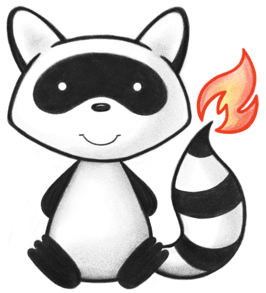
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.instance.model.api.IBaseParameters; 042import org.hl7.fhir.exceptions.FHIRException; 043 044/** 045 * This special resource type is used to represent an operation request and 046 * response (operations.html). It has no other use, and there is no RESTful 047 * endpoint associated with it. 048 */ 049@ResourceDef(name = "Parameters", profile = "http://hl7.org/fhir/Profile/Parameters") 050public class Parameters extends Resource implements IBaseParameters { 051 052 @Block() 053 public static class ParametersParameterComponent extends BackboneElement implements IBaseBackboneElement { 054 /** 055 * The name of the parameter (reference to the operation definition). 056 */ 057 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 058 @Description(shortDefinition = "Name from the definition", formalDefinition = "The name of the parameter (reference to the operation definition).") 059 protected StringType name; 060 061 /** 062 * If the parameter is a data type. 063 */ 064 @Child(name = "value", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = false) 065 @Description(shortDefinition = "If parameter is a data type", formalDefinition = "If the parameter is a data type.") 066 protected org.hl7.fhir.dstu2.model.Type value; 067 068 /** 069 * If the parameter is a whole resource. 070 */ 071 @Child(name = "resource", type = { Resource.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 072 @Description(shortDefinition = "If parameter is a whole resource", formalDefinition = "If the parameter is a whole resource.") 073 protected Resource resource; 074 075 /** 076 * A named part of a parameter. In many implementation context, a set of named 077 * parts is known as a "Tuple". 078 */ 079 @Child(name = "part", type = { 080 ParametersParameterComponent.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 081 @Description(shortDefinition = "Named part of a parameter (e.g. Tuple)", formalDefinition = "A named part of a parameter. In many implementation context, a set of named parts is known as a \"Tuple\".") 082 protected List<ParametersParameterComponent> part; 083 084 private static final long serialVersionUID = -198518915L; 085 086 /* 087 * Constructor 088 */ 089 public ParametersParameterComponent() { 090 super(); 091 } 092 093 /* 094 * Constructor 095 */ 096 public ParametersParameterComponent(StringType name) { 097 super(); 098 this.name = name; 099 } 100 101 /** 102 * @return {@link #name} (The name of the parameter (reference to the operation 103 * definition).). This is the underlying object with id, value and 104 * extensions. The accessor "getName" gives direct access to the value 105 */ 106 public StringType getNameElement() { 107 if (this.name == null) 108 if (Configuration.errorOnAutoCreate()) 109 throw new Error("Attempt to auto-create ParametersParameterComponent.name"); 110 else if (Configuration.doAutoCreate()) 111 this.name = new StringType(); // bb 112 return this.name; 113 } 114 115 public boolean hasNameElement() { 116 return this.name != null && !this.name.isEmpty(); 117 } 118 119 public boolean hasName() { 120 return this.name != null && !this.name.isEmpty(); 121 } 122 123 /** 124 * @param value {@link #name} (The name of the parameter (reference to the 125 * operation definition).). This is the underlying object with id, 126 * value and extensions. The accessor "getName" gives direct access 127 * to the value 128 */ 129 public ParametersParameterComponent setNameElement(StringType value) { 130 this.name = value; 131 return this; 132 } 133 134 /** 135 * @return The name of the parameter (reference to the operation definition). 136 */ 137 public String getName() { 138 return this.name == null ? null : this.name.getValue(); 139 } 140 141 /** 142 * @param value The name of the parameter (reference to the operation 143 * definition). 