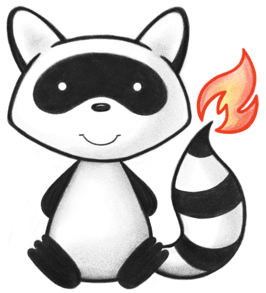
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.utilities.Utilities; 044 045/** 046 * A formally or informally recognized grouping of people or organizations 047 * formed for the purpose of achieving some form of collective action. Includes 048 * companies, institutions, corporations, departments, community groups, 049 * healthcare practice groups, etc. 050 */ 051@ResourceDef(name = "Organization", profile = "http://hl7.org/fhir/Profile/Organization") 052public class Organization extends DomainResource { 053 054 @Block() 055 public static class OrganizationContactComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * Indicates a purpose for which the contact can be reached. 058 */ 059 @Child(name = "purpose", type = { 060 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 061 @Description(shortDefinition = "The type of contact", formalDefinition = "Indicates a purpose for which the contact can be reached.") 062 protected CodeableConcept purpose; 063 064 /** 065 * A name associated with the contact. 066 */ 067 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 068 @Description(shortDefinition = "A name associated with the contact", formalDefinition = "A name associated with the contact.") 069 protected HumanName name; 070 071 /** 072 * A contact detail (e.g. a telephone number or an email address) by which the 073 * party may be contacted. 074 */ 075 @Child(name = "telecom", type = { 076 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 077 @Description(shortDefinition = "Contact details (telephone, email, etc.) for a contact", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.") 078 protected List<ContactPoint> telecom; 079 080 /** 081 * Visiting or postal addresses for the contact. 082 */ 083 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 084 @Description(shortDefinition = "Visiting or postal addresses for the contact", formalDefinition = "Visiting or postal addresses for the contact.") 085 protected Address address; 086 087 private static final long serialVersionUID = 1831121305L; 088 089 /* 090 * Constructor 091 */ 092 public OrganizationContactComponent() { 093 super(); 094 } 095 096 /** 097 * @return {@link #purpose} (Indicates a purpose for which the contact can be 098 * reached.) 099 */ 100 public CodeableConcept getPurpose() { 101 if (this.purpose == null) 102 if (Configuration.errorOnAutoCreate()) 103 throw new Error("Attempt to auto-create OrganizationContactComponent.purpose"); 104 else if (Configuration.doAutoCreate()) 105 this.purpose = new CodeableConcept(); // cc 106 return this.purpose; 107 } 108 109 public boolean hasPurpose() { 110 return this.purpose != null && !this.purpose.isEmpty(); 111 } 112 113 /** 114 * @param value {@link #purpose} (Indicates a purpose for which the contact can 115 * be reached.) 116 */ 117 public OrganizationContactComponent setPurpose(CodeableConcept value) { 118 this.purpose = value; 119 return this; 120 } 121 122 /** 123 * @return {@link #name} (A name associated with the contact.) 124 */ 125 public HumanName getName() { 126 if (this.name == null) 127 if (Configuration.errorOnAutoCreate()) 128 throw new Error("Attempt to auto-create OrganizationContactComponent.name"); 129 else if (Configuration.doAutoCreate()) 130 this.name = new HumanName(); // cc 131 return this.name; 132 } 133 134 public boolean hasName() { 135 return this.name != null && !this.name.isEmpty(); 136 } 137 138 /** 139 * @param value {@link #name} (A name associated with the contact.) 140 */ 141 public OrganizationContactComponent setName(HumanName value) { 142 this.name = value; 143 return this; 144 } 145 146 /** 147 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 148 * email address) by which the party may be contacted.) 149 */ 150 public List<ContactPoint> getTelecom() { 151 if (this.telecom == null) 152 this.telecom = new ArrayList<ContactPoint>(); 153 return this.telecom; 154 } 155 156 public boolean hasTelecom() { 157 if (this.telecom == null) 158 return false; 159 for (ContactPoint item : this.telecom) 160 if (!item.isEmpty()) 161 return true; 162 return false; 163 } 164 165 /** 166 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 167 * email address) by which the party may be contacted.) 