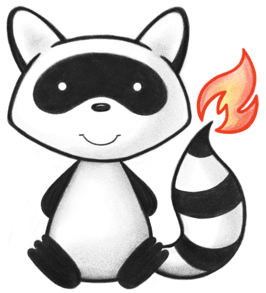
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Provenance of a resource is a record that describes entities and processes 048 * involved in producing and delivering or otherwise influencing that resource. 049 * Provenance provides a critical foundation for assessing authenticity, 050 * enabling trust, and allowing reproducibility. Provenance assertions are a 051 * form of contextual metadata and can themselves become important records with 052 * their own provenance. Provenance statement indicates clinical significance in 053 * terms of confidence in authenticity, reliability, and trustworthiness, 054 * integrity, and stage in lifecycle (e.g. Document Completion - has the 055 * artifact been legally authenticated), all of which may impact security, 056 * privacy, and trust policies. 057 */ 058@ResourceDef(name = "Provenance", profile = "http://hl7.org/fhir/Profile/Provenance") 059public class Provenance extends DomainResource { 060 061 public enum ProvenanceEntityRole { 062 /** 063 * A transformation of an entity into another, an update of an entity resulting 064 * in a new one, or the construction of a new entity based on a preexisting 065 * entity. 066 */ 067 DERIVATION, 068 /** 069 * A derivation for which the resulting entity is a revised version of some 070 * original. 071 */ 072 REVISION, 073 /** 074 * The repeat of (some or all of) an entity, such as text or image, by someone 075 * who may or may not be its original author. 076 */ 077 QUOTATION, 078 /** 079 * A primary source for a topic refers to something produced by some agent with 080 * direct experience and knowledge about the topic, at the time of the topic's 081 * study, without benefit from hindsight. 082 */ 083 SOURCE, 084 /** 085 * added to help the parsers 086 */ 087 NULL; 088 089 public static ProvenanceEntityRole fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("derivation".equals(codeString)) 093 return DERIVATION; 094 if ("revision".equals(codeString)) 095 return REVISION; 096 if ("quotation".equals(codeString)) 097 return QUOTATION; 098 if ("source".equals(codeString)) 099 return SOURCE; 100 throw new FHIRException("Unknown ProvenanceEntityRole code '" + codeString + "'"); 101 } 102 103 public String toCode() { 104 switch (this) { 105 case DERIVATION: 106 return "derivation"; 107 case REVISION: 108 return "revision"; 109 case QUOTATION: 110 return "quotation"; 111 case SOURCE: 112 return "source"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case DERIVATION: 123 return "http://hl7.org/fhir/provenance-entity-role"; 124 case REVISION: 125 return "http://hl7.org/fhir/provenance-entity-role"; 126 case QUOTATION: 127 return "http://hl7.org/fhir/provenance-entity-role"; 128 case SOURCE: 129 return "http://hl7.org/fhir/provenance-entity-role"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case DERIVATION: 140 return "A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a preexisting entity."; 141 case REVISION: 142 return "A derivation for which the resulting entity is a revised version of some original."; 143 case QUOTATION: 144 return "The repeat of (some or all of) an entity, such as text or image, by someone who may or may not be its original author."; 145 case SOURCE: 146 return "A primary source for a topic refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight."; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getDisplay() { 155 switch (this) { 156 case DERIVATION: 157 return "Derivation"; 158 case REVISION: 159 return "Revision"; 160 case QUOTATION: 161 return "Quotation"; 162 case SOURCE: 163 return "Source"; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 } 171 172 public static class ProvenanceEntityRoleEnumFactory implements EnumFactory<ProvenanceEntityRole> { 173 public ProvenanceEntityRole fromCode(String codeString) throws IllegalArgumentException { 174 if (codeString == null || "".equals(codeString)) 175 if (codeString == null || "".equals(codeString)) 176 return null; 177 if ("derivation".equals(codeString)) 178 return ProvenanceEntityRole.DERIVATION; 179 if ("revision".equals(codeString)) 180 return ProvenanceEntityRole.REVISION; 181 if ("quotation".equals(codeString)) 182 return ProvenanceEntityRole.QUOTATION; 183 if ("source".equals(codeString)) 184 return ProvenanceEntityRole.SOURCE; 185 throw new IllegalArgumentException("Unknown ProvenanceEntityRole code '" + codeString + "'"); 186 } 187 188 public Enumeration<ProvenanceEntityRole> fromType(Base code) throws FHIRException { 189 if (code == null || code.isEmpty()) 190 return null; 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("derivation".equals(codeString)) 195 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.DERIVATION); 196 if ("revision".equals(codeString)) 197 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.REVISION); 198 if ("quotation".equals(codeString)) 199 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.QUOTATION); 200 if ("source".equals(codeString)) 201 return new Enumeration<ProvenanceEntityRole>(this, ProvenanceEntityRole.SOURCE); 202 throw new FHIRException("Unknown ProvenanceEntityRole code '" + codeString + "'"); 203 } 204 205 public String toCode(ProvenanceEntityRole code) 206 { 207 if (code == ProvenanceEntityRole.NULL) 208 return null; 209 if (code == ProvenanceEntityRole.DERIVATION) 210 return "derivation"; 211 if (code == ProvenanceEntityRole.REVISION) 212 return "revision"; 213 if (code == ProvenanceEntityRole.QUOTATION) 214 return "quotation"; 215 if (code == ProvenanceEntityRole.SOURCE) 216 return "source"; 217 return "?"; 218 } 219 } 220 221 @Block() 222 public static class ProvenanceAgentComponent extends BackboneElement implements IBaseBackboneElement { 223 /** 224 * The function of the agent with respect to the activity. 225 */ 226 @Child(name = "role", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 227 @Description(shortDefinition = "What the agents involvement was", formalDefinition = "The function of the agent with respect to the activity.") 228 protected Coding role; 229 230 /** 231 * The individual, device or organization that participated in the event. 232 */ 233 @Child(name = "actor", type = { Practitioner.class, RelatedPerson.class, Patient.class, Device.class, 234 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 235 @Description(shortDefinition = "Individual, device or organization playing role", formalDefinition = "The individual, device or organization that participated in the event.") 236 protected Reference actor; 237 238 /** 239 * The actual object that is the target of the reference (The individual, device 240 * or organization that participated in the event.) 241 */ 242 protected Resource actorTarget; 243 244 /** 245 * The identity of the agent as known by the authorization system. 246 */ 247 @Child(name = "userId", type = { Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 248 @Description(shortDefinition = "Authorization-system identifier for the agent", formalDefinition = "The identity of the agent as known by the authorization system.") 249 protected Identifier userId; 250 251 /** 252 * A relationship between two the agents referenced in this resource. This is 253 * defined to allow for explicit description of the delegation between agents. 254 * For example, this human author used this device, or one person acted on 255 * another's behest. 256 */ 257 @Child(name = "relatedAgent", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 258 @Description(shortDefinition = "Track delegation between agents", formalDefinition = "A relationship between two the agents referenced in this resource. This is defined to allow for explicit description of the delegation between agents. For example, this human author used this device, or one person acted on another's behest.") 259 protected List<ProvenanceAgentRelatedAgentComponent> relatedAgent; 260 261 private static final long serialVersionUID = 1792758952L; 262 263 /* 264 * Constructor 265 */ 266 public ProvenanceAgentComponent() { 267 super(); 268 } 269 270 /* 271 * Constructor 272 */ 273 public ProvenanceAgentComponent(Coding role) { 274 super(); 275 this.role = role; 276 } 277 278 /** 279 * @return {@link #role} (The function of the agent with respect to the 280 * activity.) 