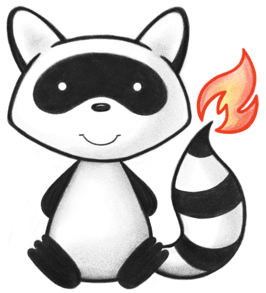
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * A slot of time on a schedule that may be available for booking appointments. 046 */ 047@ResourceDef(name = "Slot", profile = "http://hl7.org/fhir/Profile/Slot") 048public class Slot extends DomainResource { 049 050 public enum SlotStatus { 051 /** 052 * Indicates that the time interval is busy because one or more events have been 053 * scheduled for that interval. 054 */ 055 BUSY, 056 /** 057 * Indicates that the time interval is free for scheduling. 058 */ 059 FREE, 060 /** 061 * Indicates that the time interval is busy and that the interval can not be 062 * scheduled. 063 */ 064 BUSYUNAVAILABLE, 065 /** 066 * Indicates that the time interval is busy because one or more events have been 067 * tentatively scheduled for that interval. 068 */ 069 BUSYTENTATIVE, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static SlotStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("busy".equals(codeString)) 079 return BUSY; 080 if ("free".equals(codeString)) 081 return FREE; 082 if ("busy-unavailable".equals(codeString)) 083 return BUSYUNAVAILABLE; 084 if ("busy-tentative".equals(codeString)) 085 return BUSYTENTATIVE; 086 throw new FHIRException("Unknown SlotStatus code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case BUSY: 092 return "busy"; 093 case FREE: 094 return "free"; 095 case BUSYUNAVAILABLE: 096 return "busy-unavailable"; 097 case BUSYTENTATIVE: 098 return "busy-tentative"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 switch (this) { 108 case BUSY: 109 return "http://hl7.org/fhir/slotstatus"; 110 case FREE: 111 return "http://hl7.org/fhir/slotstatus"; 112 case BUSYUNAVAILABLE: 113 return "http://hl7.org/fhir/slotstatus"; 114 case BUSYTENTATIVE: 115 return "http://hl7.org/fhir/slotstatus"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case BUSY: 126 return "Indicates that the time interval is busy because one or more events have been scheduled for that interval."; 127 case FREE: 128 return "Indicates that the time interval is free for scheduling."; 129 case BUSYUNAVAILABLE: 130 return "Indicates that the time interval is busy and that the interval can not be scheduled."; 131 case BUSYTENTATIVE: 132 return "Indicates that the time interval is busy because one or more events have been tentatively scheduled for that interval."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case BUSY: 143 return "Busy"; 144 case FREE: 145 return "Free"; 146 case BUSYUNAVAILABLE: 147 return "Busy (Unavailable)"; 148 case BUSYTENTATIVE: 149 return "Busy (Tentative)"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class SlotStatusEnumFactory implements EnumFactory<SlotStatus> { 159 public SlotStatus fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("busy".equals(codeString)) 164 return SlotStatus.BUSY; 165 if ("free".equals(codeString)) 166 return SlotStatus.FREE; 167 if ("busy-unavailable".equals(codeString)) 168 return SlotStatus.BUSYUNAVAILABLE; 169 if ("busy-tentative".equals(codeString)) 170 return SlotStatus.BUSYTENTATIVE; 171 throw new IllegalArgumentException("Unknown SlotStatus code '" + codeString + "'"); 172 } 173 174 public Enumeration<SlotStatus> fromType(Base code) throws FHIRException { 175 if (code == null || code.isEmpty()) 176 return null; 177 String codeString = ((PrimitiveType) code).asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("busy".equals(codeString)) 181 return new Enumeration<SlotStatus>(this, SlotStatus.BUSY); 182 if ("free".equals(codeString)) 183 return new Enumeration<SlotStatus>(this, SlotStatus.FREE); 184 if ("busy-unavailable".equals(codeString)) 185 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYUNAVAILABLE); 186 if ("busy-tentative".equals(codeString)) 187 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYTENTATIVE); 188 throw new FHIRException("Unknown SlotStatus code '" + codeString + "'"); 189 } 190 191 public String toCode(SlotStatus code) 192 { 193 if (code == SlotStatus.NULL) 194 return null; 195 if (code == SlotStatus.BUSY) 196 return "busy"; 197 if (code == SlotStatus.FREE) 198 return "free"; 199 if (code == SlotStatus.BUSYUNAVAILABLE) 200 return "busy-unavailable"; 201 if (code == SlotStatus.BUSYTENTATIVE) 202 return "busy-tentative"; 203 return "?"; 204 } 205 } 206 207 /** 208 * External Ids for this item. 209 */ 210 @Child(name = "identifier", type = { 211 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 212 @Description(shortDefinition = "External Ids for this item", formalDefinition = "External Ids for this item.") 213 protected List<Identifier> identifier; 214 215 /** 216 * The type of appointments that can be booked into this slot (ideally this 217 * would be an identifiable service - which is at a location, rather than the 218 * location itself). If provided then this overrides the value provided on the 219 * availability resource. 