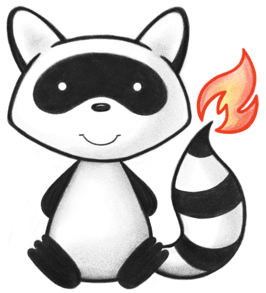
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender; 038import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046 047/** 048 * Demographics and administrative information about a person independent of a 049 * specific health-related context. 050 */ 051@ResourceDef(name = "Person", profile = "http://hl7.org/fhir/Profile/Person") 052public class Person extends DomainResource { 053 054 public enum IdentityAssuranceLevel { 055 /** 056 * Little or no confidence in the asserted identity's accuracy. 057 */ 058 LEVEL1, 059 /** 060 * Some confidence in the asserted identity's accuracy. 061 */ 062 LEVEL2, 063 /** 064 * High confidence in the asserted identity's accuracy. 065 */ 066 LEVEL3, 067 /** 068 * Very high confidence in the asserted identity's accuracy. 069 */ 070 LEVEL4, 071 /** 072 * added to help the parsers 073 */ 074 NULL; 075 076 public static IdentityAssuranceLevel fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("level1".equals(codeString)) 080 return LEVEL1; 081 if ("level2".equals(codeString)) 082 return LEVEL2; 083 if ("level3".equals(codeString)) 084 return LEVEL3; 085 if ("level4".equals(codeString)) 086 return LEVEL4; 087 throw new FHIRException("Unknown IdentityAssuranceLevel code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case LEVEL1: 093 return "level1"; 094 case LEVEL2: 095 return "level2"; 096 case LEVEL3: 097 return "level3"; 098 case LEVEL4: 099 return "level4"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case LEVEL1: 110 return "http://hl7.org/fhir/identity-assuranceLevel"; 111 case LEVEL2: 112 return "http://hl7.org/fhir/identity-assuranceLevel"; 113 case LEVEL3: 114 return "http://hl7.org/fhir/identity-assuranceLevel"; 115 case LEVEL4: 116 return "http://hl7.org/fhir/identity-assuranceLevel"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDefinition() { 125 switch (this) { 126 case LEVEL1: 127 return "Little or no confidence in the asserted identity's accuracy."; 128 case LEVEL2: 129 return "Some confidence in the asserted identity's accuracy."; 130 case LEVEL3: 131 return "High confidence in the asserted identity's accuracy."; 132 case LEVEL4: 133 return "Very high confidence in the asserted identity's accuracy."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case LEVEL1: 144 return "Level 1"; 145 case LEVEL2: 146 return "Level 2"; 147 case LEVEL3: 148 return "Level 3"; 149 case LEVEL4: 150 return "Level 4"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 } 158 159 public static class IdentityAssuranceLevelEnumFactory implements EnumFactory<IdentityAssuranceLevel> { 160 public IdentityAssuranceLevel fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("level1".equals(codeString)) 165 return IdentityAssuranceLevel.LEVEL1; 166 if ("level2".equals(codeString)) 167 return IdentityAssuranceLevel.LEVEL2; 168 if ("level3".equals(codeString)) 169 return IdentityAssuranceLevel.LEVEL3; 170 if ("level4".equals(codeString)) 171 return IdentityAssuranceLevel.LEVEL4; 172 throw new IllegalArgumentException("Unknown IdentityAssuranceLevel code '" + codeString + "'"); 173 } 174 175 public Enumeration<IdentityAssuranceLevel> fromType(Base code) throws FHIRException { 176 if (code == null || code.isEmpty()) 177 return null; 178 String codeString = ((PrimitiveType) code).asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("level1".equals(codeString)) 182 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL1); 183 if ("level2".equals(codeString)) 184 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL2); 185 if ("level3".equals(codeString)) 186 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL3); 187 if ("level4".equals(codeString)) 188 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL4); 189 throw new FHIRException("Unknown IdentityAssuranceLevel code '" + codeString + "'"); 190 } 191 192 public String toCode(IdentityAssuranceLevel code) 193 { 194 if (code == IdentityAssuranceLevel.NULL) 195 return null; 196 if (code == IdentityAssuranceLevel.LEVEL1) 197 return "level1"; 198 if (code == IdentityAssuranceLevel.LEVEL2) 199 return "level2"; 200 if (code == IdentityAssuranceLevel.LEVEL3) 201 return "level3"; 202 if (code == IdentityAssuranceLevel.LEVEL4) 203 return "level4"; 204 return "?"; 205 } 206 } 207 208 @Block() 209 public static class PersonLinkComponent extends BackboneElement implements IBaseBackboneElement { 210 /** 211 * The resource to which this actual person is associated. 212 */ 213 @Child(name = "target", type = { Patient.class, Practitioner.class, RelatedPerson.class, 214 Person.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 215 @Description(shortDefinition = "The resource to which this actual person is associated", formalDefinition = "The resource to which this actual person is associated.") 