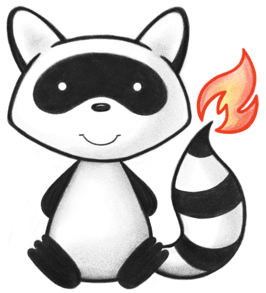
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A structured set of questions intended to guide the collection of answers. 048 * The questions are ordered and grouped into coherent subsets, corresponding to 049 * the structure of the grouping of the underlying questions. 050 */ 051@ResourceDef(name = "Questionnaire", profile = "http://hl7.org/fhir/Profile/Questionnaire") 052public class Questionnaire extends DomainResource { 053 054 public enum QuestionnaireStatus { 055 /** 056 * This Questionnaire is not ready for official use. 057 */ 058 DRAFT, 059 /** 060 * This Questionnaire is ready for use. 061 */ 062 PUBLISHED, 063 /** 064 * This Questionnaire should no longer be used to gather data. 065 */ 066 RETIRED, 067 /** 068 * added to help the parsers 069 */ 070 NULL; 071 072 public static QuestionnaireStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("draft".equals(codeString)) 076 return DRAFT; 077 if ("published".equals(codeString)) 078 return PUBLISHED; 079 if ("retired".equals(codeString)) 080 return RETIRED; 081 throw new FHIRException("Unknown QuestionnaireStatus code '" + codeString + "'"); 082 } 083 084 public String toCode() { 085 switch (this) { 086 case DRAFT: 087 return "draft"; 088 case PUBLISHED: 089 return "published"; 090 case RETIRED: 091 return "retired"; 092 case NULL: 093 return null; 094 default: 095 return "?"; 096 } 097 } 098 099 public String getSystem() { 100 switch (this) { 101 case DRAFT: 102 return "http://hl7.org/fhir/questionnaire-status"; 103 case PUBLISHED: 104 return "http://hl7.org/fhir/questionnaire-status"; 105 case RETIRED: 106 return "http://hl7.org/fhir/questionnaire-status"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getDefinition() { 115 switch (this) { 116 case DRAFT: 117 return "This Questionnaire is not ready for official use."; 118 case PUBLISHED: 119 return "This Questionnaire is ready for use."; 120 case RETIRED: 121 return "This Questionnaire should no longer be used to gather data."; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDisplay() { 130 switch (this) { 131 case DRAFT: 132 return "Draft"; 133 case PUBLISHED: 134 return "Published"; 135 case RETIRED: 136 return "Retired"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 } 144 145 public static class QuestionnaireStatusEnumFactory implements EnumFactory<QuestionnaireStatus> { 146 public QuestionnaireStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("draft".equals(codeString)) 151 return QuestionnaireStatus.DRAFT; 152 if ("published".equals(codeString)) 153 return QuestionnaireStatus.PUBLISHED; 154 if ("retired".equals(codeString)) 155 return QuestionnaireStatus.RETIRED; 156 throw new IllegalArgumentException("Unknown QuestionnaireStatus code '" + codeString + "'"); 157 } 158 159 public Enumeration<QuestionnaireStatus> fromType(Base code) throws FHIRException { 160 if (code == null || code.isEmpty()) 161 return null; 162 String codeString = ((PrimitiveType) code).asStringValue(); 163 if (codeString == null || "".equals(codeString)) 164 return null; 165 if ("draft".equals(codeString)) 166 return new Enumeration<QuestionnaireStatus>(this, QuestionnaireStatus.DRAFT); 167 if ("published".equals(codeString)) 168 return new Enumeration<QuestionnaireStatus>(this, QuestionnaireStatus.PUBLISHED); 169 if ("retired".equals(codeString)) 170 return new Enumeration<QuestionnaireStatus>(this, QuestionnaireStatus.RETIRED); 171 throw new FHIRException("Unknown QuestionnaireStatus code '" + codeString + "'"); 172 } 173 174 public String toCode(QuestionnaireStatus code) 175 { 176 if (code == QuestionnaireStatus.NULL) 177 return null; 178 if (code == QuestionnaireStatus.DRAFT) 179 return "draft"; 180 if (code == QuestionnaireStatus.PUBLISHED) 181 return "published"; 182 if (code == QuestionnaireStatus.RETIRED) 183 return "retired"; 184 return "?"; 185 } 186 } 187 188 public enum AnswerFormat { 189 /** 190 * Answer is a yes/no answer. 191 */ 192 BOOLEAN, 193 /** 194 * Answer is a floating point number. 195 */ 196 DECIMAL, 197 /** 198 * Answer is an integer. 199 */ 200 INTEGER, 201 /** 202 * Answer is a date. 203 */ 204 DATE, 205 /** 206 * Answer is a date and time. 207 */ 208 DATETIME, 209 /** 210 * Answer is a system timestamp. 211 */ 212 INSTANT, 213 /** 214 * Answer is a time (hour/minute/second) independent of date. 215 */ 216 TIME, 217 /** 218 * Answer is a short (few words to short sentence) free-text entry. 219 */ 220 STRING, 221 /** 222 * Answer is a long (potentially multi-paragraph) free-text entry (still 223 * captured as a string). 224 */ 225 TEXT, 226 /** 227 * Answer is a url (website, FTP site, etc.). 228 */ 229 URL, 230 /** 231 * Answer is a Coding drawn from a list of options. 232 */ 233 CHOICE, 234 /** 235 * Answer is a Coding drawn from a list of options or a free-text entry. 236 */ 237 OPENCHOICE, 238 /** 239 * Answer is binary content such as a image, PDF, etc. 240 */ 241 ATTACHMENT, 242 /** 243 * Answer is a reference to another resource (practitioner, organization, etc.). 244 */ 245 REFERENCE, 246 /** 247 * Answer is a combination of a numeric value and unit, potentially with a 248 * comparator (<, >, etc.). 249 */ 250 QUANTITY, 251 /** 252 * added to help the parsers 253 */ 254 NULL; 255 256 public static AnswerFormat fromCode(String codeString) throws FHIRException { 257 if (codeString == null || "".equals(codeString)) 258 return null; 259 if ("boolean".equals(codeString)) 260 return BOOLEAN; 261 if ("decimal".equals(codeString)) 262 return DECIMAL; 263 if ("integer".equals(codeString)) 264 return INTEGER; 265 if ("date".equals(codeString)) 266 return DATE; 267 if ("dateTime".equals(codeString)) 268 return DATETIME; 269 if ("instant".equals(codeString)) 270 return INSTANT; 271 if ("time".equals(codeString)) 272 return TIME; 273 if ("string".equals(codeString)) 274 return STRING; 275 if ("text".equals(codeString)) 276 return TEXT; 277 if ("url".equals(codeString)) 278 return URL; 279 if ("choice".equals(codeString)) 280 return CHOICE; 281 if ("open-choice".equals(codeString)) 282 return OPENCHOICE; 283 if ("attachment".equals(codeString)) 284 return ATTACHMENT; 285 if ("reference".equals(codeString)) 286 return REFERENCE; 287 if ("quantity".equals(codeString)) 288 return QUANTITY; 289 throw new FHIRException("Unknown AnswerFormat code '" + codeString + "'"); 290 } 291 292 public String toCode() { 293 switch (this) { 294 case BOOLEAN: 295 return "boolean"; 296 case DECIMAL: 297 return "decimal"; 298 case INTEGER: 299 return "integer"; 300 case DATE: 301 return "date"; 302 case DATETIME: 303 return "dateTime"; 304 case INSTANT: 305 return "instant"; 306 case TIME: 307 return "time"; 308 case STRING: 309 return "string"; 310 case TEXT: 311 return "text"; 312 case URL: 313 return "url"; 314 case CHOICE: 315 return "choice"; 316 case OPENCHOICE: 317 return "open-choice"; 318 case ATTACHMENT: 319 return "attachment"; 320 case REFERENCE: 321 return "reference"; 322 case QUANTITY: 323 return "quantity"; 324 case NULL: 325 return null; 326 default: 327 return "?"; 328 } 329 } 330 331 public String getSystem() { 332 switch (this) { 333 case BOOLEAN: 334 return "http://hl7.org/fhir/answer-format"; 335 case DECIMAL: 336 return "http://hl7.org/fhir/answer-format"; 337 case INTEGER: 338 return "http://hl7.org/fhir/answer-format"; 339 case DATE: 340 return "http://hl7.org/fhir/answer-format"; 341 case DATETIME: 342 return "http://hl7.org/fhir/answer-format"; 343 case INSTANT: 344 return "http://hl7.org/fhir/answer-format"; 345 case TIME: 346 return "http://hl7.org/fhir/answer-format"; 347 case STRING: 348 return "http://hl7.org/fhir/answer-format"; 349 case TEXT: 350 return "http://hl7.org/fhir/answer-format"; 351 case URL: 352 return "http://hl7.org/fhir/answer-format"; 353 case CHOICE: 354 return "http://hl7.org/fhir/answer-format"; 355 case OPENCHOICE: 356 return "http://hl7.org/fhir/answer-format"; 357 case ATTACHMENT: 358 return "http://hl7.org/fhir/answer-format"; 359 case REFERENCE: 360 return "http://hl7.org/fhir/answer-format"; 361 case QUANTITY: 362 return "http://hl7.org/fhir/answer-format"; 363 case NULL: 364 return null; 365 default: 366 return "?"; 367 } 368 } 369 370 public String getDefinition() { 371 switch (this) { 372 case BOOLEAN: 373 return "Answer is a yes/no answer."; 374 case DECIMAL: 375 return "Answer is a floating point number."; 376 case INTEGER: 377 return "Answer is an integer."; 378 case DATE: 379 return "Answer is a date."; 380 case DATETIME: 381 return "Answer is a date and time."; 382 case INSTANT: 383 return "Answer is a system timestamp."; 384 case TIME: 385 return "Answer is a time (hour/minute/second) independent of date."; 386 case STRING: 387 return "Answer is a short (few words to short sentence) free-text entry."; 388 case TEXT: 389 return "Answer is a long (potentially multi-paragraph) free-text entry (still captured as a string)."; 390 case URL: 391 return "Answer is a url (website, FTP site, etc.)."; 392 case CHOICE: 393 return "Answer is a Coding drawn from a list of options."; 394 case OPENCHOICE: 395 return "Answer is a Coding drawn from a list of options or a free-text entry."; 396 case ATTACHMENT: 397 return "Answer is binary content such as a image, PDF, etc."; 398 case REFERENCE: 399 return "Answer is a reference to another resource (practitioner, organization, etc.)."; 400 case QUANTITY: 401 return "Answer is a combination of a numeric value and unit, potentially with a comparator (<, >, etc.)