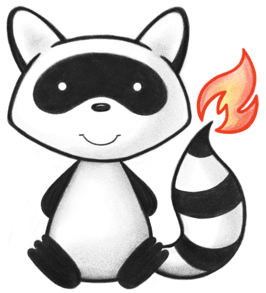
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * A response to an order. 046 */ 047@ResourceDef(name = "OrderResponse", profile = "http://hl7.org/fhir/Profile/OrderResponse") 048public class OrderResponse extends DomainResource { 049 050 public enum OrderStatus { 051 /** 052 * The order is known, but no processing has occurred at this time 053 */ 054 PENDING, 055 /** 056 * The order is undergoing initial processing to determine whether it will be 057 * accepted (usually this involves human review) 058 */ 059 REVIEW, 060 /** 061 * The order was rejected because of a workflow/business logic reason 062 */ 063 REJECTED, 064 /** 065 * The order was unable to be processed because of a technical error (i.e. 066 * unexpected error) 067 */ 068 ERROR, 069 /** 070 * The order has been accepted, and work is in progress. 071 */ 072 ACCEPTED, 073 /** 074 * Processing the order was halted at the initiators request. 075 */ 076 CANCELLED, 077 /** 078 * The order has been cancelled and replaced by another. 079 */ 080 REPLACED, 081 /** 082 * Processing the order was stopped because of some workflow/business logic 083 * reason. 084 */ 085 ABORTED, 086 /** 087 * The order has been completed. 088 */ 089 COMPLETED, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 095 public static OrderStatus fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("pending".equals(codeString)) 099 return PENDING; 100 if ("review".equals(codeString)) 101 return REVIEW; 102 if ("rejected".equals(codeString)) 103 return REJECTED; 104 if ("error".equals(codeString)) 105 return ERROR; 106 if ("accepted".equals(codeString)) 107 return ACCEPTED; 108 if ("cancelled".equals(codeString)) 109 return CANCELLED; 110 if ("replaced".equals(codeString)) 111 return REPLACED; 112 if ("aborted".equals(codeString)) 113 return ABORTED; 114 if ("completed".equals(codeString)) 115 return COMPLETED; 116 throw new FHIRException("Unknown OrderStatus code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case PENDING: 122 return "pending"; 123 case REVIEW: 124 return "review"; 125 case REJECTED: 126 return "rejected"; 127 case ERROR: 128 return "error"; 129 case ACCEPTED: 130 return "accepted"; 131 case CANCELLED: 132 return "cancelled"; 133 case REPLACED: 134 return "replaced"; 135 case ABORTED: 136 return "aborted"; 137 case COMPLETED: 138 return "completed"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getSystem() { 147 switch (this) { 148 case PENDING: 149 return "http://hl7.org/fhir/order-status"; 150 case REVIEW: 151 return "http://hl7.org/fhir/order-status"; 152 case REJECTED: 153 return "http://hl7.org/fhir/order-status"; 154 case ERROR: 155 return "http://hl7.org/fhir/order-status"; 156 case ACCEPTED: 157 return "http://hl7.org/fhir/order-status"; 158 case CANCELLED: 159 return "http://hl7.org/fhir/order-status"; 160 case REPLACED: 161 return "http://hl7.org/fhir/order-status"; 162 case ABORTED: 163 return "http://hl7.org/fhir/order-status"; 164 case COMPLETED: 165 return "http://hl7.org/fhir/order-status"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 173 public String getDefinition() { 174 switch (this) { 175 case PENDING: 176 return "The order is known, but no processing has occurred at this time"; 177 case REVIEW: 178 return "The order is undergoing initial processing to determine whether it will be accepted (usually this involves human review)"; 179 case REJECTED: 180 return "The order was rejected because of a workflow/business logic reason"; 181 case ERROR: 182 return "The order was unable to be processed because of a technical error (i.e. unexpected error)"; 183 case ACCEPTED: 184 return "The order has been accepted, and work is in progress."; 185 case CANCELLED: 186 return "Processing the order was halted at the initiators request."; 187 case REPLACED: 188 return "The order has been cancelled and replaced by another."; 189 case ABORTED: 190 return "Processing the order was stopped because of some workflow/business logic reason."; 191 case COMPLETED: 192 return "The order has been completed."; 193 case NULL: 194 return null; 195 default: 196 return "?"; 197 } 198 } 199 200 public String getDisplay() { 201 switch (this) { 202 case PENDING: 203 return "Pending"; 204 case REVIEW: 205 return "Review"; 206 case REJECTED: 207 return "Rejected"; 208 case ERROR: 209 return "Error"; 210 case ACCEPTED: 211 return "Accepted"; 212 case CANCELLED: 213 return "Cancelled"; 