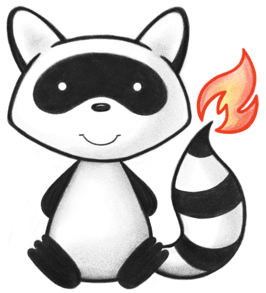
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.DatatypeDef; 039import ca.uhn.fhir.model.api.annotation.Description; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * A series of measurements taken by a device, with upper and lower limits. 045 * There may be more than one dimension in the data. 046 */ 047@DatatypeDef(name = "SampledData") 048public class SampledData extends Type implements ICompositeType { 049 050 /** 051 * The base quantity that a measured value of zero represents. In addition, this 052 * provides the units of the entire measurement series. 053 */ 054 @Child(name = "origin", type = { 055 SimpleQuantity.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 056 @Description(shortDefinition = "Zero value and units", formalDefinition = "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.") 057 protected SimpleQuantity origin; 058 059 /** 060 * The length of time between sampling times, measured in milliseconds. 061 */ 062 @Child(name = "period", type = { DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "Number of milliseconds between samples", formalDefinition = "The length of time between sampling times, measured in milliseconds.") 064 protected DecimalType period; 065 066 /** 067 * A correction factor that is applied to the sampled data points before they 068 * are added to the origin. 069 */ 070 @Child(name = "factor", type = { DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 071 @Description(shortDefinition = "Multiply data by this before adding to origin", formalDefinition = "A correction factor that is applied to the sampled data points before they are added to the origin.") 072 protected DecimalType factor; 073 074 /** 075 * The lower limit of detection of the measured points. This is needed if any of 076 * the data points have the value "L" (lower than detection limit). 077 */ 078 @Child(name = "lowerLimit", type = { 079 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 080 @Description(shortDefinition = "Lower limit of detection", formalDefinition = "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).") 081 protected DecimalType lowerLimit; 082 083 /** 084 * The upper limit of detection of the measured points. This is needed if any of 085 * the data points have the value "U" (higher than detection limit). 086 */ 087 @Child(name = "upperLimit", type = { 088 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 089 @Description(shortDefinition = "Upper limit of detection", formalDefinition = "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).") 090 protected DecimalType upperLimit; 091 092 /** 093 * The number of sample points at each time point. If this value is greater than 094 * one, then the dimensions will be interlaced - all the sample points for a 095 * point in time will be recorded at once. 096 */ 097 @Child(name = "dimensions", type = { 098 PositiveIntType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 099 @Description(shortDefinition = "Number of sample points at each time point", formalDefinition = "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.") 100 protected PositiveIntType dimensions; 101 102 /** 103 * A series of data points which are decimal values separated by a single space 104 * (character u20). The special values "E" (error), "L" (below detection limit) 105 * and "U" (above detection limit) can also be used in place of a decimal value. 106 */ 107 @Child(name = "data", type = { StringType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 108 @Description(shortDefinition = "Decimal values with spaces, or \"E\" | \"U\" | \"L\"", formalDefinition = "A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value.") 109 protected StringType data; 110 111 private static final long serialVersionUID = -1763278368L; 112 113 /* 114 * Constructor 115 */ 116 public SampledData() { 117 super(); 118 } 119 120 /* 121 * Constructor 122 */ 123 public SampledData(SimpleQuantity origin, DecimalType period, PositiveIntType dimensions, StringType data) { 124 super(); 125 this.origin = origin; 126 this.period = period; 127 this.dimensions = dimensions; 128 this.data = data; 129 } 130 131 /** 132 * @return {@link #origin} (The base quantity that a measured value of zero 133 * represents. In addition, this provides the units of the entire 134 * measurement series.) 