281 */ 282 public Coding getRole() { 283 if (this.role == null) 284 if (Configuration.errorOnAutoCreate()) 285 throw new Error("Attempt to auto-create ProvenanceAgentComponent.role"); 286 else if (Configuration.doAutoCreate()) 287 this.role = new Coding(); // cc 288 return this.role; 289 } 290 291 public boolean hasRole() { 292 return this.role != null && !this.role.isEmpty(); 293 } 294 295 /** 296 * @param value {@link #role} (The function of the agent with respect to the 297 * activity.) 298 */ 299 public ProvenanceAgentComponent setRole(Coding value) { 300 this.role = value; 301 return this; 302 } 303 304 /** 305 * @return {@link #actor} (The individual, device or organization that 306 * participated in the event.) 307 */ 308 public Reference getActor() { 309 if (this.actor == null) 310 if (Configuration.errorOnAutoCreate()) 311 throw new Error("Attempt to auto-create ProvenanceAgentComponent.actor"); 312 else if (Configuration.doAutoCreate()) 313 this.actor = new Reference(); // cc 314 return this.actor; 315 } 316 317 public boolean hasActor() { 318 return this.actor != null && !this.actor.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #actor} (The individual, device or organization that 323 * participated in the event.) 324 */ 325 public ProvenanceAgentComponent setActor(Reference value) { 326 this.actor = value; 327 return this; 328 } 329 330 /** 331 * @return {@link #actor} The actual object that is the target of the reference. 332 * The reference library doesn't populate this, but you can use it to 333 * hold the resource if you resolve it. (The individual, device or 334 * organization that participated in the event.) 335 */ 336 public Resource getActorTarget() { 337 return this.actorTarget; 338 } 339 340 /** 341 * @param value {@link #actor} The actual object that is the target of the 342 * reference. The reference library doesn't use these, but you can 343 * use it to hold the resource if you resolve it. (The individual, 344 * device or organization that participated in the event.) 345 */ 346 public ProvenanceAgentComponent setActorTarget(Resource value) { 347 this.actorTarget = value; 348 return this; 349 } 350 351 /** 352 * @return {@link #userId} (The identity of the agent as known by the 353 * authorization system.) 354 */ 355 public Identifier getUserId() { 356 if (this.userId == null) 357 if (Configuration.errorOnAutoCreate()) 358 throw new Error("Attempt to auto-create ProvenanceAgentComponent.userId"); 359 else if (Configuration.doAutoCreate()) 360 this.userId = new Identifier(); // cc 361 return this.userId; 362 } 363 364 public boolean hasUserId() { 365 return this.userId != null && !this.userId.isEmpty(); 366 } 367 368 /** 369 * @param value {@link #userId} (The identity of the agent as known by the 370 * authorization system.) 371 */ 372 public ProvenanceAgentComponent setUserId(Identifier value) { 373 this.userId = value; 374 return this; 375 } 376 377 /** 378 * @return {@link #relatedAgent} (A relationship between two the agents 379 * referenced in this resource. This is defined to allow for explicit 380 * description of the delegation between agents. For example, this human 381 * author used this device, or one person acted on another's behest.) 382 */ 383 public List<ProvenanceAgentRelatedAgentComponent> getRelatedAgent() { 384 if (this.relatedAgent == null) 385 this.relatedAgent = new ArrayList<ProvenanceAgentRelatedAgentComponent>(); 386 return this.relatedAgent; 387 } 388 389 public boolean hasRelatedAgent() { 390 if (this.relatedAgent == null) 391 return false; 392 for (ProvenanceAgentRelatedAgentComponent item : this.relatedAgent) 393 if (!item.isEmpty()) 394 return true; 395 return false; 396 } 397 398 /** 399 * @return {@link #relatedAgent} (A relationship between two the agents 400 * referenced in this resource. This is defined to allow for explicit 401 * description of the delegation between agents. For example, this human 402 * author used this device, or one person acted on another's behest.) 403 */ 404 // syntactic sugar 405 public ProvenanceAgentRelatedAgentComponent addRelatedAgent() { // 3 406 ProvenanceAgentRelatedAgentComponent t = new ProvenanceAgentRelatedAgentComponent(); 407 if (this.relatedAgent == null) 408 this.relatedAgent = new ArrayList<ProvenanceAgentRelatedAgentComponent>(); 409 this.relatedAgent.add(t); 410 return t; 411 } 412 413 // syntactic sugar 414 public ProvenanceAgentComponent addRelatedAgent(ProvenanceAgentRelatedAgentComponent t) { // 3 415 if (t == null) 416 return this; 417 if (this.relatedAgent == null) 418 this.relatedAgent = new ArrayList<ProvenanceAgentRelatedAgentComponent>(); 419 this.relatedAgent.add(t); 420 return this; 421 } 422 423 protected void listChildren(List<Property> childrenList) { 424 super.listChildren(childrenList); 425 childrenList.add(new Property("role", "Coding", "The function of the agent with respect to the activity.", 0, 426 java.lang.Integer.MAX_VALUE, role)); 427 childrenList.add(new Property("actor", "Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", 428 "The individual, device or organization that participated in the event.", 0, java.lang.Integer.MAX_VALUE, 429 actor)); 430 childrenList.add(new Property("userId", "Identifier", 431 "The identity of the agent as known by the authorization system.", 0, java.lang.Integer.MAX_VALUE, userId)); 432 childrenList.add(new Property("relatedAgent", "", 433 "A relationship between two the agents referenced in this resource. This is defined to allow for explicit description of the delegation between agents. For example, this human author used this device, or one person acted on another's behest.", 434 0, java.lang.Integer.MAX_VALUE, relatedAgent)); 435 } 436 437 @Override 438 public void setProperty(String name, Base value) throws FHIRException { 439 if (name.equals("role")) 440 this.role = castToCoding(value); // Coding 441 else if (name.equals("actor")) 442 this.actor = castToReference(value); // Reference 443 else if (name.equals("userId")) 444 this.userId = castToIdentifier(value); // Identifier 445 else if (name.equals("relatedAgent")) 446 this.getRelatedAgent().add((ProvenanceAgentRelatedAgentComponent) value); 447 else 448 super.setProperty(name, value); 449 } 450 451 @Override 452 public Base addChild(String name) throws FHIRException { 453 if (name.equals("role")) { 454 this.role = new Coding(); 455 return this.role; 456 } else if (name.equals("actor")) { 457 this.actor = new Reference(); 458 return this.actor; 459 } else if (name.equals("userId")) { 460 this.userId = new Identifier(); 461 return this.userId; 462 } else if (name.equals("relatedAgent")) { 463 return addRelatedAgent(); 464 } else 465 return super.addChild(name); 466 } 467 468 public ProvenanceAgentComponent copy() { 469 ProvenanceAgentComponent dst = new ProvenanceAgentComponent(); 470 copyValues(dst); 471 dst.role = role == null ? null : role.copy(); 472 dst.actor = actor == null ? null : actor.copy(); 473 dst.userId = userId == null ? null : userId.copy(); 474 if (relatedAgent != null) { 475 dst.relatedAgent = new ArrayList<ProvenanceAgentRelatedAgentComponent>(); 476 for (ProvenanceAgentRelatedAgentComponent i : relatedAgent) 477 dst.relatedAgent.add(i.copy()); 478 } 479 ; 480 return dst; 481 } 482 483 @Override 484 public boolean equalsDeep(Base other) { 485 if (!super.equalsDeep(other)) 486 return false; 487 if (!(other instanceof ProvenanceAgentComponent)) 488 return false; 489 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other; 490 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true) && compareDeep(userId, o.userId, true) 491 && compareDeep(relatedAgent, o.relatedAgent, true); 492 } 493 494 @Override 495 public boolean equalsShallow(Base other) { 496 if (!super.equalsShallow(other)) 497 return false; 498 if (!(other instanceof ProvenanceAgentComponent)) 499 return false; 500 ProvenanceAgentComponent o = (ProvenanceAgentComponent) other; 501 return true; 502 } 503 504 public boolean isEmpty() { 505 return super.isEmpty() && (role == null || role.isEmpty()) && (actor == null || actor.isEmpty()) 506 && (userId == null || userId.isEmpty()) && (relatedAgent == null || relatedAgent.isEmpty()); 507 } 508 509 public String fhirType() { 510 return "Provenance.agent"; 511 512 } 513 514 } 515 516 @Block() 517 public static class ProvenanceAgentRelatedAgentComponent extends BackboneElement implements IBaseBackboneElement { 518 /** 519 * The type of relationship between agents. 