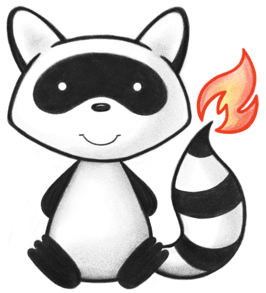
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * This resource provides processing status, errors and notes from the 048 * processing of a resource. 049 */ 050@ResourceDef(name = "ProcessResponse", profile = "http://hl7.org/fhir/Profile/ProcessResponse") 051public class ProcessResponse extends DomainResource { 052 053 @Block() 054 public static class ProcessResponseNotesComponent extends BackboneElement implements IBaseBackboneElement { 055 /** 056 * The note purpose: Print/Display. 057 */ 058 @Child(name = "type", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "display | print | printoper", formalDefinition = "The note purpose: Print/Display.") 060 protected Coding type; 061 062 /** 063 * The note text. 064 */ 065 @Child(name = "text", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 066 @Description(shortDefinition = "Notes text", formalDefinition = "The note text.") 067 protected StringType text; 068 069 private static final long serialVersionUID = 129959202L; 070 071 /* 072 * Constructor 073 */ 074 public ProcessResponseNotesComponent() { 075 super(); 076 } 077 078 /** 079 * @return {@link #type} (The note purpose: Print/Display.) 080 */ 081 public Coding getType() { 082 if (this.type == null) 083 if (Configuration.errorOnAutoCreate()) 084 throw new Error("Attempt to auto-create ProcessResponseNotesComponent.type"); 085 else if (Configuration.doAutoCreate()) 086 this.type = new Coding(); // cc 087 return this.type; 088 } 089 090 public boolean hasType() { 091 return this.type != null && !this.type.isEmpty(); 092 } 093 094 /** 095 * @param value {@link #type} (The note purpose: Print/Display.) 096 */ 097 public ProcessResponseNotesComponent setType(Coding value) { 098 this.type = value; 099 return this; 100 } 101 102 /** 103 * @return {@link #text} (The note text.). This is the underlying object with 104 * id, value and extensions. The accessor "getText" gives direct access 105 * to the value 106 */ 107 public StringType getTextElement() { 108 if (this.text == null) 109 if (Configuration.errorOnAutoCreate()) 110 throw new Error("Attempt to auto-create ProcessResponseNotesComponent.text"); 111 else if (Configuration.doAutoCreate()) 112 this.text = new StringType(); // bb 113 return this.text; 114 } 115 116 public boolean hasTextElement() { 117 return this.text != null && !this.text.isEmpty(); 118 } 119 120 public boolean hasText() { 121 return this.text != null && !this.text.isEmpty(); 122 } 123 124 /** 125 * @param value {@link #text} (The note text.). This is the underlying object 126 * with id, value and extensions. The accessor "getText" gives 127 * direct access to the value 128 */ 129 public ProcessResponseNotesComponent setTextElement(StringType value) { 130 this.text = value; 131 return this; 132 } 133 134 /** 135 * @return The note text. 136 */ 137 public String getText() { 138 return this.text == null ? null : this.text.getValue(); 139 } 140 141 /** 142 * @param value The note text. 143 */ 144 public ProcessResponseNotesComponent setText(String value) { 145 if (Utilities.noString(value)) 146 this.text = null; 147 else { 148 if (this.text == null) 149 this.text = new StringType(); 150 this.text.setValue(value); 151 } 152 return this; 153 } 154 155 protected void listChildren(List<Property> childrenList) { 156 super.listChildren(childrenList); 157 childrenList.add( 158 new Property("type", "Coding", "The note purpose: Print/Display.", 0, java.lang.Integer.MAX_VALUE, type)); 159 childrenList.add(new Property("text", "string", "The note text.", 0, java.lang.Integer.MAX_VALUE, text)); 160 } 161 162 @Override 163 public void setProperty(String name, Base value) throws FHIRException { 164 if (name.equals("type")) 165 this.type = castToCoding(value); // Coding 166 else if (name.equals("text")) 167 this.text = castToString(value); // StringType 168 else 169 super.setProperty(name, value); 170 } 171 172 @Override 173 public Base addChild(String name) throws FHIRException { 174 if (name.equals("type")) { 175 this.type = new Coding(); 176 return this.type; 177 } else if (name.equals("text")) { 178 throw new FHIRException("Cannot call addChild on a singleton property ProcessResponse.text"); 179 } else 180 return super.addChild(name); 181 } 182 183 public ProcessResponseNotesComponent copy() { 184 ProcessResponseNotesComponent dst = new ProcessResponseNotesComponent(); 185 copyValues(dst); 186 dst.type = type == null ? null : type.copy(); 187 dst.text = text == null ? null : text.copy(); 188 return dst; 189 } 190 191 @Override 192 public boolean equalsDeep(Base other) { 193 if (!super.equalsDeep(other)) 194 return false; 195 if (!