."; 402 case NULL: 403 return null; 404 default: 405 return "?"; 406 } 407 } 408 409 public String getDisplay() { 410 switch (this) { 411 case BOOLEAN: 412 return "Boolean"; 413 case DECIMAL: 414 return "Decimal"; 415 case INTEGER: 416 return "Integer"; 417 case DATE: 418 return "Date"; 419 case DATETIME: 420 return "Date Time"; 421 case INSTANT: 422 return "Instant"; 423 case TIME: 424 return "Time"; 425 case STRING: 426 return "String"; 427 case TEXT: 428 return "Text"; 429 case URL: 430 return "Url"; 431 case CHOICE: 432 return "Choice"; 433 case OPENCHOICE: 434 return "Open Choice"; 435 case ATTACHMENT: 436 return "Attachment"; 437 case REFERENCE: 438 return "Reference"; 439 case QUANTITY: 440 return "Quantity"; 441 case NULL: 442 return null; 443 default: 444 return "?"; 445 } 446 } 447 } 448 449 public static class AnswerFormatEnumFactory implements EnumFactory<AnswerFormat> { 450 public AnswerFormat fromCode(String codeString) throws IllegalArgumentException { 451 if (codeString == null || "".equals(codeString)) 452 if (codeString == null || "".equals(codeString)) 453 return null; 454 if ("boolean".equals(codeString)) 455 return AnswerFormat.BOOLEAN; 456 if ("decimal".equals(codeString)) 457 return AnswerFormat.DECIMAL; 458 if ("integer".equals(codeString)) 459 return AnswerFormat.INTEGER; 460 if ("date".equals(codeString)) 461 return AnswerFormat.DATE; 462 if ("dateTime".equals(codeString)) 463 return AnswerFormat.DATETIME; 464 if ("instant".equals(codeString)) 465 return AnswerFormat.INSTANT; 466 if ("time".equals(codeString)) 467 return AnswerFormat.TIME; 468 if ("string".equals(codeString)) 469 return AnswerFormat.STRING; 470 if ("text".equals(codeString)) 471 return AnswerFormat.TEXT; 472 if ("url".equals(codeString)) 473 return AnswerFormat.URL; 474 if ("choice".equals(codeString)) 475 return AnswerFormat.CHOICE; 476 if ("open-choice".equals(codeString)) 477 return AnswerFormat.OPENCHOICE; 478 if ("attachment".equals(codeString)) 479 return AnswerFormat.ATTACHMENT; 480 if ("reference".equals(codeString)) 481 return AnswerFormat.REFERENCE; 482 if ("quantity".equals(codeString)) 483 return AnswerFormat.QUANTITY; 484 throw new IllegalArgumentException("Unknown AnswerFormat code '" + codeString + "'"); 485 } 486 487 public Enumeration<AnswerFormat> fromType(Base code) throws FHIRException { 488 if (code == null || code.isEmpty()) 489 return null; 490 String codeString = ((PrimitiveType) code).asStringValue(); 491 if (codeString == null || "".equals(codeString)) 492 return null; 493 if ("boolean".equals(codeString)) 494 return new Enumeration<AnswerFormat>(this, AnswerFormat.BOOLEAN); 495 if ("decimal".equals(codeString)) 496 return new Enumeration<AnswerFormat>(this, AnswerFormat.DECIMAL); 497 if ("integer".equals(codeString)) 498 return new Enumeration<AnswerFormat>(this, AnswerFormat.INTEGER); 499 if ("date".equals(codeString)) 500 return new Enumeration<AnswerFormat>(this, AnswerFormat.DATE); 501 if ("dateTime".equals(codeString)) 502 return new Enumeration<AnswerFormat>(this, AnswerFormat.DATETIME); 503 if ("instant".equals(codeString)) 504 return new Enumeration<AnswerFormat>(this, AnswerFormat.INSTANT); 505 if ("time".equals(codeString)) 506 return new Enumeration<AnswerFormat>(this, AnswerFormat.TIME); 507 if ("string".equals(codeString)) 508 return new Enumeration<AnswerFormat>(this, AnswerFormat.STRING); 509 if ("text".equals(codeString)) 510 return new Enumeration<AnswerFormat>(this, AnswerFormat.TEXT); 511 if ("url".equals(codeString)) 512 return new Enumeration<AnswerFormat>(this, AnswerFormat.URL); 513 if ("choice".equals(codeString)) 514 return new Enumeration<AnswerFormat>(this, AnswerFormat.CHOICE); 515 if ("open-choice".equals(codeString)) 516 return new Enumeration<AnswerFormat>(this, AnswerFormat.OPENCHOICE); 517 if ("attachment".equals(codeString)) 518 return new Enumeration<AnswerFormat>(this, AnswerFormat.ATTACHMENT); 519 if ("reference".equals(codeString)) 520 return new Enumeration<AnswerFormat>(this, AnswerFormat.REFERENCE); 521 if ("quantity".equals(codeString)) 522 return new Enumeration<AnswerFormat>(this, AnswerFormat.QUANTITY); 523 throw new FHIRException("Unknown AnswerFormat code '" + codeString + "'"); 524 } 525 526 public String toCode(AnswerFormat code) 527 { 528 if (code == AnswerFormat.NULL) 529 return null; 530 if (code == AnswerFormat.BOOLEAN) 531 return "boolean"; 532 if (code == AnswerFormat.DECIMAL) 533 return "decimal"; 534 if (code == AnswerFormat.INTEGER) 535 return "integer"; 536 if (code == AnswerFormat.DATE) 537 return "date"; 538 if (code == AnswerFormat.DATETIME) 539 return "dateTime"; 540 if (code == AnswerFormat.INSTANT) 541 return "instant"; 542 if (code == AnswerFormat.TIME) 543 return "time"; 544 if (code == AnswerFormat.STRING) 545 return "string"; 546 if (code == AnswerFormat.TEXT) 547 return "text"; 548 if (code == AnswerFormat.URL) 549 return "url"; 550 if (code == AnswerFormat.CHOICE) 551 return "choice"; 552 if (code == AnswerFormat.OPENCHOICE) 553 return "open-choice"; 554 if (code == AnswerFormat.ATTACHMENT) 555 return "attachment"; 556 if (code == AnswerFormat.REFERENCE) 557 return "reference"; 558 if (code == AnswerFormat.QUANTITY) 559 return "quantity"; 560 return "?"; 561 } 562 } 563 564 @Block() 565 public static class GroupComponent extends BackboneElement implements IBaseBackboneElement { 566 /** 567 * An identifier that is unique within the Questionnaire allowing linkage to the 568 * equivalent group in a QuestionnaireResponse resource. 569 */ 570 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 571 @Description(shortDefinition = "To link questionnaire with questionnaire response", formalDefinition = "An identifier that is unique within the Questionnaire allowing linkage to the equivalent group in a QuestionnaireResponse resource.") 572 protected StringType linkId; 573 574 /** 575 * The human-readable name for this section of the questionnaire. 576 */ 577 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 578 @Description(shortDefinition = "Name to be displayed for group", formalDefinition = "The human-readable name for this section of the questionnaire.") 579 protected StringType title; 580 581 /** 582 * Identifies a how this group of questions is known in a particular terminology 583 * such as LOINC. 584 */ 585 @Child(name = "concept", type = { 586 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 587 @Description(shortDefinition = "Concept that represents this section in a questionnaire", formalDefinition = "Identifies a how this group of questions is known in a particular terminology such as LOINC.") 588 protected List<Coding> concept; 589 590 /** 591 * Additional text for the group, used for display purposes. 592 */ 593 @Child(name = "text", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 594 @Description(shortDefinition = "Additional text for the group", formalDefinition = "Additional text for the group, used for display purposes.") 595 protected StringType text; 596 597 /** 598 * If true, indicates that the group must be present and have required questions 599 * within it answered. If false, the group may be skipped when answering the 600 * questionnaire. 601 */ 602 @Child(name = "required", type = { 603 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 604 @Description(shortDefinition = "Whether the group must be included in data results", formalDefinition = "If true, indicates that the group must be present and have required questions within it answered. If false, the group may be skipped when answering the questionnaire.") 605 protected BooleanType required; 606 607 /** 608 * Whether the group may occur multiple times in the instance, containing 609 * multiple sets of answers. 610 */ 611 @Child(name = "repeats", type = { 612 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 613 @Description(shortDefinition = "Whether the group may repeat", formalDefinition = "Whether the group may occur multiple times in the instance, containing multiple sets of answers.") 614 protected BooleanType repeats; 615 616 /** 617 * A sub-group within a group. The ordering of groups within this group is 618 * relevant. 619 */ 620 @Child(name = "group", type = { 621 GroupComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 622 @Description(shortDefinition = "Nested questionnaire group", formalDefinition = "A sub-group within a group. The ordering of groups within this group is relevant.") 623 protected List<GroupComponent> group; 624 625 /** 626 * Set of questions within this group. The order of questions within the group 627 * is relevant. 628 */ 629 @Child(name = "question", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 630 @Description(shortDefinition = "Questions in this group", formalDefinition = "Set of questions within this group. The order of questions within the group is relevant.") 631 protected List<QuestionComponent> question; 632 633 private static final long serialVersionUID = 494129548L; 634 635 /* 636 * Constructor 637 */ 638 public GroupComponent() { 639 super(); 640 } 641 642 /** 643 * @return {@link #linkId} (An identifier that is unique within the 644 * Questionnaire allowing linkage to the equivalent group in a 645 * QuestionnaireResponse resource.). This is the underlying object with 646 * id, value and extensions. The accessor "getLinkId" gives direct 647 * access to the value 648 */ 649 public StringType getLinkIdElement() { 650 if (this.linkId == null) 651 if (Configuration.errorOnAutoCreate()) 652 throw new Error("Attempt to auto-create GroupComponent.linkId"); 653 else if (Configuration.doAutoCreate()) 654 this.linkId = new StringType(); // bb 655 return this.linkId; 656 } 657 658 public boolean hasLinkIdElement() { 659 return this.linkId != null && !this.linkId.isEmpty(); 660 } 661 662 public boolean hasLinkId() { 663 return this.linkId != null && !this.linkId.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #linkId} (An identifier that is unique within the 668 * Questionnaire allowing linkage to the equivalent group in a 669 * QuestionnaireResponse resource.). This is the underlying object 670 * with id, value and extensions. The accessor "getLinkId" gives 671 * direct access to the value 672 */ 673 public GroupComponent setLinkIdElement(StringType value) { 674 this.linkId = value; 675 return this; 676 } 677 678 /** 679 * @return An identifier that is unique within the Questionnaire allowing 680 * linkage to the equivalent group in a QuestionnaireResponse resource. 681 */ 682 public String getLinkId() { 683 return this.linkId == null ? null : this.linkId.getValue(); 684 } 685 686 /** 687 * @param value An identifier that is unique within the Questionnaire allowing 688 * linkage to the equivalent group in a QuestionnaireResponse 689 * resource. 690 */ 691 public GroupComponent setLinkId(String value) { 692 if (Utilities.noString(value)) 693 this.linkId = null; 694 else { 695 if (this.linkId == null) 696 this.linkId = new StringType(); 697 this.linkId.setValue(value); 698 } 699 return this; 700 } 701 702 /** 703 * @return {@link #title} (The human-readable name for this section of the 704 * questionnaire.). This is the underlying object with id, value and 705 * extensions. The accessor "getTitle" gives direct access to the value 706 */ 707 public StringType getTitleElement() { 708 if (this.title == null) 709 if (Configuration.errorOnAutoCreate()) 710 throw new Error("Attempt to auto-create GroupComponent.title"); 711 else if (Configuration.doAutoCreate()) 712 this.title = new StringType(); // bb 713 return this.title; 714 } 715 716 public boolean hasTitleElement() { 717 return this.title != null && !this.title.isEmpty(); 718 } 719 720 public boolean hasTitle() { 721 return this.title != null && !this.title.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #title} (The human-readable name for this section of the 726 * questionnaire.). This is the underlying object with id, value 727 * and extensions. The accessor "getTitle" gives direct access to 728 * the value 729 */ 730 public GroupComponent setTitleElement(StringType value) { 731 this.title = value; 732 return this; 733 } 734 735 /** 736 * @return The human-readable name for this section of the questionnaire. 737 */ 738 public String getTitle() { 739 return this.title == null ? null : this.title.getValue(); 740 } 741 742 /** 743 * @param value The human-readable name for this section of the questionnaire. 