 if ("on-hold".equals(codeString)) 233 return NutritionOrderStatus.ONHOLD; 234 if ("completed".equals(codeString)) 235 return NutritionOrderStatus.COMPLETED; 236 if ("cancelled".equals(codeString)) 237 return NutritionOrderStatus.CANCELLED; 238 throw new IllegalArgumentException("Unknown NutritionOrderStatus code '" + codeString + "'"); 239 } 240 241 public Enumeration<NutritionOrderStatus> fromType(Base code) throws FHIRException { 242 if (code == null || code.isEmpty()) 243 return null; 244 String codeString = ((PrimitiveType) code).asStringValue(); 245 if (codeString == null || "".equals(codeString)) 246 return null; 247 if ("proposed".equals(codeString)) 248 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.PROPOSED); 249 if ("draft".equals(codeString)) 250 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.DRAFT); 251 if ("planned".equals(codeString)) 252 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.PLANNED); 253 if ("requested".equals(codeString)) 254 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.REQUESTED); 255 if ("active".equals(codeString)) 256 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ACTIVE); 257 if ("on-hold".equals(codeString)) 258 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ONHOLD); 259 if ("completed".equals(codeString)) 260 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.COMPLETED); 261 if ("cancelled".equals(codeString)) 262 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.CANCELLED); 263 throw new FHIRException("Unknown NutritionOrderStatus code '" + codeString + "'"); 264 } 265 266 public String toCode(NutritionOrderStatus code) { 267 if (code == NutritionOrderStatus.PROPOSED) 268 return "proposed"; 269 if (code == NutritionOrderStatus.DRAFT) 270 return "draft"; 271 if (code == NutritionOrderStatus.PLANNED) 272 return "planned"; 273 if (code == NutritionOrderStatus.REQUESTED) 274 return "requested"; 275 if (code == NutritionOrderStatus.ACTIVE) 276 return "active"; 277 if (code == NutritionOrderStatus.ONHOLD) 278 return "on-hold"; 279 if (code == NutritionOrderStatus.COMPLETED) 280 return "completed"; 281 if (code == NutritionOrderStatus.CANCELLED) 282 return "cancelled"; 283 return "?"; 284 } 285 } 286 287 @Block() 288 public static class NutritionOrderOralDietComponent extends BackboneElement implements IBaseBackboneElement { 289 /** 290 * The kind of diet or dietary restriction such as fiber restricted diet or 291 * diabetic diet. 292 */ 293 @Child(name = "type", type = { 294 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 295 @Description(shortDefinition = "Type of oral diet or diet restrictions that describe what can be consumed orally", formalDefinition = "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.") 296 protected List<CodeableConcept> type; 297 298 /** 299 * The time period and frequency at which the diet should be given. 300 */ 301 @Child(name = "schedule", type = { 302 Timing.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 303 @Description(shortDefinition = "Scheduled frequency of diet", formalDefinition = "The time period and frequency at which the diet should be given.") 304 protected List<Timing> schedule; 305 306 /** 307 * Class that defines the quantity and type of nutrient modifications required 308 * for the oral diet. 309 */ 310 @Child(name = "nutrient", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 311 @Description(shortDefinition = "Required nutrient modifications", formalDefinition = "Class that defines the quantity and type of nutrient modifications required for the oral diet.") 312 protected List<NutritionOrderOralDietNutrientComponent> nutrient; 313 314 /** 315 * Class that describes any texture modifications required for the patient to 316 * safely consume various types of solid foods. 317 */ 318 @Child(name = "texture", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 319 @Description(shortDefinition = "Required texture modifications", formalDefinition = "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.") 320 protected List<NutritionOrderOralDietTextureComponent> texture; 321 322 /** 323 * The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) 324 * of liquids or fluids served to the patient. 325 */ 326 @Child(name = "fluidConsistencyType", type = { 327 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 328 @Description(shortDefinition = "The required consistency of fluids and liquids provided to the patient", formalDefinition = "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.") 329 protected List<CodeableConcept> fluidConsistencyType; 330 331 /** 332 * Free text or additional instructions or information pertaining to the oral 333 * diet. 334 */ 335 @Child(name = "instruction", type = { 336 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 337 @Description(shortDefinition = "Instructions or additional information about the oral diet", formalDefinition = "Free text or additional instructions or information pertaining to the oral diet.") 338 protected StringType instruction; 339 340 private static final long serialVersionUID = 973058412L; 341 342 /* 343 * Constructor 344 */ 345 public NutritionOrderOralDietComponent() { 346 super(); 347 } 348 349 /** 350 * @return {@link #type} (The kind of diet or dietary restriction such as fiber 351 * restricted diet or diabetic diet.) 352 */ 353 public List<CodeableConcept> getType() { 354 if (this.type == null) 355 this.type = new ArrayList<CodeableConcept>(); 356 return this.type; 357 } 358 359 public boolean hasType() { 360 if (this.type == null) 361 return false; 362 for (CodeableConcept item : this.type) 363 if (!item.isEmpty()) 364 return true; 365 return false; 366 } 367 368 /** 369 * @return {@link #type} (The kind of diet or dietary restriction such as fiber 370 * restricted diet or diabetic diet.) 371 */ 372 // syntactic sugar 373 public CodeableConcept addType() { // 3 374 CodeableConcept t = new CodeableConcept(); 375 if (this.type == null) 376 this.type = new ArrayList<CodeableConcept>(); 377 this.type.add(t); 378 return t; 379 } 380 381 // syntactic sugar 382 public NutritionOrderOralDietComponent addType(CodeableConcept t) { // 3 383 if (t == null) 384 return this; 385 if (this.type == null) 386 this.type = new ArrayList<CodeableConcept>(); 387 this.type.add(t); 388 return this; 389 } 390 391 /** 392 * @return {@link #schedule} (The time period and frequency at which the diet 393 * should be given.) 394 */ 395 public List<Timing> getSchedule() { 396 if (this.schedule == null) 397 this.schedule = new ArrayList<Timing>(); 398 return this.schedule; 399 } 400 401 public boolean hasSchedule() { 402 if (this.schedule == null) 403 return false; 404 for (Timing item : this.schedule) 405 if (!item.isEmpty()) 406 return true; 407 return false; 408 } 409 410 /** 411 * @return {@link #schedule} (The time period and frequency at which the diet 412 * should be given.) 413 */ 414 // syntactic sugar 415 public Timing addSchedule() { // 3 416 Timing t = new Timing(); 417 if (this.schedule == null) 418 this.schedule = new ArrayList<Timing>(); 419 this.schedule.add(t); 420 return t; 421 } 422 423 // syntactic sugar 424 public NutritionOrderOralDietComponent addSchedule(Timing t) { // 3 425 if (t == null) 426 return this; 427 if (this.schedule == null) 428 this.schedule = new ArrayList<Timing>(); 429 this.schedule.add(t); 430 return this; 431 } 432 433 /** 434 * @return {@link #nutrient} (Class that defines the quantity and type of 435 * nutrient modifications required for the oral diet.) 436 */ 437 public List<NutritionOrderOralDietNutrientComponent> getNutrient() { 438 if (this.nutrient == null) 439 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 440 return this.nutrient; 441 } 442 443 public boolean hasNutrient() { 444 if (this.nutrient == null) 445 return false; 446 for (NutritionOrderOralDietNutrientComponent item : this.nutrient) 447 if (!item.isEmpty()) 448 return true; 449 return false; 450 } 451 452 /** 453 * @return {@link #nutrient} (Class that defines the quantity and type of 454 * nutrient modifications required for the oral diet.) 455 */ 456 // syntactic sugar 457 public NutritionOrderOralDietNutrientComponent addNutrient() { // 3 458 NutritionOrderOralDietNutrientComponent t = new NutritionOrderOralDietNutrientComponent(); 459 if (this.nutrient == null) 460 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 461 this.nutrient.add(t); 462 return t; 463 } 464 465 // syntactic sugar 466 public NutritionOrderOralDietComponent addNutrient(NutritionOrderOralDietNutrientComponent t) { // 3 467 if (t == null) 468 return this; 469 if (this.nutrient == null) 470 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 471 this.nutrient.add(t); 472 return this; 473 } 474 475 /** 476 * @return {@link #texture} (Class that describes any texture modifications 477 * required for the patient to safely consume various types of solid 478 * foods.) 479 */ 480 public List<NutritionOrderOralDietTextureComponent> getTexture() { 481 if (this.texture == null) 482 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 483 return this.texture; 484 } 485 486 public boolean hasTexture() { 487 if (this.texture == null) 488 return false; 489 for (NutritionOrderOralDietTextureComponent item : this.texture) 490 if (!item.isEmpty()) 491 return true; 492 return false; 493 } 494 495 /** 496 * @return {@link #texture} (Class that describes any texture modifications 497 * required for the patient to safely consume various types of solid 498 * foods.) 499 */ 500 // syntactic sugar 501 public NutritionOrderOralDietTextureComponent addTexture() { // 3 502 NutritionOrderOralDietTextureComponent t = new NutritionOrderOralDietTextureComponent(); 503 if (this.texture == null) 504 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 505 this.texture.add(t); 506 return t; 507 } 508 509 // syntactic sugar 510 public NutritionOrderOralDietComponent addTexture(NutritionOrderOralDietTextureComponent t) { // 3 511 if (t == null) 512 return this; 513 if (this.texture == null) 514 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 515 this.texture.add(t); 516 return this; 517 } 518 519 /** 520 * @return {@link #fluidConsistencyType} (The required consistency (e.g. 521 * honey-thick, nectar-thick, thin, thickened.) of liquids or fluids 522 * served to the patient.) 523 */ 524 public List<CodeableConcept> getFluidConsistencyType() { 525 if (this.fluidConsistencyType == null) 526 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 527 return this.fluidConsistencyType; 528 } 529 530 public boolean hasFluidConsistencyType() { 531 if (this.fluidConsistencyType == null) 532 return false; 533 for (CodeableConcept item : this.fluidConsistencyType) 534 if (!item.isEmpty()) 535 return true; 536 return false; 537 } 538 539 /** 540 * @return {@link #fluidConsistencyType} (The required consistency (e.g. 541 * honey-thick, nectar-thick, thin, thickened.) of liquids or fluids 542 * served to the patient.) 543 */ 544 // syntactic sugar 545 public CodeableConcept addFluidConsistencyType() { // 3 546 CodeableConcept t = new CodeableConcept(); 547 if (this.fluidConsistencyType == null) 548 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 549 this.fluidConsistencyType.add(t); 550 return t; 551 } 552 553 // syntactic sugar 554 public NutritionOrderOralDietComponent addFluidConsistencyType(CodeableConcept t) { // 3 555 if (t == null) 556 return this; 557 if (this.fluidConsistencyType == null) 558 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 559 this.fluidConsistencyType.add(t); 560 return this; 561 } 562 563 /** 564 * @return {@link #instruction} (Free text or additional instructions or 565 * information pertaining to the oral diet.). This is the underlying 566 * object with id, value and extensions. The accessor "getInstruction" 567 * gives direct access to the value 568 */ 569 public StringType getInstructionElement() { 570 if (this.instruction == null) 571 if (Configuration.errorOnAutoCreate()) 572 throw new Error("Attempt to auto-create NutritionOrderOralDietComponent.instruction"); 573 else if (Configuration.doAutoCreate()) 574 this.instruction = new StringType(); // bb 575 return this.instruction; 576 } 577 578 public boolean hasInstructionElement() { 579 return this.instruction != null && !this.instruction.isEmpty(); 580 } 581 582 public boolean hasInstruction() { 583 return this.instruction != null && !this.instruction.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #instruction} (Free text or additional instructions or 588 * information pertaining to the oral diet.). This is the 589 * underlying object with id, value and extensions. The accessor 590 * "getInstruction" gives direct access to the value 591 */ 592 public NutritionOrderOralDietComponent setInstructionElement(StringType value) { 593 this.instruction = value; 594 return this; 595 } 596 597 /** 598 * @return Free text or additional instructions or information pertaining to the 599 * oral diet. 600 */ 601 public String getInstruction() { 602 return this.instruction == null ? null : this.instruction.getValue(); 603 } 604 605 /** 606 * @param value Free text or additional instructions or information pertaining 607 * to the oral diet. 608 */ 609 public NutritionOrderOralDietComponent setInstruction(String value) { 610 if (Utilities.noString(value)) 611 this.instruction = null; 612 else { 613 if (this.instruction == null) 614 this.instruction = new StringType(); 615 this.instruction.setValue(value); 616 } 617 return this; 618 } 619 620 protected void listChildren(List<Property> childrenList) { 621 super.listChildren(childrenList); 622 childrenList.add(new Property("type", "CodeableConcept", 623 "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, 624 java.lang.Integer.MAX_VALUE, type)); 625 childrenList 626 .add(new Property("schedule", "Timing", "The time period and frequency at which the diet should be given.", 0, 627 java.lang.Integer.MAX_VALUE, schedule)); 628 childrenList.add(new Property("nutrient", "", 629 "Class that defines the quantity and type of nutrient modifications required for the oral diet.", 0, 630 java.lang.Integer.MAX_VALUE, nutrient)); 631 childrenList.add(new Property("texture", "", 632 "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 633 0, java.lang.Integer.MAX_VALUE, texture)); 634 childrenList.add(new Property("fluidConsistencyType", "CodeableConcept", 635 "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 636 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType)); 637 childrenList.add(new Property("instruction", "string", 638 "Free text or additional instructions or information pertaining to the oral diet.", 0, 639 java.lang.Integer.MAX_VALUE, instruction)); 640 } 641 642 @Override 643 public void setProperty(String name, Base value) throws FHIRException { 644 if (name.equals("type")) 645 this.getType().add(castToCodeableConcept(value)); 646 else if (name.equals("schedule")) 647 this.getSchedule().add(castToTiming(value)); 648 else if (name.equals("nutrient")) 649 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); 650 else if (name.equals("texture")) 651 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); 652 else if (name.equals("fluidConsistencyType")) 653 this.getFluidConsistencyType().add(castToCodeableConcept(value)); 654 else if (name.equals("instruction")) 655 this.instruction = castToString(value); // StringType 656 else 657 super.setProperty(name, value); 658 } 659 660 @Override 661 public Base addChild(String name) throws FHIRException { 662 if (name.equals("type")) { 663 return addType(); 664 } else if (name.equals("schedule")) { 665 return addSchedule(); 666 } else if (name.equals("nutrient")) { 667 return addNutrient(); 668 } else if (name.equals("texture")) { 669 return addTexture(); 670 } else if (name.equals("fluidConsistencyType")) { 671 return addFluidConsistencyType(); 672 } else if (name.equals("instruction")) { 673 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 674 } else 675 return super.addChild(name); 676 } 677 678 public NutritionOrderOralDietComponent copy() { 679 NutritionOrderOralDietComponent dst = new NutritionOrderOralDietComponent(); 680 copyValues(dst); 681 if (type != null) { 682 dst.type = new ArrayList<CodeableConcept>(); 683 for (CodeableConcept i : type) 684 dst.type.add(i.copy()); 685 } 686 ; 687 if (schedule != null) { 688 dst.schedule = new ArrayList<Timing>(); 689 for (Timing i : schedule) 690 dst.schedule.add(i.copy()); 691 } 692 ; 693 if (nutrient != null) { 694 dst.