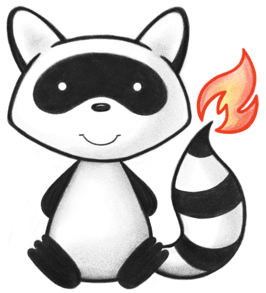
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.RemittanceOutcome; 038import org.hl7.fhir.dstu2.model.Enumerations.RemittanceOutcomeEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * This resource provides payment details and claim references supporting a bulk 050 * payment. 051 */ 052@ResourceDef(name = "PaymentReconciliation", profile = "http://hl7.org/fhir/Profile/PaymentReconciliation") 053public class PaymentReconciliation extends DomainResource { 054 055 @Block() 056 public static class DetailsComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Code to indicate the nature of the payment, adjustment, funds advance, etc. 059 */ 060 @Child(name = "type", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "Type code", formalDefinition = "Code to indicate the nature of the payment, adjustment, funds advance, etc.") 062 protected Coding type; 063 064 /** 065 * The claim or financial resource. 066 */ 067 @Child(name = "request", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "Claim", formalDefinition = "The claim or financial resource.") 069 protected Reference request; 070 071 /** 072 * The actual object that is the target of the reference (The claim or financial 073 * resource.) 074 */ 075 protected Resource requestTarget; 076 077 /** 078 * The claim response resource. 079 */ 080 @Child(name = "responce", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = true) 081 @Description(shortDefinition = "Claim Response", formalDefinition = "The claim response resource.") 082 protected Reference responce; 083 084 /** 085 * The actual object that is the target of the reference (The claim response 086 * resource.) 087 */ 088 protected Resource responceTarget; 089 090 /** 091 * The Organization which submitted the invoice or financial transaction. 092 */ 093 @Child(name = "submitter", type = { 094 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 095 @Description(shortDefinition = "Submitter", formalDefinition = "The Organization which submitted the invoice or financial transaction.") 096 protected Reference submitter; 097 098 /** 099 * The actual object that is the target of the reference (The Organization which 100 * submitted the invoice or financial transaction.) 101 */ 102 protected Organization submitterTarget; 103 104 /** 105 * The organization which is receiving the payment. 106 */ 107 @Child(name = "payee", type = { Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 108 @Description(shortDefinition = "Payee", formalDefinition = "The organization which is receiving the payment.") 109 protected Reference payee; 110 111 /** 112 * The actual object that is the target of the reference (The organization which 113 * is receiving the payment.) 114 */ 115 protected Organization payeeTarget; 116 117 /** 118 * The date of the invoice or financial resource. 119 */ 120 @Child(name = "date", type = { DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 121 @Description(shortDefinition = "Invoice date", formalDefinition = "The date of the invoice or financial resource.") 122 protected DateType date; 123 124 /** 125 * Amount paid for this detail. 126 */ 127 @Child(name = "amount", type = { Money.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 128 @Description(shortDefinition = "Detail amount", formalDefinition = "Amount paid for this detail.") 129 protected Money amount; 130 131 private static final long serialVersionUID = -1644048062L; 132 133 /* 134 * Constructor 135 */ 136 public DetailsComponent() { 137 super(); 138 } 139 140 /* 141 * Constructor 142 */ 143 public DetailsComponent(Coding type) { 144 super(); 145 this.type = type; 146 } 147 148 /** 149 * @return {@link #type} (Code to indicate the nature of the payment, 150 * adjustment, funds advance, etc.) 151 */ 152 public Coding getType() { 153 if (this.type == null) 154 if (Configuration.errorOnAutoCreate()) 155 throw new Error("Attempt to auto-create DetailsComponent.type"); 156 else if (Configuration.doAutoCreate()) 157 this.type = new Coding(); // cc 158 return this.type; 159 } 160 161 public boolean hasType() { 162 return this.type != null && !this.type.isEmpty(); 163 } 164 165 /** 166 * @param value {@link #type} (Code to indicate the nature of the payment, 167 * adjustment, funds advance, etc.) 168 */ 169 public DetailsComponent setType(Coding value) { 170 this.type = value; 171 return this; 172 } 173 174 /** 175 * @return {@link #request} (The claim or financial resource.) 176 */ 177 public Reference getRequest() { 178 if (this.request == null) 179 if (Configuration.errorOnAutoCreate()) 180 throw new Error("Attempt to auto-create DetailsComponent.request"); 181 else if (Configuration.doAutoCreate()) 182 this.request = new Reference(); // cc 183 return this.request; 184 } 185 186 public boolean hasRequest() { 187 return this.request != null && !this.request.isEmpty(); 188 } 189 190 /** 191 * @param value {@link #request} (The claim or financial resource.) 192 */ 193 public DetailsComponent setRequest(Reference value) { 194 this.request = value; 195 return this; 196 } 197 198 /** 199 * @return {@link #request} The actual object that is the target of the 200 * reference. The reference library doesn't populate this, but you can 201 * use it to hold the resource if you resolve it. (The claim or 202 * financial resource.) 203 */ 204 public Resource getRequestTarget() { 205 return this.requestTarget; 206 } 207 208 /** 209 * @param value {@link #request} The actual object that is the target of the 210 * reference. The reference library doesn't use these, but you can 211 * use it to hold the resource if you resolve it. (The claim or 212 * financial resource.) 213 */ 214 public DetailsComponent setRequestTarget(Resource value) { 215 this.requestTarget = value; 216 return this; 217 } 218 219 /** 220 * @return {@link #responce} (The claim response resource.) 221 */ 222 public Reference getResponce() { 223 if (this.responce == null) 224 if (Configuration.errorOnAutoCreate()) 225 throw new Error("Attempt to auto-create DetailsComponent.responce"); 226 else if (Configuration.doAutoCreate()) 227 this.responce = new Reference(); // cc 228 return this.responce; 229 } 230 231 public boolean hasResponce() { 232 return this.responce != null && !this.responce.isEmpty(); 233 } 234 235 /** 236 * @param value {@link #responce} (The claim response resource.) 237 */ 238 public DetailsComponent setResponce(Reference value) { 239 this.responce = value; 240 return this; 241 } 242 243 /** 244 * @return {@link #responce} The actual object that is the target of the 245 * reference. The reference library doesn't populate this, but you can 246 * use it to hold the resource if you resolve it. (The claim response 247 * resource.) 248 */ 249 public Resource getResponceTarget() { 250 return this.responceTarget; 251 } 252 253 /** 254 * @param value {@link #responce} The actual object that is the target of the 255 * reference. The reference library doesn't use these, but you can 256 * use it to hold the resource if you resolve it. (The claim 257 * response resource.) 258 */ 259 public DetailsComponent setResponceTarget(Resource value) { 260 this.responceTarget = value; 261 return this; 262 } 263 264 /** 265 * @return {@link #submitter} (The Organization which submitted the invoice or 266 * financial transaction.) 