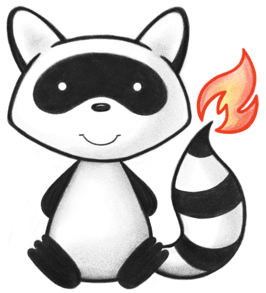
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender; 038import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046 047/** 048 * A person who is directly or indirectly involved in the provisioning of 049 * healthcare. 050 */ 051@ResourceDef(name = "Practitioner", profile = "http://hl7.org/fhir/Profile/Practitioner") 052public class Practitioner extends DomainResource { 053 054 @Block() 055 public static class PractitionerPractitionerRoleComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * The organization where the Practitioner performs the roles associated. 058 */ 059 @Child(name = "managingOrganization", type = { 060 Organization.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 061 @Description(shortDefinition = "Organization where the roles are performed", formalDefinition = "The organization where the Practitioner performs the roles associated.") 062 protected Reference managingOrganization; 063 064 /** 065 * The actual object that is the target of the reference (The organization where 066 * the Practitioner performs the roles associated.) 067 */ 068 protected Organization managingOrganizationTarget; 069 070 /** 071 * Roles which this practitioner is authorized to perform for the organization. 072 */ 073 @Child(name = "role", type = { 074 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 075 @Description(shortDefinition = "Roles which this practitioner may perform", formalDefinition = "Roles which this practitioner is authorized to perform for the organization.") 076 protected CodeableConcept role; 077 078 /** 079 * Specific specialty of the practitioner. 080 */ 081 @Child(name = "specialty", type = { 082 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 083 @Description(shortDefinition = "Specific specialty of the practitioner", formalDefinition = "Specific specialty of the practitioner.") 084 protected List<CodeableConcept> specialty; 085 086 /** 087 * The period during which the person is authorized to act as a practitioner in 088 * these role(s) for the organization. 089 */ 090 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 091 @Description(shortDefinition = "The period during which the practitioner is authorized to perform in these role(s)", formalDefinition = "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.") 092 protected Period period; 093 094 /** 095 * The location(s) at which this practitioner provides care. 096 */ 097 @Child(name = "location", type = { 098 Location.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 099 @Description(shortDefinition = "The location(s) at which this practitioner provides care", formalDefinition = "The location(s) at which this practitioner provides care.") 100 protected List<Reference> location; 101 /** 102 * The actual objects that are the target of the reference (The location(s) at 103 * which this practitioner provides care.) 104 */ 105 protected List<Location> locationTarget; 106 107 /** 108 * The list of healthcare services that this worker provides for this role's 109 * Organization/Location(s). 110 */ 111 @Child(name = "healthcareService", type = { 112 HealthcareService.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 113 @Description(shortDefinition = "The list of healthcare services that this worker provides for this role's Organization/Location(s)", formalDefinition = "The list of healthcare services that this worker provides for this role's Organization/Location(s).") 114 protected List<Reference> healthcareService; 115 /** 116 * The actual objects that are the target of the reference (The list of 117 * healthcare services that this worker provides for this role's 118 * Organization/Location(s).) 119 */ 120 protected List<HealthcareService> healthcareServiceTarget; 121 122 private static final long serialVersionUID = -2146177018L; 123 124 /* 125 * Constructor 126 */ 127 public PractitionerPractitionerRoleComponent() { 128 super(); 129 } 130 131 /** 132 * @return {@link #managingOrganization} (The organization where the 133 * Practitioner performs the roles associated.) 134 */ 135 public Reference getManagingOrganization() { 136 if (this.managingOrganization == null) 137 if (Configuration.errorOnAutoCreate()) 138 throw new Error("Attempt to auto-create PractitionerPractitionerRoleComponent.managingOrganization"); 139 else if (Configuration.doAutoCreate()) 140 this.managingOrganization = new Reference(); // cc 141 return this.managingOrganization; 142 } 143 144 public boolean hasManagingOrganization() { 145 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 146 } 147 148 /** 149 * @param value {@link #managingOrganization} (The organization where the 150 * Practitioner performs the roles associated.) 151 */ 152 public PractitionerPractitionerRoleComponent setManagingOrganization(Reference value) { 153 this.managingOrganization = value; 154 return this; 155 } 156 157 /** 158 * @return {@link #managingOrganization} The actual object that is the target of 159 * the reference. The reference library doesn't populate this, but you 160 * can use it to hold the resource if you resolve it. (The organization 161 * where the Practitioner performs the roles associated.) 162 */ 163 public Organization getManagingOrganizationTarget() { 164 if (this.managingOrganizationTarget == null) 165 if (Configuration.errorOnAutoCreate()) 166 throw new Error("Attempt to auto-create PractitionerPractitionerRoleComponent.managingOrganization"); 167 else if (Configuration.doAutoCreate()) 168 this.managingOrganizationTarget = new Organization(); // aa 169 return this.managingOrganizationTarget; 170 } 171 172 /** 173 * @param value {@link #managingOrganization} The actual object that is the 174 * target of the reference. The reference library doesn't use 175 * these, but you can use it to hold the resource if you resolve 176 * it. (The organization where the Practitioner performs the roles 177 * associated.) 178 */ 179 public PractitionerPractitionerRoleComponent setManagingOrganizationTarget(Organization value) { 180 this.managingOrganizationTarget = value; 181 return this; 182 } 183 184 /** 185 * @return {@link #role} (Roles which this practitioner is authorized to perform 186 * for the organization.) 187 */ 188 public CodeableConcept getRole() { 189 if (this.role == null) 190 if (Configuration.errorOnAutoCreate()) 191 throw new Error("Attempt to auto-create PractitionerPractitionerRoleComponent.role"); 192 else if (Configuration.doAutoCreate()) 193 this.role = new CodeableConcept(); // cc 194 return this.role; 195 } 196 197 public boolean hasRole() { 198 return this.role != null && !this.role.isEmpty(); 199 } 200 201 /** 202 * @param value {@link #role} (Roles which this practitioner is authorized to 203 * perform for the organization.) 204 */ 205 public PractitionerPractitionerRoleComponent setRole(CodeableConcept value) { 206 this.role = value; 207 return this; 208 } 209 210 /** 211 * @return {@link #specialty} (Specific specialty of the practitioner.) 212 */ 213 public List<CodeableConcept> getSpecialty() { 214 if (this.specialty == null) 215 this.specialty = new ArrayList<CodeableConcept>(); 216 return this.specialty; 217 } 218 219 public boolean hasSpecialty() { 220 if (this.specialty == null) 221 return false; 222 for (CodeableConcept item : this.specialty) 223 if (!item.isEmpty()) 224 return true; 225 return false; 226 } 227 228 /** 229 * @return {@link #specialty} (Specific specialty of the practitioner.) 