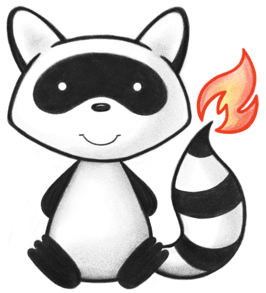
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.utilities.Utilities; 044 045/** 046 * A collection of error, warning or information messages that result from a 047 * system action. 048 */ 049@ResourceDef(name = "OperationOutcome", profile = "http://hl7.org/fhir/Profile/OperationOutcome") 050public class OperationOutcome extends DomainResource implements IBaseOperationOutcome { 051 052 public enum IssueSeverity { 053 /** 054 * The issue caused the action to fail, and no further checking could be 055 * performed. 056 */ 057 FATAL, 058 /** 059 * The issue is sufficiently important to cause the action to fail. 060 */ 061 ERROR, 062 /** 063 * The issue is not important enough to cause the action to fail, but may cause 064 * it to be performed suboptimally or in a way that is not as desired. 065 */ 066 WARNING, 067 /** 068 * The issue has no relation to the degree of success of the action. 069 */ 070 INFORMATION, 071 /** 072 * added to help the parsers 073 */ 074 NULL; 075 076 public static IssueSeverity fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("fatal".equals(codeString)) 080 return FATAL; 081 if ("error".equals(codeString)) 082 return ERROR; 083 if ("warning".equals(codeString)) 084 return WARNING; 085 if ("information".equals(codeString)) 086 return INFORMATION; 087 throw new FHIRException("Unknown IssueSeverity code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case FATAL: 093 return "fatal"; 094 case ERROR: 095 return "error"; 096 case WARNING: 097 return "warning"; 098 case INFORMATION: 099 return "information"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case FATAL: 110 return "http://hl7.org/fhir/issue-severity"; 111 case ERROR: 112 return "http://hl7.org/fhir/issue-severity"; 113 case WARNING: 114 return "http://hl7.org/fhir/issue-severity"; 115 case INFORMATION: 116 return "http://hl7.org/fhir/issue-severity"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDefinition() { 125 switch (this) { 126 case FATAL: 127 return "The issue caused the action to fail, and no further checking could be performed."; 128 case ERROR: 129 return "The issue is sufficiently important to cause the action to fail."; 130 case WARNING: 131 return "The issue is not important enough to cause the action to fail, but may cause it to be performed suboptimally or in a way that is not as desired."; 132 case INFORMATION: 133 return "The issue has no relation to the degree of success of the action."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case FATAL: 144 return "Fatal"; 145 case ERROR: 146 return "Error"; 147 case WARNING: 148 return "Warning"; 149 case INFORMATION: 150 return "Information"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 } 158 159 public static class IssueSeverityEnumFactory implements EnumFactory<IssueSeverity> { 160 public IssueSeverity fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("fatal".equals(codeString)) 165 return IssueSeverity.FATAL; 166 if ("error".equals(codeString)) 167 return IssueSeverity.ERROR; 168 if ("warning".equals(codeString)) 169 return IssueSeverity.WARNING; 170 if ("information".equals(codeString)) 171 return IssueSeverity.INFORMATION; 172 throw new IllegalArgumentException("Unknown IssueSeverity code '" + codeString + "'"); 173 } 174 175 public Enumeration<IssueSeverity> fromType(Base code) throws FHIRException { 176 if (code == null || code.isEmpty()) 177 return null; 178 String codeString = ((PrimitiveType) code).asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("fatal".equals(codeString)) 182 return new Enumeration<IssueSeverity>(this, IssueSeverity.FATAL); 183 if ("error".equals(codeString)) 184 return new Enumeration<IssueSeverity>(this, IssueSeverity.ERROR); 185 if ("warning".equals(codeString)) 186 return new Enumeration<IssueSeverity>(this, IssueSeverity.WARNING); 187 if ("information".equals(codeString)) 188 return new Enumeration<IssueSeverity>(this, IssueSeverity.INFORMATION); 189 throw new FHIRException("Unknown IssueSeverity code '" + codeString + "'"); 190 } 191 192 public String toCode(IssueSeverity code) 193 { 194 if (code == IssueSeverity.NULL) 195 return null; 196 if (code == IssueSeverity.FATAL) 197 return "fatal"; 198 if (code == IssueSeverity.ERROR) 199 return "error"; 200 if (code == IssueSeverity.WARNING) 201 return "warning"; 202 if (code == IssueSeverity.INFORMATION) 203 return "information"; 204 return "?"; 205 } 206 } 207 208 public enum IssueType { 209 /** 210 * Content invalid against the specification or a profile. 211 */ 212 INVALID, 213 /** 214 * A structural issue in the content such as wrong namespace, or unable to parse 215 * the content completely, or invalid json syntax. 216 */ 217 STRUCTURE, 218 /** 219 * A required element is missing. 220 */ 221 REQUIRED, 222 /** 223 * An element value is invalid. 224 */ 225 VALUE, 226 /** 227 * A content validation rule failed - e.g. a schematron rule. 228 */ 229 INVARIANT, 230 /** 231 * An authentication/authorization/permissions issue of some kind. 232 */ 233 SECURITY, 234 /** 235 * The client needs to initiate an authentication process. 236 */ 237 LOGIN, 238 /** 239 * The user or system was not able to be authenticated (either there is no 240 * process, or the proferred token is unacceptable). 241 */ 242 UNKNOWN, 243 /** 244 * User session expired; a login may be required. 245 */ 246 EXPIRED, 247 /** 248 * The user does not have the rights to perform this action. 249 */ 250 FORBIDDEN, 251 /** 252 * Some information was not or may not have been returned due to business rules, 253 * consent or privacy rules, or access permission constraints. This information 254 * may be accessible through alternate processes. 255 */ 256 SUPPRESSED, 257 /** 258 * Processing issues. These are expected to be final e.g. there is no point 259 * resubmitting the same content unchanged. 260 */ 261 PROCESSING, 262 /** 263 * The resource or profile is not supported. 