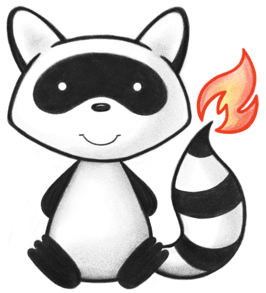
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * Used to record and send details about a request for referral service or 046 * transfer of a patient to the care of another provider or provider 047 * organization. 048 */ 049@ResourceDef(name = "ReferralRequest", profile = "http://hl7.org/fhir/Profile/ReferralRequest") 050public class ReferralRequest extends DomainResource { 051 052 public enum ReferralStatus { 053 /** 054 * A draft referral that has yet to be send. 055 */ 056 DRAFT, 057 /** 058 * The referral has been transmitted, but not yet acknowledged by the recipient. 059 */ 060 REQUESTED, 061 /** 062 * The referral has been acknowledged by the recipient, and is in the process of 063 * being actioned. 064 */ 065 ACTIVE, 066 /** 067 * The referral has been cancelled without being completed. For example it is no 068 * longer needed. 069 */ 070 CANCELLED, 071 /** 072 * The recipient has agreed to deliver the care requested by the referral. 073 */ 074 ACCEPTED, 075 /** 076 * The recipient has declined to accept the referral. 077 */ 078 REJECTED, 079 /** 080 * The referral has been completely actioned. 081 */ 082 COMPLETED, 083 /** 084 * added to help the parsers 085 */ 086 NULL; 087 088 public static ReferralStatus fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("draft".equals(codeString)) 092 return DRAFT; 093 if ("requested".equals(codeString)) 094 return REQUESTED; 095 if ("active".equals(codeString)) 096 return ACTIVE; 097 if ("cancelled".equals(codeString)) 098 return CANCELLED; 099 if ("accepted".equals(codeString)) 100 return ACCEPTED; 101 if ("rejected".equals(codeString)) 102 return REJECTED; 103 if ("completed".equals(codeString)) 104 return COMPLETED; 105 throw new FHIRException("Unknown ReferralStatus code '" + codeString + "'"); 106 } 107 108 public String toCode() { 109 switch (this) { 110 case DRAFT: 111 return "draft"; 112 case REQUESTED: 113 return "requested"; 114 case ACTIVE: 115 return "active"; 116 case CANCELLED: 117 return "cancelled"; 118 case ACCEPTED: 119 return "accepted"; 120 case REJECTED: 121 return "rejected"; 122 case COMPLETED: 123 return "completed"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getSystem() { 132 switch (this) { 133 case DRAFT: 134 return "http://hl7.org/fhir/referralstatus"; 135 case REQUESTED: 136 return "http://hl7.org/fhir/referralstatus"; 137 case ACTIVE: 138 return "http://hl7.org/fhir/referralstatus"; 139 case CANCELLED: 140 return "http://hl7.org/fhir/referralstatus"; 141 case ACCEPTED: 142 return "http://hl7.org/fhir/referralstatus"; 143 case REJECTED: 144 return "http://hl7.org/fhir/referralstatus"; 145 case COMPLETED: 146 return "http://hl7.org/fhir/referralstatus"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getDefinition() { 155 switch (this) { 156 case DRAFT: 157 return "A draft referral that has yet to be send."; 158 case REQUESTED: 159 return "The referral has been transmitted, but not yet acknowledged by the recipient."; 160 case ACTIVE: 161 return "The referral has been acknowledged by the recipient, and is in the process of being actioned."; 162 case CANCELLED: 163 return "The referral has been cancelled without being completed. For example it is no longer needed."; 164 case ACCEPTED: 165 return "The recipient has agreed to deliver the care requested by the referral."; 166 case REJECTED: 167 return "The recipient has declined to accept the referral."; 168 case COMPLETED: 169 return "The referral has been completely actioned."; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 177 public String getDisplay() { 178 switch (this) { 179 case DRAFT: 180 return "Draft"; 181 case REQUESTED: 182 return "Requested"; 183 case ACTIVE: 184 return "Active"; 185 case CANCELLED: 186 return "Cancelled"; 187 case ACCEPTED: 188 return "Accepted"; 189 case REJECTED: 190 return "Rejected"; 191 case COMPLETED: 192 return "Completed"; 193 case NULL: 194 return null; 195 default: 196 return "?"; 197 } 198 } 199 } 200 201 public static class ReferralStatusEnumFactory implements EnumFactory<ReferralStatus> { 202 public ReferralStatus fromCode(String codeString) throws IllegalArgumentException { 203 if (codeString == null || "".equals(codeString)) 204 if (codeString == null || "".equals(codeString)) 205 return null; 206 if ("draft".equals(codeString)) 207 return ReferralStatus.DRAFT; 208 if ("requested".equals(codeString)) 209 return ReferralStatus.REQUESTED; 210 if ("active".equals(codeString)) 211 return ReferralStatus.ACTIVE; 212 if ("cancelled".equals(codeString)) 213 return ReferralStatus.CANCELLED; 214 if ("accepted".equals(codeString)) 215 return ReferralStatus.ACCEPTED; 216 if ("rejected".equals(codeString)) 217 return ReferralStatus.REJECTED; 218 if ("completed".equals(codeString)) 219 return ReferralStatus.COMPLETED; 220 throw new IllegalArgumentException("Unknown ReferralStatus code '" + codeString + "'"); 221 } 222 223 public Enumeration<ReferralStatus> fromType(Base code) throws FHIRException { 224 if (code == null || code.isEmpty()) 225 return null; 226 String codeString = ((PrimitiveType) code).asStringValue(); 227 if (codeString == null || "".equals(codeString)) 228 return null; 229 if ("draft".equals(codeString)) 230 return new Enumeration<ReferralStatus>(this, ReferralStatus.DRAFT); 231 if ("requested".equals(codeString)) 232 return new Enumeration<ReferralStatus>(this, ReferralStatus.REQUESTED); 233 if ("active".equals(codeString)) 234 return new Enumeration<ReferralStatus>(this, ReferralStatus.ACTIVE); 235 if ("cancelled".equals(codeString)) 236 return new Enumeration<ReferralStatus>(this, ReferralStatus.CANCELLED); 237 if ("accepted".equals(codeString)) 238 return new Enumeration<ReferralStatus>(this, ReferralStatus.ACCEPTED); 239 if ("rejected".equals(codeString)) 240 return new Enumeration<ReferralStatus>(this, ReferralStatus.REJECTED); 241 if ("completed".equals(codeString)) 242 return new Enumeration<ReferralStatus>(this, ReferralStatus.COMPLETED); 243 throw new FHIRException("Unknown ReferralStatus code '" + codeString + "'"); 244 } 245 246 public String toCode(ReferralStatus code) 247 { 248 if (code == ReferralStatus.NULL) 249 return null; 250 if (code == ReferralStatus.DRAFT) 251 return "draft"; 252 if (code == ReferralStatus.REQUESTED) 253 return "requested"; 254 if (code == ReferralStatus.ACTIVE) 255 return "active"; 256 if (code == ReferralStatus.CANCELLED) 257 return "cancelled"; 258 if (code == ReferralStatus.ACCEPTED) 259 return "accepted"; 260 if (code == ReferralStatus.REJECTED) 261 return "rejected"; 262 if (code == ReferralStatus.COMPLETED) 263 return "completed"; 264 return "?"; 265 } 266 } 267 268 /** 269 * The workflow status of the referral or transfer of care request. 