216 protected Reference target; 217 218 /** 219 * The actual object that is the target of the reference (The resource to which 220 * this actual person is associated.) 221 */ 222 protected Resource targetTarget; 223 224 /** 225 * Level of assurance that this link is actually associated with the target 226 * resource. 227 */ 228 @Child(name = "assurance", type = { 229 CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 230 @Description(shortDefinition = "level1 | level2 | level3 | level4", formalDefinition = "Level of assurance that this link is actually associated with the target resource.") 231 protected Enumeration<IdentityAssuranceLevel> assurance; 232 233 private static final long serialVersionUID = 508763647L; 234 235 /* 236 * Constructor 237 */ 238 public PersonLinkComponent() { 239 super(); 240 } 241 242 /* 243 * Constructor 244 */ 245 public PersonLinkComponent(Reference target) { 246 super(); 247 this.target = target; 248 } 249 250 /** 251 * @return {@link #target} (The resource to which this actual person is 252 * associated.) 253 */ 254 public Reference getTarget() { 255 if (this.target == null) 256 if (Configuration.errorOnAutoCreate()) 257 throw new Error("Attempt to auto-create PersonLinkComponent.target"); 258 else if (Configuration.doAutoCreate()) 259 this.target = new Reference(); // cc 260 return this.target; 261 } 262 263 public boolean hasTarget() { 264 return this.target != null && !this.target.isEmpty(); 265 } 266 267 /** 268 * @param value {@link #target} (The resource to which this actual person is 269 * associated.) 270 */ 271 public PersonLinkComponent setTarget(Reference value) { 272 this.target = value; 273 return this; 274 } 275 276 /** 277 * @return {@link #target} The actual object that is the target of the 278 * reference. The reference library doesn't populate this, but you can 279 * use it to hold the resource if you resolve it. (The resource to which 280 * this actual person is associated.) 281 */ 282 public Resource getTargetTarget() { 283 return this.targetTarget; 284 } 285 286 /** 287 * @param value {@link #target} The actual object that is the target of the 288 * reference. The reference library doesn't use these, but you can 289 * use it to hold the resource if you resolve it. (The resource to 290 * which this actual person is associated.) 291 */ 292 public PersonLinkComponent setTargetTarget(Resource value) { 293 this.targetTarget = value; 294 return this; 295 } 296 297 /** 298 * @return {@link #assurance} (Level of assurance that this link is actually 299 * associated with the target resource.). This is the underlying object 300 * with id, value and extensions. The accessor "getAssurance" gives 301 * direct access to the value 302 */ 303 public Enumeration<IdentityAssuranceLevel> getAssuranceElement() { 304 if (this.assurance == null) 305 if (Configuration.errorOnAutoCreate()) 306 throw new Error("Attempt to auto-create PersonLinkComponent.assurance"); 307 else if (Configuration.doAutoCreate()) 308 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); // bb 309 return this.assurance; 310 } 311 312 public boolean hasAssuranceElement() { 313 return this.assurance != null && !this.assurance.isEmpty(); 314 } 315 316 public boolean hasAssurance() { 317 return this.assurance != null && !this.assurance.isEmpty(); 318 } 319 320 /** 321 * @param value {@link #assurance} (Level of assurance that this link is 322 * actually associated with the target resource.). This is the 323 * underlying object with id, value and extensions. The accessor 324 * "getAssurance" gives direct access to the value 325 */ 326 public PersonLinkComponent setAssuranceElement(Enumeration<IdentityAssuranceLevel> value) { 327 this.assurance = value; 328 return this; 329 } 330 331 /** 332 * @return Level of assurance that this link is actually associated with the 333 * target resource. 334 */ 335 public IdentityAssuranceLevel getAssurance() { 336 return this.assurance == null ? null : this.assurance.getValue(); 337 } 338 339 /** 340 * @param value Level of assurance that this link is actually associated with 341 * the target resource. 