520 */ 521 @Child(name = "type", type = { 522 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 523 @Description(shortDefinition = "Type of relationship between agents", formalDefinition = "The type of relationship between agents.") 524 protected CodeableConcept type; 525 526 /** 527 * An internal reference to another agent listed in this provenance by its 528 * identifier. 529 */ 530 @Child(name = "target", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 531 @Description(shortDefinition = "Reference to other agent in this resource by identifier", formalDefinition = "An internal reference to another agent listed in this provenance by its identifier.") 532 protected UriType target; 533 534 private static final long serialVersionUID = 794181198L; 535 536 /* 537 * Constructor 538 */ 539 public ProvenanceAgentRelatedAgentComponent() { 540 super(); 541 } 542 543 /* 544 * Constructor 545 */ 546 public ProvenanceAgentRelatedAgentComponent(CodeableConcept type, UriType target) { 547 super(); 548 this.type = type; 549 this.target = target; 550 } 551 552 /** 553 * @return {@link #type} (The type of relationship between agents.) 554 */ 555 public CodeableConcept getType() { 556 if (this.type == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create ProvenanceAgentRelatedAgentComponent.type"); 559 else if (Configuration.doAutoCreate()) 560 this.type = new CodeableConcept(); // cc 561 return this.type; 562 } 563 564 public boolean hasType() { 565 return this.type != null && !this.type.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #type} (The type of relationship between agents.) 570 */ 571 public ProvenanceAgentRelatedAgentComponent setType(CodeableConcept value) { 572 this.type = value; 573 return this; 574 } 575 576 /** 577 * @return {@link #target} (An internal reference to another agent listed in 578 * this provenance by its identifier.). This is the underlying object 579 * with id, value and extensions. The accessor "getTarget" gives direct 580 * access to the value 581 */ 582 public UriType getTargetElement() { 583 if (this.target == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create ProvenanceAgentRelatedAgentComponent.target"); 586 else if (Configuration.doAutoCreate()) 587 this.target = new UriType(); // bb 588 return this.target; 589 } 590 591 public boolean hasTargetElement() { 592 return this.target != null && !this.target.isEmpty(); 593 } 594 595 public boolean hasTarget() { 596 return this.target != null && !this.target.isEmpty(); 597 } 598 599 /** 600 * @param value {@link #target} (An internal reference to another agent listed 601 * in this provenance by its identifier.). This is the underlying 602 * object with id, value and extensions. The accessor "getTarget" 603 * gives direct access to the value 604 */ 605 public ProvenanceAgentRelatedAgentComponent setTargetElement(UriType value) { 606 this.target = value; 607 return this; 608 } 609 610 /** 611 * @return An internal reference to another agent listed in this provenance by 612 * its identifier. 613 */ 614 public String getTarget() { 615 return this.target == null ? null : this.target.getValue(); 616 } 617 618 /** 619 * @param value An internal reference to another agent listed in this provenance 620 * by its identifier. 621 */ 622 public ProvenanceAgentRelatedAgentComponent setTarget(String value) { 623 if (this.target == null) 624 this.target = new UriType(); 625 this.target.setValue(value); 626 return this; 627 } 628 629 protected void listChildren(List<Property> childrenList) { 630 super.listChildren(childrenList); 631 childrenList.add(new Property("type", "CodeableConcept", "The type of relationship between agents.", 0, 632 java.lang.Integer.MAX_VALUE, type)); 633 childrenList.add(new Property("target", "uri", 634 "An internal reference to another agent listed in this provenance by its identifier.", 0, 635 java.lang.Integer.MAX_VALUE, target)); 636 } 637 638 @Override 639 public void setProperty(String name, Base value) throws FHIRException { 640 if (name.equals("type")) 641 this.type = castToCodeableConcept(value); // CodeableConcept 642 else if (name.equals("target")) 643 this.target = castToUri(value); // UriType 644 else 645 super.setProperty(name, value); 646 } 647 648 @Override 649 public Base addChild(String name) throws FHIRException { 650 if (name.equals("type")) { 651 this.type = new CodeableConcept(); 652 return this.type; 653 } else if (name.equals("target")) { 654 throw new FHIRException("Cannot call addChild on a singleton property Provenance.target"); 655 } else 656 return super.addChild(name); 657 } 658 659 public ProvenanceAgentRelatedAgentComponent copy() { 660 ProvenanceAgentRelatedAgentComponent dst = new ProvenanceAgentRelatedAgentComponent(); 661 copyValues(dst); 662 dst.type = type == null ? null : type.copy(); 663 dst.target = target == null ? null : target.copy(); 664 return dst; 665 } 666 667 @Override 668 public boolean equalsDeep(Base other) { 669 if (!super.equalsDeep(other)) 670 return false; 671 if (!(other instanceof ProvenanceAgentRelatedAgentComponent)) 672 return false; 673 ProvenanceAgentRelatedAgentComponent o = (ProvenanceAgentRelatedAgentComponent) other; 674 return compareDeep(type, o.type, true) && compareDeep(target, o.target, true); 675 } 676 677 @Override 678 public boolean equalsShallow(Base other) { 679 if (!super.equalsShallow(other)) 680 return false; 681 if (!(other instanceof ProvenanceAgentRelatedAgentComponent)) 682 return false; 683 ProvenanceAgentRelatedAgentComponent o = (ProvenanceAgentRelatedAgentComponent) other; 684 return compareValues(target, o.target, true); 685 } 686 687 public boolean isEmpty() { 688 return super.isEmpty() && (type == null || type.isEmpty()) && (target == null || target.isEmpty()); 689 } 690 691 public String fhirType() { 692 return "Provenance.agent.relatedAgent"; 693 694 } 695 696 } 697 698 @Block() 699 public static class ProvenanceEntityComponent extends BackboneElement implements IBaseBackboneElement { 700 /** 701 * How the entity was used during the activity. 702 */ 703 @Child(name = "role", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 704 @Description(shortDefinition = "derivation | revision | quotation | source", formalDefinition = "How the entity was used during the activity.") 705 protected Enumeration<ProvenanceEntityRole> role; 706 707 /** 708 * The type of the entity. If the entity is a resource, then this is a resource 709 * type. 710 */ 711 @Child(name = "type", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 712 @Description(shortDefinition = "The type of resource in this entity", formalDefinition = "The type of the entity. If the entity is a resource, then this is a resource type.") 713 protected Coding type; 714 715 /** 716 * Identity of the Entity used. May be a logical or physical uri and maybe 717 * absolute or relative. 718 */ 719 @Child(name = "reference", type = { UriType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 720 @Description(shortDefinition = "Identity of entity", formalDefinition = "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.") 721 protected UriType reference; 722 723 /** 724 * Human-readable description of the entity. 725 */ 726 @Child(name = "display", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 727 @Description(shortDefinition = "Human description of entity", formalDefinition = "Human-readable description of the entity.") 728 protected StringType display; 729 730 /** 731 * The entity is attributed to an agent to express the agent's responsibility 732 * for that entity, possibly along with other agents. This description can be 733 * understood as shorthand for saying that the agent was responsible for the 734 * activity which generated the entity. 735 */ 736 @Child(name = "agent", type = { 737 ProvenanceAgentComponent.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 738 @Description(shortDefinition = "Entity is attributed to this agent", formalDefinition = "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.") 