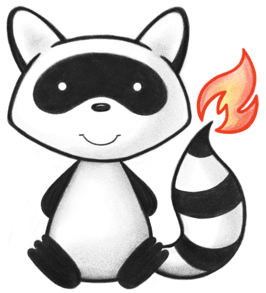
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043 044/** 045 * An action that is or was performed on a patient. This can be a physical 046 * intervention like an operation, or less invasive like counseling or 047 * hypnotherapy. 048 */ 049@ResourceDef(name = "Procedure", profile = "http://hl7.org/fhir/Profile/Procedure") 050public class Procedure extends DomainResource { 051 052 public enum ProcedureStatus { 053 /** 054 * The procedure is still occurring. 055 */ 056 INPROGRESS, 057 /** 058 * The procedure was terminated without completing successfully. 059 */ 060 ABORTED, 061 /** 062 * All actions involved in the procedure have taken place. 063 */ 064 COMPLETED, 065 /** 066 * The statement was entered in error and Is not valid. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static ProcedureStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("in-progress".equals(codeString)) 078 return INPROGRESS; 079 if ("aborted".equals(codeString)) 080 return ABORTED; 081 if ("completed".equals(codeString)) 082 return COMPLETED; 083 if ("entered-in-error".equals(codeString)) 084 return ENTEREDINERROR; 085 throw new FHIRException("Unknown ProcedureStatus code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case INPROGRESS: 091 return "in-progress"; 092 case ABORTED: 093 return "aborted"; 094 case COMPLETED: 095 return "completed"; 096 case ENTEREDINERROR: 097 return "entered-in-error"; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105 public String getSystem() { 106 switch (this) { 107 case INPROGRESS: 108 return "http://hl7.org/fhir/procedure-status"; 109 case ABORTED: 110 return "http://hl7.org/fhir/procedure-status"; 111 case COMPLETED: 112 return "http://hl7.org/fhir/procedure-status"; 113 case ENTEREDINERROR: 114 return "http://hl7.org/fhir/procedure-status"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getDefinition() { 123 switch (this) { 124 case INPROGRESS: 125 return "The procedure is still occurring."; 126 case ABORTED: 127 return "The procedure was terminated without completing successfully."; 128 case COMPLETED: 129 return "All actions involved in the procedure have taken place."; 130 case ENTEREDINERROR: 131 return "The statement was entered in error and Is not valid."; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDisplay() { 140 switch (this) { 141 case INPROGRESS: 142 return "In Progress"; 143 case ABORTED: 144 return "Aboted"; 145 case COMPLETED: 146 return "Completed"; 147 case ENTEREDINERROR: 148 return "Entered in Error"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 } 156 157 public static class ProcedureStatusEnumFactory implements EnumFactory<ProcedureStatus> { 158 public ProcedureStatus fromCode(String codeString) throws IllegalArgumentException { 159 if (codeString == null || "".equals(codeString)) 160 if (codeString == null || "".equals(codeString)) 161 return null; 162 if ("in-progress".equals(codeString)) 163 return ProcedureStatus.INPROGRESS; 164 if ("aborted".equals(codeString)) 165 return ProcedureStatus.ABORTED; 166 if ("completed".equals(codeString)) 167 return ProcedureStatus.COMPLETED; 168 if ("entered-in-error".equals(codeString)) 169 return ProcedureStatus.ENTEREDINERROR; 170 throw new IllegalArgumentException("Unknown ProcedureStatus code '" + codeString + "'"); 171 } 172 173 public Enumeration<ProcedureStatus> fromType(Base code) throws FHIRException { 174 if (code == null || code.isEmpty()) 175 return null; 176 String codeString = ((PrimitiveType) code).asStringValue(); 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("in-progress".equals(codeString)) 180 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.INPROGRESS); 181 if ("aborted".equals(codeString)) 182 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.ABORTED); 183 if ("completed".equals(codeString)) 184 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.COMPLETED); 185 if ("entered-in-error".equals(codeString)) 186 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.ENTEREDINERROR); 187 throw new FHIRException("Unknown ProcedureStatus code '" + codeString + "'"); 188 } 189 190 public String toCode(ProcedureStatus code) { 191 if (code == ProcedureStatus.INPROGRESS) 192 return "in-progress"; 193 if (code == ProcedureStatus.ABORTED) 194 return "aborted"; 195 if (code == ProcedureStatus.COMPLETED) 196 return "completed"; 197 if (code == ProcedureStatus.ENTEREDINERROR) 198 return "entered-in-error"; 199 return "?"; 200 } 201 } 202 203 @Block() 204 public static class ProcedurePerformerComponent extends BackboneElement implements IBaseBackboneElement { 205 /** 206 * The practitioner who was involved in the procedure. 207 */ 208 @Child(name = "actor", type = { Practitioner.class, Organization.class, Patient.class, 209 RelatedPerson.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 210 @Description(shortDefinition = "The reference to the practitioner", formalDefinition = "The practitioner who was involved in the procedure.") 211 protected Reference actor; 212 213 /** 214 * The actual object that is the target of the reference (The practitioner who 215 * was involved in the procedure.) 216 */ 217 protected Resource actorTarget; 218 219 /** 220 * For example: surgeon, anaethetist, endoscopist. 221 */ 222 @Child(name = "role", type = { 223 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "The role the actor was in", formalDefinition = "For example: surgeon, anaethetist, endoscopist.") 225 protected CodeableConcept role; 226 227 private static final long serialVersionUID = -843698327L; 228 229 /* 230 * Constructor 231 */ 232 public ProcedurePerformerComponent() { 233 super(); 234 } 235 236 /** 237 * @return {@link #actor} (The practitioner who was involved in the procedure.) 238 */ 239 public Reference getActor() { 240 if (this.actor == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create ProcedurePerformerComponent.actor"); 243 else if (Configuration.doAutoCreate()) 244 this.actor = new Reference(); // cc 245 return this.actor; 246 } 247 248 public boolean hasActor() { 249 return this.actor != null && !this.actor.isEmpty(); 250 } 251 252 /** 253 * @param value {@link #actor} (The practitioner who was involved in the 254 * procedure.) 255 */ 256 public ProcedurePerformerComponent setActor(Reference value) { 257 this.actor = value; 258 return this; 259 } 260 261 /** 262 * @return {@link #actor} The actual object that is the target of the reference. 263 * The reference library doesn't populate this, but you can use it to 264 * hold the resource if you resolve it. (The practitioner who was 265 * involved in the procedure.) 266 */ 267 public Resource getActorTarget() { 268 return this.actorTarget; 269 } 270 271 /** 272 * @param value {@link #actor} The actual object that is the target of the 273 * reference. The reference library doesn't use these, but you can 274 * use it to hold the resource if you resolve it. (The practitioner 275 * who was involved in the procedure.) 276 */ 277 public ProcedurePerformerComponent setActorTarget(Resource value) { 278 this.actorTarget = value; 279 return this; 280 } 281 282 /** 283 * @return {@link #role} (For example: surgeon, anaethetist, endoscopist.) 284 */ 285 public CodeableConcept getRole() { 286 if (this.role == null) 287 if (Configuration.errorOnAutoCreate()) 288 throw new Error("Attempt to auto-create ProcedurePerformerComponent.role"); 289 else if (Configuration.doAutoCreate()) 290 this.role = new CodeableConcept(); // cc 291 return this.role; 292 } 293 294 public boolean hasRole() { 295 return this.role != null && !this.role.isEmpty(); 296 } 297 298 /** 299 * @param value {@link #role} (For example: surgeon, anaethetist, endoscopist.) 300 */ 301 public ProcedurePerformerComponent setRole(CodeableConcept value) { 302 this.role = value; 303 return this; 304 } 305 306 protected void listChildren(List<Property> childrenList) { 307 super.listChildren(childrenList); 308 childrenList.add(new Property("actor", "Reference(Practitioner|Organization|Patient|RelatedPerson)", 309 "The practitioner who was involved in the procedure.", 0, java.lang.Integer.MAX_VALUE, actor)); 310 childrenList.add(new Property("role", "CodeableConcept", "For example: surgeon, anaethetist, endoscopist.", 0, 311 java.lang.Integer.MAX_VALUE, role)); 312 } 313 314 @Override 315 public void setProperty(String name, Base value) throws FHIRException { 316 if (name.equals("actor")) 317 this.actor = castToReference(value); // Reference 318 else if (name.equals("role")) 319 this.role = castToCodeableConcept(value); // CodeableConcept 320 else 321 super.setProperty(name, value); 322 } 323 324 @Override 325 public Base addChild(String name) throws FHIRException { 326 if (name.equals("actor")) { 327 this.actor = new Reference(); 328 return this.actor; 329 } else if (name.equals("role")) { 330 this.role = new CodeableConcept(); 331 return this.role; 332 } else 333 return super.addChild(name); 334 } 335 336 public ProcedurePerformerComponent copy() { 337 ProcedurePerformerComponent dst = new ProcedurePerformerComponent(); 338 copyValues(dst); 339 dst.actor = actor == null ? null : actor.copy(); 340 dst.role = role == null ? null : role.copy(); 341 return dst; 342 } 343 344 @Override 345 public boolean equalsDeep(Base other) { 346 if (!super.equalsDeep(other)) 347 return false; 348 if (!(other instanceof ProcedurePerformerComponent)) 349 return false; 350 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other; 351 return compareDeep(actor, o.actor, true) && compareDeep(role, o.role, true); 352 } 353 354 @Override 355 public boolean equalsShallow(Base other) { 356 if (!super.equalsShallow(other)) 357 return false; 358 if (!