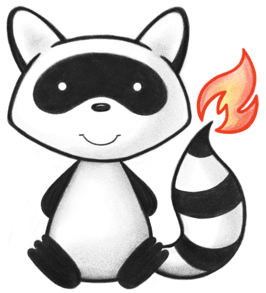
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * A request to perform an action. 047 */ 048@ResourceDef(name = "Order", profile = "http://hl7.org/fhir/Profile/Order") 049public class Order extends DomainResource { 050 051 @Block() 052 public static class OrderWhenComponent extends BackboneElement implements IBaseBackboneElement { 053 /** 054 * Code specifies when request should be done. The code may simply be a priority 055 * code. 056 */ 057 @Child(name = "code", type = { 058 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "Code specifies when request should be done. The code may simply be a priority code", formalDefinition = "Code specifies when request should be done. The code may simply be a priority code.") 060 protected CodeableConcept code; 061 062 /** 063 * A formal schedule. 064 */ 065 @Child(name = "schedule", type = { Timing.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 066 @Description(shortDefinition = "A formal schedule", formalDefinition = "A formal schedule.") 067 protected Timing schedule; 068 069 private static final long serialVersionUID = 307115287L; 070 071 /* 072 * Constructor 073 */ 074 public OrderWhenComponent() { 075 super(); 076 } 077 078 /** 079 * @return {@link #code} (Code specifies when request should be done. The code 080 * may simply be a priority code.) 081 */ 082 public CodeableConcept getCode() { 083 if (this.code == null) 084 if (Configuration.errorOnAutoCreate()) 085 throw new Error("Attempt to auto-create OrderWhenComponent.code"); 086 else if (Configuration.doAutoCreate()) 087 this.code = new CodeableConcept(); // cc 088 return this.code; 089 } 090 091 public boolean hasCode() { 092 return this.code != null && !this.code.isEmpty(); 093 } 094 095 /** 096 * @param value {@link #code} (Code specifies when request should be done. The 097 * code may simply be a priority code.) 098 */ 099 public OrderWhenComponent setCode(CodeableConcept value) { 100 this.code = value; 101 return this; 102 } 103 104 /** 105 * @return {@link #schedule} (A formal schedule.) 106 */ 107 public Timing getSchedule() { 108 if (this.schedule == null) 109 if (Configuration.errorOnAutoCreate()) 110 throw new Error("Attempt to auto-create OrderWhenComponent.schedule"); 111 else if (Configuration.doAutoCreate()) 112 this.schedule = new Timing(); // cc 113 return this.schedule; 114 } 115 116 public boolean hasSchedule() { 117 return this.schedule != null && !this.schedule.isEmpty(); 118 } 119 120 /** 121 * @param value {@link #schedule} (A formal schedule.) 122 */ 123 public OrderWhenComponent setSchedule(Timing value) { 124 this.schedule = value; 125 return this; 126 } 127 128 protected void listChildren(List<Property> childrenList) { 129 super.listChildren(childrenList); 130 childrenList.add(new Property("code", "CodeableConcept", 131 "Code specifies when request should be done. The code may simply be a priority code.", 0, 132 java.lang.Integer.MAX_VALUE, code)); 133 childrenList 134 .add(new Property("schedule", "Timing", "A formal schedule.", 0, java.lang.Integer.MAX_VALUE, schedule)); 135 } 136 137 @Override 138 public void setProperty(String name, Base value) throws FHIRException { 139 if (name.equals("code")) 140 this.code = castToCodeableConcept(value); // CodeableConcept 141 else if (name.equals("schedule")) 142 this.schedule = castToTiming(value); // Timing 143 else 144 super.setProperty(name, value); 145 } 146 147 @Override 148 public Base addChild(String name) throws FHIRException { 149 if (name.equals("code")) { 150 this.code = new CodeableConcept(); 151 return this.code; 152 } else if (name.equals("schedule")) { 153 this.schedule = new Timing(); 154 return this.schedule; 155 } else 156 return super.addChild(name); 157 } 158 159 public OrderWhenComponent copy() { 160 OrderWhenComponent dst = new OrderWhenComponent(); 161 copyValues(dst); 162 dst.code = code == null ? null : code.copy(); 163 dst.schedule = schedule == null ? null : schedule.copy(); 164 return dst; 165 } 166 167 @Override 168 public boolean equalsDeep(Base other) { 169 if (!super.equalsDeep(other)) 170 return false; 171 if (!