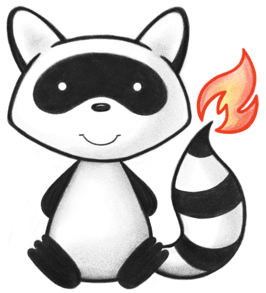
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender; 038import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046 047/** 048 * Demographics and other administrative information about an individual or 049 * animal receiving care or other health-related services. 050 */ 051@ResourceDef(name = "Patient", profile = "http://hl7.org/fhir/Profile/Patient") 052public class Patient extends DomainResource { 053 054 public enum LinkType { 055 /** 056 * The patient resource containing this link must no longer be used. The link 057 * points forward to another patient resource that must be used in lieu of the 058 * patient resource that contains this link. 059 */ 060 REPLACE, 061 /** 062 * The patient resource containing this link is in use and valid but not 063 * considered the main source of information about a patient. The link points 064 * forward to another patient resource that should be consulted to retrieve 065 * additional patient information. 066 */ 067 REFER, 068 /** 069 * The patient resource containing this link is in use and valid, but points to 070 * another patient resource that is known to contain data about the same person. 071 * Data in this resource might overlap or contradict information found in the 072 * other patient resource. This link does not indicate any relative importance 073 * of the resources concerned, and both should be regarded as equally valid. 074 */ 075 SEEALSO, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 081 public static LinkType fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("replace".equals(codeString)) 085 return REPLACE; 086 if ("refer".equals(codeString)) 087 return REFER; 088 if ("seealso".equals(codeString)) 089 return SEEALSO; 090 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 091 } 092 093 public String toCode() { 094 switch (this) { 095 case REPLACE: 096 return "replace"; 097 case REFER: 098 return "refer"; 099 case SEEALSO: 100 return "seealso"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 switch (this) { 110 case REPLACE: 111 return "http://hl7.org/fhir/link-type"; 112 case REFER: 113 return "http://hl7.org/fhir/link-type"; 114 case SEEALSO: 115 return "http://hl7.org/fhir/link-type"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case REPLACE: 126 return "The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link."; 127 case REFER: 128 return "The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information."; 129 case SEEALSO: 130 return "The patient resource containing this link is in use and valid, but points to another patient resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other patient resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid."; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDisplay() { 139 switch (this) { 140 case REPLACE: 141 return "Replace"; 142 case REFER: 143 return "Refer"; 144 case SEEALSO: 145 return "See also"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 } 153 154 public static class LinkTypeEnumFactory implements EnumFactory<LinkType> { 155 public LinkType fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("replace".equals(codeString)) 160 return LinkType.REPLACE; 161 if ("refer".equals(codeString)) 162 return LinkType.REFER; 163 if ("seealso".equals(codeString)) 164 return LinkType.SEEALSO; 165 throw new IllegalArgumentException("Unknown LinkType code '" + codeString + "'"); 166 } 167 168 public Enumeration<LinkType> fromType(Base code) throws FHIRException { 169 if (code == null || code.isEmpty()) 170 return null; 171 String codeString = ((PrimitiveType) code).asStringValue(); 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("replace".equals(codeString)) 175 return new Enumeration<LinkType>(this, LinkType.REPLACE); 176 if ("refer".equals(codeString)) 177 return new Enumeration<LinkType>(this, LinkType.REFER); 178 if ("seealso".equals(codeString)) 179 return new Enumeration<LinkType>(this, LinkType.SEEALSO); 180 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 181 } 182 183 public String toCode(LinkType code) 184 { 185 if (code == LinkType.NULL) 186 return null; 187 if (code == LinkType.REPLACE) 188 return "replace"; 189 if (code == LinkType.REFER) 190 return "refer"; 191 if (code == LinkType.SEEALSO) 192 return "seealso"; 193 return "?"; 194 } 195 } 196 197 @Block() 198 public static class ContactComponent extends BackboneElement implements IBaseBackboneElement { 199 /** 200 * The nature of the relationship between the patient and the contact person. 201 */ 202 @Child(name = "relationship", type = { 203 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 204 @Description(shortDefinition = "The kind of relationship", formalDefinition = "The nature of the relationship between the patient and the contact person.") 205 protected List<CodeableConcept> relationship; 206 207 /** 208 * A name associated with the contact person. 209 */ 210 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 211 @Description(shortDefinition = "A name associated with the contact person", formalDefinition = "A name associated with the contact person.") 212 protected HumanName name; 213 214 /** 215 * A contact detail for the person, e.g. a telephone number or an email address. 216 */ 217 @Child(name = "telecom", type = { 218 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 219 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 220 protected List<ContactPoint> telecom; 221 222 /** 223 * Address for the contact person. 224 */ 225 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 226 @Description(shortDefinition = "Address for the contact person", formalDefinition = "Address for the contact person.") 227 protected Address address; 228 229 /** 230 * Administrative Gender - the gender that the contact person is considered to 231 * have for administration and record keeping purposes. 232 */ 233 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 234 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.") 235 protected Enumeration<AdministrativeGender> gender; 236 237 /** 238 * Organization on behalf of which the contact is acting or for which the 239 * contact is working. 240 */ 241 @Child(name = "organization", type = { 242 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 243 @Description(shortDefinition = "Organization that is associated with the contact", formalDefinition = "Organization on behalf of which the contact is acting or for which the contact is working.") 244 protected Reference organization; 245 246 /** 247 * The actual object that is the target of the reference (Organization on behalf 248 * of which the contact is acting or for which the contact is working.) 249 */ 250 protected Organization organizationTarget; 251 252 /** 253 * The period during which this contact person or organization is valid to be 254 * contacted relating to this patient. 255 */ 256 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 257 @Description(shortDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient", formalDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient.") 258 protected Period period; 259 260 private static final long serialVersionUID = 364269017L; 261 262 /* 263 * Constructor 264 */ 265 public ContactComponent() { 266 super(); 267 } 268 269 /** 270 * @return {@link #relationship} (The nature of the relationship between the 271 * patient and the contact person.) 272 */ 273 public List<CodeableConcept> getRelationship() { 274 if (this.relationship == null) 275 this.relationship = new ArrayList<CodeableConcept>(); 276 return this.relationship; 277 } 278 279 public boolean hasRelationship() { 280 if (this.relationship == null) 281 return false; 282 for (CodeableConcept item : this.relationship) 283 if (!item.isEmpty()) 284 return true; 285 return false; 286 } 287 288 /** 289 * @return {@link #relationship} (The nature of the relationship between the 290 * patient and the contact person.) 291 */ 292 // syntactic sugar 293 public CodeableConcept addRelationship() { // 3 294 CodeableConcept t = new CodeableConcept(); 295 if (this.relationship == null) 296 this.relationship = new ArrayList<CodeableConcept>(); 297 this.relationship.add(t); 298 return t; 299 } 300 301 // syntactic sugar 302 public ContactComponent addRelationship(CodeableConcept t) { // 3 303 if (t == null) 304 return this; 305 if (this.relationship == null) 306 this.relationship = new ArrayList<CodeableConcept>(); 307 this.relationship.add(t); 308 return this; 309 } 310 311 /** 312 * @return {@link #name} (A name associated with the contact person.) 313 */ 314 public HumanName getName() { 315 if (this.name == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create ContactComponent.name"); 318 else if (Configuration.doAutoCreate()) 319 this.name = new HumanName(); // cc 320 return this.name; 321 } 322 323 public boolean hasName() { 324 return this.name != null && !this.name.isEmpty(); 325 } 326 327 /** 328 * @param value {@link #name} (A name associated with the contact person.) 329 */ 330 public ContactComponent setName(HumanName value) { 331 this.name = value; 332 return this; 333 } 334 335 /** 336 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 337 * number or an email address.) 338 */ 339 public List<ContactPoint> getTelecom() { 340 if (this.telecom == null) 341 this.telecom = new ArrayList<ContactPoint>(); 342 return this.telecom; 343 } 344 345 public boolean hasTelecom() { 346 if (this.telecom == null) 347 return false; 348 for (ContactPoint item : this.telecom) 349 if (!item.isEmpty()) 350 return true; 351 return false; 352 } 353 354 /** 355 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 356 * number or an email address.) 357 */ 358 // syntactic sugar 359 public ContactPoint addTelecom() { // 3 360 ContactPoint t = new ContactPoint(); 361 if (this.telecom == null) 362 this.telecom = new ArrayList<ContactPoint>(); 363 this.telecom.add(t); 364 return t; 365 } 366 367 // syntactic sugar 368 public ContactComponent addTelecom(ContactPoint t) { // 3 369 if (t == null) 370 return this; 371 if (this.telecom == null) 372 this.telecom = new ArrayList<ContactPoint>(); 373 this.telecom.add(t); 374 return this; 375 } 376 377 /** 378 * @return {@link #address} (Address for the contact person.) 379 */ 380 public Address getAddress() { 381 if (this.address == null) 382 if (Configuration.errorOnAutoCreate()) 383 throw new Error("Attempt to auto-create ContactComponent.address"); 384 else if (Configuration.doAutoCreate()) 385 this.address = new Address(); // cc 386 return this.address; 387 } 388 389 public boolean hasAddress() { 390 return this.address != null && !this.address.isEmpty(); 391 } 392 393 /** 394 * @param value {@link #address} (Address for the contact person.) 