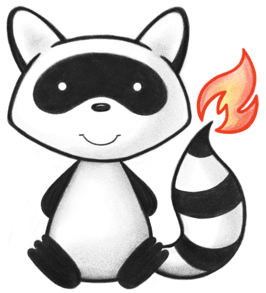
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender; 038import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046 047/** 048 * Demographics and other administrative information about an individual or 049 * animal receiving care or other health-related services. 050 */ 051@ResourceDef(name = "Patient", profile = "http://hl7.org/fhir/Profile/Patient") 052public class Patient extends DomainResource { 053 054 public enum LinkType { 055 /** 056 * The patient resource containing this link must no longer be used. The link 057 * points forward to another patient resource that must be used in lieu of the 058 * patient resource that contains this link. 059 */ 060 REPLACE, 061 /** 062 * The patient resource containing this link is in use and valid but not 063 * considered the main source of information about a patient. The link points 064 * forward to another patient resource that should be consulted to retrieve 065 * additional patient information. 066 */ 067 REFER, 068 /** 069 * The patient resource containing this link is in use and valid, but points to 070 * another patient resource that is known to contain data about the same person. 071 * Data in this resource might overlap or contradict information found in the 072 * other patient resource. This link does not indicate any relative importance 073 * of the resources concerned, and both should be regarded as equally valid. 074 */ 075 SEEALSO, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 081 public static LinkType fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("replace".equals(codeString)) 085 return REPLACE; 086 if ("refer".equals(codeString)) 087 return REFER; 088 if ("seealso".equals(codeString)) 089 return SEEALSO; 090 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 091 } 092 093 public String toCode() { 094 switch (this) { 095 case REPLACE: 096 return "replace"; 097 case REFER: 098 return "refer"; 099 case SEEALSO: 100 return "seealso"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 switch (this) { 110 case REPLACE: 111 return "http://hl7.org/fhir/link-type"; 112 case REFER: 113 return "http://hl7.org/fhir/link-type"; 114 case SEEALSO: 115 return "http://hl7.org/fhir/link-type"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case REPLACE: 126 return "The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link."; 127 case REFER: 128 return "The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information."; 129 case SEEALSO: 130 return "The patient resource containing this link is in use and valid, but points to another patient resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other patient resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid."; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDisplay() { 139 switch (this) { 140 case REPLACE: 141 return "Replace"; 142 case REFER: 143 return "Refer"; 144 case SEEALSO: 145 return "See also"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 } 153 154 public static class LinkTypeEnumFactory implements EnumFactory<LinkType> { 155 public LinkType fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("replace".equals(codeString)) 160 return LinkType.REPLACE; 161 if ("refer".equals(codeString)) 162 return LinkType.REFER; 163 if ("seealso".equals(codeString)) 164 return LinkType.SEEALSO; 165 throw new IllegalArgumentException("Unknown LinkType code '" + codeString + "'"); 166 } 167 168 public Enumeration<LinkType> fromType(Base code) throws FHIRException { 169 if (code == null || code.isEmpty()) 170 return null; 171 String codeString = ((PrimitiveType) code).asStringValue(); 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("replace".equals(codeString)) 175 return new Enumeration<LinkType>(this, LinkType.REPLACE); 176 if ("refer".equals(codeString)) 177 return new Enumeration<LinkType>(this, LinkType.REFER); 178 if ("seealso".equals(codeString)) 179 return new Enumeration<LinkType>(this, LinkType.SEEALSO); 180 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 181 } 182 183 public String toCode(LinkType code) { 184 if (code == LinkType.REPLACE) 185 return "replace"; 186 if (code == LinkType.REFER) 187 return "refer"; 188 if (code == LinkType.SEEALSO) 189 return "seealso"; 190 return "?"; 191 } 192 } 193 194 @Block() 195 public static class ContactComponent extends BackboneElement implements IBaseBackboneElement { 196 /** 197 * The nature of the relationship between the patient and the contact person. 198 */ 199 @Child(name = "relationship", type = { 200 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 201 @Description(shortDefinition = "The kind of relationship", formalDefinition = "The nature of the relationship between the patient and the contact person.") 202 protected List<CodeableConcept> relationship; 203 204 /** 205 * A name associated with the contact person. 206 */ 207 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 208 @Description(shortDefinition = "A name associated with the contact person", formalDefinition = "A name associated with the contact person.") 209 protected HumanName name; 210 211 /** 212 * A contact detail for the person, e.g. a telephone number or an email address. 213 */ 214 @Child(name = "telecom", type = { 215 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 216 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 217 protected List<ContactPoint> telecom; 218 219 /** 220 * Address for the contact person. 221 */ 222 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 223 @Description(shortDefinition = "Address for the contact person", formalDefinition = "Address for the contact person.") 224 protected Address address; 225 226 /** 227 * Administrative Gender - the gender that the contact person is considered to 228 * have for administration and record keeping purposes. 229 */ 230 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 231 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.") 232 protected Enumeration<AdministrativeGender> gender; 233 234 /** 235 * Organization on behalf of which the contact is acting or for which the 236 * contact is working. 237 */ 238 @Child(name = "organization", type = { 239 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 240 @Description(shortDefinition = "Organization that is associated with the contact", formalDefinition = "Organization on behalf of which the contact is acting or for which the contact is working.") 241 protected Reference organization; 242 243 /** 244 * The actual object that is the target of the reference (Organization on behalf 245 * of which the contact is acting or for which the contact is working.) 246 */ 247 protected Organization organizationTarget; 248 249 /** 250 * The period during which this contact person or organization is valid to be 251 * contacted relating to this patient. 252 */ 253 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 254 @Description(shortDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient", formalDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient.") 255 protected Period period; 256 257 private static final long serialVersionUID = 364269017L; 258 259 /* 260 * Constructor 261 */ 262 public ContactComponent() { 263 super(); 264 } 265 266 /** 267 * @return {@link #relationship} (The nature of the relationship between the 268 * patient and the contact person.) 269 */ 270 public List<CodeableConcept> getRelationship() { 271 if (this.relationship == null) 272 this.relationship = new ArrayList<CodeableConcept>(); 273 return this.relationship; 274 } 275 276 public boolean hasRelationship() { 277 if (this.relationship == null) 278 return false; 279 for (CodeableConcept item : this.relationship) 280 if (!item.isEmpty()) 281 return true; 282 return false; 283 } 284 285 /** 286 * @return {@link #relationship} (The nature of the relationship between the 287 * patient and the contact person.) 288 */ 289 // syntactic sugar 290 public CodeableConcept addRelationship() { // 3 291 CodeableConcept t = new CodeableConcept(); 292 if (this.relationship == null) 293 this.relationship = new ArrayList<CodeableConcept>(); 294 this.relationship.add(t); 295 return t; 296 } 297 298 // syntactic sugar 299 public ContactComponent addRelationship(CodeableConcept t) { // 3 300 if (t == null) 301 return this; 302 if (this.relationship == null) 303 this.relationship = new ArrayList<CodeableConcept>(); 304 this.relationship.add(t); 305 return this; 306 } 307 308 /** 309 * @return {@link #name} (A name associated with the contact person.) 310 */ 311 public HumanName getName() { 312 if (this.name == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create ContactComponent.name"); 315 else if (Configuration.doAutoCreate()) 316 this.name = new HumanName(); // cc 317 return this.name; 318 } 319 320 public boolean hasName() { 321 return this.name != null && !this.name.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #name} (A name associated with the contact person.) 326 */ 327 public ContactComponent setName(HumanName value) { 328 this.name = value; 329 return this; 330 } 331 332 /** 333 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 334 * number or an email address.) 335 */ 336 public List<ContactPoint> getTelecom() { 337 if (this.telecom == null) 338 this.telecom = new ArrayList<ContactPoint>(); 339 return this.telecom; 340 } 341 342 public boolean hasTelecom() { 343 if (this.telecom == null) 344 return false; 345 for (ContactPoint item : this.telecom) 346 if (!item.isEmpty()) 347 return true; 348 return false; 349 } 350 351 /** 352 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 353 * number or an email address.) 354 */ 355 // syntactic sugar 356 public ContactPoint addTelecom() { // 3 357 ContactPoint t = new ContactPoint(); 358 if (this.telecom == null) 359 this.telecom = new ArrayList<ContactPoint>(); 360 this.telecom.add(t); 361 return t; 362 } 363 364 // syntactic sugar 365 public ContactComponent addTelecom(ContactPoint t) { // 3 366 if (t == null) 367 return this; 368 if (this.telecom == null) 369 this.telecom = new ArrayList<ContactPoint>(); 370 this.telecom.add(t); 371 return this; 372 } 373 374 /** 375 * @return {@link #address} (Address for the contact person.) 376 */ 377 public Address getAddress() { 378 if (this.address == null) 379 if (Configuration.errorOnAutoCreate()) 380 throw new Error("Attempt to auto-create ContactComponent.address"); 381 else if (Configuration.doAutoCreate()) 382 this.address = new Address(); // cc 383 return this.address; 384 } 385 386 public boolean hasAddress() { 387 return this.address != null && !this.address.isEmpty(); 388 } 389 390 /** 391 * @param value {@link #address} (Address for the contact person.) 