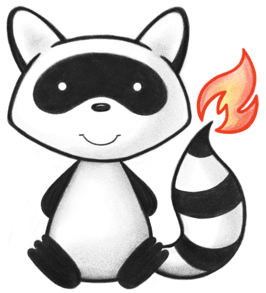
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.IAnyResource; 039import org.hl7.fhir.instance.model.api.IBaseReference; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * A reference from one resource to another. 046 */ 047@DatatypeDef(name = "Reference") 048public class Reference extends BaseReference implements IBaseReference, ICompositeType { 049 050 /** 051 * A reference to a location at which the other resource is found. The reference 052 * may be a relative reference, in which case it is relative to the service base 053 * URL, or an absolute URL that resolves to the location where the resource is 054 * found. The reference may be version specific or not. If the reference is not 055 * to a FHIR RESTful server, then it should be assumed to be version specific. 056 * Internal fragment references (start with '#') refer to contained resources. 057 */ 058 @Child(name = "reference", type = { StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "Relative, internal or absolute URL reference", formalDefinition = "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.") 060 protected StringType reference; 061 062 /** 063 * Plain text narrative that identifies the resource in addition to the resource 064 * reference. 065 */ 066 @Child(name = "display", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "Text alternative for the resource", formalDefinition = "Plain text narrative that identifies the resource in addition to the resource reference.") 068 protected StringType display; 069 070 private static final long serialVersionUID = 22777321L; 071 072 /* 073 * Constructor 074 */ 075 public Reference() { 076 super(); 077 } 078 079 /** 080 * Constructor 081 * 082 * @param theReference The given reference string (e.g. "Patient/123" or 083 * "http://example.com/Patient/123") 084 */ 085 public Reference(String theReference) { 086 super(theReference); 087 } 088 089 /** 090 * Constructor 091 * 092 * @param theReference The given reference as an IdType (e.g. "Patient/123" or 093 * "http://example.com/Patient/123") 094 */ 095 public Reference(IdType theReference) { 096 super(theReference); 097 } 098 099 /** 100 * Constructor 101 * 102 * @param theResource The resource represented by this reference 103 */ 104 public Reference(IAnyResource theResource) { 105 super(theResource); 106 } 107 108 /** 109 * @return {@link #reference} (A reference to a location at which the other 110 * resource is found. The reference may be a relative reference, in 111 * which case it is relative to the service base URL, or an absolute URL 112 * that resolves to the location where the resource is found. The 113 * reference may be version specific or not. If the reference is not to 114 * a FHIR RESTful server, then it should be assumed to be version 115 * specific. Internal fragment references (start with '#') refer to 116 * contained resources.). This is the underlying object with id, value 117 * and extensions. The accessor "getReference" gives direct access to 118 * the value 119 */ 120 public boolean hasReferenceElement() { 121 return this.reference != null && !this.reference.isEmpty(); 122 } 123 124 public boolean hasReference() { 125 return this.reference != null && !this.reference.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #reference} (A reference to a location at which the other 130 * resource is found. The reference may be a relative reference, in 131 * which case it is relative to the service base URL, or an 132 * absolute URL that resolves to the location where the resource is 133 * found. The reference may be version specific or not. If the 134 * reference is not to a FHIR RESTful server, then it should be 135 * assumed to be version specific. Internal fragment references 136 * (start with '#') refer to contained resources.). This is the 137 * underlying object with id, value and extensions. The accessor 138 * "getReference" gives direct access to the value 139 */ 140 public Reference setReferenceElement(StringType value) { 141 this.reference = value; 142 return this; 143 } 144 145 /** 146 * @return A reference to a location at which the other resource is found. The 147 * reference may be a relative reference, in which case it is relative 148 * to the service base URL, or an absolute URL that resolves to the 149 * location where the resource is found. The reference may be version 150 * specific or not. If the reference is not to a FHIR RESTful server, 151 * then it should be assumed to be version specific. Internal fragment 152 * references (start with '#') refer to contained resources. 153 */ 154 public String getReference() { 155 return this.reference == null ? null : this.reference.getValue(); 156 } 157 158 /** 159 * @param value A reference to a location at which the other resource is found. 