(other instanceof ProcessResponseNotesComponent)) 196 return false; 197 ProcessResponseNotesComponent o = (ProcessResponseNotesComponent) other; 198 return compareDeep(type, o.type, true) && compareDeep(text, o.text, true); 199 } 200 201 @Override 202 public boolean equalsShallow(Base other) { 203 if (!super.equalsShallow(other)) 204 return false; 205 if (!(other instanceof ProcessResponseNotesComponent)) 206 return false; 207 ProcessResponseNotesComponent o = (ProcessResponseNotesComponent) other; 208 return compareValues(text, o.text, true); 209 } 210 211 public boolean isEmpty() { 212 return super.isEmpty() && (type == null || type.isEmpty()) && (text == null || text.isEmpty()); 213 } 214 215 public String fhirType() { 216 return "ProcessResponse.notes"; 217 218 } 219 220 } 221 222 /** 223 * The Response business identifier. 224 */ 225 @Child(name = "identifier", type = { 226 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 227 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 228 protected List<Identifier> identifier; 229 230 /** 231 * Original request resource reference. 232 */ 233 @Child(name = "request", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = true) 234 @Description(shortDefinition = "Request reference", formalDefinition = "Original request resource reference.") 235 protected Reference request; 236 237 /** 238 * The actual object that is the target of the reference (Original request 239 * resource reference.) 240 */ 241 protected Resource requestTarget; 242 243 /** 244 * Transaction status: error, complete, held. 245 */ 246 @Child(name = "outcome", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 247 @Description(shortDefinition = "Processing outcome", formalDefinition = "Transaction status: error, complete, held.") 248 protected Coding outcome; 249 250 /** 251 * A description of the status of the adjudication or processing. 252 */ 253 @Child(name = "disposition", type = { 254 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 255 @Description(shortDefinition = "Disposition Message", formalDefinition = "A description of the status of the adjudication or processing.") 256 protected StringType disposition; 257 258 /** 259 * The version of the style of resource contents. This should be mapped to the 260 * allowable profiles for this and supporting resources. 261 */ 262 @Child(name = "ruleset", type = { Coding.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 263 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 264 protected Coding ruleset; 265 266 /** 267 * The style (standard) and version of the original material which was converted 268 * into this resource. 269 */ 270 @Child(name = "originalRuleset", type = { 271 Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 272 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 273 protected Coding originalRuleset; 274 275 /** 276 * The date when the enclosed suite of services were performed or completed. 277 */ 278 @Child(name = "created", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 279 @Description(shortDefinition = "Creation date", formalDefinition = "The date when the enclosed suite of services were performed or completed.") 280 protected DateTimeType created; 281 282 /** 283 * The organization who produced this adjudicated response. 284 */ 285 @Child(name = "organization", type = { 286 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 287 @Description(shortDefinition = "Authoring Organization", formalDefinition = "The organization who produced this adjudicated response.") 288 protected Reference organization; 289 290 /** 291 * The actual object that is the target of the reference (The organization who 292 * produced this adjudicated response.) 293 */ 294 protected Organization organizationTarget; 295 296 /** 297 * The practitioner who is responsible for the services rendered to the patient. 298 */ 299 @Child(name = "requestProvider", type = { 300 Practitioner.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 301 @Description(shortDefinition = "Responsible Practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 302 protected Reference requestProvider; 303 304 /** 305 * The actual object that is the target of the reference (The practitioner who 306 * is responsible for the services rendered to the patient.) 307 */ 308 protected Practitioner requestProviderTarget; 309 310 /** 311 * The organization which is responsible for the services rendered to the 312 * patient. 313 */ 314 @Child(name = "requestOrganization", type = { 315 Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 316 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the services rendered to the patient.") 