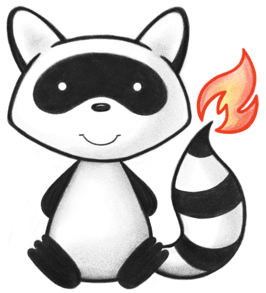
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.BindingStrength; 038import org.hl7.fhir.dstu2.model.Enumerations.BindingStrengthEnumFactory; 039import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 040import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.exceptions.FHIRException; 048import org.hl7.fhir.utilities.Utilities; 049 050/** 051 * A formal computable definition of an operation (on the RESTful interface) or 052 * a named query (using the search interaction). 053 */ 054@ResourceDef(name = "OperationDefinition", profile = "http://hl7.org/fhir/Profile/OperationDefinition") 055public class OperationDefinition extends DomainResource { 056 057 public enum OperationKind { 058 /** 059 * This operation is invoked as an operation. 060 */ 061 OPERATION, 062 /** 063 * This operation is a named query, invoked using the search mechanism. 064 */ 065 QUERY, 066 /** 067 * added to help the parsers 068 */ 069 NULL; 070 071 public static OperationKind fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("operation".equals(codeString)) 075 return OPERATION; 076 if ("query".equals(codeString)) 077 return QUERY; 078 throw new FHIRException("Unknown OperationKind code '" + codeString + "'"); 079 } 080 081 public String toCode() { 082 switch (this) { 083 case OPERATION: 084 return "operation"; 085 case QUERY: 086 return "query"; 087 case NULL: 088 return null; 089 default: 090 return "?"; 091 } 092 } 093 094 public String getSystem() { 095 switch (this) { 096 case OPERATION: 097 return "http://hl7.org/fhir/operation-kind"; 098 case QUERY: 099 return "http://hl7.org/fhir/operation-kind"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getDefinition() { 108 switch (this) { 109 case OPERATION: 110 return "This operation is invoked as an operation."; 111 case QUERY: 112 return "This operation is a named query, invoked using the search mechanism."; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getDisplay() { 121 switch (this) { 122 case OPERATION: 123 return "Operation"; 124 case QUERY: 125 return "Query"; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 } 133 134 public static class OperationKindEnumFactory implements EnumFactory<OperationKind> { 135 public OperationKind fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("operation".equals(codeString)) 140 return OperationKind.OPERATION; 141 if ("query".equals(codeString)) 142 return OperationKind.QUERY; 143 throw new IllegalArgumentException("Unknown OperationKind code '" + codeString + "'"); 144 } 145 146 public Enumeration<OperationKind> fromType(Base code) throws FHIRException { 147 if (code == null || code.isEmpty()) 148 return null; 149 String codeString = ((PrimitiveType) code).asStringValue(); 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("operation".equals(codeString)) 153 return new Enumeration<OperationKind>(this, OperationKind.OPERATION); 154 if ("query".equals(codeString)) 155 return new Enumeration<OperationKind>(this, OperationKind.QUERY); 156 throw new FHIRException("Unknown OperationKind code '" + codeString + "'"); 157 } 158 159 public String toCode(OperationKind code) 160 { 161 if (code == OperationKind.NULL) 162 return null; 163 if (code == OperationKind.OPERATION) 164 return "operation"; 165 if (code == OperationKind.QUERY) 166 return "query"; 167 return "?"; 168 } 169 } 170 171 public enum OperationParameterUse { 172 /** 173 * This is an input parameter. 174 */ 175 IN, 176 /** 177 * This is an output parameter. 178 */ 179 OUT, 180 /** 181 * added to help the parsers 182 */ 183 NULL; 184 185 public static OperationParameterUse fromCode(String codeString) throws FHIRException { 186 if (codeString == null || "".equals(codeString)) 187 return null; 188 if ("in".equals(codeString)) 189 return IN; 190 if ("out".equals(codeString)) 191 return OUT; 192 throw new FHIRException("Unknown OperationParameterUse code '" + codeString + "'"); 193 } 194 195 public String toCode() { 196 switch (this) { 197 case IN: 198 return "in"; 199 case OUT: 200 return "out"; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208 public String getSystem() { 209 switch (this) { 210 case IN: 211 return "http://hl7.org/fhir/operation-parameter-use"; 212 case OUT: 213 return "http://hl7.org/fhir/operation-parameter-use"; 214 case NULL: 215 return null; 216 default: 217 return "?"; 218 } 219 } 220 221 public String getDefinition() { 222 switch (this) { 223 case IN: 224 return "This is an input parameter."; 225 case OUT: 226 return "This is an output parameter."; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 234 public String getDisplay() { 235 switch (this) { 236 case IN: 237 return "In"; 238 case OUT: 239 return "Out"; 240 case NULL: 241 return null; 242 default: 243 return "?"; 244 } 245 } 246 } 247 248 public static class OperationParameterUseEnumFactory implements EnumFactory<OperationParameterUse> { 249 public OperationParameterUse fromCode(String codeString) throws IllegalArgumentException { 250 if (codeString == null || "".equals(codeString)) 251 if (codeString == null || "".equals(codeString)) 252 return null; 253 if ("in".equals(codeString)) 254 return OperationParameterUse.IN; 255 if ("out".equals(codeString)) 256 return OperationParameterUse.OUT; 257 throw new IllegalArgumentException("Unknown OperationParameterUse code '" + codeString + "'"); 258 } 259 260 public Enumeration<OperationParameterUse> fromType(Base code) throws FHIRException { 261 if (code == null || code.isEmpty()) 262 return null; 263 String codeString = ((PrimitiveType) code).asStringValue(); 264 if (codeString == null || "".equals(codeString)) 265 return null; 266 if ("in".equals(codeString)) 267 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.IN); 268 if ("out".equals(codeString)) 269 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.OUT); 270 throw new FHIRException("Unknown OperationParameterUse code '" + codeString + "'"); 271 } 272 273 public String toCode(OperationParameterUse code) 274 { 275 if (code == OperationParameterUse.NULL) 276 return null; 277 if (code == OperationParameterUse.IN) 278 return "in"; 279 if (code == OperationParameterUse.OUT) 280 return "out"; 281 return "?"; 282 } 283 } 284 285 @Block() 286 public static class OperationDefinitionContactComponent extends BackboneElement implements IBaseBackboneElement { 287 /** 288 * The name of an individual to contact regarding the operation definition. 289 */ 290 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 291 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the operation definition.") 292 protected StringType name; 293 294 /** 295 * Contact details for individual (if a name was provided) or the publisher. 296 */ 297 @Child(name = "telecom", type = { 298 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 299 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 300 protected List<ContactPoint> telecom; 301 302 private static final long serialVersionUID = -1179697803L; 303 304 /* 305 * Constructor 306 */ 307 public OperationDefinitionContactComponent() { 308 super(); 309 } 310 311 /** 312 * @return {@link #name} (The name of an individual to contact regarding the 313 * operation definition.). This is the underlying object with id, value 314 * and extensions. The accessor "getName" gives direct access to the 315 * value 316 */ 317 public StringType getNameElement() { 318 if (this.name == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create OperationDefinitionContactComponent.name"); 321 else if (Configuration.doAutoCreate()) 322 this.name = new StringType(); // bb 323 return this.name; 324 } 325 326 public boolean hasNameElement() { 327 return this.name != null && !this.name.isEmpty(); 328 } 329 330 public boolean hasName() { 331 return this.name != null && !this.name.isEmpty(); 332 } 333 334 /** 335 * @param value {@link #name} (The name of an individual to contact regarding 336 * the operation definition.). This is the underlying object with 337 * id, value and extensions. The accessor "getName" gives direct 338 * access to the value 339 */ 340 public OperationDefinitionContactComponent setNameElement(StringType value) { 341 this.name = value; 342 return this; 343 } 344 345 /** 346 * @return The name of an individual to contact regarding the operation 347 * definition. 348 */ 349 public String getName() { 350 return this.name == null ? null : this.name.getValue(); 351 } 352 353 /** 354 * @param value The name of an individual to contact regarding the operation 355 * definition. 356 */ 357 public OperationDefinitionContactComponent setName(String value) { 358 if (Utilities.noString(value)) 359 this.name = null; 360 else { 361 if (this.name == null) 362 this.name = new StringType(); 363 this.name.setValue(value); 364 } 365 return this; 366 } 367 368 /** 369 * @return {@link #telecom} (Contact details for individual (if a name was 370 * provided) or the publisher.) 371 */ 372 public List<ContactPoint> getTelecom() { 373 if (this.telecom == null) 374 this.telecom = new ArrayList<ContactPoint>(); 375 return this.telecom; 376 } 377 378 public boolean hasTelecom() { 379 if (this.telecom == null) 380 return false; 381 for (ContactPoint item : this.telecom) 382 if (!item.isEmpty()) 383 return true; 384 return false; 385 } 386 387 /** 388 * @return {@link #telecom} (Contact details for individual (if a name was 389 * provided) or the publisher.) 390 */ 391 // syntactic sugar 392 public ContactPoint addTelecom() { // 3 393 ContactPoint t = new ContactPoint(); 394 if (this.telecom == null) 395 this.telecom = new ArrayList<ContactPoint>(); 396 this.telecom.add(t); 397 return t; 398 } 399 400 // syntactic sugar 401 public OperationDefinitionContactComponent addTelecom(ContactPoint t) { // 3 402 if (t == null) 403 return this; 404 if (this.telecom == null) 405 this.telecom = new ArrayList<ContactPoint>(); 406 this.telecom.add(t); 407 return this; 408 } 409 410 protected void listChildren(List<Property> childrenList) { 411 super.listChildren(childrenList); 412 childrenList.add( 413 new Property("name", "string", "The name of an individual to contact regarding the operation definition.", 0, 414 java.lang.Integer.MAX_VALUE, name)); 415 childrenList.add(new Property("telecom", "ContactPoint", 416 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 417 telecom)); 418 } 419 420 @Override 421 public void setProperty(String name, Base value) throws FHIRException { 422 if (name.equals("name")) 423 this.name = castToString(value); // StringType 424 else if (name.equals("telecom")) 425 this.getTelecom().add(castToContactPoint(value)); 426 else 427 super.setProperty(name, value); 428 } 429 430 @Override 431 public Base addChild(String name) throws FHIRException { 432 if (name.equals("name")) { 433 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 434 } else if (name.equals("telecom")) { 435 return addTelecom(); 436 } else 437 return super.addChild(name); 438 } 439 440 public OperationDefinitionContactComponent copy() { 441 OperationDefinitionContactComponent dst = new OperationDefinitionContactComponent(); 442 copyValues(dst); 443 dst.name = name == null ? null : name.copy(); 444 if (telecom != null) { 445 dst.telecom = new ArrayList<ContactPoint>(); 446 for (ContactPoint i : telecom) 447 dst.telecom.add(i.copy()); 448 } 449 ; 450 return dst; 451 } 452 453 @Override 454 public boolean equalsDeep(Base other) { 455 if (!super.equalsDeep(other)) 456 return false; 457 if (!(other instanceof OperationDefinitionContactComponent)) 458 return false; 459 OperationDefinitionContactComponent o = (OperationDefinitionContactComponent) other; 460 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 461 } 462 463 @Override 464 public boolean equalsShallow(Base other) { 465 if (!super.equalsShallow(other)) 466 return false; 467 if (!(other instanceof OperationDefinitionContactComponent)) 468 return false; 469 OperationDefinitionContactComponent o = (OperationDefinitionContactComponent) other; 470 return compareValues(name, o.name, true); 471 } 472 473 public boolean isEmpty() { 474 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 475 } 476 477 public String fhirType() { 478 return "OperationDefinition.contact"; 479 480 } 481 482 } 483 484 @Block() 485 public static class OperationDefinitionParameterComponent extends BackboneElement implements IBaseBackboneElement { 486 /** 487 * The name of used to identify the parameter. 488 */ 489 @Child(name = "name", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 490 @Description(shortDefinition = "Name in Parameters.parameter.name or in URL", formalDefinition = "The name of used to identify the parameter.") 491 protected CodeType name; 492 493 /** 494 * Whether this is an input or an output parameter. 495 */ 496 @Child(name = "use", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 497 @Description(shortDefinition = "in | out", formalDefinition = "Whether this is an input or an output parameter.") 498 protected Enumeration<OperationParameterUse> use; 499 500 /** 501 * The minimum number of times this parameter SHALL appear in the request or 502 * response. 