230 */ 231 // syntactic sugar 232 public CodeableConcept addSpecialty() { // 3 233 CodeableConcept t = new CodeableConcept(); 234 if (this.specialty == null) 235 this.specialty = new ArrayList<CodeableConcept>(); 236 this.specialty.add(t); 237 return t; 238 } 239 240 // syntactic sugar 241 public PractitionerPractitionerRoleComponent addSpecialty(CodeableConcept t) { // 3 242 if (t == null) 243 return this; 244 if (this.specialty == null) 245 this.specialty = new ArrayList<CodeableConcept>(); 246 this.specialty.add(t); 247 return this; 248 } 249 250 /** 251 * @return {@link #period} (The period during which the person is authorized to 252 * act as a practitioner in these role(s) for the organization.) 253 */ 254 public Period getPeriod() { 255 if (this.period == null) 256 if (Configuration.errorOnAutoCreate()) 257 throw new Error("Attempt to auto-create PractitionerPractitionerRoleComponent.period"); 258 else if (Configuration.doAutoCreate()) 259 this.period = new Period(); // cc 260 return this.period; 261 } 262 263 public boolean hasPeriod() { 264 return this.period != null && !this.period.isEmpty(); 265 } 266 267 /** 268 * @param value {@link #period} (The period during which the person is 269 * authorized to act as a practitioner in these role(s) for the 270 * organization.) 271 */ 272 public PractitionerPractitionerRoleComponent setPeriod(Period value) { 273 this.period = value; 274 return this; 275 } 276 277 /** 278 * @return {@link #location} (The location(s) at which this practitioner 279 * provides care.) 280 */ 281 public List<Reference> getLocation() { 282 if (this.location == null) 283 this.location = new ArrayList<Reference>(); 284 return this.location; 285 } 286 287 public boolean hasLocation() { 288 if (this.location == null) 289 return false; 290 for (Reference item : this.location) 291 if (!item.isEmpty()) 292 return true; 293 return false; 294 } 295 296 /** 297 * @return {@link #location} (The location(s) at which this practitioner 298 * provides care.) 299 */ 300 // syntactic sugar 301 public Reference addLocation() { // 3 302 Reference t = new Reference(); 303 if (this.location == null) 304 this.location = new ArrayList<Reference>(); 305 this.location.add(t); 306 return t; 307 } 308 309 // syntactic sugar 310 public PractitionerPractitionerRoleComponent addLocation(Reference t) { // 3 311 if (t == null) 312 return this; 313 if (this.location == null) 314 this.location = new ArrayList<Reference>(); 315 this.location.add(t); 316 return this; 317 } 318 319 /** 320 * @return {@link #location} (The actual objects that are the target of the 321 * reference. The reference library doesn't populate this, but you can 322 * use this to hold the resources if you resolvethemt. The location(s) 323 * at which this practitioner provides care.) 324 */ 325 public List<Location> getLocationTarget() { 326 if (this.locationTarget == null) 327 this.locationTarget = new ArrayList<Location>(); 328 return this.locationTarget; 329 } 330 331 // syntactic sugar 332 /** 333 * @return {@link #location} (Add an actual object that is the target of the 334 * reference. The reference library doesn't use these, but you can use 335 * this to hold the resources if you resolvethemt. The location(s) at 336 * which this practitioner provides care.) 337 */ 338 public Location addLocationTarget() { 339 Location r = new Location(); 340 if (this.locationTarget == null) 341 this.locationTarget = new ArrayList<Location>(); 342 this.locationTarget.add(r); 343 return r; 344 } 345 346 /** 347 * @return {@link #healthcareService} (The list of healthcare services that this 348 * worker provides for this role's Organization/Location(s).) 349 */ 350 public List<Reference> getHealthcareService() { 351 if (this.healthcareService == null) 352 this.healthcareService = new ArrayList<Reference>(); 353 return this.healthcareService; 354 } 355 356 public boolean hasHealthcareService() { 357 if (this.healthcareService == null) 358 return false; 359 for (Reference item : this.healthcareService) 360 if (!item.isEmpty()) 361 return true; 362 return false; 363 } 364 365 /** 366 * @return {@link #healthcareService} (The list of healthcare services that this 367 * worker provides for this role's Organization/Location(s).) 368 */ 369 // syntactic sugar 370 public Reference addHealthcareService() { // 3 371 Reference t = new Reference(); 372 if (this.healthcareService == null) 373 this.healthcareService = new ArrayList<Reference>(); 374 this.healthcareService.add(t); 375 return t; 376 } 377 378 // syntactic sugar 379 public PractitionerPractitionerRoleComponent addHealthcareService(Reference t) { // 3 380 if (t == null) 381 return this; 382 if (this.healthcareService == null) 383 this.healthcareService = new ArrayList<Reference>(); 384 this.healthcareService.add(t); 385 return this; 386 } 387 388 /** 389 * @return {@link #healthcareService} (The actual objects that are the target of 390 * the reference. The reference library doesn't populate this, but you 391 * can use this to hold the resources if you resolvethemt. The list of 392 * healthcare services that this worker provides for this role's 393 * Organization/Location(s).) 394 */ 395 public List<HealthcareService> getHealthcareServiceTarget() { 396 if (this.healthcareServiceTarget == null) 397 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 398 return this.healthcareServiceTarget; 399 } 400 401 // syntactic sugar 402 /** 403 * @return {@link #healthcareService} (Add an actual object that is the target 404 * of the reference. The reference library doesn't use these, but you 405 * can use this to hold the resources if you resolvethemt. The list of 406 * healthcare services that this worker provides for this role's 407 * Organization/Location(s).) 408 */ 409 public HealthcareService addHealthcareServiceTarget() { 410 HealthcareService r = new HealthcareService(); 411 if (this.healthcareServiceTarget == null) 412 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 413 this.healthcareServiceTarget.add(r); 414 return r; 415 } 416 417 protected void listChildren(List<Property> childrenList) { 418 super.listChildren(childrenList); 419 childrenList.add(new Property("managingOrganization", "Reference(Organization)", 420 "The organization where the Practitioner performs the roles associated.", 0, java.lang.Integer.MAX_VALUE, 421 managingOrganization)); 422 childrenList.add(new Property("role", "CodeableConcept", 423 "Roles which this practitioner is authorized to perform for the organization.", 0, 424 java.lang.Integer.MAX_VALUE, role)); 425 childrenList.add(new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, 426 java.lang.Integer.MAX_VALUE, specialty)); 427 childrenList.add(new Property("period", "Period", 428 "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 429 0, java.lang.Integer.MAX_VALUE, period)); 430 childrenList.add(new Property("location", "Reference(Location)", 431 "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location)); 432 childrenList.add(new Property("healthcareService", "Reference(HealthcareService)", 433 "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, 434 java.lang.Integer.MAX_VALUE, healthcareService)); 435 } 436 437 @Override 438 public void setProperty(String name, Base value) throws FHIRException { 439 if (name.equals("managingOrganization")) 440 this.managingOrganization = castToReference(value); // Reference 441 else if (name.equals("role")) 442 this.role = castToCodeableConcept(value); // CodeableConcept 443 else if (name.equals("specialty")) 444 this.getSpecialty().add(castToCodeableConcept(value)); 445 else if (name.equals("period")) 446 this.period = castToPeriod(value); // Period 447 else if (name.equals("location")) 448 this.getLocation().add(castToReference(value)); 449 else if (name.equals("healthcareService")) 450 this.getHealthcareService().add(castToReference(value)); 451 else 452 super.setProperty(name, value); 453 } 454 455 @Override 456 public Base addChild(String name) throws FHIRException { 457 if (name.equals("managingOrganization")) { 458 this.managingOrganization = new Reference(); 459 return this.managingOrganization; 460 } else if (name.equals("role")) { 461 this.role = new CodeableConcept(); 462 return this.role; 463 } else if (name.equals("specialty")) { 464 return addSpecialty(); 465 } else if (name.equals("period")) { 466 this.period = new Period(); 467 return this.period; 468 } else if (name.equals("location")) { 469 return addLocation(); 470 } else if (name.equals("healthcareService")) { 471 return addHealthcareService(); 472 } else 473 return super.addChild(name); 474 } 475 476 public PractitionerPractitionerRoleComponent copy() { 477 PractitionerPractitionerRoleComponent dst = new PractitionerPractitionerRoleComponent(); 478 copyValues(dst); 479 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 480 dst.role = role == null ? null : role.copy(); 481 if (specialty != null) { 482 dst.specialty = new ArrayList<CodeableConcept>(); 483 for (CodeableConcept i : specialty) 484 dst.specialty.add(i.copy()); 485 } 486 ; 487 dst.period = period == null ? null : period.copy(); 488 if (location != null) { 489 dst.location = new ArrayList<Reference>(); 490 for (Reference i : location) 491 dst.location.add(i.copy()); 492 } 493 ; 494 if (healthcareService != null) { 495 dst.healthcareService = new ArrayList<Reference>(); 496 for (Reference i : healthcareService) 497 dst.healthcareService.add(i.copy()); 498 } 499 ; 500 return dst; 501 } 502 503 @Override 504 public boolean equalsDeep(Base other) { 505 if (!super.equalsDeep(other)) 506 return false; 507 if (!(other instanceof PractitionerPractitionerRoleComponent)) 508 return false; 509 PractitionerPractitionerRoleComponent o = (PractitionerPractitionerRoleComponent) other; 510 return compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(role, o.role, true) 511 && compareDeep(specialty, o.specialty, true) && compareDeep(period, o.period, true) 512 && compareDeep(location, o.location, true) && compareDeep(healthcareService, o.healthcareService, true); 513 } 514 515 @Override 516 public boolean equalsShallow(Base other) { 517 if (!super.equalsShallow(other)) 518 return false; 519 if (!(other instanceof PractitionerPractitionerRoleComponent)) 520 return false; 521 PractitionerPractitionerRoleComponent o = (PractitionerPractitionerRoleComponent) other; 522 return true; 523 } 524 525 public boolean isEmpty() { 526 return super.isEmpty() && (managingOrganization == null || managingOrganization.isEmpty()) 527 && (role == null || role.isEmpty()) && (specialty == null || specialty.isEmpty()) 528 && (period == null || period.isEmpty()) && (location == null || location.isEmpty()) 529 && (healthcareService == null || healthcareService.isEmpty()); 530 } 531 532 public String fhirType() { 533 return "Practitioner.practitionerRole"; 534 535 } 536 537 } 538 539 @Block() 540 public static class PractitionerQualificationComponent extends BackboneElement implements IBaseBackboneElement { 541 /** 542 * An identifier that applies to this person's qualification in this role. 543 */ 544 @Child(name = "identifier", type = { 545 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 546 @Description(shortDefinition = "An identifier for this qualification for the practitioner", formalDefinition = "An identifier that applies to this person's qualification in this role.") 547 protected List<Identifier> identifier; 548 549 /** 550 * Coded representation of the qualification. 551 */ 552 @Child(name = "code", type = { 553 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 554 @Description(shortDefinition = "Coded representation of the qualification", formalDefinition = "Coded representation of the qualification.") 555 protected CodeableConcept code; 556 557 /** 558 * Period during which the qualification is valid. 559 */ 560 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 561 @Description(shortDefinition = "Period during which the qualification is valid", formalDefinition = "Period during which the qualification is valid.") 562 protected Period period; 563 564 /** 565 * Organization that regulates and issues the qualification. 566 */ 567 @Child(name = "issuer", type = { 568 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 569 @Description(shortDefinition = "Organization that regulates and issues the qualification", formalDefinition = "Organization that regulates and issues the qualification.") 570 protected Reference issuer; 571 572 /** 573 * The actual object that is the target of the reference (Organization that 574 * regulates and issues the qualification.) 575 */ 576 protected Organization issuerTarget; 577 578 private static final long serialVersionUID = 1095219071L; 579 580 /* 581 * Constructor 582 */ 583 public PractitionerQualificationComponent() { 584 super(); 585 } 586 587 /* 588 * Constructor 589 */ 590 public PractitionerQualificationComponent(CodeableConcept code) { 591 super(); 592 this.code = code; 593 } 594 595 /** 596 * @return {@link #identifier} (An identifier that applies to this person's 597 * qualification in this role.) 598 */ 599 public List<Identifier> getIdentifier() { 600 if (this.identifier == null) 601 this.identifier = new ArrayList<Identifier>(); 602 return this.identifier; 603 } 604 605 public boolean hasIdentifier() { 606 if (this.identifier == null) 607 return false; 608 for (Identifier item : this.identifier) 609 if (!item.isEmpty()) 610 return true; 611 return false; 612 } 613 614 /** 615 * @return {@link #identifier} (An identifier that applies to this person's 616 * qualification in this role.) 617 */ 618 // syntactic sugar 619 public Identifier addIdentifier() { // 3 620 Identifier t = new Identifier(); 621 if (this.identifier == null) 622 this.identifier = new ArrayList<Identifier>(); 623 this.identifier.add(t); 624 return t; 625 } 626 627 // syntactic sugar 628 public PractitionerQualificationComponent addIdentifier(Identifier t) { // 3 629 if (t == null) 630 return this; 631 if (this.identifier == null) 632 this.identifier = new ArrayList<Identifier>(); 633 this.identifier.add(t); 634 return this; 635 } 636 637 /** 638 * @return {@link #code} (Coded representation of the qualification.) 639 */ 640 public CodeableConcept getCode() { 641 if (this.code == null) 642 if (Configuration.errorOnAutoCreate()) 643 throw new Error("Attempt to auto-create PractitionerQualificationComponent.code"); 644 else if (Configuration.doAutoCreate()) 645 this.code = new CodeableConcept(); // cc 646 return this.code; 647 } 648 649 public boolean hasCode() { 650 return this.code != null && !this.code.isEmpty(); 651 } 652 653 /** 654 * @param value {@link #code} (Coded representation of the qualification.) 