744 */ 745 public GroupComponent setTitle(String value) { 746 if (Utilities.noString(value)) 747 this.title = null; 748 else { 749 if (this.title == null) 750 this.title = new StringType(); 751 this.title.setValue(value); 752 } 753 return this; 754 } 755 756 /** 757 * @return {@link #concept} (Identifies a how this group of questions is known 758 * in a particular terminology such as LOINC.) 759 */ 760 public List<Coding> getConcept() { 761 if (this.concept == null) 762 this.concept = new ArrayList<Coding>(); 763 return this.concept; 764 } 765 766 public boolean hasConcept() { 767 if (this.concept == null) 768 return false; 769 for (Coding item : this.concept) 770 if (!item.isEmpty()) 771 return true; 772 return false; 773 } 774 775 /** 776 * @return {@link #concept} (Identifies a how this group of questions is known 777 * in a particular terminology such as LOINC.) 778 */ 779 // syntactic sugar 780 public Coding addConcept() { // 3 781 Coding t = new Coding(); 782 if (this.concept == null) 783 this.concept = new ArrayList<Coding>(); 784 this.concept.add(t); 785 return t; 786 } 787 788 // syntactic sugar 789 public GroupComponent addConcept(Coding t) { // 3 790 if (t == null) 791 return this; 792 if (this.concept == null) 793 this.concept = new ArrayList<Coding>(); 794 this.concept.add(t); 795 return this; 796 } 797 798 /** 799 * @return {@link #text} (Additional text for the group, used for display 800 * purposes.). This is the underlying object with id, value and 801 * extensions. The accessor "getText" gives direct access to the value 802 */ 803 public StringType getTextElement() { 804 if (this.text == null) 805 if (Configuration.errorOnAutoCreate()) 806 throw new Error("Attempt to auto-create GroupComponent.text"); 807 else if (Configuration.doAutoCreate()) 808 this.text = new StringType(); // bb 809 return this.text; 810 } 811 812 public boolean hasTextElement() { 813 return this.text != null && !this.text.isEmpty(); 814 } 815 816 public boolean hasText() { 817 return this.text != null && !this.text.isEmpty(); 818 } 819 820 /** 821 * @param value {@link #text} (Additional text for the group, used for display 822 * purposes.). This is the underlying object with id, value and 823 * extensions. The accessor "getText" gives direct access to the 824 * value 825 */ 826 public GroupComponent setTextElement(StringType value) { 827 this.text = value; 828 return this; 829 } 830 831 /** 832 * @return Additional text for the group, used for display purposes. 833 */ 834 public String getText() { 835 return this.text == null ? null : this.text.getValue(); 836 } 837 838 /** 839 * @param value Additional text for the group, used for display purposes. 840 */ 841 public GroupComponent setText(String value) { 842 if (Utilities.noString(value)) 843 this.text = null; 844 else { 845 if (this.text == null) 846 this.text = new StringType(); 847 this.text.setValue(value); 848 } 849 return this; 850 } 851 852 /** 853 * @return {@link #required} (If true, indicates that the group must be present 854 * and have required questions within it answered. If false, the group 855 * may be skipped when answering the questionnaire.). This is the 856 * underlying object with id, value and extensions. The accessor 857 * "getRequired" gives direct access to the value 858 */ 859 public BooleanType getRequiredElement() { 860 if (this.required == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create GroupComponent.required"); 863 else if (Configuration.doAutoCreate()) 864 this.required = new BooleanType(); // bb 865 return this.required; 866 } 867 868 public boolean hasRequiredElement() { 869 return this.required != null && !this.required.isEmpty(); 870 } 871 872 public boolean hasRequired() { 873 return this.required != null && !this.required.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #required} (If true, indicates that the group must be 878 * present and have required questions within it answered. If 879 * false, the group may be skipped when answering the 880 * questionnaire.). This is the underlying object with id, value 881 * and extensions. The accessor "getRequired" gives direct access 882 * to the value 883 */ 884 public GroupComponent setRequiredElement(BooleanType value) { 885 this.required = value; 886 return this; 887 } 888 889 /** 890 * @return If true, indicates that the group must be present and have required 891 * questions within it answered. If false, the group may be skipped when 892 * answering the questionnaire. 893 */ 894 public boolean getRequired() { 895 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 896 } 897 898 /** 899 * @param value If true, indicates that the group must be present and have 900 * required questions within it answered. If false, the group may 901 * be skipped when answering the questionnaire. 902 */ 903 public GroupComponent setRequired(boolean value) { 904 if (this.required == null) 905 this.required = new BooleanType(); 906 this.required.setValue(value); 907 return this; 908 } 909 910 /** 911 * @return {@link #repeats} (Whether the group may occur multiple times in the 912 * instance, containing multiple sets of answers.). This is the 913 * underlying object with id, value and extensions. The accessor 914 * "getRepeats" gives direct access to the value 915 */ 916 public BooleanType getRepeatsElement() { 917 if (this.repeats == null) 918 if (Configuration.errorOnAutoCreate()) 919 throw new Error("Attempt to auto-create GroupComponent.repeats"); 920 else if (Configuration.doAutoCreate()) 921 this.repeats = new BooleanType(); // bb 922 return this.repeats; 923 } 924 925 public boolean hasRepeatsElement() { 926 return this.repeats != null && !this.repeats.isEmpty(); 927 } 928 929 public boolean hasRepeats() { 930 return this.repeats != null && !this.repeats.isEmpty(); 931 } 932 933 /** 934 * @param value {@link #repeats} (Whether the group may occur multiple times in 935 * the instance, containing multiple sets of answers.). This is the 936 * underlying object with id, value and extensions. The accessor 937 * "getRepeats" gives direct access to the value 938 */ 939 public GroupComponent setRepeatsElement(BooleanType value) { 940 this.repeats = value; 941 return this; 942 } 943 944 /** 945 * @return Whether the group may occur multiple times in the instance, 946 * containing multiple sets of answers. 947 */ 948 public boolean getRepeats() { 949 return this.repeats == null || this.repeats.isEmpty() ? false : this.repeats.getValue(); 950 } 951 952 /** 953 * @param value Whether the group may occur multiple times in the instance, 954 * containing multiple sets of answers. 955 */ 956 public GroupComponent setRepeats(boolean value) { 957 if (this.repeats == null) 958 this.repeats = new BooleanType(); 959 this.repeats.setValue(value); 960 return this; 961 } 962 963 /** 964 * @return {@link #group} (A sub-group within a group. The ordering of groups 965 * within this group is relevant.) 966 */ 967 public List<GroupComponent> getGroup() { 968 if (this.group == null) 969 this.group = new ArrayList<GroupComponent>(); 970 return this.group; 971 } 972 973 public boolean hasGroup() { 974 if (this.group == null) 975 return false; 976 for (GroupComponent item : this.group) 977 if (!item.isEmpty()) 978 return true; 979 return false; 980 } 981 982 /** 983 * @return {@link #group} (A sub-group within a group. The ordering of groups 984 * within this group is relevant.) 985 */ 986 // syntactic sugar 987 public GroupComponent addGroup() { // 3 988 GroupComponent t = new GroupComponent(); 989 if (this.group == null) 990 this.group = new ArrayList<GroupComponent>(); 991 this.group.add(t); 992 return t; 993 } 994 995 // syntactic sugar 996 public GroupComponent addGroup(GroupComponent t) { // 3 997 if (t == null) 998 return this; 999 if (this.group == null) 1000 this.group = new ArrayList<GroupComponent>(); 1001 this.group.add(t); 1002 return this; 1003 } 1004 1005 /** 1006 * @return {@link #question} (Set of questions within this group. The order of 1007 * questions within the group is relevant.) 1008 */ 1009 public List<QuestionComponent> getQuestion() { 1010 if (this.question == null) 1011 this.question = new ArrayList<QuestionComponent>(); 1012 return this.question; 1013 } 1014 1015 public boolean hasQuestion() { 1016 if (this.question == null) 1017 return false; 1018 for (QuestionComponent item : this.question) 1019 if (!item.isEmpty()) 1020 return true; 1021 return false; 1022 } 1023 1024 /** 1025 * @return {@link #question} (Set of questions within this group. The order of 1026 * questions within the group is relevant.) 1027 */ 1028 // syntactic sugar 1029 public QuestionComponent addQuestion() { // 3 1030 QuestionComponent t = new QuestionComponent(); 1031 if (this.question == null) 1032 this.question = new ArrayList<QuestionComponent>(); 1033 this.question.add(t); 1034 return t; 1035 } 1036 1037 // syntactic sugar 1038 public GroupComponent addQuestion(QuestionComponent t) { // 3 1039 if (t == null) 1040 return this; 1041 if (this.question == null) 1042 this.question = new ArrayList<QuestionComponent>(); 1043 this.question.add(t); 1044 return this; 1045 } 1046 1047 protected void listChildren(List<Property> childrenList) { 1048 super.listChildren(childrenList); 1049 childrenList.add(new Property("linkId", "string", 1050 "An identifier that is unique within the Questionnaire allowing linkage to the equivalent group in a QuestionnaireResponse resource.", 1051 0, java.lang.Integer.MAX_VALUE, linkId)); 1052 childrenList.add(new Property("title", "string", "The human-readable name for this section of the questionnaire.", 1053 0, java.lang.Integer.MAX_VALUE, title)); 1054 childrenList.add(new Property("concept", "Coding", 1055 "Identifies a how this group of questions is known in a particular terminology such as LOINC.", 0, 1056 java.lang.Integer.MAX_VALUE, concept)); 1057 childrenList.add(new Property("text", "string", "Additional text for the group, used for display purposes.", 0, 1058 java.lang.Integer.MAX_VALUE, text)); 1059 childrenList.add(new Property("required", "boolean", 1060 "If true, indicates that the group must be present and have required questions within it answered. If false, the group may be skipped when answering the questionnaire.", 1061 0, java.lang.Integer.MAX_VALUE, required)); 1062 childrenList.add(new Property("repeats", "boolean", 1063 "Whether the group may occur multiple times in the instance, containing multiple sets of answers.", 0, 1064 java.lang.Integer.MAX_VALUE, repeats)); 1065 childrenList.add(new Property("group", "@Questionnaire.group", 1066 "A sub-group within a group. The ordering of groups within this group is relevant.", 0, 1067 java.lang.Integer.MAX_VALUE, group)); 1068 childrenList.add(new Property("question", "", 1069 "Set of questions within this group. The order of questions within the group is relevant.", 0, 1070 java.lang.Integer.MAX_VALUE, question)); 1071 } 1072 1073 @Override 1074 public void setProperty(String name, Base value) throws FHIRException { 1075 if (name.equals("linkId")) 1076 