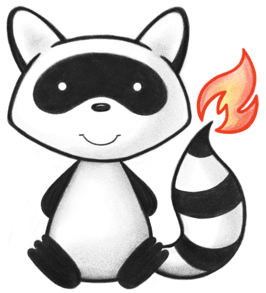
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Measurements and simple assertions made about a patient, device or other 048 * subject. 049 */ 050@ResourceDef(name = "Observation", profile = "http://hl7.org/fhir/Profile/Observation") 051public class Observation extends DomainResource { 052 053 public enum ObservationStatus { 054 /** 055 * The existence of the observation is registered, but there is no result yet 056 * available. 057 */ 058 REGISTERED, 059 /** 060 * This is an initial or interim observation: data may be incomplete or 061 * unverified. 062 */ 063 PRELIMINARY, 064 /** 065 * The observation is complete and verified by an authorized person. 066 */ 067 FINAL, 068 /** 069 * The observation has been modified subsequent to being Final, and is complete 070 * and verified by an authorized person. 071 */ 072 AMENDED, 073 /** 074 * The observation is unavailable because the measurement was not started or not 075 * completed (also sometimes called "aborted"). 076 */ 077 CANCELLED, 078 /** 079 * The observation has been withdrawn following previous final release. 080 */ 081 ENTEREDINERROR, 082 /** 083 * The observation status is unknown. Note that "unknown" is a value of last 084 * resort and every attempt should be made to provide a meaningful value other 085 * than "unknown". 086 */ 087 UNKNOWN, 088 /** 089 * added to help the parsers 090 */ 091 NULL; 092 093 public static ObservationStatus fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("registered".equals(codeString)) 097 return REGISTERED; 098 if ("preliminary".equals(codeString)) 099 return PRELIMINARY; 100 if ("final".equals(codeString)) 101 return FINAL; 102 if ("amended".equals(codeString)) 103 return AMENDED; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("entered-in-error".equals(codeString)) 107 return ENTEREDINERROR; 108 if ("unknown".equals(codeString)) 109 return UNKNOWN; 110 throw new FHIRException("Unknown ObservationStatus code '" + codeString + "'"); 111 } 112 113 public String toCode() { 114 switch (this) { 115 case REGISTERED: 116 return "registered"; 117 case PRELIMINARY: 118 return "preliminary"; 119 case FINAL: 120 return "final"; 121 case AMENDED: 122 return "amended"; 123 case CANCELLED: 124 return "cancelled"; 125 case ENTEREDINERROR: 126 return "entered-in-error"; 127 case UNKNOWN: 128 return "unknown"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getSystem() { 137 switch (this) { 138 case REGISTERED: 139 return "http://hl7.org/fhir/observation-status"; 140 case PRELIMINARY: 141 return "http://hl7.org/fhir/observation-status"; 142 case FINAL: 143 return "http://hl7.org/fhir/observation-status"; 144 case AMENDED: 145 return "http://hl7.org/fhir/observation-status"; 146 case CANCELLED: 147 return "http://hl7.org/fhir/observation-status"; 148 case ENTEREDINERROR: 149 return "http://hl7.org/fhir/observation-status"; 150 case UNKNOWN: 151 return "http://hl7.org/fhir/observation-status"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getDefinition() { 160 switch (this) { 161 case REGISTERED: 162 return "The existence of the observation is registered, but there is no result yet available."; 163 case PRELIMINARY: 164 return "This is an initial or interim observation: data may be incomplete or unverified."; 165 case FINAL: 166 return "The observation is complete and verified by an authorized person."; 167 case AMENDED: 168 return "The observation has been modified subsequent to being Final, and is complete and verified by an authorized person."; 169 case CANCELLED: 170 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 171 case ENTEREDINERROR: 172 return "The observation has been withdrawn following previous final release."; 173 case UNKNOWN: 174 return "The observation status is unknown. Note that \"unknown\" is a value of last resort and every attempt should be made to provide a meaningful value other than \"unknown\"."; 175 case NULL: 176 return null; 177 default: 178 return "?"; 179 } 180 } 181 182 public String getDisplay() { 183 switch (this) { 184 case REGISTERED: 185 return "Registered"; 186 case PRELIMINARY: 187 return "Preliminary"; 188 case FINAL: 189 return "Final"; 190 case AMENDED: 191 return "Amended"; 192 case CANCELLED: 193 return "cancelled"; 194 case ENTEREDINERROR: 195 return "Entered in Error"; 196 case UNKNOWN: 197 return "Unknown Status"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 } 205 206 public static class ObservationStatusEnumFactory implements EnumFactory<ObservationStatus> { 207 public ObservationStatus fromCode(String codeString) throws IllegalArgumentException { 208 if (codeString == null || "".equals(codeString)) 209 if (codeString == null || "".equals(codeString)) 210 return null; 211 if ("registered".equals(codeString)) 212 return ObservationStatus.REGISTERED; 213 if ("preliminary".equals(codeString)) 214 return ObservationStatus.PRELIMINARY; 215 if ("final".equals(codeString)) 216 return ObservationStatus.FINAL; 217 if ("amended".equals(codeString)) 218 return ObservationStatus.AMENDED; 219 if ("cancelled".equals(codeString)) 220 return ObservationStatus.CANCELLED; 221 if ("entered-in-error".equals(codeString)) 222 return ObservationStatus.ENTEREDINERROR; 223 if ("unknown".equals(codeString)) 224 return ObservationStatus.UNKNOWN; 225 throw new IllegalArgumentException("Unknown ObservationStatus code '" + codeString + "'"); 226 } 227 228 public Enumeration<ObservationStatus> fromType(Base code) throws FHIRException { 229 if (code == null || code.isEmpty()) 230 return null; 231 String codeString = ((PrimitiveType) code).asStringValue(); 232 if (codeString == null || "".equals(codeString)) 233 return null; 234 if ("registered".equals(codeString)) 235 return new Enumeration<ObservationStatus>(this, ObservationStatus.REGISTERED); 236 if ("preliminary".equals(codeString)) 237 return new Enumeration<ObservationStatus>(this, ObservationStatus.PRELIMINARY); 238 if ("final".equals(codeString)) 239 return new Enumeration<ObservationStatus>(this, ObservationStatus.FINAL); 240 if ("amended".equals(codeString)) 241 return new Enumeration<ObservationStatus>(this, ObservationStatus.AMENDED); 242 if ("cancelled".equals(codeString)) 243 return new Enumeration<ObservationStatus>(this, ObservationStatus.CANCELLED); 244 if ("entered-in-error".equals(codeString)) 245 return new Enumeration<ObservationStatus>(this, ObservationStatus.ENTEREDINERROR); 246 if ("unknown".equals(codeString)) 247 return new Enumeration<ObservationStatus>(this, ObservationStatus.UNKNOWN); 248 throw new FHIRException("Unknown ObservationStatus code '" + codeString + "'"); 249 } 250 251 public String toCode(ObservationStatus code) 252 { 253 if (code == ObservationStatus.NULL) 254 return null; 255 if (code == ObservationStatus.REGISTERED) 256 return "registered"; 257 if (code == ObservationStatus.PRELIMINARY) 258 return "preliminary"; 259 if (code == ObservationStatus.FINAL) 260 return "final"; 261 if (code == ObservationStatus.AMENDED) 262 return "amended"; 263 if (code == ObservationStatus.CANCELLED) 264 return "cancelled"; 265 if (code == ObservationStatus.ENTEREDINERROR) 266 return "entered-in-error"; 267 if (code == ObservationStatus.UNKNOWN) 268 return "unknown"; 269 return "?"; 270 } 271 } 272 273 public enum ObservationRelationshipType { 274 /** 275 * This observation is a group observation (e.g. a battery, a panel of tests, a 276 * set of vital sign measurements) that includes the target as a member of the 277 * group. 278 */ 279 HASMEMBER, 280 /** 281 * The target resource (Observation or QuestionnaireResponse) is part of the 282 * information from which this observation value is derived. (e.g. calculated 283 * anion gap, Apgar score) NOTE: "derived-from" is only logical choice when 284 * referencing QuestionnaireResponse. 285 */ 286 DERIVEDFROM, 287 /** 288 * This observation follows the target observation (e.g. timed tests such as 289 * Glucose Tolerance Test). 290 */ 291 SEQUELTO, 292 /** 293 * This observation replaces a previous observation (i.e. a revised value). The 294 * target observation is now obsolete. 295 */ 296 REPLACES, 297 /** 298 * The value of the target observation qualifies (refines) the semantics of the 299 * source observation (e.g. a lipemia measure target from a plasma measure). 300 */ 301 QUALIFIEDBY, 302 /** 303 * The value of the target observation interferes (degrades quality, or prevents 304 * valid observation) with the semantics of the source observation (e.g. a 305 * hemolysis measure target from a plasma potassium measure which has no value). 306 */ 307 INTERFEREDBY, 308 /** 309 * added to help the parsers 310 */ 311 NULL; 312 313 public static ObservationRelationshipType fromCode(String codeString) throws FHIRException { 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("has-member".equals(codeString)) 317 return HASMEMBER; 318 if ("derived-from".equals(codeString)) 319 return DERIVEDFROM; 320 if ("sequel-to".equals(codeString)) 321 return SEQUELTO; 322 if ("replaces".equals(codeString)) 323 return REPLACES; 324 if ("qualified-by".equals(codeString)) 325 return QUALIFIEDBY; 326 if ("interfered-by".equals(codeString)) 327 return INTERFEREDBY; 328 throw new FHIRException("Unknown ObservationRelationshipType code '" + codeString + "'"); 329 } 330 331 public String toCode() { 332 switch (this) { 333 case HASMEMBER: 334 return "has-member"; 335 case DERIVEDFROM: 336 return "derived-from"; 337 case SEQUELTO: 338 return "sequel-to"; 339 case REPLACES: 340 return "replaces"; 341 case QUALIFIEDBY: 342 return "qualified-by"; 343 case INTERFEREDBY: 344 return "interfered-by"; 345 case NULL: 346 return null; 347 default: 348 return "?"; 349 } 350 } 351 352 public String getSystem() { 353 switch (this) { 354 case HASMEMBER: 355 return "http://hl7.org/fhir/observation-relationshiptypes"; 356 case DERIVEDFROM: 357 return "http://hl7.org/fhir/observation-relationshiptypes"; 358 case SEQUELTO: 359 return "http://hl7.org/fhir/observation-relationshiptypes"; 360 case REPLACES: 361 return "http://hl7.org/fhir/observation-relationshiptypes"; 362 case QUALIFIEDBY: 363 return "http://hl7.org/fhir/observation-relationshiptypes"; 364 case INTERFEREDBY: 365 return "http://hl7.org/fhir/observation-relationshiptypes"; 366 case NULL: 367 return null; 368 default: 369 return "?"; 370 } 371 } 372 373 public String getDefinition() { 374 switch (this) { 375 case HASMEMBER: 376 return "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group."; 377 case DERIVEDFROM: 378 return "The target resource (Observation or QuestionnaireResponse) is part of the information from which this observation value is derived. (e.g. calculated anion gap, Apgar score) NOTE: \"derived-from\" is only logical choice when referencing QuestionnaireResponse."; 379 case SEQUELTO: 380 return "This observation follows the target observation (e.g. timed tests such as Glucose Tolerance Test)."; 381 case REPLACES: 382 return "This observation replaces a previous observation (i.e. a revised value). The target observation is now obsolete."; 383 case QUALIFIEDBY: 384 return "The value of the target observation qualifies (refines) the semantics of the source observation (e.g. a lipemia measure target from a plasma measure)."; 385 case INTERFEREDBY: 386 return "The value of the target observation interferes (degrades quality, or prevents valid observation) with the semantics of the source observation (e.g. a hemolysis measure target from a plasma potassium measure which has no value)."; 387 case NULL: 388 return null; 389 default: 390 return "?"; 391 } 392 } 393 394 public String getDisplay() { 395 switch (this) { 396 case HASMEMBER: 397 return "Has Member"; 398 case DERIVEDFROM: 399 return "Derived From"; 400 case SEQUELTO: 401 return "Sequel To"; 402 case REPLACES: 403 return "Replaces"; 404 case QUALIFIEDBY: 405 return "Qualified By"; 406 case INTERFEREDBY: 407 return "Interfered By"; 408 case NULL: 409 return null; 410 default: 411 return "?"; 412 } 413 } 414 } 415 416 public static class ObservationRelationshipTypeEnumFactory implements EnumFactory<ObservationRelationshipType> { 417 public ObservationRelationshipType fromCode(String codeString) throws IllegalArgumentException { 418 if (codeString == null || "".equals(codeString)) 419 if (codeString == null || "".equals(codeString)) 420 return null; 421 if ("has-member".equals(codeString)) 422 return ObservationRelationshipType.HASMEMBER; 423 if ("derived-from".equals(codeString)) 424 return ObservationRelationshipType.DERIVEDFROM; 425 if ("sequel-to".equals(codeString)) 426 return ObservationRelationshipType.SEQUELTO; 427 if ("replaces".equals(codeString)) 428 return ObservationRelationshipType.REPLACES; 429 if ("qualified-by".equals(codeString)) 430 return ObservationRelationshipType.QUALIFIEDBY; 431 if ("interfered-by".equals(codeString)) 432 return ObservationRelationshipType.INTERFEREDBY; 433 throw new IllegalArgumentException("Unknown ObservationRelationshipType code '" + codeString + "'"); 434 } 435 436 public Enumeration<ObservationRelationshipType> fromType(Base code) throws FHIRException { 437 if (code == null || code.isEmpty()) 438 return null; 439 String codeString = ((PrimitiveType) code).asStringValue(); 440 if (codeString == null || "".equals(codeString)) 441 return null; 442 if ("has-member".equals(codeString)) 443 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.HASMEMBER); 444 if ("derived-from".equals(codeString)) 445 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.DERIVEDFROM); 446 if ("sequel-to".equals(codeString)) 447 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.SEQUELTO); 448 if ("replaces".equals(codeString)) 449 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.REPLACES); 450 if ("qualified-by".equals(codeString)) 451 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.QUALIFIEDBY); 452 if ("interfered-by".equals(codeString)) 453 return new Enumeration<ObservationRelationshipType>(this, ObservationRelationshipType.INTERFEREDBY); 454 throw new FHIRException("Unknown ObservationRelationshipType code '" + codeString + "'"); 455 } 456 457 public String toCode(ObservationRelationshipType code) 458 { 459 if (code == ObservationRelationshipType.NULL) 460 return null; 461 if (code == ObservationRelationshipType.HASMEMBER) 462 return "has-member"; 463 if (code == ObservationRelationshipType.DERIVEDFROM) 464 return "derived-from"; 465 if (code == ObservationRelationshipType.SEQUELTO) 466 return "sequel-to"; 467 if (code == ObservationRelationshipType.REPLACES) 468 return "replaces"; 469 if (code == ObservationRelationshipType.QUALIFIEDBY) 470 return "qualified-by"; 471 if (code == ObservationRelationshipType.INTERFEREDBY) 472 return "interfered-by"; 473 return "?"; 474 } 475 } 476 477 @Block() 478 public static class ObservationReferenceRangeComponent extends BackboneElement implements IBaseBackboneElement { 479 /** 480 * The value of the low bound of the reference range. The low bound of the 481 * reference range endpoint is inclusive of the value (e.g. reference range is 482 * >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless 483 * (e.g. reference range is <=2.3). 