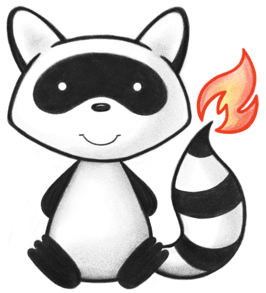
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * A request for a procedure to be performed. May be a proposal or an order. 045 */ 046@ResourceDef(name = "ProcedureRequest", profile = "http://hl7.org/fhir/Profile/ProcedureRequest") 047public class ProcedureRequest extends DomainResource { 048 049 public enum ProcedureRequestStatus { 050 /** 051 * The request has been proposed. 052 */ 053 PROPOSED, 054 /** 055 * The request is in preliminary form, prior to being requested. 056 */ 057 DRAFT, 058 /** 059 * The request has been placed. 060 */ 061 REQUESTED, 062 /** 063 * The receiving system has received the request but not yet decided whether it 064 * will be performed. 065 */ 066 RECEIVED, 067 /** 068 * The receiving system has accepted the request, but work has not yet 069 * commenced. 070 */ 071 ACCEPTED, 072 /** 073 * The work to fulfill the request is happening. 074 */ 075 INPROGRESS, 076 /** 077 * The work has been completed, the report(s) released, and no further work is 078 * planned. 079 */ 080 COMPLETED, 081 /** 082 * The request has been held by originating system/user request. 083 */ 084 SUSPENDED, 085 /** 086 * The receiving system has declined to fulfill the request. 087 */ 088 REJECTED, 089 /** 090 * The request was attempted, but due to some procedural error, it could not be 091 * completed. 092 */ 093 ABORTED, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 099 public static ProcedureRequestStatus fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("proposed".equals(codeString)) 103 return PROPOSED; 104 if ("draft".equals(codeString)) 105 return DRAFT; 106 if ("requested".equals(codeString)) 107 return REQUESTED; 108 if ("received".equals(codeString)) 109 return RECEIVED; 110 if ("accepted".equals(codeString)) 111 return ACCEPTED; 112 if ("in-progress".equals(codeString)) 113 return INPROGRESS; 114 if ("completed".equals(codeString)) 115 return COMPLETED; 116 if ("suspended".equals(codeString)) 117 return SUSPENDED; 118 if ("rejected".equals(codeString)) 119 return REJECTED; 120 if ("aborted".equals(codeString)) 121 return ABORTED; 122 throw new FHIRException("Unknown ProcedureRequestStatus code '" + codeString + "'"); 123 } 124 125 public String toCode() { 126 switch (this) { 127 case PROPOSED: 128 return "proposed"; 129 case DRAFT: 130 return "draft"; 131 case REQUESTED: 132 return "requested"; 133 case RECEIVED: 134 return "received"; 135 case ACCEPTED: 136 return "accepted"; 137 case INPROGRESS: 138 return "in-progress"; 139 case COMPLETED: 140 return "completed"; 141 case SUSPENDED: 142 return "suspended"; 143 case REJECTED: 144 return "rejected"; 145 case ABORTED: 146 return "aborted"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case PROPOSED: 157 return "http://hl7.org/fhir/procedure-request-status"; 158 case DRAFT: 159 return "http://hl7.org/fhir/procedure-request-status"; 160 case REQUESTED: 161 return "http://hl7.org/fhir/procedure-request-status"; 162 case RECEIVED: 163 return "http://hl7.org/fhir/procedure-request-status"; 164 case ACCEPTED: 165 return "http://hl7.org/fhir/procedure-request-status"; 166 case INPROGRESS: 167 return "http://hl7.org/fhir/procedure-request-status"; 168 case COMPLETED: 169 return "http://hl7.org/fhir/procedure-request-status"; 170 case SUSPENDED: 171 return "http://hl7.org/fhir/procedure-request-status"; 172 case REJECTED: 173 return "http://hl7.org/fhir/procedure-request-status"; 174 case ABORTED: 175 return "http://hl7.org/fhir/procedure-request-status"; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 183 public String getDefinition() { 184 switch (this) { 185 case PROPOSED: 186 return "The request has been proposed."; 187 case DRAFT: 188 return "The request is in preliminary form, prior to being requested."; 189 case REQUESTED: 190 return "The request has been placed."; 191 case RECEIVED: 192 return "The receiving system has received the request but not yet decided whether it will be performed."; 193 case ACCEPTED: 194 return "The receiving system has accepted the request, but work has not yet commenced."; 195 case INPROGRESS: 196 return "The work to fulfill the request is happening."; 197 case COMPLETED: 198 return "The work has been completed, the report(s) released, and no further work is planned."; 199 case SUSPENDED: 200 return "The request has been held by originating system/user request."; 201 case REJECTED: 202 return "The receiving system has declined to fulfill the request."; 203 case ABORTED: 204 return "The request was attempted, but due to some procedural error, it could not be completed."; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getDisplay() { 213 switch (this) { 214 case PROPOSED: 215 return "Proposed"; 216 case DRAFT: 217 return "Draft"; 218 case REQUESTED: 219 return "Requested"; 220 case RECEIVED: 221 return "Received"; 222 case ACCEPTED: 223 return "Accepted"; 224 case INPROGRESS: 225 return "In Progress"; 226 case COMPLETED: 227 return "Completed"; 228 case SUSPENDED: 229 return "Suspended"; 230 case REJECTED: 231 return "Rejected"; 232 case ABORTED: 233 return "Aborted"; 234 case NULL: 235 return null; 236 default: 237 return "?"; 238 } 239 } 240 } 241 242 public static class ProcedureRequestStatusEnumFactory implements EnumFactory<ProcedureRequestStatus> { 243 public ProcedureRequestStatus fromCode(String codeString) throws IllegalArgumentException { 244 if (codeString == null || "".equals(codeString)) 245 if (codeString == null || "".equals(codeString)) 246 return null; 247 if ("proposed".equals(codeString)) 248 return ProcedureRequestStatus.PROPOSED; 249 if ("draft".equals(codeString)) 250 return ProcedureRequestStatus.DRAFT; 251 if ("requested".equals(codeString)) 252 return ProcedureRequestStatus.REQUESTED; 253 if ("received".equals(codeString)) 254 return ProcedureRequestStatus.RECEIVED; 255 if ("accepted".equals(codeString)) 256 return ProcedureRequestStatus.ACCEPTED; 257 if ("in-progress".equals(codeString)) 258 return ProcedureRequestStatus.INPROGRESS; 259 if ("completed".equals(codeString)) 260 return ProcedureRequestStatus.COMPLETED; 261 if ("suspended".equals(codeString)) 262 return ProcedureRequestStatus.SUSPENDED; 263 if ("rejected".equals(codeString)) 264 return ProcedureRequestStatus.REJECTED; 265 if ("aborted".equals(codeString)) 266 return ProcedureRequestStatus.ABORTED; 267 throw new IllegalArgumentException("Unknown ProcedureRequestStatus code '" + codeString + "'"); 268 } 269 270 public Enumeration<ProcedureRequestStatus> fromType(Base code) throws FHIRException { 271 if (code == null || code.isEmpty()) 272 return null; 273 String codeString = ((PrimitiveType) code).asStringValue(); 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("proposed".equals(codeString)) 277 