264 */ 265 NOTSUPPORTED, 266 /** 267 * An attempt was made to create a duplicate record. 268 */ 269 DUPLICATE, 270 /** 271 * The reference provided was not found. In a pure RESTful environment, this 272 * would be an HTTP 404 error, but this code may be used where the content is 273 * not found further into the application architecture. 274 */ 275 NOTFOUND, 276 /** 277 * Provided content is too long (typically, this is a denial of service 278 * protection type of error). 279 */ 280 TOOLONG, 281 /** 282 * The code or system could not be understood, or it was not valid in the 283 * context of a particular ValueSet.code. 284 */ 285 CODEINVALID, 286 /** 287 * An extension was found that was not acceptable, could not be resolved, or a 288 * modifierExtension was not recognized. 289 */ 290 EXTENSION, 291 /** 292 * The operation was stopped to protect server resources; e.g. a request for a 293 * value set expansion on all of SNOMED CT. 294 */ 295 TOOCOSTLY, 296 /** 297 * The content/operation failed to pass some business rule, and so could not 298 * proceed. 299 */ 300 BUSINESSRULE, 301 /** 302 * Content could not be accepted because of an edit conflict (i.e. version aware 303 * updates) (In a pure RESTful environment, this would be an HTTP 404 error, but 304 * this code may be used where the conflict is discovered further into the 305 * application architecture.) 306 */ 307 CONFLICT, 308 /** 309 * Not all data sources typically accessed could be reached, or responded in 310 * time, so the returned information may not be complete. 311 */ 312 INCOMPLETE, 313 /** 314 * Transient processing issues. The system receiving the error may be able to 315 * resubmit the same content once an underlying issue is resolved. 316 */ 317 TRANSIENT, 318 /** 319 * A resource/record locking failure (usually in an underlying database). 320 */ 321 LOCKERROR, 322 /** 323 * The persistent store is unavailable; e.g. the database is down for 324 * maintenance or similar action. 325 */ 326 NOSTORE, 327 /** 328 * An unexpected internal error has occurred. 329 */ 330 EXCEPTION, 331 /** 332 * An internal timeout has occurred. 333 */ 334 TIMEOUT, 335 /** 336 * The system is not prepared to handle this request due to load management. 337 */ 338 THROTTLED, 339 /** 340 * A message unrelated to the processing success of the completed operation 341 * (examples of the latter include things like reminders of password expiry, 342 * system maintenance times, etc.). 343 */ 344 INFORMATIONAL, 345 /** 346 * added to help the parsers 347 */ 348 NULL; 349 350 public static IssueType fromCode(String codeString) throws FHIRException { 351 if (codeString == null || "".equals(codeString)) 352 return null; 353 if ("invalid".equals(codeString)) 354 return INVALID; 355 if ("structure".equals(codeString)) 356 return STRUCTURE; 357 if ("required".equals(codeString)) 358 return REQUIRED; 359 if ("value".equals(codeString)) 360 return VALUE; 361 if ("invariant".equals(codeString)) 362 return INVARIANT; 363 if ("security".equals(codeString)) 364 return SECURITY; 365 if ("login".equals(codeString)) 366 return LOGIN; 367 if ("unknown".equals(codeString)) 368 return UNKNOWN; 369 if ("expired".equals(codeString)) 370 return EXPIRED; 371 if ("forbidden".equals(codeString)) 372 return FORBIDDEN; 373 if ("suppressed".equals(codeString)) 374 return SUPPRESSED; 375 if ("processing".equals(codeString)) 376 return PROCESSING; 377 if ("not-supported".equals(codeString)) 378 return NOTSUPPORTED; 379 if ("duplicate".equals(codeString)) 380 return DUPLICATE; 381 if ("not-found".equals(codeString)) 382 return NOTFOUND; 383 if ("too-long".equals(codeString)) 384 return TOOLONG; 385 if ("code-invalid".equals(codeString)) 386 return CODEINVALID; 387 if ("extension".equals(codeString)) 388 return EXTENSION; 389 if ("too-costly".equals(codeString)) 390 return TOOCOSTLY; 391 if ("business-rule".equals(codeString)) 392 return BUSINESSRULE; 393 if ("conflict".equals(codeString)) 394 return CONFLICT; 395 if ("incomplete".equals(codeString)) 396 return INCOMPLETE; 397 if ("transient".equals(codeString)) 398 return TRANSIENT; 399 if ("lock-error".equals(codeString)) 400 return LOCKERROR; 401 if ("no-store".equals(codeString)) 402 return NOSTORE; 403 if ("exception".equals(codeString)) 404 return EXCEPTION; 405 if ("timeout".equals(codeString)) 406 return TIMEOUT; 407 if ("throttled".equals(codeString)) 408 return THROTTLED; 409 if ("informational".equals(codeString)) 410 return INFORMATIONAL; 411 throw new FHIRException("Unknown IssueType code '" + codeString + "'"); 412 } 413 414 public String toCode() { 415 switch (this) { 416 case INVALID: 417 return "invalid"; 418 case STRUCTURE: 419 return "structure"; 420 case REQUIRED: 421 return "required"; 422 case VALUE: 423 return "value"; 424 case INVARIANT: 425 return "invariant"; 426 case SECURITY: 427 return "security"; 428 case LOGIN: 429 return "login"; 430 case UNKNOWN: 431 return "unknown"; 432 case EXPIRED: 433 return "expired"; 434 case FORBIDDEN: 435 return "forbidden"; 436 case SUPPRESSED: 437 return "suppressed"; 438 case PROCESSING: 439 return "processing"; 440 case NOTSUPPORTED: 441 return "not-supported"; 442 case DUPLICATE: 443 return "duplicate"; 444 case NOTFOUND: 445 return "not-found"; 446 case TOOLONG: 447 return "too-long"; 448 case CODEINVALID: 449 return "code-invalid"; 450 case EXTENSION: 451 return "extension"; 452 case TOOCOSTLY: 453 return "too-costly"; 454 case BUSINESSRULE: 455 return "business-rule"; 456 case CONFLICT: 457 return "conflict"; 458 case INCOMPLETE: 459 return "incomplete"; 460 case TRANSIENT: 461 return "transient"; 462 case LOCKERROR: 463 return "lock-error"; 464 case NOSTORE: 465 return "no-store"; 466 case EXCEPTION: 467 return "exception"; 468 case TIMEOUT: 469 return "timeout"; 470 case THROTTLED: 471 return "throttled"; 472 case INFORMATIONAL: 473 return "informational"; 474 case NULL: 475 return null; 476 default: 477 return "?"; 478 } 