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 695 for (NutritionOrderOralDietNutrientComponent i : nutrient) 696 dst.nutrient.add(i.copy()); 697 } 698 ; 699 if (texture != null) { 700 dst.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 701 for (NutritionOrderOralDietTextureComponent i : texture) 702 dst.texture.add(i.copy()); 703 } 704 ; 705 if (fluidConsistencyType != null) { 706 dst.fluidConsistencyType = new ArrayList<CodeableConcept>(); 707 for (CodeableConcept i : fluidConsistencyType) 708 dst.fluidConsistencyType.add(i.copy()); 709 } 710 ; 711 dst.instruction = instruction == null ? null : instruction.copy(); 712 return dst; 713 } 714 715 @Override 716 public boolean equalsDeep(Base other) { 717 if (!super.equalsDeep(other)) 718 return false; 719 if (!(other instanceof NutritionOrderOralDietComponent)) 720 return false; 721 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other; 722 return compareDeep(type, o.type, true) && compareDeep(schedule, o.schedule, true) 723 && compareDeep(nutrient, o.nutrient, true) && compareDeep(texture, o.texture, true) 724 && compareDeep(fluidConsistencyType, o.fluidConsistencyType, true) 725 && compareDeep(instruction, o.instruction, true); 726 } 727 728 @Override 729 public boolean equalsShallow(Base other) { 730 if (!super.equalsShallow(other)) 731 return false; 732 if (!(other instanceof NutritionOrderOralDietComponent)) 733 return false; 734 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other; 735 return compareValues(instruction, o.instruction, true); 736 } 737 738 public boolean isEmpty() { 739 return super.isEmpty() && (type == null || type.isEmpty()) && (schedule == null || schedule.isEmpty()) 740 && (nutrient == null || nutrient.isEmpty()) && (texture == null || texture.isEmpty()) 741 && (fluidConsistencyType == null || fluidConsistencyType.isEmpty()) 742 && (instruction == null || instruction.isEmpty()); 743 } 744 745 public String fhirType() { 746 return "NutritionOrder.oralDiet"; 747 748 } 749 750 } 751 752 @Block() 753 public static class NutritionOrderOralDietNutrientComponent extends BackboneElement implements IBaseBackboneElement { 754 /** 755 * The nutrient that is being modified such as carbohydrate or sodium. 756 */ 757 @Child(name = "modifier", type = { 758 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 759 @Description(shortDefinition = "Type of nutrient that is being modified", formalDefinition = "The nutrient that is being modified such as carbohydrate or sodium.") 760 protected CodeableConcept modifier; 761 762 /** 763 * The quantity of the specified nutrient to include in diet. 764 */ 765 @Child(name = "amount", type = { 766 SimpleQuantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 767 @Description(shortDefinition = "Quantity of the specified nutrient", formalDefinition = "The quantity of the specified nutrient to include in diet.") 768 protected SimpleQuantity amount; 769 770 private static final long serialVersionUID = 465107295L; 771 772 /* 773 * Constructor 774 */ 775 public NutritionOrderOralDietNutrientComponent() { 776 super(); 777 } 778 779 /** 780 * @return {@link #modifier} (The nutrient that is being modified such as 781 * carbohydrate or sodium.) 782 */ 783 public CodeableConcept getModifier() { 784 if (this.modifier == null) 785 if (Configuration.errorOnAutoCreate()) 786 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.modifier"); 787 else if (Configuration.doAutoCreate()) 788 this.modifier = new CodeableConcept(); // cc 789 return this.modifier; 790 } 791 792 public boolean hasModifier() { 793 return this.modifier != null && !this.modifier.isEmpty(); 794 } 795 796 /** 797 * @param value {@link #modifier} (The nutrient that is being modified such as 798 * carbohydrate or sodium.) 799 */ 800 public NutritionOrderOralDietNutrientComponent setModifier(CodeableConcept value) { 801 this.modifier = value; 802 return this; 803 } 804 805 /** 806 * @return {@link #amount} (The quantity of the specified nutrient to include in 807 * diet.) 808 */ 809 public SimpleQuantity getAmount() { 810 if (this.amount == null) 811 if (Configuration.errorOnAutoCreate()) 812 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.amount"); 813 else if (Configuration.doAutoCreate()) 814 this.amount = new SimpleQuantity(); // cc 815 return this.amount; 816 } 817 818 public boolean hasAmount() { 819 return this.amount != null && !this.amount.isEmpty(); 820 } 821 822 /** 823 * @param value {@link #amount} (The quantity of the specified nutrient to 824 * include in diet.) 825 */ 826 public NutritionOrderOralDietNutrientComponent setAmount(SimpleQuantity value) { 827 this.amount = value; 828 return this; 829 } 830 831 protected void listChildren(List<Property> childrenList) { 832 super.listChildren(childrenList); 833 childrenList.add(new Property("modifier", "CodeableConcept", 834 "The nutrient that is being modified such as carbohydrate or sodium.", 0, java.lang.Integer.MAX_VALUE, 835 modifier)); 836 childrenList.add(new Property("amount", "SimpleQuantity", 837 "The quantity of the specified nutrient to include in diet.", 0, java.lang.Integer.MAX_VALUE, amount)); 838 } 839 840 @Override 841 public void setProperty(String name, Base value) throws FHIRException { 842 if (name.equals("modifier")) 843 this.modifier = castToCodeableConcept(value); // CodeableConcept 844 else if (name.equals("amount")) 845 this.amount = castToSimpleQuantity(value); // SimpleQuantity 846 else 847 super.setProperty(name, value); 848 } 849 850 @Override 851 public Base addChild(String name) throws FHIRException { 852 if (name.equals("modifier")) { 853 this.modifier = new CodeableConcept(); 854 return this.modifier; 855 } else if (name.equals("amount")) { 856 this.amount = new SimpleQuantity(); 857 return this.amount; 858 } else 859 return super.addChild(name); 860 } 861 862 public NutritionOrderOralDietNutrientComponent copy() { 863 NutritionOrderOralDietNutrientComponent dst = new NutritionOrderOralDietNutrientComponent(); 864 copyValues(dst); 865 dst.modifier = modifier == null ? null : modifier.copy(); 866 dst.amount = amount == null ? null : amount.copy(); 867 return dst; 868 } 869 870 @Override 871 public boolean equalsDeep(Base other) { 872 if (!super.equalsDeep(other)) 873 return false; 874 if (!(other instanceof NutritionOrderOralDietNutrientComponent)) 875 return false; 876 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other; 877 return compareDeep(modifier, o.modifier, true) && compareDeep(amount, o.amount, true); 878 } 879 880 @Override 881 public boolean equalsShallow(Base other) { 882 if (!super.equalsShallow(other)) 883 return false; 884 if (!(other instanceof NutritionOrderOralDietNutrientComponent)) 885 return false; 886 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other; 887 return true; 888 } 889 890 public boolean isEmpty() { 891 return super.isEmpty() && (modifier == null || modifier.isEmpty()) && (amount == null || amount.isEmpty()); 892 } 893 894 public String fhirType() { 895 return "NutritionOrder.oralDiet.nutrient"; 896 897 } 898 899 } 900 901 @Block() 902 public static class NutritionOrderOralDietTextureComponent extends BackboneElement implements IBaseBackboneElement { 903 /** 904 * Any texture modifications (for solid foods) that should be made, e.g. easy to 905 * chew, chopped, ground, and pureed. 906 */ 907 @Child(name = "modifier", type = { 908 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 909 @Description(shortDefinition = "Code to indicate how to alter the texture of the foods, e.g. pureed", formalDefinition = "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.") 910 protected CodeableConcept modifier; 911 912 /** 913 * The food type(s) (e.g. meats, all foods) that the texture modification 914 * applies to. This could be all foods types. 915 */ 916 @Child(name = "foodType", type = { 917 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 918 @Description(shortDefinition = "Concepts that are used to identify an entity that is ingested for nutritional purposes", formalDefinition = "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.") 919 protected CodeableConcept foodType; 920 921 private static final long serialVersionUID = -56402817L; 922 923 /* 924 * Constructor 925 */ 926 public NutritionOrderOralDietTextureComponent() { 927 super(); 928 } 929 930 /** 931 * @return {@link #modifier} (Any texture modifications (for solid foods) that 932 * should be made, e.g. easy to chew, chopped, ground, and pureed.) 933 */ 934 public CodeableConcept getModifier() { 935 if (this.modifier == null) 936 if (Configuration.errorOnAutoCreate()) 937 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.modifier"); 938 else if (Configuration.doAutoCreate()) 939 this.modifier = new CodeableConcept(); // cc 940 return this.modifier; 941 } 942 943 public boolean hasModifier() { 944 return this.modifier != null && !this.modifier.isEmpty(); 945 } 946 947 /** 948 * @param value {@link #modifier} (Any texture modifications (for solid foods) 949 * that should be made, e.g. easy to chew, chopped, ground, and 950 * pureed.) 951 */ 952 public NutritionOrderOralDietTextureComponent setModifier(CodeableConcept value) { 953 this.modifier = value; 954 return this; 955 } 956 957 /** 958 * @return {@link #foodType} (The food type(s) (e.g. meats, all foods) that the 959 * texture modification applies to. This could be all foods types.) 960 */ 961 public CodeableConcept getFoodType() { 962 if (this.foodType == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.foodType"); 965 else if (Configuration.doAutoCreate()) 966 this.foodType = new CodeableConcept(); // cc 967 return this.foodType; 968 } 969 970 public boolean hasFoodType() { 971 return this.foodType != null && !this.foodType.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #foodType} (The food type(s) (e.g. meats, all foods) that 976 * the texture modification applies to. This could be all foods 977 * types.) 978 */ 979 public NutritionOrderOralDietTextureComponent setFoodType(CodeableConcept value) { 980 this.foodType = value; 981 return this; 982 } 983 984 protected void listChildren(List<Property> childrenList) { 985 super.listChildren(childrenList); 986 childrenList.add(new Property("modifier", "CodeableConcept", 987 "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 988 0, java.lang.Integer.MAX_VALUE, modifier)); 989 childrenList.add(new Property("foodType", "CodeableConcept", 990 "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 991 0, java.lang.Integer.MAX_VALUE, foodType)); 992 } 993 994 @Override 995 public void setProperty(String name, Base value) throws FHIRException { 996 if (name.equals("modifier")) 997 this.modifier = castToCodeableConcept(value); // CodeableConcept 998 else if (name.equals("foodType")) 999 this.foodType = castToCodeableConcept(value); // CodeableConcept 1000 else 1001 super.setProperty(name, value); 1002 } 1003 1004 @Override 1005 public Base addChild(String name) throws FHIRException { 1006 if (name.equals("modifier")) { 1007 this.modifier = new CodeableConcept(); 1008 return this.modifier; 1009 } else if (name.equals("foodType")) { 1010 this.foodType = new CodeableConcept(); 1011 return this.foodType; 1012 } else 1013 return super.addChild(name); 1014 } 1015 1016 public NutritionOrderOralDietTextureComponent copy() { 1017 NutritionOrderOralDietTextureComponent dst = new NutritionOrderOralDietTextureComponent(); 1018 copyValues(dst); 1019 dst.modifier = modifier == null ? null : modifier.copy(); 1020 dst.foodType = foodType == null ? null : foodType.copy(); 1021 return dst; 1022 } 1023 1024 @Override 1025 public boolean equalsDeep(Base other) { 1026 if (!super.equalsDeep(other)) 1027 return false; 1028 if (!(other instanceof NutritionOrderOralDietTextureComponent)) 1029 return false; 1030 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other; 1031 return compareDeep(modifier, o.modifier, true) && compareDeep(foodType, o.foodType, true); 1032 } 1033 1034 @Override 1035 public boolean equalsShallow(Base other) { 1036 if (!super.equalsShallow(other)) 1037 return false; 1038 if (!(other instanceof NutritionOrderOralDietTextureComponent)) 1039 return false; 1040 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other; 1041 return true; 1042 } 1043 1044 public boolean isEmpty() { 1045 return super.isEmpty() && (modifier == null || modifier.isEmpty()) && (foodType == null || foodType.isEmpty()); 1046 } 1047 1048 public String fhirType() { 1049 return "NutritionOrder.oralDiet.texture"; 1050 1051 } 1052 1053 } 1054 1055 @Block() 1056 public static class NutritionOrderSupplementComponent extends BackboneElement implements IBaseBackboneElement { 1057 /** 1058 * The kind of nutritional supplement product required such as a high protein or 1059 * pediatric clear liquid supplement. 1060 */ 1061 @Child(name = "type", type = { 1062 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1063 @Description(shortDefinition = "Type of supplement product requested", formalDefinition = "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.") 1064 protected CodeableConcept type; 1065 1066 /** 1067 * The product or brand name of the nutritional supplement such as "Acme Protein 1068 * Shake". 