this.linkId = castToString(value); // StringType 1077 else if (name.equals("title")) 1078 this.title = castToString(value); // StringType 1079 else if (name.equals("concept")) 1080 this.getConcept().add(castToCoding(value)); 1081 else if (name.equals("text")) 1082 this.text = castToString(value); // StringType 1083 else if (name.equals("required")) 1084 this.required = castToBoolean(value); // BooleanType 1085 else if (name.equals("repeats")) 1086 this.repeats = castToBoolean(value); // BooleanType 1087 else if (name.equals("group")) 1088 this.getGroup().add((GroupComponent) value); 1089 else if (name.equals("question")) 1090 this.getQuestion().add((QuestionComponent) value); 1091 else 1092 super.setProperty(name, value); 1093 } 1094 1095 @Override 1096 public Base addChild(String name) throws FHIRException { 1097 if (name.equals("linkId")) { 1098 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.linkId"); 1099 } else if (name.equals("title")) { 1100 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.title"); 1101 } else if (name.equals("concept")) { 1102 return addConcept(); 1103 } else if (name.equals("text")) { 1104 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.text"); 1105 } else if (name.equals("required")) { 1106 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.required"); 1107 } else if (name.equals("repeats")) { 1108 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.repeats"); 1109 } else if (name.equals("group")) { 1110 return addGroup(); 1111 } else if (name.equals("question")) { 1112 return addQuestion(); 1113 } else 1114 return super.addChild(name); 1115 } 1116 1117 public GroupComponent copy() { 1118 GroupComponent dst = new GroupComponent(); 1119 copyValues(dst); 1120 dst.linkId = linkId == null ? null : linkId.copy(); 1121 dst.title = title == null ? null : title.copy(); 1122 if (concept != null) { 1123 dst.concept = new ArrayList<Coding>(); 1124 for (Coding i : concept) 1125 dst.concept.add(i.copy()); 1126 } 1127 ; 1128 dst.text = text == null ? null : text.copy(); 1129 dst.required = required == null ? null : required.copy(); 1130 dst.repeats = repeats == null ? null : repeats.copy(); 1131 if (group != null) { 1132 dst.group = new ArrayList<GroupComponent>(); 1133 for (GroupComponent i : group) 1134 dst.group.add(i.copy()); 1135 } 1136 ; 1137 if (question != null) { 1138 dst.question = new ArrayList<QuestionComponent>(); 1139 for (QuestionComponent i : question) 1140 dst.question.add(i.copy()); 1141 } 1142 ; 1143 return dst; 1144 } 1145 1146 @Override 1147 public boolean equalsDeep(Base other) { 1148 if (!super.equalsDeep(other)) 1149 return false; 1150 if (!(other instanceof GroupComponent)) 1151 return false; 1152 GroupComponent o = (GroupComponent) other; 1153 return compareDeep(linkId, o.linkId, true) && compareDeep(title, o.title, true) 1154 && compareDeep(concept, o.concept, true) && compareDeep(text, o.text, true) 1155 && compareDeep(required, o.required, true) && compareDeep(repeats, o.repeats, true) 1156 && compareDeep(group, o.group, true) && compareDeep(question, o.question, true); 1157 } 1158 1159 @Override 1160 public boolean equalsShallow(Base other) { 1161 if (!super.equalsShallow(other)) 1162 return false; 1163 if (!(other instanceof GroupComponent)) 1164 return false; 1165 GroupComponent o = (GroupComponent) other; 1166 return compareValues(linkId, o.linkId, true) && compareValues(title, o.title, true) 1167 && compareValues(text, o.text, true) && compareValues(required, o.required, true) 1168 && compareValues(repeats, o.repeats, true); 1169 } 1170 1171 public boolean isEmpty() { 1172 return super.isEmpty() && (linkId == null || linkId.isEmpty()) && (title == null || title.isEmpty()) 1173 && (concept == null || concept.isEmpty()) && (text == null || text.isEmpty()) 1174 && (required == null || required.isEmpty()) && (repeats == null || repeats.isEmpty()) 1175 && (group == null || group.isEmpty()) && (question == null || question.isEmpty()); 1176 } 1177 1178 public String fhirType() { 1179 return "Questionnaire.group"; 1180 1181 } 1182 1183 } 1184 1185 @Block() 1186 public static class QuestionComponent extends BackboneElement implements IBaseBackboneElement { 1187 /** 1188 * An identifier that is unique within the questionnaire allowing linkage to the 1189 * equivalent group in a [[[QuestionnaireResponse]]] resource. 1190 */ 1191 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1192 @Description(shortDefinition = "To link questionnaire with questionnaire response", formalDefinition = "An identifier that is unique within the questionnaire allowing linkage to the equivalent group in a [[[QuestionnaireResponse]]] resource.") 1193 protected StringType linkId; 1194 1195 /** 1196 * Identifies a how this question is known in a particular terminology such as 1197 * LOINC. 1198 */ 1199 @Child(name = "concept", type = { 1200 Coding.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1201 @Description(shortDefinition = "Concept that represents this question on a questionnaire", formalDefinition = "Identifies a how this question is known in a particular terminology such as LOINC.") 1202 protected List<Coding> concept; 1203 1204 /** 1205 * The actual question as shown to the user to prompt them for an answer. 1206 */ 1207 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1208 @Description(shortDefinition = "Text of the question as it is shown to the user", formalDefinition = "The actual question as shown to the user to prompt them for an answer.") 1209 protected StringType text; 1210 1211 /** 1212 * The expected format of the answer, e.g. the type of input (string, integer) 1213 * or whether a (multiple) choice is expected. 1214 */ 1215 @Child(name = "type", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1216 @Description(shortDefinition = "boolean | decimal | integer | date | dateTime +", formalDefinition = "The expected format of the answer, e.g. the type of input (string, integer) or whether a (multiple) choice is expected.") 1217 protected Enumeration<AnswerFormat> type; 1218 1219 /** 1220 * If true, indicates that the question must be answered and have required 1221 * groups within it also present. If false, the question and any contained 1222 * groups may be skipped when answering the questionnaire. 1223 */ 1224 @Child(name = "required", type = { 1225 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1226 @Description(shortDefinition = "Whether the question must be answered in data results", formalDefinition = "If true, indicates that the question must be answered and have required groups within it also present. If false, the question and any contained groups may be skipped when answering the questionnaire.") 1227 protected BooleanType required; 1228 1229 /** 1230 * If true, the question may have more than one answer. 1231 */ 1232 @Child(name = "repeats", type = { 1233 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1234 @Description(shortDefinition = "Whether the question can have multiple answers", formalDefinition = "If true, the question may have more than one answer.") 1235 protected BooleanType repeats; 1236 1237 /** 1238 * Reference to a value set containing a list of codes representing permitted 1239 * answers for the question. 1240 */ 1241 @Child(name = "options", type = { ValueSet.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1242 @Description(shortDefinition = "Valueset containing permitted answers", formalDefinition = "Reference to a value set containing a list of codes representing permitted answers for the question.") 1243 protected Reference options; 1244 1245 /** 1246 * The actual object that is the target of the reference (Reference to a value 1247 * set containing a list of codes representing permitted answers for the 1248 * question.) 1249 */ 1250 protected ValueSet optionsTarget; 1251 1252 /** 1253 * For a "choice" question, identifies one of the permitted answers for the 1254 * question. 1255 */ 1256 @Child(name = "option", type = { 1257 Coding.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1258 @Description(shortDefinition = "Permitted answer", formalDefinition = "For a \"choice\" question, identifies one of the permitted answers for the question.") 1259 protected List<Coding> option; 1260 1261 /** 1262 * Nested group, containing nested question for this question. The order of 1263 * groups within the question is relevant. 1264 */ 1265 @Child(name = "group", type = { 1266 GroupComponent.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1267 @Description(shortDefinition = "Nested questionnaire group", formalDefinition = "Nested group, containing nested question for this question. The order of groups within the question is relevant.") 1268 protected List<GroupComponent> group; 1269 1270 private static final long serialVersionUID = -1078951042L; 1271 1272 /* 1273 * Constructor 1274 */ 1275 public QuestionComponent() { 1276 super(); 1277 } 1278 1279 /** 1280 * @return {@link #linkId} (An identifier that is unique within the 1281 * questionnaire allowing linkage to the equivalent group in a 1282 * [[[QuestionnaireResponse]]] resource.). This is the underlying object 1283 * with id, value and extensions. The accessor "getLinkId" gives direct 1284 * access to the value 1285 */ 1286 public StringType getLinkIdElement() { 1287 if (this.linkId == null) 1288 if (Configuration.errorOnAutoCreate()) 1289 throw new Error("Attempt to auto-create QuestionComponent.linkId"); 1290 else if (Configuration.doAutoCreate()) 1291 this.linkId = new StringType(); // bb 1292 return this.linkId; 1293 } 1294 1295 public boolean hasLinkIdElement() { 1296 return this.linkId != null && !this.linkId.isEmpty(); 1297 } 1298 1299 public boolean hasLinkId() { 1300 return this.linkId != null && !this.linkId.isEmpty(); 1301 } 1302 1303 /** 1304 * @param value {@link #linkId} (An identifier that is unique within the 1305 * questionnaire allowing linkage to the equivalent group in a 1306 * [[[QuestionnaireResponse]]] resource.). This is the underlying 1307 * object with id, value and extensions. The accessor "getLinkId" 1308 * gives direct access to the value 1309 */ 1310 public QuestionComponent setLinkIdElement(StringType value) { 1311 this.linkId = value; 1312 return this; 1313 } 1314 1315 /** 1316 * @return An identifier that is unique within the questionnaire allowing 1317 * linkage to the equivalent group in a [[[QuestionnaireResponse]]] 1318 * resource. 1319 */ 1320 public String getLinkId() { 1321 return this.linkId == null ? null : this.linkId.getValue(); 1322 } 1323 1324 /** 1325 * @param value An identifier that is unique within the questionnaire allowing 1326 * linkage to the equivalent group in a [[[QuestionnaireResponse]]] 1327 * resource. 1328 */ 1329 public QuestionComponent setLinkId(String value) { 1330 if (Utilities.noString(value)) 1331 this.linkId = null; 1332 else { 1333 if (this.linkId == null) 1334 this.linkId = new StringType(); 1335 this.linkId.setValue(value); 1336 } 1337 return this; 1338 } 1339 1340 /** 1341 * @return {@link #concept} (Identifies a how this question is known in a 1342 * particular terminology such as LOINC.) 