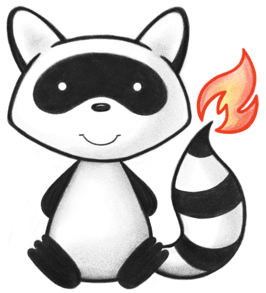
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * An assessment of the likely outcome(s) for a patient or other subject as well 050 * as the likelihood of each outcome. 051 */ 052@ResourceDef(name = "RiskAssessment", profile = "http://hl7.org/fhir/Profile/RiskAssessment") 053public class RiskAssessment extends DomainResource { 054 055 @Block() 056 public static class RiskAssessmentPredictionComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * One of the potential outcomes for the patient (e.g. remission, death, a 059 * particular condition). 060 */ 061 @Child(name = "outcome", type = { 062 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 063 @Description(shortDefinition = "Possible outcome for the subject", formalDefinition = "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).") 064 protected CodeableConcept outcome; 065 066 /** 067 * How likely is the outcome (in the specified timeframe). 068 */ 069 @Child(name = "probability", type = { DecimalType.class, Range.class, 070 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 071 @Description(shortDefinition = "Likelihood of specified outcome", formalDefinition = "How likely is the outcome (in the specified timeframe).") 072 protected Type probability; 073 074 /** 075 * Indicates the risk for this particular subject (with their specific 076 * characteristics) divided by the risk of the population in general. (Numbers 077 * greater than 1 = higher risk than the population, numbers less than 1 = lower 078 * risk.). 079 */ 080 @Child(name = "relativeRisk", type = { 081 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 082 @Description(shortDefinition = "Relative likelihood", formalDefinition = "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).") 083 protected DecimalType relativeRisk; 084 085 /** 086 * Indicates the period of time or age range of the subject to which the 087 * specified probability applies. 088 */ 089 @Child(name = "when", type = { Period.class, 090 Range.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 091 @Description(shortDefinition = "Timeframe or age range", formalDefinition = "Indicates the period of time or age range of the subject to which the specified probability applies.") 092 protected Type when; 093 094 /** 095 * Additional information explaining the basis for the prediction. 096 */ 097 @Child(name = "rationale", type = { 098 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 099 @Description(shortDefinition = "Explanation of prediction", formalDefinition = "Additional information explaining the basis for the prediction.") 100 protected StringType rationale; 101 102 private static final long serialVersionUID = 647967428L; 103 104 /* 105 * Constructor 106 */ 107 public RiskAssessmentPredictionComponent() { 108 super(); 109 } 110 111 /* 112 * Constructor 113 */ 114 public RiskAssessmentPredictionComponent(CodeableConcept outcome) { 115 super(); 116 this.outcome = outcome; 117 } 118 119 /** 120 * @return {@link #outcome} (One of the potential outcomes for the patient (e.g. 121 * remission, death, a particular condition).) 122 */ 123 public CodeableConcept getOutcome() { 124 if (this.outcome == null) 125 if (Configuration.errorOnAutoCreate()) 126 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.outcome"); 127 else if (Configuration.doAutoCreate()) 128 this.outcome = new CodeableConcept(); // cc 129 return this.outcome; 130 } 131 132 public boolean hasOutcome() { 133 return this.outcome != null && !this.outcome.isEmpty(); 134 } 135 136 /** 137 * @param value {@link #outcome} (One of the potential outcomes for the patient 138 * (e.g. remission, death, a particular condition).) 139 */ 140 public RiskAssessmentPredictionComponent setOutcome(CodeableConcept value) { 141 this.outcome = value; 142 return this; 143 } 144 145 /** 146 * @return {@link #probability} (How likely is the outcome (in the specified 147 * timeframe).) 148 */ 149 public Type getProbability() { 150 return this.probability; 151 } 152 153 /** 154 * @return {@link #probability} (How likely is the outcome (in the specified 155 * timeframe).) 156 */ 157 public DecimalType getProbabilityDecimalType() throws FHIRException { 158 if (!(this.probability instanceof DecimalType)) 159 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 160 + this.probability.getClass().getName() + " was encountered"); 161 return (DecimalType) this.probability; 162 } 163 164 public boolean hasProbabilityDecimalType() { 165 return this.probability instanceof DecimalType; 166 } 167 168 /** 169 * @return {@link #probability} (How likely is the outcome (in the specified 170 * timeframe).) 171 */ 172 public Range getProbabilityRange() throws FHIRException { 173 if (!(this.probability instanceof Range)) 174 throw new FHIRException("Type mismatch: the type Range was expected, but " 175 + this.probability.getClass().getName() + " was encountered"); 176 return (Range) this.probability; 177 } 178 179 public boolean hasProbabilityRange() { 180 return this.probability instanceof Range; 181 } 182 183 /** 184 * @return {@link #probability} (How likely is the outcome (in the specified 185 * timeframe).) 186 */ 187 public CodeableConcept getProbabilityCodeableConcept() throws FHIRException { 188 if (!(this.probability instanceof CodeableConcept)) 189 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 190 + this.probability.getClass().getName() + " was encountered"); 191 return (CodeableConcept) this.probability; 192 } 193 194 public boolean hasProbabilityCodeableConcept() { 195 return this.probability instanceof CodeableConcept; 196 } 197 198 public boolean hasProbability() { 199 return this.probability != null && !this.probability.isEmpty(); 200 } 201 202 /** 203 * @param value {@link #probability} (How likely is the outcome (in the 204 * specified timeframe).) 