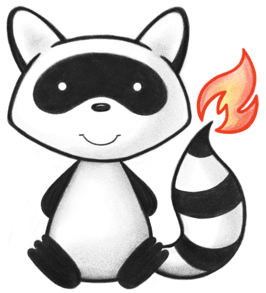
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import ca.uhn.fhir.model.api.annotation.ResourceDef; 039import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * A container for slot(s) of time that may be available for booking 045 * appointments. 046 */ 047@ResourceDef(name = "Schedule", profile = "http://hl7.org/fhir/Profile/Schedule") 048public class Schedule extends DomainResource { 049 050 /** 051 * External Ids for this item. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 055 @Description(shortDefinition = "External Ids for this item", formalDefinition = "External Ids for this item.") 056 protected List<Identifier> identifier; 057 058 /** 059 * The schedule type can be used for the categorization of healthcare services 060 * or other appointment types. 061 */ 062 @Child(name = "type", type = { 063 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 064 @Description(shortDefinition = "The schedule type can be used for the categorization of healthcare services or other appointment types", formalDefinition = "The schedule type can be used for the categorization of healthcare services or other appointment types.") 065 protected List<CodeableConcept> type; 066 067 /** 068 * The resource this Schedule resource is providing availability information 069 * for. These are expected to usually be one of HealthcareService, Location, 070 * Practitioner, Device, Patient or RelatedPerson. 071 */ 072 @Child(name = "actor", type = { Patient.class, Practitioner.class, RelatedPerson.class, Device.class, 073 HealthcareService.class, Location.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 074 @Description(shortDefinition = "The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, Device, Patient or RelatedPerson", formalDefinition = "The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, Device, Patient or RelatedPerson.") 075 protected Reference actor; 076 077 /** 078 * The actual object that is the target of the reference (The resource this 079 * Schedule resource is providing availability information for. These are 080 * expected to usually be one of HealthcareService, Location, Practitioner, 081 * Device, Patient or RelatedPerson.) 082 */ 083 protected Resource actorTarget; 084 085 /** 086 * The period of time that the slots that are attached to this Schedule resource 087 * cover (even if none exist). These cover the amount of time that an 088 * organization's planning horizon; the interval for which they are currently 089 * accepting appointments. This does not define a "template" for planning 090 * outside these dates. 091 */ 092 @Child(name = "planningHorizon", type = { 093 Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 094 @Description(shortDefinition = "The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates", formalDefinition = "The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.") 095 protected Period planningHorizon; 096 097 /** 098 * Comments on the availability to describe any extended information. Such as 099 * custom constraints on the slot(s) that may be associated. 100 */ 101 @Child(name = "comment", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 102 @Description(shortDefinition = "Comments on the availability to describe any extended information. Such as custom constraints on the slot(s) that may be associated", formalDefinition = "Comments on the availability to describe any extended information. Such as custom constraints on the slot(s) that may be associated.") 103 protected StringType comment; 104 105 private static final long serialVersionUID = 158030926L; 106 107 /* 108 * Constructor 109 */ 110 public Schedule() { 111 super(); 112 } 113 114 /* 115 * Constructor 116 */ 117 public Schedule(Reference actor) { 118 super(); 119 this.actor = actor; 120 } 121 122 /** 123 * @return {@link #identifier} (External Ids for this item.) 124 */ 125 public List<Identifier> getIdentifier() { 126 if (this.identifier == null) 127 this.identifier = new ArrayList<Identifier>(); 128 return this.identifier; 129 } 130 131 public boolean hasIdentifier() { 132 if (this.identifier == null) 133 return