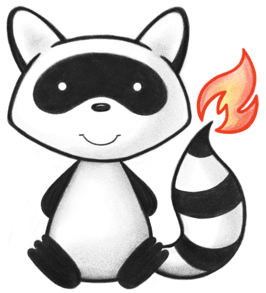
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import org.hl7.fhir.dstu2.model.Enumerations.SearchParamType; 040import org.hl7.fhir.dstu2.model.Enumerations.SearchParamTypeEnumFactory; 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.exceptions.FHIRException; 048import org.hl7.fhir.utilities.Utilities; 049 050/** 051 * A search parameter that defines a named search item that can be used to 052 * search/filter on a resource. 053 */ 054@ResourceDef(name = "SearchParameter", profile = "http://hl7.org/fhir/Profile/SearchParameter") 055public class SearchParameter extends DomainResource { 056 057 public enum XPathUsageType { 058 /** 059 * The search parameter is derived directly from the selected nodes based on the 060 * type definitions. 061 */ 062 NORMAL, 063 /** 064 * The search parameter is derived by a phonetic transform from the selected 065 * nodes. 066 */ 067 PHONETIC, 068 /** 069 * The search parameter is based on a spatial transform of the selected nodes. 070 */ 071 NEARBY, 072 /** 073 * The search parameter is based on a spatial transform of the selected nodes, 074 * using physical distance from the middle. 075 */ 076 DISTANCE, 077 /** 078 * The interpretation of the xpath statement is unknown (and can't be 079 * automated). 080 */ 081 OTHER, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 087 public static XPathUsageType fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("normal".equals(codeString)) 091 return NORMAL; 092 if ("phonetic".equals(codeString)) 093 return PHONETIC; 094 if ("nearby".equals(codeString)) 095 return NEARBY; 096 if ("distance".equals(codeString)) 097 return DISTANCE; 098 if ("other".equals(codeString)) 099 return OTHER; 100 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 101 } 102 103 public String toCode() { 104 switch (this) { 105 case NORMAL: 106 return "normal"; 107 case PHONETIC: 108 return "phonetic"; 109 case NEARBY: 110 return "nearby"; 111 case DISTANCE: 112 return "distance"; 113 case OTHER: 114 return "other"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case NORMAL: 125 return "http://hl7.org/fhir/search-xpath-usage"; 126 case PHONETIC: 127 return "http://hl7.org/fhir/search-xpath-usage"; 128 case NEARBY: 129 return "http://hl7.org/fhir/search-xpath-usage"; 130 case DISTANCE: 131 return "http://hl7.org/fhir/search-xpath-usage"; 132 case OTHER: 133 return "http://hl7.org/fhir/search-xpath-usage"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case NORMAL: 144 return "The search parameter is derived directly from the selected nodes based on the type definitions."; 145 case PHONETIC: 146 return "The search parameter is derived by a phonetic transform from the selected nodes."; 147 case NEARBY: 148 return "The search parameter is based on a spatial transform of the selected nodes."; 149 case DISTANCE: 150 return "The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle."; 151 case OTHER: 152 return "The interpretation of the xpath statement is unknown (and can't be automated)."; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDisplay() { 161 switch (this) { 162 case NORMAL: 163 return "Normal"; 164 case PHONETIC: 165 return "Phonetic"; 166 case NEARBY: 167 return "Nearby"; 168 case DISTANCE: 169 return "Distance"; 170 case OTHER: 171 return "Other"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 } 179 180 public static class XPathUsageTypeEnumFactory implements EnumFactory<XPathUsageType> { 181 public XPathUsageType fromCode(String codeString) throws IllegalArgumentException { 182 if (codeString == null || "".equals(codeString)) 183 if (codeString == null || "".equals(codeString)) 184 return null; 185 if ("normal".equals(codeString)) 186 return XPathUsageType.NORMAL; 187 if ("phonetic".equals(codeString)) 188 return XPathUsageType.PHONETIC; 189 if ("nearby".equals(codeString)) 190 return XPathUsageType.NEARBY; 191 if ("distance".equals(codeString)) 192 return XPathUsageType.DISTANCE; 193 if ("other".equals(codeString)) 194 return XPathUsageType.OTHER; 195 throw new IllegalArgumentException("Unknown XPathUsageType code '" + codeString + "'"); 196 } 197 198 public Enumeration<XPathUsageType> fromType(Base code) throws FHIRException { 199 if (code == null || code.isEmpty()) 200 return null; 201 String codeString = ((PrimitiveType) code).asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("normal".equals(codeString)) 205 return new Enumeration<XPathUsageType>(this, XPathUsageType.NORMAL); 206 if ("phonetic".equals(codeString)) 207 return new Enumeration<XPathUsageType>(this, XPathUsageType.PHONETIC); 208 if ("nearby".equals(codeString)) 209 return new Enumeration<XPathUsageType>(this, XPathUsageType.NEARBY); 210 if ("distance".equals(codeString)) 211 return new Enumeration<XPathUsageType>(this, XPathUsageType.DISTANCE); 212 if ("other".equals(codeString)) 213 return new Enumeration<XPathUsageType>(this, XPathUsageType.OTHER); 214 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 215 } 216 217 public String toCode(XPathUsageType code) { 218 if (code == XPathUsageType.NORMAL) 219 return "normal"; 220 if (code == XPathUsageType.PHONETIC) 221 return "phonetic"; 222 if (code == XPathUsageType.NEARBY) 223 return "nearby"; 224 if (code == XPathUsageType.DISTANCE) 225 return "distance"; 226 if (code == XPathUsageType.OTHER) 227 return "other"; 228 return "?"; 229 } 230 } 231 232 @Block() 233 public static class SearchParameterContactComponent extends BackboneElement implements IBaseBackboneElement { 234 /** 235 * The name of an individual to contact regarding the search parameter. 236 */ 237 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 238 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the search parameter.") 239 protected StringType name; 240 241 /** 242 * Contact details for individual (if a name was provided) or the publisher. 243 */ 244 @Child(name = "telecom", type = { 245 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 246 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 247 protected List<ContactPoint> telecom; 248 249 private static final long serialVersionUID = -1179697803L; 250 251 /* 252 * Constructor 253 */ 254 public SearchParameterContactComponent() { 255 super(); 256 } 257 258 /** 259 * @return {@link #name} (The name of an individual to contact regarding the 260 * search parameter.). This is the underlying object with id, value and 261 * extensions. The accessor "getName" gives direct access to the value 262 */ 263 public StringType getNameElement() { 264 if (this.name == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create SearchParameterContactComponent.name"); 267 else if (Configuration.doAutoCreate()) 268 this.name = new StringType(); // bb 269 return this.name; 270 } 271 272 public boolean hasNameElement() { 273 return this.name != null && !this.name.isEmpty(); 274 } 275 276 public boolean hasName() { 277 return this.name != null && !this.name.isEmpty(); 278 } 279 280 /** 281 * @param value {@link #name} (The name of an individual to contact regarding 282 * the search parameter.). This is the underlying object with id, 283 * value and extensions. The accessor "getName" gives direct access 284 * to the value 285 */ 286 public SearchParameterContactComponent setNameElement(StringType value) { 287 this.name = value; 288 return this; 289 } 290 291 /** 292 * @return The name of an individual to contact regarding the search parameter. 