205 */ 206 public RiskAssessmentPredictionComponent setProbability(Type value) { 207 this.probability = value; 208 return this; 209 } 210 211 /** 212 * @return {@link #relativeRisk} (Indicates the risk for this particular subject 213 * (with their specific characteristics) divided by the risk of the 214 * population in general. (Numbers greater than 1 = higher risk than the 215 * population, numbers less than 1 = lower risk.).). This is the 216 * underlying object with id, value and extensions. The accessor 217 * "getRelativeRisk" gives direct access to the value 218 */ 219 public DecimalType getRelativeRiskElement() { 220 if (this.relativeRisk == null) 221 if (Configuration.errorOnAutoCreate()) 222 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.relativeRisk"); 223 else if (Configuration.doAutoCreate()) 224 this.relativeRisk = new DecimalType(); // bb 225 return this.relativeRisk; 226 } 227 228 public boolean hasRelativeRiskElement() { 229 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 230 } 231 232 public boolean hasRelativeRisk() { 233 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 234 } 235 236 /** 237 * @param value {@link #relativeRisk} (Indicates the risk for this particular 238 * subject (with their specific characteristics) divided by the 239 * risk of the population in general. (Numbers greater than 1 = 240 * higher risk than the population, numbers less than 1 = lower 241 * risk.).). This is the underlying object with id, value and 242 * extensions. The accessor "getRelativeRisk" gives direct access 243 * to the value 244 */ 245 public RiskAssessmentPredictionComponent setRelativeRiskElement(DecimalType value) { 246 this.relativeRisk = value; 247 return this; 248 } 249 250 /** 251 * @return Indicates the risk for this particular subject (with their specific 252 * characteristics) divided by the risk of the population in general. 253 * (Numbers greater than 1 = higher risk than the population, numbers 254 * less than 1 = lower risk.). 255 */ 256 public BigDecimal getRelativeRisk() { 257 return this.relativeRisk == null ? null : this.relativeRisk.getValue(); 258 } 259 260 /** 261 * @param value Indicates the risk for this particular subject (with their 262 * specific characteristics) divided by the risk of the population 263 * in general. (Numbers greater than 1 = higher risk than the 264 * population, numbers less than 1 = lower risk.). 265 */ 266 public RiskAssessmentPredictionComponent setRelativeRisk(BigDecimal value) { 267 if (value == null) 268 this.relativeRisk = null; 269 else { 270 if (this.relativeRisk == null) 271 this.relativeRisk = new DecimalType(); 272 this.relativeRisk.setValue(value); 273 } 274 return this; 275 } 276 277 /** 278 * @return {@link #when} (Indicates the period of time or age range of the 279 * subject to which the specified probability applies.) 280 */ 281 public Type getWhen() { 282 return this.when; 283 } 284 285 /** 286 * @return {@link #when} (Indicates the period of time or age range of the 287 * subject to which the specified probability applies.) 288 */ 289 public Period getWhenPeriod() throws FHIRException { 290 if (!(this.when instanceof Period)) 291 throw new FHIRException( 292 "Type mismatch: the type Period was expected, but " + this.when.getClass().getName() + " was encountered"); 293 return (Period) this.when; 294 } 295 296 public boolean hasWhenPeriod() { 297 return this.when instanceof Period; 298 } 299 300 /** 301 * @return {@link #when} (Indicates the period of time or age range of the 302 * subject to which the specified probability applies.) 303 */ 304 public Range getWhenRange() throws FHIRException { 305 if (!(this.when instanceof Range)) 306 throw new FHIRException( 307 "Type mismatch: the type Range was expected, but " + this.when.getClass().getName() + " was encountered"); 308 return (Range) this.when; 309 } 310 311 public boolean hasWhenRange() { 312 return this.when instanceof Range; 313 } 314 315 public boolean hasWhen() { 316 return this.when != null && !this.when.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #when} (Indicates the period of time or age range of the 321 * subject to which the specified probability applies.) 322 */ 323 public RiskAssessmentPredictionComponent setWhen(Type value) { 324 this.when = value; 325 return this; 326 } 327 328 /** 329 * @return {@link #rationale} (Additional information explaining the basis for 330 * the prediction.). This is the underlying object with id, value and 331 * extensions. The accessor "getRationale" gives direct access to the 332 * value 333 */ 334 public StringType getRationaleElement() { 335 if (this.rationale == null) 336 if (Configuration.errorOnAutoCreate()) 337 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.rationale"); 338 else if (Configuration.doAutoCreate()) 339 this.rationale = new StringType(); // bb 340 return this.rationale; 341 } 342 343 public boolean hasRationaleElement() { 344 return this.rationale != null && !this.rationale.isEmpty(); 345 } 346 347 public boolean hasRationale() { 348 return this.rationale != null && !this.rationale.isEmpty(); 349 } 350 351 /** 352 * @param value {@link #rationale} (Additional information explaining the basis 353 * for the prediction.). This is the underlying object with id, 354 * value and extensions. The accessor "getRationale" gives direct 355 * access to the value 356 */ 357 public RiskAssessmentPredictionComponent setRationaleElement(StringType value) { 358 this.rationale = value; 359 return this; 360 } 361 362 /** 363 * @return Additional information explaining the basis for the prediction. 