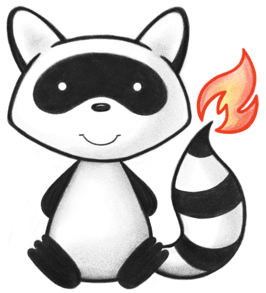
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * This resource provides the target, request and response, and action details 048 * for an action to be performed by the target on or about existing resources. 049 */ 050@ResourceDef(name = "ProcessRequest", profile = "http://hl7.org/fhir/Profile/ProcessRequest") 051public class ProcessRequest extends DomainResource { 052 053 public enum ActionList { 054 /** 055 * Cancel, reverse or nullify the target resource. 056 */ 057 CANCEL, 058 /** 059 * Check for previously un-read/ not-retrieved resources. 060 */ 061 POLL, 062 /** 063 * Re-process the target resource. 064 */ 065 REPROCESS, 066 /** 067 * Retrieve the processing status of the target resource. 068 */ 069 STATUS, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static ActionList fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("cancel".equals(codeString)) 079 return CANCEL; 080 if ("poll".equals(codeString)) 081 return POLL; 082 if ("reprocess".equals(codeString)) 083 return REPROCESS; 084 if ("status".equals(codeString)) 085 return STATUS; 086 throw new FHIRException("Unknown ActionList code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case CANCEL: 092 return "cancel"; 093 case POLL: 094 return "poll"; 095 case REPROCESS: 096 return "reprocess"; 097 case STATUS: 098 return "status"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 switch (this) { 108 case CANCEL: 109 return "http://hl7.org/fhir/actionlist"; 110 case POLL: 111 return "http://hl7.org/fhir/actionlist"; 112 case REPROCESS: 113 return "http://hl7.org/fhir/actionlist"; 114 case STATUS: 115 return "http://hl7.org/fhir/actionlist"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case CANCEL: 126 return "Cancel, reverse or nullify the target resource."; 127 case POLL: 128 return "Check for previously un-read/ not-retrieved resources."; 129 case REPROCESS: 130 return "Re-process the target resource."; 131 case STATUS: 132 return "Retrieve the processing status of the target resource."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case CANCEL: 143 return "Cancel, Reverse or Nullify"; 144 case POLL: 145 return "Poll"; 146 case REPROCESS: 147 return "Re-Process"; 148 case STATUS: 149 return "Status Check"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class ActionListEnumFactory implements EnumFactory<ActionList> { 159 public ActionList fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("cancel".equals(codeString)) 164 return ActionList.CANCEL; 165 if ("poll".equals(codeString)) 166 return ActionList.POLL; 167 if ("reprocess".equals(codeString)) 168 return ActionList.REPROCESS; 169 if ("status".equals(codeString)) 170 return ActionList.STATUS; 171 throw new IllegalArgumentException("Unknown ActionList code '" + codeString + "'"); 172 } 173 174 public Enumeration<ActionList> fromType(Base code) throws FHIRException { 175 if (code == null || code.isEmpty()) 176 return null; 177 String codeString = ((PrimitiveType) code).asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("cancel".equals(codeString)) 181 return new Enumeration<ActionList>(this, ActionList.CANCEL); 182 if ("poll".equals(codeString)) 183 return new Enumeration<ActionList>(this, ActionList.POLL); 184 if ("reprocess".equals(codeString)) 185 return new Enumeration<ActionList>(this, ActionList.REPROCESS); 186 if ("status".equals(codeString)) 187 return new Enumeration<ActionList>(this, ActionList.STATUS); 188 throw new FHIRException("Unknown ActionList code '" + codeString + "'"); 189 } 190 191 public String toCode(ActionList code) 192 { 193 if (code == ActionList.NULL) 194 return null; 195 if (code == ActionList.CANCEL) 196 return "cancel"; 197 if (code == ActionList.POLL) 198 return "poll"; 199 if (code == ActionList.REPROCESS) 200 return "reprocess"; 201 if (code == ActionList.STATUS) 202 return "status"; 203 return "?"; 204 } 205 } 206 207 @Block() 208 public static class ItemsComponent extends BackboneElement implements IBaseBackboneElement { 209 /** 210 * A service line number. 211 */ 212 @Child(name = "sequenceLinkId", type = { 213 IntegerType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 214 @Description(shortDefinition = "Service instance", formalDefinition = "A service line number.") 215 protected IntegerType sequenceLinkId; 216 217 private static final long serialVersionUID = -1598360600L; 218 219 /* 220 * Constructor 221 */ 222 public ItemsComponent() { 223 super(); 224 } 225 226 /* 227 * Constructor 228 */ 229 public ItemsComponent(IntegerType sequenceLinkId) { 230 super(); 231 this.sequenceLinkId = sequenceLinkId; 232 } 233 234 /** 235 * @return {@link #sequenceLinkId} (A service line number.). This is the 236 * underlying object with id, value and extensions. The accessor 237 * "getSequenceLinkId" gives direct access to the value 238 */ 239 public IntegerType getSequenceLinkIdElement() { 240 if (this.sequenceLinkId == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create ItemsComponent.sequenceLinkId"); 243 else if (Configuration.doAutoCreate()) 244 this.sequenceLinkId = new IntegerType(); // bb 245 return this.sequenceLinkId; 246 } 247 248 public boolean hasSequenceLinkIdElement() { 249 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 250 } 251 252 public boolean hasSequenceLinkId() { 253 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 254 } 255 256 /** 257 * @param value {@link #sequenceLinkId} (A service line number.). This is the 258 * underlying object with id, value and extensions. The accessor 259 * "getSequenceLinkId" gives direct access to the value 260 */ 261 public ItemsComponent setSequenceLinkIdElement(IntegerType value) { 262 this.sequenceLinkId = value; 263 return this; 264 } 265 266 /** 267 * @return A service line number. 268 */ 269 public int getSequenceLinkId() { 270 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 271 } 272 273 /** 274 * @param value A service line number. 