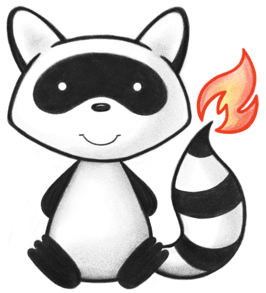
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.DatatypeDef; 039import ca.uhn.fhir.model.api.annotation.Description; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * A measured amount (or an amount that can potentially be measured). Note that 046 * measured amounts include amounts that are not precisely quantified, including 047 * amounts involving arbitrary units and floating currencies. 048 */ 049@DatatypeDef(name = "Quantity") 050public class Quantity extends Type implements ICompositeType { 051 052 public enum QuantityComparator { 053 /** 054 * The actual value is less than the given value. 055 */ 056 LESS_THAN, 057 /** 058 * The actual value is less than or equal to the given value. 059 */ 060 LESS_OR_EQUAL, 061 /** 062 * The actual value is greater than or equal to the given value. 063 */ 064 GREATER_OR_EQUAL, 065 /** 066 * The actual value is greater than the given value. 067 */ 068 GREATER_THAN, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static QuantityComparator fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("<".equals(codeString)) 078 return LESS_THAN; 079 if ("<=".equals(codeString)) 080 return LESS_OR_EQUAL; 081 if (">=".equals(codeString)) 082 return GREATER_OR_EQUAL; 083 if (">".equals(codeString)) 084 return GREATER_THAN; 085 throw new FHIRException("Unknown QuantityComparator code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case LESS_THAN: 091 return "<"; 092 case LESS_OR_EQUAL: 093 return "<="; 094 case GREATER_OR_EQUAL: 095 return ">="; 096 case GREATER_THAN: 097 return ">"; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105 public String getSystem() { 106 switch (this) { 107 case LESS_THAN: 108 return "http://hl7.org/fhir/quantity-comparator"; 109 case LESS_OR_EQUAL: 110 return "http://hl7.org/fhir/quantity-comparator"; 111 case GREATER_OR_EQUAL: 112 return "http://hl7.org/fhir/quantity-comparator"; 113 case GREATER_THAN: 114 return "http://hl7.org/fhir/quantity-comparator"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getDefinition() { 123 switch (this) { 124 case LESS_THAN: 125 return "The actual value is less than the given value."; 126 case LESS_OR_EQUAL: 127 return "The actual value is less than or equal to the given value."; 128 case GREATER_OR_EQUAL: 129 return "The actual value is greater than or equal to the given value."; 130 case GREATER_THAN: 131 return "The actual value is greater than the given value."; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDisplay() { 140 switch (this) { 141 case LESS_THAN: 142 return "Less than"; 143 case LESS_OR_EQUAL: 144 return "Less or Equal to"; 145 case GREATER_OR_EQUAL: 146 return "Greater or Equal to"; 147 case GREATER_THAN: 148 return "Greater than"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 } 156 157 public static class QuantityComparatorEnumFactory implements EnumFactory<QuantityComparator> { 158 public QuantityComparator fromCode(String codeString) throws IllegalArgumentException { 159 if (codeString == null || "".equals(codeString)) 160 if (codeString == null || "".equals(codeString)) 161 return null; 162 if ("<".equals(codeString)) 163 return QuantityComparator.LESS_THAN; 164 if ("<=".equals(codeString)) 165 return QuantityComparator.LESS_OR_EQUAL; 166 if (">=".equals(codeString)) 167 return QuantityComparator.GREATER_OR_EQUAL; 168 if (">".equals(codeString)) 169 return QuantityComparator.GREATER_THAN; 170 throw new IllegalArgumentException("Unknown QuantityComparator code '" + codeString + "'"); 171 } 172 173 public Enumeration<QuantityComparator> fromType(Base code) throws FHIRException { 174 if (code == null || code.isEmpty()) 175 return null; 176 String codeString = ((PrimitiveType) code).asStringValue(); 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("<".equals(codeString)) 180 return new Enumeration<QuantityComparator>(this, QuantityComparator.LESS_THAN); 181 if ("<=".equals(codeString)) 182 return new Enumeration<QuantityComparator>(this, QuantityComparator.LESS_OR_EQUAL); 183 if (">=".equals(codeString)) 184 return new Enumeration<QuantityComparator>(this, QuantityComparator.GREATER_OR_EQUAL); 185 if (">".equals(codeString)) 186 return new Enumeration<QuantityComparator>(this, QuantityComparator.GREATER_THAN); 187 throw new FHIRException("Unknown QuantityComparator code '" + codeString + "'"); 188 } 189 190 public String toCode(QuantityComparator code) 191 { 192 if (code == QuantityComparator.NULL) 193 return null; 194 if (code == QuantityComparator.LESS_THAN) 195 return "<"; 196 if (code == QuantityComparator.LESS_OR_EQUAL) 197 return "<="; 198 if (code == QuantityComparator.GREATER_OR_EQUAL) 199 return ">="; 200 if (code == QuantityComparator.GREATER_THAN) 201 return ">"; 202 return "?"; 203 } 204 } 205 206 /** 207 * The value of the measured amount. The value includes an implicit precision in 208 * the presentation of the value. 