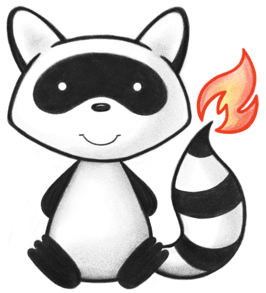
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender; 038import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * Information about a person that is involved in the care for a patient, but 047 * who is not the target of healthcare, nor has a formal responsibility in the 048 * care process. 049 */ 050@ResourceDef(name = "RelatedPerson", profile = "http://hl7.org/fhir/Profile/RelatedPerson") 051public class RelatedPerson extends DomainResource { 052 053 /** 054 * Identifier for a person within a particular scope. 055 */ 056 @Child(name = "identifier", type = { 057 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 058 @Description(shortDefinition = "A human identifier for this person", formalDefinition = "Identifier for a person within a particular scope.") 059 protected List<Identifier> identifier; 060 061 /** 062 * The patient this person is related to. 063 */ 064 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 065 @Description(shortDefinition = "The patient this person is related to", formalDefinition = "The patient this person is related to.") 066 protected Reference patient; 067 068 /** 069 * The actual object that is the target of the reference (The patient this 070 * person is related to.) 071 */ 072 protected Patient patientTarget; 073 074 /** 075 * The nature of the relationship between a patient and the related person. 076 */ 077 @Child(name = "relationship", type = { 078 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 079 @Description(shortDefinition = "The nature of the relationship", formalDefinition = "The nature of the relationship between a patient and the related person.") 080 protected CodeableConcept relationship; 081 082 /** 083 * A name associated with the person. 084 */ 085 @Child(name = "name", type = { HumanName.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 086 @Description(shortDefinition = "A name associated with the person", formalDefinition = "A name associated with the person.") 087 protected HumanName name; 088 089 /** 090 * A contact detail for the person, e.g. a telephone number or an email address. 091 */ 092 @Child(name = "telecom", type = { 093 ContactPoint.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 094 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 095 protected List<ContactPoint> telecom; 096 097 /** 098 * Administrative Gender - the gender that the person is considered to have for 099 * administration and record keeping purposes. 100 */ 101 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 102 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.") 103 protected Enumeration<AdministrativeGender> gender; 104 105 /** 106 * The date on which the related person was born. 107 */ 108 @Child(name = "birthDate", type = { DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 109 @Description(shortDefinition = "The date on which the related person was born", formalDefinition = "The date on which the related person was born.") 110 protected DateType birthDate; 111 112 /** 113 * Address where the related person can be contacted or visited. 114 */ 115 @Child(name = "address", type = { 116 Address.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 117 @Description(shortDefinition = "Address where the related person can be contacted or visited", formalDefinition = "Address where the related person can be contacted or visited.") 118 protected List<Address> address; 119 120 /** 121 * Image of the person. 122 */ 123 @Child(name = "photo", type = { 124 Attachment.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 125 @Description(shortDefinition = "Image of the person", formalDefinition = "Image of the person.") 126 protected List<Attachment> photo; 127 128 /** 129 * The period of time that this relationship is considered to be valid. If there 130 * are no dates defined, then the interval is unknown. 131 */ 132 @Child(name = "period", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 133 @Description(shortDefinition = "Period of time that this relationship is considered valid", formalDefinition = "The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown.") 134 protected Period period; 135 136 private static final long serialVersionUID = 7777543L; 137 138 /* 139 * Constructor 140 */ 141 public RelatedPerson() { 142 super(); 143 } 144 145 /* 146 * Constructor 147 */ 148 public RelatedPerson(Reference patient) { 149 super(); 150 this.patient = patient; 151 } 152 153 /** 154 * @return {@link #identifier} (Identifier for a person within a particular 155 * scope.) 