317 protected Reference requestOrganization; 318 319 /** 320 * The actual object that is the target of the reference (The organization which 321 * is responsible for the services rendered to the patient.) 322 */ 323 protected Organization requestOrganizationTarget; 324 325 /** 326 * The form to be used for printing the content. 327 */ 328 @Child(name = "form", type = { Coding.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 329 @Description(shortDefinition = "Printed Form Identifier", formalDefinition = "The form to be used for printing the content.") 330 protected Coding form; 331 332 /** 333 * Suite of processing note or additional requirements is the processing has 334 * been held. 335 */ 336 @Child(name = "notes", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 337 @Description(shortDefinition = "Notes", formalDefinition = "Suite of processing note or additional requirements is the processing has been held.") 338 protected List<ProcessResponseNotesComponent> notes; 339 340 /** 341 * Processing errors. 342 */ 343 @Child(name = "error", type = { 344 Coding.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 345 @Description(shortDefinition = "Error code", formalDefinition = "Processing errors.") 346 protected List<Coding> error; 347 348 private static final long serialVersionUID = -1668062545L; 349 350 /* 351 * Constructor 352 */ 353 public ProcessResponse() { 354 super(); 355 } 356 357 /** 358 * @return {@link #identifier} (The Response business identifier.) 359 */ 360 public List<Identifier> getIdentifier() { 361 if (this.identifier == null) 362 this.identifier = new ArrayList<Identifier>(); 363 return this.identifier; 364 } 365 366 public boolean hasIdentifier() { 367 if (this.identifier == null) 368 return false; 369 for (Identifier item : this.identifier) 370 if (!item.isEmpty()) 371 return true; 372 return false; 373 } 374 375 /** 376 * @return {@link #identifier} (The Response business identifier.) 377 */ 378 // syntactic sugar 379 public Identifier addIdentifier() { // 3 380 Identifier t = new Identifier(); 381 if (this.identifier == null) 382 this.identifier = new ArrayList<Identifier>(); 383 this.identifier.add(t); 384 return t; 385 } 386 387 // syntactic sugar 388 public ProcessResponse addIdentifier(Identifier t) { // 3 389 if (t == null) 390 return this; 391 if (this.identifier == null) 392 this.identifier = new ArrayList<Identifier>(); 393 this.identifier.add(t); 394 return this; 395 } 396 397 /** 398 * @return {@link #request} (Original request resource reference.) 399 */ 400 public Reference getRequest() { 401 if (this.request == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create ProcessResponse.request"); 404 else if (Configuration.doAutoCreate()) 405 this.request = new Reference(); // cc 406 return this.request; 407 } 408 409 public boolean hasRequest() { 410 return this.request != null && !this.request.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #request} (Original request resource reference.) 415 */ 416 public ProcessResponse setRequest(Reference value) { 417 this.request = value; 418 return this; 419 } 420 421 /** 422 * @return {@link #request} The actual object that is the target of the 423 * reference. The reference library doesn't populate this, but you can 424 * use it to hold the resource if you resolve it. (Original request 425 * resource reference.) 426 */ 427 public Resource getRequestTarget() { 428 return this.requestTarget; 429 } 430 431 /** 432 * @param value {@link #request} The actual object that is the target of the 433 * reference. The reference library doesn't use these, but you can 434 * use it to hold the resource if you resolve it. (Original request 435 * resource reference.) 436 */ 437 public ProcessResponse setRequestTarget(Resource value) { 438 this.requestTarget = value; 439 return this; 440 } 441 442 /** 443 * @return {@link #outcome} (Transaction status: error, complete, held.) 444 */ 445 public Coding getOutcome() { 446 if (this.outcome == null) 447 if (Configuration.errorOnAutoCreate()) 448 throw new Error("Attempt to auto-create ProcessResponse.outcome"); 449 else if (Configuration.doAutoCreate()) 450 this.outcome = new Coding(); // cc 451 return this.outcome; 452 } 453 454 public boolean hasOutcome() { 455 return this.outcome != null && !this.outcome.isEmpty(); 456 } 457 458 /** 459 * @param value {@link #outcome} (Transaction status: error, complete, held.) 460 */ 461 public ProcessResponse setOutcome(Coding value) { 462 this.outcome = value; 463 return this; 464 } 465 466 /** 467 * @return {@link #disposition} (A description of the status of the adjudication 468 * or processing.). This is the underlying object with id, value and 469 * extensions. The accessor "getDisposition" gives direct access to the 470 * value 471 */ 472 public StringType getDispositionElement() { 473 if (this.disposition == null) 474 if (Configuration.errorOnAutoCreate()) 475 throw new Error("Attempt to auto-create ProcessResponse.disposition"); 476 else if (Configuration.doAutoCreate()) 477 this.disposition = new StringType(); // bb 478 return this.disposition; 479 } 480 481 public boolean hasDispositionElement() { 482 return this.disposition != null && !this.disposition.isEmpty(); 483 } 484 485 public boolean hasDisposition() { 486 return this.disposition != null && !this.disposition.