270 */ 271 @Child(name = "status", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = true, summary = true) 272 @Description(shortDefinition = "draft | requested | active | cancelled | accepted | rejected | completed", formalDefinition = "The workflow status of the referral or transfer of care request.") 273 protected Enumeration<ReferralStatus> status; 274 275 /** 276 * Business identifier that uniquely identifies the referral/care transfer 277 * request instance. 278 */ 279 @Child(name = "identifier", type = { 280 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 281 @Description(shortDefinition = "Business identifier", formalDefinition = "Business identifier that uniquely identifies the referral/care transfer request instance.") 282 protected List<Identifier> identifier; 283 284 /** 285 * Date/DateTime of creation for draft requests and date of activation for 286 * active requests. 287 */ 288 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 289 @Description(shortDefinition = "Date of creation/activation", formalDefinition = "Date/DateTime of creation for draft requests and date of activation for active requests.") 290 protected DateTimeType date; 291 292 /** 293 * An indication of the type of referral (or where applicable the type of 294 * transfer of care) request. 295 */ 296 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 297 @Description(shortDefinition = "Referral/Transition of care request type", formalDefinition = "An indication of the type of referral (or where applicable the type of transfer of care) request.") 298 protected CodeableConcept type; 299 300 /** 301 * Indication of the clinical domain or discipline to which the referral or 302 * transfer of care request is sent. For example: Cardiology Gastroenterology 303 * Diabetology. 304 */ 305 @Child(name = "specialty", type = { 306 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 307 @Description(shortDefinition = "The clinical specialty (discipline) that the referral is requested for", formalDefinition = "Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology.") 308 protected CodeableConcept specialty; 309 310 /** 311 * An indication of the urgency of referral (or where applicable the type of 312 * transfer of care) request. 313 */ 314 @Child(name = "priority", type = { 315 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 316 @Description(shortDefinition = "Urgency of referral / transfer of care request", formalDefinition = "An indication of the urgency of referral (or where applicable the type of transfer of care) request.") 317 protected CodeableConcept priority; 318 319 /** 320 * The patient who is the subject of a referral or transfer of care request. 321 */ 322 @Child(name = "patient", type = { Patient.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 323 @Description(shortDefinition = "Patient referred to care or transfer", formalDefinition = "The patient who is the subject of a referral or transfer of care request.") 324 protected Reference patient; 325 326 /** 327 * The actual object that is the target of the reference (The patient who is the 328 * subject of a referral or transfer of care request.) 329 */ 330 protected Patient patientTarget; 331 332 /** 333 * The healthcare provider or provider organization who/which initiated the 334 * referral/transfer of care request. Can also be Patient (a self referral). 335 */ 336 @Child(name = "requester", type = { Practitioner.class, Organization.class, 337 Patient.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 338 @Description(shortDefinition = "Requester of referral / transfer of care", formalDefinition = "The healthcare provider or provider organization who/which initiated the referral/transfer of care request. Can also be Patient (a self referral).") 339 protected Reference requester; 340 341 /** 342 * The actual object that is the target of the reference (The healthcare 343 * provider or provider organization who/which initiated the referral/transfer 344 * of care request. Can also be Patient (a self referral).) 345 */ 346 protected Resource requesterTarget; 347 348 /** 349 * The healthcare provider(s) or provider organization(s) who/which is to 350 * receive the referral/transfer of care request. 351 */ 352 @Child(name = "recipient", type = { Practitioner.class, 353 Organization.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 354 @Description(shortDefinition = "Receiver of referral / transfer of care request", formalDefinition = "The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request.") 355 protected List<Reference> recipient; 356 /** 357 * The actual objects that are the target of the reference (The healthcare 358 * provider(s) or provider organization(s) who/which is to receive the 359 * referral/transfer of care request.) 360 */ 361 protected List<Resource> recipientTarget; 362 363 /** 364 * The encounter at which the request for referral or transfer of care is 365 * initiated. 366 */ 367 @Child(name = "encounter", type = { Encounter.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 368 @Description(shortDefinition = "Originating encounter", formalDefinition = "The encounter at which the request for referral or transfer of care is initiated.") 369 protected Reference encounter; 370 371 /** 372 * The actual object that is the target of the reference (The encounter at which 373 * the request for referral or transfer of care is initiated.) 374 */ 375 protected Encounter encounterTarget; 376 377 /** 378 * Date/DateTime the request for referral or transfer of care is sent by the 379 * author. 380 */ 381 @Child(name = "dateSent", type = { 382 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 383 @Description(shortDefinition = "Date referral/transfer of care request is sent", formalDefinition = "Date/DateTime the request for referral or transfer of care is sent by the author.") 384 protected DateTimeType dateSent; 385 386 /** 387 * Description of clinical condition indicating why referral/transfer of care is 388 * requested. For example: Pathological Anomalies, Disabled (physical or 389 * mental), Behavioral Management. 390 */ 391 @Child(name = "reason", type = { 392 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 393 @Description(shortDefinition = "Reason for referral / transfer of care request", formalDefinition = "Description of clinical condition indicating why referral/transfer of care is requested. For example: Pathological Anomalies, Disabled (physical or mental), Behavioral Management.") 394 protected CodeableConcept reason; 395 396 /** 397 * The reason element gives a short description of why the referral is being 398 * made, the description expands on this to support a more complete clinical 399 * summary. 400 */ 401 @Child(name = "description", type = { 402 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 403 @Description(shortDefinition = "A textual description of the referral", formalDefinition = "The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary.") 404 protected StringType description; 405 406 /** 407 * The service(s) that is/are requested to be provided to the patient. For 408 * example: cardiac pacemaker insertion. 