655 */ 656 public PractitionerQualificationComponent setCode(CodeableConcept value) { 657 this.code = value; 658 return this; 659 } 660 661 /** 662 * @return {@link #period} (Period during which the qualification is valid.) 663 */ 664 public Period getPeriod() { 665 if (this.period == null) 666 if (Configuration.errorOnAutoCreate()) 667 throw new Error("Attempt to auto-create PractitionerQualificationComponent.period"); 668 else if (Configuration.doAutoCreate()) 669 this.period = new Period(); // cc 670 return this.period; 671 } 672 673 public boolean hasPeriod() { 674 return this.period != null && !this.period.isEmpty(); 675 } 676 677 /** 678 * @param value {@link #period} (Period during which the qualification is 679 * valid.) 680 */ 681 public PractitionerQualificationComponent setPeriod(Period value) { 682 this.period = value; 683 return this; 684 } 685 686 /** 687 * @return {@link #issuer} (Organization that regulates and issues the 688 * qualification.) 689 */ 690 public Reference getIssuer() { 691 if (this.issuer == null) 692 if (Configuration.errorOnAutoCreate()) 693 throw new Error("Attempt to auto-create PractitionerQualificationComponent.issuer"); 694 else if (Configuration.doAutoCreate()) 695 this.issuer = new Reference(); // cc 696 return this.issuer; 697 } 698 699 public boolean hasIssuer() { 700 return this.issuer != null && !this.issuer.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #issuer} (Organization that regulates and issues the 705 * qualification.) 706 */ 707 public PractitionerQualificationComponent setIssuer(Reference value) { 708 this.issuer = value; 709 return this; 710 } 711 712 /** 713 * @return {@link #issuer} The actual object that is the target of the 714 * reference. The reference library doesn't populate this, but you can 715 * use it to hold the resource if you resolve it. (Organization that 716 * regulates and issues the qualification.) 717 */ 718 public Organization getIssuerTarget() { 719 if (this.issuerTarget == null) 720 if (Configuration.errorOnAutoCreate()) 721 throw new Error("Attempt to auto-create PractitionerQualificationComponent.issuer"); 722 else if (Configuration.doAutoCreate()) 723 this.issuerTarget = new Organization(); // aa 724 return this.issuerTarget; 725 } 726 727 /** 728 * @param value {@link #issuer} The actual object that is the target of the 729 * reference. The reference library doesn't use these, but you can 730 * use it to hold the resource if you resolve it. (Organization 731 * that regulates and issues the qualification.) 732 */ 733 public PractitionerQualificationComponent setIssuerTarget(Organization value) { 734 this.issuerTarget = value; 735 return this; 736 } 737 738 protected void listChildren(List<Property> childrenList) { 739 super.listChildren(childrenList); 740 childrenList.add(new Property("identifier", "Identifier", 741 "An identifier that applies to this person's qualification in this role.", 0, java.lang.Integer.MAX_VALUE, 742 identifier)); 743 childrenList.add(new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 744 java.lang.Integer.MAX_VALUE, code)); 745 childrenList.add(new Property("period", "Period", "Period during which the qualification is valid.", 0, 746 java.lang.Integer.MAX_VALUE, period)); 747 childrenList.add(new Property("issuer", "Reference(Organization)", 748 "Organization that regulates and issues the qualification.", 0, java.lang.Integer.MAX_VALUE, issuer)); 749 } 750 751 @Override 752 public void setProperty(String name, Base value) throws FHIRException { 753 if (name.equals("identifier")) 754 this.getIdentifier().add(castToIdentifier(value)); 755 else if (name.equals("code")) 756 this.code = castToCodeableConcept(value); // CodeableConcept 757 else if (name.equals("period")) 758 this.period = castToPeriod(value); // Period 759 else if (name.equals("issuer")) 760 this.issuer = castToReference(value); // Reference 761 else 762 super.setProperty(name, value); 763 } 764 765 @Override 766 public Base addChild(String name) throws FHIRException { 767 if (name.equals("identifier")) { 768 return addIdentifier(); 769 } else if (name.equals("code")) { 770 this.code = new CodeableConcept(); 771 return this.code; 772 } else if (name.equals("period")) { 773 this.period = new Period(); 774 return this.period; 775 } else if (name.equals("issuer")) { 776 this.issuer = new Reference(); 777 return this.issuer; 778 } else 779 return super.addChild(name); 780 } 781 782 public PractitionerQualificationComponent copy() { 783 PractitionerQualificationComponent dst = new PractitionerQualificationComponent(); 784 copyValues(dst); 785 if (identifier != null) { 786 dst.identifier = new ArrayList<Identifier>(); 787 for (Identifier i : identifier) 788 dst.identifier.add(i.copy()); 789 } 790 ; 791 dst.code = code == null ? null : code.copy(); 792 dst.period = period == null ? null : period.copy(); 793 dst.issuer = issuer == null ? null : issuer.copy(); 794 return dst; 795 } 796 797 @Override 798 public boolean equalsDeep(Base other) { 799 if (!super.equalsDeep(other)) 800 return false; 801 if (!(other instanceof PractitionerQualificationComponent)) 802 return false; 803 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other; 804 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 805 && compareDeep(period, o.period, true) && compareDeep(issuer, o.issuer, true); 806 } 807 808 @Override 809 public boolean equalsShallow(Base other) { 810 if (!super.equalsShallow(other)) 811 return false; 812 if (!(other instanceof PractitionerQualificationComponent)) 813 return false; 814 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other; 815 return true; 816 } 817 818 public boolean isEmpty() { 819 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (code == null || code.isEmpty()) 820 && (period == null || period.isEmpty()) && (issuer == null || issuer.isEmpty()); 821 } 822 823 public String fhirType() { 824 return "Practitioner.qualification"; 825 826 } 827 828 } 829 830 /** 831 * An identifier that applies to this person in this role. 832 */ 833 @Child(name = "identifier", type = { 834 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 835 @Description(shortDefinition = "A identifier for the person as this agent", formalDefinition = "An identifier that applies to this person in this role.") 836 protected List<Identifier> identifier; 837 838 /** 839 * Whether this practitioner's record is in active use. 840 */ 841 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 842 @Description(shortDefinition = "Whether this practitioner's record is in active use", formalDefinition = "Whether this practitioner's record is in active use.") 843 protected BooleanType active; 844 845 /** 846 * A name associated with the person. 847 */ 848 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 849 @Description(shortDefinition = "A name associated with the person", formalDefinition = "A name associated with the person.") 850 protected HumanName name; 851 852 /** 853 * A contact detail for the practitioner, e.g. a telephone number or an email 854 * address. 855 */ 856 @Child(name = "telecom", type = { 857 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 858 @Description(shortDefinition = "A contact detail for the practitioner", formalDefinition = "A contact detail for the practitioner, e.g. a telephone number or an email address.") 