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #disposition} (A description of the status of the 491 * adjudication or processing.). This is the underlying object with 492 * id, value and extensions. The accessor "getDisposition" gives 493 * direct access to the value 494 */ 495 public ProcessResponse setDispositionElement(StringType value) { 496 this.disposition = value; 497 return this; 498 } 499 500 /** 501 * @return A description of the status of the adjudication or processing. 502 */ 503 public String getDisposition() { 504 return this.disposition == null ? null : this.disposition.getValue(); 505 } 506 507 /** 508 * @param value A description of the status of the adjudication or processing. 509 */ 510 public ProcessResponse setDisposition(String value) { 511 if (Utilities.noString(value)) 512 this.disposition = null; 513 else { 514 if (this.disposition == null) 515 this.disposition = new StringType(); 516 this.disposition.setValue(value); 517 } 518 return this; 519 } 520 521 /** 522 * @return {@link #ruleset} (The version of the style of resource contents. This 523 * should be mapped to the allowable profiles for this and supporting 524 * resources.) 525 */ 526 public Coding getRuleset() { 527 if (this.ruleset == null) 528 if (Configuration.errorOnAutoCreate()) 529 throw new Error("Attempt to auto-create ProcessResponse.ruleset"); 530 else if (Configuration.doAutoCreate()) 531 this.ruleset = new Coding(); // cc 532 return this.ruleset; 533 } 534 535 public boolean hasRuleset() { 536 return this.ruleset != null && !this.ruleset.isEmpty(); 537 } 538 539 /** 540 * @param value {@link #ruleset} (The version of the style of resource contents. 541 * This should be mapped to the allowable profiles for this and 542 * supporting resources.) 543 */ 544 public ProcessResponse setRuleset(Coding value) { 545 this.ruleset = value; 546 return this; 547 } 548 549 /** 550 * @return {@link #originalRuleset} (The style (standard) and version of the 551 * original material which was converted into this resource.) 552 */ 553 public Coding getOriginalRuleset() { 554 if (this.originalRuleset == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create ProcessResponse.originalRuleset"); 557 else if (Configuration.doAutoCreate()) 558 this.originalRuleset = new Coding(); // cc 559 return this.originalRuleset; 560 } 561 562 public boolean hasOriginalRuleset() { 563 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 564 } 565 566 /** 567 * @param value {@link #originalRuleset} (The style (standard) and version of 568 * the original material which was converted into this resource.) 569 */ 570 public ProcessResponse setOriginalRuleset(Coding value) { 571 this.originalRuleset = value; 572 return this; 573 } 574 575 /** 576 * @return {@link #created} (The date when the enclosed suite of services were 577 * performed or completed.). This is the underlying object with id, 578 * value and extensions. The accessor "getCreated" gives direct access 579 * to the value 580 */ 581 public DateTimeType getCreatedElement() { 582 if (this.created == null) 583 if (Configuration.errorOnAutoCreate()) 584 throw new Error("Attempt to auto-create ProcessResponse.created"); 585 else if (Configuration.doAutoCreate()) 586 this.created = new DateTimeType(); // bb 587 return this.created; 588 } 589 590 public boolean hasCreatedElement() { 591 return this.created != null && !this.created.isEmpty(); 592 } 593 594 public boolean hasCreated() { 595 return this.created != null && !this.created.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #created} (The date when the enclosed suite of services 600 * were performed or completed.). This is the underlying object 601 * with id, value and extensions. The accessor "getCreated" gives 602 * direct access to the value 603 */ 604 public ProcessResponse setCreatedElement(DateTimeType value) { 605 this.created = value; 606 return this; 607 } 608 609 /** 610 * @return The date when the enclosed suite of services were performed or 611 * completed. 612 */ 613 public Date getCreated() { 614 return this.created == null ? null : this.created.getValue(); 615 } 616 617 /** 618 * @param value The date when the enclosed suite of services were performed or 619 * completed. 