409 */ 410 @Child(name = "serviceRequested", type = { 411 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 412 @Description(shortDefinition = "Actions requested as part of the referral", formalDefinition = "The service(s) that is/are requested to be provided to the patient. For example: cardiac pacemaker insertion.") 413 protected List<CodeableConcept> serviceRequested; 414 415 /** 416 * Any additional (administrative, financial or clinical) information required 417 * to support request for referral or transfer of care. For example: Presenting 418 * problems/chief complaints Medical History Family History Alerts 419 * Allergy/Intolerance and Adverse Reactions Medications 420 * Observations/Assessments (may include cognitive and fundtional assessments) 421 * Diagnostic Reports Care Plan. 422 */ 423 @Child(name = "supportingInformation", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 424 @Description(shortDefinition = "Additonal information to support referral or transfer of care request", formalDefinition = "Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan.") 425 protected List<Reference> supportingInformation; 426 /** 427 * The actual objects that are the target of the reference (Any additional 428 * (administrative, financial or clinical) information required to support 429 * request for referral or transfer of care. For example: Presenting 430 * problems/chief complaints Medical History Family History Alerts 431 * Allergy/Intolerance and Adverse Reactions Medications 432 * Observations/Assessments (may include cognitive and fundtional assessments) 433 * Diagnostic Reports Care Plan.) 434 */ 435 protected List<Resource> supportingInformationTarget; 436 437 /** 438 * The period of time within which the services identified in the 439 * referral/transfer of care is specified or required to occur. 440 */ 441 @Child(name = "fulfillmentTime", type = { 442 Period.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 443 @Description(shortDefinition = "Requested service(s) fulfillment time", formalDefinition = "The period of time within which the services identified in the referral/transfer of care is specified or required to occur.") 444 protected Period fulfillmentTime; 445 446 private static final long serialVersionUID = 1948652599L; 447 448 /* 449 * Constructor 450 */ 451 public ReferralRequest() { 452 super(); 453 } 454 455 /* 456 * Constructor 457 */ 458 public ReferralRequest(Enumeration<ReferralStatus> status) { 459 super(); 460 this.status = status; 461 } 462 463 /** 464 * @return {@link #status} (The workflow status of the referral or transfer of 465 * care request.). This is the underlying object with id, value and 466 * extensions. The accessor "getStatus" gives direct access to the value 467 */ 468 public Enumeration<ReferralStatus> getStatusElement() { 469 if (this.status == null) 470 if (Configuration.errorOnAutoCreate()) 471 throw new Error("Attempt to auto-create ReferralRequest.status"); 472 else if (Configuration.doAutoCreate()) 473 this.status = new Enumeration<ReferralStatus>(new ReferralStatusEnumFactory()); // bb 474 return this.status; 475 } 476 477 public boolean hasStatusElement() { 478 return this.status != null && !this.status.isEmpty(); 479 } 480 481 public boolean hasStatus() { 482 return this.status != null && !this.status.isEmpty(); 483 } 484 485 /** 486 * @param value {@link #status} (The workflow status of the referral or transfer 487 * of care request.). This is the underlying object with id, value 488 * and extensions. The accessor "getStatus" gives direct access to 489 * the value 490 */ 491 public ReferralRequest setStatusElement(Enumeration<ReferralStatus> value) { 492 this.status = value; 493 return this; 494 } 495 496 /** 497 * @return The workflow status of the referral or transfer of care request. 498 */ 499 public ReferralStatus getStatus() { 500 return this.status == null ? null : this.status.getValue(); 501 } 502 503 /** 504 * @param value The workflow status of the referral or transfer of care request. 505 */ 506 public ReferralRequest setStatus(ReferralStatus value) { 507 if (this.status == null) 508 this.status = new Enumeration<ReferralStatus>(new ReferralStatusEnumFactory()); 509 this.status.setValue(value); 510 return this; 511 } 512 513 /** 514 * @return {@link #identifier} (Business identifier that uniquely identifies the 515 * referral/care transfer request instance.) 516 */ 517 public List<Identifier> getIdentifier() { 518 if (this.identifier == null) 519 this.identifier = new ArrayList<Identifier>(); 520 return this.identifier; 521 } 522 523 public boolean hasIdentifier() { 524 if (this.identifier == null) 525 return false; 526 for (Identifier item : this.identifier) 527 if (!item.isEmpty()) 528 return true; 529 return false; 530 } 531 532 /** 533 * @return {@link #identifier} (Business identifier that uniquely identifies the 534 * referral/care transfer request instance.) 535 */ 536 // syntactic sugar 537 public Identifier addIdentifier() { // 3 538 Identifier t = new Identifier(); 539 if (this.identifier == null) 540 this.identifier = new ArrayList<Identifier>(); 541 this.identifier.add(t); 542 return t; 543 } 544 545 // syntactic sugar 546 public ReferralRequest addIdentifier(Identifier t) { // 3 547 if (t == null) 548 return this; 549 if (this.identifier == null) 550 this.identifier = new ArrayList<Identifier>(); 551 this.identifier.add(t); 552 return this; 553 } 554 555 /** 556 * @return {@link #date} (Date/DateTime of creation for draft requests and date 557 * of activation for active requests.). This is the underlying object 558 * with id, value and extensions. The accessor "getDate" gives direct 559 * access to the value 560 */ 561 public DateTimeType getDateElement() { 562 if (this.date == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create ReferralRequest.date"); 565 else if (Configuration.doAutoCreate()) 566 this.date = new DateTimeType(); // bb 567 return this.date; 568 } 569 570 public boolean hasDateElement() { 571 return this.date != null && !this.date.isEmpty(); 572 } 573 574 public boolean hasDate() { 575 return this.date != null && !this.date.isEmpty(); 576 } 577 578 /** 579 * @param value {@link #date} (Date/DateTime of creation for draft requests and 580 * date of activation for active requests.). This is the underlying 581 * object with id, value and extensions. The accessor "getDate" 582 * gives direct access to the value 583 */ 584 public ReferralRequest setDateElement(DateTimeType value) { 585 this.date = value; 586 return this; 587 } 588 589 /** 590 * @return Date/DateTime of creation for draft requests and date of activation 591 * for active requests. 