859 protected List<ContactPoint> telecom; 860 861 /** 862 * The postal address where the practitioner can be found or visited or to which 863 * mail can be delivered. 864 */ 865 @Child(name = "address", type = { 866 Address.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 867 @Description(shortDefinition = "Where practitioner can be found/visited", formalDefinition = "The postal address where the practitioner can be found or visited or to which mail can be delivered.") 868 protected List<Address> address; 869 870 /** 871 * Administrative Gender - the gender that the person is considered to have for 872 * administration and record keeping purposes. 873 */ 874 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 875 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.") 876 protected Enumeration<AdministrativeGender> gender; 877 878 /** 879 * The date of birth for the practitioner. 880 */ 881 @Child(name = "birthDate", type = { DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 882 @Description(shortDefinition = "The date on which the practitioner was born", formalDefinition = "The date of birth for the practitioner.") 883 protected DateType birthDate; 884 885 /** 886 * Image of the person. 887 */ 888 @Child(name = "photo", type = { 889 Attachment.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 890 @Description(shortDefinition = "Image of the person", formalDefinition = "Image of the person.") 891 protected List<Attachment> photo; 892 893 /** 894 * The list of roles/organizations that the practitioner is associated with. 895 */ 896 @Child(name = "practitionerRole", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 897 @Description(shortDefinition = "Roles/organizations the practitioner is associated with", formalDefinition = "The list of roles/organizations that the practitioner is associated with.") 898 protected List<PractitionerPractitionerRoleComponent> practitionerRole; 899 900 /** 901 * Qualifications obtained by training and certification. 902 */ 903 @Child(name = "qualification", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 904 @Description(shortDefinition = "Qualifications obtained by training and certification", formalDefinition = "Qualifications obtained by training and certification.") 905 protected List<PractitionerQualificationComponent> qualification; 906 907 /** 908 * A language the practitioner is able to use in patient communication. 909 */ 910 @Child(name = "communication", type = { 911 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 912 @Description(shortDefinition = "A language the practitioner is able to use in patient communication", formalDefinition = "A language the practitioner is able to use in patient communication.") 913 protected List<CodeableConcept> communication; 914 915 private static final long serialVersionUID = 1066276346L; 916 917 /* 918 * Constructor 919 */ 920 public Practitioner() { 921 super(); 922 } 923 924 /** 925 * @return {@link #identifier} (An identifier that applies to this person in 926 * this role.) 927 */ 928 public List<Identifier> getIdentifier() { 929 if (this.identifier == null) 930 this.identifier = new ArrayList<Identifier>(); 931 return this.identifier; 932 } 933 934 public boolean hasIdentifier() { 935 if (this.identifier == null) 936 return false; 937 for (Identifier item : this.identifier) 938 if (!item.isEmpty()) 939 return true; 940 return false; 941 } 942 943 /** 944 * @return {@link #identifier} (An identifier that applies to this person in 945 * this role.) 946 */ 947 // syntactic sugar 948 public Identifier addIdentifier() { // 3 949 Identifier t = new Identifier(); 950 if (this.identifier == null) 951 this.identifier = new ArrayList<Identifier>(); 952 this.identifier.add(t); 953 return t; 954 } 955 956 // syntactic sugar 957 public Practitioner addIdentifier(Identifier t) { // 3 958 if (t == null) 959 return this; 960 if (this.identifier == null) 961 this.identifier = new ArrayList<Identifier>(); 962 this.identifier.add(t); 963 return this; 964 } 965 966 /** 967 * @return {@link #active} (Whether this practitioner's record is in active 968 * use.). This is the underlying object with id, value and extensions. 969 * The accessor "getActive" gives direct access to the value 970 */ 971 public BooleanType getActiveElement() { 972 if (this.active == null) 973 if (Configuration.errorOnAutoCreate()) 974 throw new Error("Attempt to auto-create Practitioner.active"); 975 else if (Configuration.doAutoCreate()) 976 this.active = new BooleanType(); // bb 977 return this.active; 978 } 979 980 public boolean hasActiveElement() { 981 return this.active != null && !this.active.isEmpty(); 982 } 983 984 public boolean hasActive() { 985 return this.active != null && !this.active.isEmpty(); 986 } 987 988 /** 989 * @param value {@link #active} (Whether this practitioner's record is in active 990 * use.). This is the underlying object with id, value and 991 * extensions. The accessor "getActive" gives direct access to the 992 * value 993 */ 994 public Practitioner setActiveElement(BooleanType value) { 995 this.active = value; 996 return this; 997 } 998 999 /** 1000 * @return Whether this practitioner's record is in active use. 1001 */ 1002 public boolean getActive() { 1003 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1004 } 1005 1006 /** 1007 * @param value Whether this practitioner's record is in active use. 1008 */ 1009 public Practitioner setActive(boolean value) { 1010 if (this.active == null) 1011 this.active = new BooleanType(); 1012 this.active.setValue(value); 1013 return this; 1014 } 1015 1016 /** 1017 * @return {@link #name} (A name associated with the person.) 1018 */ 1019 public HumanName getName() { 1020 if (this.name == null) 1021 if (Configuration.errorOnAutoCreate()) 1022 throw new Error("Attempt to auto-create Practitioner.name"); 1023 else if (Configuration.doAutoCreate()) 1024 this.name = new HumanName(); // cc 1025 return this.name; 1026 } 1027 1028 public boolean hasName() { 1029 return this.name != null && !this.name.isEmpty(); 1030 } 1031 1032 /** 1033 * @param value {@link #name} (A name associated with the person.) 1034 */ 1035 public Practitioner setName(HumanName value) { 1036 this.name = value; 1037 return this; 1038 } 1039 1040 /** 1041 * @return {@link #telecom} (A contact detail for the practitioner, e.g. a 1042 * telephone number or an email address.) 1043 */ 1044 public List<ContactPoint> getTelecom() { 1045 if (this.telecom == null) 1046 this.telecom = new ArrayList<ContactPoint>(); 1047 return this.telecom; 1048 } 1049 1050 public boolean hasTelecom() { 1051 if (this.telecom == null) 1052 return false; 1053 for (ContactPoint item : this.telecom) 1054 if (!item.isEmpty()) 1055 return true; 1056 return false; 1057 } 1058 1059 /** 1060 * @return {@link #telecom} (A contact detail for the practitioner, e.g. a 1061 * telephone number or an email address.) 1062 */ 1063 // syntactic sugar 1064 public ContactPoint addTelecom() { // 3 1065 ContactPoint t = new ContactPoint(); 1066 if (this.telecom == null) 1067 this.telecom = new ArrayList<ContactPoint>(); 1068 this.telecom.add(t); 1069 return t; 1070 } 1071 1072 // syntactic sugar 1073 public Practitioner addTelecom(ContactPoint t) { // 3 1074 if (t == null) 1075 return this; 1076 if (this.telecom == null) 1077 this.telecom = new ArrayList<ContactPoint>(); 1078 this.telecom.add(t); 1079 return this; 1080 } 1081 1082 /** 1083 * @return {@link #address} (The postal address where the practitioner can be 1084 * found or visited or to which mail can be delivered.) 