364 */ 365 public String getRationale() { 366 return this.rationale == null ? null : this.rationale.getValue(); 367 } 368 369 /** 370 * @param value Additional information explaining the basis for the prediction. 371 */ 372 public RiskAssessmentPredictionComponent setRationale(String value) { 373 if (Utilities.noString(value)) 374 this.rationale = null; 375 else { 376 if (this.rationale == null) 377 this.rationale = new StringType(); 378 this.rationale.setValue(value); 379 } 380 return this; 381 } 382 383 protected void listChildren(List<Property> childrenList) { 384 super.listChildren(childrenList); 385 childrenList.add(new Property("outcome", "CodeableConcept", 386 "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 387 java.lang.Integer.MAX_VALUE, outcome)); 388 childrenList.add(new Property("probability[x]", "decimal|Range|CodeableConcept", 389 "How likely is the outcome (in the specified timeframe).", 0, java.lang.Integer.MAX_VALUE, probability)); 390 childrenList.add(new Property("relativeRisk", "decimal", 391 "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 392 0, java.lang.Integer.MAX_VALUE, relativeRisk)); 393 childrenList.add(new Property("when[x]", "Period|Range", 394 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 395 java.lang.Integer.MAX_VALUE, when)); 396 childrenList 397 .add(new Property("rationale", "string", "Additional information explaining the basis for the prediction.", 0, 398 java.lang.Integer.MAX_VALUE, rationale)); 399 } 400 401 @Override 402 public void setProperty(String name, Base value) throws FHIRException { 403 if (name.equals("outcome")) 404 this.outcome = castToCodeableConcept(value); // CodeableConcept 405 else if (name.equals("probability[x]")) 406 this.probability = (Type) value; // Type 407 else if (name.equals("relativeRisk")) 408 this.relativeRisk = castToDecimal(value); // DecimalType 409 else if (name.equals("when[x]")) 410 this.when = (Type) value; // Type 411 else if (name.equals("rationale")) 412 this.rationale = castToString(value); // StringType 413 else 414 super.setProperty(name, value); 415 } 416 417 @Override 418 public Base addChild(String name) throws FHIRException { 419 if (name.equals("outcome")) { 420 this.outcome = new CodeableConcept(); 421 return this.outcome; 422 } else if (name.equals("probabilityDecimal")) { 423 this.probability = new DecimalType(); 424 return this.probability; 425 } else if (name.equals("probabilityRange")) { 426 this.probability = new Range(); 427 return this.probability; 428 } else if (name.equals("probabilityCodeableConcept")) { 429 this.probability = new CodeableConcept(); 430 return this.probability; 431 } else if (name.equals("relativeRisk")) { 432 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.relativeRisk"); 433 } else if (name.equals("whenPeriod")) { 434 this.when = new Period(); 435 return this.when; 436 } else if (name.equals("whenRange")) { 437 this.when = new Range(); 438 return this.when; 439 } else if (name.equals("rationale")) { 440 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.rationale"); 441 } else 442 return super.addChild(name); 443 } 444 445 public RiskAssessmentPredictionComponent copy() { 446 RiskAssessmentPredictionComponent dst = new RiskAssessmentPredictionComponent(); 447 copyValues(dst); 448 dst.outcome = outcome == null ? null : outcome.copy(); 449 dst.probability = probability == null ? null : probability.copy(); 450 dst.relativeRisk = relativeRisk == null ? null : relativeRisk.copy(); 451 dst.when = when == null ? null : when.copy(); 452 dst.rationale = rationale == null ? null : rationale.copy(); 453 return dst; 454 } 455 456 @Override 457 public boolean equalsDeep(Base other) { 458 if (!super.equalsDeep(other)) 459 return false; 460 if (!(other instanceof RiskAssessmentPredictionComponent)) 461 return false; 462 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other; 463 return compareDeep(outcome, o.outcome, true) && compareDeep(probability, o.probability, true) 464 && compareDeep(relativeRisk, o.relativeRisk, true) && compareDeep(when, o.when, true) 465 && compareDeep(rationale, o.rationale, true); 466 } 467 468 @Override 469 public boolean equalsShallow(Base other) { 470 if (!super.equalsShallow(other)) 471 return false; 472 if (!(other instanceof RiskAssessmentPredictionComponent)) 473 return false; 474 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other; 475 return compareValues(relativeRisk, o.relativeRisk, true) && compareValues(rationale, o.rationale, true); 476 } 477 478 public boolean isEmpty() { 479 return super.isEmpty() && (outcome == null || outcome.isEmpty()) && (probability == null || probability.isEmpty()) 480 && (relativeRisk == null || relativeRisk.isEmpty()) && (when == null || when.isEmpty()) 481 && (rationale == null || rationale.isEmpty()); 482 } 483 484 public String fhirType() { 485 return "RiskAssessment.prediction"; 486 487 } 488 489 } 490 491 /** 492 * The patient or group the risk assessment applies to. 493 */ 494 @Child(name = "subject", type = { Patient.class, 495 Group.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 496 @Description(shortDefinition = "Who/what does assessment apply to?", formalDefinition = "The patient or group the risk assessment applies to.") 497 protected Reference subject; 498 499 /** 500 * The actual object that is the target of the reference (The patient or group 501 * the risk assessment applies to.) 502 */ 503 protected Resource subjectTarget; 504 505 /** 506 * The date (and possibly time) the risk assessment was performed. 