(other instanceof ProcedurePerformerComponent)) 359 return false; 360 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other; 361 return true; 362 } 363 364 public boolean isEmpty() { 365 return super.isEmpty() && (actor == null || actor.isEmpty()) && (role == null || role.isEmpty()); 366 } 367 368 public String fhirType() { 369 return "Procedure.performer"; 370 371 } 372 373 } 374 375 @Block() 376 public static class ProcedureFocalDeviceComponent extends BackboneElement implements IBaseBackboneElement { 377 /** 378 * The kind of change that happened to the device during the procedure. 379 */ 380 @Child(name = "action", type = { 381 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 382 @Description(shortDefinition = "Kind of change to device", formalDefinition = "The kind of change that happened to the device during the procedure.") 383 protected CodeableConcept action; 384 385 /** 386 * The device that was manipulated (changed) during the procedure. 387 */ 388 @Child(name = "manipulated", type = { 389 Device.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 390 @Description(shortDefinition = "Device that was changed", formalDefinition = "The device that was manipulated (changed) during the procedure.") 391 protected Reference manipulated; 392 393 /** 394 * The actual object that is the target of the reference (The device that was 395 * manipulated (changed) during the procedure.) 396 */ 397 protected Device manipulatedTarget; 398 399 private static final long serialVersionUID = 1779937807L; 400 401 /* 402 * Constructor 403 */ 404 public ProcedureFocalDeviceComponent() { 405 super(); 406 } 407 408 /* 409 * Constructor 410 */ 411 public ProcedureFocalDeviceComponent(Reference manipulated) { 412 super(); 413 this.manipulated = manipulated; 414 } 415 416 /** 417 * @return {@link #action} (The kind of change that happened to the device 418 * during the procedure.) 419 */ 420 public CodeableConcept getAction() { 421 if (this.action == null) 422 if (Configuration.errorOnAutoCreate()) 423 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.action"); 424 else if (Configuration.doAutoCreate()) 425 this.action = new CodeableConcept(); // cc 426 return this.action; 427 } 428 429 public boolean hasAction() { 430 return this.action != null && !this.action.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #action} (The kind of change that happened to the device 435 * during the procedure.) 436 */ 437 public ProcedureFocalDeviceComponent setAction(CodeableConcept value) { 438 this.action = value; 439 return this; 440 } 441 442 /** 443 * @return {@link #manipulated} (The device that was manipulated (changed) 444 * during the procedure.) 445 */ 446 public Reference getManipulated() { 447 if (this.manipulated == null) 448 if (Configuration.errorOnAutoCreate()) 449 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.manipulated"); 450 else if (Configuration.doAutoCreate()) 451 this.manipulated = new Reference(); // cc 452 return this.manipulated; 453 } 454 455 public boolean hasManipulated() { 456 return this.manipulated != null && !this.manipulated.isEmpty(); 457 } 458 459 /** 460 * @param value {@link #manipulated} (The device that was manipulated (changed) 461 * during the procedure.) 462 */ 463 public ProcedureFocalDeviceComponent setManipulated(Reference value) { 464 this.manipulated = value; 465 return this; 466 } 467 468 /** 469 * @return {@link #manipulated} The actual object that is the target of the 470 * reference. The reference library doesn't populate this, but you can 471 * use it to hold the resource if you resolve it. (The device that was 472 * manipulated (changed) during the procedure.) 473 */ 474 public Device getManipulatedTarget() { 475 if (this.manipulatedTarget == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.manipulated"); 478 else if (Configuration.doAutoCreate()) 479 this.manipulatedTarget = new Device(); // aa 480 return this.manipulatedTarget; 481 } 482 483 /** 484 * @param value {@link #manipulated} The actual object that is the target of the 485 * reference. The reference library doesn't use these, but you can 486 * use it to hold the resource if you resolve it. (The device that 487 * was manipulated (changed) during the procedure.) 488 */ 489 public ProcedureFocalDeviceComponent setManipulatedTarget(Device value) { 490 this.manipulatedTarget = value; 491 return this; 492 } 493 494 protected void listChildren(List<Property> childrenList) { 495 super.listChildren(childrenList); 496 childrenList.add(new Property("action", "CodeableConcept", 497 "The kind of change that happened to the device during the procedure.", 0, java.lang.Integer.MAX_VALUE, 498 action)); 499 childrenList.add(new Property("manipulated", "Reference(Device)", 500 "The device that was manipulated (changed) during the procedure.", 0, java.lang.Integer.MAX_VALUE, 501 manipulated)); 502 } 503 504 @Override 505 public void setProperty(String name, Base value) throws FHIRException { 506 if (name.equals("action")) 507 this.action = castToCodeableConcept(value); // CodeableConcept 508 else if (name.equals("manipulated")) 509 this.manipulated = castToReference(value); // Reference 510 else 511 super.setProperty(name, value); 512 } 513 514 @Override 515 public Base addChild(String name) throws FHIRException { 516 if (name.equals("action")) { 517 this.action = new CodeableConcept(); 518 return this.action; 519 } else if (name.equals("manipulated")) { 520 this.manipulated = new Reference(); 521 return this.manipulated; 522 } else 523 return super.addChild(name); 524 } 525 526 public ProcedureFocalDeviceComponent copy() { 527 ProcedureFocalDeviceComponent dst = new ProcedureFocalDeviceComponent(); 528 copyValues(dst); 529 dst.action = action == null ? null : action.copy(); 530 dst.manipulated = manipulated == null ? null : manipulated.copy(); 531 return dst; 532 } 533 534 @Override 535 public boolean equalsDeep(Base other) { 536 if (!super.equalsDeep(other)) 537 return false; 538 if (!(other instanceof ProcedureFocalDeviceComponent)) 539 return false; 540 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other; 541 return compareDeep(action, o.action, true) && compareDeep(manipulated, o.manipulated, true); 542 } 543 544 @Override 545 public boolean equalsShallow(Base other) { 546 if (!super.equalsShallow(other)) 547 return false; 548 if (!(other instanceof ProcedureFocalDeviceComponent)) 549 return false; 550 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other; 551 return true; 552 } 553 554 public boolean isEmpty() { 555 return super.isEmpty() && (action == null || action.isEmpty()) && (manipulated == null || manipulated.isEmpty()); 556 } 557 558 public String fhirType() { 559 return "Procedure.focalDevice"; 560 561 } 562 563 } 564 565 /** 566 * This records identifiers associated with this procedure that are defined by 567 * business processes and/or used to refer to it when a direct URL reference to 568 * the resource itself is not appropriate (e.g. in CDA documents, or in written 569 * / printed documentation). 570 */ 571 @Child(name = "identifier", type = { 572 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 573 @Description(shortDefinition = "External Identifiers for this procedure", formalDefinition = "This records identifiers associated with this procedure that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 574 protected List<Identifier> identifier; 575 576 /** 577 * The person, animal or group on which the procedure was performed. 578 */ 579 @Child(name = "subject", type = { Patient.class, 580 Group.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 581 @Description(shortDefinition = "Who the procedure was performed on", formalDefinition = "The person, animal or group on which the procedure was performed.") 582 protected Reference subject; 583 584 /** 585 * The actual object that is the target of the reference (The person, animal or 586 * group on which the procedure was performed.) 587 */ 588 protected Resource subjectTarget; 589 590 /** 591 * A code specifying the state of the procedure. Generally this will be 592 * in-progress or completed state. 593 */ 594 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 595 @Description(shortDefinition = "in-progress | aborted | completed | entered-in-error", formalDefinition = "A code specifying the state of the procedure. Generally this will be in-progress or completed state.") 596 protected Enumeration<ProcedureStatus> status; 597 598 /** 599 * A code that classifies the procedure for searching, sorting and display 600 * purposes (e.g. "Surgical Procedure"). 601 */ 602 @Child(name = "category", type = { 603 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 604 @Description(shortDefinition = "Classification of the procedure", formalDefinition = "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").") 605 protected CodeableConcept category; 606 607 /** 608 * The specific procedure that is performed. Use text if the exact nature of the 609 * procedure cannot be coded (e.g. "Laparoscopic Appendectomy"). 610 */ 611 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 612 @Description(shortDefinition = "Identification of the procedure", formalDefinition = "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").") 613 protected CodeableConcept code; 614 615 /** 616 * Set this to true if the record is saying that the procedure was NOT 617 * performed. 