1343 */ 1344 public List<Coding> getConcept() { 1345 if (this.concept == null) 1346 this.concept = new ArrayList<Coding>(); 1347 return this.concept; 1348 } 1349 1350 public boolean hasConcept() { 1351 if (this.concept == null) 1352 return false; 1353 for (Coding item : this.concept) 1354 if (!item.isEmpty()) 1355 return true; 1356 return false; 1357 } 1358 1359 /** 1360 * @return {@link #concept} (Identifies a how this question is known in a 1361 * particular terminology such as LOINC.) 1362 */ 1363 // syntactic sugar 1364 public Coding addConcept() { // 3 1365 Coding t = new Coding(); 1366 if (this.concept == null) 1367 this.concept = new ArrayList<Coding>(); 1368 this.concept.add(t); 1369 return t; 1370 } 1371 1372 // syntactic sugar 1373 public QuestionComponent addConcept(Coding t) { // 3 1374 if (t == null) 1375 return this; 1376 if (this.concept == null) 1377 this.concept = new ArrayList<Coding>(); 1378 this.concept.add(t); 1379 return this; 1380 } 1381 1382 /** 1383 * @return {@link #text} (The actual question as shown to the user to prompt 1384 * them for an answer.). This is the underlying object with id, value 1385 * and extensions. The accessor "getText" gives direct access to the 1386 * value 1387 */ 1388 public StringType getTextElement() { 1389 if (this.text == null) 1390 if (Configuration.errorOnAutoCreate()) 1391 throw new Error("Attempt to auto-create QuestionComponent.text"); 1392 else if (Configuration.doAutoCreate()) 1393 this.text = new StringType(); // bb 1394 return this.text; 1395 } 1396 1397 public boolean hasTextElement() { 1398 return this.text != null && !this.text.isEmpty(); 1399 } 1400 1401 public boolean hasText() { 1402 return this.text != null && !this.text.isEmpty(); 1403 } 1404 1405 /** 1406 * @param value {@link #text} (The actual question as shown to the user to 1407 * prompt them for an answer.). This is the underlying object with 1408 * id, value and extensions. The accessor "getText" gives direct 1409 * access to the value 1410 */ 1411 public QuestionComponent setTextElement(StringType value) { 1412 this.text = value; 1413 return this; 1414 } 1415 1416 /** 1417 * @return The actual question as shown to the user to prompt them for an 1418 * answer. 1419 */ 1420 public String getText() { 1421 return this.text == null ? null : this.text.getValue(); 1422 } 1423 1424 /** 1425 * @param value The actual question as shown to the user to prompt them for an 1426 * answer. 1427 */ 1428 public QuestionComponent setText(String value) { 1429 if (Utilities.noString(value)) 1430 this.text = null; 1431 else { 1432 if (this.text == null) 1433 this.text = new StringType(); 1434 this.text.setValue(value); 1435 } 1436 return this; 1437 } 1438 1439 /** 1440 * @return {@link #type} (The expected format of the answer, e.g. the type of 1441 * input (string, integer) or whether a (multiple) choice is expected.). 1442 * This is the underlying object with id, value and extensions. The 1443 * accessor "getType" gives direct access to the value 1444 */ 1445 public Enumeration<AnswerFormat> getTypeElement() { 1446 if (this.type == null) 1447 if (Configuration.errorOnAutoCreate()) 1448 throw new Error("Attempt to auto-create QuestionComponent.type"); 1449 else if (Configuration.doAutoCreate()) 1450 this.type = new Enumeration<AnswerFormat>(new AnswerFormatEnumFactory()); // bb 1451 return this.type; 1452 } 1453 1454 public boolean hasTypeElement() { 1455 return this.type != null && !this.type.isEmpty(); 1456 } 1457 1458 public boolean hasType() { 1459 return this.type != null && !this.type.isEmpty(); 1460 } 1461 1462 /** 1463 * @param value {@link #type} (The expected format of the answer, e.g. the type 1464 * of input (string, integer) or whether a (multiple) choice is 1465 * expected.). This is the underlying object with id, value and 1466 * extensions. The accessor "getType" gives direct access to the 1467 * value 1468 */ 1469 public QuestionComponent setTypeElement(Enumeration<AnswerFormat> value) { 1470 this.type = value; 1471 return this; 1472 } 1473 1474 /** 1475 * @return The expected format of the answer, e.g. the type of input (string, 1476 * integer) or whether a (multiple) choice is expected. 1477 */ 1478 public AnswerFormat getType() { 1479 return this.type == null ? null : this.type.getValue(); 1480 } 1481 1482 /** 1483 * @param value The expected format of the answer, e.g. the type of input 1484 * (string, integer) or whether a (multiple) choice is expected. 1485 */ 1486 public QuestionComponent setType(AnswerFormat value) { 1487 if (value == null) 1488 this.type = null; 1489 else { 1490 if (this.type == null) 1491 this.type = new Enumeration<AnswerFormat>(new AnswerFormatEnumFactory()); 1492 this.type.setValue(value); 1493 } 1494 return this; 1495 } 1496 1497 /** 1498 * @return {@link #required} (If true, indicates that the question must be 1499 * answered and have required groups within it also present. If false, 1500 * the question and any contained groups may be skipped when answering 1501 * the questionnaire.). This is the underlying object with id, value and 1502 * extensions. The accessor "getRequired" gives direct access to the 1503 * value 1504 */ 1505 public BooleanType getRequiredElement() { 1506 if (this.required == null) 1507 if (Configuration.errorOnAutoCreate()) 1508 throw new Error("Attempt to auto-create QuestionComponent.required"); 1509 else if (Configuration.doAutoCreate()) 1510 this.required = new BooleanType(); // bb 1511 return this.required; 1512 } 1513 1514 public boolean hasRequiredElement() { 1515 return this.required != null && !this.required.isEmpty(); 1516 } 1517 1518 public boolean hasRequired() { 1519 return this.required != null && !this.required.isEmpty(); 1520 } 1521 1522 /** 1523 * @param value {@link #required} (If true, indicates that the question must be 1524 * answered and have required groups within it also present. If 1525 * false, the question and any contained groups may be skipped when 1526 * answering the questionnaire.). This is the underlying object 1527 * with id, value and extensions. The accessor "getRequired" gives 1528 * direct access to the value 1529 */ 1530 public QuestionComponent setRequiredElement(BooleanType value) { 1531 this.required = value; 1532 return this; 1533 } 1534 1535 /** 1536 * @return If true, indicates that the question must be answered and have 1537 * required groups within it also present. If false, the question and 1538 * any contained groups may be skipped when answering the questionnaire. 1539 */ 1540 public boolean getRequired() { 1541 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 1542 } 1543 1544 /** 1545 * @param value If true, indicates that the question must be answered and have 1546 * required groups within it also present. If false, the question 1547 * and any contained groups may be skipped when answering the 1548 * questionnaire. 1549 */ 1550 public QuestionComponent setRequired(boolean value) { 1551 if (this.required == null) 1552 this.required = new BooleanType(); 1553 this.required.setValue(value); 1554 return this; 1555 } 1556 1557 /** 1558 * @return {@link #repeats} (If true, the question may have more than one 1559 * answer.). This is the underlying object with id, value and 1560 * extensions. The accessor "getRepeats" gives direct access to the 1561 * value 1562 */ 1563 public BooleanType getRepeatsElement() { 1564 if (this.repeats == null) 1565 if (Configuration.errorOnAutoCreate()) 1566 throw new Error("Attempt to auto-create QuestionComponent.repeats"); 1567 else if (Configuration.doAutoCreate()) 1568 this.repeats = new BooleanType(); // bb 1569 return this.repeats; 1570 } 1571 1572 public boolean hasRepeatsElement() { 1573 return this.repeats != null && !this.repeats.isEmpty(); 1574 } 1575 1576 public boolean hasRepeats() { 1577 return this.repeats != null && !this.repeats.isEmpty(); 1578 } 1579 1580 /** 1581 * @param value {@link #repeats} (If true, the question may have more than one 1582 * answer.). This is the underlying object with id, value and 1583 * extensions. The accessor "getRepeats" gives direct access to the 1584 * value 1585 */ 1586 public QuestionComponent setRepeatsElement(BooleanType value) { 1587 this.repeats = value; 1588 return this; 1589 } 1590 1591 /** 1592 * @return If true, the question may have more than one answer. 1593 */ 1594 public boolean getRepeats() { 1595 return this.repeats == null || this.repeats.isEmpty() ? false : this.repeats.getValue(); 1596 } 1597 1598 /** 1599 * @param value If true, the question may have more than one answer. 1600 */ 1601 public QuestionComponent setRepeats(boolean value) { 1602 if (this.repeats == null) 1603 this.repeats = new BooleanType(); 1604 this.repeats.setValue(value); 1605 return this; 1606 } 1607 1608 /** 1609 * @return {@link #options} (Reference to a value set containing a list of codes 1610 * representing permitted answers for the question.) 1611 */ 1612 public Reference getOptions() { 1613 if (this.options == null) 1614 if (Configuration.errorOnAutoCreate()) 1615 throw new Error("Attempt to auto-create QuestionComponent.options"); 1616 else if (Configuration.doAutoCreate()) 1617 this.options = new Reference(); // cc 1618 return this.options; 1619 } 1620 1621 public boolean hasOptions() { 1622 return this.options != null && !this.options.isEmpty(); 1623 } 1624 1625 /** 1626 * @param value {@link #options} (Reference to a value set containing a list of 1627 * codes representing permitted answers for the question.) 1628 */ 1629 public QuestionComponent setOptions(Reference value) { 1630 this.options = value; 1631 return this; 1632 } 1633 1634 /** 1635 * @return {@link #options} The actual object that is the target of the 1636 * reference. The reference library doesn't populate this, but you can 1637 * use it to hold the resource if you resolve it. (Reference to a value 1638 * set containing a list of codes representing permitted answers for the 1639 * question.) 1640 */ 1641 public ValueSet getOptionsTarget() { 1642 if (this.optionsTarget == null) 1643 if (Configuration.errorOnAutoCreate()) 1644 throw new Error("Attempt to auto-create QuestionComponent.options"); 1645 else if (Configuration.doAutoCreate()) 1646 this.optionsTarget = new ValueSet(); // aa 1647 return this.optionsTarget; 1648 } 1649 1650 /** 1651 * @param value {@link #options} The actual object that is the target of the 1652 * reference. The reference library doesn't use these, but you can 1653 * use it to hold the resource if you resolve it. (Reference to a 1654 * value set containing a list of codes representing permitted 1655 * answers for the question.) 1656 */ 1657 public QuestionComponent setOptionsTarget(ValueSet value) { 1658 this.optionsTarget = value; 1659 return this; 1660 } 1661 1662 /** 1663 * @return {@link #option} (For a "choice" question, identifies one of the 1664 * permitted answers for the question.) 