1069 */ 1070 @Child(name = "productName", type = { 1071 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1072 @Description(shortDefinition = "Product or brand name of the nutritional supplement", formalDefinition = "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".") 1073 protected StringType productName; 1074 1075 /** 1076 * The time period and frequency at which the supplement(s) should be given. 1077 */ 1078 @Child(name = "schedule", type = { 1079 Timing.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1080 @Description(shortDefinition = "Scheduled frequency of supplement", formalDefinition = "The time period and frequency at which the supplement(s) should be given.") 1081 protected List<Timing> schedule; 1082 1083 /** 1084 * The amount of the nutritional supplement to be given. 1085 */ 1086 @Child(name = "quantity", type = { 1087 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1088 @Description(shortDefinition = "Amount of the nutritional supplement", formalDefinition = "The amount of the nutritional supplement to be given.") 1089 protected SimpleQuantity quantity; 1090 1091 /** 1092 * Free text or additional instructions or information pertaining to the oral 1093 * supplement. 1094 */ 1095 @Child(name = "instruction", type = { 1096 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1097 @Description(shortDefinition = "Instructions or additional information about the oral supplement", formalDefinition = "Free text or additional instructions or information pertaining to the oral supplement.") 1098 protected StringType instruction; 1099 1100 private static final long serialVersionUID = 297545236L; 1101 1102 /* 1103 * Constructor 1104 */ 1105 public NutritionOrderSupplementComponent() { 1106 super(); 1107 } 1108 1109 /** 1110 * @return {@link #type} (The kind of nutritional supplement product required 1111 * such as a high protein or pediatric clear liquid supplement.) 1112 */ 1113 public CodeableConcept getType() { 1114 if (this.type == null) 1115 if (Configuration.errorOnAutoCreate()) 1116 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.type"); 1117 else if (Configuration.doAutoCreate()) 1118 this.type = new CodeableConcept(); // cc 1119 return this.type; 1120 } 1121 1122 public boolean hasType() { 1123 return this.type != null && !this.type.isEmpty(); 1124 } 1125 1126 /** 1127 * @param value {@link #type} (The kind of nutritional supplement product 1128 * required such as a high protein or pediatric clear liquid 1129 * supplement.) 1130 */ 1131 public NutritionOrderSupplementComponent setType(CodeableConcept value) { 1132 this.type = value; 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #productName} (The product or brand name of the nutritional 1138 * supplement such as "Acme Protein Shake".). This is the underlying 1139 * object with id, value and extensions. The accessor "getProductName" 1140 * gives direct access to the value 1141 */ 1142 public StringType getProductNameElement() { 1143 if (this.productName == null) 1144 if (Configuration.errorOnAutoCreate()) 1145 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.productName"); 1146 else if (Configuration.doAutoCreate()) 1147 this.productName = new StringType(); // bb 1148 return this.productName; 1149 } 1150 1151 public boolean hasProductNameElement() { 1152 return this.productName != null && !this.productName.isEmpty(); 1153 } 1154 1155 public boolean hasProductName() { 1156 return this.productName != null && !this.productName.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #productName} (The product or brand name of the 1161 * nutritional supplement such as "Acme Protein Shake".). This is 1162 * the underlying object with id, value and extensions. The 1163 * accessor "getProductName" gives direct access to the value 1164 */ 1165 public NutritionOrderSupplementComponent setProductNameElement(StringType value) { 1166 this.productName = value; 1167 return this; 1168 } 1169 1170 /** 1171 * @return The product or brand name of the nutritional supplement such as "Acme 1172 * Protein Shake". 1173 */ 1174 public String getProductName() { 1175 return this.productName == null ? null : this.productName.getValue(); 1176 } 1177 1178 /** 1179 * @param value The product or brand name of the nutritional supplement such as 1180 * "Acme Protein Shake". 1181 */ 1182 public NutritionOrderSupplementComponent setProductName(String value) { 1183 if (Utilities.noString(value)) 1184 this.productName = null; 1185 else { 1186 if (this.productName == null) 1187 this.productName = new StringType(); 1188 this.productName.setValue(value); 1189 } 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #schedule} (The time period and frequency at which the 1195 * supplement(s) should be given.) 1196 */ 1197 public List<Timing> getSchedule() { 1198 if (this.schedule == null) 1199 this.schedule = new ArrayList<Timing>(); 1200 return this.schedule; 1201 } 1202 1203 public boolean hasSchedule() { 1204 if (this.schedule == null) 1205 return false; 1206 for (Timing item : this.schedule) 1207 if (!item.isEmpty()) 1208 return true; 1209 return false; 1210 } 1211 1212 /** 1213 * @return {@link #schedule} (The time period and frequency at which the 1214 * supplement(s) should be given.) 1215 */ 1216 // syntactic sugar 1217 public Timing addSchedule() { // 3 1218 Timing t = new Timing(); 1219 if (this.schedule == null) 1220 this.schedule = new ArrayList<Timing>(); 1221 this.schedule.add(t); 1222 return t; 1223 } 1224 1225 // syntactic sugar 1226 public NutritionOrderSupplementComponent addSchedule(Timing t) { // 3 1227 if (t == null) 1228 return this; 1229 if (this.schedule == null) 1230 this.schedule = new ArrayList<Timing>(); 1231 this.schedule.add(t); 1232 return this; 1233 } 1234 1235 /** 1236 * @return {@link #quantity} (The amount of the nutritional supplement to be 1237 * given.) 1238 */ 1239 public SimpleQuantity getQuantity() { 1240 if (this.quantity == null) 1241 if (Configuration.errorOnAutoCreate()) 1242 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.quantity"); 1243 else if (Configuration.doAutoCreate()) 1244 this.quantity = new SimpleQuantity(); // cc 1245 return this.quantity; 1246 } 1247 1248 public boolean hasQuantity() { 1249 return this.quantity != null && !this.quantity.isEmpty(); 1250 } 1251 1252 /** 1253 * @param value {@link #quantity} (The amount of the nutritional supplement to 1254 * be given.) 1255 */ 1256 public NutritionOrderSupplementComponent setQuantity(SimpleQuantity value) { 1257 this.quantity = value; 1258 return this; 1259 } 1260 1261 /** 1262 * @return {@link #instruction} (Free text or additional instructions or 1263 * information pertaining to the oral supplement.). This is the 1264 * underlying object with id, value and extensions. The accessor 1265 * "getInstruction" gives direct access to the value 1266 */ 1267 public StringType getInstructionElement() { 1268 if (this.instruction == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.instruction"); 1271 else if (Configuration.doAutoCreate()) 1272 this.instruction = new StringType(); // bb 1273 return this.instruction; 1274 } 1275 1276 public boolean hasInstructionElement() { 1277 return this.instruction != null && !this.instruction.isEmpty(); 1278 } 1279 1280 public boolean hasInstruction() { 1281 return this.instruction != null && !this.instruction.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #instruction} (Free text or additional instructions or 1286 * information pertaining to the oral supplement.). This is the 1287 * underlying object with id, value and extensions. The accessor 1288 * "getInstruction" gives direct access to the value 1289 */ 1290 public NutritionOrderSupplementComponent setInstructionElement(StringType value) { 1291 this.instruction = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return Free text or additional instructions or information pertaining to the 1297 * oral supplement. 1298 */ 1299 public String getInstruction() { 1300 return this.instruction == null ? null : this.instruction.getValue(); 1301 } 1302 1303 /** 1304 * @param value Free text or additional instructions or information pertaining 1305 * to the oral supplement. 1306 */ 1307 public NutritionOrderSupplementComponent setInstruction(String value) { 1308 if (Utilities.noString(value)) 1309 this.instruction = null; 1310 else { 1311 if (this.instruction == null) 1312 this.instruction = new StringType(); 1313 this.instruction.setValue(value); 1314 } 1315 return this; 1316 } 1317 1318 protected void listChildren(List<Property> childrenList) { 1319 super.listChildren(childrenList); 1320 childrenList.add(new Property("type", "CodeableConcept", 1321 "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 1322 0, java.lang.Integer.MAX_VALUE, type)); 1323 childrenList.add(new Property("productName", "string", 1324 "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1325 java.lang.Integer.MAX_VALUE, productName)); 1326 childrenList.add(new Property("schedule", "Timing", 1327 "The time period and frequency at which the supplement(s) should be given.", 0, java.lang.Integer.MAX_VALUE, 1328 schedule)); 1329 childrenList.add(new Property("quantity", "SimpleQuantity", 1330 "The amount of the nutritional supplement to be given.", 0, java.lang.Integer.MAX_VALUE, quantity)); 1331 childrenList.add(new Property("instruction", "string", 1332 "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1333 java.lang.Integer.MAX_VALUE, instruction)); 1334 } 1335 1336 @Override 1337 public void setProperty(String name, Base value) throws FHIRException { 1338 if (name.equals("type")) 1339 this.type = castToCodeableConcept(value); // CodeableConcept 1340 else if (name.equals("productName")) 1341 this.productName = castToString(value); // StringType 1342 else if (name.equals("schedule")) 1343 this.getSchedule().add(castToTiming(value)); 1344 else if (name.equals("quantity")) 1345 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1346 else if (name.equals("instruction")) 1347 this.instruction = castToString(value); // StringType 1348 else 1349 super.setProperty(name, value); 1350 } 1351 1352 @Override 1353 public Base addChild(String name) throws FHIRException { 1354 if (name.equals("type")) { 1355 this.type = new CodeableConcept(); 1356 return this.type; 1357 } else if (name.equals("productName")) { 1358 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.productName"); 1359 } else if (name.equals("schedule")) { 1360 return addSchedule(); 1361 } else if (name.equals("quantity")) { 1362 this.quantity = new SimpleQuantity(); 1363 return this.quantity; 1364 } else if (name.equals("instruction")) { 1365 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 1366 } else 1367 return super.addChild(name); 1368 } 1369 1370 public NutritionOrderSupplementComponent copy() { 1371 NutritionOrderSupplementComponent dst = new NutritionOrderSupplementComponent(); 1372 copyValues(dst); 1373 dst.type = type == null ? null : type.copy(); 1374 dst.productName = productName == null ? null : productName.copy(); 1375 if (schedule != null) { 1376 dst.schedule = new ArrayList<Timing>(); 1377 for (Timing i : schedule) 1378 dst.schedule.add(i.copy()); 1379 } 1380 ; 1381 dst.quantity = quantity == null ? null : quantity.copy(); 1382 dst.instruction = instruction == null ? null : instruction.copy(); 1383 return dst; 1384 } 1385 1386 @Override 1387 public boolean equalsDeep(Base other) { 1388 if (!super.equalsDeep(other)) 1389 return false; 1390 if (!(other instanceof NutritionOrderSupplementComponent)) 1391 return false; 1392 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other; 1393 return compareDeep(type, o.type, true) && compareDeep(productName, o.productName, true) 1394 && compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) 1395 && compareDeep(instruction, o.instruction, true); 1396 } 1397 1398 @Override 1399 public boolean equalsShallow(Base other) { 1400 if (!super.equalsShallow(other)) 1401 return false; 1402 if (!(other instanceof NutritionOrderSupplementComponent)) 1403 return false; 1404 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other; 1405 return compareValues(productName, o.productName, true) && compareValues(instruction, o.instruction, true); 1406 } 1407 1408 public boolean isEmpty() { 1409 return super.isEmpty() && (type == null || type.isEmpty()) && (productName == null || productName.isEmpty()) 1410 && (schedule == null || schedule.isEmpty()) && (quantity == null || quantity.isEmpty()) 1411 && (instruction == null || instruction.isEmpty()); 1412 } 1413 1414 public String fhirType() { 1415 return "NutritionOrder.supplement"; 1416 1417 } 1418 1419 } 1420 1421 @Block() 1422 public static class NutritionOrderEnteralFormulaComponent extends BackboneElement implements IBaseBackboneElement { 1423 /** 1424 * The type of enteral or infant formula such as an adult standard formula with 1425 * fiber or a soy-based infant formula. 1426 */ 1427 @Child(name = "baseFormulaType", type = { 1428 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1429 @Description(shortDefinition = "Type of enteral or infant formula", formalDefinition = "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.") 1430 protected CodeableConcept baseFormulaType; 1431 1432 /** 1433 * The product or brand name of the enteral or infant formula product such as 1434 * "ACME Adult Standard Formula". 1435 */ 1436 @Child(name = "baseFormulaProductName", type = { 1437 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1438 @Description(shortDefinition = "Product or brand name of the enteral or infant formula", formalDefinition = "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".") 1439 protected StringType baseFormulaProductName; 1440 1441 /** 1442 * Indicates the type of modular component such as protein, carbohydrate, fat or 1443 * fiber to be provided in addition to or mixed with the base formula. 1444 */ 1445 @Child(name = "additiveType", type = { 1446 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1447 @Description(shortDefinition = "Type of modular component to add to the feeding", formalDefinition = "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.") 1448 protected CodeableConcept additiveType; 1449 1450 /** 1451 * The product or brand name of the type of modular component to be added to the 1452 * formula. 1453 */ 1454 @Child(name = "additiveProductName", type = { 1455 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1456 @Description(shortDefinition = "Product or brand name of the modular additive", formalDefinition = "The product or brand name of the type of modular component to be added to the formula.") 