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.PROPOSED); 278 if ("draft".equals(codeString)) 279 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.DRAFT); 280 if ("requested".equals(codeString)) 281 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.REQUESTED); 282 if ("received".equals(codeString)) 283 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.RECEIVED); 284 if ("accepted".equals(codeString)) 285 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.ACCEPTED); 286 if ("in-progress".equals(codeString)) 287 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.INPROGRESS); 288 if ("completed".equals(codeString)) 289 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.COMPLETED); 290 if ("suspended".equals(codeString)) 291 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.SUSPENDED); 292 if ("rejected".equals(codeString)) 293 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.REJECTED); 294 if ("aborted".equals(codeString)) 295 return new Enumeration<ProcedureRequestStatus>(this, ProcedureRequestStatus.ABORTED); 296 throw new FHIRException("Unknown ProcedureRequestStatus code '" + codeString + "'"); 297 } 298 299 public String toCode(ProcedureRequestStatus code) 300 { 301 if (code == ProcedureRequestStatus.NULL) 302 return null; 303 if (code == ProcedureRequestStatus.PROPOSED) 304 return "proposed"; 305 if (code == ProcedureRequestStatus.DRAFT) 306 return "draft"; 307 if (code == ProcedureRequestStatus.REQUESTED) 308 return "requested"; 309 if (code == ProcedureRequestStatus.RECEIVED) 310 return "received"; 311 if (code == ProcedureRequestStatus.ACCEPTED) 312 return "accepted"; 313 if (code == ProcedureRequestStatus.INPROGRESS) 314 return "in-progress"; 315 if (code == ProcedureRequestStatus.COMPLETED) 316 return "completed"; 317 if (code == ProcedureRequestStatus.SUSPENDED) 318 return "suspended"; 319 if (code == ProcedureRequestStatus.REJECTED) 320 return "rejected"; 321 if (code == ProcedureRequestStatus.ABORTED) 322 return "aborted"; 323 return "?"; 324 } 325 } 326 327 public enum ProcedureRequestPriority { 328 /** 329 * The request has a normal priority. 330 */ 331 ROUTINE, 332 /** 333 * The request should be done urgently. 334 */ 335 URGENT, 336 /** 337 * The request is time-critical. 338 */ 339 STAT, 340 /** 341 * The request should be acted on as soon as possible. 342 */ 343 ASAP, 344 /** 345 * added to help the parsers 346 */ 347 NULL; 348 349 public static ProcedureRequestPriority fromCode(String codeString) throws FHIRException { 350 if (codeString == null || "".equals(codeString)) 351 return null; 352 if ("routine".equals(codeString)) 353 return ROUTINE; 354 if ("urgent".equals(codeString)) 355 return URGENT; 356 if ("stat".equals(codeString)) 357 return STAT; 358 if ("asap".equals(codeString)) 359 return ASAP; 360 throw new FHIRException("Unknown ProcedureRequestPriority code '" + codeString + "'"); 361 } 362 363 public String toCode() { 364 switch (this) { 365 case ROUTINE: 366 return "routine"; 367 case URGENT: 368 return "urgent"; 369 case STAT: 370 return "stat"; 371 case ASAP: 372 return "asap"; 373 case NULL: 374 return null; 375 default: 376 return "?"; 377 } 378 } 379 380 public String getSystem() { 381 switch (this) { 382 case ROUTINE: 383 return "http://hl7.org/fhir/procedure-request-priority"; 384 case URGENT: 385 return "http://hl7.org/fhir/procedure-request-priority"; 386 case STAT: 387 return "http://hl7.org/fhir/procedure-request-priority"; 388 case ASAP: 389 return "http://hl7.org/fhir/procedure-request-priority"; 390 case NULL: 391 return null; 392 default: 393 return "?"; 394 } 395 } 396 397 public String getDefinition() { 398 switch (this) { 399 case ROUTINE: 400 return "The request has a normal priority."; 401 case URGENT: 402 return "The request should be done urgently."; 403 case STAT: 404 return "The request is time-critical."; 405 case ASAP: 406 return "The request should be acted on as soon as possible."; 407 case NULL: 408 return null; 409 default: 410 return "?"; 411 } 412 } 413 414 public String getDisplay() { 415 switch (this) { 416 case ROUTINE: 417 return "Routine"; 418 case URGENT: 419 return "Urgent"; 420 case STAT: 421 return "Stat"; 422 case ASAP: 423 return "ASAP"; 424 case NULL: 425 return null; 426 default: 427 return "?"; 428 } 429 } 430 } 431 432 public static class ProcedureRequestPriorityEnumFactory implements EnumFactory<ProcedureRequestPriority> { 433 public ProcedureRequestPriority fromCode(String codeString) throws IllegalArgumentException { 434 if (codeString == null || "".equals(codeString)) 435 if (codeString == null || "".equals(codeString)) 436 return null; 437 if ("routine".equals(codeString)) 438 return ProcedureRequestPriority.ROUTINE; 439 if ("urgent".equals(codeString)) 440 return ProcedureRequestPriority.URGENT; 441 if ("stat".equals(codeString)) 442 return ProcedureRequestPriority.STAT; 443 if ("asap".equals(codeString)) 444 return ProcedureRequestPriority.ASAP; 445 throw new IllegalArgumentException("Unknown ProcedureRequestPriority code '" + codeString + "'"); 446 } 447 448 public Enumeration<ProcedureRequestPriority> fromType(Base code) throws FHIRException { 449 if (code == null || code.isEmpty()) 450 return null; 451 String codeString = ((PrimitiveType) code).asStringValue(); 452 if (codeString == null || "".equals(codeString)) 453 return null; 454 if ("routine".equals(codeString)) 455 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.ROUTINE); 456 if ("urgent".equals(codeString)) 457 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.URGENT); 458 if ("stat".equals(codeString)) 459 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.STAT); 460 if ("asap".equals(codeString)) 461 return new Enumeration<ProcedureRequestPriority>(this, ProcedureRequestPriority.ASAP); 462 throw new FHIRException("Unknown ProcedureRequestPriority code '" + codeString + "'"); 463 } 464 465 public String toCode(ProcedureRequestPriority code) 466 { 467 if (code == ProcedureRequestPriority.NULL) 468 return null; 469 if (code == ProcedureRequestPriority.ROUTINE) 470 return "routine"; 471 if (code == ProcedureRequestPriority.URGENT) 472 return "urgent"; 473 if (code == ProcedureRequestPriority.STAT) 474 return "stat"; 475 if (code == ProcedureRequestPriority.ASAP) 476 return "asap"; 477 return "?"; 478 } 479 } 480 481 /** 482 * Identifiers assigned to this order by the order or by the receiver. 483 */ 484 @Child(name = "identifier", type = { 485 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 486 @Description(shortDefinition = "Unique identifier for the request", formalDefinition = "Identifiers assigned to this order by the order or by the receiver.") 487 protected List<Identifier> identifier; 488 489 /** 490 * The person, animal or group that should receive the procedure. 