618 */ 619 @Child(name = "notPerformed", type = { 620 BooleanType.class }, order = 5, min = 0, max = 1, modifier = true, summary = false) 621 @Description(shortDefinition = "True if procedure was not performed as scheduled", formalDefinition = "Set this to true if the record is saying that the procedure was NOT performed.") 622 protected BooleanType notPerformed; 623 624 /** 625 * A code indicating why the procedure was not performed. 626 */ 627 @Child(name = "reasonNotPerformed", type = { 628 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 629 @Description(shortDefinition = "Reason procedure was not performed", formalDefinition = "A code indicating why the procedure was not performed.") 630 protected List<CodeableConcept> reasonNotPerformed; 631 632 /** 633 * Detailed and structured anatomical location information. Multiple locations 634 * are allowed - e.g. multiple punch biopsies of a lesion. 635 */ 636 @Child(name = "bodySite", type = { 637 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 638 @Description(shortDefinition = "Target body sites", formalDefinition = "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.") 639 protected List<CodeableConcept> bodySite; 640 641 /** 642 * The reason why the procedure was performed. This may be due to a Condition, 643 * may be coded entity of some type, or may simply be present as text. 644 */ 645 @Child(name = "reason", type = { CodeableConcept.class, 646 Condition.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 647 @Description(shortDefinition = "Reason procedure performed", formalDefinition = "The reason why the procedure was performed. This may be due to a Condition, may be coded entity of some type, or may simply be present as text.") 648 protected Type reason; 649 650 /** 651 * Limited to 'real' people rather than equipment. 652 */ 653 @Child(name = "performer", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 654 @Description(shortDefinition = "The people who performed the procedure", formalDefinition = "Limited to 'real' people rather than equipment.") 655 protected List<ProcedurePerformerComponent> performer; 656 657 /** 658 * The date(time)/period over which the procedure was performed. Allows a period 659 * to support complex procedures that span more than one date, and also allows 660 * for the length of the procedure to be captured. 661 */ 662 @Child(name = "performed", type = { DateTimeType.class, 663 Period.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 664 @Description(shortDefinition = "Date/Period the procedure was performed", formalDefinition = "The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.") 665 protected Type performed; 666 667 /** 668 * The encounter during which the procedure was performed. 669 */ 670 @Child(name = "encounter", type = { Encounter.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 671 @Description(shortDefinition = "The encounter associated with the procedure", formalDefinition = "The encounter during which the procedure was performed.") 672 protected Reference encounter; 673 674 /** 675 * The actual object that is the target of the reference (The encounter during 676 * which the procedure was performed.) 677 */ 678 protected Encounter encounterTarget; 679 680 /** 681 * The location where the procedure actually happened. E.g. a newborn at home, a 682 * tracheostomy at a restaurant. 683 */ 684 @Child(name = "location", type = { Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 685 @Description(shortDefinition = "Where the procedure happened", formalDefinition = "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.") 686 protected Reference location; 687 688 /** 689 * The actual object that is the target of the reference (The location where the 690 * procedure actually happened. E.g. a newborn at home, a tracheostomy at a 691 * restaurant.) 692 */ 693 protected Location locationTarget; 694 695 /** 696 * The outcome of the procedure - did it resolve reasons for the procedure being 697 * performed? 698 */ 699 @Child(name = "outcome", type = { 700 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 701 @Description(shortDefinition = "The result of procedure", formalDefinition = "The outcome of the procedure - did it resolve reasons for the procedure being performed?") 702 protected CodeableConcept outcome; 703 704 /** 705 * This could be a histology result, pathology report, surgical report, etc.. 706 */ 707 @Child(name = "report", type = { 708 DiagnosticReport.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 709 @Description(shortDefinition = "Any report resulting from the procedure", formalDefinition = "This could be a histology result, pathology report, surgical report, etc..") 710 protected List<Reference> report; 711 /** 712 * The actual objects that are the target of the reference (This could be a 713 * histology result, pathology report, surgical report, etc..) 714 */ 715 protected List<DiagnosticReport> reportTarget; 716 717 /** 718 * Any complications that occurred during the procedure, or in the immediate 719 * post-performance period. These are generally tracked separately from the 720 * notes, which will typically describe the procedure itself rather than any 721 * 'post procedure' issues. 722 */ 723 @Child(name = "complication", type = { 724 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 725 @Description(shortDefinition = "Complication following the procedure", formalDefinition = "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.") 726 protected List<CodeableConcept> complication; 727 728 /** 729 * If the procedure required specific follow up - e.g. removal of sutures. The 730 * followup may be represented as a simple note, or could potentially be more 731 * complex in which case the CarePlan resource can be used. 732 */ 733 @Child(name = "followUp", type = { 734 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 735 @Description(shortDefinition = "Instructions for follow up", formalDefinition = "If the procedure required specific follow up - e.g. removal of sutures. The followup may be represented as a simple note, or could potentially be more complex in which case the CarePlan resource can be used.") 736 protected List<CodeableConcept> followUp; 737 738 /** 739 * A reference to a resource that contains details of the request for this 740 * procedure. 741 */ 742 @Child(name = "request", type = { CarePlan.class, DiagnosticOrder.class, ProcedureRequest.class, 743 ReferralRequest.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 744 @Description(shortDefinition = "A request for this procedure", formalDefinition = "A reference to a resource that contains details of the request for this procedure.") 745 protected Reference request; 746 747 /** 748 * The actual object that is the target of the reference (A reference to a 749 * resource that contains details of the request for this procedure.) 750 */ 751 protected Resource requestTarget; 752 753 /** 754 * Any other notes about the procedure. E.g. the operative notes. 755 */ 756 @Child(name = "notes", type = { 757 Annotation.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 758 @Description(shortDefinition = "Additional information about the procedure", formalDefinition = "Any other notes about the procedure. E.g. the operative notes.") 759 protected List<Annotation> notes; 760 761 /** 762 * A device that is implanted, removed or otherwise manipulated (calibration, 763 * battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a 764 * focal portion of the Procedure. 765 */ 766 @Child(name = "focalDevice", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 767 @Description(shortDefinition = "Device changed in procedure", formalDefinition = "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.") 768 protected List<ProcedureFocalDeviceComponent> focalDevice; 769 770 /** 771 * Identifies medications, devices and any other substance used as part of the 772 * procedure. 773 */ 774 @Child(name = "used", type = { Device.class, Medication.class, 775 Substance.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 776 @Description(shortDefinition = "Items used during procedure", formalDefinition = "Identifies medications, devices and any other substance used as part of the procedure.") 777 protected List<Reference> used; 778 /** 779 * The actual objects that are the target of the reference (Identifies 780 * medications, devices and any other substance used as part of the procedure.) 781 */ 782 protected List<Resource> usedTarget; 783 784 private static final long serialVersionUID = -489125036L; 785 786 /* 787 * Constructor 788 */ 789 public Procedure() { 790 super(); 791 } 792 793 /* 794 * Constructor 795 */ 796 public Procedure(Reference subject, Enumeration<ProcedureStatus> status, CodeableConcept code) { 797 super(); 798 this.subject = subject; 799 this.status = status; 800 this.code = code; 801 } 802 803 /** 804 * @return {@link #identifier} (This records identifiers associated with this 805 * procedure that are defined by business processes and/or used to refer 806 * to it when a direct URL reference to the resource itself is not 807 * appropriate (e.g. in CDA documents, or in written / printed 808 * documentation).) 