620 */ 621 public ProcessResponse setCreated(Date value) { 622 if (value == null) 623 this.created = null; 624 else { 625 if (this.created == null) 626 this.created = new DateTimeType(); 627 this.created.setValue(value); 628 } 629 return this; 630 } 631 632 /** 633 * @return {@link #organization} (The organization who produced this adjudicated 634 * response.) 635 */ 636 public Reference getOrganization() { 637 if (this.organization == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create ProcessResponse.organization"); 640 else if (Configuration.doAutoCreate()) 641 this.organization = new Reference(); // cc 642 return this.organization; 643 } 644 645 public boolean hasOrganization() { 646 return this.organization != null && !this.organization.isEmpty(); 647 } 648 649 /** 650 * @param value {@link #organization} (The organization who produced this 651 * adjudicated response.) 652 */ 653 public ProcessResponse setOrganization(Reference value) { 654 this.organization = value; 655 return this; 656 } 657 658 /** 659 * @return {@link #organization} The actual object that is the target of the 660 * reference. The reference library doesn't populate this, but you can 661 * use it to hold the resource if you resolve it. (The organization who 662 * produced this adjudicated response.) 663 */ 664 public Organization getOrganizationTarget() { 665 if (this.organizationTarget == null) 666 if (Configuration.errorOnAutoCreate()) 667 throw new Error("Attempt to auto-create ProcessResponse.organization"); 668 else if (Configuration.doAutoCreate()) 669 this.organizationTarget = new Organization(); // aa 670 return this.organizationTarget; 671 } 672 673 /** 674 * @param value {@link #organization} The actual object that is the target of 675 * the reference. The reference library doesn't use these, but you 676 * can use it to hold the resource if you resolve it. (The 677 * organization who produced this adjudicated response.) 678 */ 679 public ProcessResponse setOrganizationTarget(Organization value) { 680 this.organizationTarget = value; 681 return this; 682 } 683 684 /** 685 * @return {@link #requestProvider} (The practitioner who is responsible for the 686 * services rendered to the patient.) 687 */ 688 public Reference getRequestProvider() { 689 if (this.requestProvider == null) 690 if (Configuration.errorOnAutoCreate()) 691 throw new Error("Attempt to auto-create ProcessResponse.requestProvider"); 692 else if (Configuration.doAutoCreate()) 693 this.requestProvider = new Reference(); // cc 694 return this.requestProvider; 695 } 696 697 public boolean hasRequestProvider() { 698 return this.requestProvider != null && !this.requestProvider.isEmpty(); 699 } 700 701 /** 702 * @param value {@link #requestProvider} (The practitioner who is responsible 703 * for the services rendered to the patient.) 704 */ 705 public ProcessResponse setRequestProvider(Reference value) { 706 this.requestProvider = value; 707 return this; 708 } 709 710 /** 711 * @return {@link #requestProvider} The actual object that is the target of the 712 * reference. The reference library doesn't populate this, but you can 713 * use it to hold the resource if you resolve it. (The practitioner who 714 * is responsible for the services rendered to the patient.) 715 */ 716 public Practitioner getRequestProviderTarget() { 717 if (this.requestProviderTarget == null) 718 if (Configuration.errorOnAutoCreate()) 719 throw new Error("Attempt to auto-create ProcessResponse.requestProvider"); 720 else if (Configuration.doAutoCreate()) 721 this.requestProviderTarget = new Practitioner(); // aa 722 return this.requestProviderTarget; 723 } 724 725 /** 726 * @param value {@link #requestProvider} The actual object that is the target of 727 * the reference. The reference library doesn't use these, but you 728 * can use it to hold the resource if you resolve it. (The 729 * practitioner who is responsible for the services rendered to the 730 * patient.) 731 */ 732 public ProcessResponse setRequestProviderTarget(Practitioner value) { 733 this.requestProviderTarget = value; 734 return this; 735 } 736 737 /** 738 * @return {@link #requestOrganization} (The organization which is responsible 739 * for the services rendered to the patient.) 740 */ 741 public Reference getRequestOrganization() { 742 if (this.requestOrganization == null) 743 if (Configuration.errorOnAutoCreate()) 744 throw new Error("Attempt to auto-create ProcessResponse.requestOrganization"); 745 else if (Configuration.doAutoCreate()) 746 this.requestOrganization = new Reference(); // cc 747 return this.requestOrganization; 748 } 749 750 public boolean hasRequestOrganization() { 751 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #requestOrganization} (The organization which is 756 * responsible for the services rendered to the patient.) 