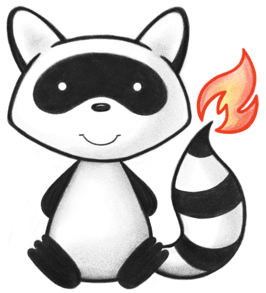
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * This resource provides the status of the payment for goods and services 045 * rendered, and the request and response resource references. 046 */ 047@ResourceDef(name = "PaymentNotice", profile = "http://hl7.org/fhir/Profile/PaymentNotice") 048public class PaymentNotice extends DomainResource { 049 050 /** 051 * The Response business identifier. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 055 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 056 protected List<Identifier> identifier; 057 058 /** 059 * The version of the style of resource contents. This should be mapped to the 060 * allowable profiles for this and supporting resources. 061 */ 062 @Child(name = "ruleset", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 064 protected Coding ruleset; 065 066 /** 067 * The style (standard) and version of the original material which was converted 068 * into this resource. 069 */ 070 @Child(name = "originalRuleset", type = { 071 Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 072 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 073 protected Coding originalRuleset; 074 075 /** 076 * The date when this resource was created. 077 */ 078 @Child(name = "created", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 079 @Description(shortDefinition = "Creation date", formalDefinition = "The date when this resource was created.") 080 protected DateTimeType created; 081 082 /** 083 * The Insurer who is target of the request. 084 */ 085 @Child(name = "target", type = { Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 086 @Description(shortDefinition = "Insurer or Regulatory body", formalDefinition = "The Insurer who is target of the request.") 087 protected Reference target; 088 089 /** 090 * The actual object that is the target of the reference (The Insurer who is 091 * target of the request.) 092 */ 093 protected Organization targetTarget; 094 095 /** 096 * The practitioner who is responsible for the services rendered to the patient. 097 */ 098 @Child(name = "provider", type = { 099 Practitioner.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 100 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 101 protected Reference provider; 102 103 /** 104 * The actual object that is the target of the reference (The practitioner who 105 * is responsible for the services rendered to the patient.) 106 */ 107 protected Practitioner providerTarget; 108 109 /** 110 * The organization which is responsible for the services rendered to the 111 * patient. 112 */ 113 @Child(name = "organization", type = { 114 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 115 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the services rendered to the patient.") 116 protected Reference organization; 117 118 /** 119 * The actual object that is the target of the reference (The organization which 120 * is responsible for the services rendered to the patient.) 121 */ 122 protected Organization organizationTarget; 123 124 /** 125 * Reference of resource to reverse. 126 */ 127 @Child(name = "request", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = true) 128 @Description(shortDefinition = "Request reference", formalDefinition = "Reference of resource to reverse.") 129 protected Reference request; 130 131 /** 132 * The actual object that is the target of the reference (Reference of resource 133 * to reverse.) 134 */ 135 protected Resource requestTarget; 136 137 /** 138 * Reference of response to resource to reverse. 139 */ 140 @Child(name = "response", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = true) 141 @Description(shortDefinition = "Response reference", formalDefinition = "Reference of response to resource to reverse.") 142 protected Reference response; 143 144 /** 145 * The actual object that is the target of the reference (Reference of response 146 * to resource to reverse.) 147 */ 148 protected Resource responseTarget; 149 150 /** 151 * The payment status, typically paid: payment sent, cleared: payment received. 152 */ 153 @Child(name = "paymentStatus", type = { Coding.