392 */ 393 public ContactComponent setAddress(Address value) { 394 this.address = value; 395 return this; 396 } 397 398 /** 399 * @return {@link #gender} (Administrative Gender - the gender that the contact 400 * person is considered to have for administration and record keeping 401 * purposes.). This is the underlying object with id, value and 402 * extensions. The accessor "getGender" gives direct access to the value 403 */ 404 public Enumeration<AdministrativeGender> getGenderElement() { 405 if (this.gender == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create ContactComponent.gender"); 408 else if (Configuration.doAutoCreate()) 409 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 410 return this.gender; 411 } 412 413 public boolean hasGenderElement() { 414 return this.gender != null && !this.gender.isEmpty(); 415 } 416 417 public boolean hasGender() { 418 return this.gender != null && !this.gender.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #gender} (Administrative Gender - the gender that the 423 * contact person is considered to have for administration and 424 * record keeping purposes.). This is the underlying object with 425 * id, value and extensions. The accessor "getGender" gives direct 426 * access to the value 427 */ 428 public ContactComponent setGenderElement(Enumeration<AdministrativeGender> value) { 429 this.gender = value; 430 return this; 431 } 432 433 /** 434 * @return Administrative Gender - the gender that the contact person is 435 * considered to have for administration and record keeping purposes. 436 */ 437 public AdministrativeGender getGender() { 438 return this.gender == null ? null : this.gender.getValue(); 439 } 440 441 /** 442 * @param value Administrative Gender - the gender that the contact person is 443 * considered to have for administration and record keeping 444 * purposes. 445 */ 446 public ContactComponent setGender(AdministrativeGender value) { 447 if (value == null) 448 this.gender = null; 449 else { 450 if (this.gender == null) 451 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 452 this.gender.setValue(value); 453 } 454 return this; 455 } 456 457 /** 458 * @return {@link #organization} (Organization on behalf of which the contact is 459 * acting or for which the contact is working.) 460 */ 461 public Reference getOrganization() { 462 if (this.organization == null) 463 if (Configuration.errorOnAutoCreate()) 464 throw new Error("Attempt to auto-create ContactComponent.organization"); 465 else if (Configuration.doAutoCreate()) 466 this.organization = new Reference(); // cc 467 return this.organization; 468 } 469 470 public boolean hasOrganization() { 471 return this.organization != null && !this.organization.isEmpty(); 472 } 473 474 /** 475 * @param value {@link #organization} (Organization on behalf of which the 476 * contact is acting or for which the contact is working.) 477 */ 478 public ContactComponent setOrganization(Reference value) { 479 this.organization = value; 480 return this; 481 } 482 483 /** 484 * @return {@link #organization} The actual object that is the target of the 485 * reference. The reference library doesn't populate this, but you can 486 * use it to hold the resource if you resolve it. (Organization on 487 * behalf of which the contact is acting or for which the contact is 488 * working.) 489 */ 490 public Organization getOrganizationTarget() { 491 if (this.organizationTarget == null) 492 if (Configuration.errorOnAutoCreate()) 493 throw new Error("Attempt to auto-create ContactComponent.organization"); 494 else if (Configuration.doAutoCreate()) 495 this.organizationTarget = new Organization(); // aa 496 return this.organizationTarget; 497 } 498 499 /** 500 * @param value {@link #organization} The actual object that is the target of 501 * the reference. The reference library doesn't use these, but you 502 * can use it to hold the resource if you resolve it. (Organization 503 * on behalf of which the contact is acting or for which the 504 * contact is working.) 505 */ 506 public ContactComponent setOrganizationTarget(Organization value) { 507 this.organizationTarget = value; 508 return this; 509 } 510 511 /** 512 * @return {@link #period} (The period during which this contact person or 513 * organization is valid to be contacted relating to this patient.) 514 */ 515 public Period getPeriod() { 516 if (this.period == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create ContactComponent.period"); 519 else if (Configuration.doAutoCreate()) 520 this.period = new Period(); // cc 521 return this.period; 522 } 523 524 public boolean hasPeriod() { 525 return this.period != null && !this.period.isEmpty(); 526 } 527 528 /** 529 * @param value {@link #period} (The period during which this contact person or 530 * organization is valid to be contacted relating to this patient.) 531 */ 532 public ContactComponent setPeriod(Period value) { 533 this.period = value; 534 return this; 535 } 536 537 protected void listChildren(List<Property> childrenList) { 538 super.listChildren(childrenList); 539 childrenList.add(new Property("relationship", "CodeableConcept", 540 "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, 541 relationship)); 542 childrenList.add(new Property("name", "HumanName", "A name associated with the contact person.", 0, 543 java.lang.Integer.MAX_VALUE, name)); 544 childrenList.add(new Property("telecom", "ContactPoint", 545 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 546 java.lang.Integer.MAX_VALUE, telecom)); 547 childrenList.add(new Property("address", "Address", "Address for the contact person.", 0, 548 java.lang.Integer.MAX_VALUE, address)); 549 childrenList.add(new Property("gender", "code", 550 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 551 0, java.lang.Integer.MAX_VALUE, gender)); 552 childrenList.add(new Property("organization", "Reference(Organization)", 553 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 554 java.lang.Integer.MAX_VALUE, organization)); 555 childrenList.add(new Property("period", "Period", 556 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 557 0, java.lang.Integer.MAX_VALUE, period)); 558 } 559 560 @Override 561 public void setProperty(String name, Base value) throws FHIRException { 562 if (name.equals("relationship")) 563 this.getRelationship().add(castToCodeableConcept(value)); 564 else if (name.equals("name")) 565 this.name = castToHumanName(value); // HumanName 566 else if (name.equals("telecom")) 567 this.getTelecom().add(castToContactPoint(value)); 568 else if (name.equals("address")) 569 this.address = castToAddress(value); // Address 570 else if (name.equals("gender")) 571 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 572 else if (name.equals("organization")) 573 this.organization = castToReference(value); // Reference 574 else if (name.equals("period")) 575 this.period = castToPeriod(value); // Period 576 else 577 super.setProperty(name, value); 578 } 579 580 @Override 581 public Base addChild(String name) throws FHIRException { 582 if (name.equals("relationship")) { 583 return addRelationship(); 584 } else if (name.equals("name")) { 585 this.name = new HumanName(); 586 return this.name; 587 } else if (name.equals("telecom")) { 588 return addTelecom(); 589 } else if (name.equals("address")) { 590 this.address = new Address(); 591 return this.address; 592 } else if (name.equals("gender")) { 593 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 594 } else if (name.equals("organization")) { 595 this.organization = new Reference(); 596 return this.organization; 597 } else if (name.equals("period")) { 598 this.period = new Period(); 599 return this.period; 600 } else 601 return super.addChild(name); 602 } 603 604 public ContactComponent copy() { 605 ContactComponent dst = new ContactComponent(); 606 copyValues(dst); 607 if (relationship != null) { 608 dst.relationship = new ArrayList<CodeableConcept>(); 609 for (CodeableConcept i : relationship) 610 dst.relationship.add(i.copy()); 611 } 612 ; 613 dst.name = name == null ? null : name.copy(); 614 if (telecom != null) { 615 dst.telecom = new ArrayList<ContactPoint>(); 616 for (ContactPoint i : telecom) 617 dst.telecom.add(i.copy()); 618 } 619 ; 620 dst.address = address == null ? null : address.copy(); 621 dst.gender = gender == null ? null : gender.copy(); 622 dst.organization = organization == null ? null : organization.copy(); 623 dst.period = period == null ? null : period.copy(); 624 return dst; 625 } 626 627 @Override 628 public boolean equalsDeep(Base other) { 629 if (!super.equalsDeep(other)) 630 return false; 631 if (!(other instanceof ContactComponent)) 632 return false; 633 ContactComponent o = (ContactComponent) other; 634 return compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) 635 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true) 636 && compareDeep(gender, o.gender, true) && compareDeep(organization, o.organization, true) 637 && compareDeep(period, o.period, true); 638 } 639 640 @Override 641 public boolean equalsShallow(Base other) { 642 if (!super.equalsShallow(other)) 643 return false; 644 if (!(other instanceof ContactComponent)) 645 return false; 646 ContactComponent o = (ContactComponent) other; 647 return compareValues(gender, o.gender, true); 648 } 649 650 public boolean isEmpty() { 651 return super.isEmpty() && (relationship == null || relationship.isEmpty()) && (name == null || name.isEmpty()) 652 && (telecom == null || telecom.isEmpty()) && (address == null || address.isEmpty()) 653 && (gender == null || gender.isEmpty()) && (organization == null || organization.isEmpty()) 654 && (period == null || period.isEmpty()); 655 } 656 657 public String fhirType() { 658 return "Patient.contact"; 659 660 } 661 662 } 663 664 @Block() 665 public static class AnimalComponent extends BackboneElement implements IBaseBackboneElement { 666 /** 667 * Identifies the high level taxonomic categorization of the kind of animal. 668 */ 669 @Child(name = "species", type = { 670 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 671 @Description(shortDefinition = "E.g. Dog, Cow", formalDefinition = "Identifies the high level taxonomic categorization of the kind of animal.") 672 protected CodeableConcept species; 673 674 /** 675 * Identifies the detailed categorization of the kind of animal. 676 */ 677 @Child(name = "breed", type = { 678 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 679 @Description(shortDefinition = "E.g. Poodle, Angus", formalDefinition = "Identifies the detailed categorization of the kind of animal.") 680 protected CodeableConcept breed; 681 682 /** 683 * Indicates the current state of the animal's reproductive organs. 684 */ 685 @Child(name = "genderStatus", type = { 686 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 687 @Description(shortDefinition = "E.g. Neutered, Intact", formalDefinition = "Indicates the current state of the animal's reproductive organs.") 