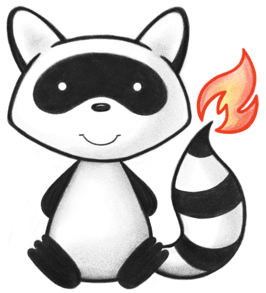
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.ArrayList; 033import java.util.List; 034 035/** 036 * A child element or property defined by the FHIR specification This class is 037 * defined as a helper class when iterating the children of an element in a 038 * generic fashion 039 * 040 * At present, iteration is only based on the specification, but this may be 041 * changed to allow profile based expression at a later date 042 * 043 * note: there's no point in creating one of these classes outside this package 044 */ 045public class Property { 046 047 /** 048 * The name of the property as found in the FHIR specification 049 */ 050 private String name; 051 052 /** 053 * The type of the property as specified in the FHIR specification (e.g. 054 * type|type|Reference(Name|Name) 055 */ 056 private String typeCode; 057 058 /** 059 * The formal definition of the element given in the FHIR specification 060 */ 061 private String definition; 062 063 /** 064 * The minimum allowed cardinality - 0 or 1 when based on the specification 065 */ 066 private int minCardinality; 067 068 /** 069 * The maximum allowed cardinality - 1 or MAX_INT when based on the 070 * specification 071 */ 072 private int maxCardinality; 073 074 /** 075 * The actual elements that exist on this instance 076 */ 077 private List<Base> values = new ArrayList<Base>(); 078 079 /** 080 * For run time, if/once a property is hooked up to it's definition 081 */ 082 private StructureDefinition structure; 083 084 /** 085 * Internal constructor 086 */ 087 public Property(String name, String typeCode, String definition, int minCardinality, int maxCardinality, Base value) { 088 super(); 089 this.name = name; 090 this.typeCode = typeCode; 091 this.definition = definition; 092 this.minCardinality = minCardinality; 093 this.maxCardinality = maxCardinality; 094 this.values.add(value); 095 } 096 097 /** 098 * Internal constructor 099 */ 100 public Property(String name, String typeCode, String definition, int minCardinality, int maxCardinality, 101 List<? extends Base> values) { 102 super(); 103 this.name = name; 104 this.typeCode = typeCode; 105 this.definition = definition; 106 this.minCardinality = minCardinality; 107 this.maxCardinality = maxCardinality; 108 if (values != null) 109 this.values.addAll(values); 110 } 111 112 /** 113 * @return The name of this property in the FHIR Specification 114 */ 115 public String getName() { 116 return name; 117 } 118 119 /** 120 * @return The stated type in the FHIR specification 121 */ 122 public String getTypeCode() { 123 return typeCode; 124 } 125 126 /** 127 * @return The definition of this element in the FHIR spec 128 */ 129 public String getDefinition() { 130 return definition; 131 } 132 133 /** 134 * @return the minimum cardinality for this element 135 */ 136 public int getMinCardinality() { 137 return minCardinality; 138 } 139 140 /** 141 * @return the maximum cardinality for this element 142 */ 143 public int getMaxCardinality() { 144 return maxCardinality; 145 } 146 147 /** 148 * @return the actual values - will only be 1 unless maximum cardinality == 149 * MAX_INT 150 */ 151 public List<Base> getValues() { 152 return values; 153 } 154 155 public boolean hasValues() { 156 for (Base e : getValues()) 157 if (e != null) 158 return true; 159 return false; 160 } 161 162 public StructureDefinition getStructure() { 163 return structure; 164 } 165 166 public void setStructure(StructureDefinition structure) { 167 this.structure = structure; 168 } 169 170}