491 */ 492 @Child(name = "subject", type = { Patient.class, 493 Group.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 494 @Description(shortDefinition = "Who the procedure should be done to", formalDefinition = "The person, animal or group that should receive the procedure.") 495 protected Reference subject; 496 497 /** 498 * The actual object that is the target of the reference (The person, animal or 499 * group that should receive the procedure.) 500 */ 501 protected Resource subjectTarget; 502 503 /** 504 * The specific procedure that is ordered. Use text if the exact nature of the 505 * procedure cannot be coded. 506 */ 507 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 508 @Description(shortDefinition = "What procedure to perform", formalDefinition = "The specific procedure that is ordered. Use text if the exact nature of the procedure cannot be coded.") 509 protected CodeableConcept code; 510 511 /** 512 * Indicates the sites on the subject's body where the procedure should be 513 * performed (I.e. the target sites). 514 */ 515 @Child(name = "bodySite", type = { 516 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 517 @Description(shortDefinition = "What part of body to perform on", formalDefinition = "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).") 518 protected List<CodeableConcept> bodySite; 519 520 /** 521 * The reason why the procedure is being proposed or ordered. This procedure 522 * request may be motivated by a Condition for instance. 523 */ 524 @Child(name = "reason", type = { CodeableConcept.class, 525 Condition.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 526 @Description(shortDefinition = "Why procedure should occur", formalDefinition = "The reason why the procedure is being proposed or ordered. This procedure request may be motivated by a Condition for instance.") 527 protected Type reason; 528 529 /** 530 * The timing schedule for the proposed or ordered procedure. The Schedule data 531 * type allows many different expressions. E.g. "Every 8 hours"; "Three times a 532 * day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 533 * 2013, 17 Oct 2013 and 1 Nov 2013". 534 */ 535 @Child(name = "scheduled", type = { DateTimeType.class, Period.class, 536 Timing.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 537 @Description(shortDefinition = "When procedure should occur", formalDefinition = "The timing schedule for the proposed or ordered procedure. The Schedule data type allows many different expressions. E.g. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".") 538 protected Type scheduled; 539 540 /** 541 * The encounter within which the procedure proposal or request was created. 542 */ 543 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 544 @Description(shortDefinition = "Encounter request created during", formalDefinition = "The encounter within which the procedure proposal or request was created.") 545 protected Reference encounter; 546 547 /** 548 * The actual object that is the target of the reference (The encounter within 549 * which the procedure proposal or request was created.) 550 */ 551 protected Encounter encounterTarget; 552 553 /** 554 * For example, the surgeon, anaethetist, endoscopist, etc. 555 */ 556 @Child(name = "performer", type = { Practitioner.class, Organization.class, Patient.class, 557 RelatedPerson.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 558 @Description(shortDefinition = "Who should perform the procedure", formalDefinition = "For example, the surgeon, anaethetist, endoscopist, etc.") 559 protected Reference performer; 560 561 /** 562 * The actual object that is the target of the reference (For example, the 563 * surgeon, anaethetist, endoscopist, etc.) 564 */ 565 protected Resource performerTarget; 566 567 /** 568 * The status of the order. 569 */ 570 @Child(name = "status", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = true, summary = true) 571 @Description(shortDefinition = "proposed | draft | requested | received | accepted | in-progress | completed | suspended | rejected | aborted", formalDefinition = "The status of the order.") 572 protected Enumeration<ProcedureRequestStatus> status; 573 574 /** 575 * Any other notes associated with this proposal or order - e.g. provider 576 * instructions. 577 */ 578 @Child(name = "notes", type = { 579 Annotation.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 580 @Description(shortDefinition = "Additional information about desired procedure", formalDefinition = "Any other notes associated with this proposal or order - e.g. provider instructions.") 581 protected List<Annotation> notes; 582 583 /** 584 * If a CodeableConcept is present, it indicates the pre-condition for 585 * performing the procedure. 586 */ 587 @Child(name = "asNeeded", type = { BooleanType.class, 588 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 589 @Description(shortDefinition = "Preconditions for procedure", formalDefinition = "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure.") 590 protected Type asNeeded; 591 592 /** 593 * The time when the request was made. 594 */ 595 @Child(name = "orderedOn", type = { 596 DateTimeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 597 @Description(shortDefinition = "When request was created", formalDefinition = "The time when the request was made.") 598 protected DateTimeType orderedOn; 599 600 /** 601 * The healthcare professional responsible for proposing or ordering the 602 * procedure. 603 */ 604 @Child(name = "orderer", type = { Practitioner.class, Patient.class, RelatedPerson.class, 605 Device.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 606 @Description(shortDefinition = "Who made request", formalDefinition = "The healthcare professional responsible for proposing or ordering the procedure.") 607 protected Reference orderer; 608 609 /** 610 * The actual object that is the target of the reference (The healthcare 611 * professional responsible for proposing or ordering the procedure.) 612 */ 613 protected Resource ordererTarget; 614 615 /** 616 * The clinical priority associated with this order. 617 */ 618 @Child(name = "priority", type = { CodeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 619 @Description(shortDefinition = "routine | urgent | stat | asap", formalDefinition = "The clinical priority associated with this order.") 620 protected Enumeration<ProcedureRequestPriority> priority; 621 622 private static final long serialVersionUID = -916650578L; 623 624 /* 625 * Constructor 626 */ 627 public ProcedureRequest() { 628 super(); 629 } 630 631 /* 632 * Constructor 633 */ 634 public ProcedureRequest(Reference subject, CodeableConcept code) { 635 super(); 636 this.subject = subject; 637 this.code = code; 638 } 639 640 /** 641 * @return {@link #identifier} (Identifiers assigned to this order by the order 642 * or by the receiver.) 