156 */ 157 public List<Identifier> getIdentifier() { 158 if (this.identifier == null) 159 this.identifier = new ArrayList<Identifier>(); 160 return this.identifier; 161 } 162 163 public boolean hasIdentifier() { 164 if (this.identifier == null) 165 return false; 166 for (Identifier item : this.identifier) 167 if (!item.isEmpty()) 168 return true; 169 return false; 170 } 171 172 /** 173 * @return {@link #identifier} (Identifier for a person within a particular 174 * scope.) 175 */ 176 // syntactic sugar 177 public Identifier addIdentifier() { // 3 178 Identifier t = new Identifier(); 179 if (this.identifier == null) 180 this.identifier = new ArrayList<Identifier>(); 181 this.identifier.add(t); 182 return t; 183 } 184 185 // syntactic sugar 186 public RelatedPerson addIdentifier(Identifier t) { // 3 187 if (t == null) 188 return this; 189 if (this.identifier == null) 190 this.identifier = new ArrayList<Identifier>(); 191 this.identifier.add(t); 192 return this; 193 } 194 195 /** 196 * @return {@link #patient} (The patient this person is related to.) 197 */ 198 public Reference getPatient() { 199 if (this.patient == null) 200 if (Configuration.errorOnAutoCreate()) 201 throw new Error("Attempt to auto-create RelatedPerson.patient"); 202 else if (Configuration.doAutoCreate()) 203 this.patient = new Reference(); // cc 204 return this.patient; 205 } 206 207 public boolean hasPatient() { 208 return this.patient != null && !this.patient.isEmpty(); 209 } 210 211 /** 212 * @param value {@link #patient} (The patient this person is related to.) 213 */ 214 public RelatedPerson setPatient(Reference value) { 215 this.patient = value; 216 return this; 217 } 218 219 /** 220 * @return {@link #patient} The actual object that is the target of the 221 * reference. The reference library doesn't populate this, but you can 222 * use it to hold the resource if you resolve it. (The patient this 223 * person is related to.) 224 */ 225 public Patient getPatientTarget() { 226 if (this.patientTarget == null) 227 if (Configuration.errorOnAutoCreate()) 228 throw new Error("Attempt to auto-create RelatedPerson.patient"); 229 else if (Configuration.doAutoCreate()) 230 this.patientTarget = new Patient(); // aa 231 return this.patientTarget; 232 } 233 234 /** 235 * @param value {@link #patient} The actual object that is the target of the 236 * reference. The reference library doesn't use these, but you can 237 * use it to hold the resource if you resolve it. (The patient this 238 * person is related to.) 239 */ 240 public RelatedPerson setPatientTarget(Patient value) { 241 this.patientTarget = value; 242 return this; 243 } 244 245 /** 246 * @return {@link #relationship} (The nature of the relationship between a 247 * patient and the related person.) 248 */ 249 public CodeableConcept getRelationship() { 250 if (this.relationship == null) 251 if (Configuration.errorOnAutoCreate()) 252 throw new Error("Attempt to auto-create RelatedPerson.relationship"); 253 else if (Configuration.doAutoCreate()) 254 this.relationship = new CodeableConcept(); // cc 255 return this.relationship; 256 } 257 258 public boolean hasRelationship() { 259 return this.relationship != null && !this.relationship.isEmpty(); 260 } 261 262 /** 263 * @param value {@link #relationship} (The nature of the relationship between a 264 * patient and the related person.) 265 */ 266 public RelatedPerson setRelationship(CodeableConcept value) { 267 this.relationship = value; 268 return this; 269 } 270 271 /** 272 * @return {@link #name} (A name associated with the person.) 273 */ 274 public HumanName getName() { 275 if (this.name == null) 276 if (Configuration.errorOnAutoCreate()) 277 throw new Error("Attempt to auto-create RelatedPerson.name"); 278 else if (Configuration.doAutoCreate()) 279 this.name = new HumanName(); // cc 280 return this.name; 281 } 282 283 public boolean hasName() { 284 return this.name != null && !this.name.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #name} (A name associated with the person.) 