275 */ 276 public ItemsComponent setSequenceLinkId(int value) { 277 if (this.sequenceLinkId == null) 278 this.sequenceLinkId = new IntegerType(); 279 this.sequenceLinkId.setValue(value); 280 return this; 281 } 282 283 protected void listChildren(List<Property> childrenList) { 284 super.listChildren(childrenList); 285 childrenList.add(new Property("sequenceLinkId", "integer", "A service line number.", 0, 286 java.lang.Integer.MAX_VALUE, sequenceLinkId)); 287 } 288 289 @Override 290 public void setProperty(String name, Base value) throws FHIRException { 291 if (name.equals("sequenceLinkId")) 292 this.sequenceLinkId = castToInteger(value); // IntegerType 293 else 294 super.setProperty(name, value); 295 } 296 297 @Override 298 public Base addChild(String name) throws FHIRException { 299 if (name.equals("sequenceLinkId")) { 300 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.sequenceLinkId"); 301 } else 302 return super.addChild(name); 303 } 304 305 public ItemsComponent copy() { 306 ItemsComponent dst = new ItemsComponent(); 307 copyValues(dst); 308 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 309 return dst; 310 } 311 312 @Override 313 public boolean equalsDeep(Base other) { 314 if (!super.equalsDeep(other)) 315 return false; 316 if (!(other instanceof ItemsComponent)) 317 return false; 318 ItemsComponent o = (ItemsComponent) other; 319 return compareDeep(sequenceLinkId, o.sequenceLinkId, true); 320 } 321 322 @Override 323 public boolean equalsShallow(Base other) { 324 if (!super.equalsShallow(other)) 325 return false; 326 if (!(other instanceof ItemsComponent)) 327 return false; 328 ItemsComponent o = (ItemsComponent) other; 329 return compareValues(sequenceLinkId, o.sequenceLinkId, true); 330 } 331 332 public boolean isEmpty() { 333 return super.isEmpty() && (sequenceLinkId == null || sequenceLinkId.isEmpty()); 334 } 335 336 public String fhirType() { 337 return "ProcessRequest.item"; 338 339 } 340 341 } 342 343 /** 344 * The type of processing action being requested, for example Reversal, 345 * Readjudication, StatusRequest,PendedRequest. 346 */ 347 @Child(name = "action", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 348 @Description(shortDefinition = "cancel | poll | reprocess | status", formalDefinition = "The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest.") 349 protected Enumeration<ActionList> action; 350 351 /** 352 * The ProcessRequest business identifier. 353 */ 354 @Child(name = "identifier", type = { 355 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 356 @Description(shortDefinition = "Business Identifier", formalDefinition = "The ProcessRequest business identifier.") 357 protected List<Identifier> identifier; 358 359 /** 360 * The version of the style of resource contents. This should be mapped to the 361 * allowable profiles for this and supporting resources. 362 */ 363 @Child(name = "ruleset", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 364 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 365 protected Coding ruleset; 366 367 /** 368 * The style (standard) and version of the original material which was converted 369 * into this resource. 370 */ 371 @Child(name = "originalRuleset", type = { 372 Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 373 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 374 protected Coding originalRuleset; 375 376 /** 377 * The date when this resource was created. 378 */ 379 @Child(name = "created", type = { DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 380 @Description(shortDefinition = "Creation date", formalDefinition = "The date when this resource was created.") 381 protected DateTimeType created; 382 383 /** 384 * The organization which is the target of the request. 385 */ 386 @Child(name = "target", type = { Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 387 @Description(shortDefinition = "Target of the request", formalDefinition = "The organization which is the target of the request.") 388 protected Reference target; 389 390 /** 391 * The actual object that is the target of the reference (The organization which 392 * is the target of the request.) 393 */ 394 protected Organization targetTarget; 395 396 /** 397 * The practitioner who is responsible for the action specified in thise 398 * request. 399 */ 400 @Child(name = "provider", type = { 401 Practitioner.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 402 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the action specified in thise request.") 403 protected Reference provider; 404 405 /** 406 * The actual object that is the target of the reference (The practitioner who 407 * is responsible for the action specified in thise request.) 408 */ 409 protected Practitioner providerTarget; 410 411 /** 412 * The organization which is responsible for the action speccified in thise 413 * request. 414 */ 415 @Child(name = "organization", type = { 416 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 417 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the action speccified in thise request.") 418 protected Reference organization; 419 420 /** 421 * The actual object that is the target of the reference (The organization which 422 * is responsible for the action speccified in thise request.) 423 */ 424 protected Organization organizationTarget; 425 426 /** 427 * Reference of resource which is the target or subject of this action. 428 */ 429 @Child(name = "request", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = true) 430 @Description(shortDefinition = "Request reference", formalDefinition = "Reference of resource which is the target or subject of this action.") 431 protected Reference request; 432 433 /** 434 * The actual object that is the target of the reference (Reference of resource 435 * which is the target or subject of this action.) 436 */ 437 protected Resource requestTarget; 438 439 /** 440 * Reference of a prior response to resource which is the target or subject of 441 * this action. 442 */ 443 @Child(name = "response", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = true) 444 @Description(shortDefinition = "Response reference", formalDefinition = "Reference of a prior response to resource which is the target or subject of this action.") 445 protected Reference response; 446 447 /** 448 * The actual object that is the target of the reference (Reference of a prior 449 * response to resource which is the target or subject of this action.) 