267 */ 268 public Reference getSubmitter() { 269 if (this.submitter == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create DetailsComponent.submitter"); 272 else if (Configuration.doAutoCreate()) 273 this.submitter = new Reference(); // cc 274 return this.submitter; 275 } 276 277 public boolean hasSubmitter() { 278 return this.submitter != null && !this.submitter.isEmpty(); 279 } 280 281 /** 282 * @param value {@link #submitter} (The Organization which submitted the invoice 283 * or financial transaction.) 284 */ 285 public DetailsComponent setSubmitter(Reference value) { 286 this.submitter = value; 287 return this; 288 } 289 290 /** 291 * @return {@link #submitter} The actual object that is the target of the 292 * reference. The reference library doesn't populate this, but you can 293 * use it to hold the resource if you resolve it. (The Organization 294 * which submitted the invoice or financial transaction.) 295 */ 296 public Organization getSubmitterTarget() { 297 if (this.submitterTarget == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create DetailsComponent.submitter"); 300 else if (Configuration.doAutoCreate()) 301 this.submitterTarget = new Organization(); // aa 302 return this.submitterTarget; 303 } 304 305 /** 306 * @param value {@link #submitter} The actual object that is the target of the 307 * reference. The reference library doesn't use these, but you can 308 * use it to hold the resource if you resolve it. (The Organization 309 * which submitted the invoice or financial transaction.) 310 */ 311 public DetailsComponent setSubmitterTarget(Organization value) { 312 this.submitterTarget = value; 313 return this; 314 } 315 316 /** 317 * @return {@link #payee} (The organization which is receiving the payment.) 318 */ 319 public Reference getPayee() { 320 if (this.payee == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create DetailsComponent.payee"); 323 else if (Configuration.doAutoCreate()) 324 this.payee = new Reference(); // cc 325 return this.payee; 326 } 327 328 public boolean hasPayee() { 329 return this.payee != null && !this.payee.isEmpty(); 330 } 331 332 /** 333 * @param value {@link #payee} (The organization which is receiving the 334 * payment.) 335 */ 336 public DetailsComponent setPayee(Reference value) { 337 this.payee = value; 338 return this; 339 } 340 341 /** 342 * @return {@link #payee} The actual object that is the target of the reference. 343 * The reference library doesn't populate this, but you can use it to 344 * hold the resource if you resolve it. (The organization which is 345 * receiving the payment.) 346 */ 347 public Organization getPayeeTarget() { 348 if (this.payeeTarget == null) 349 if (Configuration.errorOnAutoCreate()) 350 throw new Error("Attempt to auto-create DetailsComponent.payee"); 351 else if (Configuration.doAutoCreate()) 352 this.payeeTarget = new Organization(); // aa 353 return this.payeeTarget; 354 } 355 356 /** 357 * @param value {@link #payee} The actual object that is the target of the 358 * reference. The reference library doesn't use these, but you can 359 * use it to hold the resource if you resolve it. (The organization 360 * which is receiving the payment.) 361 */ 362 public DetailsComponent setPayeeTarget(Organization value) { 363 this.payeeTarget = value; 364 return this; 365 } 366 367 /** 368 * @return {@link #date} (The date of the invoice or financial resource.). This 369 * is the underlying object with id, value and extensions. The accessor 370 * "getDate" gives direct access to the value 371 */ 372 public DateType getDateElement() { 373 if (this.date == null) 374 if (Configuration.errorOnAutoCreate()) 375 throw new Error("Attempt to auto-create DetailsComponent.date"); 376 else if (Configuration.doAutoCreate()) 377 this.date = new DateType(); // bb 378 return this.date; 379 } 380 381 public boolean hasDateElement() { 382 return this.date != null && !this.date.isEmpty(); 383 } 384 385 public boolean hasDate() { 386 return this.date != null && !this.date.isEmpty(); 387 } 388 389 /** 390 * @param value {@link #date} (The date of the invoice or financial resource.). 391 * This is the underlying object with id, value and extensions. The 392 * accessor "getDate" gives direct access to the value 393 */ 394 public DetailsComponent setDateElement(DateType value) { 395 this.date = value; 396 return this; 397 } 398 399 /** 400 * @return The date of the invoice or financial resource. 401 */ 402 public Date getDate() { 403 return this.date == null ? null : this.date.getValue(); 404 } 405 406 /** 407 * @param value The date of the invoice or financial resource. 408 */ 409 public DetailsComponent setDate(Date value) { 410 if (value == null) 411 this.date = null; 412 else { 413 if (this.date == null) 414 this.date = new DateType(); 415 this.date.setValue(value); 416 } 417 return this; 418 } 419 420 /** 421 * @return {@link #amount} (Amount paid for this detail.) 422 */ 423 public Money getAmount() { 424 if (this.amount == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create DetailsComponent.amount"); 427 else if (Configuration.doAutoCreate()) 428 this.amount = new Money(); // cc 429 return this.amount; 430 } 431 432 public boolean hasAmount() { 433 return this.amount != null && !this.amount.isEmpty(); 434 } 435 436 /** 437 * @param value {@link #amount} (Amount paid for this detail.) 438 */ 439 public DetailsComponent setAmount(Money value) { 440 this.amount = value; 441 return this; 442 } 443 444 protected void listChildren(List<Property> childrenList) { 445 super.listChildren(childrenList); 446 childrenList.add( 447 new Property("type", "Coding", "Code to indicate the nature of the payment, adjustment, funds advance, etc.", 448 0, java.lang.Integer.MAX_VALUE, type)); 449 childrenList.add(new Property("request", "Reference(Any)", "The claim or financial resource.", 0, 450 java.lang.Integer.MAX_VALUE, request)); 451 childrenList.add(new Property("responce", "Reference(Any)", "The claim response resource.", 0, 452 java.lang.Integer.MAX_VALUE, responce)); 453 childrenList.add(new Property("submitter", "Reference(Organization)", 454 "The Organization which submitted the invoice or financial transaction.", 0, java.lang.Integer.MAX_VALUE, 455 submitter)); 456 childrenList.add(new Property("payee", "Reference(Organization)", 457 "The organization which is receiving the payment.", 0, java.lang.Integer.MAX_VALUE, payee)); 458 childrenList.add(new Property("date", "date", "The date of the invoice or financial resource.", 0, 459 java.lang.Integer.MAX_VALUE, date)); 460 childrenList 461 .add(new Property("amount", "Money", "Amount paid for this detail.", 0, java.lang.Integer.MAX_VALUE, amount)); 462 } 463 464 @Override 465 public void setProperty(String name, Base value) throws FHIRException { 466 if (name.equals("type")) 467 this.type = castToCoding(value); // Coding 468 else if (name.equals("request")) 469 this.request = castToReference(value); // Reference 470 else if (name.equals("responce")) 471 this.responce = castToReference(value); // Reference 472 else if (name.equals("submitter")) 473 this.submitter = castToReference(value); // Reference 474 else if (name.equals("payee")) 475 this.payee = castToReference(value); // Reference 476 else if (name.equals("date")) 477 this.date = castToDate(value); // DateType 478 else if (name.equals("amount")) 479 this.amount = castToMoney(value); // Money 480 else 481 super.setProperty(name, value); 482 } 483 484 @Override 485 public Base addChild(String name) throws FHIRException { 486 if (name.equals("type")) { 487 this.type = new Coding(); 488 return this.type; 489 } else if (name.equals("request")) { 490 this.request = new Reference(); 491 return this.request; 492 } else if (name.equals("responce")) { 493 this.responce = new Reference(); 494 return this.responce; 495 } else if (name.equals("submitter")) { 496 this.submitter = new Reference(); 497 return this.submitter; 498 } else if (name.equals("payee")) { 499 this.payee = new Reference(); 500 return this.payee; 501 } else if (name.equals("date")) { 502 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.date"); 503 } else if (name.equals("amount")) { 504 this.amount = new Money(); 505 return this.amount; 506 } else 507 return super.addChild(name); 508 } 509 510 public DetailsComponent copy() { 511 DetailsComponent dst = new DetailsComponent(); 512 copyValues(dst); 513 dst.type = type == null ? null : type.copy(); 514 dst.request = request == null ? null : request.copy(); 515 dst.responce = responce == null ? null : responce.copy(); 516 dst.submitter = submitter == null ? null : submitter.copy(); 517 dst.payee = payee == null ? null : payee.copy(); 518 dst.date = date == null ? null : date.copy(); 519 dst.amount = amount == null ? null : amount.copy(); 520 return dst; 521 } 522 523 @Override 524 public boolean equalsDeep(Base other) { 525 if (!super.equalsDeep(other)) 526 return false; 527 if (!(other instanceof DetailsComponent)) 528 return false; 529 DetailsComponent o = (DetailsComponent) other; 530 return compareDeep(type, o.type, true) && compareDeep(request, o.request, true) 531 && compareDeep(responce, o.responce, true) && compareDeep(submitter, o.submitter, true) 532 && compareDeep(payee, o.payee, true) && compareDeep(date, o.date, true) 533 && compareDeep(amount, o.amount, true); 534 } 535 536 @Override 537 public boolean equalsShallow(Base other) { 538 if (!super.equalsShallow(other)) 539 return false; 540 if (!(other instanceof DetailsComponent)) 541 return false; 542 DetailsComponent o = (DetailsComponent) other; 543 return compareValues(date, o.date, true); 544 } 545 546 public boolean isEmpty() { 547 return super.isEmpty() && (type == null || type.isEmpty()) && (request == null || request.isEmpty()) 548 && (responce == null || responce.isEmpty()) && (submitter == null || submitter.isEmpty()) 549 && (payee == null || payee.isEmpty()) && (date == null || date.isEmpty()) 550 && (amount == null || amount.isEmpty()); 551 } 552 553 public String fhirType() { 554 return "PaymentReconciliation.detail"; 555 556 } 557 558 } 559 560 @Block() 561 public static class NotesComponent extends BackboneElement implements IBaseBackboneElement { 562 /** 563 * The note purpose: Print/Display. 564 */ 565 @Child(name = "type", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 566 @Description(shortDefinition = "display | print | printoper", formalDefinition = "The note purpose: Print/Display.") 567 protected Coding type; 568 569 /** 570 * The note text. 571 */ 572 @Child(name = "text", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 573 @Description(shortDefinition = "Notes text", formalDefinition = "The note text.") 574 protected StringType text; 575 576 private static final long serialVersionUID = 129959202L; 577 578 /* 579 * Constructor 580 */ 581 public NotesComponent() { 582 super(); 583 } 584 585 /** 586 * @return {@link #type} (The note purpose: Print/Display.) 587 */ 588 public Coding getType() { 589 if (this.type == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create NotesComponent.type"); 592 else if (Configuration.doAutoCreate()) 593 this.type = new Coding(); // cc 594 return this.type; 595 } 596 597 public boolean hasType() { 598 return this.type != null && !this.type.isEmpty(); 599 } 600 601 /** 602 * @param value {@link #type} (The note purpose: Print/Display.) 603 */ 604 public NotesComponent setType(Coding value) { 605 this.type = value; 606 return this; 607 } 608 609 /** 610 * @return {@link #text} (The note text.). This is the underlying object with 611 * id, value and extensions. The accessor "getText" gives direct access 612 * to the value 613 */ 614 public StringType getTextElement() { 615 if (this.text == null) 616 if (Configuration.errorOnAutoCreate()) 617 throw new Error("Attempt to auto-create NotesComponent.text"); 618 else if (Configuration.doAutoCreate()) 619 this.text = new StringType(); // bb 620 return this.text; 621 } 622 623 public boolean hasTextElement() { 624 return this.text != null && !this.text.isEmpty(); 625 } 626 627 public boolean hasText() { 628 return this.text != null && !this.text.isEmpty(); 629 } 630 631 /** 632 * @param value {@link #text} (The note text.). This is the underlying object 633 * with id, value and extensions. The accessor "getText" gives 634 * direct access to the value 635 */ 636 public NotesComponent setTextElement(StringType value) { 637 this.text = value; 638 return this; 639 } 640 641 /** 642 * @return The note text. 643 */ 644 public String getText() { 645 return this.text == null ? null : this.text.getValue(); 646 } 647 648 /** 649 * @param value The note text. 650 */ 651 public NotesComponent setText(String value) { 652 if (Utilities.noString(value)) 653 this.text = null; 654 else { 655 if (this.text == null) 656 this.text = new StringType(); 657 this.text.setValue(value); 658 } 659 return this; 660 } 661 662 protected void listChildren(List<Property> childrenList) { 663 super.listChildren(childrenList); 664 childrenList.add( 665 new Property("type", "Coding", "The note purpose: Print/Display.", 0, java.lang.Integer.MAX_VALUE, type)); 666 childrenList.add(new Property("text", "string", "The note text.", 0, java.lang.Integer.MAX_VALUE, text)); 667 } 668 669 @Override 670 public void setProperty(String name, Base value) throws FHIRException { 671 if (name.equals("type")) 672 this.type = castToCoding(value); // Coding 673 else if (name.equals("text")) 674 this.text = castToString(value); // StringType 675 else 676 super.setProperty(name, value); 677 } 678 679 @Override 680 public Base addChild(String name) throws FHIRException { 681 if (name.equals("type")) { 682 this.type = new Coding(); 683 return this.type; 684 } else if (name.equals("text")) { 685 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.text"); 686 } else 687 return super.addChild(name); 688 } 689 690 public NotesComponent copy() { 691 NotesComponent dst = new NotesComponent(); 692 copyValues(dst); 693 dst.type = type == null ? null : type.copy(); 694 dst.text = text == null ? null : text.copy(); 695 return dst; 696 } 697 698 @Override 699 public boolean equalsDeep(Base other) { 700 if (!super.equalsDeep(other)) 701 return false; 702 if (!(other instanceof NotesComponent)) 703 return false; 704 NotesComponent o = (NotesComponent) other; 705 return compareDeep(type, o.type, true) && compareDeep(text, o.text, true); 706 } 707 708 @Override 709 public boolean equalsShallow(Base other) { 710 if (!super.equalsShallow(other)) 711 return false; 712 if (!