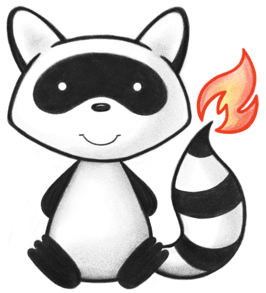
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.DatatypeDef; 039import ca.uhn.fhir.model.api.annotation.Description; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * A digital signature along with supporting context. The signature may be 045 * electronic/cryptographic in nature, or a graphical image representing a 046 * hand-written signature, or a signature process. Different Signature 047 * approaches have different utilities. 048 */ 049@DatatypeDef(name = "Signature") 050public class Signature extends Type implements ICompositeType { 051 052 /** 053 * An indication of the reason that the entity signed this document. This may be 054 * explicitly included as part of the signature information and can be used when 055 * determining accountability for various actions concerning the document. 056 */ 057 @Child(name = "type", type = { 058 Coding.class }, order = 0, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 059 @Description(shortDefinition = "Indication of the reason the entity signed the object(s)", formalDefinition = "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.") 060 protected List<Coding> type; 061 062 /** 063 * When the digital signature was signed. 064 */ 065 @Child(name = "when", type = { InstantType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 066 @Description(shortDefinition = "When the signature was created", formalDefinition = "When the digital signature was signed.") 067 protected InstantType when; 068 069 /** 070 * A reference to an application-usable description of the person that signed 071 * the certificate (e.g. the signature used their private key). 072 */ 073 @Child(name = "who", type = { UriType.class, Practitioner.class, RelatedPerson.class, Patient.class, Device.class, 074 Organization.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 075 @Description(shortDefinition = "Who signed the signature", formalDefinition = "A reference to an application-usable description of the person that signed the certificate (e.g. the signature used their private key).") 076 protected Type who; 077 078 /** 079 * A mime type that indicates the technical format of the signature. Important 080 * mime types are application/signature+xml for X ML DigSig, application/jwt for 081 * JWT, and image/* for a graphical image of a signature. 082 */ 083 @Child(name = "contentType", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 084 @Description(shortDefinition = "The technical format of the signature", formalDefinition = "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature.") 085 protected CodeType contentType; 086 087 /** 088 * The base64 encoding of the Signature content. 089 */ 090 @Child(name = "blob", type = { 091 Base64BinaryType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 092 @Description(shortDefinition = "The actual signature content (XML DigSig. JWT, picture, etc.)", formalDefinition = "The base64 encoding of the Signature content.") 093 protected Base64BinaryType blob; 094 095 private static final long serialVersionUID = -452432714L; 096 097 /* 098 * Constructor 099 */ 100 public Signature() { 101 super(); 102 } 103 104 /* 105 * Constructor 106 */ 107 public Signature(InstantType when, Type who, CodeType contentType, Base64BinaryType blob) { 108 super(); 109 this.when = when; 110 this.who = who; 111 this.contentType = contentType; 112 this.blob = blob; 113 } 114 115 /** 116 * @return {@link #type} (An indication of the reason that the entity signed 117 * this document. This may be explicitly included as part of the 118 * signature information and can be used when determining accountability 119 * for various actions concerning the document.) 120 */ 121 public List<Coding> getType() { 122 if (this.type == null) 123 this.type = new ArrayList<Coding>(); 124 return this.type; 125 } 126 127 public boolean hasType() { 128 if (this.type == null) 129 return false; 130 for (Coding item : this.type) 131 if (!item.isEmpty()) 132 return true; 133 return false; 134 } 135 136 /** 137 * @return {@link #type} (An indication of the reason that the entity signed 138 * this document. This may be explicitly included as part of the 139 * signature information and can be used when determining accountability 140 * for various actions concerning the document.) 141 */ 142 // syntactic sugar 143 public Coding addType() { // 3 144 Coding t = new Coding(); 145 if (this.type == null) 146 this.type = new ArrayList<Coding>(); 147 this.type.add(t); 148 return t; 149 } 150 151 // syntactic sugar 152 public Signature addType(Coding t) { // 3 153 if (t == null) 154 return this; 155 if (this.type == null) 156 this.type = new ArrayList<Coding>(); 157 this.type.add(t); 158 return this; 159 } 160 161 /** 162 * @return {@link #when} (When the digital signature was signed.). This is the 163 * underlying object with id, value and extensions. The accessor 164 * "getWhen" gives direct access to the value 165 */ 166 public InstantType getWhenElement() { 167 if (this.when == null) 168 if (Configuration.errorOnAutoCreate()) 169 throw new Error("Attempt to auto-create Signature.when"); 170 else if (Configuration.doAutoCreate()) 171 this.when = new InstantType(); // bb 172 return this.when; 173 } 174 175 public boolean hasWhenElement() { 176 return this.when != null && !this.when.isEmpty(); 177 } 178 179 public boolean hasWhen() { 180 return this.when != null && !this.when.isEmpty(); 181 } 182 183 /** 184 * @param value {@link #when} (When the digital signature was signed.). This is 185 * the underlying object with id, value and extensions. The 186 * accessor "getWhen" gives direct access to the value 187 */ 188 public Signature setWhenElement(InstantType value) { 189 this.when = value; 190 return this; 191 } 192 193 /** 194 * @return When the digital signature was signed. 