757 */ 758 public ProcessResponse setRequestOrganization(Reference value) { 759 this.requestOrganization = value; 760 return this; 761 } 762 763 /** 764 * @return {@link #requestOrganization} The actual object that is the target of 765 * the reference. The reference library doesn't populate this, but you 766 * can use it to hold the resource if you resolve it. (The organization 767 * which is responsible for the services rendered to the patient.) 768 */ 769 public Organization getRequestOrganizationTarget() { 770 if (this.requestOrganizationTarget == null) 771 if (Configuration.errorOnAutoCreate()) 772 throw new Error("Attempt to auto-create ProcessResponse.requestOrganization"); 773 else if (Configuration.doAutoCreate()) 774 this.requestOrganizationTarget = new Organization(); // aa 775 return this.requestOrganizationTarget; 776 } 777 778 /** 779 * @param value {@link #requestOrganization} The actual object that is the 780 * target of the reference. The reference library doesn't use 781 * these, but you can use it to hold the resource if you resolve 782 * it. (The organization which is responsible for the services 783 * rendered to the patient.) 784 */ 785 public ProcessResponse setRequestOrganizationTarget(Organization value) { 786 this.requestOrganizationTarget = value; 787 return this; 788 } 789 790 /** 791 * @return {@link #form} (The form to be used for printing the content.) 792 */ 793 public Coding getForm() { 794 if (this.form == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create ProcessResponse.form"); 797 else if (Configuration.doAutoCreate()) 798 this.form = new Coding(); // cc 799 return this.form; 800 } 801 802 public boolean hasForm() { 803 return this.form != null && !this.form.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #form} (The form to be used for printing the content.) 808 */ 809 public ProcessResponse setForm(Coding value) { 810 this.form = value; 811 return this; 812 } 813 814 /** 815 * @return {@link #notes} (Suite of processing note or additional requirements 816 * is the processing has been held.) 817 */ 818 public List<ProcessResponseNotesComponent> getNotes() { 819 if (this.notes == null) 820 this.notes = new ArrayList<ProcessResponseNotesComponent>(); 821 return this.notes; 822 } 823 824 public boolean hasNotes() { 825 if (this.notes == null) 826 return false; 827 for (ProcessResponseNotesComponent item : this.notes) 828 if (!item.isEmpty()) 829 return true; 830 return false; 831 } 832 833 /** 834 * @return {@link #notes} (Suite of processing note or additional requirements 835 * is the processing has been held.) 836 */ 837 // syntactic sugar 838 public ProcessResponseNotesComponent addNotes() { // 3 839 ProcessResponseNotesComponent t = new ProcessResponseNotesComponent(); 840 if (this.notes == null) 841 this.notes = new ArrayList<ProcessResponseNotesComponent>(); 842 this.notes.add(t); 843 return t; 844 } 845 846 // syntactic sugar 847 public ProcessResponse addNotes(ProcessResponseNotesComponent t) { // 3 848 if (t == null) 849 return this; 850 if (this.notes == null) 851 this.notes = new ArrayList<ProcessResponseNotesComponent>(); 852 this.notes.add(t); 853 return this; 854 } 855 856 /** 857 * @return {@link #error} (Processing errors.) 858 */ 859 public List<Coding> getError() { 860 if (this.error == null) 861 this.error = new ArrayList<Coding>(); 862 return this.error; 863 } 864 865 public boolean hasError() { 866 if (this.error == null) 867 return false; 868 for (Coding item : this.error) 869 if (!item.isEmpty()) 870 return true; 871 return false; 872 } 873 874 /** 875 * @return {@link #error} (Processing errors.) 876 */ 877 // syntactic sugar 878 public Coding addError() { // 3 879 Coding t = new Coding(); 880 if (this.error == null) 881 this.error = new ArrayList<Coding>(); 882 this.error.add(t); 883 return t; 884 } 885 886 // syntactic sugar 887 public ProcessResponse addError(Coding t) { // 3 888 if (t == null) 889 return this; 890 if (this.error == null) 891 this.error = new ArrayList<Coding>(); 892 this.error.add(t); 893 return this; 894 } 895 896 protected void listChildren(List<Property> childrenList) { 897 super.listChildren(childrenList); 898 childrenList.