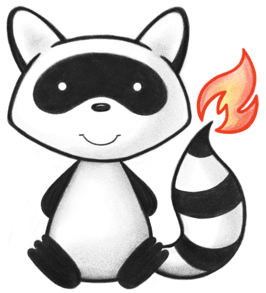
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A structured set of questions intended to guide the collection of answers. 048 * The questions are ordered and grouped into coherent subsets, corresponding to 049 * the structure of the grouping of the underlying questions. 050 */ 051@ResourceDef(name = "Questionnaire", profile = "http://hl7.org/fhir/Profile/Questionnaire") 052public class Questionnaire extends DomainResource { 053 054 public enum QuestionnaireStatus { 055 /** 056 * This Questionnaire is not ready for official use. 057 */ 058 DRAFT, 059 /** 060 * This Questionnaire is ready for use. 061 */ 062 PUBLISHED, 063 /** 064 * This Questionnaire should no longer be used to gather data. 065 */ 066 RETIRED, 067 /** 068 * added to help the parsers 069 */ 070 NULL; 071 072 public static QuestionnaireStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("draft".equals(codeString)) 076 return DRAFT; 077 if ("published".equals(codeString)) 078 return PUBLISHED; 079 if ("retired".equals(codeString)) 080 return RETIRED; 081 throw new FHIRException("Unknown QuestionnaireStatus code '" + codeString + "'"); 082 } 083 084 public String toCode() { 085 switch (this) { 086 case DRAFT: 087 return "draft"; 088 case PUBLISHED: 089 return "published"; 090 case RETIRED: 091 return "retired"; 092 case NULL: 093 return null; 094 default: 095 return "?"; 096 } 097 } 098 099 public String getSystem() { 100 switch (this) { 101 case DRAFT: 102 return "http://hl7.org/fhir/questionnaire-status"; 103 case PUBLISHED: 104 return "http://hl7.org/fhir/questionnaire-status"; 105 case RETIRED: 106 return "http://hl7.org/fhir/questionnaire-status"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getDefinition() { 115 switch (this) { 116 case DRAFT: 117 return "This Questionnaire is not ready for official use."; 118 case PUBLISHED: 119 return "This Questionnaire is ready for use."; 120 case RETIRED: 121 return "This Questionnaire should no longer be used to gather data."; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDisplay() { 130 switch (this) { 131 case DRAFT: 132 return "Draft"; 133 case PUBLISHED: 134 return "Published"; 135 case RETIRED: 136 return "Retired"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 } 144 145 public static class QuestionnaireStatusEnumFactory implements EnumFactory<QuestionnaireStatus> { 146 public QuestionnaireStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("draft".equals(codeString)) 151 return QuestionnaireStatus.DRAFT; 152 if ("published".equals(codeString)) 153 return QuestionnaireStatus.PUBLISHED; 154 if ("retired".equals(codeString)) 155 return QuestionnaireStatus.RETIRED; 156 throw new IllegalArgumentException("Unknown QuestionnaireStatus code '" + codeString + "'"); 157 } 158 159 public Enumeration<QuestionnaireStatus> fromType(Base code) throws FHIRException { 160 if (code == null || code.isEmpty()) 161 return null; 162 String codeString = ((PrimitiveType) code).asStringValue(); 163 if (codeString == null || "".equals(codeString)) 164 return null; 165 if ("draft".equals(codeString)) 166 return new Enumeration<QuestionnaireStatus>(this, QuestionnaireStatus.DRAFT); 167 if ("published".equals(codeString)) 168 return new Enumeration<QuestionnaireStatus>(this, QuestionnaireStatus.PUBLISHED); 169 if ("retired".equals(codeString)) 170 return new Enumeration<QuestionnaireStatus>(this, QuestionnaireStatus.RETIRED); 171 throw new FHIRException("Unknown QuestionnaireStatus code '" + codeString + "'"); 172 } 173 174 public String toCode(QuestionnaireStatus code) { 175 if (code == QuestionnaireStatus.DRAFT) 176 return "draft"; 177 if (code == QuestionnaireStatus.PUBLISHED) 178 return "published"; 179 if (code == QuestionnaireStatus.RETIRED) 180 return "retired"; 181 return "?"; 182 } 183 } 184 185 public enum AnswerFormat { 186 /** 187 * Answer is a yes/no answer. 188 */ 189 BOOLEAN, 190 /** 191 * Answer is a floating point number. 192 */ 193 DECIMAL, 194 /** 195 * Answer is an integer. 196 */ 197 INTEGER, 198 /** 199 * Answer is a date. 200 */ 201 DATE, 202 /** 203 * Answer is a date and time. 204 */ 205 DATETIME, 206 /** 207 * Answer is a system timestamp. 208 */ 209 INSTANT, 210 /** 211 * Answer is a time (hour/minute/second) independent of date. 212 */ 213 TIME, 214 /** 215 * Answer is a short (few words to short sentence) free-text entry. 216 */ 217 STRING, 218 /** 219 * Answer is a long (potentially multi-paragraph) free-text entry (still 220 * captured as a string). 221 */ 222 TEXT, 223 /** 224 * Answer is a url (website, FTP site, etc.). 225 */ 226 URL, 227 /** 228 * Answer is a Coding drawn from a list of options. 229 */ 230 CHOICE, 231 /** 232 * Answer is a Coding drawn from a list of options or a free-text entry. 233 */ 234 OPENCHOICE, 235 /** 236 * Answer is binary content such as a image, PDF, etc. 237 */ 238 ATTACHMENT, 239 /** 240 * Answer is a reference to another resource (practitioner, organization, etc.). 241 */ 242 REFERENCE, 243 /** 244 * Answer is a combination of a numeric value and unit, potentially with a 245 * comparator (<, >, etc.). 246 */ 247 QUANTITY, 248 /** 249 * added to help the parsers 250 */ 251 NULL; 252 253 public static AnswerFormat fromCode(String codeString) throws FHIRException { 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("boolean".equals(codeString)) 257 return BOOLEAN; 258 if ("decimal".equals(codeString)) 259 return DECIMAL; 260 if ("integer".equals(codeString)) 261 return INTEGER; 262 if ("date".equals(codeString)) 263 return DATE; 264 if ("dateTime".equals(codeString)) 265 return DATETIME; 266 if ("instant".equals(codeString)) 267 return INSTANT; 268 if ("time".equals(codeString)) 269 return TIME; 270 if ("string".equals(codeString)) 271 return STRING; 272 if ("text".equals(codeString)) 273 return TEXT; 274 if ("url".equals(codeString)) 275 return URL; 276 if ("choice".equals(codeString)) 277 return CHOICE; 278 if ("open-choice".equals(codeString)) 279 return OPENCHOICE; 280 if ("attachment".equals(codeString)) 281 return ATTACHMENT; 282 if ("reference".equals(codeString)) 283 return REFERENCE; 284 if ("quantity".equals(codeString)) 285 return QUANTITY; 286 throw new FHIRException("Unknown AnswerFormat code '" + codeString + "'"); 287 } 288 289 public String toCode() { 290 switch (this) { 291 case BOOLEAN: 292 return "boolean"; 293 case DECIMAL: 294 return "decimal"; 295 case INTEGER: 296 return "integer"; 297 case DATE: 298 return "date"; 299 case DATETIME: 300 return "dateTime"; 301 case INSTANT: 302 return "instant"; 303 case TIME: 304 return "time"; 305 case STRING: 306 return "string"; 307 case TEXT: 308 return "text"; 309 case URL: 310 return "url"; 311 case CHOICE: 312 return "choice"; 313 case OPENCHOICE: 314 return "open-choice"; 315 case ATTACHMENT: 316 return "attachment"; 317 case REFERENCE: 318 return "reference"; 319 case QUANTITY: 320 return "quantity"; 321 case NULL: 322 return null; 323 default: 324 return "?"; 325 } 326 } 327 328 public String getSystem() { 329 switch (this) { 330 case BOOLEAN: 331 return "http://hl7.org/fhir/answer-format"; 332 case DECIMAL: 333 return "http://hl7.org/fhir/answer-format"; 334 case INTEGER: 335 return "http://hl7.org/fhir/answer-format"; 336 case DATE: 337 return "http://hl7.org/fhir/answer-format"; 338 case DATETIME: 339 return "http://hl7.org/fhir/answer-format"; 340 case INSTANT: 341 return "http://hl7.org/fhir/answer-format"; 342 case TIME: 343 return "http://hl7.org/fhir/answer-format"; 344 case STRING: 345 return "http://hl7.org/fhir/answer-format"; 346 case TEXT: 347 return "http://hl7.org/fhir/answer-format"; 348 case URL: 349 return "http://hl7.org/fhir/answer-format"; 350 case CHOICE: 351 return "http://hl7.org/fhir/answer-format"; 352 case OPENCHOICE: 353 return "http://hl7.org/fhir/answer-format"; 354 case ATTACHMENT: 355 return "http://hl7.org/fhir/answer-format"; 356 case REFERENCE: 357 return "http://hl7.org/fhir/answer-format"; 358 case QUANTITY: 359 return "http://hl7.org/fhir/answer-format"; 360 case NULL: 361 return null; 362 default: 363 return "?"; 364 } 365 } 366 367 public String getDefinition() { 368 switch (this) { 369 case BOOLEAN: 370 return "Answer is a yes/no answer."; 371 case DECIMAL: 372 return "Answer is a floating point number."; 373 case INTEGER: 374 return "Answer is an integer."; 375 case DATE: 376 return "Answer is a date."; 377 case DATETIME: 378 return "Answer is a date and time."; 379 case INSTANT: 380 return "Answer is a system timestamp."; 381 case TIME: 382 return "Answer is a time (hour/minute/second) independent of date."; 383 case STRING: 384 return "Answer is a short (few words to short sentence) free-text entry."; 385 case TEXT: 386 return "Answer is a long (potentially multi-paragraph) free-text entry (still captured as a string)."; 387 case URL: 388 return "Answer is a url (website, FTP site, etc.)."; 389 case CHOICE: 390 return "Answer is a Coding drawn from a list of options."; 391 case OPENCHOICE: 392 return "Answer is a Coding drawn from a list of options or a free-text entry."; 393 case ATTACHMENT: 394 return "Answer is binary content such as a image, PDF, etc."; 395 case REFERENCE: 396 return "Answer is a reference to another resource (practitioner, organization, etc.)."; 397 case QUANTITY: 398 return "Answer is a combination of a numeric value and unit, potentially with a comparator (<, >, etc.)