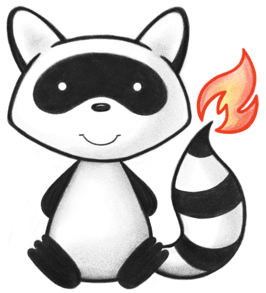
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import org.hl7.fhir.dstu2.model.Enumerations.SearchParamType; 040import org.hl7.fhir.dstu2.model.Enumerations.SearchParamTypeEnumFactory; 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.exceptions.FHIRException; 048import org.hl7.fhir.utilities.Utilities; 049 050/** 051 * A search parameter that defines a named search item that can be used to 052 * search/filter on a resource. 053 */ 054@ResourceDef(name = "SearchParameter", profile = "http://hl7.org/fhir/Profile/SearchParameter") 055public class SearchParameter extends DomainResource { 056 057 public enum XPathUsageType { 058 /** 059 * The search parameter is derived directly from the selected nodes based on the 060 * type definitions. 061 */ 062 NORMAL, 063 /** 064 * The search parameter is derived by a phonetic transform from the selected 065 * nodes. 066 */ 067 PHONETIC, 068 /** 069 * The search parameter is based on a spatial transform of the selected nodes. 070 */ 071 NEARBY, 072 /** 073 * The search parameter is based on a spatial transform of the selected nodes, 074 * using physical distance from the middle. 075 */ 076 DISTANCE, 077 /** 078 * The interpretation of the xpath statement is unknown (and can't be 079 * automated). 080 */ 081 OTHER, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 087 public static XPathUsageType fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("normal".equals(codeString)) 091 return NORMAL; 092 if ("phonetic".equals(codeString)) 093 return PHONETIC; 094 if ("nearby".equals(codeString)) 095 return NEARBY; 096 if ("distance".equals(codeString)) 097 return DISTANCE; 098 if ("other".equals(codeString)) 099 return OTHER; 100 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 101 } 102 103 public String toCode() { 104 switch (this) { 105 case NORMAL: 106 return "normal"; 107 case PHONETIC: 108 return "phonetic"; 109 case NEARBY: 110 return "nearby"; 111 case DISTANCE: 112 return "distance"; 113 case OTHER: 114 return "other"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case NORMAL: 125 return "http://hl7.org/fhir/search-xpath-usage"; 126 case PHONETIC: 127 return "http://hl7.org/fhir/search-xpath-usage"; 128 case NEARBY: 129 return "http://hl7.org/fhir/search-xpath-usage"; 130 case DISTANCE: 131 return "http://hl7.org/fhir/search-xpath-usage"; 132 case OTHER: 133 return "http://hl7.org/fhir/search-xpath-usage"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case NORMAL: 144 return "The search parameter is derived directly from the selected nodes based on the type definitions."; 145 case PHONETIC: 146 return "The search parameter is derived by a phonetic transform from the selected nodes."; 147 case NEARBY: 148 return "The search parameter is based on a spatial transform of the selected nodes."; 149 case DISTANCE: 150 return "The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle."; 151 case OTHER: 152 return "The interpretation of the xpath statement is unknown (and can't be automated)."; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDisplay() { 161 switch (this) { 162 case NORMAL: 163 return "Normal"; 164 case PHONETIC: 165 return "Phonetic"; 166 case NEARBY: 167 return "Nearby"; 168 case DISTANCE: 169 return "Distance"; 170 case OTHER: 171 return "Other"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 } 179 180 public static class XPathUsageTypeEnumFactory implements EnumFactory<XPathUsageType> { 181 public XPathUsageType fromCode(String codeString) throws IllegalArgumentException { 182 if (codeString == null || "".equals(codeString)) 183 if (codeString == null || "".equals(codeString)) 184 return null; 185 if ("normal".equals(codeString)) 186 return XPathUsageType.NORMAL; 187 if ("phonetic".equals(codeString)) 188 return XPathUsageType.PHONETIC; 189 if ("nearby".equals(codeString)) 190 return XPathUsageType.NEARBY; 191 if ("distance".equals(codeString)) 192 return XPathUsageType.DISTANCE; 193 if ("other".equals(codeString)) 194 return XPathUsageType.OTHER; 195 throw new IllegalArgumentException("Unknown XPathUsageType code '" + codeString + "'"); 196 } 197 198 public Enumeration<XPathUsageType> fromType(Base code) throws FHIRException { 199 if (code == null || code.isEmpty()) 200 return null; 201 String codeString = ((PrimitiveType) code).asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("normal".equals(codeString)) 205 return new Enumeration<XPathUsageType>(this, XPathUsageType.NORMAL); 206 if ("phonetic".equals(codeString)) 207 return new Enumeration<XPathUsageType>(this, XPathUsageType.PHONETIC); 208 if ("nearby".equals(codeString)) 209 return new Enumeration<XPathUsageType>(this, XPathUsageType.NEARBY); 210 if ("distance".equals(codeString)) 211 return new Enumeration<XPathUsageType>(this, XPathUsageType.DISTANCE); 212 if ("other".equals(codeString)) 213 return new Enumeration<XPathUsageType>(this, XPathUsageType.OTHER); 214 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 215 } 216 217 public String toCode(XPathUsageType code) 218 { 219 if (code == XPathUsageType.NULL) 220 return null; 221 if (code == XPathUsageType.NORMAL) 222 return "normal"; 223 if (code == XPathUsageType.PHONETIC) 224 return "phonetic"; 225 if (code == XPathUsageType.NEARBY) 226 return "nearby"; 227 if (code == XPathUsageType.DISTANCE) 228 return "distance"; 229 if (code == XPathUsageType.OTHER) 230 return "other"; 231 return "?"; 232 } 233 } 234 235 @Block() 236 public static class SearchParameterContactComponent extends BackboneElement implements IBaseBackboneElement { 237 /** 238 * The name of an individual to contact regarding the search parameter. 239 */ 240 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 241 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the search parameter.") 242 protected StringType name; 243 244 /** 245 * Contact details for individual (if a name was provided) or the publisher. 246 */ 247 @Child(name = "telecom", type = { 248 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 249 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 250 protected List<ContactPoint> telecom; 251 252 private static final long serialVersionUID = -1179697803L; 253 254 /* 255 * Constructor 256 */ 257 public SearchParameterContactComponent() { 258 super(); 259 } 260 261 /** 262 * @return {@link #name} (The name of an individual to contact regarding the 263 * search parameter.). This is the underlying object with id, value and 264 * extensions. The accessor "getName" gives direct access to the value 265 */ 266 public StringType getNameElement() { 267 if (this.name == null) 268 if (Configuration.errorOnAutoCreate()) 269 throw new Error("Attempt to auto-create SearchParameterContactComponent.name"); 270 else if (Configuration.doAutoCreate()) 271 this.name = new StringType(); // bb 272 return this.name; 273 } 274 275 public boolean hasNameElement() { 276 return this.name != null && !this.name.isEmpty(); 277 } 278 279 public boolean hasName() { 280 return this.name != null && !this.name.isEmpty(); 281 } 282 283 /** 284 * @param value {@link #name} (The name of an individual to contact regarding 285 * the search parameter.). This is the underlying object with id, 286 * value and extensions. The accessor "getName" gives direct access 287 * to the value 288 */ 289 public SearchParameterContactComponent setNameElement(StringType value) { 290 this.name = value; 291 return this; 292 } 293 294 /** 295 * @return The name of an individual to contact regarding the search parameter. 