395 */ 396 public ContactComponent setAddress(Address value) { 397 this.address = value; 398 return this; 399 } 400 401 /** 402 * @return {@link #gender} (Administrative Gender - the gender that the contact 403 * person is considered to have for administration and record keeping 404 * purposes.). This is the underlying object with id, value and 405 * extensions. The accessor "getGender" gives direct access to the value 406 */ 407 public Enumeration<AdministrativeGender> getGenderElement() { 408 if (this.gender == null) 409 if (Configuration.errorOnAutoCreate()) 410 throw new Error("Attempt to auto-create ContactComponent.gender"); 411 else if (Configuration.doAutoCreate()) 412 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 413 return this.gender; 414 } 415 416 public boolean hasGenderElement() { 417 return this.gender != null && !this.gender.isEmpty(); 418 } 419 420 public boolean hasGender() { 421 return this.gender != null && !this.gender.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #gender} (Administrative Gender - the gender that the 426 * contact person is considered to have for administration and 427 * record keeping purposes.). This is the underlying object with 428 * id, value and extensions. The accessor "getGender" gives direct 429 * access to the value 430 */ 431 public ContactComponent setGenderElement(Enumeration<AdministrativeGender> value) { 432 this.gender = value; 433 return this; 434 } 435 436 /** 437 * @return Administrative Gender - the gender that the contact person is 438 * considered to have for administration and record keeping purposes. 439 */ 440 public AdministrativeGender getGender() { 441 return this.gender == null ? null : this.gender.getValue(); 442 } 443 444 /** 445 * @param value Administrative Gender - the gender that the contact person is 446 * considered to have for administration and record keeping 447 * purposes. 448 */ 449 public ContactComponent setGender(AdministrativeGender value) { 450 if (value == null) 451 this.gender = null; 452 else { 453 if (this.gender == null) 454 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 455 this.gender.setValue(value); 456 } 457 return this; 458 } 459 460 /** 461 * @return {@link #organization} (Organization on behalf of which the contact is 462 * acting or for which the contact is working.) 463 */ 464 public Reference getOrganization() { 465 if (this.organization == null) 466 if (Configuration.errorOnAutoCreate()) 467 throw new Error("Attempt to auto-create ContactComponent.organization"); 468 else if (Configuration.doAutoCreate()) 469 this.organization = new Reference(); // cc 470 return this.organization; 471 } 472 473 public boolean hasOrganization() { 474 return this.organization != null && !this.organization.isEmpty(); 475 } 476 477 /** 478 * @param value {@link #organization} (Organization on behalf of which the 479 * contact is acting or for which the contact is working.) 480 */ 481 public ContactComponent setOrganization(Reference value) { 482 this.organization = value; 483 return this; 484 } 485 486 /** 487 * @return {@link #organization} The actual object that is the target of the 488 * reference. The reference library doesn't populate this, but you can 489 * use it to hold the resource if you resolve it. (Organization on 490 * behalf of which the contact is acting or for which the contact is 491 * working.) 492 */ 493 public Organization getOrganizationTarget() { 494 if (this.organizationTarget == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create ContactComponent.organization"); 497 else if (Configuration.doAutoCreate()) 498 this.organizationTarget = new Organization(); // aa 499 return this.organizationTarget; 500 } 501 502 /** 503 * @param value {@link #organization} The actual object that is the target of 504 * the reference. The reference library doesn't use these, but you 505 * can use it to hold the resource if you resolve it. (Organization 506 * on behalf of which the contact is acting or for which the 507 * contact is working.) 508 */ 509 public ContactComponent setOrganizationTarget(Organization value) { 510 this.organizationTarget = value; 511 return this; 512 } 513 514 /** 515 * @return {@link #period} (The period during which this contact person or 516 * organization is valid to be contacted relating to this patient.) 517 */ 518 public Period getPeriod() { 519 if (this.period == null) 520 if (Configuration.errorOnAutoCreate()) 521 throw new Error("Attempt to auto-create ContactComponent.period"); 522 else if (Configuration.doAutoCreate()) 523 this.period = new Period(); // cc 524 return this.period; 525 } 526 527 public boolean hasPeriod() { 528 return this.period != null && !this.period.isEmpty(); 529 } 530 531 /** 532 * @param value {@link #period} (The period during which this contact person or 533 * organization is valid to be contacted relating to this patient.) 534 */ 535 public ContactComponent setPeriod(Period value) { 536 this.period = value; 537 return this; 538 } 539 540 protected void listChildren(List<Property> childrenList) { 541 super.listChildren(childrenList); 542 childrenList.add(new Property("relationship", "CodeableConcept", 543 "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, 544 relationship)); 545 childrenList.add(new Property("name", "HumanName", "A name associated with the contact person.", 0, 546 java.lang.Integer.MAX_VALUE, name)); 547 childrenList.add(new Property("telecom", "ContactPoint", 548 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 549 java.lang.Integer.MAX_VALUE, telecom)); 550 childrenList.add(new Property("address", "Address", "Address for the contact person.", 0, 551 java.lang.Integer.MAX_VALUE, address)); 552 childrenList.add(new Property("gender", "code", 553 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 554 0, java.lang.Integer.MAX_VALUE, gender)); 555 childrenList.add(new Property("organization", "Reference(Organization)", 556 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 557 java.lang.Integer.MAX_VALUE, organization)); 558 childrenList.add(new Property("period", "Period", 559 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 560 0, java.lang.Integer.MAX_VALUE, period)); 561 } 562 563 @Override 564 public void setProperty(String name, Base value) throws FHIRException { 565 if (name.equals("relationship")) 566 this.getRelationship().add(castToCodeableConcept(value)); 567 else if (name.equals("name")) 568 this.name = castToHumanName(value); // HumanName 569 else if (name.equals("telecom")) 570 this.getTelecom().add(castToContactPoint(value)); 571 else if (name.equals("address")) 572 this.address = castToAddress(value); // Address 573 else if (name.equals("gender")) 574 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 575 else if (name.equals("organization")) 576 this.organization = castToReference(value); // Reference 577 else if (name.equals("period")) 578 this.period = castToPeriod(value); // Period 579 else 580 super.setProperty(name, value); 581 } 582 583 @Override 584 public Base addChild(String name) throws FHIRException { 585 if (name.equals("relationship")) { 586 return addRelationship(); 587 } else if (name.equals("name")) { 588 this.name = new HumanName(); 589 return this.name; 590 } else if (name.equals("telecom")) { 591 return addTelecom(); 592 } else if (name.equals("address")) { 593 this.address = new Address(); 594 return this.address; 595 } else if (name.equals("gender")) { 596 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 597 } else if (name.equals("organization")) { 598 this.organization = new Reference(); 599 return this.organization; 600 } else if (name.equals("period")) { 601 this.period = new Period(); 602 return this.period; 603 } else 604 return super.addChild(name); 605 } 606 607 public ContactComponent copy() { 608 ContactComponent dst = new ContactComponent(); 609 copyValues(dst); 610 if (relationship != null) { 611 dst.relationship = new ArrayList<CodeableConcept>(); 612 for (CodeableConcept i : relationship) 613 dst.relationship.add(i.copy()); 614 } 615 ; 616 dst.name = name == null ? null : name.copy(); 617 if (telecom != null) { 618 dst.telecom = new ArrayList<ContactPoint>(); 619 for (ContactPoint i : telecom) 620 dst.telecom.add(i.copy()); 621 } 622 ; 623 dst.address = address == null ? null : address.copy(); 624 dst.gender = gender == null ? null : gender.copy(); 625 dst.organization = organization == null ? null : organization.copy(); 626 dst.period = period == null ? null : period.copy(); 627 return dst; 628 } 629 630 @Override 631 public boolean equalsDeep(Base other) { 632 if (!super.equalsDeep(other)) 633 return false; 634 if (!(other instanceof ContactComponent)) 635 return false; 636 ContactComponent o = (ContactComponent) other; 637 return compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) 638 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true) 639 && compareDeep(gender, o.gender, true) && compareDeep(organization, o.organization, true) 640 && compareDeep(period, o.period, true); 641 } 642 643 @Override 644 public boolean equalsShallow(Base other) { 645 if (!super.equalsShallow(other)) 646 return false; 647 if (!(other instanceof ContactComponent)) 648 return false; 649 ContactComponent o = (ContactComponent) other; 650 return compareValues(gender, o.gender, true); 651 } 652 653 public boolean isEmpty() { 654 return super.isEmpty() && (relationship == null || relationship.isEmpty()) && (name == null || name.isEmpty()) 655 && (telecom == null || telecom.isEmpty()) && (address == null || address.isEmpty()) 656 && (gender == null || gender.isEmpty()) && (organization == null || organization.isEmpty()) 657 && (period == null || period.isEmpty()); 658 } 659 660 public String fhirType() { 661 return "Patient.contact"; 662 663 } 664 665 } 666 667 @Block() 668 public static class AnimalComponent extends BackboneElement implements IBaseBackboneElement { 669 /** 670 * Identifies the high level taxonomic categorization of the kind of animal. 671 */ 672 @Child(name = "species", type = { 673 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 674 @Description(shortDefinition = "E.g. Dog, Cow", formalDefinition = "Identifies the high level taxonomic categorization of the kind of animal.") 675 protected CodeableConcept species; 676 677 /** 678 * Identifies the detailed categorization of the kind of animal. 679 */ 680 @Child(name = "breed", type = { 681 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 682 @Description(shortDefinition = "E.g. Poodle, Angus", formalDefinition = "Identifies the detailed categorization of the kind of animal.") 683 protected CodeableConcept breed; 684 685 /** 686 * Indicates the current state of the animal's reproductive organs. 687 */ 688 @Child(name = "genderStatus", type = { 689 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 690 @Description(shortDefinition = "E.g. Neutered, Intact", formalDefinition = "Indicates the current state of the animal's reproductive organs.") 