643 */ 644 public List<Identifier> getIdentifier() { 645 if (this.identifier == null) 646 this.identifier = new ArrayList<Identifier>(); 647 return this.identifier; 648 } 649 650 public boolean hasIdentifier() { 651 if (this.identifier == null) 652 return false; 653 for (Identifier item : this.identifier) 654 if (!item.isEmpty()) 655 return true; 656 return false; 657 } 658 659 /** 660 * @return {@link #identifier} (Identifiers assigned to this order by the order 661 * or by the receiver.) 662 */ 663 // syntactic sugar 664 public Identifier addIdentifier() { // 3 665 Identifier t = new Identifier(); 666 if (this.identifier == null) 667 this.identifier = new ArrayList<Identifier>(); 668 this.identifier.add(t); 669 return t; 670 } 671 672 // syntactic sugar 673 public ProcedureRequest addIdentifier(Identifier t) { // 3 674 if (t == null) 675 return this; 676 if (this.identifier == null) 677 this.identifier = new ArrayList<Identifier>(); 678 this.identifier.add(t); 679 return this; 680 } 681 682 /** 683 * @return {@link #subject} (The person, animal or group that should receive the 684 * procedure.) 685 */ 686 public Reference getSubject() { 687 if (this.subject == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create ProcedureRequest.subject"); 690 else if (Configuration.doAutoCreate()) 691 this.subject = new Reference(); // cc 692 return this.subject; 693 } 694 695 public boolean hasSubject() { 696 return this.subject != null && !this.subject.isEmpty(); 697 } 698 699 /** 700 * @param value {@link #subject} (The person, animal or group that should 701 * receive the procedure.) 702 */ 703 public ProcedureRequest setSubject(Reference value) { 704 this.subject = value; 705 return this; 706 } 707 708 /** 709 * @return {@link #subject} The actual object that is the target of the 710 * reference. The reference library doesn't populate this, but you can 711 * use it to hold the resource if you resolve it. (The person, animal or 712 * group that should receive the procedure.) 713 */ 714 public Resource getSubjectTarget() { 715 return this.subjectTarget; 716 } 717 718 /** 719 * @param value {@link #subject} The actual object that is the target of the 720 * reference. The reference library doesn't use these, but you can 721 * use it to hold the resource if you resolve it. (The person, 722 * animal or group that should receive the procedure.) 723 */ 724 public ProcedureRequest setSubjectTarget(Resource value) { 725 this.subjectTarget = value; 726 return this; 727 } 728 729 /** 730 * @return {@link #code} (The specific procedure that is ordered. Use text if 731 * the exact nature of the procedure cannot be coded.) 732 */ 733 public CodeableConcept getCode() { 734 if (this.code == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create ProcedureRequest.code"); 737 else if (Configuration.doAutoCreate()) 738 this.code = new CodeableConcept(); // cc 739 return this.code; 740 } 741 742 public boolean hasCode() { 743 return this.code != null && !this.code.isEmpty(); 744 } 745 746 /** 747 * @param value {@link #code} (The specific procedure that is ordered. Use text 748 * if the exact nature of the procedure cannot be coded.) 749 */ 750 public ProcedureRequest setCode(CodeableConcept value) { 751 this.code = value; 752 return this; 753 } 754 755 /** 756 * @return {@link #bodySite} (Indicates the sites on the subject's body where 757 * the procedure should be performed (I.e. the target sites).) 758 */ 759 public List<CodeableConcept> getBodySite() { 760 if (this.bodySite == null) 761 this.bodySite = new ArrayList<CodeableConcept>(); 762 return this.bodySite; 763 } 764 765 public boolean hasBodySite() { 766 if (this.bodySite == null) 767 return false; 768 for (CodeableConcept item : this.bodySite) 769 if (!item.isEmpty()) 770 return true; 771 return false; 772 } 773 774 /** 775 * @return {@link #bodySite} (Indicates the sites on the subject's body where 776 * the procedure should be performed (I.e. the target sites).) 777 */ 778 // syntactic sugar 779 public CodeableConcept addBodySite() { // 3 780 CodeableConcept t = new CodeableConcept(); 781 if (this.bodySite == null) 782 this.bodySite = new ArrayList<CodeableConcept>(); 783 this.bodySite.add(t); 784 return t; 785 } 786 787 // syntactic sugar 788 public ProcedureRequest addBodySite(CodeableConcept t) { // 3 789 if (t == null) 790 return this; 791 if (this.bodySite == null) 792 this.bodySite = new ArrayList<CodeableConcept>(); 793 this.bodySite.add(t); 794 return this; 795 } 796 797 /** 798 * @return {@link #reason} (The reason why the procedure is being proposed or 799 * ordered. This procedure request may be motivated by a Condition for 800 * instance.) 801 */ 802 public Type getReason() { 803 return this.reason; 804 } 805 806 /** 807 * @return {@link #reason} (The reason why the procedure is being proposed or 808 * ordered. This procedure request may be motivated by a Condition for 809 * instance.) 810 */ 811 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 812 if (!(this.reason instanceof CodeableConcept)) 813 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 814 + this.reason.getClass().getName() + " was encountered"); 815 return (CodeableConcept) this.reason; 816 } 817 818 public boolean hasReasonCodeableConcept() { 819 return this.reason instanceof CodeableConcept; 820 } 821 822 /** 823 * @return {@link #reason} (The reason why the procedure is being proposed or 824 * ordered. This procedure request may be motivated by a Condition for 825 * instance.) 826 */ 827 public Reference getReasonReference() throws FHIRException { 828 if (!(this.reason instanceof Reference)) 829 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.reason.getClass().getName() 830 + " was encountered"); 831 return (Reference) this.reason; 832 } 833 834 public boolean hasReasonReference() { 835 return this.reason instanceof Reference; 836 } 837 838 public boolean hasReason() { 839 return this.reason != null && !this.reason.isEmpty(); 840 } 841 842 /** 843 * @param value {@link #reason} (The reason why the procedure is being proposed 844 * or ordered. This procedure request may be motivated by a 845 * Condition for instance.) 846 */ 847 public ProcedureRequest setReason(Type value) { 848 this.reason = value; 849 return this; 850 } 851 852 /** 853 * @return {@link #scheduled} (The timing schedule for the proposed or ordered 854 * procedure. The Schedule data type allows many different expressions. 855 * E.g. "Every 8 hours"; "Three times a day"; "1/2 an hour before 856 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 857 * and 1 Nov 2013".) 