296 */ 297 public String getName() { 298 return this.name == null ? null : this.name.getValue(); 299 } 300 301 /** 302 * @param value The name of an individual to contact regarding the search 303 * parameter. 304 */ 305 public SearchParameterContactComponent setName(String value) { 306 if (Utilities.noString(value)) 307 this.name = null; 308 else { 309 if (this.name == null) 310 this.name = new StringType(); 311 this.name.setValue(value); 312 } 313 return this; 314 } 315 316 /** 317 * @return {@link #telecom} (Contact details for individual (if a name was 318 * provided) or the publisher.) 319 */ 320 public List<ContactPoint> getTelecom() { 321 if (this.telecom == null) 322 this.telecom = new ArrayList<ContactPoint>(); 323 return this.telecom; 324 } 325 326 public boolean hasTelecom() { 327 if (this.telecom == null) 328 return false; 329 for (ContactPoint item : this.telecom) 330 if (!item.isEmpty()) 331 return true; 332 return false; 333 } 334 335 /** 336 * @return {@link #telecom} (Contact details for individual (if a name was 337 * provided) or the publisher.) 338 */ 339 // syntactic sugar 340 public ContactPoint addTelecom() { // 3 341 ContactPoint t = new ContactPoint(); 342 if (this.telecom == null) 343 this.telecom = new ArrayList<ContactPoint>(); 344 this.telecom.add(t); 345 return t; 346 } 347 348 // syntactic sugar 349 public SearchParameterContactComponent addTelecom(ContactPoint t) { // 3 350 if (t == null) 351 return this; 352 if (this.telecom == null) 353 this.telecom = new ArrayList<ContactPoint>(); 354 this.telecom.add(t); 355 return this; 356 } 357 358 protected void listChildren(List<Property> childrenList) { 359 super.listChildren(childrenList); 360 childrenList 361 .add(new Property("name", "string", "The name of an individual to contact regarding the search parameter.", 0, 362 java.lang.Integer.MAX_VALUE, name)); 363 childrenList.add(new Property("telecom", "ContactPoint", 364 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 365 telecom)); 366 } 367 368 @Override 369 public void setProperty(String name, Base value) throws FHIRException { 370 if (name.equals("name")) 371 this.name = castToString(value); // StringType 372 else if (name.equals("telecom")) 373 this.getTelecom().add(castToContactPoint(value)); 374 else 375 super.setProperty(name, value); 376 } 377 378 @Override 379 public Base addChild(String name) throws FHIRException { 380 if (name.equals("name")) { 381 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 382 } else if (name.equals("telecom")) { 383 return addTelecom(); 384 } else 385 return super.addChild(name); 386 } 387 388 public SearchParameterContactComponent copy() { 389 SearchParameterContactComponent dst = new SearchParameterContactComponent(); 390 copyValues(dst); 391 dst.name = name == null ? null : name.copy(); 392 if (telecom != null) { 393 dst.telecom = new ArrayList<ContactPoint>(); 394 for (ContactPoint i : telecom) 395 dst.telecom.add(i.copy()); 396 } 397 ; 398 return dst; 399 } 400 401 @Override 402 public boolean equalsDeep(Base other) { 403 if (!super.equalsDeep(other)) 404 return false; 405 if (!(other instanceof SearchParameterContactComponent)) 406 return false; 407 SearchParameterContactComponent o = (SearchParameterContactComponent) other; 408 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 409 } 410 411 @Override 412 public boolean equalsShallow(Base other) { 413 if (!super.equalsShallow(other)) 414 return false; 415 if (!(other instanceof SearchParameterContactComponent)) 416 return false; 417 SearchParameterContactComponent o = (SearchParameterContactComponent) other; 418 return compareValues(name, o.name, true); 419 } 420 421 public boolean isEmpty() { 422 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 423 } 424 425 public String fhirType() { 426 return "SearchParameter.contact"; 427 428 } 429 430 } 431 432 /** 433 * An absolute URL that is used to identify this search parameter when it is 434 * referenced in a specification, model, design or an instance. This SHALL be a 435 * URL, SHOULD be globally unique, and SHOULD be an address at which this search 436 * parameter is (or will be) published. 437 */ 438 @Child(name = "url", type = { UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 439 @Description(shortDefinition = "Absolute URL used to reference this search parameter", formalDefinition = "An absolute URL that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published.") 440 protected UriType url; 441 442 /** 443 * A free text natural language name identifying the search parameter. 444 */ 445 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 446 @Description(shortDefinition = "Informal name for this search parameter", formalDefinition = "A free text natural language name identifying the search parameter.") 447 protected StringType name; 448 449 /** 450 * The status of this search parameter definition. 451 */ 452 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 453 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of this search parameter definition.") 454 protected Enumeration<ConformanceResourceStatus> status; 455 456 /** 457 * A flag to indicate that this search parameter definition is authored for 458 * testing purposes (or education/evaluation/marketing), and is not intended to 459 * be used for genuine usage. 460 */ 461 @Child(name = "experimental", type = { 462 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 463 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "A flag to indicate that this search parameter definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 464 protected BooleanType experimental; 465 466 /** 467 * The name of the individual or organization that published the search 468 * parameter. 469 */ 470 @Child(name = "publisher", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 471 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the search parameter.") 472 protected StringType publisher; 473 474 /** 475 * Contacts to assist a user in finding and communicating with the publisher. 476 */ 477 @Child(name = "contact", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 478 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 479 protected List<SearchParameterContactComponent> contact; 480 481 /** 482 * The date (and optionally time) when the search parameter definition was 483 * published. The date must change when the business version changes, if it 484 * does, and it must change if the status code changes. In addition, it should 485 * change when the substantive content of the search parameter changes. 486 */ 487 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 488 @Description(shortDefinition = "Publication Date(/time)", formalDefinition = "The date (and optionally time) when the search parameter definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.") 