(other instanceof OrderWhenComponent)) 172 return false; 173 OrderWhenComponent o = (OrderWhenComponent) other; 174 return compareDeep(code, o.code, true) && compareDeep(schedule, o.schedule, true); 175 } 176 177 @Override 178 public boolean equalsShallow(Base other) { 179 if (!super.equalsShallow(other)) 180 return false; 181 if (!(other instanceof OrderWhenComponent)) 182 return false; 183 OrderWhenComponent o = (OrderWhenComponent) other; 184 return true; 185 } 186 187 public boolean isEmpty() { 188 return super.isEmpty() && (code == null || code.isEmpty()) && (schedule == null || schedule.isEmpty()); 189 } 190 191 public String fhirType() { 192 return "Order.when"; 193 194 } 195 196 } 197 198 /** 199 * Identifiers assigned to this order by the orderer or by the receiver. 200 */ 201 @Child(name = "identifier", type = { 202 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 203 @Description(shortDefinition = "Identifiers assigned to this order by the orderer or by the receiver", formalDefinition = "Identifiers assigned to this order by the orderer or by the receiver.") 204 protected List<Identifier> identifier; 205 206 /** 207 * When the order was made. 208 */ 209 @Child(name = "date", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 210 @Description(shortDefinition = "When the order was made", formalDefinition = "When the order was made.") 211 protected DateTimeType date; 212 213 /** 214 * Patient this order is about. 215 */ 216 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 217 Substance.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 218 @Description(shortDefinition = "Patient this order is about", formalDefinition = "Patient this order is about.") 219 protected Reference subject; 220 221 /** 222 * The actual object that is the target of the reference (Patient this order is 223 * about.) 224 */ 225 protected Resource subjectTarget; 226 227 /** 228 * Who initiated the order. 229 */ 230 @Child(name = "source", type = { Practitioner.class, 231 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 232 @Description(shortDefinition = "Who initiated the order", formalDefinition = "Who initiated the order.") 233 protected Reference source; 234 235 /** 236 * The actual object that is the target of the reference (Who initiated the 237 * order.) 238 */ 239 protected Resource sourceTarget; 240 241 /** 242 * Who is intended to fulfill the order. 243 */ 244 @Child(name = "target", type = { Organization.class, Device.class, 245 Practitioner.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 246 @Description(shortDefinition = "Who is intended to fulfill the order", formalDefinition = "Who is intended to fulfill the order.") 247 protected Reference target; 248 249 /** 250 * The actual object that is the target of the reference (Who is intended to 251 * fulfill the order.) 252 */ 253 protected Resource targetTarget; 254 255 /** 256 * Text - why the order was made. 257 */ 258 @Child(name = "reason", type = { 259 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 260 @Description(shortDefinition = "Text - why the order was made", formalDefinition = "Text - why the order was made.") 261 protected Type reason; 262 263 /** 264 * When order should be fulfilled. 265 */ 266 @Child(name = "when", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = true) 267 @Description(shortDefinition = "When order should be fulfilled", formalDefinition = "When order should be fulfilled.") 268 protected OrderWhenComponent when; 269 270 /** 271 * What action is being ordered. 272 */ 273 @Child(name = "detail", type = {}, order = 7, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 274 @Description(shortDefinition = "What action is being ordered", formalDefinition = "What action is being ordered.") 275 protected List<Reference> detail; 276 /** 277 * The actual objects that are the target of the reference (What action is being 278 * ordered.) 279 */ 280 protected List<Resource> detailTarget; 281 282 private static final long serialVersionUID = -1392311096L; 283 284 /* 285 * Constructor 286 */ 287 public Order() { 288 super(); 289 } 290 291 /** 292 * @return {@link #identifier} (Identifiers assigned to this order by the 293 * orderer or by the receiver.) 