450 */ 451 protected Resource responseTarget; 452 453 /** 454 * If true remove all history excluding audit. 455 */ 456 @Child(name = "nullify", type = { BooleanType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 457 @Description(shortDefinition = "Nullify", formalDefinition = "If true remove all history excluding audit.") 458 protected BooleanType nullify; 459 460 /** 461 * A reference to supply which authenticates the process. 462 */ 463 @Child(name = "reference", type = { 464 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 465 @Description(shortDefinition = "Reference number/string", formalDefinition = "A reference to supply which authenticates the process.") 466 protected StringType reference; 467 468 /** 469 * List of top level items to be re-adjudicated, if none specified then the 470 * entire submission is re-adjudicated. 471 */ 472 @Child(name = "item", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 473 @Description(shortDefinition = "Items to re-adjudicate", formalDefinition = "List of top level items to be re-adjudicated, if none specified then the entire submission is re-adjudicated.") 474 protected List<ItemsComponent> item; 475 476 /** 477 * Names of resource types to include. 478 */ 479 @Child(name = "include", type = { 480 StringType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 481 @Description(shortDefinition = "Resource type(s) to include", formalDefinition = "Names of resource types to include.") 482 protected List<StringType> include; 483 484 /** 485 * Names of resource types to exclude. 486 */ 487 @Child(name = "exclude", type = { 488 StringType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 489 @Description(shortDefinition = "Resource type(s) to exclude", formalDefinition = "Names of resource types to exclude.") 490 protected List<StringType> exclude; 491 492 /** 493 * A period of time during which the fulfilling resources would have been 494 * created. 495 */ 496 @Child(name = "period", type = { Period.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 497 @Description(shortDefinition = "Period", formalDefinition = "A period of time during which the fulfilling resources would have been created.") 498 protected Period period; 499 500 private static final long serialVersionUID = -1332331220L; 501 502 /* 503 * Constructor 504 */ 505 public ProcessRequest() { 506 super(); 507 } 508 509 /* 510 * Constructor 511 */ 512 public ProcessRequest(Enumeration<ActionList> action) { 513 super(); 514 this.action = action; 515 } 516 517 /** 518 * @return {@link #action} (The type of processing action being requested, for 519 * example Reversal, Readjudication, StatusRequest,PendedRequest.). This 520 * is the underlying object with id, value and extensions. The accessor 521 * "getAction" gives direct access to the value 522 */ 523 public Enumeration<ActionList> getActionElement() { 524 if (this.action == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create ProcessRequest.action"); 527 else if (Configuration.doAutoCreate()) 528 this.action = new Enumeration<ActionList>(new ActionListEnumFactory()); // bb 529 return this.action; 530 } 531 532 public boolean hasActionElement() { 533 return this.action != null && !this.action.isEmpty(); 534 } 535 536 public boolean hasAction() { 537 return this.action != null && !this.action.isEmpty(); 538 } 539 540 /** 541 * @param value {@link #action} (The type of processing action being requested, 542 * for example Reversal, Readjudication, 543 * StatusRequest,PendedRequest.). This is the underlying object 544 * with id, value and extensions. The accessor "getAction" gives 545 * direct access to the value 546 */ 547 public ProcessRequest setActionElement(Enumeration<ActionList> value) { 548 this.action = value; 549 return this; 550 } 551 552 /** 553 * @return The type of processing action being requested, for example Reversal, 554 * Readjudication, StatusRequest,PendedRequest. 555 */ 556 public ActionList getAction() { 557 return this.action == null ? null : this.action.getValue(); 558 } 559 560 /** 561 * @param value The type of processing action being requested, for example 562 * Reversal, Readjudication, StatusRequest,PendedRequest. 563 */ 564 public ProcessRequest setAction(ActionList value) { 565 if (this.action == null) 566 this.action = new Enumeration<ActionList>(new ActionListEnumFactory()); 567 this.action.setValue(value); 568 return this; 569 } 570 571 /** 572 * @return {@link #identifier} (The ProcessRequest business identifier.) 573 */ 574 public List<Identifier> getIdentifier() { 575 if (this.identifier == null) 576 this.identifier = new ArrayList<Identifier>(); 577 return this.identifier; 578 } 579 580 public boolean hasIdentifier() { 581 if (this.identifier == null) 582 return false; 583 for (Identifier item : this.identifier) 584 if (!item.isEmpty()) 585 return true; 586 return false; 587 } 588 589 /** 590 * @return {@link #identifier} (The ProcessRequest business identifier.) 591 */ 592 // syntactic sugar 593 public Identifier addIdentifier() { // 3 594 Identifier t = new Identifier(); 595 if (this.identifier == null) 596 this.identifier = new ArrayList<Identifier>(); 597 this.identifier.add(t); 598 return t; 599 } 600 601 // syntactic sugar 602 public ProcessRequest addIdentifier(Identifier t) { // 3 603 if (t == null) 604 return this; 605 if (this.identifier == null) 606 this.identifier = new ArrayList<Identifier>(); 607 this.identifier.add(t); 608 return this; 609 } 610 611 /** 612 * @return {@link #ruleset} (The version of the style of resource contents. This 613 * should be mapped to the allowable profiles for this and supporting 614 * resources.) 615 */ 616 public Coding getRuleset() { 617 if (this.ruleset == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create ProcessRequest.ruleset"); 620 else if (Configuration.doAutoCreate()) 621 this.ruleset = new Coding(); // cc 622 return this.ruleset; 623 } 624 625 public boolean hasRuleset() { 626 return this.ruleset != null && !this.ruleset.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #ruleset} (The version of the style of resource contents. 631 * This should be mapped to the allowable profiles for this and 632 * supporting resources.) 