503 */ 504 @Child(name = "min", type = { IntegerType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 505 @Description(shortDefinition = "Minimum Cardinality", formalDefinition = "The minimum number of times this parameter SHALL appear in the request or response.") 506 protected IntegerType min; 507 508 /** 509 * The maximum number of times this element is permitted to appear in the 510 * request or response. 511 */ 512 @Child(name = "max", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 513 @Description(shortDefinition = "Maximum Cardinality (a number or *)", formalDefinition = "The maximum number of times this element is permitted to appear in the request or response.") 514 protected StringType max; 515 516 /** 517 * Describes the meaning or use of this parameter. 518 */ 519 @Child(name = "documentation", type = { 520 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 521 @Description(shortDefinition = "Description of meaning/use", formalDefinition = "Describes the meaning or use of this parameter.") 522 protected StringType documentation; 523 524 /** 525 * The type for this parameter. 526 */ 527 @Child(name = "type", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 528 @Description(shortDefinition = "What type this parameter has", formalDefinition = "The type for this parameter.") 529 protected CodeType type; 530 531 /** 532 * A profile the specifies the rules that this parameter must conform to. 533 */ 534 @Child(name = "profile", type = { 535 StructureDefinition.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 536 @Description(shortDefinition = "Profile on the type", formalDefinition = "A profile the specifies the rules that this parameter must conform to.") 537 protected Reference profile; 538 539 /** 540 * The actual object that is the target of the reference (A profile the 541 * specifies the rules that this parameter must conform to.) 542 */ 543 protected StructureDefinition profileTarget; 544 545 /** 546 * Binds to a value set if this parameter is coded (code, Coding, 547 * CodeableConcept). 548 */ 549 @Child(name = "binding", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = false) 550 @Description(shortDefinition = "ValueSet details if this is coded", formalDefinition = "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).") 551 protected OperationDefinitionParameterBindingComponent binding; 552 553 /** 554 * The parts of a Tuple Parameter. 555 */ 556 @Child(name = "part", type = { 557 OperationDefinitionParameterComponent.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 558 @Description(shortDefinition = "Parts of a Tuple Parameter", formalDefinition = "The parts of a Tuple Parameter.") 559 protected List<OperationDefinitionParameterComponent> part; 560 561 private static final long serialVersionUID = -1514145741L; 562 563 /* 564 * Constructor 565 */ 566 public OperationDefinitionParameterComponent() { 567 super(); 568 } 569 570 /* 571 * Constructor 572 */ 573 public OperationDefinitionParameterComponent(CodeType name, Enumeration<OperationParameterUse> use, IntegerType min, 574 StringType max) { 575 super(); 576 this.name = name; 577 this.use = use; 578 this.min = min; 579 this.max = max; 580 } 581 582 /** 583 * @return {@link #name} (The name of used to identify the parameter.). This is 584 * the underlying object with id, value and extensions. The accessor 585 * "getName" gives direct access to the value 586 */ 587 public CodeType getNameElement() { 588 if (this.name == null) 589 if (Configuration.errorOnAutoCreate()) 590 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.name"); 591 else if (Configuration.doAutoCreate()) 592 this.name = new CodeType(); // bb 593 return this.name; 594 } 595 596 public boolean hasNameElement() { 597 return this.name != null && !this.name.isEmpty(); 598 } 599 600 public boolean hasName() { 601 return this.name != null && !this.name.isEmpty(); 602 } 603 604 /** 605 * @param value {@link #name} (The name of used to identify the parameter.). 606 * This is the underlying object with id, value and extensions. The 607 * accessor "getName" gives direct access to the value 608 */ 609 public OperationDefinitionParameterComponent setNameElement(CodeType value) { 610 this.name = value; 611 return this; 612 } 613 614 /** 615 * @return The name of used to identify the parameter. 616 */ 617 public String getName() { 618 return this.name == null ? null : this.name.getValue(); 619 } 620 621 /** 622 * @param value The name of used to identify the parameter. 623 */ 624 public OperationDefinitionParameterComponent setName(String value) { 625 if (this.name == null) 626 this.name = new CodeType(); 627 this.name.setValue(value); 628 return this; 629 } 630 631 /** 632 * @return {@link #use} (Whether this is an input or an output parameter.). This 633 * is the underlying object with id, value and extensions. The accessor 634 * "getUse" gives direct access to the value 635 */ 636 public Enumeration<OperationParameterUse> getUseElement() { 637 if (this.use == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.use"); 640 else if (Configuration.doAutoCreate()) 641 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); // bb 642 return this.use; 643 } 644 645 public boolean hasUseElement() { 646 return this.use != null && !this.use.isEmpty(); 647 } 648 649 public boolean hasUse() { 650 return this.use != null && !this.use.isEmpty(); 651 } 652 653 /** 654 * @param value {@link #use} (Whether this is an input or an output parameter.). 655 * This is the underlying object with id, value and extensions. The 656 * accessor "getUse" gives direct access to the value 657 */ 658 public OperationDefinitionParameterComponent setUseElement(Enumeration<OperationParameterUse> value) { 659 this.use = value; 660 return this; 661 } 662 663 /** 664 * @return Whether this is an input or an output parameter. 665 */ 666 public OperationParameterUse getUse() { 667 return this.use == null ? null : this.use.getValue(); 668 } 669 670 /** 671 * @param value Whether this is an input or an output parameter. 672 */ 673 public OperationDefinitionParameterComponent setUse(OperationParameterUse value) { 674 if (this.use == null) 675 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); 676 this.use.setValue(value); 677 return this; 678 } 679 680 /** 681 * @return {@link #min} (The minimum number of times this parameter SHALL appear 682 * in the request or response.). This is the underlying object with id, 683 * value and extensions. The accessor "getMin" gives direct access to 684 * the value 685 */ 686 public IntegerType getMinElement() { 687 if (this.min == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.min"); 690 else if (Configuration.doAutoCreate()) 691 this.min = new IntegerType(); // bb 692 return this.min; 693 } 694 695 public boolean hasMinElement() { 696 return this.min != null && !this.min.isEmpty(); 697 } 698 699 public boolean hasMin() { 700 return this.min != null && !this.min.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #min} (The minimum number of times this parameter SHALL 705 * appear in the request or response.). This is the underlying 706 * object with id, value and extensions. The accessor "getMin" 707 * gives direct access to the value 708 */ 709 public OperationDefinitionParameterComponent setMinElement(IntegerType value) { 710 this.min = value; 711 return this; 712 } 713 714 /** 715 * @return The minimum number of times this parameter SHALL appear in the 716 * request or response. 717 */ 718 public int getMin() { 719 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 720 } 721 722 /** 723 * @param value The minimum number of times this parameter SHALL appear in the 724 * request or response. 725 */ 726 public OperationDefinitionParameterComponent setMin(int value) { 727 if (this.min == null) 728 this.min = new IntegerType(); 729 this.min.setValue(value); 730 return this; 731 } 732 733 /** 734 * @return {@link #max} (The maximum number of times this element is permitted 735 * to appear in the request or response.). This is the underlying object 736 * with id, value and extensions. The accessor "getMax" gives direct 737 * access to the value 738 */ 739 public StringType getMaxElement() { 740 if (this.max == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.max"); 743 else if (Configuration.doAutoCreate()) 744 this.max = new StringType(); // bb 745 return this.max; 746 } 747 748 public boolean hasMaxElement() { 749 return this.max != null && !this.max.isEmpty(); 750 } 751 752 public boolean hasMax() { 753 return this.max != null && !this.max.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #max} (The maximum number of times this element is 758 * permitted to appear in the request or response.). This is the 759 * underlying object with id, value and extensions. The accessor 760 * "getMax" gives direct access to the value 761 */ 762 public OperationDefinitionParameterComponent setMaxElement(StringType value) { 763 this.max = value; 764 return this; 765 } 766 767 /** 768 * @return The maximum number of times this element is permitted to appear in 769 * the request or response. 770 */ 771 public String getMax() { 772 return this.max == null ? null : this.max.getValue(); 773 } 774 775 /** 776 * @param value The maximum number of times this element is permitted to appear 777 * in the request or response. 778 */ 779 public OperationDefinitionParameterComponent setMax(String value) { 780 if (this.max == null) 781 this.max = new StringType(); 782 this.max.setValue(value); 783 return this; 784 } 785 786 /** 787 * @return {@link #documentation} (Describes the meaning or use of this 788 * parameter.). This is the underlying object with id, value and 789 * extensions. The accessor "getDocumentation" gives direct access to 790 * the value 791 */ 792 public StringType getDocumentationElement() { 793 if (this.documentation == null) 794 if (Configuration.errorOnAutoCreate()) 795 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.documentation"); 796 else if (Configuration.doAutoCreate()) 797 this.documentation = new StringType(); // bb 798 return this.documentation; 799 } 800 801 public boolean hasDocumentationElement() { 802 return this.documentation != null && !this.documentation.isEmpty(); 803 } 804 805 public boolean hasDocumentation() { 806 return this.documentation != null && !this.documentation.isEmpty(); 807 } 808 809 /** 810 * @param value {@link #documentation} (Describes the meaning or use of this 811 * parameter.). This is the underlying object with id, value and 812 * extensions. The accessor "getDocumentation" gives direct access 813 * to the value 814 */ 815 public OperationDefinitionParameterComponent setDocumentationElement(StringType value) { 816 this.documentation = value; 817 return this; 818 } 819 820 /** 821 * @return Describes the meaning or use of this parameter. 822 */ 823 public String getDocumentation() { 824 return this.documentation == null ? null : this.documentation.getValue(); 825 } 826 827 /** 828 * @param value Describes the meaning or use of this parameter. 829 */ 830 public OperationDefinitionParameterComponent setDocumentation(String value) { 831 if (Utilities.noString(value)) 832 this.documentation = null; 833 else { 834 if (this.documentation == null) 835 this.documentation = new StringType(); 836 this.documentation.setValue(value); 837 } 838 return this; 839 } 840 841 /** 842 * @return {@link #type} (The type for this parameter.). This is the underlying 843 * object with id, value and extensions. The accessor "getType" gives 844 * direct access to the value 845 */ 846 public CodeType getTypeElement() { 847 if (this.type == null) 848 if (Configuration.errorOnAutoCreate()) 849 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.type"); 850 else if (Configuration.doAutoCreate()) 851 this.type = new CodeType(); // bb 852 return this.type; 853 } 854 855 public boolean hasTypeElement() { 856 return this.type != null && !this.type.isEmpty(); 857 } 858 859 public boolean hasType() { 860 return this.type != null && !this.type.isEmpty(); 861 } 862 863 /** 864 * @param value {@link #type} (The type for this parameter.). This is the 865 * underlying object with id, value and extensions. The accessor 866 * "getType" gives direct access to the value 867 */ 868 public OperationDefinitionParameterComponent setTypeElement(CodeType value) { 869 this.type = value; 870 return this; 871 } 872 873 /** 874 * @return The type for this parameter. 875 */ 876 public String getType() { 877 return this.type == null ? null : this.type.getValue(); 878 } 879 880 /** 881 * @param value The type for this parameter. 882 */ 883 public OperationDefinitionParameterComponent setType(String value) { 884 if (Utilities.noString(value)) 885 this.type = null; 886 else { 887 if (this.type == null) 888 this.type = new CodeType(); 889 this.type.setValue(value); 890 } 891 return this; 892 } 893 894 /** 895 * @return {@link #profile} (A profile the specifies the rules that this 896 * parameter must conform to.) 897 */ 898 public Reference getProfile() { 899 if (this.profile == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.profile"); 902 else if (Configuration.doAutoCreate()) 903 this.profile = new Reference(); // cc 904 return this.profile; 905 } 906 907 public boolean hasProfile() { 908 return this.profile != null && !this.profile.