858 */ 859 public Type getScheduled() { 860 return this.scheduled; 861 } 862 863 /** 864 * @return {@link #scheduled} (The timing schedule for the proposed or ordered 865 * procedure. The Schedule data type allows many different expressions. 866 * E.g. "Every 8 hours"; "Three times a day"; "1/2 an hour before 867 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 868 * and 1 Nov 2013".) 869 */ 870 public DateTimeType getScheduledDateTimeType() throws FHIRException { 871 if (!(this.scheduled instanceof DateTimeType)) 872 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 873 + this.scheduled.getClass().getName() + " was encountered"); 874 return (DateTimeType) this.scheduled; 875 } 876 877 public boolean hasScheduledDateTimeType() { 878 return this.scheduled instanceof DateTimeType; 879 } 880 881 /** 882 * @return {@link #scheduled} (The timing schedule for the proposed or ordered 883 * procedure. The Schedule data type allows many different expressions. 884 * E.g. "Every 8 hours"; "Three times a day"; "1/2 an hour before 885 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 886 * and 1 Nov 2013".) 887 */ 888 public Period getScheduledPeriod() throws FHIRException { 889 if (!(this.scheduled instanceof Period)) 890 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.scheduled.getClass().getName() 891 + " was encountered"); 892 return (Period) this.scheduled; 893 } 894 895 public boolean hasScheduledPeriod() { 896 return this.scheduled instanceof Period; 897 } 898 899 /** 900 * @return {@link #scheduled} (The timing schedule for the proposed or ordered 901 * procedure. The Schedule data type allows many different expressions. 902 * E.g. "Every 8 hours"; "Three times a day"; "1/2 an hour before 903 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 904 * and 1 Nov 2013".) 905 */ 906 public Timing getScheduledTiming() throws FHIRException { 907 if (!(this.scheduled instanceof Timing)) 908 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.scheduled.getClass().getName() 909 + " was encountered"); 910 return (Timing) this.scheduled; 911 } 912 913 public boolean hasScheduledTiming() { 914 return this.scheduled instanceof Timing; 915 } 916 917 public boolean hasScheduled() { 918 return this.scheduled != null && !this.scheduled.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #scheduled} (The timing schedule for the proposed or 923 * ordered procedure. The Schedule data type allows many different 924 * expressions. E.g. "Every 8 hours"; "Three times a day"; "1/2 an 925 * hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 926 * 2013, 17 Oct 2013 and 1 Nov 2013".) 927 */ 928 public ProcedureRequest setScheduled(Type value) { 929 this.scheduled = value; 930 return this; 931 } 932 933 /** 934 * @return {@link #encounter} (The encounter within which the procedure proposal 935 * or request was created.) 936 */ 937 public Reference getEncounter() { 938 if (this.encounter == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create ProcedureRequest.encounter"); 941 else if (Configuration.doAutoCreate()) 942 this.encounter = new Reference(); // cc 943 return this.encounter; 944 } 945 946 public boolean hasEncounter() { 947 return this.encounter != null && !this.encounter.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #encounter} (The encounter within which the procedure 952 * proposal or request was created.) 953 */ 954 public ProcedureRequest setEncounter(Reference value) { 955 this.encounter = value; 956 return this; 957 } 958 959 /** 960 * @return {@link #encounter} The actual object that is the target of the 961 * reference. The reference library doesn't populate this, but you can 962 * use it to hold the resource if you resolve it. (The encounter within 963 * which the procedure proposal or request was created.) 964 */ 965 public Encounter getEncounterTarget() { 966 if (this.encounterTarget == null) 967 if (Configuration.errorOnAutoCreate()) 968 throw new Error("Attempt to auto-create ProcedureRequest.encounter"); 969 else if (Configuration.doAutoCreate()) 970 this.encounterTarget = new Encounter(); // aa 971 return this.encounterTarget; 972 } 973 974 /** 975 * @param value {@link #encounter} The actual object that is the target of the 976 * reference. The reference library doesn't use these, but you can 977 * use it to hold the resource if you resolve it. (The encounter 978 * within which the procedure proposal or request was created.) 979 */ 980 public ProcedureRequest setEncounterTarget(Encounter value) { 981 this.encounterTarget = value; 982 return this; 983 } 984 985 /** 986 * @return {@link #performer} (For example, the surgeon, anaethetist, 987 * endoscopist, etc.) 988 */ 989 public Reference getPerformer() { 990 if (this.performer == null) 991 if (Configuration.errorOnAutoCreate()) 992 throw new Error("Attempt to auto-create ProcedureRequest.performer"); 993 else if (Configuration.doAutoCreate()) 994 this.performer = new Reference(); // cc 995 return this.performer; 996 } 997 998 public boolean hasPerformer() { 999 return this.performer != null && !this.performer.isEmpty(); 1000 } 1001 1002 /** 1003 * @param value {@link #performer} (For example, the surgeon, anaethetist, 1004 * endoscopist, etc.) 1005 */ 1006 public ProcedureRequest setPerformer(Reference value) { 1007 this.performer = value; 1008 return this; 1009 } 1010 1011 /** 1012 * @return {@link #performer} The actual object that is the target of the 1013 * reference. The reference library doesn't populate this, but you can 1014 * use it to hold the resource if you resolve it. (For example, the 1015 * surgeon, anaethetist, endoscopist, etc.) 1016 */ 1017 public Resource getPerformerTarget() { 1018 return this.performerTarget; 1019 } 1020 1021 /** 1022 * @param value {@link #performer} The actual object that is the target of the 1023 * reference. The reference library doesn't use these, but you can 1024 * use it to hold the resource if you resolve it. (For example, the 1025 * surgeon, anaethetist, endoscopist, etc.) 1026 */ 1027 public ProcedureRequest setPerformerTarget(Resource value) { 1028 this.performerTarget = value; 1029 return this; 1030 } 1031 1032 /** 1033 * @return {@link #status} (The status of the order.). This is the underlying 1034 * object with id, value and extensions. The accessor "getStatus" gives 1035 * direct access to the value 1036 */ 1037 public Enumeration<ProcedureRequestStatus> getStatusElement() { 1038 if (this.status == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create ProcedureRequest.status"); 1041 else if (Configuration.doAutoCreate()) 1042 this.status = new Enumeration<ProcedureRequestStatus>(new ProcedureRequestStatusEnumFactory()); // bb 1043 return this.status; 1044 } 1045 1046 public boolean hasStatusElement() { 1047 return this.status != null && !this.status.isEmpty(); 1048 } 1049 1050 public boolean hasStatus() { 1051 return this.status != null && !this.status.