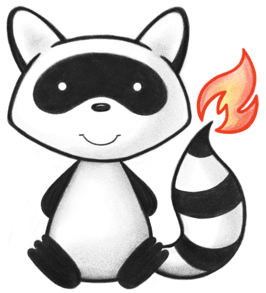
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A structured set of questions and their answers. The questions are ordered 048 * and grouped into coherent subsets, corresponding to the structure of the 049 * grouping of the underlying questions. 050 */ 051@ResourceDef(name = "QuestionnaireResponse", profile = "http://hl7.org/fhir/Profile/QuestionnaireResponse") 052public class QuestionnaireResponse extends DomainResource { 053 054 public enum QuestionnaireResponseStatus { 055 /** 056 * This QuestionnaireResponse has been partially filled out with answers, but 057 * changes or additions are still expected to be made to it. 058 */ 059 INPROGRESS, 060 /** 061 * This QuestionnaireResponse has been filled out with answers, and the current 062 * content is regarded as definitive. 063 */ 064 COMPLETED, 065 /** 066 * This QuestionnaireResponse has been filled out with answers, then marked as 067 * complete, yet changes or additions have been made to it afterwards. 068 */ 069 AMENDED, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static QuestionnaireResponseStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("in-progress".equals(codeString)) 079 return INPROGRESS; 080 if ("completed".equals(codeString)) 081 return COMPLETED; 082 if ("amended".equals(codeString)) 083 return AMENDED; 084 throw new FHIRException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case INPROGRESS: 090 return "in-progress"; 091 case COMPLETED: 092 return "completed"; 093 case AMENDED: 094 return "amended"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 switch (this) { 104 case INPROGRESS: 105 return "http://hl7.org/fhir/questionnaire-answers-status"; 106 case COMPLETED: 107 return "http://hl7.org/fhir/questionnaire-answers-status"; 108 case AMENDED: 109 return "http://hl7.org/fhir/questionnaire-answers-status"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case INPROGRESS: 120 return "This QuestionnaireResponse has been partially filled out with answers, but changes or additions are still expected to be made to it."; 121 case COMPLETED: 122 return "This QuestionnaireResponse has been filled out with answers, and the current content is regarded as definitive."; 123 case AMENDED: 124 return "This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards."; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case INPROGRESS: 135 return "In Progress"; 136 case COMPLETED: 137 return "Completed"; 138 case AMENDED: 139 return "Amended"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 } 147 148 public static class QuestionnaireResponseStatusEnumFactory implements EnumFactory<QuestionnaireResponseStatus> { 149 public QuestionnaireResponseStatus fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("in-progress".equals(codeString)) 154 return QuestionnaireResponseStatus.INPROGRESS; 155 if ("completed".equals(codeString)) 156 return QuestionnaireResponseStatus.COMPLETED; 157 if ("amended".equals(codeString)) 158 return QuestionnaireResponseStatus.AMENDED; 159 throw new IllegalArgumentException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 160 } 161 162 public Enumeration<QuestionnaireResponseStatus> fromType(Base code) throws FHIRException { 163 if (code == null || code.isEmpty()) 164 return null; 165 String codeString = ((PrimitiveType) code).asStringValue(); 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("in-progress".equals(codeString)) 169 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.INPROGRESS); 170 if ("completed".equals(codeString)) 171 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.COMPLETED); 172 if ("amended".equals(codeString)) 173 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.AMENDED); 174 throw new FHIRException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 175 } 176 177 public String toCode(QuestionnaireResponseStatus code) 178 { 179 if (code == QuestionnaireResponseStatus.NULL) 180 return null; 181 if (code == QuestionnaireResponseStatus.INPROGRESS) 182 return "in-progress"; 183 if (code == QuestionnaireResponseStatus.COMPLETED) 184 return "completed"; 185 if (code == QuestionnaireResponseStatus.AMENDED) 186 return "amended"; 187 return "?"; 188 } 189 } 190 191 @Block() 192 public static class GroupComponent extends BackboneElement implements IBaseBackboneElement { 193 /** 194 * Identifies the group from the Questionnaire that corresponds to this group in 195 * the QuestionnaireResponse resource. 196 */ 197 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 198 @Description(shortDefinition = "Corresponding group within Questionnaire", formalDefinition = "Identifies the group from the Questionnaire that corresponds to this group in the QuestionnaireResponse resource.") 199 protected StringType linkId; 200 201 /** 202 * Text that is displayed above the contents of the group. 203 */ 204 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 205 @Description(shortDefinition = "Name for this group", formalDefinition = "Text that is displayed above the contents of the group.") 206 protected StringType title; 207 208 /** 209 * Additional text for the group, used for display purposes. 210 */ 211 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 212 @Description(shortDefinition = "Additional text for the group", formalDefinition = "Additional text for the group, used for display purposes.") 213 protected StringType text; 214 215 /** 216 * More specific subject this section's answers are about, details the subject 217 * given in QuestionnaireResponse. 218 */ 219 @Child(name = "subject", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = false) 220 @Description(shortDefinition = "The subject this group's answers are about", formalDefinition = "More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.") 221 protected Reference subject; 222 223 /** 224 * The actual object that is the target of the reference (More specific subject 225 * this section's answers are about, details the subject given in 226 * QuestionnaireResponse.) 227 */ 228 protected Resource subjectTarget; 229 230 /** 231 * A sub-group within a group. The ordering of groups within this group is 232 * relevant. 233 */ 234 @Child(name = "group", type = { 235 GroupComponent.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 236 @Description(shortDefinition = "Nested questionnaire response group", formalDefinition = "A sub-group within a group. The ordering of groups within this group is relevant.") 237 protected List<GroupComponent> group; 238 239 /** 240 * Set of questions within this group. The order of questions within the group 241 * is relevant. 242 */ 243 @Child(name = "question", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 244 @Description(shortDefinition = "Questions in this group", formalDefinition = "Set of questions within this group. The order of questions within the group is relevant.") 245 protected List<QuestionComponent> question; 246 247 private static final long serialVersionUID = -1045990435L; 248 249 /* 250 * Constructor 251 */ 252 public GroupComponent() { 253 super(); 254 } 255 256 /** 257 * @return {@link #linkId} (Identifies the group from the Questionnaire that 258 * corresponds to this group in the QuestionnaireResponse resource.). 259 * This is the underlying object with id, value and extensions. The 260 * accessor "getLinkId" gives direct access to the value 261 */ 262 public StringType getLinkIdElement() { 263 if (this.linkId == null) 264 if (Configuration.errorOnAutoCreate()) 265 throw new Error("Attempt to auto-create GroupComponent.linkId"); 266 else if (Configuration.doAutoCreate()) 267 this.linkId = new StringType(); // bb 268 return this.linkId; 269 } 270 271 public boolean hasLinkIdElement() { 272 return this.linkId != null && !this.linkId.isEmpty(); 273 } 274 275 public boolean hasLinkId() { 276 return this.linkId != null && !this.linkId.isEmpty(); 277 } 278 279 /** 280 * @param value {@link #linkId} (Identifies the group from the Questionnaire 281 * that corresponds to this group in the QuestionnaireResponse 282 * resource.). This is the underlying object with id, value and 283 * extensions. The accessor "getLinkId" gives direct access to the 284 * value 285 */ 286 public GroupComponent setLinkIdElement(StringType value) { 287 this.linkId = value; 288 return this; 289 } 290 291 /** 292 * @return Identifies the group from the Questionnaire that corresponds to this 293 * group in the QuestionnaireResponse resource. 294 */ 295 public String getLinkId() { 296 return this.linkId == null ? null : this.linkId.getValue(); 297 } 298 299 /** 300 * @param value Identifies the group from the Questionnaire that corresponds to 301 * this group in the QuestionnaireResponse resource. 302 */ 303 public GroupComponent setLinkId(String value) { 304 if (Utilities.noString(value)) 305 this.linkId = null; 306 else { 307 if (this.linkId == null) 308 this.linkId = new StringType(); 309 this.linkId.setValue(value); 310 } 311 return this; 312 } 313 314 /** 315 * @return {@link #title} (Text that is displayed above the contents of the 316 * group.). This is the underlying object with id, value and extensions. 