false; 134 for (Identifier item : this.identifier) 135 if (!item.isEmpty()) 136 return true; 137 return false; 138 } 139 140 /** 141 * @return {@link #identifier} (External Ids for this item.) 142 */ 143 // syntactic sugar 144 public Identifier addIdentifier() { // 3 145 Identifier t = new Identifier(); 146 if (this.identifier == null) 147 this.identifier = new ArrayList<Identifier>(); 148 this.identifier.add(t); 149 return t; 150 } 151 152 // syntactic sugar 153 public Schedule addIdentifier(Identifier t) { // 3 154 if (t == null) 155 return this; 156 if (this.identifier == null) 157 this.identifier = new ArrayList<Identifier>(); 158 this.identifier.add(t); 159 return this; 160 } 161 162 /** 163 * @return {@link #type} (The schedule type can be used for the categorization 164 * of healthcare services or other appointment types.) 165 */ 166 public List<CodeableConcept> getType() { 167 if (this.type == null) 168 this.type = new ArrayList<CodeableConcept>(); 169 return this.type; 170 } 171 172 public boolean hasType() { 173 if (this.type == null) 174 return false; 175 for (CodeableConcept item : this.type) 176 if (!item.isEmpty()) 177 return true; 178 return false; 179 } 180 181 /** 182 * @return {@link #type} (The schedule type can be used for the categorization 183 * of healthcare services or other appointment types.) 184 */ 185 // syntactic sugar 186 public CodeableConcept addType() { // 3 187 CodeableConcept t = new CodeableConcept(); 188 if (this.type == null) 189 this.type = new ArrayList<CodeableConcept>(); 190 this.type.add(t); 191 return t; 192 } 193 194 // syntactic sugar 195 public Schedule addType(CodeableConcept t) { // 3 196 if (t == null) 197 return this; 198 if (this.type == null) 199 this.type = new ArrayList<CodeableConcept>(); 200 this.type.add(t); 201 return this; 202 } 203 204 /** 205 * @return {@link #actor} (The resource this Schedule resource is providing 206 * availability information for. These are expected to usually be one of 207 * HealthcareService, Location, Practitioner, Device, Patient or 208 * RelatedPerson.) 209 */ 210 public Reference getActor() { 211 if (this.actor == null) 212 if (Configuration.errorOnAutoCreate()) 213 throw new Error("Attempt to auto-create Schedule.actor"); 214 else if (Configuration.doAutoCreate()) 215 this.actor = new Reference(); // cc 216 return this.actor; 217 } 218 219 public boolean hasActor() { 220 return this.actor != null && !this.actor.isEmpty(); 221 } 222 223 /** 224 * @param value {@link #actor} (The resource this Schedule resource is providing 225 * availability information for. These are expected to usually be 226 * one of HealthcareService, Location, Practitioner, Device, 227 * Patient or RelatedPerson.) 228 */ 229 public Schedule setActor(Reference value) { 230 this.actor = value; 231 return this; 232 } 233 234 /** 235 * @return {@link #actor} The actual object that is the target of the reference. 236 * The reference library doesn't populate this, but you can use it to 237 * hold the resource if you resolve it. (The resource this Schedule 238 * resource is providing availability information for. These are 239 * expected to usually be one of HealthcareService, Location, 240 * Practitioner, Device, Patient or RelatedPerson.) 241 */ 242 public Resource getActorTarget() { 243 return this.actorTarget; 244 } 245 246 /** 247 * @param value {@link #actor} The actual object that is the target of the 248 * reference. The reference library doesn't use these, but you can 249 * use it to hold the resource if you resolve it. (The resource 250 * this Schedule resource is providing availability information 251 * for. These are expected to usually be one of HealthcareService, 252 * Location, Practitioner, Device, Patient or RelatedPerson.) 253 */ 254 public Schedule setActorTarget(Resource value) { 255 this.actorTarget = value; 256 return this; 257 } 258 259 /** 260 * @return {@link #planningHorizon} (The period of time that the slots that are 261 * attached to this Schedule resource cover (even if none exist). These 262 * cover the amount of time that an organization's planning horizon; the 263 * interval for which they are currently accepting appointments. This 264 * does not define a "template" for planning outside these dates.) 