168 */ 169 // syntactic sugar 170 public ContactPoint addTelecom() { // 3 171 ContactPoint t = new ContactPoint(); 172 if (this.telecom == null) 173 this.telecom = new ArrayList<ContactPoint>(); 174 this.telecom.add(t); 175 return t; 176 } 177 178 // syntactic sugar 179 public OrganizationContactComponent addTelecom(ContactPoint t) { // 3 180 if (t == null) 181 return this; 182 if (this.telecom == null) 183 this.telecom = new ArrayList<ContactPoint>(); 184 this.telecom.add(t); 185 return this; 186 } 187 188 /** 189 * @return {@link #address} (Visiting or postal addresses for the contact.) 190 */ 191 public Address getAddress() { 192 if (this.address == null) 193 if (Configuration.errorOnAutoCreate()) 194 throw new Error("Attempt to auto-create OrganizationContactComponent.address"); 195 else if (Configuration.doAutoCreate()) 196 this.address = new Address(); // cc 197 return this.address; 198 } 199 200 public boolean hasAddress() { 201 return this.address != null && !this.address.isEmpty(); 202 } 203 204 /** 205 * @param value {@link #address} (Visiting or postal addresses for the contact.) 206 */ 207 public OrganizationContactComponent setAddress(Address value) { 208 this.address = value; 209 return this; 210 } 211 212 protected void listChildren(List<Property> childrenList) { 213 super.listChildren(childrenList); 214 childrenList.add(new Property("purpose", "CodeableConcept", 215 "Indicates a purpose for which the contact can be reached.", 0, java.lang.Integer.MAX_VALUE, purpose)); 216 childrenList.add(new Property("name", "HumanName", "A name associated with the contact.", 0, 217 java.lang.Integer.MAX_VALUE, name)); 218 childrenList.add(new Property("telecom", "ContactPoint", 219 "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, 220 java.lang.Integer.MAX_VALUE, telecom)); 221 childrenList.add(new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 222 java.lang.Integer.MAX_VALUE, address)); 223 } 224 225 @Override 226 public void setProperty(String name, Base value) throws FHIRException { 227 if (name.equals("purpose")) 228 this.purpose = castToCodeableConcept(value); // CodeableConcept 229 else if (name.equals("name")) 230 this.name = castToHumanName(value); // HumanName 231 else if (name.equals("telecom")) 232 this.getTelecom().add(castToContactPoint(value)); 233 else if (name.equals("address")) 234 this.address = castToAddress(value); // Address 235 else 236 super.setProperty(name, value); 237 } 238 239 @Override 240 public Base addChild(String name) throws FHIRException { 241 if (name.equals("purpose")) { 242 this.purpose = new CodeableConcept(); 243 return this.purpose; 244 } else if (name.equals("name")) { 245 this.name = new HumanName(); 246 return this.name; 247 } else if (name.equals("telecom")) { 248 return addTelecom(); 249 } else if (name.equals("address")) { 250 this.address = new Address(); 251 return this.address; 252 } else 253 return super.addChild(name); 254 } 255 256 public OrganizationContactComponent copy() { 257 OrganizationContactComponent dst = new OrganizationContactComponent(); 258 copyValues(dst); 259 dst.purpose = purpose == null ? null : purpose.copy(); 260 dst.name = name == null ? null : name.copy(); 261 if (telecom != null) { 262 dst.telecom = new ArrayList<ContactPoint>(); 263 for (ContactPoint i : telecom) 264 dst.telecom.add(i.copy()); 265 } 266 ; 267 dst.address = address == null ? null : address.copy(); 268 return dst; 269 } 270 271 @Override 272 public boolean equalsDeep(Base other) { 273 if (!super.equalsDeep(other)) 274 return false; 275 if (!(other instanceof OrganizationContactComponent)) 276 return false; 277 OrganizationContactComponent o = (OrganizationContactComponent) other; 278 return compareDeep(purpose, o.purpose, true) && compareDeep(name, o.name, true) 279 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true); 280 } 281 282 @Override 283 public boolean equalsShallow(Base other) { 284 if (!super.equalsShallow(other)) 285 return false; 286 if (!