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 154 @Description(shortDefinition = "Status of the payment", formalDefinition = "The payment status, typically paid: payment sent, cleared: payment received.") 155 protected Coding paymentStatus; 156 157 private static final long serialVersionUID = -394826458L; 158 159 /* 160 * Constructor 161 */ 162 public PaymentNotice() { 163 super(); 164 } 165 166 /* 167 * Constructor 168 */ 169 public PaymentNotice(Coding paymentStatus) { 170 super(); 171 this.paymentStatus = paymentStatus; 172 } 173 174 /** 175 * @return {@link #identifier} (The Response business identifier.) 176 */ 177 public List<Identifier> getIdentifier() { 178 if (this.identifier == null) 179 this.identifier = new ArrayList<Identifier>(); 180 return this.identifier; 181 } 182 183 public boolean hasIdentifier() { 184 if (this.identifier == null) 185 return false; 186 for (Identifier item : this.identifier) 187 if (!item.isEmpty()) 188 return true; 189 return false; 190 } 191 192 /** 193 * @return {@link #identifier} (The Response business identifier.) 194 */ 195 // syntactic sugar 196 public Identifier addIdentifier() { // 3 197 Identifier t = new Identifier(); 198 if (this.identifier == null) 199 this.identifier = new ArrayList<Identifier>(); 200 this.identifier.add(t); 201 return t; 202 } 203 204 // syntactic sugar 205 public PaymentNotice addIdentifier(Identifier t) { // 3 206 if (t == null) 207 return this; 208 if (this.identifier == null) 209 this.identifier = new ArrayList<Identifier>(); 210 this.identifier.add(t); 211 return this; 212 } 213 214 /** 215 * @return {@link #ruleset} (The version of the style of resource contents. This 216 * should be mapped to the allowable profiles for this and supporting 217 * resources.) 218 */ 219 public Coding getRuleset() { 220 if (this.ruleset == null) 221 if (Configuration.errorOnAutoCreate()) 222 throw new Error("Attempt to auto-create PaymentNotice.ruleset"); 223 else if (Configuration.doAutoCreate()) 224 this.ruleset = new Coding(); // cc 225 return this.ruleset; 226 } 227 228 public boolean hasRuleset() { 229 return this.ruleset != null && !this.ruleset.isEmpty(); 230 } 231 232 /** 233 * @param value {@link #ruleset} (The version of the style of resource contents. 234 * This should be mapped to the allowable profiles for this and 235 * supporting resources.) 236 */ 237 public PaymentNotice setRuleset(Coding value) { 238 this.ruleset = value; 239 return this; 240 } 241 242 /** 243 * @return {@link #originalRuleset} (The style (standard) and version of the 244 * original material which was converted into this resource.) 245 */ 246 public Coding getOriginalRuleset() { 247 if (this.originalRuleset == null) 248 if (Configuration.errorOnAutoCreate()) 249 throw new Error("Attempt to auto-create PaymentNotice.originalRuleset"); 250 else if (Configuration.doAutoCreate()) 251 this.originalRuleset = new Coding(); // cc 252 return this.originalRuleset; 253 } 254 255 public boolean hasOriginalRuleset() { 256 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 257 } 258 259 /** 260 * @param value {@link #originalRuleset} (The style (standard) and version of 261 * the original material which was converted into this resource.) 262 */ 263 public PaymentNotice setOriginalRuleset(Coding value) { 264 this.originalRuleset = value; 265 return this; 266 } 267 268 /** 269 * @return {@link #created} (The date when this resource was created.). This is 270 * the underlying object with id, value and extensions. The accessor 271 * "getCreated" gives direct access to the value 272 */ 273 public DateTimeType getCreatedElement() { 274 if (this.created == null) 275 if (Configuration.errorOnAutoCreate()) 276 throw new Error("Attempt to auto-create PaymentNotice.created"); 277 else if (Configuration.doAutoCreate()) 278 this.created = new DateTimeType(); // bb 279 return this.created; 280 } 281 282 public boolean hasCreatedElement() { 283 return this.created != null && !this.created.isEmpty(); 284 } 285 286 public boolean hasCreated() { 287 return this.created != null && !this.created.isEmpty(); 288 } 289 290 /** 291 * @param value {@link #created} (The date when this resource was created.). 