214 case REPLACED: 215 return "Replaced"; 216 case ABORTED: 217 return "Aborted"; 218 case COMPLETED: 219 return "Completed"; 220 case NULL: 221 return null; 222 default: 223 return "?"; 224 } 225 } 226 } 227 228 public static class OrderStatusEnumFactory implements EnumFactory<OrderStatus> { 229 public OrderStatus fromCode(String codeString) throws IllegalArgumentException { 230 if (codeString == null || "".equals(codeString)) 231 if (codeString == null || "".equals(codeString)) 232 return null; 233 if ("pending".equals(codeString)) 234 return OrderStatus.PENDING; 235 if ("review".equals(codeString)) 236 return OrderStatus.REVIEW; 237 if ("rejected".equals(codeString)) 238 return OrderStatus.REJECTED; 239 if ("error".equals(codeString)) 240 return OrderStatus.ERROR; 241 if ("accepted".equals(codeString)) 242 return OrderStatus.ACCEPTED; 243 if ("cancelled".equals(codeString)) 244 return OrderStatus.CANCELLED; 245 if ("replaced".equals(codeString)) 246 return OrderStatus.REPLACED; 247 if ("aborted".equals(codeString)) 248 return OrderStatus.ABORTED; 249 if ("completed".equals(codeString)) 250 return OrderStatus.COMPLETED; 251 throw new IllegalArgumentException("Unknown OrderStatus code '" + codeString + "'"); 252 } 253 254 public Enumeration<OrderStatus> fromType(Base code) throws FHIRException { 255 if (code == null || code.isEmpty()) 256 return null; 257 String codeString = ((PrimitiveType) code).asStringValue(); 258 if (codeString == null || "".equals(codeString)) 259 return null; 260 if ("pending".equals(codeString)) 261 return new Enumeration<OrderStatus>(this, OrderStatus.PENDING); 262 if ("review".equals(codeString)) 263 return new Enumeration<OrderStatus>(this, OrderStatus.REVIEW); 264 if ("rejected".equals(codeString)) 265 return new Enumeration<OrderStatus>(this, OrderStatus.REJECTED); 266 if ("error".equals(codeString)) 267 return new Enumeration<OrderStatus>(this, OrderStatus.ERROR); 268 if ("accepted".equals(codeString)) 269 return new Enumeration<OrderStatus>(this, OrderStatus.ACCEPTED); 270 if ("cancelled".equals(codeString)) 271 return new Enumeration<OrderStatus>(this, OrderStatus.CANCELLED); 272 if ("replaced".equals(codeString)) 273 return new Enumeration<OrderStatus>(this, OrderStatus.REPLACED); 274 if ("aborted".equals(codeString)) 275 return new Enumeration<OrderStatus>(this, OrderStatus.ABORTED); 276 if ("completed".equals(codeString)) 277 return new Enumeration<OrderStatus>(this, OrderStatus.COMPLETED); 278 throw new FHIRException("Unknown OrderStatus code '" + codeString + "'"); 279 } 280 281 public String toCode(OrderStatus code) 282 { 283 if (code == OrderStatus.NULL) 284 return null; 285 if (code == OrderStatus.PENDING) 286 return "pending"; 287 if (code == OrderStatus.REVIEW) 288 return "review"; 289 if (code == OrderStatus.REJECTED) 290 return "rejected"; 291 if (code == OrderStatus.ERROR) 292 return "error"; 293 if (code == OrderStatus.ACCEPTED) 294 return "accepted"; 295 if (code == OrderStatus.CANCELLED) 296 return "cancelled"; 297 if (code == OrderStatus.REPLACED) 298 return "replaced"; 299 if (code == OrderStatus.ABORTED) 300 return "aborted"; 301 if (code == OrderStatus.COMPLETED) 302 return "completed"; 303 return "?"; 304 } 305 } 306 307 /** 308 * Identifiers assigned to this order. The identifiers are usually assigned by 309 * the system responding to the order, but they may be provided or added to by 310 * other systems. 311 */ 312 @Child(name = "identifier", type = { 313 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 314 @Description(shortDefinition = "Identifiers assigned to this order by the orderer or by the receiver", formalDefinition = "Identifiers assigned to this order. The identifiers are usually assigned by the system responding to the order, but they may be provided or added to by other systems.") 315 protected List<Identifier> identifier; 316 317 /** 318 * A reference to the order that this is in response to. 319 */ 320 @Child(name = "request", type = { Order.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 321 @Description(shortDefinition = "The order that this is a response to", formalDefinition = "A reference to the order that this is in response to.") 322 protected Reference request; 323 324 /** 325 * The actual object that is the target of the reference (A reference to the 326 * order that this is in response to.) 327 */ 328 protected Order requestTarget; 329 330 /** 331 * The date and time at which this order response was made (created/posted). 