507 */ 508 @Child(name = "date", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 509 @Description(shortDefinition = "When was assessment made?", formalDefinition = "The date (and possibly time) the risk assessment was performed.") 510 protected DateTimeType date; 511 512 /** 513 * For assessments or prognosis specific to a particular condition, indicates 514 * the condition being assessed. 515 */ 516 @Child(name = "condition", type = { Condition.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 517 @Description(shortDefinition = "Condition assessed", formalDefinition = "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.") 518 protected Reference condition; 519 520 /** 521 * The actual object that is the target of the reference (For assessments or 522 * prognosis specific to a particular condition, indicates the condition being 523 * assessed.) 524 */ 525 protected Condition conditionTarget; 526 527 /** 528 * The encounter where the assessment was performed. 529 */ 530 @Child(name = "encounter", type = { Encounter.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 531 @Description(shortDefinition = "Where was assessment performed?", formalDefinition = "The encounter where the assessment was performed.") 532 protected Reference encounter; 533 534 /** 535 * The actual object that is the target of the reference (The encounter where 536 * the assessment was performed.) 537 */ 538 protected Encounter encounterTarget; 539 540 /** 541 * The provider or software application that performed the assessment. 542 */ 543 @Child(name = "performer", type = { Practitioner.class, 544 Device.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 545 @Description(shortDefinition = "Who did assessment?", formalDefinition = "The provider or software application that performed the assessment.") 546 protected Reference performer; 547 548 /** 549 * The actual object that is the target of the reference (The provider or 550 * software application that performed the assessment.) 551 */ 552 protected Resource performerTarget; 553 554 /** 555 * Business identifier assigned to the risk assessment. 556 */ 557 @Child(name = "identifier", type = { 558 Identifier.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 559 @Description(shortDefinition = "Unique identifier for the assessment", formalDefinition = "Business identifier assigned to the risk assessment.") 560 protected Identifier identifier; 561 562 /** 563 * The algorithm, process or mechanism used to evaluate the risk. 564 */ 565 @Child(name = "method", type = { 566 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 567 @Description(shortDefinition = "Evaluation mechanism", formalDefinition = "The algorithm, process or mechanism used to evaluate the risk.") 568 protected CodeableConcept method; 569 570 /** 571 * Indicates the source data considered as part of the assessment 572 * (FamilyHistory, Observations, Procedures, Conditions, etc.). 573 */ 574 @Child(name = "basis", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 575 @Description(shortDefinition = "Information used in assessment", formalDefinition = "Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.).") 576 protected List<Reference> basis; 577 /** 578 * The actual objects that are the target of the reference (Indicates the source 579 * data considered as part of the assessment (FamilyHistory, Observations, 580 * Procedures, Conditions, etc.).) 581 */ 582 protected List<Resource> basisTarget; 583 584 /** 585 * Describes the expected outcome for the subject. 586 */ 587 @Child(name = "prediction", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 588 @Description(shortDefinition = "Outcome predicted", formalDefinition = "Describes the expected outcome for the subject.") 589 protected List<RiskAssessmentPredictionComponent> prediction; 590 591 /** 592 * A description of the steps that might be taken to reduce the identified 593 * risk(s). 594 */ 595 @Child(name = "mitigation", type = { 596 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 597 @Description(shortDefinition = "How to reduce risk", formalDefinition = "A description of the steps that might be taken to reduce the identified risk(s).") 598 protected StringType mitigation; 599 600 private static final long serialVersionUID = 724306293L; 601 602 /* 603 * Constructor 604 */ 605 public RiskAssessment() { 606 super(); 607 } 608 609 /** 610 * @return {@link #subject} (The patient or group the risk assessment applies 611 * to.) 612 */ 613 public Reference getSubject() { 614 if (this.subject == null) 615 if (Configuration.errorOnAutoCreate()) 616 throw new Error("Attempt to auto-create RiskAssessment.subject"); 617 else if (Configuration.doAutoCreate()) 618 this.subject = new Reference(); // cc 619 return this.subject; 620 } 621 622 public boolean hasSubject() { 623 return this.subject != null && !this.subject.