."; 399 case NULL: 400 return null; 401 default: 402 return "?"; 403 } 404 } 405 406 public String getDisplay() { 407 switch (this) { 408 case BOOLEAN: 409 return "Boolean"; 410 case DECIMAL: 411 return "Decimal"; 412 case INTEGER: 413 return "Integer"; 414 case DATE: 415 return "Date"; 416 case DATETIME: 417 return "Date Time"; 418 case INSTANT: 419 return "Instant"; 420 case TIME: 421 return "Time"; 422 case STRING: 423 return "String"; 424 case TEXT: 425 return "Text"; 426 case URL: 427 return "Url"; 428 case CHOICE: 429 return "Choice"; 430 case OPENCHOICE: 431 return "Open Choice"; 432 case ATTACHMENT: 433 return "Attachment"; 434 case REFERENCE: 435 return "Reference"; 436 case QUANTITY: 437 return "Quantity"; 438 case NULL: 439 return null; 440 default: 441 return "?"; 442 } 443 } 444 } 445 446 public static class AnswerFormatEnumFactory implements EnumFactory<AnswerFormat> { 447 public AnswerFormat fromCode(String codeString) throws IllegalArgumentException { 448 if (codeString == null || "".equals(codeString)) 449 if (codeString == null || "".equals(codeString)) 450 return null; 451 if ("boolean".equals(codeString)) 452 return AnswerFormat.BOOLEAN; 453 if ("decimal".equals(codeString)) 454 return AnswerFormat.DECIMAL; 455 if ("integer".equals(codeString)) 456 return AnswerFormat.INTEGER; 457 if ("date".equals(codeString)) 458 return AnswerFormat.DATE; 459 if ("dateTime".equals(codeString)) 460 return AnswerFormat.DATETIME; 461 if ("instant".equals(codeString)) 462 return AnswerFormat.INSTANT; 463 if ("time".equals(codeString)) 464 return AnswerFormat.TIME; 465 if ("string".equals(codeString)) 466 return AnswerFormat.STRING; 467 if ("text".equals(codeString)) 468 return AnswerFormat.TEXT; 469 if ("url".equals(codeString)) 470 return AnswerFormat.URL; 471 if ("choice".equals(codeString)) 472 return AnswerFormat.CHOICE; 473 if ("open-choice".equals(codeString)) 474 return AnswerFormat.OPENCHOICE; 475 if ("attachment".equals(codeString)) 476 return AnswerFormat.ATTACHMENT; 477 if ("reference".equals(codeString)) 478 return AnswerFormat.REFERENCE; 479 if ("quantity".equals(codeString)) 480 return AnswerFormat.QUANTITY; 481 throw new IllegalArgumentException("Unknown AnswerFormat code '" + codeString + "'"); 482 } 483 484 public Enumeration<AnswerFormat> fromType(Base code) throws FHIRException { 485 if (code == null || code.isEmpty()) 486 return null; 487 String codeString = ((PrimitiveType) code).asStringValue(); 488 if (codeString == null || "".equals(codeString)) 489 return null; 490 if ("boolean".equals(codeString)) 491 return new Enumeration<AnswerFormat>(this, AnswerFormat.BOOLEAN); 492 if ("decimal".equals(codeString)) 493 return new Enumeration<AnswerFormat>(this, AnswerFormat.DECIMAL); 494 if ("integer".equals(codeString)) 495 return new Enumeration<AnswerFormat>(this, AnswerFormat.INTEGER); 496 if ("date".equals(codeString)) 497 return new Enumeration<AnswerFormat>(this, AnswerFormat.DATE); 498 if ("dateTime".equals(codeString)) 499 return new Enumeration<AnswerFormat>(this, AnswerFormat.DATETIME); 500 if ("instant".equals(codeString)) 501 return new Enumeration<AnswerFormat>(this, AnswerFormat.INSTANT); 502 if ("time".equals(codeString)) 503 return new Enumeration<AnswerFormat>(this, AnswerFormat.TIME); 504 if ("string".equals(codeString)) 505 return new Enumeration<AnswerFormat>(this, AnswerFormat.STRING); 506 if ("text".equals(codeString)) 507 return new Enumeration<AnswerFormat>(this, AnswerFormat.TEXT); 508 if ("url".equals(codeString)) 509 return new Enumeration<AnswerFormat>(this, AnswerFormat.URL); 510 if ("choice".equals(codeString)) 511 return new Enumeration<AnswerFormat>(this, AnswerFormat.CHOICE); 512 if ("open-choice".equals(codeString)) 513 return new Enumeration<AnswerFormat>(this, AnswerFormat.OPENCHOICE); 514 if ("attachment".equals(codeString)) 515 return new Enumeration<AnswerFormat>(this, AnswerFormat.ATTACHMENT); 516 if ("reference".equals(codeString)) 517 return new Enumeration<AnswerFormat>(this, AnswerFormat.REFERENCE); 518 if ("quantity".equals(codeString)) 519 return new Enumeration<AnswerFormat>(this, AnswerFormat.QUANTITY); 520 throw new FHIRException("Unknown AnswerFormat code '" + codeString + "'"); 521 } 522 523 public String toCode(AnswerFormat code) { 524 if (code == AnswerFormat.BOOLEAN) 525 return "boolean"; 526 if (code == AnswerFormat.DECIMAL) 527 return "decimal"; 528 if (code == AnswerFormat.INTEGER) 529 return "integer"; 530 if (code == AnswerFormat.DATE) 531 return "date"; 532 if (code == AnswerFormat.DATETIME) 533 return "dateTime"; 534 if (code == AnswerFormat.INSTANT) 535 return "instant"; 536 if (code == AnswerFormat.TIME) 537 return "time"; 538 if (code == AnswerFormat.STRING) 539 return "string"; 540 if (code == AnswerFormat.TEXT) 541 return "text"; 542 if (code == AnswerFormat.URL) 543 return "url"; 544 if (code == AnswerFormat.CHOICE) 545 return "choice"; 546 if (code == AnswerFormat.OPENCHOICE) 547 return "open-choice"; 548 if (code == AnswerFormat.ATTACHMENT) 549 return "attachment"; 550 if (code == AnswerFormat.REFERENCE) 551 return "reference"; 552 if (code == AnswerFormat.QUANTITY) 553 return "quantity"; 554 return "?"; 555 } 556 } 557 558 @Block() 559 public static class GroupComponent extends BackboneElement implements IBaseBackboneElement { 560 /** 561 * An identifier that is unique within the Questionnaire allowing linkage to the 562 * equivalent group in a QuestionnaireResponse resource. 563 */ 564 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 565 @Description(shortDefinition = "To link questionnaire with questionnaire response", formalDefinition = "An identifier that is unique within the Questionnaire allowing linkage to the equivalent group in a QuestionnaireResponse resource.") 566 protected StringType linkId; 567 568 /** 569 * The human-readable name for this section of the questionnaire. 570 */ 571 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 572 @Description(shortDefinition = "Name to be displayed for group", formalDefinition = "The human-readable name for this section of the questionnaire.") 573 protected StringType title; 574 575 /** 576 * Identifies a how this group of questions is known in a particular terminology 577 * such as LOINC. 578 */ 579 @Child(name = "concept", type = { 580 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 581 @Description(shortDefinition = "Concept that represents this section in a questionnaire", formalDefinition = "Identifies a how this group of questions is known in a particular terminology such as LOINC.") 582 protected List<Coding> concept; 583 584 /** 585 * Additional text for the group, used for display purposes. 586 */ 587 @Child(name = "text", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 588 @Description(shortDefinition = "Additional text for the group", formalDefinition = "Additional text for the group, used for display purposes.") 589 protected StringType text; 590 591 /** 592 * If true, indicates that the group must be present and have required questions 593 * within it answered. If false, the group may be skipped when answering the 594 * questionnaire. 595 */ 596 @Child(name = "required", type = { 597 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 598 @Description(shortDefinition = "Whether the group must be included in data results", formalDefinition = "If true, indicates that the group must be present and have required questions within it answered. If false, the group may be skipped when answering the questionnaire.") 599 protected BooleanType required; 600 601 /** 602 * Whether the group may occur multiple times in the instance, containing 603 * multiple sets of answers. 604 */ 605 @Child(name = "repeats", type = { 606 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 607 @Description(shortDefinition = "Whether the group may repeat", formalDefinition = "Whether the group may occur multiple times in the instance, containing multiple sets of answers.") 608 protected BooleanType repeats; 609 610 /** 611 * A sub-group within a group. The ordering of groups within this group is 612 * relevant. 613 */ 614 @Child(name = "group", type = { 615 GroupComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 616 @Description(shortDefinition = "Nested questionnaire group", formalDefinition = "A sub-group within a group. The ordering of groups within this group is relevant.") 617 protected List<GroupComponent> group; 618 619 /** 620 * Set of questions within this group. The order of questions within the group 621 * is relevant. 622 */ 623 @Child(name = "question", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 624 @Description(shortDefinition = "Questions in this group", formalDefinition = "Set of questions within this group. The order of questions within the group is relevant.") 625 protected List<QuestionComponent> question; 626 627 private static final long serialVersionUID = 494129548L; 628 629 /* 630 * Constructor 631 */ 632 public GroupComponent() { 633 super(); 634 } 635 636 /** 637 * @return {@link #linkId} (An identifier that is unique within the 638 * Questionnaire allowing linkage to the equivalent group in a 639 * QuestionnaireResponse resource.). This is the underlying object with 640 * id, value and extensions. The accessor "getLinkId" gives direct 641 * access to the value 642 */ 643 public StringType getLinkIdElement() { 644 if (this.linkId == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create GroupComponent.linkId"); 647 else if (Configuration.doAutoCreate()) 648 this.linkId = new StringType(); // bb 649 return this.linkId; 650 } 651 652 public boolean hasLinkIdElement() { 653 return this.linkId != null && !this.linkId.isEmpty(); 654 } 655 656 public boolean hasLinkId() { 657 return this.linkId != null && !this.linkId.isEmpty(); 658 } 659 660 /** 661 * @param value {@link #linkId} (An identifier that is unique within the 662 * Questionnaire allowing linkage to the equivalent group in a 663 * QuestionnaireResponse resource.). This is the underlying object 664 * with id, value and extensions. The accessor "getLinkId" gives 665 * direct access to the value 666 */ 667 public GroupComponent setLinkIdElement(StringType value) { 668 this.linkId = value; 669 return this; 670 } 671 672 /** 673 * @return An identifier that is unique within the Questionnaire allowing 674 * linkage to the equivalent group in a QuestionnaireResponse resource. 675 */ 676 public String getLinkId() { 677 return this.linkId == null ? null : this.linkId.getValue(); 678 } 679 680 /** 681 * @param value An identifier that is unique within the Questionnaire allowing 682 * linkage to the equivalent group in a QuestionnaireResponse 683 * resource. 684 */ 685 public GroupComponent setLinkId(String value) { 686 if (Utilities.noString(value)) 687 this.linkId = null; 688 else { 689 if (this.linkId == null) 690 this.linkId = new StringType(); 691 this.linkId.setValue(value); 692 } 693 return this; 694 } 695 696 /** 697 * @return {@link #title} (The human-readable name for this section of the 698 * questionnaire.). This is the underlying object with id, value and 699 * extensions. The accessor "getTitle" gives direct access to the value 700 */ 701 public StringType getTitleElement() { 702 if (this.title == null) 703 if (Configuration.errorOnAutoCreate()) 704 throw new Error("Attempt to auto-create GroupComponent.title"); 705 else if (Configuration.doAutoCreate()) 706 this.title = new StringType(); // bb 707 return this.title; 708 } 709 710 public boolean hasTitleElement() { 711 return this.title != null && !this.title.isEmpty(); 712 } 713 714 public boolean hasTitle() { 715 return this.title != null && !this.title.isEmpty(); 716 } 717 718 /** 719 * @param value {@link #title} (The human-readable name for this section of the 720 * questionnaire.). This is the underlying object with id, value 721 * and extensions. The accessor "getTitle" gives direct access to 722 * the value 723 */ 724 public GroupComponent setTitleElement(StringType value) { 725 this.title = value; 726 return this; 727 } 728 729 /** 730 * @return The human-readable name for this section of the questionnaire. 731 */ 732 public String getTitle() { 733 return this.title == null ? null : this.title.getValue(); 734 } 735 736 /** 737 * @param value The human-readable name for this section of the questionnaire. 