332 */ 333 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 334 @Description(shortDefinition = "When the response was made", formalDefinition = "The date and time at which this order response was made (created/posted).") 335 protected DateTimeType date; 336 337 /** 338 * The person, organization, or device credited with making the response. 339 */ 340 @Child(name = "who", type = { Practitioner.class, Organization.class, 341 Device.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 342 @Description(shortDefinition = "Who made the response", formalDefinition = "The person, organization, or device credited with making the response.") 343 protected Reference who; 344 345 /** 346 * The actual object that is the target of the reference (The person, 347 * organization, or device credited with making the response.) 348 */ 349 protected Resource whoTarget; 350 351 /** 352 * What this response says about the status of the original order. 353 */ 354 @Child(name = "orderStatus", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 355 @Description(shortDefinition = "pending | review | rejected | error | accepted | cancelled | replaced | aborted | completed", formalDefinition = "What this response says about the status of the original order.") 356 protected Enumeration<OrderStatus> orderStatus; 357 358 /** 359 * Additional description about the response - e.g. a text description provided 360 * by a human user when making decisions about the order. 361 */ 362 @Child(name = "description", type = { 363 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 364 @Description(shortDefinition = "Additional description of the response", formalDefinition = "Additional description about the response - e.g. a text description provided by a human user when making decisions about the order.") 365 protected StringType description; 366 367 /** 368 * Links to resources that provide details of the outcome of performing the 369 * order; e.g. Diagnostic Reports in a response that is made to an order that 370 * referenced a diagnostic order. 371 */ 372 @Child(name = "fulfillment", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 373 @Description(shortDefinition = "Details of the outcome of performing the order", formalDefinition = "Links to resources that provide details of the outcome of performing the order; e.g. Diagnostic Reports in a response that is made to an order that referenced a diagnostic order.") 374 protected List<Reference> fulfillment; 375 /** 376 * The actual objects that are the target of the reference (Links to resources 377 * that provide details of the outcome of performing the order; e.g. Diagnostic 378 * Reports in a response that is made to an order that referenced a diagnostic 379 * order.) 380 */ 381 protected List<Resource> fulfillmentTarget; 382 383 private static final long serialVersionUID = -856633109L; 384 385 /* 386 * Constructor 387 */ 388 public OrderResponse() { 389 super(); 390 } 391 392 /* 393 * Constructor 394 */ 395 public OrderResponse(Reference request, Enumeration<OrderStatus> orderStatus) { 396 super(); 397 this.request = request; 398 this.orderStatus = orderStatus; 399 } 400 401 /** 402 * @return {@link #identifier} (Identifiers assigned to this order. The 403 * identifiers are usually assigned by the system responding to the 404 * order, but they may be provided or added to by other systems.) 405 */ 406 public List<Identifier> getIdentifier() { 407 if (this.identifier == null) 408 this.identifier = new ArrayList<Identifier>(); 409 return this.identifier; 410 } 411 412 public boolean hasIdentifier() { 413 if (this.identifier == null) 414 return false; 415 for (Identifier item : this.identifier) 416 if (!item.isEmpty()) 417 return true; 418 return false; 419 } 420 421 /** 422 * @return {@link #identifier} (Identifiers assigned to this order. The 423 * identifiers are usually assigned by the system responding to the 424 * order, but they may be provided or added to by other systems.) 