739 protected ProvenanceAgentComponent agent; 740 741 private static final long serialVersionUID = 1533729633L; 742 743 /* 744 * Constructor 745 */ 746 public ProvenanceEntityComponent() { 747 super(); 748 } 749 750 /* 751 * Constructor 752 */ 753 public ProvenanceEntityComponent(Enumeration<ProvenanceEntityRole> role, Coding type, UriType reference) { 754 super(); 755 this.role = role; 756 this.type = type; 757 this.reference = reference; 758 } 759 760 /** 761 * @return {@link #role} (How the entity was used during the activity.). This is 762 * the underlying object with id, value and extensions. The accessor 763 * "getRole" gives direct access to the value 764 */ 765 public Enumeration<ProvenanceEntityRole> getRoleElement() { 766 if (this.role == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create ProvenanceEntityComponent.role"); 769 else if (Configuration.doAutoCreate()) 770 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); // bb 771 return this.role; 772 } 773 774 public boolean hasRoleElement() { 775 return this.role != null && !this.role.isEmpty(); 776 } 777 778 public boolean hasRole() { 779 return this.role != null && !this.role.isEmpty(); 780 } 781 782 /** 783 * @param value {@link #role} (How the entity was used during the activity.). 784 * This is the underlying object with id, value and extensions. The 785 * accessor "getRole" gives direct access to the value 786 */ 787 public ProvenanceEntityComponent setRoleElement(Enumeration<ProvenanceEntityRole> value) { 788 this.role = value; 789 return this; 790 } 791 792 /** 793 * @return How the entity was used during the activity. 794 */ 795 public ProvenanceEntityRole getRole() { 796 return this.role == null ? null : this.role.getValue(); 797 } 798 799 /** 800 * @param value How the entity was used during the activity. 801 */ 802 public ProvenanceEntityComponent setRole(ProvenanceEntityRole value) { 803 if (this.role == null) 804 this.role = new Enumeration<ProvenanceEntityRole>(new ProvenanceEntityRoleEnumFactory()); 805 this.role.setValue(value); 806 return this; 807 } 808 809 /** 810 * @return {@link #type} (The type of the entity. If the entity is a resource, 811 * then this is a resource type.) 812 */ 813 public Coding getType() { 814 if (this.type == null) 815 if (Configuration.errorOnAutoCreate()) 816 throw new Error("Attempt to auto-create ProvenanceEntityComponent.type"); 817 else if (Configuration.doAutoCreate()) 818 this.type = new Coding(); // cc 819 return this.type; 820 } 821 822 public boolean hasType() { 823 return this.type != null && !this.type.isEmpty(); 824 } 825 826 /** 827 * @param value {@link #type} (The type of the entity. If the entity is a 828 * resource, then this is a resource type.) 829 */ 830 public ProvenanceEntityComponent setType(Coding value) { 831 this.type = value; 832 return this; 833 } 834 835 /** 836 * @return {@link #reference} (Identity of the Entity used. May be a logical or 837 * physical uri and maybe absolute or relative.). This is the underlying 838 * object with id, value and extensions. The accessor "getReference" 839 * gives direct access to the value 840 */ 841 public UriType getReferenceElement() { 842 if (this.reference == null) 843 if (Configuration.errorOnAutoCreate()) 844 throw new Error("Attempt to auto-create ProvenanceEntityComponent.reference"); 845 else if (Configuration.doAutoCreate()) 846 this.reference = new UriType(); // bb 847 return this.reference; 848 } 849 850 public boolean hasReferenceElement() { 851 return this.reference != null && !this.reference.isEmpty(); 852 } 853 854 public boolean hasReference() { 855 return this.reference != null && !this.reference.isEmpty(); 856 } 857 858 /** 859 * @param value {@link #reference} (Identity of the Entity used. May be a 860 * logical or physical uri and maybe absolute or relative.). This 861 * is the underlying object with id, value and extensions. The 862 * accessor "getReference" gives direct access to the value 863 */ 864 public ProvenanceEntityComponent setReferenceElement(UriType value) { 865 this.reference = value; 866 return this; 867 } 868 869 /** 870 * @return Identity of the Entity used. May be a logical or physical uri and 871 * maybe absolute or relative. 872 */ 873 public String getReference() { 874 return this.reference == null ? null : this.reference.getValue(); 875 } 876 877 /** 878 * @param value Identity of the Entity used. May be a logical or physical uri 879 * and maybe absolute or relative. 880 */ 881 public ProvenanceEntityComponent setReference(String value) { 882 if (this.reference == null) 883 this.reference = new UriType(); 884 this.reference.setValue(value); 885 return this; 886 } 887 888 /** 889 * @return {@link #display} (Human-readable description of the entity.). This is 890 * the underlying object with id, value and extensions. The accessor 891 * "getDisplay" gives direct access to the value 892 */ 893 public StringType getDisplayElement() { 894 if (this.display == null) 895 if (Configuration.errorOnAutoCreate()) 896 throw new Error("Attempt to auto-create ProvenanceEntityComponent.display"); 897 else if (Configuration.doAutoCreate()) 898 this.display = new StringType(); // bb 899 return this.display; 900 } 901 902 public boolean hasDisplayElement() { 903 return this.display != null && !this.display.isEmpty(); 904 } 905 906 public boolean hasDisplay() { 907 return this.display != null && !this.display.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #display} (Human-readable description of the entity.). 912 * This is the underlying object with id, value and extensions. The 913 * accessor "getDisplay" gives direct access to the value 914 */ 915 public ProvenanceEntityComponent setDisplayElement(StringType value) { 916 this.display = value; 917 return this; 918 } 919 920 /** 921 * @return Human-readable description of the entity. 922 */ 923 public String getDisplay() { 924 return this.display == null ? null : this.display.getValue(); 925 } 926 927 /** 928 * @param value Human-readable description of the entity. 929 */ 930 public ProvenanceEntityComponent setDisplay(String value) { 931 if (Utilities.noString(value)) 932 this.display = null; 933 else { 934 if (this.display == null) 935 this.display = new StringType(); 936 this.display.setValue(value); 937 } 938 return this; 939 } 940 941 /** 942 * @return {@link #agent} (The entity is attributed to an agent to express the 943 * agent's responsibility for that entity, possibly along with other 944 * agents. This description can be understood as shorthand for saying 945 * that the agent was responsible for the activity which generated the 946 * entity.) 947 */ 948 public ProvenanceAgentComponent getAgent() { 949 if (this.agent == null) 950 if (Configuration.errorOnAutoCreate()) 951 throw new Error("Attempt to auto-create ProvenanceEntityComponent.agent"); 952 else if (Configuration.doAutoCreate()) 953 this.agent = new ProvenanceAgentComponent(); // cc 954 return this.agent; 955 } 956 957 public boolean hasAgent() { 958 return this.agent != null && !this.agent.isEmpty(); 959 } 960 961 /** 962 * @param value {@link #agent} (The entity is attributed to an agent to express 963 * the agent's responsibility for that entity, possibly along with 964 * other agents. This description can be understood as shorthand 965 * for saying that the agent was responsible for the activity which 966 * generated the entity.) 