1457 protected StringType additiveProductName; 1458 1459 /** 1460 * The amount of energy (Calories) that the formula should provide per specified 1461 * volume, typically per mL or fluid oz. For example, an infant may require a 1462 * formula that provides 24 Calories per fluid ounce or an adult may require an 1463 * enteral formula that provides 1.5 Calorie/mL. 1464 */ 1465 @Child(name = "caloricDensity", type = { 1466 SimpleQuantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1467 @Description(shortDefinition = "Amount of energy per specified volume that is required", formalDefinition = "The amount of energy (Calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 Calories per fluid ounce or an adult may require an enteral formula that provides 1.5 Calorie/mL.") 1468 protected SimpleQuantity caloricDensity; 1469 1470 /** 1471 * The route or physiological path of administration into the patient's 1472 * gastrointestinal tract for purposes of providing the formula feeding, e.g. 1473 * nasogastric tube. 1474 */ 1475 @Child(name = "routeofAdministration", type = { 1476 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1477 @Description(shortDefinition = "How the formula should enter the patient's gastrointestinal tract", formalDefinition = "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.") 1478 protected CodeableConcept routeofAdministration; 1479 1480 /** 1481 * Formula administration instructions as structured data. This repeating 1482 * structure allows for changing the administration rate or volume over time for 1483 * both bolus and continuous feeding. An example of this would be an instruction 1484 * to increase the rate of continuous feeding every 2 hours. 1485 */ 1486 @Child(name = "administration", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1487 @Description(shortDefinition = "Formula feeding instruction as structured data", formalDefinition = "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.") 1488 protected List<NutritionOrderEnteralFormulaAdministrationComponent> administration; 1489 1490 /** 1491 * The maximum total quantity of formula that may be administered to a subject 1492 * over the period of time, e.g. 1440 mL over 24 hours. 1493 */ 1494 @Child(name = "maxVolumeToDeliver", type = { 1495 SimpleQuantity.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1496 @Description(shortDefinition = "Upper limit on formula volume per unit of time", formalDefinition = "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.") 1497 protected SimpleQuantity maxVolumeToDeliver; 1498 1499 /** 1500 * Free text formula administration, feeding instructions or additional 1501 * instructions or information. 1502 */ 1503 @Child(name = "administrationInstruction", type = { 1504 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1505 @Description(shortDefinition = "Formula feeding instructions expressed as text", formalDefinition = "Free text formula administration, feeding instructions or additional instructions or information.") 1506 protected StringType administrationInstruction; 1507 1508 private static final long serialVersionUID = 292116061L; 1509 1510 /* 1511 * Constructor 1512 */ 1513 public NutritionOrderEnteralFormulaComponent() { 1514 super(); 1515 } 1516 1517 /** 1518 * @return {@link #baseFormulaType} (The type of enteral or infant formula such 1519 * as an adult standard formula with fiber or a soy-based infant 1520 * formula.) 1521 */ 1522 public CodeableConcept getBaseFormulaType() { 1523 if (this.baseFormulaType == null) 1524 if (Configuration.errorOnAutoCreate()) 1525 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaType"); 1526 else if (Configuration.doAutoCreate()) 1527 this.baseFormulaType = new CodeableConcept(); // cc 1528 return this.baseFormulaType; 1529 } 1530 1531 public boolean hasBaseFormulaType() { 1532 return this.baseFormulaType != null && !this.baseFormulaType.isEmpty(); 1533 } 1534 1535 /** 1536 * @param value {@link #baseFormulaType} (The type of enteral or infant formula 1537 * such as an adult standard formula with fiber or a soy-based 1538 * infant formula.) 1539 */ 1540 public NutritionOrderEnteralFormulaComponent setBaseFormulaType(CodeableConcept value) { 1541 this.baseFormulaType = value; 1542 return this; 1543 } 1544 1545 /** 1546 * @return {@link #baseFormulaProductName} (The product or brand name of the 1547 * enteral or infant formula product such as "ACME Adult Standard 1548 * Formula".). This is the underlying object with id, value and 1549 * extensions. The accessor "getBaseFormulaProductName" gives direct 1550 * access to the value 1551 */ 1552 public StringType getBaseFormulaProductNameElement() { 1553 if (this.baseFormulaProductName == null) 1554 if (Configuration.errorOnAutoCreate()) 1555 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaProductName"); 1556 else if (Configuration.doAutoCreate()) 1557 this.baseFormulaProductName = new StringType(); // bb 1558 return this.baseFormulaProductName; 1559 } 1560 1561 public boolean hasBaseFormulaProductNameElement() { 1562 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 1563 } 1564 1565 public boolean hasBaseFormulaProductName() { 1566 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 1567 } 1568 1569 /** 1570 * @param value {@link #baseFormulaProductName} (The product or brand name of 1571 * the enteral or infant formula product such as "ACME Adult 1572 * Standard Formula".). This is the underlying object with id, 1573 * value and extensions. The accessor "getBaseFormulaProductName" 1574 * gives direct access to the value 1575 */ 1576 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductNameElement(StringType value) { 1577 this.baseFormulaProductName = value; 1578 return this; 1579 } 1580 1581 /** 1582 * @return The product or brand name of the enteral or infant formula product 1583 * such as "ACME Adult Standard Formula". 1584 */ 1585 public String getBaseFormulaProductName() { 1586 return this.baseFormulaProductName == null ? null : this.baseFormulaProductName.getValue(); 1587 } 1588 1589 /** 1590 * @param value The product or brand name of the enteral or infant formula 1591 * product such as "ACME Adult Standard Formula". 1592 */ 1593 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductName(String value) { 1594 if (Utilities.noString(value)) 1595 this.baseFormulaProductName = null; 1596 else { 1597 if (this.baseFormulaProductName == null) 1598 this.baseFormulaProductName = new StringType(); 1599 this.baseFormulaProductName.setValue(value); 1600 } 1601 return this; 1602 } 1603 1604 /** 1605 * @return {@link #additiveType} (Indicates the type of modular component such 1606 * as protein, carbohydrate, fat or fiber to be provided in addition to 1607 * or mixed with the base formula.) 1608 */ 1609 public CodeableConcept getAdditiveType() { 1610 if (this.additiveType == null) 1611 if (Configuration.errorOnAutoCreate()) 1612 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveType"); 1613 else if (Configuration.doAutoCreate()) 1614 this.additiveType = new CodeableConcept(); // cc 1615 return this.additiveType; 1616 } 1617 1618 public boolean hasAdditiveType() { 1619 return this.additiveType != null && !this.additiveType.isEmpty(); 1620 } 1621 1622 /** 1623 * @param value {@link #additiveType} (Indicates the type of modular component 1624 * such as protein, carbohydrate, fat or fiber to be provided in 1625 * addition to or mixed with the base formula.) 1626 */ 1627 public NutritionOrderEnteralFormulaComponent setAdditiveType(CodeableConcept value) { 1628 this.additiveType = value; 1629 return this; 1630 } 1631 1632 /** 1633 * @return {@link #additiveProductName} (The product or brand name of the type 1634 * of modular component to be added to the formula.). This is the 1635 * underlying object with id, value and extensions. The accessor 1636 * "getAdditiveProductName" gives direct access to the value 1637 */ 1638 public StringType getAdditiveProductNameElement() { 1639 if (this.additiveProductName == null) 1640 if (Configuration.errorOnAutoCreate()) 1641 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveProductName"); 1642 else if (Configuration.doAutoCreate()) 1643 this.additiveProductName = new StringType(); // bb 1644 return this.additiveProductName; 1645 } 1646 1647 public boolean hasAdditiveProductNameElement() { 1648 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 1649 } 1650 1651 public boolean hasAdditiveProductName() { 1652 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #additiveProductName} (The product or brand name of the 1657 * type of modular component to be added to the formula.). This is 1658 * the underlying object with id, value and extensions. The 1659 * accessor "getAdditiveProductName" gives direct access to the 1660 * value 1661 */ 1662 public NutritionOrderEnteralFormulaComponent setAdditiveProductNameElement(StringType value) { 1663 this.additiveProductName = value; 1664 return this; 1665 } 1666 1667 /** 1668 * @return The product or brand name of the type of modular component to be 1669 * added to the formula. 1670 */ 1671 public String getAdditiveProductName() { 1672 return this.additiveProductName == null ? null : this.additiveProductName.getValue(); 1673 } 1674 1675 /** 1676 * @param value The product or brand name of the type of modular component to be 1677 * added to the formula. 1678 */ 1679 public NutritionOrderEnteralFormulaComponent setAdditiveProductName(String value) { 1680 if (Utilities.noString(value)) 1681 this.additiveProductName = null; 1682 else { 1683 if (this.additiveProductName == null) 1684 this.additiveProductName = new StringType(); 1685 this.additiveProductName.setValue(value); 1686 } 1687 return this; 1688 } 1689 1690 /** 1691 * @return {@link #caloricDensity} (The amount of energy (Calories) that the 1692 * formula should provide per specified volume, typically per mL or 1693 * fluid oz. For example, an infant may require a formula that provides 1694 * 24 Calories per fluid ounce or an adult may require an enteral 1695 * formula that provides 1.5 Calorie/mL.) 1696 */ 1697 public SimpleQuantity getCaloricDensity() { 1698 if (this.caloricDensity == null) 1699 if (Configuration.errorOnAutoCreate()) 1700 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.caloricDensity"); 1701 else if (Configuration.doAutoCreate()) 1702 this.caloricDensity = new SimpleQuantity(); // cc 1703 return this.caloricDensity; 1704 } 1705 1706 public boolean hasCaloricDensity() { 1707 return this.caloricDensity != null && !this.caloricDensity.isEmpty(); 1708 } 1709 1710 /** 1711 * @param value {@link #caloricDensity} (The amount of energy (Calories) that 1712 * the formula should provide per specified volume, typically per 1713 * mL or fluid oz. For example, an infant may require a formula 1714 * that provides 24 Calories per fluid ounce or an adult may 1715 * require an enteral formula that provides 1.5 Calorie/mL.) 1716 */ 1717 public NutritionOrderEnteralFormulaComponent setCaloricDensity(SimpleQuantity value) { 1718 this.caloricDensity = value; 1719 return this; 1720 } 1721 1722 /** 1723 * @return {@link #routeofAdministration} (The route or physiological path of 1724 * administration into the patient's gastrointestinal tract for purposes 1725 * of providing the formula feeding, e.g. nasogastric tube.) 1726 */ 1727 public CodeableConcept getRouteofAdministration() { 1728 if (this.routeofAdministration == null) 1729 if (Configuration.errorOnAutoCreate()) 1730 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.routeofAdministration"); 1731 else if (Configuration.doAutoCreate()) 1732 this.routeofAdministration = new CodeableConcept(); // cc 1733 return this.routeofAdministration; 1734 } 1735 1736 public boolean hasRouteofAdministration() { 1737 return this.routeofAdministration != null && !this.routeofAdministration.isEmpty(); 1738 } 1739 1740 /** 1741 * @param value {@link #routeofAdministration} (The route or physiological path 1742 * of administration into the patient's gastrointestinal tract for 1743 * purposes of providing the formula feeding, e.g. nasogastric 1744 * tube.) 1745 */ 1746 public NutritionOrderEnteralFormulaComponent setRouteofAdministration(CodeableConcept value) { 1747 this.routeofAdministration = value; 1748 return this; 1749 } 1750 1751 /** 1752 * @return {@link #administration} (Formula administration instructions as 1753 * structured data. This repeating structure allows for changing the 1754 * administration rate or volume over time for both bolus and continuous 1755 * feeding. An example of this would be an instruction to increase the 1756 * rate of continuous feeding every 2 hours.) 1757 */ 1758 public List<NutritionOrderEnteralFormulaAdministrationComponent> getAdministration() { 1759 if (this.administration == null) 1760 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1761 return this.administration; 1762 } 1763 1764 public boolean hasAdministration() { 1765 if (this.administration == null) 1766 return false; 1767 for (NutritionOrderEnteralFormulaAdministrationComponent item : this.administration) 1768 if (!item.isEmpty()) 1769 return true; 1770 return false; 1771 } 1772 1773 /** 1774 * @return {@link #administration} (Formula administration instructions as 1775 * structured data. This repeating structure allows for changing the 1776 * administration rate or volume over time for both bolus and continuous 1777 * feeding. An example of this would be an instruction to increase the 1778 * rate of continuous feeding every 2 hours.) 1779 */ 1780 // syntactic sugar 1781 public NutritionOrderEnteralFormulaAdministrationComponent addAdministration() { // 3 1782 NutritionOrderEnteralFormulaAdministrationComponent t = new NutritionOrderEnteralFormulaAdministrationComponent(); 1783 if (this.administration == null) 1784 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1785 this.administration.add(t); 1786 return t; 1787 } 1788 1789 // syntactic sugar 1790 public NutritionOrderEnteralFormulaComponent addAdministration( 1791 NutritionOrderEnteralFormulaAdministrationComponent t) { // 3 1792 if (t == null) 1793 return this; 1794 if (this.administration == null) 1795 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1796 this.administration.add(t); 1797 return this; 1798 } 1799 1800 /** 1801 * @return {@link #maxVolumeToDeliver} (The maximum total quantity of formula 1802 * that may be administered to a subject over the period of time, e.g. 1803 * 1440 mL over 24 hours.) 1804 */ 1805 public SimpleQuantity getMaxVolumeToDeliver() { 1806 if (this.maxVolumeToDeliver == null) 1807 if (Configuration.errorOnAutoCreate()) 1808 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.maxVolumeToDeliver"); 1809 else if (Configuration.doAutoCreate()) 1810 this.maxVolumeToDeliver = new SimpleQuantity(); // cc 1811 return this.maxVolumeToDeliver; 1812 } 1813 1814 public boolean hasMaxVolumeToDeliver() { 1815 return this.maxVolumeToDeliver != null && !this.maxVolumeToDeliver.isEmpty(); 1816 } 1817 1818 /** 1819 * @param value {@link #maxVolumeToDeliver} (The maximum total quantity of 1820 * formula that may be administered to a subject over the period of 1821 * time, e.g. 1440 mL over 24 hours.) 