317 * The accessor "getTitle" gives direct access to the value 318 */ 319 public StringType getTitleElement() { 320 if (this.title == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create GroupComponent.title"); 323 else if (Configuration.doAutoCreate()) 324 this.title = new StringType(); // bb 325 return this.title; 326 } 327 328 public boolean hasTitleElement() { 329 return this.title != null && !this.title.isEmpty(); 330 } 331 332 public boolean hasTitle() { 333 return this.title != null && !this.title.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #title} (Text that is displayed above the contents of the 338 * group.). This is the underlying object with id, value and 339 * extensions. The accessor "getTitle" gives direct access to the 340 * value 341 */ 342 public GroupComponent setTitleElement(StringType value) { 343 this.title = value; 344 return this; 345 } 346 347 /** 348 * @return Text that is displayed above the contents of the group. 349 */ 350 public String getTitle() { 351 return this.title == null ? null : this.title.getValue(); 352 } 353 354 /** 355 * @param value Text that is displayed above the contents of the group. 356 */ 357 public GroupComponent setTitle(String value) { 358 if (Utilities.noString(value)) 359 this.title = null; 360 else { 361 if (this.title == null) 362 this.title = new StringType(); 363 this.title.setValue(value); 364 } 365 return this; 366 } 367 368 /** 369 * @return {@link #text} (Additional text for the group, used for display 370 * purposes.). This is the underlying object with id, value and 371 * extensions. The accessor "getText" gives direct access to the value 372 */ 373 public StringType getTextElement() { 374 if (this.text == null) 375 if (Configuration.errorOnAutoCreate()) 376 throw new Error("Attempt to auto-create GroupComponent.text"); 377 else if (Configuration.doAutoCreate()) 378 this.text = new StringType(); // bb 379 return this.text; 380 } 381 382 public boolean hasTextElement() { 383 return this.text != null && !this.text.isEmpty(); 384 } 385 386 public boolean hasText() { 387 return this.text != null && !this.text.isEmpty(); 388 } 389 390 /** 391 * @param value {@link #text} (Additional text for the group, used for display 392 * purposes.). This is the underlying object with id, value and 393 * extensions. The accessor "getText" gives direct access to the 394 * value 395 */ 396 public GroupComponent setTextElement(StringType value) { 397 this.text = value; 398 return this; 399 } 400 401 /** 402 * @return Additional text for the group, used for display purposes. 403 */ 404 public String getText() { 405 return this.text == null ? null : this.text.getValue(); 406 } 407 408 /** 409 * @param value Additional text for the group, used for display purposes. 410 */ 411 public GroupComponent setText(String value) { 412 if (Utilities.noString(value)) 413 this.text = null; 414 else { 415 if (this.text == null) 416 this.text = new StringType(); 417 this.text.setValue(value); 418 } 419 return this; 420 } 421 422 /** 423 * @return {@link #subject} (More specific subject this section's answers are 424 * about, details the subject given in QuestionnaireResponse.) 425 */ 426 public Reference getSubject() { 427 if (this.subject == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create GroupComponent.subject"); 430 else if (Configuration.doAutoCreate()) 431 this.subject = new Reference(); // cc 432 return this.subject; 433 } 434 435 public boolean hasSubject() { 436 return this.subject != null && !this.subject.isEmpty(); 437 } 438 439 /** 440 * @param value {@link #subject} (More specific subject this section's answers 441 * are about, details the subject given in QuestionnaireResponse.) 442 */ 443 public GroupComponent setSubject(Reference value) { 444 this.subject = value; 445 return this; 446 } 447 448 /** 449 * @return {@link #subject} The actual object that is the target of the 450 * reference. The reference library doesn't populate this, but you can 451 * use it to hold the resource if you resolve it. (More specific subject 452 * this section's answers are about, details the subject given in 453 * QuestionnaireResponse.) 454 */ 455 public Resource getSubjectTarget() { 456 return this.subjectTarget; 457 } 458 459 /** 460 * @param value {@link #subject} The actual object that is the target of the 461 * reference. The reference library doesn't use these, but you can 462 * use it to hold the resource if you resolve it. (More specific 463 * subject this section's answers are about, details the subject 464 * given in QuestionnaireResponse.) 465 */ 466 public GroupComponent setSubjectTarget(Resource value) { 467 this.subjectTarget = value; 468 return this; 469 } 470 471 /** 472 * @return {@link #group} (A sub-group within a group. The ordering of groups 473 * within this group is relevant.) 474 */ 475 public List<GroupComponent> getGroup() { 476 if (this.group == null) 477 this.group = new ArrayList<GroupComponent>(); 478 return this.group; 479 } 480 481 public boolean hasGroup() { 482 if (this.group == null) 483 return false; 484 for (GroupComponent item : this.group) 485 if (!item.isEmpty()) 486 return true; 487 return false; 488 } 489 490 /** 491 * @return {@link #group} (A sub-group within a group. The ordering of groups 492 * within this group is relevant.) 493 */ 494 // syntactic sugar 495 public GroupComponent addGroup() { // 3 496 GroupComponent t = new GroupComponent(); 497 if (this.group == null) 498 this.group = new ArrayList<GroupComponent>(); 499 this.group.add(t); 500 return t; 501 } 502 503 // syntactic sugar 504 public GroupComponent addGroup(GroupComponent t) { // 3 505 if (t == null) 506 return this; 507 if (this.group == null) 508 this.group = new ArrayList<GroupComponent>(); 509 this.group.add(t); 510 return this; 511 } 512 513 /** 514 * @return {@link #question} (Set of questions within this group. The order of 515 * questions within the group is relevant.) 516 */ 517 public List<QuestionComponent> getQuestion() { 518 if (this.question == null) 519 this.question = new ArrayList<QuestionComponent>(); 520 return this.question; 521 } 522 523 public boolean hasQuestion() { 524 if (this.question == null) 525 return false; 526 for (QuestionComponent item : this.question) 527 if (!item.isEmpty()) 528 return true; 529 return false; 530 } 531 532 /** 533 * @return {@link #question} (Set of questions within this group. The order of 534 * questions within the group is relevant.) 535 */ 536 // syntactic sugar 537 public QuestionComponent addQuestion() { // 3 538 QuestionComponent t = new QuestionComponent(); 539 if (this.question == null) 540 this.question = new ArrayList<QuestionComponent>(); 541 this.question.add(t); 542 return t; 543 } 544 545 // syntactic sugar 546 public GroupComponent addQuestion(QuestionComponent t) { // 3 547 if (t == null) 548 return this; 549 if (this.question == null) 550 this.question = new ArrayList<QuestionComponent>(); 551 this.question.add(t); 552 return this; 553 } 554 555 protected void listChildren(List<Property> childrenList) { 556 super.listChildren(childrenList); 557 childrenList.add(new Property("linkId", "string", 558 "Identifies the group from the Questionnaire that corresponds to this group in the QuestionnaireResponse resource.", 559 0, java.lang.Integer.MAX_VALUE, linkId)); 560 childrenList.add(new Property("title", "string", "Text that is displayed above the contents of the group.", 0, 561 java.lang.Integer.MAX_VALUE, title)); 562 childrenList.add(new Property("text", "string", "Additional text for the group, used for display purposes.", 0, 563 java.lang.Integer.MAX_VALUE, text)); 564 childrenList.add(new Property("subject", "Reference(Any)", 565 "More specific subject this section's answers are about, details the subject given in QuestionnaireResponse.", 566 0, java.lang.Integer.MAX_VALUE, subject)); 567 childrenList.add(new Property("group", "@QuestionnaireResponse.group", 568 "A sub-group within a group. The ordering of groups within this group is relevant.", 0, 569 java.lang.Integer.MAX_VALUE, group)); 570 childrenList.add(new Property("question", "", 571 "Set of questions within this group. The order of questions within the group is relevant.", 0, 572 java.lang.Integer.MAX_VALUE, question)); 573 } 574 575 @Override 576 public void setProperty(String name, Base value) throws FHIRException { 577 if (name.equals("linkId")) 578 this.linkId = castToString(value); // StringType 579 else if (name.equals("title")) 580 this.title = castToString(value); // StringType 581 else if (name.equals("text")) 582 this.text = castToString(value); // StringType 583 else if (name.equals("subject")) 584 this.subject = castToReference(value); // Reference 585 else if (name.equals("group")) 586 this.getGroup().add((GroupComponent) value); 587 else if (name.equals("question")) 588 this.getQuestion().add((QuestionComponent) value); 589 else 590 super.setProperty(name, value); 591 } 592 593 @Override 594 public Base addChild(String name) throws FHIRException { 595 if (name.equals("linkId")) { 596 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.linkId"); 597 } else if (name.equals("title")) { 598 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.title"); 599 } else if (name.equals("text")) { 600 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.text"); 601 } else if (name.equals("subject")) { 602 this.subject = new Reference(); 603 return this.subject; 604 } else if (name.equals("group")) { 605 return addGroup(); 606 } else if (name.equals("question")) { 607 return addQuestion(); 608 } else 609 return super.addChild(name); 610 } 611 612 public GroupComponent copy() { 613 GroupComponent dst = new GroupComponent(); 614 copyValues(dst); 615 dst.linkId = linkId == null ? null : linkId.copy(); 616 dst.title = title == null ? null : title.copy(); 617 dst.text = text == null ? null : text.copy(); 618 dst.subject = subject == null ? null : subject.copy(); 619 if (group != null) { 620 dst.group = new ArrayList<GroupComponent>(); 621 for (GroupComponent i : group) 622 dst.group.add(i.copy()); 623 } 624 ; 625 if (question != null) { 626 dst.question = new ArrayList<QuestionComponent>(); 627 for (QuestionComponent i : question) 628 dst.question.add(i.copy()); 629 } 630 ; 631 return dst; 632 } 633 634 @Override 635 public boolean equalsDeep(Base other) { 636 if (!super.equalsDeep(other)) 637 return false; 638 if (!