1665 */ 1666 public List<Coding> getOption() { 1667 if (this.option == null) 1668 this.option = new ArrayList<Coding>(); 1669 return this.option; 1670 } 1671 1672 public boolean hasOption() { 1673 if (this.option == null) 1674 return false; 1675 for (Coding item : this.option) 1676 if (!item.isEmpty()) 1677 return true; 1678 return false; 1679 } 1680 1681 /** 1682 * @return {@link #option} (For a "choice" question, identifies one of the 1683 * permitted answers for the question.) 1684 */ 1685 // syntactic sugar 1686 public Coding addOption() { // 3 1687 Coding t = new Coding(); 1688 if (this.option == null) 1689 this.option = new ArrayList<Coding>(); 1690 this.option.add(t); 1691 return t; 1692 } 1693 1694 // syntactic sugar 1695 public QuestionComponent addOption(Coding t) { // 3 1696 if (t == null) 1697 return this; 1698 if (this.option == null) 1699 this.option = new ArrayList<Coding>(); 1700 this.option.add(t); 1701 return this; 1702 } 1703 1704 /** 1705 * @return {@link #group} (Nested group, containing nested question for this 1706 * question. The order of groups within the question is relevant.) 1707 */ 1708 public List<GroupComponent> getGroup() { 1709 if (this.group == null) 1710 this.group = new ArrayList<GroupComponent>(); 1711 return this.group; 1712 } 1713 1714 public boolean hasGroup() { 1715 if (this.group == null) 1716 return false; 1717 for (GroupComponent item : this.group) 1718 if (!item.isEmpty()) 1719 return true; 1720 return false; 1721 } 1722 1723 /** 1724 * @return {@link #group} (Nested group, containing nested question for this 1725 * question. The order of groups within the question is relevant.) 1726 */ 1727 // syntactic sugar 1728 public GroupComponent addGroup() { // 3 1729 GroupComponent t = new GroupComponent(); 1730 if (this.group == null) 1731 this.group = new ArrayList<GroupComponent>(); 1732 this.group.add(t); 1733 return t; 1734 } 1735 1736 // syntactic sugar 1737 public QuestionComponent addGroup(GroupComponent t) { // 3 1738 if (t == null) 1739 return this; 1740 if (this.group == null) 1741 this.group = new ArrayList<GroupComponent>(); 1742 this.group.add(t); 1743 return this; 1744 } 1745 1746 protected void listChildren(List<Property> childrenList) { 1747 super.listChildren(childrenList); 1748 childrenList.add(new Property("linkId", "string", 1749 "An identifier that is unique within the questionnaire allowing linkage to the equivalent group in a [[[QuestionnaireResponse]]] resource.", 1750 0, java.lang.Integer.MAX_VALUE, linkId)); 1751 childrenList.add(new Property("concept", "Coding", 1752 "Identifies a how this question is known in a particular terminology such as LOINC.", 0, 1753 java.lang.Integer.MAX_VALUE, concept)); 1754 childrenList 1755 .add(new Property("text", "string", "The actual question as shown to the user to prompt them for an answer.", 1756 0, java.lang.Integer.MAX_VALUE, text)); 1757 childrenList.add(new Property("type", "code", 1758 "The expected format of the answer, e.g. the type of input (string, integer) or whether a (multiple) choice is expected.", 1759 0, java.lang.Integer.MAX_VALUE, type)); 1760 childrenList.add(new Property("required", "boolean", 1761 "If true, indicates that the question must be answered and have required groups within it also present. If false, the question and any contained groups may be skipped when answering the questionnaire.", 1762 0, java.lang.Integer.MAX_VALUE, required)); 1763 childrenList.add(new Property("repeats", "boolean", "If true, the question may have more than one answer.", 0, 1764 java.lang.Integer.MAX_VALUE, repeats)); 1765 childrenList.add(new Property("options", "Reference(ValueSet)", 1766 "Reference to a value set containing a list of codes representing permitted answers for the question.", 0, 1767 java.lang.Integer.MAX_VALUE, options)); 1768 childrenList.add(new Property("option", "Coding", 1769 "For a \"choice\" question, identifies one of the permitted answers for the question.", 0, 1770 java.lang.Integer.MAX_VALUE, option)); 1771 childrenList.add(new Property("group", "@Questionnaire.group", 1772 "Nested group, containing nested question for this question. The order of groups within the question is relevant.", 1773 0, java.lang.Integer.MAX_VALUE, group)); 1774 } 1775 1776 @Override 1777 public void setProperty(String name, Base value) throws FHIRException { 1778 if (name.equals("linkId")) 1779 this.linkId = castToString(value); // StringType 1780 else if (name.equals("concept")) 1781 this.getConcept().add(castToCoding(value)); 1782 else if (name.equals("text")) 1783 this.text = castToString(value); // StringType 1784 else if (name.equals("type")) 1785 this.type = new AnswerFormatEnumFactory().fromType(value); // Enumeration<AnswerFormat> 1786 else if (name.equals("required")) 1787 this.required = castToBoolean(value); // BooleanType 1788 else if (name.equals("repeats")) 1789 this.repeats = castToBoolean(value); // BooleanType 1790 else if (name.equals("options")) 1791 this.options = castToReference(value); // Reference 1792 else if (name.equals("option")) 1793 this.getOption().add(castToCoding(value)); 1794 else if (name.equals("group")) 1795 this.getGroup().add((GroupComponent) value); 1796 else 1797 super.setProperty(name, value); 1798 } 1799 1800 @Override 1801 public Base addChild(String name) throws FHIRException { 1802 if (name.equals("linkId")) { 1803 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.linkId"); 1804 } else if (name.equals("concept")) { 1805 return addConcept(); 1806 } else if (name.equals("text")) { 1807 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.text"); 1808 } else if (name.equals("type")) { 1809 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.type"); 1810 } else if (name.equals("required")) { 1811 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.required"); 1812 } else if (name.equals("repeats")) { 1813 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.repeats"); 1814 } else if (name.equals("options")) { 1815 this.options = new Reference(); 1816 return this.options; 1817 } else if (name.equals("option")) { 1818 return addOption(); 1819 } else if (name.equals("group")) { 1820 return addGroup(); 1821 } else 1822 return super.addChild(name); 1823 } 1824 1825 public QuestionComponent copy() { 1826 QuestionComponent dst = new QuestionComponent(); 1827 copyValues(dst); 1828 dst.linkId = linkId == null ? null : linkId.copy(); 1829 if (concept != null) { 1830 dst.concept = new ArrayList<Coding>(); 1831 for (Coding i : concept) 1832 dst.concept.add(i.copy()); 1833 } 1834 ; 1835 dst.text = text == null ? null : text.copy(); 1836 dst.type = type == null ? null : type.copy(); 1837 dst.required = required == null ? null : required.copy(); 1838 dst.repeats = repeats == null ? null : repeats.copy(); 1839 dst.options = options == null ? null : options.copy(); 1840 if (option != null) { 1841 dst.option = new ArrayList<Coding>(); 1842 for (Coding i : option) 1843 dst.option.add(i.copy()); 1844 } 1845 ; 1846 if (group != null) { 1847 dst.group = new ArrayList<GroupComponent>(); 1848 for (GroupComponent i : group) 1849 dst.group.add(i.copy()); 1850 } 1851 ; 1852 return dst; 1853 } 1854 1855 @Override 1856 public boolean equalsDeep(Base other) { 1857 if (!super.equalsDeep(other)) 1858 return false; 1859 if (!(other instanceof QuestionComponent)) 1860 return false; 1861 QuestionComponent o = (QuestionComponent) other; 1862 return compareDeep(linkId, o.linkId, true) && compareDeep(concept, o.concept, true) 1863 && compareDeep(text, o.text, true) && compareDeep(type, o.type, true) 1864 && compareDeep(required, o.required, true) && compareDeep(repeats, o.repeats, true) 1865 && compareDeep(options, o.options, true) && compareDeep(option, o.option, true) 1866 && compareDeep(group, o.group, true); 1867 } 1868 1869 @Override 1870 public boolean equalsShallow(Base other) { 1871 if (!super.equalsShallow(other)) 1872 return false; 1873 if (!(other instanceof QuestionComponent)) 1874 return false; 1875 QuestionComponent o = (QuestionComponent) other; 1876 return compareValues(linkId, o.linkId, true) && compareValues(text, o.text, true) 1877 && compareValues(type, o.type, true) && compareValues(required, o.required, true) 1878 && compareValues(repeats, o.repeats, true); 1879 } 1880 1881 public boolean isEmpty() { 1882 return super.isEmpty() && (linkId == null || linkId.isEmpty()) && (concept == null || concept.isEmpty()) 1883 && (text == null || text.isEmpty()) && (type == null || type.isEmpty()) 1884 && (required == null || required.isEmpty()) && (repeats == null || repeats.isEmpty()) 1885 && (options == null || options.isEmpty()) && (option == null || option.isEmpty()) 1886 && (group == null || group.isEmpty()); 1887 } 1888 1889 public String fhirType() { 1890 return "Questionnaire.group.question"; 1891 1892 } 1893 1894 } 1895 1896 /** 1897 * This records identifiers associated with this question set that are defined 1898 * by business processes and/or used to refer to it when a direct URL reference 1899 * to the resource itself is not appropriate (e.g. in CDA documents, or in 1900 * written / printed documentation). 1901 */ 1902 @Child(name = "identifier", type = { 1903 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1904 @Description(shortDefinition = "External identifiers for this questionnaire", formalDefinition = "This records identifiers associated with this question set that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 1905 protected List<Identifier> identifier; 1906 1907 /** 1908 * The version number assigned by the publisher for business reasons. It may 1909 * remain the same when the resource is updated. 1910 */ 1911 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1912 @Description(shortDefinition = "Logical identifier for this version of Questionnaire", formalDefinition = "The version number assigned by the publisher for business reasons. It may remain the same when the resource is updated.") 1913 protected StringType version; 1914 1915 /** 1916 * The lifecycle status of the questionnaire as a whole. 1917 */ 1918 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 1919 @Description(shortDefinition = "draft | published | retired", formalDefinition = "The lifecycle status of the questionnaire as a whole.") 1920 protected Enumeration<QuestionnaireStatus> status; 1921 1922 /** 1923 * The date that this questionnaire was last changed. 1924 */ 1925 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1926 @Description(shortDefinition = "Date this version was authored", formalDefinition = "The date that this questionnaire was last changed.") 1927 protected DateTimeType date; 1928 1929 /** 1930 * Organization or person responsible for developing and maintaining the 1931 * questionnaire. 1932 */ 1933 @Child(name = "publisher", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1934 @Description(shortDefinition = "Organization/individual who designed the questionnaire", formalDefinition = "Organization or person responsible for developing and maintaining the questionnaire.") 