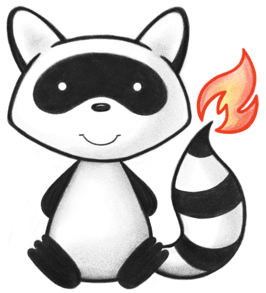
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.Date; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.DatatypeDef; 038import ca.uhn.fhir.model.api.annotation.Description; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.exceptions.FHIRException; 041 042/** 043 * A time period defined by a start and end date and optionally time. 044 */ 045@DatatypeDef(name = "Period") 046public class Period extends Type implements ICompositeType { 047 048 /** 049 * The start of the period. The boundary is inclusive. 050 */ 051 @Child(name = "start", type = { DateTimeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 052 @Description(shortDefinition = "Starting time with inclusive boundary", formalDefinition = "The start of the period. The boundary is inclusive.") 053 protected DateTimeType start; 054 055 /** 056 * The end of the period. If the end of the period is missing, it means that the 057 * period is ongoing. The start may be in the past, and the end date in the 058 * future, which means that period is expected/planned to end at that time. 059 */ 060 @Child(name = "end", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "End time with inclusive boundary, if not ongoing", formalDefinition = "The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.") 062 protected DateTimeType end; 063 064 private static final long serialVersionUID = 649791751L; 065 066 /* 067 * Constructor 068 */ 069 public Period() { 070 super(); 071 } 072 073 /** 074 * @return {@link #start} (The start of the period. The boundary is inclusive.). 075 * This is the underlying object with id, value and extensions. The 076 * accessor "getStart" gives direct access to the value 077 */ 078 public DateTimeType getStartElement() { 079 if (this.start == null) 080 if (Configuration.errorOnAutoCreate()) 081 throw new Error("Attempt to auto-create Period.start"); 082 else if (Configuration.doAutoCreate()) 083 this.start = new DateTimeType(); // bb 084 return this.start; 085 } 086 087 public boolean hasStartElement() { 088 return this.start != null && !this.start.isEmpty(); 089 } 090 091 public boolean hasStart() { 092 return this.start != null && !this.start.isEmpty(); 093 } 094 095 /** 096 * @param value {@link #start} (The start of the period. The boundary is 097 * inclusive.). This is the underlying object with id, value and 098 * extensions. The accessor "getStart" gives direct access to the 099 * value 100 */ 101 public Period setStartElement(DateTimeType value) { 102 this.start = value; 103 return this; 104 } 105 106 /** 107 * @return The start of the period. The boundary is inclusive. 108 */ 109 public Date getStart() { 110 return this.start == null ? null : this.start.getValue(); 111 } 112 113 /** 114 * @param value The start of the period. The boundary is inclusive. 115 */ 116 public Period setStart(Date value) { 117 if (value == null) 118 this.start = null; 119 else { 120 if (this.start == null) 121 this.start = new DateTimeType(); 122 this.start.setValue(value); 123 } 124 return this; 125 } 126 127 /** 128 * @return {@link #end} (The end of the period. If the end of the period is 129 * missing, it means that the period is ongoing. The start may be in the 130 * past, and the end date in the future, which means that period is 131 * expected/planned to end at that time.). This is the underlying object 132 * with id, value and extensions. The accessor "getEnd" gives direct 133 * access to the value 134 */ 135 public DateTimeType getEndElement() { 136 if (this.end == null) 137 if (Configuration.errorOnAutoCreate()) 138 throw new Error("Attempt to auto-create Period.end"); 139 else if (Configuration.doAutoCreate()) 140 this.end = new DateTimeType(); // bb 141 return this.end; 142 } 143 144 public boolean hasEndElement() { 145 return this.end != null && !this.end.isEmpty(); 146 } 147 148 public boolean hasEnd() { 149 return this.end != null && !this.end.isEmpty(); 150 } 151 152 /** 153 * @param value {@link #end} (The end of the period. If the end of the period is 154 * missing, it means that the period is ongoing. The start may be 155 * in the past, and the end date in the future, which means that 156 * period is expected/planned to end at that time.). This is the 157 * underlying object with id, value and extensions. The accessor 158 * "getEnd" gives direct access to the value 159 */ 160 public Period setEndElement(DateTimeType value) { 161 this.end = value; 162 return this; 163 } 164 165 /** 166 * @return The end of the period. If the end of the period is missing, it means 167 * that the period is ongoing. The start may be in the past, and the end 168 * date in the future, which means that period is expected/planned to 169 * end at that time. 170 */ 171 public Date getEnd() { 172 return this.end == null ? null : this.end.getValue(); 173 } 174 175 /** 176 * @param value The end of the period. If the end of the period is missing, it 177 * means that the period is ongoing. The start may be in the past, 178 * and the end date in the future, which means that period is 179 * expected/planned to end at that time. 180 */ 181 public Period setEnd(Date value) { 182 if (value == null) 183 this.end = null; 184 else { 185 if (this.end == null) 186 this.end = new DateTimeType(); 187 this.end.setValue(value); 188 } 189 return this; 190 } 191 192 protected void listChildren(List<Property> childrenList) { 193 super.listChildren(childrenList); 194 childrenList.add(new Property("start", "dateTime", "The start of the period. The boundary is inclusive.", 0, 195 java.lang.Integer.MAX_VALUE, start)); 196 childrenList.add(new Property("end", "dateTime", 197 "The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.", 198 0, java.lang.Integer.MAX_VALUE, end)); 199 } 200 201 @Override 202 public void setProperty(String name, Base value) throws FHIRException { 203 if (name.equals("start")) 204 this.start = castToDateTime(value); // DateTimeType 205 else if (name.equals("end")) 206 this.end = castToDateTime(value); // DateTimeType 207 else 208 super.setProperty(name, value); 209 } 210 211 @Override 212 public Base addChild(String name) throws FHIRException { 213 if (name.equals("start")) { 214 throw new FHIRException("Cannot call addChild on a singleton property Period.start"); 215 } else if (name.equals("end")) { 216 throw new FHIRException("Cannot call addChild on a singleton property Period.end"); 217 } else 218 return super.addChild(name); 219 } 220 221 public String fhirType() { 222 return "Period"; 223 224 } 225 226 public Period copy() { 227 Period dst = new Period(); 228 copyValues(dst); 229 dst.start = start == null ? null : start.copy(); 230 dst.end = end == null ? null : end.copy(); 231 return dst; 232 } 233 234 protected Period typedCopy() { 235 return copy(); 236 } 237 238 @Override 239 public boolean equalsDeep(Base other) { 240 if (!super.equalsDeep(other)) 241 return false; 242 if (!(other instanceof Period)) 243 return false; 244 Period o = (Period) other; 245 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 246 } 247 248 @Override 249 public boolean equalsShallow(Base other) { 250 if (!super.equalsShallow(other)) 251 return false; 252 if (!(other instanceof Period)) 253 return false; 254 Period o = (Period) other; 255 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 256 } 257 258 public boolean isEmpty() { 259 return super.isEmpty() && (start == null || start.isEmpty()) && (end == null || end.isEmpty()); 260 } 261 262}