809 */ 810 public List<Identifier> getIdentifier() { 811 if (this.identifier == null) 812 this.identifier = new ArrayList<Identifier>(); 813 return this.identifier; 814 } 815 816 public boolean hasIdentifier() { 817 if (this.identifier == null) 818 return false; 819 for (Identifier item : this.identifier) 820 if (!item.isEmpty()) 821 return true; 822 return false; 823 } 824 825 /** 826 * @return {@link #identifier} (This records identifiers associated with this 827 * procedure that are defined by business processes and/or used to refer 828 * to it when a direct URL reference to the resource itself is not 829 * appropriate (e.g. in CDA documents, or in written / printed 830 * documentation).) 831 */ 832 // syntactic sugar 833 public Identifier addIdentifier() { // 3 834 Identifier t = new Identifier(); 835 if (this.identifier == null) 836 this.identifier = new ArrayList<Identifier>(); 837 this.identifier.add(t); 838 return t; 839 } 840 841 // syntactic sugar 842 public Procedure addIdentifier(Identifier t) { // 3 843 if (t == null) 844 return this; 845 if (this.identifier == null) 846 this.identifier = new ArrayList<Identifier>(); 847 this.identifier.add(t); 848 return this; 849 } 850 851 /** 852 * @return {@link #subject} (The person, animal or group on which the procedure 853 * was performed.) 854 */ 855 public Reference getSubject() { 856 if (this.subject == null) 857 if (Configuration.errorOnAutoCreate()) 858 throw new Error("Attempt to auto-create Procedure.subject"); 859 else if (Configuration.doAutoCreate()) 860 this.subject = new Reference(); // cc 861 return this.subject; 862 } 863 864 public boolean hasSubject() { 865 return this.subject != null && !this.subject.isEmpty(); 866 } 867 868 /** 869 * @param value {@link #subject} (The person, animal or group on which the 870 * procedure was performed.) 871 */ 872 public Procedure setSubject(Reference value) { 873 this.subject = value; 874 return this; 875 } 876 877 /** 878 * @return {@link #subject} The actual object that is the target of the 879 * reference. The reference library doesn't populate this, but you can 880 * use it to hold the resource if you resolve it. (The person, animal or 881 * group on which the procedure was performed.) 882 */ 883 public Resource getSubjectTarget() { 884 return this.subjectTarget; 885 } 886 887 /** 888 * @param value {@link #subject} The actual object that is the target of the 889 * reference. The reference library doesn't use these, but you can 890 * use it to hold the resource if you resolve it. (The person, 891 * animal or group on which the procedure was performed.) 892 */ 893 public Procedure setSubjectTarget(Resource value) { 894 this.subjectTarget = value; 895 return this; 896 } 897 898 /** 899 * @return {@link #status} (A code specifying the state of the procedure. 900 * Generally this will be in-progress or completed state.). This is the 901 * underlying object with id, value and extensions. The accessor 902 * "getStatus" gives direct access to the value 903 */ 904 public Enumeration<ProcedureStatus> getStatusElement() { 905 if (this.status == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create Procedure.status"); 908 else if (Configuration.doAutoCreate()) 909 this.status = new Enumeration<ProcedureStatus>(new ProcedureStatusEnumFactory()); // bb 910 return this.status; 911 } 912 913 public boolean hasStatusElement() { 914 return this.status != null && !this.status.isEmpty(); 915 } 916 917 public boolean hasStatus() { 918 return this.status != null && !this.status.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #status} (A code specifying the state of the procedure. 923 * Generally this will be in-progress or completed state.). This is 924 * the underlying object with id, value and extensions. The 925 * accessor "getStatus" gives direct access to the value 926 */ 927 public Procedure setStatusElement(Enumeration<ProcedureStatus> value) { 928 this.status = value; 929 return this; 930 } 931 932 /** 933 * @return A code specifying the state of the procedure. Generally this will be 934 * in-progress or completed state. 935 */ 936 public ProcedureStatus getStatus() { 937 return this.status == null ? null : this.status.getValue(); 938 } 939 940 /** 941 * @param value A code specifying the state of the procedure. Generally this 942 * will be in-progress or completed state. 943 */ 944 public Procedure setStatus(ProcedureStatus value) { 945 if (this.status == null) 946 this.status = new Enumeration<ProcedureStatus>(new ProcedureStatusEnumFactory()); 947 this.status.setValue(value); 948 return this; 949 } 950 951 /** 952 * @return {@link #category} (A code that classifies the procedure for 953 * searching, sorting and display purposes (e.g. "Surgical Procedure").) 954 */ 955 public CodeableConcept getCategory() { 956 if (this.category == null) 957 if (Configuration.errorOnAutoCreate()) 958 throw new Error("Attempt to auto-create Procedure.category"); 959 else if (Configuration.doAutoCreate()) 960 this.category = new CodeableConcept(); // cc 961 return this.category; 962 } 963 964 public boolean hasCategory() { 965 return this.category != null && !this.category.isEmpty(); 966 } 967 968 /** 969 * @param value {@link #category} (A code that classifies the procedure for 970 * searching, sorting and display purposes (e.g. "Surgical 971 * Procedure").) 972 */ 973 public Procedure setCategory(CodeableConcept value) { 974 this.category = value; 975 return this; 976 } 977 978 /** 979 * @return {@link #code} (The specific procedure that is performed. Use text if 980 * the exact nature of the procedure cannot be coded (e.g. "Laparoscopic 981 * Appendectomy").) 982 */ 983 public CodeableConcept getCode() { 984 if (this.code == null) 985 if (Configuration.errorOnAutoCreate()) 986 throw new Error("Attempt to auto-create Procedure.code"); 987 else if (Configuration.doAutoCreate()) 988 this.code = new CodeableConcept(); // cc 989 return this.code; 990 } 991 992 public boolean hasCode() { 993 return this.code != null && !this.code.isEmpty(); 994 } 995 996 /** 997 * @param value {@link #code} (The specific procedure that is performed. Use 998 * text if the exact nature of the procedure cannot be coded (e.g. 999 * "Laparoscopic Appendectomy").) 1000 */ 1001 public Procedure setCode(CodeableConcept value) { 1002 this.code = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #notPerformed} (Set this to true if the record is saying that 1008 * the procedure was NOT performed.). This is the underlying object with 1009 * id, value and extensions. The accessor "getNotPerformed" gives direct 1010 * access to the value 1011 */ 1012 public BooleanType getNotPerformedElement() { 1013 if (this.notPerformed == null) 1014 if (Configuration.errorOnAutoCreate()) 1015 throw new Error("Attempt to auto-create Procedure.notPerformed"); 1016 else if (Configuration.doAutoCreate()) 1017 this.notPerformed = new BooleanType(); // bb 1018 return this.notPerformed; 1019 } 1020 1021 public boolean hasNotPerformedElement() { 1022 return this.notPerformed != null && !this.notPerformed.isEmpty(); 1023 } 1024 1025 public boolean hasNotPerformed() { 1026 return this.notPerformed != null && !this.notPerformed.isEmpty(); 1027 } 1028 1029 /** 1030 * @param value {@link #notPerformed} (Set this to true if the record is saying 1031 * that the procedure was NOT performed.). This is the underlying 1032 * object with id, value and extensions. The accessor 1033 * "getNotPerformed" gives direct access to the value 1034 */ 1035 public Procedure setNotPerformedElement(BooleanType value) { 1036 this.notPerformed = value; 1037 return this; 1038 } 1039 1040 /** 1041 * @return Set this to true if the record is saying that the procedure was NOT 1042 * performed. 1043 */ 1044 public boolean getNotPerformed() { 1045 return this.notPerformed == null || this.notPerformed.isEmpty() ? false : this.notPerformed.getValue(); 1046 } 1047 1048 /** 1049 * @param value Set this to true if the record is saying that the procedure was 1050 * NOT performed. 1051 */ 1052 public Procedure setNotPerformed(boolean value) { 1053 if (this.notPerformed == null) 1054 this.notPerformed = new BooleanType(); 1055 this.notPerformed.setValue(value); 1056 return this; 1057 } 1058 1059 /** 1060 * @return {@link #reasonNotPerformed} (A code indicating why the procedure was 1061 * not performed.) 1062 */ 1063 public List<CodeableConcept> getReasonNotPerformed() { 1064 if (this.reasonNotPerformed == null) 1065 this.reasonNotPerformed = new ArrayList<CodeableConcept>(); 1066 return this.reasonNotPerformed; 1067 } 1068 1069 public boolean hasReasonNotPerformed() { 1070 if (this.reasonNotPerformed == null) 1071 return false; 1072 for (CodeableConcept item : this.reasonNotPerformed) 1073 if (!item.isEmpty()) 1074 return true; 1075 return false; 1076 } 1077 1078 /** 1079 * @return {@link #reasonNotPerformed} (A code indicating why the procedure was 1080 * not performed.) 1081 */ 1082 // syntactic sugar 1083 public CodeableConcept addReasonNotPerformed() { // 3 1084 CodeableConcept t = new CodeableConcept(); 1085 if (this.reasonNotPerformed == null) 1086 this.reasonNotPerformed = new ArrayList<CodeableConcept>(); 1087 this.reasonNotPerformed.add(t); 1088 return t; 1089 } 1090 1091 // syntactic sugar 1092 public Procedure addReasonNotPerformed(CodeableConcept t) { // 3 1093 if (t == null) 1094 return this; 1095 if (this.reasonNotPerformed == null) 1096 this.reasonNotPerformed = new ArrayList<CodeableConcept>(); 1097 this.reasonNotPerformed.add(t); 1098 return this; 1099 } 1100 1101 /** 1102 * @return {@link #bodySite} (Detailed and structured anatomical location 1103 * information. Multiple locations are allowed - e.g. multiple punch 1104 * biopsies of a lesion.) 