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 899 java.lang.Integer.MAX_VALUE, identifier)); 900 childrenList.add(new Property("request", "Reference(Any)", "Original request resource reference.", 0, 901 java.lang.Integer.MAX_VALUE, request)); 902 childrenList.add(new Property("outcome", "Coding", "Transaction status: error, complete, held.", 0, 903 java.lang.Integer.MAX_VALUE, outcome)); 904 childrenList.add(new Property("disposition", "string", 905 "A description of the status of the adjudication or processing.", 0, java.lang.Integer.MAX_VALUE, disposition)); 906 childrenList.add(new Property("ruleset", "Coding", 907 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 908 0, java.lang.Integer.MAX_VALUE, ruleset)); 909 childrenList.add(new Property("originalRuleset", "Coding", 910 "The style (standard) and version of the original material which was converted into this resource.", 0, 911 java.lang.Integer.MAX_VALUE, originalRuleset)); 912 childrenList.add( 913 new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 914 0, java.lang.Integer.MAX_VALUE, created)); 915 childrenList.add(new Property("organization", "Reference(Organization)", 916 "The organization who produced this adjudicated response.", 0, java.lang.Integer.MAX_VALUE, organization)); 917 childrenList.add(new Property("requestProvider", "Reference(Practitioner)", 918 "The practitioner who is responsible for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 919 requestProvider)); 920 childrenList.add(new Property("requestOrganization", "Reference(Organization)", 921 "The organization which is responsible for the services rendered to the patient.", 0, 922 java.lang.Integer.MAX_VALUE, requestOrganization)); 923 childrenList.add(new Property("form", "Coding", "The form to be used for printing the content.", 0, 924 java.lang.Integer.MAX_VALUE, form)); 925 childrenList.add(new Property("notes", "", 926 "Suite of processing note or additional requirements is the processing has been held.", 0, 927 java.lang.Integer.MAX_VALUE, notes)); 928 childrenList.add(new Property("error", "Coding", "Processing errors.", 0, java.lang.Integer.MAX_VALUE, error)); 929 } 930 931 @Override 932 public void setProperty(String name, Base value) throws FHIRException { 933 if (name.equals("identifier")) 934 this.getIdentifier().add(castToIdentifier(value)); 935 else if (name.equals("request")) 936 this.request = castToReference(value); // Reference 937 else if (name.equals("outcome")) 938 this.outcome = castToCoding(value); // Coding 939 else if (name.equals("disposition")) 940 this.disposition = castToString(value); // StringType 941 else if (name.equals("ruleset")) 942 this.ruleset = castToCoding(value); // Coding 943 else if (name.equals("originalRuleset")) 944 this.originalRuleset = castToCoding(value); // Coding 945 else if (name.equals("created")) 946 this.created = castToDateTime(value); // DateTimeType 947 else if (name.equals("organization")) 948 this.organization = castToReference(value); // Reference 949 else if (name.equals("requestProvider")) 950 this.requestProvider = castToReference(value); // Reference 951 else if (name.equals("requestOrganization")) 952 this.requestOrganization = castToReference(value); // Reference 953 else if (name.equals("form")) 954 this.form = castToCoding(value); // Coding 955 else if (name.equals("notes")) 956 this.getNotes().add((ProcessResponseNotesComponent) value); 957 else if (name.equals("error")) 958 this.getError().add(castToCoding(value)); 959 else 960 super.setProperty(name, value); 961 } 962 963 @Override 964 public Base addChild(String name) throws FHIRException { 965 if (name.equals("identifier")) { 966 return addIdentifier(); 967 } else if (name.equals("request")) { 968 this.request = new Reference(); 969 return this.request; 970 } else if (name.equals("outcome")) { 971 this.outcome = new Coding(); 972 return this.outcome; 973 } else if (name.equals("disposition")) { 974 throw new FHIRException("Cannot call addChild on a singleton property ProcessResponse.disposition"); 975 } else if (name.equals("ruleset")) { 976 this.ruleset = new Coding(); 977 return this.ruleset; 978 } else if (name.equals("originalRuleset")) { 979 this.originalRuleset = new Coding(); 980 return this.originalRuleset; 981 } else if (name.equals("created")) { 982 throw new FHIRException("Cannot call addChild on a singleton property ProcessResponse.created"); 983 } else if (name.equals("organization")) { 984 this.organization = new Reference(); 985 return this.organization; 986 } else if (name.equals("requestProvider")) { 987 this.requestProvider = new Reference(); 988 return this.requestProvider; 989 } else if (name.equals("requestOrganization")) { 990 this.requestOrganization = new Reference(); 991 return this.requestOrganization; 992 } else if (name.equals("form")) { 993 this.form = new Coding(); 994 return this.form; 995 } else if (name.equals("notes")) { 996 return addNotes(); 997 } else if (name.equals("error")) { 998 return