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #profile} (A profile the specifies the rules that this 913 * parameter must conform to.) 914 */ 915 public OperationDefinitionParameterComponent setProfile(Reference value) { 916 this.profile = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #profile} The actual object that is the target of the 922 * reference. The reference library doesn't populate this, but you can 923 * use it to hold the resource if you resolve it. (A profile the 924 * specifies the rules that this parameter must conform to.) 925 */ 926 public StructureDefinition getProfileTarget() { 927 if (this.profileTarget == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.profile"); 930 else if (Configuration.doAutoCreate()) 931 this.profileTarget = new StructureDefinition(); // aa 932 return this.profileTarget; 933 } 934 935 /** 936 * @param value {@link #profile} The actual object that is the target of the 937 * reference. The reference library doesn't use these, but you can 938 * use it to hold the resource if you resolve it. (A profile the 939 * specifies the rules that this parameter must conform to.) 940 */ 941 public OperationDefinitionParameterComponent setProfileTarget(StructureDefinition value) { 942 this.profileTarget = value; 943 return this; 944 } 945 946 /** 947 * @return {@link #binding} (Binds to a value set if this parameter is coded 948 * (code, Coding, CodeableConcept).) 949 */ 950 public OperationDefinitionParameterBindingComponent getBinding() { 951 if (this.binding == null) 952 if (Configuration.errorOnAutoCreate()) 953 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.binding"); 954 else if (Configuration.doAutoCreate()) 955 this.binding = new OperationDefinitionParameterBindingComponent(); // cc 956 return this.binding; 957 } 958 959 public boolean hasBinding() { 960 return this.binding != null && !this.binding.isEmpty(); 961 } 962 963 /** 964 * @param value {@link #binding} (Binds to a value set if this parameter is 965 * coded (code, Coding, CodeableConcept).) 966 */ 967 public OperationDefinitionParameterComponent setBinding(OperationDefinitionParameterBindingComponent value) { 968 this.binding = value; 969 return this; 970 } 971 972 /** 973 * @return {@link #part} (The parts of a Tuple Parameter.) 974 */ 975 public List<OperationDefinitionParameterComponent> getPart() { 976 if (this.part == null) 977 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 978 return this.part; 979 } 980 981 public boolean hasPart() { 982 if (this.part == null) 983 return false; 984 for (OperationDefinitionParameterComponent item : this.part) 985 if (!item.isEmpty()) 986 return true; 987 return false; 988 } 989 990 /** 991 * @return {@link #part} (The parts of a Tuple Parameter.) 992 */ 993 // syntactic sugar 994 public OperationDefinitionParameterComponent addPart() { // 3 995 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 996 if (this.part == null) 997 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 998 this.part.add(t); 999 return t; 1000 } 1001 1002 // syntactic sugar 1003 public OperationDefinitionParameterComponent addPart(OperationDefinitionParameterComponent t) { // 3 1004 if (t == null) 1005 return this; 1006 if (this.part == null) 1007 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 1008 this.part.add(t); 1009 return this; 1010 } 1011 1012 protected void listChildren(List<Property> childrenList) { 1013 super.listChildren(childrenList); 1014 childrenList.add(new Property("name", "code", "The name of used to identify the parameter.", 0, 1015 java.lang.Integer.MAX_VALUE, name)); 1016 childrenList.add(new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1017 java.lang.Integer.MAX_VALUE, use)); 1018 childrenList.add(new Property("min", "integer", 1019 "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1020 java.lang.Integer.MAX_VALUE, min)); 1021 childrenList.add(new Property("max", "string", 1022 "The maximum number of times this element is permitted to appear in the request or response.", 0, 1023 java.lang.Integer.MAX_VALUE, max)); 1024 childrenList.add(new Property("documentation", "string", "Describes the meaning or use of this parameter.", 0, 1025 java.lang.Integer.MAX_VALUE, documentation)); 1026 childrenList 1027 .add(new Property("type", "code", "The type for this parameter.", 0, java.lang.Integer.MAX_VALUE, type)); 1028 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 1029 "A profile the specifies the rules that this parameter must conform to.", 0, java.lang.Integer.MAX_VALUE, 1030 profile)); 1031 childrenList.add(new Property("binding", "", 1032 "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1033 java.lang.Integer.MAX_VALUE, binding)); 1034 childrenList.add(new Property("part", "@OperationDefinition.parameter", "The parts of a Tuple Parameter.", 0, 1035 java.lang.Integer.MAX_VALUE, part)); 1036 } 1037 1038 @Override 1039 public void setProperty(String name, Base value) throws FHIRException { 1040 if (name.equals("name")) 1041 this.name = castToCode(value); // CodeType 1042 else if (name.equals("use")) 1043 this.use = new OperationParameterUseEnumFactory().fromType(value); // Enumeration<OperationParameterUse> 1044 else if (name.equals("min")) 1045 this.min = castToInteger(value); // IntegerType 1046 else if (name.equals("max")) 1047 this.max = castToString(value); // StringType 1048 else if (name.equals("documentation")) 1049 this.documentation = castToString(value); // StringType 1050 else if (name.equals("type")) 1051 this.type = castToCode(value); // CodeType 1052 else if (name.equals("profile")) 1053 this.profile = castToReference(value); // Reference 1054 else if (name.equals("binding")) 1055 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1056 else if (name.equals("part")) 1057 this.getPart().add((OperationDefinitionParameterComponent) value); 1058 else 1059 super.setProperty(name, value); 1060 } 1061 1062 @Override 1063 public Base addChild(String name) throws FHIRException { 1064 if (name.equals("name")) { 1065 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 1066 } else if (name.equals("use")) { 1067 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.use"); 1068 } else if (name.equals("min")) { 1069 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.min"); 1070 } else if (name.equals("max")) { 1071 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.max"); 1072 } else if (name.equals("documentation")) { 1073 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.documentation"); 1074 } else if (name.equals("type")) { 1075 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 1076 } else if (name.equals("profile")) { 1077 this.profile = new Reference(); 1078 return this.profile; 1079 } else if (name.equals("binding")) { 1080 this.binding = new OperationDefinitionParameterBindingComponent(); 1081 return this.binding; 1082 } else if (name.equals("part")) { 1083 return addPart(); 1084 } else 1085 return super.addChild(name); 1086 } 1087 1088 public OperationDefinitionParameterComponent copy() { 1089 OperationDefinitionParameterComponent dst = new OperationDefinitionParameterComponent(); 1090 copyValues(dst); 1091 dst.name = name == null ? null : name.copy(); 1092 dst.use = use == null ? null : use.copy(); 1093 dst.min = min == null ? null : min.copy(); 1094 dst.max = max == null ? null : max.copy(); 1095 dst.documentation = documentation == null ? null : documentation.copy(); 1096 dst.type = type == null ? null : type.copy(); 1097 dst.profile = profile == null ? null : profile.copy(); 1098 dst.binding = binding == null ? null : binding.copy(); 1099 if (part != null) { 1100 dst.part = new ArrayList<OperationDefinitionParameterComponent>(); 1101 for (OperationDefinitionParameterComponent i : part) 1102 dst.part.add(i.copy()); 1103 } 1104 ; 1105 return dst; 1106 } 1107 1108 @Override 1109 public boolean equalsDeep(Base other) { 1110 if (!super.equalsDeep(other)) 1111 return false; 1112 if (!(other instanceof OperationDefinitionParameterComponent)) 1113 return false; 1114 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other; 1115 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(min, o.min, true) 1116 && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) 1117 && compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) 1118 && compareDeep(binding, o.binding, true) && compareDeep(part, o.part, true); 1119 } 1120 1121 @Override 1122 public boolean equalsShallow(Base other) { 1123 if (!super.equalsShallow(other)) 1124 return false; 1125 if (!(other instanceof OperationDefinitionParameterComponent)) 1126 return false; 1127 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other; 1128 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(min, o.min, true) 1129 && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) 1130 && compareValues(type, o.type, true); 1131 } 1132 1133 public boolean isEmpty() { 1134 return super.isEmpty() && (name == null || name.isEmpty()) && (use == null || use.isEmpty()) 1135 && (min == null || min.isEmpty()) && (max == null || max.isEmpty()) 1136 && (documentation == null || documentation.isEmpty()) && (type == null || type.isEmpty()) 1137 && (profile == null || profile.isEmpty()) && (binding == null || binding.isEmpty()) 1138 && (part == null || part.isEmpty()); 1139 } 1140 1141 public String fhirType() { 1142 return "OperationDefinition.parameter"; 1143 1144 } 1145 1146 } 1147 1148 @Block() 1149 public static class OperationDefinitionParameterBindingComponent extends BackboneElement 1150 implements IBaseBackboneElement { 1151 /** 1152 * Indicates the degree of conformance expectations associated with this binding 1153 * - that is, the degree to which the provided value set must be adhered to in 1154 * the instances. 1155 */ 1156 @Child(name = "strength", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1157 @Description(shortDefinition = "required | extensible | preferred | example", formalDefinition = "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.") 1158 protected Enumeration<BindingStrength> strength; 1159 1160 /** 1161 * Points to the value set or external definition (e.g. implicit value set) that 1162 * identifies the set of codes to be used. 1163 */ 1164 @Child(name = "valueSet", type = { UriType.class, 1165 ValueSet.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1166 @Description(shortDefinition = "Source of value set", formalDefinition = "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.") 1167 protected Type valueSet; 1168 1169 private static final long serialVersionUID = 857140521L; 1170 1171 /* 1172 * Constructor 1173 */ 1174 public OperationDefinitionParameterBindingComponent() { 1175 super(); 1176 } 1177 1178 /* 1179 * Constructor 1180 */ 1181 public OperationDefinitionParameterBindingComponent(Enumeration<BindingStrength> strength, Type valueSet) { 1182 super(); 1183 this.strength = strength; 1184 this.valueSet = valueSet; 1185 } 1186 1187 /** 1188 * @return {@link #strength} (Indicates the degree of conformance expectations 1189 * associated with this binding - that is, the degree to which the 1190 * provided value set must be adhered to in the instances.). This is the 1191 * underlying object with id, value and extensions. The accessor 1192 * "getStrength" gives direct access to the value 1193 */ 1194 public Enumeration<BindingStrength> getStrengthElement() { 1195 if (this.strength == null) 1196 if (Configuration.errorOnAutoCreate()) 1197 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.strength"); 1198 else if (Configuration.doAutoCreate()) 1199 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 1200 return this.strength; 1201 } 1202 1203 public boolean hasStrengthElement() { 1204 return this.strength != null && !this.strength.isEmpty(); 1205 } 1206 1207 public boolean hasStrength() { 1208 return this.strength != null && !this.strength.isEmpty(); 1209 } 1210 1211 /** 1212 * @param value {@link #strength} (Indicates the degree of conformance 1213 * expectations associated with this binding - that is, the degree 1214 * to which the provided value set must be adhered to in the 1215 * instances.). This is the underlying object with id, value and 1216 * extensions. The accessor "getStrength" gives direct access to 1217 * the value 1218 */ 1219 public OperationDefinitionParameterBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 1220 this.strength = value; 1221 return this; 1222 } 1223 1224 /** 1225 * @return Indicates the degree of conformance expectations associated with this 1226 * binding - that is, the degree to which the provided value set must be 1227 * adhered to in the instances. 1228 */ 1229 public BindingStrength getStrength() { 1230 return this.strength == null ? null : this.strength.getValue(); 1231 } 1232 1233 /** 1234 * @param value Indicates the degree of conformance expectations associated with 1235 * this binding - that is, the degree to which the provided value 1236 * set must be adhered to in the instances. 