342 */ 343 public PersonLinkComponent setAssurance(IdentityAssuranceLevel value) { 344 if (value == null) 345 this.assurance = null; 346 else { 347 if (this.assurance == null) 348 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); 349 this.assurance.setValue(value); 350 } 351 return this; 352 } 353 354 protected void listChildren(List<Property> childrenList) { 355 super.listChildren(childrenList); 356 childrenList.add(new Property("target", "Reference(Patient|Practitioner|RelatedPerson|Person)", 357 "The resource to which this actual person is associated.", 0, java.lang.Integer.MAX_VALUE, target)); 358 childrenList.add(new Property("assurance", "code", 359 "Level of assurance that this link is actually associated with the target resource.", 0, 360 java.lang.Integer.MAX_VALUE, assurance)); 361 } 362 363 @Override 364 public void setProperty(String name, Base value) throws FHIRException { 365 if (name.equals("target")) 366 this.target = castToReference(value); // Reference 367 else if (name.equals("assurance")) 368 this.assurance = new IdentityAssuranceLevelEnumFactory().fromType(value); // Enumeration<IdentityAssuranceLevel> 369 else 370 super.setProperty(name, value); 371 } 372 373 @Override 374 public Base addChild(String name) throws FHIRException { 375 if (name.equals("target")) { 376 this.target = new Reference(); 377 return this.target; 378 } else if (name.equals("assurance")) { 379 throw new FHIRException("Cannot call addChild on a singleton property Person.assurance"); 380 } else 381 return super.addChild(name); 382 } 383 384 public PersonLinkComponent copy() { 385 PersonLinkComponent dst = new PersonLinkComponent(); 386 copyValues(dst); 387 dst.target = target == null ? null : target.copy(); 388 dst.assurance = assurance == null ? null : assurance.copy(); 389 return dst; 390 } 391 392 @Override 393 public boolean equalsDeep(Base other) { 394 if (!super.equalsDeep(other)) 395 return false; 396 if (!(other instanceof PersonLinkComponent)) 397 return false; 398 PersonLinkComponent o = (PersonLinkComponent) other; 399 return compareDeep(target, o.target, true) && compareDeep(assurance, o.assurance, true); 400 } 401 402 @Override 403 public boolean equalsShallow(Base other) { 404 if (!super.equalsShallow(other)) 405 return false; 406 if (!(other instanceof PersonLinkComponent)) 407 return false; 408 PersonLinkComponent o = (PersonLinkComponent) other; 409 return compareValues(assurance, o.assurance, true); 410 } 411 412 public boolean isEmpty() { 413 return super.isEmpty() && (target == null || target.isEmpty()) && (assurance == null || assurance.isEmpty()); 414 } 415 416 public String fhirType() { 417 return "Person.link"; 418 419 } 420 421 } 422 423 /** 424 * Identifier for a person within a particular scope. 425 */ 426 @Child(name = "identifier", type = { 427 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 428 @Description(shortDefinition = "A human identifier for this person", formalDefinition = "Identifier for a person within a particular scope.") 429 protected List<Identifier> identifier; 430 431 /** 432 * A name associated with the person. 433 */ 434 @Child(name = "name", type = { 435 HumanName.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 436 @Description(shortDefinition = "A name associated with the person", formalDefinition = "A name associated with the person.") 437 protected List<HumanName> name; 438 439 /** 440 * A contact detail for the person, e.g. a telephone number or an email address. 441 */ 442 @Child(name = "telecom", type = { 443 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 444 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 445 protected List<ContactPoint> telecom; 446 447 /** 448 * Administrative Gender. 449 */ 450 @Child(name = "gender", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 451 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender.") 452 protected Enumeration<AdministrativeGender> gender; 453 454 /** 455 * The birth date for the person. 456 */ 457 @Child(name = "birthDate", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 458 @Description(shortDefinition = "The date on which the person was born", formalDefinition = "The birth date for the person.") 459 protected DateType birthDate; 460 461 /** 462 * One or more addresses for the person. 463 */ 464 @Child(name = "address", type = { 465 Address.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 466 @Description(shortDefinition = "One or more addresses for the person", formalDefinition = "One or more addresses for the person.") 467 protected List<Address> address; 468 469 /** 470 * An image that can be displayed as a thumbnail of the person to enhance the 471 * identification of the individual. 472 */ 473 @Child(name = "photo", type = { Attachment.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 474 @Description(shortDefinition = "Image of the person", formalDefinition = "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.") 475 protected Attachment photo; 476 477 /** 478 * The organization that is the custodian of the person record. 479 */ 480 @Child(name = "managingOrganization", type = { 481 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 482 @Description(shortDefinition = "The organization that is the custodian of the person record", formalDefinition = "The organization that is the custodian of the person record.") 483 protected Reference managingOrganization; 484 485 /** 486 * The actual object that is the target of the reference (The organization that 487 * is the custodian of the person record.) 