489 protected DateTimeType date; 490 491 /** 492 * The Scope and Usage that this search parameter was created to meet. 493 */ 494 @Child(name = "requirements", type = { 495 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 496 @Description(shortDefinition = "Why this search parameter is defined", formalDefinition = "The Scope and Usage that this search parameter was created to meet.") 497 protected StringType requirements; 498 499 /** 500 * The code used in the URL or the parameter name in a parameters resource for 501 * this search parameter. 502 */ 503 @Child(name = "code", type = { CodeType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 504 @Description(shortDefinition = "Code used in URL", formalDefinition = "The code used in the URL or the parameter name in a parameters resource for this search parameter.") 505 protected CodeType code; 506 507 /** 508 * The base resource type that this search parameter refers to. 509 */ 510 @Child(name = "base", type = { CodeType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 511 @Description(shortDefinition = "The resource type this search parameter applies to", formalDefinition = "The base resource type that this search parameter refers to.") 512 protected CodeType base; 513 514 /** 515 * The type of value a search parameter refers to, and how the content is 516 * interpreted. 517 */ 518 @Child(name = "type", type = { CodeType.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 519 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri", formalDefinition = "The type of value a search parameter refers to, and how the content is interpreted.") 520 protected Enumeration<SearchParamType> type; 521 522 /** 523 * A description of the search parameters and how it used. 524 */ 525 @Child(name = "description", type = { 526 StringType.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 527 @Description(shortDefinition = "Documentation for search parameter", formalDefinition = "A description of the search parameters and how it used.") 528 protected StringType description; 529 530 /** 531 * An XPath expression that returns a set of elements for the search parameter. 532 */ 533 @Child(name = "xpath", type = { StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 534 @Description(shortDefinition = "XPath that extracts the values", formalDefinition = "An XPath expression that returns a set of elements for the search parameter.") 535 protected StringType xpath; 536 537 /** 538 * How the search parameter relates to the set of elements returned by 539 * evaluating the xpath query. 540 */ 541 @Child(name = "xpathUsage", type = { 542 CodeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 543 @Description(shortDefinition = "normal | phonetic | nearby | distance | other", formalDefinition = "How the search parameter relates to the set of elements returned by evaluating the xpath query.") 544 protected Enumeration<XPathUsageType> xpathUsage; 545 546 /** 547 * Types of resource (if a resource is referenced). 548 */ 549 @Child(name = "target", type = { 550 CodeType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 551 @Description(shortDefinition = "Types of resource (if a resource reference)", formalDefinition = "Types of resource (if a resource is referenced).") 552 protected List<CodeType> target; 553 554 private static final long serialVersionUID = -742596414L; 555 556 /* 557 * Constructor 558 */ 559 public SearchParameter() { 560 super(); 561 } 562 563 /* 564 * Constructor 565 */ 566 public SearchParameter(UriType url, StringType name, CodeType code, CodeType base, Enumeration<SearchParamType> type, 567 StringType description) { 568 super(); 569 this.url = url; 570 this.name = name; 571 this.code = code; 572 this.base = base; 573 this.type = type; 574 this.description = description; 575 } 576 577 /** 578 * @return {@link #url} (An absolute URL that is used to identify this search 579 * parameter when it is referenced in a specification, model, design or 580 * an instance. This SHALL be a URL, SHOULD be globally unique, and 581 * SHOULD be an address at which this search parameter is (or will be) 582 * published.). This is the underlying object with id, value and 583 * extensions. The accessor "getUrl" gives direct access to the value 584 */ 585 public UriType getUrlElement() { 586 if (this.url == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create SearchParameter.url"); 589 else if (Configuration.doAutoCreate()) 590 this.url = new UriType(); // bb 591 return this.url; 592 } 593 594 public boolean hasUrlElement() { 595 return this.url != null && !this.url.isEmpty(); 596 } 597 598 public boolean hasUrl() { 599 return this.url != null && !this.url.isEmpty(); 600 } 601 602 /** 603 * @param value {@link #url} (An absolute URL that is used to identify this 604 * search parameter when it is referenced in a specification, 605 * model, design or an instance. This SHALL be a URL, SHOULD be 606 * globally unique, and SHOULD be an address at which this search 607 * parameter is (or will be) published.). This is the underlying 608 * object with id, value and extensions. The accessor "getUrl" 609 * gives direct access to the value 610 */ 611 public SearchParameter setUrlElement(UriType value) { 612 this.url = value; 613 return this; 614 } 615 616 /** 617 * @return An absolute URL that is used to identify this search parameter when 618 * it is referenced in a specification, model, design or an instance. 619 * This SHALL be a URL, SHOULD be globally unique, and SHOULD be an 620 * address at which this search parameter is (or will be) published. 621 */ 622 public String getUrl() { 623 return this.url == null ? null : this.url.getValue(); 624 } 625 626 /** 627 * @param value An absolute URL that is used to identify this search parameter 628 * when it is referenced in a specification, model, design or an 629 * instance. This SHALL be a URL, SHOULD be globally unique, and 630 * SHOULD be an address at which this search parameter is (or will 631 * be) published. 632 */ 633 public SearchParameter setUrl(String value) { 634 if (this.url == null) 635 this.url = new UriType(); 636 this.url.setValue(value); 637 return this; 638 } 639 640 /** 641 * @return {@link #name} (A free text natural language name identifying the 642 * search parameter.). This is the underlying object with id, value and 643 * extensions. The accessor "getName" gives direct access to the value 644 */ 645 public StringType getNameElement() { 646 if (this.name == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create SearchParameter.name"); 649 else if (Configuration.doAutoCreate()) 650 this.name = new StringType(); // bb 651 return this.name; 652 } 653 654 public boolean hasNameElement() { 655 return this.name != null && !this.name.isEmpty(); 656 } 657 658 public boolean hasName() { 659 return this.name != null && !this.name.isEmpty(); 660 } 661 662 /** 663 * @param value {@link #name} (A free text natural language name identifying the 664 * search parameter.). This is the underlying object with id, value 665 * and extensions. The accessor "getName" gives direct access to 666 * the value 667 */ 668 public SearchParameter setNameElement(StringType value) { 669 this.name = value; 670 return this; 671 } 672 673 /** 674 * @return A free text natural language name identifying the search parameter. 