135 */ 136 public SimpleQuantity getOrigin() { 137 if (this.origin == null) 138 if (Configuration.errorOnAutoCreate()) 139 throw new Error("Attempt to auto-create SampledData.origin"); 140 else if (Configuration.doAutoCreate()) 141 this.origin = new SimpleQuantity(); // cc 142 return this.origin; 143 } 144 145 public boolean hasOrigin() { 146 return this.origin != null && !this.origin.isEmpty(); 147 } 148 149 /** 150 * @param value {@link #origin} (The base quantity that a measured value of zero 151 * represents. In addition, this provides the units of the entire 152 * measurement series.) 153 */ 154 public SampledData setOrigin(SimpleQuantity value) { 155 this.origin = value; 156 return this; 157 } 158 159 /** 160 * @return {@link #period} (The length of time between sampling times, measured 161 * in milliseconds.). This is the underlying object with id, value and 162 * extensions. The accessor "getPeriod" gives direct access to the value 163 */ 164 public DecimalType getPeriodElement() { 165 if (this.period == null) 166 if (Configuration.errorOnAutoCreate()) 167 throw new Error("Attempt to auto-create SampledData.period"); 168 else if (Configuration.doAutoCreate()) 169 this.period = new DecimalType(); // bb 170 return this.period; 171 } 172 173 public boolean hasPeriodElement() { 174 return this.period != null && !this.period.isEmpty(); 175 } 176 177 public boolean hasPeriod() { 178 return this.period != null && !this.period.isEmpty(); 179 } 180 181 /** 182 * @param value {@link #period} (The length of time between sampling times, 183 * measured in milliseconds.). This is the underlying object with 184 * id, value and extensions. The accessor "getPeriod" gives direct 185 * access to the value 186 */ 187 public SampledData setPeriodElement(DecimalType value) { 188 this.period = value; 189 return this; 190 } 191 192 /** 193 * @return The length of time between sampling times, measured in milliseconds. 194 */ 195 public BigDecimal getPeriod() { 196 return this.period == null ? null : this.period.getValue(); 197 } 198 199 /** 200 * @param value The length of time between sampling times, measured in 201 * milliseconds. 202 */ 203 public SampledData setPeriod(BigDecimal value) { 204 if (this.period == null) 205 this.period = new DecimalType(); 206 this.period.setValue(value); 207 return this; 208 } 209 210 /** 211 * @return {@link #factor} (A correction factor that is applied to the sampled 212 * data points before they are added to the origin.). This is the 213 * underlying object with id, value and extensions. The accessor 214 * "getFactor" gives direct access to the value 215 */ 216 public DecimalType getFactorElement() { 217 if (this.factor == null) 218 if (Configuration.errorOnAutoCreate()) 219 throw new Error("Attempt to auto-create SampledData.factor"); 220 else if (Configuration.doAutoCreate()) 221 this.factor = new DecimalType(); // bb 222 return this.factor; 223 } 224 225 public boolean hasFactorElement() { 226 return this.factor != null && !this.factor.isEmpty(); 227 } 228 229 public boolean hasFactor() { 230 return this.factor != null && !this.factor.isEmpty(); 231 } 232 233 /** 234 * @param value {@link #factor} (A correction factor that is applied to the 235 * sampled data points before they are added to the origin.). This 236 * is the underlying object with id, value and extensions. The 237 * accessor "getFactor" gives direct access to the value 238 */ 239 public SampledData setFactorElement(DecimalType value) { 240 this.factor = value; 241 return this; 242 } 243 244 /** 245 * @return A correction factor that is applied to the sampled data points before 246 * they are added to the origin. 247 */ 248 public BigDecimal getFactor() { 249 return this.factor == null ? null : this.factor.getValue(); 250 } 251 252 /** 253 * @param value A correction factor that is applied to the sampled data points 254 * before they are added to the origin. 255 */ 256 public SampledData setFactor(BigDecimal value) { 257 if (value == null) 258 this.factor = null; 259 else { 260 if (this.factor == null) 261 this.factor = new DecimalType(); 262 this.factor.setValue(value); 263 } 264 return this; 265 } 266 267 /** 268 * @return {@link #lowerLimit} (The lower limit of detection of the measured 269 * points. This is needed if any of the data points have the value "L" 270 * (lower than detection limit).). This is the underlying object with 271 * id, value and extensions. The accessor "getLowerLimit" gives direct 272 * access to the value 273 */ 274 public DecimalType getLowerLimitElement() { 275 if (this.lowerLimit == null) 276 if (Configuration.errorOnAutoCreate()) 277 throw new Error("Attempt to auto-create SampledData.lowerLimit"); 