(other instanceof GroupComponent)) 639 return false; 640 GroupComponent o = (GroupComponent) other; 641 return compareDeep(linkId, o.linkId, true) && compareDeep(title, o.title, true) && compareDeep(text, o.text, true) 642 && compareDeep(subject, o.subject, true) && compareDeep(group, o.group, true) 643 && compareDeep(question, o.question, true); 644 } 645 646 @Override 647 public boolean equalsShallow(Base other) { 648 if (!super.equalsShallow(other)) 649 return false; 650 if (!(other instanceof GroupComponent)) 651 return false; 652 GroupComponent o = (GroupComponent) other; 653 return compareValues(linkId, o.linkId, true) && compareValues(title, o.title, true) 654 && compareValues(text, o.text, true); 655 } 656 657 public boolean isEmpty() { 658 return super.isEmpty() && (linkId == null || linkId.isEmpty()) && (title == null || title.isEmpty()) 659 && (text == null || text.isEmpty()) && (subject == null || subject.isEmpty()) 660 && (group == null || group.isEmpty()) && (question == null || question.isEmpty()); 661 } 662 663 public String fhirType() { 664 return "QuestionnaireResponse.group"; 665 666 } 667 668 } 669 670 @Block() 671 public static class QuestionComponent extends BackboneElement implements IBaseBackboneElement { 672 /** 673 * Identifies the question from the Questionnaire that corresponds to this 674 * question in the QuestionnaireResponse resource. 675 */ 676 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 677 @Description(shortDefinition = "Corresponding question within Questionnaire", formalDefinition = "Identifies the question from the Questionnaire that corresponds to this question in the QuestionnaireResponse resource.") 678 protected StringType linkId; 679 680 /** 681 * The actual question as shown to the user to prompt them for an answer. 682 */ 683 @Child(name = "text", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 684 @Description(shortDefinition = "Text of the question as it is shown to the user", formalDefinition = "The actual question as shown to the user to prompt them for an answer.") 685 protected StringType text; 686 687 /** 688 * The respondent's answer(s) to the question. 689 */ 690 @Child(name = "answer", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 691 @Description(shortDefinition = "The response(s) to the question", formalDefinition = "The respondent's answer(s) to the question.") 692 protected List<QuestionAnswerComponent> answer; 693 694 private static final long serialVersionUID = -265263901L; 695 696 /* 697 * Constructor 698 */ 699 public QuestionComponent() { 700 super(); 701 } 702 703 /** 704 * @return {@link #linkId} (Identifies the question from the Questionnaire that 705 * corresponds to this question in the QuestionnaireResponse resource.). 706 * This is the underlying object with id, value and extensions. The 707 * accessor "getLinkId" gives direct access to the value 708 */ 709 public StringType getLinkIdElement() { 710 if (this.linkId == null) 711 if (Configuration.errorOnAutoCreate()) 712 throw new Error("Attempt to auto-create QuestionComponent.linkId"); 713 else if (Configuration.doAutoCreate()) 714 this.linkId = new StringType(); // bb 715 return this.linkId; 716 } 717 718 public boolean hasLinkIdElement() { 719 return this.linkId != null && !this.linkId.isEmpty(); 720 } 721 722 public boolean hasLinkId() { 723 return this.linkId != null && !this.linkId.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #linkId} (Identifies the question from the Questionnaire 728 * that corresponds to this question in the QuestionnaireResponse 729 * resource.). This is the underlying object with id, value and 730 * extensions. The accessor "getLinkId" gives direct access to the 731 * value 732 */ 733 public QuestionComponent setLinkIdElement(StringType value) { 734 this.linkId = value; 735 return this; 736 } 737 738 /** 739 * @return Identifies the question from the Questionnaire that corresponds to 740 * this question in the QuestionnaireResponse resource. 741 */ 742 public String getLinkId() { 743 return this.linkId == null ? null : this.linkId.getValue(); 744 } 745 746 /** 747 * @param value Identifies the question from the Questionnaire that corresponds 748 * to this question in the QuestionnaireResponse resource. 749 */ 750 public QuestionComponent setLinkId(String value) { 751 if (Utilities.noString(value)) 752 this.linkId = null; 753 else { 754 if (this.linkId == null) 755 this.linkId = new StringType(); 756 this.linkId.setValue(value); 757 } 758 return this; 759 } 760 761 /** 762 * @return {@link #text} (The actual question as shown to the user to prompt 763 * them for an answer.). This is the underlying object with id, value 764 * and extensions. The accessor "getText" gives direct access to the 765 * value 766 */ 767 public StringType getTextElement() { 768 if (this.text == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create QuestionComponent.text"); 771 else if (Configuration.doAutoCreate()) 772 this.text = new StringType(); // bb 773 return this.text; 774 } 775 776 public boolean hasTextElement() { 777 return this.text != null && !this.text.isEmpty(); 778 } 779 780 public boolean hasText() { 781 return this.text != null && !this.text.isEmpty(); 782 } 783 784 /** 785 * @param value {@link #text} (The actual question as shown to the user to 786 * prompt them for an answer.). This is the underlying object with 787 * id, value and extensions. The accessor "getText" gives direct 788 * access to the value 789 */ 790 public QuestionComponent setTextElement(StringType value) { 791 this.text = value; 792 return this; 793 } 794 795 /** 796 * @return The actual question as shown to the user to prompt them for an 797 * answer. 798 */ 799 public String getText() { 800 return this.text == null ? null : this.text.getValue(); 801 } 802 803 /** 804 * @param value The actual question as shown to the user to prompt them for an 805 * answer. 806 */ 807 public QuestionComponent setText(String value) { 808 if (Utilities.noString(value)) 809 this.text = null; 810 else { 811 if (this.text == null) 812 this.text = new StringType(); 813 this.text.setValue(value); 814 } 815 return this; 816 } 817 818 /** 819 * @return {@link #answer} (The respondent's answer(s) to the question.) 820 */ 821 public List<QuestionAnswerComponent> getAnswer() { 822 if (this.answer == null) 823 this.answer = new ArrayList<QuestionAnswerComponent>(); 824 return this.answer; 825 } 826 827 public boolean hasAnswer() { 828 if (this.answer == null) 829 return false; 830 for (QuestionAnswerComponent item : this.answer) 831 if (!item.isEmpty()) 832 return true; 833 return false; 834 } 835 836 /** 837 * @return {@link #answer} (The respondent's answer(s) to the question.) 