675 */ 676 public String getName() { 677 return this.name == null ? null : this.name.getValue(); 678 } 679 680 /** 681 * @param value A free text natural language name identifying the search 682 * parameter. 683 */ 684 public SearchParameter setName(String value) { 685 if (this.name == null) 686 this.name = new StringType(); 687 this.name.setValue(value); 688 return this; 689 } 690 691 /** 692 * @return {@link #status} (The status of this search parameter definition.). 693 * This is the underlying object with id, value and extensions. The 694 * accessor "getStatus" gives direct access to the value 695 */ 696 public Enumeration<ConformanceResourceStatus> getStatusElement() { 697 if (this.status == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create SearchParameter.status"); 700 else if (Configuration.doAutoCreate()) 701 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 702 return this.status; 703 } 704 705 public boolean hasStatusElement() { 706 return this.status != null && !this.status.isEmpty(); 707 } 708 709 public boolean hasStatus() { 710 return this.status != null && !this.status.isEmpty(); 711 } 712 713 /** 714 * @param value {@link #status} (The status of this search parameter 715 * definition.). This is the underlying object with id, value and 716 * extensions. The accessor "getStatus" gives direct access to the 717 * value 718 */ 719 public SearchParameter setStatusElement(Enumeration<ConformanceResourceStatus> value) { 720 this.status = value; 721 return this; 722 } 723 724 /** 725 * @return The status of this search parameter definition. 726 */ 727 public ConformanceResourceStatus getStatus() { 728 return this.status == null ? null : this.status.getValue(); 729 } 730 731 /** 732 * @param value The status of this search parameter definition. 733 */ 734 public SearchParameter setStatus(ConformanceResourceStatus value) { 735 if (value == null) 736 this.status = null; 737 else { 738 if (this.status == null) 739 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 740 this.status.setValue(value); 741 } 742 return this; 743 } 744 745 /** 746 * @return {@link #experimental} (A flag to indicate that this search parameter 747 * definition is authored for testing purposes (or 748 * education/evaluation/marketing), and is not intended to be used for 749 * genuine usage.). This is the underlying object with id, value and 750 * extensions. The accessor "getExperimental" gives direct access to the 751 * value 752 */ 753 public BooleanType getExperimentalElement() { 754 if (this.experimental == null) 755 if (Configuration.errorOnAutoCreate()) 756 throw new Error("Attempt to auto-create SearchParameter.experimental"); 757 else if (Configuration.doAutoCreate()) 758 this.experimental = new BooleanType(); // bb 759 return this.experimental; 760 } 761 762 public boolean hasExperimentalElement() { 763 return this.experimental != null && !this.experimental.isEmpty(); 764 } 765 766 public boolean hasExperimental() { 767 return this.experimental != null && !this.experimental.isEmpty(); 768 } 769 770 /** 771 * @param value {@link #experimental} (A flag to indicate that this search 772 * parameter definition is authored for testing purposes (or 773 * education/evaluation/marketing), and is not intended to be used 774 * for genuine usage.). This is the underlying object with id, 775 * value and extensions. The accessor "getExperimental" gives 776 * direct access to the value 777 */ 778 public SearchParameter setExperimentalElement(BooleanType value) { 779 this.experimental = value; 780 return this; 781 } 782 783 /** 784 * @return A flag to indicate that this search parameter definition is authored 785 * for testing purposes (or education/evaluation/marketing), and is not 786 * intended to be used for genuine usage. 787 */ 788 public boolean getExperimental() { 789 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 790 } 791 792 /** 793 * @param value A flag to indicate that this search parameter definition is 794 * authored for testing purposes (or 795 * education/evaluation/marketing), and is not intended to be used 796 * for genuine usage. 797 */ 798 public SearchParameter setExperimental(boolean value) { 799 if (this.experimental == null) 800 this.experimental = new BooleanType(); 801 this.experimental.setValue(value); 802 return this; 803 } 804 805 /** 806 * @return {@link #publisher} (The name of the individual or organization that 807 * published the search parameter.). This is the underlying object with 808 * id, value and extensions. The accessor "getPublisher" gives direct 809 * access to the value 810 */ 811 public StringType getPublisherElement() { 812 if (this.publisher == null) 813 if (Configuration.errorOnAutoCreate()) 814 throw new Error("Attempt to auto-create SearchParameter.publisher"); 815 else if (Configuration.doAutoCreate()) 816 this.publisher = new StringType(); // bb 817 return this.publisher; 818 } 819 820 public boolean hasPublisherElement() { 821 return this.publisher != null && !this.publisher.isEmpty(); 822 } 823 824 public boolean hasPublisher() { 825 return this.publisher != null && !this.publisher.isEmpty(); 826 } 827 828 /** 829 * @param value {@link #publisher} (The name of the individual or organization 830 * that published the search parameter.). This is the underlying 831 * object with id, value and extensions. The accessor 832 * "getPublisher" gives direct access to the value 833 */ 834 public SearchParameter setPublisherElement(StringType value) { 835 this.publisher = value; 836 return this; 837 } 838 839 /** 840 * @return The name of the individual or organization that published the search 841 * parameter. 842 */ 843 public String getPublisher() { 844 return this.publisher == null ? null : this.publisher.getValue(); 845 } 846 847 /** 848 * @param value The name of the individual or organization that published the 849 * search parameter. 850 */ 851 public SearchParameter setPublisher(String value) { 852 if (Utilities.noString(value)) 853 this.publisher = null; 854 else { 855 if (this.publisher == null) 856 this.publisher = new StringType(); 857 this.publisher.setValue(value); 858 } 859 return this; 860 } 861 862 /** 863 * @return {@link #contact} (Contacts to assist a user in finding and 864 * communicating with the publisher.) 865 */ 866 public List<SearchParameterContactComponent> getContact() { 867 if (this.contact == null) 868 this.contact = new ArrayList<SearchParameterContactComponent>(); 869 return this.contact; 870 } 871 872 public boolean hasContact() { 873 if (this.contact == null) 874 return false; 875 for (SearchParameterContactComponent item : this.contact) 876 if (!item.isEmpty()) 877 return true; 878 return false; 879 } 880 881 /** 882 * @return {@link #contact} (Contacts to assist a user in finding and 883 * communicating with the publisher.) 