592 */ 593 public Date getDate() { 594 return this.date == null ? null : this.date.getValue(); 595 } 596 597 /** 598 * @param value Date/DateTime of creation for draft requests and date of 599 * activation for active requests. 600 */ 601 public ReferralRequest setDate(Date value) { 602 if (value == null) 603 this.date = null; 604 else { 605 if (this.date == null) 606 this.date = new DateTimeType(); 607 this.date.setValue(value); 608 } 609 return this; 610 } 611 612 /** 613 * @return {@link #type} (An indication of the type of referral (or where 614 * applicable the type of transfer of care) request.) 615 */ 616 public CodeableConcept getType() { 617 if (this.type == null) 618 if (Configuration.errorOnAutoCreate()) 619 throw new Error("Attempt to auto-create ReferralRequest.type"); 620 else if (Configuration.doAutoCreate()) 621 this.type = new CodeableConcept(); // cc 622 return this.type; 623 } 624 625 public boolean hasType() { 626 return this.type != null && !this.type.isEmpty(); 627 } 628 629 /** 630 * @param value {@link #type} (An indication of the type of referral (or where 631 * applicable the type of transfer of care) request.) 632 */ 633 public ReferralRequest setType(CodeableConcept value) { 634 this.type = value; 635 return this; 636 } 637 638 /** 639 * @return {@link #specialty} (Indication of the clinical domain or discipline 640 * to which the referral or transfer of care request is sent. For 641 * example: Cardiology Gastroenterology Diabetology.) 642 */ 643 public CodeableConcept getSpecialty() { 644 if (this.specialty == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create ReferralRequest.specialty"); 647 else if (Configuration.doAutoCreate()) 648 this.specialty = new CodeableConcept(); // cc 649 return this.specialty; 650 } 651 652 public boolean hasSpecialty() { 653 return this.specialty != null && !this.specialty.isEmpty(); 654 } 655 656 /** 657 * @param value {@link #specialty} (Indication of the clinical domain or 658 * discipline to which the referral or transfer of care request is 659 * sent. For example: Cardiology Gastroenterology Diabetology.) 660 */ 661 public ReferralRequest setSpecialty(CodeableConcept value) { 662 this.specialty = value; 663 return this; 664 } 665 666 /** 667 * @return {@link #priority} (An indication of the urgency of referral (or where 668 * applicable the type of transfer of care) request.) 669 */ 670 public CodeableConcept getPriority() { 671 if (this.priority == null) 672 if (Configuration.errorOnAutoCreate()) 673 throw new Error("Attempt to auto-create ReferralRequest.priority"); 674 else if (Configuration.doAutoCreate()) 675 this.priority = new CodeableConcept(); // cc 676 return this.priority; 677 } 678 679 public boolean hasPriority() { 680 return this.priority != null && !this.priority.isEmpty(); 681 } 682 683 /** 684 * @param value {@link #priority} (An indication of the urgency of referral (or 685 * where applicable the type of transfer of care) request.) 686 */ 687 public ReferralRequest setPriority(CodeableConcept value) { 688 this.priority = value; 689 return this; 690 } 691 692 /** 693 * @return {@link #patient} (The patient who is the subject of a referral or 694 * transfer of care request.) 695 */ 696 public Reference getPatient() { 697 if (this.patient == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create ReferralRequest.patient"); 700 else if (Configuration.doAutoCreate()) 701 this.patient = new Reference(); // cc 702 return this.patient; 703 } 704 705 public boolean hasPatient() { 706 return this.patient != null && !this.patient.isEmpty(); 707 } 708 709 /** 710 * @param value {@link #patient} (The patient who is the subject of a referral 711 * or transfer of care request.) 712 */ 713 public ReferralRequest setPatient(Reference value) { 714 this.patient = value; 715 return this; 716 } 717 718 /** 719 * @return {@link #patient} The actual object that is the target of the 720 * reference. The reference library doesn't populate this, but you can 721 * use it to hold the resource if you resolve it. (The patient who is 722 * the subject of a referral or transfer of care request.) 723 */ 724 public Patient getPatientTarget() { 725 if (this.patientTarget == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create ReferralRequest.patient"); 728 else if (Configuration.doAutoCreate()) 729 this.patientTarget = new Patient(); // aa 730 return this.patientTarget; 731 } 732 733 /** 734 * @param value {@link #patient} The actual object that is the target of the 735 * reference. The reference library doesn't use these, but you can 736 * use it to hold the resource if you resolve it. (The patient who 737 * is the subject of a referral or transfer of care request.) 738 */ 739 public ReferralRequest setPatientTarget(Patient value) { 740 this.patientTarget = value; 741 return this; 742 } 743 744 /** 745 * @return {@link #requester} (The healthcare provider or provider organization 746 * who/which initiated the referral/transfer of care request. Can also 747 * be Patient (a self referral).) 748 */ 749 public Reference getRequester() { 750 if (this.requester == null) 751 if (Configuration.errorOnAutoCreate()) 752 throw new Error("Attempt to auto-create ReferralRequest.requester"); 753 else if (Configuration.doAutoCreate()) 754 this.requester = new Reference(); // cc 755 return this.requester; 756 } 757 758 public boolean hasRequester() { 759 return this.requester != null && !this.requester.isEmpty(); 760 } 761 762 /** 763 * @param value {@link #requester} (The healthcare provider or provider 764 * organization who/which initiated the referral/transfer of care 765 * request. Can also be Patient (a self referral).) 766 */ 767 public ReferralRequest setRequester(Reference value) { 768 this.requester = value; 769 return this; 770 } 771 772 /** 773 * @return {@link #requester} The actual object that is the target of the 774 * reference. The reference library doesn't populate this, but you can 775 * use it to hold the resource if you resolve it. (The healthcare 776 * provider or provider organization who/which initiated the 777 * referral/transfer of care request. Can also be Patient (a self 778 * referral).) 779 */ 780 public Resource getRequesterTarget() { 781 return this.requesterTarget; 782 } 783 784 /** 785 * @param value {@link #requester} The actual object that is the target of the 786 * reference. The reference library doesn't use these, but you can 787 * use it to hold the resource if you resolve it. (The healthcare 788 * provider or provider organization who/which initiated the 789 * referral/transfer of care request. Can also be Patient (a self 790 * referral).) 791 */ 792 public ReferralRequest setRequesterTarget(Resource value) { 793 this.requesterTarget = value; 794 return this; 795 } 796 797 /** 798 * @return {@link #recipient} (The healthcare provider(s) or provider 799 * organization(s) who/which is to receive the referral/transfer of care 800 * request.) 