278 else if (Configuration.doAutoCreate()) 279 this.lowerLimit = new DecimalType(); // bb 280 return this.lowerLimit; 281 } 282 283 public boolean hasLowerLimitElement() { 284 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 285 } 286 287 public boolean hasLowerLimit() { 288 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 289 } 290 291 /** 292 * @param value {@link #lowerLimit} (The lower limit of detection of the 293 * measured points. This is needed if any of the data points have 294 * the value "L" (lower than detection limit).). This is the 295 * underlying object with id, value and extensions. The accessor 296 * "getLowerLimit" gives direct access to the value 297 */ 298 public SampledData setLowerLimitElement(DecimalType value) { 299 this.lowerLimit = value; 300 return this; 301 } 302 303 /** 304 * @return The lower limit of detection of the measured points. This is needed 305 * if any of the data points have the value "L" (lower than detection 306 * limit). 307 */ 308 public BigDecimal getLowerLimit() { 309 return this.lowerLimit == null ? null : this.lowerLimit.getValue(); 310 } 311 312 /** 313 * @param value The lower limit of detection of the measured points. This is 314 * needed if any of the data points have the value "L" (lower than 315 * detection limit). 316 */ 317 public SampledData setLowerLimit(BigDecimal value) { 318 if (value == null) 319 this.lowerLimit = null; 320 else { 321 if (this.lowerLimit == null) 322 this.lowerLimit = new DecimalType(); 323 this.lowerLimit.setValue(value); 324 } 325 return this; 326 } 327 328 /** 329 * @return {@link #upperLimit} (The upper limit of detection of the measured 330 * points. This is needed if any of the data points have the value "U" 331 * (higher than detection limit).). This is the underlying object with 332 * id, value and extensions. The accessor "getUpperLimit" gives direct 333 * access to the value 334 */ 335 public DecimalType getUpperLimitElement() { 336 if (this.upperLimit == null) 337 if (Configuration.errorOnAutoCreate()) 338 throw new Error("Attempt to auto-create SampledData.upperLimit"); 339 else if (Configuration.doAutoCreate()) 340 this.upperLimit = new DecimalType(); // bb 341 return this.upperLimit; 342 } 343 344 public boolean hasUpperLimitElement() { 345 return this.upperLimit != null && !this.upperLimit.isEmpty(); 346 } 347 348 public boolean hasUpperLimit() { 349 return this.upperLimit != null && !this.upperLimit.isEmpty(); 350 } 351 352 /** 353 * @param value {@link #upperLimit} (The upper limit of detection of the 354 * measured points. This is needed if any of the data points have 355 * the value "U" (higher than detection limit).). This is the 356 * underlying object with id, value and extensions. The accessor 357 * "getUpperLimit" gives direct access to the value 358 */ 359 public SampledData setUpperLimitElement(DecimalType value) { 360 this.upperLimit = value; 361 return this; 362 } 363 364 /** 365 * @return The upper limit of detection of the measured points. This is needed 366 * if any of the data points have the value "U" (higher than detection 367 * limit). 368 */ 369 public BigDecimal getUpperLimit() { 370 return this.upperLimit == null ? null : this.upperLimit.getValue(); 371 } 372 373 /** 374 * @param value The upper limit of detection of the measured points. This is 375 * needed if any of the data points have the value "U" (higher than 376 * detection limit). 377 */ 378 public SampledData setUpperLimit(BigDecimal value) { 379 if (value == null) 380 this.upperLimit = null; 381 else { 382 if (this.upperLimit == null) 383 this.upperLimit = new DecimalType(); 384 this.upperLimit.setValue(value); 385 } 386 return this; 387 } 388 389 /** 390 * @return {@link #dimensions} (The number of sample points at each time point. 391 * If this value is greater than one, then the dimensions will be 392 * interlaced - all the sample points for a point in time will be 393 * recorded at once.). This is the underlying object with id, value and 394 * extensions. The accessor "getDimensions" gives direct access to the 395 * value 396 */ 397 public PositiveIntType getDimensionsElement() { 398 if (this.dimensions == null) 399 if (Configuration.errorOnAutoCreate()) 400 throw new Error("Attempt to auto-create SampledData.dimensions"); 401 else if (Configuration.doAutoCreate()) 402 this.dimensions = new PositiveIntType(); // bb 403 return this.dimensions; 404 } 405 406 public boolean hasDimensionsElement() { 407 return this.dimensions != null && !this.dimensions.isEmpty(); 408 } 409 410 public boolean hasDimensions() { 411 return this.dimensions != null && !this.dimensions.isEmpty(); 412 } 413 414 /** 415 * @param value {@link #dimensions} (The number of sample points at each time 416 * point. If this value is greater than one, then the dimensions 417 * will be interlaced - all the sample points for a point in time 418 * will be recorded at once.). This is the underlying object with 419 * id, value and extensions. The accessor "getDimensions" gives 420 * direct access to the value 421 */ 422 public SampledData setDimensionsElement(PositiveIntType value) { 423 this.dimensions = value; 424 return this; 425 } 426 427 /** 428 * @return The number of sample points at each time point. If this value is 429 * greater than one, then the dimensions will be interlaced - all the 430 * sample points for a point in time will be recorded at once. 431 */ 432 public int getDimensions() { 433 return this.dimensions == null || this.dimensions.isEmpty() ? 0 : this.dimensions.getValue(); 434 } 435 436 /** 437 * @param value The number of sample points at each time point. If this value is 438 * greater than one, then the dimensions will be interlaced - all 439 * the sample points for a point in time will be recorded at once. 440 */ 441 public SampledData setDimensions(int value) { 442 if (this.dimensions == null) 443 this.dimensions = new PositiveIntType(); 444 this.dimensions.setValue(value); 445 return this; 446 } 447 448 /** 449 * @return {@link #data} (A series of data points which are decimal values 450 * separated by a single space (character u20). The special values "E" 451 * (error), "L" (below detection limit) and "U" (above detection limit) 452 * can also be used in place of a decimal value.). This is the 453 * underlying object with id, value and extensions. The accessor 454 * "getData" gives direct access to the value 455 */ 456 public StringType getDataElement() { 457 if (this.data == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create SampledData.data"); 460 else if (Configuration.doAutoCreate()) 461 this.data = new StringType(); // bb 462 return this.data; 463 } 464 465 public boolean hasDataElement() { 466 return this.data != null && !this.data.isEmpty(); 467 } 468 469 public boolean hasData() { 470 return this.data != null && !this.data.isEmpty(); 471 } 472 473 /** 474 * @param value {@link #data} (A series of data points which are decimal values 475 * separated by a single space (character u20). The special values 476 * "E" (error), "L" (below detection limit) and "U" (above 477 * detection limit) can also be used in place of a decimal value.). 478 * This is the underlying object with id, value and extensions. The 479 * accessor "getData" gives direct access to the value 480 */ 481 public SampledData setDataElement(StringType value) { 482 this.data = value; 483 return this; 484 } 485 486 /** 487 * @return A series of data points which are decimal values separated by a 488 * single space (character u20). The special values "E" (error), "L" 489 * (below detection limit) and "U" (above detection limit) can also be 490 * used in place of a decimal value. 491 */ 492 public String getData() { 493 return this.data == null ? null : this.data.getValue(); 494 } 495 496 /** 497 * @param value A series of data points which are decimal values separated by a 498 * single space (character u20). The special values "E" (error), 499 * "L" (below detection limit) and "U" (above detection limit) can 500 * also be used in place of a decimal value. 501 */ 502 public SampledData setData(String value) { 503 if (this.data == null) 504 this.data = new StringType(); 505 this.data.setValue(value); 506 return this; 507 } 508 509 protected void listChildren(List<Property> childrenList) { 510 super.listChildren(childrenList); 511 childrenList.add(new Property("origin", "SimpleQuantity", 512 "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.", 513 0, java.lang.Integer.MAX_VALUE, origin)); 514 childrenList 515 .add(new Property("period", "decimal", "The length of time between sampling times, measured in milliseconds.", 516 0, java.lang.Integer.MAX_VALUE, period)); 517 childrenList.add(new Property("factor", "decimal", 518 "A correction factor that is applied to the sampled data points before they are added to the origin.", 0, 519 java.lang.Integer.MAX_VALUE, factor)); 520 childrenList.add(new Property("lowerLimit", "decimal", 521 "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).", 522 0, java.lang.Integer.MAX_VALUE, lowerLimit)); 