1085 */ 1086 public List<Address> getAddress() { 1087 if (this.address == null) 1088 this.address = new ArrayList<Address>(); 1089 return this.address; 1090 } 1091 1092 public boolean hasAddress() { 1093 if (this.address == null) 1094 return false; 1095 for (Address item : this.address) 1096 if (!item.isEmpty()) 1097 return true; 1098 return false; 1099 } 1100 1101 /** 1102 * @return {@link #address} (The postal address where the practitioner can be 1103 * found or visited or to which mail can be delivered.) 1104 */ 1105 // syntactic sugar 1106 public Address addAddress() { // 3 1107 Address t = new Address(); 1108 if (this.address == null) 1109 this.address = new ArrayList<Address>(); 1110 this.address.add(t); 1111 return t; 1112 } 1113 1114 // syntactic sugar 1115 public Practitioner addAddress(Address t) { // 3 1116 if (t == null) 1117 return this; 1118 if (this.address == null) 1119 this.address = new ArrayList<Address>(); 1120 this.address.add(t); 1121 return this; 1122 } 1123 1124 /** 1125 * @return {@link #gender} (Administrative Gender - the gender that the person 1126 * is considered to have for administration and record keeping 1127 * purposes.). This is the underlying object with id, value and 1128 * extensions. The accessor "getGender" gives direct access to the value 1129 */ 1130 public Enumeration<AdministrativeGender> getGenderElement() { 1131 if (this.gender == null) 1132 if (Configuration.errorOnAutoCreate()) 1133 throw new Error("Attempt to auto-create Practitioner.gender"); 1134 else if (Configuration.doAutoCreate()) 1135 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1136 return this.gender; 1137 } 1138 1139 public boolean hasGenderElement() { 1140 return this.gender != null && !this.gender.isEmpty(); 1141 } 1142 1143 public boolean hasGender() { 1144 return this.gender != null && !this.gender.isEmpty(); 1145 } 1146 1147 /** 1148 * @param value {@link #gender} (Administrative Gender - the gender that the 1149 * person is considered to have for administration and record 1150 * keeping purposes.). This is the underlying object with id, value 1151 * and extensions. The accessor "getGender" gives direct access to 1152 * the value 1153 */ 1154 public Practitioner setGenderElement(Enumeration<AdministrativeGender> value) { 1155 this.gender = value; 1156 return this; 1157 } 1158 1159 /** 1160 * @return Administrative Gender - the gender that the person is considered to 1161 * have for administration and record keeping purposes. 1162 */ 1163 public AdministrativeGender getGender() { 1164 return this.gender == null ? null : this.gender.getValue(); 1165 } 1166 1167 /** 1168 * @param value Administrative Gender - the gender that the person is considered 1169 * to have for administration and record keeping purposes. 1170 */ 1171 public Practitioner setGender(AdministrativeGender value) { 1172 if (value == null) 1173 this.gender = null; 1174 else { 1175 if (this.gender == null) 1176 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1177 this.gender.setValue(value); 1178 } 1179 return this; 1180 } 1181 1182 /** 1183 * @return {@link #birthDate} (The date of birth for the practitioner.). This is 1184 * the underlying object with id, value and extensions. The accessor 1185 * "getBirthDate" gives direct access to the value 1186 */ 1187 public DateType getBirthDateElement() { 1188 if (this.birthDate == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create Practitioner.birthDate"); 1191 else if (Configuration.doAutoCreate()) 1192 this.birthDate = new DateType(); // bb 1193 return this.birthDate; 1194 } 1195 1196 public boolean hasBirthDateElement() { 1197 return this.birthDate != null && !this.birthDate.isEmpty(); 1198 } 1199 1200 public boolean hasBirthDate() { 1201 return this.birthDate != null && !this.birthDate.isEmpty(); 1202 } 1203 1204 /** 1205 * @param value {@link #birthDate} (The date of birth for the practitioner.). 1206 * This is the underlying object with id, value and extensions. The 1207 * accessor "getBirthDate" gives direct access to the value 1208 */ 1209 public Practitioner setBirthDateElement(DateType value) { 1210 this.birthDate = value; 1211 return this; 1212 } 1213 1214 /** 1215 * @return The date of birth for the practitioner. 1216 */ 1217 public Date getBirthDate() { 1218 return this.birthDate == null ? null : this.birthDate.getValue(); 1219 } 1220 1221 /** 1222 * @param value The date of birth for the practitioner. 1223 */ 1224 public Practitioner setBirthDate(Date value) { 1225 if (value == null) 1226 this.birthDate = null; 1227 else { 1228 if (this.birthDate == null) 1229 this.birthDate = new DateType(); 1230 this.birthDate.setValue(value); 1231 } 1232 return this; 1233 } 1234 1235 /** 1236 * @return {@link #photo} (Image of the person.) 1237 */ 1238 public List<Attachment> getPhoto() { 1239 if (this.photo == null) 1240 this.photo = new ArrayList<Attachment>(); 1241 return this.photo; 1242 } 1243 1244 public boolean hasPhoto() { 1245 if (this.photo == null) 1246 return false; 1247 for (Attachment item : this.photo) 1248 if (!item.isEmpty()) 1249 return true; 1250 return false; 1251 } 1252 1253 /** 1254 * @return {@link #photo} (Image of the person.) 1255 */ 1256 // syntactic sugar 1257 public Attachment addPhoto() { // 3 1258 Attachment t = new Attachment(); 1259 if (this.photo == null) 1260 this.photo = new ArrayList<Attachment>(); 1261 this.photo.add(t); 1262 return t; 1263 } 1264 1265 // syntactic sugar 1266 public Practitioner addPhoto(Attachment t) { // 3 1267 if (t == null) 1268 return this; 1269 if (this.photo == null) 1270 this.photo = new ArrayList<Attachment>(); 1271 this.photo.add(t); 1272 return this; 1273 } 1274 1275 /** 1276 * @return {@link #practitionerRole} (The list of roles/organizations that the 1277 * practitioner is associated with.) 1278 */ 1279 public List<PractitionerPractitionerRoleComponent> getPractitionerRole() { 1280 if (this.practitionerRole == null) 1281 this.practitionerRole = new ArrayList<PractitionerPractitionerRoleComponent>(); 1282 return this.practitionerRole; 1283 } 1284 1285 public boolean hasPractitionerRole() { 1286 if (this.practitionerRole == null) 1287 return false; 1288 for (PractitionerPractitionerRoleComponent item : this.practitionerRole) 1289 if (!item.isEmpty()) 1290 return true; 1291 return false; 1292 } 1293 1294 /** 1295 * @return {@link #practitionerRole} (The list of roles/organizations that the 1296 * practitioner is associated with.) 1297 */ 1298 // syntactic sugar 1299 public PractitionerPractitionerRoleComponent addPractitionerRole() { // 3 1300 PractitionerPractitionerRoleComponent t = new PractitionerPractitionerRoleComponent(); 1301 if (this.practitionerRole == null) 1302 this.practitionerRole = new ArrayList<PractitionerPractitionerRoleComponent>(); 1303 this.practitionerRole.add(t); 1304 return t; 1305 } 1306 1307 // syntactic sugar 1308 public Practitioner addPractitionerRole(PractitionerPractitionerRoleComponent t) { // 3 1309 if (t == null) 1310 return this; 1311 if (this.practitionerRole == null) 1312 this.practitionerRole = new ArrayList<PractitionerPractitionerRoleComponent>(); 1313 this.practitionerRole.add(t); 1314 return this; 1315 } 1316 1317 /** 1318 * @return {@link #qualification} (Qualifications obtained by training and 1319 * certification.) 