addError(); 999 } else 1000 return super.addChild(name); 1001 } 1002 1003 public String fhirType() { 1004 return "ProcessResponse"; 1005 1006 } 1007 1008 public ProcessResponse copy() { 1009 ProcessResponse dst = new ProcessResponse(); 1010 copyValues(dst); 1011 if (identifier != null) { 1012 dst.identifier = new ArrayList<Identifier>(); 1013 for (Identifier i : identifier) 1014 dst.identifier.add(i.copy()); 1015 } 1016 ; 1017 dst.request = request == null ? null : request.copy(); 1018 dst.outcome = outcome == null ? null : outcome.copy(); 1019 dst.disposition = disposition == null ? null : disposition.copy(); 1020 dst.ruleset = ruleset == null ? null : ruleset.copy(); 1021 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 1022 dst.created = created == null ? null : created.copy(); 1023 dst.organization = organization == null ? null : organization.copy(); 1024 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 1025 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 1026 dst.form = form == null ? null : form.copy(); 1027 if (notes != null) { 1028 dst.notes = new ArrayList<ProcessResponseNotesComponent>(); 1029 for (ProcessResponseNotesComponent i : notes) 1030 dst.notes.add(i.copy()); 1031 } 1032 ; 1033 if (error != null) { 1034 dst.error = new ArrayList<Coding>(); 1035 for (Coding i : error) 1036 dst.error.add(i.copy()); 1037 } 1038 ; 1039 return dst; 1040 } 1041 1042 protected ProcessResponse typedCopy() { 1043 return copy(); 1044 } 1045 1046 @Override 1047 public boolean equalsDeep(Base other) { 1048 if (!super.equalsDeep(other)) 1049 return false; 1050 if (!(other instanceof ProcessResponse)) 1051 return false; 1052 ProcessResponse o = (ProcessResponse) other; 1053 return compareDeep(identifier, o.identifier, true) && compareDeep(request, o.request, true) 1054 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) 1055 && compareDeep(ruleset, o.ruleset, true) && compareDeep(originalRuleset, o.originalRuleset, true) 1056 && compareDeep(created, o.created, true) && compareDeep(organization, o.organization, true) 1057 && compareDeep(requestProvider, o.requestProvider, true) 1058 && compareDeep(requestOrganization, o.requestOrganization, true) && compareDeep(form, o.form, true) 1059 && compareDeep(notes, o.notes, true) && compareDeep(error, o.error, true); 1060 } 1061 1062 @Override 1063 public boolean equalsShallow(Base other) { 1064 if (!super.equalsShallow(other)) 1065 return false; 1066 if (!(other instanceof ProcessResponse)) 1067 return false; 1068 ProcessResponse o = (ProcessResponse) other; 1069 return compareValues(disposition, o.disposition, true) && compareValues(created, o.created, true); 1070 } 1071 1072 public boolean isEmpty() { 1073 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (request == null || request.isEmpty()) 1074 && (outcome == null || outcome.isEmpty()) && (disposition == null || disposition.isEmpty()) 1075 && (ruleset == null || ruleset.isEmpty()) && (originalRuleset == null || originalRuleset.isEmpty()) 1076 && (created == null || created.isEmpty()) && (organization == null || organization.isEmpty()) 1077 && (requestProvider == null || requestProvider.isEmpty()) 1078 && (requestOrganization == null || requestOrganization.isEmpty()) && (form == null || form.isEmpty()) 1079 && (notes == null || notes.isEmpty()) && (error == null || error.isEmpty()); 1080 } 1081 1082 @Override 1083 public ResourceType getResourceType() { 1084 return ResourceType.ProcessResponse; 1085 } 1086 1087 @SearchParamDefinition(name = "identifier", path = "ProcessResponse.identifier", description = "The business identifier of the Explanation of Benefit", type = "token") 1088 public static final String SP_IDENTIFIER = "identifier"; 1089 @SearchParamDefinition(name = "request", path = "ProcessResponse.request", description = "The reference to the claim", type = "reference") 1090 public static final String SP_REQUEST = "request"; 1091 @SearchParamDefinition(name = "organization", path = "ProcessResponse.organization", description = "The organization who generated this resource", type = "reference") 1092 public static final String SP_ORGANIZATION = "organization"; 1093 @SearchParamDefinition(name = "requestprovider", path = "ProcessResponse.requestProvider", description = "The Provider who is responsible the request transaction", type = "reference") 1094 public static final String SP_REQUESTPROVIDER = "requestprovider"; 1095 @SearchParamDefinition(name = "requestorganization", path = "ProcessResponse.requestOrganization", description = "The Organization who is responsible the request transaction", type = "reference") 1096 public static final String SP_REQUESTORGANIZATION = "requestorganization"; 1097 1098}