691 protected CodeableConcept genderStatus; 692 693 private static final long serialVersionUID = -549738382L; 694 695 /* 696 * Constructor 697 */ 698 public AnimalComponent() { 699 super(); 700 } 701 702 /* 703 * Constructor 704 */ 705 public AnimalComponent(CodeableConcept species) { 706 super(); 707 this.species = species; 708 } 709 710 /** 711 * @return {@link #species} (Identifies the high level taxonomic categorization 712 * of the kind of animal.) 713 */ 714 public CodeableConcept getSpecies() { 715 if (this.species == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create AnimalComponent.species"); 718 else if (Configuration.doAutoCreate()) 719 this.species = new CodeableConcept(); // cc 720 return this.species; 721 } 722 723 public boolean hasSpecies() { 724 return this.species != null && !this.species.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #species} (Identifies the high level taxonomic 729 * categorization of the kind of animal.) 730 */ 731 public AnimalComponent setSpecies(CodeableConcept value) { 732 this.species = value; 733 return this; 734 } 735 736 /** 737 * @return {@link #breed} (Identifies the detailed categorization of the kind of 738 * animal.) 739 */ 740 public CodeableConcept getBreed() { 741 if (this.breed == null) 742 if (Configuration.errorOnAutoCreate()) 743 throw new Error("Attempt to auto-create AnimalComponent.breed"); 744 else if (Configuration.doAutoCreate()) 745 this.breed = new CodeableConcept(); // cc 746 return this.breed; 747 } 748 749 public boolean hasBreed() { 750 return this.breed != null && !this.breed.isEmpty(); 751 } 752 753 /** 754 * @param value {@link #breed} (Identifies the detailed categorization of the 755 * kind of animal.) 756 */ 757 public AnimalComponent setBreed(CodeableConcept value) { 758 this.breed = value; 759 return this; 760 } 761 762 /** 763 * @return {@link #genderStatus} (Indicates the current state of the animal's 764 * reproductive organs.) 765 */ 766 public CodeableConcept getGenderStatus() { 767 if (this.genderStatus == null) 768 if (Configuration.errorOnAutoCreate()) 769 throw new Error("Attempt to auto-create AnimalComponent.genderStatus"); 770 else if (Configuration.doAutoCreate()) 771 this.genderStatus = new CodeableConcept(); // cc 772 return this.genderStatus; 773 } 774 775 public boolean hasGenderStatus() { 776 return this.genderStatus != null && !this.genderStatus.isEmpty(); 777 } 778 779 /** 780 * @param value {@link #genderStatus} (Indicates the current state of the 781 * animal's reproductive organs.) 782 */ 783 public AnimalComponent setGenderStatus(CodeableConcept value) { 784 this.genderStatus = value; 785 return this; 786 } 787 788 protected void listChildren(List<Property> childrenList) { 789 super.listChildren(childrenList); 790 childrenList.add(new Property("species", "CodeableConcept", 791 "Identifies the high level taxonomic categorization of the kind of animal.", 0, java.lang.Integer.MAX_VALUE, 792 species)); 793 childrenList.add(new Property("breed", "CodeableConcept", 794 "Identifies the detailed categorization of the kind of animal.", 0, java.lang.Integer.MAX_VALUE, breed)); 795 childrenList.add(new Property("genderStatus", "CodeableConcept", 796 "Indicates the current state of the animal's reproductive organs.", 0, java.lang.Integer.MAX_VALUE, 797 genderStatus)); 798 } 799 800 @Override 801 public void setProperty(String name, Base value) throws FHIRException { 802 if (name.equals("species")) 803 this.species = castToCodeableConcept(value); // CodeableConcept 804 else if (name.equals("breed")) 805 this.breed = castToCodeableConcept(value); // CodeableConcept 806 else if (name.equals("genderStatus")) 807 this.genderStatus = castToCodeableConcept(value); // CodeableConcept 808 else 809 super.setProperty(name, value); 810 } 811 812 @Override 813 public Base addChild(String name) throws FHIRException { 814 if (name.equals("species")) { 815 this.species = new CodeableConcept(); 816 return this.species; 817 } else if (name.equals("breed")) { 818 this.breed = new CodeableConcept(); 819 return this.breed; 820 } else if (name.equals("genderStatus")) { 821 this.genderStatus = new CodeableConcept(); 822 return this.genderStatus; 823 } else 824 return super.addChild(name); 825 } 826 827 public AnimalComponent copy() { 828 AnimalComponent dst = new AnimalComponent(); 829 copyValues(dst); 830 dst.species = species == null ? null : species.copy(); 831 dst.breed = breed == null ? null : breed.copy(); 832 dst.genderStatus = genderStatus == null ? null : genderStatus.copy(); 833 return dst; 834 } 835 836 @Override 837 public boolean equalsDeep(Base other) { 838 if (!super.equalsDeep(other)) 839 return false; 840 if (!(other instanceof AnimalComponent)) 841 return false; 842 AnimalComponent o = (AnimalComponent) other; 843 return compareDeep(species, o.species, true) && compareDeep(breed, o.breed, true) 844 && compareDeep(genderStatus, o.genderStatus, true); 845 } 846 847 @Override 848 public boolean equalsShallow(Base other) { 849 if (!super.equalsShallow(other)) 850 return false; 851 if (!(other instanceof AnimalComponent)) 852 return false; 853 AnimalComponent o = (AnimalComponent) other; 854 return true; 855 } 856 857 public boolean isEmpty() { 858 return super.isEmpty() && (species == null || species.isEmpty()) && (breed == null || breed.isEmpty()) 859 && (genderStatus == null || genderStatus.isEmpty()); 860 } 861 862 public String fhirType() { 863 return "Patient.animal"; 864 865 } 866 867 } 868 869 @Block() 870 public static class PatientCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 871 /** 872 * The ISO-639-1 alpha 2 code in lower case for the language, optionally 873 * followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper 874 * case; e.g. "en" for English, or "en-US" for American English versus "en-EN" 875 * for England English. 876 */ 877 @Child(name = "language", type = { 878 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 879 @Description(shortDefinition = "The language which can be used to communicate with the patient about his or her health", formalDefinition = "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.") 880 protected CodeableConcept language; 881 882 /** 883 * Indicates whether or not the patient prefers this language (over other 884 * languages he masters up a certain level). 885 */ 886 @Child(name = "preferred", type = { 887 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 888 @Description(shortDefinition = "Language preference indicator", formalDefinition = "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).") 889 protected BooleanType preferred; 890 891 private static final long serialVersionUID = 633792918L; 892 893 /* 894 * Constructor 895 */ 896 public PatientCommunicationComponent() { 897 super(); 898 } 899 900 /* 901 * Constructor 902 */ 903 public PatientCommunicationComponent(CodeableConcept language) { 904 super(); 905 this.language = language; 906 } 907 908 /** 909 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the 910 * language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 911 * code for the region in upper case; e.g. "en" for English, or "en-US" 912 * for American English versus "en-EN" for England English.) 913 */ 914 public CodeableConcept getLanguage() { 915 if (this.language == null) 916 if (Configuration.errorOnAutoCreate()) 917 throw new Error("Attempt to auto-create PatientCommunicationComponent.language"); 918 else if (Configuration.doAutoCreate()) 919 this.language = new CodeableConcept(); // cc 920 return this.language; 921 } 922 923 public boolean hasLanguage() { 924 return this.language != null && !this.language.isEmpty(); 925 } 926 927 /** 928 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for 929 * the language, optionally followed by a hyphen and the ISO-3166-1 930 * alpha 2 code for the region in upper case; e.g. "en" for 931 * English, or "en-US" for American English versus "en-EN" for 932 * England English.) 933 */ 934 public PatientCommunicationComponent setLanguage(CodeableConcept value) { 935 this.language = value; 936 return this; 937 } 938 939 /** 940 * @return {@link #preferred} (Indicates whether or not the patient prefers this 941 * language (over other languages he masters up a certain level).). This 942 * is the underlying object with id, value and extensions. The accessor 943 * "getPreferred" gives direct access to the value 944 */ 945 public BooleanType getPreferredElement() { 946 if (this.preferred == null) 947 if (Configuration.errorOnAutoCreate()) 948 throw new Error("Attempt to auto-create PatientCommunicationComponent.preferred"); 949 else if (Configuration.doAutoCreate()) 950 this.preferred = new BooleanType(); // bb 951 return this.preferred; 952 } 953 954 public boolean hasPreferredElement() { 955 return this.preferred != null && !this.preferred.isEmpty(); 956 } 957 958 public boolean hasPreferred() { 959 return this.preferred != null && !this.preferred.isEmpty(); 960 } 961 962 /** 963 * @param value {@link #preferred} (Indicates whether or not the patient prefers 964 * this language (over other languages he masters up a certain 965 * level).). This is the underlying object with id, value and 966 * extensions. The accessor "getPreferred" gives direct access to 967 * the value 968 */ 969 public PatientCommunicationComponent setPreferredElement(BooleanType value) { 970 this.preferred = value; 971 return this; 972 } 973 974 /** 975 * @return Indicates whether or not the patient prefers this language (over 976 * other languages he masters up a certain level). 977 */ 978 public boolean getPreferred() { 979 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 980 } 981 982 /** 983 * @param value Indicates whether or not the patient prefers this language (over 984 * other languages he masters up a certain level). 985 */ 986 public PatientCommunicationComponent setPreferred(boolean value) { 987 if (this.preferred == null) 988 this.preferred = new BooleanType(); 989 this.preferred.setValue(value); 990 return this; 991 } 992 993 protected void listChildren(List<Property> childrenList) { 994 super.listChildren(childrenList); 995 childrenList.add(new Property("language", "CodeableConcept", 996 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 997 0, java.lang.Integer.MAX_VALUE, language)); 998 childrenList.add(new Property("preferred", "boolean", 999 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 1000 0, java.lang.Integer.MAX_VALUE, preferred)); 1001 } 1002 1003 @Override 1004 public void setProperty(String name, Base value) throws FHIRException { 1005 if (name.equals("language")) 1006 this.language = castToCodeableConcept(value); // CodeableConcept 1007 else if (name.equals("preferred")) 1008 this.preferred = castToBoolean(value); // BooleanType 1009 else 1010 super.setProperty(name, value); 1011 } 1012 1013 @Override 1014 public Base addChild(String name) throws FHIRException { 1015 if (name.equals("language")) { 1016 this.language = new CodeableConcept(); 1017 return this.language; 1018 } else if (name.equals("preferred")) { 1019 throw new FHIRException("Cannot call addChild on a singleton property Patient.preferred"); 1020 } else 1021 return super.addChild(name); 1022 } 1023 1024 public PatientCommunicationComponent copy() { 1025 PatientCommunicationComponent dst = new PatientCommunicationComponent(); 1026 copyValues(dst); 1027 dst.language = language == null ? null : language.copy(); 1028 dst.preferred = preferred == null ? null : preferred.copy(); 1029 return dst; 1030 } 1031 1032 @Override 1033 public boolean equalsDeep(Base other) { 1034 if (!super.equalsDeep(other)) 1035 return false; 1036 if (!