1822 */ 1823 public NutritionOrderEnteralFormulaComponent setMaxVolumeToDeliver(SimpleQuantity value) { 1824 this.maxVolumeToDeliver = value; 1825 return this; 1826 } 1827 1828 /** 1829 * @return {@link #administrationInstruction} (Free text formula administration, 1830 * feeding instructions or additional instructions or information.). 1831 * This is the underlying object with id, value and extensions. The 1832 * accessor "getAdministrationInstruction" gives direct access to the 1833 * value 1834 */ 1835 public StringType getAdministrationInstructionElement() { 1836 if (this.administrationInstruction == null) 1837 if (Configuration.errorOnAutoCreate()) 1838 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.administrationInstruction"); 1839 else if (Configuration.doAutoCreate()) 1840 this.administrationInstruction = new StringType(); // bb 1841 return this.administrationInstruction; 1842 } 1843 1844 public boolean hasAdministrationInstructionElement() { 1845 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 1846 } 1847 1848 public boolean hasAdministrationInstruction() { 1849 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 1850 } 1851 1852 /** 1853 * @param value {@link #administrationInstruction} (Free text formula 1854 * administration, feeding instructions or additional instructions 1855 * or information.). This is the underlying object with id, value 1856 * and extensions. The accessor "getAdministrationInstruction" 1857 * gives direct access to the value 1858 */ 1859 public NutritionOrderEnteralFormulaComponent setAdministrationInstructionElement(StringType value) { 1860 this.administrationInstruction = value; 1861 return this; 1862 } 1863 1864 /** 1865 * @return Free text formula administration, feeding instructions or additional 1866 * instructions or information. 1867 */ 1868 public String getAdministrationInstruction() { 1869 return this.administrationInstruction == null ? null : this.administrationInstruction.getValue(); 1870 } 1871 1872 /** 1873 * @param value Free text formula administration, feeding instructions or 1874 * additional instructions or information. 1875 */ 1876 public NutritionOrderEnteralFormulaComponent setAdministrationInstruction(String value) { 1877 if (Utilities.noString(value)) 1878 this.administrationInstruction = null; 1879 else { 1880 if (this.administrationInstruction == null) 1881 this.administrationInstruction = new StringType(); 1882 this.administrationInstruction.setValue(value); 1883 } 1884 return this; 1885 } 1886 1887 protected void listChildren(List<Property> childrenList) { 1888 super.listChildren(childrenList); 1889 childrenList.add(new Property("baseFormulaType", "CodeableConcept", 1890 "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 1891 0, java.lang.Integer.MAX_VALUE, baseFormulaType)); 1892 childrenList.add(new Property("baseFormulaProductName", "string", 1893 "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 1894 0, java.lang.Integer.MAX_VALUE, baseFormulaProductName)); 1895 childrenList.add(new Property("additiveType", "CodeableConcept", 1896 "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 1897 0, java.lang.Integer.MAX_VALUE, additiveType)); 1898 childrenList.add(new Property("additiveProductName", "string", 1899 "The product or brand name of the type of modular component to be added to the formula.", 0, 1900 java.lang.Integer.MAX_VALUE, additiveProductName)); 1901 childrenList.add(new Property("caloricDensity", "SimpleQuantity", 1902 "The amount of energy (Calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 Calories per fluid ounce or an adult may require an enteral formula that provides 1.5 Calorie/mL.", 1903 0, java.lang.Integer.MAX_VALUE, caloricDensity)); 1904 childrenList.add(new Property("routeofAdministration", "CodeableConcept", 1905 "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 1906 0, java.lang.Integer.MAX_VALUE, routeofAdministration)); 1907 childrenList.add(new Property("administration", "", 1908 "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 1909 0, java.lang.Integer.MAX_VALUE, administration)); 1910 childrenList.add(new Property("maxVolumeToDeliver", "SimpleQuantity", 1911 "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 1912 0, java.lang.Integer.MAX_VALUE, maxVolumeToDeliver)); 1913 childrenList.add(new Property("administrationInstruction", "string", 1914 "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1915 java.lang.Integer.MAX_VALUE, administrationInstruction)); 1916 } 1917 1918 @Override 1919 public void setProperty(String name, Base value) throws FHIRException { 1920 if (name.equals("baseFormulaType")) 1921 this.baseFormulaType = castToCodeableConcept(value); // CodeableConcept 1922 else if (name.equals("baseFormulaProductName")) 1923 this.baseFormulaProductName = castToString(value); // StringType 1924 else if (name.equals("additiveType")) 1925 this.additiveType = castToCodeableConcept(value); // CodeableConcept 1926 else if (name.equals("additiveProductName")) 1927 this.additiveProductName = castToString(value); // StringType 1928 else if (name.equals("caloricDensity")) 1929 this.caloricDensity = castToSimpleQuantity(value); // SimpleQuantity 1930 else if (name.equals("routeofAdministration")) 1931 this.routeofAdministration = castToCodeableConcept(value); // CodeableConcept 1932 else if (name.equals("administration")) 1933 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); 1934 else if (name.equals("maxVolumeToDeliver")) 1935 this.maxVolumeToDeliver = castToSimpleQuantity(value); // SimpleQuantity 1936 else if (name.equals("administrationInstruction")) 1937 this.administrationInstruction = castToString(value); // StringType 1938 else 1939 super.setProperty(name, value); 1940 } 1941 1942 @Override 1943 public Base addChild(String name) throws FHIRException { 1944 if (name.equals("baseFormulaType")) { 1945 this.baseFormulaType = new CodeableConcept(); 1946 return this.baseFormulaType; 1947 } else if (name.equals("baseFormulaProductName")) { 1948 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.baseFormulaProductName"); 1949 } else if (name.equals("additiveType")) { 1950 this.additiveType = new CodeableConcept(); 1951 return this.additiveType; 1952 } else if (name.equals("additiveProductName")) { 1953 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.additiveProductName"); 1954 } else if (name.equals("caloricDensity")) { 1955 this.caloricDensity = new SimpleQuantity(); 1956 return this.caloricDensity; 1957 } else if (name.equals("routeofAdministration")) { 1958 this.routeofAdministration = new CodeableConcept(); 1959 return this.routeofAdministration; 1960 } else if (name.equals("administration")) { 1961 return addAdministration(); 1962 } else if (name.equals("maxVolumeToDeliver")) { 1963 this.maxVolumeToDeliver = new SimpleQuantity(); 1964 return this.maxVolumeToDeliver; 1965 } else if (name.equals("administrationInstruction")) { 1966 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.administrationInstruction"); 1967 } else 1968 return super.addChild(name); 1969 } 1970 1971 public NutritionOrderEnteralFormulaComponent copy() { 1972 NutritionOrderEnteralFormulaComponent dst = new NutritionOrderEnteralFormulaComponent(); 1973 copyValues(dst); 1974 dst.baseFormulaType = baseFormulaType == null ? null : baseFormulaType.copy(); 1975 dst.baseFormulaProductName = baseFormulaProductName == null ? null : baseFormulaProductName.copy(); 1976 dst.additiveType = additiveType == null ? null : additiveType.copy(); 1977 dst.additiveProductName = additiveProductName == null ? null : additiveProductName.copy(); 1978 dst.caloricDensity = caloricDensity == null ? null : caloricDensity.copy(); 1979 dst.routeofAdministration = routeofAdministration == null ? null : routeofAdministration.copy(); 1980 if (administration != null) { 1981 dst.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 1982 for (NutritionOrderEnteralFormulaAdministrationComponent i : administration) 1983 dst.administration.add(i.copy()); 1984 } 1985 ; 1986 dst.maxVolumeToDeliver = maxVolumeToDeliver == null ? null : maxVolumeToDeliver.copy(); 1987 dst.administrationInstruction = administrationInstruction == null ? null : administrationInstruction.copy(); 1988 return dst; 1989 } 1990 1991 @Override 1992 public boolean equalsDeep(Base other) { 1993 if (!super.equalsDeep(other)) 1994 return false; 1995 if (!(other instanceof NutritionOrderEnteralFormulaComponent)) 1996 return false; 1997 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other; 1998 return compareDeep(baseFormulaType, o.baseFormulaType, true) 1999 && compareDeep(baseFormulaProductName, o.baseFormulaProductName, true) 2000 && compareDeep(additiveType, o.additiveType, true) 2001 && compareDeep(additiveProductName, o.additiveProductName, true) 2002 && compareDeep(caloricDensity, o.caloricDensity, true) 2003 && compareDeep(routeofAdministration, o.routeofAdministration, true) 2004 && compareDeep(administration, o.administration, true) 2005 && compareDeep(maxVolumeToDeliver, o.maxVolumeToDeliver, true) 2006 && compareDeep(administrationInstruction, o.administrationInstruction, true); 2007 } 2008 2009 @Override 2010 public boolean equalsShallow(Base other) { 2011 if (!super.equalsShallow(other)) 2012 return false; 2013 if (!(other instanceof NutritionOrderEnteralFormulaComponent)) 2014 return false; 2015 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other; 2016 return compareValues(baseFormulaProductName, o.baseFormulaProductName, true) 2017 && compareValues(additiveProductName, o.additiveProductName, true) 2018 && compareValues(administrationInstruction, o.administrationInstruction, true); 2019 } 2020 2021 public boolean isEmpty() { 2022 return super.isEmpty() && (baseFormulaType == null || baseFormulaType.isEmpty()) 2023 && (baseFormulaProductName == null || baseFormulaProductName.isEmpty()) 2024 && (additiveType == null || additiveType.isEmpty()) 2025 && (additiveProductName == null || additiveProductName.isEmpty()) 2026 && (caloricDensity == null || caloricDensity.isEmpty()) 2027 && (routeofAdministration == null || routeofAdministration.isEmpty()) 2028 && (administration == null || administration.isEmpty()) 2029 && (maxVolumeToDeliver == null || maxVolumeToDeliver.isEmpty()) 2030 && (administrationInstruction == null || administrationInstruction.isEmpty()); 2031 } 2032 2033 public String fhirType() { 2034 return "NutritionOrder.enteralFormula"; 2035 2036 } 2037 2038 } 2039 2040 @Block() 2041 public static class NutritionOrderEnteralFormulaAdministrationComponent extends BackboneElement 2042 implements IBaseBackboneElement { 2043 /** 2044 * The time period and frequency at which the enteral formula should be 2045 * delivered to the patient. 2046 */ 2047 @Child(name = "schedule", type = { Timing.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2048 @Description(shortDefinition = "Scheduled frequency of enteral feeding", formalDefinition = "The time period and frequency at which the enteral formula should be delivered to the patient.") 2049 protected Timing schedule; 2050 2051 /** 2052 * The volume of formula to provide to the patient per the specified 2053 * administration schedule. 2054 */ 2055 @Child(name = "quantity", type = { 2056 SimpleQuantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2057 @Description(shortDefinition = "The volume of formula to provide", formalDefinition = "The volume of formula to provide to the patient per the specified administration schedule.") 2058 protected SimpleQuantity quantity; 2059 2060 /** 2061 * The rate of administration of formula via a feeding pump, e.g. 60 mL per 2062 * hour, according to the specified schedule. 2063 */ 2064 @Child(name = "rate", type = { SimpleQuantity.class, 2065 Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2066 @Description(shortDefinition = "Speed with which the formula is provided per period of time", formalDefinition = "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.") 2067 protected Type rate; 2068 2069 private static final long serialVersionUID = 1895031997L; 2070 2071 /* 2072 * Constructor 2073 */ 2074 public NutritionOrderEnteralFormulaAdministrationComponent() { 2075 super(); 2076 } 2077 2078 /** 2079 * @return {@link #schedule} (The time period and frequency at which the enteral 2080 * formula should be delivered to the patient.) 2081 */ 2082 public Timing getSchedule() { 2083 if (this.schedule == null) 2084 if (Configuration.errorOnAutoCreate()) 2085 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.schedule"); 2086 else if (Configuration.doAutoCreate()) 2087 this.schedule = new Timing(); // cc 2088 return this.schedule; 2089 } 2090 2091 public boolean hasSchedule() { 2092 return this.schedule != null && !this.schedule.isEmpty(); 2093 } 2094 2095 /** 2096 * @param value {@link #schedule} (The time period and frequency at which the 2097 * enteral formula should be delivered to the patient.) 2098 */ 2099 public NutritionOrderEnteralFormulaAdministrationComponent setSchedule(Timing value) { 2100 this.schedule = value; 2101 return this; 2102 } 2103 2104 /** 2105 * @return {@link #quantity} (The volume of formula to provide to the patient 2106 * per the specified administration schedule.) 2107 */ 2108 public SimpleQuantity getQuantity() { 2109 if (this.quantity == null) 2110 if (Configuration.errorOnAutoCreate()) 2111 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.quantity"); 2112 else if (Configuration.doAutoCreate()) 2113 this.quantity = new SimpleQuantity(); // cc 2114 return this.quantity; 2115 } 2116 2117 public boolean hasQuantity() { 2118 return this.quantity != null && !this.quantity.isEmpty(); 2119 } 2120 2121 /** 2122 * @param value {@link #quantity} (The volume of formula to provide to the 2123 * patient per the specified administration schedule.) 2124 */ 2125 public NutritionOrderEnteralFormulaAdministrationComponent setQuantity(SimpleQuantity value) { 2126 this.quantity = value; 2127 return this; 2128 } 2129 2130 /** 2131 * @return {@link #rate} (The rate of administration of formula via a feeding 2132 * pump, e.g. 60 mL per hour, according to the specified schedule.) 2133 */ 2134 public Type getRate() { 2135 return this.rate; 2136 } 2137 2138 /** 2139 * @return {@link #rate} (The rate of administration of formula via a feeding 2140 * pump, e.g. 60 mL per hour, according to the specified schedule.) 2141 */ 2142 public SimpleQuantity getRateSimpleQuantity() throws FHIRException { 2143 if (!(this.rate instanceof SimpleQuantity)) 2144 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but " 2145 + this.rate.getClass().getName() + " was encountered"); 2146 return (SimpleQuantity) this.rate; 2147 } 2148 2149 public boolean hasRateSimpleQuantity() { 2150 return this.rate instanceof SimpleQuantity; 2151 } 2152 2153 /** 2154 * @return {@link #rate} (The rate of administration of formula via a feeding 2155 * pump, e.g. 60 mL per hour, according to the specified schedule.) 