144 */ 145 public ParametersParameterComponent setName(String value) { 146 if (this.name == null) 147 this.name = new StringType(); 148 this.name.setValue(value); 149 return this; 150 } 151 152 /** 153 * @return {@link #value} (If the parameter is a data type.) 154 */ 155 public org.hl7.fhir.dstu2.model.Type getValue() { 156 return this.value; 157 } 158 159 public boolean hasValue() { 160 return this.value != null && !this.value.isEmpty(); 161 } 162 163 /** 164 * @param value {@link #value} (If the parameter is a data type.) 165 */ 166 public ParametersParameterComponent setValue(org.hl7.fhir.dstu2.model.Type value) { 167 this.value = value; 168 return this; 169 } 170 171 /** 172 * @return {@link #resource} (If the parameter is a whole resource.) 173 */ 174 public Resource getResource() { 175 return this.resource; 176 } 177 178 public boolean hasResource() { 179 return this.resource != null && !this.resource.isEmpty(); 180 } 181 182 /** 183 * @param value {@link #resource} (If the parameter is a whole resource.) 184 */ 185 public ParametersParameterComponent setResource(Resource value) { 186 this.resource = value; 187 return this; 188 } 189 190 /** 191 * @return {@link #part} (A named part of a parameter. In many implementation 192 * context, a set of named parts is known as a "Tuple".) 193 */ 194 public List<ParametersParameterComponent> getPart() { 195 if (this.part == null) 196 this.part = new ArrayList<ParametersParameterComponent>(); 197 return this.part; 198 } 199 200 public boolean hasPart() { 201 if (this.part == null) 202 return false; 203 for (ParametersParameterComponent item : this.part) 204 if (!item.isEmpty()) 205 return true; 206 return false; 207 } 208 209 /** 210 * @return {@link #part} (A named part of a parameter. In many implementation 211 * context, a set of named parts is known as a "Tuple".) 212 */ 213 // syntactic sugar 214 public ParametersParameterComponent addPart() { // 3 215 ParametersParameterComponent t = new ParametersParameterComponent(); 216 if (this.part == null) 217 this.part = new ArrayList<ParametersParameterComponent>(); 218 this.part.add(t); 219 return t; 220 } 221 222 // syntactic sugar 223 public ParametersParameterComponent addPart(ParametersParameterComponent t) { // 3 224 if (t == null) 225 return this; 226 if (this.part == null) 227 this.part = new ArrayList<ParametersParameterComponent>(); 228 this.part.add(t); 229 return this; 230 } 231 232 protected void listChildren(List<Property> childrenList) { 233 super.listChildren(childrenList); 234 childrenList.add(new Property("name", "string", 235 "The name of the parameter (reference to the operation definition).", 0, java.lang.Integer.MAX_VALUE, name)); 236 childrenList.add( 237 new Property("value[x]", "*", "If the parameter is a data type.", 0, java.lang.Integer.MAX_VALUE, value)); 238 childrenList.add(new Property("resource", "Resource", "If the parameter is a whole resource.", 0, 239 java.lang.Integer.MAX_VALUE, resource)); 240 childrenList.add(new Property("part", "@Parameters.parameter", 241 "A named part of a parameter. In many implementation context, a set of named parts is known as a \"Tuple\".", 242 0, java.lang.Integer.MAX_VALUE, part)); 243 } 244 245 @Override 246 public void setProperty(String name, Base value) throws FHIRException { 247 if (name.equals("name")) 248 this.name = castToString(value); // StringType 249 else if (name.equals("value[x]")) 250 this.value = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 251 else if (name.equals("resource")) 252 this.resource = castToResource(value); // Resource 253 else if (name.equals("part")) 254 this.getPart().add((ParametersParameterComponent) value); 255 else 256 super.setProperty(name, value); 257 } 258 259 @Override 260 public Base addChild(String name) throws FHIRException { 261 if (name.equals("name")) { 262 throw new FHIRException("Cannot call addChild on a singleton property Parameters.name"); 263 } else if (name.equals("valueBoolean")) { 264 this.value = new BooleanType(); 265 return this.value; 266 } else if (name.equals("valueInteger")) { 267 this.value = new IntegerType(); 268 return this.value; 269 } else if (name.equals("valueDecimal")) { 270 this.value = new DecimalType(); 271 return this.value; 272 } else if (name.equals("valueBase64Binary")) { 273 this.value = new Base64BinaryType(); 274 return this.value; 275 } else if (name.equals("valueInstant")) { 276 this.value = new InstantType(); 277 