838 */ 839 // syntactic sugar 840 public QuestionAnswerComponent addAnswer() { // 3 841 QuestionAnswerComponent t = new QuestionAnswerComponent(); 842 if (this.answer == null) 843 this.answer = new ArrayList<QuestionAnswerComponent>(); 844 this.answer.add(t); 845 return t; 846 } 847 848 // syntactic sugar 849 public QuestionComponent addAnswer(QuestionAnswerComponent t) { // 3 850 if (t == null) 851 return this; 852 if (this.answer == null) 853 this.answer = new ArrayList<QuestionAnswerComponent>(); 854 this.answer.add(t); 855 return this; 856 } 857 858 protected void listChildren(List<Property> childrenList) { 859 super.listChildren(childrenList); 860 childrenList.add(new Property("linkId", "string", 861 "Identifies the question from the Questionnaire that corresponds to this question in the QuestionnaireResponse resource.", 862 0, java.lang.Integer.MAX_VALUE, linkId)); 863 childrenList 864 .add(new Property("text", "string", "The actual question as shown to the user to prompt them for an answer.", 865 0, java.lang.Integer.MAX_VALUE, text)); 866 childrenList.add(new Property("answer", "", "The respondent's answer(s) to the question.", 0, 867 java.lang.Integer.MAX_VALUE, answer)); 868 } 869 870 @Override 871 public void setProperty(String name, Base value) throws FHIRException { 872 if (name.equals("linkId")) 873 this.linkId = castToString(value); // StringType 874 else if (name.equals("text")) 875 this.text = castToString(value); // StringType 876 else if (name.equals("answer")) 877 this.getAnswer().add((QuestionAnswerComponent) value); 878 else 879 super.setProperty(name, value); 880 } 881 882 @Override 883 public Base addChild(String name) throws FHIRException { 884 if (name.equals("linkId")) { 885 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.linkId"); 886 } else if (name.equals("text")) { 887 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.text"); 888 } else if (name.equals("answer")) { 889 return addAnswer(); 890 } else 891 return super.addChild(name); 892 } 893 894 public QuestionComponent copy() { 895 QuestionComponent dst = new QuestionComponent(); 896 copyValues(dst); 897 dst.linkId = linkId == null ? null : linkId.copy(); 898 dst.text = text == null ? null : text.copy(); 899 if (answer != null) { 900 dst.answer = new ArrayList<QuestionAnswerComponent>(); 901 for (QuestionAnswerComponent i : answer) 902 dst.answer.add(i.copy()); 903 } 904 ; 905 return dst; 906 } 907 908 @Override 909 public boolean equalsDeep(Base other) { 910 if (!super.equalsDeep(other)) 911 return false; 912 if (!(other instanceof QuestionComponent)) 913 return false; 914 QuestionComponent o = (QuestionComponent) other; 915 return compareDeep(linkId, o.linkId, true) && compareDeep(text, o.text, true) 916 && compareDeep(answer, o.answer, true); 917 } 918 919 @Override 920 public boolean equalsShallow(Base other) { 921 if (!super.equalsShallow(other)) 922 return false; 923 if (!(other instanceof QuestionComponent)) 924 return false; 925 QuestionComponent o = (QuestionComponent) other; 926 return compareValues(linkId, o.linkId, true) && compareValues(text, o.text, true); 927 } 928 929 public boolean isEmpty() { 930 return super.isEmpty() && (linkId == null || linkId.isEmpty()) && (text == null || text.isEmpty()) 931 && (answer == null || answer.isEmpty()); 932 } 933 934 public String fhirType() { 935 return "QuestionnaireResponse.group.question"; 936 937 } 938 939 } 940 941 @Block() 942 public static class QuestionAnswerComponent extends BackboneElement implements IBaseBackboneElement { 943 /** 944 * The answer (or one of the answers) provided by the respondent to the 945 * question. 946 */ 947 @Child(name = "value", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, 948 DateTimeType.class, InstantType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, 949 Coding.class, Quantity.class, Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 950 @Description(shortDefinition = "Single-valued answer to the question", formalDefinition = "The answer (or one of the answers) provided by the respondent to the question.") 951 protected Type value; 952 953 /** 954 * Nested group, containing nested question for this question. The order of 955 * groups within the question is relevant. 956 */ 957 @Child(name = "group", type = { 958 GroupComponent.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 959 @Description(shortDefinition = "Nested questionnaire group", formalDefinition = "Nested group, containing nested question for this question. The order of groups within the question is relevant.") 960 protected List<GroupComponent> group; 961 962 private static final long serialVersionUID = -1223680118L; 963 964 /* 965 * Constructor 966 */ 967 public QuestionAnswerComponent() { 968 super(); 969 } 970 971 /** 972 * @return {@link #value} (The answer (or one of the answers) provided by the 973 * respondent to the question.) 974 */ 975 public Type getValue() { 976 return this.value; 977 } 978 979 /** 980 * @return {@link #value} (The answer (or one of the answers) provided by the 981 * respondent to the question.) 982 */ 983 public BooleanType getValueBooleanType() throws FHIRException { 984 if (!(this.value instanceof BooleanType)) 985 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 986 + this.value.getClass().getName() + " was encountered"); 987 return (BooleanType) this.value; 988 } 989 990 public boolean hasValueBooleanType() { 991 return this.value instanceof BooleanType; 992 } 993 994 /** 995 * @return {@link #value} (The answer (or one of the answers) provided by the 996 * respondent to the question.) 997 */ 998 public DecimalType getValueDecimalType() throws FHIRException { 999 if (!(this.value instanceof DecimalType)) 1000 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 1001 + this.value.getClass().getName() + " was encountered"); 1002 return (DecimalType) this.value; 1003 } 1004 1005 public boolean hasValueDecimalType() { 1006 return this.value instanceof DecimalType; 1007 } 1008 1009 /** 1010 * @return {@link #value} (The answer (or one of the answers) provided by the 1011 * respondent to the question.) 1012 */ 1013 public IntegerType getValueIntegerType() throws FHIRException { 1014 if (!(this.value instanceof IntegerType)) 1015 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 1016 + this.value.getClass().getName() + " was encountered"); 1017 return (IntegerType) this.value; 1018 } 1019 1020 public boolean hasValueIntegerType() { 1021 return this.value instanceof IntegerType; 1022 } 1023 1024 /** 1025 * @return {@link #value} (The answer (or one of the answers) provided by the 1026 * respondent to the question.) 1027 */ 1028 public DateType getValueDateType() throws FHIRException { 1029 if (!(this.value instanceof DateType)) 1030 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName() 1031 + " was encountered"); 1032 return (DateType) this.value; 1033 } 1034 1035 public boolean hasValueDateType() { 1036 return this.value instanceof DateType; 1037 } 1038 1039 /** 1040 * @return {@link #value} (The answer (or one of the answers) provided by the 1041 * respondent to the question.) 1042 */ 1043 public DateTimeType getValueDateTimeType() throws FHIRException { 1044 if (!(this.value instanceof DateTimeType)) 1045 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1046 + this.value.getClass().getName() + " was encountered"); 1047 return (DateTimeType) this.value; 1048 } 1049 1050 public boolean hasValueDateTimeType() { 1051 return this.value instanceof DateTimeType; 1052 } 1053 1054 /** 1055 * @return {@link #value} (The answer (or one of the answers) provided by the 1056 * respondent to the question.) 1057 */ 1058 public InstantType getValueInstantType() throws FHIRException { 1059 if (!(this.value instanceof InstantType)) 1060 throw new FHIRException("Type mismatch: the type InstantType was expected, but " 1061 + this.value.getClass().getName() + " was encountered"); 1062 return (InstantType) this.value; 1063 } 1064 1065 public boolean hasValueInstantType() { 1066 return this.value instanceof InstantType; 1067 } 1068 1069 /** 1070 * @return {@link #value} (The answer (or one of the answers) provided by the 1071 * respondent to the question.) 1072 */ 1073 public TimeType getValueTimeType() throws FHIRException { 1074 if (!(this.value instanceof TimeType)) 1075 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 1076 + " was encountered"); 1077 return (TimeType) this.value; 1078 } 1079 1080 public boolean hasValueTimeType() { 1081 return this.value instanceof TimeType; 1082 } 1083 1084 /** 1085 * @return {@link #value} (The answer (or one of the answers) provided by the 1086 * respondent to the question.) 1087 */ 1088 public StringType getValueStringType() throws FHIRException { 1089 if (!(this.value instanceof StringType)) 1090 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1091 + this.value.getClass().getName() + " was encountered"); 1092 return (StringType) this.value; 1093 } 1094 1095 public boolean hasValueStringType() { 1096 return this.value instanceof StringType; 1097 } 1098 1099 /** 1100 * @return {@link #value} (The answer (or one of the answers) provided by the 1101 * respondent to the question.) 1102 */ 1103 public UriType getValueUriType() throws FHIRException { 1104 if (!(this.value instanceof UriType)) 1105 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 1106 + " was encountered"); 1107 return (UriType) this.value; 1108 } 1109 1110 public boolean hasValueUriType() { 1111 return this.value instanceof UriType; 1112 } 1113 1114 /** 1115 * @return {@link #value} (The answer (or one of the answers) provided by the 1116 * respondent to the question.) 1117 */ 1118 public Attachment getValueAttachment() throws FHIRException { 1119 if (!(this.value instanceof Attachment)) 1120 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 1121 + this.value.getClass().getName() + " was encountered"); 1122 return (Attachment) this.value; 1123 } 1124 1125 public boolean hasValueAttachment() { 1126 return this.value instanceof Attachment; 1127 } 1128 1129 /** 1130 * @return {@link #value} (The answer (or one of the answers) provided by the 1131 * respondent to the question.) 1132 */ 1133 public Coding getValueCoding() throws FHIRException { 1134 if (!