2156 */ 2157 public Ratio getRateRatio() throws FHIRException { 2158 if (!(this.rate instanceof Ratio)) 2159 throw new FHIRException( 2160 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 2161 return (Ratio) this.rate; 2162 } 2163 2164 public boolean hasRateRatio() { 2165 return this.rate instanceof Ratio; 2166 } 2167 2168 public boolean hasRate() { 2169 return this.rate != null && !this.rate.isEmpty(); 2170 } 2171 2172 /** 2173 * @param value {@link #rate} (The rate of administration of formula via a 2174 * feeding pump, e.g. 60 mL per hour, according to the specified 2175 * schedule.) 2176 */ 2177 public NutritionOrderEnteralFormulaAdministrationComponent setRate(Type value) { 2178 this.rate = value; 2179 return this; 2180 } 2181 2182 protected void listChildren(List<Property> childrenList) { 2183 super.listChildren(childrenList); 2184 childrenList.add(new Property("schedule", "Timing", 2185 "The time period and frequency at which the enteral formula should be delivered to the patient.", 0, 2186 java.lang.Integer.MAX_VALUE, schedule)); 2187 childrenList.add(new Property("quantity", "SimpleQuantity", 2188 "The volume of formula to provide to the patient per the specified administration schedule.", 0, 2189 java.lang.Integer.MAX_VALUE, quantity)); 2190 childrenList.add(new Property("rate[x]", "SimpleQuantity|Ratio", 2191 "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 2192 0, java.lang.Integer.MAX_VALUE, rate)); 2193 } 2194 2195 @Override 2196 public void setProperty(String name, Base value) throws FHIRException { 2197 if (name.equals("schedule")) 2198 this.schedule = castToTiming(value); // Timing 2199 else if (name.equals("quantity")) 2200 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2201 else if (name.equals("rate[x]")) 2202 this.rate = (Type) value; // Type 2203 else 2204 super.setProperty(name, value); 2205 } 2206 2207 @Override 2208 public Base addChild(String name) throws FHIRException { 2209 if (name.equals("schedule")) { 2210 this.schedule = new Timing(); 2211 return this.schedule; 2212 } else if (name.equals("quantity")) { 2213 this.quantity = new SimpleQuantity(); 2214 return this.quantity; 2215 } else if (name.equals("rateSimpleQuantity")) { 2216 this.rate = new SimpleQuantity(); 2217 return this.rate; 2218 } else if (name.equals("rateRatio")) { 2219 this.rate = new Ratio(); 2220 return this.rate; 2221 } else 2222 return super.addChild(name); 2223 } 2224 2225 public NutritionOrderEnteralFormulaAdministrationComponent copy() { 2226 NutritionOrderEnteralFormulaAdministrationComponent dst = new NutritionOrderEnteralFormulaAdministrationComponent(); 2227 copyValues(dst); 2228 dst.schedule = schedule == null ? null : schedule.copy(); 2229 dst.quantity = quantity == null ? null : quantity.copy(); 2230 dst.rate = rate == null ? null : rate.copy(); 2231 return dst; 2232 } 2233 2234 @Override 2235 public boolean equalsDeep(Base other) { 2236 if (!super.equalsDeep(other)) 2237 return false; 2238 if (!(other instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 2239 return false; 2240 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other; 2241 return compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) 2242 && compareDeep(rate, o.rate, true); 2243 } 2244 2245 @Override 2246 public boolean equalsShallow(Base other) { 2247 if (!super.equalsShallow(other)) 2248 return false; 2249 if (!(other instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 2250 return false; 2251 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other; 2252 return true; 2253 } 2254 2255 public boolean isEmpty() { 2256 return super.isEmpty() && (schedule == null || schedule.isEmpty()) && (quantity == null || quantity.isEmpty()) 2257 && (rate == null || rate.isEmpty()); 2258 } 2259 2260 public String fhirType() { 2261 return "NutritionOrder.enteralFormula.administration"; 2262 2263 } 2264 2265 } 2266 2267 /** 2268 * The person (patient) who needs the nutrition order for an oral diet, 2269 * nutritional supplement and/or enteral or formula feeding. 2270 */ 2271 @Child(name = "patient", type = { Patient.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 2272 @Description(shortDefinition = "The person who requires the diet, formula or nutritional supplement", formalDefinition = "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.") 2273 protected Reference patient; 2274 2275 /** 2276 * The actual object that is the target of the reference (The person (patient) 2277 * who needs the nutrition order for an oral diet, nutritional supplement and/or 2278 * enteral or formula feeding.) 2279 */ 2280 protected Patient patientTarget; 2281 2282 /** 2283 * The practitioner that holds legal responsibility for ordering the diet, 2284 * nutritional supplement, or formula feedings. 2285 */ 2286 @Child(name = "orderer", type = { Practitioner.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2287 @Description(shortDefinition = "Who ordered the diet, formula or nutritional supplement", formalDefinition = "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.") 2288 protected Reference orderer; 2289 2290 /** 2291 * The actual object that is the target of the reference (The practitioner that 2292 * holds legal responsibility for ordering the diet, nutritional supplement, or 2293 * formula feedings.) 2294 */ 2295 protected Practitioner ordererTarget; 2296 2297 /** 2298 * Identifiers assigned to this order by the order sender or by the order 2299 * receiver. 2300 */ 2301 @Child(name = "identifier", type = { 2302 Identifier.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2303 @Description(shortDefinition = "Identifiers assigned to this order", formalDefinition = "Identifiers assigned to this order by the order sender or by the order receiver.") 2304 protected List<Identifier> identifier; 2305 2306 /** 2307 * An encounter that provides additional information about the healthcare 2308 * context in which this request is made. 2309 */ 2310 @Child(name = "encounter", type = { Encounter.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2311 @Description(shortDefinition = "The encounter associated with this nutrition order", formalDefinition = "An encounter that provides additional information about the healthcare context in which this request is made.") 2312 protected Reference encounter; 2313 2314 /** 2315 * The actual object that is the target of the reference (An encounter that 2316 * provides additional information about the healthcare context in which this 2317 * request is made.) 2318 */ 2319 protected Encounter encounterTarget; 2320 2321 /** 2322 * The date and time that this nutrition order was requested. 2323 */ 2324 @Child(name = "dateTime", type = { 2325 DateTimeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 2326 @Description(shortDefinition = "Date and time the nutrition order was requested", formalDefinition = "The date and time that this nutrition order was requested.") 2327 protected DateTimeType dateTime; 2328 2329 /** 2330 * The workflow status of the nutrition order/request. 2331 */ 2332 @Child(name = "status", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = true, summary = true) 2333 @Description(shortDefinition = "proposed | draft | planned | requested | active | on-hold | completed | cancelled", formalDefinition = "The workflow status of the nutrition order/request.") 2334 protected Enumeration<NutritionOrderStatus> status; 2335 2336 /** 2337 * A link to a record of allergies or intolerances which should be included in 2338 * the nutrition order. 2339 */ 2340 @Child(name = "allergyIntolerance", type = { 2341 AllergyIntolerance.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2342 @Description(shortDefinition = "List of the patient's food and nutrition-related allergies and intolerances", formalDefinition = "A link to a record of allergies or intolerances which should be included in the nutrition order.") 2343 protected List<Reference> allergyIntolerance; 2344 /** 2345 * The actual objects that are the target of the reference (A link to a record 2346 * of allergies or intolerances which should be included in the nutrition 2347 * order.) 2348 */ 2349 protected List<AllergyIntolerance> allergyIntoleranceTarget; 2350 2351 /** 2352 * This modifier is used to convey order-specific modifiers about the type of 2353 * food that should be given. These can be derived from patient allergies, 2354 * intolerances, or preferences such as Halal, Vegan or Kosher. This modifier 2355 * applies to the entire nutrition order inclusive of the oral diet, nutritional 2356 * supplements and enteral formula feedings. 2357 */ 2358 @Child(name = "foodPreferenceModifier", type = { 2359 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2360 @Description(shortDefinition = "Order-specific modifier about the type of food that should be given", formalDefinition = "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.") 2361 protected List<CodeableConcept> foodPreferenceModifier; 2362 2363 /** 2364 * This modifier is used to convey order-specific modifiers about the type of 2365 * food that should NOT be given. These can be derived from patient allergies, 2366 * intolerances, or preferences such as No Red Meat, No Soy or No Wheat or 2367 * Gluten-Free. While it should not be necessary to repeat allergy or 2368 * intolerance information captured in the referenced allergyIntolerance 2369 * resource in the excludeFoodModifier, this element may be used to convey 2370 * additional specificity related to foods that should be eliminated from the 2371 * patient?s diet for any reason. This modifier applies to the entire nutrition 2372 * order inclusive of the oral diet, nutritional supplements and enteral formula 2373 * feedings. 2374 */ 2375 @Child(name = "excludeFoodModifier", type = { 2376 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2377 @Description(shortDefinition = "Order-specific modifier about the type of food that should not be given", formalDefinition = "This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced allergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.") 2378 protected List<CodeableConcept> excludeFoodModifier; 2379 2380 /** 2381 * Diet given orally in contrast to enteral (tube) feeding. 2382 */ 2383 @Child(name = "oralDiet", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 2384 @Description(shortDefinition = "Oral diet components", formalDefinition = "Diet given orally in contrast to enteral (tube) feeding.") 2385 protected NutritionOrderOralDietComponent oralDiet; 2386 2387 /** 2388 * Oral nutritional products given in order to add further nutritional value to 2389 * the patient's diet. 2390 */ 2391 @Child(name = "supplement", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2392 @Description(shortDefinition = "Supplement components", formalDefinition = "Oral nutritional products given in order to add further nutritional value to the patient's diet.") 2393 protected List<NutritionOrderSupplementComponent> supplement; 2394 2395 /** 2396 * Feeding provided through the gastrointestinal tract via a tube, catheter, or 2397 * stoma that delivers nutrition distal to the oral cavity. 2398 */ 2399 @Child(name = "enteralFormula", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = false) 2400 @Description(shortDefinition = "Enteral formula components", formalDefinition = "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.") 2401 protected NutritionOrderEnteralFormulaComponent enteralFormula; 2402 2403 private static final long serialVersionUID = 1139624085L; 2404 2405 /* 2406 * Constructor 2407 */ 2408 public NutritionOrder() { 2409 super(); 2410 } 2411 2412 /* 2413 * Constructor 2414 */ 2415 public NutritionOrder(Reference patient, DateTimeType dateTime) { 2416 super(); 2417 this.patient = patient; 2418 this.dateTime = dateTime; 2419 } 2420 2421 /** 2422 * @return {@link #patient} (The person (patient) who needs the nutrition order 2423 * for an oral diet, nutritional supplement and/or enteral or formula 2424 * feeding.) 2425 */ 2426 public Reference getPatient() { 2427 if (this.patient == null) 2428 if (Configuration.errorOnAutoCreate()) 2429 throw new Error("Attempt to auto-create NutritionOrder.patient"); 2430 else if (Configuration.doAutoCreate()) 2431 this.patient = new Reference(); // cc 2432 return this.patient; 2433 } 2434 2435 public boolean hasPatient() { 2436 return this.patient != null && !this.patient.isEmpty(); 2437 } 2438 2439 /** 2440 * @param value {@link #patient} (The person (patient) who needs the nutrition 2441 * order for an oral diet, nutritional supplement and/or enteral or 2442 * formula feeding.) 2443 */ 2444 public NutritionOrder setPatient(Reference value) { 2445 this.patient = value; 2446 return this; 2447 } 2448 2449 /** 2450 * @return {@link #patient} The actual object that is the target of the 2451 * reference. The reference library doesn't populate this, but you can 2452 * use it to hold the resource if you resolve it. (The person (patient) 2453 * who needs the nutrition order for an oral diet, nutritional 2454 * supplement and/or enteral or formula feeding.) 2455 */ 2456 public Patient getPatientTarget() { 2457 if (this.patientTarget == null) 2458 if (Configuration.errorOnAutoCreate()) 2459 throw new Error("Attempt to auto-create NutritionOrder.patient"); 2460 else if (Configuration.doAutoCreate()) 2461 this.patientTarget = new Patient(); // aa 2462 return this.patientTarget; 2463 } 2464 2465 /** 2466 * @param value {@link #patient} The actual object that is the target of the 2467 * reference. The reference library doesn't use these, but you can 2468 * use it to hold the resource if you resolve it. (The person 2469 * (patient) who needs the nutrition order for an oral diet, 2470 * nutritional supplement and/or enteral or formula feeding.) 2471 */ 2472 public NutritionOrder setPatientTarget(Patient value) { 2473 this.patientTarget = value; 2474 return this; 2475 } 2476 2477 /** 2478 * @return {@link #orderer} (The practitioner that holds legal responsibility 2479 * for ordering the diet, nutritional supplement, or formula feedings.) 2480 */ 2481 public Reference getOrderer() { 2482 if (this.orderer == null) 2483 if (Configuration.errorOnAutoCreate()) 2484 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 2485 else if (Configuration.doAutoCreate()) 2486 this.orderer = new Reference(); // cc 2487 return this.orderer; 2488 } 2489 2490 public boolean hasOrderer() { 2491 return this.orderer != null && !this.orderer.isEmpty(); 2492 } 2493 2494 /** 2495 * @param value {@link #orderer} (The practitioner that holds legal 2496 * responsibility for ordering the diet, nutritional supplement, or 2497 * formula feedings.) 