425 */ 426 // syntactic sugar 427 public Identifier addIdentifier() { // 3 428 Identifier t = new Identifier(); 429 if (this.identifier == null) 430 this.identifier = new ArrayList<Identifier>(); 431 this.identifier.add(t); 432 return t; 433 } 434 435 // syntactic sugar 436 public OrderResponse addIdentifier(Identifier t) { // 3 437 if (t == null) 438 return this; 439 if (this.identifier == null) 440 this.identifier = new ArrayList<Identifier>(); 441 this.identifier.add(t); 442 return this; 443 } 444 445 /** 446 * @return {@link #request} (A reference to the order that this is in response 447 * to.) 448 */ 449 public Reference getRequest() { 450 if (this.request == null) 451 if (Configuration.errorOnAutoCreate()) 452 throw new Error("Attempt to auto-create OrderResponse.request"); 453 else if (Configuration.doAutoCreate()) 454 this.request = new Reference(); // cc 455 return this.request; 456 } 457 458 public boolean hasRequest() { 459 return this.request != null && !this.request.isEmpty(); 460 } 461 462 /** 463 * @param value {@link #request} (A reference to the order that this is in 464 * response to.) 465 */ 466 public OrderResponse setRequest(Reference value) { 467 this.request = value; 468 return this; 469 } 470 471 /** 472 * @return {@link #request} The actual object that is the target of the 473 * reference. The reference library doesn't populate this, but you can 474 * use it to hold the resource if you resolve it. (A reference to the 475 * order that this is in response to.) 476 */ 477 public Order getRequestTarget() { 478 if (this.requestTarget == null) 479 if (Configuration.errorOnAutoCreate()) 480 throw new Error("Attempt to auto-create OrderResponse.request"); 481 else if (Configuration.doAutoCreate()) 482 this.requestTarget = new Order(); // aa 483 return this.requestTarget; 484 } 485 486 /** 487 * @param value {@link #request} The actual object that is the target of the 488 * reference. The reference library doesn't use these, but you can 489 * use it to hold the resource if you resolve it. (A reference to 490 * the order that this is in response to.) 491 */ 492 public OrderResponse setRequestTarget(Order value) { 493 this.requestTarget = value; 494 return this; 495 } 496 497 /** 498 * @return {@link #date} (The date and time at which this order response was 499 * made (created/posted).). This is the underlying object with id, value 500 * and extensions. The accessor "getDate" gives direct access to the 501 * value 502 */ 503 public DateTimeType getDateElement() { 504 if (this.date == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create OrderResponse.date"); 507 else if (Configuration.doAutoCreate()) 508 this.date = new DateTimeType(); // bb 509 return this.date; 510 } 511 512 public boolean hasDateElement() { 513 return this.date != null && !this.date.isEmpty(); 514 } 515 516 public boolean hasDate() { 517 return this.date != null && !this.date.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #date} (The date and time at which this order response 522 * was made (created/posted).). This is the underlying object with 523 * id, value and extensions. The accessor "getDate" gives direct 524 * access to the value 525 */ 526 public OrderResponse setDateElement(DateTimeType value) { 527 this.date = value; 528 return this; 529 } 530 531 /** 532 * @return The date and time at which this order response was made 533 * (created/posted). 534 */ 535 public Date getDate() { 536 return this.date == null ? null : this.date.getValue(); 537 } 538 539 /** 540 * @param value The date and time at which this order response was made 541 * (created/posted). 542 */ 543 public OrderResponse setDate(Date value) { 544 if (value == null) 545 this.date = null; 546 else { 547 if (this.date == null) 548 this.date = new DateTimeType(); 549 this.date.setValue(value); 550 } 551 return this; 552 } 553 554 /** 555 * @return {@link #who} (The person, organization, or device credited with 556 * making the response.) 557 */ 558 public Reference getWho() { 559 if (this.who == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create OrderResponse.who"); 562 else if (Configuration.doAutoCreate()) 563 this.who = new Reference(); // cc 564 return this.who; 565 } 566 567 public boolean hasWho() { 568 return this.who != null && !this.who.isEmpty(); 569 } 570 571 /** 572 * @param value {@link #who} (The person, organization, or device credited with 573 * making the response.) 574 */ 575 public OrderResponse setWho(Reference value) { 576 this.who = value; 577 return this; 578 } 579 580 /** 581 * @return {@link #who} The actual object that is the target of the reference. 582 * The reference library doesn't populate this, but you can use it to 583 * hold the resource if you resolve it. (The person, organization, or 584 * device credited with making the response.) 