479 } 480 481 public String getSystem() { 482 switch (this) { 483 case INVALID: 484 return "http://hl7.org/fhir/issue-type"; 485 case STRUCTURE: 486 return "http://hl7.org/fhir/issue-type"; 487 case REQUIRED: 488 return "http://hl7.org/fhir/issue-type"; 489 case VALUE: 490 return "http://hl7.org/fhir/issue-type"; 491 case INVARIANT: 492 return "http://hl7.org/fhir/issue-type"; 493 case SECURITY: 494 return "http://hl7.org/fhir/issue-type"; 495 case LOGIN: 496 return "http://hl7.org/fhir/issue-type"; 497 case UNKNOWN: 498 return "http://hl7.org/fhir/issue-type"; 499 case EXPIRED: 500 return "http://hl7.org/fhir/issue-type"; 501 case FORBIDDEN: 502 return "http://hl7.org/fhir/issue-type"; 503 case SUPPRESSED: 504 return "http://hl7.org/fhir/issue-type"; 505 case PROCESSING: 506 return "http://hl7.org/fhir/issue-type"; 507 case NOTSUPPORTED: 508 return "http://hl7.org/fhir/issue-type"; 509 case DUPLICATE: 510 return "http://hl7.org/fhir/issue-type"; 511 case NOTFOUND: 512 return "http://hl7.org/fhir/issue-type"; 513 case TOOLONG: 514 return "http://hl7.org/fhir/issue-type"; 515 case CODEINVALID: 516 return "http://hl7.org/fhir/issue-type"; 517 case EXTENSION: 518 return "http://hl7.org/fhir/issue-type"; 519 case TOOCOSTLY: 520 return "http://hl7.org/fhir/issue-type"; 521 case BUSINESSRULE: 522 return "http://hl7.org/fhir/issue-type"; 523 case CONFLICT: 524 return "http://hl7.org/fhir/issue-type"; 525 case INCOMPLETE: 526 return "http://hl7.org/fhir/issue-type"; 527 case TRANSIENT: 528 return "http://hl7.org/fhir/issue-type"; 529 case LOCKERROR: 530 return "http://hl7.org/fhir/issue-type"; 531 case NOSTORE: 532 return "http://hl7.org/fhir/issue-type"; 533 case EXCEPTION: 534 return "http://hl7.org/fhir/issue-type"; 535 case TIMEOUT: 536 return "http://hl7.org/fhir/issue-type"; 537 case THROTTLED: 538 return "http://hl7.org/fhir/issue-type"; 539 case INFORMATIONAL: 540 return "http://hl7.org/fhir/issue-type"; 541 case NULL: 542 return null; 543 default: 544 return "?"; 545 } 546 } 547 548 public String getDefinition() { 549 switch (this) { 550 case INVALID: 551 return "Content invalid against the specification or a profile."; 552 case STRUCTURE: 553 return "A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax."; 554 case REQUIRED: 555 return "A required element is missing."; 556 case VALUE: 557 return "An element value is invalid."; 558 case INVARIANT: 559 return "A content validation rule failed - e.g. a schematron rule."; 560 case SECURITY: 561 return "An authentication/authorization/permissions issue of some kind."; 562 case LOGIN: 563 return "The client needs to initiate an authentication process."; 564 case UNKNOWN: 565 return "The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable)."; 566 case EXPIRED: 567 return "User session expired; a login may be required."; 568 case FORBIDDEN: 569 return "The user does not have the rights to perform this action."; 570 case SUPPRESSED: 571 return "Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes."; 572 case PROCESSING: 573 return "Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged."; 574 case NOTSUPPORTED: 575 return "The resource or profile is not supported."; 576 case DUPLICATE: 577 return "An attempt was made to create a duplicate record."; 578 case NOTFOUND: 579 return "The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture."; 580 case TOOLONG: 581 return "Provided content is too long (typically, this is a denial of service protection type of error)."; 582 case CODEINVALID: 583 return "The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code."; 584 case EXTENSION: 585 return "An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized."; 586 case TOOCOSTLY: 587 return "The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT."; 588 case BUSINESSRULE: 589 return "The content/operation failed to pass some business rule, and so could not proceed."; 590 case CONFLICT: 591 return "Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.)"; 592 case INCOMPLETE: 593 return "Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete."; 594 case TRANSIENT: 595 return "Transient processing issues. The system receiving the error may be able to resubmit the same content once an underlying issue is resolved."; 596 case LOCKERROR: 597 return "A resource/record locking failure (usually in an underlying database)."; 598 case NOSTORE: 599 return "The persistent store is unavailable; e.g. the database is down for maintenance or similar action."; 600 case EXCEPTION: 601 return "An unexpected internal error has occurred."; 602 case TIMEOUT: 603 return "An internal timeout has occurred."; 604 case THROTTLED: 605 return "The system is not prepared to handle this request due to load management."; 606 case INFORMATIONAL: 607 return "A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.)."; 608 case NULL: 609 return null; 610 default: 611 return "?"; 612 } 613 } 614 615 public String getDisplay() { 616 switch (this) { 617 case INVALID: 618 return "Invalid Content"; 619 case STRUCTURE: 620 return "Structural Issue"; 621 case REQUIRED: 622 return "Required element missing"; 623 case VALUE: 624 return "Element value invalid"; 625 case INVARIANT: 626 return "Validation rule failed"; 627 case SECURITY: 628 return "Security Problem"; 629 case LOGIN: 630 return "Login Required"; 631 case UNKNOWN: 632 return "Unknown User"; 633 case EXPIRED: 634 return "Session Expired"; 635 case FORBIDDEN: 636 return "Forbidden"; 637 case SUPPRESSED: 638 return "Information Suppressed"; 639 case PROCESSING: 640 return "Processing Failure"; 641 case NOTSUPPORTED: 642 return "Content not supported"; 643 case DUPLICATE: 644 return "Duplicate"; 645 case NOTFOUND: 646 return "Not Found"; 647 case TOOLONG: 648 return "Content Too Long"; 649 case CODEINVALID: 650 return "Invalid Code"; 651 case EXTENSION: 652 return "Unacceptable Extension"; 653 case TOOCOSTLY: 654 return "Operation Too Costly"; 655 case BUSINESSRULE: 