633 */ 634 public ProcessRequest setRuleset(Coding value) { 635 this.ruleset = value; 636 return this; 637 } 638 639 /** 640 * @return {@link #originalRuleset} (The style (standard) and version of the 641 * original material which was converted into this resource.) 642 */ 643 public Coding getOriginalRuleset() { 644 if (this.originalRuleset == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create ProcessRequest.originalRuleset"); 647 else if (Configuration.doAutoCreate()) 648 this.originalRuleset = new Coding(); // cc 649 return this.originalRuleset; 650 } 651 652 public boolean hasOriginalRuleset() { 653 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 654 } 655 656 /** 657 * @param value {@link #originalRuleset} (The style (standard) and version of 658 * the original material which was converted into this resource.) 659 */ 660 public ProcessRequest setOriginalRuleset(Coding value) { 661 this.originalRuleset = value; 662 return this; 663 } 664 665 /** 666 * @return {@link #created} (The date when this resource was created.). This is 667 * the underlying object with id, value and extensions. The accessor 668 * "getCreated" gives direct access to the value 669 */ 670 public DateTimeType getCreatedElement() { 671 if (this.created == null) 672 if (Configuration.errorOnAutoCreate()) 673 throw new Error("Attempt to auto-create ProcessRequest.created"); 674 else if (Configuration.doAutoCreate()) 675 this.created = new DateTimeType(); // bb 676 return this.created; 677 } 678 679 public boolean hasCreatedElement() { 680 return this.created != null && !this.created.isEmpty(); 681 } 682 683 public boolean hasCreated() { 684 return this.created != null && !this.created.isEmpty(); 685 } 686 687 /** 688 * @param value {@link #created} (The date when this resource was created.). 689 * This is the underlying object with id, value and extensions. The 690 * accessor "getCreated" gives direct access to the value 691 */ 692 public ProcessRequest setCreatedElement(DateTimeType value) { 693 this.created = value; 694 return this; 695 } 696 697 /** 698 * @return The date when this resource was created. 699 */ 700 public Date getCreated() { 701 return this.created == null ? null : this.created.getValue(); 702 } 703 704 /** 705 * @param value The date when this resource was created. 706 */ 707 public ProcessRequest setCreated(Date value) { 708 if (value == null) 709 this.created = null; 710 else { 711 if (this.created == null) 712 this.created = new DateTimeType(); 713 this.created.setValue(value); 714 } 715 return this; 716 } 717 718 /** 719 * @return {@link #target} (The organization which is the target of the 720 * request.) 721 */ 722 public Reference getTarget() { 723 if (this.target == null) 724 if (Configuration.errorOnAutoCreate()) 725 throw new Error("Attempt to auto-create ProcessRequest.target"); 726 else if (Configuration.doAutoCreate()) 727 this.target = new Reference(); // cc 728 return this.target; 729 } 730 731 public boolean hasTarget() { 732 return this.target != null && !this.target.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #target} (The organization which is the target of the 737 * request.) 738 */ 739 public ProcessRequest setTarget(Reference value) { 740 this.target = value; 741 return this; 742 } 743 744 /** 745 * @return {@link #target} The actual object that is the target of the 746 * reference. The reference library doesn't populate this, but you can 747 * use it to hold the resource if you resolve it. (The organization 748 * which is the target of the request.) 749 */ 750 public Organization getTargetTarget() { 751 if (this.targetTarget == null) 752 if (Configuration.errorOnAutoCreate()) 753 throw new Error("Attempt to auto-create ProcessRequest.target"); 754 else if (Configuration.doAutoCreate()) 755 this.targetTarget = new Organization(); // aa 756 return this.targetTarget; 757 } 758 759 /** 760 * @param value {@link #target} The actual object that is the target of the 761 * reference. The reference library doesn't use these, but you can 762 * use it to hold the resource if you resolve it. (The organization 763 * which is the target of the request.) 764 */ 765 public ProcessRequest setTargetTarget(Organization value) { 766 this.targetTarget = value; 767 return this; 768 } 769 770 /** 771 * @return {@link #provider} (The practitioner who is responsible for the action 772 * specified in thise request.) 773 */ 774 public Reference getProvider() { 775 if (this.provider == null) 776 if (Configuration.errorOnAutoCreate()) 777 throw new Error("Attempt to auto-create ProcessRequest.provider"); 778 else if (Configuration.doAutoCreate()) 779 this.provider = new Reference(); // cc 780 return this.provider; 781 } 782 783 public boolean hasProvider() { 784 return this.provider != null && !this.provider.isEmpty(); 785 } 786 787 /** 788 * @param value {@link #provider} (The practitioner who is responsible for the 789 * action specified in thise request.) 790 */ 791 public ProcessRequest setProvider(Reference value) { 792 this.provider = value; 793 return this; 794 } 795 796 /** 797 * @return {@link #provider} The actual object that is the target of the 798 * reference. The reference library doesn't populate this, but you can 799 * use it to hold the resource if you resolve it. (The practitioner who 800 * is responsible for the action specified in thise request.) 801 */ 802 public Practitioner getProviderTarget() { 803 if (this.providerTarget == null) 804 if (Configuration.errorOnAutoCreate()) 805 throw new Error("Attempt to auto-create ProcessRequest.provider"); 806 else if (Configuration.doAutoCreate()) 807 this.providerTarget = new Practitioner(); // aa 808 return this.providerTarget; 809 } 810 811 /** 812 * @param value {@link #provider} The actual object that is the target of the 813 * reference. The reference library doesn't use these, but you can 814 * use it to hold the resource if you resolve it. (The practitioner 815 * who is responsible for the action specified in thise request.) 816 */ 817 public ProcessRequest setProviderTarget(Practitioner value) { 818 this.providerTarget = value; 819 return this; 820 } 821 822 /** 823 * @return {@link #organization} (The organization which is responsible for the 824 * action speccified in thise request.) 