289 */ 290 public RelatedPerson setName(HumanName value) { 291 this.name = value; 292 return this; 293 } 294 295 /** 296 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 297 * number or an email address.) 298 */ 299 public List<ContactPoint> getTelecom() { 300 if (this.telecom == null) 301 this.telecom = new ArrayList<ContactPoint>(); 302 return this.telecom; 303 } 304 305 public boolean hasTelecom() { 306 if (this.telecom == null) 307 return false; 308 for (ContactPoint item : this.telecom) 309 if (!item.isEmpty()) 310 return true; 311 return false; 312 } 313 314 /** 315 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 316 * number or an email address.) 317 */ 318 // syntactic sugar 319 public ContactPoint addTelecom() { // 3 320 ContactPoint t = new ContactPoint(); 321 if (this.telecom == null) 322 this.telecom = new ArrayList<ContactPoint>(); 323 this.telecom.add(t); 324 return t; 325 } 326 327 // syntactic sugar 328 public RelatedPerson addTelecom(ContactPoint t) { // 3 329 if (t == null) 330 return this; 331 if (this.telecom == null) 332 this.telecom = new ArrayList<ContactPoint>(); 333 this.telecom.add(t); 334 return this; 335 } 336 337 /** 338 * @return {@link #gender} (Administrative Gender - the gender that the person 339 * is considered to have for administration and record keeping 340 * purposes.). This is the underlying object with id, value and 341 * extensions. The accessor "getGender" gives direct access to the value 342 */ 343 public Enumeration<AdministrativeGender> getGenderElement() { 344 if (this.gender == null) 345 if (Configuration.errorOnAutoCreate()) 346 throw new Error("Attempt to auto-create RelatedPerson.gender"); 347 else if (Configuration.doAutoCreate()) 348 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 349 return this.gender; 350 } 351 352 public boolean hasGenderElement() { 353 return this.gender != null && !this.gender.isEmpty(); 354 } 355 356 public boolean hasGender() { 357 return this.gender != null && !this.gender.isEmpty(); 358 } 359 360 /** 361 * @param value {@link #gender} (Administrative Gender - the gender that the 362 * person is considered to have for administration and record 363 * keeping purposes.). This is the underlying object with id, value 364 * and extensions. The accessor "getGender" gives direct access to 365 * the value 366 */ 367 public RelatedPerson setGenderElement(Enumeration<AdministrativeGender> value) { 368 this.gender = value; 369 return this; 370 } 371 372 /** 373 * @return Administrative Gender - the gender that the person is considered to 374 * have for administration and record keeping purposes. 375 */ 376 public AdministrativeGender getGender() { 377 return this.gender == null ? null : this.gender.getValue(); 378 } 379 380 /** 381 * @param value Administrative Gender - the gender that the person is considered 382 * to have for administration and record keeping purposes. 383 */ 384 public RelatedPerson setGender(AdministrativeGender value) { 385 if (value == null) 386 this.gender = null; 387 else { 388 if (this.gender == null) 389 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 390 this.gender.setValue(value); 391 } 392 return this; 393 } 394 395 /** 396 * @return {@link #birthDate} (The date on which the related person was born.). 397 * This is the underlying object with id, value and extensions. The 398 * accessor "getBirthDate" gives direct access to the value 399 */ 400 public DateType getBirthDateElement() { 401 if (this.birthDate == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create RelatedPerson.birthDate"); 404 else if (Configuration.doAutoCreate()) 405 this.birthDate = new DateType(); // bb 406 return this.birthDate; 407 } 408 409 public boolean hasBirthDateElement() { 410 return this.birthDate != null && !this.birthDate.isEmpty(); 411 } 412 413 public boolean hasBirthDate() { 414 return this.birthDate != null && !this.birthDate.