1237 */ 1238 public OperationDefinitionParameterBindingComponent setStrength(BindingStrength value) { 1239 if (this.strength == null) 1240 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 1241 this.strength.setValue(value); 1242 return this; 1243 } 1244 1245 /** 1246 * @return {@link #valueSet} (Points to the value set or external definition 1247 * (e.g. implicit value set) that identifies the set of codes to be 1248 * used.) 1249 */ 1250 public Type getValueSet() { 1251 return this.valueSet; 1252 } 1253 1254 /** 1255 * @return {@link #valueSet} (Points to the value set or external definition 1256 * (e.g. implicit value set) that identifies the set of codes to be 1257 * used.) 1258 */ 1259 public UriType getValueSetUriType() throws FHIRException { 1260 if (!(this.valueSet instanceof UriType)) 1261 throw new FHIRException("Type mismatch: the type UriType was expected, but " 1262 + this.valueSet.getClass().getName() + " was encountered"); 1263 return (UriType) this.valueSet; 1264 } 1265 1266 public boolean hasValueSetUriType() { 1267 return this.valueSet instanceof UriType; 1268 } 1269 1270 /** 1271 * @return {@link #valueSet} (Points to the value set or external definition 1272 * (e.g. implicit value set) that identifies the set of codes to be 1273 * used.) 1274 */ 1275 public Reference getValueSetReference() throws FHIRException { 1276 if (!(this.valueSet instanceof Reference)) 1277 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1278 + this.valueSet.getClass().getName() + " was encountered"); 1279 return (Reference) this.valueSet; 1280 } 1281 1282 public boolean hasValueSetReference() { 1283 return this.valueSet instanceof Reference; 1284 } 1285 1286 public boolean hasValueSet() { 1287 return this.valueSet != null && !this.valueSet.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #valueSet} (Points to the value set or external 1292 * definition (e.g. implicit value set) that identifies the set of 1293 * codes to be used.) 1294 */ 1295 public OperationDefinitionParameterBindingComponent setValueSet(Type value) { 1296 this.valueSet = value; 1297 return this; 1298 } 1299 1300 protected void listChildren(List<Property> childrenList) { 1301 super.listChildren(childrenList); 1302 childrenList.add(new Property("strength", "code", 1303 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 1304 0, java.lang.Integer.MAX_VALUE, strength)); 1305 childrenList.add(new Property("valueSet[x]", "uri|Reference(ValueSet)", 1306 "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 1307 0, java.lang.Integer.MAX_VALUE, valueSet)); 1308 } 1309 1310 @Override 1311 public void setProperty(String name, Base value) throws FHIRException { 1312 if (name.equals("strength")) 1313 this.strength = new BindingStrengthEnumFactory().fromType(value); // Enumeration<BindingStrength> 1314 else if (name.equals("valueSet[x]")) 1315 this.valueSet = (Type) value; // Type 1316 else 1317 super.setProperty(name, value); 1318 } 1319 1320 @Override 1321 public Base addChild(String name) throws FHIRException { 1322 if (name.equals("strength")) { 1323 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.strength"); 1324 } else if (name.equals("valueSetUri")) { 1325 this.valueSet = new UriType(); 1326 return this.valueSet; 1327 } else if (name.equals("valueSetReference")) { 1328 this.valueSet = new Reference(); 1329 return this.valueSet; 1330 } else 1331 return super.addChild(name); 1332 } 1333 1334 public OperationDefinitionParameterBindingComponent copy() { 1335 OperationDefinitionParameterBindingComponent dst = new OperationDefinitionParameterBindingComponent(); 1336 copyValues(dst); 1337 dst.strength = strength == null ? null : strength.copy(); 1338 dst.valueSet = valueSet == null ? null : valueSet.copy(); 1339 return dst; 1340 } 1341 1342 @Override 1343 public boolean equalsDeep(Base other) { 1344 if (!super.equalsDeep(other)) 1345 return false; 1346 if (!(other instanceof OperationDefinitionParameterBindingComponent)) 1347 return false; 1348 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other; 1349 return compareDeep(strength, o.strength, true) && compareDeep(valueSet, o.valueSet, true); 1350 } 1351 1352 @Override 1353 public boolean equalsShallow(Base other) { 1354 if (!super.equalsShallow(other)) 1355 return false; 1356 if (!(other instanceof OperationDefinitionParameterBindingComponent)) 1357 return false; 1358 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other; 1359 return compareValues(strength, o.strength, true); 1360 } 1361 1362 public boolean isEmpty() { 1363 return super.isEmpty() && (strength == null || strength.isEmpty()) && (valueSet == null || valueSet.isEmpty()); 1364 } 1365 1366 public String fhirType() { 1367 return "OperationDefinition.parameter.binding"; 1368 1369 } 1370 1371 } 1372 1373 /** 1374 * An absolute URL that is used to identify this operation definition when it is 1375 * referenced in a specification, model, design or an instance. This SHALL be a 1376 * URL, SHOULD be globally unique, and SHOULD be an address at which this 1377 * operation definition is (or will be) published. 1378 */ 1379 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 1380 @Description(shortDefinition = "Logical URL to reference this operation definition", formalDefinition = "An absolute URL that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published.") 1381 protected UriType url; 1382 1383 /** 1384 * The identifier that is used to identify this version of the profile when it 1385 * is referenced in a specification, model, design or instance. This is an 1386 * arbitrary value managed by the profile author manually and the value should 1387 * be a timestamp. 1388 */ 1389 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1390 @Description(shortDefinition = "Logical id for this version of the operation definition", formalDefinition = "The identifier that is used to identify this version of the profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.") 1391 protected StringType version; 1392 1393 /** 1394 * A free text natural language name identifying the operation. 1395 */ 1396 @Child(name = "name", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1397 @Description(shortDefinition = "Informal name for this operation", formalDefinition = "A free text natural language name identifying the operation.") 1398 protected StringType name; 1399 1400 /** 1401 * The status of the profile. 1402 */ 1403 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = false) 1404 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the profile.") 1405 protected Enumeration<ConformanceResourceStatus> status; 1406 1407 /** 1408 * Whether this is an operation or a named query. 1409 */ 1410 @Child(name = "kind", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 1411 @Description(shortDefinition = "operation | query", formalDefinition = "Whether this is an operation or a named query.") 1412 protected Enumeration<OperationKind> kind; 1413 1414 /** 1415 * This profile was authored for testing purposes (or 1416 * education/evaluation/marketing), and is not intended to be used for genuine 1417 * usage. 1418 */ 1419 @Child(name = "experimental", type = { 1420 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1421 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "This profile was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 1422 protected BooleanType experimental; 1423 1424 /** 1425 * The name of the individual or organization that published the operation 1426 * definition. 1427 */ 1428 @Child(name = "publisher", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1429 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the operation definition.") 1430 protected StringType publisher; 1431 1432 /** 1433 * Contacts to assist a user in finding and communicating with the publisher. 1434 */ 1435 @Child(name = "contact", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1436 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 1437 protected List<OperationDefinitionContactComponent> contact; 1438 1439 /** 1440 * The date this version of the operation definition was published. The date 1441 * must change when the business version changes, if it does, and it must change 1442 * if the status code changes. In addition, it should change when the 1443 * substantive content of the Operation Definition changes. 1444 */ 1445 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1446 @Description(shortDefinition = "Date for this version of the operation definition", formalDefinition = "The date this version of the operation definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the Operation Definition changes.") 1447 protected DateTimeType date; 1448 1449 /** 1450 * A free text natural language description of the profile and its use. 1451 */ 1452 @Child(name = "description", type = { 1453 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1454 @Description(shortDefinition = "Natural language description of the operation", formalDefinition = "A free text natural language description of the profile and its use.") 1455 protected StringType description; 1456 1457 /** 1458 * Explains why this operation definition is needed and why it's been 1459 * constrained as it has. 1460 */ 1461 @Child(name = "requirements", type = { 1462 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1463 @Description(shortDefinition = "Why is this needed?", formalDefinition = "Explains why this operation definition is needed and why it's been constrained as it has.") 1464 protected StringType requirements; 1465 1466 /** 1467 * Operations that are idempotent (see [HTTP specification definition of 1468 * idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be 1469 * invoked by performing an HTTP GET operation instead of a POST. 1470 */ 1471 @Child(name = "idempotent", type = { 1472 BooleanType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1473 @Description(shortDefinition = "Whether content is unchanged by operation", formalDefinition = "Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST.") 1474 protected BooleanType idempotent; 1475 1476 /** 1477 * The name used to invoke the operation. 1478 */ 1479 @Child(name = "code", type = { CodeType.class }, order = 12, min = 1, max = 1, modifier = false, summary = false) 1480 @Description(shortDefinition = "Name used to invoke the operation", formalDefinition = "The name used to invoke the operation.") 1481 protected CodeType code; 1482 1483 /** 1484 * Additional information about how to use this operation or named query. 1485 */ 1486 @Child(name = "notes", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1487 @Description(shortDefinition = "Additional information about use", formalDefinition = "Additional information about how to use this operation or named query.") 1488 protected StringType notes; 1489 1490 /** 1491 * Indicates that this operation definition is a constraining profile on the 1492 * base. 1493 */ 1494 @Child(name = "base", type = { 1495 OperationDefinition.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1496 @Description(shortDefinition = "Marks this as a profile of the base", formalDefinition = "Indicates that this operation definition is a constraining profile on the base.") 1497 protected Reference base; 1498 1499 /** 1500 * The actual object that is the target of the reference (Indicates that this 1501 * operation definition is a constraining profile on the base.) 1502 */ 1503 protected OperationDefinition baseTarget; 1504 1505 /** 1506 * Indicates whether this operation or named query can be invoked at the system 1507 * level (e.g. without needing to choose a resource type for the context). 1508 */ 1509 @Child(name = "system", type = { BooleanType.class }, order = 15, min = 1, max = 1, modifier = false, summary = false) 1510 @Description(shortDefinition = "Invoke at the system level?", formalDefinition = "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).") 1511 protected BooleanType system; 1512 1513 /** 1514 * Indicates whether this operation or named query can be invoked at the 1515 * resource type level for any given resource type level (e.g. without needing 1516 * to choose a resource type for the context). 1517 */ 1518 @Child(name = "type", type = { 1519 CodeType.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1520 @Description(shortDefinition = "Invoke at resource level for these type", formalDefinition = "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a resource type for the context).") 1521 protected List<CodeType> type; 1522 1523 /** 1524 * Indicates whether this operation can be invoked on a particular instance of 1525 * one of the given types. 1526 */ 1527 @Child(name = "instance", type = { 1528 BooleanType.class }, order = 17, min = 1, max = 1, modifier = false, summary = false) 1529 @Description(shortDefinition = "Invoke on an instance?", formalDefinition = "Indicates whether this operation can be invoked on a particular instance of one of the given types.") 1530 protected BooleanType instance; 1531 1532 /** 1533 * The parameters for the operation/query. 1534 */ 1535 @Child(name = "parameter", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1536 @Description(shortDefinition = "Parameters for the operation/query", formalDefinition = "The parameters for the operation/query.") 