801 */ 802 public List<Reference> getRecipient() { 803 if (this.recipient == null) 804 this.recipient = new ArrayList<Reference>(); 805 return this.recipient; 806 } 807 808 public boolean hasRecipient() { 809 if (this.recipient == null) 810 return false; 811 for (Reference item : this.recipient) 812 if (!item.isEmpty()) 813 return true; 814 return false; 815 } 816 817 /** 818 * @return {@link #recipient} (The healthcare provider(s) or provider 819 * organization(s) who/which is to receive the referral/transfer of care 820 * request.) 821 */ 822 // syntactic sugar 823 public Reference addRecipient() { // 3 824 Reference t = new Reference(); 825 if (this.recipient == null) 826 this.recipient = new ArrayList<Reference>(); 827 this.recipient.add(t); 828 return t; 829 } 830 831 // syntactic sugar 832 public ReferralRequest addRecipient(Reference t) { // 3 833 if (t == null) 834 return this; 835 if (this.recipient == null) 836 this.recipient = new ArrayList<Reference>(); 837 this.recipient.add(t); 838 return this; 839 } 840 841 /** 842 * @return {@link #recipient} (The actual objects that are the target of the 843 * reference. The reference library doesn't populate this, but you can 844 * use this to hold the resources if you resolvethemt. The healthcare 845 * provider(s) or provider organization(s) who/which is to receive the 846 * referral/transfer of care request.) 847 */ 848 public List<Resource> getRecipientTarget() { 849 if (this.recipientTarget == null) 850 this.recipientTarget = new ArrayList<Resource>(); 851 return this.recipientTarget; 852 } 853 854 /** 855 * @return {@link #encounter} (The encounter at which the request for referral 856 * or transfer of care is initiated.) 857 */ 858 public Reference getEncounter() { 859 if (this.encounter == null) 860 if (Configuration.errorOnAutoCreate()) 861 throw new Error("Attempt to auto-create ReferralRequest.encounter"); 862 else if (Configuration.doAutoCreate()) 863 this.encounter = new Reference(); // cc 864 return this.encounter; 865 } 866 867 public boolean hasEncounter() { 868 return this.encounter != null && !this.encounter.isEmpty(); 869 } 870 871 /** 872 * @param value {@link #encounter} (The encounter at which the request for 873 * referral or transfer of care is initiated.) 874 */ 875 public ReferralRequest setEncounter(Reference value) { 876 this.encounter = value; 877 return this; 878 } 879 880 /** 881 * @return {@link #encounter} The actual object that is the target of the 882 * reference. The reference library doesn't populate this, but you can 883 * use it to hold the resource if you resolve it. (The encounter at 884 * which the request for referral or transfer of care is initiated.) 885 */ 886 public Encounter getEncounterTarget() { 887 if (this.encounterTarget == null) 888 if (Configuration.errorOnAutoCreate()) 889 throw new Error("Attempt to auto-create ReferralRequest.encounter"); 890 else if (Configuration.doAutoCreate()) 891 this.encounterTarget = new Encounter(); // aa 892 return this.encounterTarget; 893 } 894 895 /** 896 * @param value {@link #encounter} The actual object that is the target of the 897 * reference. The reference library doesn't use these, but you can 898 * use it to hold the resource if you resolve it. (The encounter at 899 * which the request for referral or transfer of care is 900 * initiated.) 901 */ 902 public ReferralRequest setEncounterTarget(Encounter value) { 903 this.encounterTarget = value; 904 return this; 905 } 906 907 /** 908 * @return {@link #dateSent} (Date/DateTime the request for referral or transfer 909 * of care is sent by the author.). This is the underlying object with 910 * id, value and extensions. The accessor "getDateSent" gives direct 911 * access to the value 912 */ 913 public DateTimeType getDateSentElement() { 914 if (this.dateSent == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create ReferralRequest.dateSent"); 917 else if (Configuration.doAutoCreate()) 918 this.dateSent = new DateTimeType(); // bb 919 return this.dateSent; 920 } 921 922 public boolean hasDateSentElement() { 923 return this.dateSent != null && !this.dateSent.isEmpty(); 924 } 925 926 public boolean hasDateSent() { 927 return this.dateSent != null && !this.dateSent.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #dateSent} (Date/DateTime the request for referral or 932 * transfer of care is sent by the author.). This is the underlying 933 * object with id, value and extensions. The accessor "getDateSent" 934 * gives direct access to the value 935 */ 936 public ReferralRequest setDateSentElement(DateTimeType value) { 937 this.dateSent = value; 938 return this; 939 } 940 941 /** 942 * @return Date/DateTime the request for referral or transfer of care is sent by 943 * the author. 944 */ 945 public Date getDateSent() { 946 return this.dateSent == null ? null : this.dateSent.getValue(); 947 } 948 949 /** 950 * @param value Date/DateTime the request for referral or transfer of care is 951 * sent by the author. 952 */ 953 public ReferralRequest setDateSent(Date value) { 954 if (value == null) 955 this.dateSent = null; 956 else { 957 if (this.dateSent == null) 958 this.dateSent = new DateTimeType(); 959 this.dateSent.setValue(value); 960 } 961 return this; 962 } 963 964 /** 965 * @return {@link #reason} (Description of clinical condition indicating why 966 * referral/transfer of care is requested. For example: Pathological 967 * Anomalies, Disabled (physical or mental), Behavioral Management.) 968 */ 969 public CodeableConcept getReason() { 970 if (this.reason == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create ReferralRequest.reason"); 973 else if (Configuration.doAutoCreate()) 974 this.reason = new CodeableConcept(); // cc 975 return this.reason; 976 } 977 978 public boolean hasReason() { 979 return this.reason != null && !this.reason.isEmpty(); 980 } 981 982 /** 983 * @param value {@link #reason} (Description of clinical condition indicating 984 * why referral/transfer of care is requested. For example: 985 * Pathological Anomalies, Disabled (physical or mental), 986 * Behavioral Management.) 987 */ 988 public ReferralRequest setReason(CodeableConcept value) { 989 this.reason = value; 990 return this; 991 } 992 993 /** 994 * @return {@link #description} (The reason element gives a short description of 995 * why the referral is being made, the description expands on this to 996 * support a more complete clinical summary.). This is the underlying 997 * object with id, value and extensions. The accessor "getDescription" 998 * gives direct access to the value 999 */ 1000 public StringType getDescriptionElement() { 1001 if (this.description == null) 1002 if (Configuration.errorOnAutoCreate()) 1003 throw new Error("Attempt to auto-create ReferralRequest.description"); 1004 else if (Configuration.doAutoCreate()) 1005 this.description = new StringType(); // bb 1006 return this.description; 1007 } 1008 1009 public boolean hasDescriptionElement() { 1010 return this.description != null && !this.description.isEmpty(); 1011 } 1012 1013 public boolean hasDescription() { 1014 return this.description != null && !this.description.isEmpty(); 1015 } 1016 1017 /** 1018 * @param value {@link #description} (The reason element gives a short 1019 * description of why the referral is being made, the description 1020 * expands on this to support a more complete clinical summary.). 