688 protected CodeableConcept genderStatus; 689 690 private static final long serialVersionUID = -549738382L; 691 692 /* 693 * Constructor 694 */ 695 public AnimalComponent() { 696 super(); 697 } 698 699 /* 700 * Constructor 701 */ 702 public AnimalComponent(CodeableConcept species) { 703 super(); 704 this.species = species; 705 } 706 707 /** 708 * @return {@link #species} (Identifies the high level taxonomic categorization 709 * of the kind of animal.) 710 */ 711 public CodeableConcept getSpecies() { 712 if (this.species == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create AnimalComponent.species"); 715 else if (Configuration.doAutoCreate()) 716 this.species = new CodeableConcept(); // cc 717 return this.species; 718 } 719 720 public boolean hasSpecies() { 721 return this.species != null && !this.species.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #species} (Identifies the high level taxonomic 726 * categorization of the kind of animal.) 727 */ 728 public AnimalComponent setSpecies(CodeableConcept value) { 729 this.species = value; 730 return this; 731 } 732 733 /** 734 * @return {@link #breed} (Identifies the detailed categorization of the kind of 735 * animal.) 736 */ 737 public CodeableConcept getBreed() { 738 if (this.breed == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create AnimalComponent.breed"); 741 else if (Configuration.doAutoCreate()) 742 this.breed = new CodeableConcept(); // cc 743 return this.breed; 744 } 745 746 public boolean hasBreed() { 747 return this.breed != null && !this.breed.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #breed} (Identifies the detailed categorization of the 752 * kind of animal.) 753 */ 754 public AnimalComponent setBreed(CodeableConcept value) { 755 this.breed = value; 756 return this; 757 } 758 759 /** 760 * @return {@link #genderStatus} (Indicates the current state of the animal's 761 * reproductive organs.) 762 */ 763 public CodeableConcept getGenderStatus() { 764 if (this.genderStatus == null) 765 if (Configuration.errorOnAutoCreate()) 766 throw new Error("Attempt to auto-create AnimalComponent.genderStatus"); 767 else if (Configuration.doAutoCreate()) 768 this.genderStatus = new CodeableConcept(); // cc 769 return this.genderStatus; 770 } 771 772 public boolean hasGenderStatus() { 773 return this.genderStatus != null && !this.genderStatus.isEmpty(); 774 } 775 776 /** 777 * @param value {@link #genderStatus} (Indicates the current state of the 778 * animal's reproductive organs.) 779 */ 780 public AnimalComponent setGenderStatus(CodeableConcept value) { 781 this.genderStatus = value; 782 return this; 783 } 784 785 protected void listChildren(List<Property> childrenList) { 786 super.listChildren(childrenList); 787 childrenList.add(new Property("species", "CodeableConcept", 788 "Identifies the high level taxonomic categorization of the kind of animal.", 0, java.lang.Integer.MAX_VALUE, 789 species)); 790 childrenList.add(new Property("breed", "CodeableConcept", 791 "Identifies the detailed categorization of the kind of animal.", 0, java.lang.Integer.MAX_VALUE, breed)); 792 childrenList.add(new Property("genderStatus", "CodeableConcept", 793 "Indicates the current state of the animal's reproductive organs.", 0, java.lang.Integer.MAX_VALUE, 794 genderStatus)); 795 } 796 797 @Override 798 public void setProperty(String name, Base value) throws FHIRException { 799 if (name.equals("species")) 800 this.species = castToCodeableConcept(value); // CodeableConcept 801 else if (name.equals("breed")) 802 this.breed = castToCodeableConcept(value); // CodeableConcept 803 else if (name.equals("genderStatus")) 804 this.genderStatus = castToCodeableConcept(value); // CodeableConcept 805 else 806 super.setProperty(name, value); 807 } 808 809 @Override 810 public Base addChild(String name) throws FHIRException { 811 if (name.equals("species")) { 812 this.species = new CodeableConcept(); 813 return this.species; 814 } else if (name.equals("breed")) { 815 this.breed = new CodeableConcept(); 816 return this.breed; 817 } else if (name.equals("genderStatus")) { 818 this.genderStatus = new CodeableConcept(); 819 return this.genderStatus; 820 } else 821 return super.addChild(name); 822 } 823 824 public AnimalComponent copy() { 825 AnimalComponent dst = new AnimalComponent(); 826 copyValues(dst); 827 dst.species = species == null ? null : species.copy(); 828 dst.breed = breed == null ? null : breed.copy(); 829 dst.genderStatus = genderStatus == null ? null : genderStatus.copy(); 830 return dst; 831 } 832 833 @Override 834 public boolean equalsDeep(Base other) { 835 if (!super.equalsDeep(other)) 836 return false; 837 if (!(other instanceof AnimalComponent)) 838 return false; 839 AnimalComponent o = (AnimalComponent) other; 840 return compareDeep(species, o.species, true) && compareDeep(breed, o.breed, true) 841 && compareDeep(genderStatus, o.genderStatus, true); 842 } 843 844 @Override 845 public boolean equalsShallow(Base other) { 846 if (!super.equalsShallow(other)) 847 return false; 848 if (!(other instanceof AnimalComponent)) 849 return false; 850 AnimalComponent o = (AnimalComponent) other; 851 return true; 852 } 853 854 public boolean isEmpty() { 855 return super.isEmpty() && (species == null || species.isEmpty()) && (breed == null || breed.isEmpty()) 856 && (genderStatus == null || genderStatus.isEmpty()); 857 } 858 859 public String fhirType() { 860 return "Patient.animal"; 861 862 } 863 864 } 865 866 @Block() 867 public static class PatientCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 868 /** 869 * The ISO-639-1 alpha 2 code in lower case for the language, optionally 870 * followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper 871 * case; e.g. "en" for English, or "en-US" for American English versus "en-EN" 872 * for England English. 873 */ 874 @Child(name = "language", type = { 875 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 876 @Description(shortDefinition = "The language which can be used to communicate with the patient about his or her health", formalDefinition = "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.") 877 protected CodeableConcept language; 878 879 /** 880 * Indicates whether or not the patient prefers this language (over other 881 * languages he masters up a certain level). 882 */ 883 @Child(name = "preferred", type = { 884 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 885 @Description(shortDefinition = "Language preference indicator", formalDefinition = "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).") 886 protected BooleanType preferred; 887 888 private static final long serialVersionUID = 633792918L; 889 890 /* 891 * Constructor 892 */ 893 public PatientCommunicationComponent() { 894 super(); 895 } 896 897 /* 898 * Constructor 899 */ 900 public PatientCommunicationComponent(CodeableConcept language) { 901 super(); 902 this.language = language; 903 } 904 905 /** 906 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the 907 * language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 908 * code for the region in upper case; e.g. "en" for English, or "en-US" 909 * for American English versus "en-EN" for England English.) 910 */ 911 public CodeableConcept getLanguage() { 912 if (this.language == null) 913 if (Configuration.errorOnAutoCreate()) 914 throw new Error("Attempt to auto-create PatientCommunicationComponent.language"); 915 else if (Configuration.doAutoCreate()) 916 this.language = new CodeableConcept(); // cc 917 return this.language; 918 } 919 920 public boolean hasLanguage() { 921 return this.language != null && !this.language.isEmpty(); 922 } 923 924 /** 925 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for 926 * the language, optionally followed by a hyphen and the ISO-3166-1 927 * alpha 2 code for the region in upper case; e.g. "en" for 928 * English, or "en-US" for American English versus "en-EN" for 929 * England English.) 930 */ 931 public PatientCommunicationComponent setLanguage(CodeableConcept value) { 932 this.language = value; 933 return this; 934 } 935 936 /** 937 * @return {@link #preferred} (Indicates whether or not the patient prefers this 938 * language (over other languages he masters up a certain level).). This 939 * is the underlying object with id, value and extensions. The accessor 940 * "getPreferred" gives direct access to the value 941 */ 942 public BooleanType getPreferredElement() { 943 if (this.preferred == null) 944 if (Configuration.errorOnAutoCreate()) 945 throw new Error("Attempt to auto-create PatientCommunicationComponent.preferred"); 946 else if (Configuration.doAutoCreate()) 947 this.preferred = new BooleanType(); // bb 948 return this.preferred; 949 } 950 951 public boolean hasPreferredElement() { 952 return this.preferred != null && !this.preferred.isEmpty(); 953 } 954 955 public boolean hasPreferred() { 956 return this.preferred != null && !this.preferred.isEmpty(); 957 } 958 959 /** 960 * @param value {@link #preferred} (Indicates whether or not the patient prefers 961 * this language (over other languages he masters up a certain 962 * level).). This is the underlying object with id, value and 963 * extensions. The accessor "getPreferred" gives direct access to 964 * the value 965 */ 966 public PatientCommunicationComponent setPreferredElement(BooleanType value) { 967 this.preferred = value; 968 return this; 969 } 970 971 /** 972 * @return Indicates whether or not the patient prefers this language (over 973 * other languages he masters up a certain level). 974 */ 975 public boolean getPreferred() { 976 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 977 } 978 979 /** 980 * @param value Indicates whether or not the patient prefers this language (over 981 * other languages he masters up a certain level). 982 */ 983 public PatientCommunicationComponent setPreferred(boolean value) { 984 if (this.preferred == null) 985 this.preferred = new BooleanType(); 986 this.preferred.setValue(value); 987 return this; 988 } 989 990 protected void listChildren(List<Property> childrenList) { 991 super.listChildren(childrenList); 992 childrenList.add(new Property("language", "CodeableConcept", 993 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 994 0, java.lang.Integer.MAX_VALUE, language)); 995 childrenList.add(new Property("preferred", "boolean", 996 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 997 0, java.lang.Integer.MAX_VALUE, preferred)); 998 } 999 1000 @Override 1001 public void setProperty(String name, Base value) throws FHIRException { 1002 if (name.equals("language")) 1003 this.language = castToCodeableConcept(value); // CodeableConcept 1004 else if (name.equals("preferred")) 1005 this.preferred = castToBoolean(value); // BooleanType 1006 else 1007 super.setProperty(name, value); 1008 } 1009 1010 @Override 1011 public Base addChild(String name) throws FHIRException { 1012 if (name.equals("language")) { 1013 this.language = new CodeableConcept(); 1014 return this.language; 1015 } else if (name.equals("preferred")) { 1016 throw new FHIRException("Cannot call addChild on a singleton property Patient.preferred"); 1017 } else 1018 return super.addChild(name); 1019 } 1020 1021 public PatientCommunicationComponent copy() { 1022 PatientCommunicationComponent dst = new PatientCommunicationComponent(); 1023 copyValues(dst); 1024 dst.language = language == null ? null : language.copy(); 1025 dst.preferred = preferred == null ? null : preferred.copy(); 1026 return dst; 1027 } 1028 1029 @Override 1030 public boolean equalsDeep(Base other) { 1031 if (!super.equalsDeep(other)) 1032 return false; 1033 if (!