294 */ 295 public List<Identifier> getIdentifier() { 296 if (this.identifier == null) 297 this.identifier = new ArrayList<Identifier>(); 298 return this.identifier; 299 } 300 301 public boolean hasIdentifier() { 302 if (this.identifier == null) 303 return false; 304 for (Identifier item : this.identifier) 305 if (!item.isEmpty()) 306 return true; 307 return false; 308 } 309 310 /** 311 * @return {@link #identifier} (Identifiers assigned to this order by the 312 * orderer or by the receiver.) 313 */ 314 // syntactic sugar 315 public Identifier addIdentifier() { // 3 316 Identifier t = new Identifier(); 317 if (this.identifier == null) 318 this.identifier = new ArrayList<Identifier>(); 319 this.identifier.add(t); 320 return t; 321 } 322 323 // syntactic sugar 324 public Order addIdentifier(Identifier t) { // 3 325 if (t == null) 326 return this; 327 if (this.identifier == null) 328 this.identifier = new ArrayList<Identifier>(); 329 this.identifier.add(t); 330 return this; 331 } 332 333 /** 334 * @return {@link #date} (When the order was made.). This is the underlying 335 * object with id, value and extensions. The accessor "getDate" gives 336 * direct access to the value 337 */ 338 public DateTimeType getDateElement() { 339 if (this.date == null) 340 if (Configuration.errorOnAutoCreate()) 341 throw new Error("Attempt to auto-create Order.date"); 342 else if (Configuration.doAutoCreate()) 343 this.date = new DateTimeType(); // bb 344 return this.date; 345 } 346 347 public boolean hasDateElement() { 348 return this.date != null && !this.date.isEmpty(); 349 } 350 351 public boolean hasDate() { 352 return this.date != null && !this.date.isEmpty(); 353 } 354 355 /** 356 * @param value {@link #date} (When the order was made.). This is the underlying 357 * object with id, value and extensions. The accessor "getDate" 358 * gives direct access to the value 359 */ 360 public Order setDateElement(DateTimeType value) { 361 this.date = value; 362 return this; 363 } 364 365 /** 366 * @return When the order was made. 367 */ 368 public Date getDate() { 369 return this.date == null ? null : this.date.getValue(); 370 } 371 372 /** 373 * @param value When the order was made. 374 */ 375 public Order setDate(Date value) { 376 if (value == null) 377 this.date = null; 378 else { 379 if (this.date == null) 380 this.date = new DateTimeType(); 381 this.date.setValue(value); 382 } 383 return this; 384 } 385 386 /** 387 * @return {@link #subject} (Patient this order is about.) 388 */ 389 public Reference getSubject() { 390 if (this.subject == null) 391 if (Configuration.errorOnAutoCreate()) 392 throw new Error("Attempt to auto-create Order.subject"); 393 else if (Configuration.doAutoCreate()) 394 this.subject = new Reference(); // cc 395 return this.subject; 396 } 397 398 public boolean hasSubject() { 399 return this.subject != null && !this.subject.isEmpty(); 400 } 401 402 /** 403 * @param value {@link #subject} (Patient this order is about.) 404 */ 405 public Order setSubject(Reference value) { 406 this.subject = value; 407 return this; 408 } 409 410 /** 411 * @return {@link #subject} The actual object that is the target of the 412 * reference. The reference library doesn't populate this, but you can 413 * use it to hold the resource if you resolve it. (Patient this order is 414 * about.) 415 */ 416 public Resource getSubjectTarget() { 417 return this.subjectTarget; 418 } 419 420 /** 421 * @param value {@link #subject} The actual object that is the target of the 422 * reference. The reference library doesn't use these, but you can 423 * use it to hold the resource if you resolve it. (Patient this 424 * order is about.) 425 */ 426 public Order setSubjectTarget(Resource value) { 427 this.subjectTarget = value; 428 return this; 429 } 430 431 /** 432 * @return {@link #source} (Who initiated the order.) 433 */ 434 public Reference getSource() { 435 if (this.source == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create Order.source"); 438 else if (Configuration.doAutoCreate()) 439 this.source = new Reference(); // cc 440 return this.source; 441 } 442 443 public boolean hasSource() { 444 return this.source != null && !this.source.isEmpty(); 445 } 446 447 /** 448 * @param value {@link #source} (Who initiated the order.) 