265 */ 266 public Period getPlanningHorizon() { 267 if (this.planningHorizon == null) 268 if (Configuration.errorOnAutoCreate()) 269 throw new Error("Attempt to auto-create Schedule.planningHorizon"); 270 else if (Configuration.doAutoCreate()) 271 this.planningHorizon = new Period(); // cc 272 return this.planningHorizon; 273 } 274 275 public boolean hasPlanningHorizon() { 276 return this.planningHorizon != null && !this.planningHorizon.isEmpty(); 277 } 278 279 /** 280 * @param value {@link #planningHorizon} (The period of time that the slots that 281 * are attached to this Schedule resource cover (even if none 282 * exist). These cover the amount of time that an organization's 283 * planning horizon; the interval for which they are currently 284 * accepting appointments. This does not define a "template" for 285 * planning outside these dates.) 286 */ 287 public Schedule setPlanningHorizon(Period value) { 288 this.planningHorizon = value; 289 return this; 290 } 291 292 /** 293 * @return {@link #comment} (Comments on the availability to describe any 294 * extended information. Such as custom constraints on the slot(s) that 295 * may be associated.). This is the underlying object with id, value and 296 * extensions. The accessor "getComment" gives direct access to the 297 * value 298 */ 299 public StringType getCommentElement() { 300 if (this.comment == null) 301 if (Configuration.errorOnAutoCreate()) 302 throw new Error("Attempt to auto-create Schedule.comment"); 303 else if (Configuration.doAutoCreate()) 304 this.comment = new StringType(); // bb 305 return this.comment; 306 } 307 308 public boolean hasCommentElement() { 309 return this.comment != null && !this.comment.isEmpty(); 310 } 311 312 public boolean hasComment() { 313 return this.comment != null && !this.comment.isEmpty(); 314 } 315 316 /** 317 * @param value {@link #comment} (Comments on the availability to describe any 318 * extended information. Such as custom constraints on the slot(s) 319 * that may be associated.). This is the underlying object with id, 320 * value and extensions. The accessor "getComment" gives direct 321 * access to the value 322 */ 323 public Schedule setCommentElement(StringType value) { 324 this.comment = value; 325 return this; 326 } 327 328 /** 329 * @return Comments on the availability to describe any extended information. 330 * Such as custom constraints on the slot(s) that may be associated. 331 */ 332 public String getComment() { 333 return this.comment == null ? null : this.comment.getValue(); 334 } 335 336 /** 337 * @param value Comments on the availability to describe any extended 338 * information. Such as custom constraints on the slot(s) that may 339 * be associated. 340 */ 341 public Schedule setComment(String value) { 342 if (Utilities.noString(value)) 343 this.comment = null; 344 else { 345 if (this.comment == null) 346 this.comment = new StringType(); 347 this.comment.setValue(value); 348 } 349 return this; 350 } 351 352 protected void listChildren(List<Property> childrenList) { 353 super.listChildren(childrenList); 354 childrenList.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, 355 java.lang.Integer.MAX_VALUE, identifier)); 356 childrenList.add(new Property("type", "CodeableConcept", 357 "The schedule type can be used for the categorization of healthcare services or other appointment types.", 0, 358 java.lang.Integer.MAX_VALUE, type)); 359 childrenList.add(new Property("actor", 360 "Reference(Patient|Practitioner|RelatedPerson|Device|HealthcareService|Location)", 361 "The resource this Schedule resource is providing availability information for. These are expected to usually be one of HealthcareService, Location, Practitioner, Device, Patient or RelatedPerson.", 362 0, java.lang.Integer.MAX_VALUE, actor)); 363 childrenList.add(new Property("planningHorizon", "Period", 364 "The period of time that the slots that are attached to this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 365 0, java.lang.Integer.MAX_VALUE, planningHorizon)); 366 