(other instanceof PatientCommunicationComponent)) 1034 return false; 1035 PatientCommunicationComponent o = (PatientCommunicationComponent) other; 1036 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 1037 } 1038 1039 @Override 1040 public boolean equalsShallow(Base other) { 1041 if (!super.equalsShallow(other)) 1042 return false; 1043 if (!(other instanceof PatientCommunicationComponent)) 1044 return false; 1045 PatientCommunicationComponent o = (PatientCommunicationComponent) other; 1046 return compareValues(preferred, o.preferred, true); 1047 } 1048 1049 public boolean isEmpty() { 1050 return super.isEmpty() && (language == null || language.isEmpty()) && (preferred == null || preferred.isEmpty()); 1051 } 1052 1053 public String fhirType() { 1054 return "Patient.communication"; 1055 1056 } 1057 1058 } 1059 1060 @Block() 1061 public static class PatientLinkComponent extends BackboneElement implements IBaseBackboneElement { 1062 /** 1063 * The other patient resource that the link refers to. 1064 */ 1065 @Child(name = "other", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = true, summary = false) 1066 @Description(shortDefinition = "The other patient resource that the link refers to", formalDefinition = "The other patient resource that the link refers to.") 1067 protected Reference other; 1068 1069 /** 1070 * The actual object that is the target of the reference (The other patient 1071 * resource that the link refers to.) 1072 */ 1073 protected Patient otherTarget; 1074 1075 /** 1076 * The type of link between this patient resource and another patient resource. 1077 */ 1078 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = false) 1079 @Description(shortDefinition = "replace | refer | seealso - type of link", formalDefinition = "The type of link between this patient resource and another patient resource.") 1080 protected Enumeration<LinkType> type; 1081 1082 private static final long serialVersionUID = -1942104050L; 1083 1084 /* 1085 * Constructor 1086 */ 1087 public PatientLinkComponent() { 1088 super(); 1089 } 1090 1091 /* 1092 * Constructor 1093 */ 1094 public PatientLinkComponent(Reference other, Enumeration<LinkType> type) { 1095 super(); 1096 this.other = other; 1097 this.type = type; 1098 } 1099 1100 /** 1101 * @return {@link #other} (The other patient resource that the link refers to.) 1102 */ 1103 public Reference getOther() { 1104 if (this.other == null) 1105 if (Configuration.errorOnAutoCreate()) 1106 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1107 else if (Configuration.doAutoCreate()) 1108 this.other = new Reference(); // cc 1109 return this.other; 1110 } 1111 1112 public boolean hasOther() { 1113 return this.other != null && !this.other.isEmpty(); 1114 } 1115 1116 /** 1117 * @param value {@link #other} (The other patient resource that the link refers 1118 * to.) 1119 */ 1120 public PatientLinkComponent setOther(Reference value) { 1121 this.other = value; 1122 return this; 1123 } 1124 1125 /** 1126 * @return {@link #other} The actual object that is the target of the reference. 1127 * The reference library doesn't populate this, but you can use it to 1128 * hold the resource if you resolve it. (The other patient resource that 1129 * the link refers to.) 1130 */ 1131 public Patient getOtherTarget() { 1132 if (this.otherTarget == null) 1133 if (Configuration.errorOnAutoCreate()) 1134 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1135 else if (Configuration.doAutoCreate()) 1136 this.otherTarget = new Patient(); // aa 1137 return this.otherTarget; 1138 } 1139 1140 /** 1141 * @param value {@link #other} The actual object that is the target of the 1142 * reference. The reference library doesn't use these, but you can 1143 * use it to hold the resource if you resolve it. (The other 1144 * patient resource that the link refers to.) 1145 */ 1146 public PatientLinkComponent setOtherTarget(Patient value) { 1147 this.otherTarget = value; 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #type} (The type of link between this patient resource and 1153 * another patient resource.). This is the underlying object with id, 1154 * value and extensions. The accessor "getType" gives direct access to 1155 * the value 1156 */ 1157 public Enumeration<LinkType> getTypeElement() { 1158 if (this.type == null) 1159 if (Configuration.errorOnAutoCreate()) 1160 throw new Error("Attempt to auto-create PatientLinkComponent.type"); 1161 else if (Configuration.doAutoCreate()) 1162 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); // bb 1163 return this.type; 1164 } 1165 1166 public boolean hasTypeElement() { 1167 return this.type != null && !this.type.isEmpty(); 1168 } 1169 1170 public boolean hasType() { 1171 return this.type != null && !this.type.isEmpty(); 1172 } 1173 1174 /** 1175 * @param value {@link #type} (The type of link between this patient resource 1176 * and another patient resource.). This is the underlying object 1177 * with id, value and extensions. The accessor "getType" gives 1178 * direct access to the value 1179 */ 1180 public PatientLinkComponent setTypeElement(Enumeration<LinkType> value) { 1181 this.type = value; 1182 return this; 1183 } 1184 1185 /** 1186 * @return The type of link between this patient resource and another patient 1187 * resource. 1188 */ 1189 public LinkType getType() { 1190 return this.type == null ? null : this.type.getValue(); 1191 } 1192 1193 /** 1194 * @param value The type of link between this patient resource and another 1195 * patient resource. 1196 */ 1197 public PatientLinkComponent setType(LinkType value) { 1198 if (this.type == null) 1199 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); 1200 this.type.setValue(value); 1201 return this; 1202 } 1203 1204 protected void listChildren(List<Property> childrenList) { 1205 super.listChildren(childrenList); 1206 childrenList.add(new Property("other", "Reference(Patient)", 1207 "The other patient resource that the link refers to.", 0, java.lang.Integer.MAX_VALUE, other)); 1208 childrenList.add( 1209 new Property("type", "code", "The type of link between this patient resource and another patient resource.", 1210 0, java.lang.Integer.MAX_VALUE, type)); 1211 } 1212 1213 @Override 1214 public void setProperty(String name, Base value) throws FHIRException { 1215 if (name.equals("other")) 1216 this.other = castToReference(value); // Reference 1217 else if (name.equals("type")) 1218 this.type = new LinkTypeEnumFactory().fromType(value); // Enumeration<LinkType> 1219 else 1220 super.setProperty(name, value); 1221 } 1222 1223 @Override 1224 public Base addChild(String name) throws FHIRException { 1225 if (name.equals("other")) { 1226 this.other = new Reference(); 1227 return this.other; 1228 } else if (name.equals("type")) { 1229 throw new FHIRException("Cannot call addChild on a singleton property Patient.type"); 1230 } else 1231 return super.addChild(name); 1232 } 1233 1234 public PatientLinkComponent copy() { 1235 PatientLinkComponent dst = new PatientLinkComponent(); 1236 copyValues(dst); 1237 dst.other = other == null ? null : other.copy(); 1238 dst.type = type == null ? null : type.copy(); 1239 return dst; 1240 } 1241 1242 @Override 1243 public boolean equalsDeep(Base other) { 1244 if (!super.equalsDeep(other)) 1245 return false; 1246 if (!(other instanceof PatientLinkComponent)) 1247 return false; 1248 PatientLinkComponent o = (PatientLinkComponent) other; 1249 return compareDeep(other, o.other, true) && compareDeep(type, o.type, true); 1250 } 1251 1252 @Override 1253 public boolean equalsShallow(Base other) { 1254 if (!super.equalsShallow(other)) 1255 return false; 1256 if (!(other instanceof PatientLinkComponent)) 1257 return false; 1258 PatientLinkComponent o = (PatientLinkComponent) other; 1259 return compareValues(type, o.type, true); 1260 } 1261 1262 public boolean isEmpty() { 1263 return super.isEmpty() && (other == null || other.isEmpty()) && (type == null || type.isEmpty()); 1264 } 1265 1266 public String fhirType() { 1267 return "Patient.link"; 1268 1269 } 1270 1271 } 1272 1273 /** 1274 * An identifier for this patient. 1275 */ 1276 @Child(name = "identifier", type = { 1277 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1278 @Description(shortDefinition = "An identifier for this patient", formalDefinition = "An identifier for this patient.") 1279 protected List<Identifier> identifier; 1280 1281 /** 1282 * Whether this patient record is in active use. 1283 */ 1284 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1285 @Description(shortDefinition = "Whether this patient's record is in active use", formalDefinition = "Whether this patient record is in active use.") 1286 protected BooleanType active; 1287 1288 /** 1289 * A name associated with the individual. 1290 */ 1291 @Child(name = "name", type = { 1292 HumanName.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1293 @Description(shortDefinition = "A name associated with the patient", formalDefinition = "A name associated with the individual.") 1294 protected List<HumanName> name; 1295 1296 /** 1297 * A contact detail (e.g. a telephone number or an email address) by which the 1298 * individual may be contacted. 1299 */ 1300 @Child(name = "telecom", type = { 1301 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1302 @Description(shortDefinition = "A contact detail for the individual", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.") 1303 protected List<ContactPoint> telecom; 1304 1305 /** 1306 * Administrative Gender - the gender that the patient is considered to have for 1307 * administration and record keeping purposes. 1308 */ 1309 @Child(name = "gender", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1310 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.") 1311 protected Enumeration<AdministrativeGender> gender; 1312 1313 /** 1314 * The date of birth for the individual. 1315 */ 1316 @Child(name = "birthDate", type = { DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1317 @Description(shortDefinition = "The date of birth for the individual", formalDefinition = "The date of birth for the individual.") 