195 */ 196 public Date getWhen() { 197 return this.when == null ? null : this.when.getValue(); 198 } 199 200 /** 201 * @param value When the digital signature was signed. 202 */ 203 public Signature setWhen(Date value) { 204 if (this.when == null) 205 this.when = new InstantType(); 206 this.when.setValue(value); 207 return this; 208 } 209 210 /** 211 * @return {@link #who} (A reference to an application-usable description of the 212 * person that signed the certificate (e.g. the signature used their 213 * private key).) 214 */ 215 public Type getWho() { 216 return this.who; 217 } 218 219 /** 220 * @return {@link #who} (A reference to an application-usable description of the 221 * person that signed the certificate (e.g. the signature used their 222 * private key).) 223 */ 224 public UriType getWhoUriType() throws FHIRException { 225 if (!(this.who instanceof UriType)) 226 throw new FHIRException( 227 "Type mismatch: the type UriType was expected, but " + this.who.getClass().getName() + " was encountered"); 228 return (UriType) this.who; 229 } 230 231 public boolean hasWhoUriType() { 232 return this.who instanceof UriType; 233 } 234 235 /** 236 * @return {@link #who} (A reference to an application-usable description of the 237 * person that signed the certificate (e.g. the signature used their 238 * private key).) 239 */ 240 public Reference getWhoReference() throws FHIRException { 241 if (!(this.who instanceof Reference)) 242 throw new FHIRException( 243 "Type mismatch: the type Reference was expected, but " + this.who.getClass().getName() + " was encountered"); 244 return (Reference) this.who; 245 } 246 247 public boolean hasWhoReference() { 248 return this.who instanceof Reference; 249 } 250 251 public boolean hasWho() { 252 return this.who != null && !this.who.isEmpty(); 253 } 254 255 /** 256 * @param value {@link #who} (A reference to an application-usable description 257 * of the person that signed the certificate (e.g. the signature 258 * used their private key).) 259 */ 260 public Signature setWho(Type value) { 261 this.who = value; 262 return this; 263 } 264 265 /** 266 * @return {@link #contentType} (A mime type that indicates the technical format 267 * of the signature. Important mime types are application/signature+xml 268 * for X ML DigSig, application/jwt for JWT, and image/* for a graphical 269 * image of a signature.). This is the underlying object with id, value 270 * and extensions. The accessor "getContentType" gives direct access to 271 * the value 272 */ 273 public CodeType getContentTypeElement() { 274 if (this.contentType == null) 275 if (Configuration.errorOnAutoCreate()) 276 throw new Error("Attempt to auto-create Signature.contentType"); 277 else if (Configuration.doAutoCreate()) 278 this.contentType = new CodeType(); // bb 279 return this.contentType; 280 } 281 282 public boolean hasContentTypeElement() { 283 return this.contentType != null && !this.contentType.isEmpty(); 284 } 285 286 public boolean hasContentType() { 287 return this.contentType != null && !this.contentType.isEmpty(); 288 } 289 290 /** 291 * @param value {@link #contentType} (A mime type that indicates the technical 292 * format of the signature. Important mime types are 293 * application/signature+xml for X ML DigSig, application/jwt for 294 * JWT, and image/* for a graphical image of a signature.). This is 295 * the underlying object with id, value and extensions. The 296 * accessor "getContentType" gives direct access to the value 297 */ 298 public Signature setContentTypeElement(CodeType value) { 299 this.contentType = value; 300 return this; 301 } 302 303 /** 304 * @return A mime type that indicates the technical format of the signature. 305 * Important mime types are application/signature+xml for X ML DigSig, 306 * application/jwt for JWT, and image/* for a graphical image of a 307 * signature. 308 */ 309 public String getContentType() { 310 return this.contentType == null ? null : this.contentType.getValue(); 311 } 312 313 /** 314 * @param value A mime type that indicates the technical format of the 315 * signature. Important mime types are application/signature+xml 316 * for X ML DigSig, application/jwt for JWT, and image/* for a 317 * graphical image of a signature. 