484 */ 485 @Child(name = "low", type = { 486 SimpleQuantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 487 @Description(shortDefinition = "Low Range, if relevant", formalDefinition = "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).") 488 protected SimpleQuantity low; 489 490 /** 491 * The value of the high bound of the reference range. The high bound of the 492 * reference range endpoint is inclusive of the value (e.g. reference range is 493 * >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless 494 * (e.g. reference range is >= 2.3). 495 */ 496 @Child(name = "high", type = { 497 SimpleQuantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 498 @Description(shortDefinition = "High Range, if relevant", formalDefinition = "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).") 499 protected SimpleQuantity high; 500 501 /** 502 * Code for the meaning of the reference range. 503 */ 504 @Child(name = "meaning", type = { 505 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 506 @Description(shortDefinition = "Indicates the meaning/use of this range of this range", formalDefinition = "Code for the meaning of the reference range.") 507 protected CodeableConcept meaning; 508 509 /** 510 * The age at which this reference range is applicable. This is a neonatal age 511 * (e.g. number of weeks at term) if the meaning says so. 512 */ 513 @Child(name = "age", type = { Range.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 514 @Description(shortDefinition = "Applicable age range, if relevant", formalDefinition = "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.") 515 protected Range age; 516 517 /** 518 * Text based reference range in an observation which may be used when a 519 * quantitative range is not appropriate for an observation. An example would be 520 * a reference value of "Negative" or a list or table of 'normals'. 521 */ 522 @Child(name = "text", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 523 @Description(shortDefinition = "Text based reference range in an observation", formalDefinition = "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of 'normals'.") 524 protected StringType text; 525 526 private static final long serialVersionUID = -238694788L; 527 528 /* 529 * Constructor 530 */ 531 public ObservationReferenceRangeComponent() { 532 super(); 533 } 534 535 /** 536 * @return {@link #low} (The value of the low bound of the reference range. The 537 * low bound of the reference range endpoint is inclusive of the value 538 * (e.g. reference range is >=5 - <=9). If the low bound is omitted, it 539 * is assumed to be meaningless (e.g. reference range is <=2.3).) 540 */ 541 public SimpleQuantity getLow() { 542 if (this.low == null) 543 if (Configuration.errorOnAutoCreate()) 544 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.low"); 545 else if (Configuration.doAutoCreate()) 546 this.low = new SimpleQuantity(); // cc 547 return this.low; 548 } 549 550 public boolean hasLow() { 551 return this.low != null && !this.low.isEmpty(); 552 } 553 554 /** 555 * @param value {@link #low} (The value of the low bound of the reference range. 556 * The low bound of the reference range endpoint is inclusive of 557 * the value (e.g. reference range is >=5 - <=9). If the low bound 558 * is omitted, it is assumed to be meaningless (e.g. reference 559 * range is <=2.3).) 560 */ 561 public ObservationReferenceRangeComponent setLow(SimpleQuantity value) { 562 this.low = value; 563 return this; 564 } 565 566 /** 567 * @return {@link #high} (The value of the high bound of the reference range. 568 * The high bound of the reference range endpoint is inclusive of the 569 * value (e.g. reference range is >=5 - <=9). If the high bound is 570 * omitted, it is assumed to be meaningless (e.g. reference range is >= 571 * 2.3).) 572 */ 573 public SimpleQuantity getHigh() { 574 if (this.high == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.high"); 577 else if (Configuration.doAutoCreate()) 578 this.high = new SimpleQuantity(); // cc 579 return this.high; 580 } 581 582 public boolean hasHigh() { 583 return this.high != null && !this.high.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #high} (The value of the high bound of the reference 588 * range. The high bound of the reference range endpoint is 589 * inclusive of the value (e.g. reference range is >=5 - <=9). If 590 * the high bound is omitted, it is assumed to be meaningless (e.g. 591 * reference range is >= 2.3).) 592 */ 593 public ObservationReferenceRangeComponent setHigh(SimpleQuantity value) { 594 this.high = value; 595 return this; 596 } 597 598 /** 599 * @return {@link #meaning} (Code for the meaning of the reference range.) 600 */ 601 public CodeableConcept getMeaning() { 602 if (this.meaning == null) 603 if (Configuration.errorOnAutoCreate()) 604 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.meaning"); 605 else if (Configuration.doAutoCreate()) 606 this.meaning = new CodeableConcept(); // cc 607 return this.meaning; 608 } 609 610 public boolean hasMeaning() { 611 return this.meaning != null && !this.meaning.isEmpty(); 612 } 613 614 /** 615 * @param value {@link #meaning} (Code for the meaning of the reference range.) 616 */ 617 public ObservationReferenceRangeComponent setMeaning(CodeableConcept value) { 618 this.meaning = value; 619 return this; 620 } 621 622 /** 623 * @return {@link #age} (The age at which this reference range is applicable. 624 * This is a neonatal age (e.g. number of weeks at term) if the meaning 625 * says so.) 626 */ 627 public Range getAge() { 628 if (this.age == null) 629 if (Configuration.errorOnAutoCreate()) 630 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.age"); 631 else if (Configuration.doAutoCreate()) 632 this.age = new Range(); // cc 633 return this.age; 634 } 635 636 public boolean hasAge() { 637 return this.age != null && !this.age.isEmpty(); 638 } 639 640 /** 641 * @param value {@link #age} (The age at which this reference range is 642 * applicable. This is a neonatal age (e.g. number of weeks at 643 * term) if the meaning says so.) 644 */ 645 public ObservationReferenceRangeComponent setAge(Range value) { 646 this.age = value; 647 return this; 648 } 649 650 /** 651 * @return {@link #text} (Text based reference range in an observation which may 652 * be used when a quantitative range is not appropriate for an 653 * observation. An example would be a reference value of "Negative" or a 654 * list or table of 'normals'.). This is the underlying object with id, 655 * value and extensions. The accessor "getText" gives direct access to 656 * the value 657 */ 658 public StringType getTextElement() { 659 if (this.text == null) 660 if (Configuration.errorOnAutoCreate()) 661 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.text"); 662 else if (Configuration.doAutoCreate()) 663 this.text = new StringType(); // bb 664 return this.text; 665 } 666 667 public boolean hasTextElement() { 668 return this.text != null && !this.text.isEmpty(); 669 } 670 671 public boolean hasText() { 672 return this.text != null && !this.text.isEmpty(); 673 } 674 675 /** 676 * @param value {@link #text} (Text based reference range in an observation 677 * which may be used when a quantitative range is not appropriate 678 * for an observation. An example would be a reference value of 679 * "Negative" or a list or table of 'normals'.). This is the 680 * underlying object with id, value and extensions. The accessor 681 * "getText" gives direct access to the value 682 */ 683 public ObservationReferenceRangeComponent setTextElement(StringType value) { 684 this.text = value; 685 return this; 686 } 687 688 /** 689 * @return Text based reference range in an observation which may be used when a 690 * quantitative range is not appropriate for an observation. An example 691 * would be a reference value of "Negative" or a list or table of 692 * 'normals'. 693 */ 694 public String getText() { 695 return this.text == null ? null : this.text.getValue(); 696 } 697 698 /** 699 * @param value Text based reference range in an observation which may be used 700 * when a quantitative range is not appropriate for an observation. 701 * An example would be a reference value of "Negative" or a list or 702 * table of 'normals'. 703 */ 704 public ObservationReferenceRangeComponent setText(String value) { 705 if (Utilities.noString(value)) 706 this.text = null; 707 else { 708 if (this.text == null) 709 this.text = new StringType(); 710 this.text.setValue(value); 711 } 712 return this; 713 } 714 715 protected void listChildren(List<Property> childrenList) { 716 super.listChildren(childrenList); 717 childrenList.add(new Property("low", "SimpleQuantity", 718 "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 719 0, java.lang.Integer.MAX_VALUE, low)); 720 childrenList.add(new Property("high", "SimpleQuantity", 721 "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 722 0, java.lang.Integer.MAX_VALUE, high)); 723 childrenList.add(new Property("meaning", "CodeableConcept", "Code for the meaning of the reference range.", 0, 724 java.lang.Integer.MAX_VALUE, meaning)); 725 childrenList.add(new Property("age", "Range", 726 "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 727 0, java.lang.Integer.MAX_VALUE, age)); 728 childrenList.add(new Property("text", "string", 729 "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of 'normals'.", 730 0, java.lang.Integer.MAX_VALUE, text)); 731 } 732 733 @Override 734 public void setProperty(String name, Base value) throws FHIRException { 735 if (name.equals("low")) 736 this.low = castToSimpleQuantity(value); // SimpleQuantity 737 else if (name.equals("high")) 738 this.high = castToSimpleQuantity(value); // SimpleQuantity 739 else if (name.equals("meaning")) 740 this.meaning = castToCodeableConcept(value); // CodeableConcept 741 else if (name.equals("age")) 742 this.age = castToRange(value); // Range 743 else if (name.equals("text")) 744 this.text = castToString(value); // StringType 745 else 746 super.setProperty(name, value); 747 } 748 749 @Override 750 public Base addChild(String name) throws FHIRException { 751 if (name.equals("low")) { 752 this.low = new SimpleQuantity(); 753 return this.low; 754 } else if (name.equals("high")) { 755 this.high = new SimpleQuantity(); 756 return this.high; 757 } else if (name.equals("meaning")) { 758 this.meaning = new CodeableConcept(); 759 return this.meaning; 760 } else if (name.equals("age")) { 761 this.age = new Range(); 762 return this.age; 763 } else if (name.equals("text")) { 764 throw new FHIRException("Cannot call addChild on a singleton property Observation.text"); 765 } else 766 return super.addChild(name); 767 } 768 769 public ObservationReferenceRangeComponent copy() { 770 ObservationReferenceRangeComponent dst = new ObservationReferenceRangeComponent(); 771 copyValues(dst); 772 dst.low = low == null ? null : low.copy(); 773 dst.high = high == null ? null : high.copy(); 774 dst.meaning = meaning == null ? null : meaning.copy(); 775 dst.age = age == null ? null : age.copy(); 776 dst.text = text == null ? null : text.copy(); 777 return dst; 778 } 779 780 @Override 781 public boolean equalsDeep(Base other) { 782 if (!super.equalsDeep(other)) 783 return false; 784 if (!(other instanceof ObservationReferenceRangeComponent)) 785 return false; 786 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other; 787 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true) && compareDeep(meaning, o.meaning, true) 788 && compareDeep(age, o.age, true) && compareDeep(text, o.text, true); 789 } 790 791 @Override 792 public boolean equalsShallow(Base other) { 793 if (!super.equalsShallow(other)) 794 return false; 795 if (!(other instanceof ObservationReferenceRangeComponent)) 796 return false; 797 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other; 798 return compareValues(text, o.text, true); 799 } 800 801 public boolean isEmpty() { 802 return super.isEmpty() && (low == null || low.isEmpty()) && (high == null || high.isEmpty()) 803 && (meaning == null || meaning.isEmpty()) && (age == null || age.isEmpty()) 804 && (text == null || text.isEmpty()); 805 } 806 807 public String fhirType() { 808 return "Observation.referenceRange"; 809 810 } 811 812 } 813 814 @Block() 815 public static class ObservationRelatedComponent extends BackboneElement implements IBaseBackboneElement { 816 /** 817 * A code specifying the kind of relationship that exists with the target 818 * resource. 819 */ 820 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 821 @Description(shortDefinition = "has-member | derived-from | sequel-to | replaces | qualified-by | interfered-by", formalDefinition = "A code specifying the kind of relationship that exists with the target resource.") 822 protected Enumeration<ObservationRelationshipType> type; 823 824 /** 825 * A reference to the observation or [[[QuestionnaireResponse]]] resource that 826 * is related to this observation. 827 */ 828 @Child(name = "target", type = { Observation.class, 829 QuestionnaireResponse.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 830 @Description(shortDefinition = "Resource that is related to this one", formalDefinition = "A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.") 831 protected Reference target; 832 833 /** 834 * The actual object that is the target of the reference (A reference to the 835 * observation or [[[QuestionnaireResponse]]] resource that is related to this 836 * observation.) 837 */ 838 protected Resource targetTarget; 839 840 private static final long serialVersionUID = 1541802577L; 841 842 /* 843 * Constructor 844 */ 845 public ObservationRelatedComponent() { 846 super(); 847 } 848 849 /* 850 * Constructor 851 */ 852 public ObservationRelatedComponent(Reference target) { 853 super(); 854 this.target = target; 855 } 856 857 /** 858 * @return {@link #type} (A code specifying the kind of relationship that exists 859 * with the target resource.). This is the underlying object with id, 860 * value and extensions. The accessor "getType" gives direct access to 861 * the value 862 */ 863 public Enumeration<ObservationRelationshipType> getTypeElement() { 864 if (this.type == null) 865 if (Configuration.errorOnAutoCreate()) 866 throw new Error("Attempt to auto-create ObservationRelatedComponent.type"); 867 else if (Configuration.doAutoCreate()) 868 this.type = new Enumeration<ObservationRelationshipType>(new ObservationRelationshipTypeEnumFactory()); // bb 869 return this.type; 870 } 871 872 public boolean hasTypeElement() { 873 return this.type != null && !this.type.isEmpty(); 874 } 875 876 public boolean hasType() { 877 return this.type != null && !this.type.isEmpty(); 878 } 879 880 /** 881 * @param value {@link #type} (A code specifying the kind of relationship that 882 * exists with the target resource.). This is the underlying object 883 * with id, value and extensions. The accessor "getType" gives 884 * direct access to the value 885 */ 886 public ObservationRelatedComponent setTypeElement(Enumeration<ObservationRelationshipType> value) { 887 this.type = value; 888 return this; 889 } 890 891 /** 892 * @return A code specifying the kind of relationship that exists with the 893 * target resource. 