(other instanceof NotesComponent)) 713 return false; 714 NotesComponent o = (NotesComponent) other; 715 return compareValues(text, o.text, true); 716 } 717 718 public boolean isEmpty() { 719 return super.isEmpty() && (type == null || type.isEmpty()) && (text == null || text.isEmpty()); 720 } 721 722 public String fhirType() { 723 return "PaymentReconciliation.note"; 724 725 } 726 727 } 728 729 /** 730 * The Response business identifier. 731 */ 732 @Child(name = "identifier", type = { 733 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 734 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 735 protected List<Identifier> identifier; 736 737 /** 738 * Original request resource reference. 739 */ 740 @Child(name = "request", type = { 741 ProcessRequest.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 742 @Description(shortDefinition = "Claim reference", formalDefinition = "Original request resource reference.") 743 protected Reference request; 744 745 /** 746 * The actual object that is the target of the reference (Original request 747 * resource reference.) 748 */ 749 protected ProcessRequest requestTarget; 750 751 /** 752 * Transaction status: error, complete. 753 */ 754 @Child(name = "outcome", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 755 @Description(shortDefinition = "complete | error", formalDefinition = "Transaction status: error, complete.") 756 protected Enumeration<RemittanceOutcome> outcome; 757 758 /** 759 * A description of the status of the adjudication. 760 */ 761 @Child(name = "disposition", type = { 762 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 763 @Description(shortDefinition = "Disposition Message", formalDefinition = "A description of the status of the adjudication.") 764 protected StringType disposition; 765 766 /** 767 * The version of the style of resource contents. This should be mapped to the 768 * allowable profiles for this and supporting resources. 769 */ 770 @Child(name = "ruleset", type = { Coding.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 771 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 772 protected Coding ruleset; 773 774 /** 775 * The style (standard) and version of the original material which was converted 776 * into this resource. 777 */ 778 @Child(name = "originalRuleset", type = { 779 Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 780 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 781 protected Coding originalRuleset; 782 783 /** 784 * The date when the enclosed suite of services were performed or completed. 785 */ 786 @Child(name = "created", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 787 @Description(shortDefinition = "Creation date", formalDefinition = "The date when the enclosed suite of services were performed or completed.") 788 protected DateTimeType created; 789 790 /** 791 * The period of time for which payments have been gathered into this bulk 792 * payment for settlement. 793 */ 794 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 795 @Description(shortDefinition = "Period covered", formalDefinition = "The period of time for which payments have been gathered into this bulk payment for settlement.") 796 protected Period period; 797 798 /** 799 * The Insurer who produced this adjudicated response. 800 */ 801 @Child(name = "organization", type = { 802 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 803 @Description(shortDefinition = "Insurer", formalDefinition = "The Insurer who produced this adjudicated response.") 804 protected Reference organization; 805 806 /** 807 * The actual object that is the target of the reference (The Insurer who 808 * produced this adjudicated response.) 809 */ 810 protected Organization organizationTarget; 811 812 /** 813 * The practitioner who is responsible for the services rendered to the patient. 814 */ 815 @Child(name = "requestProvider", type = { 816 Practitioner.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 817 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 818 protected Reference requestProvider; 819 820 /** 821 * The actual object that is the target of the reference (The practitioner who 822 * is responsible for the services rendered to the patient.) 823 */ 824 protected Practitioner requestProviderTarget; 825 826 /** 827 * The organization which is responsible for the services rendered to the 828 * patient. 829 */ 830 @Child(name = "requestOrganization", type = { 831 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 832 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the services rendered to the patient.") 833 protected Reference requestOrganization; 834 835 /** 836 * The actual object that is the target of the reference (The organization which 837 * is responsible for the services rendered to the patient.) 838 */ 839 protected Organization requestOrganizationTarget; 840 841 /** 842 * List of individual settlement amounts and the corresponding transaction. 843 */ 844 @Child(name = "detail", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 845 @Description(shortDefinition = "Details", formalDefinition = "List of individual settlement amounts and the corresponding transaction.") 846 protected List<DetailsComponent> detail; 847 848 /** 849 * The form to be used for printing the content. 850 */ 851 @Child(name = "form", type = { Coding.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 852 @Description(shortDefinition = "Printed Form Identifier", formalDefinition = "The form to be used for printing the content.") 853 protected Coding form; 854 855 /** 856 * Total payment amount. 857 */ 858 @Child(name = "total", type = { Money.class }, order = 13, min = 1, max = 1, modifier = false, summary = true) 859 @Description(shortDefinition = "Total amount of Payment", formalDefinition = "Total payment amount.") 860 protected Money total; 861 862 /** 863 * Suite of notes. 864 */ 865 @Child(name = "note", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 866 @Description(shortDefinition = "Note text", formalDefinition = "Suite of notes.") 867 protected List<NotesComponent> note; 868 869 private static final long serialVersionUID = 454328025L; 870 871 /* 872 * Constructor 873 */ 874 public PaymentReconciliation() { 875 super(); 876 } 877 878 /* 879 * Constructor 880 */ 881 public PaymentReconciliation(Money total) { 882 super(); 883 this.total = total; 884 } 885 886 /** 887 * @return {@link #identifier} (The Response business identifier.) 888 */ 889 public List<Identifier> getIdentifier() { 890 if (this.identifier == null) 891 this.identifier = new ArrayList<Identifier>(); 892 return this.identifier; 893 } 894 895 public boolean hasIdentifier() { 896 if (this.identifier == null) 897 return false; 898 for (Identifier item : this.identifier) 899 if (!item.isEmpty()) 900 return true; 901 return false; 902 } 903 904 /** 905 * @return {@link #identifier} (The Response business identifier.) 906 */ 907 // syntactic sugar 908 public Identifier addIdentifier() { // 3 909 Identifier t = new Identifier(); 910 if (this.identifier == null) 911 this.identifier = new ArrayList<Identifier>(); 912 this.identifier.add(t); 913 return t; 914 } 915 916 // syntactic sugar 917 public PaymentReconciliation addIdentifier(Identifier t) { // 3 918 if (t == null) 919 return this; 920 if (this.identifier == null) 921 this.identifier = new ArrayList<Identifier>(); 922 this.identifier.add(t); 923 return this; 924 } 925 926 /** 927 * @return {@link #request} (Original request resource reference.) 