293 */ 294 public String getName() { 295 return this.name == null ? null : this.name.getValue(); 296 } 297 298 /** 299 * @param value The name of an individual to contact regarding the search 300 * parameter. 301 */ 302 public SearchParameterContactComponent setName(String value) { 303 if (Utilities.noString(value)) 304 this.name = null; 305 else { 306 if (this.name == null) 307 this.name = new StringType(); 308 this.name.setValue(value); 309 } 310 return this; 311 } 312 313 /** 314 * @return {@link #telecom} (Contact details for individual (if a name was 315 * provided) or the publisher.) 316 */ 317 public List<ContactPoint> getTelecom() { 318 if (this.telecom == null) 319 this.telecom = new ArrayList<ContactPoint>(); 320 return this.telecom; 321 } 322 323 public boolean hasTelecom() { 324 if (this.telecom == null) 325 return false; 326 for (ContactPoint item : this.telecom) 327 if (!item.isEmpty()) 328 return true; 329 return false; 330 } 331 332 /** 333 * @return {@link #telecom} (Contact details for individual (if a name was 334 * provided) or the publisher.) 335 */ 336 // syntactic sugar 337 public ContactPoint addTelecom() { // 3 338 ContactPoint t = new ContactPoint(); 339 if (this.telecom == null) 340 this.telecom = new ArrayList<ContactPoint>(); 341 this.telecom.add(t); 342 return t; 343 } 344 345 // syntactic sugar 346 public SearchParameterContactComponent addTelecom(ContactPoint t) { // 3 347 if (t == null) 348 return this; 349 if (this.telecom == null) 350 this.telecom = new ArrayList<ContactPoint>(); 351 this.telecom.add(t); 352 return this; 353 } 354 355 protected void listChildren(List<Property> childrenList) { 356 super.listChildren(childrenList); 357 childrenList 358 .add(new Property("name", "string", "The name of an individual to contact regarding the search parameter.", 0, 359 java.lang.Integer.MAX_VALUE, name)); 360 childrenList.add(new Property("telecom", "ContactPoint", 361 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 362 telecom)); 363 } 364 365 @Override 366 public void setProperty(String name, Base value) throws FHIRException { 367 if (name.equals("name")) 368 this.name = castToString(value); // StringType 369 else if (name.equals("telecom")) 370 this.getTelecom().add(castToContactPoint(value)); 371 else 372 super.setProperty(name, value); 373 } 374 375 @Override 376 public Base addChild(String name) throws FHIRException { 377 if (name.equals("name")) { 378 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 379 } else if (name.equals("telecom")) { 380 return addTelecom(); 381 } else 382 return super.addChild(name); 383 } 384 385 public SearchParameterContactComponent copy() { 386 SearchParameterContactComponent dst = new SearchParameterContactComponent(); 387 copyValues(dst); 388 dst.name = name == null ? null : name.copy(); 389 if (telecom != null) { 390 dst.telecom = new ArrayList<ContactPoint>(); 391 for (ContactPoint i : telecom) 392 dst.telecom.add(i.copy()); 393 } 394 ; 395 return dst; 396 } 397 398 @Override 399 public boolean equalsDeep(Base other) { 400 if (!super.equalsDeep(other)) 401 return false; 402 if (!(other instanceof SearchParameterContactComponent)) 403 return false; 404 SearchParameterContactComponent o = (SearchParameterContactComponent) other; 405 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 406 } 407 408 @Override 409 public boolean equalsShallow(Base other) { 410 if (!super.equalsShallow(other)) 411 return false; 412 if (!(other instanceof SearchParameterContactComponent)) 413 return false; 414 SearchParameterContactComponent o = (SearchParameterContactComponent) other; 415 return compareValues(name, o.name, true); 416 } 417 418 public boolean isEmpty() { 419 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 420 } 421 422 public String fhirType() { 423 return "SearchParameter.contact"; 424 425 } 426 427 } 428 429 /** 430 * An absolute URL that is used to identify this search parameter when it is 431 * referenced in a specification, model, design or an instance. This SHALL be a 432 * URL, SHOULD be globally unique, and SHOULD be an address at which this search 433 * parameter is (or will be) published. 434 */ 435 @Child(name = "url", type = { UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 436 @Description(shortDefinition = "Absolute URL used to reference this search parameter", formalDefinition = "An absolute URL that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published.") 437 protected UriType url; 438 439 /** 440 * A free text natural language name identifying the search parameter. 441 */ 442 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 443 @Description(shortDefinition = "Informal name for this search parameter", formalDefinition = "A free text natural language name identifying the search parameter.") 444 protected StringType name; 445 446 /** 447 * The status of this search parameter definition. 448 */ 449 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 450 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of this search parameter definition.") 451 protected Enumeration<ConformanceResourceStatus> status; 452 453 /** 454 * A flag to indicate that this search parameter definition is authored for 455 * testing purposes (or education/evaluation/marketing), and is not intended to 456 * be used for genuine usage. 457 */ 458 @Child(name = "experimental", type = { 459 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 460 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "A flag to indicate that this search parameter definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 461 protected BooleanType experimental; 462 463 /** 464 * The name of the individual or organization that published the search 465 * parameter. 466 */ 467 @Child(name = "publisher", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 468 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the search parameter.") 469 protected StringType publisher; 470 471 /** 472 * Contacts to assist a user in finding and communicating with the publisher. 473 */ 474 @Child(name = "contact", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 475 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 476 protected List<SearchParameterContactComponent> contact; 477 478 /** 479 * The date (and optionally time) when the search parameter definition was 480 * published. The date must change when the business version changes, if it 481 * does, and it must change if the status code changes. In addition, it should 482 * change when the substantive content of the search parameter changes. 483 */ 484 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 485 @Description(shortDefinition = "Publication Date(/time)", formalDefinition = "The date (and optionally time) when the search parameter definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.") 