220 */ 221 @Child(name = "type", type = { 222 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 223 @Description(shortDefinition = "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource", formalDefinition = "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.") 224 protected CodeableConcept type; 225 226 /** 227 * The schedule resource that this slot defines an interval of status 228 * information. 229 */ 230 @Child(name = "schedule", type = { Schedule.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 231 @Description(shortDefinition = "The schedule resource that this slot defines an interval of status information", formalDefinition = "The schedule resource that this slot defines an interval of status information.") 232 protected Reference schedule; 233 234 /** 235 * The actual object that is the target of the reference (The schedule resource 236 * that this slot defines an interval of status information.) 237 */ 238 protected Schedule scheduleTarget; 239 240 /** 241 * busy | free | busy-unavailable | busy-tentative. 242 */ 243 @Child(name = "freeBusyType", type = { 244 CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 245 @Description(shortDefinition = "busy | free | busy-unavailable | busy-tentative", formalDefinition = "busy | free | busy-unavailable | busy-tentative.") 246 protected Enumeration<SlotStatus> freeBusyType; 247 248 /** 249 * Date/Time that the slot is to begin. 250 */ 251 @Child(name = "start", type = { InstantType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 252 @Description(shortDefinition = "Date/Time that the slot is to begin", formalDefinition = "Date/Time that the slot is to begin.") 253 protected InstantType start; 254 255 /** 256 * Date/Time that the slot is to conclude. 257 */ 258 @Child(name = "end", type = { InstantType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 259 @Description(shortDefinition = "Date/Time that the slot is to conclude", formalDefinition = "Date/Time that the slot is to conclude.") 260 protected InstantType end; 261 262 /** 263 * This slot has already been overbooked, appointments are unlikely to be 264 * accepted for this time. 265 */ 266 @Child(name = "overbooked", type = { 267 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 268 @Description(shortDefinition = "This slot has already been overbooked, appointments are unlikely to be accepted for this time", formalDefinition = "This slot has already been overbooked, appointments are unlikely to be accepted for this time.") 269 protected BooleanType overbooked; 270 271 /** 272 * Comments on the slot to describe any extended information. Such as custom 273 * constraints on the slot. 274 */ 275 @Child(name = "comment", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 276 @Description(shortDefinition = "Comments on the slot to describe any extended information. Such as custom constraints on the slot", formalDefinition = "Comments on the slot to describe any extended information. Such as custom constraints on the slot.") 277 protected StringType comment; 278 279 private static final long serialVersionUID = 1984269299L; 280 281 /* 282 * Constructor 283 */ 284 public Slot() { 285 super(); 286 } 287 288 /* 289 * Constructor 290 */ 291 public Slot(Reference schedule, Enumeration<SlotStatus> freeBusyType, InstantType start, InstantType end) { 292 super(); 293 this.schedule = schedule; 294 this.freeBusyType = freeBusyType; 295 this.start = start; 296 this.end = end; 297 } 298 299 /** 300 * @return {@link #identifier} (External Ids for this item.) 301 */ 302 public List<Identifier> getIdentifier() { 303 if (this.identifier == null) 304 this.identifier = new ArrayList<Identifier>(); 305 return this.identifier; 306 } 307 308 public boolean hasIdentifier() { 309 if (this.identifier == null) 310 return false; 311 for (Identifier item : this.identifier) 312 if (!item.isEmpty()) 313 return true; 314 return false; 315 } 316 317 /** 318 * @return {@link #identifier} (External Ids for this item.) 319 */ 320 // syntactic sugar 321 public Identifier addIdentifier() { // 3 322 Identifier t = new Identifier(); 323 if (this.identifier == null) 324 this.identifier = new ArrayList<Identifier>(); 325 this.identifier.add(t); 326 return t; 327 } 328 329 // syntactic sugar 330 public Slot addIdentifier(Identifier t) { // 3 331 if (t == null) 332 return this; 333 if (this.identifier == null) 334 this.identifier = new ArrayList<Identifier>(); 335 this.identifier.add(t); 336 return this; 337 } 338 339 /** 340 * @return {@link #type} (The type of appointments that can be booked into this 341 * slot (ideally this would be an identifiable service - which is at a 342 * location, rather than the location itself). If provided then this 343 * overrides the value provided on the availability resource.) 