738 */ 739 public GroupComponent setTitle(String value) { 740 if (Utilities.noString(value)) 741 this.title = null; 742 else { 743 if (this.title == null) 744 this.title = new StringType(); 745 this.title.setValue(value); 746 } 747 return this; 748 } 749 750 /** 751 * @return {@link #concept} (Identifies a how this group of questions is known 752 * in a particular terminology such as LOINC.) 753 */ 754 public List<Coding> getConcept() { 755 if (this.concept == null) 756 this.concept = new ArrayList<Coding>(); 757 return this.concept; 758 } 759 760 public boolean hasConcept() { 761 if (this.concept == null) 762 return false; 763 for (Coding item : this.concept) 764 if (!item.isEmpty()) 765 return true; 766 return false; 767 } 768 769 /** 770 * @return {@link #concept} (Identifies a how this group of questions is known 771 * in a particular terminology such as LOINC.) 772 */ 773 // syntactic sugar 774 public Coding addConcept() { // 3 775 Coding t = new Coding(); 776 if (this.concept == null) 777 this.concept = new ArrayList<Coding>(); 778 this.concept.add(t); 779 return t; 780 } 781 782 // syntactic sugar 783 public GroupComponent addConcept(Coding t) { // 3 784 if (t == null) 785 return this; 786 if (this.concept == null) 787 this.concept = new ArrayList<Coding>(); 788 this.concept.add(t); 789 return this; 790 } 791 792 /** 793 * @return {@link #text} (Additional text for the group, used for display 794 * purposes.). This is the underlying object with id, value and 795 * extensions. The accessor "getText" gives direct access to the value 796 */ 797 public StringType getTextElement() { 798 if (this.text == null) 799 if (Configuration.errorOnAutoCreate()) 800 throw new Error("Attempt to auto-create GroupComponent.text"); 801 else if (Configuration.doAutoCreate()) 802 this.text = new StringType(); // bb 803 return this.text; 804 } 805 806 public boolean hasTextElement() { 807 return this.text != null && !this.text.isEmpty(); 808 } 809 810 public boolean hasText() { 811 return this.text != null && !this.text.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #text} (Additional text for the group, used for display 816 * purposes.). This is the underlying object with id, value and 817 * extensions. The accessor "getText" gives direct access to the 818 * value 819 */ 820 public GroupComponent setTextElement(StringType value) { 821 this.text = value; 822 return this; 823 } 824 825 /** 826 * @return Additional text for the group, used for display purposes. 827 */ 828 public String getText() { 829 return this.text == null ? null : this.text.getValue(); 830 } 831 832 /** 833 * @param value Additional text for the group, used for display purposes. 834 */ 835 public GroupComponent setText(String value) { 836 if (Utilities.noString(value)) 837 this.text = null; 838 else { 839 if (this.text == null) 840 this.text = new StringType(); 841 this.text.setValue(value); 842 } 843 return this; 844 } 845 846 /** 847 * @return {@link #required} (If true, indicates that the group must be present 848 * and have required questions within it answered. If false, the group 849 * may be skipped when answering the questionnaire.). This is the 850 * underlying object with id, value and extensions. The accessor 851 * "getRequired" gives direct access to the value 852 */ 853 public BooleanType getRequiredElement() { 854 if (this.required == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create GroupComponent.required"); 857 else if (Configuration.doAutoCreate()) 858 this.required = new BooleanType(); // bb 859 return this.required; 860 } 861 862 public boolean hasRequiredElement() { 863 return this.required != null && !this.required.isEmpty(); 864 } 865 866 public boolean hasRequired() { 867 return this.required != null && !this.required.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #required} (If true, indicates that the group must be 872 * present and have required questions within it answered. If 873 * false, the group may be skipped when answering the 874 * questionnaire.). This is the underlying object with id, value 875 * and extensions. The accessor "getRequired" gives direct access 876 * to the value 877 */ 878 public GroupComponent setRequiredElement(BooleanType value) { 879 this.required = value; 880 return this; 881 } 882 883 /** 884 * @return If true, indicates that the group must be present and have required 885 * questions within it answered. If false, the group may be skipped when 886 * answering the questionnaire. 887 */ 888 public boolean getRequired() { 889 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 890 } 891 892 /** 893 * @param value If true, indicates that the group must be present and have 894 * required questions within it answered. If false, the group may 895 * be skipped when answering the questionnaire. 896 */ 897 public GroupComponent setRequired(boolean value) { 898 if (this.required == null) 899 this.required = new BooleanType(); 900 this.required.setValue(value); 901 return this; 902 } 903 904 /** 905 * @return {@link #repeats} (Whether the group may occur multiple times in the 906 * instance, containing multiple sets of answers.). This is the 907 * underlying object with id, value and extensions. The accessor 908 * "getRepeats" gives direct access to the value 909 */ 910 public BooleanType getRepeatsElement() { 911 if (this.repeats == null) 912 if (Configuration.errorOnAutoCreate()) 913 throw new Error("Attempt to auto-create GroupComponent.repeats"); 914 else if (Configuration.doAutoCreate()) 915 this.repeats = new BooleanType(); // bb 916 return this.repeats; 917 } 918 919 public boolean hasRepeatsElement() { 920 return this.repeats != null && !this.repeats.isEmpty(); 921 } 922 923 public boolean hasRepeats() { 924 return this.repeats != null && !this.repeats.isEmpty(); 925 } 926 927 /** 928 * @param value {@link #repeats} (Whether the group may occur multiple times in 929 * the instance, containing multiple sets of answers.). This is the 930 * underlying object with id, value and extensions. The accessor 931 * "getRepeats" gives direct access to the value 932 */ 933 public GroupComponent setRepeatsElement(BooleanType value) { 934 this.repeats = value; 935 return this; 936 } 937 938 /** 939 * @return Whether the group may occur multiple times in the instance, 940 * containing multiple sets of answers. 941 */ 942 public boolean getRepeats() { 943 return this.repeats == null || this.repeats.isEmpty() ? false : this.repeats.getValue(); 944 } 945 946 /** 947 * @param value Whether the group may occur multiple times in the instance, 948 * containing multiple sets of answers. 949 */ 950 public GroupComponent setRepeats(boolean value) { 951 if (this.repeats == null) 952 this.repeats = new BooleanType(); 953 this.repeats.setValue(value); 954 return this; 955 } 956 957 /** 958 * @return {@link #group} (A sub-group within a group. The ordering of groups 959 * within this group is relevant.) 960 */ 961 public List<GroupComponent> getGroup() { 962 if (this.group == null) 963 this.group = new ArrayList<GroupComponent>(); 964 return this.group; 965 } 966 967 public boolean hasGroup() { 968 if (this.group == null) 969 return false; 970 for (GroupComponent item : this.group) 971 if (!item.isEmpty()) 972 return true; 973 return false; 974 } 975 976 /** 977 * @return {@link #group} (A sub-group within a group. The ordering of groups 978 * within this group is relevant.) 979 */ 980 // syntactic sugar 981 public GroupComponent addGroup() { // 3 982 GroupComponent t = new GroupComponent(); 983 if (this.group == null) 984 this.group = new ArrayList<GroupComponent>(); 985 this.group.add(t); 986 return t; 987 } 988 989 // syntactic sugar 990 public GroupComponent addGroup(GroupComponent t) { // 3 991 if (t == null) 992 return this; 993 if (this.group == null) 994 this.group = new ArrayList<GroupComponent>(); 995 this.group.add(t); 996 return this; 997 } 998 999 /** 1000 * @return {@link #question} (Set of questions within this group. The order of 1001 * questions within the group is relevant.) 1002 */ 1003 public List<QuestionComponent> getQuestion() { 1004 if (this.question == null) 1005 this.question = new ArrayList<QuestionComponent>(); 1006 return this.question; 1007 } 1008 1009 public boolean hasQuestion() { 1010 if (this.question == null) 1011 return false; 1012 for (QuestionComponent item : this.question) 1013 if (!item.isEmpty()) 1014 return true; 1015 return false; 1016 } 1017 1018 /** 1019 * @return {@link #question} (Set of questions within this group. The order of 1020 * questions within the group is relevant.) 1021 */ 1022 // syntactic sugar 1023 public QuestionComponent addQuestion() { // 3 1024 QuestionComponent t = new QuestionComponent(); 1025 if (this.question == null) 1026 this.question = new ArrayList<QuestionComponent>(); 1027 this.question.add(t); 1028 return t; 1029 } 1030 1031 // syntactic sugar 1032 public GroupComponent addQuestion(QuestionComponent t) { // 3 1033 if (t == null) 1034 return this; 1035 if (this.question == null) 1036 this.question = new ArrayList<QuestionComponent>(); 1037 this.question.add(t); 1038 return this; 1039 } 1040 1041 protected void listChildren(List<Property> childrenList) { 1042 super.listChildren(childrenList); 1043 childrenList.add(new Property("linkId", "string", 1044 "An identifier that is unique within the Questionnaire allowing linkage to the equivalent group in a QuestionnaireResponse resource.", 1045 0, java.lang.Integer.MAX_VALUE, linkId)); 1046 childrenList.add(new Property("title", "string", "The human-readable name for this section of the questionnaire.", 1047 0, java.lang.Integer.MAX_VALUE, title)); 1048 childrenList.add(new Property("concept", "Coding", 1049 "Identifies a how this group of questions is known in a particular terminology such as LOINC.", 0, 1050 java.lang.Integer.MAX_VALUE, concept)); 1051 childrenList.add(new Property("text", "string", "Additional text for the group, used for display purposes.", 0, 1052 java.lang.Integer.MAX_VALUE, text)); 1053 childrenList.add(new Property("required", "boolean", 1054 "If true, indicates that the group must be present and have required questions within it answered. If false, the group may be skipped when answering the questionnaire.", 1055 0, java.lang.Integer.MAX_VALUE, required)); 1056 childrenList.add(new Property("repeats", "boolean", 1057 "Whether the group may occur multiple times in the instance, containing multiple sets of answers.", 0, 1058 java.lang.Integer.MAX_VALUE, repeats)); 1059 childrenList.add(new Property("group", "@Questionnaire.group", 1060 "A sub-group within a group. The ordering of groups within this group is relevant.", 0, 1061 java.lang.Integer.MAX_VALUE, group)); 1062 childrenList.add(new Property("question", "", 1063 "Set of questions within this group. The order of questions within the group is relevant.", 0, 1064 java.lang.Integer.MAX_VALUE, question)); 1065 } 1066 1067 @Override 1068 public void setProperty(String name, Base value) throws FHIRException { 1069 if (name.equals("linkId")) 1070 this.linkId = castToString(value); // StringType 1071 else if (name.equals("title")) 1072 this.title = castToString(value); // StringType 1073 else if (name.equals("concept")) 1074 this.getConcept().add(castToCoding(value)); 1075 else if (name.equals("text")) 1076 this.text = castToString(value); // StringType 1077 else if (name.equals("required")) 1078 this.required = castToBoolean(value); // BooleanType 1079 else if (name.equals("repeats")) 1080 this.repeats = castToBoolean(value); // BooleanType 1081 else if (name.equals("group")) 1082 this.getGroup().add((GroupComponent) value); 1083 else if (name.equals("question")) 1084 this.getQuestion().add((QuestionComponent) value); 1085 else 1086 super.setProperty(name, value); 1087 } 1088 1089 @Override 1090 public Base addChild(String name) throws FHIRException { 1091 if (name.equals("linkId")) { 1092 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.linkId"); 1093 } else if (name.equals("title")) { 1094 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.title"); 1095 } else if (name.equals("concept")) { 1096 return addConcept(); 1097 } else if (name.equals("text")) { 1098 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.text"); 1099 } else if (name.equals("required")) { 1100 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.required"); 1101 } else if (name.equals("repeats")) { 1102 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.repeats"); 1103 } else if (name.equals("group")) { 1104 return addGroup(); 1105 } else if (name.equals("question")) { 1106 return addQuestion(); 1107 } else 1108 return super.addChild(name); 1109 } 1110 1111 public GroupComponent copy() { 1112 GroupComponent dst = new GroupComponent(); 1113 copyValues(dst); 1114 dst.linkId = linkId == null ? null : linkId.copy(); 1115 dst.title = title == null ? null : title.copy(); 1116 if (concept != null) { 1117 dst.concept = new ArrayList<Coding>(); 1118 for (Coding i : concept) 1119 dst.concept.add(i.copy()); 1120 } 1121 ; 1122 dst.text = text == null ? null : text.copy(); 1123 dst.required = required == null ? null : required.copy(); 1124 dst.repeats = repeats == null ? null : repeats.copy(); 1125 if (group != null) { 1126 dst.group = new ArrayList<GroupComponent>(); 1127 for (GroupComponent i : group) 1128 dst.group.add(i.copy()); 1129 } 1130 ; 1131 if (question != null) { 1132 dst.question = new ArrayList<QuestionComponent>(); 1133 for (QuestionComponent i : question) 1134 dst.question.add(i.copy()); 1135 } 1136 ; 1137 return dst; 1138 } 1139 1140 @Override 1141 public boolean equalsDeep(Base other) { 1142 if (!super.equalsDeep(other)) 1143 return false; 1144 if (!(other instanceof GroupComponent)) 1145 return false; 1146 GroupComponent o = (GroupComponent) other; 1147 return compareDeep(linkId, o.linkId, true) && compareDeep(title, o.title, true) 1148 && compareDeep(concept, o.concept, true) && compareDeep(text, o.text, true) 1149 && compareDeep(required, o.required, true) && compareDeep(repeats, o.repeats, true) 1150 && compareDeep(group, o.group, true) && compareDeep(question, o.question, true); 1151 } 1152 1153 @Override 1154 public boolean equalsShallow(Base other) { 1155 if (!super.equalsShallow(other)) 1156 return false; 1157 if (!(other instanceof GroupComponent)) 1158 return false; 1159 GroupComponent o = (GroupComponent) other; 1160 return compareValues(linkId, o.linkId, true) && compareValues(title, o.title, true) 1161 && compareValues(text, o.text, true) && compareValues(required, o.required, true) 1162 && compareValues(repeats, o.repeats, true); 1163 } 1164 1165 public boolean isEmpty() { 1166 return super.isEmpty() && (linkId == null || linkId.isEmpty()) && (title == null || title.isEmpty()) 1167 && (concept == null || concept.isEmpty()) && (text == null || text.isEmpty()) 1168 && (required == null || required.isEmpty()) && (repeats == null || repeats.isEmpty()) 1169 && (group == null || group.isEmpty()) && (question == null || question.isEmpty()); 1170 } 1171 1172 public String fhirType() { 1173 return "Questionnaire.group"; 1174 1175 } 1176 1177 } 1178 1179 @Block() 1180 public static class QuestionComponent extends BackboneElement implements IBaseBackboneElement { 1181 /** 1182 * An identifier that is unique within the questionnaire allowing linkage to the 1183 * equivalent group in a [[[QuestionnaireResponse]]] resource. 1184 */ 1185 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1186 @Description(shortDefinition = "To link questionnaire with questionnaire response", formalDefinition = "An identifier that is unique within the questionnaire allowing linkage to the equivalent group in a [[[QuestionnaireResponse]]] resource.") 1187 protected StringType linkId; 1188 1189 /** 1190 * Identifies a how this question is known in a particular terminology such as 1191 * LOINC. 1192 */ 1193 @Child(name = "concept", type = { 1194 Coding.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1195 @Description(shortDefinition = "Concept that represents this question on a questionnaire", formalDefinition = "Identifies a how this question is known in a particular terminology such as LOINC.") 1196 protected List<Coding> concept; 1197 1198 /** 1199 * The actual question as shown to the user to prompt them for an answer. 1200 */ 1201 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1202 @Description(shortDefinition = "Text of the question as it is shown to the user", formalDefinition = "The actual question as shown to the user to prompt them for an answer.") 1203 protected StringType text; 1204 1205 /** 1206 * The expected format of the answer, e.g. the type of input (string, integer) 1207 * or whether a (multiple) choice is expected. 1208 */ 1209 @Child(name = "type", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1210 @Description(shortDefinition = "boolean | decimal | integer | date | dateTime +", formalDefinition = "The expected format of the answer, e.g. the type of input (string, integer) or whether a (multiple) choice is expected.") 1211 protected Enumeration<AnswerFormat> type; 1212 1213 /** 1214 * If true, indicates that the question must be answered and have required 1215 * groups within it also present. If false, the question and any contained 1216 * groups may be skipped when answering the questionnaire. 1217 */ 1218 @Child(name = "required", type = { 1219 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1220 @Description(shortDefinition = "Whether the question must be answered in data results", formalDefinition = "If true, indicates that the question must be answered and have required groups within it also present. If false, the question and any contained groups may be skipped when answering the questionnaire.") 1221 protected BooleanType required; 1222 1223 /** 1224 * If true, the question may have more than one answer. 1225 */ 1226 @Child(name = "repeats", type = { 1227 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1228 @Description(shortDefinition = "Whether the question can have multiple answers", formalDefinition = "If true, the question may have more than one answer.") 1229 protected BooleanType repeats; 1230 1231 /** 1232 * Reference to a value set containing a list of codes representing permitted 1233 * answers for the question. 1234 */ 1235 @Child(name = "options", type = { ValueSet.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1236 @Description(shortDefinition = "Valueset containing permitted answers", formalDefinition = "Reference to a value set containing a list of codes representing permitted answers for the question.") 1237 protected Reference options; 1238 1239 /** 1240 * The actual object that is the target of the reference (Reference to a value 1241 * set containing a list of codes representing permitted answers for the 1242 * question.) 1243 */ 1244 protected ValueSet optionsTarget; 1245 1246 /** 1247 * For a "choice" question, identifies one of the permitted answers for the 1248 * question. 1249 */ 1250 @Child(name = "option", type = { 1251 Coding.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1252 @Description(shortDefinition = "Permitted answer", formalDefinition = "For a \"choice\" question, identifies one of the permitted answers for the question.") 1253 protected List<Coding> option; 1254 1255 /** 1256 * Nested group, containing nested question for this question. The order of 1257 * groups within the question is relevant. 1258 */ 1259 @Child(name = "group", type = { 1260 GroupComponent.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1261 @Description(shortDefinition = "Nested questionnaire group", formalDefinition = "Nested group, containing nested question for this question. The order of groups within the question is relevant.") 1262 protected List<GroupComponent> group; 1263 1264 private static final long serialVersionUID = -1078951042L; 1265 1266 /* 1267 * Constructor 1268 */ 1269 public QuestionComponent() { 1270 super(); 1271 } 1272 1273 /** 1274 * @return {@link #linkId} (An identifier that is unique within the 1275 * questionnaire allowing linkage to the equivalent group in a 1276 * [[[QuestionnaireResponse]]] resource.). This is the underlying object 1277 * with id, value and extensions. The accessor "getLinkId" gives direct 1278 * access to the value 1279 */ 1280 public StringType getLinkIdElement() { 1281 if (this.linkId == null) 1282 if (Configuration.errorOnAutoCreate()) 1283 throw new Error("Attempt to auto-create QuestionComponent.linkId"); 1284 else if (Configuration.doAutoCreate()) 1285 this.linkId = new StringType(); // bb 1286 return this.linkId; 1287 } 1288 1289 public boolean hasLinkIdElement() { 1290 return this.linkId != null && !this.linkId.isEmpty(); 1291 } 1292 1293 public boolean hasLinkId() { 1294 return this.linkId != null && !this.linkId.isEmpty(); 1295 } 1296 1297 /** 1298 * @param value {@link #linkId} (An identifier that is unique within the 1299 * questionnaire allowing linkage to the equivalent group in a 1300 * [[[QuestionnaireResponse]]] resource.). This is the underlying 1301 * object with id, value and extensions. The accessor "getLinkId" 1302 * gives direct access to the value 1303 */ 1304 public QuestionComponent setLinkIdElement(StringType value) { 1305 this.linkId = value; 1306 return this; 1307 } 1308 1309 /** 1310 * @return An identifier that is unique within the questionnaire allowing 1311 * linkage to the equivalent group in a [[[QuestionnaireResponse]]] 1312 * resource. 1313 */ 1314 public String getLinkId() { 1315 return this.linkId == null ? null : this.linkId.getValue(); 1316 } 1317 1318 /** 1319 * @param value An identifier that is unique within the questionnaire allowing 1320 * linkage to the equivalent group in a [[[QuestionnaireResponse]]] 1321 * resource. 1322 */ 1323 public QuestionComponent setLinkId(String value) { 1324 if (Utilities.noString(value)) 1325 this.linkId = null; 1326 else { 1327 if (this.linkId == null) 1328 this.linkId = new StringType(); 1329 this.linkId.setValue(value); 1330 } 1331 return this; 1332 } 1333 1334 /** 1335 * @return {@link #concept} (Identifies a how this question is known in a 1336 * particular terminology such as LOINC.) 