486 protected DateTimeType date; 487 488 /** 489 * The Scope and Usage that this search parameter was created to meet. 490 */ 491 @Child(name = "requirements", type = { 492 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 493 @Description(shortDefinition = "Why this search parameter is defined", formalDefinition = "The Scope and Usage that this search parameter was created to meet.") 494 protected StringType requirements; 495 496 /** 497 * The code used in the URL or the parameter name in a parameters resource for 498 * this search parameter. 499 */ 500 @Child(name = "code", type = { CodeType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 501 @Description(shortDefinition = "Code used in URL", formalDefinition = "The code used in the URL or the parameter name in a parameters resource for this search parameter.") 502 protected CodeType code; 503 504 /** 505 * The base resource type that this search parameter refers to. 506 */ 507 @Child(name = "base", type = { CodeType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 508 @Description(shortDefinition = "The resource type this search parameter applies to", formalDefinition = "The base resource type that this search parameter refers to.") 509 protected CodeType base; 510 511 /** 512 * The type of value a search parameter refers to, and how the content is 513 * interpreted. 514 */ 515 @Child(name = "type", type = { CodeType.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 516 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri", formalDefinition = "The type of value a search parameter refers to, and how the content is interpreted.") 517 protected Enumeration<SearchParamType> type; 518 519 /** 520 * A description of the search parameters and how it used. 521 */ 522 @Child(name = "description", type = { 523 StringType.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 524 @Description(shortDefinition = "Documentation for search parameter", formalDefinition = "A description of the search parameters and how it used.") 525 protected StringType description; 526 527 /** 528 * An XPath expression that returns a set of elements for the search parameter. 529 */ 530 @Child(name = "xpath", type = { StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 531 @Description(shortDefinition = "XPath that extracts the values", formalDefinition = "An XPath expression that returns a set of elements for the search parameter.") 532 protected StringType xpath; 533 534 /** 535 * How the search parameter relates to the set of elements returned by 536 * evaluating the xpath query. 537 */ 538 @Child(name = "xpathUsage", type = { 539 CodeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 540 @Description(shortDefinition = "normal | phonetic | nearby | distance | other", formalDefinition = "How the search parameter relates to the set of elements returned by evaluating the xpath query.") 541 protected Enumeration<XPathUsageType> xpathUsage; 542 543 /** 544 * Types of resource (if a resource is referenced). 545 */ 546 @Child(name = "target", type = { 547 CodeType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 548 @Description(shortDefinition = "Types of resource (if a resource reference)", formalDefinition = "Types of resource (if a resource is referenced).") 549 protected List<CodeType> target; 550 551 private static final long serialVersionUID = -742596414L; 552 553 /* 554 * Constructor 555 */ 556 public SearchParameter() { 557 super(); 558 } 559 560 /* 561 * Constructor 562 */ 563 public SearchParameter(UriType url, StringType name, CodeType code, CodeType base, Enumeration<SearchParamType> type, 564 StringType description) { 565 super(); 566 this.url = url; 567 this.name = name; 568 this.code = code; 569 this.base = base; 570 this.type = type; 571 this.description = description; 572 } 573 574 /** 575 * @return {@link #url} (An absolute URL that is used to identify this search 576 * parameter when it is referenced in a specification, model, design or 577 * an instance. This SHALL be a URL, SHOULD be globally unique, and 578 * SHOULD be an address at which this search parameter is (or will be) 579 * published.). This is the underlying object with id, value and 580 * extensions. The accessor "getUrl" gives direct access to the value 581 */ 582 public UriType getUrlElement() { 583 if (this.url == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create SearchParameter.url"); 586 else if (Configuration.doAutoCreate()) 587 this.url = new UriType(); // bb 588 return this.url; 589 } 590 591 public boolean hasUrlElement() { 592 return this.url != null && !this.url.isEmpty(); 593 } 594 595 public boolean hasUrl() { 596 return this.url != null && !this.url.isEmpty(); 597 } 598 599 /** 600 * @param value {@link #url} (An absolute URL that is used to identify this 601 * search parameter when it is referenced in a specification, 602 * model, design or an instance. This SHALL be a URL, SHOULD be 603 * globally unique, and SHOULD be an address at which this search 604 * parameter is (or will be) published.). This is the underlying 605 * object with id, value and extensions. The accessor "getUrl" 606 * gives direct access to the value 607 */ 608 public SearchParameter setUrlElement(UriType value) { 609 this.url = value; 610 return this; 611 } 612 613 /** 614 * @return An absolute URL that is used to identify this search parameter when 615 * it is referenced in a specification, model, design or an instance. 616 * This SHALL be a URL, SHOULD be globally unique, and SHOULD be an 617 * address at which this search parameter is (or will be) published. 618 */ 619 public String getUrl() { 620 return this.url == null ? null : this.url.getValue(); 621 } 622 623 /** 624 * @param value An absolute URL that is used to identify this search parameter 625 * when it is referenced in a specification, model, design or an 626 * instance. This SHALL be a URL, SHOULD be globally unique, and 627 * SHOULD be an address at which this search parameter is (or will 628 * be) published. 629 */ 630 public SearchParameter setUrl(String value) { 631 if (this.url == null) 632 this.url = new UriType(); 633 this.url.setValue(value); 634 return this; 635 } 636 637 /** 638 * @return {@link #name} (A free text natural language name identifying the 639 * search parameter.). This is the underlying object with id, value and 640 * extensions. The accessor "getName" gives direct access to the value 641 */ 642 public StringType getNameElement() { 643 if (this.name == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create SearchParameter.name"); 646 else if (Configuration.doAutoCreate()) 647 this.name = new StringType(); // bb 648 return this.name; 649 } 650 651 public boolean hasNameElement() { 652 return this.name != null && !this.name.isEmpty(); 653 } 654 655 public boolean hasName() { 656 return this.name != null && !this.name.isEmpty(); 657 } 658 659 /** 660 * @param value {@link #name} (A free text natural language name identifying the 661 * search parameter.). This is the underlying object with id, value 662 * and extensions. The accessor "getName" gives direct access to 663 * the value 664 */ 665 public SearchParameter setNameElement(StringType value) { 666 this.name = value; 667 return this; 668 } 669 670 /** 671 * @return A free text natural language name identifying the search parameter. 