1935 protected StringType publisher; 1936 1937 /** 1938 * Contact details to assist a user in finding and communicating with the 1939 * publisher. 1940 */ 1941 @Child(name = "telecom", type = { 1942 ContactPoint.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1943 @Description(shortDefinition = "Contact information of the publisher", formalDefinition = "Contact details to assist a user in finding and communicating with the publisher.") 1944 protected List<ContactPoint> telecom; 1945 1946 /** 1947 * Identifies the types of subjects that can be the subject of the 1948 * questionnaire. 1949 */ 1950 @Child(name = "subjectType", type = { 1951 CodeType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1952 @Description(shortDefinition = "Resource that can be subject of QuestionnaireResponse", formalDefinition = "Identifies the types of subjects that can be the subject of the questionnaire.") 1953 protected List<CodeType> subjectType; 1954 1955 /** 1956 * A collection of related questions (or further groupings of questions). 1957 */ 1958 @Child(name = "group", type = {}, order = 7, min = 1, max = 1, modifier = false, summary = true) 1959 @Description(shortDefinition = "Grouped questions", formalDefinition = "A collection of related questions (or further groupings of questions).") 1960 protected GroupComponent group; 1961 1962 private static final long serialVersionUID = -1348292652L; 1963 1964 /* 1965 * Constructor 1966 */ 1967 public Questionnaire() { 1968 super(); 1969 } 1970 1971 /* 1972 * Constructor 1973 */ 1974 public Questionnaire(Enumeration<QuestionnaireStatus> status, GroupComponent group) { 1975 super(); 1976 this.status = status; 1977 this.group = group; 1978 } 1979 1980 /** 1981 * @return {@link #identifier} (This records identifiers associated with this 1982 * question set that are defined by business processes and/or used to 1983 * refer to it when a direct URL reference to the resource itself is not 1984 * appropriate (e.g. in CDA documents, or in written / printed 1985 * documentation).) 1986 */ 1987 public List<Identifier> getIdentifier() { 1988 if (this.identifier == null) 1989 this.identifier = new ArrayList<Identifier>(); 1990 return this.identifier; 1991 } 1992 1993 public boolean hasIdentifier() { 1994 if (this.identifier == null) 1995 return false; 1996 for (Identifier item : this.identifier) 1997 if (!item.isEmpty()) 1998 return true; 1999 return false; 2000 } 2001 2002 /** 2003 * @return {@link #identifier} (This records identifiers associated with this 2004 * question set that are defined by business processes and/or used to 2005 * refer to it when a direct URL reference to the resource itself is not 2006 * appropriate (e.g. in CDA documents, or in written / printed 2007 * documentation).) 2008 */ 2009 // syntactic sugar 2010 public Identifier addIdentifier() { // 3 2011 Identifier t = new Identifier(); 2012 if (this.identifier == null) 2013 this.identifier = new ArrayList<Identifier>(); 2014 this.identifier.add(t); 2015 return t; 2016 } 2017 2018 // syntactic sugar 2019 public Questionnaire addIdentifier(Identifier t) { // 3 2020 if (t == null) 2021 return this; 2022 if (this.identifier == null) 2023 this.identifier = new ArrayList<Identifier>(); 2024 this.identifier.add(t); 2025 return this; 2026 } 2027 2028 /** 2029 * @return {@link #version} (The version number assigned by the publisher for 2030 * business reasons. It may remain the same when the resource is 2031 * updated.). This is the underlying object with id, value and 2032 * extensions. The accessor "getVersion" gives direct access to the 2033 * value 2034 */ 2035 public StringType getVersionElement() { 2036 if (this.version == null) 2037 if (Configuration.errorOnAutoCreate()) 2038 throw new Error("Attempt to auto-create Questionnaire.version"); 2039 else if (Configuration.doAutoCreate()) 2040 this.version = new StringType(); // bb 2041 return this.version; 2042 } 2043 2044 public boolean hasVersionElement() { 2045 return this.version != null && !this.version.isEmpty(); 2046 } 2047 2048 public boolean hasVersion() { 2049 return this.version != null && !this.version.isEmpty(); 2050 } 2051 2052 /** 2053 * @param value {@link #version} (The version number assigned by the publisher 2054 * for business reasons. It may remain the same when the resource 2055 * is updated.). This is the underlying object with id, value and 2056 * extensions. The accessor "getVersion" gives direct access to the 2057 * value 2058 */ 2059 public Questionnaire setVersionElement(StringType value) { 2060 this.version = value; 2061 return this; 2062 } 2063 2064 /** 2065 * @return The version number assigned by the publisher for business reasons. It 2066 * may remain the same when the resource is updated. 2067 */ 2068 public String getVersion() { 2069 return this.version == null ? null : this.version.getValue(); 2070 } 2071 2072 /** 2073 * @param value The version number assigned by the publisher for business 2074 * reasons. It may remain the same when the resource is updated. 2075 */ 2076 public Questionnaire setVersion(String value) { 2077 if (Utilities.noString(value)) 2078 this.version = null; 2079 else { 2080 if (this.version == null) 2081 this.version = new StringType(); 2082 this.version.setValue(value); 2083 } 2084 return this; 2085 } 2086 2087 /** 2088 * @return {@link #status} (The lifecycle status of the questionnaire as a 2089 * whole.). This is the underlying object with id, value and extensions. 2090 * The accessor "getStatus" gives direct access to the value 2091 */ 2092 public Enumeration<QuestionnaireStatus> getStatusElement() { 2093 if (this.status == null) 2094 if (Configuration.errorOnAutoCreate()) 2095 throw new Error("Attempt to auto-create Questionnaire.status"); 2096 else if (Configuration.doAutoCreate()) 2097 this.status = new Enumeration<QuestionnaireStatus>(new QuestionnaireStatusEnumFactory()); // bb 2098 return this.status; 2099 } 2100 2101 public boolean hasStatusElement() { 2102 return this.status != null && !this.status.isEmpty(); 2103 } 2104 2105 public boolean hasStatus() { 2106 return this.status != null && !this.status.isEmpty(); 2107 } 2108 2109 /** 2110 * @param value {@link #status} (The lifecycle status of the questionnaire as a 2111 * whole.). This is the underlying object with id, value and 2112 * extensions. The accessor "getStatus" gives direct access to the 2113 * value 2114 */ 2115 public Questionnaire setStatusElement(Enumeration<QuestionnaireStatus> value) { 2116 this.status = value; 2117 return this; 2118 } 2119 2120 /** 2121 * @return The lifecycle status of the questionnaire as a whole. 2122 */ 2123 public QuestionnaireStatus getStatus() { 2124 return this.status == null ? null : this.status.getValue(); 2125 } 2126 2127 /** 2128 * @param value The lifecycle status of the questionnaire as a whole. 2129 */ 2130 public Questionnaire setStatus(QuestionnaireStatus value) { 2131 if (this.status == null) 2132 this.status = new Enumeration<QuestionnaireStatus>(new QuestionnaireStatusEnumFactory()); 2133 this.status.setValue(value); 2134 return this; 2135 } 2136 2137 /** 2138 * @return {@link #date} (The date that this questionnaire was last changed.). 2139 * This is the underlying object with id, value and extensions. The 2140 * accessor "getDate" gives direct access to the value 2141 */ 2142 public DateTimeType getDateElement() { 2143 if (this.date == null) 2144 if (Configuration.errorOnAutoCreate()) 2145 throw new Error("Attempt to auto-create Questionnaire.date"); 2146 else if (Configuration.doAutoCreate()) 2147 this.date = new DateTimeType(); // bb 2148 return this.date; 2149 } 2150 2151 public boolean hasDateElement() { 2152 return this.date != null && !this.date.isEmpty(); 2153 } 2154 2155 public boolean hasDate() { 2156 return this.date != null && !this.date.isEmpty(); 2157 } 2158 2159 /** 2160 * @param value {@link #date} (The date that this questionnaire was last 2161 * changed.). This is the underlying object with id, value and 2162 * extensions. The accessor "getDate" gives direct access to the 2163 * value 2164 */ 2165 public Questionnaire setDateElement(DateTimeType value) { 2166 this.date = value; 2167 return this; 2168 } 2169 2170 /** 2171 * @return The date that this questionnaire was last changed. 2172 */ 2173 public Date getDate() { 2174 return this.date == null ? null : this.date.getValue(); 2175 } 2176 2177 /** 2178 * @param value The date that this questionnaire was last changed. 2179 */ 2180 public Questionnaire setDate(Date value) { 2181 if (value == null) 2182 this.date = null; 2183 else { 2184 if (this.date == null) 2185 this.date = new DateTimeType(); 2186 this.date.setValue(value); 2187 } 2188 return this; 2189 } 2190 2191 /** 2192 * @return {@link #publisher} (Organization or person responsible for developing 2193 * and maintaining the questionnaire.). This is the underlying object 2194 * with id, value and extensions. The accessor "getPublisher" gives 2195 * direct access to the value 2196 */ 2197 public StringType getPublisherElement() { 2198 if (this.publisher == null) 2199 if (Configuration.errorOnAutoCreate()) 2200 throw new Error("Attempt to auto-create Questionnaire.publisher"); 2201 else if (Configuration.doAutoCreate()) 2202 this.publisher = new StringType(); // bb 2203 return this.publisher; 2204 } 2205 2206 public boolean hasPublisherElement() { 2207 return this.publisher != null && !this.publisher.isEmpty(); 2208 } 2209 2210 public boolean hasPublisher() { 2211 return this.publisher != null && !this.publisher.isEmpty(); 2212 } 2213 2214 /** 2215 * @param value {@link #publisher} (Organization or person responsible for 2216 * developing and maintaining the questionnaire.). This is the 2217 * underlying object with id, value and extensions. The accessor 2218 * "getPublisher" gives direct access to the value 2219 */ 2220 public Questionnaire setPublisherElement(StringType value) { 2221 this.publisher = value; 2222 return this; 2223 } 2224 2225 /** 2226 * @return Organization or person responsible for developing and maintaining the 2227 * questionnaire. 2228 */ 2229 public String getPublisher() { 2230 return this.publisher == null ? null : this.publisher.getValue(); 2231 } 2232 2233 /** 2234 * @param value Organization or person responsible for developing and 2235 * maintaining the questionnaire. 2236 */ 2237 public Questionnaire setPublisher(String value) { 2238 if (Utilities.noString(value)) 2239 this.publisher = null; 2240 else { 2241 if (this.publisher == null) 2242 this.publisher = new StringType(); 2243 this.publisher.setValue(value); 2244 } 2245 return this; 2246 } 2247 2248 /** 2249 * @return {@link #telecom} (Contact details to assist a user in finding and 2250 * communicating with the publisher.) 