967 */ 968 public ProvenanceEntityComponent setAgent(ProvenanceAgentComponent value) { 969 this.agent = value; 970 return this; 971 } 972 973 protected void listChildren(List<Property> childrenList) { 974 super.listChildren(childrenList); 975 childrenList.add(new Property("role", "code", "How the entity was used during the activity.", 0, 976 java.lang.Integer.MAX_VALUE, role)); 977 childrenList.add(new Property("type", "Coding", 978 "The type of the entity. If the entity is a resource, then this is a resource type.", 0, 979 java.lang.Integer.MAX_VALUE, type)); 980 childrenList.add(new Property("reference", "uri", 981 "Identity of the Entity used. May be a logical or physical uri and maybe absolute or relative.", 0, 982 java.lang.Integer.MAX_VALUE, reference)); 983 childrenList.add(new Property("display", "string", "Human-readable description of the entity.", 0, 984 java.lang.Integer.MAX_VALUE, display)); 985 childrenList.add(new Property("agent", "@Provenance.agent", 986 "The entity is attributed to an agent to express the agent's responsibility for that entity, possibly along with other agents. This description can be understood as shorthand for saying that the agent was responsible for the activity which generated the entity.", 987 0, java.lang.Integer.MAX_VALUE, agent)); 988 } 989 990 @Override 991 public void setProperty(String name, Base value) throws FHIRException { 992 if (name.equals("role")) 993 this.role = new ProvenanceEntityRoleEnumFactory().fromType(value); // Enumeration<ProvenanceEntityRole> 994 else if (name.equals("type")) 995 this.type = castToCoding(value); // Coding 996 else if (name.equals("reference")) 997 this.reference = castToUri(value); // UriType 998 else if (name.equals("display")) 999 this.display = castToString(value); // StringType 1000 else if (name.equals("agent")) 1001 this.agent = (ProvenanceAgentComponent) value; // ProvenanceAgentComponent 1002 else 1003 super.setProperty(name, value); 1004 } 1005 1006 @Override 1007 public Base addChild(String name) throws FHIRException { 1008 if (name.equals("role")) { 1009 throw new FHIRException("Cannot call addChild on a singleton property Provenance.role"); 1010 } else if (name.equals("type")) { 1011 this.type = new Coding(); 1012 return this.type; 1013 } else if (name.equals("reference")) { 1014 throw new FHIRException("Cannot call addChild on a singleton property Provenance.reference"); 1015 } else if (name.equals("display")) { 1016 throw new FHIRException("Cannot call addChild on a singleton property Provenance.display"); 1017 } else if (name.equals("agent")) { 1018 this.agent = new ProvenanceAgentComponent(); 1019 return this.agent; 1020 } else 1021 return super.addChild(name); 1022 } 1023 1024 public ProvenanceEntityComponent copy() { 1025 ProvenanceEntityComponent dst = new ProvenanceEntityComponent(); 1026 copyValues(dst); 1027 dst.role = role == null ? null : role.copy(); 1028 dst.type = type == null ? null : type.copy(); 1029 dst.reference = reference == null ? null : reference.copy(); 1030 dst.display = display == null ? null : display.copy(); 1031 dst.agent = agent == null ? null : agent.copy(); 1032 return dst; 1033 } 1034 1035 @Override 1036 public boolean equalsDeep(Base other) { 1037 if (!super.equalsDeep(other)) 1038 return false; 1039 if (!(other instanceof ProvenanceEntityComponent)) 1040 return false; 1041 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other; 1042 return compareDeep(role, o.role, true) && compareDeep(type, o.type, true) 1043 && compareDeep(reference, o.reference, true) && compareDeep(display, o.display, true) 1044 && compareDeep(agent, o.agent, true); 1045 } 1046 1047 @Override 1048 public boolean equalsShallow(Base other) { 1049 if (!super.equalsShallow(other)) 1050 return false; 1051 if (!(other instanceof ProvenanceEntityComponent)) 1052 return false; 1053 ProvenanceEntityComponent o = (ProvenanceEntityComponent) other; 1054 return compareValues(role, o.role, true) && compareValues(reference, o.reference, true) 1055 && compareValues(display, o.display, true); 1056 } 1057 1058 public boolean isEmpty() { 1059 return super.isEmpty() && (role == null || role.isEmpty()) && (type == null || type.isEmpty()) 1060 && (reference == null || reference.isEmpty()) && (display == null || display.isEmpty()) 1061 && (agent == null || agent.isEmpty()); 1062 } 1063 1064 public String fhirType() { 1065 return "Provenance.entity"; 1066 1067 } 1068 1069 } 1070 1071 /** 1072 * The Reference(s) that were generated or updated by the activity described in 1073 * this resource. A provenance can point to more than one target if multiple 1074 * resources were created/updated by the same activity. 1075 */ 1076 @Child(name = "target", type = {}, order = 0, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1077 @Description(shortDefinition = "Target Reference(s) (usually version specific)", formalDefinition = "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.") 1078 protected List<Reference> target; 1079 /** 1080 * The actual objects that are the target of the reference (The Reference(s) 1081 * that were generated or updated by the activity described in this resource. A 1082 * provenance can point to more than one target if multiple resources were 1083 * created/updated by the same activity.) 1084 */ 1085 protected List<Resource> targetTarget; 1086 1087 /** 1088 * The period during which the activity occurred. 1089 */ 1090 @Child(name = "period", type = { Period.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1091 @Description(shortDefinition = "When the activity occurred", formalDefinition = "The period during which the activity occurred.") 1092 protected Period period; 1093 1094 /** 1095 * The instant of time at which the activity was recorded. 1096 */ 1097 @Child(name = "recorded", type = { InstantType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1098 @Description(shortDefinition = "When the activity was recorded / updated", formalDefinition = "The instant of time at which the activity was recorded.") 1099 protected InstantType recorded; 1100 1101 /** 1102 * The reason that the activity was taking place. 1103 */ 1104 @Child(name = "reason", type = { 1105 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1106 @Description(shortDefinition = "Reason the activity is occurring", formalDefinition = "The reason that the activity was taking place.") 1107 protected List<CodeableConcept> reason; 1108 1109 /** 1110 * An activity is something that occurs over a period of time and acts upon or 1111 * with entities; it may include consuming, processing, transforming, modifying, 1112 * relocating, using, or generating entities. 1113 */ 1114 @Child(name = "activity", type = { 1115 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1116 @Description(shortDefinition = "Activity that occurred", formalDefinition = "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.") 1117 protected CodeableConcept activity; 1118 1119 /** 1120 * Where the activity occurred, if relevant. 1121 */ 1122 @Child(name = "location", type = { Location.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1123 @Description(shortDefinition = "Where the activity occurred, if relevant", formalDefinition = "Where the activity occurred, if relevant.") 1124 protected Reference location; 1125 1126 /** 1127 * The actual object that is the target of the reference (Where the activity 1128 * occurred, if relevant.) 1129 */ 1130 protected Location locationTarget; 1131 1132 /** 1133 * Policy or plan the activity was defined by. Typically, a single activity may 1134 * have multiple applicable policy documents, such as patient consent, guarantor 1135 * funding, etc. 1136 */ 1137 @Child(name = "policy", type = { 1138 UriType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1139 @Description(shortDefinition = "Policy or plan the activity was defined by", formalDefinition = "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.") 1140 protected List<UriType> policy; 1141 1142 /** 1143 * An agent takes a role in an activity such that the agent can be assigned some 1144 * degree of responsibility for the activity taking place. An agent can be a 1145 * person, an organization, software, or other entities that may be ascribed 1146 * responsibility. 1147 */ 1148 @Child(name = "agent", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1149 @Description(shortDefinition = "Agents involved in creating resource", formalDefinition = "An agent takes a role in an activity such that the agent can be assigned some degree of responsibility for the activity taking place. An agent can be a person, an organization, software, or other entities that may be ascribed responsibility.") 1150 protected List<ProvenanceAgentComponent> agent; 1151 1152 /** 1153 * An entity used in this activity. 1154 */ 1155 @Child(name = "entity", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1156 @Description(shortDefinition = "An entity used in this activity", formalDefinition = "An entity used in this activity.") 