672 */ 673 public String getName() { 674 return this.name == null ? null : this.name.getValue(); 675 } 676 677 /** 678 * @param value A free text natural language name identifying the search 679 * parameter. 680 */ 681 public SearchParameter setName(String value) { 682 if (this.name == null) 683 this.name = new StringType(); 684 this.name.setValue(value); 685 return this; 686 } 687 688 /** 689 * @return {@link #status} (The status of this search parameter definition.). 690 * This is the underlying object with id, value and extensions. The 691 * accessor "getStatus" gives direct access to the value 692 */ 693 public Enumeration<ConformanceResourceStatus> getStatusElement() { 694 if (this.status == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create SearchParameter.status"); 697 else if (Configuration.doAutoCreate()) 698 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 699 return this.status; 700 } 701 702 public boolean hasStatusElement() { 703 return this.status != null && !this.status.isEmpty(); 704 } 705 706 public boolean hasStatus() { 707 return this.status != null && !this.status.isEmpty(); 708 } 709 710 /** 711 * @param value {@link #status} (The status of this search parameter 712 * definition.). This is the underlying object with id, value and 713 * extensions. The accessor "getStatus" gives direct access to the 714 * value 715 */ 716 public SearchParameter setStatusElement(Enumeration<ConformanceResourceStatus> value) { 717 this.status = value; 718 return this; 719 } 720 721 /** 722 * @return The status of this search parameter definition. 723 */ 724 public ConformanceResourceStatus getStatus() { 725 return this.status == null ? null : this.status.getValue(); 726 } 727 728 /** 729 * @param value The status of this search parameter definition. 730 */ 731 public SearchParameter setStatus(ConformanceResourceStatus value) { 732 if (value == null) 733 this.status = null; 734 else { 735 if (this.status == null) 736 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 737 this.status.setValue(value); 738 } 739 return this; 740 } 741 742 /** 743 * @return {@link #experimental} (A flag to indicate that this search parameter 744 * definition is authored for testing purposes (or 745 * education/evaluation/marketing), and is not intended to be used for 746 * genuine usage.). This is the underlying object with id, value and 747 * extensions. The accessor "getExperimental" gives direct access to the 748 * value 749 */ 750 public BooleanType getExperimentalElement() { 751 if (this.experimental == null) 752 if (Configuration.errorOnAutoCreate()) 753 throw new Error("Attempt to auto-create SearchParameter.experimental"); 754 else if (Configuration.doAutoCreate()) 755 this.experimental = new BooleanType(); // bb 756 return this.experimental; 757 } 758 759 public boolean hasExperimentalElement() { 760 return this.experimental != null && !this.experimental.isEmpty(); 761 } 762 763 public boolean hasExperimental() { 764 return this.experimental != null && !this.experimental.isEmpty(); 765 } 766 767 /** 768 * @param value {@link #experimental} (A flag to indicate that this search 769 * parameter definition is authored for testing purposes (or 770 * education/evaluation/marketing), and is not intended to be used 771 * for genuine usage.). This is the underlying object with id, 772 * value and extensions. The accessor "getExperimental" gives 773 * direct access to the value 774 */ 775 public SearchParameter setExperimentalElement(BooleanType value) { 776 this.experimental = value; 777 return this; 778 } 779 780 /** 781 * @return A flag to indicate that this search parameter definition is authored 782 * for testing purposes (or education/evaluation/marketing), and is not 783 * intended to be used for genuine usage. 784 */ 785 public boolean getExperimental() { 786 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 787 } 788 789 /** 790 * @param value A flag to indicate that this search parameter definition is 791 * authored for testing purposes (or 792 * education/evaluation/marketing), and is not intended to be used 793 * for genuine usage. 794 */ 795 public SearchParameter setExperimental(boolean value) { 796 if (this.experimental == null) 797 this.experimental = new BooleanType(); 798 this.experimental.setValue(value); 799 return this; 800 } 801 802 /** 803 * @return {@link #publisher} (The name of the individual or organization that 804 * published the search parameter.). This is the underlying object with 805 * id, value and extensions. The accessor "getPublisher" gives direct 806 * access to the value 807 */ 808 public StringType getPublisherElement() { 809 if (this.publisher == null) 810 if (Configuration.errorOnAutoCreate()) 811 throw new Error("Attempt to auto-create SearchParameter.publisher"); 812 else if (Configuration.doAutoCreate()) 813 this.publisher = new StringType(); // bb 814 return this.publisher; 815 } 816 817 public boolean hasPublisherElement() { 818 return this.publisher != null && !this.publisher.isEmpty(); 819 } 820 821 public boolean hasPublisher() { 822 return this.publisher != null && !this.publisher.isEmpty(); 823 } 824 825 /** 826 * @param value {@link #publisher} (The name of the individual or organization 827 * that published the search parameter.). This is the underlying 828 * object with id, value and extensions. The accessor 829 * "getPublisher" gives direct access to the value 830 */ 831 public SearchParameter setPublisherElement(StringType value) { 832 this.publisher = value; 833 return this; 834 } 835 836 /** 837 * @return The name of the individual or organization that published the search 838 * parameter. 839 */ 840 public String getPublisher() { 841 return this.publisher == null ? null : this.publisher.getValue(); 842 } 843 844 /** 845 * @param value The name of the individual or organization that published the 846 * search parameter. 847 */ 848 public SearchParameter setPublisher(String value) { 849 if (Utilities.noString(value)) 850 this.publisher = null; 851 else { 852 if (this.publisher == null) 853 this.publisher = new StringType(); 854 this.publisher.setValue(value); 855 } 856 return this; 857 } 858 859 /** 860 * @return {@link #contact} (Contacts to assist a user in finding and 861 * communicating with the publisher.) 862 */ 863 public List<SearchParameterContactComponent> getContact() { 864 if (this.contact == null) 865 this.contact = new ArrayList<SearchParameterContactComponent>(); 866 return this.contact; 867 } 868 869 public boolean hasContact() { 870 if (this.contact == null) 871 return false; 872 for (SearchParameterContactComponent item : this.contact) 873 if (!item.isEmpty()) 874 return true; 875 return false; 876 } 877 878 /** 879 * @return {@link #contact} (Contacts to assist a user in finding and 880 * communicating with the publisher.) 