884 */ 885 // syntactic sugar 886 public SearchParameterContactComponent addContact() { // 3 887 SearchParameterContactComponent t = new SearchParameterContactComponent(); 888 if (this.contact == null) 889 this.contact = new ArrayList<SearchParameterContactComponent>(); 890 this.contact.add(t); 891 return t; 892 } 893 894 // syntactic sugar 895 public SearchParameter addContact(SearchParameterContactComponent t) { // 3 896 if (t == null) 897 return this; 898 if (this.contact == null) 899 this.contact = new ArrayList<SearchParameterContactComponent>(); 900 this.contact.add(t); 901 return this; 902 } 903 904 /** 905 * @return {@link #date} (The date (and optionally time) when the search 906 * parameter definition was published. The date must change when the 907 * business version changes, if it does, and it must change if the 908 * status code changes. In addition, it should change when the 909 * substantive content of the search parameter changes.). This is the 910 * underlying object with id, value and extensions. The accessor 911 * "getDate" gives direct access to the value 912 */ 913 public DateTimeType getDateElement() { 914 if (this.date == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create SearchParameter.date"); 917 else if (Configuration.doAutoCreate()) 918 this.date = new DateTimeType(); // bb 919 return this.date; 920 } 921 922 public boolean hasDateElement() { 923 return this.date != null && !this.date.isEmpty(); 924 } 925 926 public boolean hasDate() { 927 return this.date != null && !this.date.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #date} (The date (and optionally time) when the search 932 * parameter definition was published. The date must change when 933 * the business version changes, if it does, and it must change if 934 * the status code changes. In addition, it should change when the 935 * substantive content of the search parameter changes.). This is 936 * the underlying object with id, value and extensions. The 937 * accessor "getDate" gives direct access to the value 938 */ 939 public SearchParameter setDateElement(DateTimeType value) { 940 this.date = value; 941 return this; 942 } 943 944 /** 945 * @return The date (and optionally time) when the search parameter definition 946 * was published. The date must change when the business version 947 * changes, if it does, and it must change if the status code changes. 948 * In addition, it should change when the substantive content of the 949 * search parameter changes. 950 */ 951 public Date getDate() { 952 return this.date == null ? null : this.date.getValue(); 953 } 954 955 /** 956 * @param value The date (and optionally time) when the search parameter 957 * definition was published. The date must change when the business 958 * version changes, if it does, and it must change if the status 959 * code changes. In addition, it should change when the substantive 960 * content of the search parameter changes. 961 */ 962 public SearchParameter setDate(Date value) { 963 if (value == null) 964 this.date = null; 965 else { 966 if (this.date == null) 967 this.date = new DateTimeType(); 968 this.date.setValue(value); 969 } 970 return this; 971 } 972 973 /** 974 * @return {@link #requirements} (The Scope and Usage that this search parameter 975 * was created to meet.). This is the underlying object with id, value 976 * and extensions. The accessor "getRequirements" gives direct access to 977 * the value 978 */ 979 public StringType getRequirementsElement() { 980 if (this.requirements == null) 981 if (Configuration.errorOnAutoCreate()) 982 throw new Error("Attempt to auto-create SearchParameter.requirements"); 983 else if (Configuration.doAutoCreate()) 984 this.requirements = new StringType(); // bb 985 return this.requirements; 986 } 987 988 public boolean hasRequirementsElement() { 989 return this.requirements != null && !this.requirements.isEmpty(); 990 } 991 992 public boolean hasRequirements() { 993 return this.requirements != null && !this.requirements.isEmpty(); 994 } 995 996 /** 997 * @param value {@link #requirements} (The Scope and Usage that this search 998 * parameter was created to meet.). This is the underlying object 999 * with id, value and extensions. The accessor "getRequirements" 1000 * gives direct access to the value 1001 */ 1002 public SearchParameter setRequirementsElement(StringType value) { 1003 this.requirements = value; 1004 return this; 1005 } 1006 1007 /** 1008 * @return The Scope and Usage that this search parameter was created to meet. 1009 */ 1010 public String getRequirements() { 1011 return this.requirements == null ? null : this.requirements.getValue(); 1012 } 1013 1014 /** 1015 * @param value The Scope and Usage that this search parameter was created to 1016 * meet. 1017 */ 1018 public SearchParameter setRequirements(String value) { 1019 if (Utilities.noString(value)) 1020 this.requirements = null; 1021 else { 1022 if (this.requirements == null) 1023 this.requirements = new StringType(); 1024 this.requirements.setValue(value); 1025 } 1026 return this; 1027 } 1028 1029 /** 1030 * @return {@link #code} (The code used in the URL or the parameter name in a 1031 * parameters resource for this search parameter.). This is the 1032 * underlying object with id, value and extensions. The accessor 1033 * "getCode" gives direct access to the value 1034 */ 1035 public CodeType getCodeElement() { 1036 if (this.code == null) 1037 if (Configuration.errorOnAutoCreate()) 1038 throw new Error("Attempt to auto-create SearchParameter.code"); 1039 else if (Configuration.doAutoCreate()) 1040 this.code = new CodeType(); // bb 1041 return this.code; 1042 } 1043 1044 public boolean hasCodeElement() { 1045 return this.code != null && !this.code.isEmpty(); 1046 } 1047 1048 public boolean hasCode() { 1049 return this.code != null && !this.code.isEmpty(); 1050 } 1051 1052 /** 1053 * @param value {@link #code} (The code used in the URL or the parameter name in 1054 * a parameters resource for this search parameter.). This is the 1055 * underlying object with id, value and extensions. The accessor 1056 * "getCode" gives direct access to the value 1057 */ 1058 public SearchParameter setCodeElement(CodeType value) { 1059 this.code = value; 1060 return this; 1061 } 1062 1063 /** 1064 * @return The code used in the URL or the parameter name in a parameters 1065 * resource for this search parameter. 1066 */ 1067 public String getCode() { 1068 return this.code == null ? null : this.code.getValue(); 1069 } 1070 1071 /** 1072 * @param value The code used in the URL or the parameter name in a parameters 1073 * resource for this search parameter. 