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #status} (The status of the order.). This is the 1056 * underlying object with id, value and extensions. The accessor 1057 * "getStatus" gives direct access to the value 1058 */ 1059 public ProcedureRequest setStatusElement(Enumeration<ProcedureRequestStatus> value) { 1060 this.status = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return The status of the order. 1066 */ 1067 public ProcedureRequestStatus getStatus() { 1068 return this.status == null ? null : this.status.getValue(); 1069 } 1070 1071 /** 1072 * @param value The status of the order. 1073 */ 1074 public ProcedureRequest setStatus(ProcedureRequestStatus value) { 1075 if (value == null) 1076 this.status = null; 1077 else { 1078 if (this.status == null) 1079 this.status = new Enumeration<ProcedureRequestStatus>(new ProcedureRequestStatusEnumFactory()); 1080 this.status.setValue(value); 1081 } 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #notes} (Any other notes associated with this proposal or 1087 * order - e.g. provider instructions.) 1088 */ 1089 public List<Annotation> getNotes() { 1090 if (this.notes == null) 1091 this.notes = new ArrayList<Annotation>(); 1092 return this.notes; 1093 } 1094 1095 public boolean hasNotes() { 1096 if (this.notes == null) 1097 return false; 1098 for (Annotation item : this.notes) 1099 if (!item.isEmpty()) 1100 return true; 1101 return false; 1102 } 1103 1104 /** 1105 * @return {@link #notes} (Any other notes associated with this proposal or 1106 * order - e.g. provider instructions.) 1107 */ 1108 // syntactic sugar 1109 public Annotation addNotes() { // 3 1110 Annotation t = new Annotation(); 1111 if (this.notes == null) 1112 this.notes = new ArrayList<Annotation>(); 1113 this.notes.add(t); 1114 return t; 1115 } 1116 1117 // syntactic sugar 1118 public ProcedureRequest addNotes(Annotation t) { // 3 1119 if (t == null) 1120 return this; 1121 if (this.notes == null) 1122 this.notes = new ArrayList<Annotation>(); 1123 this.notes.add(t); 1124 return this; 1125 } 1126 1127 /** 1128 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the 1129 * pre-condition for performing the procedure.) 1130 */ 1131 public Type getAsNeeded() { 1132 return this.asNeeded; 1133 } 1134 1135 /** 1136 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the 1137 * pre-condition for performing the procedure.) 1138 */ 1139 public BooleanType getAsNeededBooleanType() throws FHIRException { 1140 if (!(this.asNeeded instanceof BooleanType)) 1141 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1142 + this.asNeeded.getClass().getName() + " was encountered"); 1143 return (BooleanType) this.asNeeded; 1144 } 1145 1146 public boolean hasAsNeededBooleanType() { 1147 return this.asNeeded instanceof BooleanType; 1148 } 1149 1150 /** 1151 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the 1152 * pre-condition for performing the procedure.) 1153 */ 1154 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 1155 if (!(this.asNeeded instanceof CodeableConcept)) 1156 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1157 + this.asNeeded.getClass().getName() + " was encountered"); 1158 return (CodeableConcept) this.asNeeded; 1159 } 1160 1161 public boolean hasAsNeededCodeableConcept() { 1162 return this.asNeeded instanceof CodeableConcept; 1163 } 1164 1165 public boolean hasAsNeeded() { 1166 return this.asNeeded != null && !this.asNeeded.isEmpty(); 1167 } 1168 1169 /** 1170 * @param value {@link #asNeeded} (If a CodeableConcept is present, it indicates 1171 * the pre-condition for performing the procedure.) 1172 */ 1173 public ProcedureRequest setAsNeeded(Type value) { 1174 this.asNeeded = value; 1175 return this; 1176 } 1177 1178 /** 1179 * @return {@link #orderedOn} (The time when the request was made.). This is the 1180 * underlying object with id, value and extensions. The accessor 1181 * "getOrderedOn" gives direct access to the value 1182 */ 1183 public DateTimeType getOrderedOnElement() { 1184 if (this.orderedOn == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create ProcedureRequest.orderedOn"); 1187 else if (Configuration.doAutoCreate()) 1188 this.orderedOn = new DateTimeType(); // bb 1189 return this.orderedOn; 1190 } 1191 1192 public boolean hasOrderedOnElement() { 1193 return this.orderedOn != null && !this.orderedOn.isEmpty(); 1194 } 1195 1196 public boolean hasOrderedOn() { 1197 return this.orderedOn != null && !this.orderedOn.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #orderedOn} (The time when the request was made.). This 1202 * is the underlying object with id, value and extensions. The 1203 * accessor "getOrderedOn" gives direct access to the value 1204 */ 1205 public ProcedureRequest setOrderedOnElement(DateTimeType value) { 1206 this.orderedOn = value; 1207 return this; 1208 } 1209 1210 /** 1211 * @return The time when the request was made. 1212 */ 1213 public Date getOrderedOn() { 1214 return this.orderedOn == null ? null : this.orderedOn.getValue(); 1215 } 1216 1217 /** 1218 * @param value The time when the request was made. 1219 */ 1220 public ProcedureRequest setOrderedOn(Date value) { 1221 if (value == null) 1222 this.orderedOn = null; 1223 else { 1224 if (this.orderedOn == null) 1225 this.orderedOn = new DateTimeType(); 1226 this.orderedOn.setValue(value); 1227 } 1228 return this; 1229 } 1230 1231 /** 1232 * @return {@link #orderer} (The healthcare professional responsible for 1233 * proposing or ordering the procedure.) 1234 */ 1235 public Reference getOrderer() { 1236 if (this.orderer == null) 1237 if (Configuration.errorOnAutoCreate()) 1238 throw new Error("Attempt to auto-create ProcedureRequest.orderer"); 1239 else if (Configuration.doAutoCreate()) 1240 this.orderer = new Reference(); // cc 1241 return this.orderer; 1242 } 1243 1244 public boolean hasOrderer() { 1245 return this.orderer != null && !this.orderer.isEmpty(); 1246 } 1247 1248 /** 1249 * @param value {@link #orderer} (The healthcare professional responsible for 1250 * proposing or ordering the procedure.) 1251 */ 1252 public ProcedureRequest setOrderer(Reference value) { 1253 this.orderer = value; 1254 return this; 1255 } 1256 1257 /** 1258 * @return {@link #orderer} The actual object that is the target of the 1259 * reference. The reference library doesn't populate this, but you can 1260 * use it to hold the resource if you resolve it. (The healthcare 1261 * professional responsible for proposing or ordering the procedure.) 1262 */ 1263 public Resource getOrdererTarget() { 1264 return this.ordererTarget; 1265 } 1266 1267 /** 1268 * @param value {@link #orderer} The actual object that is the target of the 1269 * reference. The reference library doesn't use these, but you can 1270 * use it to hold the resource if you resolve it. (The healthcare 1271 * professional responsible for proposing or ordering the 1272 * procedure.) 