1537 protected List<OperationDefinitionParameterComponent> parameter; 1538 1539 private static final long serialVersionUID = 148203484L; 1540 1541 /* 1542 * Constructor 1543 */ 1544 public OperationDefinition() { 1545 super(); 1546 } 1547 1548 /* 1549 * Constructor 1550 */ 1551 public OperationDefinition(StringType name, Enumeration<ConformanceResourceStatus> status, 1552 Enumeration<OperationKind> kind, CodeType code, BooleanType system, BooleanType instance) { 1553 super(); 1554 this.name = name; 1555 this.status = status; 1556 this.kind = kind; 1557 this.code = code; 1558 this.system = system; 1559 this.instance = instance; 1560 } 1561 1562 /** 1563 * @return {@link #url} (An absolute URL that is used to identify this operation 1564 * definition when it is referenced in a specification, model, design or 1565 * an instance. This SHALL be a URL, SHOULD be globally unique, and 1566 * SHOULD be an address at which this operation definition is (or will 1567 * be) published.). This is the underlying object with id, value and 1568 * extensions. The accessor "getUrl" gives direct access to the value 1569 */ 1570 public UriType getUrlElement() { 1571 if (this.url == null) 1572 if (Configuration.errorOnAutoCreate()) 1573 throw new Error("Attempt to auto-create OperationDefinition.url"); 1574 else if (Configuration.doAutoCreate()) 1575 this.url = new UriType(); // bb 1576 return this.url; 1577 } 1578 1579 public boolean hasUrlElement() { 1580 return this.url != null && !this.url.isEmpty(); 1581 } 1582 1583 public boolean hasUrl() { 1584 return this.url != null && !this.url.isEmpty(); 1585 } 1586 1587 /** 1588 * @param value {@link #url} (An absolute URL that is used to identify this 1589 * operation definition when it is referenced in a specification, 1590 * model, design or an instance. This SHALL be a URL, SHOULD be 1591 * globally unique, and SHOULD be an address at which this 1592 * operation definition is (or will be) published.). This is the 1593 * underlying object with id, value and extensions. The accessor 1594 * "getUrl" gives direct access to the value 1595 */ 1596 public OperationDefinition setUrlElement(UriType value) { 1597 this.url = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return An absolute URL that is used to identify this operation definition 1603 * when it is referenced in a specification, model, design or an 1604 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 1605 * be an address at which this operation definition is (or will be) 1606 * published. 1607 */ 1608 public String getUrl() { 1609 return this.url == null ? null : this.url.getValue(); 1610 } 1611 1612 /** 1613 * @param value An absolute URL that is used to identify this operation 1614 * definition when it is referenced in a specification, model, 1615 * design or an instance. This SHALL be a URL, SHOULD be globally 1616 * unique, and SHOULD be an address at which this operation 1617 * definition is (or will be) published. 1618 */ 1619 public OperationDefinition setUrl(String value) { 1620 if (Utilities.noString(value)) 1621 this.url = null; 1622 else { 1623 if (this.url == null) 1624 this.url = new UriType(); 1625 this.url.setValue(value); 1626 } 1627 return this; 1628 } 1629 1630 /** 1631 * @return {@link #version} (The identifier that is used to identify this 1632 * version of the profile when it is referenced in a specification, 1633 * model, design or instance. This is an arbitrary value managed by the 1634 * profile author manually and the value should be a timestamp.). This 1635 * is the underlying object with id, value and extensions. The accessor 1636 * "getVersion" gives direct access to the value 1637 */ 1638 public StringType getVersionElement() { 1639 if (this.version == null) 1640 if (Configuration.errorOnAutoCreate()) 1641 throw new Error("Attempt to auto-create OperationDefinition.version"); 1642 else if (Configuration.doAutoCreate()) 1643 this.version = new StringType(); // bb 1644 return this.version; 1645 } 1646 1647 public boolean hasVersionElement() { 1648 return this.version != null && !this.version.isEmpty(); 1649 } 1650 1651 public boolean hasVersion() { 1652 return this.version != null && !this.version.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #version} (The identifier that is used to identify this 1657 * version of the profile when it is referenced in a specification, 1658 * model, design or instance. This is an arbitrary value managed by 1659 * the profile author manually and the value should be a 1660 * timestamp.). This is the underlying object with id, value and 1661 * extensions. The accessor "getVersion" gives direct access to the 1662 * value 1663 */ 1664 public OperationDefinition setVersionElement(StringType value) { 1665 this.version = value; 1666 return this; 1667 } 1668 1669 /** 1670 * @return The identifier that is used to identify this version of the profile 1671 * when it is referenced in a specification, model, design or instance. 1672 * This is an arbitrary value managed by the profile author manually and 1673 * the value should be a timestamp. 1674 */ 1675 public String getVersion() { 1676 return this.version == null ? null : this.version.getValue(); 1677 } 1678 1679 /** 1680 * @param value The identifier that is used to identify this version of the 1681 * profile when it is referenced in a specification, model, design 1682 * or instance. This is an arbitrary value managed by the profile 1683 * author manually and the value should be a timestamp. 1684 */ 1685 public OperationDefinition setVersion(String value) { 1686 if (Utilities.noString(value)) 1687 this.version = null; 1688 else { 1689 if (this.version == null) 1690 this.version = new StringType(); 1691 this.version.setValue(value); 1692 } 1693 return this; 1694 } 1695 1696 /** 1697 * @return {@link #name} (A free text natural language name identifying the 1698 * operation.). This is the underlying object with id, value and 1699 * extensions. The accessor "getName" gives direct access to the value 1700 */ 1701 public StringType getNameElement() { 1702 if (this.name == null) 1703 if (Configuration.errorOnAutoCreate()) 1704 throw new Error("Attempt to auto-create OperationDefinition.name"); 1705 else if (Configuration.doAutoCreate()) 1706 this.name = new StringType(); // bb 1707 return this.name; 1708 } 1709 1710 public boolean hasNameElement() { 1711 return this.name != null && !this.name.isEmpty(); 1712 } 1713 1714 public boolean hasName() { 1715 return this.name != null && !this.name.isEmpty(); 1716 } 1717 1718 /** 1719 * @param value {@link #name} (A free text natural language name identifying the 1720 * operation.). This is the underlying object with id, value and 1721 * extensions. The accessor "getName" gives direct access to the 1722 * value 1723 */ 1724 public OperationDefinition setNameElement(StringType value) { 1725 this.name = value; 1726 return this; 1727 } 1728 1729 /** 1730 * @return A free text natural language name identifying the operation. 1731 */ 1732 public String getName() { 1733 return this.name == null ? null : this.name.getValue(); 1734 } 1735 1736 /** 1737 * @param value A free text natural language name identifying the operation. 1738 */ 1739 public OperationDefinition setName(String value) { 1740 if (this.name == null) 1741 this.name = new StringType(); 1742 this.name.setValue(value); 1743 return this; 1744 } 1745 1746 /** 1747 * @return {@link #status} (The status of the profile.). This is the underlying 1748 * object with id, value and extensions. The accessor "getStatus" gives 1749 * direct access to the value 1750 */ 1751 public Enumeration<ConformanceResourceStatus> getStatusElement() { 1752 if (this.status == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create OperationDefinition.status"); 1755 else if (Configuration.doAutoCreate()) 1756 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 1757 return this.status; 1758 } 1759 1760 public boolean hasStatusElement() { 1761 return this.status != null && !this.status.isEmpty(); 1762 } 1763 1764 public boolean hasStatus() { 1765 return this.status != null && !this.status.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #status} (The status of the profile.). This is the 1770 * underlying object with id, value and extensions. The accessor 1771 * "getStatus" gives direct access to the value 1772 */ 1773 public OperationDefinition setStatusElement(Enumeration<ConformanceResourceStatus> value) { 1774 this.status = value; 1775 return this; 1776 } 1777 1778 /** 1779 * @return The status of the profile. 1780 */ 1781 public ConformanceResourceStatus getStatus() { 1782 return this.status == null ? null : this.status.getValue(); 1783 } 1784 1785 /** 1786 * @param value The status of the profile. 1787 */ 1788 public OperationDefinition setStatus(ConformanceResourceStatus value) { 1789 if (this.status == null) 1790 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 1791 this.status.setValue(value); 1792 return this; 1793 } 1794 1795 /** 1796 * @return {@link #kind} (Whether this is an operation or a named query.). This 1797 * is the underlying object with id, value and extensions. The accessor 1798 * "getKind" gives direct access to the value 1799 */ 1800 public Enumeration<OperationKind> getKindElement() { 1801 if (this.kind == null) 1802 if (Configuration.errorOnAutoCreate()) 1803 throw new Error("Attempt to auto-create OperationDefinition.kind"); 1804 else if (Configuration.doAutoCreate()) 1805 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); // bb 1806 return this.kind; 1807 } 1808 1809 public boolean hasKindElement() { 1810 return this.kind != null && !this.kind.isEmpty(); 1811 } 1812 1813 public boolean hasKind() { 1814 return this.kind != null && !this.kind.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #kind} (Whether this is an operation or a named query.). 1819 * This is the underlying object with id, value and extensions. The 1820 * accessor "getKind" gives direct access to the value 1821 */ 1822 public OperationDefinition setKindElement(Enumeration<OperationKind> value) { 1823 this.kind = value; 1824 return this; 1825 } 1826 1827 /** 1828 * @return Whether this is an operation or a named query. 1829 */ 1830 public OperationKind getKind() { 1831 return this.kind == null ? null : this.kind.getValue(); 1832 } 1833 1834 /** 1835 * @param value Whether this is an operation or a named query. 1836 */ 1837 public OperationDefinition setKind(OperationKind value) { 1838 if (this.kind == null) 1839 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); 1840 this.kind.setValue(value); 1841 return this; 1842 } 1843 1844 /** 1845 * @return {@link #experimental} (This profile was authored for testing purposes 1846 * (or education/evaluation/marketing), and is not intended to be used 1847 * for genuine usage.). This is the underlying object with id, value and 1848 * extensions. The accessor "getExperimental" gives direct access to the 1849 * value 1850 */ 1851 public BooleanType getExperimentalElement() { 1852 if (this.experimental == null) 1853 if (Configuration.errorOnAutoCreate()) 1854 throw new Error("Attempt to auto-create OperationDefinition.experimental"); 1855 else if (Configuration.doAutoCreate()) 1856 this.experimental = new BooleanType(); // bb 1857 return this.experimental; 1858 } 1859 1860 public boolean hasExperimentalElement() { 1861 return this.experimental != null && !this.experimental.isEmpty(); 1862 } 1863 1864 public boolean hasExperimental() { 1865 return this.experimental != null && !this.experimental.isEmpty(); 1866 } 1867 1868 /** 1869 * @param value {@link #experimental} (This profile was authored for testing 1870 * purposes (or education/evaluation/marketing), and is not 1871 * intended to be used for genuine usage.). This is the underlying 1872 * object with id, value and extensions. The accessor 1873 * "getExperimental" gives direct access to the value 1874 */ 1875 public OperationDefinition setExperimentalElement(BooleanType value) { 1876 this.experimental = value; 1877 return this; 1878 } 1879 1880 /** 1881 * @return This profile was authored for testing purposes (or 1882 * education/evaluation/marketing), and is not intended to be used for 1883 * genuine usage. 1884 */ 1885 public boolean getExperimental() { 1886 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1887 } 1888 1889 /** 1890 * @param value This profile was authored for testing purposes (or 1891 * education/evaluation/marketing), and is not intended to be used 1892 * for genuine usage. 