(this.value instanceof Coding)) 1135 throw new FHIRException( 1136 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 1137 return (Coding) this.value; 1138 } 1139 1140 public boolean hasValueCoding() { 1141 return this.value instanceof Coding; 1142 } 1143 1144 /** 1145 * @return {@link #value} (The answer (or one of the answers) provided by the 1146 * respondent to the question.) 1147 */ 1148 public Quantity getValueQuantity() throws FHIRException { 1149 if (!(this.value instanceof Quantity)) 1150 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 1151 + " was encountered"); 1152 return (Quantity) this.value; 1153 } 1154 1155 public boolean hasValueQuantity() { 1156 return this.value instanceof Quantity; 1157 } 1158 1159 /** 1160 * @return {@link #value} (The answer (or one of the answers) provided by the 1161 * respondent to the question.) 1162 */ 1163 public Reference getValueReference() throws FHIRException { 1164 if (!(this.value instanceof Reference)) 1165 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 1166 + " was encountered"); 1167 return (Reference) this.value; 1168 } 1169 1170 public boolean hasValueReference() { 1171 return this.value instanceof Reference; 1172 } 1173 1174 public boolean hasValue() { 1175 return this.value != null && !this.value.isEmpty(); 1176 } 1177 1178 /** 1179 * @param value {@link #value} (The answer (or one of the answers) provided by 1180 * the respondent to the question.) 1181 */ 1182 public QuestionAnswerComponent setValue(Type value) { 1183 this.value = value; 1184 return this; 1185 } 1186 1187 /** 1188 * @return {@link #group} (Nested group, containing nested question for this 1189 * question. The order of groups within the question is relevant.) 1190 */ 1191 public List<GroupComponent> getGroup() { 1192 if (this.group == null) 1193 this.group = new ArrayList<GroupComponent>(); 1194 return this.group; 1195 } 1196 1197 public boolean hasGroup() { 1198 if (this.group == null) 1199 return false; 1200 for (GroupComponent item : this.group) 1201 if (!item.isEmpty()) 1202 return true; 1203 return false; 1204 } 1205 1206 /** 1207 * @return {@link #group} (Nested group, containing nested question for this 1208 * question. The order of groups within the question is relevant.) 1209 */ 1210 // syntactic sugar 1211 public GroupComponent addGroup() { // 3 1212 GroupComponent t = new GroupComponent(); 1213 if (this.group == null) 1214 this.group = new ArrayList<GroupComponent>(); 1215 this.group.add(t); 1216 return t; 1217 } 1218 1219 // syntactic sugar 1220 public QuestionAnswerComponent addGroup(GroupComponent t) { // 3 1221 if (t == null) 1222 return this; 1223 if (this.group == null) 1224 this.group = new ArrayList<GroupComponent>(); 1225 this.group.add(t); 1226 return this; 1227 } 1228 1229 protected void listChildren(List<Property> childrenList) { 1230 super.listChildren(childrenList); 1231 childrenList.add(new Property("value[x]", 1232 "boolean|decimal|integer|date|dateTime|instant|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1233 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1234 java.lang.Integer.MAX_VALUE, value)); 1235 childrenList.add(new Property("group", "@QuestionnaireResponse.group", 1236 "Nested group, containing nested question for this question. The order of groups within the question is relevant.", 1237 0, java.lang.Integer.MAX_VALUE, group)); 1238 } 1239 1240 @Override 1241 public void setProperty(String name, Base value) throws FHIRException { 1242 if (name.equals("value[x]")) 1243 this.value = (Type) value; // Type 1244 else if (name.equals("group")) 1245 this.getGroup().add((GroupComponent) value); 1246 else 1247 super.setProperty(name, value); 1248 } 1249 1250 @Override 1251 public Base addChild(String name) throws FHIRException { 1252 if (name.equals("valueBoolean")) { 1253 this.value = new BooleanType(); 1254 return this.value; 1255 } else if (name.equals("valueDecimal")) { 1256 this.value = new DecimalType(); 1257 return this.value; 1258 } else if (name.equals("valueInteger")) { 1259 this.value = new IntegerType(); 1260 return this.value; 1261 } else if (name.equals("valueDate")) { 1262 this.value = new DateType(); 1263 return this.value; 1264 } else if (name.equals("valueDateTime")) { 1265 this.value = new DateTimeType(); 1266 return this.value; 1267 } else if (name.equals("valueInstant")) { 1268 this.value = new InstantType(); 1269 return this.value; 1270 } else if (name.equals("valueTime")) { 1271 this.value = new TimeType(); 1272 return this.value; 1273 } else if (name.equals("valueString")) { 1274 this.value = new StringType(); 1275 return this.value; 1276 } else if (name.equals("valueUri")) { 1277 this.value = new UriType(); 1278 return this.value; 1279 } else if (name.equals("valueAttachment")) { 1280 this.value = new Attachment(); 1281 return this.value; 1282 } else if (name.equals("valueCoding")) { 1283 this.value = new Coding(); 1284 return this.value; 1285 } else if (name.equals("valueQuantity")) { 1286 this.value = new Quantity(); 1287 return this.value; 1288 } else if (name.equals("valueReference")) { 1289 this.value = new Reference(); 1290 return this.value; 1291 } else if (name.equals("group")) { 1292 return addGroup(); 1293 } else 1294 return super.addChild(name); 1295 } 1296 1297 public QuestionAnswerComponent copy() { 1298 QuestionAnswerComponent dst = new QuestionAnswerComponent(); 1299 copyValues(dst); 1300 dst.value = value == null ? null : value.copy(); 1301 if (group != null) { 1302 dst.group = new ArrayList<GroupComponent>(); 1303 for (GroupComponent i : group) 1304 dst.group.add(i.copy()); 1305 } 1306 ; 1307 return dst; 1308 } 1309 1310 @Override 1311 public boolean equalsDeep(Base other) { 1312 if (!super.equalsDeep(other)) 1313 return false; 1314 if (!(other instanceof QuestionAnswerComponent)) 1315 return false; 1316 QuestionAnswerComponent o = (QuestionAnswerComponent) other; 1317 return compareDeep(value, o.value, true) && compareDeep(group, o.group, true); 1318 } 1319 1320 @Override 1321 public boolean equalsShallow(Base other) { 1322 if (!super.equalsShallow(other)) 1323 return false; 1324 if (!(other instanceof QuestionAnswerComponent)) 1325 return false; 1326 QuestionAnswerComponent o = (QuestionAnswerComponent) other; 1327 return true; 1328 } 1329 1330 public boolean isEmpty() { 1331 return super.isEmpty() && (value == null || value.isEmpty()) && (group == null || group.isEmpty()); 1332 } 1333 1334 public String fhirType() { 1335 return "QuestionnaireResponse.group.question.answer"; 1336 1337 } 1338 1339 } 1340 1341 /** 1342 * A business identifier assigned to a particular completed (or partially 1343 * completed) questionnaire. 1344 */ 1345 @Child(name = "identifier", type = { 1346 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1347 @Description(shortDefinition = "Unique id for this set of answers", formalDefinition = "A business identifier assigned to a particular completed (or partially completed) questionnaire.") 1348 protected Identifier identifier; 1349 1350 /** 1351 * Indicates the Questionnaire resource that defines the form for which answers 1352 * are being provided. 1353 */ 1354 @Child(name = "questionnaire", type = { 1355 Questionnaire.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1356 @Description(shortDefinition = "Form being answered", formalDefinition = "Indicates the Questionnaire resource that defines the form for which answers are being provided.") 1357 protected Reference questionnaire; 1358 1359 /** 1360 * The actual object that is the target of the reference (Indicates the 1361 * Questionnaire resource that defines the form for which answers are being 1362 * provided.) 1363 */ 1364 protected Questionnaire questionnaireTarget; 1365 1366 /** 1367 * The lifecycle status of the questionnaire response as a whole. 1368 */ 1369 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 1370 @Description(shortDefinition = "in-progress | completed | amended", formalDefinition = "The lifecycle status of the questionnaire response as a whole.") 1371 protected Enumeration<QuestionnaireResponseStatus> status; 1372 1373 /** 1374 * The subject of the questionnaire response. This could be a patient, 1375 * organization, practitioner, device, etc. This is who/what the answers apply 1376 * to, but is not necessarily the source of information. 1377 */ 1378 @Child(name = "subject", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = true) 1379 @Description(shortDefinition = "The subject of the questions", formalDefinition = "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.") 1380 protected Reference subject; 1381 1382 /** 1383 * The actual object that is the target of the reference (The subject of the 1384 * questionnaire response. This could be a patient, organization, practitioner, 1385 * device, etc. This is who/what the answers apply to, but is not necessarily 1386 * the source of information.) 1387 */ 1388 protected Resource subjectTarget; 1389 1390 /** 1391 * Person who received the answers to the questions in the QuestionnaireResponse 1392 * and recorded them in the system. 