449 */ 450 public Order setSource(Reference value) { 451 this.source = value; 452 return this; 453 } 454 455 /** 456 * @return {@link #source} The actual object that is the target of the 457 * reference. The reference library doesn't populate this, but you can 458 * use it to hold the resource if you resolve it. (Who initiated the 459 * order.) 460 */ 461 public Resource getSourceTarget() { 462 return this.sourceTarget; 463 } 464 465 /** 466 * @param value {@link #source} The actual object that is the target of the 467 * reference. The reference library doesn't use these, but you can 468 * use it to hold the resource if you resolve it. (Who initiated 469 * the order.) 470 */ 471 public Order setSourceTarget(Resource value) { 472 this.sourceTarget = value; 473 return this; 474 } 475 476 /** 477 * @return {@link #target} (Who is intended to fulfill the order.) 478 */ 479 public Reference getTarget() { 480 if (this.target == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create Order.target"); 483 else if (Configuration.doAutoCreate()) 484 this.target = new Reference(); // cc 485 return this.target; 486 } 487 488 public boolean hasTarget() { 489 return this.target != null && !this.target.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #target} (Who is intended to fulfill the order.) 494 */ 495 public Order setTarget(Reference value) { 496 this.target = value; 497 return this; 498 } 499 500 /** 501 * @return {@link #target} The actual object that is the target of the 502 * reference. The reference library doesn't populate this, but you can 503 * use it to hold the resource if you resolve it. (Who is intended to 504 * fulfill the order.) 505 */ 506 public Resource getTargetTarget() { 507 return this.targetTarget; 508 } 509 510 /** 511 * @param value {@link #target} The actual object that is the target of the 512 * reference. The reference library doesn't use these, but you can 513 * use it to hold the resource if you resolve it. (Who is intended 514 * to fulfill the order.) 515 */ 516 public Order setTargetTarget(Resource value) { 517 this.targetTarget = value; 518 return this; 519 } 520 521 /** 522 * @return {@link #reason} (Text - why the order was made.) 523 */ 524 public Type getReason() { 525 return this.reason; 526 } 527 528 /** 529 * @return {@link #reason} (Text - why the order was made.) 530 */ 531 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 532 if (!(this.reason instanceof CodeableConcept)) 533 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 534 + this.reason.getClass().getName() + " was encountered"); 535 return (CodeableConcept) this.reason; 536 } 537 538 public boolean hasReasonCodeableConcept() { 539 return this.reason instanceof CodeableConcept; 540 } 541 542 /** 543 * @return {@link #reason} (Text - why the order was made.) 544 */ 545 public Reference getReasonReference() throws FHIRException { 546 if (!(this.reason instanceof Reference)) 547 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.reason.getClass().getName() 548 + " was encountered"); 549 return (Reference) this.reason; 550 } 551 552 public boolean hasReasonReference() { 553 return this.reason instanceof Reference; 554 } 555 556 public boolean hasReason() { 557 return this.reason != null && !this.reason.isEmpty(); 558 } 559 560 /** 561 * @param value {@link #reason} (Text - why the order was made.) 562 */ 563 public Order setReason(Type value) { 564 this.reason = value; 565 return this; 566 } 567 568 /** 569 * @return {@link #when} (When order should be fulfilled.) 570 */ 571 public OrderWhenComponent getWhen() { 572 if (this.when == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create Order.when"); 575 else if (Configuration.doAutoCreate()) 576 this.when = new OrderWhenComponent(); // cc 577 return this.when; 578 } 579 580 public boolean hasWhen() { 581 return this.when != null && !this.when.isEmpty(); 582 } 583 584 /** 585 * @param value {@link #when} (When order should be fulfilled.) 586 */ 587 public Order setWhen(OrderWhenComponent value) { 588 this.when = value; 589 return this; 590 } 591 592 /** 593 * @return {@link #detail} (What action is being ordered.) 