1337 */ 1338 public List<Coding> getConcept() { 1339 if (this.concept == null) 1340 this.concept = new ArrayList<Coding>(); 1341 return this.concept; 1342 } 1343 1344 public boolean hasConcept() { 1345 if (this.concept == null) 1346 return false; 1347 for (Coding item : this.concept) 1348 if (!item.isEmpty()) 1349 return true; 1350 return false; 1351 } 1352 1353 /** 1354 * @return {@link #concept} (Identifies a how this question is known in a 1355 * particular terminology such as LOINC.) 1356 */ 1357 // syntactic sugar 1358 public Coding addConcept() { // 3 1359 Coding t = new Coding(); 1360 if (this.concept == null) 1361 this.concept = new ArrayList<Coding>(); 1362 this.concept.add(t); 1363 return t; 1364 } 1365 1366 // syntactic sugar 1367 public QuestionComponent addConcept(Coding t) { // 3 1368 if (t == null) 1369 return this; 1370 if (this.concept == null) 1371 this.concept = new ArrayList<Coding>(); 1372 this.concept.add(t); 1373 return this; 1374 } 1375 1376 /** 1377 * @return {@link #text} (The actual question as shown to the user to prompt 1378 * them for an answer.). This is the underlying object with id, value 1379 * and extensions. The accessor "getText" gives direct access to the 1380 * value 1381 */ 1382 public StringType getTextElement() { 1383 if (this.text == null) 1384 if (Configuration.errorOnAutoCreate()) 1385 throw new Error("Attempt to auto-create QuestionComponent.text"); 1386 else if (Configuration.doAutoCreate()) 1387 this.text = new StringType(); // bb 1388 return this.text; 1389 } 1390 1391 public boolean hasTextElement() { 1392 return this.text != null && !this.text.isEmpty(); 1393 } 1394 1395 public boolean hasText() { 1396 return this.text != null && !this.text.isEmpty(); 1397 } 1398 1399 /** 1400 * @param value {@link #text} (The actual question as shown to the user to 1401 * prompt them for an answer.). This is the underlying object with 1402 * id, value and extensions. The accessor "getText" gives direct 1403 * access to the value 1404 */ 1405 public QuestionComponent setTextElement(StringType value) { 1406 this.text = value; 1407 return this; 1408 } 1409 1410 /** 1411 * @return The actual question as shown to the user to prompt them for an 1412 * answer. 1413 */ 1414 public String getText() { 1415 return this.text == null ? null : this.text.getValue(); 1416 } 1417 1418 /** 1419 * @param value The actual question as shown to the user to prompt them for an 1420 * answer. 1421 */ 1422 public QuestionComponent setText(String value) { 1423 if (Utilities.noString(value)) 1424 this.text = null; 1425 else { 1426 if (this.text == null) 1427 this.text = new StringType(); 1428 this.text.setValue(value); 1429 } 1430 return this; 1431 } 1432 1433 /** 1434 * @return {@link #type} (The expected format of the answer, e.g. the type of 1435 * input (string, integer) or whether a (multiple) choice is expected.). 1436 * This is the underlying object with id, value and extensions. The 1437 * accessor "getType" gives direct access to the value 1438 */ 1439 public Enumeration<AnswerFormat> getTypeElement() { 1440 if (this.type == null) 1441 if (Configuration.errorOnAutoCreate()) 1442 throw new Error("Attempt to auto-create QuestionComponent.type"); 1443 else if (Configuration.doAutoCreate()) 1444 this.type = new Enumeration<AnswerFormat>(new AnswerFormatEnumFactory()); // bb 1445 return this.type; 1446 } 1447 1448 public boolean hasTypeElement() { 1449 return this.type != null && !this.type.isEmpty(); 1450 } 1451 1452 public boolean hasType() { 1453 return this.type != null && !this.type.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #type} (The expected format of the answer, e.g. the type 1458 * of input (string, integer) or whether a (multiple) choice is 1459 * expected.). This is the underlying object with id, value and 1460 * extensions. The accessor "getType" gives direct access to the 1461 * value 1462 */ 1463 public QuestionComponent setTypeElement(Enumeration<AnswerFormat> value) { 1464 this.type = value; 1465 return this; 1466 } 1467 1468 /** 1469 * @return The expected format of the answer, e.g. the type of input (string, 1470 * integer) or whether a (multiple) choice is expected. 1471 */ 1472 public AnswerFormat getType() { 1473 return this.type == null ? null : this.type.getValue(); 1474 } 1475 1476 /** 1477 * @param value The expected format of the answer, e.g. the type of input 1478 * (string, integer) or whether a (multiple) choice is expected. 1479 */ 1480 public QuestionComponent setType(AnswerFormat value) { 1481 if (value == null) 1482 this.type = null; 1483 else { 1484 if (this.type == null) 1485 this.type = new Enumeration<AnswerFormat>(new AnswerFormatEnumFactory()); 1486 this.type.setValue(value); 1487 } 1488 return this; 1489 } 1490 1491 /** 1492 * @return {@link #required} (If true, indicates that the question must be 1493 * answered and have required groups within it also present. If false, 1494 * the question and any contained groups may be skipped when answering 1495 * the questionnaire.). This is the underlying object with id, value and 1496 * extensions. The accessor "getRequired" gives direct access to the 1497 * value 1498 */ 1499 public BooleanType getRequiredElement() { 1500 if (this.required == null) 1501 if (Configuration.errorOnAutoCreate()) 1502 throw new Error("Attempt to auto-create QuestionComponent.required"); 1503 else if (Configuration.doAutoCreate()) 1504 this.required = new BooleanType(); // bb 1505 return this.required; 1506 } 1507 1508 public boolean hasRequiredElement() { 1509 return this.required != null && !this.required.isEmpty(); 1510 } 1511 1512 public boolean hasRequired() { 1513 return this.required != null && !this.required.isEmpty(); 1514 } 1515 1516 /** 1517 * @param value {@link #required} (If true, indicates that the question must be 1518 * answered and have required groups within it also present. If 1519 * false, the question and any contained groups may be skipped when 1520 * answering the questionnaire.). This is the underlying object 1521 * with id, value and extensions. The accessor "getRequired" gives 1522 * direct access to the value 1523 */ 1524 public QuestionComponent setRequiredElement(BooleanType value) { 1525 this.required = value; 1526 return this; 1527 } 1528 1529 /** 1530 * @return If true, indicates that the question must be answered and have 1531 * required groups within it also present. If false, the question and 1532 * any contained groups may be skipped when answering the questionnaire. 1533 */ 1534 public boolean getRequired() { 1535 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 1536 } 1537 1538 /** 1539 * @param value If true, indicates that the question must be answered and have 1540 * required groups within it also present. If false, the question 1541 * and any contained groups may be skipped when answering the 1542 * questionnaire. 1543 */ 1544 public QuestionComponent setRequired(boolean value) { 1545 if (this.required == null) 1546 this.required = new BooleanType(); 1547 this.required.setValue(value); 1548 return this; 1549 } 1550 1551 /** 1552 * @return {@link #repeats} (If true, the question may have more than one 1553 * answer.). This is the underlying object with id, value and 1554 * extensions. The accessor "getRepeats" gives direct access to the 1555 * value 1556 */ 1557 public BooleanType getRepeatsElement() { 1558 if (this.repeats == null) 1559 if (Configuration.errorOnAutoCreate()) 1560 throw new Error("Attempt to auto-create QuestionComponent.repeats"); 1561 else if (Configuration.doAutoCreate()) 1562 this.repeats = new BooleanType(); // bb 1563 return this.repeats; 1564 } 1565 1566 public boolean hasRepeatsElement() { 1567 return this.repeats != null && !this.repeats.isEmpty(); 1568 } 1569 1570 public boolean hasRepeats() { 1571 return this.repeats != null && !this.repeats.isEmpty(); 1572 } 1573 1574 /** 1575 * @param value {@link #repeats} (If true, the question may have more than one 1576 * answer.). This is the underlying object with id, value and 1577 * extensions. The accessor "getRepeats" gives direct access to the 1578 * value 1579 */ 1580 public QuestionComponent setRepeatsElement(BooleanType value) { 1581 this.repeats = value; 1582 return this; 1583 } 1584 1585 /** 1586 * @return If true, the question may have more than one answer. 1587 */ 1588 public boolean getRepeats() { 1589 return this.repeats == null || this.repeats.isEmpty() ? false : this.repeats.getValue(); 1590 } 1591 1592 /** 1593 * @param value If true, the question may have more than one answer. 1594 */ 1595 public QuestionComponent setRepeats(boolean value) { 1596 if (this.repeats == null) 1597 this.repeats = new BooleanType(); 1598 this.repeats.setValue(value); 1599 return this; 1600 } 1601 1602 /** 1603 * @return {@link #options} (Reference to a value set containing a list of codes 1604 * representing permitted answers for the question.) 1605 */ 1606 public Reference getOptions() { 1607 if (this.options == null) 1608 if (Configuration.errorOnAutoCreate()) 1609 throw new Error("Attempt to auto-create QuestionComponent.options"); 1610 else if (Configuration.doAutoCreate()) 1611 this.options = new Reference(); // cc 1612 return this.options; 1613 } 1614 1615 public boolean hasOptions() { 1616 return this.options != null && !this.options.isEmpty(); 1617 } 1618 1619 /** 1620 * @param value {@link #options} (Reference to a value set containing a list of 1621 * codes representing permitted answers for the question.) 1622 */ 1623 public QuestionComponent setOptions(Reference value) { 1624 this.options = value; 1625 return this; 1626 } 1627 1628 /** 1629 * @return {@link #options} The actual object that is the target of the 1630 * reference. The reference library doesn't populate this, but you can 1631 * use it to hold the resource if you resolve it. (Reference to a value 1632 * set containing a list of codes representing permitted answers for the 1633 * question.) 1634 */ 1635 public ValueSet getOptionsTarget() { 1636 if (this.optionsTarget == null) 1637 if (Configuration.errorOnAutoCreate()) 1638 throw new Error("Attempt to auto-create QuestionComponent.options"); 1639 else if (Configuration.doAutoCreate()) 1640 this.optionsTarget = new ValueSet(); // aa 1641 return this.optionsTarget; 1642 } 1643 1644 /** 1645 * @param value {@link #options} The actual object that is the target of the 1646 * reference. The reference library doesn't use these, but you can 1647 * use it to hold the resource if you resolve it. (Reference to a 1648 * value set containing a list of codes representing permitted 1649 * answers for the question.) 1650 */ 1651 public QuestionComponent setOptionsTarget(ValueSet value) { 1652 this.optionsTarget = value; 1653 return this; 1654 } 1655 1656 /** 1657 * @return {@link #option} (For a "choice" question, identifies one of the 1658 * permitted answers for the question.) 