1393 */ 1394 @Child(name = "author", type = { Device.class, Practitioner.class, Patient.class, 1395 RelatedPerson.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1396 @Description(shortDefinition = "Person who received and recorded the answers", formalDefinition = "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.") 1397 protected Reference author; 1398 1399 /** 1400 * The actual object that is the target of the reference (Person who received 1401 * the answers to the questions in the QuestionnaireResponse and recorded them 1402 * in the system.) 1403 */ 1404 protected Resource authorTarget; 1405 1406 /** 1407 * The date and/or time that this version of the questionnaire response was 1408 * authored. 1409 */ 1410 @Child(name = "authored", type = { 1411 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1412 @Description(shortDefinition = "Date this version was authored", formalDefinition = "The date and/or time that this version of the questionnaire response was authored.") 1413 protected DateTimeType authored; 1414 1415 /** 1416 * The person who answered the questions about the subject. 1417 */ 1418 @Child(name = "source", type = { Patient.class, Practitioner.class, 1419 RelatedPerson.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1420 @Description(shortDefinition = "The person who answered the questions", formalDefinition = "The person who answered the questions about the subject.") 1421 protected Reference source; 1422 1423 /** 1424 * The actual object that is the target of the reference (The person who 1425 * answered the questions about the subject.) 1426 */ 1427 protected Resource sourceTarget; 1428 1429 /** 1430 * Encounter during which this set of questionnaire response were collected. 1431 * When there were multiple encounters, this is the one considered most relevant 1432 * to the context of the answers. 1433 */ 1434 @Child(name = "encounter", type = { Encounter.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1435 @Description(shortDefinition = "Primary encounter during which the answers were collected", formalDefinition = "Encounter during which this set of questionnaire response were collected. When there were multiple encounters, this is the one considered most relevant to the context of the answers.") 1436 protected Reference encounter; 1437 1438 /** 1439 * The actual object that is the target of the reference (Encounter during which 1440 * this set of questionnaire response were collected. When there were multiple 1441 * encounters, this is the one considered most relevant to the context of the 1442 * answers.) 1443 */ 1444 protected Encounter encounterTarget; 1445 1446 /** 1447 * A group of questions to a possibly similarly grouped set of questions in the 1448 * questionnaire response. 1449 */ 1450 @Child(name = "group", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = false) 1451 @Description(shortDefinition = "Grouped questions", formalDefinition = "A group of questions to a possibly similarly grouped set of questions in the questionnaire response.") 1452 protected GroupComponent group; 1453 1454 private static final long serialVersionUID = -1081988635L; 1455 1456 /* 1457 * Constructor 1458 */ 1459 public QuestionnaireResponse() { 1460 super(); 1461 } 1462 1463 /* 1464 * Constructor 1465 */ 1466 public QuestionnaireResponse(Enumeration<QuestionnaireResponseStatus> status) { 1467 super(); 1468 this.status = status; 1469 } 1470 1471 /** 1472 * @return {@link #identifier} (A business identifier assigned to a particular 1473 * completed (or partially completed) questionnaire.) 1474 */ 1475 public Identifier getIdentifier() { 1476 if (this.identifier == null) 1477 if (Configuration.errorOnAutoCreate()) 1478 throw new Error("Attempt to auto-create QuestionnaireResponse.identifier"); 1479 else if (Configuration.doAutoCreate()) 1480 this.identifier = new Identifier(); // cc 1481 return this.identifier; 1482 } 1483 1484 public boolean hasIdentifier() { 1485 return this.identifier != null && !this.identifier.isEmpty(); 1486 } 1487 1488 /** 1489 * @param value {@link #identifier} (A business identifier assigned to a 1490 * particular completed (or partially completed) questionnaire.) 1491 */ 1492 public QuestionnaireResponse setIdentifier(Identifier value) { 1493 this.identifier = value; 1494 return this; 1495 } 1496 1497 /** 1498 * @return {@link #questionnaire} (Indicates the Questionnaire resource that 1499 * defines the form for which answers are being provided.) 1500 */ 1501 public Reference getQuestionnaire() { 1502 if (this.questionnaire == null) 1503 if (Configuration.errorOnAutoCreate()) 1504 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1505 else if (Configuration.doAutoCreate()) 1506 this.questionnaire = new Reference(); // cc 1507 return this.questionnaire; 1508 } 1509 1510 public boolean hasQuestionnaire() { 1511 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1512 } 1513 1514 /** 1515 * @param value {@link #questionnaire} (Indicates the Questionnaire resource 1516 * that defines the form for which answers are being provided.) 1517 */ 1518 public QuestionnaireResponse setQuestionnaire(Reference value) { 1519 this.questionnaire = value; 1520 return this; 1521 } 1522 1523 /** 1524 * @return {@link #questionnaire} The actual object that is the target of the 1525 * reference. The reference library doesn't populate this, but you can 1526 * use it to hold the resource if you resolve it. (Indicates the 1527 * Questionnaire resource that defines the form for which answers are 1528 * being provided.) 1529 */ 1530 public Questionnaire getQuestionnaireTarget() { 1531 if (this.questionnaireTarget == null) 1532 if (Configuration.errorOnAutoCreate()) 1533 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1534 else if (Configuration.doAutoCreate()) 1535 this.questionnaireTarget = new Questionnaire(); // aa 1536 return this.questionnaireTarget; 1537 } 1538 1539 /** 1540 * @param value {@link #questionnaire} The actual object that is the target of 1541 * the reference. The reference library doesn't use these, but you 1542 * can use it to hold the resource if you resolve it. (Indicates 1543 * the Questionnaire resource that defines the form for which 1544 * answers are being provided.) 1545 */ 1546 public QuestionnaireResponse setQuestionnaireTarget(Questionnaire value) { 1547 this.questionnaireTarget = value; 1548 return this; 1549 } 1550 1551 /** 1552 * @return {@link #status} (The lifecycle status of the questionnaire response 1553 * as a whole.). This is the underlying object with id, value and 1554 * extensions. The accessor "getStatus" gives direct access to the value 1555 */ 1556 public Enumeration<QuestionnaireResponseStatus> getStatusElement() { 1557 if (this.status == null) 1558 if (Configuration.errorOnAutoCreate()) 1559 throw new Error("Attempt to auto-create QuestionnaireResponse.status"); 1560 else if (Configuration.doAutoCreate()) 1561 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); // bb 1562 return this.status; 1563 } 1564 1565 public boolean hasStatusElement() { 1566 return this.status != null && !this.status.isEmpty(); 1567 } 1568 1569 public boolean hasStatus() { 1570 return this.status != null && !this.status.isEmpty(); 1571 } 1572 1573 /** 1574 * @param value {@link #status} (The lifecycle status of the questionnaire 1575 * response as a whole.). This is the underlying object with id, 1576 * value and extensions. The accessor "getStatus" gives direct 1577 * access to the value 1578 */ 1579 public QuestionnaireResponse setStatusElement(Enumeration<QuestionnaireResponseStatus> value) { 1580 this.status = value; 1581 return this; 1582 } 1583 1584 /** 1585 * @return The lifecycle status of the questionnaire response as a whole. 1586 */ 1587 public QuestionnaireResponseStatus getStatus() { 1588 return this.status == null ? null : this.status.getValue(); 1589 } 1590 1591 /** 1592 * @param value The lifecycle status of the questionnaire response as a whole. 1593 */ 1594 public QuestionnaireResponse setStatus(QuestionnaireResponseStatus value) { 1595 if (this.status == null) 1596 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); 1597 this.status.setValue(value); 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #subject} (The subject of the questionnaire response. This 1603 * could be a patient, organization, practitioner, device, etc. This is 1604 * who/what the answers apply to, but is not necessarily the source of 1605 * information.) 1606 */ 1607 public Reference getSubject() { 1608 if (this.subject == null) 1609 if (Configuration.errorOnAutoCreate()) 1610 throw new Error("Attempt to auto-create QuestionnaireResponse.subject"); 1611 else if (Configuration.doAutoCreate()) 1612 this.subject = new Reference(); // cc 1613 return this.subject; 1614 } 1615 1616 public boolean hasSubject() { 1617 return this.subject != null && !this.subject.isEmpty(); 1618 } 1619 1620 /** 1621 * @param value {@link #subject} (The subject of the questionnaire response. 1622 * This could be a patient, organization, practitioner, device, 1623 * etc. This is who/what the answers apply to, but is not 1624 * necessarily the source of information.) 