(other instanceof PatientCommunicationComponent)) 1037 return false; 1038 PatientCommunicationComponent o = (PatientCommunicationComponent) other; 1039 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 1040 } 1041 1042 @Override 1043 public boolean equalsShallow(Base other) { 1044 if (!super.equalsShallow(other)) 1045 return false; 1046 if (!(other instanceof PatientCommunicationComponent)) 1047 return false; 1048 PatientCommunicationComponent o = (PatientCommunicationComponent) other; 1049 return compareValues(preferred, o.preferred, true); 1050 } 1051 1052 public boolean isEmpty() { 1053 return super.isEmpty() && (language == null || language.isEmpty()) && (preferred == null || preferred.isEmpty()); 1054 } 1055 1056 public String fhirType() { 1057 return "Patient.communication"; 1058 1059 } 1060 1061 } 1062 1063 @Block() 1064 public static class PatientLinkComponent extends BackboneElement implements IBaseBackboneElement { 1065 /** 1066 * The other patient resource that the link refers to. 1067 */ 1068 @Child(name = "other", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = true, summary = false) 1069 @Description(shortDefinition = "The other patient resource that the link refers to", formalDefinition = "The other patient resource that the link refers to.") 1070 protected Reference other; 1071 1072 /** 1073 * The actual object that is the target of the reference (The other patient 1074 * resource that the link refers to.) 1075 */ 1076 protected Patient otherTarget; 1077 1078 /** 1079 * The type of link between this patient resource and another patient resource. 1080 */ 1081 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = false) 1082 @Description(shortDefinition = "replace | refer | seealso - type of link", formalDefinition = "The type of link between this patient resource and another patient resource.") 1083 protected Enumeration<LinkType> type; 1084 1085 private static final long serialVersionUID = -1942104050L; 1086 1087 /* 1088 * Constructor 1089 */ 1090 public PatientLinkComponent() { 1091 super(); 1092 } 1093 1094 /* 1095 * Constructor 1096 */ 1097 public PatientLinkComponent(Reference other, Enumeration<LinkType> type) { 1098 super(); 1099 this.other = other; 1100 this.type = type; 1101 } 1102 1103 /** 1104 * @return {@link #other} (The other patient resource that the link refers to.) 1105 */ 1106 public Reference getOther() { 1107 if (this.other == null) 1108 if (Configuration.errorOnAutoCreate()) 1109 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1110 else if (Configuration.doAutoCreate()) 1111 this.other = new Reference(); // cc 1112 return this.other; 1113 } 1114 1115 public boolean hasOther() { 1116 return this.other != null && !this.other.isEmpty(); 1117 } 1118 1119 /** 1120 * @param value {@link #other} (The other patient resource that the link refers 1121 * to.) 1122 */ 1123 public PatientLinkComponent setOther(Reference value) { 1124 this.other = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #other} The actual object that is the target of the reference. 1130 * The reference library doesn't populate this, but you can use it to 1131 * hold the resource if you resolve it. (The other patient resource that 1132 * the link refers to.) 1133 */ 1134 public Patient getOtherTarget() { 1135 if (this.otherTarget == null) 1136 if (Configuration.errorOnAutoCreate()) 1137 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1138 else if (Configuration.doAutoCreate()) 1139 this.otherTarget = new Patient(); // aa 1140 return this.otherTarget; 1141 } 1142 1143 /** 1144 * @param value {@link #other} The actual object that is the target of the 1145 * reference. The reference library doesn't use these, but you can 1146 * use it to hold the resource if you resolve it. (The other 1147 * patient resource that the link refers to.) 1148 */ 1149 public PatientLinkComponent setOtherTarget(Patient value) { 1150 this.otherTarget = value; 1151 return this; 1152 } 1153 1154 /** 1155 * @return {@link #type} (The type of link between this patient resource and 1156 * another patient resource.). This is the underlying object with id, 1157 * value and extensions. The accessor "getType" gives direct access to 1158 * the value 1159 */ 1160 public Enumeration<LinkType> getTypeElement() { 1161 if (this.type == null) 1162 if (Configuration.errorOnAutoCreate()) 1163 throw new Error("Attempt to auto-create PatientLinkComponent.type"); 1164 else if (Configuration.doAutoCreate()) 1165 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); // bb 1166 return this.type; 1167 } 1168 1169 public boolean hasTypeElement() { 1170 return this.type != null && !this.type.isEmpty(); 1171 } 1172 1173 public boolean hasType() { 1174 return this.type != null && !this.type.isEmpty(); 1175 } 1176 1177 /** 1178 * @param value {@link #type} (The type of link between this patient resource 1179 * and another patient resource.). This is the underlying object 1180 * with id, value and extensions. The accessor "getType" gives 1181 * direct access to the value 1182 */ 1183 public PatientLinkComponent setTypeElement(Enumeration<LinkType> value) { 1184 this.type = value; 1185 return this; 1186 } 1187 1188 /** 1189 * @return The type of link between this patient resource and another patient 1190 * resource. 1191 */ 1192 public LinkType getType() { 1193 return this.type == null ? null : this.type.getValue(); 1194 } 1195 1196 /** 1197 * @param value The type of link between this patient resource and another 1198 * patient resource. 1199 */ 1200 public PatientLinkComponent setType(LinkType value) { 1201 if (this.type == null) 1202 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); 1203 this.type.setValue(value); 1204 return this; 1205 } 1206 1207 protected void listChildren(List<Property> childrenList) { 1208 super.listChildren(childrenList); 1209 childrenList.add(new Property("other", "Reference(Patient)", 1210 "The other patient resource that the link refers to.", 0, java.lang.Integer.MAX_VALUE, other)); 1211 childrenList.add( 1212 new Property("type", "code", "The type of link between this patient resource and another patient resource.", 1213 0, java.lang.Integer.MAX_VALUE, type)); 1214 } 1215 1216 @Override 1217 public void setProperty(String name, Base value) throws FHIRException { 1218 if (name.equals("other")) 1219 this.other = castToReference(value); // Reference 1220 else if (name.equals("type")) 1221 this.type = new LinkTypeEnumFactory().fromType(value); // Enumeration<LinkType> 1222 else 1223 super.setProperty(name, value); 1224 } 1225 1226 @Override 1227 public Base addChild(String name) throws FHIRException { 1228 if (name.equals("other")) { 1229 this.other = new Reference(); 1230 return this.other; 1231 } else if (name.equals("type")) { 1232 throw new FHIRException("Cannot call addChild on a singleton property Patient.type"); 1233 } else 1234 return super.addChild(name); 1235 } 1236 1237 public PatientLinkComponent copy() { 1238 PatientLinkComponent dst = new PatientLinkComponent(); 1239 copyValues(dst); 1240 dst.other = other == null ? null : other.copy(); 1241 dst.type = type == null ? null : type.copy(); 1242 return dst; 1243 } 1244 1245 @Override 1246 public boolean equalsDeep(Base other) { 1247 if (!super.equalsDeep(other)) 1248 return false; 1249 if (!(other instanceof PatientLinkComponent)) 1250 return false; 1251 PatientLinkComponent o = (PatientLinkComponent) other; 1252 return compareDeep(other, o.other, true) && compareDeep(type, o.type, true); 1253 } 1254 1255 @Override 1256 public boolean equalsShallow(Base other) { 1257 if (!super.equalsShallow(other)) 1258 return false; 1259 if (!(other instanceof PatientLinkComponent)) 1260 return false; 1261 PatientLinkComponent o = (PatientLinkComponent) other; 1262 return compareValues(type, o.type, true); 1263 } 1264 1265 public boolean isEmpty() { 1266 return super.isEmpty() && (other == null || other.isEmpty()) && (type == null || type.isEmpty()); 1267 } 1268 1269 public String fhirType() { 1270 return "Patient.link"; 1271 1272 } 1273 1274 } 1275 1276 /** 1277 * An identifier for this patient. 1278 */ 1279 @Child(name = "identifier", type = { 1280 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1281 @Description(shortDefinition = "An identifier for this patient", formalDefinition = "An identifier for this patient.") 1282 protected List<Identifier> identifier; 1283 1284 /** 1285 * Whether this patient record is in active use. 1286 */ 1287 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1288 @Description(shortDefinition = "Whether this patient's record is in active use", formalDefinition = "Whether this patient record is in active use.") 1289 protected BooleanType active; 1290 1291 /** 1292 * A name associated with the individual. 1293 */ 1294 @Child(name = "name", type = { 1295 HumanName.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1296 @Description(shortDefinition = "A name associated with the patient", formalDefinition = "A name associated with the individual.") 1297 protected List<HumanName> name; 1298 1299 /** 1300 * A contact detail (e.g. a telephone number or an email address) by which the 1301 * individual may be contacted. 1302 */ 1303 @Child(name = "telecom", type = { 1304 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1305 @Description(shortDefinition = "A contact detail for the individual", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.") 1306 protected List<ContactPoint> telecom; 1307 1308 /** 1309 * Administrative Gender - the gender that the patient is considered to have for 1310 * administration and record keeping purposes. 1311 */ 1312 @Child(name = "gender", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1313 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.") 1314 protected Enumeration<AdministrativeGender> gender; 1315 1316 /** 1317 * The date of birth for the individual. 1318 */ 1319 @Child(name = "birthDate", type = { DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1320 @Description(shortDefinition = "The date of birth for the individual", formalDefinition = "The date of birth for the individual.") 