(other instanceof OrganizationContactComponent)) 287 return false; 288 OrganizationContactComponent o = (OrganizationContactComponent) other; 289 return true; 290 } 291 292 public boolean isEmpty() { 293 return super.isEmpty() && (purpose == null || purpose.isEmpty()) && (name == null || name.isEmpty()) 294 && (telecom == null || telecom.isEmpty()) && (address == null || address.isEmpty()); 295 } 296 297 public String fhirType() { 298 return "Organization.contact"; 299 300 } 301 302 } 303 304 /** 305 * Identifier for the organization that is used to identify the organization 306 * across multiple disparate systems. 307 */ 308 @Child(name = "identifier", type = { 309 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 310 @Description(shortDefinition = "Identifies this organization across multiple systems", formalDefinition = "Identifier for the organization that is used to identify the organization across multiple disparate systems.") 311 protected List<Identifier> identifier; 312 313 /** 314 * Whether the organization's record is still in active use. 315 */ 316 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 317 @Description(shortDefinition = "Whether the organization's record is still in active use", formalDefinition = "Whether the organization's record is still in active use.") 318 protected BooleanType active; 319 320 /** 321 * The kind of organization that this is. 322 */ 323 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 324 @Description(shortDefinition = "Kind of organization", formalDefinition = "The kind of organization that this is.") 325 protected CodeableConcept type; 326 327 /** 328 * A name associated with the organization. 329 */ 330 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 331 @Description(shortDefinition = "Name used for the organization", formalDefinition = "A name associated with the organization.") 332 protected StringType name; 333 334 /** 335 * A contact detail for the organization. 336 */ 337 @Child(name = "telecom", type = { 338 ContactPoint.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 339 @Description(shortDefinition = "A contact detail for the organization", formalDefinition = "A contact detail for the organization.") 340 protected List<ContactPoint> telecom; 341 342 /** 343 * An address for the organization. 344 */ 345 @Child(name = "address", type = { 346 Address.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 347 @Description(shortDefinition = "An address for the organization", formalDefinition = "An address for the organization.") 348 protected List<Address> address; 349 350 /** 351 * The organization of which this organization forms a part. 352 */ 353 @Child(name = "partOf", type = { Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 354 @Description(shortDefinition = "The organization of which this organization forms a part", formalDefinition = "The organization of which this organization forms a part.") 355 protected Reference partOf; 356 357 /** 358 * The actual object that is the target of the reference (The organization of 359 * which this organization forms a part.) 360 */ 361 protected Organization partOfTarget; 362 363 /** 364 * Contact for the organization for a certain purpose. 365 */ 366 @Child(name = "contact", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 367 @Description(shortDefinition = "Contact for the organization for a certain purpose", formalDefinition = "Contact for the organization for a certain purpose.") 368 protected List<OrganizationContactComponent> contact; 369 370 private static final long serialVersionUID = -749567123L; 371 372 /* 373 * Constructor 374 */ 375 public Organization() { 376 super(); 377 } 378 379 /** 380 * @return {@link #identifier} (Identifier for the organization that is used to 381 * identify the organization across multiple disparate systems.) 382 */ 383 public List<Identifier> getIdentifier() { 384 if (this.identifier == null) 385 this.identifier = new ArrayList<Identifier>(); 386 return this.identifier; 387 } 388 389 public boolean hasIdentifier() { 390 if (this.identifier == null) 391 return false; 392 for (Identifier item : this.identifier) 393 if (!item.isEmpty()) 394 return true; 395 return false; 396 } 397 398 /** 399 * @return {@link #identifier} (Identifier for the organization that is used to 400 * identify the organization across multiple disparate systems.) 