344 */ 345 public CodeableConcept getType() { 346 if (this.type == null) 347 if (Configuration.errorOnAutoCreate()) 348 throw new Error("Attempt to auto-create Slot.type"); 349 else if (Configuration.doAutoCreate()) 350 this.type = new CodeableConcept(); // cc 351 return this.type; 352 } 353 354 public boolean hasType() { 355 return this.type != null && !this.type.isEmpty(); 356 } 357 358 /** 359 * @param value {@link #type} (The type of appointments that can be booked into 360 * this slot (ideally this would be an identifiable service - which 361 * is at a location, rather than the location itself). If provided 362 * then this overrides the value provided on the availability 363 * resource.) 364 */ 365 public Slot setType(CodeableConcept value) { 366 this.type = value; 367 return this; 368 } 369 370 /** 371 * @return {@link #schedule} (The schedule resource that this slot defines an 372 * interval of status information.) 373 */ 374 public Reference getSchedule() { 375 if (this.schedule == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create Slot.schedule"); 378 else if (Configuration.doAutoCreate()) 379 this.schedule = new Reference(); // cc 380 return this.schedule; 381 } 382 383 public boolean hasSchedule() { 384 return this.schedule != null && !this.schedule.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #schedule} (The schedule resource that this slot defines 389 * an interval of status information.) 390 */ 391 public Slot setSchedule(Reference value) { 392 this.schedule = value; 393 return this; 394 } 395 396 /** 397 * @return {@link #schedule} The actual object that is the target of the 398 * reference. The reference library doesn't populate this, but you can 399 * use it to hold the resource if you resolve it. (The schedule resource 400 * that this slot defines an interval of status information.) 401 */ 402 public Schedule getScheduleTarget() { 403 if (this.scheduleTarget == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create Slot.schedule"); 406 else if (Configuration.doAutoCreate()) 407 this.scheduleTarget = new Schedule(); // aa 408 return this.scheduleTarget; 409 } 410 411 /** 412 * @param value {@link #schedule} The actual object that is the target of the 413 * reference. The reference library doesn't use these, but you can 414 * use it to hold the resource if you resolve it. (The schedule 415 * resource that this slot defines an interval of status 416 * information.) 417 */ 418 public Slot setScheduleTarget(Schedule value) { 419 this.scheduleTarget = value; 420 return this; 421 } 422 423 /** 424 * @return {@link #freeBusyType} (busy | free | busy-unavailable | 425 * busy-tentative.). This is the underlying object with id, value and 426 * extensions. The accessor "getFreeBusyType" gives direct access to the 427 * value 428 */ 429 public Enumeration<SlotStatus> getFreeBusyTypeElement() { 430 if (this.freeBusyType == null) 431 if (Configuration.errorOnAutoCreate()) 432 throw new Error("Attempt to auto-create Slot.freeBusyType"); 433 else if (Configuration.doAutoCreate()) 434 this.freeBusyType = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); // bb 435 return this.freeBusyType; 436 } 437 438 public boolean hasFreeBusyTypeElement() { 439 return this.freeBusyType != null && !this.freeBusyType.isEmpty(); 440 } 441 442 public boolean hasFreeBusyType() { 443 return this.freeBusyType != null && !this.freeBusyType.isEmpty(); 444 } 445 446 /** 447 * @param value {@link #freeBusyType} (busy | free | busy-unavailable | 448 * busy-tentative.). This is the underlying object with id, value 449 * and extensions. The accessor "getFreeBusyType" gives direct 450 * access to the value 451 */ 452 public Slot setFreeBusyTypeElement(Enumeration<SlotStatus> value) { 453 this.freeBusyType = value; 454 return this; 455 } 456 457 /** 458 * @return busy | free | busy-unavailable | busy-tentative. 