209 */ 210 @Child(name = "value", type = { DecimalType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 211 @Description(shortDefinition = "Numerical value (with implicit precision)", formalDefinition = "The value of the measured amount. The value includes an implicit precision in the presentation of the value.") 212 protected DecimalType value; 213 214 /** 215 * How the value should be understood and represented - whether the actual value 216 * is greater or less than the stated value due to measurement issues; e.g. if 217 * the comparator is "<" , then the real value is < stated value. 218 */ 219 @Child(name = "comparator", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 220 @Description(shortDefinition = "< | <= | >= | > - how to understand the value", formalDefinition = "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.") 221 protected Enumeration<QuantityComparator> comparator; 222 223 /** 224 * A human-readable form of the unit. 225 */ 226 @Child(name = "unit", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 227 @Description(shortDefinition = "Unit representation", formalDefinition = "A human-readable form of the unit.") 228 protected StringType unit; 229 230 /** 231 * The identification of the system that provides the coded form of the unit. 232 */ 233 @Child(name = "system", type = { UriType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 234 @Description(shortDefinition = "System that defines coded unit form", formalDefinition = "The identification of the system that provides the coded form of the unit.") 235 protected UriType system; 236 237 /** 238 * A computer processable form of the unit in some unit representation system. 239 */ 240 @Child(name = "code", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 241 @Description(shortDefinition = "Coded form of the unit", formalDefinition = "A computer processable form of the unit in some unit representation system.") 242 protected CodeType code; 243 244 private static final long serialVersionUID = 1069574054L; 245 246 /* 247 * Constructor 248 */ 249 public Quantity() { 250 super(); 251 } 252 253 /** 254 * @return {@link #value} (The value of the measured amount. The value includes 255 * an implicit precision in the presentation of the value.). This is the 256 * underlying object with id, value and extensions. The accessor 257 * "getValue" gives direct access to the value 258 */ 259 public DecimalType getValueElement() { 260 if (this.value == null) 261 if (Configuration.errorOnAutoCreate()) 262 throw new Error("Attempt to auto-create Quantity.value"); 263 else if (Configuration.doAutoCreate()) 264 this.value = new DecimalType(); // bb 265 return this.value; 266 } 267 268 public boolean hasValueElement() { 269 return this.value != null && !this.value.isEmpty(); 270 } 271 272 public boolean hasValue() { 273 return this.value != null && !this.value.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #value} (The value of the measured amount. The value 278 * includes an implicit precision in the presentation of the 279 * value.). This is the underlying object with id, value and 280 * extensions. The accessor "getValue" gives direct access to the 281 * value 282 */ 283 public Quantity setValueElement(DecimalType value) { 284 this.value = value; 285 return this; 286 } 287 288 /** 289 * @return The value of the measured amount. The value includes an implicit 290 * precision in the presentation of the value. 291 */ 292 public BigDecimal getValue() { 293 return this.value == null ? null : this.value.getValue(); 294 } 295 296 /** 297 * @param value The value of the measured amount. The value includes an implicit 298 * precision in the presentation of the value. 299 */ 300 public Quantity setValue(BigDecimal value) { 301 if (value == null) 302 this.value = null; 303 else { 304 if (this.value == null) 305 this.value = new DecimalType(); 306 this.value.setValue(value); 307 } 308 return this; 309 } 310 311 /** 312 * @return {@link #comparator} (How the value should be understood and 313 * represented - whether the actual value is greater or less than the 314 * stated value due to measurement issues; e.g. if the comparator is "<" 315 * , then the real value is < stated value.). This is the underlying 316 * object with id, value and extensions. The accessor "getComparator" 317 * gives direct access to the value 318 */ 319 public Enumeration<QuantityComparator> getComparatorElement() { 320 if (this.comparator == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create Quantity.comparator"); 323 else if (Configuration.doAutoCreate()) 324 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); // bb 325 return this.comparator; 326 } 327 328 public boolean hasComparatorElement() { 329 return this.comparator != null && !this.comparator.isEmpty(); 330 } 331 332 public boolean hasComparator() { 333 return this.comparator != null && !this.comparator.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #comparator} (How the value should be understood and 338 * represented - whether the actual value is greater or less than 339 * the stated value due to measurement issues; e.g. if the 340 * comparator is "<" , then the real value is < stated value.). 