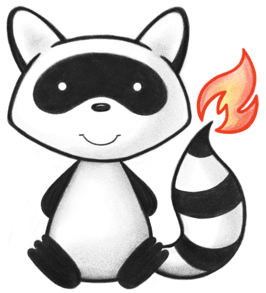
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.exceptions.FHIRException; 040 041/** 042 * A relationship of two Quantity values - expressed as a numerator and a 043 * denominator. 044 */ 045@DatatypeDef(name = "Ratio") 046public class Ratio extends Type implements ICompositeType { 047 048 /** 049 * The value of the numerator. 050 */ 051 @Child(name = "numerator", type = { Quantity.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 052 @Description(shortDefinition = "Numerator value", formalDefinition = "The value of the numerator.") 053 protected Quantity numerator; 054 055 /** 056 * The value of the denominator. 057 */ 058 @Child(name = "denominator", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "Denominator value", formalDefinition = "The value of the denominator.") 060 protected Quantity denominator; 061 062 private static final long serialVersionUID = 479922563L; 063 064 /* 065 * Constructor 066 */ 067 public Ratio() { 068 super(); 069 } 070 071 /** 072 * @return {@link #numerator} (The value of the numerator.) 073 */ 074 public Quantity getNumerator() { 075 if (this.numerator == null) 076 if (Configuration.errorOnAutoCreate()) 077 throw new Error("Attempt to auto-create Ratio.numerator"); 078 else if (Configuration.doAutoCreate()) 079 this.numerator = new Quantity(); // cc 080 return this.numerator; 081 } 082 083 public boolean hasNumerator() { 084 return this.numerator != null && !this.numerator.isEmpty(); 085 } 086 087 /** 088 * @param value {@link #numerator} (The value of the numerator.) 089 */ 090 public Ratio setNumerator(Quantity value) { 091 this.numerator = value; 092 return this; 093 } 094 095 /** 096 * @return {@link #denominator} (The value of the denominator.) 097 */ 098 public Quantity getDenominator() { 099 if (this.denominator == null) 100 if (Configuration.errorOnAutoCreate()) 101 throw new Error("Attempt to auto-create Ratio.denominator"); 102 else if (Configuration.doAutoCreate()) 103 this.denominator = new Quantity(); // cc 104 return this.denominator; 105 } 106 107 public boolean hasDenominator() { 108 return this.denominator != null && !this.denominator.isEmpty(); 109 } 110 111 /** 112 * @param value {@link #denominator} (The value of the denominator.) 113 */ 114 public Ratio setDenominator(Quantity value) { 115 this.denominator = value; 116 return this; 117 } 118 119 protected void listChildren(List<Property> childrenList) { 120 super.listChildren(childrenList); 121 childrenList.add(new Property("numerator", "Quantity", "The value of the numerator.", 0, 122 java.lang.Integer.MAX_VALUE, numerator)); 123 childrenList.add(new Property("denominator", "Quantity", "The value of the denominator.", 0, 124 java.lang.Integer.MAX_VALUE, denominator)); 125 } 126 127 @Override 128 public void setProperty(String name, Base value) throws FHIRException { 129 if (name.equals("numerator")) 130 this.numerator = castToQuantity(value); // Quantity 131 else if (name.equals("denominator")) 132 this.denominator = castToQuantity(value); // Quantity 133 else 134 super.setProperty(name, value); 135 } 136 137 @Override 138 public Base addChild(String name) throws FHIRException { 139 if (name.equals("numerator")) { 140 this.numerator = new Quantity(); 141 return this.numerator; 142 } else if (name.equals("denominator")) { 143 this.denominator = new Quantity(); 144 return this.denominator; 145 } else 146 return super.addChild(name); 147 } 148 149 public String fhirType() { 150 return "Ratio"; 151 152 } 153 154 public Ratio copy() { 155 Ratio dst = new Ratio(); 156 copyValues(dst); 157 dst.numerator = numerator == null ? null : numerator.copy(); 158 dst.denominator = denominator == null ? null : denominator.copy(); 159 return dst; 160 } 161 162 protected Ratio typedCopy() { 163 return copy(); 164 } 165 166 @Override 167 public boolean equalsDeep(Base other) { 168 if (!super.equalsDeep(other)) 169 return false; 170 if (!(other instanceof Ratio)) 171 return false; 172 Ratio o = (Ratio) other; 173 return compareDeep(numerator, o.numerator, true) && compareDeep(denominator, o.denominator, true); 174 } 175 176 @Override 177 public boolean equalsShallow(Base other) { 178 if (!super.equalsShallow(other)) 179 return false; 180 if (!(other instanceof Ratio)) 181 return false; 182 Ratio o = (Ratio) other; 183 return true; 184 } 185 186 public boolean isEmpty() { 187 return super.isEmpty() && (numerator == null || numerator.isEmpty()) 188 && (denominator == null || denominator.isEmpty()); 189 } 190 191}