825 */ 826 public Reference getOrganization() { 827 if (this.organization == null) 828 if (Configuration.errorOnAutoCreate()) 829 throw new Error("Attempt to auto-create ProcessRequest.organization"); 830 else if (Configuration.doAutoCreate()) 831 this.organization = new Reference(); // cc 832 return this.organization; 833 } 834 835 public boolean hasOrganization() { 836 return this.organization != null && !this.organization.isEmpty(); 837 } 838 839 /** 840 * @param value {@link #organization} (The organization which is responsible for 841 * the action speccified in thise request.) 842 */ 843 public ProcessRequest setOrganization(Reference value) { 844 this.organization = value; 845 return this; 846 } 847 848 /** 849 * @return {@link #organization} The actual object that is the target of the 850 * reference. The reference library doesn't populate this, but you can 851 * use it to hold the resource if you resolve it. (The organization 852 * which is responsible for the action speccified in thise request.) 853 */ 854 public Organization getOrganizationTarget() { 855 if (this.organizationTarget == null) 856 if (Configuration.errorOnAutoCreate()) 857 throw new Error("Attempt to auto-create ProcessRequest.organization"); 858 else if (Configuration.doAutoCreate()) 859 this.organizationTarget = new Organization(); // aa 860 return this.organizationTarget; 861 } 862 863 /** 864 * @param value {@link #organization} The actual object that is the target of 865 * the reference. The reference library doesn't use these, but you 866 * can use it to hold the resource if you resolve it. (The 867 * organization which is responsible for the action speccified in 868 * thise request.) 869 */ 870 public ProcessRequest setOrganizationTarget(Organization value) { 871 this.organizationTarget = value; 872 return this; 873 } 874 875 /** 876 * @return {@link #request} (Reference of resource which is the target or 877 * subject of this action.) 878 */ 879 public Reference getRequest() { 880 if (this.request == null) 881 if (Configuration.errorOnAutoCreate()) 882 throw new Error("Attempt to auto-create ProcessRequest.request"); 883 else if (Configuration.doAutoCreate()) 884 this.request = new Reference(); // cc 885 return this.request; 886 } 887 888 public boolean hasRequest() { 889 return this.request != null && !this.request.isEmpty(); 890 } 891 892 /** 893 * @param value {@link #request} (Reference of resource which is the target or 894 * subject of this action.) 895 */ 896 public ProcessRequest setRequest(Reference value) { 897 this.request = value; 898 return this; 899 } 900 901 /** 902 * @return {@link #request} The actual object that is the target of the 903 * reference. The reference library doesn't populate this, but you can 904 * use it to hold the resource if you resolve it. (Reference of resource 905 * which is the target or subject of this action.) 906 */ 907 public Resource getRequestTarget() { 908 return this.requestTarget; 909 } 910 911 /** 912 * @param value {@link #request} The actual object that is the target of the 913 * reference. The reference library doesn't use these, but you can 914 * use it to hold the resource if you resolve it. (Reference of 915 * resource which is the target or subject of this action.) 916 */ 917 public ProcessRequest setRequestTarget(Resource value) { 918 this.requestTarget = value; 919 return this; 920 } 921 922 /** 923 * @return {@link #response} (Reference of a prior response to resource which is 924 * the target or subject of this action.) 925 */ 926 public Reference getResponse() { 927 if (this.response == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create ProcessRequest.response"); 930 else if (Configuration.doAutoCreate()) 931 this.response = new Reference(); // cc 932 return this.response; 933 } 934 935 public boolean hasResponse() { 936 return this.response != null && !this.response.isEmpty(); 937 } 938 939 /** 940 * @param value {@link #response} (Reference of a prior response to resource 941 * which is the target or subject of this action.) 942 */ 943 public ProcessRequest setResponse(Reference value) { 944 this.response = value; 945 return this; 946 } 947 948 /** 949 * @return {@link #response} The actual object that is the target of the 950 * reference. The reference library doesn't populate this, but you can 951 * use it to hold the resource if you resolve it. (Reference of a prior 952 * response to resource which is the target or subject of this action.) 953 */ 954 public Resource getResponseTarget() { 955 return this.responseTarget; 956 } 957 958 /** 959 * @param value {@link #response} The actual object that is the target of the 960 * reference. The reference library doesn't use these, but you can 961 * use it to hold the resource if you resolve it. (Reference of a 962 * prior response to resource which is the target or subject of 963 * this action.) 964 */ 965 public ProcessRequest setResponseTarget(Resource value) { 966 this.responseTarget = value; 967 return this; 968 } 969 970 /** 971 * @return {@link #nullify} (If true remove all history excluding audit.). This 972 * is the underlying object with id, value and extensions. The accessor 973 * "getNullify" gives direct access to the value 974 */ 975 public BooleanType getNullifyElement() { 976 if (this.nullify == null) 977 if (Configuration.errorOnAutoCreate()) 978 throw new Error("Attempt to auto-create ProcessRequest.nullify"); 979 else if (Configuration.doAutoCreate()) 980 this.nullify = new BooleanType(); // bb 981 return this.nullify; 982 } 983 984 public boolean hasNullifyElement() { 985 return this.nullify != null && !this.nullify.isEmpty(); 986 } 987 988 public boolean hasNullify() { 989 return this.nullify != null && !this.nullify.isEmpty(); 990 } 991 992 /** 993 * @param value {@link #nullify} (If true remove all history excluding audit.). 994 * This is the underlying object with id, value and extensions. The 995 * accessor "getNullify" gives direct access to the value 996 */ 997 public ProcessRequest setNullifyElement(BooleanType value) { 998 this.nullify = value; 999 return this; 1000 } 1001 1002 /** 1003 * @return If true remove all history excluding audit. 