2498 */ 2499 public NutritionOrder setOrderer(Reference value) { 2500 this.orderer = value; 2501 return this; 2502 } 2503 2504 /** 2505 * @return {@link #orderer} The actual object that is the target of the 2506 * reference. The reference library doesn't populate this, but you can 2507 * use it to hold the resource if you resolve it. (The practitioner that 2508 * holds legal responsibility for ordering the diet, nutritional 2509 * supplement, or formula feedings.) 2510 */ 2511 public Practitioner getOrdererTarget() { 2512 if (this.ordererTarget == null) 2513 if (Configuration.errorOnAutoCreate()) 2514 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 2515 else if (Configuration.doAutoCreate()) 2516 this.ordererTarget = new Practitioner(); // aa 2517 return this.ordererTarget; 2518 } 2519 2520 /** 2521 * @param value {@link #orderer} The actual object that is the target of the 2522 * reference. The reference library doesn't use these, but you can 2523 * use it to hold the resource if you resolve it. (The practitioner 2524 * that holds legal responsibility for ordering the diet, 2525 * nutritional supplement, or formula feedings.) 2526 */ 2527 public NutritionOrder setOrdererTarget(Practitioner value) { 2528 this.ordererTarget = value; 2529 return this; 2530 } 2531 2532 /** 2533 * @return {@link #identifier} (Identifiers assigned to this order by the order 2534 * sender or by the order receiver.) 2535 */ 2536 public List<Identifier> getIdentifier() { 2537 if (this.identifier == null) 2538 this.identifier = new ArrayList<Identifier>(); 2539 return this.identifier; 2540 } 2541 2542 public boolean hasIdentifier() { 2543 if (this.identifier == null) 2544 return false; 2545 for (Identifier item : this.identifier) 2546 if (!item.isEmpty()) 2547 return true; 2548 return false; 2549 } 2550 2551 /** 2552 * @return {@link #identifier} (Identifiers assigned to this order by the order 2553 * sender or by the order receiver.) 2554 */ 2555 // syntactic sugar 2556 public Identifier addIdentifier() { // 3 2557 Identifier t = new Identifier(); 2558 if (this.identifier == null) 2559 this.identifier = new ArrayList<Identifier>(); 2560 this.identifier.add(t); 2561 return t; 2562 } 2563 2564 // syntactic sugar 2565 public NutritionOrder addIdentifier(Identifier t) { // 3 2566 if (t == null) 2567 return this; 2568 if (this.identifier == null) 2569 this.identifier = new ArrayList<Identifier>(); 2570 this.identifier.add(t); 2571 return this; 2572 } 2573 2574 /** 2575 * @return {@link #encounter} (An encounter that provides additional information 2576 * about the healthcare context in which this request is made.) 2577 */ 2578 public Reference getEncounter() { 2579 if (this.encounter == null) 2580 if (Configuration.errorOnAutoCreate()) 2581 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 2582 else if (Configuration.doAutoCreate()) 2583 this.encounter = new Reference(); // cc 2584 return this.encounter; 2585 } 2586 2587 public boolean hasEncounter() { 2588 return this.encounter != null && !this.encounter.isEmpty(); 2589 } 2590 2591 /** 2592 * @param value {@link #encounter} (An encounter that provides additional 2593 * information about the healthcare context in which this request 2594 * is made.) 2595 */ 2596 public NutritionOrder setEncounter(Reference value) { 2597 this.encounter = value; 2598 return this; 2599 } 2600 2601 /** 2602 * @return {@link #encounter} The actual object that is the target of the 2603 * reference. The reference library doesn't populate this, but you can 2604 * use it to hold the resource if you resolve it. (An encounter that 2605 * provides additional information about the healthcare context in which 2606 * this request is made.) 2607 */ 2608 public Encounter getEncounterTarget() { 2609 if (this.encounterTarget == null) 2610 if (Configuration.errorOnAutoCreate()) 2611 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 2612 else if (Configuration.doAutoCreate()) 2613 this.encounterTarget = new Encounter(); // aa 2614 return this.encounterTarget; 2615 } 2616 2617 /** 2618 * @param value {@link #encounter} The actual object that is the target of the 2619 * reference. The reference library doesn't use these, but you can 2620 * use it to hold the resource if you resolve it. (An encounter 2621 * that provides additional information about the healthcare 2622 * context in which this request is made.) 2623 */ 2624 public NutritionOrder setEncounterTarget(Encounter value) { 2625 this.encounterTarget = value; 2626 return this; 2627 } 2628 2629 /** 2630 * @return {@link #dateTime} (The date and time that this nutrition order was 2631 * requested.). This is the underlying object with id, value and 2632 * extensions. The accessor "getDateTime" gives direct access to the 2633 * value 2634 */ 2635 public DateTimeType getDateTimeElement() { 2636 if (this.dateTime == null) 2637 if (Configuration.errorOnAutoCreate()) 2638 throw new Error("Attempt to auto-create NutritionOrder.dateTime"); 2639 else if (Configuration.doAutoCreate()) 2640 this.dateTime = new DateTimeType(); // bb 2641 return this.dateTime; 2642 } 2643 2644 public boolean hasDateTimeElement() { 2645 return this.dateTime != null && !this.dateTime.isEmpty(); 2646 } 2647 2648 public boolean hasDateTime() { 2649 return this.dateTime != null && !this.dateTime.isEmpty(); 2650 } 2651 2652 /** 2653 * @param value {@link #dateTime} (The date and time that this nutrition order 2654 * was requested.). This is the underlying object with id, value 2655 * and extensions. The accessor "getDateTime" gives direct access 2656 * to the value 2657 */ 2658 public NutritionOrder setDateTimeElement(DateTimeType value) { 2659 this.dateTime = value; 2660 return this; 2661 } 2662 2663 /** 2664 * @return The date and time that this nutrition order was requested. 2665 */ 2666 public Date getDateTime() { 2667 return this.dateTime == null ? null : this.dateTime.getValue(); 2668 } 2669 2670 /** 2671 * @param value The date and time that this nutrition order was requested. 2672 */ 2673 public NutritionOrder setDateTime(Date value) { 2674 if (this.dateTime == null) 2675 this.dateTime = new DateTimeType(); 2676 this.dateTime.setValue(value); 2677 return this; 2678 } 2679 2680 /** 2681 * @return {@link #status} (The workflow status of the nutrition 2682 * order/request.). This is the underlying object with id, value and 2683 * extensions. The accessor "getStatus" gives direct access to the value 2684 */ 2685 public Enumeration<NutritionOrderStatus> getStatusElement() { 2686 if (this.status == null) 2687 if (Configuration.errorOnAutoCreate()) 2688 throw new Error("Attempt to auto-create NutritionOrder.status"); 2689 else if (Configuration.doAutoCreate()) 2690 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); // bb 2691 return this.status; 2692 } 2693 2694 public boolean hasStatusElement() { 2695 return this.status != null && !this.status.isEmpty(); 2696 } 2697 2698 public boolean hasStatus() { 2699 return this.status != null && !this.status.isEmpty(); 2700 } 2701 2702 /** 2703 * @param value {@link #status} (The workflow status of the nutrition 2704 * order/request.). This is the underlying object with id, value 2705 * and extensions. The accessor "getStatus" gives direct access to 2706 * the value 2707 */ 2708 public NutritionOrder setStatusElement(Enumeration<NutritionOrderStatus> value) { 2709 this.status = value; 2710 return this; 2711 } 2712 2713 /** 2714 * @return The workflow status of the nutrition order/request. 2715 */ 2716 public NutritionOrderStatus getStatus() { 2717 return this.status == null ? null : this.status.getValue(); 2718 } 2719 2720 /** 2721 * @param value The workflow status of the nutrition order/request. 2722 */ 2723 public NutritionOrder setStatus(NutritionOrderStatus value) { 2724 if (value == null) 2725 this.status = null; 2726 else { 2727 if (this.status == null) 2728 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); 2729 this.status.setValue(value); 2730 } 2731 return this; 2732 } 2733 2734 /** 2735 * @return {@link #allergyIntolerance} (A link to a record of allergies or 2736 * intolerances which should be included in the nutrition order.) 2737 */ 2738 public List<Reference> getAllergyIntolerance() { 2739 if (this.allergyIntolerance == null) 2740 this.allergyIntolerance = new ArrayList<Reference>(); 2741 return this.allergyIntolerance; 2742 } 2743 2744 public boolean hasAllergyIntolerance() { 2745 if (this.allergyIntolerance == null) 2746 return false; 2747 for (Reference item : this.allergyIntolerance) 2748 if (!item.isEmpty()) 2749 return true; 2750 return false; 2751 } 2752 2753 /** 2754 * @return {@link #allergyIntolerance} (A link to a record of allergies or 2755 * intolerances which should be included in the nutrition order.) 2756 */ 2757 // syntactic sugar 2758 public Reference addAllergyIntolerance() { // 3 2759 Reference t = new Reference(); 2760 if (this.allergyIntolerance == null) 2761 this.allergyIntolerance = new ArrayList<Reference>(); 2762 this.allergyIntolerance.add(t); 2763 return t; 2764 } 2765 2766 // syntactic sugar 2767 public NutritionOrder addAllergyIntolerance(Reference t) { // 3 2768 if (t == null) 2769 return this; 2770 if (this.allergyIntolerance == null) 2771 this.allergyIntolerance = new ArrayList<Reference>(); 2772 this.allergyIntolerance.add(t); 2773 return this; 2774 } 2775 2776 /** 2777 * @return {@link #allergyIntolerance} (The actual objects that are the target 2778 * of the reference. The reference library doesn't populate this, but 2779 * you can use this to hold the resources if you resolvethemt. A link to 2780 * a record of allergies or intolerances which should be included in the 2781 * nutrition order.) 2782 */ 2783 public List<AllergyIntolerance> getAllergyIntoleranceTarget() { 2784 if (this.allergyIntoleranceTarget == null) 2785 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 2786 return this.allergyIntoleranceTarget; 2787 } 2788 2789 // syntactic sugar 2790 /** 2791 * @return {@link #allergyIntolerance} (Add an actual object that is the target 2792 * of the reference. The reference library doesn't use these, but you 2793 * can use this to hold the resources if you resolvethemt. A link to a 2794 * record of allergies or intolerances which should be included in the 2795 * nutrition order.) 2796 */ 2797 public AllergyIntolerance addAllergyIntoleranceTarget() { 2798 AllergyIntolerance r = new AllergyIntolerance(); 2799 if (this.allergyIntoleranceTarget == null) 2800 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 2801 this.allergyIntoleranceTarget.add(r); 2802 return r; 2803 } 2804 2805 /** 2806 * @return {@link #foodPreferenceModifier} (This modifier is used to convey 2807 * order-specific modifiers about the type of food that should be given. 2808 * These can be derived from patient allergies, intolerances, or 2809 * preferences such as Halal, Vegan or Kosher. This modifier applies to 2810 * the entire nutrition order inclusive of the oral diet, nutritional 2811 * supplements and enteral formula feedings.) 2812 */ 2813 public List<CodeableConcept> getFoodPreferenceModifier() { 2814 if (this.foodPreferenceModifier == null) 2815 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 2816 return this.foodPreferenceModifier; 2817 } 2818 2819 public boolean hasFoodPreferenceModifier() { 2820 if (this.foodPreferenceModifier == null) 2821 return false; 2822 for (CodeableConcept item : this.foodPreferenceModifier) 2823 if (!item.isEmpty()) 2824 return true; 2825 return false; 2826 } 2827 2828 /** 2829 * @return {@link #foodPreferenceModifier} (This modifier is used to convey 2830 * order-specific modifiers about the type of food that should be given. 2831 * These can be derived from patient allergies, intolerances, or 2832 * preferences such as Halal, Vegan or Kosher. This modifier applies to 2833 * the entire nutrition order inclusive of the oral diet, nutritional 2834 * supplements and enteral formula feedings.) 2835 */ 2836 // syntactic sugar 2837 public CodeableConcept addFoodPreferenceModifier() { // 3 2838 CodeableConcept t = new CodeableConcept(); 2839 if (this.foodPreferenceModifier == null) 2840 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 2841 this.foodPreferenceModifier.add(t); 2842 return t; 2843 } 2844 2845 // syntactic sugar 2846 public NutritionOrder addFoodPreferenceModifier(CodeableConcept t) { // 3 2847 if (t == null) 2848 return this; 2849 if (this.foodPreferenceModifier == null) 2850 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 2851 this.foodPreferenceModifier.add(t); 2852 return this; 2853 } 2854 2855 /** 2856 * @return {@link #excludeFoodModifier} (This modifier is used to convey 2857 * order-specific modifiers about the type of food that should NOT be 2858 * given. These can be derived from patient allergies, intolerances, or 2859 * preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. 2860 * While it should not be necessary to repeat allergy or intolerance 2861 * information captured in the referenced allergyIntolerance resource in 2862 * the excludeFoodModifier, this element may be used to convey 2863 * additional specificity related to foods that should be eliminated 2864 * from the patient?s diet for any reason. This modifier applies to the 2865 * entire nutrition order inclusive of the oral diet, nutritional 2866 * supplements and enteral formula feedings.) 2867 */ 2868 public List<CodeableConcept> getExcludeFoodModifier() { 2869 if (this.excludeFoodModifier == null) 2870 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 2871 return this.excludeFoodModifier; 2872 } 2873 2874 public boolean hasExcludeFoodModifier() { 2875 if (this.excludeFoodModifier == null) 2876 return false; 2877 for (CodeableConcept item : this.excludeFoodModifier) 2878 if (!item.isEmpty()) 2879 return true; 2880 return false; 2881 } 2882 2883 /** 2884 * @return {@link #excludeFoodModifier} (This modifier is used to convey 2885 * order-specific modifiers about the type of food that should NOT be 2886 * given. These can be derived from patient allergies, intolerances, or 2887 * preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. 2888 * While it should not be necessary to repeat allergy or intolerance 2889 * information captured in the referenced allergyIntolerance resource in 2890 * the excludeFoodModifier, this element may be used to convey 2891 * additional specificity related to foods that should be eliminated 2892 * from the patient?s diet for any reason. This modifier applies to the 2893 * entire nutrition order inclusive of the oral diet, nutritional 2894 * supplements and enteral formula feedings.) 2895 */ 2896 // syntactic sugar 2897 public CodeableConcept addExcludeFoodModifier() { // 3 2898 CodeableConcept t = new CodeableConcept(); 2899 if (this.excludeFoodModifier == null) 2900 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 2901 this.excludeFoodModifier.add(t); 2902 return t; 2903 } 2904 2905 // syntactic sugar 2906 public NutritionOrder addExcludeFoodModifier(CodeableConcept t) { // 3 2907 if (t == null) 2908 return this; 2909 if (this.excludeFoodModifier == null) 2910 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 2911 this.excludeFoodModifier.add(t); 2912 return this; 2913 } 2914 2915 /** 2916 * @return {@link #oralDiet} (Diet given orally in contrast to enteral (tube) 2917 * feeding.) 