1321 protected DateType birthDate; 1322 1323 /** 1324 * Indicates if the individual is deceased or not. 1325 */ 1326 @Child(name = "deceased", type = { BooleanType.class, 1327 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1328 @Description(shortDefinition = "Indicates if the individual is deceased or not", formalDefinition = "Indicates if the individual is deceased or not.") 1329 protected Type deceased; 1330 1331 /** 1332 * Addresses for the individual. 1333 */ 1334 @Child(name = "address", type = { 1335 Address.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1336 @Description(shortDefinition = "Addresses for the individual", formalDefinition = "Addresses for the individual.") 1337 protected List<Address> address; 1338 1339 /** 1340 * This field contains a patient's most recent marital (civil) status. 1341 */ 1342 @Child(name = "maritalStatus", type = { 1343 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1344 @Description(shortDefinition = "Marital (civil) status of a patient", formalDefinition = "This field contains a patient's most recent marital (civil) status.") 1345 protected CodeableConcept maritalStatus; 1346 1347 /** 1348 * Indicates whether the patient is part of a multiple or indicates the actual 1349 * birth order. 1350 */ 1351 @Child(name = "multipleBirth", type = { BooleanType.class, 1352 IntegerType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1353 @Description(shortDefinition = "Whether patient is part of a multiple birth", formalDefinition = "Indicates whether the patient is part of a multiple or indicates the actual birth order.") 1354 protected Type multipleBirth; 1355 1356 /** 1357 * Image of the patient. 1358 */ 1359 @Child(name = "photo", type = { 1360 Attachment.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1361 @Description(shortDefinition = "Image of the patient", formalDefinition = "Image of the patient.") 1362 protected List<Attachment> photo; 1363 1364 /** 1365 * A contact party (e.g. guardian, partner, friend) for the patient. 1366 */ 1367 @Child(name = "contact", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1368 @Description(shortDefinition = "A contact party (e.g. guardian, partner, friend) for the patient", formalDefinition = "A contact party (e.g. guardian, partner, friend) for the patient.") 1369 protected List<ContactComponent> contact; 1370 1371 /** 1372 * This patient is known to be an animal. 1373 */ 1374 @Child(name = "animal", type = {}, order = 12, min = 0, max = 1, modifier = true, summary = true) 1375 @Description(shortDefinition = "This patient is known to be an animal (non-human)", formalDefinition = "This patient is known to be an animal.") 1376 protected AnimalComponent animal; 1377 1378 /** 1379 * Languages which may be used to communicate with the patient about his or her 1380 * health. 1381 */ 1382 @Child(name = "communication", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1383 @Description(shortDefinition = "A list of Languages which may be used to communicate with the patient about his or her health", formalDefinition = "Languages which may be used to communicate with the patient about his or her health.") 1384 protected List<PatientCommunicationComponent> communication; 1385 1386 /** 1387 * Patient's nominated care provider. 1388 */ 1389 @Child(name = "careProvider", type = { Organization.class, 1390 Practitioner.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1391 @Description(shortDefinition = "Patient's nominated primary care provider", formalDefinition = "Patient's nominated care provider.") 1392 protected List<Reference> careProvider; 1393 /** 1394 * The actual objects that are the target of the reference (Patient's nominated 1395 * care provider.) 1396 */ 1397 protected List<Resource> careProviderTarget; 1398 1399 /** 1400 * Organization that is the custodian of the patient record. 1401 */ 1402 @Child(name = "managingOrganization", type = { 1403 Organization.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1404 @Description(shortDefinition = "Organization that is the custodian of the patient record", formalDefinition = "Organization that is the custodian of the patient record.") 1405 protected Reference managingOrganization; 1406 1407 /** 1408 * The actual object that is the target of the reference (Organization that is 1409 * the custodian of the patient record.) 1410 */ 1411 protected Organization managingOrganizationTarget; 1412 1413 /** 1414 * Link to another patient resource that concerns the same actual patient. 1415 */ 1416 @Child(name = "link", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = false) 1417 @Description(shortDefinition = "Link to another patient resource that concerns the same actual person", formalDefinition = "Link to another patient resource that concerns the same actual patient.") 1418 protected List<PatientLinkComponent> link; 1419 1420 private static final long serialVersionUID = 2019992554L; 1421 1422 /* 1423 * Constructor 1424 */ 1425 public Patient() { 1426 super(); 1427 } 1428 1429 /** 1430 * @return {@link #identifier} (An identifier for this patient.) 1431 */ 1432 public List<Identifier> getIdentifier() { 1433 if (this.identifier == null) 1434 this.identifier = new ArrayList<Identifier>(); 1435 return this.identifier; 1436 } 1437 1438 public boolean hasIdentifier() { 1439 if (this.identifier == null) 1440 return false; 1441 for (Identifier item : this.identifier) 1442 if (!item.isEmpty()) 1443 return true; 1444 return false; 1445 } 1446 1447 /** 1448 * @return {@link #identifier} (An identifier for this patient.) 1449 */ 1450 // syntactic sugar 1451 public Identifier addIdentifier() { // 3 1452 Identifier t = new Identifier(); 1453 if (this.identifier == null) 1454 this.identifier = new ArrayList<Identifier>(); 1455 this.identifier.add(t); 1456 return t; 1457 } 1458 1459 // syntactic sugar 1460 public Patient addIdentifier(Identifier t) { // 3 1461 if (t == null) 1462 return this; 1463 if (this.identifier == null) 1464 this.identifier = new ArrayList<Identifier>(); 1465 this.identifier.add(t); 1466 return this; 1467 } 1468 1469 /** 1470 * @return {@link #active} (Whether this patient record is in active use.). This 1471 * is the underlying object with id, value and extensions. The accessor 1472 * "getActive" gives direct access to the value 1473 */ 1474 public BooleanType getActiveElement() { 1475 if (this.active == null) 1476 if (Configuration.errorOnAutoCreate()) 1477 throw new Error("Attempt to auto-create Patient.active"); 1478 else if (Configuration.doAutoCreate()) 1479 this.active = new BooleanType(); // bb 1480 return this.active; 1481 } 1482 1483 public boolean hasActiveElement() { 1484 return this.active != null && !this.active.isEmpty(); 1485 } 1486 1487 public boolean hasActive() { 1488 return this.active != null && !this.active.isEmpty(); 1489 } 1490 1491 /** 1492 * @param value {@link #active} (Whether this patient record is in active use.). 1493 * This is the underlying object with id, value and extensions. The 1494 * accessor "getActive" gives direct access to the value 1495 */ 1496 public Patient setActiveElement(BooleanType value) { 1497 this.active = value; 1498 return this; 1499 } 1500 1501 /** 1502 * @return Whether this patient record is in active use. 1503 */ 1504 public boolean getActive() { 1505 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1506 } 1507 1508 /** 1509 * @param value Whether this patient record is in active use. 1510 */ 1511 public Patient setActive(boolean value) { 1512 if (this.active == null) 1513 this.active = new BooleanType(); 1514 this.active.setValue(value); 1515 return this; 1516 } 1517 1518 /** 1519 * @return {@link #name} (A name associated with the individual.) 1520 */ 1521 public List<HumanName> getName() { 1522 if (this.name == null) 1523 this.name = new ArrayList<HumanName>(); 1524 return this.name; 1525 } 1526 1527 public boolean hasName() { 1528 if (this.name == null) 1529 return false; 1530 for (HumanName item : this.name) 1531 if (!item.isEmpty()) 1532 return true; 1533 return false; 1534 } 1535 1536 /** 1537 * @return {@link #name} (A name associated with the individual.) 1538 */ 1539 // syntactic sugar 1540 public HumanName addName() { // 3 1541 HumanName t = new HumanName(); 1542 if (this.name == null) 1543 this.name = new ArrayList<HumanName>(); 1544 this.name.add(t); 1545 return t; 1546 } 1547 1548 // syntactic sugar 1549 public Patient addName(HumanName t) { // 3 1550 if (t == null) 1551 return this; 1552 if (this.name == null) 1553 this.name = new ArrayList<HumanName>(); 1554 this.name.add(t); 1555 return this; 1556 } 1557 1558 /** 1559 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 1560 * email address) by which the individual may be contacted.) 1561 */ 1562 public List<ContactPoint> getTelecom() { 1563 if (this.telecom == null) 1564 this.telecom = new ArrayList<ContactPoint>(); 1565 return this.telecom; 1566 } 1567 1568 public boolean hasTelecom() { 1569 if (this.telecom == null) 1570 return false; 1571 for (ContactPoint item : this.telecom) 1572 if (!item.isEmpty()) 1573 return true; 1574 return false; 1575 } 1576 1577 /** 1578 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 1579 * email address) by which the individual may be contacted.) 1580 */ 1581 // syntactic sugar 1582 public ContactPoint addTelecom() { // 3 1583 ContactPoint t = new ContactPoint(); 1584 if (this.telecom == null) 1585 this.telecom = new ArrayList<ContactPoint>(); 1586 this.telecom.add(t); 1587 return t; 1588 } 1589 1590 // syntactic sugar 1591 public Patient addTelecom(ContactPoint t) { // 3 1592 if (t == null) 1593 return this; 1594 if (this.telecom == null) 1595 this.telecom = new ArrayList<ContactPoint>(); 1596 this.telecom.add(t); 1597 return this; 1598 } 1599 1600 /** 1601 * @return {@link #gender} (Administrative Gender - the gender that the patient 1602 * is considered to have for administration and record keeping 1603 * purposes.). This is the underlying object with id, value and 1604 * extensions. The accessor "getGender" gives direct access to the value 1605 */ 1606 public Enumeration<AdministrativeGender> getGenderElement() { 1607 if (this.gender == null) 1608 if (Configuration.errorOnAutoCreate()) 1609 throw new Error("Attempt to auto-create Patient.gender"); 1610 else if (Configuration.doAutoCreate()) 1611 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1612 return this.gender; 1613 } 1614 1615 public boolean hasGenderElement() { 1616 return this.gender != null && !this.gender.isEmpty(); 1617 } 1618 1619 public boolean hasGender() { 1620 return this.gender != null && !this.gender.isEmpty(); 1621 } 1622 1623 /** 1624 * @param value {@link #gender} (Administrative Gender - the gender that the 1625 * patient is considered to have for administration and record 1626 * keeping purposes.). This is the underlying object with id, value 1627 * and extensions. The accessor "getGender" gives direct access to 1628 * the value 1629 */ 1630 public Patient setGenderElement(Enumeration<AdministrativeGender> value) { 1631 this.gender = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return Administrative Gender - the gender that the patient is considered to 1637 * have for administration and record keeping purposes. 