1004 */ 1005 public boolean getNullify() { 1006 return this.nullify == null || this.nullify.isEmpty() ? false : this.nullify.getValue(); 1007 } 1008 1009 /** 1010 * @param value If true remove all history excluding audit. 1011 */ 1012 public ProcessRequest setNullify(boolean value) { 1013 if (this.nullify == null) 1014 this.nullify = new BooleanType(); 1015 this.nullify.setValue(value); 1016 return this; 1017 } 1018 1019 /** 1020 * @return {@link #reference} (A reference to supply which authenticates the 1021 * process.). This is the underlying object with id, value and 1022 * extensions. The accessor "getReference" gives direct access to the 1023 * value 1024 */ 1025 public StringType getReferenceElement() { 1026 if (this.reference == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create ProcessRequest.reference"); 1029 else if (Configuration.doAutoCreate()) 1030 this.reference = new StringType(); // bb 1031 return this.reference; 1032 } 1033 1034 public boolean hasReferenceElement() { 1035 return this.reference != null && !this.reference.isEmpty(); 1036 } 1037 1038 public boolean hasReference() { 1039 return this.reference != null && !this.reference.isEmpty(); 1040 } 1041 1042 /** 1043 * @param value {@link #reference} (A reference to supply which authenticates 1044 * the process.). This is the underlying object with id, value and 1045 * extensions. The accessor "getReference" gives direct access to 1046 * the value 1047 */ 1048 public ProcessRequest setReferenceElement(StringType value) { 1049 this.reference = value; 1050 return this; 1051 } 1052 1053 /** 1054 * @return A reference to supply which authenticates the process. 1055 */ 1056 public String getReference() { 1057 return this.reference == null ? null : this.reference.getValue(); 1058 } 1059 1060 /** 1061 * @param value A reference to supply which authenticates the process. 1062 */ 1063 public ProcessRequest setReference(String value) { 1064 if (Utilities.noString(value)) 1065 this.reference = null; 1066 else { 1067 if (this.reference == null) 1068 this.reference = new StringType(); 1069 this.reference.setValue(value); 1070 } 1071 return this; 1072 } 1073 1074 /** 1075 * @return {@link #item} (List of top level items to be re-adjudicated, if none 1076 * specified then the entire submission is re-adjudicated.) 1077 */ 1078 public List<ItemsComponent> getItem() { 1079 if (this.item == null) 1080 this.item = new ArrayList<ItemsComponent>(); 1081 return this.item; 1082 } 1083 1084 public boolean hasItem() { 1085 if (this.item == null) 1086 return false; 1087 for (ItemsComponent item : this.item) 1088 if (!item.isEmpty()) 1089 return true; 1090 return false; 1091 } 1092 1093 /** 1094 * @return {@link #item} (List of top level items to be re-adjudicated, if none 1095 * specified then the entire submission is re-adjudicated.) 1096 */ 1097 // syntactic sugar 1098 public ItemsComponent addItem() { // 3 1099 ItemsComponent t = new ItemsComponent(); 1100 if (this.item == null) 1101 this.item = new ArrayList<ItemsComponent>(); 1102 this.item.add(t); 1103 return t; 1104 } 1105 1106 // syntactic sugar 1107 public ProcessRequest addItem(ItemsComponent t) { // 3 1108 if (t == null) 1109 return this; 1110 if (this.item == null) 1111 this.item = new ArrayList<ItemsComponent>(); 1112 this.item.add(t); 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #include} (Names of resource types to include.) 1118 */ 1119 public List<StringType> getInclude() { 1120 if (this.include == null) 1121 this.include = new ArrayList<StringType>(); 1122 return this.include; 1123 } 1124 1125 public boolean hasInclude() { 1126 if (this.include == null) 1127 return false; 1128 for (StringType item : this.include) 1129 if (!item.isEmpty()) 1130 return true; 1131 return false; 1132 } 1133 1134 /** 1135 * @return {@link #include} (Names of resource types to include.) 1136 */ 1137 // syntactic sugar 1138 public StringType addIncludeElement() {// 2 1139 StringType t = new StringType(); 1140 if (this.include == null) 1141 this.include = new ArrayList<StringType>(); 1142 this.include.add(t); 1143 return t; 1144 } 1145 1146 /** 1147 * @param value {@link #include} (Names of resource types to include.) 1148 */ 1149 public ProcessRequest addInclude(String value) { // 1 1150 StringType t = new StringType(); 1151 t.setValue(value); 1152 if (this.include == null) 1153 this.include = new ArrayList<StringType>(); 1154 this.include.add(t); 1155 return this; 1156 } 1157 1158 /** 1159 * @param value {@link #include} (Names of resource types to include.) 1160 */ 1161 public boolean hasInclude(String value) { 1162 if (this.include == null) 1163 return false; 1164 for (StringType v : this.include) 1165 if (v.equals(value)) // string 1166 return true; 1167 return false; 1168 } 1169 1170 /** 1171 * @return {@link #exclude} (Names of resource types to exclude.) 1172 */ 1173 public List<StringType> getExclude() { 1174 if (this.exclude == null) 1175 this.exclude = new ArrayList<StringType>(); 1176 return this.exclude; 1177 } 1178 1179 public boolean hasExclude() { 1180 if (this.exclude == null) 1181 return false; 1182 for (StringType item : this.exclude) 1183 if (!item.isEmpty()) 1184 return true; 1185 return false; 1186 } 1187 1188 /** 1189 * @return {@link #exclude} (Names of resource types to exclude.) 1190 */ 1191 // syntactic sugar 1192 public StringType addExcludeElement() {// 2 1193 StringType t = new StringType(); 1194 if (this.exclude == null) 1195 this.exclude = new ArrayList<StringType>(); 1196 this.exclude.add(t); 1197 return t; 1198 } 1199 1200 /** 1201 * @param value {@link #exclude} (Names of resource types to exclude.) 1202 */ 1203 public ProcessRequest addExclude(String value) { // 1 1204 StringType t = new StringType(); 1205 t.setValue(value); 1206 if (this.exclude == null) 1207 this.exclude = new ArrayList<StringType>(); 1208 this.exclude.add(t); 1209 return this; 1210 } 1211 1212 /** 1213 * @param value {@link #exclude} (Names of resource types to exclude.) 