292 * This is the underlying object with id, value and extensions. The 293 * accessor "getCreated" gives direct access to the value 294 */ 295 public PaymentNotice setCreatedElement(DateTimeType value) { 296 this.created = value; 297 return this; 298 } 299 300 /** 301 * @return The date when this resource was created. 302 */ 303 public Date getCreated() { 304 return this.created == null ? null : this.created.getValue(); 305 } 306 307 /** 308 * @param value The date when this resource was created. 309 */ 310 public PaymentNotice setCreated(Date value) { 311 if (value == null) 312 this.created = null; 313 else { 314 if (this.created == null) 315 this.created = new DateTimeType(); 316 this.created.setValue(value); 317 } 318 return this; 319 } 320 321 /** 322 * @return {@link #target} (The Insurer who is target of the request.) 323 */ 324 public Reference getTarget() { 325 if (this.target == null) 326 if (Configuration.errorOnAutoCreate()) 327 throw new Error("Attempt to auto-create PaymentNotice.target"); 328 else if (Configuration.doAutoCreate()) 329 this.target = new Reference(); // cc 330 return this.target; 331 } 332 333 public boolean hasTarget() { 334 return this.target != null && !this.target.isEmpty(); 335 } 336 337 /** 338 * @param value {@link #target} (The Insurer who is target of the request.) 339 */ 340 public PaymentNotice setTarget(Reference value) { 341 this.target = value; 342 return this; 343 } 344 345 /** 346 * @return {@link #target} The actual object that is the target of the 347 * reference. The reference library doesn't populate this, but you can 348 * use it to hold the resource if you resolve it. (The Insurer who is 349 * target of the request.) 350 */ 351 public Organization getTargetTarget() { 352 if (this.targetTarget == null) 353 if (Configuration.errorOnAutoCreate()) 354 throw new Error("Attempt to auto-create PaymentNotice.target"); 355 else if (Configuration.doAutoCreate()) 356 this.targetTarget = new Organization(); // aa 357 return this.targetTarget; 358 } 359 360 /** 361 * @param value {@link #target} The actual object that is the target of the 362 * reference. The reference library doesn't use these, but you can 363 * use it to hold the resource if you resolve it. (The Insurer who 364 * is target of the request.) 365 */ 366 public PaymentNotice setTargetTarget(Organization value) { 367 this.targetTarget = value; 368 return this; 369 } 370 371 /** 372 * @return {@link #provider} (The practitioner who is responsible for the 373 * services rendered to the patient.) 374 */ 375 public Reference getProvider() { 376 if (this.provider == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create PaymentNotice.provider"); 379 else if (Configuration.doAutoCreate()) 380 this.provider = new Reference(); // cc 381 return this.provider; 382 } 383 384 public boolean hasProvider() { 385 return this.provider != null && !this.provider.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #provider} (The practitioner who is responsible for the 390 * services rendered to the patient.) 391 */ 392 public PaymentNotice setProvider(Reference value) { 393 this.provider = value; 394 return this; 395 } 396 397 /** 398 * @return {@link #provider} The actual object that is the target of the 399 * reference. The reference library doesn't populate this, but you can 400 * use it to hold the resource if you resolve it. (The practitioner who 401 * is responsible for the services rendered to the patient.) 402 */ 403 public Practitioner getProviderTarget() { 404 if (this.providerTarget == null) 405 if (Configuration.errorOnAutoCreate()) 406 throw new Error("Attempt to auto-create PaymentNotice.provider"); 407 else if (Configuration.doAutoCreate()) 408 this.providerTarget = new Practitioner(); // aa 409 return this.providerTarget; 410 } 411 412 /** 413 * @param value {@link #provider} The actual object that is the target of the 414 * reference. The reference library doesn't use these, but you can 415 * use it to hold the resource if you resolve it. (The practitioner 416 * who is responsible for the services rendered to the patient.) 