1320 */ 1321 public List<PractitionerQualificationComponent> getQualification() { 1322 if (this.qualification == null) 1323 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1324 return this.qualification; 1325 } 1326 1327 public boolean hasQualification() { 1328 if (this.qualification == null) 1329 return false; 1330 for (PractitionerQualificationComponent item : this.qualification) 1331 if (!item.isEmpty()) 1332 return true; 1333 return false; 1334 } 1335 1336 /** 1337 * @return {@link #qualification} (Qualifications obtained by training and 1338 * certification.) 1339 */ 1340 // syntactic sugar 1341 public PractitionerQualificationComponent addQualification() { // 3 1342 PractitionerQualificationComponent t = new PractitionerQualificationComponent(); 1343 if (this.qualification == null) 1344 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1345 this.qualification.add(t); 1346 return t; 1347 } 1348 1349 // syntactic sugar 1350 public Practitioner addQualification(PractitionerQualificationComponent t) { // 3 1351 if (t == null) 1352 return this; 1353 if (this.qualification == null) 1354 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1355 this.qualification.add(t); 1356 return this; 1357 } 1358 1359 /** 1360 * @return {@link #communication} (A language the practitioner is able to use in 1361 * patient communication.) 1362 */ 1363 public List<CodeableConcept> getCommunication() { 1364 if (this.communication == null) 1365 this.communication = new ArrayList<CodeableConcept>(); 1366 return this.communication; 1367 } 1368 1369 public boolean hasCommunication() { 1370 if (this.communication == null) 1371 return false; 1372 for (CodeableConcept item : this.communication) 1373 if (!item.isEmpty()) 1374 return true; 1375 return false; 1376 } 1377 1378 /** 1379 * @return {@link #communication} (A language the practitioner is able to use in 1380 * patient communication.) 1381 */ 1382 // syntactic sugar 1383 public CodeableConcept addCommunication() { // 3 1384 CodeableConcept t = new CodeableConcept(); 1385 if (this.communication == null) 1386 this.communication = new ArrayList<CodeableConcept>(); 1387 this.communication.add(t); 1388 return t; 1389 } 1390 1391 // syntactic sugar 1392 public Practitioner addCommunication(CodeableConcept t) { // 3 1393 if (t == null) 1394 return this; 1395 if (this.communication == null) 1396 this.communication = new ArrayList<CodeableConcept>(); 1397 this.communication.add(t); 1398 return this; 1399 } 1400 1401 protected void listChildren(List<Property> childrenList) { 1402 super.listChildren(childrenList); 1403 childrenList.add(new Property("identifier", "Identifier", "An identifier that applies to this person in this role.", 1404 0, java.lang.Integer.MAX_VALUE, identifier)); 1405 childrenList.add(new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1406 java.lang.Integer.MAX_VALUE, active)); 1407 childrenList.add( 1408 new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 1409 childrenList.add(new Property("telecom", "ContactPoint", 1410 "A contact detail for the practitioner, e.g. a telephone number or an email address.", 0, 1411 java.lang.Integer.MAX_VALUE, telecom)); 1412 childrenList.add(new Property("address", "Address", 1413 "The postal address where the practitioner can be found or visited or to which mail can be delivered.", 0, 1414 java.lang.Integer.MAX_VALUE, address)); 1415 childrenList.add(new Property("gender", "code", 1416 "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 1417 0, java.lang.Integer.MAX_VALUE, gender)); 1418 childrenList.add(new Property("birthDate", "date", "The date of birth for the practitioner.", 0, 1419 java.lang.Integer.MAX_VALUE, birthDate)); 1420 childrenList 1421 .add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 1422 childrenList.add(new Property("practitionerRole", "", 1423 "The list of roles/organizations that the practitioner is associated with.", 0, java.lang.Integer.MAX_VALUE, 1424 practitionerRole)); 1425 childrenList.add(new Property("qualification", "", "Qualifications obtained by training and certification.", 0, 1426 java.lang.Integer.MAX_VALUE, qualification)); 1427 childrenList.add(new Property("communication", "CodeableConcept", 1428 "A language the practitioner is able to use in patient communication.", 0, java.lang.Integer.MAX_VALUE, 1429 communication)); 1430 } 1431 1432 @Override 1433 public void setProperty(String name, Base value) throws FHIRException { 1434 if (name.equals("identifier")) 1435 this.getIdentifier().add(castToIdentifier(value)); 1436 else if (name.equals("active")) 1437 this.active = castToBoolean(value); // BooleanType 1438 else if (name.equals("name")) 1439 this.name = castToHumanName(value); // HumanName 1440 else if (name.equals("telecom")) 1441 this.getTelecom().add(castToContactPoint(value)); 1442 else if (name.equals("address")) 1443 this.getAddress().add(castToAddress(value)); 1444 else if (name.equals("gender")) 1445 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 1446 else if (name.equals("birthDate")) 1447 this.birthDate = castToDate(value); // DateType 1448 else if (name.equals("photo")) 1449 this.getPhoto().add(castToAttachment(value)); 1450 else if (name.equals("practitionerRole")) 1451 this.getPractitionerRole().add((PractitionerPractitionerRoleComponent) value); 1452 else if (name.equals("qualification")) 1453 this.getQualification().add((PractitionerQualificationComponent) value); 1454 else if (name.equals("communication")) 1455 this.getCommunication().add(castToCodeableConcept(value)); 1456 else 1457 super.setProperty(name, value); 1458 } 1459 1460 @Override 1461 public Base addChild(String name) throws FHIRException { 1462 if (name.equals("identifier")) { 1463 return addIdentifier(); 1464 } else if (name.equals("active")) { 1465 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.active"); 1466 } else if (name.equals("name")) { 1467 this.name = new HumanName(); 1468 return this.name; 1469 } else if (name.equals("telecom")) { 1470 return addTelecom(); 1471 } else if (name.equals("address")) { 1472 return addAddress(); 1473 } else if (name.equals("gender")) { 1474 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.gender"); 1475 } else if (name.equals("birthDate")) { 1476 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.birthDate"); 1477 } else if (name.equals("photo")) { 1478 return addPhoto(); 1479 } else if (name.equals("practitionerRole")) { 1480 return addPractitionerRole(); 1481 } else if (name.equals("qualification")) { 1482 return addQualification(); 1483 } else if (name.equals("communication")) { 1484 return addCommunication(); 1485 } else 1486 return super.addChild(name); 1487 } 1488 1489 public String fhirType() { 1490 return "Practitioner"; 1491 1492 } 1493 1494 public Practitioner copy() { 1495 Practitioner dst = new Practitioner(); 1496 copyValues(dst); 1497 if (identifier != null) { 1498 dst.identifier = new ArrayList<Identifier>(); 1499 for (Identifier i : identifier) 1500 dst.identifier.add(i.copy()); 1501 } 1502 ; 1503 dst.active = active == null ? null : active.copy(); 1504 dst.name = name == null ? null : name.copy(); 1505 if (telecom != null) { 1506 dst.telecom = new ArrayList<ContactPoint>(); 1507 for (ContactPoint i : telecom) 1508 