656 return "Business Rule Violation"; 657 case CONFLICT: 658 return "Edit Version Conflict"; 659 case INCOMPLETE: 660 return "Incomplete Results"; 661 case TRANSIENT: 662 return "Transient Issue"; 663 case LOCKERROR: 664 return "Lock Error"; 665 case NOSTORE: 666 return "No Store Available"; 667 case EXCEPTION: 668 return "Exception"; 669 case TIMEOUT: 670 return "Timeout"; 671 case THROTTLED: 672 return "Throttled"; 673 case INFORMATIONAL: 674 return "Informational Note"; 675 case NULL: 676 return null; 677 default: 678 return "?"; 679 } 680 } 681 } 682 683 public static class IssueTypeEnumFactory implements EnumFactory<IssueType> { 684 public IssueType fromCode(String codeString) throws IllegalArgumentException { 685 if (codeString == null || "".equals(codeString)) 686 if (codeString == null || "".equals(codeString)) 687 return null; 688 if ("invalid".equals(codeString)) 689 return IssueType.INVALID; 690 if ("structure".equals(codeString)) 691 return IssueType.STRUCTURE; 692 if ("required".equals(codeString)) 693 return IssueType.REQUIRED; 694 if ("value".equals(codeString)) 695 return IssueType.VALUE; 696 if ("invariant".equals(codeString)) 697 return IssueType.INVARIANT; 698 if ("security".equals(codeString)) 699 return IssueType.SECURITY; 700 if ("login".equals(codeString)) 701 return IssueType.LOGIN; 702 if ("unknown".equals(codeString)) 703 return IssueType.UNKNOWN; 704 if ("expired".equals(codeString)) 705 return IssueType.EXPIRED; 706 if ("forbidden".equals(codeString)) 707 return IssueType.FORBIDDEN; 708 if ("suppressed".equals(codeString)) 709 return IssueType.SUPPRESSED; 710 if ("processing".equals(codeString)) 711 return IssueType.PROCESSING; 712 if ("not-supported".equals(codeString)) 713 return IssueType.NOTSUPPORTED; 714 if ("duplicate".equals(codeString)) 715 return IssueType.DUPLICATE; 716 if ("not-found".equals(codeString)) 717 return IssueType.NOTFOUND; 718 if ("too-long".equals(codeString)) 719 return IssueType.TOOLONG; 720 if ("code-invalid".equals(codeString)) 721 return IssueType.CODEINVALID; 722 if ("extension".equals(codeString)) 723 return IssueType.EXTENSION; 724 if ("too-costly".equals(codeString)) 725 return IssueType.TOOCOSTLY; 726 if ("business-rule".equals(codeString)) 727 return IssueType.BUSINESSRULE; 728 if ("conflict".equals(codeString)) 729 return IssueType.CONFLICT; 730 if ("incomplete".equals(codeString)) 731 return IssueType.INCOMPLETE; 732 if ("transient".equals(codeString)) 733 return IssueType.TRANSIENT; 734 if ("lock-error".equals(codeString)) 735 return IssueType.LOCKERROR; 736 if ("no-store".equals(codeString)) 737 return IssueType.NOSTORE; 738 if ("exception".equals(codeString)) 739 return IssueType.EXCEPTION; 740 if ("timeout".equals(codeString)) 741 return IssueType.TIMEOUT; 742 if ("throttled".equals(codeString)) 743 return IssueType.THROTTLED; 744 if ("informational".equals(codeString)) 745 return IssueType.INFORMATIONAL; 746 throw new IllegalArgumentException("Unknown IssueType code '" + codeString + "'"); 747 } 748 749 public Enumeration<IssueType> fromType(Base code) throws FHIRException { 750 if (code == null || code.isEmpty()) 751 return null; 752 String codeString = ((PrimitiveType) code).asStringValue(); 753 if (codeString == null || "".equals(codeString)) 754 return null; 755 if ("invalid".equals(codeString)) 756 return new Enumeration<IssueType>(this, IssueType.INVALID); 757 if ("structure".equals(codeString)) 758 return new Enumeration<IssueType>(this, IssueType.STRUCTURE); 759 if ("required".equals(codeString)) 760 return new Enumeration<IssueType>(this, IssueType.REQUIRED); 761 if ("value".equals(codeString)) 762 return new Enumeration<IssueType>(this, IssueType.VALUE); 763 if ("invariant".equals(codeString)) 764 return new Enumeration<IssueType>(this, IssueType.INVARIANT); 765 if ("security".equals(codeString)) 766 return new Enumeration<IssueType>(this, IssueType.SECURITY); 767 if ("login".equals(codeString)) 768 return new Enumeration<IssueType>(this, IssueType.LOGIN); 769 if ("unknown".equals(codeString)) 770 return new Enumeration<IssueType>(this, IssueType.UNKNOWN); 771 if ("expired".equals(codeString)) 772 return new Enumeration<IssueType>(this, IssueType.EXPIRED); 773 if ("forbidden".equals(codeString)) 774 return new Enumeration<IssueType>(this, IssueType.FORBIDDEN); 775 if ("suppressed".equals(codeString)) 776 return new Enumeration<IssueType>(this, IssueType.SUPPRESSED); 777 if ("processing".equals(codeString)) 778 return new Enumeration<IssueType>(this, IssueType.PROCESSING); 779 if ("not-supported".equals(codeString)) 780 return new Enumeration<IssueType>(this, IssueType.NOTSUPPORTED); 781 if ("duplicate".equals(codeString)) 782 return new Enumeration<IssueType>(this, IssueType.DUPLICATE); 783 if ("not-found".equals(codeString)) 784 return new Enumeration<IssueType>(this, IssueType.NOTFOUND); 785 if ("too-long".equals(codeString)) 786 return new Enumeration<IssueType>(this, IssueType.TOOLONG); 787 if ("code-invalid".equals(codeString)) 788 return new Enumeration<IssueType>(this, IssueType.CODEINVALID); 789 if ("extension".equals(codeString)) 790 return new Enumeration<IssueType>(this, IssueType.EXTENSION); 791 if ("too-costly".equals(codeString)) 792 return new Enumeration<IssueType>(this, IssueType.TOOCOSTLY); 793 if ("business-rule".equals(codeString)) 794 return new Enumeration<IssueType>(this, IssueType.BUSINESSRULE); 795 if ("conflict".equals(codeString)) 796 return new Enumeration<IssueType>(this, IssueType.CONFLICT); 797 if ("incomplete".equals(codeString)) 798 return new Enumeration<IssueType>(this, IssueType.INCOMPLETE); 799 if ("transient".equals(codeString)) 800 return new Enumeration<IssueType>(this, IssueType.TRANSIENT); 801 if ("lock-error".equals(codeString)) 802 return new Enumeration<IssueType>(this, IssueType.LOCKERROR); 803 if ("no-store".equals(codeString)) 804 return new Enumeration<IssueType>(this, IssueType.NOSTORE); 805 if ("exception".equals(codeString)) 806 return new Enumeration<IssueType>(this, IssueType.EXCEPTION); 807 if ("timeout".equals(codeString)) 808 return new Enumeration<IssueType>(this, IssueType.TIMEOUT); 809 if ("throttled".equals(codeString)) 810 return new Enumeration<IssueType>(this, IssueType.THROTTLED); 811 if ("informational".equals(codeString)) 812 return new Enumeration<IssueType>(this, IssueType.INFORMATIONAL); 813 throw new FHIRException("Unknown IssueType code '" + codeString + "'"); 814 } 815 816 public String toCode(IssueType code) 817 { 818 if (code == IssueType.NULL) 819 return null; 820 if (code == IssueType.INVALID) 821 return "invalid"; 822 if (code == IssueType.STRUCTURE) 823 return "structure"; 824 if (code == IssueType.REQUIRED) 825 return "required"; 826 if (code == IssueType.VALUE) 827 return "value"; 828 if (code == IssueType.INVARIANT) 829 return "invariant"; 830 if (code == IssueType.SECURITY) 831 return "security"; 832 if (code == IssueType.LOGIN) 833 return "login"; 834 if (code == IssueType.UNKNOWN) 835 return "unknown"; 836 if (code == IssueType.EXPIRED) 837 return "expired"; 838 if (code == IssueType.FORBIDDEN) 839 return "forbidden"; 840 if (code == IssueType.SUPPRESSED) 841 return "suppressed"; 842 if (code == IssueType.PROCESSING) 843 return "processing"; 844 if (code == IssueType.NOTSUPPORTED) 845 return "not-supported"; 846 if (code == IssueType.DUPLICATE) 847 return "duplicate"; 848 if (code == IssueType.NOTFOUND) 849 return "not-found"; 850 if (code == IssueType.TOOLONG) 851 return "too-long"; 852 if (code == IssueType.CODEINVALID) 853 return "code-invalid"; 854 if (code == IssueType.EXTENSION) 855 return "extension"; 856 if (code == IssueType.TOOCOSTLY) 857 return "too-costly"; 858 if (code == IssueType.BUSINESSRULE) 859 return "business-rule"; 860 if (code == IssueType.CONFLICT) 861 return "conflict"; 862 if (code == IssueType.INCOMPLETE) 863 return "incomplete"; 864 if (code == IssueType.TRANSIENT) 865 return "transient"; 866 if (code == IssueType.LOCKERROR) 867 return "lock-error"; 868 if (code == IssueType.NOSTORE) 869 return "no-store"; 870 if (code == IssueType.EXCEPTION) 871 return "exception"; 872 if (code == IssueType.TIMEOUT) 873 return "timeout"; 874 if (code == IssueType.THROTTLED) 875 return "throttled"; 876 if (code == IssueType.INFORMATIONAL) 877 return "informational"; 878 return "?"; 879 } 880 } 881 882 @Block() 883 public static class OperationOutcomeIssueComponent extends BackboneElement implements IBaseBackboneElement { 884 /** 885 * Indicates whether the issue indicates a variation from successful processing. 886 */ 887 @Child(name = "severity", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 888 @Description(shortDefinition = "fatal | error | warning | information", formalDefinition = "Indicates whether the issue indicates a variation from successful processing.") 889 protected Enumeration<IssueSeverity> severity; 890 891 /** 892 * Describes the type of the issue. The system that creates an OperationOutcome 893 * SHALL choose the most applicable code from the IssueType value set, and may 894 * additional provide its own code for the error in the details element. 895 */ 896 @Child(name = "code", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 897 @Description(shortDefinition = "Error or warning code", formalDefinition = "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.") 898 protected Enumeration<IssueType> code; 899 900 /** 901 * Additional details about the error. This may be a text description of the 902 * error, or a system code that identifies the error. 903 */ 904 @Child(name = "details", type = { 905 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 906 @Description(shortDefinition = "Additional details about the error", formalDefinition = "Additional details about the error. This may be a text description of the error, or a system code that identifies the error.") 907 protected CodeableConcept details; 908 909 /** 910 * Additional diagnostic information about the issue. Typically, this may be a 911 * description of how a value is erroneous, or a stack dump to help trace the 912 * issue. 913 */ 914 @Child(name = "diagnostics", type = { 915 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 916 @Description(shortDefinition = "Additional diagnostic information about the issue", formalDefinition = "Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue.") 917 protected StringType diagnostics; 918 919 /** 920 * A simple XPath limited to element names, repetition indicators and the 921 * default child access that identifies one of the elements in the resource that 922 * caused this issue to be raised. 923 */ 924 @Child(name = "location", type = { 925 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 926 @Description(shortDefinition = "XPath of element(s) related to issue", formalDefinition = "A simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.") 927 protected List<StringType> location; 928 929 private static final long serialVersionUID = 930165515L; 930 931 /* 932 * Constructor 933 */ 934 public OperationOutcomeIssueComponent() { 935 super(); 936 } 937 938 /* 939 * Constructor 940 */ 941 public OperationOutcomeIssueComponent(Enumeration<IssueSeverity> severity, Enumeration<IssueType> code) { 942 super(); 943 this.severity = severity; 944 this.code = code; 945 } 946 947 /** 948 * @return {@link #severity} (Indicates whether the issue indicates a variation 949 * from successful processing.). This is the underlying object with id, 950 * value and extensions. The accessor "getSeverity" gives direct access 951 * to the value 952 */ 953 public Enumeration<IssueSeverity> getSeverityElement() { 954 if (this.severity == null) 955 if (Configuration.errorOnAutoCreate()) 956 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.severity"); 957 else if (Configuration.doAutoCreate()) 958 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); // bb 959 return this.severity; 960 } 961 962 public boolean hasSeverityElement() { 963 return this.severity != null && !this.severity.isEmpty(); 964 } 965 966 public boolean hasSeverity() { 967 return this.severity != null && !this.severity.isEmpty(); 968 } 969 970 /** 971 * @param value {@link #severity} (Indicates whether the issue indicates a 972 * variation from successful processing.). This is the underlying 973 * object with id, value and extensions. The accessor "getSeverity" 974 * gives direct access to the value 975 */ 976 public OperationOutcomeIssueComponent setSeverityElement(Enumeration<IssueSeverity> value) { 977 this.severity = value; 978 return this; 979 } 980 981 /** 982 * @return Indicates whether the issue indicates a variation from successful 983 * processing. 