881 */ 882 // syntactic sugar 883 public SearchParameterContactComponent addContact() { // 3 884 SearchParameterContactComponent t = new SearchParameterContactComponent(); 885 if (this.contact == null) 886 this.contact = new ArrayList<SearchParameterContactComponent>(); 887 this.contact.add(t); 888 return t; 889 } 890 891 // syntactic sugar 892 public SearchParameter addContact(SearchParameterContactComponent t) { // 3 893 if (t == null) 894 return this; 895 if (this.contact == null) 896 this.contact = new ArrayList<SearchParameterContactComponent>(); 897 this.contact.add(t); 898 return this; 899 } 900 901 /** 902 * @return {@link #date} (The date (and optionally time) when the search 903 * parameter definition was published. The date must change when the 904 * business version changes, if it does, and it must change if the 905 * status code changes. In addition, it should change when the 906 * substantive content of the search parameter changes.). This is the 907 * underlying object with id, value and extensions. The accessor 908 * "getDate" gives direct access to the value 909 */ 910 public DateTimeType getDateElement() { 911 if (this.date == null) 912 if (Configuration.errorOnAutoCreate()) 913 throw new Error("Attempt to auto-create SearchParameter.date"); 914 else if (Configuration.doAutoCreate()) 915 this.date = new DateTimeType(); // bb 916 return this.date; 917 } 918 919 public boolean hasDateElement() { 920 return this.date != null && !this.date.isEmpty(); 921 } 922 923 public boolean hasDate() { 924 return this.date != null && !this.date.isEmpty(); 925 } 926 927 /** 928 * @param value {@link #date} (The date (and optionally time) when the search 929 * parameter definition was published. The date must change when 930 * the business version changes, if it does, and it must change if 931 * the status code changes. In addition, it should change when the 932 * substantive content of the search parameter changes.). This is 933 * the underlying object with id, value and extensions. The 934 * accessor "getDate" gives direct access to the value 935 */ 936 public SearchParameter setDateElement(DateTimeType value) { 937 this.date = value; 938 return this; 939 } 940 941 /** 942 * @return The date (and optionally time) when the search parameter definition 943 * was published. The date must change when the business version 944 * changes, if it does, and it must change if the status code changes. 945 * In addition, it should change when the substantive content of the 946 * search parameter changes. 947 */ 948 public Date getDate() { 949 return this.date == null ? null : this.date.getValue(); 950 } 951 952 /** 953 * @param value The date (and optionally time) when the search parameter 954 * definition was published. The date must change when the business 955 * version changes, if it does, and it must change if the status 956 * code changes. In addition, it should change when the substantive 957 * content of the search parameter changes. 958 */ 959 public SearchParameter setDate(Date value) { 960 if (value == null) 961 this.date = null; 962 else { 963 if (this.date == null) 964 this.date = new DateTimeType(); 965 this.date.setValue(value); 966 } 967 return this; 968 } 969 970 /** 971 * @return {@link #requirements} (The Scope and Usage that this search parameter 972 * was created to meet.). This is the underlying object with id, value 973 * and extensions. The accessor "getRequirements" gives direct access to 974 * the value 975 */ 976 public StringType getRequirementsElement() { 977 if (this.requirements == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create SearchParameter.requirements"); 980 else if (Configuration.doAutoCreate()) 981 this.requirements = new StringType(); // bb 982 return this.requirements; 983 } 984 985 public boolean hasRequirementsElement() { 986 return this.requirements != null && !this.requirements.isEmpty(); 987 } 988 989 public boolean hasRequirements() { 990 return this.requirements != null && !this.requirements.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #requirements} (The Scope and Usage that this search 995 * parameter was created to meet.). This is the underlying object 996 * with id, value and extensions. The accessor "getRequirements" 997 * gives direct access to the value 998 */ 999 public SearchParameter setRequirementsElement(StringType value) { 1000 this.requirements = value; 1001 return this; 1002 } 1003 1004 /** 1005 * @return The Scope and Usage that this search parameter was created to meet. 1006 */ 1007 public String getRequirements() { 1008 return this.requirements == null ? null : this.requirements.getValue(); 1009 } 1010 1011 /** 1012 * @param value The Scope and Usage that this search parameter was created to 1013 * meet. 1014 */ 1015 public SearchParameter setRequirements(String value) { 1016 if (Utilities.noString(value)) 1017 this.requirements = null; 1018 else { 1019 if (this.requirements == null) 1020 this.requirements = new StringType(); 1021 this.requirements.setValue(value); 1022 } 1023 return this; 1024 } 1025 1026 /** 1027 * @return {@link #code} (The code used in the URL or the parameter name in a 1028 * parameters resource for this search parameter.). This is the 1029 * underlying object with id, value and extensions. The accessor 1030 * "getCode" gives direct access to the value 1031 */ 1032 public CodeType getCodeElement() { 1033 if (this.code == null) 1034 if (Configuration.errorOnAutoCreate()) 1035 throw new Error("Attempt to auto-create SearchParameter.code"); 1036 else if (Configuration.doAutoCreate()) 1037 this.code = new CodeType(); // bb 1038 return this.code; 1039 } 1040 1041 public boolean hasCodeElement() { 1042 return this.code != null && !this.code.isEmpty(); 1043 } 1044 1045 public boolean hasCode() { 1046 return this.code != null && !this.code.isEmpty(); 1047 } 1048 1049 /** 1050 * @param value {@link #code} (The code used in the URL or the parameter name in 1051 * a parameters resource for this search parameter.). This is the 1052 * underlying object with id, value and extensions. The accessor 1053 * "getCode" gives direct access to the value 1054 */ 1055 public SearchParameter setCodeElement(CodeType value) { 1056 this.code = value; 1057 return this; 1058 } 1059 1060 /** 1061 * @return The code used in the URL or the parameter name in a parameters 1062 * resource for this search parameter. 1063 */ 1064 public String getCode() { 1065 return this.code == null ? null : this.code.getValue(); 1066 } 1067 1068 /** 1069 * @param value The code used in the URL or the parameter name in a parameters 1070 * resource for this search parameter. 