1105 */ 1106 public List<CodeableConcept> getBodySite() { 1107 if (this.bodySite == null) 1108 this.bodySite = new ArrayList<CodeableConcept>(); 1109 return this.bodySite; 1110 } 1111 1112 public boolean hasBodySite() { 1113 if (this.bodySite == null) 1114 return false; 1115 for (CodeableConcept item : this.bodySite) 1116 if (!item.isEmpty()) 1117 return true; 1118 return false; 1119 } 1120 1121 /** 1122 * @return {@link #bodySite} (Detailed and structured anatomical location 1123 * information. Multiple locations are allowed - e.g. multiple punch 1124 * biopsies of a lesion.) 1125 */ 1126 // syntactic sugar 1127 public CodeableConcept addBodySite() { // 3 1128 CodeableConcept t = new CodeableConcept(); 1129 if (this.bodySite == null) 1130 this.bodySite = new ArrayList<CodeableConcept>(); 1131 this.bodySite.add(t); 1132 return t; 1133 } 1134 1135 // syntactic sugar 1136 public Procedure addBodySite(CodeableConcept t) { // 3 1137 if (t == null) 1138 return this; 1139 if (this.bodySite == null) 1140 this.bodySite = new ArrayList<CodeableConcept>(); 1141 this.bodySite.add(t); 1142 return this; 1143 } 1144 1145 /** 1146 * @return {@link #reason} (The reason why the procedure was performed. This may 1147 * be due to a Condition, may be coded entity of some type, or may 1148 * simply be present as text.) 1149 */ 1150 public Type getReason() { 1151 return this.reason; 1152 } 1153 1154 /** 1155 * @return {@link #reason} (The reason why the procedure was performed. This may 1156 * be due to a Condition, may be coded entity of some type, or may 1157 * simply be present as text.) 1158 */ 1159 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 1160 if (!(this.reason instanceof CodeableConcept)) 1161 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1162 + this.reason.getClass().getName() + " was encountered"); 1163 return (CodeableConcept) this.reason; 1164 } 1165 1166 public boolean hasReasonCodeableConcept() { 1167 return this.reason instanceof CodeableConcept; 1168 } 1169 1170 /** 1171 * @return {@link #reason} (The reason why the procedure was performed. This may 1172 * be due to a Condition, may be coded entity of some type, or may 1173 * simply be present as text.) 1174 */ 1175 public Reference getReasonReference() throws FHIRException { 1176 if (!(this.reason instanceof Reference)) 1177 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.reason.getClass().getName() 1178 + " was encountered"); 1179 return (Reference) this.reason; 1180 } 1181 1182 public boolean hasReasonReference() { 1183 return this.reason instanceof Reference; 1184 } 1185 1186 public boolean hasReason() { 1187 return this.reason != null && !this.reason.isEmpty(); 1188 } 1189 1190 /** 1191 * @param value {@link #reason} (The reason why the procedure was performed. 1192 * This may be due to a Condition, may be coded entity of some 1193 * type, or may simply be present as text.) 1194 */ 1195 public Procedure setReason(Type value) { 1196 this.reason = value; 1197 return this; 1198 } 1199 1200 /** 1201 * @return {@link #performer} (Limited to 'real' people rather than equipment.) 1202 */ 1203 public List<ProcedurePerformerComponent> getPerformer() { 1204 if (this.performer == null) 1205 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1206 return this.performer; 1207 } 1208 1209 public boolean hasPerformer() { 1210 if (this.performer == null) 1211 return false; 1212 for (ProcedurePerformerComponent item : this.performer) 1213 if (!item.isEmpty()) 1214 return true; 1215 return false; 1216 } 1217 1218 /** 1219 * @return {@link #performer} (Limited to 'real' people rather than equipment.) 1220 */ 1221 // syntactic sugar 1222 public ProcedurePerformerComponent addPerformer() { // 3 1223 ProcedurePerformerComponent t = new ProcedurePerformerComponent(); 1224 if (this.performer == null) 1225 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1226 this.performer.add(t); 1227 return t; 1228 } 1229 1230 // syntactic sugar 1231 public Procedure addPerformer(ProcedurePerformerComponent t) { // 3 1232 if (t == null) 1233 return this; 1234 if (this.performer == null) 1235 this.performer = new ArrayList<ProcedurePerformerComponent>(); 1236 this.performer.add(t); 1237 return this; 1238 } 1239 1240 /** 1241 * @return {@link #performed} (The date(time)/period over which the procedure 1242 * was performed. Allows a period to support complex procedures that 1243 * span more than one date, and also allows for the length of the 1244 * procedure to be captured.) 1245 */ 1246 public Type getPerformed() { 1247 return this.performed; 1248 } 1249 1250 /** 1251 * @return {@link #performed} (The date(time)/period over which the procedure 1252 * was performed. Allows a period to support complex procedures that 1253 * span more than one date, and also allows for the length of the 1254 * procedure to be captured.) 1255 */ 1256 public DateTimeType getPerformedDateTimeType() throws FHIRException { 1257 if (!(this.performed instanceof DateTimeType)) 1258 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1259 + this.performed.getClass().getName() + " was encountered"); 1260 return (DateTimeType) this.performed; 1261 } 1262 1263 public boolean hasPerformedDateTimeType() { 1264 return this.performed instanceof DateTimeType; 1265 } 1266 1267 /** 1268 * @return {@link #performed} (The date(time)/period over which the procedure 1269 * was performed. Allows a period to support complex procedures that 1270 * span more than one date, and also allows for the length of the 1271 * procedure to be captured.) 1272 */ 1273 public Period getPerformedPeriod() throws FHIRException { 1274 if (!(this.performed instanceof Period)) 1275 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.performed.getClass().getName() 1276 + " was encountered"); 1277 return (Period) this.performed; 1278 } 1279 1280 public boolean hasPerformedPeriod() { 1281 return this.performed instanceof Period; 1282 } 1283 1284 public boolean hasPerformed() { 1285 return this.performed != null && !this.performed.isEmpty(); 1286 } 1287 1288 /** 1289 * @param value {@link #performed} (The date(time)/period over which the 1290 * procedure was performed. Allows a period to support complex 1291 * procedures that span more than one date, and also allows for the 1292 * length of the procedure to be captured.) 1293 */ 1294 public Procedure setPerformed(Type value) { 1295 this.performed = value; 1296 return this; 1297 } 1298 1299 /** 1300 * @return {@link #encounter} (The encounter during which the procedure was 1301 * performed.) 1302 */ 1303 public Reference getEncounter() { 1304 if (this.encounter == null) 1305 if (Configuration.errorOnAutoCreate()) 1306 throw new Error("Attempt to auto-create Procedure.encounter"); 1307 else if (Configuration.doAutoCreate()) 1308 this.encounter = new Reference(); // cc 1309 return this.encounter; 1310 } 1311 1312 public boolean hasEncounter() { 1313 return this.encounter != null && !this.encounter.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #encounter} (The encounter during which the procedure was 1318 * performed.) 1319 */ 1320 public Procedure setEncounter(Reference value) { 1321 this.encounter = value; 1322 return this; 1323 } 1324 1325 /** 1326 * @return {@link #encounter} The actual object that is the target of the 1327 * reference. The reference library doesn't populate this, but you can 1328 * use it to hold the resource if you resolve it. (The encounter during 1329 * which the procedure was performed.) 1330 */ 1331 public Encounter getEncounterTarget() { 1332 if (this.encounterTarget == null) 1333 if (Configuration.errorOnAutoCreate()) 1334 throw new Error("Attempt to auto-create Procedure.encounter"); 1335 else if (Configuration.doAutoCreate()) 1336 this.encounterTarget = new Encounter(); // aa 1337 return this.encounterTarget; 1338 } 1339 1340 /** 1341 * @param value {@link #encounter} The actual object that is the target of the 1342 * reference. The reference library doesn't use these, but you can 1343 * use it to hold the resource if you resolve it. (The encounter 1344 * during which the procedure was performed.) 1345 */ 1346 public Procedure setEncounterTarget(Encounter value) { 1347 this.encounterTarget = value; 1348 return this; 1349 } 1350 1351 /** 1352 * @return {@link #location} (The location where the procedure actually 1353 * happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 1354 */ 1355 public Reference getLocation() { 1356 if (this.location == null) 1357 if (Configuration.errorOnAutoCreate()) 1358 throw new Error("Attempt to auto-create Procedure.location"); 1359 else if (Configuration.doAutoCreate()) 1360 this.location = new Reference(); // cc 1361 return this.location; 1362 } 1363 1364 public boolean hasLocation() { 1365 return this.location != null && !this.location.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #location} (The location where the procedure actually 1370 * happened. E.g. a newborn at home, a tracheostomy at a 1371 * restaurant.) 1372 */ 1373 public Procedure setLocation(Reference value) { 1374 this.location = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #location} The actual object that is the target of the 1380 * reference. The reference library doesn't populate this, but you can 1381 * use it to hold the resource if you resolve it. (The location where 1382 * the procedure actually happened. E.g. a newborn at home, a 1383 * tracheostomy at a restaurant.) 