585 */ 586 public Resource getWhoTarget() { 587 return this.whoTarget; 588 } 589 590 /** 591 * @param value {@link #who} The actual object that is the target of the 592 * reference. The reference library doesn't use these, but you can 593 * use it to hold the resource if you resolve it. (The person, 594 * organization, or device credited with making the response.) 595 */ 596 public OrderResponse setWhoTarget(Resource value) { 597 this.whoTarget = value; 598 return this; 599 } 600 601 /** 602 * @return {@link #orderStatus} (What this response says about the status of the 603 * original order.). This is the underlying object with id, value and 604 * extensions. The accessor "getOrderStatus" gives direct access to the 605 * value 606 */ 607 public Enumeration<OrderStatus> getOrderStatusElement() { 608 if (this.orderStatus == null) 609 if (Configuration.errorOnAutoCreate()) 610 throw new Error("Attempt to auto-create OrderResponse.orderStatus"); 611 else if (Configuration.doAutoCreate()) 612 this.orderStatus = new Enumeration<OrderStatus>(new OrderStatusEnumFactory()); // bb 613 return this.orderStatus; 614 } 615 616 public boolean hasOrderStatusElement() { 617 return this.orderStatus != null && !this.orderStatus.isEmpty(); 618 } 619 620 public boolean hasOrderStatus() { 621 return this.orderStatus != null && !this.orderStatus.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #orderStatus} (What this response says about the status 626 * of the original order.). This is the underlying object with id, 627 * value and extensions. The accessor "getOrderStatus" gives direct 628 * access to the value 629 */ 630 public OrderResponse setOrderStatusElement(Enumeration<OrderStatus> value) { 631 this.orderStatus = value; 632 return this; 633 } 634 635 /** 636 * @return What this response says about the status of the original order. 637 */ 638 public OrderStatus getOrderStatus() { 639 return this.orderStatus == null ? null : this.orderStatus.getValue(); 640 } 641 642 /** 643 * @param value What this response says about the status of the original order. 644 */ 645 public OrderResponse setOrderStatus(OrderStatus value) { 646 if (this.orderStatus == null) 647 this.orderStatus = new Enumeration<OrderStatus>(new OrderStatusEnumFactory()); 648 this.orderStatus.setValue(value); 649 return this; 650 } 651 652 /** 653 * @return {@link #description} (Additional description about the response - 654 * e.g. a text description provided by a human user when making 655 * decisions about the order.). This is the underlying object with id, 656 * value and extensions. The accessor "getDescription" gives direct 657 * access to the value 658 */ 659 public StringType getDescriptionElement() { 660 if (this.description == null) 661 if (Configuration.errorOnAutoCreate()) 662 throw new Error("Attempt to auto-create OrderResponse.description"); 663 else if (Configuration.doAutoCreate()) 664 this.description = new StringType(); // bb 665 return this.description; 666 } 667 668 public boolean hasDescriptionElement() { 669 return this.description != null && !this.description.isEmpty(); 670 } 671 672 public boolean hasDescription() { 673 return this.description != null && !this.description.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #description} (Additional description about the response 678 * - e.g. a text description provided by a human user when making 679 * decisions about the order.). This is the underlying object with 680 * id, value and extensions. The accessor "getDescription" gives 681 * direct access to the value 682 */ 683 public OrderResponse setDescriptionElement(StringType value) { 684 this.description = value; 685 return this; 686 } 687 688 /** 689 * @return Additional description about the response - e.g. a text description 690 * provided by a human user when making decisions about the order. 691 */ 692 public String getDescription() { 693 return this.description == null ? null : this.description.getValue(); 694 } 695 696 /** 697 * @param value Additional description about the response - e.g. a text 698 * description provided by a human user when making decisions about 699 * the order. 700 */ 701 public OrderResponse setDescription(String value) { 702 if (Utilities.noString(value)) 703 this.description = null; 704 else { 705 if (this.description == null) 706 this.description = new StringType(); 707 this.description.setValue(value); 708 } 709 return this; 710 } 711 712 /** 713 * @return {@link #fulfillment} (Links to resources that provide details of the 714 * outcome of performing the order; e.g. Diagnostic Reports in a 715 * response that is made to an order that referenced a diagnostic 716 * order.) 