984 */ 985 public IssueSeverity getSeverity() { 986 return this.severity == null ? null : this.severity.getValue(); 987 } 988 989 /** 990 * @param value Indicates whether the issue indicates a variation from 991 * successful processing. 992 */ 993 public OperationOutcomeIssueComponent setSeverity(IssueSeverity value) { 994 if (this.severity == null) 995 this.severity = new Enumeration<IssueSeverity>(new IssueSeverityEnumFactory()); 996 this.severity.setValue(value); 997 return this; 998 } 999 1000 /** 1001 * @return {@link #code} (Describes the type of the issue. The system that 1002 * creates an OperationOutcome SHALL choose the most applicable code 1003 * from the IssueType value set, and may additional provide its own code 1004 * for the error in the details element.). This is the underlying object 1005 * with id, value and extensions. The accessor "getCode" gives direct 1006 * access to the value 1007 */ 1008 public Enumeration<IssueType> getCodeElement() { 1009 if (this.code == null) 1010 if (Configuration.errorOnAutoCreate()) 1011 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.code"); 1012 else if (Configuration.doAutoCreate()) 1013 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); // bb 1014 return this.code; 1015 } 1016 1017 public boolean hasCodeElement() { 1018 return this.code != null && !this.code.isEmpty(); 1019 } 1020 1021 public boolean hasCode() { 1022 return this.code != null && !this.code.isEmpty(); 1023 } 1024 1025 /** 1026 * @param value {@link #code} (Describes the type of the issue. The system that 1027 * creates an OperationOutcome SHALL choose the most applicable 1028 * code from the IssueType value set, and may additional provide 1029 * its own code for the error in the details element.). This is the 1030 * underlying object with id, value and extensions. The accessor 1031 * "getCode" gives direct access to the value 1032 */ 1033 public OperationOutcomeIssueComponent setCodeElement(Enumeration<IssueType> value) { 1034 this.code = value; 1035 return this; 1036 } 1037 1038 /** 1039 * @return Describes the type of the issue. The system that creates an 1040 * OperationOutcome SHALL choose the most applicable code from the 1041 * IssueType value set, and may additional provide its own code for the 1042 * error in the details element. 1043 */ 1044 public IssueType getCode() { 1045 return this.code == null ? null : this.code.getValue(); 1046 } 1047 1048 /** 1049 * @param value Describes the type of the issue. The system that creates an 1050 * OperationOutcome SHALL choose the most applicable code from the 1051 * IssueType value set, and may additional provide its own code for 1052 * the error in the details element. 1053 */ 1054 public OperationOutcomeIssueComponent setCode(IssueType value) { 1055 if (this.code == null) 1056 this.code = new Enumeration<IssueType>(new IssueTypeEnumFactory()); 1057 this.code.setValue(value); 1058 return this; 1059 } 1060 1061 /** 1062 * @return {@link #details} (Additional details about the error. This may be a 1063 * text description of the error, or a system code that identifies the 1064 * error.) 1065 */ 1066 public CodeableConcept getDetails() { 1067 if (this.details == null) 1068 if (Configuration.errorOnAutoCreate()) 1069 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.details"); 1070 else if (Configuration.doAutoCreate()) 1071 this.details = new CodeableConcept(); // cc 1072 return this.details; 1073 } 1074 1075 public boolean hasDetails() { 1076 return this.details != null && !this.details.isEmpty(); 1077 } 1078 1079 /** 1080 * @param value {@link #details} (Additional details about the error. This may 1081 * be a text description of the error, or a system code that 1082 * identifies the error.) 1083 */ 1084 public OperationOutcomeIssueComponent setDetails(CodeableConcept value) { 1085 this.details = value; 1086 return this; 1087 } 1088 1089 /** 1090 * @return {@link #diagnostics} (Additional diagnostic information about the 1091 * issue. Typically, this may be a description of how a value is 1092 * erroneous, or a stack dump to help trace the issue.). This is the 1093 * underlying object with id, value and extensions. The accessor 1094 * "getDiagnostics" gives direct access to the value 1095 */ 1096 public StringType getDiagnosticsElement() { 1097 if (this.diagnostics == null) 1098 if (Configuration.errorOnAutoCreate()) 1099 throw new Error("Attempt to auto-create OperationOutcomeIssueComponent.diagnostics"); 1100 else if (Configuration.doAutoCreate()) 1101 this.diagnostics = new StringType(); // bb 1102 return this.diagnostics; 1103 } 1104 1105 public boolean hasDiagnosticsElement() { 1106 return this.diagnostics != null && !this.diagnostics.isEmpty(); 1107 } 1108 1109 public boolean hasDiagnostics() { 1110 return this.diagnostics != null && !this.diagnostics.isEmpty(); 1111 } 1112 1113 /** 1114 * @param value {@link #diagnostics} (Additional diagnostic information about 1115 * the issue. Typically, this may be a description of how a value 1116 * is erroneous, or a stack dump to help trace the issue.). This is 1117 * the underlying object with id, value and extensions. The 1118 * accessor "getDiagnostics" gives direct access to the value 1119 */ 1120 public OperationOutcomeIssueComponent setDiagnosticsElement(StringType value) { 1121 this.diagnostics = value; 1122 return this; 1123 } 1124 1125 /** 1126 * @return Additional diagnostic information about the issue. Typically, this 1127 * may be a description of how a value is erroneous, or a stack dump to 1128 * help trace the issue. 