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #birthDate} (The date on which the related person was 419 * born.). This is the underlying object with id, value and 420 * extensions. The accessor "getBirthDate" gives direct access to 421 * the value 422 */ 423 public RelatedPerson setBirthDateElement(DateType value) { 424 this.birthDate = value; 425 return this; 426 } 427 428 /** 429 * @return The date on which the related person was born. 430 */ 431 public Date getBirthDate() { 432 return this.birthDate == null ? null : this.birthDate.getValue(); 433 } 434 435 /** 436 * @param value The date on which the related person was born. 437 */ 438 public RelatedPerson setBirthDate(Date value) { 439 if (value == null) 440 this.birthDate = null; 441 else { 442 if (this.birthDate == null) 443 this.birthDate = new DateType(); 444 this.birthDate.setValue(value); 445 } 446 return this; 447 } 448 449 /** 450 * @return {@link #address} (Address where the related person can be contacted 451 * or visited.) 452 */ 453 public List<Address> getAddress() { 454 if (this.address == null) 455 this.address = new ArrayList<Address>(); 456 return this.address; 457 } 458 459 public boolean hasAddress() { 460 if (this.address == null) 461 return false; 462 for (Address item : this.address) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 /** 469 * @return {@link #address} (Address where the related person can be contacted 470 * or visited.) 471 */ 472 // syntactic sugar 473 public Address addAddress() { // 3 474 Address t = new Address(); 475 if (this.address == null) 476 this.address = new ArrayList<Address>(); 477 this.address.add(t); 478 return t; 479 } 480 481 // syntactic sugar 482 public RelatedPerson addAddress(Address t) { // 3 483 if (t == null) 484 return this; 485 if (this.address == null) 486 this.address = new ArrayList<Address>(); 487 this.address.add(t); 488 return this; 489 } 490 491 /** 492 * @return {@link #photo} (Image of the person.) 493 */ 494 public List<Attachment> getPhoto() { 495 if (this.photo == null) 496 this.photo = new ArrayList<Attachment>(); 497 return this.photo; 498 } 499 500 public boolean hasPhoto() { 501 if (this.photo == null) 502 return false; 503 for (Attachment item : this.photo) 504 if (!item.isEmpty()) 505 return true; 506 return false; 507 } 508 509 /** 510 * @return {@link #photo} (Image of the person.) 511 */ 512 // syntactic sugar 513 public Attachment addPhoto() { // 3 514 Attachment t = new Attachment(); 515 if (this.photo == null) 516 this.photo = new ArrayList<Attachment>(); 517 this.photo.add(t); 518 return t; 519 } 520 521 // syntactic sugar 522 public RelatedPerson addPhoto(Attachment t) { // 3 523 if (t == null) 524 return this; 525 if (this.photo == null) 526 this.photo = new ArrayList<Attachment>(); 527 this.photo.add(t); 528 return this; 529 } 530 531 /** 532 * @return {@link #period} (The period of time that this relationship is 533 * considered to be valid. If there are no dates defined, then the 534 * interval is unknown.) 535 */ 536 public Period getPeriod() { 537 if (this.period == null) 538 if (Configuration.errorOnAutoCreate()) 539 throw new Error("Attempt to auto-create RelatedPerson.period"); 540 else if (Configuration.doAutoCreate()) 541 this.period = new Period(); // cc 542 return this.period; 543 } 544 545 public boolean hasPeriod() { 546 return this.period != null && !this.period.isEmpty(); 547 } 548 549 /** 550 * @param value {@link #period} (The period of time that this relationship is 551 * considered to be valid. If there are no dates defined, then the 552 * interval is unknown.) 553 */ 554 public RelatedPerson setPeriod(Period value) { 555 this.period = value; 556 return this; 557 } 558 559 protected void listChildren(List<Property> childrenList) { 560 super.listChildren(childrenList); 561 childrenList.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, 562 java.lang.Integer.MAX_VALUE, identifier)); 563 childrenList.add(new Property("patient", "Reference(Patient)", "The patient this person is related to.", 0, 564 java.lang.Integer.MAX_VALUE, patient)); 565 childrenList.add(new Property("relationship", "CodeableConcept", 566 "The nature of the relationship between a patient and the related person.", 0, java.lang.Integer.MAX_VALUE, 567 relationship)); 568 childrenList.add( 569 new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 570 childrenList.add(new Property("telecom", "ContactPoint", 571 "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, 572 telecom)); 573 childrenList.add(new Property("gender", "code", 574 "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 575 0, java.lang.Integer.MAX_VALUE, gender)); 576 childrenList.add(new