1214 */ 1215 public boolean hasExclude(String value) { 1216 if (this.exclude == null) 1217 return false; 1218 for (StringType v : this.exclude) 1219 if (v.equals(value)) // string 1220 return true; 1221 return false; 1222 } 1223 1224 /** 1225 * @return {@link #period} (A period of time during which the fulfilling 1226 * resources would have been created.) 1227 */ 1228 public Period getPeriod() { 1229 if (this.period == null) 1230 if (Configuration.errorOnAutoCreate()) 1231 throw new Error("Attempt to auto-create ProcessRequest.period"); 1232 else if (Configuration.doAutoCreate()) 1233 this.period = new Period(); // cc 1234 return this.period; 1235 } 1236 1237 public boolean hasPeriod() { 1238 return this.period != null && !this.period.isEmpty(); 1239 } 1240 1241 /** 1242 * @param value {@link #period} (A period of time during which the fulfilling 1243 * resources would have been created.) 1244 */ 1245 public ProcessRequest setPeriod(Period value) { 1246 this.period = value; 1247 return this; 1248 } 1249 1250 protected void listChildren(List<Property> childrenList) { 1251 super.listChildren(childrenList); 1252 childrenList.add(new Property("action", "code", 1253 "The type of processing action being requested, for example Reversal, Readjudication, StatusRequest,PendedRequest.", 1254 0, java.lang.Integer.MAX_VALUE, action)); 1255 childrenList.add(new Property("identifier", "Identifier", "The ProcessRequest business identifier.", 0, 1256 java.lang.Integer.MAX_VALUE, identifier)); 1257 childrenList.add(new Property("ruleset", "Coding", 1258 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 1259 0, java.lang.Integer.MAX_VALUE, ruleset)); 1260 childrenList.add(new Property("originalRuleset", "Coding", 1261 "The style (standard) and version of the original material which was converted into this resource.", 0, 1262 java.lang.Integer.MAX_VALUE, originalRuleset)); 1263 childrenList.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1264 java.lang.Integer.MAX_VALUE, created)); 1265 childrenList.add(new Property("target", "Reference(Organization)", 1266 "The organization which is the target of the request.", 0, java.lang.Integer.MAX_VALUE, target)); 1267 childrenList.add(new Property("provider", "Reference(Practitioner)", 1268 "The practitioner who is responsible for the action specified in thise request.", 0, 1269 java.lang.Integer.MAX_VALUE, provider)); 1270 childrenList.add(new Property("organization", "Reference(Organization)", 1271 "The organization which is responsible for the action speccified in thise request.", 0, 1272 java.lang.Integer.MAX_VALUE, organization)); 1273 childrenList.add(new Property("request", "Reference(Any)", 1274 "Reference of resource which is the target or subject of this action.", 0, java.lang.Integer.MAX_VALUE, 1275 request)); 1276 childrenList.add(new Property("response", "Reference(Any)", 1277 "Reference of a prior response to resource which is the target or subject of this action.", 0, 1278 java.lang.Integer.MAX_VALUE, response)); 1279 childrenList.add(new Property("nullify", "boolean", "If true remove all history excluding audit.", 0, 1280 java.lang.Integer.MAX_VALUE, nullify)); 1281 childrenList.add(new Property("reference", "string", "A reference to supply which authenticates the process.", 0, 1282 java.lang.Integer.MAX_VALUE, reference)); 1283 childrenList.add(new Property("item", "", 1284 "List of top level items to be re-adjudicated, if none specified then the entire submission is re-adjudicated.", 1285 0, java.lang.Integer.MAX_VALUE, item)); 1286 childrenList.add(new Property("include", "string", "Names of resource types to include.", 0, 1287 java.lang.Integer.MAX_VALUE, include)); 1288 childrenList.add(new Property("exclude", "string", "Names of resource types to exclude.", 0, 1289 java.lang.Integer.MAX_VALUE, exclude)); 1290 childrenList.add(new Property("period", "Period", 1291 "A period of time during which the fulfilling resources would have been created.", 0, 1292 java.lang.Integer.MAX_VALUE, period)); 1293 } 1294 1295 @Override 1296 public void setProperty(String name, Base value) throws FHIRException { 1297 if (name.equals("action")) 1298 this.action = new ActionListEnumFactory().fromType(value); // Enumeration<ActionList> 1299 else if (name.equals("identifier")) 1300 this.getIdentifier().add(castToIdentifier(value)); 1301 else if (name.equals("ruleset")) 1302 this.ruleset = castToCoding(value); // Coding 1303 else if (name.equals("originalRuleset")) 1304 this.originalRuleset = castToCoding(value); // Coding 1305 else if (name.equals("created")) 1306 this.created = castToDateTime(value); // DateTimeType 1307 else if (name.equals("target")) 1308 this.target = castToReference(value); // Reference 1309 else if (name.equals("provider")) 1310 this.provider = castToReference(value); // Reference 1311 else if (name.equals("organization")) 1312 this.organization = castToReference(value); // Reference 1313 else if (name.equals("request")) 1314 this.request = castToReference(value); // Reference 1315 else if (name.equals("response")) 1316 this.response = castToReference(value); // Reference 1317 else if (name.equals("nullify")) 1318 this.nullify = castToBoolean(value); // BooleanType 1319 else if (name.equals("reference")) 1320 this.reference = castToString(value); // StringType 1321 else if (name.equals("item")) 1322 this.getItem().add((ItemsComponent) value); 1323 else if (name.equals("include")) 1324 this.getInclude().add(castToString(value)); 1325 else if (name.equals("exclude")) 1326 this.getExclude().add(castToString(value)); 1327 else if (name.equals("period")) 1328 this.period = castToPeriod(value); // Period 1329 else 1330 super.setProperty(name, value); 1331 } 1332 1333 @Override 1334 public Base addChild(String name) throws FHIRException { 1335 if (name.equals("action")) { 1336 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.action"); 1337 } else if (name.equals("identifier")) { 1338 return addIdentifier(); 1339 } else if (name.equals("ruleset")) { 1340 this.ruleset = new Coding(); 1341 return this.ruleset; 1342 } else if (name.equals("originalRuleset")) { 1343 this.originalRuleset = new Coding(); 1344 return this.originalRuleset; 1345 } else if (name.equals("created")) { 1346 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.created"); 