1273 */ 1274 public ProcedureRequest setOrdererTarget(Resource value) { 1275 this.ordererTarget = value; 1276 return this; 1277 } 1278 1279 /** 1280 * @return {@link #priority} (The clinical priority associated with this 1281 * order.). This is the underlying object with id, value and extensions. 1282 * The accessor "getPriority" gives direct access to the value 1283 */ 1284 public Enumeration<ProcedureRequestPriority> getPriorityElement() { 1285 if (this.priority == null) 1286 if (Configuration.errorOnAutoCreate()) 1287 throw new Error("Attempt to auto-create ProcedureRequest.priority"); 1288 else if (Configuration.doAutoCreate()) 1289 this.priority = new Enumeration<ProcedureRequestPriority>(new ProcedureRequestPriorityEnumFactory()); // bb 1290 return this.priority; 1291 } 1292 1293 public boolean hasPriorityElement() { 1294 return this.priority != null && !this.priority.isEmpty(); 1295 } 1296 1297 public boolean hasPriority() { 1298 return this.priority != null && !this.priority.isEmpty(); 1299 } 1300 1301 /** 1302 * @param value {@link #priority} (The clinical priority associated with this 1303 * order.). This is the underlying object with id, value and 1304 * extensions. The accessor "getPriority" gives direct access to 1305 * the value 1306 */ 1307 public ProcedureRequest setPriorityElement(Enumeration<ProcedureRequestPriority> value) { 1308 this.priority = value; 1309 return this; 1310 } 1311 1312 /** 1313 * @return The clinical priority associated with this order. 1314 */ 1315 public ProcedureRequestPriority getPriority() { 1316 return this.priority == null ? null : this.priority.getValue(); 1317 } 1318 1319 /** 1320 * @param value The clinical priority associated with this order. 1321 */ 1322 public ProcedureRequest setPriority(ProcedureRequestPriority value) { 1323 if (value == null) 1324 this.priority = null; 1325 else { 1326 if (this.priority == null) 1327 this.priority = new Enumeration<ProcedureRequestPriority>(new ProcedureRequestPriorityEnumFactory()); 1328 this.priority.setValue(value); 1329 } 1330 return this; 1331 } 1332 1333 protected void listChildren(List<Property> childrenList) { 1334 super.listChildren(childrenList); 1335 childrenList.add( 1336 new Property("identifier", "Identifier", "Identifiers assigned to this order by the order or by the receiver.", 1337 0, java.lang.Integer.MAX_VALUE, identifier)); 1338 childrenList.add(new Property("subject", "Reference(Patient|Group)", 1339 "The person, animal or group that should receive the procedure.", 0, java.lang.Integer.MAX_VALUE, subject)); 1340 childrenList.add(new Property("code", "CodeableConcept", 1341 "The specific procedure that is ordered. Use text if the exact nature of the procedure cannot be coded.", 0, 1342 java.lang.Integer.MAX_VALUE, code)); 1343 childrenList.add(new Property("bodySite", "CodeableConcept", 1344 "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 0, 1345 java.lang.Integer.MAX_VALUE, bodySite)); 1346 childrenList.add(new Property("reason[x]", "CodeableConcept|Reference(Condition)", 1347 "The reason why the procedure is being proposed or ordered. This procedure request may be motivated by a Condition for instance.", 1348 0, java.lang.Integer.MAX_VALUE, reason)); 1349 childrenList.add(new Property("scheduled[x]", "dateTime|Period|Timing", 1350 "The timing schedule for the proposed or ordered procedure. The Schedule data type allows many different expressions. E.g. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 1351 0, java.lang.Integer.MAX_VALUE, scheduled)); 1352 childrenList.add(new Property("encounter", "Reference(Encounter)", 1353 "The encounter within which the procedure proposal or request was created.", 0, java.lang.Integer.MAX_VALUE, 1354 encounter)); 1355 childrenList.add(new Property("performer", "Reference(Practitioner|Organization|Patient|RelatedPerson)", 1356 "For example, the surgeon, anaethetist, endoscopist, etc.", 0, java.lang.Integer.MAX_VALUE, performer)); 1357 childrenList 1358 .add(new Property("status", "code", "The status of the order.", 0, java.lang.Integer.MAX_VALUE, status)); 1359 childrenList.add(new Property("notes", "Annotation", 1360 "Any other notes associated with this proposal or order - e.g. provider instructions.", 0, 1361 java.lang.Integer.MAX_VALUE, notes)); 1362 childrenList.add(new Property("asNeeded[x]", "boolean|CodeableConcept", 1363 "If a CodeableConcept is present, it indicates the pre-condition for performing the procedure.", 0, 1364 java.lang.Integer.MAX_VALUE, asNeeded)); 1365 childrenList.add(new Property("orderedOn", "dateTime", "The time when the request was made.", 0, 1366 java.lang.Integer.MAX_VALUE, orderedOn)); 1367 childrenList.add(new Property("orderer", "Reference(Practitioner|Patient|RelatedPerson|Device)", 1368 "The healthcare professional responsible for proposing or ordering the procedure.", 0, 1369 java.lang.Integer.MAX_VALUE, orderer)); 1370 childrenList.add(new Property("priority", "code", "The clinical priority associated with this order.", 0, 1371 java.lang.Integer.MAX_VALUE, priority)); 1372 } 1373 1374 @Override 1375 public void setProperty(String name, Base value) throws FHIRException { 1376 if (name.equals("identifier")) 1377 this.getIdentifier().add(castToIdentifier(value)); 1378 else if (name.equals("subject")) 1379 this.subject = castToReference(value); // Reference 1380 else if (name.equals("code")) 1381 this.code = castToCodeableConcept(value); // CodeableConcept 1382 else if (name.equals("bodySite")) 1383 this.getBodySite().add(castToCodeableConcept(value)); 1384 else if (name.equals("reason[x]")) 1385 this.reason = (Type) value; // Type 1386 else if (name.equals("scheduled[x]")) 1387 this.scheduled = (Type) value; // Type 1388 else if (name.equals("encounter")) 1389 this.encounter = castToReference(value); // Reference 1390 else if (name.equals("performer")) 1391 this.performer = castToReference(value); // Reference 1392 else if (name.equals("status")) 1393 this.status = new ProcedureRequestStatusEnumFactory().fromType(value); // Enumeration<ProcedureRequestStatus> 1394 else if (name.equals("notes")) 1395 this.getNotes().add(castToAnnotation(value)); 1396 else if (name.equals("asNeeded[x]")) 1397 this.asNeeded = (Type) value; // Type 1398 else if (name.equals("orderedOn")) 1399 this.orderedOn = castToDateTime(value); // DateTimeType 1400 else if (name.equals("orderer")) 1401 this.orderer = castToReference(value); // Reference 1402 else if (name.equals("priority")) 1403 this.priority = new ProcedureRequestPriorityEnumFactory().fromType(value); // Enumeration<ProcedureRequestPriority> 1404 else 1405 super.setProperty(name, value); 1406 } 1407 1408 @Override 1409 public