488 */ 489 protected Organization managingOrganizationTarget; 490 491 /** 492 * Whether this person's record is in active use. 493 */ 494 @Child(name = "active", type = { BooleanType.class }, order = 8, min = 0, max = 1, modifier = true, summary = true) 495 @Description(shortDefinition = "This person's record is in active use", formalDefinition = "Whether this person's record is in active use.") 496 protected BooleanType active; 497 498 /** 499 * Link to a resource that concerns the same actual person. 500 */ 501 @Child(name = "link", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 502 @Description(shortDefinition = "Link to a resource that concerns the same actual person", formalDefinition = "Link to a resource that concerns the same actual person.") 503 protected List<PersonLinkComponent> link; 504 505 private static final long serialVersionUID = -117464654L; 506 507 /* 508 * Constructor 509 */ 510 public Person() { 511 super(); 512 } 513 514 /** 515 * @return {@link #identifier} (Identifier for a person within a particular 516 * scope.) 517 */ 518 public List<Identifier> getIdentifier() { 519 if (this.identifier == null) 520 this.identifier = new ArrayList<Identifier>(); 521 return this.identifier; 522 } 523 524 public boolean hasIdentifier() { 525 if (this.identifier == null) 526 return false; 527 for (Identifier item : this.identifier) 528 if (!item.isEmpty()) 529 return true; 530 return false; 531 } 532 533 /** 534 * @return {@link #identifier} (Identifier for a person within a particular 535 * scope.) 536 */ 537 // syntactic sugar 538 public Identifier addIdentifier() { // 3 539 Identifier t = new Identifier(); 540 if (this.identifier == null) 541 this.identifier = new ArrayList<Identifier>(); 542 this.identifier.add(t); 543 return t; 544 } 545 546 // syntactic sugar 547 public Person addIdentifier(Identifier t) { // 3 548 if (t == null) 549 return this; 550 if (this.identifier == null) 551 this.identifier = new ArrayList<Identifier>(); 552 this.identifier.add(t); 553 return this; 554 } 555 556 /** 557 * @return {@link #name} (A name associated with the person.) 558 */ 559 public List<HumanName> getName() { 560 if (this.name == null) 561 this.name = new ArrayList<HumanName>(); 562 return this.name; 563 } 564 565 public boolean hasName() { 566 if (this.name == null) 567 return false; 568 for (HumanName item : this.name) 569 if (!item.isEmpty()) 570 return true; 571 return false; 572 } 573 574 /** 575 * @return {@link #name} (A name associated with the person.) 576 */ 577 // syntactic sugar 578 public HumanName addName() { // 3 579 HumanName t = new HumanName(); 580 if (this.name == null) 581 this.name = new ArrayList<HumanName>(); 582 this.name.add(t); 583 return t; 584 } 585 586 // syntactic sugar 587 public Person addName(HumanName t) { // 3 588 if (t == null) 589 return this; 590 if (this.name == null) 591 this.name = new ArrayList<HumanName>(); 592 this.name.add(t); 593 return this; 594 } 595 596 /** 597 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 598 * number or an email address.) 599 */ 600 public List<ContactPoint> getTelecom() { 601 if (this.telecom == null) 602 this.telecom = new ArrayList<ContactPoint>(); 603 return this.telecom; 604 } 605 606 public boolean hasTelecom() { 607 if (this.telecom == null) 608 return false; 609 for (ContactPoint item : this.telecom) 610 if (!item.isEmpty()) 611 return true; 612 return false; 613 } 614 615 /** 616 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 617 * number or an email address.) 618 */ 619 // syntactic sugar 620 public ContactPoint addTelecom() { // 3 621 ContactPoint t = new ContactPoint(); 622 if (this.telecom == null) 623 this.telecom = new ArrayList<ContactPoint>(); 624 this.telecom.add(t); 625 return t; 626 } 627 628 // syntactic sugar 629 public Person addTelecom(ContactPoint t) { // 3 630 if (t == null) 631 return this; 632 if (this.telecom == null) 633 this.telecom = new ArrayList<ContactPoint>(); 634 this.telecom.add(t); 635 return this; 636 } 637 638 /** 639 * @return {@link #gender} (Administrative Gender.). This is the underlying 640 * object with id, value and extensions. The accessor "getGender" gives 641 * direct access to the value 642 */ 643 public Enumeration<AdministrativeGender> getGenderElement() { 644 if (this.gender == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create Person.gender"); 647 else if (Configuration.doAutoCreate()) 648 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 649 return this.gender; 650 } 651 652 public boolean hasGenderElement() { 653 return this.gender != null && !this.gender.isEmpty(); 654 } 655 656 public boolean hasGender() { 657 return this.gender != null && !this.gender.isEmpty(); 658 } 659 660 /** 661 * @param value {@link #gender} (Administrative Gender.). This is the underlying 662 * object with id, value and extensions. The accessor "getGender" 663 * gives direct access to the value 664 */ 665 public Person setGenderElement(Enumeration<AdministrativeGender> value) { 666 this.gender = value; 667 return this; 668 } 669 670 /** 671 * @return Administrative Gender. 