894 */ 895 public ObservationRelationshipType getType() { 896 return this.type == null ? null : this.type.getValue(); 897 } 898 899 /** 900 * @param value A code specifying the kind of relationship that exists with the 901 * target resource. 902 */ 903 public ObservationRelatedComponent setType(ObservationRelationshipType value) { 904 if (value == null) 905 this.type = null; 906 else { 907 if (this.type == null) 908 this.type = new Enumeration<ObservationRelationshipType>(new ObservationRelationshipTypeEnumFactory()); 909 this.type.setValue(value); 910 } 911 return this; 912 } 913 914 /** 915 * @return {@link #target} (A reference to the observation or 916 * [[[QuestionnaireResponse]]] resource that is related to this 917 * observation.) 918 */ 919 public Reference getTarget() { 920 if (this.target == null) 921 if (Configuration.errorOnAutoCreate()) 922 throw new Error("Attempt to auto-create ObservationRelatedComponent.target"); 923 else if (Configuration.doAutoCreate()) 924 this.target = new Reference(); // cc 925 return this.target; 926 } 927 928 public boolean hasTarget() { 929 return this.target != null && !this.target.isEmpty(); 930 } 931 932 /** 933 * @param value {@link #target} (A reference to the observation or 934 * [[[QuestionnaireResponse]]] resource that is related to this 935 * observation.) 936 */ 937 public ObservationRelatedComponent setTarget(Reference value) { 938 this.target = value; 939 return this; 940 } 941 942 /** 943 * @return {@link #target} The actual object that is the target of the 944 * reference. The reference library doesn't populate this, but you can 945 * use it to hold the resource if you resolve it. (A reference to the 946 * observation or [[[QuestionnaireResponse]]] resource that is related 947 * to this observation.) 948 */ 949 public Resource getTargetTarget() { 950 return this.targetTarget; 951 } 952 953 /** 954 * @param value {@link #target} The actual object that is the target of the 955 * reference. The reference library doesn't use these, but you can 956 * use it to hold the resource if you resolve it. (A reference to 957 * the observation or [[[QuestionnaireResponse]]] resource that is 958 * related to this observation.) 959 */ 960 public ObservationRelatedComponent setTargetTarget(Resource value) { 961 this.targetTarget = value; 962 return this; 963 } 964 965 protected void listChildren(List<Property> childrenList) { 966 super.listChildren(childrenList); 967 childrenList.add(new Property("type", "code", 968 "A code specifying the kind of relationship that exists with the target resource.", 0, 969 java.lang.Integer.MAX_VALUE, type)); 970 childrenList.add(new Property("target", "Reference(Observation|QuestionnaireResponse)", 971 "A reference to the observation or [[[QuestionnaireResponse]]] resource that is related to this observation.", 972 0, java.lang.Integer.MAX_VALUE, target)); 973 } 974 975 @Override 976 public void setProperty(String name, Base value) throws FHIRException { 977 if (name.equals("type")) 978 this.type = new ObservationRelationshipTypeEnumFactory().fromType(value); // Enumeration<ObservationRelationshipType> 979 else if (name.equals("target")) 980 this.target = castToReference(value); // Reference 981 else 982 super.setProperty(name, value); 983 } 984 985 @Override 986 public Base addChild(String name) throws FHIRException { 987 if (name.equals("type")) { 988 throw new FHIRException("Cannot call addChild on a singleton property Observation.type"); 989 } else if (name.equals("target")) { 990 this.target = new Reference(); 991 return this.target; 992 } else 993 return super.addChild(name); 994 } 995 996 public ObservationRelatedComponent copy() { 997 ObservationRelatedComponent dst = new ObservationRelatedComponent(); 998 copyValues(dst); 999 dst.type = type == null ? null : type.copy(); 1000 dst.target = target == null ? null : target.copy(); 1001 return dst; 1002 } 1003 1004 @Override 1005 public boolean equalsDeep(Base other) { 1006 if (!super.equalsDeep(other)) 1007 return false; 1008 if (!(other instanceof ObservationRelatedComponent)) 1009 return false; 1010 ObservationRelatedComponent o = (ObservationRelatedComponent) other; 1011 return compareDeep(type, o.type, true) && compareDeep(target, o.target, true); 1012 } 1013 1014 @Override 1015 public boolean equalsShallow(Base other) { 1016 if (!super.equalsShallow(other)) 1017 return false; 1018 if (!(other instanceof ObservationRelatedComponent)) 1019 return false; 1020 ObservationRelatedComponent o = (ObservationRelatedComponent) other; 1021 return compareValues(type, o.type, true); 1022 } 1023 1024 public boolean isEmpty() { 1025 return super.isEmpty() && (type == null || type.isEmpty()) && (target == null || target.isEmpty()); 1026 } 1027 1028 public String fhirType() { 1029 return "Observation.related"; 1030 1031 } 1032 1033 } 1034 1035 @Block() 1036 public static class ObservationComponentComponent extends BackboneElement implements IBaseBackboneElement { 1037 /** 1038 * Describes what was observed. Sometimes this is called the observation "code". 1039 */ 1040 @Child(name = "code", type = { 1041 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1042 @Description(shortDefinition = "Type of component observation (code / type)", formalDefinition = "Describes what was observed. Sometimes this is called the observation \"code\".") 1043 protected CodeableConcept code; 1044 1045 /** 1046 * The information determined as a result of making the observation, if the 1047 * information has a simple value. 1048 */ 1049 @Child(name = "value", type = { Quantity.class, CodeableConcept.class, StringType.class, Range.class, Ratio.class, 1050 SampledData.class, Attachment.class, TimeType.class, DateTimeType.class, 1051 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1052 @Description(shortDefinition = "Actual component result", formalDefinition = "The information determined as a result of making the observation, if the information has a simple value.") 1053 protected Type value; 1054 1055 /** 1056 * Provides a reason why the expected value in the element Observation.value[x] 1057 * is missing. 1058 */ 1059 @Child(name = "dataAbsentReason", type = { 1060 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1061 @Description(shortDefinition = "Why the component result is missing", formalDefinition = "Provides a reason why the expected value in the element Observation.value[x] is missing.") 1062 protected CodeableConcept dataAbsentReason; 1063 1064 /** 1065 * Guidance on how to interpret the value by comparison to a normal or 1066 * recommended range. 1067 */ 1068 @Child(name = "referenceRange", type = { 1069 ObservationReferenceRangeComponent.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1070 @Description(shortDefinition = "Provides guide for interpretation of component result", formalDefinition = "Guidance on how to interpret the value by comparison to a normal or recommended range.") 1071 protected List<ObservationReferenceRangeComponent> referenceRange; 1072 1073 private static final long serialVersionUID = 946602904L; 1074 1075 /* 1076 * Constructor 1077 */ 1078 public ObservationComponentComponent() { 1079 super(); 1080 } 1081 1082 /* 1083 * Constructor 1084 */ 1085 public ObservationComponentComponent(CodeableConcept code) { 1086 super(); 1087 this.code = code; 1088 } 1089 1090 /** 1091 * @return {@link #code} (Describes what was observed. Sometimes this is called 1092 * the observation "code".) 1093 */ 1094 public CodeableConcept getCode() { 1095 if (this.code == null) 1096 if (Configuration.errorOnAutoCreate()) 1097 throw new Error("Attempt to auto-create ObservationComponentComponent.code"); 1098 else if (Configuration.doAutoCreate()) 1099 this.code = new CodeableConcept(); // cc 1100 return this.code; 1101 } 1102 1103 public boolean hasCode() { 1104 return this.code != null && !this.code.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #code} (Describes what was observed. Sometimes this is 1109 * called the observation "code".) 1110 */ 1111 public ObservationComponentComponent setCode(CodeableConcept value) { 1112 this.code = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #value} (The information determined as a result of making the 1118 * observation, if the information has a simple value.) 1119 */ 1120 public Type getValue() { 1121 return this.value; 1122 } 1123 1124 /** 1125 * @return {@link #value} (The information determined as a result of making the 1126 * observation, if the information has a simple value.) 1127 */ 1128 public Quantity getValueQuantity() throws FHIRException { 1129 if (!(this.value instanceof Quantity)) 1130 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 1131 + " was encountered"); 1132 return (Quantity) this.value; 1133 } 1134 1135 public boolean hasValueQuantity() { 1136 return this.value instanceof Quantity; 1137 } 1138 1139 /** 1140 * @return {@link #value} (The information determined as a result of making the 1141 * observation, if the information has a simple value.) 1142 */ 1143 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1144 if (!(this.value instanceof CodeableConcept)) 1145 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1146 + this.value.getClass().getName() + " was encountered"); 1147 return (CodeableConcept) this.value; 1148 } 1149 1150 public boolean hasValueCodeableConcept() { 1151 return this.value instanceof CodeableConcept; 1152 } 1153 1154 /** 1155 * @return {@link #value} (The information determined as a result of making the 1156 * observation, if the information has a simple value.) 1157 */ 1158 public StringType getValueStringType() throws FHIRException { 1159 if (!(this.value instanceof StringType)) 1160 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1161 + this.value.getClass().getName() + " was encountered"); 1162 return (StringType) this.value; 1163 } 1164 1165 public boolean hasValueStringType() { 1166 return this.value instanceof StringType; 1167 } 1168 1169 /** 1170 * @return {@link #value} (The information determined as a result of making the 1171 * observation, if the information has a simple value.) 1172 */ 1173 public Range getValueRange() throws FHIRException { 1174 if (!(this.value instanceof Range)) 1175 throw new FHIRException( 1176 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 1177 return (Range) this.value; 1178 } 1179 1180 public boolean hasValueRange() { 1181 return this.value instanceof Range; 1182 } 1183 1184 /** 1185 * @return {@link #value} (The information determined as a result of making the 1186 * observation, if the information has a simple value.) 1187 */ 1188 public Ratio getValueRatio() throws FHIRException { 1189 if (!(this.value instanceof Ratio)) 1190 throw new FHIRException( 1191 "Type mismatch: the type Ratio was expected, but " + this.value.getClass().getName() + " was encountered"); 1192 return (Ratio) this.value; 1193 } 1194 1195 public boolean hasValueRatio() { 1196 return this.value instanceof Ratio; 1197 } 1198 1199 /** 1200 * @return {@link #value} (The information determined as a result of making the 1201 * observation, if the information has a simple value.) 1202 */ 1203 public SampledData getValueSampledData() throws FHIRException { 1204 if (!(this.value instanceof SampledData)) 1205 throw new FHIRException("Type mismatch: the type SampledData was expected, but " 1206 + this.value.getClass().getName() + " was encountered"); 1207 return (SampledData) this.value; 1208 } 1209 1210 public boolean hasValueSampledData() { 1211 return this.value instanceof SampledData; 1212 } 1213 1214 /** 1215 * @return {@link #value} (The information determined as a result of making the 1216 * observation, if the information has a simple value.) 1217 */ 1218 public Attachment getValueAttachment() throws FHIRException { 1219 if (!(this.value instanceof Attachment)) 1220 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 1221 + this.value.getClass().getName() + " was encountered"); 1222 return (Attachment) this.value; 1223 } 1224 1225 public boolean hasValueAttachment() { 1226 return this.value instanceof Attachment; 1227 } 1228 1229 /** 1230 * @return {@link #value} (The information determined as a result of making the 1231 * observation, if the information has a simple value.) 1232 */ 1233 public TimeType getValueTimeType() throws FHIRException { 1234 if (!(this.value instanceof TimeType)) 1235 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 1236 + " was encountered"); 1237 return (TimeType) this.value; 1238 } 1239 1240 public boolean hasValueTimeType() { 1241 return this.value instanceof TimeType; 1242 } 1243 1244 /** 1245 * @return {@link #value} (The information determined as a result of making the 1246 * observation, if the information has a simple value.) 1247 */ 1248 public DateTimeType getValueDateTimeType() throws FHIRException { 1249 if (!(this.value instanceof DateTimeType)) 1250 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1251 + this.value.getClass().getName() + " was encountered"); 1252 return (DateTimeType) this.value; 1253 } 1254 1255 public boolean hasValueDateTimeType() { 1256 return this.value instanceof DateTimeType; 1257 } 1258 1259 /** 1260 * @return {@link #value} (The information determined as a result of making the 1261 * observation, if the information has a simple value.) 1262 */ 1263 public Period getValuePeriod() throws FHIRException { 1264 if (!(this.value instanceof Period)) 1265 throw new FHIRException( 1266 "Type mismatch: the type Period was expected, but " + this.value.getClass().getName() + " was encountered"); 1267 return (Period) this.value; 1268 } 1269 1270 public boolean hasValuePeriod() { 1271 return this.value instanceof Period; 1272 } 1273 1274 public boolean hasValue() { 1275 return this.value != null && !this.value.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #value} (The information determined as a result of making 1280 * the observation, if the information has a simple value.) 1281 */ 1282 public ObservationComponentComponent setValue(Type value) { 1283 this.value = value; 1284 return this; 1285 } 1286 1287 /** 1288 * @return {@link #dataAbsentReason} (Provides a reason why the expected value 1289 * in the element Observation.value[x] is missing.) 1290 */ 1291 public CodeableConcept getDataAbsentReason() { 1292 if (this.dataAbsentReason == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create ObservationComponentComponent.dataAbsentReason"); 1295 else if (Configuration.doAutoCreate()) 1296 this.dataAbsentReason = new CodeableConcept(); // cc 1297 return this.dataAbsentReason; 1298 } 1299 1300 public boolean hasDataAbsentReason() { 1301 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #dataAbsentReason} (Provides a reason why the expected 1306 * value in the element Observation.value[x] is missing.) 