1071 */ 1072 public SearchParameter setCode(String value) { 1073 if (this.code == null) 1074 this.code = new CodeType(); 1075 this.code.setValue(value); 1076 return this; 1077 } 1078 1079 /** 1080 * @return {@link #base} (The base resource type that this search parameter 1081 * refers to.). This is the underlying object with id, value and 1082 * extensions. The accessor "getBase" gives direct access to the value 1083 */ 1084 public CodeType getBaseElement() { 1085 if (this.base == null) 1086 if (Configuration.errorOnAutoCreate()) 1087 throw new Error("Attempt to auto-create SearchParameter.base"); 1088 else if (Configuration.doAutoCreate()) 1089 this.base = new CodeType(); // bb 1090 return this.base; 1091 } 1092 1093 public boolean hasBaseElement() { 1094 return this.base != null && !this.base.isEmpty(); 1095 } 1096 1097 public boolean hasBase() { 1098 return this.base != null && !this.base.isEmpty(); 1099 } 1100 1101 /** 1102 * @param value {@link #base} (The base resource type that this search parameter 1103 * refers to.). This is the underlying object with id, value and 1104 * extensions. The accessor "getBase" gives direct access to the 1105 * value 1106 */ 1107 public SearchParameter setBaseElement(CodeType value) { 1108 this.base = value; 1109 return this; 1110 } 1111 1112 /** 1113 * @return The base resource type that this search parameter refers to. 1114 */ 1115 public String getBase() { 1116 return this.base == null ? null : this.base.getValue(); 1117 } 1118 1119 /** 1120 * @param value The base resource type that this search parameter refers to. 1121 */ 1122 public SearchParameter setBase(String value) { 1123 if (this.base == null) 1124 this.base = new CodeType(); 1125 this.base.setValue(value); 1126 return this; 1127 } 1128 1129 /** 1130 * @return {@link #type} (The type of value a search parameter refers to, and 1131 * how the content is interpreted.). This is the underlying object with 1132 * id, value and extensions. The accessor "getType" gives direct access 1133 * to the value 1134 */ 1135 public Enumeration<SearchParamType> getTypeElement() { 1136 if (this.type == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error("Attempt to auto-create SearchParameter.type"); 1139 else if (Configuration.doAutoCreate()) 1140 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 1141 return this.type; 1142 } 1143 1144 public boolean hasTypeElement() { 1145 return this.type != null && !this.type.isEmpty(); 1146 } 1147 1148 public boolean hasType() { 1149 return this.type != null && !this.type.isEmpty(); 1150 } 1151 1152 /** 1153 * @param value {@link #type} (The type of value a search parameter refers to, 1154 * and how the content is interpreted.). This is the underlying 1155 * object with id, value and extensions. The accessor "getType" 1156 * gives direct access to the value 1157 */ 1158 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 1159 this.type = value; 1160 return this; 1161 } 1162 1163 /** 1164 * @return The type of value a search parameter refers to, and how the content 1165 * is interpreted. 1166 */ 1167 public SearchParamType getType() { 1168 return this.type == null ? null : this.type.getValue(); 1169 } 1170 1171 /** 1172 * @param value The type of value a search parameter refers to, and how the 1173 * content is interpreted. 1174 */ 1175 public SearchParameter setType(SearchParamType value) { 1176 if (this.type == null) 1177 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 1178 this.type.setValue(value); 1179 return this; 1180 } 1181 1182 /** 1183 * @return {@link #description} (A description of the search parameters and how 1184 * it used.). This is the underlying object with id, value and 1185 * extensions. The accessor "getDescription" gives direct access to the 1186 * value 1187 */ 1188 public StringType getDescriptionElement() { 1189 if (this.description == null) 1190 if (Configuration.errorOnAutoCreate()) 1191 throw new Error("Attempt to auto-create SearchParameter.description"); 1192 else if (Configuration.doAutoCreate()) 1193 this.description = new StringType(); // bb 1194 return this.description; 1195 } 1196 1197 public boolean hasDescriptionElement() { 1198 return this.description != null && !this.description.isEmpty(); 1199 } 1200 1201 public boolean hasDescription() { 1202 return this.description != null && !this.description.isEmpty(); 1203 } 1204 1205 /** 1206 * @param value {@link #description} (A description of the search parameters and 1207 * how it used.). This is the underlying object with id, value and 1208 * extensions. The accessor "getDescription" gives direct access to 1209 * the value 1210 */ 1211 public SearchParameter setDescriptionElement(StringType value) { 1212 this.description = value; 1213 return this; 1214 } 1215 1216 /** 1217 * @return A description of the search parameters and how it used. 1218 */ 1219 public String getDescription() { 1220 return this.description == null ? null : this.description.getValue(); 1221 } 1222 1223 /** 1224 * @param value A description of the search parameters and how it used. 1225 */ 1226 public SearchParameter setDescription(String value) { 1227 if (this.description == null) 1228 this.description = new StringType(); 1229 this.description.setValue(value); 1230 return this; 1231 } 1232 1233 /** 1234 * @return {@link #xpath} (An XPath expression that returns a set of elements 1235 * for the search parameter.). This is the underlying object with id, 1236 * value and extensions. The accessor "getXpath" gives direct access to 1237 * the value 1238 */ 1239 public StringType getXpathElement() { 1240 if (this.xpath == null) 1241 if (Configuration.errorOnAutoCreate()) 1242 throw new Error("Attempt to auto-create SearchParameter.xpath"); 1243 else if (Configuration.doAutoCreate()) 1244 this.xpath = new StringType(); // bb 1245 return this.xpath; 1246 } 1247 1248 public boolean hasXpathElement() { 1249 return this.xpath != null && !this.xpath.isEmpty(); 1250 } 1251 1252 public boolean hasXpath() { 1253 return this.xpath != null && !this.xpath.isEmpty(); 1254 } 1255 1256 /** 1257 * @param value {@link #xpath} (An XPath expression that returns a set of 1258 * elements for the search parameter.). This is the underlying 1259 * object with id, value and extensions. The accessor "getXpath" 1260 * gives direct access to the value 1261 */ 1262 public SearchParameter setXpathElement(StringType value) { 1263 this.xpath = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return An XPath expression that returns a set of elements for the search 1269 * parameter. 1270 */ 1271 public String getXpath() { 1272 return this.xpath == null ? null : this.xpath.getValue(); 1273 } 1274 1275 /** 1276 * @param value An XPath expression that returns a set of elements for the 1277 * search parameter. 1278 */ 1279 public SearchParameter setXpath(String value) { 1280 if (Utilities.noString(value)) 1281 this.xpath = null; 1282 else { 1283 if (this.xpath == null) 1284 this.xpath = new StringType(); 1285 this.xpath.setValue(value); 1286 } 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #xpathUsage} (How the search parameter relates to the set of 1292 * elements returned by evaluating the xpath query.). This is the 1293 * underlying object with id, value and extensions. The accessor 1294 * "getXpathUsage" gives direct access to the value 1295 */ 1296 public Enumeration<XPathUsageType> getXpathUsageElement() { 1297 if (this.xpathUsage == null) 1298 if (Configuration.errorOnAutoCreate()) 1299 throw new Error("Attempt to auto-create SearchParameter.xpathUsage"); 1300 else if (Configuration.doAutoCreate()) 1301 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); // bb 1302 return this.xpathUsage; 1303 } 1304 1305 public boolean hasXpathUsageElement() { 1306 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1307 } 1308 1309 public boolean hasXpathUsage() { 1310 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 1311 } 1312 1313 /** 1314 * @param value {@link #xpathUsage} (How the search parameter relates to the set 1315 * of elements returned by evaluating the xpath query.). This is 1316 * the underlying object with id, value and extensions. The 1317 * accessor "getXpathUsage" gives direct access to the value 1318 */ 1319 public SearchParameter setXpathUsageElement(Enumeration<XPathUsageType> value) { 1320 this.xpathUsage = value; 1321 return this; 1322 } 1323 1324 /** 1325 * @return How the search parameter relates to the set of elements returned by 1326 * evaluating the xpath query. 