Property("birthDate", "date", "The date on which the related person was born.", 0, 577 java.lang.Integer.MAX_VALUE, birthDate)); 578 childrenList.add(new Property("address", "Address", "Address where the related person can be contacted or visited.", 579 0, java.lang.Integer.MAX_VALUE, address)); 580 childrenList 581 .add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 582 childrenList.add(new Property("period", "Period", 583 "The period of time that this relationship is considered to be valid. If there are no dates defined, then the interval is unknown.", 584 0, java.lang.Integer.MAX_VALUE, period)); 585 } 586 587 @Override 588 public void setProperty(String name, Base value) throws FHIRException { 589 if (name.equals("identifier")) 590 this.getIdentifier().add(castToIdentifier(value)); 591 else if (name.equals("patient")) 592 this.patient = castToReference(value); // Reference 593 else if (name.equals("relationship")) 594 this.relationship = castToCodeableConcept(value); // CodeableConcept 595 else if (name.equals("name")) 596 this.name = castToHumanName(value); // HumanName 597 else if (name.equals("telecom")) 598 this.getTelecom().add(castToContactPoint(value)); 599 else if (name.equals("gender")) 600 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 601 else if (name.equals("birthDate")) 602 this.birthDate = castToDate(value); // DateType 603 else if (name.equals("address")) 604 this.getAddress().add(castToAddress(value)); 605 else if (name.equals("photo")) 606 this.getPhoto().add(castToAttachment(value)); 607 else if (name.equals("period")) 608 this.period = castToPeriod(value); // Period 609 else 610 super.setProperty(name, value); 611 } 612 613 @Override 614 public Base addChild(String name) throws FHIRException { 615 if (name.equals("identifier")) { 616 return addIdentifier(); 617 } else if (name.equals("patient")) { 618 this.patient = new Reference(); 619 return this.patient; 620 } else if (name.equals("relationship")) { 621 this.relationship = new CodeableConcept(); 622 return this.relationship; 623 } else if (name.equals("name")) { 624 this.name = new HumanName(); 625 return this.name; 626 } else if (name.equals("telecom")) { 627 return addTelecom(); 628 } else if (name.equals("gender")) { 629 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.gender"); 630 } else if (name.equals("birthDate")) { 631 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.birthDate"); 632 } else if (name.equals("address")) { 633 return addAddress(); 634 } else if (name.equals("photo")) { 635 return addPhoto(); 636 } else if (name.equals("period")) { 637 this.period = new Period(); 638 return this.period; 639 } else 640 return super.addChild(name); 641 } 642 643 public String fhirType() { 644 return "RelatedPerson"; 645 646 } 647 648 public RelatedPerson copy() { 649 RelatedPerson dst = new RelatedPerson(); 650 copyValues(dst); 651 if (identifier != null) { 652 dst.identifier = new ArrayList<Identifier>(); 653 for (Identifier i : identifier) 654 dst.identifier.add(i.copy()); 655 } 656 ; 657 dst.patient = patient == null ? null : patient.copy(); 658 dst.relationship = relationship == null ? null : relationship.copy(); 659 dst.name = name == null ? null : name.copy(); 660 if (telecom != null) { 661 dst.telecom = new ArrayList<ContactPoint>(); 662 for (ContactPoint i : telecom) 663 dst.telecom.add(i.copy()); 664 } 665 ; 666 dst.gender = gender == null ? null : gender.copy(); 667 dst.birthDate = birthDate == null ? null : birthDate.copy(); 668 if (address != null) { 669 dst.address = new ArrayList<Address>(); 670 for (Address i : address) 671 dst.address.add(i.copy()); 672 } 673 ; 674 if (photo != null) { 675 dst.photo = new ArrayList<Attachment>(); 676 for (Attachment i : photo) 677 dst.photo.add(i.copy()); 678 } 679 ; 680 dst.period = period == null ? null : period.copy(); 681 return dst; 682 } 683 684 protected RelatedPerson typedCopy() { 685 return copy(); 686 } 687 688 @Override 689 public boolean equalsDeep(Base other) { 690 if (!super.equalsDeep(other)) 691 return false; 692 if (!