Base addChild(String name) throws FHIRException { 1410 if (name.equals("identifier")) { 1411 return addIdentifier(); 1412 } else if (name.equals("subject")) { 1413 this.subject = new Reference(); 1414 return this.subject; 1415 } else if (name.equals("code")) { 1416 this.code = new CodeableConcept(); 1417 return this.code; 1418 } else if (name.equals("bodySite")) { 1419 return addBodySite(); 1420 } else if (name.equals("reasonCodeableConcept")) { 1421 this.reason = new CodeableConcept(); 1422 return this.reason; 1423 } else if (name.equals("reasonReference")) { 1424 this.reason = new Reference(); 1425 return this.reason; 1426 } else if (name.equals("scheduledDateTime")) { 1427 this.scheduled = new DateTimeType(); 1428 return this.scheduled; 1429 } else if (name.equals("scheduledPeriod")) { 1430 this.scheduled = new Period(); 1431 return this.scheduled; 1432 } else if (name.equals("scheduledTiming")) { 1433 this.scheduled = new Timing(); 1434 return this.scheduled; 1435 } else if (name.equals("encounter")) { 1436 this.encounter = new Reference(); 1437 return this.encounter; 1438 } else if (name.equals("performer")) { 1439 this.performer = new Reference(); 1440 return this.performer; 1441 } else if (name.equals("status")) { 1442 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.status"); 1443 } else if (name.equals("notes")) { 1444 return addNotes(); 1445 } else if (name.equals("asNeededBoolean")) { 1446 this.asNeeded = new BooleanType(); 1447 return this.asNeeded; 1448 } else if (name.equals("asNeededCodeableConcept")) { 1449 this.asNeeded = new CodeableConcept(); 1450 return this.asNeeded; 1451 } else if (name.equals("orderedOn")) { 1452 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.orderedOn"); 1453 } else if (name.equals("orderer")) { 1454 this.orderer = new Reference(); 1455 return this.orderer; 1456 } else if (name.equals("priority")) { 1457 throw new FHIRException("Cannot call addChild on a singleton property ProcedureRequest.priority"); 1458 } else 1459 return super.addChild(name); 1460 } 1461 1462 public String fhirType() { 1463 return "ProcedureRequest"; 1464 1465 } 1466 1467 public ProcedureRequest copy() { 1468 ProcedureRequest dst = new ProcedureRequest(); 1469 copyValues(dst); 1470 if (identifier != null) { 1471 dst.identifier = new ArrayList<Identifier>(); 1472 for (Identifier i : identifier) 1473 dst.identifier.add(i.copy()); 1474 } 1475 ; 1476 dst.subject = subject == null ? null : subject.copy(); 1477 dst.code = code == null ? null : code.copy(); 1478 if (bodySite != null) { 1479 dst.bodySite = new ArrayList<CodeableConcept>(); 1480 for (CodeableConcept i : bodySite) 1481 dst.bodySite.add(i.copy()); 1482 } 1483 ; 1484 dst.reason = reason == null ? null : reason.copy(); 1485 dst.scheduled = scheduled == null ? null : scheduled.copy(); 1486 dst.encounter = encounter == null ? null : encounter.copy(); 1487 dst.performer = performer == null ? null : performer.copy(); 1488 dst.status = status == null ? null : status.copy(); 1489 if (notes != null) { 1490 dst.notes = new ArrayList<Annotation>(); 1491 for (Annotation i : notes) 1492 dst.notes.add(i.copy()); 1493 } 1494 ; 1495 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 1496 dst.orderedOn = orderedOn == null ? null : orderedOn.copy(); 1497 dst.orderer = orderer == null ? null : orderer.copy(); 1498 dst.priority = priority == null ? null : priority.copy(); 1499 return dst; 1500 } 1501 1502 protected ProcedureRequest typedCopy() { 1503 return copy(); 1504 } 1505 1506 @Override 1507 public boolean equalsDeep(Base other) { 1508 if (!super.equalsDeep(other)) 1509 return false; 1510 if (!(other instanceof ProcedureRequest)) 1511 return false; 1512 ProcedureRequest o = (ProcedureRequest) other; 1513 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) 1514 && compareDeep(code, o.code, true) && compareDeep(bodySite, o.bodySite, true) 1515 && compareDeep(reason, o.reason, true) && compareDeep(scheduled, o.scheduled, true) 1516 && compareDeep(encounter, o.encounter, true) && compareDeep(performer, o.performer, true) 1517 && compareDeep(status, o.status, true) && compareDeep(notes, o.notes, true) 1518 && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(orderedOn, o.orderedOn, true) 1519 && compareDeep(orderer, o.orderer, true) && compareDeep(priority, o.priority, true); 1520 } 1521 1522 @Override 1523 public boolean equalsShallow(Base other) { 1524 if (!super.equalsShallow(other)) 1525 return false; 1526 if (!(other instanceof ProcedureRequest)) 1527 return false; 1528 ProcedureRequest o = (ProcedureRequest) other; 1529 return compareValues(status, o.status, true) && compareValues(orderedOn, o.orderedOn, true) 1530 && compareValues(priority, o.priority, true); 1531 } 1532 1533 public boolean isEmpty() { 1534 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (subject == null || subject.isEmpty()) 1535 && (code == null || code.isEmpty()) && (bodySite == null || bodySite.isEmpty()) 1536 && (reason == null || reason.isEmpty()) && (scheduled == null || scheduled.isEmpty()) 1537 && (encounter == null || encounter.isEmpty()) && (performer == null || performer.isEmpty()) 1538 && (status == null || status.isEmpty()) && (notes == null || notes.isEmpty()) 1539 && (asNeeded == null || asNeeded.isEmpty()) && (orderedOn == null || orderedOn.isEmpty()) 1540 && (orderer == null || orderer.isEmpty()) && (priority == null || priority.isEmpty()); 1541 } 1542 1543 @Override 1544 public ResourceType getResourceType() { 1545 return ResourceType.ProcedureRequest; 1546 } 1547 1548 @SearchParamDefinition(name = "identifier", path = "ProcedureRequest.identifier", description = "A unique identifier of the Procedure Request", type = "token") 1549 public static final String SP_IDENTIFIER = "identifier"; 1550 @SearchParamDefinition(name = "performer", path = "ProcedureRequest.performer", description = "Who should perform the procedure", type = "reference") 1551 public static final String SP_PERFORMER = "performer"; 1552 @SearchParamDefinition(name = "subject", path = "ProcedureRequest.subject", description = "Search by subject", type = "reference") 1553 public static final String SP_SUBJECT = "subject"; 1554 @SearchParamDefinition(name = "patient", path = "ProcedureRequest.subject", description = "Search by subject - a patient", type = "reference") 1555 public static final String SP_PATIENT = "patient"; 1556 @SearchParamDefinition(name = "orderer", path = "ProcedureRequest.orderer", description = "Who made request", type = "reference") 1557 public static final String SP_ORDERER = "orderer"; 1558 @SearchParamDefinition(name = "encounter", path = "ProcedureRequest.encounter", description = "Encounter request created during", type = "reference") 1559 public static final String SP_ENCOUNTER = "encounter"; 1560 1561}