1021 * This is the underlying object with id, value and extensions. The 1022 * accessor "getDescription" gives direct access to the value 1023 */ 1024 public ReferralRequest setDescriptionElement(StringType value) { 1025 this.description = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return The reason element gives a short description of why the referral is 1031 * being made, the description expands on this to support a more 1032 * complete clinical summary. 1033 */ 1034 public String getDescription() { 1035 return this.description == null ? null : this.description.getValue(); 1036 } 1037 1038 /** 1039 * @param value The reason element gives a short description of why the referral 1040 * is being made, the description expands on this to support a more 1041 * complete clinical summary. 1042 */ 1043 public ReferralRequest setDescription(String value) { 1044 if (Utilities.noString(value)) 1045 this.description = null; 1046 else { 1047 if (this.description == null) 1048 this.description = new StringType(); 1049 this.description.setValue(value); 1050 } 1051 return this; 1052 } 1053 1054 /** 1055 * @return {@link #serviceRequested} (The service(s) that is/are requested to be 1056 * provided to the patient. For example: cardiac pacemaker insertion.) 1057 */ 1058 public List<CodeableConcept> getServiceRequested() { 1059 if (this.serviceRequested == null) 1060 this.serviceRequested = new ArrayList<CodeableConcept>(); 1061 return this.serviceRequested; 1062 } 1063 1064 public boolean hasServiceRequested() { 1065 if (this.serviceRequested == null) 1066 return false; 1067 for (CodeableConcept item : this.serviceRequested) 1068 if (!item.isEmpty()) 1069 return true; 1070 return false; 1071 } 1072 1073 /** 1074 * @return {@link #serviceRequested} (The service(s) that is/are requested to be 1075 * provided to the patient. For example: cardiac pacemaker insertion.) 1076 */ 1077 // syntactic sugar 1078 public CodeableConcept addServiceRequested() { // 3 1079 CodeableConcept t = new CodeableConcept(); 1080 if (this.serviceRequested == null) 1081 this.serviceRequested = new ArrayList<CodeableConcept>(); 1082 this.serviceRequested.add(t); 1083 return t; 1084 } 1085 1086 // syntactic sugar 1087 public ReferralRequest addServiceRequested(CodeableConcept t) { // 3 1088 if (t == null) 1089 return this; 1090 if (this.serviceRequested == null) 1091 this.serviceRequested = new ArrayList<CodeableConcept>(); 1092 this.serviceRequested.add(t); 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #supportingInformation} (Any additional (administrative, 1098 * financial or clinical) information required to support request for 1099 * referral or transfer of care. For example: Presenting problems/chief 1100 * complaints Medical History Family History Alerts Allergy/Intolerance 1101 * and Adverse Reactions Medications Observations/Assessments (may 1102 * include cognitive and fundtional assessments) Diagnostic Reports Care 1103 * Plan.) 1104 */ 1105 public List<Reference> getSupportingInformation() { 1106 if (this.supportingInformation == null) 1107 this.supportingInformation = new ArrayList<Reference>(); 1108 return this.supportingInformation; 1109 } 1110 1111 public boolean hasSupportingInformation() { 1112 if (this.supportingInformation == null) 1113 return false; 1114 for (Reference item : this.supportingInformation) 1115 if (!item.isEmpty()) 1116 return true; 1117 return false; 1118 } 1119 1120 /** 1121 * @return {@link #supportingInformation} (Any additional (administrative, 1122 * financial or clinical) information required to support request for 1123 * referral or transfer of care. For example: Presenting problems/chief 1124 * complaints Medical History Family History Alerts Allergy/Intolerance 1125 * and Adverse Reactions Medications Observations/Assessments (may 1126 * include cognitive and fundtional assessments) Diagnostic Reports Care 1127 * Plan.) 1128 */ 1129 // syntactic sugar 1130 public Reference addSupportingInformation() { // 3 1131 Reference t = new Reference(); 1132 if (this.supportingInformation == null) 1133 this.supportingInformation = new ArrayList<Reference>(); 1134 this.supportingInformation.add(t); 1135 return t; 1136 } 1137 1138 // syntactic sugar 1139 public ReferralRequest addSupportingInformation(Reference t) { // 3 1140 if (t == null) 1141 return this; 1142 if (this.supportingInformation == null) 1143 this.supportingInformation = new ArrayList<Reference>(); 1144 this.supportingInformation.add(t); 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #supportingInformation} (The actual objects that are the 1150 * target of the reference. The reference library doesn't populate this, 1151 * but you can use this to hold the resources if you resolvethemt. Any 1152 * additional (administrative, financial or clinical) information 1153 * required to support request for referral or transfer of care. For 1154 * example: Presenting problems/chief complaints Medical History Family 1155 * History Alerts Allergy/Intolerance and Adverse Reactions Medications 1156 * Observations/Assessments (may include cognitive and fundtional 1157 * assessments) Diagnostic Reports Care Plan.) 1158 */ 1159 public List<Resource> getSupportingInformationTarget() { 1160 if (this.supportingInformationTarget == null) 1161 this.supportingInformationTarget = new ArrayList<Resource>(); 1162 return this.supportingInformationTarget; 1163 } 1164 1165 /** 1166 * @return {@link #fulfillmentTime} (The period of time within which the 1167 * services identified in the referral/transfer of care is specified or 1168 * required to occur.) 1169 */ 1170 public Period getFulfillmentTime() { 1171 if (this.fulfillmentTime == null) 1172 if (Configuration.errorOnAutoCreate()) 1173 throw new Error("Attempt to auto-create ReferralRequest.fulfillmentTime"); 1174 else if (Configuration.doAutoCreate()) 1175 this.fulfillmentTime = new Period(); // cc 1176 return this.fulfillmentTime; 1177 } 1178 1179 public boolean hasFulfillmentTime() { 1180 return this.fulfillmentTime != null && !this.fulfillmentTime.isEmpty(); 1181 } 1182 1183 /** 1184 * @param value {@link #fulfillmentTime} (The period of time within which the 1185 * services identified in the referral/transfer of care is 1186 * specified or required to occur.) 