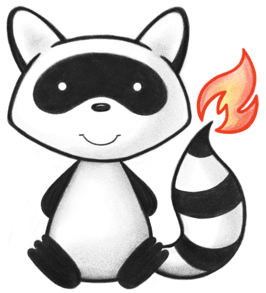
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.Description; 037import org.hl7.fhir.instance.model.api.IAnyResource; 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.utilities.Utilities; 040 041/** 042 * This is the base resource type for everything. 043 */ 044public abstract class Resource extends BaseResource implements IAnyResource { 045 046 /** 047 * The logical id of the resource, as used in the URL for the resource. Once 048 * assigned, this value never changes. 049 */ 050 @Child(name = "id", type = { IdType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 051 @Description(shortDefinition = "Logical id of this artifact", formalDefinition = "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.") 052 protected IdType id; 053 054 /** 055 * The metadata about the resource. This is content that is maintained by the 056 * infrastructure. Changes to the content may not always be associated with 057 * version changes to the resource. 058 */ 059 @Child(name = "meta", type = { Meta.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "Metadata about the resource", formalDefinition = "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource.") 061 protected Meta meta; 062 063 /** 064 * A reference to a set of rules that were followed when the resource was 065 * constructed, and which must be understood when processing the content. 066 */ 067 @Child(name = "implicitRules", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 068 @Description(shortDefinition = "A set of rules under which this content was created", formalDefinition = "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content.") 069 protected UriType implicitRules; 070 071 /** 072 * The base language in which the resource is written. 073 */ 074 @Child(name = "language", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 075 @Description(shortDefinition = "Language of the resource content", formalDefinition = "The base language in which the resource is written.") 076 protected CodeType language; 077 078 private static final long serialVersionUID = -559462759L; 079 080 /* 081 * Constructor 082 */ 083 public Resource() { 084 super(); 085 } 086 087 /** 088 * @return {@link #id} (The logical id of the resource, as used in the URL for 089 * the resource. Once assigned, this value never changes.). This is the 090 * underlying object with id, value and extensions. The accessor "getId" 091 * gives direct access to the value 092 */ 093 public IdType getIdElement() { 094 if (this.id == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create Resource.id"); 097 else if (Configuration.doAutoCreate()) 098 this.id = new IdType(); // bb 099 return this.id; 100 } 101 102 public boolean hasIdElement() { 103 return this.id != null && !this.id.isEmpty(); 104 } 105 106 public boolean hasId() { 107 return this.id != null && !this.id.isEmpty(); 108 } 109 110 /** 111 * @param value {@link #id} (The logical id of the resource, as used in the URL 112 * for the resource. Once assigned, this value never changes.). 113 * This is the underlying object with id, value and extensions. The 114 * accessor "getId" gives direct access to the value 115 */ 116 public Resource setIdElement(IdType value) { 117 this.id = value; 118 return this; 119 } 120 121 /** 122 * @return The logical id of the resource, as used in the URL for the resource. 123 * Once assigned, this value never changes. 124 */ 125 public String getId() { 126 return this.id == null ? null : this.id.getValue(); 127 } 128 129 /** 130 * @param value The logical id of the resource, as used in the URL for the 131 * resource. Once assigned, this value never changes. 132 */ 133 public Resource setId(String value) { 134 if (Utilities.noString(value)) 135 this.id = null; 136 else { 137 if (this.id == null) 138 this.id = new IdType(); 139 this.id.setValue(value); 140 } 141 return this; 142 } 143 144 /** 145 * @return {@link #meta} (The metadata about the resource. This is content that 146 * is maintained by the infrastructure. Changes to the content may not 147 * always be associated with version changes to the resource.) 148 */ 149 public Meta getMeta() { 150 if (this.meta == null) 151 if (Configuration.errorOnAutoCreate()) 152 throw new Error("Attempt to auto-create Resource.meta"); 153 else if (Configuration.doAutoCreate()) 154 this.meta = new Meta(); // cc 155 return this.meta; 156 } 157 158 public boolean hasMeta() { 159 return this.meta != null && !this.meta.isEmpty(); 160 } 161 162 /** 163 * @param value {@link #meta} (The metadata about the resource. This is content 164 * that is maintained by the infrastructure. Changes to the content 165 * may not always be associated with version changes to the 166 * resource.) 167 */ 168 public Resource setMeta(Meta value) { 169 this.meta = value; 170 return this; 171 } 172 173 /** 174 * @return {@link #implicitRules} (A reference to a set of rules that were 175 * followed when the resource was constructed, and which must be 176 * understood when processing the content.). This is the underlying 177 * object with id, value and extensions. The accessor "getImplicitRules" 178 * gives direct access to the value 179 */ 180 public UriType getImplicitRulesElement() { 181 if (this.implicitRules == null) 182 if (Configuration.errorOnAutoCreate()) 183 throw new Error("Attempt to auto-create Resource.implicitRules"); 184 else if (Configuration.doAutoCreate()) 185 this.implicitRules = new UriType(); // bb 186 return this.implicitRules; 187 } 188 189 public boolean hasImplicitRulesElement() { 190 return this.implicitRules != null && !this.implicitRules.isEmpty(); 191 } 192 193 public boolean hasImplicitRules() { 194 return this.implicitRules != null && !this.implicitRules.isEmpty(); 195 } 196 197 /** 198 * @param value {@link #implicitRules} (A reference to a set of rules that were 199 * followed when the resource was constructed, and which must be 200 * understood when processing the content.). This is the underlying 201 * object with id, value and extensions. The accessor 202 * "getImplicitRules" gives direct access to the value 203 */ 204 public Resource setImplicitRulesElement(UriType value) { 205 this.implicitRules = value; 206 return this; 207 } 208 209 /** 210 * @return A reference to a set of rules that were followed when the resource 211 * was constructed, and which must be understood when processing the 212 * content. 