341 * This is the underlying object with id, value and extensions. The 342 * accessor "getComparator" gives direct access to the value 343 */ 344 public Quantity setComparatorElement(Enumeration<QuantityComparator> value) { 345 this.comparator = value; 346 return this; 347 } 348 349 /** 350 * @return How the value should be understood and represented - whether the 351 * actual value is greater or less than the stated value due to 352 * measurement issues; e.g. if the comparator is "<" , then the real 353 * value is < stated value. 354 */ 355 public QuantityComparator getComparator() { 356 return this.comparator == null ? null : this.comparator.getValue(); 357 } 358 359 /** 360 * @param value How the value should be understood and represented - whether the 361 * actual value is greater or less than the stated value due to 362 * measurement issues; e.g. if the comparator is "<" , then the 363 * real value is < stated value. 364 */ 365 public Quantity setComparator(QuantityComparator value) { 366 if (value == null) 367 this.comparator = null; 368 else { 369 if (this.comparator == null) 370 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); 371 this.comparator.setValue(value); 372 } 373 return this; 374 } 375 376 /** 377 * @return {@link #unit} (A human-readable form of the unit.). This is the 378 * underlying object with id, value and extensions. The accessor 379 * "getUnit" gives direct access to the value 380 */ 381 public StringType getUnitElement() { 382 if (this.unit == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create Quantity.unit"); 385 else if (Configuration.doAutoCreate()) 386 this.unit = new StringType(); // bb 387 return this.unit; 388 } 389 390 public boolean hasUnitElement() { 391 return this.unit != null && !this.unit.isEmpty(); 392 } 393 394 public boolean hasUnit() { 395 return this.unit != null && !this.unit.isEmpty(); 396 } 397 398 /** 399 * @param value {@link #unit} (A human-readable form of the unit.). This is the 400 * underlying object with id, value and extensions. The accessor 401 * "getUnit" gives direct access to the value 402 */ 403 public Quantity setUnitElement(StringType value) { 404 this.unit = value; 405 return this; 406 } 407 408 /** 409 * @return A human-readable form of the unit. 410 */ 411 public String getUnit() { 412 return this.unit == null ? null : this.unit.getValue(); 413 } 414 415 /** 416 * @param value A human-readable form of the unit. 417 */ 418 public Quantity setUnit(String value) { 419 if (Utilities.noString(value)) 420 this.unit = null; 421 else { 422 if (this.unit == null) 423 this.unit = new StringType(); 424 this.unit.setValue(value); 425 } 426 return this; 427 } 428 429 /** 430 * @return {@link #system} (The identification of the system that provides the 431 * coded form of the unit.). This is the underlying object with id, 432 * value and extensions. The accessor "getSystem" gives direct access to 433 * the value 434 */ 435 public UriType getSystemElement() { 436 if (this.system == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create Quantity.system"); 439 else if (Configuration.doAutoCreate()) 440 this.system = new UriType(); // bb 441 return this.system; 442 } 443 444 public boolean hasSystemElement() { 445 return this.system != null && !this.system.isEmpty(); 446 } 447 448 public boolean hasSystem() { 449 return this.system != null && !this.system.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #system} (The identification of the system that provides 454 * the coded form of the unit.). This is the underlying object with 455 * id, value and extensions. The accessor "getSystem" gives direct 456 * access to the value 457 */ 458 public Quantity setSystemElement(UriType value) { 459 this.system = value; 460 return this; 461 } 462 463 /** 464 * @return The identification of the system that provides the coded form of the 465 * unit. 466 */ 467 public String getSystem() { 468 return this.system == null ? null : this.system.getValue(); 469 } 470 471 /** 472 * @param value The identification of the system that provides the coded form of 473 * the unit. 474 */ 475 public Quantity setSystem(String value) { 476 if (Utilities.noString(value)) 477 this.system = null; 478 else { 479 if (this.system == null) 480 this.system = new UriType(); 481 this.system.setValue(value); 482 } 483 return this; 484 } 485 486 /** 487 * @return {@link #code} (A computer processable form of the unit in some unit 488 * representation system.). This is the underlying object with id, value 489 * and extensions. The accessor "getCode" gives direct access to the 490 * value 491 */ 492 public CodeType getCodeElement() { 493 if (this.code == null) 494 if (Configuration.errorOnAutoCreate()) 495 throw new Error("Attempt to auto-create Quantity.code"); 496 else if (Configuration.doAutoCreate()) 497 this.code = new CodeType(); // bb 498 return this.code; 499 } 500 501 public boolean hasCodeElement() { 502 return this.code != null && !this.code.isEmpty(); 503 } 504 505 public boolean hasCode() { 506 return this.code != null && !this.code.isEmpty(); 507 } 508 509 /** 510 * @param value {@link #code} (A computer processable form of the unit in some 511 * unit representation system.). This is the underlying object with 512 * id, value and extensions. The accessor "getCode" gives direct 513 * access to the value 514 */ 515 public Quantity setCodeElement(CodeType value) { 516 this.code = value; 517 return this; 518 } 519 520 /** 521 * @return A computer processable form of the unit in some unit representation 522 * system. 