928 */ 929 public Reference getRequest() { 930 if (this.request == null) 931 if (Configuration.errorOnAutoCreate()) 932 throw new Error("Attempt to auto-create PaymentReconciliation.request"); 933 else if (Configuration.doAutoCreate()) 934 this.request = new Reference(); // cc 935 return this.request; 936 } 937 938 public boolean hasRequest() { 939 return this.request != null && !this.request.isEmpty(); 940 } 941 942 /** 943 * @param value {@link #request} (Original request resource reference.) 944 */ 945 public PaymentReconciliation setRequest(Reference value) { 946 this.request = value; 947 return this; 948 } 949 950 /** 951 * @return {@link #request} The actual object that is the target of the 952 * reference. The reference library doesn't populate this, but you can 953 * use it to hold the resource if you resolve it. (Original request 954 * resource reference.) 955 */ 956 public ProcessRequest getRequestTarget() { 957 if (this.requestTarget == null) 958 if (Configuration.errorOnAutoCreate()) 959 throw new Error("Attempt to auto-create PaymentReconciliation.request"); 960 else if (Configuration.doAutoCreate()) 961 this.requestTarget = new ProcessRequest(); // aa 962 return this.requestTarget; 963 } 964 965 /** 966 * @param value {@link #request} The actual object that is the target of the 967 * reference. The reference library doesn't use these, but you can 968 * use it to hold the resource if you resolve it. (Original request 969 * resource reference.) 970 */ 971 public PaymentReconciliation setRequestTarget(ProcessRequest value) { 972 this.requestTarget = value; 973 return this; 974 } 975 976 /** 977 * @return {@link #outcome} (Transaction status: error, complete.). This is the 978 * underlying object with id, value and extensions. The accessor 979 * "getOutcome" gives direct access to the value 980 */ 981 public Enumeration<RemittanceOutcome> getOutcomeElement() { 982 if (this.outcome == null) 983 if (Configuration.errorOnAutoCreate()) 984 throw new Error("Attempt to auto-create PaymentReconciliation.outcome"); 985 else if (Configuration.doAutoCreate()) 986 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 987 return this.outcome; 988 } 989 990 public boolean hasOutcomeElement() { 991 return this.outcome != null && !this.outcome.isEmpty(); 992 } 993 994 public boolean hasOutcome() { 995 return this.outcome != null && !this.outcome.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #outcome} (Transaction status: error, complete.). This is 1000 * the underlying object with id, value and extensions. The 1001 * accessor "getOutcome" gives direct access to the value 1002 */ 1003 public PaymentReconciliation setOutcomeElement(Enumeration<RemittanceOutcome> value) { 1004 this.outcome = value; 1005 return this; 1006 } 1007 1008 /** 1009 * @return Transaction status: error, complete. 1010 */ 1011 public RemittanceOutcome getOutcome() { 1012 return this.outcome == null ? null : this.outcome.getValue(); 1013 } 1014 1015 /** 1016 * @param value Transaction status: error, complete. 1017 */ 1018 public PaymentReconciliation setOutcome(RemittanceOutcome value) { 1019 if (value == null) 1020 this.outcome = null; 1021 else { 1022 if (this.outcome == null) 1023 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 1024 this.outcome.setValue(value); 1025 } 1026 return this; 1027 } 1028 1029 /** 1030 * @return {@link #disposition} (A description of the status of the 1031 * adjudication.). This is the underlying object with id, value and 1032 * extensions. The accessor "getDisposition" gives direct access to the 1033 * value 1034 */ 1035 public StringType getDispositionElement() { 1036 if (this.disposition == null) 1037 if (Configuration.errorOnAutoCreate()) 1038 throw new Error("Attempt to auto-create PaymentReconciliation.disposition"); 1039 else if (Configuration.doAutoCreate()) 1040 this.disposition = new StringType(); // bb 1041 return this.disposition; 1042 } 1043 1044 public boolean hasDispositionElement() { 1045 return this.disposition != null && !this.disposition.isEmpty(); 1046 } 1047 1048 public boolean hasDisposition() { 1049 return this.disposition != null && !this.disposition.isEmpty(); 1050 } 1051 1052 /** 1053 * @param value {@link #disposition} (A description of the status of the 1054 * adjudication.). This is the underlying object with id, value and 1055 * extensions. The accessor "getDisposition" gives direct access to 1056 * the value 1057 */ 1058 public PaymentReconciliation setDispositionElement(StringType value) { 1059 this.disposition = value; 1060 return this; 1061 } 1062 1063 /** 1064 * @return A description of the status of the adjudication. 1065 */ 1066 public String getDisposition() { 1067 return this.disposition == null ? null : this.disposition.getValue(); 1068 } 1069 1070 /** 1071 * @param value A description of the status of the adjudication. 1072 */ 1073 public PaymentReconciliation setDisposition(String value) { 1074 if (Utilities.noString(value)) 1075 this.disposition = null; 1076 else { 1077 if (this.disposition == null) 1078 this.disposition = new StringType(); 1079 this.disposition.setValue(value); 1080 } 1081 return this; 1082 } 1083 1084 /** 1085 * @return {@link #ruleset} (The version of the style of resource contents. This 1086 * should be mapped to the allowable profiles for this and supporting 1087 * resources.) 1088 */ 1089 public Coding getRuleset() { 1090 if (this.ruleset == null) 1091 if (Configuration.errorOnAutoCreate()) 1092 throw new Error("Attempt to auto-create PaymentReconciliation.ruleset"); 1093 else if (Configuration.doAutoCreate()) 1094 this.ruleset = new Coding(); // cc 1095 return this.ruleset; 1096 } 1097 1098 public boolean hasRuleset() { 1099 return this.ruleset != null && !this.ruleset.isEmpty(); 1100 } 1101 1102 /** 1103 * @param value {@link #ruleset} (The version of the style of resource contents. 1104 * This should be mapped to the allowable profiles for this and 1105 * supporting resources.) 1106 */ 1107 public PaymentReconciliation setRuleset(Coding value) { 1108 this.ruleset = value; 1109 return this; 1110 } 1111 1112 /** 1113 * @return {@link #originalRuleset} (The style (standard) and version of the 1114 * original material which was converted into this resource.) 1115 */ 1116 public Coding getOriginalRuleset() { 1117 if (this.originalRuleset == null) 1118 if (Configuration.errorOnAutoCreate()) 1119 throw new Error("Attempt to auto-create PaymentReconciliation.originalRuleset"); 1120 else if (Configuration.doAutoCreate()) 1121 this.originalRuleset = new Coding(); // cc 1122 return this.originalRuleset; 1123 } 1124 1125 public boolean hasOriginalRuleset() { 1126 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 1127 } 1128 1129 /** 1130 * @param value {@link #originalRuleset} (The style (standard) and version of 1131 * the original material which was converted into this resource.) 