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #subject} (The patient or group the risk assessment 628 * applies to.) 629 */ 630 public RiskAssessment setSubject(Reference value) { 631 this.subject = value; 632 return this; 633 } 634 635 /** 636 * @return {@link #subject} The actual object that is the target of the 637 * reference. The reference library doesn't populate this, but you can 638 * use it to hold the resource if you resolve it. (The patient or group 639 * the risk assessment applies to.) 640 */ 641 public Resource getSubjectTarget() { 642 return this.subjectTarget; 643 } 644 645 /** 646 * @param value {@link #subject} The actual object that is the target of the 647 * reference. The reference library doesn't use these, but you can 648 * use it to hold the resource if you resolve it. (The patient or 649 * group the risk assessment applies to.) 650 */ 651 public RiskAssessment setSubjectTarget(Resource value) { 652 this.subjectTarget = value; 653 return this; 654 } 655 656 /** 657 * @return {@link #date} (The date (and possibly time) the risk assessment was 658 * performed.). This is the underlying object with id, value and 659 * extensions. The accessor "getDate" gives direct access to the value 660 */ 661 public DateTimeType getDateElement() { 662 if (this.date == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create RiskAssessment.date"); 665 else if (Configuration.doAutoCreate()) 666 this.date = new DateTimeType(); // bb 667 return this.date; 668 } 669 670 public boolean hasDateElement() { 671 return this.date != null && !this.date.isEmpty(); 672 } 673 674 public boolean hasDate() { 675 return this.date != null && !this.date.isEmpty(); 676 } 677 678 /** 679 * @param value {@link #date} (The date (and possibly time) the risk assessment 680 * was performed.). This is the underlying object with id, value 681 * and extensions. The accessor "getDate" gives direct access to 682 * the value 683 */ 684 public RiskAssessment setDateElement(DateTimeType value) { 685 this.date = value; 686 return this; 687 } 688 689 /** 690 * @return The date (and possibly time) the risk assessment was performed. 691 */ 692 public Date getDate() { 693 return this.date == null ? null : this.date.getValue(); 694 } 695 696 /** 697 * @param value The date (and possibly time) the risk assessment was performed. 698 */ 699 public RiskAssessment setDate(Date value) { 700 if (value == null) 701 this.date = null; 702 else { 703 if (this.date == null) 704 this.date = new DateTimeType(); 705 this.date.setValue(value); 706 } 707 return this; 708 } 709 710 /** 711 * @return {@link #condition} (For assessments or prognosis specific to a 712 * particular condition, indicates the condition being assessed.) 713 */ 714 public Reference getCondition() { 715 if (this.condition == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create RiskAssessment.condition"); 718 else if (Configuration.doAutoCreate()) 719 this.condition = new Reference(); // cc 720 return this.condition; 721 } 722 723 public boolean hasCondition() { 724 return this.condition != null && !this.condition.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #condition} (For assessments or prognosis specific to a 729 * particular condition, indicates the condition being assessed.) 730 */ 731 public RiskAssessment setCondition(Reference value) { 732 this.condition = value; 733 return this; 734 } 735 736 /** 737 * @return {@link #condition} The actual object that is the target of the 738 * reference. The reference library doesn't populate this, but you can 739 * use it to hold the resource if you resolve it. (For assessments or 740 * prognosis specific to a particular condition, indicates the condition 741 * being assessed.) 742 */ 743 public Condition getConditionTarget() { 744 if (this.conditionTarget == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create RiskAssessment.condition"); 747 else if (Configuration.doAutoCreate()) 748 this.conditionTarget = new Condition(); // aa 749 return this.conditionTarget; 750 } 751 752 /** 753 * @param value {@link #condition} The actual object that is the target of the 754 * reference. The reference library doesn't use these, but you can 755 * use it to hold the resource if you resolve it. (For assessments 756 * or prognosis specific to a particular condition, indicates the 757 * condition being assessed.) 758 */ 759 public RiskAssessment setConditionTarget(Condition value) { 760 this.conditionTarget = value; 761 return this; 762 } 763 764 /** 765 * @return {@link #encounter} (The encounter where the assessment was 766 * performed.) 767 */ 768 public Reference getEncounter() { 769 if (this.encounter == null) 770 if (Configuration.errorOnAutoCreate()) 771 throw new Error("Attempt to auto-create RiskAssessment.encounter"); 772 else if (Configuration.doAutoCreate()) 773 this.encounter = new Reference(); // cc 774 return this.encounter; 775 } 776 777 public boolean hasEncounter() { 778 return this.encounter != null && !this.encounter.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #encounter} (The encounter where the assessment was 783 * performed.) 784 */ 785 public RiskAssessment setEncounter(Reference value) { 786 this.encounter = value; 787 return this; 788 } 789 790 /** 791 * @return {@link #encounter} The actual object that is the target of the 792 * reference. The reference library doesn't populate this, but you can 793 * use it to hold the resource if you resolve it. (The encounter where 794 * the assessment was performed.) 795 */ 796 public Encounter getEncounterTarget() { 797 if (this.encounterTarget == null) 798 if (Configuration.errorOnAutoCreate()) 799 throw new Error("Attempt to auto-create RiskAssessment.encounter"); 800 else if (Configuration.doAutoCreate()) 801 this.encounterTarget = new Encounter(); // aa 802 return this.encounterTarget; 803 } 804 805 /** 806 * @param value {@link #encounter} The actual object that is the target of the 807 * reference. The reference library doesn't use these, but you can 808 * use it to hold the resource if you resolve it. (The encounter 809 * where the assessment was performed.) 