1893 */ 1894 public OperationDefinition setExperimental(boolean value) { 1895 if (this.experimental == null) 1896 this.experimental = new BooleanType(); 1897 this.experimental.setValue(value); 1898 return this; 1899 } 1900 1901 /** 1902 * @return {@link #publisher} (The name of the individual or organization that 1903 * published the operation definition.). This is the underlying object 1904 * with id, value and extensions. The accessor "getPublisher" gives 1905 * direct access to the value 1906 */ 1907 public StringType getPublisherElement() { 1908 if (this.publisher == null) 1909 if (Configuration.errorOnAutoCreate()) 1910 throw new Error("Attempt to auto-create OperationDefinition.publisher"); 1911 else if (Configuration.doAutoCreate()) 1912 this.publisher = new StringType(); // bb 1913 return this.publisher; 1914 } 1915 1916 public boolean hasPublisherElement() { 1917 return this.publisher != null && !this.publisher.isEmpty(); 1918 } 1919 1920 public boolean hasPublisher() { 1921 return this.publisher != null && !this.publisher.isEmpty(); 1922 } 1923 1924 /** 1925 * @param value {@link #publisher} (The name of the individual or organization 1926 * that published the operation definition.). This is the 1927 * underlying object with id, value and extensions. The accessor 1928 * "getPublisher" gives direct access to the value 1929 */ 1930 public OperationDefinition setPublisherElement(StringType value) { 1931 this.publisher = value; 1932 return this; 1933 } 1934 1935 /** 1936 * @return The name of the individual or organization that published the 1937 * operation definition. 1938 */ 1939 public String getPublisher() { 1940 return this.publisher == null ? null : this.publisher.getValue(); 1941 } 1942 1943 /** 1944 * @param value The name of the individual or organization that published the 1945 * operation definition. 1946 */ 1947 public OperationDefinition setPublisher(String value) { 1948 if (Utilities.noString(value)) 1949 this.publisher = null; 1950 else { 1951 if (this.publisher == null) 1952 this.publisher = new StringType(); 1953 this.publisher.setValue(value); 1954 } 1955 return this; 1956 } 1957 1958 /** 1959 * @return {@link #contact} (Contacts to assist a user in finding and 1960 * communicating with the publisher.) 1961 */ 1962 public List<OperationDefinitionContactComponent> getContact() { 1963 if (this.contact == null) 1964 this.contact = new ArrayList<OperationDefinitionContactComponent>(); 1965 return this.contact; 1966 } 1967 1968 public boolean hasContact() { 1969 if (this.contact == null) 1970 return false; 1971 for (OperationDefinitionContactComponent item : this.contact) 1972 if (!item.isEmpty()) 1973 return true; 1974 return false; 1975 } 1976 1977 /** 1978 * @return {@link #contact} (Contacts to assist a user in finding and 1979 * communicating with the publisher.) 1980 */ 1981 // syntactic sugar 1982 public OperationDefinitionContactComponent addContact() { // 3 1983 OperationDefinitionContactComponent t = new OperationDefinitionContactComponent(); 1984 if (this.contact == null) 1985 this.contact = new ArrayList<OperationDefinitionContactComponent>(); 1986 this.contact.add(t); 1987 return t; 1988 } 1989 1990 // syntactic sugar 1991 public OperationDefinition addContact(OperationDefinitionContactComponent t) { // 3 1992 if (t == null) 1993 return this; 1994 if (this.contact == null) 1995 this.contact = new ArrayList<OperationDefinitionContactComponent>(); 1996 this.contact.add(t); 1997 return this; 1998 } 1999 2000 /** 2001 * @return {@link #date} (The date this version of the operation definition was 2002 * published. The date must change when the business version changes, if 2003 * it does, and it must change if the status code changes. In addition, 2004 * it should change when the substantive content of the Operation 2005 * Definition changes.). This is the underlying object with id, value 2006 * and extensions. The accessor "getDate" gives direct access to the 2007 * value 2008 */ 2009 public DateTimeType getDateElement() { 2010 if (this.date == null) 2011 if (Configuration.errorOnAutoCreate()) 2012 throw new Error("Attempt to auto-create OperationDefinition.date"); 2013 else if (Configuration.doAutoCreate()) 2014 this.date = new DateTimeType(); // bb 2015 return this.date; 2016 } 2017 2018 public boolean hasDateElement() { 2019 return this.date != null && !this.date.isEmpty(); 2020 } 2021 2022 public boolean hasDate() { 2023 return this.date != null && !this.date.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #date} (The date this version of the operation definition 2028 * was published. The date must change when the business version 2029 * changes, if it does, and it must change if the status code 2030 * changes. In addition, it should change when the substantive 2031 * content of the Operation Definition changes.). This is the 2032 * underlying object with id, value and extensions. The accessor 2033 * "getDate" gives direct access to the value 2034 */ 2035 public OperationDefinition setDateElement(DateTimeType value) { 2036 this.date = value; 2037 return this; 2038 } 2039 2040 /** 2041 * @return The date this version of the operation definition was published. The 2042 * date must change when the business version changes, if it does, and 2043 * it must change if the status code changes. In addition, it should 2044 * change when the substantive content of the Operation Definition 2045 * changes. 2046 */ 2047 public Date getDate() { 2048 return this.date == null ? null : this.date.getValue(); 2049 } 2050 2051 /** 2052 * @param value The date this version of the operation definition was published. 2053 * The date must change when the business version changes, if it 2054 * does, and it must change if the status code changes. In 2055 * addition, it should change when the substantive content of the 2056 * Operation Definition changes. 2057 */ 2058 public OperationDefinition setDate(Date value) { 2059 if (value == null) 2060 this.date = null; 2061 else { 2062 if (this.date == null) 2063 this.date = new DateTimeType(); 2064 this.date.setValue(value); 2065 } 2066 return this; 2067 } 2068 2069 /** 2070 * @return {@link #description} (A free text natural language description of the 2071 * profile and its use.). This is the underlying object with id, value 2072 * and extensions. The accessor "getDescription" gives direct access to 2073 * the value 2074 */ 2075 public StringType getDescriptionElement() { 2076 if (this.description == null) 2077 if (Configuration.errorOnAutoCreate()) 2078 throw new Error("Attempt to auto-create OperationDefinition.description"); 2079 else if (Configuration.doAutoCreate()) 2080 this.description = new StringType(); // bb 2081 return this.description; 2082 } 2083 2084 public boolean hasDescriptionElement() { 2085 return this.description != null && !this.description.isEmpty(); 2086 } 2087 2088 public boolean hasDescription() { 2089 return this.description != null && !this.description.isEmpty(); 2090 } 2091 2092 /** 2093 * @param value {@link #description} (A free text natural language description 2094 * of the profile and its use.). This is the underlying object with 2095 * id, value and extensions. The accessor "getDescription" gives 2096 * direct access to the value 2097 */ 2098 public OperationDefinition setDescriptionElement(StringType value) { 2099 this.description = value; 2100 return this; 2101 } 2102 2103 /** 2104 * @return A free text natural language description of the profile and its use. 2105 */ 2106 public String getDescription() { 2107 return this.description == null ? null : this.description.getValue(); 2108 } 2109 2110 /** 2111 * @param value A free text natural language description of the profile and its 2112 * use. 2113 */ 2114 public OperationDefinition setDescription(String value) { 2115 if (Utilities.noString(value)) 2116 this.description = null; 2117 else { 2118 if (this.description == null) 2119 this.description = new StringType(); 2120 this.description.setValue(value); 2121 } 2122 return this; 2123 } 2124 2125 /** 2126 * @return {@link #requirements} (Explains why this operation definition is 2127 * needed and why it's been constrained as it has.). This is the 2128 * underlying object with id, value and extensions. The accessor 2129 * "getRequirements" gives direct access to the value 2130 */ 2131 public StringType getRequirementsElement() { 2132 if (this.requirements == null) 2133 if (Configuration.errorOnAutoCreate()) 2134 throw new Error("Attempt to auto-create OperationDefinition.requirements"); 2135 else if (Configuration.doAutoCreate()) 2136 this.requirements = new StringType(); // bb 2137 return this.requirements; 2138 } 2139 2140 public boolean hasRequirementsElement() { 2141 return this.requirements != null && !this.requirements.isEmpty(); 2142 } 2143 2144 public boolean hasRequirements() { 2145 return this.requirements != null && !this.requirements.isEmpty(); 2146 } 2147 2148 /** 2149 * @param value {@link #requirements} (Explains why this operation definition is 2150 * needed and why it's been constrained as it has.). This is the 2151 * underlying object with id, value and extensions. The accessor 2152 * "getRequirements" gives direct access to the value 2153 */ 2154 public OperationDefinition setRequirementsElement(StringType value) { 2155 this.requirements = value; 2156 return this; 2157 } 2158 2159 /** 2160 * @return Explains why this operation definition is needed and why it's been 2161 * constrained as it has. 2162 */ 2163 public String getRequirements() { 2164 return this.requirements == null ? null : this.requirements.getValue(); 2165 } 2166 2167 /** 2168 * @param value Explains why this operation definition is needed and why it's 2169 * been constrained as it has. 2170 */ 2171 public OperationDefinition setRequirements(String value) { 2172 if (Utilities.noString(value)) 2173 this.requirements = null; 2174 else { 2175 if (this.requirements == null) 2176 this.requirements = new StringType(); 2177 this.requirements.setValue(value); 2178 } 2179 return this; 2180 } 2181 2182 /** 2183 * @return {@link #idempotent} (Operations that are idempotent (see [HTTP 2184 * specification definition of 2185 * idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) 2186 * may be invoked by performing an HTTP GET operation instead of a 2187 * POST.). This is the underlying object with id, value and extensions. 2188 * The accessor "getIdempotent" gives direct access to the value 2189 */ 2190 public BooleanType getIdempotentElement() { 2191 if (this.idempotent == null) 2192 if (Configuration.errorOnAutoCreate()) 2193 throw new Error("Attempt to auto-create OperationDefinition.idempotent"); 2194 else if (Configuration.doAutoCreate()) 2195 this.idempotent = new BooleanType(); // bb 2196 return this.idempotent; 2197 } 2198 2199 public boolean hasIdempotentElement() { 2200 return this.idempotent != null && !this.idempotent.isEmpty(); 2201 } 2202 2203 public boolean hasIdempotent() { 2204 return this.idempotent != null && !this.idempotent.isEmpty(); 2205 } 2206 2207 /** 2208 * @param value {@link #idempotent} (Operations that are idempotent (see [HTTP 2209 * specification definition of 2210 * idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) 2211 * may be invoked by performing an HTTP GET operation instead of a 2212 * POST.). This is the underlying object with id, value and 2213 * extensions. The accessor "getIdempotent" gives direct access to 2214 * the value 2215 */ 2216 public OperationDefinition setIdempotentElement(BooleanType value) { 2217 this.idempotent = value; 2218 return this; 2219 } 2220 2221 /** 2222 * @return Operations that are idempotent (see [HTTP specification definition of 2223 * idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) 2224 * may be invoked by performing an HTTP GET operation instead of a POST. 2225 */ 2226 public boolean getIdempotent() { 2227 return this.idempotent == null || this.idempotent.isEmpty() ? false : this.idempotent.getValue(); 2228 } 2229 2230 /** 2231 * @param value Operations that are idempotent (see [HTTP specification 2232 * definition of 2233 * idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) 2234 * may be invoked by performing an HTTP GET operation instead of a 2235 * POST. 2236 */ 2237 public OperationDefinition setIdempotent(boolean value) { 2238 if (this.idempotent == null) 2239 this.idempotent = new BooleanType(); 2240 this.idempotent.setValue(value); 2241 return this; 2242 } 2243 2244 /** 2245 * @return {@link #code} (The name used to invoke the operation.). This is the 2246 * underlying object with id, value and extensions. The accessor 2247 * "getCode" gives direct access to the value 2248 */ 2249 public CodeType getCodeElement() { 2250 if (this.code == null) 2251 if (Configuration.errorOnAutoCreate()) 2252 throw new Error("Attempt to auto-create OperationDefinition.code"); 2253 else if (Configuration.doAutoCreate()) 2254 this.code = new CodeType(); // bb 2255 return this.code; 2256 } 2257 2258 public boolean hasCodeElement() { 2259 return this.code != null && !this.code.isEmpty(); 2260 } 2261 2262 public boolean hasCode() { 2263 return this.code != null && !this.code.isEmpty(); 2264 } 2265 2266 /** 2267 * @param value {@link #code} (The name used to invoke the operation.). This is 2268 * the underlying object with id, value and extensions. The 2269 * accessor "getCode" gives direct access to the value 2270 */ 2271 public OperationDefinition setCodeElement(CodeType value) { 2272 this.code = value; 2273 return this; 2274 } 2275 2276 /** 2277 * @return The name used to invoke the operation. 2278 */ 2279 public String getCode() { 2280 return this.code == null ? null : this.code.getValue(); 2281 } 2282 2283 /** 2284 * @param value The name used to invoke the operation. 