417 */ 418 public PaymentNotice setProviderTarget(Practitioner value) { 419 this.providerTarget = value; 420 return this; 421 } 422 423 /** 424 * @return {@link #organization} (The organization which is responsible for the 425 * services rendered to the patient.) 426 */ 427 public Reference getOrganization() { 428 if (this.organization == null) 429 if (Configuration.errorOnAutoCreate()) 430 throw new Error("Attempt to auto-create PaymentNotice.organization"); 431 else if (Configuration.doAutoCreate()) 432 this.organization = new Reference(); // cc 433 return this.organization; 434 } 435 436 public boolean hasOrganization() { 437 return this.organization != null && !this.organization.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #organization} (The organization which is responsible for 442 * the services rendered to the patient.) 443 */ 444 public PaymentNotice setOrganization(Reference value) { 445 this.organization = value; 446 return this; 447 } 448 449 /** 450 * @return {@link #organization} The actual object that is the target of the 451 * reference. The reference library doesn't populate this, but you can 452 * use it to hold the resource if you resolve it. (The organization 453 * which is responsible for the services rendered to the patient.) 454 */ 455 public Organization getOrganizationTarget() { 456 if (this.organizationTarget == null) 457 if (Configuration.errorOnAutoCreate()) 458 throw new Error("Attempt to auto-create PaymentNotice.organization"); 459 else if (Configuration.doAutoCreate()) 460 this.organizationTarget = new Organization(); // aa 461 return this.organizationTarget; 462 } 463 464 /** 465 * @param value {@link #organization} The actual object that is the target of 466 * the reference. The reference library doesn't use these, but you 467 * can use it to hold the resource if you resolve it. (The 468 * organization which is responsible for the services rendered to 469 * the patient.) 470 */ 471 public PaymentNotice setOrganizationTarget(Organization value) { 472 this.organizationTarget = value; 473 return this; 474 } 475 476 /** 477 * @return {@link #request} (Reference of resource to reverse.) 478 */ 479 public Reference getRequest() { 480 if (this.request == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create PaymentNotice.request"); 483 else if (Configuration.doAutoCreate()) 484 this.request = new Reference(); // cc 485 return this.request; 486 } 487 488 public boolean hasRequest() { 489 return this.request != null && !this.request.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #request} (Reference of resource to reverse.) 494 */ 495 public PaymentNotice setRequest(Reference value) { 496 this.request = value; 497 return this; 498 } 499 500 /** 501 * @return {@link #request} The actual object that is the target of the 502 * reference. The reference library doesn't populate this, but you can 503 * use it to hold the resource if you resolve it. (Reference of resource 504 * to reverse.) 505 */ 506 public Resource getRequestTarget() { 507 return this.requestTarget; 508 } 509 510 /** 511 * @param value {@link #request} The actual object that is the target of the 512 * reference. The reference library doesn't use these, but you can 513 * use it to hold the resource if you resolve it. (Reference of 514 * resource to reverse.) 515 */ 516 public PaymentNotice setRequestTarget(Resource value) { 517 this.requestTarget = value; 518 return this; 519 } 520 521 /** 522 * @return {@link #response} (Reference of response to resource to reverse.) 523 */ 524 public Reference getResponse() { 525 if (this.response == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create PaymentNotice.response"); 528 else if (Configuration.doAutoCreate()) 529 this.response = new Reference(); // cc 530 return this.response; 531 } 532 533 public boolean hasResponse() { 534 return this.response != null && !this.response.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #response} (Reference of response to resource to 539 * reverse.) 540 */ 541 public PaymentNotice setResponse(Reference value) { 542 this.response = value; 543 return this; 544 } 545 546 /** 547 * @return {@link #response} The actual object that is the target of the 548 * reference. The reference library doesn't populate this, but you can 549 * use it to hold the resource if you resolve it. (Reference of response 550 * to resource to reverse.) 