1327 */ 1328 public XPathUsageType getXpathUsage() { 1329 return this.xpathUsage == null ? null : this.xpathUsage.getValue(); 1330 } 1331 1332 /** 1333 * @param value How the search parameter relates to the set of elements returned 1334 * by evaluating the xpath query. 1335 */ 1336 public SearchParameter setXpathUsage(XPathUsageType value) { 1337 if (value == null) 1338 this.xpathUsage = null; 1339 else { 1340 if (this.xpathUsage == null) 1341 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); 1342 this.xpathUsage.setValue(value); 1343 } 1344 return this; 1345 } 1346 1347 /** 1348 * @return {@link #target} (Types of resource (if a resource is referenced).) 1349 */ 1350 public List<CodeType> getTarget() { 1351 if (this.target == null) 1352 this.target = new ArrayList<CodeType>(); 1353 return this.target; 1354 } 1355 1356 public boolean hasTarget() { 1357 if (this.target == null) 1358 return false; 1359 for (CodeType item : this.target) 1360 if (!item.isEmpty()) 1361 return true; 1362 return false; 1363 } 1364 1365 /** 1366 * @return {@link #target} (Types of resource (if a resource is referenced).) 1367 */ 1368 // syntactic sugar 1369 public CodeType addTargetElement() {// 2 1370 CodeType t = new CodeType(); 1371 if (this.target == null) 1372 this.target = new ArrayList<CodeType>(); 1373 this.target.add(t); 1374 return t; 1375 } 1376 1377 /** 1378 * @param value {@link #target} (Types of resource (if a resource is 1379 * referenced).) 1380 */ 1381 public SearchParameter addTarget(String value) { // 1 1382 CodeType t = new CodeType(); 1383 t.setValue(value); 1384 if (this.target == null) 1385 this.target = new ArrayList<CodeType>(); 1386 this.target.add(t); 1387 return this; 1388 } 1389 1390 /** 1391 * @param value {@link #target} (Types of resource (if a resource is 1392 * referenced).) 1393 */ 1394 public boolean hasTarget(String value) { 1395 if (this.target == null) 1396 return false; 1397 for (CodeType v : this.target) 1398 if (v.equals(value)) // code 1399 return true; 1400 return false; 1401 } 1402 1403 protected void listChildren(List<Property> childrenList) { 1404 super.listChildren(childrenList); 1405 childrenList.add(new Property("url", "uri", 1406 "An absolute URL that is used to identify this search parameter when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this search parameter is (or will be) published.", 1407 0, java.lang.Integer.MAX_VALUE, url)); 1408 childrenList.add(new Property("name", "string", 1409 "A free text natural language name identifying the search parameter.", 0, java.lang.Integer.MAX_VALUE, name)); 1410 childrenList.add(new Property("status", "code", "The status of this search parameter definition.", 0, 1411 java.lang.Integer.MAX_VALUE, status)); 1412 childrenList.add(new Property("experimental", "boolean", 1413 "A flag to indicate that this search parameter definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 1414 0, java.lang.Integer.MAX_VALUE, experimental)); 1415 childrenList.add(new Property("publisher", "string", 1416 "The name of the individual or organization that published the search parameter.", 0, 1417 java.lang.Integer.MAX_VALUE, publisher)); 1418 childrenList 1419 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 1420 0, java.lang.Integer.MAX_VALUE, contact)); 1421 childrenList.add(new Property("date", "dateTime", 1422 "The date (and optionally time) when the search parameter definition was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 1423 0, java.lang.Integer.MAX_VALUE, date)); 1424 childrenList.add( 1425 new Property("requirements", "string", "The Scope and Usage that this search parameter was created to meet.", 0, 1426 java.lang.Integer.MAX_VALUE, requirements)); 1427 childrenList.add(new Property("code", "code", 1428 "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1429 java.lang.Integer.MAX_VALUE, code)); 1430 childrenList.add(new Property("base", "code", "The base resource type that this search parameter refers to.", 0, 1431 java.lang.Integer.MAX_VALUE, base)); 1432 childrenList.add(new Property("type", "code", 1433 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1434 java.lang.Integer.MAX_VALUE, type)); 1435 childrenList.add(new Property("description", "string", "A description of the search parameters and how it used.", 0, 1436 java.lang.Integer.MAX_VALUE, description)); 1437 childrenList.add( 1438 new Property("xpath", "string", "An XPath expression that returns a set of elements for the search parameter.", 1439 0, java.lang.Integer.MAX_VALUE, xpath)); 1440 childrenList.add(new Property("xpathUsage", "code", 1441 "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1442 java.lang.Integer.MAX_VALUE, xpathUsage)); 1443 childrenList.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 1444 java.lang.Integer.MAX_VALUE, target)); 1445 } 1446 1447 @Override 1448 public void setProperty(String name, Base value) throws FHIRException { 1449 if (name.equals("url")) 1450 this.url = castToUri(value); // UriType 1451 else if (name.equals("name")) 1452 this.name = castToString(value); // StringType 1453 else if (name.equals("status")) 1454 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 1455 else if (name.equals("experimental")) 1456 this.experimental = castToBoolean(value); // BooleanType 1457 else if (name.equals("publisher")) 1458 this.publisher = castToString(value); // StringType 1459 else if (name.equals("contact")) 1460 this.getContact().add((SearchParameterContactComponent) value); 1461 else if (name.equals("date")) 1462 this.date = castToDateTime(value); // DateTimeType 1463 else if (name.equals("requirements")) 1464 this.requirements = castToString(value); // StringType 1465 else if (name.equals("code")) 1466 this.code = castToCode(value); // CodeType 1467 else if (name.equals("base")) 1468 this.base = castToCode(value); // CodeType 1469 else if (name.equals("type")) 1470 this.type = new SearchParamTypeEnumFactory().fromType(value); // Enumeration<SearchParamType> 1471 else if (name.equals("description")) 1472 this.description = castToString(value); // StringType 1473 else if (name.equals("xpath")) 1474 this.xpath = castToString(value); // StringType 1475 else if (name.equals("xpathUsage")) 1476 this.xpathUsage = new XPathUsageTypeEnumFactory().fromType(value); // Enumeration<XPathUsageType> 1477 else if (name.equals("target")) 1478 this.getTarget().add(castToCode(value)); 