childrenList.add(new Property("comment", "string", 367 "Comments on the availability to describe any extended information. Such as custom constraints on the slot(s) that may be associated.", 368 0, java.lang.Integer.MAX_VALUE, comment)); 369 } 370 371 @Override 372 public void setProperty(String name, Base value) throws FHIRException { 373 if (name.equals("identifier")) 374 this.getIdentifier().add(castToIdentifier(value)); 375 else if (name.equals("type")) 376 this.getType().add(castToCodeableConcept(value)); 377 else if (name.equals("actor")) 378 this.actor = castToReference(value); // Reference 379 else if (name.equals("planningHorizon")) 380 this.planningHorizon = castToPeriod(value); // Period 381 else if (name.equals("comment")) 382 this.comment = castToString(value); // StringType 383 else 384 super.setProperty(name, value); 385 } 386 387 @Override 388 public Base addChild(String name) throws FHIRException { 389 if (name.equals("identifier")) { 390 return addIdentifier(); 391 } else if (name.equals("type")) { 392 return addType(); 393 } else if (name.equals("actor")) { 394 this.actor = new Reference(); 395 return this.actor; 396 } else if (name.equals("planningHorizon")) { 397 this.planningHorizon = new Period(); 398 return this.planningHorizon; 399 } else if (name.equals("comment")) { 400 throw new FHIRException("Cannot call addChild on a singleton property Schedule.comment"); 401 } else 402 return super.addChild(name); 403 } 404 405 public String fhirType() { 406 return "Schedule"; 407 408 } 409 410 public Schedule copy() { 411 Schedule dst = new Schedule(); 412 copyValues(dst); 413 if (identifier != null) { 414 dst.identifier = new ArrayList<Identifier>(); 415 for (Identifier i : identifier) 416 dst.identifier.add(i.copy()); 417 } 418 ; 419 if (type != null) { 420 dst.type = new ArrayList<CodeableConcept>(); 421 for (CodeableConcept i : type) 422 dst.type.add(i.copy()); 423 } 424 ; 425 dst.actor = actor == null ? null : actor.copy(); 426 dst.planningHorizon = planningHorizon == null ? null : planningHorizon.copy(); 427 dst.comment = comment == null ? null : comment.copy(); 428 return dst; 429 } 430 431 protected Schedule typedCopy() { 432 return copy(); 433 } 434 435 @Override 436 public boolean equalsDeep(Base other) { 437 if (!super.equalsDeep(other)) 438 return false; 439 if (!(other instanceof Schedule)) 440 return false; 441 Schedule o = (Schedule) other; 442 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 443 && compareDeep(actor, o.actor, true) && compareDeep(planningHorizon, o.planningHorizon, true) 444 && compareDeep(comment, o.comment, true); 445 } 446 447 @Override 448 public boolean equalsShallow(Base other) { 449 if (!super.equalsShallow(other)) 450 return false; 451 if (!(other instanceof Schedule)) 452 return false; 453 Schedule o = (Schedule) other; 454 return compareValues(comment, o.comment, true); 455 } 456 457 public boolean isEmpty() { 458 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (type == null || type.isEmpty()) 459 && (actor == null || actor.isEmpty()) && (planningHorizon == null || planningHorizon.isEmpty()) 460 && (comment == null || comment.isEmpty()); 461 } 462 463 @Override 464 public ResourceType getResourceType() { 465 return ResourceType.Schedule; 466 } 467 468 @SearchParamDefinition(name = "actor", path = "Schedule.actor", description = "The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for", type = "reference") 469 public static final String SP_ACTOR = "actor"; 470 @SearchParamDefinition(name = "date", path = "Schedule.planningHorizon", description = "Search for Schedule resources that have a period that contains this date specified", type = "date") 471 public static final String SP_DATE = "date"; 472 @SearchParamDefinition(name = "identifier", path = "Schedule.identifier", description = "A Schedule Identifier", type = "token") 473 public static final String SP_IDENTIFIER = "identifier"; 474 @SearchParamDefinition(name = "type", path = "Schedule.type", description = "The type of appointments that can be booked into associated slot(s)", type = "token") 475 public static final String SP_TYPE = "type"; 476 477}