1384 */ 1385 public Location getLocationTarget() { 1386 if (this.locationTarget == null) 1387 if (Configuration.errorOnAutoCreate()) 1388 throw new Error("Attempt to auto-create Procedure.location"); 1389 else if (Configuration.doAutoCreate()) 1390 this.locationTarget = new Location(); // aa 1391 return this.locationTarget; 1392 } 1393 1394 /** 1395 * @param value {@link #location} The actual object that is the target of the 1396 * reference. The reference library doesn't use these, but you can 1397 * use it to hold the resource if you resolve it. (The location 1398 * where the procedure actually happened. E.g. a newborn at home, a 1399 * tracheostomy at a restaurant.) 1400 */ 1401 public Procedure setLocationTarget(Location value) { 1402 this.locationTarget = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #outcome} (The outcome of the procedure - did it resolve 1408 * reasons for the procedure being performed?) 1409 */ 1410 public CodeableConcept getOutcome() { 1411 if (this.outcome == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create Procedure.outcome"); 1414 else if (Configuration.doAutoCreate()) 1415 this.outcome = new CodeableConcept(); // cc 1416 return this.outcome; 1417 } 1418 1419 public boolean hasOutcome() { 1420 return this.outcome != null && !this.outcome.isEmpty(); 1421 } 1422 1423 /** 1424 * @param value {@link #outcome} (The outcome of the procedure - did it resolve 1425 * reasons for the procedure being performed?) 1426 */ 1427 public Procedure setOutcome(CodeableConcept value) { 1428 this.outcome = value; 1429 return this; 1430 } 1431 1432 /** 1433 * @return {@link #report} (This could be a histology result, pathology report, 1434 * surgical report, etc..) 1435 */ 1436 public List<Reference> getReport() { 1437 if (this.report == null) 1438 this.report = new ArrayList<Reference>(); 1439 return this.report; 1440 } 1441 1442 public boolean hasReport() { 1443 if (this.report == null) 1444 return false; 1445 for (Reference item : this.report) 1446 if (!item.isEmpty()) 1447 return true; 1448 return false; 1449 } 1450 1451 /** 1452 * @return {@link #report} (This could be a histology result, pathology report, 1453 * surgical report, etc..) 1454 */ 1455 // syntactic sugar 1456 public Reference addReport() { // 3 1457 Reference t = new Reference(); 1458 if (this.report == null) 1459 this.report = new ArrayList<Reference>(); 1460 this.report.add(t); 1461 return t; 1462 } 1463 1464 // syntactic sugar 1465 public Procedure addReport(Reference t) { // 3 1466 if (t == null) 1467 return this; 1468 if (this.report == null) 1469 this.report = new ArrayList<Reference>(); 1470 this.report.add(t); 1471 return this; 1472 } 1473 1474 /** 1475 * @return {@link #report} (The actual objects that are the target of the 1476 * reference. The reference library doesn't populate this, but you can 1477 * use this to hold the resources if you resolvethemt. This could be a 1478 * histology result, pathology report, surgical report, etc..) 1479 */ 1480 public List<DiagnosticReport> getReportTarget() { 1481 if (this.reportTarget == null) 1482 this.reportTarget = new ArrayList<DiagnosticReport>(); 1483 return this.reportTarget; 1484 } 1485 1486 // syntactic sugar 1487 /** 1488 * @return {@link #report} (Add an actual object that is the target of the 1489 * reference. The reference library doesn't use these, but you can use 1490 * this to hold the resources if you resolvethemt. This could be a 1491 * histology result, pathology report, surgical report, etc..) 1492 */ 1493 public DiagnosticReport addReportTarget() { 1494 DiagnosticReport r = new DiagnosticReport(); 1495 if (this.reportTarget == null) 1496 this.reportTarget = new ArrayList<DiagnosticReport>(); 1497 this.reportTarget.add(r); 1498 return r; 1499 } 1500 1501 /** 1502 * @return {@link #complication} (Any complications that occurred during the 1503 * procedure, or in the immediate post-performance period. These are 1504 * generally tracked separately from the notes, which will typically 1505 * describe the procedure itself rather than any 'post procedure' 1506 * issues.) 1507 */ 1508 public List<CodeableConcept> getComplication() { 1509 if (this.complication == null) 1510 this.complication = new ArrayList<CodeableConcept>(); 1511 return this.complication; 1512 } 1513 1514 public boolean hasComplication() { 1515 if (this.complication == null) 1516 return false; 1517 for (CodeableConcept item : this.complication) 1518 if (!item.isEmpty()) 1519 return true; 1520 return false; 1521 } 1522 1523 /** 1524 * @return {@link #complication} (Any complications that occurred during the 1525 * procedure, or in the immediate post-performance period. These are 1526 * generally tracked separately from the notes, which will typically 1527 * describe the procedure itself rather than any 'post procedure' 1528 * issues.) 1529 */ 1530 // syntactic sugar 1531 public CodeableConcept addComplication() { // 3 1532 CodeableConcept t = new CodeableConcept(); 1533 if (this.complication == null) 1534 this.complication = new ArrayList<CodeableConcept>(); 1535 this.complication.add(t); 1536 return t; 1537 } 1538 1539 // syntactic sugar 1540 public Procedure addComplication(CodeableConcept t) { // 3 1541 if (t == null) 1542 return this; 1543 if (this.complication == null) 1544 this.complication = new ArrayList<CodeableConcept>(); 1545 this.complication.add(t); 1546 return this; 1547 } 1548 1549 /** 1550 * @return {@link #followUp} (If the procedure required specific follow up - 1551 * e.g. removal of sutures. The followup may be represented as a simple 1552 * note, or could potentially be more complex in which case the CarePlan 1553 * resource can be used.) 1554 */ 1555 public List<CodeableConcept> getFollowUp() { 1556 if (this.followUp == null) 1557 this.followUp = new ArrayList<CodeableConcept>(); 1558 return this.followUp; 1559 } 1560 1561 public boolean hasFollowUp() { 1562 if (this.followUp == null) 1563 return false; 1564 for (CodeableConcept item : this.followUp) 1565 if (!item.isEmpty()) 1566 return true; 1567 return false; 1568 } 1569 1570 /** 1571 * @return {@link #followUp} (If the procedure required specific follow up - 1572 * e.g. removal of sutures. The followup may be represented as a simple 1573 * note, or could potentially be more complex in which case the CarePlan 1574 * resource can be used.) 1575 */ 1576 // syntactic sugar 1577 public CodeableConcept addFollowUp() { // 3 1578 CodeableConcept t = new CodeableConcept(); 1579 if (this.followUp == null) 1580 this.followUp = new ArrayList<CodeableConcept>(); 1581 this.followUp.add(t); 1582 return t; 1583 } 1584 1585 // syntactic sugar 1586 public Procedure addFollowUp(CodeableConcept t) { // 3 1587 if (t == null) 1588 return this; 1589 if (this.followUp == null) 1590 this.followUp = new ArrayList<CodeableConcept>(); 1591 this.followUp.add(t); 1592 return this; 1593 } 1594 1595 /** 1596 * @return {@link #request} (A reference to a resource that contains details of 1597 * the request for this procedure.) 1598 */ 1599 public Reference getRequest() { 1600 if (this.request == null) 1601 if (Configuration.errorOnAutoCreate()) 1602 throw new Error("Attempt to auto-create Procedure.request"); 1603 else if (Configuration.doAutoCreate()) 1604 this.request = new Reference(); // cc 1605 return this.request; 1606 } 1607 1608 public boolean hasRequest() { 1609 return this.request != null && !this.request.isEmpty(); 1610 } 1611 1612 /** 1613 * @param value {@link #request} (A reference to a resource that contains 1614 * details of the request for this procedure.) 1615 */ 1616 public Procedure setRequest(Reference value) { 1617 this.request = value; 1618 return this; 1619 } 1620 1621 /** 1622 * @return {@link #request} The actual object that is the target of the 1623 * reference. The reference library doesn't populate this, but you can 1624 * use it to hold the resource if you resolve it. (A reference to a 1625 * resource that contains details of the request for this procedure.) 1626 */ 1627 public Resource getRequestTarget() { 1628 return this.requestTarget; 1629 } 1630 1631 /** 1632 * @param value {@link #request} The actual object that is the target of the 1633 * reference. The reference library doesn't use these, but you can 1634 * use it to hold the resource if you resolve it. (A reference to a 1635 * resource that contains details of the request for this 1636 * procedure.) 1637 */ 1638 public Procedure setRequestTarget(Resource value) { 1639 this.requestTarget = value; 1640 return this; 1641 } 1642 1643 /** 1644 * @return {@link #notes} (Any other notes about the procedure. E.g. the 1645 * operative notes.) 1646 */ 1647 public List<Annotation> getNotes() { 1648 if (this.notes == null) 1649 this.notes = new ArrayList<Annotation>(); 1650 return this.notes; 1651 } 1652 1653 public boolean hasNotes() { 1654 if (this.notes == null) 1655 return false; 1656 for (Annotation item : this.notes) 1657 if (!item.isEmpty()) 1658 return true; 1659 return false; 1660 } 1661 1662 /** 1663 * @return {@link #notes} (Any other notes about the procedure. E.g. the 1664 * operative notes.) 1665 */ 1666 // syntactic sugar 1667 public Annotation addNotes() { // 3 1668 Annotation t = new Annotation(); 1669 if (this.notes == null) 1670 this.notes = new ArrayList<Annotation>(); 1671 this.notes.add(t); 1672 return t; 1673 } 1674 1675 // syntactic sugar 1676 public Procedure addNotes(Annotation t) { // 3 1677 if (t == null) 1678 return this; 1679 if (this.notes == null) 1680 this.notes = new ArrayList<Annotation>(); 1681 this.notes.add(t); 1682 return this; 1683 } 1684 1685 /** 1686 * @return {@link #focalDevice} (A device that is implanted, removed or 1687 * otherwise manipulated (calibration, battery replacement, fitting a 1688 * prosthesis, attaching a wound-vac, etc.) as a focal portion of the 1689 * Procedure.) 