160 * The reference may be a relative reference, in which case it is 161 * relative to the service base URL, or an absolute URL that 162 * resolves to the location where the resource is found. The 163 * reference may be version specific or not. If the reference is 164 * not to a FHIR RESTful server, then it should be assumed to be 165 * version specific. Internal fragment references (start with '#') 166 * refer to contained resources. 167 */ 168 public Reference setReference(String value) { 169 if (Utilities.noString(value)) 170 this.reference = null; 171 else { 172 if (this.reference == null) 173 this.reference = new StringType(); 174 this.reference.setValue(value); 175 } 176 return this; 177 } 178 179 /** 180 * @return {@link #display} (Plain text narrative that identifies the resource 181 * in addition to the resource reference.). This is the underlying 182 * object with id, value and extensions. The accessor "getDisplay" gives 183 * direct access to the value 184 */ 185 public StringType getDisplayElement() { 186 if (this.display == null) 187 if (Configuration.errorOnAutoCreate()) 188 throw new Error("Attempt to auto-create Reference.display"); 189 else if (Configuration.doAutoCreate()) 190 this.display = new StringType(); // bb 191 return this.display; 192 } 193 194 public boolean hasDisplayElement() { 195 return this.display != null && !this.display.isEmpty(); 196 } 197 198 public boolean hasDisplay() { 199 return this.display != null && !this.display.isEmpty(); 200 } 201 202 /** 203 * @param value {@link #display} (Plain text narrative that identifies the 204 * resource in addition to the resource reference.). This is the 205 * underlying object with id, value and extensions. The accessor 206 * "getDisplay" gives direct access to the value 207 */ 208 public Reference setDisplayElement(StringType value) { 209 this.display = value; 210 return this; 211 } 212 213 /** 214 * @return Plain text narrative that identifies the resource in addition to the 215 * resource reference. 216 */ 217 public String getDisplay() { 218 return this.display == null ? null : this.display.getValue(); 219 } 220 221 /** 222 * @param value Plain text narrative that identifies the resource in addition to 223 * the resource reference. 224 */ 225 public Reference setDisplay(String value) { 226 if (Utilities.noString(value)) 227 this.display = null; 228 else { 229 if (this.display == null) 230 this.display = new StringType(); 231 this.display.setValue(value); 232 } 233 return this; 234 } 235 236 protected void listChildren(List<Property> childrenList) { 237 super.listChildren(childrenList); 238 childrenList.add(new Property("reference", "string", 239 "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.", 240 0, java.lang.Integer.MAX_VALUE, reference)); 241 childrenList.add(new Property("display", "string", 242 "Plain text narrative that identifies the resource in addition to the resource reference.", 0, 243 java.lang.Integer.MAX_VALUE, display)); 244 } 245 246 @Override 247 public void setProperty(String name, Base value) throws FHIRException { 248 if (name.equals("reference")) 249 this.reference = castToString(value); // StringType 250 else if (name.equals("display")) 251 this.display = castToString(value); // StringType 252 else 253 super.setProperty(name, value); 254 } 255 256 @Override 257 public Base addChild(String name) throws FHIRException { 258 if (name.equals("reference")) { 259 throw new FHIRException("Cannot call addChild on a singleton property Reference.reference"); 260 } else if (name.equals("display")) { 261 throw new FHIRException("Cannot call addChild on a singleton property Reference.display"); 262 } else 263 return super.addChild(name); 264 } 265 266 public String fhirType() { 267 return "Reference"; 268 269 } 270 271 public Reference copy() { 272 Reference dst = new Reference(); 273 copyValues(dst); 274 dst.reference = reference == null ? null : reference.copy(); 275 dst.display = display == null ? null : display.copy(); 276 return dst; 277 } 278 279 protected Reference typedCopy() { 280 return copy(); 281 } 282 283 @Override 284 public boolean equalsDeep(Base other) { 285 if (!super.equalsDeep(other)) 286 return false; 287 if (!(other instanceof Reference)) 288 return false; 289 Reference o = (Reference) other; 290 return compareDeep(reference, o.reference, true) && compareDeep(display, o.display, true); 291 } 292 293 @Override 294 public boolean equalsShallow(Base other) { 295 if (!super.equalsShallow(other)) 296 return false; 297 if (!(other instanceof Reference)) 298 return false; 299 Reference o = (Reference) other; 300 return compareValues(reference, o.reference, true) && compareValues(display, o.display, true); 301 } 302 303 public boolean isEmpty() { 304 return super.isEmpty() && (reference == null || reference.isEmpty()) && (display == null || display.isEmpty()); 305 } 306 307}