2251 */ 2252 public List<ContactPoint> getTelecom() { 2253 if (this.telecom == null) 2254 this.telecom = new ArrayList<ContactPoint>(); 2255 return this.telecom; 2256 } 2257 2258 public boolean hasTelecom() { 2259 if (this.telecom == null) 2260 return false; 2261 for (ContactPoint item : this.telecom) 2262 if (!item.isEmpty()) 2263 return true; 2264 return false; 2265 } 2266 2267 /** 2268 * @return {@link #telecom} (Contact details to assist a user in finding and 2269 * communicating with the publisher.) 2270 */ 2271 // syntactic sugar 2272 public ContactPoint addTelecom() { // 3 2273 ContactPoint t = new ContactPoint(); 2274 if (this.telecom == null) 2275 this.telecom = new ArrayList<ContactPoint>(); 2276 this.telecom.add(t); 2277 return t; 2278 } 2279 2280 // syntactic sugar 2281 public Questionnaire addTelecom(ContactPoint t) { // 3 2282 if (t == null) 2283 return this; 2284 if (this.telecom == null) 2285 this.telecom = new ArrayList<ContactPoint>(); 2286 this.telecom.add(t); 2287 return this; 2288 } 2289 2290 /** 2291 * @return {@link #subjectType} (Identifies the types of subjects that can be 2292 * the subject of the questionnaire.) 2293 */ 2294 public List<CodeType> getSubjectType() { 2295 if (this.subjectType == null) 2296 this.subjectType = new ArrayList<CodeType>(); 2297 return this.subjectType; 2298 } 2299 2300 public boolean hasSubjectType() { 2301 if (this.subjectType == null) 2302 return false; 2303 for (CodeType item : this.subjectType) 2304 if (!item.isEmpty()) 2305 return true; 2306 return false; 2307 } 2308 2309 /** 2310 * @return {@link #subjectType} (Identifies the types of subjects that can be 2311 * the subject of the questionnaire.) 2312 */ 2313 // syntactic sugar 2314 public CodeType addSubjectTypeElement() {// 2 2315 CodeType t = new CodeType(); 2316 if (this.subjectType == null) 2317 this.subjectType = new ArrayList<CodeType>(); 2318 this.subjectType.add(t); 2319 return t; 2320 } 2321 2322 /** 2323 * @param value {@link #subjectType} (Identifies the types of subjects that can 2324 * be the subject of the questionnaire.) 2325 */ 2326 public Questionnaire addSubjectType(String value) { // 1 2327 CodeType t = new CodeType(); 2328 t.setValue(value); 2329 if (this.subjectType == null) 2330 this.subjectType = new ArrayList<CodeType>(); 2331 this.subjectType.add(t); 2332 return this; 2333 } 2334 2335 /** 2336 * @param value {@link #subjectType} (Identifies the types of subjects that can 2337 * be the subject of the questionnaire.) 2338 */ 2339 public boolean hasSubjectType(String value) { 2340 if (this.subjectType == null) 2341 return false; 2342 for (CodeType v : this.subjectType) 2343 if (v.equals(value)) // code 2344 return true; 2345 return false; 2346 } 2347 2348 /** 2349 * @return {@link #group} (A collection of related questions (or further 2350 * groupings of questions).) 2351 */ 2352 public GroupComponent getGroup() { 2353 if (this.group == null) 2354 if (Configuration.errorOnAutoCreate()) 2355 throw new Error("Attempt to auto-create Questionnaire.group"); 2356 else if (Configuration.doAutoCreate()) 2357 this.group = new GroupComponent(); // cc 2358 return this.group; 2359 } 2360 2361 public boolean hasGroup() { 2362 return this.group != null && !this.group.isEmpty(); 2363 } 2364 2365 /** 2366 * @param value {@link #group} (A collection of related questions (or further 2367 * groupings of questions).) 2368 */ 2369 public Questionnaire setGroup(GroupComponent value) { 2370 this.group = value; 2371 return this; 2372 } 2373 2374 protected void listChildren(List<Property> childrenList) { 2375 super.listChildren(childrenList); 2376 childrenList.add(new Property("identifier", "Identifier", 2377 "This records identifiers associated with this question set that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2378 0, java.lang.Integer.MAX_VALUE, identifier)); 2379 childrenList.add(new Property("version", "string", 2380 "The version number assigned by the publisher for business reasons. It may remain the same when the resource is updated.", 2381 0, java.lang.Integer.MAX_VALUE, version)); 2382 childrenList.add(new Property("status", "code", "The lifecycle status of the questionnaire as a whole.", 0, 2383 java.lang.Integer.MAX_VALUE, status)); 2384 childrenList.add(new Property("date", "dateTime", "The date that this questionnaire was last changed.", 0, 2385 java.lang.Integer.MAX_VALUE, date)); 2386 childrenList.add(new Property("publisher", "string", 2387 "Organization or person responsible for developing and maintaining the questionnaire.", 0, 2388 java.lang.Integer.MAX_VALUE, publisher)); 2389 childrenList.add(new Property("telecom", "ContactPoint", 2390 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2391 java.lang.Integer.MAX_VALUE, telecom)); 2392 childrenList.add(new Property("subjectType", "code", 2393 "Identifies the types of subjects that can be the subject of the questionnaire.", 0, 2394 java.lang.Integer.MAX_VALUE, subjectType)); 2395 childrenList.add(new Property("group", "", "A collection of related questions (or further groupings of questions).", 2396 0, java.lang.Integer.MAX_VALUE, group)); 2397 } 2398 2399 @Override 2400 public void setProperty(String name, Base value) throws FHIRException { 2401 if (name.equals("identifier")) 2402 this.getIdentifier().add(castToIdentifier(value)); 2403 else if (name.equals("version")) 2404 this.version = castToString(value); // StringType 2405 else if (name.equals("status")) 2406 this.status = new QuestionnaireStatusEnumFactory().fromType(value); // Enumeration<QuestionnaireStatus> 2407 else if (name.equals("date")) 2408 this.date = castToDateTime(value); // DateTimeType 2409 else if (name.equals("publisher")) 2410 this.publisher = castToString(value); // StringType 2411 else if (name.equals("telecom")) 2412 this.getTelecom().add(castToContactPoint(value)); 2413 else if (name.equals("subjectType")) 2414 this.getSubjectType().add(castToCode(value)); 2415 else if (name.equals("group")) 2416 this.group = (GroupComponent) value; // GroupComponent 2417 else 2418 super.setProperty(name, value); 2419 } 2420 2421 @Override 2422 public Base addChild(String name) throws FHIRException { 2423 if (name.equals("identifier")) { 2424 return addIdentifier(); 2425 } else if (name.equals("version")) { 2426 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.version"); 2427 } else if (name.equals("status")) { 2428 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.status"); 2429 } else if (name.equals("date")) { 2430 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.date"); 2431 } else if (name.equals("publisher")) { 2432 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.publisher"); 2433 } else if (name.equals("telecom")) { 2434 return addTelecom(); 2435 } else if (name.equals("subjectType")) { 2436 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.subjectType"); 2437 } else if (name.equals("group")) { 2438 this.group = new GroupComponent(); 2439 return this.group; 2440 } else 2441 return super.addChild(name); 2442 } 2443 2444 public String fhirType() { 2445 return "Questionnaire"; 2446 2447 } 2448 2449 public Questionnaire copy() { 2450 Questionnaire dst = new Questionnaire(); 2451 copyValues(dst); 2452 if (identifier != null) { 2453 dst.identifier = new ArrayList<Identifier>(); 2454 for (Identifier i : identifier) 2455 dst.identifier.add(i.copy()); 2456 } 2457 ; 2458 dst.version = version == null ? null : version.copy(); 2459 dst.status = status == null ? null : status.copy(); 2460 dst.date = date == null ? null : date.copy(); 2461 dst.publisher = publisher == null ? null : publisher.copy(); 2462 if (telecom != null) { 2463 dst.telecom = new ArrayList<ContactPoint>(); 2464 for (ContactPoint i : telecom) 2465 dst.telecom.add(i.copy()); 2466 } 2467 ; 2468 if (subjectType != null) { 2469 dst.subjectType = new ArrayList<CodeType>(); 2470 for (CodeType i : subjectType) 2471 dst.subjectType.add(i.copy()); 2472 } 2473 ; 2474 dst.group = group == null ? null : group.copy(); 2475 return dst; 2476 } 2477 2478 protected Questionnaire typedCopy() { 2479 return copy(); 2480 } 2481 2482 @Override 2483 public boolean equalsDeep(Base other) { 2484 if (!super.equalsDeep(other)) 2485 return false; 2486 if (!(other instanceof Questionnaire)) 2487 return false; 2488 Questionnaire o = (Questionnaire) other; 2489 return compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2490 && compareDeep(status, o.status, true) && compareDeep(date, o.date, true) 2491 && compareDeep(publisher, o.publisher, true) && compareDeep(telecom, o.telecom, true) 2492 && compareDeep(subjectType, o.subjectType, true) && compareDeep(group, o.group, true); 2493 } 2494 2495 @Override 2496 public boolean equalsShallow(Base other) { 2497 if (!super.equalsShallow(other)) 2498 return false; 2499 if (!(other instanceof Questionnaire)) 2500 return false; 2501 Questionnaire o = (Questionnaire) other; 2502 return compareValues(version, o.version, true) && compareValues(status, o.status, true) 2503 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 2504 && compareValues(subjectType, o.subjectType, true); 2505 } 2506 2507 public boolean isEmpty() { 2508 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (version == null || version.isEmpty()) 2509 && (status == null || status.isEmpty()) && (date == null || date.isEmpty()) 2510 && (publisher == null || publisher.isEmpty()) && (telecom == null || telecom.isEmpty()) 2511 && (subjectType == null || subjectType.isEmpty()) && (group == null || group.isEmpty()); 2512 } 2513 2514 @Override 2515 public ResourceType getResourceType() { 2516 return ResourceType.Questionnaire; 2517 } 2518 2519 @SearchParamDefinition(name = "date", path = "Questionnaire.date", description = "When the questionnaire was last changed", type = "date") 2520 public static final String SP_DATE = "date"; 2521 @SearchParamDefinition(name = "identifier", path = "Questionnaire.identifier", description = "An identifier for the questionnaire", type = "token") 2522 public static final String SP_IDENTIFIER = "identifier"; 2523 @SearchParamDefinition(name = "code", path = "Questionnaire.group.concept", description = "A code that corresponds to the questionnaire or one of its groups", type = "token") 2524 public static final String SP_CODE = "code"; 2525 @SearchParamDefinition(name = "publisher", path = "Questionnaire.publisher", description = "The author of the questionnaire", type = "string") 2526 public static final String SP_PUBLISHER = "publisher"; 2527 @SearchParamDefinition(name = "title", path = "Questionnaire.group.title", description = "All or part of the name of the questionnaire (title for the root group of the questionnaire)", type = "string") 2528 public static final String SP_TITLE = "title"; 2529 @SearchParamDefinition(name = "version", path = "Questionnaire.version", description = "The business version of the questionnaire", type = "string") 2530 public static final String SP_VERSION = "version"; 2531 @SearchParamDefinition(name = "status", path = "Questionnaire.status", description = "The status of the questionnaire", type = "token") 2532 public static final String SP_STATUS = "status"; 2533 2534}