1157 protected List<ProvenanceEntityComponent> entity; 1158 1159 /** 1160 * A digital signature on the target Reference(s). The signer should match a 1161 * Provenance.agent. The purpose of the signature is indicated. 1162 */ 1163 @Child(name = "signature", type = { 1164 Signature.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1165 @Description(shortDefinition = "Signature on target", formalDefinition = "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.") 1166 protected List<Signature> signature; 1167 1168 private static final long serialVersionUID = -1053458671L; 1169 1170 /* 1171 * Constructor 1172 */ 1173 public Provenance() { 1174 super(); 1175 } 1176 1177 /* 1178 * Constructor 1179 */ 1180 public Provenance(InstantType recorded) { 1181 super(); 1182 this.recorded = recorded; 1183 } 1184 1185 /** 1186 * @return {@link #target} (The Reference(s) that were generated or updated by 1187 * the activity described in this resource. A provenance can point to 1188 * more than one target if multiple resources were created/updated by 1189 * the same activity.) 1190 */ 1191 public List<Reference> getTarget() { 1192 if (this.target == null) 1193 this.target = new ArrayList<Reference>(); 1194 return this.target; 1195 } 1196 1197 public boolean hasTarget() { 1198 if (this.target == null) 1199 return false; 1200 for (Reference item : this.target) 1201 if (!item.isEmpty()) 1202 return true; 1203 return false; 1204 } 1205 1206 /** 1207 * @return {@link #target} (The Reference(s) that were generated or updated by 1208 * the activity described in this resource. A provenance can point to 1209 * more than one target if multiple resources were created/updated by 1210 * the same activity.) 1211 */ 1212 // syntactic sugar 1213 public Reference addTarget() { // 3 1214 Reference t = new Reference(); 1215 if (this.target == null) 1216 this.target = new ArrayList<Reference>(); 1217 this.target.add(t); 1218 return t; 1219 } 1220 1221 // syntactic sugar 1222 public Provenance addTarget(Reference t) { // 3 1223 if (t == null) 1224 return this; 1225 if (this.target == null) 1226 this.target = new ArrayList<Reference>(); 1227 this.target.add(t); 1228 return this; 1229 } 1230 1231 /** 1232 * @return {@link #target} (The actual objects that are the target of the 1233 * reference. The reference library doesn't populate this, but you can 1234 * use this to hold the resources if you resolvethemt. The Reference(s) 1235 * that were generated or updated by the activity described in this 1236 * resource. A provenance can point to more than one target if multiple 1237 * resources were created/updated by the same activity.) 1238 */ 1239 public List<Resource> getTargetTarget() { 1240 if (this.targetTarget == null) 1241 this.targetTarget = new ArrayList<Resource>(); 1242 return this.targetTarget; 1243 } 1244 1245 /** 1246 * @return {@link #period} (The period during which the activity occurred.) 1247 */ 1248 public Period getPeriod() { 1249 if (this.period == null) 1250 if (Configuration.errorOnAutoCreate()) 1251 throw new Error("Attempt to auto-create Provenance.period"); 1252 else if (Configuration.doAutoCreate()) 1253 this.period = new Period(); // cc 1254 return this.period; 1255 } 1256 1257 public boolean hasPeriod() { 1258 return this.period != null && !this.period.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #period} (The period during which the activity occurred.) 1263 */ 1264 public Provenance setPeriod(Period value) { 1265 this.period = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return {@link #recorded} (The instant of time at which the activity was 1271 * recorded.). This is the underlying object with id, value and 1272 * extensions. The accessor "getRecorded" gives direct access to the 1273 * value 1274 */ 1275 public InstantType getRecordedElement() { 1276 if (this.recorded == null) 1277 if (Configuration.errorOnAutoCreate()) 1278 throw new Error("Attempt to auto-create Provenance.recorded"); 1279 else if (Configuration.doAutoCreate()) 1280 this.recorded = new InstantType(); // bb 1281 return this.recorded; 1282 } 1283 1284 public boolean hasRecordedElement() { 1285 return this.recorded != null && !this.recorded.isEmpty(); 1286 } 1287 1288 public boolean hasRecorded() { 1289 return this.recorded != null && !this.recorded.isEmpty(); 1290 } 1291 1292 /** 1293 * @param value {@link #recorded} (The instant of time at which the activity was 1294 * recorded.). This is the underlying object with id, value and 1295 * extensions. The accessor "getRecorded" gives direct access to 1296 * the value 1297 */ 1298 public Provenance setRecordedElement(InstantType value) { 1299 this.recorded = value; 1300 return this; 1301 } 1302 1303 /** 1304 * @return The instant of time at which the activity was recorded. 1305 */ 1306 public Date getRecorded() { 1307 return this.recorded == null ? null : this.recorded.getValue(); 1308 } 1309 1310 /** 1311 * @param value The instant of time at which the activity was recorded. 1312 */ 1313 public Provenance setRecorded(Date value) { 1314 if (this.recorded == null) 1315 this.recorded = new InstantType(); 1316 this.recorded.setValue(value); 1317 return this; 1318 } 1319 1320 /** 1321 * @return {@link #reason} (The reason that the activity was taking place.) 1322 */ 1323 public List<CodeableConcept> getReason() { 1324 if (this.reason == null) 1325 this.reason = new ArrayList<CodeableConcept>(); 1326 return this.reason; 1327 } 1328 1329 public boolean hasReason() { 1330 if (this.reason == null) 1331 return false; 1332 for (CodeableConcept item : this.reason) 1333 if (!item.isEmpty()) 1334 return true; 1335 return false; 1336 } 1337 1338 /** 1339 * @return {@link #reason} (The reason that the activity was taking place.) 1340 */ 1341 // syntactic sugar 1342 public CodeableConcept addReason() { // 3 1343 CodeableConcept t = new CodeableConcept(); 1344 if (this.reason == null) 1345 this.reason = new ArrayList<CodeableConcept>(); 1346 this.reason.add(t); 1347 return t; 1348 } 1349 1350 // syntactic sugar 1351 public Provenance addReason(CodeableConcept t) { // 3 1352 if (t == null) 1353 return this; 1354 if (this.reason == null) 1355 this.reason = new ArrayList<CodeableConcept>(); 1356 this.reason.add(t); 1357 return this; 1358 } 1359 1360 /** 1361 * @return {@link #activity} (An activity is something that occurs over a period 1362 * of time and acts upon or with entities; it may include consuming, 1363 * processing, transforming, modifying, relocating, using, or generating 1364 * entities.) 1365 */ 1366 public CodeableConcept getActivity() { 1367 if (this.activity == null) 1368 if (Configuration.errorOnAutoCreate()) 1369 throw new Error("Attempt to auto-create Provenance.activity"); 1370 else if (Configuration.doAutoCreate()) 1371 this.activity = new CodeableConcept(); // cc 1372 return this.activity; 1373 } 1374 1375 public boolean hasActivity() { 1376 return this.activity != null && !this.activity.isEmpty(); 1377 } 1378 1379 /** 1380 * @param value {@link #activity} (An activity is something that occurs over a 1381 * period of time and acts upon or with entities; it may include 1382 * consuming, processing, transforming, modifying, relocating, 1383 * using, or generating entities.) 1384 */ 1385 public Provenance setActivity(CodeableConcept value) { 1386 this.activity = value; 1387 return this; 1388 } 1389 1390 /** 1391 * @return {@link #location} (Where the activity occurred, if relevant.) 1392 */ 1393 public Reference getLocation() { 1394 if (this.location == null) 1395 if (Configuration.errorOnAutoCreate()) 1396 throw new Error("Attempt to auto-create Provenance.location"); 1397 else if (Configuration.doAutoCreate()) 1398 this.location = new Reference(); // cc 1399 return this.location; 1400 } 1401 1402 public boolean hasLocation() { 1403 return this.location != null && !this.location.isEmpty(); 1404 } 1405 1406 /** 1407 * @param value {@link #location} (Where the activity occurred, if relevant.) 1408 */ 1409 public Provenance setLocation(Reference value) { 1410 this.location = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return {@link #location} The actual object that is the target of the 1416 * reference. The reference library doesn't populate this, but you can 1417 * use it to hold the resource if you resolve it. (Where the activity 1418 * occurred, if relevant.) 