1347 } else if (name.equals("target")) { 1348 this.target = new Reference(); 1349 return this.target; 1350 } else if (name.equals("provider")) { 1351 this.provider = new Reference(); 1352 return this.provider; 1353 } else if (name.equals("organization")) { 1354 this.organization = new Reference(); 1355 return this.organization; 1356 } else if (name.equals("request")) { 1357 this.request = new Reference(); 1358 return this.request; 1359 } else if (name.equals("response")) { 1360 this.response = new Reference(); 1361 return this.response; 1362 } else if (name.equals("nullify")) { 1363 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.nullify"); 1364 } else if (name.equals("reference")) { 1365 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.reference"); 1366 } else if (name.equals("item")) { 1367 return addItem(); 1368 } else if (name.equals("include")) { 1369 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.include"); 1370 } else if (name.equals("exclude")) { 1371 throw new FHIRException("Cannot call addChild on a singleton property ProcessRequest.exclude"); 1372 } else if (name.equals("period")) { 1373 this.period = new Period(); 1374 return this.period; 1375 } else 1376 return super.addChild(name); 1377 } 1378 1379 public String fhirType() { 1380 return "ProcessRequest"; 1381 1382 } 1383 1384 public ProcessRequest copy() { 1385 ProcessRequest dst = new ProcessRequest(); 1386 copyValues(dst); 1387 dst.action = action == null ? null : action.copy(); 1388 if (identifier != null) { 1389 dst.identifier = new ArrayList<Identifier>(); 1390 for (Identifier i : identifier) 1391 dst.identifier.add(i.copy()); 1392 } 1393 ; 1394 dst.ruleset = ruleset == null ? null : ruleset.copy(); 1395 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 1396 dst.created = created == null ? null : created.copy(); 1397 dst.target = target == null ? null : target.copy(); 1398 dst.provider = provider == null ? null : provider.copy(); 1399 dst.organization = organization == null ? null : organization.copy(); 1400 dst.request = request == null ? null : request.copy(); 1401 dst.response = response == null ? null : response.copy(); 1402 dst.nullify = nullify == null ? null : nullify.copy(); 1403 dst.reference = reference == null ? null : reference.copy(); 1404 if (item != null) { 1405 dst.item = new ArrayList<ItemsComponent>(); 1406 for (ItemsComponent i : item) 1407 dst.item.add(i.copy()); 1408 } 1409 ; 1410 if (include != null) { 1411 dst.include = new ArrayList<StringType>(); 1412 for (StringType i : include) 1413 dst.include.add(i.copy()); 1414 } 1415 ; 1416 if (exclude != null) { 1417 dst.exclude = new ArrayList<StringType>(); 1418 for (StringType i : exclude) 1419 dst.exclude.add(i.copy()); 1420 } 1421 ; 1422 dst.period = period == null ? null : period.copy(); 1423 return dst; 1424 } 1425 1426 protected ProcessRequest typedCopy() { 1427 return copy(); 1428 } 1429 1430 @Override 1431 public boolean equalsDeep(Base other) { 1432 if (!super.equalsDeep(other)) 1433 return false; 1434 if (!(other instanceof ProcessRequest)) 1435 return false; 1436 ProcessRequest o = (ProcessRequest) other; 1437 return compareDeep(action, o.action, true) && compareDeep(identifier, o.identifier, true) 1438 && compareDeep(ruleset, o.ruleset, true) && compareDeep(originalRuleset, o.originalRuleset, true) 1439 && compareDeep(created, o.created, true) && compareDeep(target, o.target, true) 1440 && compareDeep(provider, o.provider, true) && compareDeep(organization, o.organization, true) 1441 && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 1442 && compareDeep(nullify, o.nullify, true) && compareDeep(reference, o.reference, true) 1443 && compareDeep(item, o.item, true) && compareDeep(include, o.include, true) 1444 && compareDeep(exclude, o.exclude, true) && compareDeep(period, o.period, true); 1445 } 1446 1447 @Override 1448 public boolean equalsShallow(Base other) { 1449 if (!super.equalsShallow(other)) 1450 return false; 1451 if (!(other instanceof ProcessRequest)) 1452 return false; 1453 ProcessRequest o = (ProcessRequest) other; 1454 return compareValues(action, o.action, true) && compareValues(created, o.created, true) 1455 && compareValues(nullify, o.nullify, true) && compareValues(reference, o.reference, true) 1456 && compareValues(include, o.include, true) && compareValues(exclude, o.exclude, true); 1457 } 1458 1459 public boolean isEmpty() { 1460 return super.isEmpty() && (action == null || action.isEmpty()) && (identifier == null || identifier.isEmpty()) 1461 && (ruleset == null || ruleset.isEmpty()) && (originalRuleset == null || originalRuleset.isEmpty()) 1462 && (created == null || created.isEmpty()) && (target == null || target.isEmpty()) 1463 && (provider == null || provider.isEmpty()) && (organization == null || organization.isEmpty()) 1464 && (request == null || request.isEmpty()) && (response == null || response.isEmpty()) 1465 && (nullify == null || nullify.isEmpty()) && (reference == null || reference.isEmpty()) 1466 && (item == null || item.isEmpty()) && (include == null || include.isEmpty()) 1467 && (exclude == null || exclude.isEmpty()) && (period == null || period.isEmpty()); 1468 } 1469 1470 @Override 1471 public ResourceType getResourceType() { 1472 return ResourceType.ProcessRequest; 1473 } 1474 1475 @SearchParamDefinition(name = "identifier", path = "ProcessRequest.identifier", description = "The business identifier of the ProcessRequest", type = "token") 1476 public static final String SP_IDENTIFIER = "identifier"; 1477 @SearchParamDefinition(name = "provider", path = "ProcessRequest.provider", description = "The provider who regenerated this request", type = "reference") 1478 public static final String SP_PROVIDER = "provider"; 1479 @SearchParamDefinition(name = "organization", path = "ProcessRequest.organization", description = "The organization who generated this request", type = "reference") 1480 public static final String SP_ORGANIZATION = "organization"; 1481 @SearchParamDefinition(name = "action", path = "ProcessRequest.action", description = "The action requested by this resource", type = "token") 1482 public static final String SP_ACTION = "action"; 1483 1484}