213 */ 214 public String getImplicitRules() { 215 return this.implicitRules == null ? null : this.implicitRules.getValue(); 216 } 217 218 /** 219 * @param value A reference to a set of rules that were followed when the 220 * resource was constructed, and which must be understood when 221 * processing the content. 222 */ 223 public Resource setImplicitRules(String value) { 224 if (Utilities.noString(value)) 225 this.implicitRules = null; 226 else { 227 if (this.implicitRules == null) 228 this.implicitRules = new UriType(); 229 this.implicitRules.setValue(value); 230 } 231 return this; 232 } 233 234 /** 235 * @return {@link #language} (The base language in which the resource is 236 * written.). This is the underlying object with id, value and 237 * extensions. The accessor "getLanguage" gives direct access to the 238 * value 239 */ 240 public CodeType getLanguageElement() { 241 if (this.language == null) 242 if (Configuration.errorOnAutoCreate()) 243 throw new Error("Attempt to auto-create Resource.language"); 244 else if (Configuration.doAutoCreate()) 245 this.language = new CodeType(); // bb 246 return this.language; 247 } 248 249 public boolean hasLanguageElement() { 250 return this.language != null && !this.language.isEmpty(); 251 } 252 253 public boolean hasLanguage() { 254 return this.language != null && !this.language.isEmpty(); 255 } 256 257 /** 258 * @param value {@link #language} (The base language in which the resource is 259 * written.). This is the underlying object with id, value and 260 * extensions. The accessor "getLanguage" gives direct access to 261 * the value 262 */ 263 public Resource setLanguageElement(CodeType value) { 264 this.language = value; 265 return this; 266 } 267 268 /** 269 * @return The base language in which the resource is written. 270 */ 271 public String getLanguage() { 272 return this.language == null ? null : this.language.getValue(); 273 } 274 275 /** 276 * @param value The base language in which the resource is written. 277 */ 278 public Resource setLanguage(String value) { 279 if (Utilities.noString(value)) 280 this.language = null; 281 else { 282 if (this.language == null) 283 this.language = new CodeType(); 284 this.language.setValue(value); 285 } 286 return this; 287 } 288 289 protected void listChildren(List<Property> childrenList) { 290 childrenList.add(new Property("id", "id", 291 "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.", 292 0, java.lang.Integer.MAX_VALUE, id)); 293 childrenList.add(new Property("meta", "Meta", 294 "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource.", 295 0, java.lang.Integer.MAX_VALUE, meta)); 296 childrenList.add(new Property("implicitRules", "uri", 297 "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content.", 298 0, java.lang.Integer.MAX_VALUE, implicitRules)); 299 childrenList.add(new Property("language", "code", "The base language in which the resource is written.", 0, 300 java.lang.Integer.MAX_VALUE, language)); 301 } 302 303 @Override 304 public void setProperty(String name, Base value) throws FHIRException { 305 if (name.equals("id")) 306 this.id = castToId(value); // IdType 307 else if (name.equals("meta")) 308 this.meta = castToMeta(value); // Meta 309 else if (name.equals("implicitRules")) 310 this.implicitRules = castToUri(value); // UriType 311 else if (name.equals("language")) 312 this.language = castToCode(value); // CodeType 313 else 314 super.setProperty(name, value); 315 } 316 317 @Override 318 public Base addChild(String name) throws FHIRException { 319 if (name.equals("id")) { 320 throw new FHIRException("Cannot call addChild on a singleton property Resource.id"); 321 } else if (name.equals("meta")) { 322 this.meta = new Meta(); 323 return this.meta; 324 } else if (name.equals("implicitRules")) { 325 throw new FHIRException("Cannot call addChild on a singleton property Resource.implicitRules"); 326 } else if (name.equals("language")) { 327 throw new FHIRException("Cannot call addChild on a singleton property Resource.language"); 328 } else 329 return super.addChild(name); 330 } 331 332 public String fhirType() { 333 return "Resource"; 334 335 } 336 337 public abstract Resource copy(); 338 339 public void copyValues(Resource dst) { 340 dst.id = id == null ? null : id.copy(); 341 dst.meta = meta == null ? null : meta.copy(); 342 dst.implicitRules = implicitRules == null ? null : implicitRules.copy(); 343 dst.language = language == null ? null : language.copy(); 344 } 345 346 @Override 347 public boolean equalsDeep(Base other) { 348 if (!super.equalsDeep(other)) 349 return false; 350 if (!(other instanceof Resource)) 351 return false; 352 Resource o = (Resource) other; 353 return compareDeep(id, o.id, true) && compareDeep(meta, o.meta, true) 354 && compareDeep(implicitRules, o.implicitRules, true) && compareDeep(language, o.language, true); 355 } 356 357 @Override 358 public boolean equalsShallow(Base other) { 359 if (!super.equalsShallow(other)) 360 return false; 361 if (!(other instanceof Resource)) 362 return false; 363 Resource o = (Resource) other; 364 return compareValues(id, o.id, true) && compareValues(implicitRules, o.implicitRules, true) 365 && compareValues(language, o.language, true); 366 } 367 368 public boolean isEmpty() { 369 return super.isEmpty() && (id == null || id.isEmpty()) && (meta == null || meta.isEmpty()) 370 && (implicitRules == null || implicitRules.isEmpty()) && (language == null || language.isEmpty()); 371 } 372 373 public abstract ResourceType getResourceType(); 374 375}