401 */ 402 // syntactic sugar 403 public Identifier addIdentifier() { // 3 404 Identifier t = new Identifier(); 405 if (this.identifier == null) 406 this.identifier = new ArrayList<Identifier>(); 407 this.identifier.add(t); 408 return t; 409 } 410 411 // syntactic sugar 412 public Organization addIdentifier(Identifier t) { // 3 413 if (t == null) 414 return this; 415 if (this.identifier == null) 416 this.identifier = new ArrayList<Identifier>(); 417 this.identifier.add(t); 418 return this; 419 } 420 421 /** 422 * @return {@link #active} (Whether the organization's record is still in active 423 * use.). This is the underlying object with id, value and extensions. 424 * The accessor "getActive" gives direct access to the value 425 */ 426 public BooleanType getActiveElement() { 427 if (this.active == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create Organization.active"); 430 else if (Configuration.doAutoCreate()) 431 this.active = new BooleanType(); // bb 432 return this.active; 433 } 434 435 public boolean hasActiveElement() { 436 return this.active != null && !this.active.isEmpty(); 437 } 438 439 public boolean hasActive() { 440 return this.active != null && !this.active.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #active} (Whether the organization's record is still in 445 * active use.). This is the underlying object with id, value and 446 * extensions. The accessor "getActive" gives direct access to the 447 * value 448 */ 449 public Organization setActiveElement(BooleanType value) { 450 this.active = value; 451 return this; 452 } 453 454 /** 455 * @return Whether the organization's record is still in active use. 456 */ 457 public boolean getActive() { 458 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 459 } 460 461 /** 462 * @param value Whether the organization's record is still in active use. 463 */ 464 public Organization setActive(boolean value) { 465 if (this.active == null) 466 this.active = new BooleanType(); 467 this.active.setValue(value); 468 return this; 469 } 470 471 /** 472 * @return {@link #type} (The kind of organization that this is.) 473 */ 474 public CodeableConcept getType() { 475 if (this.type == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create Organization.type"); 478 else if (Configuration.doAutoCreate()) 479 this.type = new CodeableConcept(); // cc 480 return this.type; 481 } 482 483 public boolean hasType() { 484 return this.type != null && !this.type.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #type} (The kind of organization that this is.) 489 */ 490 public Organization setType(CodeableConcept value) { 491 this.type = value; 492 return this; 493 } 494 495 /** 496 * @return {@link #name} (A name associated with the organization.). This is the 497 * underlying object with id, value and extensions. The accessor 498 * "getName" gives direct access to the value 499 */ 500 public StringType getNameElement() { 501 if (this.name == null) 502 if (Configuration.errorOnAutoCreate()) 503 throw new Error("Attempt to auto-create Organization.name"); 504 else if (Configuration.doAutoCreate()) 505 this.name = new StringType(); // bb 506 return this.name; 507 } 508 509 public boolean hasNameElement() { 510 return this.name != null && !this.name.isEmpty(); 511 } 512 513 public boolean hasName() { 514 return this.name != null && !this.name.isEmpty(); 515 } 516 517 /** 518 * @param value {@link #name} (A name associated with the organization.). This 519 * is the underlying object with id, value and extensions. The 520 * accessor "getName" gives direct access to the value 521 */ 522 public Organization setNameElement(StringType value) { 523 this.name = value; 524 return this; 525 } 526 527 /** 528 * @return A name associated with the organization. 529 */ 530 public String getName() { 531 return this.name == null ? null : this.name.getValue(); 532 } 533 534 /** 535 * @param value A name associated with the organization. 536 */ 537 public Organization setName(String value) { 538 if (Utilities.noString(value)) 539 this.name = null; 540 else { 541 if (this.name == null) 542 this.name = new StringType(); 543 this.name.setValue(value); 544 } 545 return this; 546 } 547 548 /** 549 * @return {@link #telecom} (A contact detail for the organization.) 