810 */ 811 public RiskAssessment setEncounterTarget(Encounter value) { 812 this.encounterTarget = value; 813 return this; 814 } 815 816 /** 817 * @return {@link #performer} (The provider or software application that 818 * performed the assessment.) 819 */ 820 public Reference getPerformer() { 821 if (this.performer == null) 822 if (Configuration.errorOnAutoCreate()) 823 throw new Error("Attempt to auto-create RiskAssessment.performer"); 824 else if (Configuration.doAutoCreate()) 825 this.performer = new Reference(); // cc 826 return this.performer; 827 } 828 829 public boolean hasPerformer() { 830 return this.performer != null && !this.performer.isEmpty(); 831 } 832 833 /** 834 * @param value {@link #performer} (The provider or software application that 835 * performed the assessment.) 836 */ 837 public RiskAssessment setPerformer(Reference value) { 838 this.performer = value; 839 return this; 840 } 841 842 /** 843 * @return {@link #performer} The actual object that is the target of the 844 * reference. The reference library doesn't populate this, but you can 845 * use it to hold the resource if you resolve it. (The provider or 846 * software application that performed the assessment.) 847 */ 848 public Resource getPerformerTarget() { 849 return this.performerTarget; 850 } 851 852 /** 853 * @param value {@link #performer} The actual object that is the target of the 854 * reference. The reference library doesn't use these, but you can 855 * use it to hold the resource if you resolve it. (The provider or 856 * software application that performed the assessment.) 857 */ 858 public RiskAssessment setPerformerTarget(Resource value) { 859 this.performerTarget = value; 860 return this; 861 } 862 863 /** 864 * @return {@link #identifier} (Business identifier assigned to the risk 865 * assessment.) 866 */ 867 public Identifier getIdentifier() { 868 if (this.identifier == null) 869 if (Configuration.errorOnAutoCreate()) 870 throw new Error("Attempt to auto-create RiskAssessment.identifier"); 871 else if (Configuration.doAutoCreate()) 872 this.identifier = new Identifier(); // cc 873 return this.identifier; 874 } 875 876 public boolean hasIdentifier() { 877 return this.identifier != null && !this.identifier.isEmpty(); 878 } 879 880 /** 881 * @param value {@link #identifier} (Business identifier assigned to the risk 882 * assessment.) 883 */ 884 public RiskAssessment setIdentifier(Identifier value) { 885 this.identifier = value; 886 return this; 887 } 888 889 /** 890 * @return {@link #method} (The algorithm, process or mechanism used to evaluate 891 * the risk.) 892 */ 893 public CodeableConcept getMethod() { 894 if (this.method == null) 895 if (Configuration.errorOnAutoCreate()) 896 throw new Error("Attempt to auto-create RiskAssessment.method"); 897 else if (Configuration.doAutoCreate()) 898 this.method = new CodeableConcept(); // cc 899 return this.method; 900 } 901 902 public boolean hasMethod() { 903 return this.method != null && !this.method.isEmpty(); 904 } 905 906 /** 907 * @param value {@link #method} (The algorithm, process or mechanism used to 908 * evaluate the risk.) 909 */ 910 public RiskAssessment setMethod(CodeableConcept value) { 911 this.method = value; 912 return this; 913 } 914 915 /** 916 * @return {@link #basis} (Indicates the source data considered as part of the 917 * assessment (FamilyHistory, Observations, Procedures, Conditions, 918 * etc.).) 919 */ 920 public List<Reference> getBasis() { 921 if (this.basis == null) 922 this.basis = new ArrayList<Reference>(); 923 return this.basis; 924 } 925 926 public boolean hasBasis() { 927 if (this.basis == null) 928 return false; 929 for (Reference item : this.basis) 930 if (!item.isEmpty()) 931 return true; 932 return false; 933 } 934 935 /** 936 * @return {@link #basis} (Indicates the source data considered as part of the 937 * assessment (FamilyHistory, Observations, Procedures, Conditions, 938 * etc.).) 939 */ 940 // syntactic sugar 941 public Reference addBasis() { // 3 942 Reference t = new Reference(); 943 if (this.basis == null) 944 this.basis = new ArrayList<Reference>(); 945 this.basis.add(t); 946 return t; 947 } 948 949 // syntactic sugar 950 public RiskAssessment addBasis(Reference t) { // 3 951 if (t == null) 952 return this; 953 if (this.basis == null) 954 this.basis = new ArrayList<Reference>(); 955 this.basis.add(t); 956 return this; 957 } 958 959 /** 960 * @return {@link #basis} (The actual objects that are the target of the 961 * reference. The reference library doesn't populate this, but you can 962 * use this to hold the resources if you resolvethemt. Indicates the 963 * source data considered as part of the assessment (FamilyHistory, 964 * Observations, Procedures, Conditions, etc.).) 965 */ 966 public List<Resource> getBasisTarget() { 967 if (this.basisTarget == null) 968 this.basisTarget = new ArrayList<Resource>(); 969 return this.basisTarget; 970 } 971 972 /** 973 * @return {@link #prediction} (Describes the expected outcome for the subject.) 974 */ 975 public List<RiskAssessmentPredictionComponent> getPrediction() { 976 if (this.prediction == null) 977 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 978 return this.prediction; 979 } 980 981 public boolean hasPrediction() { 982 if (this.prediction == null) 983 return false; 984 for (RiskAssessmentPredictionComponent item : this.prediction) 985 if (!item.isEmpty()) 986 return true; 987 return false; 988 } 989 990 /** 991 * @return {@link #prediction} (Describes the expected outcome for the subject.) 