1074 */ 1075 public SearchParameter setCode(String value) { 1076 if (this.code == null) 1077 this.code = new CodeType(); 1078 this.code.setValue(value); 1079 return this; 1080 } 1081 1082 /** 1083 * @return {@link #base} (The base resource type that this search parameter 1084 * refers to.). This is the underlying object with id, value and 1085 * extensions. The accessor "getBase" gives direct access to the value 1086 */ 1087 public CodeType getBaseElement() { 1088 if (this.base == null) 1089 if (Configuration.errorOnAutoCreate()) 1090 throw new Error("Attempt to auto-create SearchParameter.base"); 1091 else if (Configuration.doAutoCreate()) 1092 this.base = new CodeType(); // bb 1093 return this.base; 1094 } 1095 1096 public boolean hasBaseElement() { 1097 return this.base != null && !this.base.isEmpty(); 1098 } 1099 1100 public boolean hasBase() { 1101 return this.base != null && !this.base.isEmpty(); 1102 } 1103 1104 /** 1105 * @param value {@link #base} (The base resource type that this search parameter 1106 * refers to.). This is the underlying object with id, value and 1107 * extensions. The accessor "getBase" gives direct access to the 1108 * value 1109 */ 1110 public SearchParameter setBaseElement(CodeType value) { 1111 this.base = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return The base resource type that this search parameter refers to. 1117 */ 1118 public String getBase() { 1119 return this.base == null ? null : this.base.getValue(); 1120 } 1121 1122 /** 1123 * @param value The base resource type that this search parameter refers to. 1124 */ 1125 public SearchParameter setBase(String value) { 1126 if (this.base == null) 1127 this.base = new CodeType(); 1128 this.base.setValue(value); 1129 return this; 1130 } 1131 1132 /** 1133 * @return {@link #type} (The type of value a search parameter refers to, and 1134 * how the content is interpreted.). This is the underlying object with 1135 * id, value and extensions. The accessor "getType" gives direct access 1136 * to the value 1137 */ 1138 public Enumeration<SearchParamType> getTypeElement() { 1139 if (this.type == null) 1140 if (Configuration.errorOnAutoCreate()) 1141 throw new Error("Attempt to auto-create SearchParameter.type"); 1142 else if (Configuration.doAutoCreate()) 1143 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 1144 return this.type; 1145 } 1146 1147 public boolean hasTypeElement() { 1148 return this.type != null && !this.type.isEmpty(); 1149 } 1150 1151 public boolean hasType() { 1152 return this.type != null && !this.type.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #type} (The type of value a search parameter refers to, 1157 * and how the content is interpreted.). This is the underlying 1158 * object with id, value and extensions. The accessor "getType" 1159 * gives direct access to the value 1160 */ 1161 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 1162 this.type = value; 1163 return this; 1164 } 1165 1166 /** 1167 * @return The type of value a search parameter refers to, and how the content 1168 * is interpreted. 1169 */ 1170 public SearchParamType getType() { 1171 return this.type == null ? null : this.type.getValue(); 1172 } 1173 1174 /** 1175 * @param value The type of value a search parameter refers to, and how the 1176 * content is interpreted. 1177 */ 1178 public SearchParameter setType(SearchParamType value) { 1179 if (this.type == null) 1180 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 1181 this.type.setValue(value); 1182 return this; 1183 } 1184 1185 /** 1186 * @return {@link #description} (A description of the search parameters and how 1187 * it used.). This is the underlying object with id, value and 1188 * extensions. The accessor "getDescription" gives direct access to the 1189 * value 1190 */ 1191 public StringType getDescriptionElement() { 1192 if (this.description == null) 1193 if (Configuration.errorOnAutoCreate()) 1194 throw new Error("Attempt to auto-create SearchParameter.description"); 1195 else if (Configuration.doAutoCreate()) 1196 this.description = new StringType(); // bb 1197 return this.description; 1198 } 1199 1200 public boolean hasDescriptionElement() { 1201 return this.description != null && !this.description.isEmpty(); 1202 } 1203 1204 public boolean hasDescription() { 1205 return this.description != null && !this.description.isEmpty(); 1206 } 1207 1208 /** 1209 * @param value {@link #description} (A description of the search parameters and 1210 * how it used.). This is the underlying object with id, value and 1211 * extensions. The accessor "getDescription" gives direct access to 1212 * the value 1213 */ 1214 public SearchParameter setDescriptionElement(StringType value) { 1215 this.description = value; 1216 return this; 1217 } 1218 1219 /** 1220 * @return A description of the search parameters and how it used. 1221 */ 1222 public String getDescription() { 1223 return this.description == null ? null : this.description.getValue(); 1224 } 1225 1226 /** 1227 * @param value A description of the search parameters and how it used. 1228 */ 1229 public SearchParameter setDescription(String value) { 1230 if (this.description == null) 1231 this.description = new StringType(); 1232 this.description.setValue(value); 1233 return this; 1234 } 1235 1236 /** 1237 * @return {@link #xpath} (An XPath expression that returns a set of elements 1238 * for the search parameter.). This is the underlying object with id, 1239 * value and extensions. The accessor "getXpath" gives direct access to 1240 * the value 1241 */ 1242 public StringType getXpathElement() { 1243 if (this.xpath == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create SearchParameter.xpath"); 1246 else if (Configuration.doAutoCreate()) 1247 this.xpath = new StringType(); // bb 1248 return this.xpath; 1249 } 1250 1251 public boolean hasXpathElement() { 1252 return this.xpath != null && !this.xpath.isEmpty(); 1253 } 1254 1255 public boolean hasXpath() { 1256 return this.xpath != null && !this.xpath.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #xpath} (An XPath expression that returns a set of 1261 * elements for the search parameter.). This is the underlying 1262 * object with id, value and extensions. The accessor "getXpath" 1263 * gives direct access to the value 1264 */ 1265 public SearchParameter setXpathElement(StringType value) { 1266 this.xpath = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return An XPath expression that returns a set of elements for the search 1272 * parameter. 1273 */ 1274 public String getXpath() { 1275 return this.xpath == null ? null : this.xpath.getValue(); 1276 } 1277 1278 /** 1279 * @param value An XPath expression that returns a set of elements for the 1280 * search parameter. 