1187 */ 1188 public ReferralRequest setFulfillmentTime(Period value) { 1189 this.fulfillmentTime = value; 1190 return this; 1191 } 1192 1193 protected void listChildren(List<Property> childrenList) { 1194 super.listChildren(childrenList); 1195 childrenList.add(new Property("status", "code", "The workflow status of the referral or transfer of care request.", 1196 0, java.lang.Integer.MAX_VALUE, status)); 1197 childrenList.add(new Property("identifier", "Identifier", 1198 "Business identifier that uniquely identifies the referral/care transfer request instance.", 0, 1199 java.lang.Integer.MAX_VALUE, identifier)); 1200 childrenList.add(new Property("date", "dateTime", 1201 "Date/DateTime of creation for draft requests and date of activation for active requests.", 0, 1202 java.lang.Integer.MAX_VALUE, date)); 1203 childrenList.add(new Property("type", "CodeableConcept", 1204 "An indication of the type of referral (or where applicable the type of transfer of care) request.", 0, 1205 java.lang.Integer.MAX_VALUE, type)); 1206 childrenList.add(new Property("specialty", "CodeableConcept", 1207 "Indication of the clinical domain or discipline to which the referral or transfer of care request is sent. For example: Cardiology Gastroenterology Diabetology.", 1208 0, java.lang.Integer.MAX_VALUE, specialty)); 1209 childrenList.add(new Property("priority", "CodeableConcept", 1210 "An indication of the urgency of referral (or where applicable the type of transfer of care) request.", 0, 1211 java.lang.Integer.MAX_VALUE, priority)); 1212 childrenList.add(new Property("patient", "Reference(Patient)", 1213 "The patient who is the subject of a referral or transfer of care request.", 0, java.lang.Integer.MAX_VALUE, 1214 patient)); 1215 childrenList.add(new Property("requester", "Reference(Practitioner|Organization|Patient)", 1216 "The healthcare provider or provider organization who/which initiated the referral/transfer of care request. Can also be Patient (a self referral).", 1217 0, java.lang.Integer.MAX_VALUE, requester)); 1218 childrenList.add(new Property("recipient", "Reference(Practitioner|Organization)", 1219 "The healthcare provider(s) or provider organization(s) who/which is to receive the referral/transfer of care request.", 1220 0, java.lang.Integer.MAX_VALUE, recipient)); 1221 childrenList.add(new Property("encounter", "Reference(Encounter)", 1222 "The encounter at which the request for referral or transfer of care is initiated.", 0, 1223 java.lang.Integer.MAX_VALUE, encounter)); 1224 childrenList.add(new Property("dateSent", "dateTime", 1225 "Date/DateTime the request for referral or transfer of care is sent by the author.", 0, 1226 java.lang.Integer.MAX_VALUE, dateSent)); 1227 childrenList.add(new Property("reason", "CodeableConcept", 1228 "Description of clinical condition indicating why referral/transfer of care is requested. For example: Pathological Anomalies, Disabled (physical or mental), Behavioral Management.", 1229 0, java.lang.Integer.MAX_VALUE, reason)); 1230 childrenList.add(new Property("description", "string", 1231 "The reason element gives a short description of why the referral is being made, the description expands on this to support a more complete clinical summary.", 1232 0, java.lang.Integer.MAX_VALUE, description)); 1233 childrenList.add(new Property("serviceRequested", "CodeableConcept", 1234 "The service(s) that is/are requested to be provided to the patient. For example: cardiac pacemaker insertion.", 1235 0, java.lang.Integer.MAX_VALUE, serviceRequested)); 1236 childrenList.add(new Property("supportingInformation", "Reference(Any)", 1237 "Any additional (administrative, financial or clinical) information required to support request for referral or transfer of care. For example: Presenting problems/chief complaints Medical History Family History Alerts Allergy/Intolerance and Adverse Reactions Medications Observations/Assessments (may include cognitive and fundtional assessments) Diagnostic Reports Care Plan.", 1238 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 1239 childrenList.add(new Property("fulfillmentTime", "Period", 1240 "The period of time within which the services identified in the referral/transfer of care is specified or required to occur.", 1241 0, java.lang.Integer.MAX_VALUE, fulfillmentTime)); 1242 } 1243 1244 @Override 1245 public void setProperty(String name, Base value) throws FHIRException { 1246 if (name.equals("status")) 1247 this.status = new ReferralStatusEnumFactory().fromType(value); // Enumeration<ReferralStatus> 1248 else if (name.equals("identifier")) 1249 this.getIdentifier().add(castToIdentifier(value)); 1250 else if (name.equals("date")) 1251 this.date = castToDateTime(value); // DateTimeType 1252 else if (name.equals("type")) 1253 this.type = castToCodeableConcept(value); // CodeableConcept 1254 else if (name.equals("specialty")) 1255 this.specialty = castToCodeableConcept(value); // CodeableConcept 1256 else if (name.equals("priority")) 1257 this.priority = castToCodeableConcept(value); // CodeableConcept 1258 else if (name.equals("patient")) 1259 this.patient = castToReference(value); // Reference 1260 else if (name.equals("requester")) 1261 this.requester = castToReference(value); // Reference 1262 else if (name.equals("recipient")) 1263 this.getRecipient().add(castToReference(value)); 1264 else if (name.equals("encounter")) 1265 this.encounter = castToReference(value); // Reference 1266 else if (name.equals("dateSent")) 1267 this.dateSent = castToDateTime(value); // DateTimeType 1268 else if (name.equals("reason")) 1269 this.reason = castToCodeableConcept(value); // CodeableConcept 1270 else if (name.equals("description")) 1271 this.description = castToString(value); // StringType 1272 else if (name.equals("serviceRequested")) 1273 this.getServiceRequested().add(castToCodeableConcept(value)); 1274 else if (name.equals("supportingInformation")) 1275 this.getSupportingInformation().add(castToReference(value)); 1276 else if (name.equals("fulfillmentTime")) 1277 this.fulfillmentTime = castToPeriod(value); // Period 1278 else 1279 super.setProperty(name, value); 1280 } 1281 1282 @Override 1283 public Base addChild(String name) throws FHIRException { 1284 if (name.equals("status")) { 1285 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.status"); 1286 } else if (name.equals("identifier")) { 1287 return addIdentifier(); 1288 } else if (name.equals("date")) { 1289 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.date"); 1290 } else if (name.equals("type")) { 1291 this.type = new CodeableConcept(); 1292 return this.type; 1293 } else if (name.equals("specialty")) { 1294 this.specialty = new CodeableConcept(); 1295 return this.specialty; 1296 } else if (name.equals("priority")) { 1297 this.priority = new CodeableConcept(); 1298 return this.priority; 1299 } else if (name.equals("patient")) { 1300 this.patient = new Reference(); 1301 return this.patient; 1302 } else if (name.equals("requester")) { 1303 this.requester = new Reference(); 1304 return this.requester; 1305 } else if (name.equals("recipient")) { 1306 return addRecipient(); 1307 } else if (name.equals("encounter")) { 1308 this.encounter = new Reference(); 1309 return this.encounter; 1310 } else if (name.equals("dateSent")) { 1311 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.dateSent"); 1312 } else if (name.equals("reason")) { 1313 this.reason = new CodeableConcept(); 1314 return this.reason; 1315 } else if (name.equals("description")) { 1316 throw new FHIRException("Cannot call addChild on a singleton property ReferralRequest.description"); 1317 } else if (name.equals("serviceRequested")) { 1318 return addServiceRequested(); 1319 } else if (name.equals("supportingInformation")) { 1320 return addSupportingInformation(); 1321 } else if (name.equals("fulfillmentTime")) { 1322 this.fulfillmentTime = new Period(); 1323 return this.fulfillmentTime; 1324 } else 1325 return super.addChild(name); 1326 } 1327 1328 public String fhirType() { 1329 return "ReferralRequest"; 1330 1331 } 1332 1333 public ReferralRequest copy() { 1334 ReferralRequest dst = new ReferralRequest(); 1335 copyValues(dst); 1336 dst.status = status == null ? null : status.copy(); 1337 if (identifier != null) { 1338 dst.identifier = new ArrayList<Identifier>(); 1339 for (Identifier i : identifier) 1340 dst.identifier.add(i.copy()); 1341 } 1342 ; 1343 dst.date = date == null ? null : date.copy(); 1344 dst.type = type == null ? null : type.copy(); 1345 dst.specialty = specialty == null ? null : specialty.copy(); 1346 dst.priority = priority == null ? null : priority.copy(); 1347 dst.patient = patient == null ? null : patient.copy(); 1348 dst.requester = requester == null ? null : requester.copy(); 1349 if (recipient != null) { 1350 dst.recipient = new ArrayList<Reference>(); 1351 for (Reference i : recipient) 1352 dst.recipient.add(i.copy()); 1353 } 1354 ; 1355 dst.encounter = encounter == null ? null : encounter.copy(); 1356 dst.dateSent = dateSent == null ? null : dateSent.copy(); 1357 dst.reason = reason == null ? null : reason.copy(); 1358 dst.description = description == null ? null : description.copy(); 1359 if (serviceRequested != null) { 1360 dst.serviceRequested = new ArrayList<CodeableConcept>(); 1361 for (CodeableConcept i : serviceRequested) 1362 dst.serviceRequested.add(i.copy()); 1363 } 1364 ; 1365 if (supportingInformation != null) { 1366 dst.supportingInformation = new ArrayList<Reference>(); 1367 for (Reference i : supportingInformation) 1368 dst.supportingInformation.add(i.copy()); 1369 } 1370 ; 1371 dst.fulfillmentTime = fulfillmentTime == null ? null : fulfillmentTime.copy(); 1372 return dst; 1373 } 1374 1375 protected ReferralRequest typedCopy() { 1376 return copy(); 1377 } 1378 1379 @Override 1380 public boolean equalsDeep(Base other) { 1381 if (!super.equalsDeep(other)) 1382 return false; 1383 if (!(other instanceof ReferralRequest)) 1384 return false; 1385 ReferralRequest o = (ReferralRequest) other; 1386 return compareDeep(status, o.status, true) && compareDeep(identifier, o.identifier, true) 1387 && compareDeep(date, o.date, true) && compareDeep(type, o.type, true) 1388 && compareDeep(specialty, o.specialty, true) && compareDeep(priority, o.priority, true) 1389 && compareDeep(patient, o.patient, true) && compareDeep(requester, o.requester, true) 1390 && compareDeep(recipient, o.recipient, true) && compareDeep(encounter, o.encounter, true) 1391 && compareDeep(dateSent, o.dateSent, true) && compareDeep(reason, o.reason, true) 1392 && compareDeep(description, o.description, true) && compareDeep(serviceRequested, o.serviceRequested, true) 1393 && compareDeep(supportingInformation, o.supportingInformation, true) 1394 && compareDeep(fulfillmentTime, o.fulfillmentTime, true); 1395 } 1396 1397 @Override 1398 public boolean equalsShallow(Base other) { 1399 if (!super.equalsShallow(other)) 1400 return false; 1401 if (!(other instanceof ReferralRequest)) 1402 return false; 1403 ReferralRequest o = (ReferralRequest) other; 1404 return compareValues(status, o.status, true) && compareValues(date, o.date, true) 1405 && compareValues(dateSent, o.dateSent, true) && compareValues(description, o.description, true); 1406 } 1407 1408 public boolean isEmpty() { 1409 return super.isEmpty() && (status == null || status.isEmpty()) && (identifier == null || identifier.isEmpty()) 1410 && (date == null || date.isEmpty()) && (type == null || type.isEmpty()) 1411 && (specialty == null || specialty.isEmpty()) && (priority == null || priority.isEmpty()) 1412 && (patient == null || patient.isEmpty()) && (requester == null || requester.isEmpty()) 1413 && (recipient == null || recipient.isEmpty()) && (encounter == null || encounter.isEmpty()) 1414 && (dateSent == null || dateSent.isEmpty()) && (reason == null || reason.isEmpty()) 1415 && (description == null || description.isEmpty()) && (serviceRequested == null || serviceRequested.isEmpty()) 1416 && (supportingInformation == null || supportingInformation.isEmpty()) 1417 && (fulfillmentTime == null || fulfillmentTime.isEmpty()); 1418 } 1419 1420 @Override 1421 public ResourceType getResourceType() { 1422 return ResourceType.ReferralRequest; 1423 } 1424 1425 @SearchParamDefinition(name = "date", path = "ReferralRequest.date", description = "Creation or activation date", type = "date") 1426 public static final String SP_DATE = "date"; 1427 @SearchParamDefinition(name = "requester", path = "ReferralRequest.requester", description = "Requester of referral / transfer of care", type = "reference") 1428 public static final String SP_REQUESTER = "requester"; 1429 @SearchParamDefinition(name = "specialty", path = "ReferralRequest.specialty", description = "The specialty that the referral is for", type = "token") 1430 public static final String SP_SPECIALTY = "specialty"; 1431 @SearchParamDefinition(name = "patient", path = "ReferralRequest.patient", description = "Who the referral is about", type = "reference") 1432 public static final String SP_PATIENT = "patient"; 1433 @SearchParamDefinition(name = "recipient", path = "ReferralRequest.recipient", description = "The person that the referral was sent to", type = "reference") 1434 public static final String SP_RECIPIENT = "recipient"; 1435 @SearchParamDefinition(name = "type", path = "ReferralRequest.type", description = "The type of the referral", type = "token") 1436 public static final String SP_TYPE = "type"; 1437 @SearchParamDefinition(name = "priority", path = "ReferralRequest.priority", description = "The priority assigned to the referral", type = "token") 1438 public static final String SP_PRIORITY = "priority"; 1439 @SearchParamDefinition(name = "status", path = "ReferralRequest.status", description = "The status of the referral", type = "token") 1440 public static final String SP_STATUS = "status"; 1441 1442}