672 */ 673 public AdministrativeGender getGender() { 674 return this.gender == null ? null : this.gender.getValue(); 675 } 676 677 /** 678 * @param value Administrative Gender. 679 */ 680 public Person setGender(AdministrativeGender value) { 681 if (value == null) 682 this.gender = null; 683 else { 684 if (this.gender == null) 685 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 686 this.gender.setValue(value); 687 } 688 return this; 689 } 690 691 /** 692 * @return {@link #birthDate} (The birth date for the person.). This is the 693 * underlying object with id, value and extensions. The accessor 694 * "getBirthDate" gives direct access to the value 695 */ 696 public DateType getBirthDateElement() { 697 if (this.birthDate == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create Person.birthDate"); 700 else if (Configuration.doAutoCreate()) 701 this.birthDate = new DateType(); // bb 702 return this.birthDate; 703 } 704 705 public boolean hasBirthDateElement() { 706 return this.birthDate != null && !this.birthDate.isEmpty(); 707 } 708 709 public boolean hasBirthDate() { 710 return this.birthDate != null && !this.birthDate.isEmpty(); 711 } 712 713 /** 714 * @param value {@link #birthDate} (The birth date for the person.). This is the 715 * underlying object with id, value and extensions. The accessor 716 * "getBirthDate" gives direct access to the value 717 */ 718 public Person setBirthDateElement(DateType value) { 719 this.birthDate = value; 720 return this; 721 } 722 723 /** 724 * @return The birth date for the person. 725 */ 726 public Date getBirthDate() { 727 return this.birthDate == null ? null : this.birthDate.getValue(); 728 } 729 730 /** 731 * @param value The birth date for the person. 732 */ 733 public Person setBirthDate(Date value) { 734 if (value == null) 735 this.birthDate = null; 736 else { 737 if (this.birthDate == null) 738 this.birthDate = new DateType(); 739 this.birthDate.setValue(value); 740 } 741 return this; 742 } 743 744 /** 745 * @return {@link #address} (One or more addresses for the person.) 746 */ 747 public List<Address> getAddress() { 748 if (this.address == null) 749 this.address = new ArrayList<Address>(); 750 return this.address; 751 } 752 753 public boolean hasAddress() { 754 if (this.address == null) 755 return false; 756 for (Address item : this.address) 757 if (!item.isEmpty()) 758 return true; 759 return false; 760 } 761 762 /** 763 * @return {@link #address} (One or more addresses for the person.) 764 */ 765 // syntactic sugar 766 public Address addAddress() { // 3 767 Address t = new Address(); 768 if (this.address == null) 769 this.address = new ArrayList<Address>(); 770 this.address.add(t); 771 return t; 772 } 773 774 // syntactic sugar 775 public Person addAddress(Address t) { // 3 776 if (t == null) 777 return this; 778 if (this.address == null) 779 this.address = new ArrayList<Address>(); 780 this.address.add(t); 781 return this; 782 } 783 784 /** 785 * @return {@link #photo} (An image that can be displayed as a thumbnail of the 786 * person to enhance the identification of the individual.) 787 */ 788 public Attachment getPhoto() { 789 if (this.photo == null) 790 if (Configuration.errorOnAutoCreate()) 791 throw new Error("Attempt to auto-create Person.photo"); 792 else if (Configuration.doAutoCreate()) 793 this.photo = new Attachment(); // cc 794 return this.photo; 795 } 796 797 public boolean hasPhoto() { 798 return this.photo != null && !this.photo.isEmpty(); 799 } 800 801 /** 802 * @param value {@link #photo} (An image that can be displayed as a thumbnail of 803 * the person to enhance the identification of the individual.) 804 */ 805 public Person setPhoto(Attachment value) { 806 this.photo = value; 807 return this; 808 } 809 810 /** 811 * @return {@link #managingOrganization} (The organization that is the custodian 812 * of the person record.) 813 */ 814 public Reference getManagingOrganization() { 815 if (this.managingOrganization == null) 816 if (Configuration.errorOnAutoCreate()) 817 throw new Error("Attempt to auto-create Person.managingOrganization"); 818 else if (Configuration.doAutoCreate()) 819 this.managingOrganization = new Reference(); // cc 820 return this.managingOrganization; 821 } 822 823 public boolean hasManagingOrganization() { 824 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #managingOrganization} (The organization that is the 829 * custodian of the person record.) 