dst.telecom.add(i.copy()); 1509 } 1510 ; 1511 if (address != null) { 1512 dst.address = new ArrayList<Address>(); 1513 for (Address i : address) 1514 dst.address.add(i.copy()); 1515 } 1516 ; 1517 dst.gender = gender == null ? null : gender.copy(); 1518 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1519 if (photo != null) { 1520 dst.photo = new ArrayList<Attachment>(); 1521 for (Attachment i : photo) 1522 dst.photo.add(i.copy()); 1523 } 1524 ; 1525 if (practitionerRole != null) { 1526 dst.practitionerRole = new ArrayList<PractitionerPractitionerRoleComponent>(); 1527 for (PractitionerPractitionerRoleComponent i : practitionerRole) 1528 dst.practitionerRole.add(i.copy()); 1529 } 1530 ; 1531 if (qualification != null) { 1532 dst.qualification = new ArrayList<PractitionerQualificationComponent>(); 1533 for (PractitionerQualificationComponent i : qualification) 1534 dst.qualification.add(i.copy()); 1535 } 1536 ; 1537 if (communication != null) { 1538 dst.communication = new ArrayList<CodeableConcept>(); 1539 for (CodeableConcept i : communication) 1540 dst.communication.add(i.copy()); 1541 } 1542 ; 1543 return dst; 1544 } 1545 1546 protected Practitioner typedCopy() { 1547 return copy(); 1548 } 1549 1550 @Override 1551 public boolean equalsDeep(Base other) { 1552 if (!super.equalsDeep(other)) 1553 return false; 1554 if (!(other instanceof Practitioner)) 1555 return false; 1556 Practitioner o = (Practitioner) other; 1557 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 1558 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 1559 && compareDeep(address, o.address, true) && compareDeep(gender, o.gender, true) 1560 && compareDeep(birthDate, o.birthDate, true) && compareDeep(photo, o.photo, true) 1561 && compareDeep(practitionerRole, o.practitionerRole, true) && compareDeep(qualification, o.qualification, true) 1562 && compareDeep(communication, o.communication, true); 1563 } 1564 1565 @Override 1566 public boolean equalsShallow(Base other) { 1567 if (!super.equalsShallow(other)) 1568 return false; 1569 if (!(other instanceof Practitioner)) 1570 return false; 1571 Practitioner o = (Practitioner) other; 1572 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 1573 && compareValues(birthDate, o.birthDate, true); 1574 } 1575 1576 public boolean isEmpty() { 1577 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (active == null || active.isEmpty()) 1578 && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()) 1579 && (address == null || address.isEmpty()) && (gender == null || gender.isEmpty()) 1580 && (birthDate == null || birthDate.isEmpty()) && (photo == null || photo.isEmpty()) 1581 && (practitionerRole == null || practitionerRole.isEmpty()) 1582 && (qualification == null || qualification.isEmpty()) && (communication == null || communication.isEmpty()); 1583 } 1584 1585 @Override 1586 public ResourceType getResourceType() { 1587 return ResourceType.Practitioner; 1588 } 1589 1590 @SearchParamDefinition(name = "identifier", path = "Practitioner.identifier", description = "A practitioner's Identifier", type = "token") 1591 public static final String SP_IDENTIFIER = "identifier"; 1592 @SearchParamDefinition(name = "given", path = "Practitioner.name.given", description = "A portion of the given name", type = "string") 1593 public static final String SP_GIVEN = "given"; 1594 @SearchParamDefinition(name = "specialty", path = "Practitioner.practitionerRole.specialty", description = "The practitioner has this specialty at an organization", type = "token") 1595 public static final String SP_SPECIALTY = "specialty"; 1596 @SearchParamDefinition(name = "address", path = "Practitioner.address", description = "An address in any kind of address/part", type = "string") 1597 public static final String SP_ADDRESS = "address"; 1598 @SearchParamDefinition(name = "role", path = "Practitioner.practitionerRole.role", description = "The practitioner can perform this role at for the organization", type = "token") 1599 public static final String SP_ROLE = "role"; 1600 @SearchParamDefinition(name = "address-state", path = "Practitioner.address.state", description = "A state specified in an address", type = "string") 1601 public static final String SP_ADDRESSSTATE = "address-state"; 1602 @SearchParamDefinition(name = "gender", path = "Practitioner.gender", description = "Gender of the practitioner", type = "token") 1603 public static final String SP_GENDER = "gender"; 1604 @SearchParamDefinition(name = "address-postalcode", path = "Practitioner.address.postalCode", description = "A postalCode specified in an address", type = "string") 1605 public static final String SP_ADDRESSPOSTALCODE = "address-postalcode"; 1606 @SearchParamDefinition(name = "address-country", path = "Practitioner.address.country", description = "A country specified in an address", type = "string") 1607 public static final String SP_ADDRESSCOUNTRY = "address-country"; 1608 @SearchParamDefinition(name = "phonetic", path = "Practitioner.name", description = "A portion of either family or given name using some kind of phonetic matching algorithm", type = "string") 1609 public static final String SP_PHONETIC = "phonetic"; 1610 @SearchParamDefinition(name = "phone", path = "Practitioner.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 1611 public static final String SP_PHONE = "phone"; 1612 @SearchParamDefinition(name = "organization", path = "Practitioner.practitionerRole.managingOrganization", description = "The identity of the organization the practitioner represents / acts on behalf of", type = "reference") 1613 public static final String SP_ORGANIZATION = "organization"; 1614 @SearchParamDefinition(name = "name", path = "Practitioner.name", description = "A portion of either family or given name", type = "string") 1615 public static final String SP_NAME = "name"; 1616 @SearchParamDefinition(name = "address-use", path = "Practitioner.address.use", description = "A use code specified in an address", type = "token") 1617 public static final String SP_ADDRESSUSE = "address-use"; 1618 @SearchParamDefinition(name = "telecom", path = "Practitioner.telecom", description = "The value in any kind of contact", type = "token") 1619 public static final String SP_TELECOM = "telecom"; 1620 @SearchParamDefinition(name = "location", path = "Practitioner.practitionerRole.location", description = "One of the locations at which this practitioner provides care", type = "reference") 1621 public static final String SP_LOCATION = "location"; 1622 @SearchParamDefinition(name = "family", path = "Practitioner.name.family", description = "A portion of the family name", type = "string") 1623 public static final String SP_FAMILY = "family"; 1624 @SearchParamDefinition(name = "address-city", path = "Practitioner.address.city", description = "A city specified in an address", type = "string") 1625 public static final String SP_ADDRESSCITY = "address-city"; 1626 @SearchParamDefinition(name = "communication", path = "Practitioner.communication", description = "One of the languages that the practitioner can communicate with", type = "token") 1627 public static final String SP_COMMUNICATION = "communication"; 1628 @SearchParamDefinition(name = "email", path = "Practitioner.telecom.where(system='email')", description = "A value in an email contact", type = "token") 1629 public static final String SP_EMAIL = "email"; 1630 1631}