1129 */ 1130 public String getDiagnostics() { 1131 return this.diagnostics == null ? null : this.diagnostics.getValue(); 1132 } 1133 1134 /** 1135 * @param value Additional diagnostic information about the issue. Typically, 1136 * this may be a description of how a value is erroneous, or a 1137 * stack dump to help trace the issue. 1138 */ 1139 public OperationOutcomeIssueComponent setDiagnostics(String value) { 1140 if (Utilities.noString(value)) 1141 this.diagnostics = null; 1142 else { 1143 if (this.diagnostics == null) 1144 this.diagnostics = new StringType(); 1145 this.diagnostics.setValue(value); 1146 } 1147 return this; 1148 } 1149 1150 /** 1151 * @return {@link #location} (A simple XPath limited to element names, 1152 * repetition indicators and the default child access that identifies 1153 * one of the elements in the resource that caused this issue to be 1154 * raised.) 1155 */ 1156 public List<StringType> getLocation() { 1157 if (this.location == null) 1158 this.location = new ArrayList<StringType>(); 1159 return this.location; 1160 } 1161 1162 public boolean hasLocation() { 1163 if (this.location == null) 1164 return false; 1165 for (StringType item : this.location) 1166 if (!item.isEmpty()) 1167 return true; 1168 return false; 1169 } 1170 1171 /** 1172 * @return {@link #location} (A simple XPath limited to element names, 1173 * repetition indicators and the default child access that identifies 1174 * one of the elements in the resource that caused this issue to be 1175 * raised.) 1176 */ 1177 // syntactic sugar 1178 public StringType addLocationElement() {// 2 1179 StringType t = new StringType(); 1180 if (this.location == null) 1181 this.location = new ArrayList<StringType>(); 1182 this.location.add(t); 1183 return t; 1184 } 1185 1186 /** 1187 * @param value {@link #location} (A simple XPath limited to element names, 1188 * repetition indicators and the default child access that 1189 * identifies one of the elements in the resource that caused this 1190 * issue to be raised.) 1191 */ 1192 public OperationOutcomeIssueComponent addLocation(String value) { // 1 1193 StringType t = new StringType(); 1194 t.setValue(value); 1195 if (this.location == null) 1196 this.location = new ArrayList<StringType>(); 1197 this.location.add(t); 1198 return this; 1199 } 1200 1201 /** 1202 * @param value {@link #location} (A simple XPath limited to element names, 1203 * repetition indicators and the default child access that 1204 * identifies one of the elements in the resource that caused this 1205 * issue to be raised.) 1206 */ 1207 public boolean hasLocation(String value) { 1208 if (this.location == null) 1209 return false; 1210 for (StringType v : this.location) 1211 if (v.equals(value)) // string 1212 return true; 1213 return false; 1214 } 1215 1216 protected void listChildren(List<Property> childrenList) { 1217 super.listChildren(childrenList); 1218 childrenList.add(new Property("severity", "code", 1219 "Indicates whether the issue indicates a variation from successful processing.", 0, 1220 java.lang.Integer.MAX_VALUE, severity)); 1221 childrenList.add(new Property("code", "code", 1222 "Describes the type of the issue. The system that creates an OperationOutcome SHALL choose the most applicable code from the IssueType value set, and may additional provide its own code for the error in the details element.", 1223 0, java.lang.Integer.MAX_VALUE, code)); 1224 childrenList.add(new Property("details", "CodeableConcept", 1225 "Additional details about the error. This may be a text description of the error, or a system code that identifies the error.", 1226 0, java.lang.Integer.MAX_VALUE, details)); 1227 childrenList.add(new Property("diagnostics", "string", 1228 "Additional diagnostic information about the issue. Typically, this may be a description of how a value is erroneous, or a stack dump to help trace the issue.", 1229 0, java.lang.Integer.MAX_VALUE, diagnostics)); 1230 childrenList.add(new Property("location", "string", 1231 "A simple XPath limited to element names, repetition indicators and the default child access that identifies one of the elements in the resource that caused this issue to be raised.", 1232 0, java.lang.Integer.MAX_VALUE, location)); 1233 } 1234 1235 @Override 1236 public void setProperty(String name, Base value) throws FHIRException { 1237 if (name.equals("severity")) 1238 this.severity = new IssueSeverityEnumFactory().fromType(value); // Enumeration<IssueSeverity> 1239 else if (name.equals("code")) 1240 this.code = new IssueTypeEnumFactory().fromType(value); // Enumeration<IssueType> 1241 else if (name.equals("details")) 1242 this.details = castToCodeableConcept(value); // CodeableConcept 1243 else if (name.equals("diagnostics")) 1244 this.diagnostics = castToString(value); // StringType 1245 else if (name.equals("location")) 1246 this.getLocation().add(castToString(value)); 1247 else 1248 super.setProperty(name, value); 1249 } 1250 1251 @Override 1252 public Base addChild(String name) throws FHIRException { 1253 if (name.equals("severity")) { 1254 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.severity"); 1255 } else if (name.equals("code")) { 1256 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.code"); 1257 } else if (name.equals("details")) { 1258 this.details = new CodeableConcept(); 1259 return this.details; 1260 } else if (name.equals("diagnostics")) { 1261 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.diagnostics"); 1262 } else if (name.equals("location")) { 1263 throw new FHIRException("Cannot call addChild on a singleton property OperationOutcome.location"); 1264 } else 1265 return super.addChild(name); 1266 } 1267 1268 public OperationOutcomeIssueComponent copy() { 1269 OperationOutcomeIssueComponent dst = new OperationOutcomeIssueComponent(); 1270 copyValues(dst); 1271 dst.severity = severity == null ? null : severity.copy(); 1272 dst.code = code == null ? null : code.copy(); 1273 dst.details = details == null ? null : details.copy(); 1274 dst.diagnostics = diagnostics == null ? null : diagnostics.copy(); 1275 if (location != null) { 1276 dst.location = new ArrayList<StringType>(); 1277 for (StringType i : location) 1278 dst.location.add(i.copy()); 1279 } 1280 ; 1281 return dst; 1282 } 1283 1284 @Override 1285 public boolean equalsDeep(Base other) { 1286 if (!super.equalsDeep(other)) 1287 return false; 1288 if (!