1690 */ 1691 public List<ProcedureFocalDeviceComponent> getFocalDevice() { 1692 if (this.focalDevice == null) 1693 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 1694 return this.focalDevice; 1695 } 1696 1697 public boolean hasFocalDevice() { 1698 if (this.focalDevice == null) 1699 return false; 1700 for (ProcedureFocalDeviceComponent item : this.focalDevice) 1701 if (!item.isEmpty()) 1702 return true; 1703 return false; 1704 } 1705 1706 /** 1707 * @return {@link #focalDevice} (A device that is implanted, removed or 1708 * otherwise manipulated (calibration, battery replacement, fitting a 1709 * prosthesis, attaching a wound-vac, etc.) as a focal portion of the 1710 * Procedure.) 1711 */ 1712 // syntactic sugar 1713 public ProcedureFocalDeviceComponent addFocalDevice() { // 3 1714 ProcedureFocalDeviceComponent t = new ProcedureFocalDeviceComponent(); 1715 if (this.focalDevice == null) 1716 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 1717 this.focalDevice.add(t); 1718 return t; 1719 } 1720 1721 // syntactic sugar 1722 public Procedure addFocalDevice(ProcedureFocalDeviceComponent t) { // 3 1723 if (t == null) 1724 return this; 1725 if (this.focalDevice == null) 1726 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 1727 this.focalDevice.add(t); 1728 return this; 1729 } 1730 1731 /** 1732 * @return {@link #used} (Identifies medications, devices and any other 1733 * substance used as part of the procedure.) 1734 */ 1735 public List<Reference> getUsed() { 1736 if (this.used == null) 1737 this.used = new ArrayList<Reference>(); 1738 return this.used; 1739 } 1740 1741 public boolean hasUsed() { 1742 if (this.used == null) 1743 return false; 1744 for (Reference item : this.used) 1745 if (!item.isEmpty()) 1746 return true; 1747 return false; 1748 } 1749 1750 /** 1751 * @return {@link #used} (Identifies medications, devices and any other 1752 * substance used as part of the procedure.) 1753 */ 1754 // syntactic sugar 1755 public Reference addUsed() { // 3 1756 Reference t = new Reference(); 1757 if (this.used == null) 1758 this.used = new ArrayList<Reference>(); 1759 this.used.add(t); 1760 return t; 1761 } 1762 1763 // syntactic sugar 1764 public Procedure addUsed(Reference t) { // 3 1765 if (t == null) 1766 return this; 1767 if (this.used == null) 1768 this.used = new ArrayList<Reference>(); 1769 this.used.add(t); 1770 return this; 1771 } 1772 1773 /** 1774 * @return {@link #used} (The actual objects that are the target of the 1775 * reference. The reference library doesn't populate this, but you can 1776 * use this to hold the resources if you resolvethemt. Identifies 1777 * medications, devices and any other substance used as part of the 1778 * procedure.) 1779 */ 1780 public List<Resource> getUsedTarget() { 1781 if (this.usedTarget == null) 1782 this.usedTarget = new ArrayList<Resource>(); 1783 return this.usedTarget; 1784 } 1785 1786 protected void listChildren(List<Property> childrenList) { 1787 super.listChildren(childrenList); 1788 childrenList.add(new Property("identifier", "Identifier", 1789 "This records identifiers associated with this procedure that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 1790 0, java.lang.Integer.MAX_VALUE, identifier)); 1791 childrenList.add(new Property("subject", "Reference(Patient|Group)", 1792 "The person, animal or group on which the procedure was performed.", 0, java.lang.Integer.MAX_VALUE, subject)); 1793 childrenList.add(new Property("status", "code", 1794 "A code specifying the state of the procedure. Generally this will be in-progress or completed state.", 0, 1795 java.lang.Integer.MAX_VALUE, status)); 1796 childrenList.add(new Property("category", "CodeableConcept", 1797 "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 1798 0, java.lang.Integer.MAX_VALUE, category)); 1799 childrenList.add(new Property("code", "CodeableConcept", 1800 "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").", 1801 0, java.lang.Integer.MAX_VALUE, code)); 1802 childrenList.add(new Property("notPerformed", "boolean", 1803 "Set this to true if the record is saying that the procedure was NOT performed.", 0, 1804 java.lang.Integer.MAX_VALUE, notPerformed)); 1805 childrenList.add(new Property("reasonNotPerformed", "CodeableConcept", 1806 "A code indicating why the procedure was not performed.", 0, java.lang.Integer.MAX_VALUE, reasonNotPerformed)); 1807 childrenList.add(new Property("bodySite", "CodeableConcept", 1808 "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.", 1809 0, java.lang.Integer.MAX_VALUE, bodySite)); 1810 childrenList.add(new Property("reason[x]", "CodeableConcept|Reference(Condition)", 1811 "The reason why the procedure was performed. This may be due to a Condition, may be coded entity of some type, or may simply be present as text.", 1812 0, java.lang.Integer.MAX_VALUE, reason)); 1813 childrenList.add(new Property("performer", "", "Limited to 'real' people rather than equipment.", 0, 1814 java.lang.Integer.MAX_VALUE, performer)); 1815 childrenList.add(new Property("performed[x]", "dateTime|Period", 1816 "The date(time)/period over which the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 1817 0, java.lang.Integer.MAX_VALUE, performed)); 1818 childrenList.add(new Property("encounter", "Reference(Encounter)", 1819 "The encounter during which the procedure was performed.", 0, java.lang.Integer.MAX_VALUE, encounter)); 1820 childrenList.add(new Property("location", "Reference(Location)", 1821 "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.", 1822 0, java.lang.Integer.MAX_VALUE, location)); 1823 childrenList.add(new Property("outcome", "CodeableConcept", 1824 "The outcome of the procedure - did it resolve reasons for the procedure being performed?", 0, 1825 java.lang.Integer.MAX_VALUE, outcome)); 1826 childrenList.add(new Property("report", "Reference(DiagnosticReport)", 1827 "This could be a histology result, pathology report, surgical report, etc..", 0, java.lang.Integer.MAX_VALUE, 1828 report)); 1829 childrenList.add(new Property("complication", "CodeableConcept", 1830 "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.", 1831 0, java.lang.Integer.MAX_VALUE, complication)); 1832 childrenList.add(new Property("followUp", "CodeableConcept", 1833 "If the procedure required specific follow up - e.g. removal of sutures. The followup may be represented as a simple note, or could potentially be more complex in which case the CarePlan resource can be used.", 1834 0, java.lang.Integer.MAX_VALUE, followUp)); 1835 childrenList.add(new Property("request", "Reference(CarePlan|DiagnosticOrder|ProcedureRequest|ReferralRequest)", 1836 "A reference to a resource that contains details of the request for this procedure.", 0, 1837 java.lang.Integer.MAX_VALUE, request)); 1838 childrenList.add(new Property("notes", "Annotation", 1839 "Any other notes about the procedure. E.g. the operative notes.", 0, java.lang.Integer.MAX_VALUE, notes)); 1840 childrenList.add(new Property("focalDevice", "", 1841 "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.", 1842 0, java.lang.Integer.MAX_VALUE, focalDevice)); 1843 childrenList.add(new Property("used", "Reference(Device|Medication|Substance)", 1844 "Identifies medications, devices and any other substance used as part of the procedure.", 0, 1845 java.lang.Integer.MAX_VALUE, used)); 1846 } 1847 1848 @Override 1849 public void setProperty(String name, Base value) throws FHIRException { 1850 if (name.equals("identifier")) 1851 this.getIdentifier().add(castToIdentifier(value)); 1852 else if (name.equals("subject")) 1853 this.subject = castToReference(value); // Reference 1854 else if (name.equals("status")) 1855 this.status = new ProcedureStatusEnumFactory().fromType(value); // Enumeration<ProcedureStatus> 1856 else if (name.equals("category")) 1857 this.category = castToCodeableConcept(value); // CodeableConcept 1858 else if (name.equals("code")) 1859 this.code = castToCodeableConcept(value); // CodeableConcept 1860 else if (name.equals("notPerformed")) 1861 this.notPerformed = castToBoolean(value); // BooleanType 1862 else if (name.equals("reasonNotPerformed")) 1863 this.getReasonNotPerformed().add(castToCodeableConcept(value)); 1864 else if (name.equals("bodySite")) 1865 this.getBodySite().add(castToCodeableConcept(value)); 1866 else if (name.equals("reason[x]")) 1867 this.reason = (Type) value; // Type 1868 else if (name.equals("performer")) 1869 this.getPerformer().add((ProcedurePerformerComponent) value); 1870 else if (name.equals("performed[x]")) 1871 this.performed = (Type) value; // Type 1872 else if (name.equals("encounter")) 1873 this.encounter = castToReference(value); // Reference 1874 else if (name.equals("location")) 1875 this.location = castToReference(value); // Reference 1876 else if (name.equals("outcome")) 1877 this.outcome = castToCodeableConcept(value); // CodeableConcept 1878 else if (name.equals("report")) 1879 this.getReport().add(castToReference(value)); 1880 else if (name.equals("complication")) 1881 this.getComplication().add(castToCodeableConcept(value)); 1882 else if (name.equals("followUp")) 1883 this.getFollowUp().add(castToCodeableConcept(value)); 1884 else if (name.equals("request")) 1885 this.request = castToReference(value); // Reference 1886 else if (name.equals("notes")) 1887 this.getNotes().add(castToAnnotation(value)); 1888 else