550 */ 551 public List<ContactPoint> getTelecom() { 552 if (this.telecom == null) 553 this.telecom = new ArrayList<ContactPoint>(); 554 return this.telecom; 555 } 556 557 public boolean hasTelecom() { 558 if (this.telecom == null) 559 return false; 560 for (ContactPoint item : this.telecom) 561 if (!item.isEmpty()) 562 return true; 563 return false; 564 } 565 566 /** 567 * @return {@link #telecom} (A contact detail for the organization.) 568 */ 569 // syntactic sugar 570 public ContactPoint addTelecom() { // 3 571 ContactPoint t = new ContactPoint(); 572 if (this.telecom == null) 573 this.telecom = new ArrayList<ContactPoint>(); 574 this.telecom.add(t); 575 return t; 576 } 577 578 // syntactic sugar 579 public Organization addTelecom(ContactPoint t) { // 3 580 if (t == null) 581 return this; 582 if (this.telecom == null) 583 this.telecom = new ArrayList<ContactPoint>(); 584 this.telecom.add(t); 585 return this; 586 } 587 588 /** 589 * @return {@link #address} (An address for the organization.) 590 */ 591 public List<Address> getAddress() { 592 if (this.address == null) 593 this.address = new ArrayList<Address>(); 594 return this.address; 595 } 596 597 public boolean hasAddress() { 598 if (this.address == null) 599 return false; 600 for (Address item : this.address) 601 if (!item.isEmpty()) 602 return true; 603 return false; 604 } 605 606 /** 607 * @return {@link #address} (An address for the organization.) 608 */ 609 // syntactic sugar 610 public Address addAddress() { // 3 611 Address t = new Address(); 612 if (this.address == null) 613 this.address = new ArrayList<Address>(); 614 this.address.add(t); 615 return t; 616 } 617 618 // syntactic sugar 619 public Organization addAddress(Address t) { // 3 620 if (t == null) 621 return this; 622 if (this.address == null) 623 this.address = new ArrayList<Address>(); 624 this.address.add(t); 625 return this; 626 } 627 628 /** 629 * @return {@link #partOf} (The organization of which this organization forms a 630 * part.) 631 */ 632 public Reference getPartOf() { 633 if (this.partOf == null) 634 if (Configuration.errorOnAutoCreate()) 635 throw new Error("Attempt to auto-create Organization.partOf"); 636 else if (Configuration.doAutoCreate()) 637 this.partOf = new Reference(); // cc 638 return this.partOf; 639 } 640 641 public boolean hasPartOf() { 642 return this.partOf != null && !this.partOf.isEmpty(); 643 } 644 645 /** 646 * @param value {@link #partOf} (The organization of which this organization 647 * forms a part.) 648 */ 649 public Organization setPartOf(Reference value) { 650 this.partOf = value; 651 return this; 652 } 653 654 /** 655 * @return {@link #partOf} The actual object that is the target of the 656 * reference. The reference library doesn't populate this, but you can 657 * use it to hold the resource if you resolve it. (The organization of 658 * which this organization forms a part.) 659 */ 660 public Organization getPartOfTarget() { 661 if (this.partOfTarget == null) 662 if (Configuration.errorOnAutoCreate()) 663 throw new Error("Attempt to auto-create Organization.partOf"); 664 else if (Configuration.doAutoCreate()) 665 this.partOfTarget = new Organization(); // aa 666 return this.partOfTarget; 667 } 668 669 /** 670 * @param value {@link #partOf} The actual object that is the target of the 671 * reference. The reference library doesn't use these, but you can 672 * use it to hold the resource if you resolve it. (The organization 673 * of which this organization forms a part.) 674 */ 675 public Organization setPartOfTarget(Organization value) { 676 this.partOfTarget = value; 677 return this; 678 } 679 680 /** 681 * @return {@link #contact} (Contact for the organization for a certain 682 * purpose.) 683 */ 684 public List<OrganizationContactComponent> getContact() { 685 if (this.contact == null) 686 this.contact = new ArrayList<OrganizationContactComponent>(); 687 return this.contact; 688 } 689 690 public boolean hasContact() { 691 if (this.contact == null) 692 return false; 693 for (OrganizationContactComponent item : this.contact) 694 if (!item.isEmpty()) 695 return true; 696 return false; 697 } 698 699 /** 700 * @return {@link #contact} (Contact for the organization for a certain 701 * purpose.) 