1625 */ 1626 public QuestionnaireResponse setSubject(Reference value) { 1627 this.subject = value; 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #subject} The actual object that is the target of the 1633 * reference. The reference library doesn't populate this, but you can 1634 * use it to hold the resource if you resolve it. (The subject of the 1635 * questionnaire response. This could be a patient, organization, 1636 * practitioner, device, etc. This is who/what the answers apply to, but 1637 * is not necessarily the source of information.) 1638 */ 1639 public Resource getSubjectTarget() { 1640 return this.subjectTarget; 1641 } 1642 1643 /** 1644 * @param value {@link #subject} The actual object that is the target of the 1645 * reference. The reference library doesn't use these, but you can 1646 * use it to hold the resource if you resolve it. (The subject of 1647 * the questionnaire response. This could be a patient, 1648 * organization, practitioner, device, etc. This is who/what the 1649 * answers apply to, but is not necessarily the source of 1650 * information.) 1651 */ 1652 public QuestionnaireResponse setSubjectTarget(Resource value) { 1653 this.subjectTarget = value; 1654 return this; 1655 } 1656 1657 /** 1658 * @return {@link #author} (Person who received the answers to the questions in 1659 * the QuestionnaireResponse and recorded them in the system.) 1660 */ 1661 public Reference getAuthor() { 1662 if (this.author == null) 1663 if (Configuration.errorOnAutoCreate()) 1664 throw new Error("Attempt to auto-create QuestionnaireResponse.author"); 1665 else if (Configuration.doAutoCreate()) 1666 this.author = new Reference(); // cc 1667 return this.author; 1668 } 1669 1670 public boolean hasAuthor() { 1671 return this.author != null && !this.author.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #author} (Person who received the answers to the 1676 * questions in the QuestionnaireResponse and recorded them in the 1677 * system.) 1678 */ 1679 public QuestionnaireResponse setAuthor(Reference value) { 1680 this.author = value; 1681 return this; 1682 } 1683 1684 /** 1685 * @return {@link #author} The actual object that is the target of the 1686 * reference. The reference library doesn't populate this, but you can 1687 * use it to hold the resource if you resolve it. (Person who received 1688 * the answers to the questions in the QuestionnaireResponse and 1689 * recorded them in the system.) 1690 */ 1691 public Resource getAuthorTarget() { 1692 return this.authorTarget; 1693 } 1694 1695 /** 1696 * @param value {@link #author} The actual object that is the target of the 1697 * reference. The reference library doesn't use these, but you can 1698 * use it to hold the resource if you resolve it. (Person who 1699 * received the answers to the questions in the 1700 * QuestionnaireResponse and recorded them in the system.) 1701 */ 1702 public QuestionnaireResponse setAuthorTarget(Resource value) { 1703 this.authorTarget = value; 1704 return this; 1705 } 1706 1707 /** 1708 * @return {@link #authored} (The date and/or time that this version of the 1709 * questionnaire response was authored.). This is the underlying object 1710 * with id, value and extensions. The accessor "getAuthored" gives 1711 * direct access to the value 1712 */ 1713 public DateTimeType getAuthoredElement() { 1714 if (this.authored == null) 1715 if (Configuration.errorOnAutoCreate()) 1716 throw new Error("Attempt to auto-create QuestionnaireResponse.authored"); 1717 else if (Configuration.doAutoCreate()) 1718 this.authored = new DateTimeType(); // bb 1719 return this.authored; 1720 } 1721 1722 public boolean hasAuthoredElement() { 1723 return this.authored != null && !this.authored.isEmpty(); 1724 } 1725 1726 public boolean hasAuthored() { 1727 return this.authored != null && !this.authored.isEmpty(); 1728 } 1729 1730 /** 1731 * @param value {@link #authored} (The date and/or time that this version of the 1732 * questionnaire response was authored.). This is the underlying 1733 * object with id, value and extensions. The accessor "getAuthored" 1734 * gives direct access to the value 1735 */ 1736 public QuestionnaireResponse setAuthoredElement(DateTimeType value) { 1737 this.authored = value; 1738 return this; 1739 } 1740 1741 /** 1742 * @return The date and/or time that this version of the questionnaire response 1743 * was authored. 1744 */ 1745 public Date getAuthored() { 1746 return this.authored == null ? null : this.authored.getValue(); 1747 } 1748 1749 /** 1750 * @param value The date and/or time that this version of the questionnaire 1751 * response was authored. 1752 */ 1753 public QuestionnaireResponse setAuthored(Date value) { 1754 if (value == null) 1755 this.authored = null; 1756 else { 1757 if (this.authored == null) 1758 this.authored = new DateTimeType(); 1759 this.authored.setValue(value); 1760 } 1761 return this; 1762 } 1763 1764 /** 1765 * @return {@link #source} (The person who answered the questions about the 1766 * subject.) 1767 */ 1768 public Reference getSource() { 1769 if (this.source == null) 1770 if (Configuration.errorOnAutoCreate()) 1771 throw new Error("Attempt to auto-create QuestionnaireResponse.source"); 1772 else if (Configuration.doAutoCreate()) 1773 this.source = new Reference(); // cc 1774 return this.source; 1775 } 1776 1777 public boolean hasSource() { 1778 return this.source != null && !this.source.isEmpty(); 1779 } 1780 1781 /** 1782 * @param value {@link #source} (The person who answered the questions about the 1783 * subject.) 1784 */ 1785 public QuestionnaireResponse setSource(Reference value) { 1786 this.source = value; 1787 return this; 1788 } 1789 1790 /** 1791 * @return {@link #source} The actual object that is the target of the 1792 * reference. The reference library doesn't populate this, but you can 1793 * use it to hold the resource if you resolve it. (The person who 1794 * answered the questions about the subject.) 1795 */ 1796 public Resource getSourceTarget() { 1797 return this.sourceTarget; 1798 } 1799 1800 /** 1801 * @param value {@link #source} The actual object that is the target of the 1802 * reference. The reference library doesn't use these, but you can 1803 * use it to hold the resource if you resolve it. (The person who 1804 * answered the questions about the subject.) 1805 */ 1806 public QuestionnaireResponse setSourceTarget(Resource value) { 1807 this.sourceTarget = value; 1808 return this; 1809 } 1810 1811 /** 1812 * @return {@link #encounter} (Encounter during which this set of questionnaire 1813 * response were collected. When there were multiple encounters, this is 1814 * the one considered most relevant to the context of the answers.) 1815 */ 1816 public Reference getEncounter() { 1817 if (this.encounter == null) 1818 if (Configuration.errorOnAutoCreate()) 1819 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1820 else if (Configuration.doAutoCreate()) 1821 this.encounter = new Reference(); // cc 1822 return this.encounter; 1823 } 1824 1825 public boolean hasEncounter() { 1826 return this.encounter != null && !this.encounter.isEmpty(); 1827 } 1828 1829 /** 1830 * @param value {@link #encounter} (Encounter during which this set of 1831 * questionnaire response were collected. When there were multiple 1832 * encounters, this is the one considered most relevant to the 1833 * context of the answers.) 1834 */ 1835 public QuestionnaireResponse setEncounter(Reference value) { 1836 this.encounter = value; 1837 return this; 1838 } 1839 1840 /** 1841 * @return {@link #encounter} The actual object that is the target of the 1842 * reference. The reference library doesn't populate this, but you can 1843 * use it to hold the resource if you resolve it. (Encounter during 1844 * which this set of questionnaire response were collected. When there 1845 * were multiple encounters, this is the one considered most relevant to 1846 * the context of the answers.) 1847 */ 1848 public Encounter getEncounterTarget() { 1849 if (this.encounterTarget == null) 1850 if (Configuration.errorOnAutoCreate()) 1851 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1852 else if (Configuration.doAutoCreate()) 1853 this.encounterTarget = new Encounter(); // aa 1854 return this.encounterTarget; 1855 } 1856 1857 /** 1858 * @param value {@link #encounter} The actual object that is the target of the 1859 * reference. The reference library doesn't use these, but you can 1860 * use it to hold the resource if you resolve it. (Encounter during 1861 * which this set of questionnaire response were collected. When 1862 * there were multiple encounters, this is the one considered most 1863 * relevant to the context of the answers.) 1864 */ 1865 public QuestionnaireResponse setEncounterTarget(Encounter value) { 1866 this.encounterTarget = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return {@link #group} (A group of questions to a possibly similarly grouped 1872 * set of questions in the questionnaire response.) 