2285 */ 2286 public OperationDefinition setCode(String value) { 2287 if (this.code == null) 2288 this.code = new CodeType(); 2289 this.code.setValue(value); 2290 return this; 2291 } 2292 2293 /** 2294 * @return {@link #notes} (Additional information about how to use this 2295 * operation or named query.). This is the underlying object with id, 2296 * value and extensions. The accessor "getNotes" gives direct access to 2297 * the value 2298 */ 2299 public StringType getNotesElement() { 2300 if (this.notes == null) 2301 if (Configuration.errorOnAutoCreate()) 2302 throw new Error("Attempt to auto-create OperationDefinition.notes"); 2303 else if (Configuration.doAutoCreate()) 2304 this.notes = new StringType(); // bb 2305 return this.notes; 2306 } 2307 2308 public boolean hasNotesElement() { 2309 return this.notes != null && !this.notes.isEmpty(); 2310 } 2311 2312 public boolean hasNotes() { 2313 return this.notes != null && !this.notes.isEmpty(); 2314 } 2315 2316 /** 2317 * @param value {@link #notes} (Additional information about how to use this 2318 * operation or named query.). This is the underlying object with 2319 * id, value and extensions. The accessor "getNotes" gives direct 2320 * access to the value 2321 */ 2322 public OperationDefinition setNotesElement(StringType value) { 2323 this.notes = value; 2324 return this; 2325 } 2326 2327 /** 2328 * @return Additional information about how to use this operation or named 2329 * query. 2330 */ 2331 public String getNotes() { 2332 return this.notes == null ? null : this.notes.getValue(); 2333 } 2334 2335 /** 2336 * @param value Additional information about how to use this operation or named 2337 * query. 2338 */ 2339 public OperationDefinition setNotes(String value) { 2340 if (Utilities.noString(value)) 2341 this.notes = null; 2342 else { 2343 if (this.notes == null) 2344 this.notes = new StringType(); 2345 this.notes.setValue(value); 2346 } 2347 return this; 2348 } 2349 2350 /** 2351 * @return {@link #base} (Indicates that this operation definition is a 2352 * constraining profile on the base.) 2353 */ 2354 public Reference getBase() { 2355 if (this.base == null) 2356 if (Configuration.errorOnAutoCreate()) 2357 throw new Error("Attempt to auto-create OperationDefinition.base"); 2358 else if (Configuration.doAutoCreate()) 2359 this.base = new Reference(); // cc 2360 return this.base; 2361 } 2362 2363 public boolean hasBase() { 2364 return this.base != null && !this.base.isEmpty(); 2365 } 2366 2367 /** 2368 * @param value {@link #base} (Indicates that this operation definition is a 2369 * constraining profile on the base.) 2370 */ 2371 public OperationDefinition setBase(Reference value) { 2372 this.base = value; 2373 return this; 2374 } 2375 2376 /** 2377 * @return {@link #base} The actual object that is the target of the reference. 2378 * The reference library doesn't populate this, but you can use it to 2379 * hold the resource if you resolve it. (Indicates that this operation 2380 * definition is a constraining profile on the base.) 2381 */ 2382 public OperationDefinition getBaseTarget() { 2383 if (this.baseTarget == null) 2384 if (Configuration.errorOnAutoCreate()) 2385 throw new Error("Attempt to auto-create OperationDefinition.base"); 2386 else if (Configuration.doAutoCreate()) 2387 this.baseTarget = new OperationDefinition(); // aa 2388 return this.baseTarget; 2389 } 2390 2391 /** 2392 * @param value {@link #base} The actual object that is the target of the 2393 * reference. The reference library doesn't use these, but you can 2394 * use it to hold the resource if you resolve it. (Indicates that 2395 * this operation definition is a constraining profile on the 2396 * base.) 2397 */ 2398 public OperationDefinition setBaseTarget(OperationDefinition value) { 2399 this.baseTarget = value; 2400 return this; 2401 } 2402 2403 /** 2404 * @return {@link #system} (Indicates whether this operation or named query can 2405 * be invoked at the system level (e.g. without needing to choose a 2406 * resource type for the context).). This is the underlying object with 2407 * id, value and extensions. The accessor "getSystem" gives direct 2408 * access to the value 2409 */ 2410 public BooleanType getSystemElement() { 2411 if (this.system == null) 2412 if (Configuration.errorOnAutoCreate()) 2413 throw new Error("Attempt to auto-create OperationDefinition.system"); 2414 else if (Configuration.doAutoCreate()) 2415 this.system = new BooleanType(); // bb 2416 return this.system; 2417 } 2418 2419 public boolean hasSystemElement() { 2420 return this.system != null && !this.system.isEmpty(); 2421 } 2422 2423 public boolean hasSystem() { 2424 return this.system != null && !this.system.isEmpty(); 2425 } 2426 2427 /** 2428 * @param value {@link #system} (Indicates whether this operation or named query 2429 * can be invoked at the system level (e.g. without needing to 2430 * choose a resource type for the context).). This is the 2431 * underlying object with id, value and extensions. The accessor 2432 * "getSystem" gives direct access to the value 2433 */ 2434 public OperationDefinition setSystemElement(BooleanType value) { 2435 this.system = value; 2436 return this; 2437 } 2438 2439 /** 2440 * @return Indicates whether this operation or named query can be invoked at the 2441 * system level (e.g. without needing to choose a resource type for the 2442 * context). 2443 */ 2444 public boolean getSystem() { 2445 return this.system == null || this.system.isEmpty() ? false : this.system.getValue(); 2446 } 2447 2448 /** 2449 * @param value Indicates whether this operation or named query can be invoked 2450 * at the system level (e.g. without needing to choose a resource 2451 * type for the context). 2452 */ 2453 public OperationDefinition setSystem(boolean value) { 2454 if (this.system == null) 2455 this.system = new BooleanType(); 2456 this.system.setValue(value); 2457 return this; 2458 } 2459 2460 /** 2461 * @return {@link #type} (Indicates whether this operation or named query can be 2462 * invoked at the resource type level for any given resource type level 2463 * (e.g. without needing to choose a resource type for the context).) 2464 */ 2465 public List<CodeType> getType() { 2466 if (this.type == null) 2467 this.type = new ArrayList<CodeType>(); 2468 return this.type; 2469 } 2470 2471 public boolean hasType() { 2472 if (this.type == null) 2473 return false; 2474 for (CodeType item : this.type) 2475 if (!item.isEmpty()) 2476 return true; 2477 return false; 2478 } 2479 2480 /** 2481 * @return {@link #type} (Indicates whether this operation or named query can be 2482 * invoked at the resource type level for any given resource type level 2483 * (e.g. without needing to choose a resource type for the context).) 2484 */ 2485 // syntactic sugar 2486 public CodeType addTypeElement() {// 2 2487 CodeType t = new CodeType(); 2488 if (this.type == null) 2489 this.type = new ArrayList<CodeType>(); 2490 this.type.add(t); 2491 return t; 2492 } 2493 2494 /** 2495 * @param value {@link #type} (Indicates whether this operation or named query 2496 * can be invoked at the resource type level for any given resource 2497 * type level (e.g. without needing to choose a resource type for 2498 * the context).) 2499 */ 2500 public OperationDefinition addType(String value) { // 1 2501 CodeType t = new CodeType(); 2502 t.setValue(value); 2503 if (this.type == null) 2504 this.type = new ArrayList<CodeType>(); 2505 this.type.add(t); 2506 return this; 2507 } 2508 2509 /** 2510 * @param value {@link #type} (Indicates whether this operation or named query 2511 * can be invoked at the resource type level for any given resource 2512 * type level (e.g. without needing to choose a resource type for 2513 * the context).) 2514 */ 2515 public boolean hasType(String value) { 2516 if (this.type == null) 2517 return false; 2518 for (CodeType v : this.type) 2519 if (v.equals(value)) // code 2520 return true; 2521 return false; 2522 } 2523 2524 /** 2525 * @return {@link #instance} (Indicates whether this operation can be invoked on 2526 * a particular instance of one of the given types.). This is the 2527 * underlying object with id, value and extensions. The accessor 2528 * "getInstance" gives direct access to the value 2529 */ 2530 public BooleanType getInstanceElement() { 2531 if (this.instance == null) 2532 if (Configuration.errorOnAutoCreate()) 2533 throw new Error("Attempt to auto-create OperationDefinition.instance"); 2534 else if (Configuration.doAutoCreate()) 2535 this.instance = new BooleanType(); // bb 2536 return this.instance; 2537 } 2538 2539 public boolean hasInstanceElement() { 2540 return this.instance != null && !this.instance.isEmpty(); 2541 } 2542 2543 public boolean hasInstance() { 2544 return this.instance != null && !this.instance.isEmpty(); 2545 } 2546 2547 /** 2548 * @param value {@link #instance} (Indicates whether this operation can be 2549 * invoked on a particular instance of one of the given types.). 2550 * This is the underlying object with id, value and extensions. The 2551 * accessor "getInstance" gives direct access to the value 2552 */ 2553 public OperationDefinition setInstanceElement(BooleanType value) { 2554 this.instance = value; 2555 return this; 2556 } 2557 2558 /** 2559 * @return Indicates whether this operation can be invoked on a particular 2560 * instance of one of the given types. 2561 */ 2562 public boolean getInstance() { 2563 return this.instance == null || this.instance.isEmpty() ? false : this.instance.getValue(); 2564 } 2565 2566 /** 2567 * @param value Indicates whether this operation can be invoked on a particular 2568 * instance of one of the given types. 2569 */ 2570 public OperationDefinition setInstance(boolean value) { 2571 if (this.instance == null) 2572 this.instance = new BooleanType(); 2573 this.instance.setValue(value); 2574 return this; 2575 } 2576 2577 /** 2578 * @return {@link #parameter} (The parameters for the operation/query.) 2579 */ 2580 public List<OperationDefinitionParameterComponent> getParameter() { 2581 if (this.parameter == null) 2582 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 2583 return this.parameter; 2584 } 2585 2586 public boolean hasParameter() { 2587 if (this.parameter == null) 2588 return false; 2589 for (OperationDefinitionParameterComponent item : this.parameter) 2590 if (!item.isEmpty()) 2591 return true; 2592 return false; 2593 } 2594 2595 /** 2596 * @return {@link #parameter} (The parameters for the operation/query.) 2597 */ 2598 // syntactic sugar 2599 public OperationDefinitionParameterComponent addParameter() { // 3 2600 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 2601 if (this.parameter == null) 2602 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 2603 this.parameter.add(t); 2604 return t; 2605 } 2606 2607 // syntactic sugar 2608 public OperationDefinition addParameter(OperationDefinitionParameterComponent t) { // 3 2609 if (t == null) 2610 return this; 2611 if (this.parameter == null) 2612 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 2613 this.parameter.add(t); 2614 return this; 2615 } 2616 2617 protected void listChildren(List<Property> childrenList) { 2618 super.listChildren(childrenList); 2619 childrenList.add(new Property("url", "uri", 2620 "An absolute URL that is used to identify this operation definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this operation definition is (or will be) published.", 2621 0, java.lang.Integer.MAX_VALUE, url)); 2622 childrenList.add(new Property("version", "string", 2623 "The identifier that is used to identify this version of the profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.", 2624 0, java.lang.Integer.MAX_VALUE, version)); 2625 childrenList.add(new Property("name", "string", "A free text natural language name identifying the operation.", 0, 2626 java.lang.Integer.MAX_VALUE, name)); 2627 childrenList 2628 .add(new Property("status", "code", "The status of the profile.", 0, java.lang.Integer.MAX_VALUE, status)); 2629 childrenList.add(new Property("kind", "code", "Whether this is an operation or a named query.", 0, 2630 java.lang.Integer.MAX_VALUE, kind)); 2631 childrenList.add(new Property("experimental", "boolean", 2632 "This profile was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 2633 0, java.lang.Integer.MAX_VALUE, experimental)); 2634 childrenList.add(new Property("publisher", "string", 2635 "The name of the individual or organization that published the operation definition.", 0, 2636 java.lang.Integer.MAX_VALUE, publisher)); 2637 childrenList 2638 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 2639 0, java.lang.Integer.MAX_VALUE, contact)); 2640 childrenList.add(new Property("date", "dateTime", 2641 "The date this version of the operation definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the Operation Definition changes.", 2642 0, java.lang.Integer.MAX_VALUE, date)); 2643 childrenList.add( 2644 new Property("description", "string", "A free text natural language description of the profile and its use.", 0, 2645 java.lang.Integer.MAX_VALUE, description)); 2646 childrenList.add(new Property("requirements", "string", 2647 "Explains why this operation definition is needed