if (name.equals("focalDevice")) 1889 this.getFocalDevice().add((ProcedureFocalDeviceComponent) value); 1890 else if (name.equals("used")) 1891 this.getUsed().add(castToReference(value)); 1892 else 1893 super.setProperty(name, value); 1894 } 1895 1896 @Override 1897 public Base addChild(String name) throws FHIRException { 1898 if (name.equals("identifier")) { 1899 return addIdentifier(); 1900 } else if (name.equals("subject")) { 1901 this.subject = new Reference(); 1902 return this.subject; 1903 } else if (name.equals("status")) { 1904 throw new FHIRException("Cannot call addChild on a singleton property Procedure.status"); 1905 } else if (name.equals("category")) { 1906 this.category = new CodeableConcept(); 1907 return this.category; 1908 } else if (name.equals("code")) { 1909 this.code = new CodeableConcept(); 1910 return this.code; 1911 } else if (name.equals("notPerformed")) { 1912 throw new FHIRException("Cannot call addChild on a singleton property Procedure.notPerformed"); 1913 } else if (name.equals("reasonNotPerformed")) { 1914 return addReasonNotPerformed(); 1915 } else if (name.equals("bodySite")) { 1916 return addBodySite(); 1917 } else if (name.equals("reasonCodeableConcept")) { 1918 this.reason = new CodeableConcept(); 1919 return this.reason; 1920 } else if (name.equals("reasonReference")) { 1921 this.reason = new Reference(); 1922 return this.reason; 1923 } else if (name.equals("performer")) { 1924 return addPerformer(); 1925 } else if (name.equals("performedDateTime")) { 1926 this.performed = new DateTimeType(); 1927 return this.performed; 1928 } else if (name.equals("performedPeriod")) { 1929 this.performed = new Period(); 1930 return this.performed; 1931 } else if (name.equals("encounter")) { 1932 this.encounter = new Reference(); 1933 return this.encounter; 1934 } else if (name.equals("location")) { 1935 this.location = new Reference(); 1936 return this.location; 1937 } else if (name.equals("outcome")) { 1938 this.outcome = new CodeableConcept(); 1939 return this.outcome; 1940 } else if (name.equals("report")) { 1941 return addReport(); 1942 } else if (name.equals("complication")) { 1943 return addComplication(); 1944 } else if (name.equals("followUp")) { 1945 return addFollowUp(); 1946 } else if (name.equals("request")) { 1947 this.request = new Reference(); 1948 return this.request; 1949 } else if (name.equals("notes")) { 1950 return addNotes(); 1951 } else if (name.equals("focalDevice")) { 1952 return addFocalDevice(); 1953 } else if (name.equals("used")) { 1954 return addUsed(); 1955 } else 1956 return super.addChild(name); 1957 } 1958 1959 public String fhirType() { 1960 return "Procedure"; 1961 1962 } 1963 1964 public Procedure copy() { 1965 Procedure dst = new Procedure(); 1966 copyValues(dst); 1967 if (identifier != null) { 1968 dst.identifier = new ArrayList<Identifier>(); 1969 for (Identifier i : identifier) 1970 dst.identifier.add(i.copy()); 1971 } 1972 ; 1973 dst.subject = subject == null ? null : subject.copy(); 1974 dst.status = status == null ? null : status.copy(); 1975 dst.category = category == null ? null : category.copy(); 1976 dst.code = code == null ? null : code.copy(); 1977 dst.notPerformed = notPerformed == null ? null : notPerformed.copy(); 1978 if (reasonNotPerformed != null) { 1979 dst.reasonNotPerformed = new ArrayList<CodeableConcept>(); 1980 for (CodeableConcept i : reasonNotPerformed) 1981 dst.reasonNotPerformed.add(i.copy()); 1982 } 1983 ; 1984 if (bodySite != null) { 1985 dst.bodySite = new ArrayList<CodeableConcept>(); 1986 for (CodeableConcept i : bodySite) 1987 dst.bodySite.add(i.copy()); 1988 } 1989 ; 1990 dst.reason = reason == null ? null : reason.copy(); 1991 if (performer != null) { 1992 dst.performer = new ArrayList<ProcedurePerformerComponent>(); 1993 for (ProcedurePerformerComponent i : performer) 1994 dst.performer.add(i.copy()); 1995 } 1996 ; 1997 dst.performed = performed == null ? null : performed.copy(); 1998 dst.encounter = encounter == null ? null : encounter.copy(); 1999 dst.location = location == null ? null : location.copy(); 2000 dst.outcome = outcome == null ? null : outcome.copy(); 2001 if (report != null) { 2002 dst.report = new ArrayList<Reference>(); 2003 for (Reference i : report) 2004 dst.report.add(i.copy()); 2005 } 2006 ; 2007 if (complication != null) { 2008 dst.complication = new ArrayList<CodeableConcept>(); 2009 for (CodeableConcept i : complication) 2010 dst.complication.add(i.copy()); 2011 } 2012 ; 2013 if (followUp != null) { 2014 dst.followUp = new ArrayList<CodeableConcept>(); 2015 for (CodeableConcept i : followUp) 2016 dst.followUp.add(i.copy()); 2017 } 2018 ; 2019 dst.request = request == null ? null : request.copy(); 2020 if (notes != null) { 2021 dst.notes = new ArrayList<Annotation>(); 2022 for (Annotation i : notes) 2023 dst.notes.add(i.copy()); 2024 } 2025 ; 2026 if (focalDevice != null) { 2027 dst.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2028 for (ProcedureFocalDeviceComponent i : focalDevice) 2029 dst.focalDevice.add(i.copy()); 2030 } 2031 ; 2032 if (used != null) { 2033 dst.used = new ArrayList<Reference>(); 2034 for (Reference i : used) 2035 dst.used.add(i.copy()); 2036 } 2037 ; 2038 return dst; 2039 } 2040 2041 protected Procedure typedCopy() { 2042 return copy(); 2043 } 2044 2045 @Override 2046 public boolean equalsDeep(Base other) { 2047 if (!super.equalsDeep(other)) 2048 return false; 2049 if (!(other instanceof Procedure)) 2050 return false; 2051 Procedure o = (Procedure) other; 2052 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) 2053 && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 2054 && compareDeep(code, o.code, true) && compareDeep(notPerformed, o.notPerformed, true) 2055 && compareDeep(reasonNotPerformed, o.reasonNotPerformed, true) && compareDeep(bodySite, o.bodySite, true) 2056 && compareDeep(reason, o.reason, true) && compareDeep(performer, o.performer, true) 2057 && compareDeep(performed, o.performed, true) && compareDeep(encounter, o.encounter, true) 2058 && compareDeep(location, o.location, true) && compareDeep(outcome, o.outcome, true) 2059 && compareDeep(report, o.report, true) && compareDeep(complication, o.complication, true) 2060 && compareDeep(followUp, o.followUp, true) && compareDeep(request, o.request, true) 2061 && compareDeep(notes, o.notes, true) && compareDeep(focalDevice, o.focalDevice, true) 2062 && compareDeep(used, o.used, true); 2063 } 2064 2065 @Override 2066 public boolean equalsShallow(Base other) { 2067 if (!super.equalsShallow(other)) 2068 return false; 2069 if (!(other instanceof Procedure)) 2070 return false; 2071 Procedure o = (Procedure) other; 2072 return compareValues(status, o.status, true) && compareValues(notPerformed, o.notPerformed, true); 2073 } 2074 2075 public boolean isEmpty() { 2076 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (subject == null || subject.isEmpty()) 2077 && (status == null || status.isEmpty()) && (category == null || category.isEmpty()) 2078 && (code == null || code.isEmpty()) && (notPerformed == null || notPerformed.isEmpty()) 2079 && (reasonNotPerformed == null || reasonNotPerformed.isEmpty()) && (bodySite == null || bodySite.isEmpty()) 2080 && (reason == null || reason.isEmpty()) && (performer == null || performer.isEmpty()) 2081 && (performed == null || performed.isEmpty()) && (encounter == null || encounter.isEmpty()) 2082 && (location == null || location.isEmpty()) && (outcome == null || outcome.isEmpty()) 2083 && (report == null || report.isEmpty()) && (complication == null || complication.isEmpty()) 2084 && (followUp == null || followUp.isEmpty()) && (request == null || request.isEmpty()) 2085 && (notes == null || notes.isEmpty()) && (focalDevice == null || focalDevice.isEmpty()) 2086 && (used == null || used.isEmpty()); 2087 } 2088 2089 @Override 2090 public ResourceType getResourceType() { 2091 return ResourceType.Procedure; 2092 } 2093 2094 @SearchParamDefinition(name = "date", path = "Procedure.performed[x]", description = "Date/Period the procedure was performed", type = "date") 2095 public static final String SP_DATE = "date"; 2096 @SearchParamDefinition(name = "identifier", path = "Procedure.identifier", description = "A unique identifier for a procedure", type = "token") 2097 public static final String SP_IDENTIFIER = "identifier"; 2098 @SearchParamDefinition(name = "code", path = "Procedure.code", description = "A code to identify a procedure", type = "token") 2099 public static final String SP_CODE = "code"; 2100 @SearchParamDefinition(name = "performer", path = "Procedure.performer.actor", description = "The reference to the practitioner", type = "reference") 2101 public static final String SP_PERFORMER = "performer"; 2102 @SearchParamDefinition(name = "subject", path = "Procedure.subject", description = "Search by subject", type = "reference") 2103 public static final String SP_SUBJECT = "subject"; 2104 @SearchParamDefinition(name = "patient", path = "Procedure.subject", description = "Search by subject - a patient", type = "reference") 2105 public static final String SP_PATIENT = "patient"; 2106 @SearchParamDefinition(name = "location", path = "Procedure.location", description = "Where the procedure happened", type = "reference") 2107 public static final String SP_LOCATION = "location"; 2108 @SearchParamDefinition(name = "encounter", path = "Procedure.encounter", description = "The encounter associated with the procedure", type = "reference") 2109 public static final String SP_ENCOUNTER = "encounter"; 2110 2111}