702 */ 703 // syntactic sugar 704 public OrganizationContactComponent addContact() { // 3 705 OrganizationContactComponent t = new OrganizationContactComponent(); 706 if (this.contact == null) 707 this.contact = new ArrayList<OrganizationContactComponent>(); 708 this.contact.add(t); 709 return t; 710 } 711 712 // syntactic sugar 713 public Organization addContact(OrganizationContactComponent t) { // 3 714 if (t == null) 715 return this; 716 if (this.contact == null) 717 this.contact = new ArrayList<OrganizationContactComponent>(); 718 this.contact.add(t); 719 return this; 720 } 721 722 protected void listChildren(List<Property> childrenList) { 723 super.listChildren(childrenList); 724 childrenList.add(new Property("identifier", "Identifier", 725 "Identifier for the organization that is used to identify the organization across multiple disparate systems.", 726 0, java.lang.Integer.MAX_VALUE, identifier)); 727 childrenList.add(new Property("active", "boolean", "Whether the organization's record is still in active use.", 0, 728 java.lang.Integer.MAX_VALUE, active)); 729 childrenList.add(new Property("type", "CodeableConcept", "The kind of organization that this is.", 0, 730 java.lang.Integer.MAX_VALUE, type)); 731 childrenList.add(new Property("name", "string", "A name associated with the organization.", 0, 732 java.lang.Integer.MAX_VALUE, name)); 733 childrenList.add(new Property("telecom", "ContactPoint", "A contact detail for the organization.", 0, 734 java.lang.Integer.MAX_VALUE, telecom)); 735 childrenList.add(new Property("address", "Address", "An address for the organization.", 0, 736 java.lang.Integer.MAX_VALUE, address)); 737 childrenList.add(new Property("partOf", "Reference(Organization)", 738 "The organization of which this organization forms a part.", 0, java.lang.Integer.MAX_VALUE, partOf)); 739 childrenList.add(new Property("contact", "", "Contact for the organization for a certain purpose.", 0, 740 java.lang.Integer.MAX_VALUE, contact)); 741 } 742 743 @Override 744 public void setProperty(String name, Base value) throws FHIRException { 745 if (name.equals("identifier")) 746 this.getIdentifier().add(castToIdentifier(value)); 747 else if (name.equals("active")) 748 this.active = castToBoolean(value); // BooleanType 749 else if (name.equals("type")) 750 this.type = castToCodeableConcept(value); // CodeableConcept 751 else if (name.equals("name")) 752 this.name = castToString(value); // StringType 753 else if (name.equals("telecom")) 754 this.getTelecom().add(castToContactPoint(value)); 755 else if (name.equals("address")) 756 this.getAddress().add(castToAddress(value)); 757 else if (name.equals("partOf")) 758 this.partOf = castToReference(value); // Reference 759 else if (name.equals("contact")) 760 this.getContact().add((OrganizationContactComponent) value); 761 else 762 super.setProperty(name, value); 763 } 764 765 @Override 766 public Base addChild(String name) throws FHIRException { 767 if (name.equals("identifier")) { 768 return addIdentifier(); 769 } else if (name.equals("active")) { 770 throw new FHIRException("Cannot call addChild on a singleton property Organization.active"); 771 } else if (name.equals("type")) { 772 this.type = new CodeableConcept(); 773 return this.type; 774 } else if (name.equals("name")) { 775 throw new FHIRException("Cannot call addChild on a singleton property Organization.name"); 776 } else if (name.equals("telecom")) { 777 return addTelecom(); 778 } else if (name.equals("address")) { 779 return addAddress(); 780 } else if (name.equals("partOf")) { 781 this.partOf = new Reference(); 782 return this.partOf; 783 } else if (name.equals("contact")) { 784 return addContact(); 785 } else 786 return super.addChild(name); 787 } 788 789 public String fhirType() { 790 return "Organization"; 791 792 } 793 794 public Organization copy() { 795 Organization dst = new Organization(); 796 copyValues(dst); 797 if (identifier != null) { 798 dst.identifier = new ArrayList<Identifier>(); 799 for (Identifier i : identifier) 800 dst.identifier.add(i.copy()); 801 } 802 ; 803 dst.active = active == null ? null : active.copy(); 804 dst.type = type == null ? null : type.copy(); 805 dst.name = name == null ? null : name.copy(); 806 if (telecom != null) { 807 dst.telecom = new ArrayList<ContactPoint>(); 808 for (ContactPoint i : telecom) 809 dst.telecom.add(i.copy()); 810 } 811 ; 812 if (address != null) { 813 dst.address = new ArrayList<Address>(); 814 for (Address i : address) 815 dst.address.add(i.copy()); 816 } 817 ; 818 dst.partOf = partOf == null ? null : partOf.copy(); 819 if (contact != null) { 820 dst.contact = new ArrayList<OrganizationContactComponent>(); 821 for (OrganizationContactComponent i : contact) 822 dst.contact.add(i.copy()); 823 } 824 ; 825 return dst; 826 } 827 828 protected Organization typedCopy() { 829 return copy(); 830 } 831 832 @Override 833 public boolean equalsDeep(Base other) { 834 if (!super.equalsDeep(other)) 835 return false; 836 if (!