(other instanceof OperationOutcomeIssueComponent)) 1289 return false; 1290 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other; 1291 return compareDeep(severity, o.severity, true) && compareDeep(code, o.code, true) 1292 && compareDeep(details, o.details, true) && compareDeep(diagnostics, o.diagnostics, true) 1293 && compareDeep(location, o.location, true); 1294 } 1295 1296 @Override 1297 public boolean equalsShallow(Base other) { 1298 if (!super.equalsShallow(other)) 1299 return false; 1300 if (!(other instanceof OperationOutcomeIssueComponent)) 1301 return false; 1302 OperationOutcomeIssueComponent o = (OperationOutcomeIssueComponent) other; 1303 return compareValues(severity, o.severity, true) && compareValues(code, o.code, true) 1304 && compareValues(diagnostics, o.diagnostics, true) && compareValues(location, o.location, true); 1305 } 1306 1307 public boolean isEmpty() { 1308 return super.isEmpty() && (severity == null || severity.isEmpty()) && (code == null || code.isEmpty()) 1309 && (details == null || details.isEmpty()) && (diagnostics == null || diagnostics.isEmpty()) 1310 && (location == null || location.isEmpty()); 1311 } 1312 1313 public String fhirType() { 1314 return "OperationOutcome.issue"; 1315 1316 } 1317 1318 } 1319 1320 /** 1321 * An error, warning or information message that results from a system action. 1322 */ 1323 @Child(name = "issue", type = {}, order = 0, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1324 @Description(shortDefinition = "A single issue associated with the action", formalDefinition = "An error, warning or information message that results from a system action.") 1325 protected List<OperationOutcomeIssueComponent> issue; 1326 1327 private static final long serialVersionUID = -152150052L; 1328 1329 /* 1330 * Constructor 1331 */ 1332 public OperationOutcome() { 1333 super(); 1334 } 1335 1336 /** 1337 * @return {@link #issue} (An error, warning or information message that results 1338 * from a system action.) 1339 */ 1340 public List<OperationOutcomeIssueComponent> getIssue() { 1341 if (this.issue == null) 1342 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1343 return this.issue; 1344 } 1345 1346 public boolean hasIssue() { 1347 if (this.issue == null) 1348 return false; 1349 for (OperationOutcomeIssueComponent item : this.issue) 1350 if (!item.isEmpty()) 1351 return true; 1352 return false; 1353 } 1354 1355 /** 1356 * @return {@link #issue} (An error, warning or information message that results 1357 * from a system action.) 1358 */ 1359 // syntactic sugar 1360 public OperationOutcomeIssueComponent addIssue() { // 3 1361 OperationOutcomeIssueComponent t = new OperationOutcomeIssueComponent(); 1362 if (this.issue == null) 1363 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1364 this.issue.add(t); 1365 return t; 1366 } 1367 1368 // syntactic sugar 1369 public OperationOutcome addIssue(OperationOutcomeIssueComponent t) { // 3 1370 if (t == null) 1371 return this; 1372 if (this.issue == null) 1373 this.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1374 this.issue.add(t); 1375 return this; 1376 } 1377 1378 protected void listChildren(List<Property> childrenList) { 1379 super.listChildren(childrenList); 1380 childrenList 1381 .add(new Property("issue", "", "An error, warning or information message that results from a system action.", 0, 1382 java.lang.Integer.MAX_VALUE, issue)); 1383 } 1384 1385 @Override 1386 public void setProperty(String name, Base value) throws FHIRException { 1387 if (name.equals("issue")) 1388 this.getIssue().add((OperationOutcomeIssueComponent) value); 1389 else 1390 super.setProperty(name, value); 1391 } 1392 1393 @Override 1394 public Base addChild(String name) throws FHIRException { 1395 if (name.equals("issue")) { 1396 return addIssue(); 1397 } else 1398 return super.addChild(name); 1399 } 1400 1401 public String fhirType() { 1402 return "OperationOutcome"; 1403 1404 } 1405 1406 public OperationOutcome copy() { 1407 OperationOutcome dst = new OperationOutcome(); 1408 copyValues(dst); 1409 if (issue != null) { 1410 dst.issue = new ArrayList<OperationOutcomeIssueComponent>(); 1411 for (OperationOutcomeIssueComponent i : issue) 1412 dst.issue.add(i.copy()); 1413 } 1414 ; 1415 return dst; 1416 } 1417 1418 protected OperationOutcome typedCopy() { 1419 return copy(); 1420 } 1421 1422 @Override 1423 public boolean equalsDeep(Base other) { 1424 if (!super.equalsDeep(other)) 1425 return false; 1426 if (!(other instanceof OperationOutcome)) 1427 return false; 1428 OperationOutcome o = (OperationOutcome) other; 1429 return compareDeep(issue, o.issue, true); 1430 } 1431 1432 @Override 1433 public boolean equalsShallow(Base other) { 1434 if (!super.equalsShallow(other)) 1435 return false; 1436 if (!(other instanceof OperationOutcome)) 1437 return false; 1438 OperationOutcome o = (OperationOutcome) other; 1439 return true; 1440 } 1441 1442 public boolean isEmpty() { 1443 return super.isEmpty() && (issue == null || issue.isEmpty()); 1444 } 1445 1446 @Override 1447 public ResourceType getResourceType() { 1448 return ResourceType.OperationOutcome; 1449 } 1450 1451}