830 */ 831 public Person setManagingOrganization(Reference value) { 832 this.managingOrganization = value; 833 return this; 834 } 835 836 /** 837 * @return {@link #managingOrganization} The actual object that is the target of 838 * the reference. The reference library doesn't populate this, but you 839 * can use it to hold the resource if you resolve it. (The organization 840 * that is the custodian of the person record.) 841 */ 842 public Organization getManagingOrganizationTarget() { 843 if (this.managingOrganizationTarget == null) 844 if (Configuration.errorOnAutoCreate()) 845 throw new Error("Attempt to auto-create Person.managingOrganization"); 846 else if (Configuration.doAutoCreate()) 847 this.managingOrganizationTarget = new Organization(); // aa 848 return this.managingOrganizationTarget; 849 } 850 851 /** 852 * @param value {@link #managingOrganization} The actual object that is the 853 * target of the reference. The reference library doesn't use 854 * these, but you can use it to hold the resource if you resolve 855 * it. (The organization that is the custodian of the person 856 * record.) 857 */ 858 public Person setManagingOrganizationTarget(Organization value) { 859 this.managingOrganizationTarget = value; 860 return this; 861 } 862 863 /** 864 * @return {@link #active} (Whether this person's record is in active use.). 865 * This is the underlying object with id, value and extensions. The 866 * accessor "getActive" gives direct access to the value 867 */ 868 public BooleanType getActiveElement() { 869 if (this.active == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create Person.active"); 872 else if (Configuration.doAutoCreate()) 873 this.active = new BooleanType(); // bb 874 return this.active; 875 } 876 877 public boolean hasActiveElement() { 878 return this.active != null && !this.active.isEmpty(); 879 } 880 881 public boolean hasActive() { 882 return this.active != null && !this.active.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #active} (Whether this person's record is in active 887 * use.). This is the underlying object with id, value and 888 * extensions. The accessor "getActive" gives direct access to the 889 * value 890 */ 891 public Person setActiveElement(BooleanType value) { 892 this.active = value; 893 return this; 894 } 895 896 /** 897 * @return Whether this person's record is in active use. 898 */ 899 public boolean getActive() { 900 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 901 } 902 903 /** 904 * @param value Whether this person's record is in active use. 905 */ 906 public Person setActive(boolean value) { 907 if (this.active == null) 908 this.active = new BooleanType(); 909 this.active.setValue(value); 910 return this; 911 } 912 913 /** 914 * @return {@link #link} (Link to a resource that concerns the same actual 915 * person.) 916 */ 917 public List<PersonLinkComponent> getLink() { 918 if (this.link == null) 919 this.link = new ArrayList<PersonLinkComponent>(); 920 return this.link; 921 } 922 923 public boolean hasLink() { 924 if (this.link == null) 925 return false; 926 for (PersonLinkComponent item : this.link) 927 if (!item.isEmpty()) 928 return true; 929 return false; 930 } 931 932 /** 933 * @return {@link #link} (Link to a resource that concerns the same actual 934 * person.) 935 */ 936 // syntactic sugar 937 public PersonLinkComponent addLink() { // 3 938 PersonLinkComponent t = new PersonLinkComponent(); 939 if (this.link == null) 940 this.link = new ArrayList<PersonLinkComponent>(); 941 this.link.add(t); 942 return t; 943 } 944 945 // syntactic sugar 946 public Person addLink(PersonLinkComponent t) { // 3 947 if (t == null) 948 return this; 949 if (this.link == null) 950 this.link = new ArrayList<PersonLinkComponent>(); 951 this.link.add(t); 952 return this; 953 } 954 955 protected void listChildren(List<Property> childrenList) { 956 super.listChildren(childrenList); 957 childrenList.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, 958 java.lang.Integer.MAX_VALUE, identifier)); 959 childrenList.add( 960 new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 961 childrenList.add(new Property("telecom", "ContactPoint", 962 "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, 963 telecom)); 964 childrenList.add(new Property("gender", "code", "Administrative Gender.", 0, java.lang.Integer.MAX_VALUE, gender)); 965 childrenList.add( 966 new Property("birthDate", "date", "The birth date for the person.", 0, java.lang.Integer.MAX_VALUE, birthDate)); 967 childrenList.add(new Property("address", "Address", "One or more addresses for the person.", 0, 968 java.lang.Integer.MAX_VALUE, address)); 969 childrenList.add(new Property("photo", "Attachment", 970 "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.", 971 