(other instanceof Organization)) 837 return false; 838 Organization o = (Organization) other; 839 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 840 && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 841 && compareDeep(address, o.address, true) && compareDeep(partOf, o.partOf, true) 842 && compareDeep(contact, o.contact, true); 843 } 844 845 @Override 846 public boolean equalsShallow(Base other) { 847 if (!super.equalsShallow(other)) 848 return false; 849 if (!(other instanceof Organization)) 850 return false; 851 Organization o = (Organization) other; 852 return compareValues(active, o.active, true) && compareValues(name, o.name, true); 853 } 854 855 public boolean isEmpty() { 856 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (active == null || active.isEmpty()) 857 && (type == null || type.isEmpty()) && (name == null || name.isEmpty()) 858 && (telecom == null || telecom.isEmpty()) && (address == null || address.isEmpty()) 859 && (partOf == null || partOf.isEmpty()) && (contact == null || contact.isEmpty()); 860 } 861 862 @Override 863 public ResourceType getResourceType() { 864 return ResourceType.Organization; 865 } 866 867 @SearchParamDefinition(name = "identifier", path = "Organization.identifier", description = "Any identifier for the organization (not the accreditation issuer's identifier)", type = "token") 868 public static final String SP_IDENTIFIER = "identifier"; 869 @SearchParamDefinition(name = "partof", path = "Organization.partOf", description = "Search all organizations that are part of the given organization", type = "reference") 870 public static final String SP_PARTOF = "partof"; 871 @SearchParamDefinition(name = "phonetic", path = "Organization.name", description = "A portion of the organization's name using some kind of phonetic matching algorithm", type = "string") 872 public static final String SP_PHONETIC = "phonetic"; 873 @SearchParamDefinition(name = "address", path = "Organization.address", description = "A (part of the) address of the Organization", type = "string") 874 public static final String SP_ADDRESS = "address"; 875 @SearchParamDefinition(name = "address-state", path = "Organization.address.state", description = "A state specified in an address", type = "string") 876 public static final String SP_ADDRESSSTATE = "address-state"; 877 @SearchParamDefinition(name = "name", path = "Organization.name", description = "A portion of the organization's name", type = "string") 878 public static final String SP_NAME = "name"; 879 @SearchParamDefinition(name = "address-use", path = "Organization.address.use", description = "A use code specified in an address", type = "token") 880 public static final String SP_ADDRESSUSE = "address-use"; 881 @SearchParamDefinition(name = "active", path = "Organization.active", description = "Whether the organization's record is active", type = "token") 882 public static final String SP_ACTIVE = "active"; 883 @SearchParamDefinition(name = "type", path = "Organization.type", description = "A code for the type of organization", type = "token") 884 public static final String SP_TYPE = "type"; 885 @SearchParamDefinition(name = "address-city", path = "Organization.address.city", description = "A city specified in an address", type = "string") 886 public static final String SP_ADDRESSCITY = "address-city"; 887 @SearchParamDefinition(name = "address-postalcode", path = "Organization.address.postalCode", description = "A postal code specified in an address", type = "string") 888 public static final String SP_ADDRESSPOSTALCODE = "address-postalcode"; 889 @SearchParamDefinition(name = "address-country", path = "Organization.address.country", description = "A country specified in an address", type = "string") 890 public static final String SP_ADDRESSCOUNTRY = "address-country"; 891 892}