1873 */ 1874 public GroupComponent getGroup() { 1875 if (this.group == null) 1876 if (Configuration.errorOnAutoCreate()) 1877 throw new Error("Attempt to auto-create QuestionnaireResponse.group"); 1878 else if (Configuration.doAutoCreate()) 1879 this.group = new GroupComponent(); // cc 1880 return this.group; 1881 } 1882 1883 public boolean hasGroup() { 1884 return this.group != null && !this.group.isEmpty(); 1885 } 1886 1887 /** 1888 * @param value {@link #group} (A group of questions to a possibly similarly 1889 * grouped set of questions in the questionnaire response.) 1890 */ 1891 public QuestionnaireResponse setGroup(GroupComponent value) { 1892 this.group = value; 1893 return this; 1894 } 1895 1896 protected void listChildren(List<Property> childrenList) { 1897 super.listChildren(childrenList); 1898 childrenList.add(new Property("identifier", "Identifier", 1899 "A business identifier assigned to a particular completed (or partially completed) questionnaire.", 0, 1900 java.lang.Integer.MAX_VALUE, identifier)); 1901 childrenList.add(new Property("questionnaire", "Reference(Questionnaire)", 1902 "Indicates the Questionnaire resource that defines the form for which answers are being provided.", 0, 1903 java.lang.Integer.MAX_VALUE, questionnaire)); 1904 childrenList.add(new Property("status", "code", "The lifecycle status of the questionnaire response as a whole.", 0, 1905 java.lang.Integer.MAX_VALUE, status)); 1906 childrenList.add(new Property("subject", "Reference(Any)", 1907 "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 1908 0, java.lang.Integer.MAX_VALUE, subject)); 1909 childrenList.add(new Property("author", "Reference(Device|Practitioner|Patient|RelatedPerson)", 1910 "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 1911 0, java.lang.Integer.MAX_VALUE, author)); 1912 childrenList.add(new Property("authored", "dateTime", 1913 "The date and/or time that this version of the questionnaire response was authored.", 0, 1914 java.lang.Integer.MAX_VALUE, authored)); 1915 childrenList.add(new Property("source", "Reference(Patient|Practitioner|RelatedPerson)", 1916 "The person who answered the questions about the subject.", 0, java.lang.Integer.MAX_VALUE, source)); 1917 childrenList.add(new Property("encounter", "Reference(Encounter)", 1918 "Encounter during which this set of questionnaire response were collected. When there were multiple encounters, this is the one considered most relevant to the context of the answers.", 1919 0, java.lang.Integer.MAX_VALUE, encounter)); 1920 childrenList.add(new Property("group", "", 1921 "A group of questions to a possibly similarly grouped set of questions in the questionnaire response.", 0, 1922 java.lang.Integer.MAX_VALUE, group)); 1923 } 1924 1925 @Override 1926 public void setProperty(String name, Base value) throws FHIRException { 1927 if (name.equals("identifier")) 1928 this.identifier = castToIdentifier(value); // Identifier 1929 else if (name.equals("questionnaire")) 1930 this.questionnaire = castToReference(value); // Reference 1931 else if (name.equals("status")) 1932 this.status = new QuestionnaireResponseStatusEnumFactory().fromType(value); // Enumeration<QuestionnaireResponseStatus> 1933 else if (name.equals("subject")) 1934 this.subject = castToReference(value); // Reference 1935 else if (name.equals("author")) 1936 this.author = castToReference(value); // Reference 1937 else if (name.equals("authored")) 1938 this.authored = castToDateTime(value); // DateTimeType 1939 else if (name.equals("source")) 1940 this.source = castToReference(value); // Reference 1941 else if (name.equals("encounter")) 1942 this.encounter = castToReference(value); // Reference 1943 else if (name.equals("group")) 1944 this.group = (GroupComponent) value; // GroupComponent 1945 else 1946 super.setProperty(name, value); 1947 } 1948 1949 @Override 1950 public Base addChild(String name) throws FHIRException { 1951 if (name.equals("identifier")) { 1952 this.identifier = new Identifier(); 1953 return this.identifier; 1954 } else if (name.equals("questionnaire")) { 1955 this.questionnaire = new Reference(); 1956 return this.questionnaire; 1957 } else if (name.equals("status")) { 1958 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.status"); 1959 } else if (name.equals("subject")) { 1960 this.subject = new Reference(); 1961 return this.subject; 1962 } else if (name.equals("author")) { 1963 this.author = new Reference(); 1964 return this.author; 1965 } else if (name.equals("authored")) { 1966 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.authored"); 1967 } else if (name.equals("source")) { 1968 this.source = new Reference(); 1969 return this.source; 1970 } else if (name.equals("encounter")) { 1971 this.encounter = new Reference(); 1972 return this.encounter; 1973 } else if (name.equals("group")) { 1974 this.group = new GroupComponent(); 1975 return this.group; 1976 } else 1977 return super.addChild(name); 1978 } 1979 1980 public String fhirType() { 1981 return "QuestionnaireResponse"; 1982 1983 } 1984 1985 public QuestionnaireResponse copy() { 1986 QuestionnaireResponse dst = new QuestionnaireResponse(); 1987 copyValues(dst); 1988 dst.identifier = identifier == null ? null : identifier.copy(); 1989 dst.questionnaire = questionnaire == null ? null : questionnaire.copy(); 1990 dst.status = status == null ? null : status.copy(); 1991 dst.subject = subject == null ? null : subject.copy(); 1992 dst.author = author == null ? null : author.copy(); 1993 dst.authored = authored == null ? null : authored.copy(); 1994 dst.source = source == null ? null : source.copy(); 1995 dst.encounter = encounter == null ? null : encounter.copy(); 1996 dst.group = group == null ? null : group.copy(); 1997 return dst; 1998 } 1999 2000 protected QuestionnaireResponse typedCopy() { 2001 return copy(); 2002 } 2003 2004 @Override 2005 public boolean equalsDeep(Base other) { 2006 if (!super.equalsDeep(other)) 2007 return false; 2008 if (!(other instanceof QuestionnaireResponse)) 2009 return false; 2010 QuestionnaireResponse o = (QuestionnaireResponse) other; 2011 return compareDeep(identifier, o.identifier, true) && compareDeep(questionnaire, o.questionnaire, true) 2012 && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 2013 && compareDeep(author, o.author, true) && compareDeep(authored, o.authored, true) 2014 && compareDeep(source, o.source, true) && compareDeep(encounter, o.encounter, true) 2015 && compareDeep(group, o.group, true); 2016 } 2017 2018 @Override 2019 public boolean equalsShallow(Base other) { 2020 if (!super.equalsShallow(other)) 2021 return false; 2022 if (!(other instanceof QuestionnaireResponse)) 2023 return false; 2024 QuestionnaireResponse o = (QuestionnaireResponse) other; 2025 return compareValues(status, o.status, true) && compareValues(authored, o.authored, true); 2026 } 2027 2028 public boolean isEmpty() { 2029 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 2030 && (questionnaire == null || questionnaire.isEmpty()) && (status == null || status.isEmpty()) 2031 && (subject == null || subject.isEmpty()) && (author == null || author.isEmpty()) 2032 && (authored == null || authored.isEmpty()) && (source == null || source.isEmpty()) 2033 && (encounter == null || encounter.isEmpty()) && (group == null || group.isEmpty()); 2034 } 2035 2036 @Override 2037 public ResourceType getResourceType() { 2038 return ResourceType.QuestionnaireResponse; 2039 } 2040 2041 @SearchParamDefinition(name = "authored", path = "QuestionnaireResponse.authored", description = "When the questionnaire was authored", type = "date") 2042 public static final String SP_AUTHORED = "authored"; 2043 @SearchParamDefinition(name = "questionnaire", path = "QuestionnaireResponse.questionnaire", description = "The questionnaire the answers are provided for", type = "reference") 2044 public static final String SP_QUESTIONNAIRE = "questionnaire"; 2045 @SearchParamDefinition(name = "subject", path = "QuestionnaireResponse.subject", description = "The subject of the questionnaire", type = "reference") 2046 public static final String SP_SUBJECT = "subject"; 2047 @SearchParamDefinition(name = "author", path = "QuestionnaireResponse.author", description = "The author of the questionnaire", type = "reference") 2048 public static final String SP_AUTHOR = "author"; 2049 @SearchParamDefinition(name = "patient", path = "QuestionnaireResponse.subject", description = "The patient that is the subject of the questionnaire", type = "reference") 2050 public static final String SP_PATIENT = "patient"; 2051 @SearchParamDefinition(name = "encounter", path = "QuestionnaireResponse.encounter", description = "Encounter during which questionnaire was authored", type = "reference") 2052 public static final String SP_ENCOUNTER = "encounter"; 2053 @SearchParamDefinition(name = "source", path = "QuestionnaireResponse.source", description = "The person who answered the questions", type = "reference") 2054 public static final String SP_SOURCE = "source"; 2055 @SearchParamDefinition(name = "status", path = "QuestionnaireResponse.status", description = "The status of the questionnaire response", type = "token") 2056 public static final String SP_STATUS = "status"; 2057 2058}