1479 else 1480 super.setProperty(name, value); 1481 } 1482 1483 @Override 1484 public Base addChild(String name) throws FHIRException { 1485 if (name.equals("url")) { 1486 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 1487 } else if (name.equals("name")) { 1488 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 1489 } else if (name.equals("status")) { 1490 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 1491 } else if (name.equals("experimental")) { 1492 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 1493 } else if (name.equals("publisher")) { 1494 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 1495 } else if (name.equals("contact")) { 1496 return addContact(); 1497 } else if (name.equals("date")) { 1498 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 1499 } else if (name.equals("requirements")) { 1500 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.requirements"); 1501 } else if (name.equals("code")) { 1502 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 1503 } else if (name.equals("base")) { 1504 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 1505 } else if (name.equals("type")) { 1506 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 1507 } else if (name.equals("description")) { 1508 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 1509 } else if (name.equals("xpath")) { 1510 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpath"); 1511 } else if (name.equals("xpathUsage")) { 1512 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpathUsage"); 1513 } else if (name.equals("target")) { 1514 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 1515 } else 1516 return super.addChild(name); 1517 } 1518 1519 public String fhirType() { 1520 return "SearchParameter"; 1521 1522 } 1523 1524 public SearchParameter copy() { 1525 SearchParameter dst = new SearchParameter(); 1526 copyValues(dst); 1527 dst.url = url == null ? null : url.copy(); 1528 dst.name = name == null ? null : name.copy(); 1529 dst.status = status == null ? null : status.copy(); 1530 dst.experimental = experimental == null ? null : experimental.copy(); 1531 dst.publisher = publisher == null ? null : publisher.copy(); 1532 if (contact != null) { 1533 dst.contact = new ArrayList<SearchParameterContactComponent>(); 1534 for (SearchParameterContactComponent i : contact) 1535 dst.contact.add(i.copy()); 1536 } 1537 ; 1538 dst.date = date == null ? null : date.copy(); 1539 dst.requirements = requirements == null ? null : requirements.copy(); 1540 dst.code = code == null ? null : code.copy(); 1541 dst.base = base == null ? null : base.copy(); 1542 dst.type = type == null ? null : type.copy(); 1543 dst.description = description == null ? null : description.copy(); 1544 dst.xpath = xpath == null ? null : xpath.copy(); 1545 dst.xpathUsage = xpathUsage == null ? null : xpathUsage.copy(); 1546 if (target != null) { 1547 dst.target = new ArrayList<CodeType>(); 1548 for (CodeType i : target) 1549 dst.target.add(i.copy()); 1550 } 1551 ; 1552 return dst; 1553 } 1554 1555 protected SearchParameter typedCopy() { 1556 return copy(); 1557 } 1558 1559 @Override 1560 public boolean equalsDeep(Base other) { 1561 if (!super.equalsDeep(other)) 1562 return false; 1563 if (!(other instanceof SearchParameter)) 1564 return false; 1565 SearchParameter o = (SearchParameter) other; 1566 return compareDeep(url, o.url, true) && compareDeep(name, o.name, true) && compareDeep(status, o.status, true) 1567 && compareDeep(experimental, o.experimental, true) && compareDeep(publisher, o.publisher, true) 1568 && compareDeep(contact, o.contact, true) && compareDeep(date, o.date, true) 1569 && compareDeep(requirements, o.requirements, true) && compareDeep(code, o.code, true) 1570 && compareDeep(base, o.base, true) && compareDeep(type, o.type, true) 1571 && compareDeep(description, o.description, true) && compareDeep(xpath, o.xpath, true) 1572 && compareDeep(xpathUsage, o.xpathUsage, true) && compareDeep(target, o.target, true); 1573 } 1574 1575 @Override 1576 public boolean equalsShallow(Base other) { 1577 if (!super.equalsShallow(other)) 1578 return false; 1579 if (!(other instanceof SearchParameter)) 1580 return false; 1581 SearchParameter o = (SearchParameter) other; 1582 return compareValues(url, o.url, true) && compareValues(name, o.name, true) && compareValues(status, o.status, true) 1583 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 1584 && compareValues(date, o.date, true) && compareValues(requirements, o.requirements, true) 1585 && compareValues(code, o.code, true) && compareValues(base, o.base, true) && compareValues(type, o.type, true) 1586 && compareValues(description, o.description, true) && compareValues(xpath, o.xpath, true) 1587 && compareValues(xpathUsage, o.xpathUsage, true) && compareValues(target, o.target, true); 1588 } 1589 1590 public boolean isEmpty() { 1591 return super.isEmpty() && (url == null || url.isEmpty()) && (name == null || name.isEmpty()) 1592 && (status == null || status.isEmpty()) && (experimental == null || experimental.isEmpty()) 1593 && (publisher == null || publisher.isEmpty()) && (contact == null || contact.isEmpty()) 1594 && (date == null || date.isEmpty()) && (requirements == null || requirements.isEmpty()) 1595 && (code == null || code.isEmpty()) && (base == null || base.isEmpty()) && (type == null || type.isEmpty()) 1596 && (description == null || description.isEmpty()) && (xpath == null || xpath.isEmpty()) 1597 && (xpathUsage == null || xpathUsage.isEmpty()) && (target == null || target.isEmpty()); 1598 } 1599 1600 @Override 1601 public ResourceType getResourceType() { 1602 return ResourceType.SearchParameter; 1603 } 1604 1605 @SearchParamDefinition(name = "code", path = "SearchParameter.code", description = "Code used in URL", type = "token") 1606 public static final String SP_CODE = "code"; 1607 @SearchParamDefinition(name = "name", path = "SearchParameter.name", description = "Informal name for this search parameter", type = "string") 1608 public static final String SP_NAME = "name"; 1609 @SearchParamDefinition(name = "description", path = "SearchParameter.description", description = "Documentation for search parameter", type = "string") 1610 public static final String SP_DESCRIPTION = "description"; 1611 @SearchParamDefinition(name = "type", path = "SearchParameter.type", description = "number | date | string | token | reference | composite | quantity | uri", type = "token") 1612 public static final String SP_TYPE = "type"; 1613 @SearchParamDefinition(name = "url", path = "SearchParameter.url", description = "Absolute URL used to reference this search parameter", type = "uri") 1614 public static final String SP_URL = "url"; 1615 @SearchParamDefinition(name = "base", path = "SearchParameter.base", description = "The resource type this search parameter applies to", type = "token") 1616 public static final String SP_BASE = "base"; 1617 @SearchParamDefinition(name = "target", path = "SearchParameter.target", description = "Types of resource (if a resource reference)", type = "token") 1618 public static final String SP_TARGET = "target"; 1619 1620}