1318 protected DateType birthDate; 1319 1320 /** 1321 * Indicates if the individual is deceased or not. 1322 */ 1323 @Child(name = "deceased", type = { BooleanType.class, 1324 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1325 @Description(shortDefinition = "Indicates if the individual is deceased or not", formalDefinition = "Indicates if the individual is deceased or not.") 1326 protected Type deceased; 1327 1328 /** 1329 * Addresses for the individual. 1330 */ 1331 @Child(name = "address", type = { 1332 Address.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1333 @Description(shortDefinition = "Addresses for the individual", formalDefinition = "Addresses for the individual.") 1334 protected List<Address> address; 1335 1336 /** 1337 * This field contains a patient's most recent marital (civil) status. 1338 */ 1339 @Child(name = "maritalStatus", type = { 1340 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1341 @Description(shortDefinition = "Marital (civil) status of a patient", formalDefinition = "This field contains a patient's most recent marital (civil) status.") 1342 protected CodeableConcept maritalStatus; 1343 1344 /** 1345 * Indicates whether the patient is part of a multiple or indicates the actual 1346 * birth order. 1347 */ 1348 @Child(name = "multipleBirth", type = { BooleanType.class, 1349 IntegerType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1350 @Description(shortDefinition = "Whether patient is part of a multiple birth", formalDefinition = "Indicates whether the patient is part of a multiple or indicates the actual birth order.") 1351 protected Type multipleBirth; 1352 1353 /** 1354 * Image of the patient. 1355 */ 1356 @Child(name = "photo", type = { 1357 Attachment.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1358 @Description(shortDefinition = "Image of the patient", formalDefinition = "Image of the patient.") 1359 protected List<Attachment> photo; 1360 1361 /** 1362 * A contact party (e.g. guardian, partner, friend) for the patient. 1363 */ 1364 @Child(name = "contact", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1365 @Description(shortDefinition = "A contact party (e.g. guardian, partner, friend) for the patient", formalDefinition = "A contact party (e.g. guardian, partner, friend) for the patient.") 1366 protected List<ContactComponent> contact; 1367 1368 /** 1369 * This patient is known to be an animal. 1370 */ 1371 @Child(name = "animal", type = {}, order = 12, min = 0, max = 1, modifier = true, summary = true) 1372 @Description(shortDefinition = "This patient is known to be an animal (non-human)", formalDefinition = "This patient is known to be an animal.") 1373 protected AnimalComponent animal; 1374 1375 /** 1376 * Languages which may be used to communicate with the patient about his or her 1377 * health. 1378 */ 1379 @Child(name = "communication", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1380 @Description(shortDefinition = "A list of Languages which may be used to communicate with the patient about his or her health", formalDefinition = "Languages which may be used to communicate with the patient about his or her health.") 1381 protected List<PatientCommunicationComponent> communication; 1382 1383 /** 1384 * Patient's nominated care provider. 1385 */ 1386 @Child(name = "careProvider", type = { Organization.class, 1387 Practitioner.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1388 @Description(shortDefinition = "Patient's nominated primary care provider", formalDefinition = "Patient's nominated care provider.") 1389 protected List<Reference> careProvider; 1390 /** 1391 * The actual objects that are the target of the reference (Patient's nominated 1392 * care provider.) 1393 */ 1394 protected List<Resource> careProviderTarget; 1395 1396 /** 1397 * Organization that is the custodian of the patient record. 1398 */ 1399 @Child(name = "managingOrganization", type = { 1400 Organization.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1401 @Description(shortDefinition = "Organization that is the custodian of the patient record", formalDefinition = "Organization that is the custodian of the patient record.") 1402 protected Reference managingOrganization; 1403 1404 /** 1405 * The actual object that is the target of the reference (Organization that is 1406 * the custodian of the patient record.) 1407 */ 1408 protected Organization managingOrganizationTarget; 1409 1410 /** 1411 * Link to another patient resource that concerns the same actual patient. 1412 */ 1413 @Child(name = "link", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = false) 1414 @Description(shortDefinition = "Link to another patient resource that concerns the same actual person", formalDefinition = "Link to another patient resource that concerns the same actual patient.") 1415 protected List<PatientLinkComponent> link; 1416 1417 private static final long serialVersionUID = 2019992554L; 1418 1419 /* 1420 * Constructor 1421 */ 1422 public Patient() { 1423 super(); 1424 } 1425 1426 /** 1427 * @return {@link #identifier} (An identifier for this patient.) 1428 */ 1429 public List<Identifier> getIdentifier() { 1430 if (this.identifier == null) 1431 this.identifier = new ArrayList<Identifier>(); 1432 return this.identifier; 1433 } 1434 1435 public boolean hasIdentifier() { 1436 if (this.identifier == null) 1437 return false; 1438 for (Identifier item : this.identifier) 1439 if (!item.isEmpty()) 1440 return true; 1441 return false; 1442 } 1443 1444 /** 1445 * @return {@link #identifier} (An identifier for this patient.) 1446 */ 1447 // syntactic sugar 1448 public Identifier addIdentifier() { // 3 1449 Identifier t = new Identifier(); 1450 if (this.identifier == null) 1451 this.identifier = new ArrayList<Identifier>(); 1452 this.identifier.add(t); 1453 return t; 1454 } 1455 1456 // syntactic sugar 1457 public Patient addIdentifier(Identifier t) { // 3 1458 if (t == null) 1459 return this; 1460 if (this.identifier == null) 1461 this.identifier = new ArrayList<Identifier>(); 1462 this.identifier.add(t); 1463 return this; 1464 } 1465 1466 /** 1467 * @return {@link #active} (Whether this patient record is in active use.). This 1468 * is the underlying object with id, value and extensions. The accessor 1469 * "getActive" gives direct access to the value 1470 */ 1471 public BooleanType getActiveElement() { 1472 if (this.active == null) 1473 if (Configuration.errorOnAutoCreate()) 1474 throw new Error("Attempt to auto-create Patient.active"); 1475 else if (Configuration.doAutoCreate()) 1476 this.active = new BooleanType(); // bb 1477 return this.active; 1478 } 1479 1480 public boolean hasActiveElement() { 1481 return this.active != null && !this.active.isEmpty(); 1482 } 1483 1484 public boolean hasActive() { 1485 return this.active != null && !this.active.isEmpty(); 1486 } 1487 1488 /** 1489 * @param value {@link #active} (Whether this patient record is in active use.). 1490 * This is the underlying object with id, value and extensions. The 1491 * accessor "getActive" gives direct access to the value 1492 */ 1493 public Patient setActiveElement(BooleanType value) { 1494 this.active = value; 1495 return this; 1496 } 1497 1498 /** 1499 * @return Whether this patient record is in active use. 1500 */ 1501 public boolean getActive() { 1502 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1503 } 1504 1505 /** 1506 * @param value Whether this patient record is in active use. 1507 */ 1508 public Patient setActive(boolean value) { 1509 if (this.active == null) 1510 this.active = new BooleanType(); 1511 this.active.setValue(value); 1512 return this; 1513 } 1514 1515 /** 1516 * @return {@link #name} (A name associated with the individual.) 1517 */ 1518 public List<HumanName> getName() { 1519 if (this.name == null) 1520 this.name = new ArrayList<HumanName>(); 1521 return this.name; 1522 } 1523 1524 public boolean hasName() { 1525 if (this.name == null) 1526 return false; 1527 for (HumanName item : this.name) 1528 if (!item.isEmpty()) 1529 return true; 1530 return false; 1531 } 1532 1533 /** 1534 * @return {@link #name} (A name associated with the individual.) 1535 */ 1536 // syntactic sugar 1537 public HumanName addName() { // 3 1538 HumanName t = new HumanName(); 1539 if (this.name == null) 1540 this.name = new ArrayList<HumanName>(); 1541 this.name.add(t); 1542 return t; 1543 } 1544 1545 // syntactic sugar 1546 public Patient addName(HumanName t) { // 3 1547 if (t == null) 1548 return this; 1549 if (this.name == null) 1550 this.name = new ArrayList<HumanName>(); 1551 this.name.add(t); 1552 return this; 1553 } 1554 1555 /** 1556 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 1557 * email address) by which the individual may be contacted.) 1558 */ 1559 public List<ContactPoint> getTelecom() { 1560 if (this.telecom == null) 1561 this.telecom = new ArrayList<ContactPoint>(); 1562 return this.telecom; 1563 } 1564 1565 public boolean hasTelecom() { 1566 if (this.telecom == null) 1567 return false; 1568 for (ContactPoint item : this.telecom) 1569 if (!item.isEmpty()) 1570 return true; 1571 return false; 1572 } 1573 1574 /** 1575 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 1576 * email address) by which the individual may be contacted.) 1577 */ 1578 // syntactic sugar 1579 public ContactPoint addTelecom() { // 3 1580 ContactPoint t = new ContactPoint(); 1581 if (this.telecom == null) 1582 this.telecom = new ArrayList<ContactPoint>(); 1583 this.telecom.add(t); 1584 return t; 1585 } 1586 1587 // syntactic sugar 1588 public Patient addTelecom(ContactPoint t) { // 3 1589 if (t == null) 1590 return this; 1591 if (this.telecom == null) 1592 this.telecom = new ArrayList<ContactPoint>(); 1593 this.telecom.add(t); 1594 return this; 1595 } 1596 1597 /** 1598 * @return {@link #gender} (Administrative Gender - the gender that the patient 1599 * is considered to have for administration and record keeping 1600 * purposes.). This is the underlying object with id, value and 1601 * extensions. The accessor "getGender" gives direct access to the value 1602 */ 1603 public Enumeration<AdministrativeGender> getGenderElement() { 1604 if (this.gender == null) 1605 if (Configuration.errorOnAutoCreate()) 1606 throw new Error("Attempt to auto-create Patient.gender"); 1607 else if (Configuration.doAutoCreate()) 1608 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1609 return this.gender; 1610 } 1611 1612 public boolean hasGenderElement() { 1613 return this.gender != null && !this.gender.isEmpty(); 1614 } 1615 1616 public boolean hasGender() { 1617 return this.gender != null && !this.gender.isEmpty(); 1618 } 1619 1620 /** 1621 * @param value {@link #gender} (Administrative Gender - the gender that the 1622 * patient is considered to have for administration and record 1623 * keeping purposes.). This is the underlying object with id, value 1624 * and extensions. The accessor "getGender" gives direct access to 1625 * the value 1626 */ 1627 public Patient setGenderElement(Enumeration<AdministrativeGender> value) { 1628 this.gender = value; 1629 return this; 1630 } 1631 1632 /** 1633 * @return Administrative Gender - the gender that the patient is considered to 1634 * have for administration and record keeping purposes. 