717 */ 718 public List<Reference> getFulfillment() { 719 if (this.fulfillment == null) 720 this.fulfillment = new ArrayList<Reference>(); 721 return this.fulfillment; 722 } 723 724 public boolean hasFulfillment() { 725 if (this.fulfillment == null) 726 return false; 727 for (Reference item : this.fulfillment) 728 if (!item.isEmpty()) 729 return true; 730 return false; 731 } 732 733 /** 734 * @return {@link #fulfillment} (Links to resources that provide details of the 735 * outcome of performing the order; e.g. Diagnostic Reports in a 736 * response that is made to an order that referenced a diagnostic 737 * order.) 738 */ 739 // syntactic sugar 740 public Reference addFulfillment() { // 3 741 Reference t = new Reference(); 742 if (this.fulfillment == null) 743 this.fulfillment = new ArrayList<Reference>(); 744 this.fulfillment.add(t); 745 return t; 746 } 747 748 // syntactic sugar 749 public OrderResponse addFulfillment(Reference t) { // 3 750 if (t == null) 751 return this; 752 if (this.fulfillment == null) 753 this.fulfillment = new ArrayList<Reference>(); 754 this.fulfillment.add(t); 755 return this; 756 } 757 758 /** 759 * @return {@link #fulfillment} (The actual objects that are the target of the 760 * reference. The reference library doesn't populate this, but you can 761 * use this to hold the resources if you resolvethemt. Links to 762 * resources that provide details of the outcome of performing the 763 * order; e.g. Diagnostic Reports in a response that is made to an order 764 * that referenced a diagnostic order.) 765 */ 766 public List<Resource> getFulfillmentTarget() { 767 if (this.fulfillmentTarget == null) 768 this.fulfillmentTarget = new ArrayList<Resource>(); 769 return this.fulfillmentTarget; 770 } 771 772 protected void listChildren(List<Property> childrenList) { 773 super.listChildren(childrenList); 774 childrenList.add(new Property("identifier", "Identifier", 775 "Identifiers assigned to this order. The identifiers are usually assigned by the system responding to the order, but they may be provided or added to by other systems.", 776 0, java.lang.Integer.MAX_VALUE, identifier)); 777 childrenList.add(new Property("request", "Reference(Order)", 778 "A reference to the order that this is in response to.", 0, java.lang.Integer.MAX_VALUE, request)); 779 childrenList.add( 780 new Property("date", "dateTime", "The date and time at which this order response was made (created/posted).", 0, 781 java.lang.Integer.MAX_VALUE, date)); 782 childrenList.add(new Property("who", "Reference(Practitioner|Organization|Device)", 783 "The person, organization, or device credited with making the response.", 0, java.lang.Integer.MAX_VALUE, who)); 784 childrenList 785 .add(new Property("orderStatus", "code", "What this response says about the status of the original order.", 0, 786 java.lang.Integer.MAX_VALUE, orderStatus)); 787 childrenList.add(new Property("description", "string", 788 "Additional description about the response - e.g. a text description provided by a human user when making decisions about the order.", 789 0, java.lang.Integer.MAX_VALUE, description)); 790 childrenList.add(new Property("fulfillment", "Reference(Any)", 791 "Links to resources that provide details of the outcome of performing the order; e.g. Diagnostic Reports in a response that is made to an order that referenced a diagnostic order.", 792 0, java.lang.Integer.MAX_VALUE, fulfillment)); 793 } 794 795 @Override 796 public void setProperty(String name, Base value) throws FHIRException { 797 if (name.equals("identifier")) 798 this.getIdentifier().add(castToIdentifier(value)); 799 else if (name.equals("request")) 800 this.request = castToReference(value); // Reference 801 else if (name.equals("date")) 802 this.date = castToDateTime(value); // DateTimeType 803 else if (name.equals("who")) 804 this.who = castToReference(value); // Reference 805 else if (name.equals("orderStatus")) 806 this.orderStatus = new OrderStatusEnumFactory().fromType(value); // Enumeration<OrderStatus> 807 else if (name.equals("description")) 808 this.description = castToString(value); // StringType 809 else if (name.equals("fulfillment")) 810 this.getFulfillment().add(castToReference(value)); 811 else 812 super.setProperty(name, value); 813 } 814 815 @Override 816 public Base