return this.value; 278 } else if (name.equals("valueString")) { 279 this.value = new StringType(); 280 return this.value; 281 } else if (name.equals("valueUri")) { 282 this.value = new UriType(); 283 return this.value; 284 } else if (name.equals("valueDate")) { 285 this.value = new DateType(); 286 return this.value; 287 } else if (name.equals("valueDateTime")) { 288 this.value = new DateTimeType(); 289 return this.value; 290 } else if (name.equals("valueTime")) { 291 this.value = new TimeType(); 292 return this.value; 293 } else if (name.equals("valueCode")) { 294 this.value = new CodeType(); 295 return this.value; 296 } else if (name.equals("valueOid")) { 297 this.value = new OidType(); 298 return this.value; 299 } else if (name.equals("valueId")) { 300 this.value = new IdType(); 301 return this.value; 302 } else if (name.equals("valueUnsignedInt")) { 303 this.value = new UnsignedIntType(); 304 return this.value; 305 } else if (name.equals("valuePositiveInt")) { 306 this.value = new PositiveIntType(); 307 return this.value; 308 } else if (name.equals("valueMarkdown")) { 309 this.value = new MarkdownType(); 310 return this.value; 311 } else if (name.equals("valueAnnotation")) { 312 this.value = new Annotation(); 313 return this.value; 314 } else if (name.equals("valueAttachment")) { 315 this.value = new Attachment(); 316 return this.value; 317 } else if (name.equals("valueIdentifier")) { 318 this.value = new Identifier(); 319 return this.value; 320 } else if (name.equals("valueCodeableConcept")) { 321 this.value = new CodeableConcept(); 322 return this.value; 323 } else if (name.equals("valueCoding")) { 324 this.value = new Coding(); 325 return this.value; 326 } else if (name.equals("valueQuantity")) { 327 this.value = new Quantity(); 328 return this.value; 329 } else if (name.equals("valueRange")) { 330 this.value = new Range(); 331 return this.value; 332 } else if (name.equals("valuePeriod")) { 333 this.value = new Period(); 334 return this.value; 335 } else if (name.equals("valueRatio")) { 336 this.value = new Ratio(); 337 return this.value; 338 } else if (name.equals("valueSampledData")) { 339 this.value = new SampledData(); 340 return this.value; 341 } else if (name.equals("valueSignature")) { 342 this.value = new Signature(); 343 return this.value; 344 } else if (name.equals("valueHumanName")) { 345 this.value = new HumanName(); 346 return this.value; 347 } else if (name.equals("valueAddress")) { 348 this.value = new Address(); 349 return this.value; 350 } else if (name.equals("valueContactPoint")) { 351 this.value = new ContactPoint(); 352 return this.value; 353 } else if (name.equals("valueTiming")) { 354 this.value = new Timing(); 355 return this.value; 356 } else if (name.equals("valueReference")) { 357 this.value = new Reference(); 358 return this.value; 359 } else if (name.equals("valueMeta")) { 360 this.value = new Meta(); 361 return this.value; 362 } else if (name.equals("resource")) { 363 throw new FHIRException("Cannot call addChild on an abstract type Parameters.resource"); 364 } else if (name.equals("part")) { 365 return addPart(); 366 } else 367 return super.addChild(name); 368 } 369 370 public ParametersParameterComponent copy() { 371 ParametersParameterComponent dst = new ParametersParameterComponent(); 372 copyValues(dst); 373 dst.name = name == null ? null : name.copy(); 374 dst.value = value == null ? null : value.copy(); 375 dst.resource = resource == null ? null : resource.copy(); 376 if (part != null) { 377 dst.part = new ArrayList<ParametersParameterComponent>(); 378 for (ParametersParameterComponent i : part) 379 dst.part.add(i.copy()); 380 } 381 ; 382 return dst; 383 } 384 385 @Override 386 public boolean equalsDeep(Base other) { 387 if (!super.equalsDeep(other)) 388 return false; 389 if (!(other instanceof ParametersParameterComponent)) 390 return false; 391 ParametersParameterComponent o = (ParametersParameterComponent) other; 392 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true) 393 && compareDeep(resource, o.resource, true) && compareDeep(part, o.part, true); 394 } 395 396 @Override 397 public boolean equalsShallow(Base other) { 398 if (!super.equalsShallow(other)) 399 return false; 400 if (!