1635 */ 1636 public AdministrativeGender getGender() { 1637 return this.gender == null ? null : this.gender.getValue(); 1638 } 1639 1640 /** 1641 * @param value Administrative Gender - the gender that the patient is 1642 * considered to have for administration and record keeping 1643 * purposes. 1644 */ 1645 public Patient setGender(AdministrativeGender value) { 1646 if (value == null) 1647 this.gender = null; 1648 else { 1649 if (this.gender == null) 1650 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1651 this.gender.setValue(value); 1652 } 1653 return this; 1654 } 1655 1656 /** 1657 * @return {@link #birthDate} (The date of birth for the individual.). This is 1658 * the underlying object with id, value and extensions. The accessor 1659 * "getBirthDate" gives direct access to the value 1660 */ 1661 public DateType getBirthDateElement() { 1662 if (this.birthDate == null) 1663 if (Configuration.errorOnAutoCreate()) 1664 throw new Error("Attempt to auto-create Patient.birthDate"); 1665 else if (Configuration.doAutoCreate()) 1666 this.birthDate = new DateType(); // bb 1667 return this.birthDate; 1668 } 1669 1670 public boolean hasBirthDateElement() { 1671 return this.birthDate != null && !this.birthDate.isEmpty(); 1672 } 1673 1674 public boolean hasBirthDate() { 1675 return this.birthDate != null && !this.birthDate.isEmpty(); 1676 } 1677 1678 /** 1679 * @param value {@link #birthDate} (The date of birth for the individual.). This 1680 * is the underlying object with id, value and extensions. The 1681 * accessor "getBirthDate" gives direct access to the value 1682 */ 1683 public Patient setBirthDateElement(DateType value) { 1684 this.birthDate = value; 1685 return this; 1686 } 1687 1688 /** 1689 * @return The date of birth for the individual. 1690 */ 1691 public Date getBirthDate() { 1692 return this.birthDate == null ? null : this.birthDate.getValue(); 1693 } 1694 1695 /** 1696 * @param value The date of birth for the individual. 1697 */ 1698 public Patient setBirthDate(Date value) { 1699 if (value == null) 1700 this.birthDate = null; 1701 else { 1702 if (this.birthDate == null) 1703 this.birthDate = new DateType(); 1704 this.birthDate.setValue(value); 1705 } 1706 return this; 1707 } 1708 1709 /** 1710 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1711 */ 1712 public Type getDeceased() { 1713 return this.deceased; 1714 } 1715 1716 /** 1717 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1718 */ 1719 public BooleanType getDeceasedBooleanType() throws FHIRException { 1720 if (!(this.deceased instanceof BooleanType)) 1721 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1722 + this.deceased.getClass().getName() + " was encountered"); 1723 return (BooleanType) this.deceased; 1724 } 1725 1726 public boolean hasDeceasedBooleanType() { 1727 return this.deceased instanceof BooleanType; 1728 } 1729 1730 /** 1731 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1732 */ 1733 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 1734 if (!(this.deceased instanceof DateTimeType)) 1735 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1736 + this.deceased.getClass().getName() + " was encountered"); 1737 return (DateTimeType) this.deceased; 1738 } 1739 1740 public boolean hasDeceasedDateTimeType() { 1741 return this.deceased instanceof DateTimeType; 1742 } 1743 1744 public boolean hasDeceased() { 1745 return this.deceased != null && !this.deceased.isEmpty(); 1746 } 1747 1748 /** 1749 * @param value {@link #deceased} (Indicates if the individual is deceased or 1750 * not.) 1751 */ 1752 public Patient setDeceased(Type value) { 1753 this.deceased = value; 1754 return this; 1755 } 1756 1757 /** 1758 * @return {@link #address} (Addresses for the individual.) 1759 */ 1760 public List<Address> getAddress() { 1761 if (this.address == null) 1762 this.address = new ArrayList<Address>(); 1763 return this.address; 1764 } 1765 1766 public boolean hasAddress() { 1767 if (this.address == null) 1768 return false; 1769 for (Address item : this.address) 1770 if (!item.isEmpty()) 1771 return true; 1772 return false; 1773 } 1774 1775 /** 1776 * @return {@link #address} (Addresses for the individual.) 1777 */ 1778 // syntactic sugar 1779 public Address addAddress() { // 3 1780 Address t = new Address(); 1781 if (this.address == null) 1782 this.address = new ArrayList<Address>(); 1783 this.address.add(t); 1784 return t; 1785 } 1786 1787 // syntactic sugar 1788 public Patient addAddress(Address t) { // 3 1789 if (t == null) 1790 return this; 1791 if (this.address == null) 1792 this.address = new ArrayList<Address>(); 1793 this.address.add(t); 1794 return this; 1795 } 1796 1797 /** 1798 * @return {@link #maritalStatus} (This field contains a patient's most recent 1799 * marital (civil) status.) 1800 */ 1801 public CodeableConcept getMaritalStatus() { 1802 if (this.maritalStatus == null) 1803 if (Configuration.errorOnAutoCreate()) 1804 throw new Error("Attempt to auto-create Patient.maritalStatus"); 1805 else if (Configuration.doAutoCreate()) 1806 this.maritalStatus = new CodeableConcept(); // cc 1807 return this.maritalStatus; 1808 } 1809 1810 public boolean hasMaritalStatus() { 1811 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 1812 } 1813 1814 /** 1815 * @param value {@link #maritalStatus} (This field contains a patient's most 1816 * recent marital (civil) status.) 1817 */ 1818 public Patient setMaritalStatus(CodeableConcept value) { 1819 this.maritalStatus = value; 1820 return this; 1821 } 1822 1823 /** 1824 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 1825 * multiple or indicates the actual birth order.) 1826 */ 1827 public Type getMultipleBirth() { 1828 return this.multipleBirth; 1829 } 1830 1831 /** 1832 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 1833 * multiple or indicates the actual birth order.) 1834 */ 1835 public BooleanType getMultipleBirthBooleanType() throws FHIRException { 1836 if (!(this.multipleBirth instanceof BooleanType)) 1837 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1838 + this.multipleBirth.getClass().getName() + " was encountered"); 1839 return (BooleanType) this.multipleBirth; 1840 } 1841 1842 public boolean hasMultipleBirthBooleanType() { 1843 return this.multipleBirth instanceof BooleanType; 1844 } 1845 1846 /** 1847 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 1848 * multiple or indicates the actual birth order.) 1849 */ 1850 public IntegerType getMultipleBirthIntegerType() throws FHIRException { 1851 if (!(this.multipleBirth instanceof IntegerType)) 1852 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 1853 + this.multipleBirth.getClass().getName() + " was encountered"); 1854 return (IntegerType) this.multipleBirth; 1855 } 1856 1857 public boolean hasMultipleBirthIntegerType() { 1858 return this.multipleBirth instanceof IntegerType; 1859 } 1860 1861 public boolean hasMultipleBirth() { 1862 return this.multipleBirth != null && !this.multipleBirth.isEmpty(); 1863 } 1864 1865 /** 1866 * @param value {@link #multipleBirth} (Indicates whether the patient is part of 1867 * a multiple or indicates the actual birth order.) 1868 */ 1869 public Patient setMultipleBirth(Type value) { 1870 this.multipleBirth = value; 1871 return this; 1872 } 1873 1874 /** 1875 * @return {@link #photo} (Image of the patient.) 1876 */ 1877 public List<Attachment> getPhoto() { 1878 if (this.photo == null) 1879 this.photo = new ArrayList<Attachment>(); 1880 return this.photo; 1881 } 1882 1883 public boolean hasPhoto() { 1884 if (this.photo == null) 1885 return false; 1886 for (Attachment item : this.photo) 1887 if (!item.isEmpty()) 1888 return true; 1889 return false; 1890 } 1891 1892 /** 1893 * @return {@link #photo} (Image of the patient.) 1894 */ 1895 // syntactic sugar 1896 public Attachment addPhoto() { // 3 1897 Attachment t = new Attachment(); 1898 if (this.photo == null) 1899 this.photo = new ArrayList<Attachment>(); 1900 this.photo.add(t); 1901 return t; 1902 } 1903 1904 // syntactic sugar 1905 public Patient addPhoto(Attachment t) { // 3 1906 if (t == null) 1907 return this; 1908 if (this.photo == null) 1909 this.photo = new ArrayList<Attachment>(); 1910 this.photo.add(t); 1911 return this; 1912 } 1913 1914 /** 1915 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) 1916 * for the patient.) 1917 */ 1918 public List<ContactComponent> getContact() { 1919 if (this.contact == null) 1920 this.contact = new ArrayList<ContactComponent>(); 1921 return this.contact; 1922 } 1923 1924 public boolean hasContact() { 1925 if (this.contact == null) 1926 return false; 1927 for (ContactComponent item : this.contact) 1928 if (!item.isEmpty()) 1929 return true; 1930 return false; 1931 } 1932 1933 /** 1934 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) 1935 * for the patient.) 1936 */ 1937 // syntactic sugar 1938 public ContactComponent addContact() { // 3 1939 ContactComponent t = new ContactComponent(); 1940 if (this.contact == null) 1941 this.contact = new ArrayList<ContactComponent>(); 1942 this.contact.add(t); 1943 return t; 1944 } 1945 1946 // syntactic sugar 1947 public Patient addContact(ContactComponent t) { // 3 1948 if (t == null) 1949 return this; 1950 if (this.contact == null) 1951 this.contact = new ArrayList<ContactComponent>(); 1952 this.contact.add(t); 1953 return this; 1954 } 1955 1956 /** 1957 * @return {@link #animal} (This patient is known to be an animal.) 1958 */ 1959 public AnimalComponent getAnimal() { 1960 if (this.animal == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create Patient.animal"); 1963 else if (Configuration.doAutoCreate()) 1964 this.animal = new AnimalComponent(); // cc 1965 return this.animal; 1966 } 1967 1968 public boolean hasAnimal() { 1969 return this.animal != null && !this.animal.isEmpty(); 1970 } 1971 1972 /** 1973 * @param value {@link #animal} (This patient is known to be an animal.) 1974 */ 1975 public Patient setAnimal(AnimalComponent value) { 1976 this.animal = value; 1977 return this; 1978 } 1979 1980 /** 1981 * @return {@link #communication} (Languages which may be used to communicate 1982 * with the patient about his or her health.) 1983 */ 1984 public List<PatientCommunicationComponent> getCommunication() { 1985 if (this.communication == null) 1986 this.communication = new ArrayList<PatientCommunicationComponent>(); 1987 return this.communication; 1988 } 1989 1990 public boolean hasCommunication() { 1991 if (this.communication == null) 1992 return false; 1993 for (PatientCommunicationComponent item : this.communication) 1994 if (!item.isEmpty()) 1995 return true; 1996 return false; 1997 } 1998 1999 /** 2000 * @return {@link #communication} (Languages which may be used to communicate 2001 * with the patient about his or her health.) 