addChild(String name) throws FHIRException { 817 if (name.equals("identifier")) { 818 return addIdentifier(); 819 } else if (name.equals("request")) { 820 this.request = new Reference(); 821 return this.request; 822 } else if (name.equals("date")) { 823 throw new FHIRException("Cannot call addChild on a singleton property OrderResponse.date"); 824 } else if (name.equals("who")) { 825 this.who = new Reference(); 826 return this.who; 827 } else if (name.equals("orderStatus")) { 828 throw new FHIRException("Cannot call addChild on a singleton property OrderResponse.orderStatus"); 829 } else if (name.equals("description")) { 830 throw new FHIRException("Cannot call addChild on a singleton property OrderResponse.description"); 831 } else if (name.equals("fulfillment")) { 832 return addFulfillment(); 833 } else 834 return super.addChild(name); 835 } 836 837 public String fhirType() { 838 return "OrderResponse"; 839 840 } 841 842 public OrderResponse copy() { 843 OrderResponse dst = new OrderResponse(); 844 copyValues(dst); 845 if (identifier != null) { 846 dst.identifier = new ArrayList<Identifier>(); 847 for (Identifier i : identifier) 848 dst.identifier.add(i.copy()); 849 } 850 ; 851 dst.request = request == null ? null : request.copy(); 852 dst.date = date == null ? null : date.copy(); 853 dst.who = who == null ? null : who.copy(); 854 dst.orderStatus = orderStatus == null ? null : orderStatus.copy(); 855 dst.description = description == null ? null : description.copy(); 856 if (fulfillment != null) { 857 dst.fulfillment = new ArrayList<Reference>(); 858 for (Reference i : fulfillment) 859 dst.fulfillment.add(i.copy()); 860 } 861 ; 862 return dst; 863 } 864 865 protected OrderResponse typedCopy() { 866 return copy(); 867 } 868 869 @Override 870 public boolean equalsDeep(Base other) { 871 if (!super.equalsDeep(other)) 872 return false; 873 if (!(other instanceof OrderResponse)) 874 return false; 875 OrderResponse o = (OrderResponse) other; 876 return compareDeep(identifier, o.identifier, true) && compareDeep(request, o.request, true) 877 && compareDeep(date, o.date, true) && compareDeep(who, o.who, true) 878 && compareDeep(orderStatus, o.orderStatus, true) && compareDeep(description, o.description, true) 879 && compareDeep(fulfillment, o.fulfillment, true); 880 } 881 882 @Override 883 public boolean equalsShallow(Base other) { 884 if (!super.equalsShallow(other)) 885 return false; 886 if (!(other instanceof OrderResponse)) 887 return false; 888 OrderResponse o = (OrderResponse) other; 889 return compareValues(date, o.date, true) && compareValues(orderStatus, o.orderStatus, true) 890 && compareValues(description, o.description, true); 891 } 892 893 public boolean isEmpty() { 894 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (request == null || request.isEmpty()) 895 && (date == null || date.isEmpty()) && (who == null || who.isEmpty()) 896 && (orderStatus == null || orderStatus.isEmpty()) && (description == null || description.isEmpty()) 897 && (fulfillment == null || fulfillment.isEmpty()); 898 } 899 900 @Override 901 public ResourceType getResourceType() { 902 return ResourceType.OrderResponse; 903 } 904 905 @SearchParamDefinition(name = "date", path = "OrderResponse.date", description = "When the response was made", type = "date") 906 public static final String SP_DATE = "date"; 907 @SearchParamDefinition(name = "request", path = "OrderResponse.request", description = "The order that this is a response to", type = "reference") 908 public static final String SP_REQUEST = "request"; 909 @SearchParamDefinition(name = "identifier", path = "OrderResponse.identifier", description = "Identifiers assigned to this order by the orderer or by the receiver", type = "token") 910 public static final String SP_IDENTIFIER = "identifier"; 911 @SearchParamDefinition(name = "code", path = "OrderResponse.orderStatus", description = "pending | review | rejected | error | accepted | cancelled | replaced | aborted | completed", type = "token") 912 public static final String SP_CODE = "code"; 913 @SearchParamDefinition(name = "fulfillment", path = "OrderResponse.fulfillment", description = "Details of the outcome of performing the order", type = "reference") 914 public static final String SP_FULFILLMENT = "fulfillment"; 915 @SearchParamDefinition(name = "who", path = "OrderResponse.who", description = "Who made the response", type = "reference") 916 public static final String SP_WHO = "who"; 917 918}