1419 */ 1420 public Location getLocationTarget() { 1421 if (this.locationTarget == null) 1422 if (Configuration.errorOnAutoCreate()) 1423 throw new Error("Attempt to auto-create Provenance.location"); 1424 else if (Configuration.doAutoCreate()) 1425 this.locationTarget = new Location(); // aa 1426 return this.locationTarget; 1427 } 1428 1429 /** 1430 * @param value {@link #location} The actual object that is the target of the 1431 * reference. The reference library doesn't use these, but you can 1432 * use it to hold the resource if you resolve it. (Where the 1433 * activity occurred, if relevant.) 1434 */ 1435 public Provenance setLocationTarget(Location value) { 1436 this.locationTarget = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #policy} (Policy or plan the activity was defined by. 1442 * Typically, a single activity may have multiple applicable policy 1443 * documents, such as patient consent, guarantor funding, etc.) 1444 */ 1445 public List<UriType> getPolicy() { 1446 if (this.policy == null) 1447 this.policy = new ArrayList<UriType>(); 1448 return this.policy; 1449 } 1450 1451 public boolean hasPolicy() { 1452 if (this.policy == null) 1453 return false; 1454 for (UriType item : this.policy) 1455 if (!item.isEmpty()) 1456 return true; 1457 return false; 1458 } 1459 1460 /** 1461 * @return {@link #policy} (Policy or plan the activity was defined by. 1462 * Typically, a single activity may have multiple applicable policy 1463 * documents, such as patient consent, guarantor funding, etc.) 1464 */ 1465 // syntactic sugar 1466 public UriType addPolicyElement() {// 2 1467 UriType t = new UriType(); 1468 if (this.policy == null) 1469 this.policy = new ArrayList<UriType>(); 1470 this.policy.add(t); 1471 return t; 1472 } 1473 1474 /** 1475 * @param value {@link #policy} (Policy or plan the activity was defined by. 1476 * Typically, a single activity may have multiple applicable policy 1477 * documents, such as patient consent, guarantor funding, etc.) 1478 */ 1479 public Provenance addPolicy(String value) { // 1 1480 UriType t = new UriType(); 1481 t.setValue(value); 1482 if (this.policy == null) 1483 this.policy = new ArrayList<UriType>(); 1484 this.policy.add(t); 1485 return this; 1486 } 1487 1488 /** 1489 * @param value {@link #policy} (Policy or plan the activity was defined by. 1490 * Typically, a single activity may have multiple applicable policy 1491 * documents, such as patient consent, guarantor funding, etc.) 1492 */ 1493 public boolean hasPolicy(String value) { 1494 if (this.policy == null) 1495 return false; 1496 for (UriType v : this.policy) 1497 if (v.equals(value)) // uri 1498 return true; 1499 return false; 1500 } 1501 1502 /** 1503 * @return {@link #agent} (An agent takes a role in an activity such that the 1504 * agent can be assigned some degree of responsibility for the activity 1505 * taking place. An agent can be a person, an organization, software, or 1506 * other entities that may be ascribed responsibility.) 1507 */ 1508 public List<ProvenanceAgentComponent> getAgent() { 1509 if (this.agent == null) 1510 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1511 return this.agent; 1512 } 1513 1514 public boolean hasAgent() { 1515 if (this.agent == null) 1516 return false; 1517 for (ProvenanceAgentComponent item : this.agent) 1518 if (!item.isEmpty()) 1519 return true; 1520 return false; 1521 } 1522 1523 /** 1524 * @return {@link #agent} (An agent takes a role in an activity such that the 1525 * agent can be assigned some degree of responsibility for the activity 1526 * taking place. An agent can be a person, an organization, software, or 1527 * other entities that may be ascribed responsibility.) 1528 */ 1529 // syntactic sugar 1530 public ProvenanceAgentComponent addAgent() { // 3 1531 ProvenanceAgentComponent t = new ProvenanceAgentComponent(); 1532 if (this.agent == null) 1533 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1534 this.agent.add(t); 1535 return t; 1536 } 1537 1538 // syntactic sugar 1539 public Provenance addAgent(ProvenanceAgentComponent t) { // 3 1540 if (t == null) 1541 return this; 1542 if (this.agent == null) 1543 this.agent = new ArrayList<ProvenanceAgentComponent>(); 1544 this.agent.add(t); 1545 return this; 1546 } 1547 1548 /** 1549 * @return {@link #entity} (An entity used in this activity.) 1550 */ 1551 public List<ProvenanceEntityComponent> getEntity() { 1552 if (this.entity == null) 1553 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1554 return this.entity; 1555 } 1556 1557 public boolean hasEntity() { 1558 if (this.entity == null) 1559 return false; 1560 for (ProvenanceEntityComponent item : this.entity) 1561 if (!item.isEmpty()) 1562 return true; 1563 return false; 1564 } 1565 1566 /** 1567 * @return {@link #entity} (An entity used in this activity.) 1568 */ 1569 // syntactic sugar 1570 public ProvenanceEntityComponent addEntity() { // 3 1571 ProvenanceEntityComponent t = new ProvenanceEntityComponent(); 1572 if (this.entity == null) 1573 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1574 this.entity.add(t); 1575 return t; 1576 } 1577 1578 // syntactic sugar 1579 public Provenance addEntity(ProvenanceEntityComponent t) { // 3 1580 if (t == null) 1581 return this; 1582 if (this.entity == null) 1583 this.entity = new ArrayList<ProvenanceEntityComponent>(); 1584 this.entity.add(t); 1585 return this; 1586 } 1587 1588 /** 1589 * @return {@link #signature} (A digital signature on the target Reference(s). 1590 * The signer should match a Provenance.agent. The purpose of the 1591 * signature is indicated.) 1592 */ 1593 public List<Signature> getSignature() { 1594 if (this.signature == null) 1595 this.signature = new ArrayList<Signature>(); 1596 return this.signature; 1597 } 1598 1599 public boolean hasSignature() { 1600 if (this.signature == null) 1601 return false; 1602 for (Signature item : this.signature) 1603 if (!item.isEmpty()) 1604 return true; 1605 return false; 1606 } 1607 1608 /** 1609 * @return {@link #signature} (A digital signature on the target Reference(s). 1610 * The signer should match a Provenance.agent. The purpose of the 1611 * signature is indicated.) 1612 */ 1613 // syntactic sugar 1614 public Signature addSignature() { // 3 1615 Signature t = new Signature(); 1616 if (this.signature == null) 1617 this.signature = new ArrayList<Signature>(); 1618 this.signature.add(t); 1619 return t; 1620 } 1621 1622 // syntactic sugar 1623 public Provenance addSignature(Signature t) { // 3 1624 if (t == null) 1625 return this; 1626 if (this.signature == null) 1627 this.signature = new ArrayList<Signature>(); 1628 this.signature.add(t); 1629 return this; 1630 } 1631 1632 protected void listChildren(List<Property> childrenList) { 1633 super.listChildren(childrenList); 1634 childrenList.add(new Property("target", "Reference(Any)", 1635 "The Reference(s) that were generated or updated by the activity described in this resource. A provenance can point to more than one target if multiple resources were created/updated by the same activity.", 1636 0, java.lang.Integer.MAX_VALUE, target)); 1637 childrenList.add(new Property("period", "Period", "The period during which the activity occurred.", 0, 1638 java.lang.Integer.MAX_VALUE, period)); 1639 childrenList.add(new Property("recorded", "instant", "The instant of time at which the activity was recorded.", 0, 1640 java.lang.Integer.MAX_VALUE, recorded)); 1641 childrenList.add(new Property("reason", "CodeableConcept", "The reason that the activity was taking place.", 0, 1642 java.lang.Integer.MAX_VALUE, reason)); 1643 childrenList.add(new Property("activity", "CodeableConcept", 1644 "An activity is something that occurs over a period of time and acts upon or with entities; it may include consuming, processing, transforming, modifying, relocating, using, or generating entities.", 1645 0, java.lang.Integer.MAX_VALUE, activity)); 1646 childrenList.add(new Property("location", "Reference(Location)", "Where the activity occurred, if relevant.", 0, 1647 java.lang.Integer.MAX_VALUE, location)); 1648 childrenList.add(new Property("policy", "uri", 1649 "Policy or plan the activity was defined by. Typically, a single activity