2002 */ 2003 // syntactic sugar 2004 public PatientCommunicationComponent addCommunication() { // 3 2005 PatientCommunicationComponent t = new PatientCommunicationComponent(); 2006 if (this.communication == null) 2007 this.communication = new ArrayList<PatientCommunicationComponent>(); 2008 this.communication.add(t); 2009 return t; 2010 } 2011 2012 // syntactic sugar 2013 public Patient addCommunication(PatientCommunicationComponent t) { // 3 2014 if (t == null) 2015 return this; 2016 if (this.communication == null) 2017 this.communication = new ArrayList<PatientCommunicationComponent>(); 2018 this.communication.add(t); 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #careProvider} (Patient's nominated care provider.) 2024 */ 2025 public List<Reference> getCareProvider() { 2026 if (this.careProvider == null) 2027 this.careProvider = new ArrayList<Reference>(); 2028 return this.careProvider; 2029 } 2030 2031 public boolean hasCareProvider() { 2032 if (this.careProvider == null) 2033 return false; 2034 for (Reference item : this.careProvider) 2035 if (!item.isEmpty()) 2036 return true; 2037 return false; 2038 } 2039 2040 /** 2041 * @return {@link #careProvider} (Patient's nominated care provider.) 2042 */ 2043 // syntactic sugar 2044 public Reference addCareProvider() { // 3 2045 Reference t = new Reference(); 2046 if (this.careProvider == null) 2047 this.careProvider = new ArrayList<Reference>(); 2048 this.careProvider.add(t); 2049 return t; 2050 } 2051 2052 // syntactic sugar 2053 public Patient addCareProvider(Reference t) { // 3 2054 if (t == null) 2055 return this; 2056 if (this.careProvider == null) 2057 this.careProvider = new ArrayList<Reference>(); 2058 this.careProvider.add(t); 2059 return this; 2060 } 2061 2062 /** 2063 * @return {@link #careProvider} (The actual objects that are the target of the 2064 * reference. The reference library doesn't populate this, but you can 2065 * use this to hold the resources if you resolvethemt. Patient's 2066 * nominated care provider.) 2067 */ 2068 public List<Resource> getCareProviderTarget() { 2069 if (this.careProviderTarget == null) 2070 this.careProviderTarget = new ArrayList<Resource>(); 2071 return this.careProviderTarget; 2072 } 2073 2074 /** 2075 * @return {@link #managingOrganization} (Organization that is the custodian of 2076 * the patient record.) 2077 */ 2078 public Reference getManagingOrganization() { 2079 if (this.managingOrganization == null) 2080 if (Configuration.errorOnAutoCreate()) 2081 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2082 else if (Configuration.doAutoCreate()) 2083 this.managingOrganization = new Reference(); // cc 2084 return this.managingOrganization; 2085 } 2086 2087 public boolean hasManagingOrganization() { 2088 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2089 } 2090 2091 /** 2092 * @param value {@link #managingOrganization} (Organization that is the 2093 * custodian of the patient record.) 2094 */ 2095 public Patient setManagingOrganization(Reference value) { 2096 this.managingOrganization = value; 2097 return this; 2098 } 2099 2100 /** 2101 * @return {@link #managingOrganization} The actual object that is the target of 2102 * the reference. The reference library doesn't populate this, but you 2103 * can use it to hold the resource if you resolve it. (Organization that 2104 * is the custodian of the patient record.) 2105 */ 2106 public Organization getManagingOrganizationTarget() { 2107 if (this.managingOrganizationTarget == null) 2108 if (Configuration.errorOnAutoCreate()) 2109 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2110 else if (Configuration.doAutoCreate()) 2111 this.managingOrganizationTarget = new Organization(); // aa 2112 return this.managingOrganizationTarget; 2113 } 2114 2115 /** 2116 * @param value {@link #managingOrganization} The actual object that is the 2117 * target of the reference. The reference library doesn't use 2118 * these, but you can use it to hold the resource if you resolve 2119 * it. (Organization that is the custodian of the patient record.) 2120 */ 2121 public Patient setManagingOrganizationTarget(Organization value) { 2122 this.managingOrganizationTarget = value; 2123 return this; 2124 } 2125 2126 /** 2127 * @return {@link #link} (Link to another patient resource that concerns the 2128 * same actual patient.) 2129 */ 2130 public List<PatientLinkComponent> getLink() { 2131 if (this.link == null) 2132 this.link = new ArrayList<PatientLinkComponent>(); 2133 return this.link; 2134 } 2135 2136 public boolean hasLink() { 2137 if (this.link == null) 2138 return false; 2139 for (PatientLinkComponent item : this.link) 2140 if (!item.isEmpty()) 2141 return true; 2142 return false; 2143 } 2144 2145 /** 2146 * @return {@link #link} (Link to another patient resource that concerns the 2147 * same actual patient.) 2148 */ 2149 // syntactic sugar 2150 public PatientLinkComponent addLink() { // 3 2151 PatientLinkComponent t = new PatientLinkComponent(); 2152 if (this.link == null) 2153 this.link = new ArrayList<PatientLinkComponent>(); 2154 this.link.add(t); 2155 return t; 2156 } 2157 2158 // syntactic sugar 2159 public Patient addLink(PatientLinkComponent t) { // 3 2160 if (t == null) 2161 return this; 2162 if (this.link == null) 2163 this.link = new ArrayList<PatientLinkComponent>(); 2164 this.link.add(t); 2165 return this; 2166 } 2167 2168 protected void listChildren(List<Property> childrenList) { 2169 super.listChildren(childrenList); 2170 childrenList.add(new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2171 java.lang.Integer.MAX_VALUE, identifier)); 2172 childrenList.add(new Property("active", "boolean", "Whether this patient record is in active use.", 0, 2173 java.lang.Integer.MAX_VALUE, active)); 2174 childrenList.add(new Property("name", "HumanName", "A name associated with the individual.", 0, 2175 java.lang.Integer.MAX_VALUE, name)); 2176 childrenList.add(new Property("telecom", "ContactPoint", 2177 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2178 java.lang.Integer.MAX_VALUE, telecom)); 2179 childrenList.add(new Property("gender", "code", 2180 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2181 0, java.lang.Integer.MAX_VALUE, gender)); 2182 childrenList.add(new Property("birthDate", "date", "The date of birth for the individual.", 0, 2183 java.lang.Integer.MAX_VALUE, birthDate)); 2184 childrenList.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 2185 0, java.lang.Integer.MAX_VALUE, deceased)); 2186 childrenList.add( 2187 new Property("address", "Address", "Addresses for the individual.", 0, java.lang.Integer.MAX_VALUE, address)); 2188 childrenList.add(new Property("maritalStatus", "CodeableConcept", 2189 "This field contains a patient's most recent marital (civil) status.", 0, java.lang.Integer.MAX_VALUE, 2190 maritalStatus)); 2191 childrenList.add(new Property("multipleBirth[x]", "boolean|integer", 2192 "Indicates whether the patient is part of a multiple or indicates the actual birth order.", 0, 2193 java.lang.Integer.MAX_VALUE, multipleBirth)); 2194 childrenList 2195 .add(new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo)); 2196 childrenList.add(new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, 2197 java.lang.Integer.MAX_VALUE, contact)); 2198 childrenList.add( 2199 new Property("animal", "", "This patient is known to be an animal.", 0, java.lang.Integer.MAX_VALUE, animal)); 2200 childrenList.add(new Property("communication", "", 2201 "Languages which may be used to communicate with the patient about his or her health.", 0, 2202 java.lang.Integer.MAX_VALUE, communication)); 2203 childrenList.add(new Property("careProvider", "Reference(Organization|Practitioner)", 2204 "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, careProvider)); 2205 childrenList.add(new Property("managingOrganization", "Reference(Organization)", 2206 "Organization that is the custodian of the patient record.", 0, java.lang.Integer.MAX_VALUE, 2207 managingOrganization)); 2208 childrenList.add(new Property("link", "", "Link to another patient resource that concerns the same actual patient.", 2209 0, java.lang.Integer.MAX_VALUE, link)); 2210 } 2211 2212 @Override 2213 public void setProperty(String name, Base value) throws FHIRException { 2214 if (name.equals("identifier")) 2215 this.getIdentifier().add(castToIdentifier(value)); 2216 else if (name.equals("active")) 2217 this.active = castToBoolean(value); // BooleanType 2218 else if (name.equals("name")) 2219 this.getName().add(castToHumanName(value)); 2220 else if (name.equals("telecom")) 2221 this.getTelecom().add(castToContactPoint(value)); 2222 else if (name.equals("gender")) 2223 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 2224 else if (name.equals("birthDate")) 2225 this.birthDate = castToDate(value); // DateType 2226 else if (name.equals("deceased[x]")) 2227 this.deceased = (Type) value; // Type 2228 else if (name.equals("address")) 2229 this.getAddress().add(castToAddress(value)); 2230 else if (name.equals("maritalStatus")) 2231 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2232 else if (name.equals("multipleBirth[x]")) 2233 this.multipleBirth = (Type) value; // Type 2234 else if (name.equals("photo")) 2235 this.getPhoto().add(castToAttachment(value)); 2236 else if (name.equals("contact")) 2237 this.getContact().add((ContactComponent) value); 2238 else if (name.equals("animal")) 2239 this.animal = (AnimalComponent) value; // AnimalComponent 2240 else if (name.equals("communication")) 2241 this.getCommunication().add((PatientCommunicationComponent) value); 2242 else if (name.equals("careProvider")) 2243 this.getCareProvider().add(castToReference(value)); 2244 else if (name.equals("managingOrganization")) 2245 this.managingOrganization = castToReference(value); // Reference 2246 else if (name.equals("link")) 2247 this.getLink().add((PatientLinkComponent) value); 2248 else 2249 super.setProperty(name, value); 2250 } 2251 2252 @Override 2253 public Base addChild(String name) throws FHIRException { 2254 if (name.equals("identifier")) { 2255 return addIdentifier(); 2256 } else if (name.equals("active")) { 2257 throw new FHIRException("Cannot call addChild on a singleton property Patient.active"); 2258 } else if (name.equals("name")) { 2259 return addName(); 2260 } else if (name.equals("telecom")) { 2261 return addTelecom(); 2262 } else if (name.equals("gender")) { 2263 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 2264 } else if (name.equals("birthDate")) { 2265 throw new FHIRException("Cannot call addChild on a singleton property Patient.birthDate"); 2266 } else if (name.equals("deceasedBoolean")) { 2267 this.deceased = new BooleanType(); 2268 return this.deceased; 2269 } else if (name.equals("deceasedDateTime")) { 2270 this.deceased = new DateTimeType(); 2271 return this.deceased; 2272 } else if (name.equals("address")) { 2273 return addAddress(); 