1659 */ 1660 public List<Coding> getOption() { 1661 if (this.option == null) 1662 this.option = new ArrayList<Coding>(); 1663 return this.option; 1664 } 1665 1666 public boolean hasOption() { 1667 if (this.option == null) 1668 return false; 1669 for (Coding item : this.option) 1670 if (!item.isEmpty()) 1671 return true; 1672 return false; 1673 } 1674 1675 /** 1676 * @return {@link #option} (For a "choice" question, identifies one of the 1677 * permitted answers for the question.) 1678 */ 1679 // syntactic sugar 1680 public Coding addOption() { // 3 1681 Coding t = new Coding(); 1682 if (this.option == null) 1683 this.option = new ArrayList<Coding>(); 1684 this.option.add(t); 1685 return t; 1686 } 1687 1688 // syntactic sugar 1689 public QuestionComponent addOption(Coding t) { // 3 1690 if (t == null) 1691 return this; 1692 if (this.option == null) 1693 this.option = new ArrayList<Coding>(); 1694 this.option.add(t); 1695 return this; 1696 } 1697 1698 /** 1699 * @return {@link #group} (Nested group, containing nested question for this 1700 * question. The order of groups within the question is relevant.) 1701 */ 1702 public List<GroupComponent> getGroup() { 1703 if (this.group == null) 1704 this.group = new ArrayList<GroupComponent>(); 1705 return this.group; 1706 } 1707 1708 public boolean hasGroup() { 1709 if (this.group == null) 1710 return false; 1711 for (GroupComponent item : this.group) 1712 if (!item.isEmpty()) 1713 return true; 1714 return false; 1715 } 1716 1717 /** 1718 * @return {@link #group} (Nested group, containing nested question for this 1719 * question. The order of groups within the question is relevant.) 1720 */ 1721 // syntactic sugar 1722 public GroupComponent addGroup() { // 3 1723 GroupComponent t = new GroupComponent(); 1724 if (this.group == null) 1725 this.group = new ArrayList<GroupComponent>(); 1726 this.group.add(t); 1727 return t; 1728 } 1729 1730 // syntactic sugar 1731 public QuestionComponent addGroup(GroupComponent t) { // 3 1732 if (t == null) 1733 return this; 1734 if (this.group == null) 1735 this.group = new ArrayList<GroupComponent>(); 1736 this.group.add(t); 1737 return this; 1738 } 1739 1740 protected void listChildren(List<Property> childrenList) { 1741 super.listChildren(childrenList); 1742 childrenList.add(new Property("linkId", "string", 1743 "An identifier that is unique within the questionnaire allowing linkage to the equivalent group in a [[[QuestionnaireResponse]]] resource.", 1744 0, java.lang.Integer.MAX_VALUE, linkId)); 1745 childrenList.add(new Property("concept", "Coding", 1746 "Identifies a how this question is known in a particular terminology such as LOINC.", 0, 1747 java.lang.Integer.MAX_VALUE, concept)); 1748 childrenList 1749 .add(new Property("text", "string", "The actual question as shown to the user to prompt them for an answer.", 1750 0, java.lang.Integer.MAX_VALUE, text)); 1751 childrenList.add(new Property("type", "code", 1752 "The expected format of the answer, e.g. the type of input (string, integer) or whether a (multiple) choice is expected.", 1753 0, java.lang.Integer.MAX_VALUE, type)); 1754 childrenList.add(new Property("required", "boolean", 1755 "If true, indicates that the question must be answered and have required groups within it also present. If false, the question and any contained groups may be skipped when answering the questionnaire.", 1756 0, java.lang.Integer.MAX_VALUE, required)); 1757 childrenList.add(new Property("repeats", "boolean", "If true, the question may have more than one answer.", 0, 1758 java.lang.Integer.MAX_VALUE, repeats)); 1759 childrenList.add(new Property("options", "Reference(ValueSet)", 1760 "Reference to a value set containing a list of codes representing permitted answers for the question.", 0, 1761 java.lang.Integer.MAX_VALUE, options)); 1762 childrenList.add(new Property("option", "Coding", 1763 "For a \"choice\" question, identifies one of the permitted answers for the question.", 0, 1764 java.lang.Integer.MAX_VALUE, option)); 1765 childrenList.add(new Property("group", "@Questionnaire.group", 1766 "Nested group, containing nested question for this question. The order of groups within the question is relevant.", 1767 0, java.lang.Integer.MAX_VALUE, group)); 1768 } 1769 1770 @Override 1771 public void setProperty(String name, Base value) throws FHIRException { 1772 if (name.equals("linkId")) 1773 this.linkId = castToString(value); // StringType 1774 else if (name.equals("concept")) 1775 this.getConcept().add(castToCoding(value)); 1776 else if (name.equals("text")) 1777 this.text = castToString(value); // StringType 1778 else if (name.equals("type")) 1779 this.type = new AnswerFormatEnumFactory().fromType(value); // Enumeration<AnswerFormat> 1780 else if (name.equals("required")) 1781 this.required = castToBoolean(value); // BooleanType 1782 else if (name.equals("repeats")) 1783 this.repeats = castToBoolean(value); // BooleanType 1784 else if (name.equals("options")) 1785 this.options = castToReference(value); // Reference 1786 else if (name.equals("option")) 1787 this.getOption().add(castToCoding(value)); 1788 else if (name.equals("group")) 1789 this.getGroup().add((GroupComponent) value); 1790 else 1791 super.setProperty(name, value); 1792 } 1793 1794 @Override 1795 public Base addChild(String name) throws FHIRException { 1796 if (name.equals("linkId")) { 1797 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.linkId"); 1798 } else if (name.equals("concept")) { 1799 return addConcept(); 1800 } else if (name.equals("text")) { 1801 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.text"); 1802 } else if (name.equals("type")) { 1803 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.type"); 1804 } else if (name.equals("required")) { 1805 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.required"); 1806 } else if (name.equals("repeats")) { 1807 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.repeats"); 1808 } else if (name.equals("options")) { 1809 this.options = new Reference(); 1810 return this.options; 1811 } else if (name.equals("option")) { 1812 return addOption(); 1813 } else if (name.equals("group")) { 1814 return addGroup(); 1815 } else 1816 return super.addChild(name); 1817 } 1818 1819 public QuestionComponent copy() { 1820 QuestionComponent dst = new QuestionComponent(); 1821 copyValues(dst); 1822 dst.linkId = linkId == null ? null : linkId.copy(); 1823 if (concept != null) { 1824 dst.concept = new ArrayList<Coding>(); 1825 for (Coding i : concept) 1826 dst.concept.add(i.copy()); 1827 } 1828 ; 1829 dst.text = text == null ? null : text.copy(); 1830 dst.type = type == null ? null : type.copy(); 1831 dst.required = required == null ? null : required.copy(); 1832 dst.repeats = repeats == null ? null : repeats.copy(); 1833 dst.options = options == null ? null : options.copy(); 1834 if (option != null) { 1835 dst.option = new ArrayList<Coding>(); 1836 for (Coding i : option) 1837 dst.option.add(i.copy()); 1838 } 1839 ; 1840 if (group != null) { 1841 dst.group = new ArrayList<GroupComponent>(); 1842 for (GroupComponent i : group) 1843 dst.group.add(i.copy()); 1844 } 1845 ; 1846 return dst; 1847 } 1848 1849 @Override 1850 public boolean equalsDeep(Base other) { 1851 if (!super.equalsDeep(other)) 1852 return false; 1853 if (!(other instanceof QuestionComponent)) 1854 return false; 1855 QuestionComponent o = (QuestionComponent) other; 1856 return compareDeep(linkId, o.linkId, true) && compareDeep(concept, o.concept, true) 1857 && compareDeep(text, o.text, true) && compareDeep(type, o.type, true) 1858 && compareDeep(required, o.required, true) && compareDeep(repeats, o.repeats, true) 1859 && compareDeep(options, o.options, true) && compareDeep(option, o.option, true) 1860 && compareDeep(group, o.group, true); 1861 } 1862 1863 @Override 1864 public boolean equalsShallow(Base other) { 1865 if (!super.equalsShallow(other)) 1866 return false; 1867 if (!(other instanceof QuestionComponent)) 1868 return false; 1869 QuestionComponent o = (QuestionComponent) other; 1870 return compareValues(linkId, o.linkId, true) && compareValues(text, o.text, true) 1871 && compareValues(type, o.type, true) && compareValues(required, o.required, true) 1872 && compareValues(repeats, o.repeats, true); 1873 } 1874 1875 public boolean isEmpty() { 1876 return super.isEmpty() && (linkId == null || linkId.isEmpty()) && (concept == null || concept.isEmpty()) 1877 && (text == null || text.isEmpty()) && (type == null || type.isEmpty()) 1878 && (required == null || required.isEmpty()) && (repeats == null || repeats.isEmpty()) 1879 && (options == null || options.isEmpty()) && (option == null || option.isEmpty()) 1880 && (group == null || group.isEmpty()); 1881 } 1882 1883 public String fhirType() { 1884 return "Questionnaire.group.question"; 1885 1886 } 1887 1888 } 1889 1890 /** 1891 * This records identifiers associated with this question set that are defined 1892 * by business processes and/or used to refer to it when a direct URL reference 1893 * to the resource itself is not appropriate (e.g. in CDA documents, or in 1894 * written / printed documentation). 1895 */ 1896 @Child(name = "identifier", type = { 1897 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1898 @Description(shortDefinition = "External identifiers for this questionnaire", formalDefinition = "This records identifiers associated with this question set that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 1899 protected List<Identifier> identifier; 1900 1901 /** 1902 * The version number assigned by the publisher for business reasons. It may 1903 * remain the same when the resource is updated. 1904 */ 1905 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1906 @Description(shortDefinition = "Logical identifier for this version of Questionnaire", formalDefinition = "The version number assigned by the publisher for business reasons. It may remain the same when the resource is updated.") 1907 protected StringType version; 1908 1909 /** 1910 * The lifecycle status of the questionnaire as a whole. 1911 */ 1912 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 1913 @Description(shortDefinition = "draft | published | retired", formalDefinition = "The lifecycle status of the questionnaire as a whole.") 1914 protected Enumeration<QuestionnaireStatus> status; 1915 1916 /** 1917 * The date that this questionnaire was last changed. 1918 */ 1919 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1920 @Description(shortDefinition = "Date this version was authored", formalDefinition = "The date that this questionnaire was last changed.") 1921 protected DateTimeType date; 1922 1923 /** 1924 * Organization or person responsible for developing and maintaining the 1925 * questionnaire. 1926 */ 1927 @Child(name = "publisher", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1928 @Description(shortDefinition = "Organization/individual who designed the questionnaire", formalDefinition = "Organization or person responsible for developing and maintaining the questionnaire.") 