(other instanceof RelatedPerson)) 693 return false; 694 RelatedPerson o = (RelatedPerson) other; 695 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) 696 && compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) 697 && compareDeep(telecom, o.telecom, true) && compareDeep(gender, o.gender, true) 698 && compareDeep(birthDate, o.birthDate, true) && compareDeep(address, o.address, true) 699 && compareDeep(photo, o.photo, true) && compareDeep(period, o.period, true); 700 } 701 702 @Override 703 public boolean equalsShallow(Base other) { 704 if (!super.equalsShallow(other)) 705 return false; 706 if (!(other instanceof RelatedPerson)) 707 return false; 708 RelatedPerson o = (RelatedPerson) other; 709 return compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true); 710 } 711 712 public boolean isEmpty() { 713 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (patient == null || patient.isEmpty()) 714 && (relationship == null || relationship.isEmpty()) && (name == null || name.isEmpty()) 715 && (telecom == null || telecom.isEmpty()) && (gender == null || gender.isEmpty()) 716 && (birthDate == null || birthDate.isEmpty()) && (address == null || address.isEmpty()) 717 && (photo == null || photo.isEmpty()) && (period == null || period.isEmpty()); 718 } 719 720 @Override 721 public ResourceType getResourceType() { 722 return ResourceType.RelatedPerson; 723 } 724 725 @SearchParamDefinition(name = "identifier", path = "RelatedPerson.identifier", description = "A patient Identifier", type = "token") 726 public static final String SP_IDENTIFIER = "identifier"; 727 @SearchParamDefinition(name = "address", path = "RelatedPerson.address", description = "An address in any kind of address/part", type = "string") 728 public static final String SP_ADDRESS = "address"; 729 @SearchParamDefinition(name = "birthdate", path = "RelatedPerson.birthDate", description = "The Related Person's date of birth", type = "date") 730 public static final String SP_BIRTHDATE = "birthdate"; 731 @SearchParamDefinition(name = "address-state", path = "RelatedPerson.address.state", description = "A state specified in an address", type = "string") 732 public static final String SP_ADDRESSSTATE = "address-state"; 733 @SearchParamDefinition(name = "gender", path = "RelatedPerson.gender", description = "Gender of the person", type = "token") 734 public static final String SP_GENDER = "gender"; 735 @SearchParamDefinition(name = "address-postalcode", path = "RelatedPerson.address.postalCode", description = "A postal code specified in an address", type = "string") 736 public static final String SP_ADDRESSPOSTALCODE = "address-postalcode"; 737 @SearchParamDefinition(name = "address-country", path = "RelatedPerson.address.country", description = "A country specified in an address", type = "string") 738 public static final String SP_ADDRESSCOUNTRY = "address-country"; 739 @SearchParamDefinition(name = "phonetic", path = "RelatedPerson.name", description = "A portion of name using some kind of phonetic matching algorithm", type = "string") 740 public static final String SP_PHONETIC = "phonetic"; 741 @SearchParamDefinition(name = "phone", path = "RelatedPerson.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 742 public static final String SP_PHONE = "phone"; 743 @SearchParamDefinition(name = "patient", path = "RelatedPerson.patient", description = "The patient this person is related to", type = "reference") 744 public static final String SP_PATIENT = "patient"; 745 @SearchParamDefinition(name = "name", path = "RelatedPerson.name", description = "A portion of name in any name part", type = "string") 746 public static final String SP_NAME = "name"; 747 @SearchParamDefinition(name = "address-use", path = "RelatedPerson.address.use", description = "A use code specified in an address", type = "token") 748 public static final String SP_ADDRESSUSE = "address-use"; 749 @SearchParamDefinition(name = "telecom", path = "RelatedPerson.telecom", description = "The value in any kind of contact", type = "token") 750 public static final String SP_TELECOM = "telecom"; 751 @SearchParamDefinition(name = "address-city", path = "RelatedPerson.address.city", description = "A city specified in an address", type = "string") 752 public static final String SP_ADDRESSCITY = "address-city"; 753 @SearchParamDefinition(name = "email", path = "RelatedPerson.telecom.where(system='email')", description = "A value in an email contact", type = "token") 754 public static final String SP_EMAIL = "email"; 755 756}