523 childrenList.add(new Property("upperLimit", "decimal", 524 "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).", 525 0, java.lang.Integer.MAX_VALUE, upperLimit)); 526 childrenList.add(new Property("dimensions", "positiveInt", 527 "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.", 528 0, java.lang.Integer.MAX_VALUE, dimensions)); 529 childrenList.add(new Property("data", "string", 530 "A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value.", 531 0, java.lang.Integer.MAX_VALUE, data)); 532 } 533 534 @Override 535 public void setProperty(String name, Base value) throws FHIRException { 536 if (name.equals("origin")) 537 this.origin = castToSimpleQuantity(value); // SimpleQuantity 538 else if (name.equals("period")) 539 this.period = castToDecimal(value); // DecimalType 540 else if (name.equals("factor")) 541 this.factor = castToDecimal(value); // DecimalType 542 else if (name.equals("lowerLimit")) 543 this.lowerLimit = castToDecimal(value); // DecimalType 544 else if (name.equals("upperLimit")) 545 this.upperLimit = castToDecimal(value); // DecimalType 546 else if (name.equals("dimensions")) 547 this.dimensions = castToPositiveInt(value); // PositiveIntType 548 else if (name.equals("data")) 549 this.data = castToString(value); // StringType 550 else 551 super.setProperty(name, value); 552 } 553 554 @Override 555 public Base addChild(String name) throws FHIRException { 556 if (name.equals("origin")) { 557 this.origin = new SimpleQuantity(); 558 return this.origin; 559 } else if (name.equals("period")) { 560 throw new FHIRException("Cannot call addChild on a singleton property SampledData.period"); 561 } else if (name.equals("factor")) { 562 throw new FHIRException("Cannot call addChild on a singleton property SampledData.factor"); 563 } else if (name.equals("lowerLimit")) { 564 throw new FHIRException("Cannot call addChild on a singleton property SampledData.lowerLimit"); 565 } else if (name.equals("upperLimit")) { 566 throw new FHIRException("Cannot call addChild on a singleton property SampledData.upperLimit"); 567 } else if (name.equals("dimensions")) { 568 throw new FHIRException("Cannot call addChild on a singleton property SampledData.dimensions"); 569 } else if (name.equals("data")) { 570 throw new FHIRException("Cannot call addChild on a singleton property SampledData.data"); 571 } else 572 return super.addChild(name); 573 } 574 575 public String fhirType() { 576 return "SampledData"; 577 578 } 579 580 public SampledData copy() { 581 SampledData dst = new SampledData(); 582 copyValues(dst); 583 dst.origin = origin == null ? null : origin.copy(); 584 dst.period = period == null ? null : period.copy(); 585 dst.factor = factor == null ? null : factor.copy(); 586 dst.lowerLimit = lowerLimit == null ? null : lowerLimit.copy(); 587 dst.upperLimit = upperLimit == null ? null : upperLimit.copy(); 588 dst.dimensions = dimensions == null ? null : dimensions.copy(); 589 dst.data = data == null ? null : data.copy(); 590 return dst; 591 } 592 593 protected SampledData typedCopy() { 594 return copy(); 595 } 596 597 @Override 598 public boolean equalsDeep(Base other) { 599 if (!super.equalsDeep(other)) 600 return false; 601 if (!(other instanceof SampledData)) 602 return false; 603 SampledData o = (SampledData) other; 604 return compareDeep(origin, o.origin, true) && compareDeep(period, o.period, true) 605 && compareDeep(factor, o.factor, true) && compareDeep(lowerLimit, o.lowerLimit, true) 606 && compareDeep(upperLimit, o.upperLimit, true) && compareDeep(dimensions, o.dimensions, true) 607 && compareDeep(data, o.data, true); 608 } 609 610 @Override 611 public boolean equalsShallow(Base other) { 612 if (!super.equalsShallow(other)) 613 return false; 614 if (!(other instanceof SampledData)) 615 return false; 616 SampledData o = (SampledData) other; 617 return compareValues(period, o.period, true) && compareValues(factor, o.factor, true) 618 && compareValues(lowerLimit, o.lowerLimit, true) && compareValues(upperLimit, o.upperLimit, true) 619 && compareValues(dimensions, o.dimensions, true) && compareValues(data, o.data, true); 620 } 621 622 public boolean isEmpty() { 623 return super.isEmpty() && (origin == null || origin.isEmpty()) && (period == null || period.isEmpty()) 624 && (factor == null || factor.isEmpty()) && (lowerLimit == null || lowerLimit.isEmpty()) 625 && (upperLimit == null || upperLimit.isEmpty()) && (dimensions == null || dimensions.isEmpty()) 626 && (data == null || data.isEmpty()); 627 } 628 629}