1281 */ 1282 public SearchParameter setXpath(String value) { 1283 if (Utilities.noString(value)) 1284 this.xpath = null; 1285 else { 1286 if (this.xpath == null) 1287 this.xpath = new StringType(); 1288 this.xpath.setValue(value); 1289 } 1290 return this; 1291 } 1292 1293 /** 1294 * @return {@link #xpathUsage} (How the search parameter relates to the set of 1295 * elements returned by evaluating the xpath query.). This is the 1296 * underlying object with id, value and extensions. The accessor 1297 * "getXpathUsage" gives direct access to the value 1298 */ 1299 public Enumeration<XPathUsageType> getXpathUsageElement() { 1300 if (this.xpathUsage == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create SearchParameter.xpathUsage"); 1303 else if (Configuration.doAutoCreate()) 1304 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); // bb 1305 return this.xpathUsage; 1306 } 1307 1308 public boolean hasXpathUsageElement() { 1309 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1310 } 1311 1312 public boolean hasXpathUsage() { 1313 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #xpathUsage} (How the search parameter relates to the set 1318 * of elements returned by evaluating the xpath query.). This is 1319 * the underlying object with id, value and extensions. The 1320 * accessor "getXpathUsage" gives direct access to the value 1321 */ 1322 public SearchParameter setXpathUsageElement(Enumeration<XPathUsageType> value) { 1323 this.xpathUsage = value; 1324 return this; 1325 } 1326 1327 /** 1328 * @return How the search parameter relates to the set of elements returned by 1329 * evaluating the xpath query. 1330 */ 1331 public XPathUsageType getXpathUsage() { 1332 return this.xpathUsage == null ? null : this.xpathUsage.getValue(); 1333 } 1334 1335 /** 1336 * @param value How the search parameter relates to the set of elements returned 1337 * by evaluating the xpath query. 1338 */ 1339 public SearchParameter setXpathUsage(XPathUsageType value) { 1340 if (value == null) 1341 this.xpathUsage = null; 1342 else { 1343 if (this.xpathUsage == null) 1344 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); 1345 this.xpathUsage.setValue(value); 1346 } 1347 return this; 1348 } 1349 1350 /** 1351 * @return {@link #target} (Types of resource (if a resource is referenced).) 1352 */ 1353 public List<CodeType> getTarget() { 1354 if (this.target == null) 1355 this.target = new ArrayList<CodeType>(); 1356 return this.target; 1357 } 1358 1359 public boolean hasTarget() { 1360 if (this.target == null) 1361 return false; 1362 for (CodeType item : this.target) 1363 if (!item.isEmpty()) 1364 return true; 1365 return false; 1366 } 1367 1368 /** 1369 * @return {@link #target} (Types of resource (if a resource is referenced).) 1370 */ 1371 // syntactic sugar 1372 public CodeType addTargetElement() {// 2 1373 CodeType t = new CodeType(); 1374 if (this.target == null) 1375 this.target = new ArrayList<CodeType>(); 1376 this.target.add(t); 1377 return t; 1378 } 1379 1380 /** 1381 * @param value {@link #target} (Types of resource (if a resource is 1382 * referenced).) 1383 */ 1384 public SearchParameter addTarget(String value) { // 1 1385 CodeType t = new CodeType(); 1386 t.setValue(value); 1387 if (this.target == null) 1388 this.target = new ArrayList<CodeType>(); 1389 this.target.add(t); 1390 return this; 1391 } 1392 1393 /** 1394 * @param value {@link #target} (Types of resource (if a resource is 1395 * referenced).) 1396 */ 1397 public boolean hasTarget(String value) { 1398 if (this.target == null) 1399 return false; 1400 for (CodeType v : this.target) 1401 if (v.equals(value)) // code 1402 return true; 1403 return false; 1404 } 1405 1406 protected void listChildren(List<Property> childrenList) { 1407 super.listChildren(childrenList); 1408 childrenList.add(new Property("url", "uri", 1409 "An absolute URL that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published.", 1410 0, java.lang.Integer.MAX_VALUE, url)); 1411 childrenList.add(new Property("name", "string", 1412 "A free text natural language name identifying the search parameter.", 0, java.lang.Integer.MAX_VALUE, name)); 1413 childrenList.add(new Property("status", "code", "The status of this search parameter definition.", 0, 1414 java.lang.Integer.MAX_VALUE, status)); 1415 childrenList.add(new Property("experimental", "boolean", 1416 "A flag to indicate that this search parameter definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 1417 0, java.lang.Integer.MAX_VALUE, experimental)); 1418 childrenList.add(new Property("publisher", "string", 1419 "The name of the individual or organization that published the search parameter.", 0, 1420 java.lang.Integer.MAX_VALUE, publisher)); 1421 childrenList 1422 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 1423 0, java.lang.Integer.MAX_VALUE, contact)); 1424 childrenList.add(new Property("date", "dateTime", 1425 "The date (and optionally time) when the search parameter definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 1426 0, java.lang.Integer.MAX_VALUE, date)); 1427 childrenList.add( 1428 new Property("requirements", "string", "The Scope and Usage that this search parameter was created to meet.", 0, 1429 java.lang.Integer.MAX_VALUE, requirements)); 1430 childrenList.add(new Property("code", "code", 1431 "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1432 java.lang.Integer.MAX_VALUE, code)); 1433 childrenList.add(new Property("base", "code", "The base resource type that this search parameter refers to.", 0, 1434 java.lang.Integer.MAX_VALUE, base)); 1435 childrenList.add(new Property("type", "code", 1436 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1437 java.lang.Integer.MAX_VALUE, type)); 1438 childrenList.add(new Property("description", "string", "A description of the search parameters and how it used.", 0, 1439 java.lang.Integer.MAX_VALUE, description)); 1440 childrenList.add( 1441 new Property("xpath", "string", "An XPath expression that returns a set of elements for the search parameter.", 1442 0, java.lang.Integer.MAX_VALUE, xpath)); 1443 childrenList.add(new Property("xpathUsage", "code", 1444 "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1445 java.lang.Integer.MAX_VALUE, xpathUsage)); 1446 childrenList.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 1447 java.lang.Integer.MAX_VALUE, target)); 1448 } 1449 1450 @Override 1451 public void setProperty(String name, Base value) throws FHIRException { 1452 if (name.equals("url")) 1453 this.url = castToUri(value); // UriType 1454 else if (name.equals("name")) 1455 this.name = castToString(value); // StringType 1456 else if (name.equals("status")) 1457 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 1458 else if (name.equals("experimental")) 1459 this.experimental = castToBoolean(value); // BooleanType 1460 else if (name.equals("publisher")) 1461 this.publisher = castToString(value); // StringType 1462 else if (name.equals("contact")) 1463 this.getContact().add((SearchParameterContactComponent) value); 1464 else if (name.equals("date")) 1465 this.date = castToDateTime(value); // DateTimeType 1466 else if (name.equals("requirements")) 1467 this.requirements = castToString(value); // StringType 1468 else if (name.equals("code")) 1469 this.code = castToCode(value); // CodeType 1470 else if (name.equals("base")) 1471 this.base = castToCode(value); // CodeType 1472 else if (name.equals("type")) 1473 this.type = new SearchParamTypeEnumFactory().fromType(value); // Enumeration<SearchParamType> 1474 else if (name.equals("description")) 1475 this.description = castToString(value); // StringType 1476 else if (name.equals("xpath")) 1477 this.xpath = castToString(value); // StringType 1478 else if (name.equals("xpathUsage")) 1479 this.xpathUsage = new XPathUsageTypeEnumFactory().fromType(value); // Enumeration<XPathUsageType> 1480 else if (name.equals("target")) 1481 this.getTarget().add(castToCode(value)); 