2918 */ 2919 public NutritionOrderOralDietComponent getOralDiet() { 2920 if (this.oralDiet == null) 2921 if (Configuration.errorOnAutoCreate()) 2922 throw new Error("Attempt to auto-create NutritionOrder.oralDiet"); 2923 else if (Configuration.doAutoCreate()) 2924 this.oralDiet = new NutritionOrderOralDietComponent(); // cc 2925 return this.oralDiet; 2926 } 2927 2928 public boolean hasOralDiet() { 2929 return this.oralDiet != null && !this.oralDiet.isEmpty(); 2930 } 2931 2932 /** 2933 * @param value {@link #oralDiet} (Diet given orally in contrast to enteral 2934 * (tube) feeding.) 2935 */ 2936 public NutritionOrder setOralDiet(NutritionOrderOralDietComponent value) { 2937 this.oralDiet = value; 2938 return this; 2939 } 2940 2941 /** 2942 * @return {@link #supplement} (Oral nutritional products given in order to add 2943 * further nutritional value to the patient's diet.) 2944 */ 2945 public List<NutritionOrderSupplementComponent> getSupplement() { 2946 if (this.supplement == null) 2947 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 2948 return this.supplement; 2949 } 2950 2951 public boolean hasSupplement() { 2952 if (this.supplement == null) 2953 return false; 2954 for (NutritionOrderSupplementComponent item : this.supplement) 2955 if (!item.isEmpty()) 2956 return true; 2957 return false; 2958 } 2959 2960 /** 2961 * @return {@link #supplement} (Oral nutritional products given in order to add 2962 * further nutritional value to the patient's diet.) 2963 */ 2964 // syntactic sugar 2965 public NutritionOrderSupplementComponent addSupplement() { // 3 2966 NutritionOrderSupplementComponent t = new NutritionOrderSupplementComponent(); 2967 if (this.supplement == null) 2968 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 2969 this.supplement.add(t); 2970 return t; 2971 } 2972 2973 // syntactic sugar 2974 public NutritionOrder addSupplement(NutritionOrderSupplementComponent t) { // 3 2975 if (t == null) 2976 return this; 2977 if (this.supplement == null) 2978 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 2979 this.supplement.add(t); 2980 return this; 2981 } 2982 2983 /** 2984 * @return {@link #enteralFormula} (Feeding provided through the 2985 * gastrointestinal tract via a tube, catheter, or stoma that delivers 2986 * nutrition distal to the oral cavity.) 2987 */ 2988 public NutritionOrderEnteralFormulaComponent getEnteralFormula() { 2989 if (this.enteralFormula == null) 2990 if (Configuration.errorOnAutoCreate()) 2991 throw new Error("Attempt to auto-create NutritionOrder.enteralFormula"); 2992 else if (Configuration.doAutoCreate()) 2993 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); // cc 2994 return this.enteralFormula; 2995 } 2996 2997 public boolean hasEnteralFormula() { 2998 return this.enteralFormula != null && !this.enteralFormula.isEmpty(); 2999 } 3000 3001 /** 3002 * @param value {@link #enteralFormula} (Feeding provided through the 3003 * gastrointestinal tract via a tube, catheter, or stoma that 3004 * delivers nutrition distal to the oral cavity.) 3005 */ 3006 public NutritionOrder setEnteralFormula(NutritionOrderEnteralFormulaComponent value) { 3007 this.enteralFormula = value; 3008 return this; 3009 } 3010 3011 protected void listChildren(List<Property> childrenList) { 3012 super.listChildren(childrenList); 3013 childrenList.add(new Property("patient", "Reference(Patient)", 3014 "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 3015 0, java.lang.Integer.MAX_VALUE, patient)); 3016 childrenList.add(new Property("orderer", "Reference(Practitioner)", 3017 "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 3018 0, java.lang.Integer.MAX_VALUE, orderer)); 3019 childrenList.add(new Property("identifier", "Identifier", 3020 "Identifiers assigned to this order by the order sender or by the order receiver.", 0, 3021 java.lang.Integer.MAX_VALUE, identifier)); 3022 childrenList.add(new Property("encounter", "Reference(Encounter)", 3023 "An encounter that provides additional information about the healthcare context in which this request is made.", 3024 0, java.lang.Integer.MAX_VALUE, encounter)); 3025 childrenList.add(new Property("dateTime", "dateTime", "The date and time that this nutrition order was requested.", 3026 0, java.lang.Integer.MAX_VALUE, dateTime)); 3027 childrenList.add(new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 3028 java.lang.Integer.MAX_VALUE, status)); 3029 childrenList.add(new Property("allergyIntolerance", "Reference(AllergyIntolerance)", 3030 "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, 3031 java.lang.Integer.MAX_VALUE, allergyIntolerance)); 3032 childrenList.add(new Property("foodPreferenceModifier", "CodeableConcept", 3033 "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 3034 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier)); 3035 childrenList.add(new Property("excludeFoodModifier", "CodeableConcept", 3036 "This modifier is used to convey order-specific modifiers about the type of food that should NOT be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced allergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 3037 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier)); 3038 childrenList.add(new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 3039 java.lang.Integer.MAX_VALUE, oralDiet)); 3040 childrenList.add(new Property("supplement", "", 3041 "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, 3042 java.lang.Integer.MAX_VALUE, supplement)); 3043 childrenList.add(new Property("enteralFormula", "", 3044 "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 3045 0, java.lang.Integer.MAX_VALUE, enteralFormula)); 3046 } 3047 3048 @Override 3049 public void setProperty(String name, Base value) throws FHIRException { 3050 if (name.equals("patient")) 3051 this.patient = castToReference(value); // Reference 3052 else if (name.equals("orderer")) 3053 this.orderer = castToReference(value); // Reference 3054 else if (name.equals("identifier")) 3055 this.getIdentifier().add(castToIdentifier(value)); 3056 else if (name.equals("encounter")) 3057 this.encounter = castToReference(value); // Reference 3058 else if (name.equals("dateTime")) 3059 this.dateTime = castToDateTime(value); // DateTimeType 3060 else if (name.equals("status")) 3061 this.status = new NutritionOrderStatusEnumFactory().fromType(value); // Enumeration<NutritionOrderStatus> 3062 else if (name.equals("allergyIntolerance")) 3063 this.getAllergyIntolerance().add(castToReference(value)); 3064 else if (name.equals("foodPreferenceModifier")) 3065 this.getFoodPreferenceModifier().add(castToCodeableConcept(value)); 3066 else if (name.equals("excludeFoodModifier")) 3067 this.getExcludeFoodModifier().add(castToCodeableConcept(value)); 3068 else if (name.equals("oralDiet")) 3069 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 3070 else if (name.equals("supplement")) 3071 this.getSupplement().add((NutritionOrderSupplementComponent) value); 3072 else if (name.equals("enteralFormula")) 3073 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 3074 else 3075 super.setProperty(name, value); 3076 } 3077 3078 @Override 3079 public Base addChild(String name) throws FHIRException { 3080 if (name.equals("patient")) { 3081 this.patient = new Reference(); 3082 return this.patient; 3083 } else if (name.equals("orderer")) { 3084 this.orderer = new Reference(); 3085 return this.orderer; 3086 } else if (name.equals("identifier")) { 3087 return addIdentifier(); 3088 } else if (name.equals("encounter")) { 3089 this.encounter = new Reference(); 3090 return this.encounter; 3091 } else if (name.equals("dateTime")) { 3092 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.dateTime"); 3093 } else if (name.equals("status")) { 3094 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.status"); 3095 } else if (name.equals("allergyIntolerance")) { 3096 return addAllergyIntolerance(); 3097 } else if (name.equals("foodPreferenceModifier")) { 3098 return addFoodPreferenceModifier(); 3099 } else if (name.equals("excludeFoodModifier")) { 3100 return addExcludeFoodModifier(); 3101 } else if (name.equals("oralDiet")) { 3102 this.oralDiet = new NutritionOrderOralDietComponent(); 3103 return this.oralDiet; 3104 } else if (name.equals("supplement")) { 3105 return addSupplement(); 3106 } else if (name.equals("enteralFormula")) { 3107 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); 3108 return this.enteralFormula; 3109 } else 3110 return super.addChild(name); 3111 } 3112 3113 public String fhirType() { 3114 return "NutritionOrder"; 3115 3116 } 3117 3118 public NutritionOrder copy() { 3119 NutritionOrder dst = new NutritionOrder(); 3120 copyValues(dst); 3121 dst.patient = patient == null ? null : patient.copy(); 3122 dst.orderer = orderer == null ? null : orderer.copy(); 3123 if (identifier != null) { 3124 dst.identifier = new ArrayList<Identifier>(); 3125 for (Identifier i : identifier) 3126 dst.identifier.add(i.copy()); 3127 } 3128 ; 3129 dst.encounter = encounter == null ? null : encounter.copy(); 3130 dst.dateTime = dateTime == null ? null : dateTime.copy(); 3131 dst.status = status == null ? null : status.copy(); 3132 if (allergyIntolerance != null) { 3133 dst.allergyIntolerance = new ArrayList<Reference>(); 3134 for (Reference i : allergyIntolerance) 3135 dst.allergyIntolerance.add(i.copy()); 3136 } 3137 ; 3138 if (foodPreferenceModifier != null) { 3139 dst.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 3140 for (CodeableConcept i : foodPreferenceModifier) 3141 dst.foodPreferenceModifier.add(i.copy()); 3142 } 3143 ; 3144 if (excludeFoodModifier != null) { 3145 dst.excludeFoodModifier = new ArrayList<CodeableConcept>(); 3146 for (CodeableConcept i : excludeFoodModifier) 3147 dst.excludeFoodModifier.add(i.copy()); 3148 } 3149 ; 3150 dst.oralDiet = oralDiet == null ? null : oralDiet.copy(); 3151 if (supplement != null) { 3152 dst.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 3153 for (NutritionOrderSupplementComponent i : supplement) 3154 dst.supplement.add(i.copy()); 3155 } 3156 ; 3157 dst.enteralFormula = enteralFormula == null ? null : enteralFormula.copy(); 3158 return dst; 3159 } 3160 3161 protected NutritionOrder typedCopy() { 3162 return copy(); 3163 } 3164 3165 @Override 3166 public boolean equalsDeep(Base other) { 3167 if (!super.equalsDeep(other)) 3168 return false; 3169 if (!(other instanceof NutritionOrder)) 3170 return false; 3171 NutritionOrder o = (NutritionOrder) other; 3172 return compareDeep(patient, o.patient, true) && compareDeep(orderer, o.orderer, true) 3173 && compareDeep(identifier, o.identifier, true) && compareDeep(encounter, o.encounter, true) 3174 && compareDeep(dateTime, o.dateTime, true) && compareDeep(status, o.status, true) 3175 && compareDeep(allergyIntolerance, o.allergyIntolerance, true) 3176 && compareDeep(foodPreferenceModifier, o.foodPreferenceModifier, true) 3177 && compareDeep(excludeFoodModifier, o.excludeFoodModifier, true) && compareDeep(oralDiet, o.oralDiet, true) 3178 && compareDeep(supplement, o.supplement, true) && compareDeep(enteralFormula, o.enteralFormula, true); 3179 } 3180 3181 @Override 3182 public boolean equalsShallow(Base other) { 3183 if (!super.equalsShallow(other)) 3184 return false; 3185 if (!(other instanceof NutritionOrder)) 3186 return false; 3187 NutritionOrder o = (NutritionOrder) other; 3188 return compareValues(dateTime, o.dateTime, true) && compareValues(status, o.status, true); 3189 } 3190 3191 public boolean isEmpty() { 3192 return super.isEmpty() && (patient == null || patient.isEmpty()) && (orderer == null || orderer.isEmpty()) 3193 && (identifier == null || identifier.isEmpty()) && (encounter == null || encounter.isEmpty()) 3194 && (dateTime == null || dateTime.isEmpty()) && (status == null || status.isEmpty()) 3195 && (allergyIntolerance == null || allergyIntolerance.isEmpty()) 3196 && (foodPreferenceModifier == null || foodPreferenceModifier.isEmpty()) 3197 && (excludeFoodModifier == null || excludeFoodModifier.isEmpty()) && (oralDiet == null || oralDiet.isEmpty()) 3198 && (supplement == null || supplement.isEmpty()) && (enteralFormula == null || enteralFormula.isEmpty()); 3199 } 3200 3201 @Override 3202 public ResourceType getResourceType() { 3203 return ResourceType.NutritionOrder; 3204 } 3205 3206 @SearchParamDefinition(name = "identifier", path = "NutritionOrder.identifier", description = "Return nutrition orders with this external identifier", type = "token") 3207 public static final String SP_IDENTIFIER = "identifier"; 3208 @SearchParamDefinition(name = "datetime", path = "NutritionOrder.dateTime", description = "Return nutrition orders requested on this date", type = "date") 3209 public static final String SP_DATETIME = "datetime"; 3210 @SearchParamDefinition(name = "provider", path = "NutritionOrder.orderer", description = "The identify of the provider who placed the nutrition order", type = "reference") 3211 public static final String SP_PROVIDER = "provider"; 3212 @SearchParamDefinition(name = "patient", path = "NutritionOrder.patient", description = "The identity of the person who requires the diet, formula or nutritional supplement", type = "reference") 3213 public static final String SP_PATIENT = "patient"; 3214 @SearchParamDefinition(name = "supplement", path = "NutritionOrder.supplement.type", description = "Type of supplement product requested", type = "token") 3215 public static final String SP_SUPPLEMENT = "supplement"; 3216 @SearchParamDefinition(name = "formula", path = "NutritionOrder.enteralFormula.baseFormulaType", description = "Type of enteral or infant formula", type = "token") 3217 public static final String SP_FORMULA = "formula"; 3218 @SearchParamDefinition(name = "encounter", path = "NutritionOrder.encounter", description = "Return nutrition orders with this encounter identifier", type = "reference") 3219 public static final String SP_ENCOUNTER = "encounter"; 3220 @SearchParamDefinition(name = "oraldiet", path = "NutritionOrder.oralDiet.type", description = "Type of diet that can be consumed orally (i.e., take via the mouth).", type = "token") 3221 public static final String SP_ORALDIET = "oraldiet"; 3222 @SearchParamDefinition(name = "status", path = "NutritionOrder.status", description = "Status of the nutrition order.", type = "token") 3223 public static final String SP_STATUS = "status"; 3224 @SearchParamDefinition(name = "additive", path = "NutritionOrder.enteralFormula.additiveType", description = "Type of module component to add to the feeding", type = "token") 3225 public static final String SP_ADDITIVE = "additive"; 3226 3227}