1307 */ 1308 public ObservationComponentComponent setDataAbsentReason(CodeableConcept value) { 1309 this.dataAbsentReason = value; 1310 return this; 1311 } 1312 1313 /** 1314 * @return {@link #referenceRange} (Guidance on how to interpret the value by 1315 * comparison to a normal or recommended range.) 1316 */ 1317 public List<ObservationReferenceRangeComponent> getReferenceRange() { 1318 if (this.referenceRange == null) 1319 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1320 return this.referenceRange; 1321 } 1322 1323 public boolean hasReferenceRange() { 1324 if (this.referenceRange == null) 1325 return false; 1326 for (ObservationReferenceRangeComponent item : this.referenceRange) 1327 if (!item.isEmpty()) 1328 return true; 1329 return false; 1330 } 1331 1332 /** 1333 * @return {@link #referenceRange} (Guidance on how to interpret the value by 1334 * comparison to a normal or recommended range.) 1335 */ 1336 // syntactic sugar 1337 public ObservationReferenceRangeComponent addReferenceRange() { // 3 1338 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 1339 if (this.referenceRange == null) 1340 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1341 this.referenceRange.add(t); 1342 return t; 1343 } 1344 1345 // syntactic sugar 1346 public ObservationComponentComponent addReferenceRange(ObservationReferenceRangeComponent t) { // 3 1347 if (t == null) 1348 return this; 1349 if (this.referenceRange == null) 1350 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1351 this.referenceRange.add(t); 1352 return this; 1353 } 1354 1355 protected void listChildren(List<Property> childrenList) { 1356 super.listChildren(childrenList); 1357 childrenList.add(new Property("code", "CodeableConcept", 1358 "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1359 java.lang.Integer.MAX_VALUE, code)); 1360 childrenList.add(new Property("value[x]", 1361 "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", 1362 "The information determined as a result of making the observation, if the information has a simple value.", 0, 1363 java.lang.Integer.MAX_VALUE, value)); 1364 childrenList.add(new Property("dataAbsentReason", "CodeableConcept", 1365 "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1366 java.lang.Integer.MAX_VALUE, dataAbsentReason)); 1367 childrenList.add(new Property("referenceRange", "@Observation.referenceRange", 1368 "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, 1369 java.lang.Integer.MAX_VALUE, referenceRange)); 1370 } 1371 1372 @Override 1373 public void setProperty(String name, Base value) throws FHIRException { 1374 if (name.equals("code")) 1375 this.code = castToCodeableConcept(value); // CodeableConcept 1376 else if (name.equals("value[x]")) 1377 this.value = (Type) value; // Type 1378 else if (name.equals("dataAbsentReason")) 1379 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 1380 else if (name.equals("referenceRange")) 1381 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 1382 else 1383 super.setProperty(name, value); 1384 } 1385 1386 @Override 1387 public Base addChild(String name) throws FHIRException { 1388 if (name.equals("code")) { 1389 this.code = new CodeableConcept(); 1390 return this.code; 1391 } else if (name.equals("valueQuantity")) { 1392 this.value = new Quantity(); 1393 return this.value; 1394 } else if (name.equals("valueCodeableConcept")) { 1395 this.value = new CodeableConcept(); 1396 return this.value; 1397 } else if (name.equals("valueString")) { 1398 this.value = new StringType(); 1399 return this.value; 1400 } else if (name.equals("valueRange")) { 1401 this.value = new Range(); 1402 return this.value; 1403 } else if (name.equals("valueRatio")) { 1404 this.value = new Ratio(); 1405 return this.value; 1406 } else if (name.equals("valueSampledData")) { 1407 this.value = new SampledData(); 1408 return this.value; 1409 } else if (name.equals("valueAttachment")) { 1410 this.value = new Attachment(); 1411 return this.value; 1412 } else if (name.equals("valueTime")) { 1413 this.value = new TimeType(); 1414 return this.value; 1415 } else if (name.equals("valueDateTime")) { 1416 this.value = new DateTimeType(); 1417 return this.value; 1418 } else if (name.equals("valuePeriod")) { 1419 this.value = new Period(); 1420 return this.value; 1421 } else if (name.equals("dataAbsentReason")) { 1422 this.dataAbsentReason = new CodeableConcept(); 1423 return this.dataAbsentReason; 1424 } else if (name.equals("referenceRange")) { 1425 return addReferenceRange(); 1426 } else 1427 return super.addChild(name); 1428 } 1429 1430 public ObservationComponentComponent copy() { 1431 ObservationComponentComponent dst = new ObservationComponentComponent(); 1432 copyValues(dst); 1433 dst.code = code == null ? null : code.copy(); 1434 dst.value = value == null ? null : value.copy(); 1435 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 1436 if (referenceRange != null) { 1437 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1438 for (ObservationReferenceRangeComponent i : referenceRange) 1439 dst.referenceRange.add(i.copy()); 1440 } 1441 ; 1442 return dst; 1443 } 1444 1445 @Override 1446 public boolean equalsDeep(Base other) { 1447 if (!super.equalsDeep(other)) 1448 return false; 1449 if (!(other instanceof ObservationComponentComponent)) 1450 return false; 1451 ObservationComponentComponent o = (ObservationComponentComponent) other; 1452 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 1453 && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 1454 && compareDeep(referenceRange, o.referenceRange, true); 1455 } 1456 1457 @Override 1458 public boolean equalsShallow(Base other) { 1459 if (!super.equalsShallow(other)) 1460 return false; 1461 if (!(other instanceof ObservationComponentComponent)) 1462 return false; 1463 ObservationComponentComponent o = (ObservationComponentComponent) other; 1464 return true; 1465 } 1466 1467 public boolean isEmpty() { 1468 return super.isEmpty() && (code == null || code.isEmpty()) && (value == null || value.isEmpty()) 1469 && (dataAbsentReason == null || dataAbsentReason.isEmpty()) 1470 && (referenceRange == null || referenceRange.isEmpty()); 1471 } 1472 1473 public String fhirType() { 1474 return "Observation.component"; 1475 1476 } 1477 1478 } 1479 1480 /** 1481 * A unique identifier for the simple observation instance. 1482 */ 1483 @Child(name = "identifier", type = { 1484 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1485 @Description(shortDefinition = "Unique Id for this particular observation", formalDefinition = "A unique identifier for the simple observation instance.") 1486 protected List<Identifier> identifier; 1487 1488 /** 1489 * The status of the result value. 1490 */ 1491 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1492 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "The status of the result value.") 1493 protected Enumeration<ObservationStatus> status; 1494 1495 /** 1496 * A code that classifies the general type of observation being made. This is 1497 * used for searching, sorting and display purposes. 1498 */ 1499 @Child(name = "category", type = { 1500 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1501 @Description(shortDefinition = "Classification of type of observation", formalDefinition = "A code that classifies the general type of observation being made. This is used for searching, sorting and display purposes.") 1502 protected CodeableConcept category; 1503 1504 /** 1505 * Describes what was observed. Sometimes this is called the observation "name". 1506 */ 1507 @Child(name = "code", type = { CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1508 @Description(shortDefinition = "Type of observation (code / type)", formalDefinition = "Describes what was observed. Sometimes this is called the observation \"name\".") 1509 protected CodeableConcept code; 1510 1511 /** 1512 * The patient, or group of patients, location, or device whose characteristics 1513 * (direct or indirect) are described by the observation and into whose record 1514 * the observation is placed. Comments: Indirect characteristics may be those of 1515 * a specimen, fetus, donor, other observer (for example a relative or EMT), or 1516 * any observation made about the subject. 1517 */ 1518 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 1519 Location.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1520 @Description(shortDefinition = "Who and/or what this is about", formalDefinition = "The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.") 1521 protected Reference subject; 1522 1523 /** 1524 * The actual object that is the target of the reference (The patient, or group 1525 * of patients, location, or device whose characteristics (direct or indirect) 1526 * are described by the observation and into whose record the observation is 1527 * placed. Comments: Indirect characteristics may be those of a specimen, fetus, 1528 * donor, other observer (for example a relative or EMT), or any observation 1529 * made about the subject.) 1530 */ 1531 protected Resource subjectTarget; 1532 1533 /** 1534 * The healthcare event (e.g. a patient and healthcare provider interaction) 1535 * during which this observation is made. 1536 */ 1537 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1538 @Description(shortDefinition = "Healthcare event during which this observation is made", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.") 1539 protected Reference encounter; 1540 1541 /** 1542 * The actual object that is the target of the reference (The healthcare event 1543 * (e.g. a patient and healthcare provider interaction) during which this 1544 * observation is made.) 1545 */ 1546 protected Encounter encounterTarget; 1547 1548 /** 1549 * The time or time-period the observed value is asserted as being true. For 1550 * biological subjects - e.g. human patients - this is usually called the 1551 * "physiologically relevant time". This is usually either the time of the 1552 * procedure or of specimen collection, but very often the source of the 1553 * date/time is not known, only the date/time itself. 1554 */ 1555 @Child(name = "effective", type = { DateTimeType.class, 1556 Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1557 @Description(shortDefinition = "Clinically relevant time/time-period for observation", formalDefinition = "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.") 1558 protected Type effective; 1559 1560 /** 1561 * The date and time this observation was made available to providers, typically 1562 * after the results have been reviewed and verified. 1563 */ 1564 @Child(name = "issued", type = { InstantType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1565 @Description(shortDefinition = "Date/Time this was made available", formalDefinition = "The date and time this observation was made available to providers, typically after the results have been reviewed and verified.") 1566 protected InstantType issued; 1567 1568 /** 1569 * Who was responsible for asserting the observed value as "true". 1570 */ 1571 @Child(name = "performer", type = { Practitioner.class, Organization.class, Patient.class, 1572 RelatedPerson.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1573 @Description(shortDefinition = "Who is responsible for the observation", formalDefinition = "Who was responsible for asserting the observed value as \"true\".") 1574 protected List<Reference> performer; 1575 /** 1576 * The actual objects that are the target of the reference (Who was responsible 1577 * for asserting the observed value as "true".) 1578 */ 1579 protected List<Resource> performerTarget; 1580 1581 /** 1582 * The information determined as a result of making the observation, if the 1583 * information has a simple value. 1584 */ 1585 @Child(name = "value", type = { Quantity.class, CodeableConcept.class, StringType.class, Range.class, Ratio.class, 1586 SampledData.class, Attachment.class, TimeType.class, DateTimeType.class, 1587 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1588 @Description(shortDefinition = "Actual result", formalDefinition = "The information determined as a result of making the observation, if the information has a simple value.") 1589 protected Type value; 1590 1591 /** 1592 * Provides a reason why the expected value in the element Observation.value[x] 1593 * is missing. 1594 */ 1595 @Child(name = "dataAbsentReason", type = { 1596 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1597 @Description(shortDefinition = "Why the result is missing", formalDefinition = "Provides a reason why the expected value in the element Observation.value[x] is missing.") 1598 protected CodeableConcept dataAbsentReason; 1599 1600 /** 1601 * The assessment made based on the result of the observation. Intended as a 1602 * simple compact code often placed adjacent to the result value in reports and 1603 * flow sheets to signal the meaning/normalcy status of the result. Otherwise 1604 * known as abnormal flag. 1605 */ 1606 @Child(name = "interpretation", type = { 1607 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1608 @Description(shortDefinition = "High, low, normal, etc.", formalDefinition = "The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.") 1609 protected CodeableConcept interpretation; 1610 1611 /** 1612 * May include statements about significant, unexpected or unreliable values, or 1613 * information about the source of the value where this may be relevant to the 1614 * interpretation of the result. 1615 */ 1616 @Child(name = "comments", type = { 1617 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1618 @Description(shortDefinition = "Comments about result", formalDefinition = "May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result.") 1619 protected StringType comments; 1620 1621 /** 1622 * Indicates the site on the subject's body where the observation was made (i.e. 1623 * the target site). 1624 */ 1625 @Child(name = "bodySite", type = { 1626 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1627 @Description(shortDefinition = "Observed body part", formalDefinition = "Indicates the site on the subject's body where the observation was made (i.e. the target site).") 1628 protected CodeableConcept bodySite; 1629 1630 /** 1631 * Indicates the mechanism used to perform the observation. 1632 */ 1633 @Child(name = "method", type = { 1634 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1635 @Description(shortDefinition = "How it was done", formalDefinition = "Indicates the mechanism used to perform the observation.") 1636 protected CodeableConcept method; 1637 1638 /** 1639 * The specimen that was used when this observation was made. 1640 */ 1641 @Child(name = "specimen", type = { Specimen.