459 */ 460 public SlotStatus getFreeBusyType() { 461 return this.freeBusyType == null ? null : this.freeBusyType.getValue(); 462 } 463 464 /** 465 * @param value busy | free | busy-unavailable | busy-tentative. 466 */ 467 public Slot setFreeBusyType(SlotStatus value) { 468 if (this.freeBusyType == null) 469 this.freeBusyType = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); 470 this.freeBusyType.setValue(value); 471 return this; 472 } 473 474 /** 475 * @return {@link #start} (Date/Time that the slot is to begin.). This is the 476 * underlying object with id, value and extensions. The accessor 477 * "getStart" gives direct access to the value 478 */ 479 public InstantType getStartElement() { 480 if (this.start == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create Slot.start"); 483 else if (Configuration.doAutoCreate()) 484 this.start = new InstantType(); // bb 485 return this.start; 486 } 487 488 public boolean hasStartElement() { 489 return this.start != null && !this.start.isEmpty(); 490 } 491 492 public boolean hasStart() { 493 return this.start != null && !this.start.isEmpty(); 494 } 495 496 /** 497 * @param value {@link #start} (Date/Time that the slot is to begin.). This is 498 * the underlying object with id, value and extensions. The 499 * accessor "getStart" gives direct access to the value 500 */ 501 public Slot setStartElement(InstantType value) { 502 this.start = value; 503 return this; 504 } 505 506 /** 507 * @return Date/Time that the slot is to begin. 508 */ 509 public Date getStart() { 510 return this.start == null ? null : this.start.getValue(); 511 } 512 513 /** 514 * @param value Date/Time that the slot is to begin. 515 */ 516 public Slot setStart(Date value) { 517 if (this.start == null) 518 this.start = new InstantType(); 519 this.start.setValue(value); 520 return this; 521 } 522 523 /** 524 * @return {@link #end} (Date/Time that the slot is to conclude.). This is the 525 * underlying object with id, value and extensions. The accessor 526 * "getEnd" gives direct access to the value 527 */ 528 public InstantType getEndElement() { 529 if (this.end == null) 530 if (Configuration.errorOnAutoCreate()) 531 throw new Error("Attempt to auto-create Slot.end"); 532 else if (Configuration.doAutoCreate()) 533 this.end = new InstantType(); // bb 534 return this.end; 535 } 536 537 public boolean hasEndElement() { 538 return this.end != null && !this.end.isEmpty(); 539 } 540 541 public boolean hasEnd() { 542 return this.end != null && !this.end.isEmpty(); 543 } 544 545 /** 546 * @param value {@link #end} (Date/Time that the slot is to conclude.). This is 547 * the underlying object with id, value and extensions. The 548 * accessor "getEnd" gives direct access to the value 549 */ 550 public Slot setEndElement(InstantType value) { 551 this.end = value; 552 return this; 553 } 554 555 /** 556 * @return Date/Time that the slot is to conclude. 557 */ 558 public Date getEnd() { 559 return this.end == null ? null : this.end.getValue(); 560 } 561 562 /** 563 * @param value Date/Time that the slot is to conclude. 564 */ 565 public Slot setEnd(Date value) { 566 if (this.end == null) 567 this.end = new InstantType(); 568 this.end.setValue(value); 569 return this; 570 } 571 572 /** 573 * @return {@link #overbooked} (This slot has already been overbooked, 574 * appointments are unlikely to be accepted for this time.). This is the 575 * underlying object with id, value and extensions. The accessor 576 * "getOverbooked" gives direct access to the value 577 */ 578 public BooleanType getOverbookedElement() { 579 if (this.overbooked == null) 580 if (Configuration.errorOnAutoCreate()) 581 throw new Error("Attempt to auto-create Slot.overbooked"); 582 else if (Configuration.doAutoCreate()) 583 this.overbooked = new BooleanType(); // bb 584 return this.overbooked; 585 } 586 587 public boolean hasOverbookedElement() { 588 return this.overbooked != null && !this.overbooked.isEmpty(); 589 } 590 591 public boolean hasOverbooked() { 592 return this.overbooked != null && !this.overbooked.