0, java.lang.Integer.MAX_VALUE, photo)); 972 childrenList.add(new Property("managingOrganization", "Reference(Organization)", 973 "The organization that is the custodian of the person record.", 0, java.lang.Integer.MAX_VALUE, 974 managingOrganization)); 975 childrenList.add(new Property("active", "boolean", "Whether this person's record is in active use.", 0, 976 java.lang.Integer.MAX_VALUE, active)); 977 childrenList.add(new Property("link", "", "Link to a resource that concerns the same actual person.", 0, 978 java.lang.Integer.MAX_VALUE, link)); 979 } 980 981 @Override 982 public void setProperty(String name, Base value) throws FHIRException { 983 if (name.equals("identifier")) 984 this.getIdentifier().add(castToIdentifier(value)); 985 else if (name.equals("name")) 986 this.getName().add(castToHumanName(value)); 987 else if (name.equals("telecom")) 988 this.getTelecom().add(castToContactPoint(value)); 989 else if (name.equals("gender")) 990 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 991 else if (name.equals("birthDate")) 992 this.birthDate = castToDate(value); // DateType 993 else if (name.equals("address")) 994 this.getAddress().add(castToAddress(value)); 995 else if (name.equals("photo")) 996 this.photo = castToAttachment(value); // Attachment 997 else if (name.equals("managingOrganization")) 998 this.managingOrganization = castToReference(value); // Reference 999 else if (name.equals("active")) 1000 this.active = castToBoolean(value); // BooleanType 1001 else if (name.equals("link")) 1002 this.getLink().add((PersonLinkComponent) value); 1003 else 1004 super.setProperty(name, value); 1005 } 1006 1007 @Override 1008 public Base addChild(String name) throws FHIRException { 1009 if (name.equals("identifier")) { 1010 return addIdentifier(); 1011 } else if (name.equals("name")) { 1012 return addName(); 1013 } else if (name.equals("telecom")) { 1014 return addTelecom(); 1015 } else if (name.equals("gender")) { 1016 throw new FHIRException("Cannot call addChild on a singleton property Person.gender"); 1017 } else if (name.equals("birthDate")) { 1018 throw new FHIRException("Cannot call addChild on a singleton property Person.birthDate"); 1019 } else if (name.equals("address")) { 1020 return addAddress(); 1021 } else if (name.equals("photo")) { 1022 this.photo = new Attachment(); 1023 return this.photo; 1024 } else if (name.equals("managingOrganization")) { 1025 this.managingOrganization = new Reference(); 1026 return this.managingOrganization; 1027 } else if (name.equals("active")) { 1028 throw new FHIRException("Cannot call addChild on a singleton property Person.active"); 1029 } else if (name.equals("link")) { 1030 return addLink(); 1031 } else 1032 return super.addChild(name); 1033 } 1034 1035 public String fhirType() { 1036 return "Person"; 1037 1038 } 1039 1040 public Person copy() { 1041 Person dst = new Person(); 1042 copyValues(dst); 1043 if (identifier != null) { 1044 dst.identifier = new ArrayList<Identifier>(); 1045 for (Identifier i : identifier) 1046 dst.identifier.add(i.copy()); 1047 } 1048 ; 1049 if (name != null) { 1050 dst.name = new ArrayList<HumanName>(); 1051 for (HumanName i : name) 1052 dst.name.add(i.copy()); 1053 } 1054 ; 1055 if (telecom != null) { 1056 dst.telecom = new ArrayList<ContactPoint>(); 1057 for (ContactPoint i : telecom) 1058 dst.telecom.add(i.copy()); 1059 } 1060 ; 1061 dst.gender = gender == null ? null : gender.copy(); 1062 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1063 if (address != null) { 1064 dst.address = new ArrayList<Address>(); 1065 for (Address i : address) 1066 dst.address.add(i.copy()); 1067 } 1068 ; 1069 dst.photo = photo == null ? null : photo.copy(); 1070 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1071 dst.active = active == null ? null : active.copy(); 1072 if (link != null) { 1073 dst.link = new ArrayList<PersonLinkComponent>(); 1074 for (PersonLinkComponent i : link) 1075 dst.link.add(i.copy()); 1076 } 1077 ; 1078 return dst; 1079 } 1080 1081 protected Person typedCopy() { 1082 return copy(); 1083 } 1084 1085 @Override 1086 public boolean equalsDeep(Base other) { 1087 if (!super.equalsDeep(other)) 1088 return false; 1089 if (!(other instanceof Person)) 1090 return false; 1091 Person o = (Person) other; 1092 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 1093 && compareDeep(telecom, o.telecom, true) && compareDeep(gender, o.gender, true) 1094 && compareDeep(birthDate, o.birthDate, true) && compareDeep(address, o.address, true) 1095 && compareDeep(photo, o.photo, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1096 && compareDeep(active, o.active, true) && compareDeep(link, o.link, true); 1097 } 1098 1099 @Override 1100 public boolean equalsShallow(Base other) { 1101 if (!super.equalsShallow(other)) 1102 return false; 1103 if (!