318 */ 319 public Signature setContentType(String value) { 320 if (this.contentType == null) 321 this.contentType = new CodeType(); 322 this.contentType.setValue(value); 323 return this; 324 } 325 326 /** 327 * @return {@link #blob} (The base64 encoding of the Signature content.). This 328 * is the underlying object with id, value and extensions. The accessor 329 * "getBlob" gives direct access to the value 330 */ 331 public Base64BinaryType getBlobElement() { 332 if (this.blob == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create Signature.blob"); 335 else if (Configuration.doAutoCreate()) 336 this.blob = new Base64BinaryType(); // bb 337 return this.blob; 338 } 339 340 public boolean hasBlobElement() { 341 return this.blob != null && !this.blob.isEmpty(); 342 } 343 344 public boolean hasBlob() { 345 return this.blob != null && !this.blob.isEmpty(); 346 } 347 348 /** 349 * @param value {@link #blob} (The base64 encoding of the Signature content.). 350 * This is the underlying object with id, value and extensions. The 351 * accessor "getBlob" gives direct access to the value 352 */ 353 public Signature setBlobElement(Base64BinaryType value) { 354 this.blob = value; 355 return this; 356 } 357 358 /** 359 * @return The base64 encoding of the Signature content. 360 */ 361 public byte[] getBlob() { 362 return this.blob == null ? null : this.blob.getValue(); 363 } 364 365 /** 366 * @param value The base64 encoding of the Signature content. 367 */ 368 public Signature setBlob(byte[] value) { 369 if (this.blob == null) 370 this.blob = new Base64BinaryType(); 371 this.blob.setValue(value); 372 return this; 373 } 374 375 protected void listChildren(List<Property> childrenList) { 376 super.listChildren(childrenList); 377 childrenList.add(new Property("type", "Coding", 378 "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.", 379 0, java.lang.Integer.MAX_VALUE, type)); 380 childrenList.add(new Property("when", "instant", "When the digital signature was signed.", 0, 381 java.lang.Integer.MAX_VALUE, when)); 382 childrenList.add(new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", 383 "A reference to an application-usable description of the person that signed the certificate (e.g. the signature used their private key).", 384 0, java.lang.Integer.MAX_VALUE, who)); 385 childrenList.add(new Property("contentType", "code", 386 "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature.", 387 0, java.lang.Integer.MAX_VALUE, contentType)); 388 childrenList.add(new Property("blob", "base64Binary", "The base64 encoding of the Signature content.", 0, 389 java.lang.Integer.MAX_VALUE, blob)); 390 } 391 392 @Override 393 public void setProperty(String name, Base value) throws FHIRException { 394 if (name.equals("type")) 395 this.getType().add(castToCoding(value)); 396 else if (name.equals("when")) 397 this.when = castToInstant(value); // InstantType 398 else if (name.equals("who[x]")) 399 this.who = (Type) value; // Type 400 else if (name.equals("contentType")) 401 this.contentType = castToCode(value); // CodeType 402 else if (name.equals("blob")) 403 this.blob = castToBase64Binary(value); // Base64BinaryType 404 else 405 super.setProperty(name, value); 406 } 407 408 @Override 409 public Base addChild(String name) throws FHIRException { 410 if (name.equals("type")) { 411 return addType(); 412 } else if (name.equals("when")) { 413 throw new FHIRException("Cannot call addChild on a singleton property Signature.when"); 414 } else if (name.equals("whoUri")) { 415 this.who = new UriType(); 416 return this.who; 417 } else if (name.equals("whoReference")) { 418 this.who = new Reference(); 419 return this.who; 420 } else if (name.equals("contentType")) { 421 throw new FHIRException("Cannot call addChild on a singleton property Signature.contentType"); 422 } else if (name.equals("blob")) { 423 throw new FHIRException("Cannot call addChild on a singleton property Signature.blob"); 424 } else 425 return super.addChild(name); 426 } 427 428 public String fhirType() { 429 return "Signature"; 430 431 } 432 433 public Signature copy() { 434 Signature dst = new Signature(); 435 copyValues(dst); 436 if (type != null) { 437 dst.type = new ArrayList<Coding>(); 438 for (Coding i : type) 439 dst.type.add(i.copy()); 440 } 441 ; 442 dst.when = when == null ? null : when.copy(); 443 dst.who = who == null ? null : who.copy(); 444 dst.contentType = contentType == null ? null : contentType.copy(); 445 dst.blob = blob == null ? null : blob.copy(); 446 return dst; 447 } 448 449 protected Signature typedCopy() { 450 return copy(); 451 } 452 453 @Override 454 public boolean equalsDeep(Base other) { 455 if (!super.equalsDeep(other)) 456 return false; 457 if (!(other instanceof Signature)) 458 return false; 459 Signature o = (Signature) other; 460 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true) && compareDeep(who, o.who, true) 461 && compareDeep(contentType, o.contentType, true) && compareDeep(blob, o.blob, true); 462 } 463 464 @Override 465 public boolean equalsShallow(Base other) { 466 if (!super.equalsShallow(other)) 467 return false; 468 if (!(other instanceof Signature)) 469 return false; 470 Signature o = (Signature) other; 471 return compareValues(when, o.when, true) && compareValues(contentType, o.contentType, true) 472 && compareValues(blob, o.blob, true); 473 } 474 475 public boolean isEmpty() { 476 return super.isEmpty() && (type == null || type.isEmpty()) && (when == null || when.isEmpty()) 477 && (who == null || who.isEmpty()) && (contentType == null || contentType.isEmpty()) 478 && (blob == null || blob.isEmpty()); 479 } 480 481}