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1642 @Description(shortDefinition = "Specimen used for this observation", formalDefinition = "The specimen that was used when this observation was made.") 1643 protected Reference specimen; 1644 1645 /** 1646 * The actual object that is the target of the reference (The specimen that was 1647 * used when this observation was made.) 1648 */ 1649 protected Specimen specimenTarget; 1650 1651 /** 1652 * The device used to generate the observation data. 1653 */ 1654 @Child(name = "device", type = { Device.class, 1655 DeviceMetric.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1656 @Description(shortDefinition = "(Measurement) Device", formalDefinition = "The device used to generate the observation data.") 1657 protected Reference device; 1658 1659 /** 1660 * The actual object that is the target of the reference (The device used to 1661 * generate the observation data.) 1662 */ 1663 protected Resource deviceTarget; 1664 1665 /** 1666 * Guidance on how to interpret the value by comparison to a normal or 1667 * recommended range. 1668 */ 1669 @Child(name = "referenceRange", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1670 @Description(shortDefinition = "Provides guide for interpretation", formalDefinition = "Guidance on how to interpret the value by comparison to a normal or recommended range.") 1671 protected List<ObservationReferenceRangeComponent> referenceRange; 1672 1673 /** 1674 * A reference to another resource (usually another Observation but could also 1675 * be a QuestionnaireAnswer) whose relationship is defined by the relationship 1676 * type code. 1677 */ 1678 @Child(name = "related", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1679 @Description(shortDefinition = "Resource related to this observation", formalDefinition = "A reference to another resource (usually another Observation but could also be a QuestionnaireAnswer) whose relationship is defined by the relationship type code.") 1680 protected List<ObservationRelatedComponent> related; 1681 1682 /** 1683 * Some observations have multiple component observations. These component 1684 * observations are expressed as separate code value pairs that share the same 1685 * attributes. Examples include systolic and diastolic component observations 1686 * for blood pressure measurement and multiple component observations for 1687 * genetics observations. 1688 */ 1689 @Child(name = "component", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1690 @Description(shortDefinition = "Component results", formalDefinition = "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.") 1691 protected List<ObservationComponentComponent> component; 1692 1693 private static final long serialVersionUID = -931593572L; 1694 1695 /* 1696 * Constructor 1697 */ 1698 public Observation() { 1699 super(); 1700 } 1701 1702 /* 1703 * Constructor 1704 */ 1705 public Observation(Enumeration<ObservationStatus> status, CodeableConcept code) { 1706 super(); 1707 this.status = status; 1708 this.code = code; 1709 } 1710 1711 /** 1712 * @return {@link #identifier} (A unique identifier for the simple observation 1713 * instance.) 1714 */ 1715 public List<Identifier> getIdentifier() { 1716 if (this.identifier == null) 1717 this.identifier = new ArrayList<Identifier>(); 1718 return this.identifier; 1719 } 1720 1721 public boolean hasIdentifier() { 1722 if (this.identifier == null) 1723 return false; 1724 for (Identifier item : this.identifier) 1725 if (!item.isEmpty()) 1726 return true; 1727 return false; 1728 } 1729 1730 /** 1731 * @return {@link #identifier} (A unique identifier for the simple observation 1732 * instance.) 1733 */ 1734 // syntactic sugar 1735 public Identifier addIdentifier() { // 3 1736 Identifier t = new Identifier(); 1737 if (this.identifier == null) 1738 this.identifier = new ArrayList<Identifier>(); 1739 this.identifier.add(t); 1740 return t; 1741 } 1742 1743 // syntactic sugar 1744 public Observation addIdentifier(Identifier t) { // 3 1745 if (t == null) 1746 return this; 1747 if (this.identifier == null) 1748 this.identifier = new ArrayList<Identifier>(); 1749 this.identifier.add(t); 1750 return this; 1751 } 1752 1753 /** 1754 * @return {@link #status} (The status of the result value.). This is the 1755 * underlying object with id, value and extensions. The accessor 1756 * "getStatus" gives direct access to the value 1757 */ 1758 public Enumeration<ObservationStatus> getStatusElement() { 1759 if (this.status == null) 1760 if (Configuration.errorOnAutoCreate()) 1761 throw new Error("Attempt to auto-create Observation.status"); 1762 else if (Configuration.doAutoCreate()) 1763 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); // bb 1764 return this.status; 1765 } 1766 1767 public boolean hasStatusElement() { 1768 return this.status != null && !this.status.isEmpty(); 1769 } 1770 1771 public boolean hasStatus() { 1772 return this.status != null && !this.status.isEmpty(); 1773 } 1774 1775 /** 1776 * @param value {@link #status} (The status of the result value.). This is the 1777 * underlying object with id, value and extensions. The accessor 1778 * "getStatus" gives direct access to the value 1779 */ 1780 public Observation setStatusElement(Enumeration<ObservationStatus> value) { 1781 this.status = value; 1782 return this; 1783 } 1784 1785 /** 1786 * @return The status of the result value. 1787 */ 1788 public ObservationStatus getStatus() { 1789 return this.status == null ? null : this.status.getValue(); 1790 } 1791 1792 /** 1793 * @param value The status of the result value. 1794 */ 1795 public Observation setStatus(ObservationStatus value) { 1796 if (this.status == null) 1797 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); 1798 this.status.setValue(value); 1799 return this; 1800 } 1801 1802 /** 1803 * @return {@link #category} (A code that classifies the general type of 1804 * observation being made. This is used for searching, sorting and 1805 * display purposes.) 1806 */ 1807 public CodeableConcept getCategory() { 1808 if (this.category == null) 1809 if (Configuration.errorOnAutoCreate()) 1810 throw new Error("Attempt to auto-create Observation.category"); 1811 else if (Configuration.doAutoCreate()) 1812 this.category = new CodeableConcept(); // cc 1813 return this.category; 1814 } 1815 1816 public boolean hasCategory() { 1817 return this.category != null && !this.category.isEmpty(); 1818 } 1819 1820 /** 1821 * @param value {@link #category} (A code that classifies the general type of 1822 * observation being made. This is used for searching, sorting and 1823 * display purposes.) 1824 */ 1825 public Observation setCategory(CodeableConcept value) { 1826 this.category = value; 1827 return this; 1828 } 1829 1830 /** 1831 * @return {@link #code} (Describes what was observed. Sometimes this is called 1832 * the observation "name".) 1833 */ 1834 public CodeableConcept getCode() { 1835 if (this.code == null) 1836 if (Configuration.errorOnAutoCreate()) 1837 throw new Error("Attempt to auto-create Observation.code"); 1838 else if (Configuration.doAutoCreate()) 1839 this.code = new CodeableConcept(); // cc 1840 return this.code; 1841 } 1842 1843 public boolean hasCode() { 1844 return this.code != null && !this.code.isEmpty(); 1845 } 1846 1847 /** 1848 * @param value {@link #code} (Describes what was observed. Sometimes this is 1849 * called the observation "name".) 1850 */ 1851 public Observation setCode(CodeableConcept value) { 1852 this.code = value; 1853 return this; 1854 } 1855 1856 /** 1857 * @return {@link #subject} (The patient, or group of patients, location, or 1858 * device whose characteristics (direct or indirect) are described by 1859 * the observation and into whose record the observation is placed. 1860 * Comments: Indirect characteristics may be those of a specimen, fetus, 1861 * donor, other observer (for example a relative or EMT), or any 1862 * observation made about the subject.) 1863 */ 1864 public Reference getSubject() { 1865 if (this.subject == null) 1866 if (Configuration.errorOnAutoCreate()) 1867 throw new Error("Attempt to auto-create Observation.subject"); 1868 else if (Configuration.doAutoCreate()) 1869 this.subject = new Reference(); // cc 1870 return this.subject; 1871 } 1872 1873 public boolean hasSubject() { 1874 return this.subject != null && !this.subject.isEmpty(); 1875 } 1876 1877 /** 1878 * @param value {@link #subject} (The patient, or group of patients, location, 1879 * or device whose characteristics (direct or indirect) are 1880 * described by the observation and into whose record the 1881 * observation is placed. Comments: Indirect characteristics may be 1882 * those of a specimen, fetus, donor, other observer (for example a 1883 * relative or EMT), or any observation made about the subject.) 1884 */ 1885 public Observation setSubject(Reference value) { 1886 this.subject = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return {@link #subject} The actual object that is the target of the 1892 * reference. The reference library doesn't populate this, but you can 1893 * use it to hold the resource if you resolve it. (The patient, or group 1894 * of patients, location, or device whose characteristics (direct or 1895 * indirect) are described by the observation and into whose record the 1896 * observation is placed. Comments: Indirect characteristics may be 1897 * those of a specimen, fetus, donor, other observer (for example a 1898 * relative or EMT), or any observation made about the subject.) 1899 */ 1900 public Resource getSubjectTarget() { 1901 return this.subjectTarget; 1902 } 1903 1904 /** 1905 * @param value {@link #subject} The actual object that is the target of the 1906 * reference. The reference library doesn't use these, but you can 1907 * use it to hold the resource if you resolve it. (The patient, or 1908 * group of patients, location, or device whose characteristics 1909 * (direct or indirect) are described by the observation and into 1910 * whose record the observation is placed. Comments: Indirect 1911 * characteristics may be those of a specimen, fetus, donor, other 1912 * observer (for example a relative or EMT), or any observation 1913 * made about the subject.) 1914 */ 1915 public Observation setSubjectTarget(Resource value) { 1916 this.subjectTarget = value; 1917 return this; 1918 } 1919 1920 /** 1921 * @return {@link #encounter} (The healthcare event (e.g. a patient and 1922 * healthcare provider interaction) during which this observation is 1923 * made.) 1924 */ 1925 public Reference getEncounter() { 1926 if (this.encounter == null) 1927 if (Configuration.errorOnAutoCreate()) 1928 throw new Error("Attempt to auto-create Observation.encounter"); 1929 else if (Configuration.doAutoCreate()) 1930 this.encounter = new Reference(); // cc 1931 return this.encounter; 1932 } 1933 1934 public boolean hasEncounter() { 1935 return this.encounter != null && !this.encounter.isEmpty(); 1936 } 1937 1938 /** 1939 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 1940 * healthcare provider interaction) during which this observation 1941 * is made.) 1942 */ 1943 public Observation setEncounter(Reference value) { 1944 this.encounter = value; 1945 return this; 1946 } 1947 1948 /** 1949 * @return {@link #encounter} The actual object that is the target of the 1950 * reference. The reference library doesn't populate this, but you can 1951 * use it to hold the resource if you resolve it. (The healthcare event 1952 * (e.g. a patient and healthcare provider interaction) during which 1953 * this observation is made.) 1954 */ 1955 public Encounter getEncounterTarget() { 1956 if (this.encounterTarget == null) 1957 if (Configuration.errorOnAutoCreate()) 1958 throw new Error("Attempt to auto-create Observation.encounter"); 1959 else if (Configuration.doAutoCreate()) 1960 this.encounterTarget = new Encounter(); // aa 1961 return this.encounterTarget; 1962 } 1963 1964 /** 1965 * @param value {@link #encounter} The actual object that is the target of the 1966 * reference. The reference library doesn't use these, but you can 1967 * use it to hold the resource if you resolve it. (The healthcare 1968 * event (e.g. a patient and healthcare provider interaction) 1969 * during which this observation is made.) 1970 */ 1971 public Observation setEncounterTarget(Encounter value) { 1972 this.encounterTarget = value; 1973 return this; 1974 } 1975 1976 /** 1977 * @return {@link #effective} (The time or time-period the observed value is 1978 * asserted as being true. For biological subjects - e.g. human patients 1979 * - this is usually called the "physiologically relevant time". This is 1980 * usually either the time of the procedure or of specimen collection, 1981 * but very often the source of the date/time is not known, only the 1982 * date/time itself.) 1983 */ 1984 public Type getEffective() { 1985 return this.effective; 1986 } 1987 1988 /** 1989 * @return {@link #effective} (The time or time-period the observed value is 1990 * asserted as being true. For biological subjects - e.g. human patients 1991 * - this is usually called the "physiologically relevant time". This is 1992 * usually either the time of the procedure or of specimen collection, 1993 * but very often the source of the date/time is not known, only the 1994 * date/time itself.) 1995 */ 1996 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1997 if (!(this.effective instanceof DateTimeType)) 1998 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1999 + this.effective.getClass().getName() + " was encountered"); 2000 return (DateTimeType) this.effective; 2001 } 2002 2003 public boolean hasEffectiveDateTimeType() { 2004 return this.effective instanceof DateTimeType; 2005 } 2006 2007 /** 2008 * @return {@link #effective} (The time or time-period the observed value is 2009 * asserted as being true. For biological subjects - e.g. human patients 2010 * - this is usually called the "physiologically relevant time". This is 2011 * usually either the time of the procedure or of specimen collection, 2012 * but very often the source of the date/time is not known, only the 2013 * date/time itself.) 2014 */ 2015 public Period getEffectivePeriod() throws FHIRException { 2016 if (!(this.effective instanceof Period)) 2017 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 2018 + " was encountered"); 2019 return (Period) this.effective; 2020 } 2021 2022 public boolean hasEffectivePeriod() { 2023 return this.effective instanceof Period; 2024 } 2025 2026 public boolean hasEffective() { 2027 return this.effective != null && !this.effective.isEmpty(); 2028 } 2029 2030 /** 2031 * @param value {@link #effective} (The time or time-period the observed value 2032 * is asserted as being true. For biological subjects - e.g. human 2033 * patients - this is usually called the "physiologically relevant 2034 * time". This is usually either the time of the procedure or of 2035 * specimen collection, but very often the source of the date/time 2036 * is not known, only the date/time itself.) 