992 */ 993 // syntactic sugar 994 public RiskAssessmentPredictionComponent addPrediction() { // 3 995 RiskAssessmentPredictionComponent t = new RiskAssessmentPredictionComponent(); 996 if (this.prediction == null) 997 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 998 this.prediction.add(t); 999 return t; 1000 } 1001 1002 // syntactic sugar 1003 public RiskAssessment addPrediction(RiskAssessmentPredictionComponent t) { // 3 1004 if (t == null) 1005 return this; 1006 if (this.prediction == null) 1007 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1008 this.prediction.add(t); 1009 return this; 1010 } 1011 1012 /** 1013 * @return {@link #mitigation} (A description of the steps that might be taken 1014 * to reduce the identified risk(s).). This is the underlying object 1015 * with id, value and extensions. The accessor "getMitigation" gives 1016 * direct access to the value 1017 */ 1018 public StringType getMitigationElement() { 1019 if (this.mitigation == null) 1020 if (Configuration.errorOnAutoCreate()) 1021 throw new Error("Attempt to auto-create RiskAssessment.mitigation"); 1022 else if (Configuration.doAutoCreate()) 1023 this.mitigation = new StringType(); // bb 1024 return this.mitigation; 1025 } 1026 1027 public boolean hasMitigationElement() { 1028 return this.mitigation != null && !this.mitigation.isEmpty(); 1029 } 1030 1031 public boolean hasMitigation() { 1032 return this.mitigation != null && !this.mitigation.isEmpty(); 1033 } 1034 1035 /** 1036 * @param value {@link #mitigation} (A description of the steps that might be 1037 * taken to reduce the identified risk(s).). This is the underlying 1038 * object with id, value and extensions. The accessor 1039 * "getMitigation" gives direct access to the value 1040 */ 1041 public RiskAssessment setMitigationElement(StringType value) { 1042 this.mitigation = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return A description of the steps that might be taken to reduce the 1048 * identified risk(s). 1049 */ 1050 public String getMitigation() { 1051 return this.mitigation == null ? null : this.mitigation.getValue(); 1052 } 1053 1054 /** 1055 * @param value A description of the steps that might be taken to reduce the 1056 * identified risk(s). 1057 */ 1058 public RiskAssessment setMitigation(String value) { 1059 if (Utilities.noString(value)) 1060 this.mitigation = null; 1061 else { 1062 if (this.mitigation == null) 1063 this.mitigation = new StringType(); 1064 this.mitigation.setValue(value); 1065 } 1066 return this; 1067 } 1068 1069 protected void listChildren(List<Property> childrenList) { 1070 super.listChildren(childrenList); 1071 childrenList.add(new Property("subject", "Reference(Patient|Group)", 1072 "The patient or group the risk assessment applies to.", 0, java.lang.Integer.MAX_VALUE, subject)); 1073 childrenList.add(new Property("date", "dateTime", "The date (and possibly time) the risk assessment was performed.", 1074 0, java.lang.Integer.MAX_VALUE, date)); 1075 childrenList.add(new Property("condition", "Reference(Condition)", 1076 "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 1077 java.lang.Integer.MAX_VALUE, condition)); 1078 childrenList.add(new Property("encounter", "Reference(Encounter)", 1079 "The encounter where the assessment was performed.", 0, java.lang.Integer.MAX_VALUE, encounter)); 1080 childrenList.add(new Property("performer", "Reference(Practitioner|Device)", 1081 "The provider or software application that performed the assessment.", 0, java.lang.Integer.MAX_VALUE, 1082 performer)); 1083 childrenList.add(new Property("identifier", "Identifier", "Business identifier assigned to the risk assessment.", 0, 1084 java.lang.Integer.MAX_VALUE, identifier)); 1085 childrenList.add(new Property("method", "CodeableConcept", 1086 "The algorithm, process or mechanism used to evaluate the risk.", 0, java.lang.Integer.MAX_VALUE, method)); 1087 childrenList.add(new Property("basis", "Reference(Any)", 1088 "Indicates the source data considered as part of the assessment (FamilyHistory, Observations, Procedures, Conditions, etc.).", 1089 0, java.lang.Integer.MAX_VALUE, basis)); 1090 childrenList.add(new Property("prediction", "", "Describes the expected outcome for the subject.", 0, 1091 java.lang.Integer.MAX_VALUE, prediction)); 1092 childrenList.add(new Property("mitigation", "string", 1093 "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1094 java.lang.Integer.MAX_VALUE, mitigation)); 1095 } 1096 1097 @Override 1098 public void setProperty(String name, Base value) throws FHIRException { 1099 if (name.equals("subject")) 1100 this.subject = castToReference(value); // Reference 1101 else if (name.equals("date")) 1102 this.date = castToDateTime(value); // DateTimeType 1103 else if (name.equals("condition")) 1104 this.condition = castToReference(value); // Reference 1105 else if (name.equals("encounter")) 1106 this.encounter = castToReference(value); // Reference 1107 else if (name.equals("performer")) 1108 this.performer = castToReference(value); // Reference 1109 else if (name.equals("identifier")) 1110 this.identifier = castToIdentifier(value); // Identifier 1111 else if (name.equals("method")) 1112 this.method = castToCodeableConcept(value); // CodeableConcept 1113 else if (name.equals("basis")) 1114 this.getBasis().add(castToReference(value)); 1115 else if (name.equals("prediction")) 1116 this.getPrediction().add((RiskAssessmentPredictionComponent) value); 1117 else if (name.equals("mitigation")) 1118 this.mitigation = castToString(value); // StringType 1119 else 1120 super.setProperty(name, value); 