1132 */ 1133 public PaymentReconciliation setOriginalRuleset(Coding value) { 1134 this.originalRuleset = value; 1135 return this; 1136 } 1137 1138 /** 1139 * @return {@link #created} (The date when the enclosed suite of services were 1140 * performed or completed.). This is the underlying object with id, 1141 * value and extensions. The accessor "getCreated" gives direct access 1142 * to the value 1143 */ 1144 public DateTimeType getCreatedElement() { 1145 if (this.created == null) 1146 if (Configuration.errorOnAutoCreate()) 1147 throw new Error("Attempt to auto-create PaymentReconciliation.created"); 1148 else if (Configuration.doAutoCreate()) 1149 this.created = new DateTimeType(); // bb 1150 return this.created; 1151 } 1152 1153 public boolean hasCreatedElement() { 1154 return this.created != null && !this.created.isEmpty(); 1155 } 1156 1157 public boolean hasCreated() { 1158 return this.created != null && !this.created.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #created} (The date when the enclosed suite of services 1163 * were performed or completed.). This is the underlying object 1164 * with id, value and extensions. The accessor "getCreated" gives 1165 * direct access to the value 1166 */ 1167 public PaymentReconciliation setCreatedElement(DateTimeType value) { 1168 this.created = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return The date when the enclosed suite of services were performed or 1174 * completed. 1175 */ 1176 public Date getCreated() { 1177 return this.created == null ? null : this.created.getValue(); 1178 } 1179 1180 /** 1181 * @param value The date when the enclosed suite of services were performed or 1182 * completed. 1183 */ 1184 public PaymentReconciliation setCreated(Date value) { 1185 if (value == null) 1186 this.created = null; 1187 else { 1188 if (this.created == null) 1189 this.created = new DateTimeType(); 1190 this.created.setValue(value); 1191 } 1192 return this; 1193 } 1194 1195 /** 1196 * @return {@link #period} (The period of time for which payments have been 1197 * gathered into this bulk payment for settlement.) 1198 */ 1199 public Period getPeriod() { 1200 if (this.period == null) 1201 if (Configuration.errorOnAutoCreate()) 1202 throw new Error("Attempt to auto-create PaymentReconciliation.period"); 1203 else if (Configuration.doAutoCreate()) 1204 this.period = new Period(); // cc 1205 return this.period; 1206 } 1207 1208 public boolean hasPeriod() { 1209 return this.period != null && !this.period.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #period} (The period of time for which payments have been 1214 * gathered into this bulk payment for settlement.) 1215 */ 1216 public PaymentReconciliation setPeriod(Period value) { 1217 this.period = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #organization} (The Insurer who produced this adjudicated 1223 * response.) 1224 */ 1225 public Reference getOrganization() { 1226 if (this.organization == null) 1227 if (Configuration.errorOnAutoCreate()) 1228 throw new Error("Attempt to auto-create PaymentReconciliation.organization"); 1229 else if (Configuration.doAutoCreate()) 1230 this.organization = new Reference(); // cc 1231 return this.organization; 1232 } 1233 1234 public boolean hasOrganization() { 1235 return this.organization != null && !this.organization.isEmpty(); 1236 } 1237 1238 /** 1239 * @param value {@link #organization} (The Insurer who produced this adjudicated 1240 * response.) 1241 */ 1242 public PaymentReconciliation setOrganization(Reference value) { 1243 this.organization = value; 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #organization} The actual object that is the target of the 1249 * reference. The reference library doesn't populate this, but you can 1250 * use it to hold the resource if you resolve it. (The Insurer who 1251 * produced this adjudicated response.) 1252 */ 1253 public Organization getOrganizationTarget() { 1254 if (this.organizationTarget == null) 1255 if (Configuration.errorOnAutoCreate()) 1256 throw new Error("Attempt to auto-create PaymentReconciliation.organization"); 1257 else if (Configuration.doAutoCreate()) 1258 this.organizationTarget = new Organization(); // aa 1259 return this.organizationTarget; 1260 } 1261 1262 /** 1263 * @param value {@link #organization} The actual object that is the target of 1264 * the reference. The reference library doesn't use these, but you 1265 * can use it to hold the resource if you resolve it. (The Insurer 1266 * who produced this adjudicated response.) 1267 */ 1268 public PaymentReconciliation setOrganizationTarget(Organization value) { 1269 this.organizationTarget = value; 1270 return this; 1271 } 1272 1273 /** 1274 * @return {@link #requestProvider} (The practitioner who is responsible for the 1275 * services rendered to the patient.) 1276 */ 1277 public Reference getRequestProvider() { 1278 if (this.requestProvider == null) 1279 if (Configuration.errorOnAutoCreate()) 1280 throw new Error("Attempt to auto-create PaymentReconciliation.requestProvider"); 1281 else if (Configuration.doAutoCreate()) 1282 this.requestProvider = new Reference(); // cc 1283 return this.requestProvider; 1284 } 1285 1286 public boolean hasRequestProvider() { 1287 return this.requestProvider != null && !this.requestProvider.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #requestProvider} (The practitioner who is responsible 1292 * for the services rendered to the patient.) 1293 */ 1294 public PaymentReconciliation setRequestProvider(Reference value) { 1295 this.requestProvider = value; 1296 return this; 1297 } 1298 1299 /** 1300 * @return {@link #requestProvider} The actual object that is the target of the 1301 * reference. The reference library doesn't populate this, but you can 1302 * use it to hold the resource if you resolve it. (The practitioner who 1303 * is responsible for the services rendered to the patient.) 1304 */ 1305 public Practitioner getRequestProviderTarget() { 1306 if (this.requestProviderTarget == null) 1307 if (Configuration.errorOnAutoCreate()) 1308 throw new Error("Attempt to auto-create PaymentReconciliation.requestProvider"); 1309 else if (Configuration.doAutoCreate()) 1310 this.requestProviderTarget = new Practitioner(); // aa 1311 return this.requestProviderTarget; 1312 } 1313 1314 /** 1315 * @param value {@link #requestProvider} The actual object that is the target of 1316 * the reference. The reference library doesn't use these, but you 1317 * can use it to hold the resource if you resolve it. (The 1318 * practitioner who is responsible for the services rendered to the 1319 * patient.) 1320 */ 1321 public PaymentReconciliation setRequestProviderTarget(Practitioner value) { 1322 this.requestProviderTarget = value; 1323 return this; 1324 } 1325 1326 /** 1327 * @return {@link #requestOrganization} (The organization which is responsible 1328 * for the services rendered to the patient.) 1329 */ 1330 public Reference getRequestOrganization() { 1331 if (this.requestOrganization == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create PaymentReconciliation.requestOrganization"); 1334 else if (Configuration.doAutoCreate()) 1335 this.requestOrganization = new Reference(); // cc 1336 return this.requestOrganization; 1337 } 1338 1339 public boolean hasRequestOrganization() { 1340 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 1341 } 1342 1343 /** 1344 * @param value {@link #requestOrganization} (The organization which is 1345 * responsible for the services rendered to the patient.) 