1638 */ 1639 public AdministrativeGender getGender() { 1640 return this.gender == null ? null : this.gender.getValue(); 1641 } 1642 1643 /** 1644 * @param value Administrative Gender - the gender that the patient is 1645 * considered to have for administration and record keeping 1646 * purposes. 1647 */ 1648 public Patient setGender(AdministrativeGender value) { 1649 if (value == null) 1650 this.gender = null; 1651 else { 1652 if (this.gender == null) 1653 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1654 this.gender.setValue(value); 1655 } 1656 return this; 1657 } 1658 1659 /** 1660 * @return {@link #birthDate} (The date of birth for the individual.). This is 1661 * the underlying object with id, value and extensions. The accessor 1662 * "getBirthDate" gives direct access to the value 1663 */ 1664 public DateType getBirthDateElement() { 1665 if (this.birthDate == null) 1666 if (Configuration.errorOnAutoCreate()) 1667 throw new Error("Attempt to auto-create Patient.birthDate"); 1668 else if (Configuration.doAutoCreate()) 1669 this.birthDate = new DateType(); // bb 1670 return this.birthDate; 1671 } 1672 1673 public boolean hasBirthDateElement() { 1674 return this.birthDate != null && !this.birthDate.isEmpty(); 1675 } 1676 1677 public boolean hasBirthDate() { 1678 return this.birthDate != null && !this.birthDate.isEmpty(); 1679 } 1680 1681 /** 1682 * @param value {@link #birthDate} (The date of birth for the individual.). This 1683 * is the underlying object with id, value and extensions. The 1684 * accessor "getBirthDate" gives direct access to the value 1685 */ 1686 public Patient setBirthDateElement(DateType value) { 1687 this.birthDate = value; 1688 return this; 1689 } 1690 1691 /** 1692 * @return The date of birth for the individual. 1693 */ 1694 public Date getBirthDate() { 1695 return this.birthDate == null ? null : this.birthDate.getValue(); 1696 } 1697 1698 /** 1699 * @param value The date of birth for the individual. 1700 */ 1701 public Patient setBirthDate(Date value) { 1702 if (value == null) 1703 this.birthDate = null; 1704 else { 1705 if (this.birthDate == null) 1706 this.birthDate = new DateType(); 1707 this.birthDate.setValue(value); 1708 } 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1714 */ 1715 public Type getDeceased() { 1716 return this.deceased; 1717 } 1718 1719 /** 1720 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1721 */ 1722 public BooleanType getDeceasedBooleanType() throws FHIRException { 1723 if (!(this.deceased instanceof BooleanType)) 1724 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1725 + this.deceased.getClass().getName() + " was encountered"); 1726 return (BooleanType) this.deceased; 1727 } 1728 1729 public boolean hasDeceasedBooleanType() { 1730 return this.deceased instanceof BooleanType; 1731 } 1732 1733 /** 1734 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1735 */ 1736 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 1737 if (!(this.deceased instanceof DateTimeType)) 1738 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1739 + this.deceased.getClass().getName() + " was encountered"); 1740 return (DateTimeType) this.deceased; 1741 } 1742 1743 public boolean hasDeceasedDateTimeType() { 1744 return this.deceased instanceof DateTimeType; 1745 } 1746 1747 public boolean hasDeceased() { 1748 return this.deceased != null && !this.deceased.isEmpty(); 1749 } 1750 1751 /** 1752 * @param value {@link #deceased} (Indicates if the individual is deceased or 1753 * not.) 1754 */ 1755 public Patient setDeceased(Type value) { 1756 this.deceased = value; 1757 return this; 1758 } 1759 1760 /** 1761 * @return {@link #address} (Addresses for the individual.) 1762 */ 1763 public List<Address> getAddress() { 1764 if (this.address == null) 1765 this.address = new ArrayList<Address>(); 1766 return this.address; 1767 } 1768 1769 public boolean hasAddress() { 1770 if (this.address == null) 1771 return false; 1772 for (Address item : this.address) 1773 if (!item.isEmpty()) 1774 return true; 1775 return false; 1776 } 1777 1778 /** 1779 * @return {@link #address} (Addresses for the individual.) 1780 */ 1781 // syntactic sugar 1782 public Address addAddress() { // 3 1783 Address t = new Address(); 1784 if (this.address == null) 1785 this.address = new ArrayList<Address>(); 1786 this.address.add(t); 1787 return t; 1788 } 1789 1790 // syntactic sugar 1791 public Patient addAddress(Address t) { // 3 1792 if (t == null) 1793 return this; 1794 if (this.address == null) 1795 this.address = new ArrayList<Address>(); 1796 this.address.add(t); 1797 return this; 1798 } 1799 1800 /** 1801 * @return {@link #maritalStatus} (This field contains a patient's most recent 1802 * marital (civil) status.) 1803 */ 1804 public CodeableConcept getMaritalStatus() { 1805 if (this.maritalStatus == null) 1806 if (Configuration.errorOnAutoCreate()) 1807 throw new Error("Attempt to auto-create Patient.maritalStatus"); 1808 else if (Configuration.doAutoCreate()) 1809 this.maritalStatus = new CodeableConcept(); // cc 1810 return this.maritalStatus; 1811 } 1812 1813 public boolean hasMaritalStatus() { 1814 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #maritalStatus} (This field contains a patient's most 1819 * recent marital (civil) status.) 1820 */ 1821 public Patient setMaritalStatus(CodeableConcept value) { 1822 this.maritalStatus = value; 1823 return this; 1824 } 1825 1826 /** 1827 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 1828 * multiple or indicates the actual birth order.) 1829 */ 1830 public Type getMultipleBirth() { 1831 return this.multipleBirth; 1832 } 1833 1834 /** 1835 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 1836 * multiple or indicates the actual birth order.) 1837 */ 1838 public BooleanType getMultipleBirthBooleanType() throws FHIRException { 1839 if (!(this.multipleBirth instanceof BooleanType)) 1840 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1841 + this.multipleBirth.getClass().getName() + " was encountered"); 1842 return (BooleanType) this.multipleBirth; 1843 } 1844 1845 public boolean hasMultipleBirthBooleanType() { 1846 return this.multipleBirth instanceof BooleanType; 1847 } 1848 1849 /** 1850 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 1851 * multiple or indicates the actual birth order.) 1852 */ 1853 public IntegerType getMultipleBirthIntegerType() throws FHIRException { 1854 if (!(this.multipleBirth instanceof IntegerType)) 1855 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 1856 + this.multipleBirth.getClass().getName() + " was encountered"); 1857 return (IntegerType) this.multipleBirth; 1858 } 1859 1860 public boolean hasMultipleBirthIntegerType() { 1861 return this.multipleBirth instanceof IntegerType; 1862 } 1863 1864 public boolean hasMultipleBirth() { 1865 return this.multipleBirth != null && !this.multipleBirth.isEmpty(); 1866 } 1867 1868 /** 1869 * @param value {@link #multipleBirth} (Indicates whether the patient is part of 1870 * a multiple or indicates the actual birth order.) 1871 */ 1872 public Patient setMultipleBirth(Type value) { 1873 this.multipleBirth = value; 1874 return this; 1875 } 1876 1877 /** 1878 * @return {@link #photo} (Image of the patient.) 1879 */ 1880 public List<Attachment> getPhoto() { 1881 if (this.photo == null) 1882 this.photo = new ArrayList<Attachment>(); 1883 return this.photo; 1884 } 1885 1886 public boolean hasPhoto() { 1887 if (this.photo == null) 1888 return false; 1889 for (Attachment item : this.photo) 1890 if (!item.isEmpty()) 1891 return true; 1892 return false; 1893 } 1894 1895 /** 1896 * @return {@link #photo} (Image of the patient.) 1897 */ 1898 // syntactic sugar 1899 public Attachment addPhoto() { // 3 1900 Attachment t = new Attachment(); 1901 if (this.photo == null) 1902 this.photo = new ArrayList<Attachment>(); 1903 this.photo.add(t); 1904 return t; 1905 } 1906 1907 // syntactic sugar 1908 public Patient addPhoto(Attachment t) { // 3 1909 if (t == null) 1910 return this; 1911 if (this.photo == null) 1912 this.photo = new ArrayList<Attachment>(); 1913 this.photo.add(t); 1914 return this; 1915 } 1916 1917 /** 1918 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) 1919 * for the patient.) 1920 */ 1921 public List<ContactComponent> getContact() { 1922 if (this.contact == null) 1923 this.contact = new ArrayList<ContactComponent>(); 1924 return this.contact; 1925 } 1926 1927 public boolean hasContact() { 1928 if (this.contact == null) 1929 return false; 1930 for (ContactComponent item : this.contact) 1931 if (!item.isEmpty()) 1932 return true; 1933 return false; 1934 } 1935 1936 /** 1937 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) 1938 * for the patient.) 1939 */ 1940 // syntactic sugar 1941 public ContactComponent addContact() { // 3 1942 ContactComponent t = new ContactComponent(); 1943 if (this.contact == null) 1944 this.contact = new ArrayList<ContactComponent>(); 1945 this.contact.add(t); 1946 return t; 1947 } 1948 1949 // syntactic sugar 1950 public Patient addContact(ContactComponent t) { // 3 1951 if (t == null) 1952 return this; 1953 if (this.contact == null) 1954 this.contact = new ArrayList<ContactComponent>(); 1955 this.contact.add(t); 1956 return this; 1957 } 1958 1959 /** 1960 * @return {@link #animal} (This patient is known to be an animal.) 1961 */ 1962 public AnimalComponent getAnimal() { 1963 if (this.animal == null) 1964 if (Configuration.errorOnAutoCreate()) 1965 throw new Error("Attempt to auto-create Patient.animal"); 1966 else if (Configuration.doAutoCreate()) 1967 this.animal = new AnimalComponent(); // cc 1968 return this.animal; 1969 } 1970 1971 public boolean hasAnimal() { 1972 return this.animal != null && !this.animal.isEmpty(); 1973 } 1974 1975 /** 1976 * @param value {@link #animal} (This patient is known to be an animal.) 1977 */ 1978 public Patient setAnimal(AnimalComponent value) { 1979 this.animal = value; 1980 return this; 1981 } 1982 1983 /** 1984 * @return {@link #communication} (Languages which may be used to communicate 1985 * with the patient about his or her health.) 1986 */ 1987 public List<PatientCommunicationComponent> getCommunication() { 1988 if (this.communication == null) 1989 this.communication = new ArrayList<PatientCommunicationComponent>(); 1990 return this.communication; 1991 } 1992 1993 public boolean hasCommunication() { 1994 if (this.communication == null) 1995 return false; 1996 for (PatientCommunicationComponent item : this.communication) 1997 if (!item.isEmpty()) 1998 return true; 1999 return false; 2000 } 2001 2002 /** 2003 * @return {@link #communication} (Languages which may be used to communicate 2004 * with the patient about his or her health.) 