2274 } else if (name.equals("maritalStatus")) { 2275 this.maritalStatus = new CodeableConcept(); 2276 return this.maritalStatus; 2277 } else if (name.equals("multipleBirthBoolean")) { 2278 this.multipleBirth = new BooleanType(); 2279 return this.multipleBirth; 2280 } else if (name.equals("multipleBirthInteger")) { 2281 this.multipleBirth = new IntegerType(); 2282 return this.multipleBirth; 2283 } else if (name.equals("photo")) { 2284 return addPhoto(); 2285 } else if (name.equals("contact")) { 2286 return addContact(); 2287 } else if (name.equals("animal")) { 2288 this.animal = new AnimalComponent(); 2289 return this.animal; 2290 } else if (name.equals("communication")) { 2291 return addCommunication(); 2292 } else if (name.equals("careProvider")) { 2293 return addCareProvider(); 2294 } else if (name.equals("managingOrganization")) { 2295 this.managingOrganization = new Reference(); 2296 return this.managingOrganization; 2297 } else if (name.equals("link")) { 2298 return addLink(); 2299 } else 2300 return super.addChild(name); 2301 } 2302 2303 public String fhirType() { 2304 return "Patient"; 2305 2306 } 2307 2308 public Patient copy() { 2309 Patient dst = new Patient(); 2310 copyValues(dst); 2311 if (identifier != null) { 2312 dst.identifier = new ArrayList<Identifier>(); 2313 for (Identifier i : identifier) 2314 dst.identifier.add(i.copy()); 2315 } 2316 ; 2317 dst.active = active == null ? null : active.copy(); 2318 if (name != null) { 2319 dst.name = new ArrayList<HumanName>(); 2320 for (HumanName i : name) 2321 dst.name.add(i.copy()); 2322 } 2323 ; 2324 if (telecom != null) { 2325 dst.telecom = new ArrayList<ContactPoint>(); 2326 for (ContactPoint i : telecom) 2327 dst.telecom.add(i.copy()); 2328 } 2329 ; 2330 dst.gender = gender == null ? null : gender.copy(); 2331 dst.birthDate = birthDate == null ? null : birthDate.copy(); 2332 dst.deceased = deceased == null ? null : deceased.copy(); 2333 if (address != null) { 2334 dst.address = new ArrayList<Address>(); 2335 for (Address i : address) 2336 dst.address.add(i.copy()); 2337 } 2338 ; 2339 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 2340 dst.multipleBirth = multipleBirth == null ? null : multipleBirth.copy(); 2341 if (photo != null) { 2342 dst.photo = new ArrayList<Attachment>(); 2343 for (Attachment i : photo) 2344 dst.photo.add(i.copy()); 2345 } 2346 ; 2347 if (contact != null) { 2348 dst.contact = new ArrayList<ContactComponent>(); 2349 for (ContactComponent i : contact) 2350 dst.contact.add(i.copy()); 2351 } 2352 ; 2353 dst.animal = animal == null ? null : animal.copy(); 2354 if (communication != null) { 2355 dst.communication = new ArrayList<PatientCommunicationComponent>(); 2356 for (PatientCommunicationComponent i : communication) 2357 dst.communication.add(i.copy()); 2358 } 2359 ; 2360 if (careProvider != null) { 2361 dst.careProvider = new ArrayList<Reference>(); 2362 for (Reference i : careProvider) 2363 dst.careProvider.add(i.copy()); 2364 } 2365 ; 2366 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 2367 if (link != null) { 2368 dst.link = new ArrayList<PatientLinkComponent>(); 2369 for (PatientLinkComponent i : link) 2370 dst.link.add(i.copy()); 2371 } 2372 ; 2373 return dst; 2374 } 2375 2376 protected Patient typedCopy() { 2377 return copy(); 2378 } 2379 2380 @Override 2381 public boolean equalsDeep(Base other) { 2382 if (!super.equalsDeep(other)) 2383 return false; 2384 if (!(other instanceof Patient)) 2385 return false; 2386 Patient o = (Patient) other; 2387 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 2388 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 2389 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 2390 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) 2391 && compareDeep(maritalStatus, o.maritalStatus, true) && compareDeep(multipleBirth, o.multipleBirth, true) 2392 && compareDeep(photo, o.photo, true) && compareDeep(contact, o.contact, true) 2393 && compareDeep(animal, o.animal, true) && compareDeep(communication, o.communication, true) 2394 && compareDeep(careProvider, o.careProvider, true) 2395 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(link, o.link, true); 2396 } 2397 2398 @Override 2399 public boolean equalsShallow(Base other) { 2400 if (!super.equalsShallow(other)) 2401 return false; 2402 if (!(other instanceof Patient)) 2403 return false; 2404 Patient o = (Patient) other; 2405 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 2406 && compareValues(birthDate, o.birthDate, true); 2407 } 2408 2409 public boolean isEmpty() { 2410 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (active == null || active.isEmpty()) 2411 && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()) 2412 && (gender == null || gender.isEmpty()) && (birthDate == null || birthDate.isEmpty()) 2413 && (deceased == null || deceased.isEmpty()) && (address == null || address.isEmpty()) 2414 && (maritalStatus == null || maritalStatus.isEmpty()) && (multipleBirth == null || multipleBirth.isEmpty()) 2415 && (photo == null || photo.isEmpty()) && (contact == null || contact.isEmpty()) 2416 && (animal == null || animal.isEmpty()) && (communication == null || communication.isEmpty()) 2417 && (careProvider == null || careProvider.isEmpty()) 2418 && (managingOrganization == null || managingOrganization.isEmpty()) && (link == null || link.isEmpty()); 2419 } 2420 2421 @Override 2422 public ResourceType getResourceType() { 2423 return ResourceType.Patient; 2424 } 2425 2426 @SearchParamDefinition(name = "birthdate", path = "Patient.birthDate", description = "The patient's date of birth", type = "date") 2427 public static final String SP_BIRTHDATE = "birthdate"; 2428 @SearchParamDefinition(name = "deceased", path = "Patient.deceased[x]", description = "This patient has been marked as deceased, or as a death date entered", type = "token") 2429 public static final String SP_DECEASED = "deceased"; 2430 @SearchParamDefinition(name = "address-state", path = "Patient.address.state", description = "A state specified in an address", type = "string") 2431 public static final String SP_ADDRESSSTATE = "address-state"; 2432 @SearchParamDefinition(name = "gender", path = "Patient.gender", description = "Gender of the patient", type = "token") 2433 public static final String SP_GENDER = "gender"; 2434 @SearchParamDefinition(name = "animal-species", path = "Patient.animal.species", description = "The species for animal patients", type = "token") 2435 public static final String SP_ANIMALSPECIES = "animal-species"; 2436 @SearchParamDefinition(name = "link", path = "Patient.link.other", description = "All patients linked to the given patient", type = "reference") 2437 public static final String SP_LINK = "link"; 2438 @SearchParamDefinition(name = "language", path = "Patient.communication.language", description = "Language code (irrespective of use value)", type = "token") 2439 public static final String SP_LANGUAGE = "language"; 2440 @SearchParamDefinition(name = "deathdate", path = "Patient.deceasedDateTime", description = "The date of death has been provided and satisfies this search value", type = "date") 2441 public static final String SP_DEATHDATE = "deathdate"; 2442 @SearchParamDefinition(name = "animal-breed", path = "Patient.animal.breed", description = "The breed for animal patients", type = "token") 2443 public static final String SP_ANIMALBREED = "animal-breed"; 2444 @SearchParamDefinition(name = "address-country", path = "Patient.address.country", description = "A country specified in an address", type = "string") 2445 public static final String SP_ADDRESSCOUNTRY = "address-country"; 2446 @SearchParamDefinition(name = "phonetic", path = "Patient.name", description = "A portion of either family or given name using some kind of phonetic matching algorithm", type = "string") 2447 public static final String SP_PHONETIC = "phonetic"; 2448 @SearchParamDefinition(name = "telecom", path = "Patient.telecom", description = "The value in any kind of telecom details of the patient", type = "token") 2449 public static final String SP_TELECOM = "telecom"; 2450 @SearchParamDefinition(name = "address-city", path = "Patient.address.city", description = "A city specified in an address", type = "string") 2451 public static final String SP_ADDRESSCITY = "address-city"; 2452 @SearchParamDefinition(name = "email", path = "Patient.telecom.where(system='email')", description = "A value in an email contact", type = "token") 2453 public static final String SP_EMAIL = "email"; 2454 @SearchParamDefinition(name = "identifier", path = "Patient.identifier", description = "A patient identifier", type = "token") 2455 public static final String SP_IDENTIFIER = "identifier"; 2456 @SearchParamDefinition(name = "given", path = "Patient.name.given", description = "A portion of the given name of the patient", type = "string") 2457 public static final String SP_GIVEN = "given"; 2458 @SearchParamDefinition(name = "address", path = "Patient.address", description = "An address in any kind of address/part of the patient", type = "string") 2459 public static final String SP_ADDRESS = "address"; 2460 @SearchParamDefinition(name = "active", path = "Patient.active", description = "Whether the patient record is active", type = "token") 2461 public static final String SP_ACTIVE = "active"; 2462 @SearchParamDefinition(name = "address-postalcode", path = "Patient.address.postalCode", description = "A postalCode specified in an address", type = "string") 2463 public static final String SP_ADDRESSPOSTALCODE = "address-postalcode"; 2464 @SearchParamDefinition(name = "careprovider", path = "Patient.careProvider", description = "Patient's nominated care provider, could be a care manager, not the organization that manages the record", type = "reference") 2465 public static final String SP_CAREPROVIDER = "careprovider"; 2466 @SearchParamDefinition(name = "phone", path = "Patient.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 2467 public static final String SP_PHONE = "phone"; 2468 @SearchParamDefinition(name = "organization", path = "Patient.managingOrganization", description = "The organization at which this person is a patient", type = "reference") 2469 public static final String SP_ORGANIZATION = "organization"; 2470 @SearchParamDefinition(name = "name", path = "Patient.name", description = "A portion of either family or given name of the patient", type = "string") 2471 public static final String SP_NAME = "name"; 2472 @SearchParamDefinition(name = "address-use", path = "Patient.address.use", description = "A use code specified in an address", type = "token") 2473 public static final String SP_ADDRESSUSE = "address-use"; 2474 @SearchParamDefinition(name = "family", path = "Patient.name.family", description = "A portion of the family name of the patient", type = "string") 2475 public static final String SP_FAMILY = "family"; 2476 2477}