1346 */ 1347 public PaymentReconciliation setRequestOrganization(Reference value) { 1348 this.requestOrganization = value; 1349 return this; 1350 } 1351 1352 /** 1353 * @return {@link #requestOrganization} The actual object that is the target of 1354 * the reference. The reference library doesn't populate this, but you 1355 * can use it to hold the resource if you resolve it. (The organization 1356 * which is responsible for the services rendered to the patient.) 1357 */ 1358 public Organization getRequestOrganizationTarget() { 1359 if (this.requestOrganizationTarget == null) 1360 if (Configuration.errorOnAutoCreate()) 1361 throw new Error("Attempt to auto-create PaymentReconciliation.requestOrganization"); 1362 else if (Configuration.doAutoCreate()) 1363 this.requestOrganizationTarget = new Organization(); // aa 1364 return this.requestOrganizationTarget; 1365 } 1366 1367 /** 1368 * @param value {@link #requestOrganization} The actual object that is the 1369 * target of the reference. The reference library doesn't use 1370 * these, but you can use it to hold the resource if you resolve 1371 * it. (The organization which is responsible for the services 1372 * rendered to the patient.) 1373 */ 1374 public PaymentReconciliation setRequestOrganizationTarget(Organization value) { 1375 this.requestOrganizationTarget = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #detail} (List of individual settlement amounts and the 1381 * corresponding transaction.) 1382 */ 1383 public List<DetailsComponent> getDetail() { 1384 if (this.detail == null) 1385 this.detail = new ArrayList<DetailsComponent>(); 1386 return this.detail; 1387 } 1388 1389 public boolean hasDetail() { 1390 if (this.detail == null) 1391 return false; 1392 for (DetailsComponent item : this.detail) 1393 if (!item.isEmpty()) 1394 return true; 1395 return false; 1396 } 1397 1398 /** 1399 * @return {@link #detail} (List of individual settlement amounts and the 1400 * corresponding transaction.) 1401 */ 1402 // syntactic sugar 1403 public DetailsComponent addDetail() { // 3 1404 DetailsComponent t = new DetailsComponent(); 1405 if (this.detail == null) 1406 this.detail = new ArrayList<DetailsComponent>(); 1407 this.detail.add(t); 1408 return t; 1409 } 1410 1411 // syntactic sugar 1412 public PaymentReconciliation addDetail(DetailsComponent t) { // 3 1413 if (t == null) 1414 return this; 1415 if (this.detail == null) 1416 this.detail = new ArrayList<DetailsComponent>(); 1417 this.detail.add(t); 1418 return this; 1419 } 1420 1421 /** 1422 * @return {@link #form} (The form to be used for printing the content.) 1423 */ 1424 public Coding getForm() { 1425 if (this.form == null) 1426 if (Configuration.errorOnAutoCreate()) 1427 throw new Error("Attempt to auto-create PaymentReconciliation.form"); 1428 else if (Configuration.doAutoCreate()) 1429 this.form = new Coding(); // cc 1430 return this.form; 1431 } 1432 1433 public boolean hasForm() { 1434 return this.form != null && !this.form.isEmpty(); 1435 } 1436 1437 /** 1438 * @param value {@link #form} (The form to be used for printing the content.) 1439 */ 1440 public PaymentReconciliation setForm(Coding value) { 1441 this.form = value; 1442 return this; 1443 } 1444 1445 /** 1446 * @return {@link #total} (Total payment amount.) 1447 */ 1448 public Money getTotal() { 1449 if (this.total == null) 1450 if (Configuration.errorOnAutoCreate()) 1451 throw new Error("Attempt to auto-create PaymentReconciliation.total"); 1452 else if (Configuration.doAutoCreate()) 1453 this.total = new Money(); // cc 1454 return this.total; 1455 } 1456 1457 public boolean hasTotal() { 1458 return this.total != null && !this.total.isEmpty(); 1459 } 1460 1461 /** 1462 * @param value {@link #total} (Total payment amount.) 1463 */ 1464 public PaymentReconciliation setTotal(Money value) { 1465 this.total = value; 1466 return this; 1467 } 1468 1469 /** 1470 * @return {@link #note} (Suite of notes.) 1471 */ 1472 public List<NotesComponent> getNote() { 1473 if (this.note == null) 1474 this.note = new ArrayList<NotesComponent>(); 1475 return this.note; 1476 } 1477 1478 public boolean hasNote() { 1479 if (this.note == null) 1480 return false; 1481 for (NotesComponent item : this.note) 1482 if (!item.isEmpty()) 1483 return true; 1484 return false; 1485 } 1486 1487 /** 1488 * @return {@link #note} (Suite of notes.) 1489 */ 1490 // syntactic sugar 1491 public NotesComponent addNote() { // 3 1492 NotesComponent t = new NotesComponent(); 1493 if (this.note == null) 1494 this.note = new ArrayList<NotesComponent>(); 1495 this.note.add(t); 1496 return t; 1497 } 1498 1499 // syntactic sugar 1500 public PaymentReconciliation addNote(NotesComponent t) { // 3 1501 if (t == null) 1502 return this; 1503 if (this.note == null) 1504 this.note = new ArrayList<NotesComponent>(); 1505 this.note.add(t); 1506 return this; 1507 } 1508 1509 protected void listChildren(List<Property> childrenList) { 1510 super.listChildren(childrenList); 1511 childrenList.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 1512 java.lang.Integer.MAX_VALUE, identifier)); 1513 childrenList.add(new Property("request", "Reference(ProcessRequest)", "Original request resource reference.", 0, 1514 java.lang.Integer.MAX_VALUE, request)); 1515 childrenList.add(new Property("outcome", "code", "Transaction status: error, complete.", 0, 1516 java.lang.Integer.MAX_VALUE, outcome)); 1517 childrenList.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1518 java.lang.Integer.MAX_VALUE, disposition)); 1519 childrenList.add(new Property("ruleset", "Coding", 1520 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 1521 0, java.lang.Integer.MAX_VALUE, ruleset)); 1522 childrenList.add(new Property("originalRuleset", "Coding", 1523 "The style (standard) and version of the original material which was converted into this resource.", 0, 1524 java.lang.Integer.MAX_VALUE, originalRuleset)); 1525 childrenList.add( 1526 new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 1527 0, java.lang.Integer.MAX_VALUE, created)); 1528 childrenList.add(new Property("period", "Period", 1529 "The period of time for which payments have been gathered into this bulk payment for settlement.", 0, 1530 java.lang.Integer.MAX_VALUE, period)); 1531 childrenList.add(new Property("organization", "Reference(Organization)", 1532 "The Insurer who produced this adjudicated response.", 0, java.lang.Integer.MAX_VALUE, organization)); 1533 childrenList.add(new Property("requestProvider", "Reference(Practitioner)", 1534 "The practitioner who is responsible for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 1535 requestProvider)); 1536 childrenList.add(new Property("requestOrganization", "Reference(Organization)", 1537 "The organization which is responsible for the services rendered to the patient.", 0, 1538 java.lang.Integer.MAX_VALUE, requestOrganization)); 1539 childrenList 1540 .add(new Property("detail", "", "List of individual settlement amounts and the corresponding transaction.", 0, 1541 java.lang.Integer.MAX_VALUE, detail)); 1542 childrenList.add(new Property("form", "Coding", "The form to be used for printing the content.", 0, 1543 java.lang.Integer.MAX_VALUE, form)); 1544 childrenList.add(new Property("total", "Money", "Total payment amount.", 0, java.lang.Integer.MAX_VALUE, total)); 1545 childrenList.add(new Property("note", "", "Suite of notes.", 0, java.lang.Integer.MAX_VALUE, note)); 1546 } 1547 1548 @Override 1549 public void