2005 */ 2006 // syntactic sugar 2007 public PatientCommunicationComponent addCommunication() { // 3 2008 PatientCommunicationComponent t = new PatientCommunicationComponent(); 2009 if (this.communication == null) 2010 this.communication = new ArrayList<PatientCommunicationComponent>(); 2011 this.communication.add(t); 2012 return t; 2013 } 2014 2015 // syntactic sugar 2016 public Patient addCommunication(PatientCommunicationComponent t) { // 3 2017 if (t == null) 2018 return this; 2019 if (this.communication == null) 2020 this.communication = new ArrayList<PatientCommunicationComponent>(); 2021 this.communication.add(t); 2022 return this; 2023 } 2024 2025 /** 2026 * @return {@link #careProvider} (Patient's nominated care provider.) 2027 */ 2028 public List<Reference> getCareProvider() { 2029 if (this.careProvider == null) 2030 this.careProvider = new ArrayList<Reference>(); 2031 return this.careProvider; 2032 } 2033 2034 public boolean hasCareProvider() { 2035 if (this.careProvider == null) 2036 return false; 2037 for (Reference item : this.careProvider) 2038 if (!item.isEmpty()) 2039 return true; 2040 return false; 2041 } 2042 2043 /** 2044 * @return {@link #careProvider} (Patient's nominated care provider.) 2045 */ 2046 // syntactic sugar 2047 public Reference addCareProvider() { // 3 2048 Reference t = new Reference(); 2049 if (this.careProvider == null) 2050 this.careProvider = new ArrayList<Reference>(); 2051 this.careProvider.add(t); 2052 return t; 2053 } 2054 2055 // syntactic sugar 2056 public Patient addCareProvider(Reference t) { // 3 2057 if (t == null) 2058 return this; 2059 if (this.careProvider == null) 2060 this.careProvider = new ArrayList<Reference>(); 2061 this.careProvider.add(t); 2062 return this; 2063 } 2064 2065 /** 2066 * @return {@link #careProvider} (The actual objects that are the target of the 2067 * reference. The reference library doesn't populate this, but you can 2068 * use this to hold the resources if you resolvethemt. Patient's 2069 * nominated care provider.) 2070 */ 2071 public List<Resource> getCareProviderTarget() { 2072 if (this.careProviderTarget == null) 2073 this.careProviderTarget = new ArrayList<Resource>(); 2074 return this.careProviderTarget; 2075 } 2076 2077 /** 2078 * @return {@link #managingOrganization} (Organization that is the custodian of 2079 * the patient record.) 2080 */ 2081 public Reference getManagingOrganization() { 2082 if (this.managingOrganization == null) 2083 if (Configuration.errorOnAutoCreate()) 2084 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2085 else if (Configuration.doAutoCreate()) 2086 this.managingOrganization = new Reference(); // cc 2087 return this.managingOrganization; 2088 } 2089 2090 public boolean hasManagingOrganization() { 2091 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2092 } 2093 2094 /** 2095 * @param value {@link #managingOrganization} (Organization that is the 2096 * custodian of the patient record.) 2097 */ 2098 public Patient setManagingOrganization(Reference value) { 2099 this.managingOrganization = value; 2100 return this; 2101 } 2102 2103 /** 2104 * @return {@link #managingOrganization} The actual object that is the target of 2105 * the reference. The reference library doesn't populate this, but you 2106 * can use it to hold the resource if you resolve it. (Organization that 2107 * is the custodian of the patient record.) 2108 */ 2109 public Organization getManagingOrganizationTarget() { 2110 if (this.managingOrganizationTarget == null) 2111 if (Configuration.errorOnAutoCreate()) 2112 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2113 else if (Configuration.doAutoCreate()) 2114 this.managingOrganizationTarget = new Organization(); // aa 2115 return this.managingOrganizationTarget; 2116 } 2117 2118 /** 2119 * @param value {@link #managingOrganization} The actual object that is the 2120 * target of the reference. The reference library doesn't use 2121 * these, but you can use it to hold the resource if you resolve 2122 * it. (Organization that is the custodian of the patient record.) 2123 */ 2124 public Patient setManagingOrganizationTarget(Organization value) { 2125 this.managingOrganizationTarget = value; 2126 return this; 2127 } 2128 2129 /** 2130 * @return {@link #link} (Link to another patient resource that concerns the 2131 * same actual patient.) 2132 */ 2133 public List<PatientLinkComponent> getLink() { 2134 if (this.link == null) 2135 this.link = new ArrayList<PatientLinkComponent>(); 2136 return this.link; 2137 } 2138 2139 public boolean hasLink() { 2140 if (this.link == null) 2141 return false; 2142 for (PatientLinkComponent item : this.link) 2143 if (!item.isEmpty()) 2144 return true; 2145 return false; 2146 } 2147 2148 /** 2149 * @return {@link #link} (Link to another patient resource that concerns the 2150 * same actual patient.) 2151 */ 2152 // syntactic sugar 2153 public PatientLinkComponent addLink() { // 3 2154 PatientLinkComponent t = new PatientLinkComponent(); 2155 if (this.link == null) 2156 this.link = new ArrayList<PatientLinkComponent>(); 2157 this.link.add(t); 2158 return t; 2159 } 2160 2161 // syntactic sugar 2162 public Patient addLink(PatientLinkComponent t) { // 3 2163 if (t == null) 2164 return this; 2165 if (this.link == null) 2166 this.link = new ArrayList<PatientLinkComponent>(); 2167 this.link.add(t); 2168 return this; 2169 } 2170 2171 protected void listChildren(List<Property> childrenList) { 2172 super.listChildren(childrenList); 2173 childrenList.add(new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2174 java.lang.Integer.MAX_VALUE, identifier)); 2175 childrenList.add(new Property("active", "boolean", "Whether this patient record is in active use.", 0, 2176 java.lang.Integer.MAX_VALUE, active)); 2177 childrenList.add(new Property("name", "HumanName", "A name associated with the individual.", 0, 2178 java.lang.Integer.MAX_VALUE, name)); 2179 childrenList.add(new Property("telecom", "ContactPoint", 2180 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2181 java.lang.Integer.MAX_VALUE, telecom)); 2182 childrenList.add(new Property("gender", "code", 2183 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2184 0, java.lang.Integer.MAX_VALUE, gender)); 2185 childrenList.add(new Property("birthDate", "date", "The date of birth for the individual.", 0, 2186 java.lang.Integer.MAX_VALUE, birthDate)); 2187 childrenList.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 2188 0, java.lang.Integer.MAX_VALUE, deceased)); 2189 childrenList.add( 2190 new Property("address", "Address", "Addresses for the individual.", 0, java.lang.Integer.MAX_VALUE, address)); 2191 childrenList.add(new Property("maritalStatus", "CodeableConcept", 2192 "This field contains a patient's most recent marital (civil) status.", 0, java.lang.Integer.MAX_VALUE, 2193 maritalStatus)); 2194 childrenList.add(new Property("multipleBirth[x]", "boolean|integer", 2195 "Indicates whether the patient is part of a multiple or indicates the actual birth order.", 0, 2196 java.lang.Integer.MAX_VALUE, multipleBirth)); 2197 childrenList 2198 .add(new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo)); 2199 childrenList.add(new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, 2200 java.lang.Integer.MAX_VALUE, contact)); 2201 childrenList.add( 2202 new Property("animal", "", "This patient is known to be an animal.", 0, java.lang.Integer.MAX_VALUE, animal)); 2203 childrenList.add(new Property("communication", "", 2204 "Languages which may be used to communicate with the patient about his or her health.", 0, 2205 java.lang.Integer.MAX_VALUE, communication)); 2206 childrenList.add(new Property("careProvider", "Reference(Organization|Practitioner)", 2207 "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, careProvider)); 2208 childrenList.add(new Property("managingOrganization", "Reference(Organization)", 2209 "Organization that is the custodian of the patient record.", 0, java.lang.Integer.MAX_VALUE, 2210 managingOrganization)); 2211 childrenList.add(new Property("link", "", "Link to another patient resource that concerns the same actual patient.", 2212 0, java.lang.Integer.MAX_VALUE, link)); 2213 } 2214 2215 @Override 2216 public void setProperty(String name, Base value) throws FHIRException { 2217 if (name.equals("identifier")) 2218 this.getIdentifier().add(castToIdentifier(value)); 2219 else if (name.equals("active")) 2220 this.active = castToBoolean(value); // BooleanType 2221 else if (name.equals("name")) 2222 this.getName().add(castToHumanName(value)); 2223 else if (name.equals("telecom")) 2224 this.getTelecom().add(castToContactPoint(value)); 2225 else if (name.equals("gender")) 2226 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 2227 else if (name.equals("birthDate")) 2228 this.birthDate = castToDate(value); // DateType 2229 else if (name.equals("deceased[x]")) 2230 this.deceased = (Type) value; // Type 2231 else if (name.equals("address")) 2232 this.getAddress().add(castToAddress(value)); 2233 else if (name.equals("maritalStatus")) 2234 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2235 else if (name.equals("multipleBirth[x]")) 2236 this.multipleBirth = (Type) value; // Type 2237 else if (name.equals("photo")) 2238 this.getPhoto().add(castToAttachment(value)); 2239 else if (name.equals("contact")) 2240 this.getContact().add((ContactComponent) value); 2241 else if (name.equals("animal")) 2242 this.animal = (AnimalComponent) value; // AnimalComponent 2243 else if (name.equals("communication")) 2244 this.getCommunication().add((PatientCommunicationComponent) value); 2245 else if (name.equals("careProvider")) 2246 this.getCareProvider().add(castToReference(value)); 2247 else if (name.equals("managingOrganization")) 2248 this.managingOrganization = castToReference(value); // Reference 2249 else if (name.equals("link")) 2250 this.getLink().add((PatientLinkComponent) value); 2251 else 2252 super.setProperty(name, value); 2253 } 2254 2255 @Override 2256 public Base addChild(String name) throws FHIRException { 2257 if (name.equals("identifier")) { 2258 return addIdentifier(); 2259 } else if (name.equals("active")) { 2260 throw new FHIRException("Cannot call addChild on a singleton property Patient.active"); 2261 } else if (name.equals("name")) { 2262 return addName(); 2263 } else if (name.equals("telecom")) { 2264 return addTelecom(); 2265 } else if (name.equals("gender")) { 2266 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 2267 } else if (name.equals("birthDate")) { 2268 throw new FHIRException("Cannot call addChild on a singleton property Patient.birthDate"); 2269 } else if (name.equals("deceasedBoolean")) { 2270 this.deceased = new BooleanType(); 2271 return this.deceased; 2272 } else if (name.equals("deceasedDateTime")) { 2273 this.deceased = new DateTimeType(); 2274 return this.deceased; 2275 } else if (name.equals("address")) { 2276 return