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #overbooked} (This slot has already been overbooked, 597 * appointments are unlikely to be accepted for this time.). This 598 * is the underlying object with id, value and extensions. The 599 * accessor "getOverbooked" gives direct access to the value 600 */ 601 public Slot setOverbookedElement(BooleanType value) { 602 this.overbooked = value; 603 return this; 604 } 605 606 /** 607 * @return This slot has already been overbooked, appointments are unlikely to 608 * be accepted for this time. 609 */ 610 public boolean getOverbooked() { 611 return this.overbooked == null || this.overbooked.isEmpty() ? false : this.overbooked.getValue(); 612 } 613 614 /** 615 * @param value This slot has already been overbooked, appointments are unlikely 616 * to be accepted for this time. 617 */ 618 public Slot setOverbooked(boolean value) { 619 if (this.overbooked == null) 620 this.overbooked = new BooleanType(); 621 this.overbooked.setValue(value); 622 return this; 623 } 624 625 /** 626 * @return {@link #comment} (Comments on the slot to describe any extended 627 * information. Such as custom constraints on the slot.). This is the 628 * underlying object with id, value and extensions. The accessor 629 * "getComment" gives direct access to the value 630 */ 631 public StringType getCommentElement() { 632 if (this.comment == null) 633 if (Configuration.errorOnAutoCreate()) 634 throw new Error("Attempt to auto-create Slot.comment"); 635 else if (Configuration.doAutoCreate()) 636 this.comment = new StringType(); // bb 637 return this.comment; 638 } 639 640 public boolean hasCommentElement() { 641 return this.comment != null && !this.comment.isEmpty(); 642 } 643 644 public boolean hasComment() { 645 return this.comment != null && !this.comment.isEmpty(); 646 } 647 648 /** 649 * @param value {@link #comment} (Comments on the slot to describe any extended 650 * information. Such as custom constraints on the slot.). This is 651 * the underlying object with id, value and extensions. The 652 * accessor "getComment" gives direct access to the value 653 */ 654 public Slot setCommentElement(StringType value) { 655 this.comment = value; 656 return this; 657 } 658 659 /** 660 * @return Comments on the slot to describe any extended information. Such as 661 * custom constraints on the slot. 662 */ 663 public String getComment() { 664 return this.comment == null ? null : this.comment.getValue(); 665 } 666 667 /** 668 * @param value Comments on the slot to describe any extended information. Such 669 * as custom constraints on the slot. 670 */ 671 public Slot setComment(String value) { 672 if (Utilities.noString(value)) 673 this.comment = null; 674 else { 675 if (this.comment == null) 676 this.comment = new StringType(); 677 this.comment.setValue(value); 678 } 679 return this; 680 } 681 682 protected void listChildren(List<Property> childrenList) { 683 super.listChildren(childrenList); 684 childrenList.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, 685 java.lang.Integer.MAX_VALUE, identifier)); 686 childrenList.add(new Property("type", "CodeableConcept", 687 "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.", 688 0, java.lang.Integer.MAX_VALUE, type)); 689 childrenList.add(new Property("schedule", "Reference(Schedule)", 690 "The schedule resource that this slot defines an interval of status information.", 0, 691 java.lang.Integer.MAX_VALUE, schedule)); 692 childrenList.add(new Property("freeBusyType", "code", "busy | free | busy-unavailable | busy-tentative.", 0, 693 java.lang.Integer.MAX_VALUE, freeBusyType)); 694 childrenList.add(new Property("start", "instant", "Date/Time that the slot is to begin.", 0, 695 java.lang.Integer.MAX_VALUE, start)); 696 childrenList.add( 697 new Property("end", "instant", "Date/Time that the slot is to conclude.", 0, java.lang.Integer.MAX_VALUE, end)); 698 childrenList.add(new Property("overbooked", "boolean", 699 "This slot has already been overbooked, appointments are unlikely to be accepted for this time.", 0, 700 java.lang.Integer.MAX_VALUE, overbooked)); 701 childrenList.add(new Property("comment", "string", 702 "Comments on the slot to describe any extended information. Such as custom constraints on the slot.", 0, 703 java.lang.Integer.MAX_VALUE, comment)); 704 } 705 706 @Override 707 public void setProperty(String name, Base value) throws FHIRException { 708 if (name.equals("identifier")) 709 this.getIdentifier().add(castToIdentifier(value)); 710 else if (name.equals("type")) 711 this.type = castToCodeableConcept(value); // CodeableConcept 712 else if (name.equals("schedule")) 713 this.schedule = castToReference(value); // Reference 714 else if (name.equals("freeBusyType")) 715 this.freeBusyType = new SlotStatusEnumFactory().fromType(value); // Enumeration<SlotStatus> 716 else if (name.equals("start")) 717 this.start = castToInstant(value); // InstantType 718 else if (name.equals("end")) 719 this.end = castToInstant(value); // InstantType 720 else if (name.equals("overbooked")) 721 this.overbooked = castToBoolean(value); // BooleanType 722 else if (name.equals("comment")) 723 this.comment = castToString(value); // StringType 724 else 725 super.setProperty(name, value); 726 } 727 728 @Override 729 public Base addChild(String name) throws FHIRException { 730 if (name.equals("identifier")) { 731 return addIdentifier(); 732 } else if (name.equals("type")) { 733 this.type = new CodeableConcept(); 734 return this.type; 735 } else if (name.equals("schedule")) { 736 this.schedule = new Reference(); 737 return this.schedule; 738 } else if (name.equals("freeBusyType")) { 739 throw new FHIRException("Cannot call addChild on a singleton property Slot.freeBusyType"); 740 } else if (name.equals("start")) { 741 throw new FHIRException("Cannot call addChild on a singleton property Slot.start"); 742 } else if (name.equals("end")) { 743 throw new FHIRException("Cannot call addChild on a singleton property Slot.end"); 744 } else if (name.equals("overbooked")) { 745 throw new FHIRException("Cannot call addChild on a singleton property Slot.overbooked"); 746 } else if (name.equals("comment")) { 747 throw new FHIRException("Cannot call addChild on a singleton property Slot.comment"); 748 } else 749 return super.addChild(name); 750 } 751 752 public String fhirType() { 753 return "Slot"; 754 755 } 756 757 public Slot copy() { 758 Slot dst = new Slot(); 759 copyValues(dst); 760 if (identifier != null) { 761 dst.identifier = new ArrayList<Identifier>(); 762 for (Identifier i : identifier) 763 dst.identifier.add(i.copy()); 764 } 765 ; 766 dst.type = type == null ? null : type.copy(); 767 dst.schedule = schedule == null ? null : schedule.copy(); 768 dst.freeBusyType = freeBusyType == null ? null : freeBusyType.copy(); 769 dst.start = start == null ? null : start.copy(); 770 dst.end = end == null ? null : end.copy(); 771 dst.overbooked = overbooked == null ? null : overbooked.copy(); 772 dst.comment = comment == null ? null : comment.copy(); 773 return dst; 774 } 775 776 protected Slot typedCopy() { 777 return copy(); 778 } 779 780 @Override 781 public boolean equalsDeep(Base other) { 782 if (!super.equalsDeep(other)) 783 return false; 784 if (!(other instanceof Slot)) 785 return false; 786 Slot o = (Slot) other; 787 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 788 && compareDeep(schedule, o.schedule, true) && compareDeep(freeBusyType, o.freeBusyType, true) 789 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 790 && compareDeep(overbooked, o.overbooked, true) && compareDeep(comment, o.comment, true); 791 } 792 793 @Override 794 public boolean equalsShallow(Base other) { 795 if (!super.equalsShallow(other)) 796 return false; 797 if (!(other instanceof Slot)) 798 return false; 799 Slot o = (Slot) other; 800 return compareValues(freeBusyType, o.freeBusyType, true) && compareValues(start, o.start, true) 801 && compareValues(end, o.end, true) && compareValues(overbooked, o.overbooked, true) 802 && compareValues(comment, o.comment, true); 803 } 804 805 public boolean isEmpty() { 806 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (type == null || type.isEmpty()) 807 && (schedule == null || schedule.isEmpty()) && (freeBusyType == null || freeBusyType.isEmpty()) 808 && (start == null || start.isEmpty()) && (end == null || end.isEmpty()) 809 && (overbooked == null || overbooked.isEmpty()) && (comment == null || comment.isEmpty()); 810 } 811 812 @Override 813 public ResourceType getResourceType() { 814 return ResourceType.Slot; 815 } 816 817 @SearchParamDefinition(name = "schedule", path = "Slot.schedule", description = "The Schedule Resource that we are seeking a slot within", type = "reference") 818 public static final String SP_SCHEDULE = "schedule"; 819 @SearchParamDefinition(name = "identifier", path = "Slot.identifier", description = "A Slot Identifier", type = "token") 820 public static final String SP_IDENTIFIER = "identifier"; 821 @SearchParamDefinition(name = "start", path = "Slot.start", description = "Appointment date/time.", type = "date") 822 public static final String SP_START = "start"; 823 @SearchParamDefinition(name = "slot-type", path = "Slot.type", description = "The type of appointments that can be booked into the slot", type = "token") 824 public static final String SP_SLOTTYPE = "slot-type"; 825 @SearchParamDefinition(name = "fb-type", path = "Slot.freeBusyType", description = "The free/busy status of the appointment", type = "token") 826 public static final String SP_FBTYPE = "fb-type"; 827 828}