1121 } 1122 1123 @Override 1124 public Base addChild(String name) throws FHIRException { 1125 if (name.equals("subject")) { 1126 this.subject = new Reference(); 1127 return this.subject; 1128 } else if (name.equals("date")) { 1129 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.date"); 1130 } else if (name.equals("condition")) { 1131 this.condition = new Reference(); 1132 return this.condition; 1133 } else if (name.equals("encounter")) { 1134 this.encounter = new Reference(); 1135 return this.encounter; 1136 } else if (name.equals("performer")) { 1137 this.performer = new Reference(); 1138 return this.performer; 1139 } else if (name.equals("identifier")) { 1140 this.identifier = new Identifier(); 1141 return this.identifier; 1142 } else if (name.equals("method")) { 1143 this.method = new CodeableConcept(); 1144 return this.method; 1145 } else if (name.equals("basis")) { 1146 return addBasis(); 1147 } else if (name.equals("prediction")) { 1148 return addPrediction(); 1149 } else if (name.equals("mitigation")) { 1150 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.mitigation"); 1151 } else 1152 return super.addChild(name); 1153 } 1154 1155 public String fhirType() { 1156 return "RiskAssessment"; 1157 1158 } 1159 1160 public RiskAssessment copy() { 1161 RiskAssessment dst = new RiskAssessment(); 1162 copyValues(dst); 1163 dst.subject = subject == null ? null : subject.copy(); 1164 dst.date = date == null ? null : date.copy(); 1165 dst.condition = condition == null ? null : condition.copy(); 1166 dst.encounter = encounter == null ? null : encounter.copy(); 1167 dst.performer = performer == null ? null : performer.copy(); 1168 dst.identifier = identifier == null ? null : identifier.copy(); 1169 dst.method = method == null ? null : method.copy(); 1170 if (basis != null) { 1171 dst.basis = new ArrayList<Reference>(); 1172 for (Reference i : basis) 1173 dst.basis.add(i.copy()); 1174 } 1175 ; 1176 if (prediction != null) { 1177 dst.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1178 for (RiskAssessmentPredictionComponent i : prediction) 1179 dst.prediction.add(i.copy()); 1180 } 1181 ; 1182 dst.mitigation = mitigation == null ? null : mitigation.copy(); 1183 return dst; 1184 } 1185 1186 protected RiskAssessment typedCopy() { 1187 return copy(); 1188 } 1189 1190 @Override 1191 public boolean equalsDeep(Base other) { 1192 if (!super.equalsDeep(other)) 1193 return false; 1194 if (!(other instanceof RiskAssessment)) 1195 return false; 1196 RiskAssessment o = (RiskAssessment) other; 1197 return compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) 1198 && compareDeep(condition, o.condition, true) && compareDeep(encounter, o.encounter, true) 1199 && compareDeep(performer, o.performer, true) && compareDeep(identifier, o.identifier, true) 1200 && compareDeep(method, o.method, true) && compareDeep(basis, o.basis, true) 1201 && compareDeep(prediction, o.prediction, true) && compareDeep(mitigation, o.mitigation, true); 1202 } 1203 1204 @Override 1205 public boolean equalsShallow(Base other) { 1206 if (!super.equalsShallow(other)) 1207 return false; 1208 if (!(other instanceof RiskAssessment)) 1209 return false; 1210 RiskAssessment o = (RiskAssessment) other; 1211 return compareValues(date, o.date, true) && compareValues(mitigation, o.mitigation, true); 1212 } 1213 1214 public boolean isEmpty() { 1215 return super.isEmpty() && (subject == null || subject.isEmpty()) && (date == null || date.isEmpty()) 1216 && (condition == null || condition.isEmpty()) && (encounter == null || encounter.isEmpty()) 1217 && (performer == null || performer.isEmpty()) && (identifier == null || identifier.isEmpty()) 1218 && (method == null || method.isEmpty()) && (basis == null || basis.isEmpty()) 1219 && (prediction == null || prediction.isEmpty()) && (mitigation == null || mitigation.isEmpty()); 1220 } 1221 1222 @Override 1223 public ResourceType getResourceType() { 1224 return ResourceType.RiskAssessment; 1225 } 1226 1227 @SearchParamDefinition(name = "date", path = "RiskAssessment.date", description = "When was assessment made?", type = "date") 1228 public static final String SP_DATE = "date"; 1229 @SearchParamDefinition(name = "identifier", path = "RiskAssessment.identifier", description = "Unique identifier for the assessment", type = "token") 1230 public static final String SP_IDENTIFIER = "identifier"; 1231 @SearchParamDefinition(name = "condition", path = "RiskAssessment.condition", description = "Condition assessed", type = "reference") 1232 public static final String SP_CONDITION = "condition"; 1233 @SearchParamDefinition(name = "performer", path = "RiskAssessment.performer", description = "Who did assessment?", type = "reference") 1234 public static final String SP_PERFORMER = "performer"; 1235 @SearchParamDefinition(name = "method", path = "RiskAssessment.method", description = "Evaluation mechanism", type = "token") 1236 public static final String SP_METHOD = "method"; 1237 @SearchParamDefinition(name = "subject", path = "RiskAssessment.subject", description = "Who/what does assessment apply to?", type = "reference") 1238 public static final String SP_SUBJECT = "subject"; 1239 @SearchParamDefinition(name = "patient", path = "RiskAssessment.subject", description = "Who/what does assessment apply to?", type = "reference") 1240 public static final String SP_PATIENT = "patient"; 1241 @SearchParamDefinition(name = "encounter", path = "RiskAssessment.encounter", description = "Where was assessment performed?", type = "reference") 1242 public static final String SP_ENCOUNTER = "encounter"; 1243 1244}