addAddress(); 2277 } else if (name.equals("maritalStatus")) { 2278 this.maritalStatus = new CodeableConcept(); 2279 return this.maritalStatus; 2280 } else if (name.equals("multipleBirthBoolean")) { 2281 this.multipleBirth = new BooleanType(); 2282 return this.multipleBirth; 2283 } else if (name.equals("multipleBirthInteger")) { 2284 this.multipleBirth = new IntegerType(); 2285 return this.multipleBirth; 2286 } else if (name.equals("photo")) { 2287 return addPhoto(); 2288 } else if (name.equals("contact")) { 2289 return addContact(); 2290 } else if (name.equals("animal")) { 2291 this.animal = new AnimalComponent(); 2292 return this.animal; 2293 } else if (name.equals("communication")) { 2294 return addCommunication(); 2295 } else if (name.equals("careProvider")) { 2296 return addCareProvider(); 2297 } else if (name.equals("managingOrganization")) { 2298 this.managingOrganization = new Reference(); 2299 return this.managingOrganization; 2300 } else if (name.equals("link")) { 2301 return addLink(); 2302 } else 2303 return super.addChild(name); 2304 } 2305 2306 public String fhirType() { 2307 return "Patient"; 2308 2309 } 2310 2311 public Patient copy() { 2312 Patient dst = new Patient(); 2313 copyValues(dst); 2314 if (identifier != null) { 2315 dst.identifier = new ArrayList<Identifier>(); 2316 for (Identifier i : identifier) 2317 dst.identifier.add(i.copy()); 2318 } 2319 ; 2320 dst.active = active == null ? null : active.copy(); 2321 if (name != null) { 2322 dst.name = new ArrayList<HumanName>(); 2323 for (HumanName i : name) 2324 dst.name.add(i.copy()); 2325 } 2326 ; 2327 if (telecom != null) { 2328 dst.telecom = new ArrayList<ContactPoint>(); 2329 for (ContactPoint i : telecom) 2330 dst.telecom.add(i.copy()); 2331 } 2332 ; 2333 dst.gender = gender == null ? null : gender.copy(); 2334 dst.birthDate = birthDate == null ? null : birthDate.copy(); 2335 dst.deceased = deceased == null ? null : deceased.copy(); 2336 if (address != null) { 2337 dst.address = new ArrayList<Address>(); 2338 for (Address i : address) 2339 dst.address.add(i.copy()); 2340 } 2341 ; 2342 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 2343 dst.multipleBirth = multipleBirth == null ? null : multipleBirth.copy(); 2344 if (photo != null) { 2345 dst.photo = new ArrayList<Attachment>(); 2346 for (Attachment i : photo) 2347 dst.photo.add(i.copy()); 2348 } 2349 ; 2350 if (contact != null) { 2351 dst.contact = new ArrayList<ContactComponent>(); 2352 for (ContactComponent i : contact) 2353 dst.contact.add(i.copy()); 2354 } 2355 ; 2356 dst.animal = animal == null ? null : animal.copy(); 2357 if (communication != null) { 2358 dst.communication = new ArrayList<PatientCommunicationComponent>(); 2359 for (PatientCommunicationComponent i : communication) 2360 dst.communication.add(i.copy()); 2361 } 2362 ; 2363 if (careProvider != null) { 2364 dst.careProvider = new ArrayList<Reference>(); 2365 for (Reference i : careProvider) 2366 dst.careProvider.add(i.copy()); 2367 } 2368 ; 2369 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 2370 if (link != null) { 2371 dst.link = new ArrayList<PatientLinkComponent>(); 2372 for (PatientLinkComponent i : link) 2373 dst.link.add(i.copy()); 2374 } 2375 ; 2376 return dst; 2377 } 2378 2379 protected Patient typedCopy() { 2380 return copy(); 2381 } 2382 2383 @Override 2384 public boolean equalsDeep(Base other) { 2385 if (!super.equalsDeep(other)) 2386 return false; 2387 if (!(other instanceof Patient)) 2388 return false; 2389 Patient o = (Patient) other; 2390 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 2391 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 2392 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 2393 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) 2394 && compareDeep(maritalStatus, o.maritalStatus, true) && compareDeep(multipleBirth, o.multipleBirth, true) 2395 && compareDeep(photo, o.photo, true) && compareDeep(contact, o.contact, true) 2396 && compareDeep(animal, o.animal, true) && compareDeep(communication, o.communication, true) 2397 && compareDeep(careProvider, o.careProvider, true) 2398 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(link, o.link, true); 2399 } 2400 2401 @Override 2402 public boolean equalsShallow(Base other) { 2403 if (!super.equalsShallow(other)) 2404 return false; 2405 if (!(other instanceof Patient)) 2406 return false; 2407 Patient o = (Patient) other; 2408 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 2409 && compareValues(birthDate, o.birthDate, true); 2410 } 2411 2412 public boolean isEmpty() { 2413 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (active == null || active.isEmpty()) 2414 && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()) 2415 && (gender == null || gender.isEmpty()) && (birthDate == null || birthDate.isEmpty()) 2416 && (deceased == null || deceased.isEmpty()) && (address == null || address.isEmpty()) 2417 && (maritalStatus == null || maritalStatus.isEmpty()) && (multipleBirth == null || multipleBirth.isEmpty()) 2418 && (photo == null || photo.isEmpty()) && (contact == null || contact.isEmpty()) 2419 && (animal == null || animal.isEmpty()) && (communication == null || communication.isEmpty()) 2420 && (careProvider == null || careProvider.isEmpty()) 2421 && (managingOrganization == null || managingOrganization.isEmpty()) && (link == null || link.isEmpty()); 2422 } 2423 2424 @Override 2425 public ResourceType getResourceType() { 2426 return ResourceType.Patient; 2427 } 2428 2429 @SearchParamDefinition(name = "birthdate", path = "Patient.birthDate", description = "The patient's date of birth", type = "date") 2430 public static final String SP_BIRTHDATE = "birthdate"; 2431 @SearchParamDefinition(name = "deceased", path = "Patient.deceased[x]", description = "This patient has been marked as deceased, or as a death date entered", type = "token") 2432 public static final String SP_DECEASED = "deceased"; 2433 @SearchParamDefinition(name = "address-state", path = "Patient.address.state", description = "A state specified in an address", type = "string") 2434 public static final String SP_ADDRESSSTATE = "address-state"; 2435 @SearchParamDefinition(name = "gender", path = "Patient.gender", description = "Gender of the patient", type = "token") 2436 public static final String SP_GENDER = "gender"; 2437 @SearchParamDefinition(name = "animal-species", path = "Patient.animal.species", description = "The species for animal patients", type = "token") 2438 public static final String SP_ANIMALSPECIES = "animal-species"; 2439 @SearchParamDefinition(name = "link", path = "Patient.link.other", description = "All patients linked to the given patient", type = "reference") 2440 public static final String SP_LINK = "link"; 2441 @SearchParamDefinition(name = "language", path = "Patient.communication.language", description = "Language code (irrespective of use value)", type = "token") 2442 public static final String SP_LANGUAGE = "language"; 2443 @SearchParamDefinition(name = "deathdate", path = "Patient.deceasedDateTime", description = "The date of death has been provided and satisfies this search value", type = "date") 2444 public static final String SP_DEATHDATE = "deathdate"; 2445 @SearchParamDefinition(name = "animal-breed", path = "Patient.animal.breed", description = "The breed for animal patients", type = "token") 2446 public static final String SP_ANIMALBREED = "animal-breed"; 2447 @SearchParamDefinition(name = "address-country", path = "Patient.address.country", description = "A country specified in an address", type = "string") 2448 public static final String SP_ADDRESSCOUNTRY = "address-country"; 2449 @SearchParamDefinition(name = "phonetic", path = "Patient.name", description = "A portion of either family or given name using some kind of phonetic matching algorithm", type = "string") 2450 public static final String SP_PHONETIC = "phonetic"; 2451 @SearchParamDefinition(name = "telecom", path = "Patient.telecom", description = "The value in any kind of telecom details of the patient", type = "token") 2452 public static final String SP_TELECOM = "telecom"; 2453 @SearchParamDefinition(name = "address-city", path = "Patient.address.city", description = "A city specified in an address", type = "string") 2454 public static final String SP_ADDRESSCITY = "address-city"; 2455 @SearchParamDefinition(name = "email", path = "Patient.telecom.where(system='email')", description = "A value in an email contact", type = "token") 2456 public static final String SP_EMAIL = "email"; 2457 @SearchParamDefinition(name = "identifier", path = "Patient.identifier", description = "A patient identifier", type = "token") 2458 public static final String SP_IDENTIFIER = "identifier"; 2459 @SearchParamDefinition(name = "given", path = "Patient.name.given", description = "A portion of the given name of the patient", type = "string") 2460 public static final String SP_GIVEN = "given"; 2461 @SearchParamDefinition(name = "address", path = "Patient.address", description = "An address in any kind of address/part of the patient", type = "string") 2462 public static final String SP_ADDRESS = "address"; 2463 @SearchParamDefinition(name = "active", path = "Patient.active", description = "Whether the patient record is active", type = "token") 2464 public static final String SP_ACTIVE = "active"; 2465 @SearchParamDefinition(name = "address-postalcode", path = "Patient.address.postalCode", description = "A postalCode specified in an address", type = "string") 2466 public static final String SP_ADDRESSPOSTALCODE = "address-postalcode"; 2467 @SearchParamDefinition(name = "careprovider", path = "Patient.careProvider", description = "Patient's nominated care provider, could be a care manager, not the organization that manages the record", type = "reference") 2468 public static final String SP_CAREPROVIDER = "careprovider"; 2469 @SearchParamDefinition(name = "phone", path = "Patient.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 2470 public static final String SP_PHONE = "phone"; 2471 @SearchParamDefinition(name = "organization", path = "Patient.managingOrganization", description = "The organization at which this person is a patient", type = "reference") 2472 public static final String SP_ORGANIZATION = "organization"; 2473 @SearchParamDefinition(name = "name", path = "Patient.name", description = "A portion of either family or given name of the patient", type = "string") 2474 public static final String SP_NAME = "name"; 2475 @SearchParamDefinition(name = "address-use", path = "Patient.address.use", description = "A use code specified in an address", type = "token") 2476 public static final String SP_ADDRESSUSE = "address-use"; 2477 @SearchParamDefinition(name = "family", path = "Patient.name.family", description = "A portion of the family name of the patient", type = "string") 2478 public static final String SP_FAMILY = "family"; 2479 2480}