(other instanceof Person)) 1104 return false; 1105 Person o = (Person) other; 1106 return compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 1107 && compareValues(active, o.active, true); 1108 } 1109 1110 public boolean isEmpty() { 1111 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (name == null || name.isEmpty()) 1112 && (telecom == null || telecom.isEmpty()) && (gender == null || gender.isEmpty()) 1113 && (birthDate == null || birthDate.isEmpty()) && (address == null || address.isEmpty()) 1114 && (photo == null || photo.isEmpty()) && (managingOrganization == null || managingOrganization.isEmpty()) 1115 && (active == null || active.isEmpty()) && (link == null || link.isEmpty()); 1116 } 1117 1118 @Override 1119 public ResourceType getResourceType() { 1120 return ResourceType.Person; 1121 } 1122 1123 @SearchParamDefinition(name = "identifier", path = "Person.identifier", description = "A person Identifier", type = "token") 1124 public static final String SP_IDENTIFIER = "identifier"; 1125 @SearchParamDefinition(name = "address", path = "Person.address", description = "An address in any kind of address/part", type = "string") 1126 public static final String SP_ADDRESS = "address"; 1127 @SearchParamDefinition(name = "birthdate", path = "Person.birthDate", description = "The person's date of birth", type = "date") 1128 public static final String SP_BIRTHDATE = "birthdate"; 1129 @SearchParamDefinition(name = "address-state", path = "Person.address.state", description = "A state specified in an address", type = "string") 1130 public static final String SP_ADDRESSSTATE = "address-state"; 1131 @SearchParamDefinition(name = "gender", path = "Person.gender", description = "The gender of the person", type = "token") 1132 public static final String SP_GENDER = "gender"; 1133 @SearchParamDefinition(name = "practitioner", path = "Person.link.target", description = "The Person links to this Practitioner", type = "reference") 1134 public static final String SP_PRACTITIONER = "practitioner"; 1135 @SearchParamDefinition(name = "link", path = "Person.link.target", description = "Any link has this Patient, Person, RelatedPerson or Practitioner reference", type = "reference") 1136 public static final String SP_LINK = "link"; 1137 @SearchParamDefinition(name = "relatedperson", path = "Person.link.target", description = "The Person links to this RelatedPerson", type = "reference") 1138 public static final String SP_RELATEDPERSON = "relatedperson"; 1139 @SearchParamDefinition(name = "address-postalcode", path = "Person.address.postalCode", description = "A postal code specified in an address", type = "string") 1140 public static final String SP_ADDRESSPOSTALCODE = "address-postalcode"; 1141 @SearchParamDefinition(name = "address-country", path = "Person.address.country", description = "A country specified in an address", type = "string") 1142 public static final String SP_ADDRESSCOUNTRY = "address-country"; 1143 @SearchParamDefinition(name = "phonetic", path = "Person.name", description = "A portion of name using some kind of phonetic matching algorithm", type = "string") 1144 public static final String SP_PHONETIC = "phonetic"; 1145 @SearchParamDefinition(name = "phone", path = "Person.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 1146 public static final String SP_PHONE = "phone"; 1147 @SearchParamDefinition(name = "patient", path = "Person.link.target", description = "The Person links to this Patient", type = "reference") 1148 public static final String SP_PATIENT = "patient"; 1149 @SearchParamDefinition(name = "organization", path = "Person.managingOrganization", description = "The organization at which this person record is being managed", type = "reference") 1150 public static final String SP_ORGANIZATION = "organization"; 1151 @SearchParamDefinition(name = "name", path = "Person.name", description = "A portion of name in any name part", type = "string") 1152 public static final String SP_NAME = "name"; 1153 @SearchParamDefinition(name = "address-use", path = "Person.address.use", description = "A use code specified in an address", type = "token") 1154 public static final String SP_ADDRESSUSE = "address-use"; 1155 @SearchParamDefinition(name = "telecom", path = "Person.telecom", description = "The value in any kind of contact", type = "token") 1156 public static final String SP_TELECOM = "telecom"; 1157 @SearchParamDefinition(name = "address-city", path = "Person.address.city", description = "A city specified in an address", type = "string") 1158 public static final String SP_ADDRESSCITY = "address-city"; 1159 @SearchParamDefinition(name = "email", path = "Person.telecom.where(system='email')", description = "A value in an email contact", type = "token") 1160 public static final String SP_EMAIL = "email"; 1161 1162}