1929 protected StringType publisher; 1930 1931 /** 1932 * Contact details to assist a user in finding and communicating with the 1933 * publisher. 1934 */ 1935 @Child(name = "telecom", type = { 1936 ContactPoint.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1937 @Description(shortDefinition = "Contact information of the publisher", formalDefinition = "Contact details to assist a user in finding and communicating with the publisher.") 1938 protected List<ContactPoint> telecom; 1939 1940 /** 1941 * Identifies the types of subjects that can be the subject of the 1942 * questionnaire. 1943 */ 1944 @Child(name = "subjectType", type = { 1945 CodeType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1946 @Description(shortDefinition = "Resource that can be subject of QuestionnaireResponse", formalDefinition = "Identifies the types of subjects that can be the subject of the questionnaire.") 1947 protected List<CodeType> subjectType; 1948 1949 /** 1950 * A collection of related questions (or further groupings of questions). 1951 */ 1952 @Child(name = "group", type = {}, order = 7, min = 1, max = 1, modifier = false, summary = true) 1953 @Description(shortDefinition = "Grouped questions", formalDefinition = "A collection of related questions (or further groupings of questions).") 1954 protected GroupComponent group; 1955 1956 private static final long serialVersionUID = -1348292652L; 1957 1958 /* 1959 * Constructor 1960 */ 1961 public Questionnaire() { 1962 super(); 1963 } 1964 1965 /* 1966 * Constructor 1967 */ 1968 public Questionnaire(Enumeration<QuestionnaireStatus> status, GroupComponent group) { 1969 super(); 1970 this.status = status; 1971 this.group = group; 1972 } 1973 1974 /** 1975 * @return {@link #identifier} (This records identifiers associated with this 1976 * question set that are defined by business processes and/or used to 1977 * refer to it when a direct URL reference to the resource itself is not 1978 * appropriate (e.g. in CDA documents, or in written / printed 1979 * documentation).) 1980 */ 1981 public List<Identifier> getIdentifier() { 1982 if (this.identifier == null) 1983 this.identifier = new ArrayList<Identifier>(); 1984 return this.identifier; 1985 } 1986 1987 public boolean hasIdentifier() { 1988 if (this.identifier == null) 1989 return false; 1990 for (Identifier item : this.identifier) 1991 if (!item.isEmpty()) 1992 return true; 1993 return false; 1994 } 1995 1996 /** 1997 * @return {@link #identifier} (This records identifiers associated with this 1998 * question set that are defined by business processes and/or used to 1999 * refer to it when a direct URL reference to the resource itself is not 2000 * appropriate (e.g. in CDA documents, or in written / printed 2001 * documentation).) 2002 */ 2003 // syntactic sugar 2004 public Identifier addIdentifier() { // 3 2005 Identifier t = new Identifier(); 2006 if (this.identifier == null) 2007 this.identifier = new ArrayList<Identifier>(); 2008 this.identifier.add(t); 2009 return t; 2010 } 2011 2012 // syntactic sugar 2013 public Questionnaire addIdentifier(Identifier t) { // 3 2014 if (t == null) 2015 return this; 2016 if (this.identifier == null) 2017 this.identifier = new ArrayList<Identifier>(); 2018 this.identifier.add(t); 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #version} (The version number assigned by the publisher for 2024 * business reasons. It may remain the same when the resource is 2025 * updated.). This is the underlying object with id, value and 2026 * extensions. The accessor "getVersion" gives direct access to the 2027 * value 2028 */ 2029 public StringType getVersionElement() { 2030 if (this.version == null) 2031 if (Configuration.errorOnAutoCreate()) 2032 throw new Error("Attempt to auto-create Questionnaire.version"); 2033 else if (Configuration.doAutoCreate()) 2034 this.version = new StringType(); // bb 2035 return this.version; 2036 } 2037 2038 public boolean hasVersionElement() { 2039 return this.version != null && !this.version.isEmpty(); 2040 } 2041 2042 public boolean hasVersion() { 2043 return this.version != null && !this.version.isEmpty(); 2044 } 2045 2046 /** 2047 * @param value {@link #version} (The version number assigned by the publisher 2048 * for business reasons. It may remain the same when the resource 2049 * is updated.). This is the underlying object with id, value and 2050 * extensions. The accessor "getVersion" gives direct access to the 2051 * value 2052 */ 2053 public Questionnaire setVersionElement(StringType value) { 2054 this.version = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return The version number assigned by the publisher for business reasons. It 2060 * may remain the same when the resource is updated. 2061 */ 2062 public String getVersion() { 2063 return this.version == null ? null : this.version.getValue(); 2064 } 2065 2066 /** 2067 * @param value The version number assigned by the publisher for business 2068 * reasons. It may remain the same when the resource is updated. 2069 */ 2070 public Questionnaire setVersion(String value) { 2071 if (Utilities.noString(value)) 2072 this.version = null; 2073 else { 2074 if (this.version == null) 2075 this.version = new StringType(); 2076 this.version.setValue(value); 2077 } 2078 return this; 2079 } 2080 2081 /** 2082 * @return {@link #status} (The lifecycle status of the questionnaire as a 2083 * whole.). This is the underlying object with id, value and extensions. 2084 * The accessor "getStatus" gives direct access to the value 2085 */ 2086 public Enumeration<QuestionnaireStatus> getStatusElement() { 2087 if (this.status == null) 2088 if (Configuration.errorOnAutoCreate()) 2089 throw new Error("Attempt to auto-create Questionnaire.status"); 2090 else if (Configuration.doAutoCreate()) 2091 this.status = new Enumeration<QuestionnaireStatus>(new QuestionnaireStatusEnumFactory()); // bb 2092 return this.status; 2093 } 2094 2095 public boolean hasStatusElement() { 2096 return this.status != null && !this.status.isEmpty(); 2097 } 2098 2099 public boolean hasStatus() { 2100 return this.status != null && !this.status.isEmpty(); 2101 } 2102 2103 /** 2104 * @param value {@link #status} (The lifecycle status of the questionnaire as a 2105 * whole.). This is the underlying object with id, value and 2106 * extensions. The accessor "getStatus" gives direct access to the 2107 * value 2108 */ 2109 public Questionnaire setStatusElement(Enumeration<QuestionnaireStatus> value) { 2110 this.status = value; 2111 return this; 2112 } 2113 2114 /** 2115 * @return The lifecycle status of the questionnaire as a whole. 2116 */ 2117 public QuestionnaireStatus getStatus() { 2118 return this.status == null ? null : this.status.getValue(); 2119 } 2120 2121 /** 2122 * @param value The lifecycle status of the questionnaire as a whole. 2123 */ 2124 public Questionnaire setStatus(QuestionnaireStatus value) { 2125 if (this.status == null) 2126 this.status = new Enumeration<QuestionnaireStatus>(new QuestionnaireStatusEnumFactory()); 2127 this.status.setValue(value); 2128 return this; 2129 } 2130 2131 /** 2132 * @return {@link #date} (The date that this questionnaire was last changed.). 2133 * This is the underlying object with id, value and extensions. The 2134 * accessor "getDate" gives direct access to the value 2135 */ 2136 public DateTimeType getDateElement() { 2137 if (this.date == null) 2138 if (Configuration.errorOnAutoCreate()) 2139 throw new Error("Attempt to auto-create Questionnaire.date"); 2140 else if (Configuration.doAutoCreate()) 2141 this.date = new DateTimeType(); // bb 2142 return this.date; 2143 } 2144 2145 public boolean hasDateElement() { 2146 return this.date != null && !this.date.isEmpty(); 2147 } 2148 2149 public boolean hasDate() { 2150 return this.date != null && !this.date.isEmpty(); 2151 } 2152 2153 /** 2154 * @param value {@link #date} (The date that this questionnaire was last 2155 * changed.). This is the underlying object with id, value and 2156 * extensions. The accessor "getDate" gives direct access to the 2157 * value 2158 */ 2159 public Questionnaire setDateElement(DateTimeType value) { 2160 this.date = value; 2161 return this; 2162 } 2163 2164 /** 2165 * @return The date that this questionnaire was last changed. 2166 */ 2167 public Date getDate() { 2168 return this.date == null ? null : this.date.getValue(); 2169 } 2170 2171 /** 2172 * @param value The date that this questionnaire was last changed. 2173 */ 2174 public Questionnaire setDate(Date value) { 2175 if (value == null) 2176 this.date = null; 2177 else { 2178 if (this.date == null) 2179 this.date = new DateTimeType(); 2180 this.date.setValue(value); 2181 } 2182 return this; 2183 } 2184 2185 /** 2186 * @return {@link #publisher} (Organization or person responsible for developing 2187 * and maintaining the questionnaire.). This is the underlying object 2188 * with id, value and extensions. The accessor "getPublisher" gives 2189 * direct access to the value 2190 */ 2191 public StringType getPublisherElement() { 2192 if (this.publisher == null) 2193 if (Configuration.errorOnAutoCreate()) 2194 throw new Error("Attempt to auto-create Questionnaire.publisher"); 2195 else if (Configuration.doAutoCreate()) 2196 this.publisher = new StringType(); // bb 2197 return this.publisher; 2198 } 2199 2200 public boolean hasPublisherElement() { 2201 return this.publisher != null && !this.publisher.isEmpty(); 2202 } 2203 2204 public boolean hasPublisher() { 2205 return this.publisher != null && !this.publisher.isEmpty(); 2206 } 2207 2208 /** 2209 * @param value {@link #publisher} (Organization or person responsible for 2210 * developing and maintaining the questionnaire.). This is the 2211 * underlying object with id, value and extensions. The accessor 2212 * "getPublisher" gives direct access to the value 2213 */ 2214 public Questionnaire setPublisherElement(StringType value) { 2215 this.publisher = value; 2216 return this; 2217 } 2218 2219 /** 2220 * @return Organization or person responsible for developing and maintaining the 2221 * questionnaire. 2222 */ 2223 public String getPublisher() { 2224 return this.publisher == null ? null : this.publisher.getValue(); 2225 } 2226 2227 /** 2228 * @param value Organization or person responsible for developing and 2229 * maintaining the questionnaire. 2230 */ 2231 public Questionnaire setPublisher(String value) { 2232 if (Utilities.noString(value)) 2233 this.publisher = null; 2234 else { 2235 if (this.publisher == null) 2236 this.publisher = new StringType(); 2237 this.publisher.setValue(value); 2238 } 2239 return this; 2240 } 2241 2242 /** 2243 * @return {@link #telecom} (Contact details to assist a user in finding and 2244 * communicating with the publisher.) 