(other instanceof ParametersParameterComponent)) 401 return false; 402 ParametersParameterComponent o = (ParametersParameterComponent) other; 403 return compareValues(name, o.name, true); 404 } 405 406 public boolean isEmpty() { 407 return super.isEmpty() && (name == null || name.isEmpty()) && (value == null || value.isEmpty()) 408 && (resource == null || resource.isEmpty()) && (part == null || part.isEmpty()); 409 } 410 411 public String fhirType() { 412 return "null"; 413 414 } 415 416 } 417 418 /** 419 * A parameter passed to or received from the operation. 420 */ 421 @Child(name = "parameter", type = {}, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 422 @Description(shortDefinition = "Operation Parameter", formalDefinition = "A parameter passed to or received from the operation.") 423 protected List<ParametersParameterComponent> parameter; 424 425 private static final long serialVersionUID = -1495940293L; 426 427 /* 428 * Constructor 429 */ 430 public Parameters() { 431 super(); 432 } 433 434 /** 435 * @return {@link #parameter} (A parameter passed to or received from the 436 * operation.) 437 */ 438 public List<ParametersParameterComponent> getParameter() { 439 if (this.parameter == null) 440 this.parameter = new ArrayList<ParametersParameterComponent>(); 441 return this.parameter; 442 } 443 444 public boolean hasParameter() { 445 if (this.parameter == null) 446 return false; 447 for (ParametersParameterComponent item : this.parameter) 448 if (!item.isEmpty()) 449 return true; 450 return false; 451 } 452 453 /** 454 * @return {@link #parameter} (A parameter passed to or received from the 455 * operation.) 456 */ 457 // syntactic sugar 458 public ParametersParameterComponent addParameter() { // 3 459 ParametersParameterComponent t = new ParametersParameterComponent(); 460 if (this.parameter == null) 461 this.parameter = new ArrayList<ParametersParameterComponent>(); 462 this.parameter.add(t); 463 return t; 464 } 465 466 // syntactic sugar 467 public Parameters addParameter(ParametersParameterComponent t) { // 3 468 if (t == null) 469 return this; 470 if (this.parameter == null) 471 this.parameter = new ArrayList<ParametersParameterComponent>(); 472 this.parameter.add(t); 473 return this; 474 } 475 476 protected void listChildren(List<Property> childrenList) { 477 super.listChildren(childrenList); 478 childrenList.add(new Property("parameter", "", "A parameter passed to or received from the operation.", 0, 479 java.lang.Integer.MAX_VALUE, parameter)); 480 } 481 482 @Override 483 public void setProperty(String name, Base value) throws FHIRException { 484 if (name.equals("parameter")) 485 this.getParameter().add((ParametersParameterComponent) value); 486 else 487 super.setProperty(name, value); 488 } 489 490 @Override 491 public Base addChild(String name) throws FHIRException { 492 if (name.equals("parameter")) { 493 return addParameter(); 494 } else 495 return super.addChild(name); 496 } 497 498 public String fhirType() { 499 return "Parameters"; 500 501 } 502 503 public Parameters copy() { 504 Parameters dst = new Parameters(); 505 copyValues(dst); 506 if (parameter != null) { 507 dst.parameter = new ArrayList<ParametersParameterComponent>(); 508 for (ParametersParameterComponent i : parameter) 509 dst.parameter.add(i.copy()); 510 } 511 ; 512 return dst; 513 } 514 515 protected Parameters typedCopy() { 516 return copy(); 517 } 518 519 @Override 520 public boolean equalsDeep(Base other) { 521 if (!super.equalsDeep(other)) 522 return false; 523 if (!(other instanceof Parameters)) 524 return false; 525 Parameters o = (Parameters) other; 526 return compareDeep(parameter, o.parameter, true); 527 } 528 529 @Override 530 public boolean equalsShallow(Base other) { 531 if (!super.equalsShallow(other)) 532 return false; 533 if (!(other instanceof Parameters)) 534 return false; 535 Parameters o = (Parameters) other; 536 return true; 537 } 538 539 public boolean isEmpty() { 540 return super.isEmpty() && (parameter == null || parameter.isEmpty()); 541 } 542 543 @Override 544 public ResourceType getResourceType() { 545 return ResourceType.Parameters; 546 } 547 548}