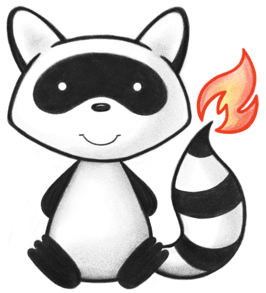
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.exceptions.FHIRException; 040 041/** 042 * A set of ordered Quantities defined by a low and high limit. 043 */ 044@DatatypeDef(name = "Range") 045public class Range extends Type implements ICompositeType { 046 047 /** 048 * The low limit. The boundary is inclusive. 049 */ 050 @Child(name = "low", type = { SimpleQuantity.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 051 @Description(shortDefinition = "Low limit", formalDefinition = "The low limit. The boundary is inclusive.") 052 protected SimpleQuantity low; 053 054 /** 055 * The high limit. The boundary is inclusive. 056 */ 057 @Child(name = "high", type = { SimpleQuantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 058 @Description(shortDefinition = "High limit", formalDefinition = "The high limit. The boundary is inclusive.") 059 protected SimpleQuantity high; 060 061 private static final long serialVersionUID = 1699187994L; 062 063 /* 064 * Constructor 065 */ 066 public Range() { 067 super(); 068 } 069 070 /** 071 * @return {@link #low} (The low limit. The boundary is inclusive.) 072 */ 073 public SimpleQuantity getLow() { 074 if (this.low == null) 075 if (Configuration.errorOnAutoCreate()) 076 throw new Error("Attempt to auto-create Range.low"); 077 else if (Configuration.doAutoCreate()) 078 this.low = new SimpleQuantity(); // cc 079 return this.low; 080 } 081 082 public boolean hasLow() { 083 return this.low != null && !this.low.isEmpty(); 084 } 085 086 /** 087 * @param value {@link #low} (The low limit. The boundary is inclusive.) 088 */ 089 public Range setLow(SimpleQuantity value) { 090 this.low = value; 091 return this; 092 } 093 094 /** 095 * @return {@link #high} (The high limit. The boundary is inclusive.) 096 */ 097 public SimpleQuantity getHigh() { 098 if (this.high == null) 099 if (Configuration.errorOnAutoCreate()) 100 throw new Error("Attempt to auto-create Range.high"); 101 else if (Configuration.doAutoCreate()) 102 this.high = new SimpleQuantity(); // cc 103 return this.high; 104 } 105 106 public boolean hasHigh() { 107 return this.high != null && !this.high.isEmpty(); 108 } 109 110 /** 111 * @param value {@link #high} (The high limit. The boundary is inclusive.) 112 */ 113 public Range setHigh(SimpleQuantity value) { 114 this.high = value; 115 return this; 116 } 117 118 protected void listChildren(List<Property> childrenList) { 119 super.listChildren(childrenList); 120 childrenList.add(new Property("low", "SimpleQuantity", "The low limit. The boundary is inclusive.", 0, 121 java.lang.Integer.MAX_VALUE, low)); 122 childrenList.add(new Property("high", "SimpleQuantity", "The high limit. The boundary is inclusive.", 0, 123 java.lang.Integer.MAX_VALUE, high)); 124 } 125 126 @Override 127 public void setProperty(String name, Base value) throws FHIRException { 128 if (name.equals("low")) 129 this.low = castToSimpleQuantity(value); // SimpleQuantity 130 else if (name.equals("high")) 131 this.high = castToSimpleQuantity(value); // SimpleQuantity 132 else 133 super.setProperty(name, value); 134 } 135 136 @Override 137 public Base addChild(String name) throws FHIRException { 138 if (name.equals("low")) { 139 this.low = new SimpleQuantity(); 140 return this.low; 141 } else if (name.equals("high")) { 142 this.high = new SimpleQuantity(); 143 return this.high; 144 } else 145 return super.addChild(name); 146 } 147 148 public String fhirType() { 149 return "Range"; 150 151 } 152 153 public Range copy() { 154 Range dst = new Range(); 155 copyValues(dst); 156 dst.low = low == null ? null : low.copy(); 157 dst.high = high == null ? null : high.copy(); 158 return dst; 159 } 160 161 protected Range typedCopy() { 162 return copy(); 163 } 164 165 @Override 166 public boolean equalsDeep(Base other) { 167 if (!super.equalsDeep(other)) 168 return false; 169 if (!(other instanceof Range)) 170 return false; 171 Range o = (Range) other; 172 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true); 173 } 174 175 @Override 176 public boolean equalsShallow(Base other) { 177 if (!super.equalsShallow(other)) 178 return false; 179 if (!(other instanceof Range)) 180 return false; 181 Range o = (Range) other; 182 return true; 183 } 184 185 public boolean isEmpty() { 186 return super.isEmpty() && (low == null || low.isEmpty()) && (high == null || high.isEmpty()); 187 } 188 189}