1482 else 1483 super.setProperty(name, value); 1484 } 1485 1486 @Override 1487 public Base addChild(String name) throws FHIRException { 1488 if (name.equals("url")) { 1489 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 1490 } else if (name.equals("name")) { 1491 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 1492 } else if (name.equals("status")) { 1493 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 1494 } else if (name.equals("experimental")) { 1495 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 1496 } else if (name.equals("publisher")) { 1497 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 1498 } else if (name.equals("contact")) { 1499 return addContact(); 1500 } else if (name.equals("date")) { 1501 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 1502 } else if (name.equals("requirements")) { 1503 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.requirements"); 1504 } else if (name.equals("code")) { 1505 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 1506 } else if (name.equals("base")) { 1507 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 1508 } else if (name.equals("type")) { 1509 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 1510 } else if (name.equals("description")) { 1511 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 1512 } else if (name.equals("xpath")) { 1513 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpath"); 1514 } else if (name.equals("xpathUsage")) { 1515 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpathUsage"); 1516 } else if (name.equals("target")) { 1517 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 1518 } else 1519 return super.addChild(name); 1520 } 1521 1522 public String fhirType() { 1523 return "SearchParameter"; 1524 1525 } 1526 1527 public SearchParameter copy() { 1528 SearchParameter dst = new SearchParameter(); 1529 copyValues(dst); 1530 dst.url = url == null ? null : url.copy(); 1531 dst.name = name == null ? null : name.copy(); 1532 dst.status = status == null ? null : status.copy(); 1533 dst.experimental = experimental == null ? null : experimental.copy(); 1534 dst.publisher = publisher == null ? null : publisher.copy(); 1535 if (contact != null) { 1536 dst.contact = new ArrayList<SearchParameterContactComponent>(); 1537 for (SearchParameterContactComponent i : contact) 1538 dst.contact.add(i.copy()); 1539 } 1540 ; 1541 dst.date = date == null ? null : date.copy(); 1542 dst.requirements = requirements == null ? null : requirements.copy(); 1543 dst.code = code == null ? null : code.copy(); 1544 dst.base = base == null ? null : base.copy(); 1545 dst.type = type == null ? null : type.copy(); 1546 dst.description = description == null ? null : description.copy(); 1547 dst.xpath = xpath == null ? null : xpath.copy(); 1548 dst.xpathUsage = xpathUsage == null ? null : xpathUsage.copy(); 1549 if (target != null) { 1550 dst.target = new ArrayList<CodeType>(); 1551 for (CodeType i : target) 1552 dst.target.add(i.copy()); 1553 } 1554 ; 1555 return dst; 1556 } 1557 1558 protected SearchParameter typedCopy() { 1559 return copy(); 1560 } 1561 1562 @Override 1563 public boolean equalsDeep(Base other) { 1564 if (!super.equalsDeep(other)) 1565 return false; 1566 if (!(other instanceof SearchParameter)) 1567 return false; 1568 SearchParameter o = (SearchParameter) other; 1569 return compareDeep(url, o.url, true) && compareDeep(name, o.name, true) && compareDeep(status, o.status, true) 1570 && compareDeep(experimental, o.experimental, true) && compareDeep(publisher, o.publisher, true) 1571 && compareDeep(contact, o.contact, true) && compareDeep(date, o.date, true) 1572 && compareDeep(requirements, o.requirements, true) && compareDeep(code, o.code, true) 1573 && compareDeep(base, o.base, true) && compareDeep(type, o.type, true) 1574 && compareDeep(description, o.description, true) && compareDeep(xpath, o.xpath, true) 1575 && compareDeep(xpathUsage, o.xpathUsage, true) && compareDeep(target, o.target, true); 1576 } 1577 1578 @Override 1579 public boolean equalsShallow(Base other) { 1580 if (!super.equalsShallow(other)) 1581 return false; 1582 if (!(other instanceof SearchParameter)) 1583 return false; 1584 SearchParameter o = (SearchParameter) other; 1585 return compareValues(url, o.url, true) && compareValues(name, o.name, true) && compareValues(status, o.status, true) 1586 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 1587 && compareValues(date, o.date, true) && compareValues(requirements, o.requirements, true) 1588 && compareValues(code, o.code, true) && compareValues(base, o.base, true) && compareValues(type, o.type, true) 1589 && compareValues(description, o.description, true) && compareValues(xpath, o.xpath, true) 1590 && compareValues(xpathUsage, o.xpathUsage, true) && compareValues(target, o.target, true); 1591 } 1592 1593 public boolean isEmpty() { 1594 return super.isEmpty() && (url == null || url.isEmpty()) && (name == null || name.isEmpty()) 1595 && (status == null || status.isEmpty()) && (experimental == null || experimental.isEmpty()) 1596 && (publisher == null || publisher.isEmpty()) && (contact == null || contact.isEmpty()) 1597 && (date == null || date.isEmpty()) && (requirements == null || requirements.isEmpty()) 1598 && (code == null || code.isEmpty()) && (base == null || base.isEmpty()) && (type == null || type.isEmpty()) 1599 && (description == null || description.isEmpty()) && (xpath == null || xpath.isEmpty()) 1600 && (xpathUsage == null || xpathUsage.isEmpty()) && (target == null || target.isEmpty()); 1601 } 1602 1603 @Override 1604 public ResourceType getResourceType() { 1605 return ResourceType.SearchParameter; 1606 } 1607 1608 @SearchParamDefinition(name = "code", path = "SearchParameter.code", description = "Code used in URL", type = "token") 1609 public static final String SP_CODE = "code"; 1610 @SearchParamDefinition(name = "name", path = "SearchParameter.name", description = "Informal name for this search parameter", type = "string") 1611 public static final String SP_NAME = "name"; 1612 @SearchParamDefinition(name = "description", path = "SearchParameter.description", description = "Documentation for search parameter", type = "string") 1613 public static final String SP_DESCRIPTION = "description"; 1614 @SearchParamDefinition(name = "type", path = "SearchParameter.type", description = "number | date | string | token | reference | composite | quantity | uri", type = "token") 1615 public static final String SP_TYPE = "type"; 1616 @SearchParamDefinition(name = "url", path = "SearchParameter.url", description = "Absolute URL used to reference this search parameter", type = "uri") 1617 public static final String SP_URL = "url"; 1618 @SearchParamDefinition(name = "base", path = "SearchParameter.base", description = "The resource type this search parameter applies to", type = "token") 1619 public static final String SP_BASE = "base"; 1620 @SearchParamDefinition(name = "target", path = "SearchParameter.target", description = "Types of resource (if a resource reference)", type = "token") 1621 public static final String SP_TARGET = "target"; 1622 1623}