2037 */ 2038 public Observation setEffective(Type value) { 2039 this.effective = value; 2040 return this; 2041 } 2042 2043 /** 2044 * @return {@link #issued} (The date and time this observation was made 2045 * available to providers, typically after the results have been 2046 * reviewed and verified.). This is the underlying object with id, value 2047 * and extensions. The accessor "getIssued" gives direct access to the 2048 * value 2049 */ 2050 public InstantType getIssuedElement() { 2051 if (this.issued == null) 2052 if (Configuration.errorOnAutoCreate()) 2053 throw new Error("Attempt to auto-create Observation.issued"); 2054 else if (Configuration.doAutoCreate()) 2055 this.issued = new InstantType(); // bb 2056 return this.issued; 2057 } 2058 2059 public boolean hasIssuedElement() { 2060 return this.issued != null && !this.issued.isEmpty(); 2061 } 2062 2063 public boolean hasIssued() { 2064 return this.issued != null && !this.issued.isEmpty(); 2065 } 2066 2067 /** 2068 * @param value {@link #issued} (The date and time this observation was made 2069 * available to providers, typically after the results have been 2070 * reviewed and verified.). This is the underlying object with id, 2071 * value and extensions. The accessor "getIssued" gives direct 2072 * access to the value 2073 */ 2074 public Observation setIssuedElement(InstantType value) { 2075 this.issued = value; 2076 return this; 2077 } 2078 2079 /** 2080 * @return The date and time this observation was made available to providers, 2081 * typically after the results have been reviewed and verified. 2082 */ 2083 public Date getIssued() { 2084 return this.issued == null ? null : this.issued.getValue(); 2085 } 2086 2087 /** 2088 * @param value The date and time this observation was made available to 2089 * providers, typically after the results have been reviewed and 2090 * verified. 2091 */ 2092 public Observation setIssued(Date value) { 2093 if (value == null) 2094 this.issued = null; 2095 else { 2096 if (this.issued == null) 2097 this.issued = new InstantType(); 2098 this.issued.setValue(value); 2099 } 2100 return this; 2101 } 2102 2103 /** 2104 * @return {@link #performer} (Who was responsible for asserting the observed 2105 * value as "true".) 2106 */ 2107 public List<Reference> getPerformer() { 2108 if (this.performer == null) 2109 this.performer = new ArrayList<Reference>(); 2110 return this.performer; 2111 } 2112 2113 public boolean hasPerformer() { 2114 if (this.performer == null) 2115 return false; 2116 for (Reference item : this.performer) 2117 if (!item.isEmpty()) 2118 return true; 2119 return false; 2120 } 2121 2122 /** 2123 * @return {@link #performer} (Who was responsible for asserting the observed 2124 * value as "true".) 2125 */ 2126 // syntactic sugar 2127 public Reference addPerformer() { // 3 2128 Reference t = new Reference(); 2129 if (this.performer == null) 2130 this.performer = new ArrayList<Reference>(); 2131 this.performer.add(t); 2132 return t; 2133 } 2134 2135 // syntactic sugar 2136 public Observation addPerformer(Reference t) { // 3 2137 if (t == null) 2138 return this; 2139 if (this.performer == null) 2140 this.performer = new ArrayList<Reference>(); 2141 this.performer.add(t); 2142 return this; 2143 } 2144 2145 /** 2146 * @return {@link #performer} (The actual objects that are the target of the 2147 * reference. The reference library doesn't populate this, but you can 2148 * use this to hold the resources if you resolvethemt. Who was 2149 * responsible for asserting the observed value as "true".) 2150 */ 2151 public List<Resource> getPerformerTarget() { 2152 if (this.performerTarget == null) 2153 this.performerTarget = new ArrayList<Resource>(); 2154 return this.performerTarget; 2155 } 2156 2157 /** 2158 * @return {@link #value} (The information determined as a result of making the 2159 * observation, if the information has a simple value.) 2160 */ 2161 public Type getValue() { 2162 return this.value; 2163 } 2164 2165 /** 2166 * @return {@link #value} (The information determined as a result of making the 2167 * observation, if the information has a simple value.) 2168 */ 2169 public Quantity getValueQuantity() throws FHIRException { 2170 if (!(this.value instanceof Quantity)) 2171 throw new FHIRException( 2172 "Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() + " was encountered"); 2173 return (Quantity) this.value; 2174 } 2175 2176 public boolean hasValueQuantity() { 2177 return this.value instanceof Quantity; 2178 } 2179 2180 /** 2181 * @return {@link #value} (The information determined as a result of making the 2182 * observation, if the information has a simple value.) 2183 */ 2184 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2185 if (!(this.value instanceof CodeableConcept)) 2186 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2187 + this.value.getClass().getName() + " was encountered"); 2188 return (CodeableConcept) this.value; 2189 } 2190 2191 public boolean hasValueCodeableConcept() { 2192 return this.value instanceof CodeableConcept; 2193 } 2194 2195 /** 2196 * @return {@link #value} (The information determined as a result of making the 2197 * observation, if the information has a simple value.) 2198 */ 2199 public StringType getValueStringType() throws FHIRException { 2200 if (!(this.value instanceof StringType)) 2201 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.value.getClass().getName() 2202 + " was encountered"); 2203 return (StringType) this.value; 2204 } 2205 2206 public boolean hasValueStringType() { 2207 return this.value instanceof StringType; 2208 } 2209 2210 /** 2211 * @return {@link #value} (The information determined as a result of making the 2212 * observation, if the information has a simple value.) 2213 */ 2214 public Range getValueRange() throws FHIRException { 2215 if (!(this.value instanceof Range)) 2216 throw new FHIRException( 2217 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 2218 return (Range) this.value; 2219 } 2220 2221 public boolean hasValueRange() { 2222 return this.value instanceof Range; 2223 } 2224 2225 /** 2226 * @return {@link #value} (The information determined as a result of making the 2227 * observation, if the information has a simple value.) 2228 */ 2229 public Ratio getValueRatio() throws FHIRException { 2230 if (!(this.value instanceof Ratio)) 2231 throw new FHIRException( 2232 "Type mismatch: the type Ratio was expected, but " + this.value.getClass().getName() + " was encountered"); 2233 return (Ratio) this.value; 2234 } 2235 2236 public boolean hasValueRatio() { 2237 return this.value instanceof Ratio; 2238 } 2239 2240 /** 2241 * @return {@link #value} (The information determined as a result of making the 2242 * observation, if the information has a simple value.) 2243 */ 2244 public SampledData getValueSampledData() throws FHIRException { 2245 if (!(this.value instanceof SampledData)) 2246 throw new FHIRException("Type mismatch: the type SampledData was expected, but " + this.value.getClass().getName() 2247 + " was encountered"); 2248 return (SampledData) this.value; 2249 } 2250 2251 public boolean hasValueSampledData() { 2252 return this.value instanceof SampledData; 2253 } 2254 2255 /** 2256 * @return {@link #value} (The information determined as a result of making the 2257 * observation, if the information has a simple value.) 2258 */ 2259 public Attachment getValueAttachment() throws FHIRException { 2260 if (!(this.value instanceof Attachment)) 2261 throw new FHIRException("Type mismatch: the type Attachment was expected, but " + this.value.getClass().getName() 2262 + " was encountered"); 2263 return (Attachment) this.value; 2264 } 2265 2266 public boolean hasValueAttachment() { 2267 return this.value instanceof Attachment; 2268 } 2269 2270 /** 2271 * @return {@link #value} (The information determined as a result of making the 2272 * observation, if the information has a simple value.) 2273 */ 2274 public TimeType getValueTimeType() throws FHIRException { 2275 if (!(this.value instanceof TimeType)) 2276 throw new FHIRException( 2277 "Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() + " was encountered"); 2278 return (TimeType) this.value; 2279 } 2280 2281 public boolean hasValueTimeType() { 2282 return this.value instanceof TimeType; 2283 } 2284 2285 /** 2286 * @return {@link #value} (The information determined as a result of making the 2287 * observation, if the information has a simple value.) 2288 */ 2289 public DateTimeType getValueDateTimeType() throws FHIRException { 2290 if (!(this.value instanceof DateTimeType)) 2291 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2292 + this.value.getClass().getName() + " was encountered"); 2293 return (DateTimeType) this.value; 2294 } 2295 2296 public boolean hasValueDateTimeType() { 2297 return this.value instanceof DateTimeType; 2298 } 2299 2300 /** 2301 * @return {@link #value} (The information determined as a result of making the 2302 * observation, if the information has a simple value.) 2303 */ 2304 public Period getValuePeriod() throws FHIRException { 2305 if (!(this.value instanceof Period)) 2306 throw new FHIRException( 2307 "Type mismatch: the type Period was expected, but " + this.value.getClass().getName() + " was encountered"); 2308 return (Period) this.value; 2309 } 2310 2311 public boolean hasValuePeriod() { 2312 return this.value instanceof Period; 2313 } 2314 2315 public boolean hasValue() { 2316 return this.value != null && !this.value.isEmpty(); 2317 } 2318 2319 /** 2320 * @param value {@link #value} (The information determined as a result of making 2321 * the observation, if the information has a simple value.) 2322 */ 2323 public Observation setValue(Type value) { 2324 this.value = value; 2325 return this; 2326 } 2327 2328 /** 2329 * @return {@link #dataAbsentReason} (Provides a reason why the expected value 2330 * in the element Observation.value[x] is missing.) 2331 */ 2332 public CodeableConcept getDataAbsentReason() { 2333 if (this.dataAbsentReason == null) 2334 if (Configuration.errorOnAutoCreate()) 2335 throw new Error("Attempt to auto-create Observation.dataAbsentReason"); 2336 else if (Configuration.doAutoCreate()) 2337 this.dataAbsentReason = new CodeableConcept(); // cc 2338 return this.dataAbsentReason; 2339 } 2340 2341 public boolean hasDataAbsentReason() { 2342 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 2343 } 2344 2345 /** 2346 * @param value {@link #dataAbsentReason} (Provides a reason why the expected 2347 * value in the element Observation.value[x] is missing.) 2348 */ 2349 public Observation setDataAbsentReason(CodeableConcept value) { 2350 this.dataAbsentReason = value; 2351 return this; 2352 } 2353 2354 /** 2355 * @return {@link #interpretation} (The assessment made based on the result of 2356 * the observation. Intended as a simple compact code often placed 2357 * adjacent to the result value in reports and flow sheets to signal the 2358 * meaning/normalcy status of the result. Otherwise known as abnormal 2359 * flag.) 2360 */ 2361 public CodeableConcept getInterpretation() { 2362 if (this.interpretation == null) 2363 if (Configuration.errorOnAutoCreate()) 2364 throw new Error("Attempt to auto-create Observation.interpretation"); 2365 else if (Configuration.doAutoCreate()) 2366 this.interpretation = new CodeableConcept(); // cc 2367 return this.interpretation; 2368 } 2369 2370 public boolean hasInterpretation() { 2371 return this.interpretation != null && !this.interpretation.isEmpty(); 2372 } 2373 2374 /** 2375 * @param value {@link #interpretation} (The assessment made based on the result 2376 * of the observation. Intended as a simple compact code often 2377 * placed adjacent to the result value in reports and flow sheets 2378 * to signal the meaning/normalcy status of the result. Otherwise 2379 * known as abnormal flag.) 2380 */ 2381 public Observation setInterpretation(CodeableConcept value) { 2382 this.interpretation = value; 2383 return this; 2384 } 2385 2386 /** 2387 * @return {@link #comments} (May include statements about significant, 2388 * unexpected or unreliable values, or information about the source of 2389 * the value where this may be relevant to the interpretation of the 2390 * result.). This is the underlying object with id, value and 2391 * extensions. The accessor "getComments" gives direct access to the 2392 * value 2393 */ 2394 public StringType getCommentsElement() { 2395 if (this.comments == null) 2396 if (Configuration.errorOnAutoCreate()) 2397 throw new Error("Attempt to auto-create Observation.comments"); 2398 else if (Configuration.doAutoCreate()) 2399 this.comments = new StringType(); // bb 2400 return this.comments; 2401 } 2402 2403 public boolean hasCommentsElement() { 2404 return this.comments != null && !this.comments.isEmpty(); 2405 } 2406 2407 public boolean hasComments() { 2408 return this.comments != null && !this.comments.isEmpty(); 2409 } 2410 2411 /** 2412 * @param value {@link #comments} (May include statements about significant, 2413 * unexpected or unreliable values, or information about the source 2414 * of the value where this may be relevant to the interpretation of 2415 * the result.). This is the underlying object with id, value and 2416 * extensions. The accessor "getComments" gives direct access to 2417 * the value 2418 */ 2419 public Observation setCommentsElement(StringType value) { 2420 this.comments = value; 2421 return this; 2422 } 2423 2424 /** 2425 * @return May include statements about significant, unexpected or unreliable 2426 * values, or information about the source of the value where this may 2427 * be relevant to the interpretation of the result. 2428 */ 2429 public String getComments() { 2430 return this.comments == null ? null : this.comments.getValue(); 2431 } 2432 2433 /** 2434 * @param value May include statements about significant, unexpected or 2435 * unreliable values, or information about the source of the value 2436 * where this may be relevant to the interpretation of the result. 2437 */ 2438 public Observation setComments(String value) { 2439 if (Utilities.noString(value)) 2440 this.comments = null; 2441 else { 2442 if (this.comments == null) 2443 this.comments = new StringType(); 2444 this.comments.setValue(value); 2445 } 2446 return this; 2447 } 2448 2449 /** 2450 * @return {@link #bodySite} (Indicates the site on the subject's body where the 2451 * observation was made (i.e. the target site).) 2452 */ 2453 public CodeableConcept getBodySite() { 2454 if (this.bodySite == null) 2455 if (Configuration.errorOnAutoCreate()) 2456 throw new Error("Attempt to auto-create Observation.bodySite"); 2457 else if (Configuration.doAutoCreate()) 2458 this.bodySite = new CodeableConcept(); // cc 2459 return this.bodySite; 2460 } 2461 2462 public boolean hasBodySite() { 2463 return this.bodySite != null && !this.bodySite.isEmpty(); 2464 } 2465 2466 /** 2467 * @param value {@link #bodySite} (Indicates the site on the subject's body 2468 * where the observation was made (i.e. the target site).) 