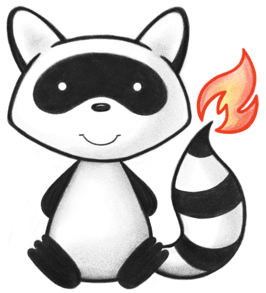
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public class ResourceFactory extends Factory { 037 038 public static Resource createResource(String name) throws FHIRException { 039 if ("Appointment".equals(name)) 040 return new Appointment(); 041 if ("ReferralRequest".equals(name)) 042 return new ReferralRequest(); 043 if ("Account".equals(name)) 044 return new Account(); 045 if ("Provenance".equals(name)) 046 return new Provenance(); 047 if ("Questionnaire".equals(name)) 048 return new Questionnaire(); 049 if ("ExplanationOfBenefit".equals(name)) 050 return new ExplanationOfBenefit(); 051 if ("DocumentManifest".equals(name)) 052 return new DocumentManifest(); 053 if ("Specimen".equals(name)) 054 return new Specimen(); 055 if ("AllergyIntolerance".equals(name)) 056 return new AllergyIntolerance(); 057 if ("CarePlan".equals(name)) 058 return new CarePlan(); 059 if ("Goal".equals(name)) 060 return new Goal(); 061 if ("StructureDefinition".equals(name)) 062 return new StructureDefinition(); 063 if ("EnrollmentRequest".equals(name)) 064 return new EnrollmentRequest(); 065 if ("EpisodeOfCare".equals(name)) 066 return new EpisodeOfCare(); 067 if ("OperationOutcome".equals(name)) 068 return new OperationOutcome(); 069 if ("Medication".equals(name)) 070 return new Medication(); 071 if ("Procedure".equals(name)) 072 return new Procedure(); 073 if ("List".equals(name)) 074 return new List_(); 075 if ("ConceptMap".equals(name)) 076 return new ConceptMap(); 077 if ("Subscription".equals(name)) 078 return new Subscription(); 079 if ("ValueSet".equals(name)) 080 return new ValueSet(); 081 if ("OperationDefinition".equals(name)) 082 return new OperationDefinition(); 083 if ("DocumentReference".equals(name)) 084 return new DocumentReference(); 085 if ("Order".equals(name)) 086 return new Order(); 087 if ("Immunization".equals(name)) 088 return new Immunization(); 089 if ("Parameters".equals(name)) 090 return new Parameters(); 091 if ("Device".equals(name)) 092 return new Device(); 093 if ("VisionPrescription".equals(name)) 094 return new VisionPrescription(); 095 if ("Media".equals(name)) 096 return new Media(); 097 if ("Conformance".equals(name)) 098 return new Conformance(); 099 if ("ProcedureRequest".equals(name)) 100 return new ProcedureRequest(); 101 if ("EligibilityResponse".equals(name)) 102 return new EligibilityResponse(); 103 if ("DeviceUseRequest".equals(name)) 104 return new DeviceUseRequest(); 105 if ("DeviceMetric".equals(name)) 106 return new DeviceMetric(); 107 if ("Flag".equals(name)) 108 return new Flag(); 109 if ("RelatedPerson".equals(name)) 110 return new RelatedPerson(); 111 if ("SupplyRequest".equals(name)) 112 return new SupplyRequest(); 113 if ("Practitioner".equals(name)) 114 return new Practitioner(); 115 if ("AppointmentResponse".equals(name)) 116 return new AppointmentResponse(); 117 if ("Observation".equals(name)) 118 return new Observation(); 119 if ("MedicationAdministration".equals(name)) 120 return new MedicationAdministration(); 121 if ("Slot".equals(name)) 122 return new Slot(); 123 if ("EnrollmentResponse".equals(name)) 124 return new EnrollmentResponse(); 125 if ("Binary".equals(name)) 126 return new Binary(); 127 if ("MedicationStatement".equals(name)) 128 return new MedicationStatement(); 129 if ("Person".equals(name)) 130 return new Person(); 131 if ("Contract".equals(name)) 132 return new Contract(); 133 if ("CommunicationRequest".equals(name)) 134 return new CommunicationRequest(); 135 if ("RiskAssessment".equals(name)) 136 return new RiskAssessment(); 137 if ("TestScript".equals(name)) 138 return new TestScript(); 139 if ("Basic".equals(name)) 140 return new Basic(); 141 if ("Group".equals(name)) 142 return new Group(); 143 if ("PaymentNotice".equals(name)) 144 return new PaymentNotice(); 145 if ("Organization".equals(name)) 146 return new Organization(); 147 if ("ImplementationGuide".equals(name)) 148 return new ImplementationGuide(); 149 if ("ClaimResponse".equals(name)) 150 return new ClaimResponse(); 151 if ("EligibilityRequest".equals(name)) 152 return new EligibilityRequest(); 153 if ("ProcessRequest".equals(name)) 154 return new ProcessRequest(); 155 if ("MedicationDispense".equals(name)) 156 return new MedicationDispense(); 157 if ("DiagnosticReport".equals(name)) 158 return new DiagnosticReport(); 159 if ("ImagingStudy".equals(name)) 160 return new ImagingStudy(); 161 if ("ImagingObjectSelection".equals(name)) 162 return new ImagingObjectSelection(); 163 if ("HealthcareService".equals(name)) 164 return new HealthcareService(); 165 if ("DataElement".equals(name)) 166 return new DataElement(); 167 if ("DeviceComponent".equals(name)) 168 return new DeviceComponent(); 169 if ("FamilyMemberHistory".equals(name)) 170 return new FamilyMemberHistory(); 171 if ("NutritionOrder".equals(name)) 172 return new NutritionOrder(); 173 if ("Encounter".equals(name)) 174 return new Encounter(); 175 if ("Substance".equals(name)) 176 return new Substance(); 177 if ("AuditEvent".equals(name)) 178 return new AuditEvent(); 179 if ("MedicationOrder".equals(name)) 180 return new MedicationOrder(); 181 if ("SearchParameter".equals(name)) 182 return new SearchParameter(); 183 if ("PaymentReconciliation".equals(name)) 184 return new PaymentReconciliation(); 185 if ("Communication".equals(name)) 186 return new Communication(); 187 if ("Condition".equals(name)) 188 return new Condition(); 189 if ("Composition".equals(name)) 190 return new Composition(); 191 if ("DetectedIssue".equals(name)) 192 return new DetectedIssue(); 193 if ("Bundle".equals(name)) 194 return new Bundle(); 195 if ("DiagnosticOrder".equals(name)) 196 return new DiagnosticOrder(); 197 if ("Patient".equals(name)) 198 return new Patient(); 199 if ("OrderResponse".equals(name)) 200 return new OrderResponse(); 201 if ("Coverage".equals(name)) 202 return new Coverage(); 203 if ("QuestionnaireResponse".equals(name)) 204 return new QuestionnaireResponse(); 205 if ("DeviceUseStatement".equals(name)) 206 return new DeviceUseStatement(); 207 if ("ProcessResponse".equals(name)) 208 return new ProcessResponse(); 209 if ("NamingSystem".equals(name)) 210 return new NamingSystem(); 211 if ("Schedule".equals(name)) 212 return new Schedule(); 213 if ("SupplyDelivery".equals(name)) 214 return new SupplyDelivery(); 215 if ("ClinicalImpression".equals(name)) 216 return new ClinicalImpression(); 217 if ("MessageHeader".equals(name)) 218 return new MessageHeader(); 219 if ("Claim".equals(name)) 220 return new Claim(); 221 if ("ImmunizationRecommendation".equals(name)) 222 return new ImmunizationRecommendation(); 223 if ("Location".equals(name)) 224 return new Location(); 225 if ("BodySite".equals(name)) 226 return new BodySite(); 227 else 228 throw new FHIRException("Unknown Resource Name '" + name + "'"); 229 } 230 231 public static Element createType(String name) throws FHIRException { 232 if ("Meta".equals(name)) 233 return new Meta(); 234 if ("Address".equals(name)) 235 return new Address(); 236 if ("Attachment".equals(name)) 237 return new Attachment(); 238 if ("Count".equals(name)) 239 return new Count(); 240 if ("Money".equals(name)) 241 return new Money(); 242 if ("HumanName".equals(name)) 243 return new HumanName(); 244 if ("ContactPoint".equals(name)) 245 return new ContactPoint(); 246 if ("Identifier".equals(name)) 247 return new Identifier(); 248 if ("Narrative".equals(name)) 249 return new Narrative(); 250 if ("Coding".equals(name)) 251 return new Coding(); 252 if ("SampledData".equals(name)) 253 return new SampledData(); 254 if ("Ratio".equals(name)) 255 return new Ratio(); 256 if ("ElementDefinition".equals(name)) 257 return new ElementDefinition(); 258 if ("Distance".equals(name)) 259 return new Distance(); 260 if ("Age".equals(name)) 261 return new Age(); 262 if ("Reference".equals(name)) 263 return new Reference(); 264 if ("SimpleQuantity".equals(name)) 265 return new SimpleQuantity(); 266 if ("Quantity".equals(name)) 267 return new Quantity(); 268 if ("Period".equals(name)) 269 return new Period(); 270 if ("Duration".equals(name)) 271 return new Duration(); 272 if ("Range".equals(name)) 273 return new Range(); 274 if ("Annotation".equals(name)) 275 return new Annotation(); 276 if ("Extension".equals(name)) 277 return new Extension(); 278 if ("Signature".equals(name)) 279 return new Signature(); 280 if ("Timing".equals(name)) 281 return new Timing(); 282 if ("CodeableConcept".equals(name)) 283 return new CodeableConcept(); 284 else 285 throw new FHIRException("Unknown Type Name '" + name + "'"); 286 } 287 288}