and why it's been constrained as it has.", 0, 2648 java.lang.Integer.MAX_VALUE, requirements)); 2649 childrenList.add(new Property("idempotent", "boolean", 2650 "Operations that are idempotent (see [HTTP specification definition of idempotent](http://www.w3.org/Protocols/rfc2616/rfc2616-sec9.html)) may be invoked by performing an HTTP GET operation instead of a POST.", 2651 0, java.lang.Integer.MAX_VALUE, idempotent)); 2652 childrenList.add( 2653 new Property("code", "code", "The name used to invoke the operation.", 0, java.lang.Integer.MAX_VALUE, code)); 2654 childrenList 2655 .add(new Property("notes", "string", "Additional information about how to use this operation or named query.", 2656 0, java.lang.Integer.MAX_VALUE, notes)); 2657 childrenList.add(new Property("base", "Reference(OperationDefinition)", 2658 "Indicates that this operation definition is a constraining profile on the base.", 0, 2659 java.lang.Integer.MAX_VALUE, base)); 2660 childrenList.add(new Property("system", "boolean", 2661 "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 2662 0, java.lang.Integer.MAX_VALUE, system)); 2663 childrenList.add(new Property("type", "code", 2664 "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a resource type for the context).", 2665 0, java.lang.Integer.MAX_VALUE, type)); 2666 childrenList.add(new Property("instance", "boolean", 2667 "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 2668 java.lang.Integer.MAX_VALUE, instance)); 2669 childrenList.add(new Property("parameter", "", "The parameters for the operation/query.", 0, 2670 java.lang.Integer.MAX_VALUE, parameter)); 2671 } 2672 2673 @Override 2674 public void setProperty(String name, Base value) throws FHIRException { 2675 if (name.equals("url")) 2676 this.url = castToUri(value); // UriType 2677 else if (name.equals("version")) 2678 this.version = castToString(value); // StringType 2679 else if (name.equals("name")) 2680 this.name = castToString(value); // StringType 2681 else if (name.equals("status")) 2682 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 2683 else if (name.equals("kind")) 2684 this.kind = new OperationKindEnumFactory().fromType(value); // Enumeration<OperationKind> 2685 else if (name.equals("experimental")) 2686 this.experimental = castToBoolean(value); // BooleanType 2687 else if (name.equals("publisher")) 2688 this.publisher = castToString(value); // StringType 2689 else if (name.equals("contact")) 2690 this.getContact().add((OperationDefinitionContactComponent) value); 2691 else if (name.equals("date")) 2692 this.date = castToDateTime(value); // DateTimeType 2693 else if (name.equals("description")) 2694 this.description = castToString(value); // StringType 2695 else if (name.equals("requirements")) 2696 this.requirements = castToString(value); // StringType 2697 else if (name.equals("idempotent")) 2698 this.idempotent = castToBoolean(value); // BooleanType 2699 else if (name.equals("code")) 2700 this.code = castToCode(value); // CodeType 2701 else if (name.equals("notes")) 2702 this.notes = castToString(value); // StringType 2703 else if (name.equals("base")) 2704 this.base = castToReference(value); // Reference 2705 else if (name.equals("system")) 2706 this.system = castToBoolean(value); // BooleanType 2707 else if (name.equals("type")) 2708 this.getType().add(castToCode(value)); 2709 else if (name.equals("instance")) 2710 this.instance = castToBoolean(value); // BooleanType 2711 else if (name.equals("parameter")) 2712 this.getParameter().add((OperationDefinitionParameterComponent) value); 2713 else 2714 super.setProperty(name, value); 2715 } 2716 2717 @Override 2718 public Base addChild(String name) throws FHIRException { 2719 if (name.equals("url")) { 2720 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.url"); 2721 } else if (name.equals("version")) { 2722 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.version"); 2723 } else if (name.equals("name")) { 2724 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 2725 } else if (name.equals("status")) { 2726 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.status"); 2727 } else if (name.equals("kind")) { 2728 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.kind"); 2729 } else if (name.equals("experimental")) { 2730 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.experimental"); 2731 } else if (name.equals("publisher")) { 2732 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.publisher"); 2733 } else if (name.equals("contact")) { 2734 return addContact(); 2735 } else if (name.equals("date")) { 2736 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.date"); 2737 } else if (name.equals("description")) { 2738 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.description"); 2739 } else if (name.equals("requirements")) { 2740 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.requirements"); 2741 } else if (name.equals("idempotent")) { 2742 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.idempotent"); 2743 } else if (name.equals("code")) { 2744 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.code"); 2745 } else if (name.equals("notes")) { 2746 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.notes"); 2747 } else if (name.equals("base")) { 2748 this.base = new Reference(); 2749 return this.base; 2750 } else if (name.equals("system")) { 2751 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.system"); 2752 } else if (name.equals("type")) { 2753 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 2754 } else if (name.equals("instance")) { 2755 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.instance"); 2756 } else if (name.equals("parameter")) { 2757 return addParameter(); 2758 } else 2759 return super.addChild(name); 2760 } 2761 2762 public String fhirType() { 2763 return "OperationDefinition"; 2764 2765 } 2766 2767 public OperationDefinition copy() { 2768 OperationDefinition dst = new OperationDefinition(); 2769 copyValues(dst); 2770 dst.url = url == null ? null : url.copy(); 2771 dst.version = version == null ? null : version.copy(); 2772 dst.name = name == null ? null : name.copy(); 2773 dst.status = status == null ? null : status.copy(); 2774 dst.kind = kind == null ? null : kind.copy(); 2775 dst.experimental = experimental == null ? null : experimental.copy(); 2776 dst.publisher = publisher == null ? null : publisher.copy(); 2777 if (contact != null) { 2778 dst.contact = new ArrayList<OperationDefinitionContactComponent>(); 2779 for (OperationDefinitionContactComponent i : contact) 2780 dst.contact.add(i.copy()); 2781 } 2782 ; 2783 dst.date = date == null ? null : date.copy(); 2784 dst.description = description == null ? null : description.copy(); 2785 dst.requirements = requirements == null ? null : requirements.copy(); 2786 dst.idempotent = idempotent == null ? null : idempotent.copy(); 2787 dst.code = code == null ? null : code.copy(); 2788 dst.notes = notes == null ? null : notes.copy(); 2789 dst.base = base == null ? null : base.copy(); 2790 dst.system = system == null ? null : system.copy(); 2791 if (type != null) { 2792 dst.type = new ArrayList<CodeType>(); 2793 for (CodeType i : type) 2794 dst.type.add(i.copy()); 2795 } 2796 ; 2797 dst.instance = instance == null ? null : instance.copy(); 2798 if (parameter != null) { 2799 dst.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 2800 for (OperationDefinitionParameterComponent i : parameter) 2801 dst.parameter.add(i.copy()); 2802 } 2803 ; 2804 return dst; 2805 } 2806 2807 protected OperationDefinition typedCopy() { 2808 return copy(); 2809 } 2810 2811 @Override 2812 public boolean equalsDeep(Base other) { 2813 if (!super.equalsDeep(other)) 2814 return false; 2815 if (!(other instanceof OperationDefinition)) 2816 return false; 2817 OperationDefinition o = (OperationDefinition) other; 2818 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 2819 && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) 2820 && compareDeep(experimental, o.experimental, true) && compareDeep(publisher, o.publisher, true) 2821 && compareDeep(contact, o.contact, true) && compareDeep(date, o.date, true) 2822 && compareDeep(description, o.description, true) && compareDeep(requirements, o.requirements, true) 2823 && compareDeep(idempotent, o.idempotent, true) && compareDeep(code, o.code, true) 2824 && compareDeep(notes, o.notes, true) && compareDeep(base, o.base, true) && compareDeep(system, o.system, true) 2825 && compareDeep(type, o.type, true) && compareDeep(instance, o.instance, true) 2826 && compareDeep(parameter, o.parameter, true); 2827 } 2828 2829 @Override 2830 public boolean equalsShallow(Base other) { 2831 if (!super.equalsShallow(other)) 2832 return false; 2833 if (!(other instanceof OperationDefinition)) 2834 return false; 2835 OperationDefinition o = (OperationDefinition) other; 2836 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 2837 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 2838 && compareValues(kind, o.kind, true) && compareValues(experimental, o.experimental, true) 2839 && compareValues(publisher, o.publisher, true) && compareValues(date, o.date, true) 2840 && compareValues(description, o.description, true) && compareValues(requirements, o.requirements, true) 2841 && compareValues(idempotent, o.idempotent, true) && compareValues(code, o.code, true) 2842 && compareValues(notes, o.notes, true) && compareValues(system, o.system, true) 2843 && compareValues(type, o.type, true) && compareValues(instance, o.instance, true); 2844 } 2845 2846 public boolean isEmpty() { 2847 return super.isEmpty() && (url == null || url.isEmpty()) && (version == null || version.isEmpty()) 2848 && (name == null || name.isEmpty()) && (status == null || status.isEmpty()) && (kind == null || kind.isEmpty()) 2849 && (experimental == null || experimental.isEmpty()) && (publisher == null || publisher.isEmpty()) 2850 && (contact == null || contact.isEmpty()) && (date == null || date.isEmpty()) 2851 && (description == null || description.isEmpty()) && (requirements == null || requirements.isEmpty()) 2852 && (idempotent == null || idempotent.isEmpty()) && (code == null || code.isEmpty()) 2853 && (notes == null || notes.isEmpty()) && (base == null || base.isEmpty()) 2854 && (system == null || system.isEmpty()) && (type == null || type.isEmpty()) 2855 && (instance == null || instance.isEmpty()) && (parameter == null || parameter.isEmpty()); 2856 } 2857 2858 @Override 2859 public ResourceType getResourceType() { 2860 return ResourceType.OperationDefinition; 2861 } 2862 2863 @SearchParamDefinition(name = "date", path = "OperationDefinition.date", description = "Date for this version of the operation definition", type = "date") 2864 public static final String SP_DATE = "date"; 2865 @SearchParamDefinition(name = "code", path = "OperationDefinition.code", description = "Name used to invoke the operation", type = "token") 2866 public static final String SP_CODE = "code"; 2867 @SearchParamDefinition(name = "instance", path = "OperationDefinition.instance", description = "Invoke on an instance?", type = "token") 2868 public static final String SP_INSTANCE = "instance"; 2869 @SearchParamDefinition(name = "kind", path = "OperationDefinition.kind", description = "operation | query", type = "token") 2870 public static final String SP_KIND = "kind"; 2871 @SearchParamDefinition(name = "profile", path = "OperationDefinition.parameter.profile", description = "Profile on the type", type = "reference") 2872 public static final String SP_PROFILE = "profile"; 2873 @SearchParamDefinition(name = "type", path = "OperationDefinition.type", description = "Invoke at resource level for these type", type = "token") 2874 public static final String SP_TYPE = "type"; 2875 @SearchParamDefinition(name = "version", path = "OperationDefinition.version", description = "Logical id for this version of the operation definition", type = "token") 2876 public static final String SP_VERSION = "version"; 2877 @SearchParamDefinition(name = "url", path = "OperationDefinition.url", description = "Logical URL to reference this operation definition", type = "uri") 2878 public static final String SP_URL = "url"; 2879 @SearchParamDefinition(name = "system", path = "OperationDefinition.system", description = "Invoke at the system level?", type = "token") 2880 public static final String SP_SYSTEM = "system"; 2881 @SearchParamDefinition(name = "name", path = "OperationDefinition.name", description = "Informal name for this operation", type = "string") 2882 public static final String SP_NAME = "name"; 2883 @SearchParamDefinition(name = "publisher", path = "OperationDefinition.publisher", description = "Name of the publisher (Organization or individual)", type = "string") 2884 public static final String SP_PUBLISHER = "publisher"; 2885 @SearchParamDefinition(name = "status", path = "OperationDefinition.status", description = "draft | active | retired", type = "token") 2886 public static final String SP_STATUS = "status"; 2887 @SearchParamDefinition(name = "base", path = "OperationDefinition.base", description = "Marks this as a profile of the base", type = "reference") 2888 public static final String SP_BASE = "base"; 2889 2890}