523 */ 524 public String getCode() { 525 return this.code == null ? null : this.code.getValue(); 526 } 527 528 /** 529 * @param value A computer processable form of the unit in some unit 530 * representation system. 531 */ 532 public Quantity setCode(String value) { 533 if (Utilities.noString(value)) 534 this.code = null; 535 else { 536 if (this.code == null) 537 this.code = new CodeType(); 538 this.code.setValue(value); 539 } 540 return this; 541 } 542 543 protected void listChildren(List<Property> childrenList) { 544 super.listChildren(childrenList); 545 childrenList.add(new Property("value", "decimal", 546 "The value of the measured amount. The value includes an implicit precision in the presentation of the value.", 547 0, java.lang.Integer.MAX_VALUE, value)); 548 childrenList.add(new Property("comparator", "code", 549 "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.", 550 0, java.lang.Integer.MAX_VALUE, comparator)); 551 childrenList.add( 552 new Property("unit", "string", "A human-readable form of the unit.", 0, java.lang.Integer.MAX_VALUE, unit)); 553 childrenList 554 .add(new Property("system", "uri", "The identification of the system that provides the coded form of the unit.", 555 0, java.lang.Integer.MAX_VALUE, system)); 556 childrenList 557 .add(new Property("code", "code", "A computer processable form of the unit in some unit representation system.", 558 0, java.lang.Integer.MAX_VALUE, code)); 559 } 560 561 @Override 562 public void setProperty(String name, Base value) throws FHIRException { 563 if (name.equals("value")) 564 this.value = castToDecimal(value); // DecimalType 565 else if (name.equals("comparator")) 566 this.comparator = new QuantityComparatorEnumFactory().fromType(value); // Enumeration<QuantityComparator> 567 else if (name.equals("unit")) 568 this.unit = castToString(value); // StringType 569 else if (name.equals("system")) 570 this.system = castToUri(value); // UriType 571 else if (name.equals("code")) 572 this.code = castToCode(value); // CodeType 573 else 574 super.setProperty(name, value); 575 } 576 577 @Override 578 public Base addChild(String name) throws FHIRException { 579 if (name.equals("value")) { 580 throw new FHIRException("Cannot call addChild on a singleton property Quantity.value"); 581 } else if (name.equals("comparator")) { 582 throw new FHIRException("Cannot call addChild on a singleton property Quantity.comparator"); 583 } else if (name.equals("unit")) { 584 throw new FHIRException("Cannot call addChild on a singleton property Quantity.unit"); 585 } else if (name.equals("system")) { 586 throw new FHIRException("Cannot call addChild on a singleton property Quantity.system"); 587 } else if (name.equals("code")) { 588 throw new FHIRException("Cannot call addChild on a singleton property Quantity.code"); 589 } else 590 return super.addChild(name); 591 } 592 593 public String fhirType() { 594 return "Quantity"; 595 596 } 597 598 public Quantity copy() { 599 Quantity dst = new Quantity(); 600 copyValues(dst); 601 dst.value = value == null ? null : value.copy(); 602 dst.comparator = comparator == null ? null : comparator.copy(); 603 dst.unit = unit == null ? null : unit.copy(); 604 dst.system = system == null ? null : system.copy(); 605 dst.code = code == null ? null : code.copy(); 606 return dst; 607 } 608 609 protected Quantity typedCopy() { 610 return copy(); 611 } 612 613 @Override 614 public boolean equalsDeep(Base other) { 615 if (!super.equalsDeep(other)) 616 return false; 617 if (!(other instanceof Quantity)) 618 return false; 619 Quantity o = (Quantity) other; 620 return compareDeep(value, o.value, true) && compareDeep(comparator, o.comparator, true) 621 && compareDeep(unit, o.unit, true) && compareDeep(system, o.system, true) && compareDeep(code, o.code, true); 622 } 623 624 @Override 625 public boolean equalsShallow(Base other) { 626 if (!super.equalsShallow(other)) 627 return false; 628 if (!(other instanceof Quantity)) 629 return false; 630 Quantity o = (Quantity) other; 631 return compareValues(value, o.value, true) && compareValues(comparator, o.comparator, true) 632 && compareValues(unit, o.unit, true) && compareValues(system, o.system, true) 633 && compareValues(code, o.code, true); 634 } 635 636 public boolean isEmpty() { 637 return super.isEmpty() && (value == null || value.isEmpty()) && (comparator == null || comparator.isEmpty()) 638 && (unit == null || unit.isEmpty()) && (system == null || system.isEmpty()) && (code == null || code.isEmpty()); 639 } 640 641}