may have multiple applicable policy documents, such as patient consent, guarantor funding, etc.", 1650 0, java.lang.Integer.MAX_VALUE, policy)); 1651 childrenList.add(new Property("agent", "", 1652 "An agent takes a role in an activity such that the agent can be assigned some degree of responsibility for the activity taking place. An agent can be a person, an organization, software, or other entities that may be ascribed responsibility.", 1653 0, java.lang.Integer.MAX_VALUE, agent)); 1654 childrenList 1655 .add(new Property("entity", "", "An entity used in this activity.", 0, java.lang.Integer.MAX_VALUE, entity)); 1656 childrenList.add(new Property("signature", "Signature", 1657 "A digital signature on the target Reference(s). The signer should match a Provenance.agent. The purpose of the signature is indicated.", 1658 0, java.lang.Integer.MAX_VALUE, signature)); 1659 } 1660 1661 @Override 1662 public void setProperty(String name, Base value) throws FHIRException { 1663 if (name.equals("target")) 1664 this.getTarget().add(castToReference(value)); 1665 else if (name.equals("period")) 1666 this.period = castToPeriod(value); // Period 1667 else if (name.equals("recorded")) 1668 this.recorded = castToInstant(value); // InstantType 1669 else if (name.equals("reason")) 1670 this.getReason().add(castToCodeableConcept(value)); 1671 else if (name.equals("activity")) 1672 this.activity = castToCodeableConcept(value); // CodeableConcept 1673 else if (name.equals("location")) 1674 this.location = castToReference(value); // Reference 1675 else if (name.equals("policy")) 1676 this.getPolicy().add(castToUri(value)); 1677 else if (name.equals("agent")) 1678 this.getAgent().add((ProvenanceAgentComponent) value); 1679 else if (name.equals("entity")) 1680 this.getEntity().add((ProvenanceEntityComponent) value); 1681 else if (name.equals("signature")) 1682 this.getSignature().add(castToSignature(value)); 1683 else 1684 super.setProperty(name, value); 1685 } 1686 1687 @Override 1688 public Base addChild(String name) throws FHIRException { 1689 if (name.equals("target")) { 1690 return addTarget(); 1691 } else if (name.equals("period")) { 1692 this.period = new Period(); 1693 return this.period; 1694 } else if (name.equals("recorded")) { 1695 throw new FHIRException("Cannot call addChild on a singleton property Provenance.recorded"); 1696 } else if (name.equals("reason")) { 1697 return addReason(); 1698 } else if (name.equals("activity")) { 1699 this.activity = new CodeableConcept(); 1700 return this.activity; 1701 } else if (name.equals("location")) { 1702 this.location = new Reference(); 1703 return this.location; 1704 } else if (name.equals("policy")) { 1705 throw new FHIRException("Cannot call addChild on a singleton property Provenance.policy"); 1706 } else if (name.equals("agent")) { 1707 return addAgent(); 1708 } else if (name.equals("entity")) { 1709 return addEntity(); 1710 } else if (name.equals("signature")) { 1711 return addSignature(); 1712 } else 1713 return super.addChild(name); 1714 } 1715 1716 public String fhirType() { 1717 return "Provenance"; 1718 1719 } 1720 1721 public Provenance copy() { 1722 Provenance dst = new Provenance(); 1723 copyValues(dst); 1724 if (target != null) { 1725 dst.target = new ArrayList<Reference>(); 1726 for (Reference i : target) 1727 dst.target.add(i.copy()); 1728 } 1729 ; 1730 dst.period = period == null ? null : period.copy(); 1731 dst.recorded = recorded == null ? null : recorded.copy(); 1732 if (reason != null) { 1733 dst.reason = new ArrayList<CodeableConcept>(); 1734 for (CodeableConcept i : reason) 1735 dst.reason.add(i.copy()); 1736 } 1737 ; 1738 dst.activity = activity == null ? null : activity.copy(); 1739 dst.location = location == null ? null : location.copy(); 1740 if (policy != null) { 1741 dst.policy = new ArrayList<UriType>(); 1742 for (UriType i : policy) 1743 dst.policy.add(i.copy()); 1744 } 1745 ; 1746 if (agent != null) { 1747 dst.agent = new ArrayList<ProvenanceAgentComponent>(); 1748 for (ProvenanceAgentComponent i : agent) 1749 dst.agent.add(i.copy()); 1750 } 1751 ; 1752 if (entity != null) { 1753 dst.entity = new ArrayList<ProvenanceEntityComponent>(); 1754 for (ProvenanceEntityComponent i : entity) 1755 dst.entity.add(i.copy()); 1756 } 1757 ; 1758 if (signature != null) { 1759 dst.signature = new ArrayList<Signature>(); 1760 for (Signature i : signature) 1761 dst.signature.add(i.copy()); 1762 } 1763 ; 1764 return dst; 1765 } 1766 1767 protected Provenance typedCopy() { 1768 return copy(); 1769 } 1770 1771 @Override 1772 public boolean equalsDeep(Base other) { 1773 if (!super.equalsDeep(other)) 1774 return false; 1775 if (!(other instanceof Provenance)) 1776 return false; 1777 Provenance o = (Provenance) other; 1778 return compareDeep(target, o.target, true) && compareDeep(period, o.period, true) 1779 && compareDeep(recorded, o.recorded, true) && compareDeep(reason, o.reason, true) 1780 && compareDeep(activity, o.activity, true) && compareDeep(location, o.location, true) 1781 && compareDeep(policy, o.policy, true) && compareDeep(agent, o.agent, true) 1782 && compareDeep(entity, o.entity, true) && compareDeep(signature, o.signature, true); 1783 } 1784 1785 @Override 1786 public boolean equalsShallow(Base other) { 1787 if (!super.equalsShallow(other)) 1788 return false; 1789 if (!(other instanceof Provenance)) 1790 return false; 1791 Provenance o = (Provenance) other; 1792 return compareValues(recorded, o.recorded, true) && compareValues(policy, o.policy, true); 1793 } 1794 1795 public boolean isEmpty() { 1796 return super.isEmpty() && (target == null || target.isEmpty()) && (period == null || period.isEmpty()) 1797 && (recorded == null || recorded.isEmpty()) && (reason == null || reason.isEmpty()) 1798 && (activity == null || activity.isEmpty()) && (location == null || location.isEmpty()) 1799 && (policy == null || policy.isEmpty()) && (agent == null || agent.isEmpty()) 1800 && (entity == null || entity.isEmpty()) && (signature == null || signature.isEmpty()); 1801 } 1802 1803 @Override 1804 public ResourceType getResourceType() { 1805 return ResourceType.Provenance; 1806 } 1807 1808 @SearchParamDefinition(name = "sigtype", path = "Provenance.signature.type", description = "Indication of the reason the entity signed the object(s)", type = "token") 1809 public static final String SP_SIGTYPE = "sigtype"; 1810 @SearchParamDefinition(name = "agent", path = "Provenance.agent.actor", description = "Individual, device or organization playing role", type = "reference") 1811 public static final String SP_AGENT = "agent"; 1812 @SearchParamDefinition(name = "entitytype", path = "Provenance.entity.type", description = "The type of resource in this entity", type = "token") 1813 public static final String SP_ENTITYTYPE = "entitytype"; 1814 @SearchParamDefinition(name = "patient", path = "Provenance.target", description = "Target Reference(s) (usually version specific)", type = "reference") 1815 public static final String SP_PATIENT = "patient"; 1816 @SearchParamDefinition(name = "start", path = "Provenance.period.start", description = "Starting time with inclusive boundary", type = "date") 1817 public static final String SP_START = "start"; 1818 @SearchParamDefinition(name = "end", path = "Provenance.period.end", description = "End time with inclusive boundary, if not ongoing", type = "date") 1819 public static final String SP_END = "end"; 1820 @SearchParamDefinition(name = "location", path = "Provenance.location", description = "Where the activity occurred, if relevant", type = "reference") 1821 public static final String SP_LOCATION = "location"; 1822 @SearchParamDefinition(name = "userid", path = "Provenance.agent.userId", description = "Authorization-system identifier for the agent", type = "token") 1823 public static final String SP_USERID = "userid"; 1824 @SearchParamDefinition(name = "entity", path = "Provenance.entity.reference", description = "Identity of entity", type = "uri") 1825 public static final String SP_ENTITY = "entity"; 1826 @SearchParamDefinition(name = "target", path = "Provenance.target", description = "Target Reference(s) (usually version specific)", type = "reference") 1827 public static final String SP_TARGET = "target"; 1828 1829}