2245 */ 2246 public List<ContactPoint> getTelecom() { 2247 if (this.telecom == null) 2248 this.telecom = new ArrayList<ContactPoint>(); 2249 return this.telecom; 2250 } 2251 2252 public boolean hasTelecom() { 2253 if (this.telecom == null) 2254 return false; 2255 for (ContactPoint item : this.telecom) 2256 if (!item.isEmpty()) 2257 return true; 2258 return false; 2259 } 2260 2261 /** 2262 * @return {@link #telecom} (Contact details to assist a user in finding and 2263 * communicating with the publisher.) 2264 */ 2265 // syntactic sugar 2266 public ContactPoint addTelecom() { // 3 2267 ContactPoint t = new ContactPoint(); 2268 if (this.telecom == null) 2269 this.telecom = new ArrayList<ContactPoint>(); 2270 this.telecom.add(t); 2271 return t; 2272 } 2273 2274 // syntactic sugar 2275 public Questionnaire addTelecom(ContactPoint t) { // 3 2276 if (t == null) 2277 return this; 2278 if (this.telecom == null) 2279 this.telecom = new ArrayList<ContactPoint>(); 2280 this.telecom.add(t); 2281 return this; 2282 } 2283 2284 /** 2285 * @return {@link #subjectType} (Identifies the types of subjects that can be 2286 * the subject of the questionnaire.) 2287 */ 2288 public List<CodeType> getSubjectType() { 2289 if (this.subjectType == null) 2290 this.subjectType = new ArrayList<CodeType>(); 2291 return this.subjectType; 2292 } 2293 2294 public boolean hasSubjectType() { 2295 if (this.subjectType == null) 2296 return false; 2297 for (CodeType item : this.subjectType) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 /** 2304 * @return {@link #subjectType} (Identifies the types of subjects that can be 2305 * the subject of the questionnaire.) 2306 */ 2307 // syntactic sugar 2308 public CodeType addSubjectTypeElement() {// 2 2309 CodeType t = new CodeType(); 2310 if (this.subjectType == null) 2311 this.subjectType = new ArrayList<CodeType>(); 2312 this.subjectType.add(t); 2313 return t; 2314 } 2315 2316 /** 2317 * @param value {@link #subjectType} (Identifies the types of subjects that can 2318 * be the subject of the questionnaire.) 2319 */ 2320 public Questionnaire addSubjectType(String value) { // 1 2321 CodeType t = new CodeType(); 2322 t.setValue(value); 2323 if (this.subjectType == null) 2324 this.subjectType = new ArrayList<CodeType>(); 2325 this.subjectType.add(t); 2326 return this; 2327 } 2328 2329 /** 2330 * @param value {@link #subjectType} (Identifies the types of subjects that can 2331 * be the subject of the questionnaire.) 2332 */ 2333 public boolean hasSubjectType(String value) { 2334 if (this.subjectType == null) 2335 return false; 2336 for (CodeType v : this.subjectType) 2337 if (v.equals(value)) // code 2338 return true; 2339 return false; 2340 } 2341 2342 /** 2343 * @return {@link #group} (A collection of related questions (or further 2344 * groupings of questions).) 2345 */ 2346 public GroupComponent getGroup() { 2347 if (this.group == null) 2348 if (Configuration.errorOnAutoCreate()) 2349 throw new Error("Attempt to auto-create Questionnaire.group"); 2350 else if (Configuration.doAutoCreate()) 2351 this.group = new GroupComponent(); // cc 2352 return this.group; 2353 } 2354 2355 public boolean hasGroup() { 2356 return this.group != null && !this.group.isEmpty(); 2357 } 2358 2359 /** 2360 * @param value {@link #group} (A collection of related questions (or further 2361 * groupings of questions).) 2362 */ 2363 public Questionnaire setGroup(GroupComponent value) { 2364 this.group = value; 2365 return this; 2366 } 2367 2368 protected void listChildren(List<Property> childrenList) { 2369 super.listChildren(childrenList); 2370 childrenList.add(new Property("identifier", "Identifier", 2371 "This records identifiers associated with this question set that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2372 0, java.lang.Integer.MAX_VALUE, identifier)); 2373 childrenList.add(new Property("version", "string", 2374 "The version number assigned by the publisher for business reasons. It may remain the same when the resource is updated.", 2375 0, java.lang.Integer.MAX_VALUE, version)); 2376 childrenList.add(new Property("status", "code", "The lifecycle status of the questionnaire as a whole.", 0, 2377 java.lang.Integer.MAX_VALUE, status)); 2378 childrenList.add(new Property("date", "dateTime", "The date that this questionnaire was last changed.", 0, 2379 java.lang.Integer.MAX_VALUE, date)); 2380 childrenList.add(new Property("publisher", "string", 2381 "Organization or person responsible for developing and maintaining the questionnaire.", 0, 2382 java.lang.Integer.MAX_VALUE, publisher)); 2383 childrenList.add(new Property("telecom", "ContactPoint", 2384 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2385 java.lang.Integer.MAX_VALUE, telecom)); 2386 childrenList.add(new Property("subjectType", "code", 2387 "Identifies the types of subjects that can be the subject of the questionnaire.", 0, 2388 java.lang.Integer.MAX_VALUE, subjectType)); 2389 childrenList.add(new Property("group", "", "A collection of related questions (or further groupings of questions).", 2390 0, java.lang.Integer.MAX_VALUE, group)); 2391 } 2392 2393 @Override 2394 public void setProperty(String name, Base value) throws FHIRException { 2395 if (name.equals("identifier")) 2396 this.getIdentifier().add(castToIdentifier(value)); 2397 else if (name.equals("version")) 2398 this.version = castToString(value); // StringType 2399 else if (name.equals("status")) 2400 this.status = new QuestionnaireStatusEnumFactory().fromType(value); // Enumeration<QuestionnaireStatus> 2401 else if (name.equals("date")) 2402 this.date = castToDateTime(value); // DateTimeType 2403 else if (name.equals("publisher")) 2404 this.publisher = castToString(value); // StringType 2405 else if (name.equals("telecom")) 2406 this.getTelecom().add(castToContactPoint(value)); 2407 else if (name.equals("subjectType")) 2408 this.getSubjectType().add(castToCode(value)); 2409 else if (name.equals("group")) 2410 this.group = (GroupComponent) value; // GroupComponent 2411 else 2412 super.setProperty(name, value); 2413 } 2414 2415 @Override 2416 public Base addChild(String name) throws FHIRException { 2417 if (name.equals("identifier")) { 2418 return addIdentifier(); 2419 } else if (name.equals("version")) { 2420 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.version"); 2421 } else if (name.equals("status")) { 2422 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.status"); 2423 } else if (name.equals("date")) { 2424 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.date"); 2425 } else if (name.equals("publisher")) { 2426 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.publisher"); 2427 } else if (name.equals("telecom")) { 2428 return addTelecom(); 2429 } else if (name.equals("subjectType")) { 2430 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.subjectType"); 2431 } else if (name.equals("group")) { 2432 this.group = new GroupComponent(); 2433 return this.group; 2434 } else 2435 return super.addChild(name); 2436 } 2437 2438 public String fhirType() { 2439 return "Questionnaire"; 2440 2441 } 2442 2443 public Questionnaire copy() { 2444 Questionnaire dst = new Questionnaire(); 2445 copyValues(dst); 2446 if (identifier != null) { 2447 dst.identifier = new ArrayList<Identifier>(); 2448 for (Identifier i : identifier) 2449 dst.identifier.add(i.copy()); 2450 } 2451 ; 2452 dst.version = version == null ? null : version.copy(); 2453 dst.status = status == null ? null : status.copy(); 2454 dst.date = date == null ? null : date.copy(); 2455 dst.publisher = publisher == null ? null : publisher.copy(); 2456 if (telecom != null) { 2457 dst.telecom = new ArrayList<ContactPoint>(); 2458 for (ContactPoint i : telecom) 2459 dst.telecom.add(i.copy()); 2460 } 2461 ; 2462 if (subjectType != null) { 2463 dst.subjectType = new ArrayList<CodeType>(); 2464 for (CodeType i : subjectType) 2465 dst.subjectType.add(i.copy()); 2466 } 2467 ; 2468 dst.group = group == null ? null : group.copy(); 2469 return dst; 2470 } 2471 2472 protected Questionnaire typedCopy() { 2473 return copy(); 2474 } 2475 2476 @Override 2477 public boolean equalsDeep(Base other) { 2478 if (!super.equalsDeep(other)) 2479 return false; 2480 if (!(other instanceof Questionnaire)) 2481 return false; 2482 Questionnaire o = (Questionnaire) other; 2483 return compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2484 && compareDeep(status, o.status, true) && compareDeep(date, o.date, true) 2485 && compareDeep(publisher, o.publisher, true) && compareDeep(telecom, o.telecom, true) 2486 && compareDeep(subjectType, o.subjectType, true) && compareDeep(group, o.group, true); 2487 } 2488 2489 @Override 2490 public boolean equalsShallow(Base other) { 2491 if (!super.equalsShallow(other)) 2492 return false; 2493 if (!(other instanceof Questionnaire)) 2494 return false; 2495 Questionnaire o = (Questionnaire) other; 2496 return compareValues(version, o.version, true) && compareValues(status, o.status, true) 2497 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 2498 && compareValues(subjectType, o.subjectType, true); 2499 } 2500 2501 public boolean isEmpty() { 2502 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (version == null || version.isEmpty()) 2503 && (status == null || status.isEmpty()) && (date == null || date.isEmpty()) 2504 && (publisher == null || publisher.isEmpty()) && (telecom == null || telecom.isEmpty()) 2505 && (subjectType == null || subjectType.isEmpty()) && (group == null || group.isEmpty()); 2506 } 2507 2508 @Override 2509 public ResourceType getResourceType() { 2510 return ResourceType.Questionnaire; 2511 } 2512 2513 @SearchParamDefinition(name = "date", path = "Questionnaire.date", description = "When the questionnaire was last changed", type = "date") 2514 public static final String SP_DATE = "date"; 2515 @SearchParamDefinition(name = "identifier", path = "Questionnaire.identifier", description = "An identifier for the questionnaire", type = "token") 2516 public static final String SP_IDENTIFIER = "identifier"; 2517 @SearchParamDefinition(name = "code", path = "Questionnaire.group.concept", description = "A code that corresponds to the questionnaire or one of its groups", type = "token") 2518 public static final String SP_CODE = "code"; 2519 @SearchParamDefinition(name = "publisher", path = "Questionnaire.publisher", description = "The author of the questionnaire", type = "string") 2520 public static final String SP_PUBLISHER = "publisher"; 2521 @SearchParamDefinition(name = "title", path = "Questionnaire.group.title", description = "All or part of the name of the questionnaire (title for the root group of the questionnaire)", type = "string") 2522 public static final String SP_TITLE = "title"; 2523 @SearchParamDefinition(name = "version", path = "Questionnaire.version", description = "The business version of the questionnaire", type = "string") 2524 public static final String SP_VERSION = "version"; 2525 @SearchParamDefinition(name = "status", path = "Questionnaire.status", description = "The status of the questionnaire", type = "token") 2526 public static final String SP_STATUS = "status"; 2527 2528}