setProperty(String name, Base value) throws FHIRException { 1550 if (name.equals("identifier")) 1551 this.getIdentifier().add(castToIdentifier(value)); 1552 else if (name.equals("request")) 1553 this.request = castToReference(value); // Reference 1554 else if (name.equals("outcome")) 1555 this.outcome = new RemittanceOutcomeEnumFactory().fromType(value); // Enumeration<RemittanceOutcome> 1556 else if (name.equals("disposition")) 1557 this.disposition = castToString(value); // StringType 1558 else if (name.equals("ruleset")) 1559 this.ruleset = castToCoding(value); // Coding 1560 else if (name.equals("originalRuleset")) 1561 this.originalRuleset = castToCoding(value); // Coding 1562 else if (name.equals("created")) 1563 this.created = castToDateTime(value); // DateTimeType 1564 else if (name.equals("period")) 1565 this.period = castToPeriod(value); // Period 1566 else if (name.equals("organization")) 1567 this.organization = castToReference(value); // Reference 1568 else if (name.equals("requestProvider")) 1569 this.requestProvider = castToReference(value); // Reference 1570 else if (name.equals("requestOrganization")) 1571 this.requestOrganization = castToReference(value); // Reference 1572 else if (name.equals("detail")) 1573 this.getDetail().add((DetailsComponent) value); 1574 else if (name.equals("form")) 1575 this.form = castToCoding(value); // Coding 1576 else if (name.equals("total")) 1577 this.total = castToMoney(value); // Money 1578 else if (name.equals("note")) 1579 this.getNote().add((NotesComponent) value); 1580 else 1581 super.setProperty(name, value); 1582 } 1583 1584 @Override 1585 public Base addChild(String name) throws FHIRException { 1586 if (name.equals("identifier")) { 1587 return addIdentifier(); 1588 } else if (name.equals("request")) { 1589 this.request = new Reference(); 1590 return this.request; 1591 } else if (name.equals("outcome")) { 1592 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.outcome"); 1593 } else if (name.equals("disposition")) { 1594 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.disposition"); 1595 } else if (name.equals("ruleset")) { 1596 this.ruleset = new Coding(); 1597 return this.ruleset; 1598 } else if (name.equals("originalRuleset")) { 1599 this.originalRuleset = new Coding(); 1600 return this.originalRuleset; 1601 } else if (name.equals("created")) { 1602 throw new FHIRException("Cannot call addChild on a singleton property PaymentReconciliation.created"); 1603 } else if (name.equals("period")) { 1604 this.period = new Period(); 1605 return this.period; 1606 } else if (name.equals("organization")) { 1607 this.organization = new Reference(); 1608 return this.organization; 1609 } else if (name.equals("requestProvider")) { 1610 this.requestProvider = new Reference(); 1611 return this.requestProvider; 1612 } else if (name.equals("requestOrganization")) { 1613 this.requestOrganization = new Reference(); 1614 return this.requestOrganization; 1615 } else if (name.equals("detail")) { 1616 return addDetail(); 1617 } else if (name.equals("form")) { 1618 this.form = new Coding(); 1619 return this.form; 1620 } else if (name.equals("total")) { 1621 this.total = new Money(); 1622 return this.total; 1623 } else if (name.equals("note")) { 1624 return addNote(); 1625 } else 1626 return super.addChild(name); 1627 } 1628 1629 public String fhirType() { 1630 return "PaymentReconciliation"; 1631 1632 } 1633 1634 public PaymentReconciliation copy() { 1635 PaymentReconciliation dst = new PaymentReconciliation(); 1636 copyValues(dst); 1637 if (identifier != null) { 1638 dst.identifier = new ArrayList<Identifier>(); 1639 for (Identifier i : identifier) 1640 dst.identifier.add(i.copy()); 1641 } 1642 ; 1643 dst.request = request == null ? null : request.copy(); 1644 dst.outcome = outcome == null ? null : outcome.copy(); 1645 dst.disposition = disposition == null ? null : disposition.copy(); 1646 dst.ruleset = ruleset == null ? null : ruleset.copy(); 1647 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 1648 dst.created = created == null ? null : created.copy(); 1649 dst.period = period == null ? null : period.copy(); 1650 dst.organization = organization == null ? null : organization.copy(); 1651 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 1652 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 1653 if (detail != null) { 1654 dst.detail = new ArrayList<DetailsComponent>(); 1655 for (DetailsComponent i : detail) 1656 dst.detail.add(i.copy()); 1657 } 1658 ; 1659 dst.form = form == null ? null : form.copy(); 1660 dst.total = total == null ? null : total.copy(); 1661 if (note != null) { 1662 dst.note = new ArrayList<NotesComponent>(); 1663 for (NotesComponent i : note) 1664 dst.note.add(i.copy()); 1665 } 1666 ; 1667 return dst; 1668 } 1669 1670 protected PaymentReconciliation typedCopy() { 1671 return copy(); 1672 } 1673 1674 @Override 1675 public boolean equalsDeep(Base other) { 1676 if (!super.equalsDeep(other)) 1677 return false; 1678 if (!(other instanceof PaymentReconciliation)) 1679 return false; 1680 PaymentReconciliation o = (PaymentReconciliation) other; 1681 return compareDeep(identifier, o.identifier, true) && compareDeep(request, o.request, true) 1682 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) 1683 && compareDeep(ruleset, o.ruleset, true) && compareDeep(originalRuleset, o.originalRuleset, true) 1684 && compareDeep(created, o.created, true) && compareDeep(period, o.period, true) 1685 && compareDeep(organization, o.organization, true) && compareDeep(requestProvider, o.requestProvider, true) 1686 && compareDeep(requestOrganization, o.requestOrganization, true) && compareDeep(detail, o.detail, true) 1687 && compareDeep(form, o.form, true) && compareDeep(total, o.total, true) && compareDeep(note, o.note, true); 1688 } 1689 1690 @Override 1691 public boolean equalsShallow(Base other) { 1692 if (!super.equalsShallow(other)) 1693 return false; 1694 if (!(other instanceof PaymentReconciliation)) 1695 return false; 1696 PaymentReconciliation o = (PaymentReconciliation) other; 1697 return compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) 1698 && compareValues(created, o.created, true); 1699 } 1700 1701 public boolean isEmpty() { 1702 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (request == null || request.isEmpty()) 1703 && (outcome == null || outcome.isEmpty()) && (disposition == null || disposition.isEmpty()) 1704 && (ruleset == null || ruleset.isEmpty()) && (originalRuleset == null || originalRuleset.isEmpty()) 1705 && (created == null || created.isEmpty()) && (period == null || period.isEmpty()) 1706 && (organization == null || organization.isEmpty()) && (requestProvider == null || requestProvider.isEmpty()) 1707 && (requestOrganization == null || requestOrganization.isEmpty()) && (detail == null || detail.isEmpty()) 1708 && (form == null || form.isEmpty()) && (total == null || total.isEmpty()) && (note == null || note.isEmpty()); 1709 } 1710 1711 @Override 1712 public ResourceType getResourceType() { 1713 return ResourceType.PaymentReconciliation; 1714 } 1715 1716 @SearchParamDefinition(name = "identifier", path = "PaymentReconciliation.identifier", description = "The business identifier of the Explanation of Benefit", type = "token") 1717 public static final String SP_IDENTIFIER = "identifier"; 1718 1719}