2469 */ 2470 public Observation setBodySite(CodeableConcept value) { 2471 this.bodySite = value; 2472 return this; 2473 } 2474 2475 /** 2476 * @return {@link #method} (Indicates the mechanism used to perform the 2477 * observation.) 2478 */ 2479 public CodeableConcept getMethod() { 2480 if (this.method == null) 2481 if (Configuration.errorOnAutoCreate()) 2482 throw new Error("Attempt to auto-create Observation.method"); 2483 else if (Configuration.doAutoCreate()) 2484 this.method = new CodeableConcept(); // cc 2485 return this.method; 2486 } 2487 2488 public boolean hasMethod() { 2489 return this.method != null && !this.method.isEmpty(); 2490 } 2491 2492 /** 2493 * @param value {@link #method} (Indicates the mechanism used to perform the 2494 * observation.) 2495 */ 2496 public Observation setMethod(CodeableConcept value) { 2497 this.method = value; 2498 return this; 2499 } 2500 2501 /** 2502 * @return {@link #specimen} (The specimen that was used when this observation 2503 * was made.) 2504 */ 2505 public Reference getSpecimen() { 2506 if (this.specimen == null) 2507 if (Configuration.errorOnAutoCreate()) 2508 throw new Error("Attempt to auto-create Observation.specimen"); 2509 else if (Configuration.doAutoCreate()) 2510 this.specimen = new Reference(); // cc 2511 return this.specimen; 2512 } 2513 2514 public boolean hasSpecimen() { 2515 return this.specimen != null && !this.specimen.isEmpty(); 2516 } 2517 2518 /** 2519 * @param value {@link #specimen} (The specimen that was used when this 2520 * observation was made.) 2521 */ 2522 public Observation setSpecimen(Reference value) { 2523 this.specimen = value; 2524 return this; 2525 } 2526 2527 /** 2528 * @return {@link #specimen} The actual object that is the target of the 2529 * reference. The reference library doesn't populate this, but you can 2530 * use it to hold the resource if you resolve it. (The specimen that was 2531 * used when this observation was made.) 2532 */ 2533 public Specimen getSpecimenTarget() { 2534 if (this.specimenTarget == null) 2535 if (Configuration.errorOnAutoCreate()) 2536 throw new Error("Attempt to auto-create Observation.specimen"); 2537 else if (Configuration.doAutoCreate()) 2538 this.specimenTarget = new Specimen(); // aa 2539 return this.specimenTarget; 2540 } 2541 2542 /** 2543 * @param value {@link #specimen} The actual object that is the target of the 2544 * reference. The reference library doesn't use these, but you can 2545 * use it to hold the resource if you resolve it. (The specimen 2546 * that was used when this observation was made.) 2547 */ 2548 public Observation setSpecimenTarget(Specimen value) { 2549 this.specimenTarget = value; 2550 return this; 2551 } 2552 2553 /** 2554 * @return {@link #device} (The device used to generate the observation data.) 2555 */ 2556 public Reference getDevice() { 2557 if (this.device == null) 2558 if (Configuration.errorOnAutoCreate()) 2559 throw new Error("Attempt to auto-create Observation.device"); 2560 else if (Configuration.doAutoCreate()) 2561 this.device = new Reference(); // cc 2562 return this.device; 2563 } 2564 2565 public boolean hasDevice() { 2566 return this.device != null && !this.device.isEmpty(); 2567 } 2568 2569 /** 2570 * @param value {@link #device} (The device used to generate the observation 2571 * data.) 2572 */ 2573 public Observation setDevice(Reference value) { 2574 this.device = value; 2575 return this; 2576 } 2577 2578 /** 2579 * @return {@link #device} The actual object that is the target of the 2580 * reference. The reference library doesn't populate this, but you can 2581 * use it to hold the resource if you resolve it. (The device used to 2582 * generate the observation data.) 2583 */ 2584 public Resource getDeviceTarget() { 2585 return this.deviceTarget; 2586 } 2587 2588 /** 2589 * @param value {@link #device} The actual object that is the target of the 2590 * reference. The reference library doesn't use these, but you can 2591 * use it to hold the resource if you resolve it. (The device used 2592 * to generate the observation data.) 2593 */ 2594 public Observation setDeviceTarget(Resource value) { 2595 this.deviceTarget = value; 2596 return this; 2597 } 2598 2599 /** 2600 * @return {@link #referenceRange} (Guidance on how to interpret the value by 2601 * comparison to a normal or recommended range.) 2602 */ 2603 public List<ObservationReferenceRangeComponent> getReferenceRange() { 2604 if (this.referenceRange == null) 2605 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2606 return this.referenceRange; 2607 } 2608 2609 public boolean hasReferenceRange() { 2610 if (this.referenceRange == null) 2611 return false; 2612 for (ObservationReferenceRangeComponent item : this.referenceRange) 2613 if (!item.isEmpty()) 2614 return true; 2615 return false; 2616 } 2617 2618 /** 2619 * @return {@link #referenceRange} (Guidance on how to interpret the value by 2620 * comparison to a normal or recommended range.) 2621 */ 2622 // syntactic sugar 2623 public ObservationReferenceRangeComponent addReferenceRange() { // 3 2624 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 2625 if (this.referenceRange == null) 2626 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2627 this.referenceRange.add(t); 2628 return t; 2629 } 2630 2631 // syntactic sugar 2632 public Observation addReferenceRange(ObservationReferenceRangeComponent t) { // 3 2633 if (t == null) 2634 return this; 2635 if (this.referenceRange == null) 2636 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2637 this.referenceRange.add(t); 2638 return this; 2639 } 2640 2641 /** 2642 * @return {@link #related} (A reference to another resource (usually another 2643 * Observation but could also be a QuestionnaireAnswer) whose 2644 * relationship is defined by the relationship type code.) 2645 */ 2646 public List<ObservationRelatedComponent> getRelated() { 2647 if (this.related == null) 2648 this.related = new ArrayList<ObservationRelatedComponent>(); 2649 return this.related; 2650 } 2651 2652 public boolean hasRelated() { 2653 if (this.related == null) 2654 return false; 2655 for (ObservationRelatedComponent item : this.related) 2656 if (!item.isEmpty()) 2657 return true; 2658 return false; 2659 } 2660 2661 /** 2662 * @return {@link #related} (A reference to another resource (usually another 2663 * Observation but could also be a QuestionnaireAnswer) whose 2664 * relationship is defined by the relationship type code.) 2665 */ 2666 // syntactic sugar 2667 public ObservationRelatedComponent addRelated() { // 3 2668 ObservationRelatedComponent t = new ObservationRelatedComponent(); 2669 if (this.related == null) 2670 this.related = new ArrayList<ObservationRelatedComponent>(); 2671 this.related.add(t); 2672 return t; 2673 } 2674 2675 // syntactic sugar 2676 public Observation addRelated(ObservationRelatedComponent t) { // 3 2677 if (t == null) 2678 return this; 2679 if (this.related == null) 2680 this.related = new ArrayList<ObservationRelatedComponent>(); 2681 this.related.add(t); 2682 return this; 2683 } 2684 2685 /** 2686 * @return {@link #component} (Some observations have multiple component 2687 * observations. These component observations are expressed as separate 2688 * code value pairs that share the same attributes. Examples include 2689 * systolic and diastolic component observations for blood pressure 2690 * measurement and multiple component observations for genetics 2691 * observations.) 2692 */ 2693 public List<ObservationComponentComponent> getComponent() { 2694 if (this.component == null) 2695 this.component = new ArrayList<ObservationComponentComponent>(); 2696 return this.component; 2697 } 2698 2699 public boolean hasComponent() { 2700 if (this.component == null) 2701 return false; 2702 for (ObservationComponentComponent item : this.component) 2703 if (!item.isEmpty()) 2704 return true; 2705 return false; 2706 } 2707 2708 /** 2709 * @return {@link #component} (Some observations have multiple component 2710 * observations. These component observations are expressed as separate 2711 * code value pairs that share the same attributes. Examples include 2712 * systolic and diastolic component observations for blood pressure 2713 * measurement and multiple component observations for genetics 2714 * observations.) 2715 */ 2716 // syntactic sugar 2717 public ObservationComponentComponent addComponent() { // 3 2718 ObservationComponentComponent t = new ObservationComponentComponent(); 2719 if (this.component == null) 2720 this.component = new ArrayList<ObservationComponentComponent>(); 2721 this.component.add(t); 2722 return t; 2723 } 2724 2725 // syntactic sugar 2726 public Observation addComponent(ObservationComponentComponent t) { // 3 2727 if (t == null) 2728 return this; 2729 if (this.component == null) 2730 this.component = new ArrayList<ObservationComponentComponent>(); 2731 this.component.add(t); 2732 return this; 2733 } 2734 2735 protected void listChildren(List<Property> childrenList) { 2736 super.listChildren(childrenList); 2737 childrenList.add(new Property("identifier", "Identifier", 2738 "A unique identifier for the simple observation instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2739 childrenList 2740 .add(new Property("status", "code", "The status of the result value.", 0, java.lang.Integer.MAX_VALUE, status)); 2741 childrenList.add(new Property("category", "CodeableConcept", 2742 "A code that classifies the general type of observation being made. This is used for searching, sorting and display purposes.", 2743 0, java.lang.Integer.MAX_VALUE, category)); 2744 childrenList.add(new Property("code", "CodeableConcept", 2745 "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 2746 java.lang.Integer.MAX_VALUE, code)); 2747 childrenList.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 2748 "The patient, or group of patients, location, or device whose characteristics (direct or indirect) are described by the observation and into whose record the observation is placed. Comments: Indirect characteristics may be those of a specimen, fetus, donor, other observer (for example a relative or EMT), or any observation made about the subject.", 2749 0, java.lang.Integer.MAX_VALUE, subject)); 2750 childrenList.add(new Property("encounter", "Reference(Encounter)", 2751 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 2752 0, java.lang.Integer.MAX_VALUE, encounter)); 2753 childrenList.add(new Property("effective[x]", "dateTime|Period", 2754 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 2755 0, java.lang.Integer.MAX_VALUE, effective)); 2756 childrenList.add(new Property("issued", "instant", 2757 "The date and time this observation was made available to providers, typically after the results have been reviewed and verified.", 2758 0, java.lang.Integer.MAX_VALUE, issued)); 2759 childrenList.add(new Property("performer", "Reference(Practitioner|Organization|Patient|RelatedPerson)", 2760 "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, 2761 performer)); 2762 childrenList.add(new Property("value[x]", 2763 "Quantity|CodeableConcept|string|Range|Ratio|SampledData|Attachment|time|dateTime|Period", 2764 "The information determined as a result of making the observation, if the information has a simple value.", 0, 2765 java.lang.Integer.MAX_VALUE, value)); 2766 childrenList.add(new Property("dataAbsentReason", "CodeableConcept", 2767 "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 2768 java.lang.Integer.MAX_VALUE, dataAbsentReason)); 2769 childrenList.add(new Property("interpretation", "CodeableConcept", 2770 "The assessment made based on the result of the observation. Intended as a simple compact code often placed adjacent to the result value in reports and flow sheets to signal the meaning/normalcy status of the result. Otherwise known as abnormal flag.", 2771 0, java.lang.Integer.MAX_VALUE, interpretation)); 2772 childrenList.add(new Property("comments", "string", 2773 "May include statements about significant, unexpected or unreliable values, or information about the source of the value where this may be relevant to the interpretation of the result.", 2774 0, java.lang.Integer.MAX_VALUE, comments)); 2775 childrenList.add(new Property("bodySite", "CodeableConcept", 2776 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 2777 java.lang.Integer.MAX_VALUE, bodySite)); 2778 childrenList.add(new Property("method", "CodeableConcept", 2779 "Indicates the mechanism used to perform the observation.", 0, java.lang.Integer.MAX_VALUE, method)); 2780 childrenList.add(new Property("specimen", "Reference(Specimen)", 2781 "The specimen that was used when this observation was made.", 0, java.lang.Integer.MAX_VALUE, specimen)); 2782 childrenList.add(new Property("device", "Reference(Device|DeviceMetric)", 2783 "The device used to generate the observation data.", 0, java.lang.Integer.MAX_VALUE, device)); 2784 childrenList.add(new Property("referenceRange", "", 2785 "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, 2786 java.lang.Integer.MAX_VALUE, referenceRange)); 2787 childrenList.add(new Property("related", "", 2788 "A reference to another resource (usually another Observation but could also be a QuestionnaireAnswer) whose relationship is defined by the relationship type code.", 2789 0, java.lang.Integer.MAX_VALUE, related)); 2790 childrenList.add(new Property("component", "", 2791 "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 2792 0, java.lang.Integer.MAX_VALUE, component)); 2793 } 2794 2795 @Override 2796 public void setProperty(String name, Base value) throws FHIRException { 2797 if (name.equals("identifier")) 2798 this.getIdentifier().add(castToIdentifier(value)); 2799 else if (name.equals("status")) 2800 this.status = new ObservationStatusEnumFactory().fromType(value); // Enumeration<ObservationStatus> 2801 else if (name.equals("category")) 2802 this.category = castToCodeableConcept(value); // CodeableConcept 2803 else if (name.equals("code")) 2804 this.code = castToCodeableConcept(value); // CodeableConcept 2805 else if (name.equals("subject")) 2806 this.subject = castToReference(value); // Reference 2807 else if (name.equals("encounter")) 2808 this.encounter = castToReference(value); // Reference 2809 else if (name.equals("effective[x]")) 2810 this.effective = (Type) value; // Type 2811 else if (name.equals("issued")) 2812 this.issued = castToInstant(value); // InstantType 2813 else if (name.equals("performer")) 2814 this.getPerformer().add(castToReference(value)); 2815 else if (name.equals("value[x]")) 2816 this.value = (Type) value; // Type 2817 else if (name.equals("dataAbsentReason")) 2818 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 2819 else if (name.equals("interpretation")) 2820 this.interpretation = castToCodeableConcept(value); // CodeableConcept 2821 else if (name.equals("comments")) 2822 this.comments = castToString(value); // StringType 2823 else if (name.equals("bodySite")) 2824 this.bodySite = castToCodeableConcept(value); // CodeableConcept 2825 else if (name.equals("method")) 2826 this.method = castToCodeableConcept(value); // CodeableConcept 2827 else if (name.equals("specimen")) 2828 this.specimen = castToReference(value); // Reference 2829 else if (name.equals("device")) 2830 this.device = castToReference(value); // Reference 2831 else if (name.equals("referenceRange")) 2832 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 2833 else if (name.equals("related")) 2834 this.getRelated().add((ObservationRelatedComponent) value); 2835 else if (name.equals("component")) 2836 this.getComponent().add((ObservationComponentComponent) value); 2837 else 2838 super.setProperty(name, value); 2839 } 2840 2841 @Override 2842 public Base addChild(String name) throws FHIRException { 2843 if (name.equals("identifier")) { 2844 return addIdentifier(); 2845 } else if (name.equals("status")) { 2846 throw new FHIRException("Cannot call addChild on a singleton property Observation.status"); 2847 } else if (name.equals("category")) { 2848 this.category = new CodeableConcept(); 2849 return this.category; 2850 } else if (name.equals("code")) { 2851 this.code = new CodeableConcept(); 2852 return this.code; 2853 } else if (name.equals("subject")) { 2854 this.subject = new Reference(); 2855 return this.subject; 2856 } else if (name.equals("encounter")) { 2857 this.encounter = new Reference(); 2858 return this.encounter; 2859 } else if (name.equals("effectiveDateTime")) { 2860 this.effective = new DateTimeType(); 2861 return this.effective; 2862 } else if (name.equals("effectivePeriod")) { 2863 this.effective = new Period(); 2864 return this.effective; 2865 } else if (name.equals("issued")) { 2866 throw new FHIRException("Cannot call addChild on a singleton property Observation.issued"); 2867 } else if (name.equals("performer")) { 2868 return addPerformer(); 2869 } else if (name.equals("valueQuantity")) { 2870 this.value = new Quantity(); 2871 return this.value; 2872 } else if (name.equals("valueCodeableConcept")) { 2873 this.value = new CodeableConcept(); 2874 return this.value; 2875 } else if (name.equals("valueString")) { 2876 this.value = new StringType(); 2877 return this.value; 2878 } else if (name.equals("valueRange")) { 2879 this.value = new Range(); 2880 return this.value; 2881 } else if (name.equals("valueRatio")) { 2882 this.value = new Ratio(); 2883 return this.value; 2884 } else if (name.equals("valueSampledData")) { 2885 this.value = new SampledData(); 2886 return this.value; 2887 } else if (name.equals("valueAttachment")) { 2888 this.value = new Attachment(); 2889 return this.value; 2890 } else if (name.equals("valueTime")) { 2891 this.value = new TimeType(); 2892 return this.value; 2893 } else if (name.equals("valueDateTime")) { 2894 this.value = new DateTimeType(); 2895 return this.value; 2896 } else if (name.equals("valuePeriod")) { 2897 this.value = new Period(); 2898 return this.value; 2899 } else if (name.equals("dataAbsentReason")) { 2900 this.dataAbsentReason = new CodeableConcept(); 2901 return this.dataAbsentReason; 2902 } else if (name.equals("interpretation")) { 2903 this.interpretation = new CodeableConcept(); 2904 return this.interpretation; 2905 } else if (name.equals("comments")) { 2906 throw new FHIRException("Cannot call addChild on a singleton property Observation.comments"); 2907 } else if (name.equals("bodySite")) { 2908 this.bodySite = new CodeableConcept(); 2909 return this.bodySite; 2910 } else if (name.equals("method")) { 2911 this.method = new CodeableConcept(); 2912 return this.method; 2913 } else if (name.equals("specimen")) { 2914 this.specimen = new Reference(); 2915 return this.specimen; 2916 } else if (name.equals("device")) { 2917 this.device = new Reference(); 2918 return this.device; 2919 } else if (name.equals("referenceRange")) { 2920 return addReferenceRange(); 2921 } else if (name.equals("related")) { 2922 return addRelated(); 2923 } else if (name.equals("component")) { 2924 return addComponent(); 2925 } else 2926 return super.addChild(name); 2927 } 2928 2929 public String fhirType() { 2930 return "Observation"; 2931 2932 } 2933 2934 public Observation copy() { 2935 Observation dst = new Observation(); 2936 copyValues(dst); 2937 if (identifier != null) { 2938 dst.identifier = new ArrayList<Identifier>(); 2939 for (Identifier i : identifier) 2940 dst.identifier.add(i.copy()); 2941 } 2942 ; 2943 dst.status = status == null ? null : status.copy(); 2944 dst.category = category == null ? null : category.copy(); 2945 dst.code = code == null ? null : code.copy(); 2946 dst.subject = subject == null ? null : subject.copy(); 2947 dst.encounter = encounter == null ? null : encounter.copy(); 2948 dst.effective = effective == null ? null : effective.copy(); 2949 dst.issued = issued == null ? null : issued.copy(); 2950 if (performer != null) { 2951 dst.performer = new ArrayList<Reference>(); 2952 for (Reference i : performer) 2953 dst.performer.add(i.copy()); 2954 } 2955 ; 2956 dst.value = value == null ? null : value.copy(); 2957 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 2958 dst.interpretation = interpretation == null ? null : interpretation.copy(); 2959 dst.comments = comments == null ? null : comments.copy(); 2960 dst.bodySite = bodySite == null ? null : bodySite.copy(); 2961 dst.method = method == null ? null : method.copy(); 2962 dst.specimen = specimen == null ? null : specimen.copy(); 2963 dst.device = device == null ? null : device.copy(); 2964 if (referenceRange != null) { 2965 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 2966 for (ObservationReferenceRangeComponent i : referenceRange) 2967 dst.referenceRange.add(i.copy()); 2968 } 2969 ; 2970 if (related != null) { 2971 dst.related = new ArrayList<ObservationRelatedComponent>(); 2972 for (ObservationRelatedComponent i : related) 2973 dst.related.add(i.copy()); 2974 } 2975 ; 2976 if (component != null) { 2977 dst.component = new ArrayList<ObservationComponentComponent>(); 2978 for (ObservationComponentComponent i : component) 2979 dst.component.add(i.copy()); 2980 } 2981 ; 2982 return dst; 2983 } 2984 2985 protected Observation typedCopy() { 2986 return copy(); 2987 } 2988 2989 @Override 2990 public boolean equalsDeep(Base other) { 2991 if (!super.equalsDeep(other)) 2992 return false; 2993 if (!(other instanceof Observation)) 2994 return false; 2995 Observation o = (Observation) other; 2996 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2997 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 2998 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2999 && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) 3000 && compareDeep(performer, o.performer, true) && compareDeep(value, o.value, true) 3001 && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 3002 && compareDeep(interpretation, o.interpretation, true) && compareDeep(comments, o.comments, true) 3003 && compareDeep(bodySite, o.bodySite, true) && compareDeep(method, o.method, true) 3004 && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 3005 && compareDeep(referenceRange, o.referenceRange, true) && compareDeep(related, o.related, true) 3006 && compareDeep(component, o.component, true); 3007 } 3008 3009 @Override 3010 public boolean equalsShallow(Base other) { 3011 if (!super.equalsShallow(other)) 3012 return false; 3013 if (!(other instanceof Observation)) 3014 return false; 3015 Observation o = (Observation) other; 3016 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) 3017 && compareValues(comments, o.comments, true); 3018 } 3019 3020 public boolean isEmpty() { 3021 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 3022 && (category == null || category.isEmpty()) && (code == null || code.isEmpty()) 3023 && (subject == null || subject.isEmpty()) && (encounter == null || encounter.isEmpty()) 3024 && (effective == null || effective.isEmpty()) && (issued == null || issued.isEmpty()) 3025 && (performer == null || performer.isEmpty()) && (value == null || value.isEmpty()) 3026 && (dataAbsentReason == null || dataAbsentReason.isEmpty()) 3027 && (interpretation == null || interpretation.isEmpty()) && (comments == null || comments.isEmpty()) 3028 && (bodySite == null || bodySite.isEmpty()) && (method == null || method.isEmpty()) 3029 && (specimen == null || specimen.isEmpty()) && (device == null || device.isEmpty()) 3030 && (referenceRange == null || referenceRange.isEmpty()) && (related == null || related.isEmpty()) 3031 && (component == null || component.isEmpty()); 3032 } 3033 3034 @Override 3035 public ResourceType getResourceType() { 3036 return ResourceType.Observation; 3037 } 3038 3039 @SearchParamDefinition(name = "date", path = "Observation.effective[x]", description = "Obtained date/time. If the obtained element is a period, a date that falls in the period", type = "date") 3040 public static final String SP_DATE = "date"; 3041 @SearchParamDefinition(name = "code", path = "Observation.code", description = "The code of the observation type", type = "token") 3042 public static final String SP_CODE = "code"; 3043 @SearchParamDefinition(name = "subject", path = "Observation.subject", description = "The subject that the observation is about", type = "reference") 3044 public static final String SP_SUBJECT = "subject"; 3045 @SearchParamDefinition(name = "component-data-absent-reason", path = "Observation.component.dataAbsentReason", description = "The reason why the expected value in the element Observation.component.value[x] is missing.", type = "token") 3046 public static final String SP_COMPONENTDATAABSENTREASON = "component-data-absent-reason"; 3047 @SearchParamDefinition(name = "value-concept", path = "Observation.valueCodeableConcept", description = "The value of the observation, if the value is a CodeableConcept", type = "token") 3048 public static final String SP_VALUECONCEPT = "value-concept"; 3049 @SearchParamDefinition(name = "value-date", path = "Observation.valueDateTime | Observation.valuePeriod", description = "The value of the observation, if the value is a date or period of time", type = "date") 3050 public static final String SP_VALUEDATE = "value-date"; 3051 @SearchParamDefinition(name = "related", path = "null", description = "Related Observations - search on related-type and related-target together", type = "composite") 3052 public static final String SP_RELATED = "related"; 3053 @SearchParamDefinition(name = "patient", path = "Observation.subject", description = "The subject that the observation is about (if patient)", type = "reference") 3054 public static final String SP_PATIENT = "patient"; 3055 @SearchParamDefinition(name = "specimen", path = "Observation.specimen", description = "Specimen used for this observation", type = "reference") 3056 public static final String SP_SPECIMEN = "specimen"; 3057 @SearchParamDefinition(name = "component-code", path = "Observation.component.code", description = "The component code of the observation type", type = "token") 3058 public static final String SP_COMPONENTCODE = "component-code"; 3059 @SearchParamDefinition(name = "value-string", path = "Observation.valueString", description = "The value of the observation, if the value is a string, and also searches in CodeableConcept.text", type = "string") 3060 public static final String SP_VALUESTRING = "value-string"; 3061 @SearchParamDefinition(name = "identifier", path = "Observation.identifier", description = "The unique id for a particular observation", type = "token") 3062 public static final String SP_IDENTIFIER = "identifier"; 3063 @SearchParamDefinition(name = "component-code-value-[x]", path = "null", description = "Both component code and one of the component value parameters", type = "composite") 3064 public static final String SP_COMPONENTCODEVALUEX = "component-code-value-[x]"; 3065 @SearchParamDefinition(name = "code-value-[x]", path = "null", description = "Both code and one of the value parameters", type = "composite") 3066 public static final String SP_CODEVALUEX = "code-value-[x]"; 3067 @SearchParamDefinition(name = "performer", path = "Observation.performer", description = "Who performed the observation", type = "reference") 3068 public static final String SP_PERFORMER = "performer"; 3069 @SearchParamDefinition(name = "value-quantity", path = "Observation.valueQuantity", description = "The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 3070 public static final String SP_VALUEQUANTITY = "value-quantity"; 3071 @SearchParamDefinition(name = "component-value-quantity", path = "Observation.component.valueQuantity", description = "The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 3072 public static final String SP_COMPONENTVALUEQUANTITY = "component-value-quantity"; 3073 @SearchParamDefinition(name = "data-absent-reason", path = "Observation.dataAbsentReason", description = "The reason why the expected value in the element Observation.value[x] is missing.", type = "token") 3074 public static final String SP_DATAABSENTREASON = "data-absent-reason"; 3075 @SearchParamDefinition(name = "encounter", path = "Observation.encounter", description = "Healthcare event related to the observation", type = "reference") 3076 public static final String SP_ENCOUNTER = "encounter"; 3077 @SearchParamDefinition(name = "related-type", path = "Observation.related.type", description = "has-member | derived-from | sequel-to | replaces | qualified-by | interfered-by", type = "token") 3078 public static final String SP_RELATEDTYPE = "related-type"; 3079 @SearchParamDefinition(name = "related-target", path = "Observation.related.target", description = "Resource that is related to this one", type = "reference") 3080 public static final String SP_RELATEDTARGET = "related-target"; 3081 @SearchParamDefinition(name = "component-value-string", path = "Observation.component.valueString", description = "The value of the component observation, if the value is a string, and also searches in CodeableConcept.text", type = "string") 3082 public static final String SP_COMPONENTVALUESTRING = "component-value-string"; 3083 @SearchParamDefinition(name = "component-value-concept", path = "Observation.component.valueCodeableConcept", description = "The value of the component observation, if the value is a CodeableConcept", type = "token") 3084 public static final String SP_COMPONENTVALUECONCEPT = "component-value-concept"; 3085 @SearchParamDefinition(name = "category", path = "Observation.category", description = "The classification of the type of observation", type = "token") 3086 public static final String SP_CATEGORY = "category"; 3087 @SearchParamDefinition(name = "device", path = "Observation.device", description = "The Device that generated the observation data.", type = "reference") 3088 public static final String SP_DEVICE = "device"; 3089 @SearchParamDefinition(name = "status", path = "Observation.status", description = "The status of the observation", type = "token") 3090 public static final String SP_STATUS = "status"; 3091 3092}