594 */ 595 public List<Reference> getDetail() { 596 if (this.detail == null) 597 this.detail = new ArrayList<Reference>(); 598 return this.detail; 599 } 600 601 public boolean hasDetail() { 602 if (this.detail == null) 603 return false; 604 for (Reference item : this.detail) 605 if (!item.isEmpty()) 606 return true; 607 return false; 608 } 609 610 /** 611 * @return {@link #detail} (What action is being ordered.) 612 */ 613 // syntactic sugar 614 public Reference addDetail() { // 3 615 Reference t = new Reference(); 616 if (this.detail == null) 617 this.detail = new ArrayList<Reference>(); 618 this.detail.add(t); 619 return t; 620 } 621 622 // syntactic sugar 623 public Order addDetail(Reference t) { // 3 624 if (t == null) 625 return this; 626 if (this.detail == null) 627 this.detail = new ArrayList<Reference>(); 628 this.detail.add(t); 629 return this; 630 } 631 632 /** 633 * @return {@link #detail} (The actual objects that are the target of the 634 * reference. The reference library doesn't populate this, but you can 635 * use this to hold the resources if you resolvethemt. What action is 636 * being ordered.) 637 */ 638 public List<Resource> getDetailTarget() { 639 if (this.detailTarget == null) 640 this.detailTarget = new ArrayList<Resource>(); 641 return this.detailTarget; 642 } 643 644 protected void listChildren(List<Property> childrenList) { 645 super.listChildren(childrenList); 646 childrenList.add(new Property("identifier", "Identifier", 647 "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, 648 identifier)); 649 childrenList 650 .add(new Property("date", "dateTime", "When the order was made.", 0, java.lang.Integer.MAX_VALUE, date)); 651 childrenList.add(new Property("subject", "Reference(Patient|Group|Device|Substance)", 652 "Patient this order is about.", 0, java.lang.Integer.MAX_VALUE, subject)); 653 childrenList.add(new Property("source", "Reference(Practitioner|Organization)", "Who initiated the order.", 0, 654 java.lang.Integer.MAX_VALUE, source)); 655 childrenList.add(new Property("target", "Reference(Organization|Device|Practitioner)", 656 "Who is intended to fulfill the order.", 0, java.lang.Integer.MAX_VALUE, target)); 657 childrenList.add(new Property("reason[x]", "CodeableConcept|Reference(Any)", "Text - why the order was made.", 0, 658 java.lang.Integer.MAX_VALUE, reason)); 659 childrenList.add(new Property("when", "", "When order should be fulfilled.", 0, java.lang.Integer.MAX_VALUE, when)); 660 childrenList.add(new Property("detail", "Reference(Any)", "What action is being ordered.", 0, 661 java.lang.Integer.MAX_VALUE, detail)); 662 } 663 664 @Override 665 public void setProperty(String name, Base value) throws FHIRException { 666 if (name.equals("identifier")) 667 this.getIdentifier().add(castToIdentifier(value)); 668 else if (name.equals("date")) 669 this.date = castToDateTime(value); // DateTimeType 670 else if (name.equals("subject")) 671 this.subject = castToReference(value); // Reference 672 else if (name.equals("source")) 673 this.source = castToReference(value); // Reference 674 else if (name.equals("target")) 675 this.target = castToReference(value); // Reference 676 else if (name.equals("reason[x]")) 677 this.reason = (Type) value; // Type 678 else if (name.equals("when")) 679 this.when = (OrderWhenComponent) value; // OrderWhenComponent 680 else if (name.equals("detail")) 681 this.getDetail().add(castToReference(value)); 682 else 683 super.setProperty(name, value); 684 } 685 686 @Override 687 public Base addChild(String name) throws FHIRException { 688 if (name.equals("identifier")) { 689 return addIdentifier(); 690 } else if (name.equals("date")) { 691 throw new FHIRException("Cannot call addChild on a singleton property Order.date"); 692 } else if (name.equals("subject")) { 693 this.subject = new Reference(); 694 return this.subject; 695 } else if (name.equals("source")) { 696 this.source = new Reference(); 697 return this.source; 698 } else if (name.equals("target")) { 699 this.target = new Reference(); 700 return this.target; 701 } else if (name.equals("reasonCodeableConcept")) { 702 this.reason = new CodeableConcept(); 703 return this.reason; 704 } else if (name.equals("reasonReference")) { 705 this.reason = new Reference(); 706 return this.reason; 707 } else if (name.equals("when")) { 708 this.when = new OrderWhenComponent(); 709 return this.when; 710 } else if (name.equals("detail")) { 711 return addDetail(); 712 } else 713 return super.addChild(name); 714 } 715 716 public String fhirType() { 717 return "Order"; 718 719 } 720 721 public Order copy() { 722 Order dst = new Order(); 723 copyValues(dst); 724 if (identifier != null) { 725 dst.identifier = new ArrayList<Identifier>(); 726 for (Identifier i : identifier) 727 dst.identifier.add(i.copy()); 728 } 729 ; 730 dst.date = date == null ? null : date.copy(); 731 dst.subject = subject == null ? null : subject.copy(); 732 dst.source = source == null ? null : source.copy(); 733 dst.target = target == null ? null : target.copy(); 734 dst.reason = reason == null ? null : reason.copy(); 735 dst.when = when == null ? null : when.copy(); 736 if (detail != null) { 737 dst.detail = new ArrayList<Reference>(); 738 for (Reference i : detail) 739 dst.detail.add(i.copy()); 740 } 741 ; 742 return dst; 743 } 744 745 protected Order typedCopy() { 746 return copy(); 747 } 748 749 @Override 750 public boolean equalsDeep(Base other) { 751 if (!super.equalsDeep(other)) 752 return false; 753 if (!(other instanceof Order)) 754 return false; 755 Order o = (Order) other; 756 return compareDeep(identifier, o.identifier, true) && compareDeep(date, o.date, true) 757 && compareDeep(subject, o.subject, true) && compareDeep(source, o.source, true) 758 && compareDeep(target, o.target, true) && compareDeep(reason, o.reason, true) && compareDeep(when, o.when, true) 759 && compareDeep(detail, o.detail, true); 760 } 761 762 @Override 763 public boolean equalsShallow(Base other) { 764 if (!super.equalsShallow(other)) 765 return false; 766 if (!(other instanceof Order)) 767 return false; 768 Order o = (Order) other; 769 return compareValues(date, o.date, true); 770 } 771 772 public boolean isEmpty() { 773 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (date == null || date.isEmpty()) 774 && (subject == null || subject.isEmpty()) && (source == null || source.isEmpty()) 775 && (target == null || target.isEmpty()) && (reason == null || reason.isEmpty()) 776 && (when == null || when.isEmpty()) && (detail == null || detail.isEmpty()); 777 } 778 779 @Override 780 public ResourceType getResourceType() { 781 return ResourceType.Order; 782 } 783 784 @SearchParamDefinition(name = "date", path = "Order.date", description = "When the order was made", type = "date") 785 public static final String SP_DATE = "date"; 786 @SearchParamDefinition(name = "identifier", path = "Order.identifier", description = "Instance id from source, target, and/or others", type = "token") 787 public static final String SP_IDENTIFIER = "identifier"; 788 @SearchParamDefinition(name = "subject", path = "Order.subject", description = "Patient this order is about", type = "reference") 789 public static final String SP_SUBJECT = "subject"; 790 @SearchParamDefinition(name = "patient", path = "Order.subject", description = "Patient this order is about", type = "reference") 791 public static final String SP_PATIENT = "patient"; 792 @SearchParamDefinition(name = "source", path = "Order.source", description = "Who initiated the order", type = "reference") 793 public static final String SP_SOURCE = "source"; 794 @SearchParamDefinition(name = "detail", path = "Order.detail", description = "What action is being ordered", type = "reference") 795 public static final String SP_DETAIL = "detail"; 796 @SearchParamDefinition(name = "when", path = "Order.when.schedule", description = "A formal schedule", type = "date") 797 public static final String SP_WHEN = "when"; 798 @SearchParamDefinition(name = "target", path = "Order.target", description = "Who is intended to fulfill the order", type = "reference") 799 public static final String SP_TARGET = "target"; 800 @SearchParamDefinition(name = "when_code", path = "Order.when.code", description = "Code specifies when request should be done. The code may simply be a priority code", type = "token") 801 public static final String SP_WHENCODE = "when_code"; 802 803}