551 */ 552 public Resource getResponseTarget() { 553 return this.responseTarget; 554 } 555 556 /** 557 * @param value {@link #response} The actual object that is the target of the 558 * reference. The reference library doesn't use these, but you can 559 * use it to hold the resource if you resolve it. (Reference of 560 * response to resource to reverse.) 561 */ 562 public PaymentNotice setResponseTarget(Resource value) { 563 this.responseTarget = value; 564 return this; 565 } 566 567 /** 568 * @return {@link #paymentStatus} (The payment status, typically paid: payment 569 * sent, cleared: payment received.) 570 */ 571 public Coding getPaymentStatus() { 572 if (this.paymentStatus == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create PaymentNotice.paymentStatus"); 575 else if (Configuration.doAutoCreate()) 576 this.paymentStatus = new Coding(); // cc 577 return this.paymentStatus; 578 } 579 580 public boolean hasPaymentStatus() { 581 return this.paymentStatus != null && !this.paymentStatus.isEmpty(); 582 } 583 584 /** 585 * @param value {@link #paymentStatus} (The payment status, typically paid: 586 * payment sent, cleared: payment received.) 587 */ 588 public PaymentNotice setPaymentStatus(Coding value) { 589 this.paymentStatus = value; 590 return this; 591 } 592 593 protected void listChildren(List<Property> childrenList) { 594 super.listChildren(childrenList); 595 childrenList.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 596 java.lang.Integer.MAX_VALUE, identifier)); 597 childrenList.add(new Property("ruleset", "Coding", 598 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 599 0, java.lang.Integer.MAX_VALUE, ruleset)); 600 childrenList.add(new Property("originalRuleset", "Coding", 601 "The style (standard) and version of the original material which was converted into this resource.", 0, 602 java.lang.Integer.MAX_VALUE, originalRuleset)); 603 childrenList.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 604 java.lang.Integer.MAX_VALUE, created)); 605 childrenList.add(new Property("target", "Reference(Organization)", "The Insurer who is target of the request.", 0, 606 java.lang.Integer.MAX_VALUE, target)); 607 childrenList.add(new Property("provider", "Reference(Practitioner)", 608 "The practitioner who is responsible for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 609 provider)); 610 childrenList.add(new Property("organization", "Reference(Organization)", 611 "The organization which is responsible for the services rendered to the patient.", 0, 612 java.lang.Integer.MAX_VALUE, organization)); 613 childrenList.add(new Property("request", "Reference(Any)", "Reference of resource to reverse.", 0, 614 java.lang.Integer.MAX_VALUE, request)); 615 childrenList.add(new Property("response", "Reference(Any)", "Reference of response to resource to reverse.", 0, 616 java.lang.Integer.MAX_VALUE, response)); 617 childrenList.add(new Property("paymentStatus", "Coding", 618 "The payment status, typically paid: payment sent, cleared: payment received.", 0, java.lang.Integer.MAX_VALUE, 619 paymentStatus)); 620 } 621 622 @Override 623 public void setProperty(String name, Base value) throws FHIRException { 624 if (name.equals("identifier")) 625 this.getIdentifier().add(castToIdentifier(value)); 626 else if (name.equals("ruleset")) 627 this.ruleset = castToCoding(value); // Coding 628 else if (name.equals("originalRuleset")) 629 this.originalRuleset = castToCoding(value); // Coding 630 else if (name.equals("created")) 631 this.created = castToDateTime(value); // DateTimeType 632 else if (name.equals("target")) 633 this.target = castToReference(value); // Reference 634 else if (name.equals("provider")) 635 this.provider = castToReference(value); // Reference 636 else if (name.equals("organization")) 637 this.organization = castToReference(value); // Reference 638 else if (name.equals("request")) 639 this.request = castToReference(value); // Reference 640 else if (name.equals("response")) 641 this.response = castToReference(value); // Reference 642 else if (name.equals("paymentStatus")) 643 this.paymentStatus = castToCoding(value); // Coding 644 else 645 super.setProperty(name, value); 646 } 647 648 @Override 649 public Base addChild(String name) throws FHIRException { 650 if (name.equals("identifier")) { 651 return addIdentifier(); 652 } else if (name.equals("ruleset")) { 653 this.ruleset = new Coding(); 654 return this.ruleset; 655 } else if (name.equals("originalRuleset")) { 656 this.originalRuleset = new Coding(); 657 return this.originalRuleset; 658 } else if (name.equals("created")) { 659 throw new FHIRException("Cannot call addChild on a singleton property PaymentNotice.created"); 660 } else if (name.equals("target")) { 661 this.target = new Reference(); 662 return this.target; 663 } else if (name.equals("provider")) { 664 this.provider = new Reference(); 665 return this.provider; 666 } else if (name.equals("organization")) { 667 this.organization = new Reference(); 668 return this.organization; 669 } else if (name.equals("request")) { 670 this.request = new Reference(); 671 return this.request; 672 } else if (name.equals("response")) { 673 this.response = new Reference(); 674 return this.response; 675 } else if (name.equals("paymentStatus")) { 676 this.paymentStatus = new Coding(); 677 return this.paymentStatus; 678 } else 679 return super.addChild(name); 680 } 681 682 public String fhirType() { 683 return "PaymentNotice"; 684 685 } 686 687 public PaymentNotice copy() { 688 PaymentNotice dst = new PaymentNotice(); 689 copyValues(dst); 690 if (identifier != null) { 691 dst.identifier = new ArrayList<Identifier>(); 692 for (Identifier i : identifier) 693 dst.identifier.add(i.copy()); 694 } 695 ; 696 dst.ruleset = ruleset == null ? null : ruleset.copy(); 697 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 698 dst.created = created == null ? null : created.copy(); 699 dst.target = target == null ? null : target.copy(); 700 dst.provider = provider == null ? null : provider.copy(); 701 dst.organization = organization == null ? null : organization.copy(); 702 dst.request = request == null ? null : request.copy(); 703 dst.response = response == null ? null : response.copy(); 704 dst.paymentStatus = paymentStatus == null ? null : paymentStatus.copy(); 705 return dst; 706 } 707 708 protected PaymentNotice typedCopy() { 709 return copy(); 710 } 711 712 @Override 713 public boolean equalsDeep(Base other) { 714 if (!super.equalsDeep(other)) 715 return false; 716 if (!(other instanceof PaymentNotice)) 717 return false; 718 PaymentNotice o = (PaymentNotice) other; 719 return compareDeep(identifier, o.identifier, true) && compareDeep(ruleset, o.ruleset, true) 720 && compareDeep(originalRuleset, o.originalRuleset, true) && compareDeep(created, o.created, true) 721 && compareDeep(target, o.target, true) && compareDeep(provider, o.provider, true) 722 && compareDeep(organization, o.organization, true) && compareDeep(request, o.request, true) 723 && compareDeep(response, o.response, true) && compareDeep(paymentStatus, o.paymentStatus, true); 724 } 725 726 @Override 727 public boolean equalsShallow(Base other) { 728 if (!super.equalsShallow(other)) 729 return false; 730 if (!(other instanceof PaymentNotice)) 731 return false; 732 PaymentNotice o = (PaymentNotice) other; 733 return compareValues(created, o.created, true); 734 } 735 736 public boolean isEmpty() { 737 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (ruleset == null || ruleset.isEmpty()) 738 && (originalRuleset == null || originalRuleset.isEmpty()) && (created == null || created.isEmpty()) 739 && (target == null || target.isEmpty()) && (provider == null || provider.isEmpty()) 740 && (organization == null || organization.isEmpty()) && (request == null || request.isEmpty()) 741 && (response == null || response.isEmpty()) && (paymentStatus == null || paymentStatus.isEmpty()); 742 } 743 744 @Override 745 public ResourceType getResourceType() { 746 return ResourceType.PaymentNotice; 747 } 748 749 @SearchParamDefinition(name = "identifier", path = "PaymentNotice.identifier", description = "The business identifier of the Eligibility", type = "token") 750 public static final String SP_IDENTIFIER = "identifier"; 751 752}